Linked Lists in Action Chapter 5 introduces the
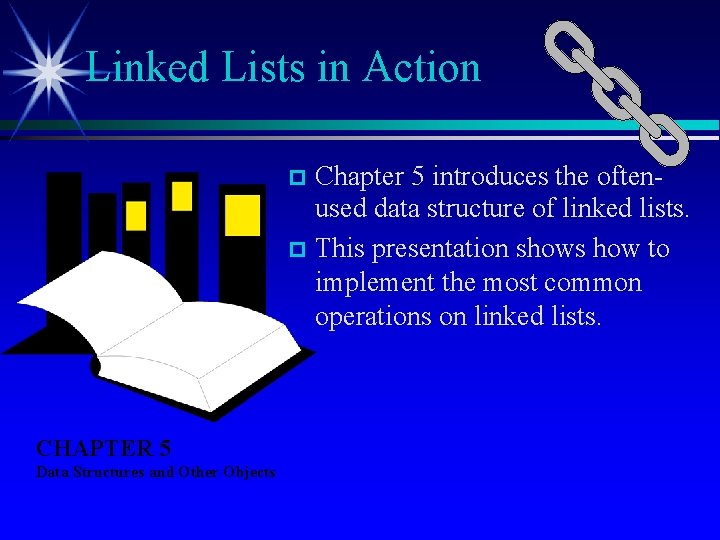
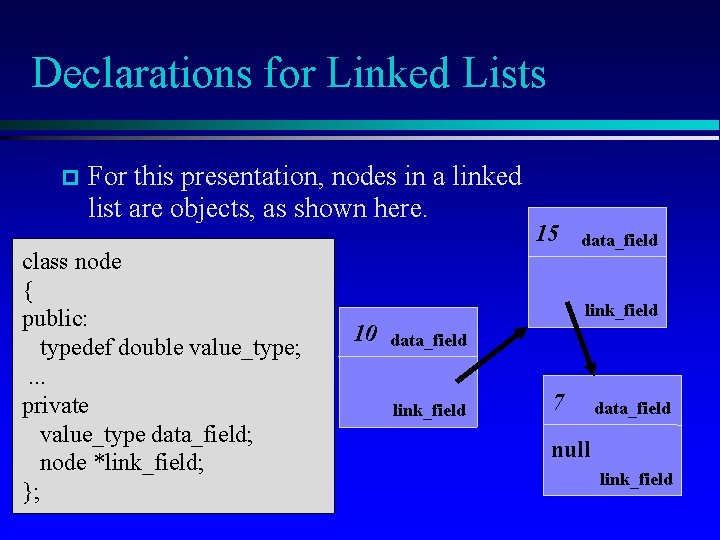
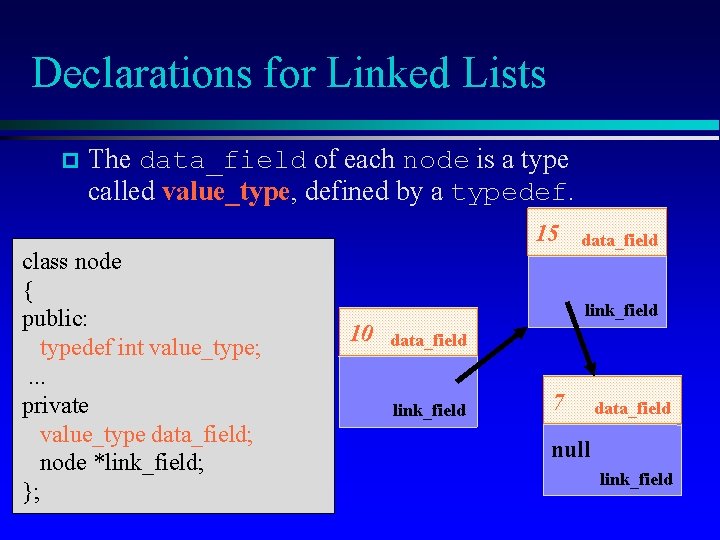
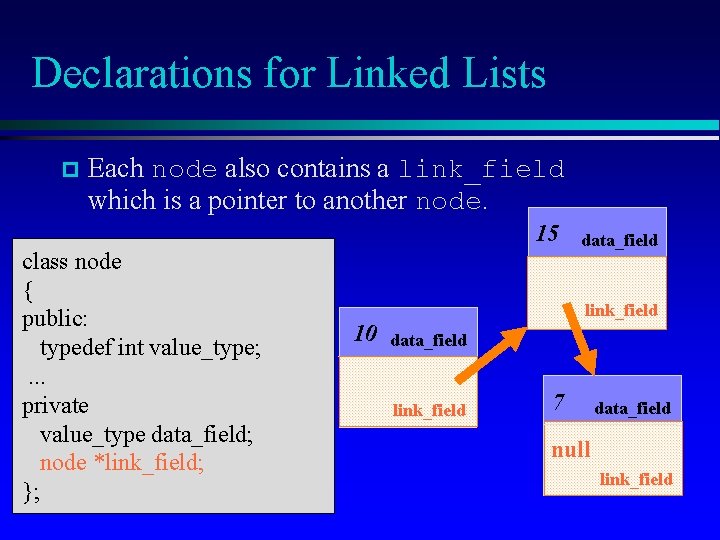
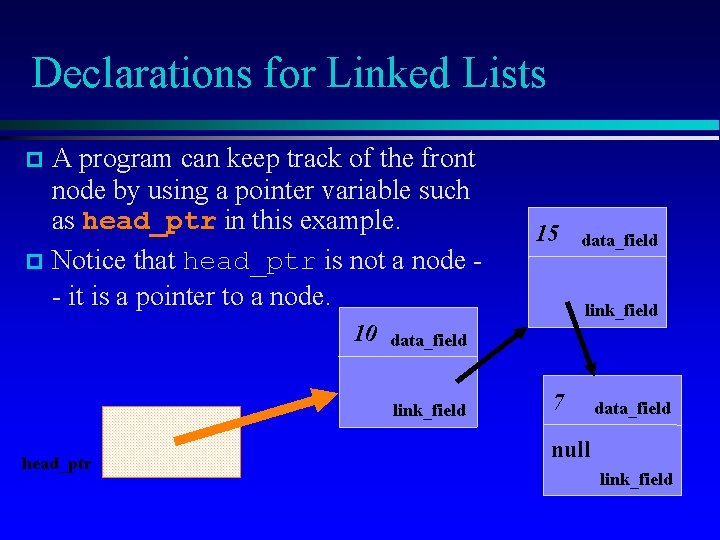
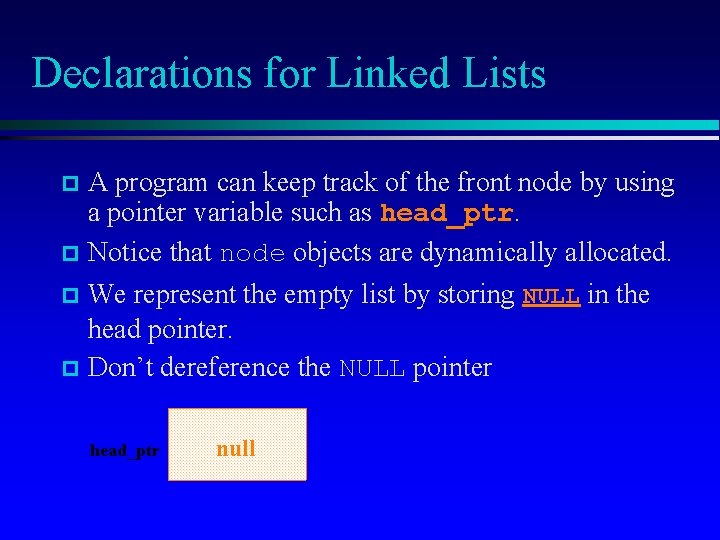
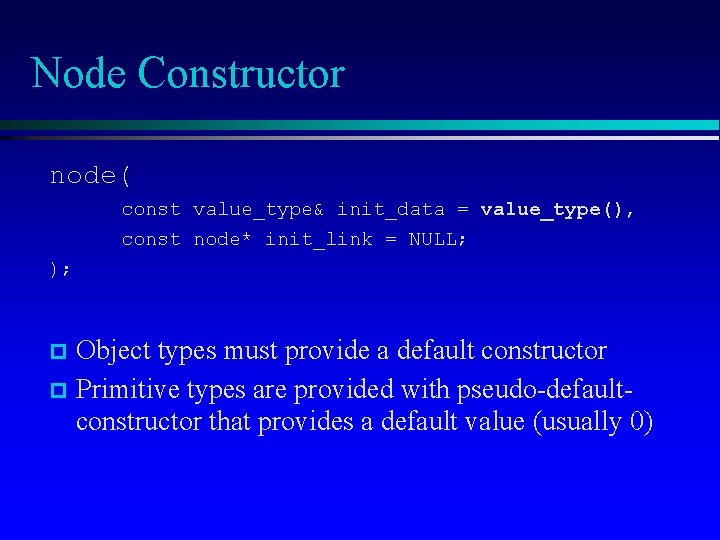
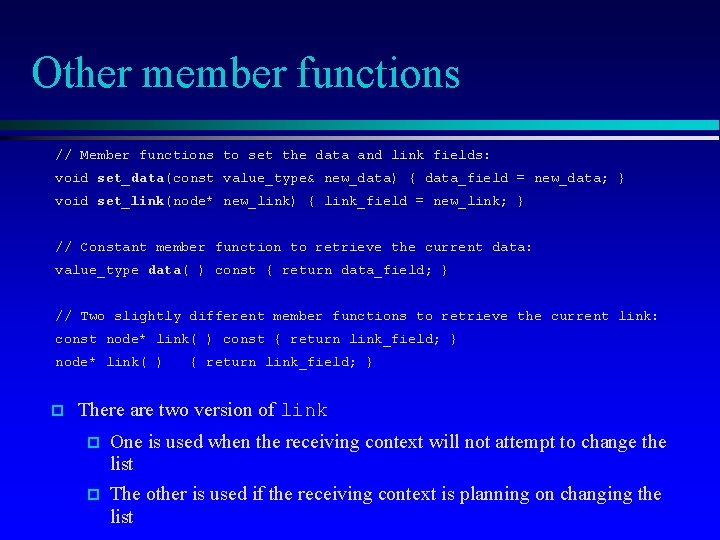
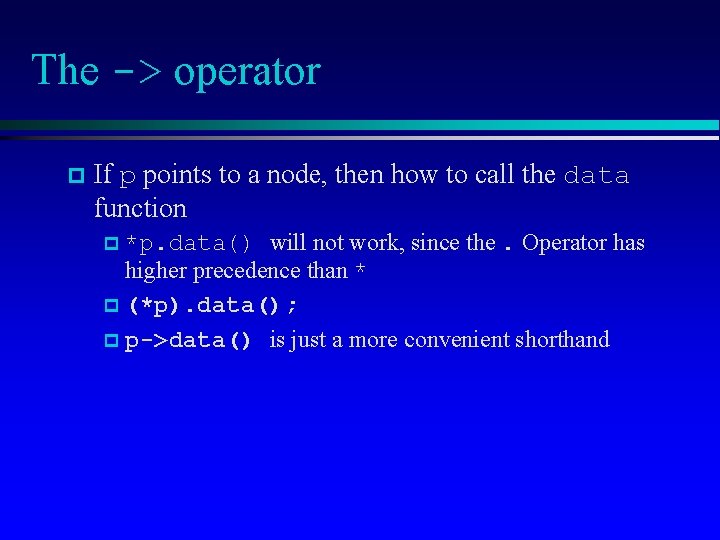
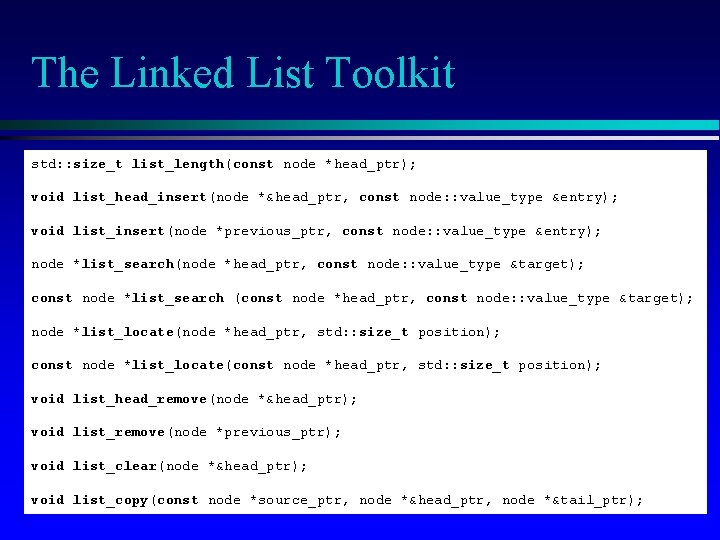
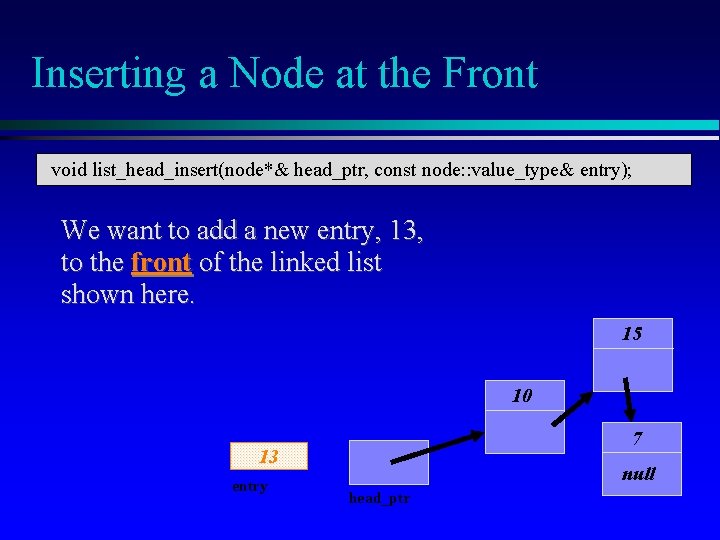
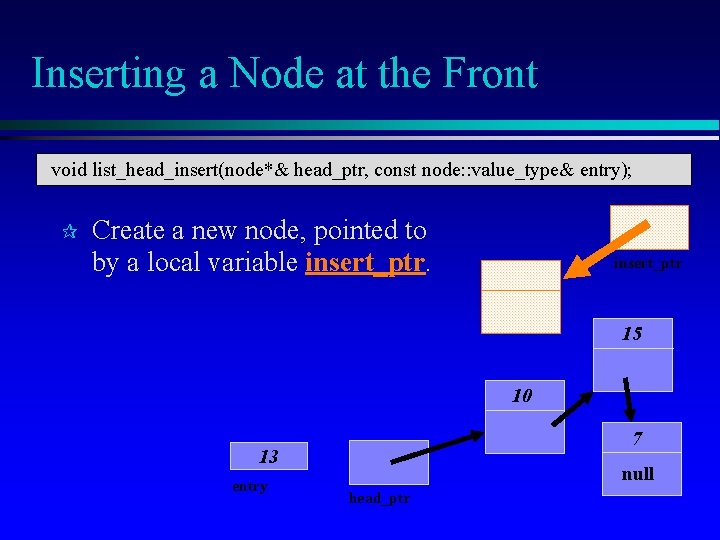
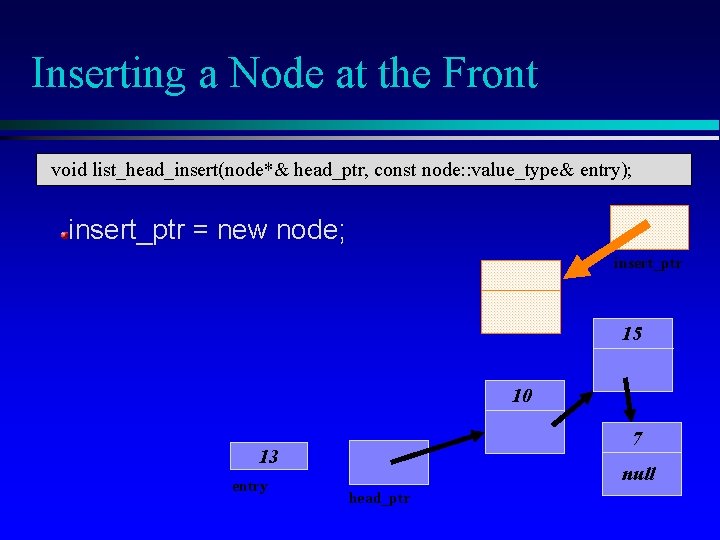
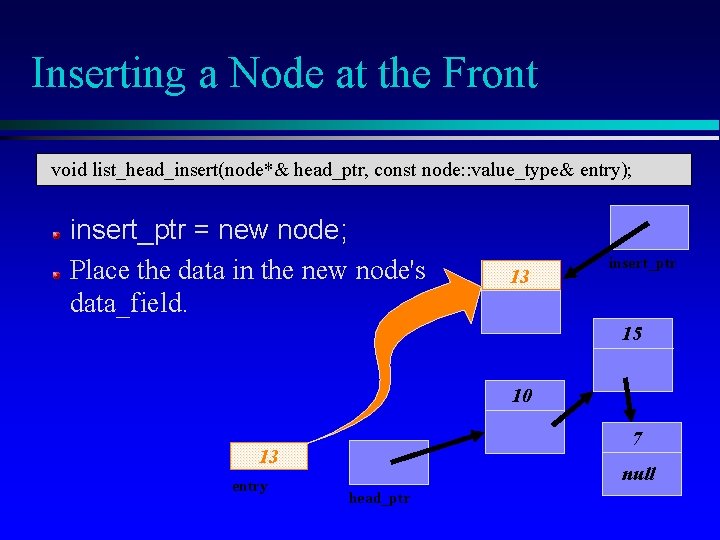
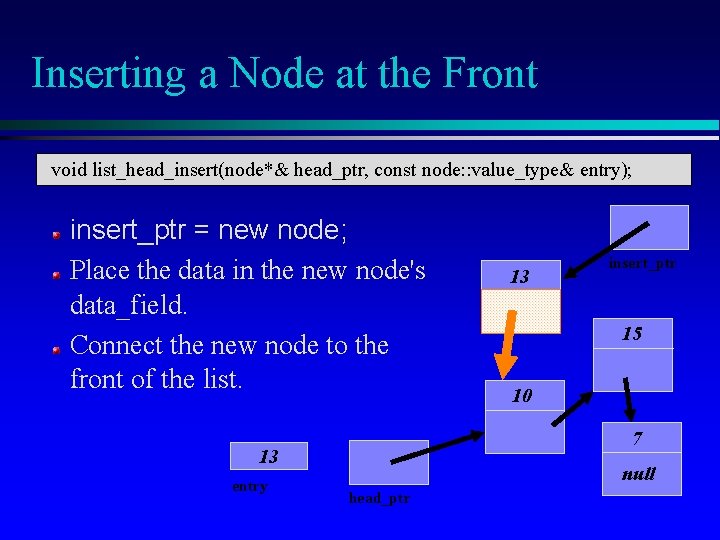
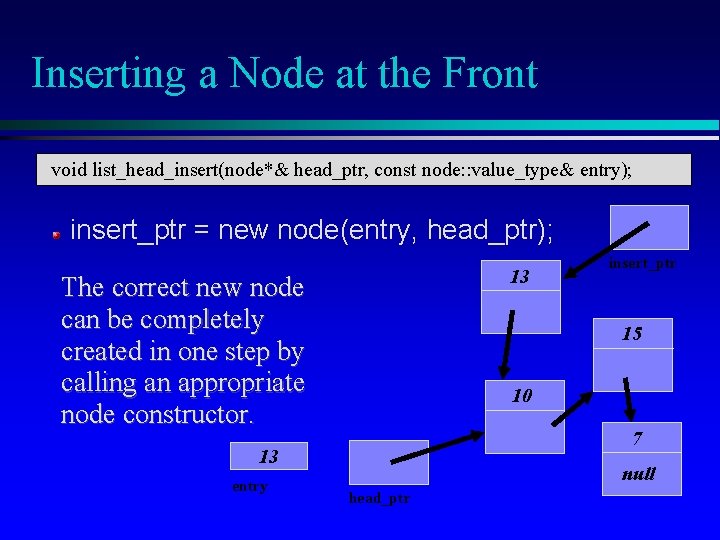
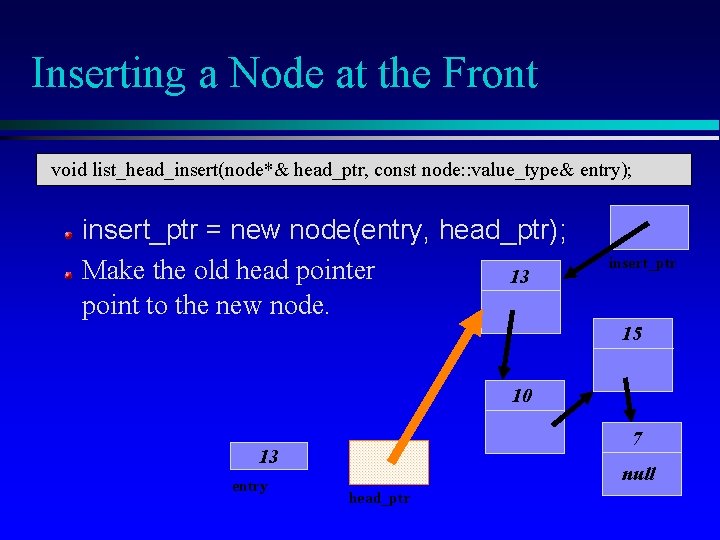
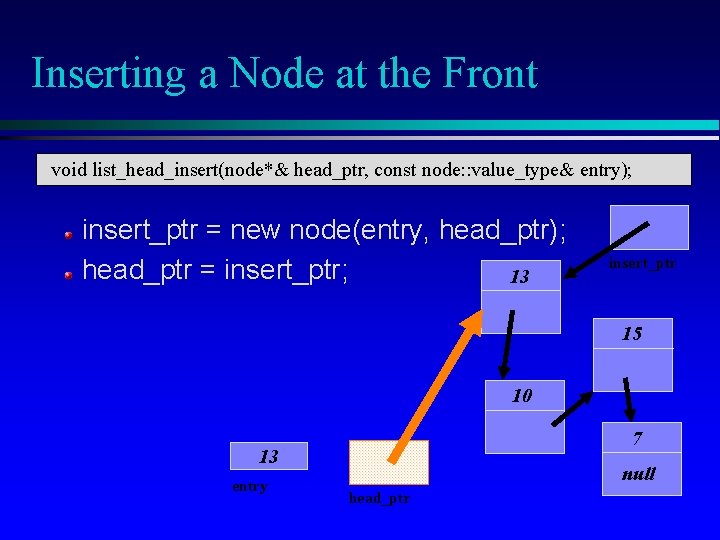
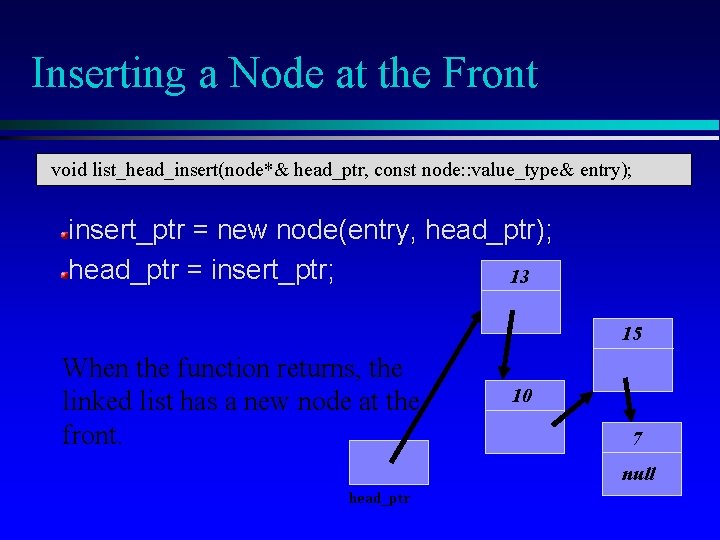
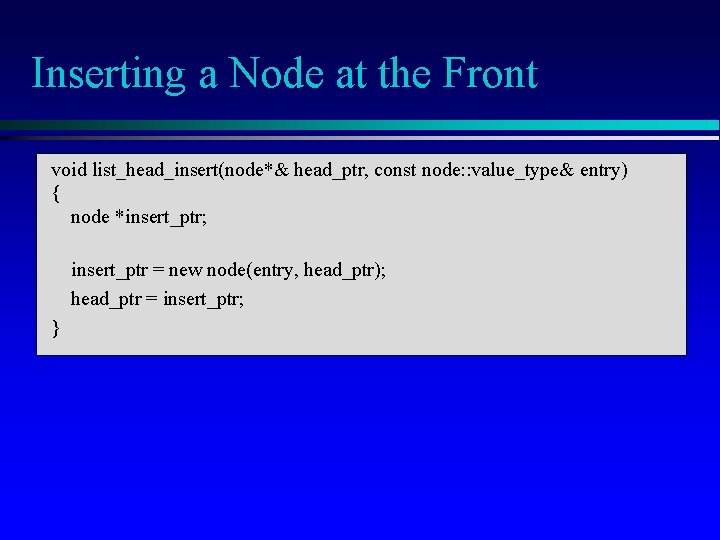
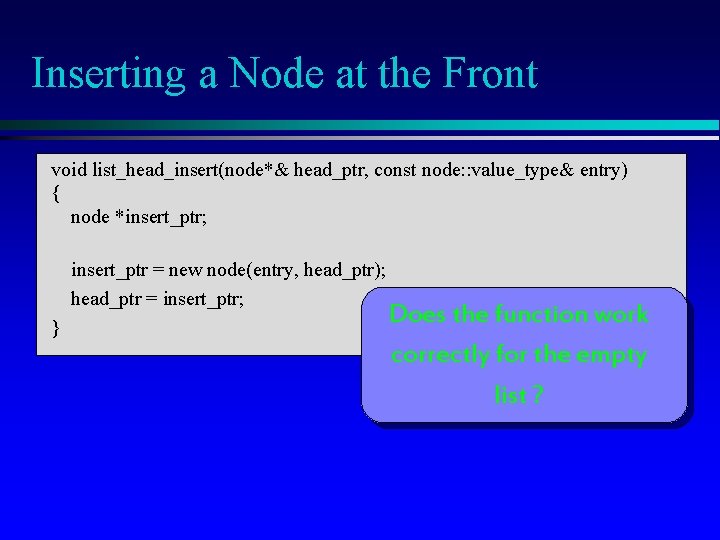
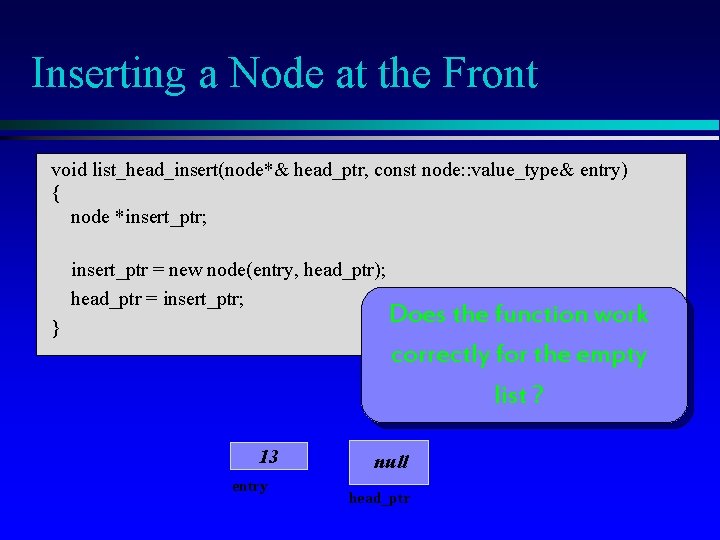
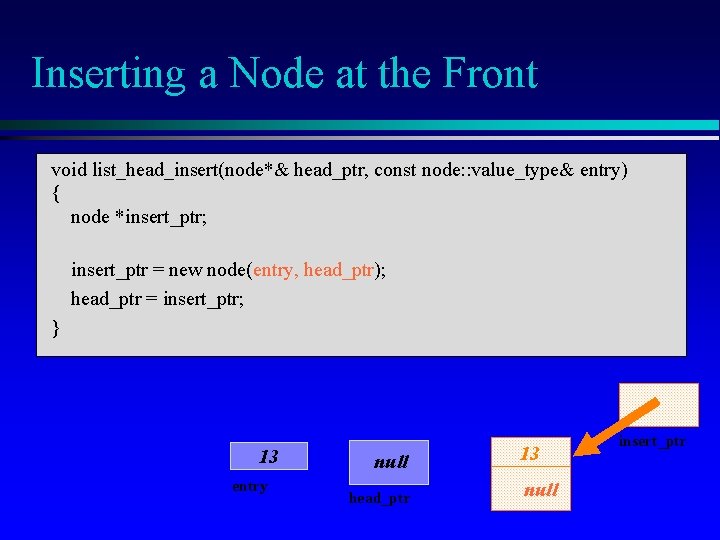
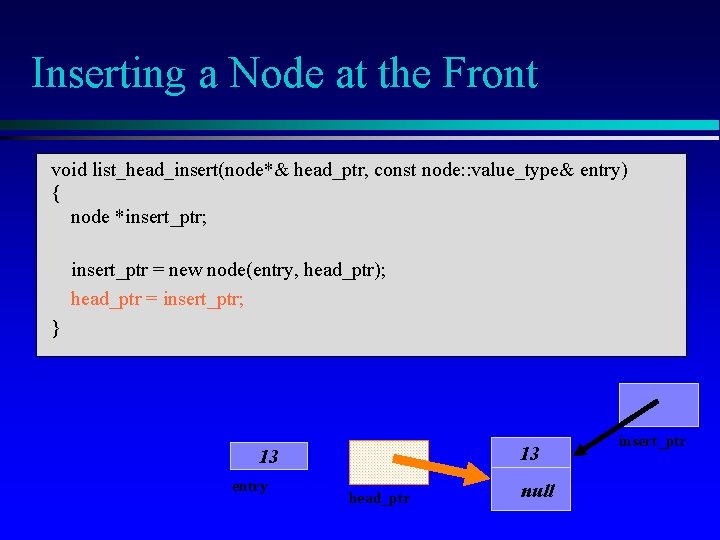
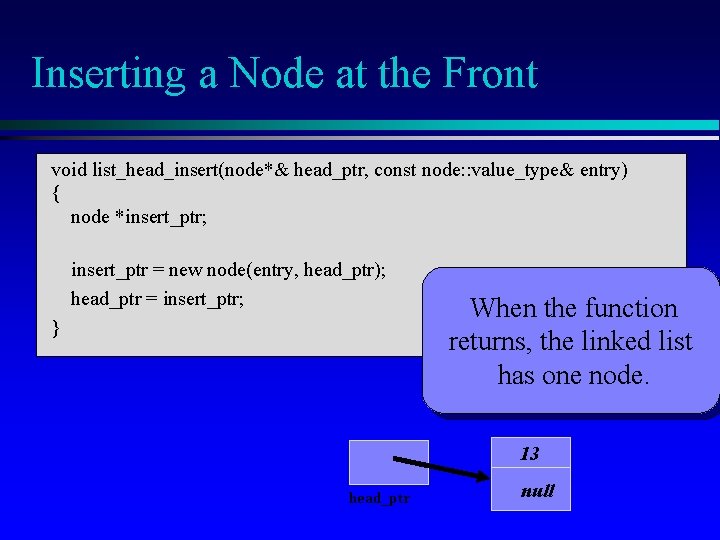
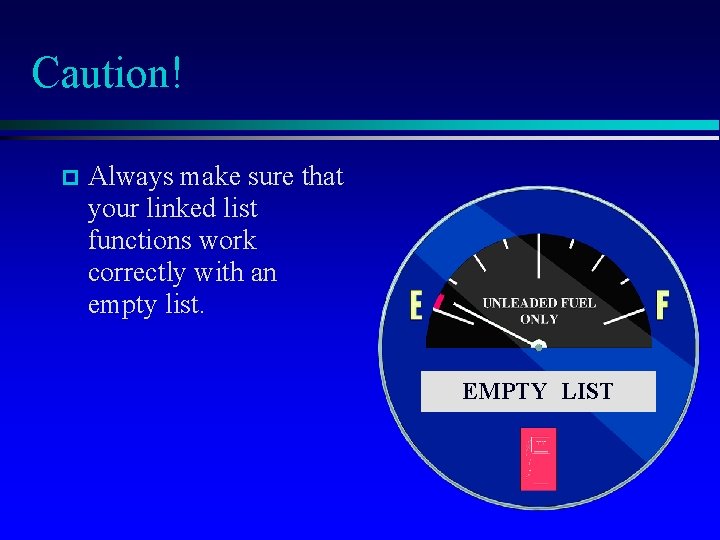
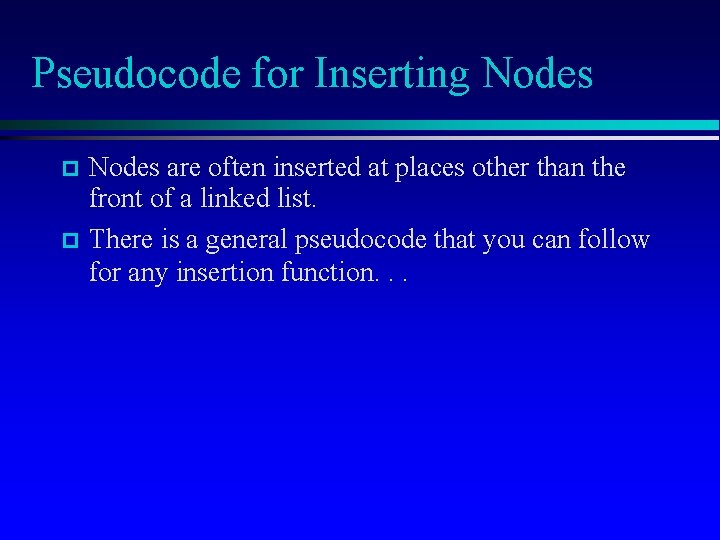
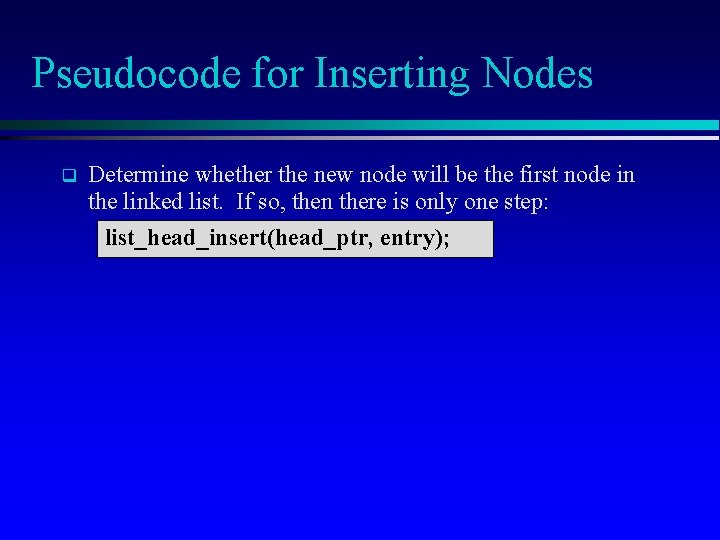
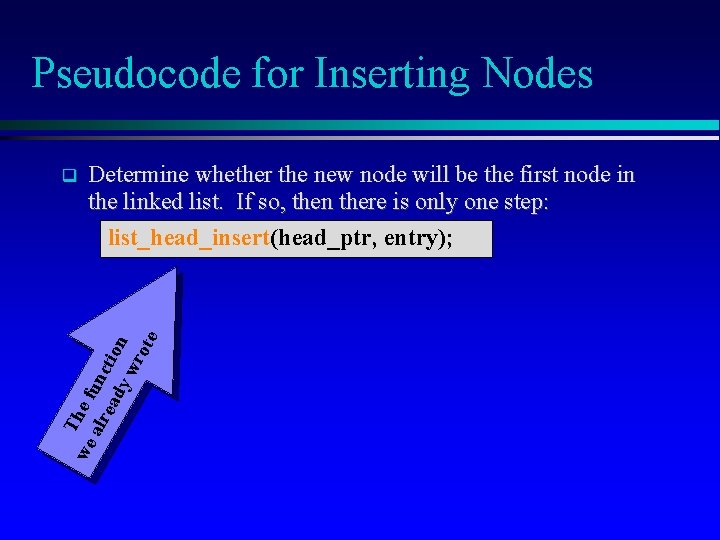
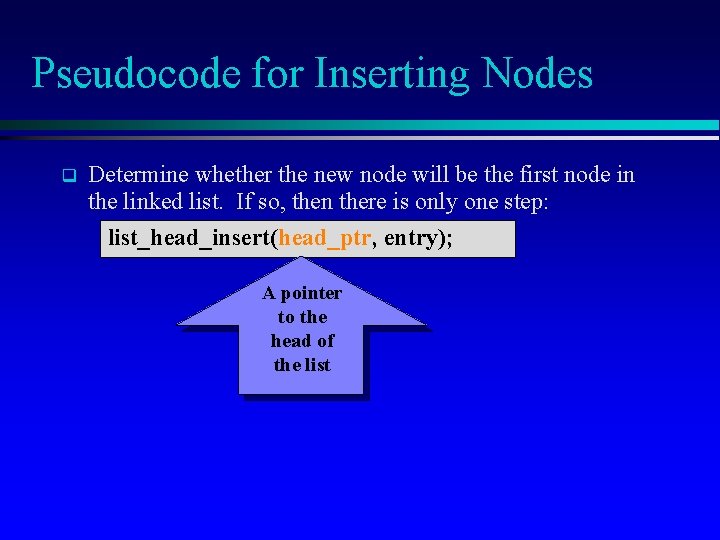
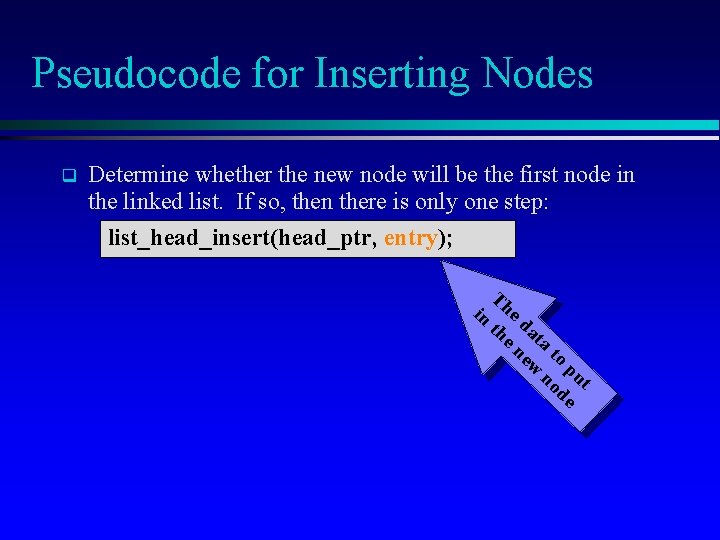
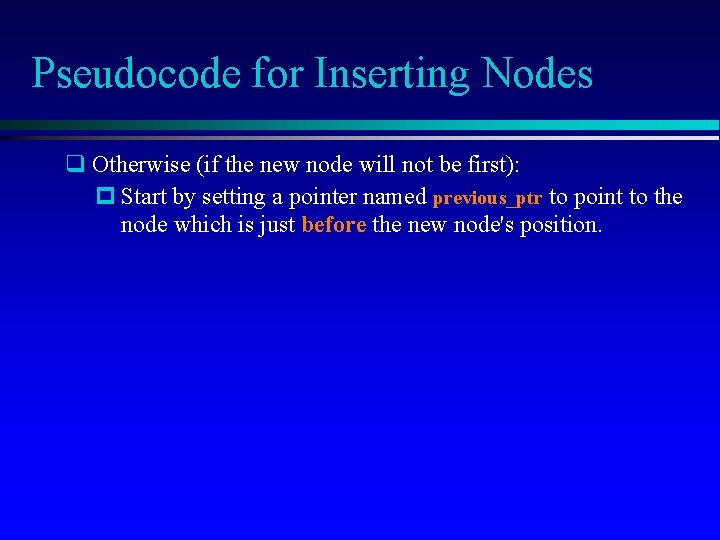
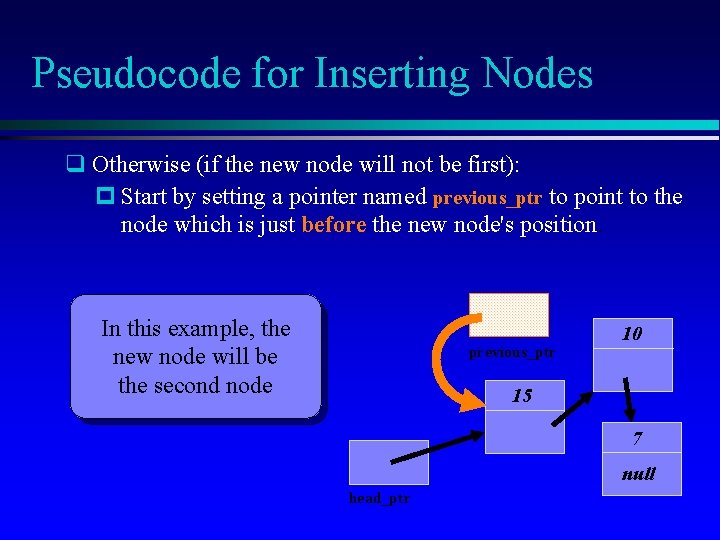
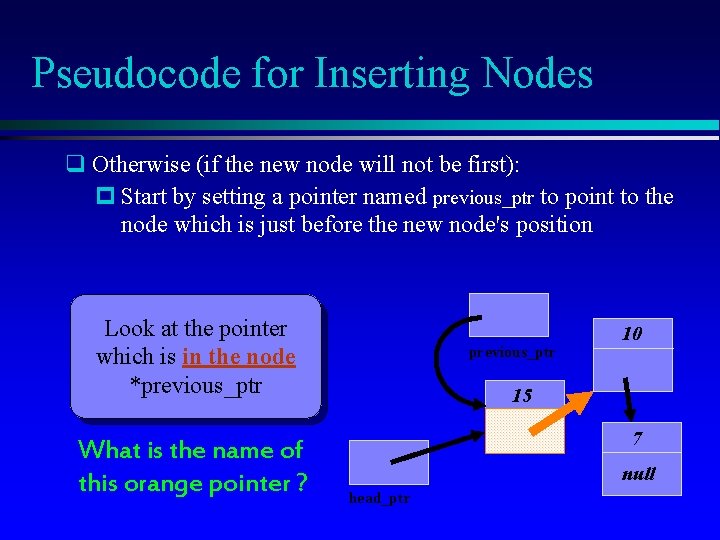
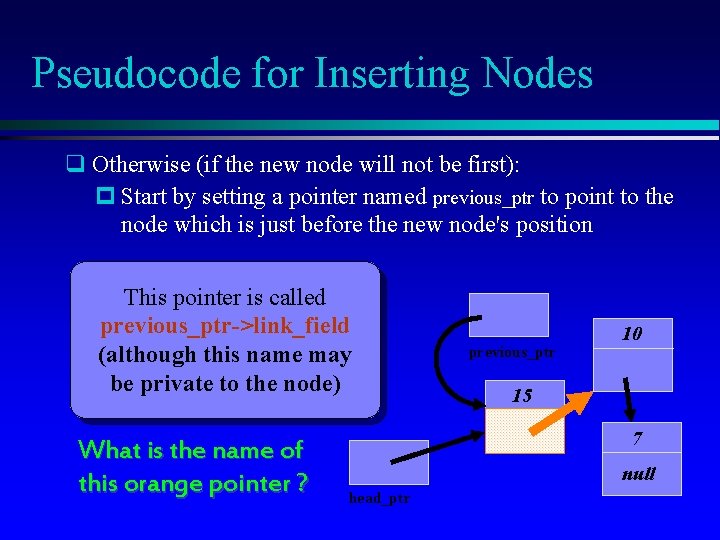
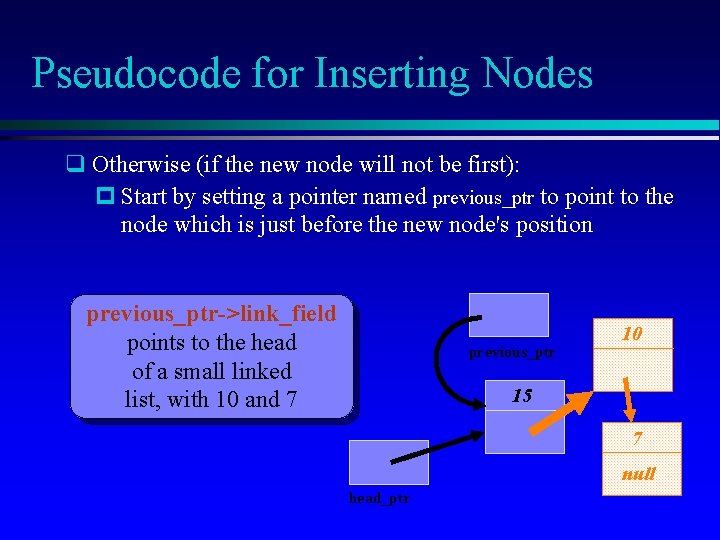
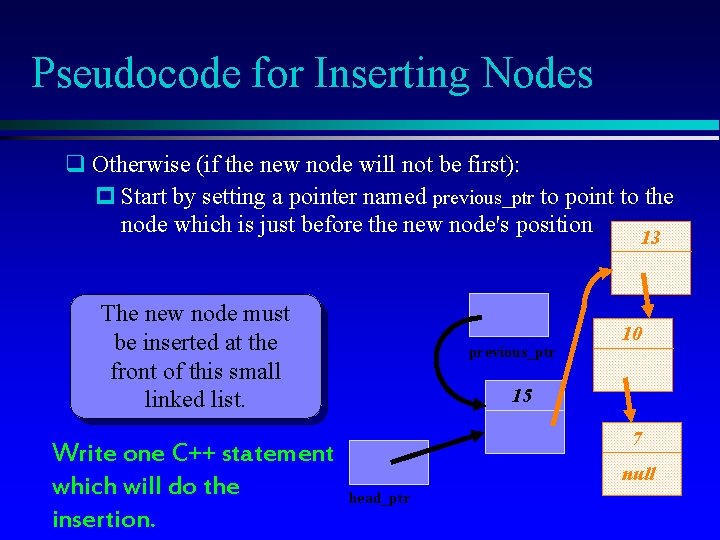
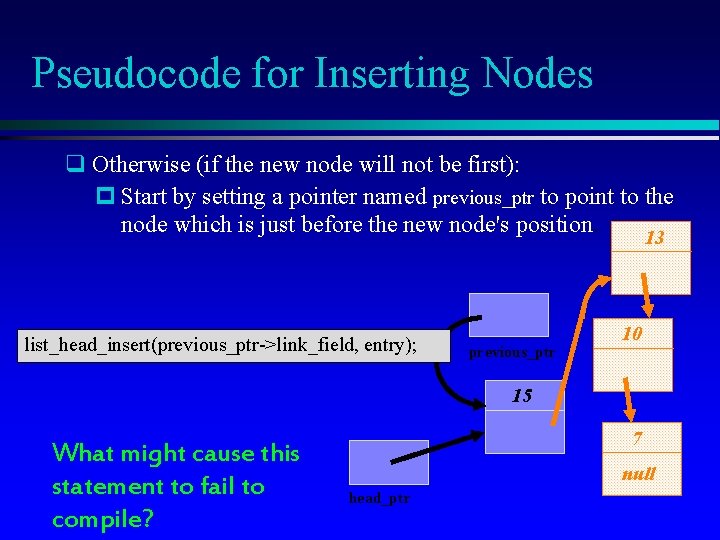
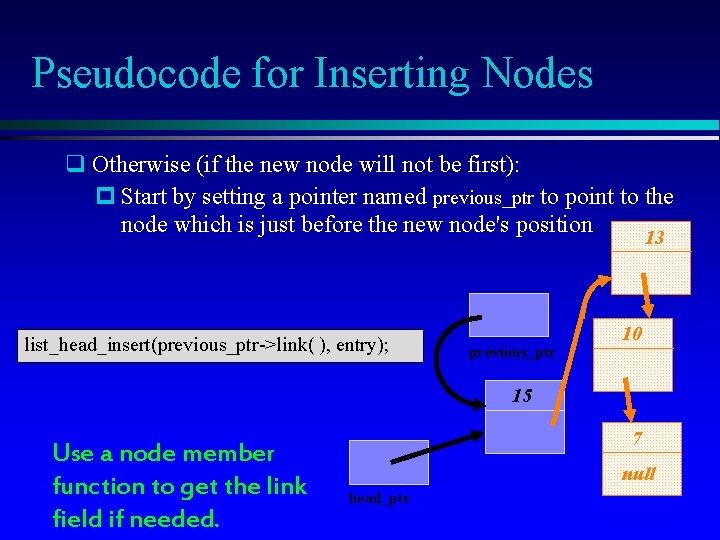
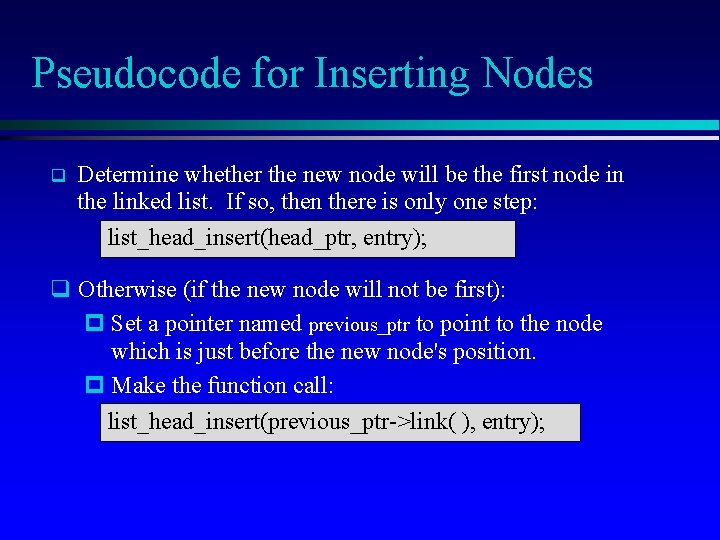
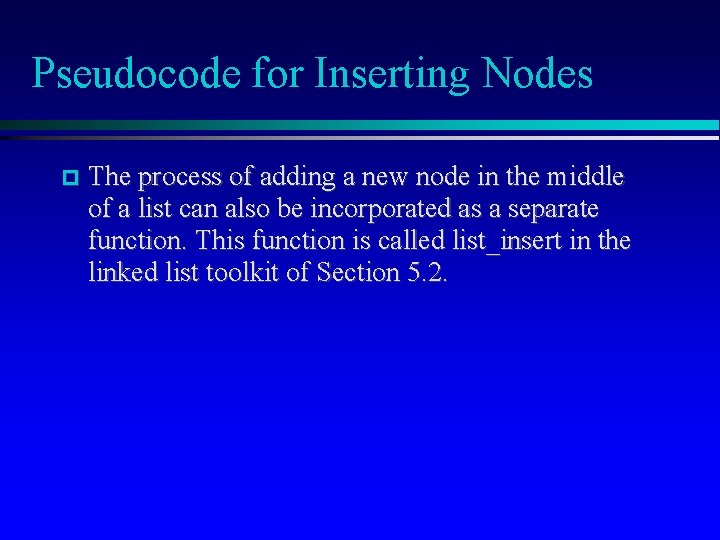
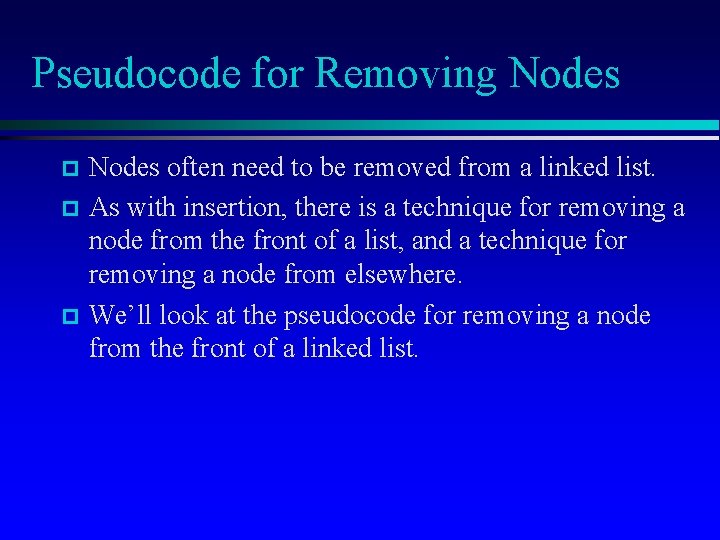
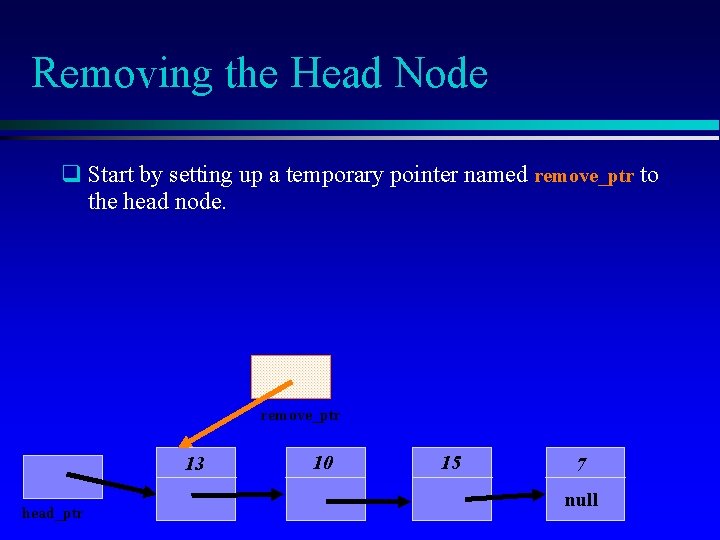
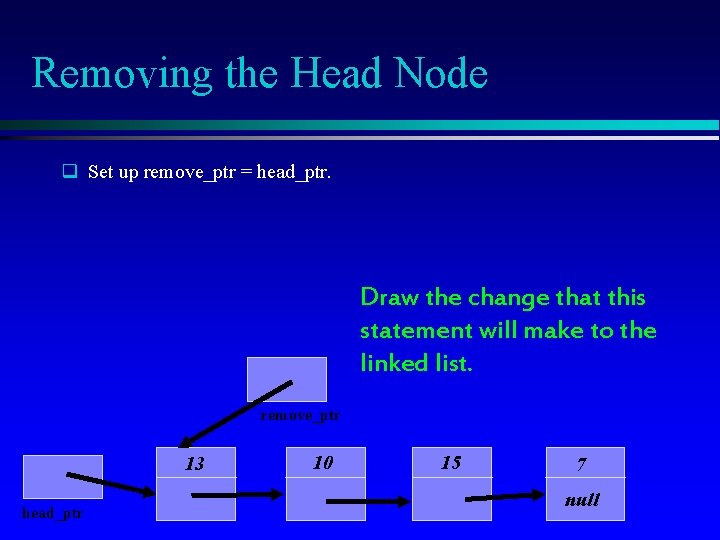
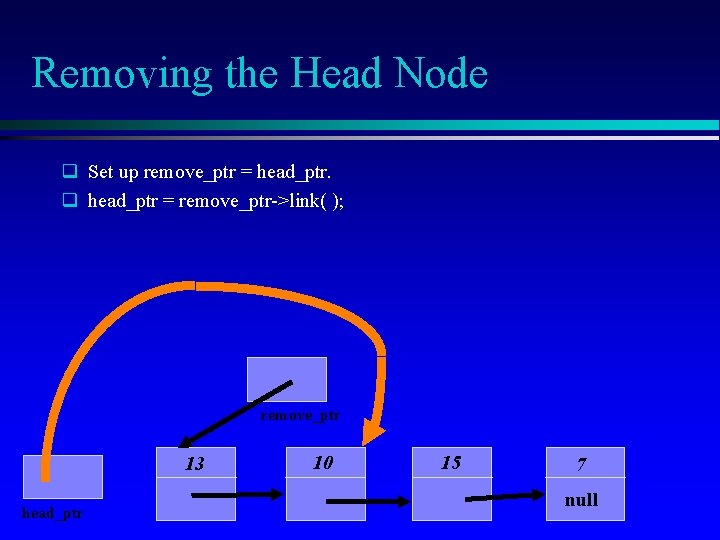
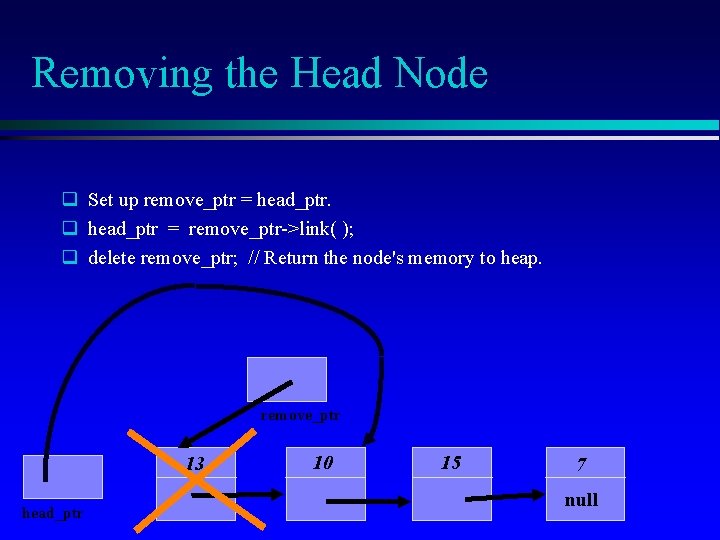
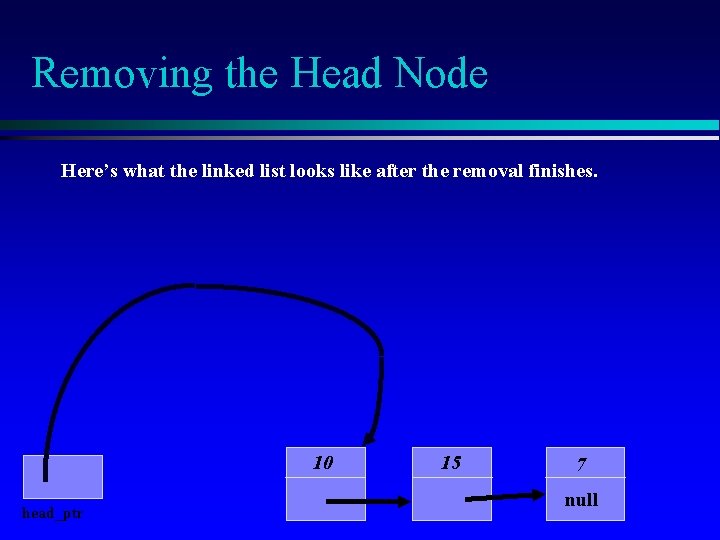
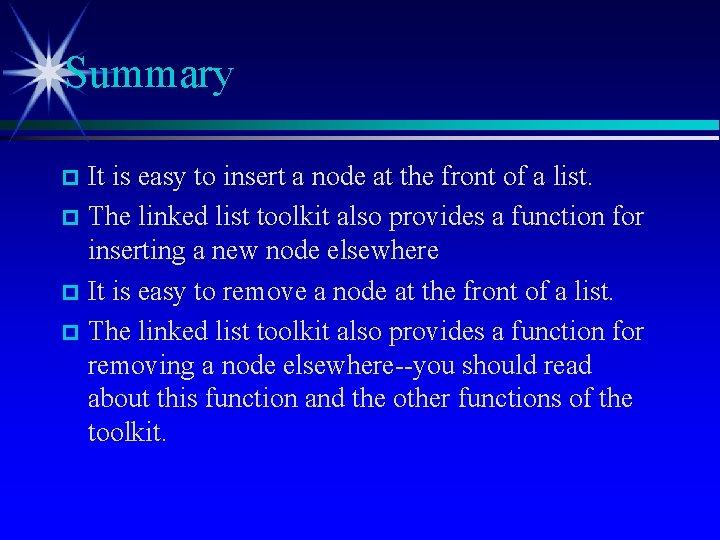
- Slides: 48
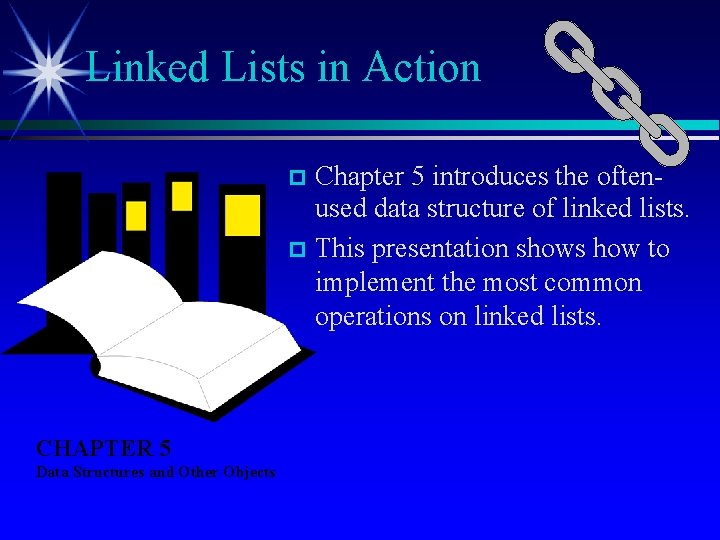
Linked Lists in Action Chapter 5 introduces the oftenused data structure of linked lists. This presentation shows how to implement the most common operations on linked lists. CHAPTER 5 Data Structures and Other Objects
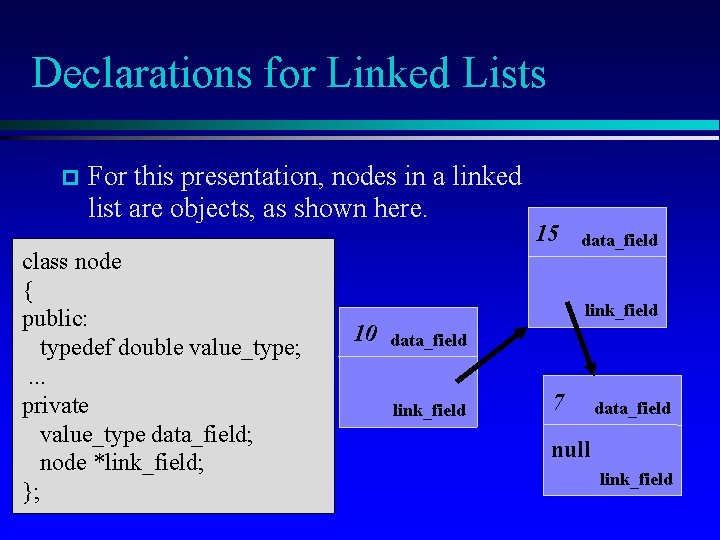
Declarations for Linked Lists For this presentation, nodes in a linked list are objects, as shown here. class node { public: typedef double value_type; . . . private value_type data_field; node *link_field; }; 10 15 data_field link_field 7 data_field null link_field
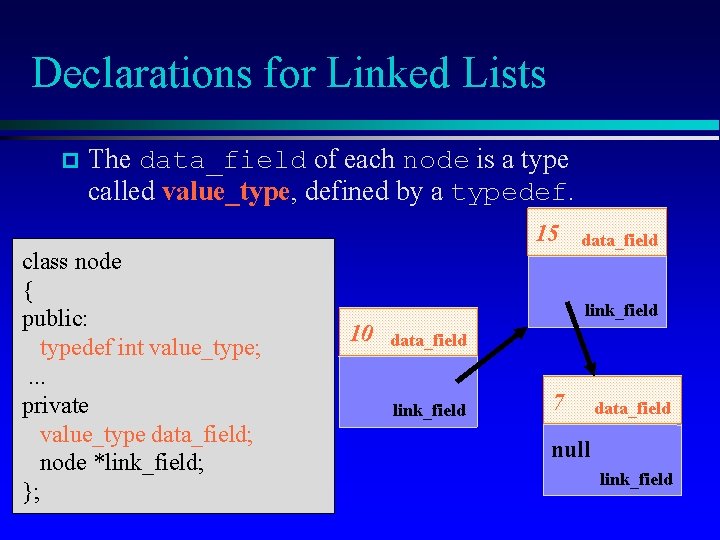
Declarations for Linked Lists The data_field of each node is a type called value_type, defined by a typedef. 15 class node { public: typedef int value_type; . . . private value_type data_field; node *link_field; }; 10 data_field link_field 7 data_field null link_field
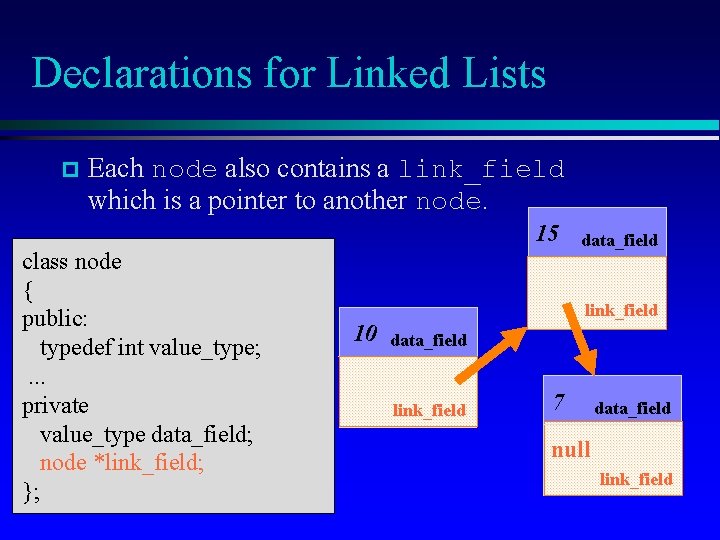
Declarations for Linked Lists Each node also contains a link_field which is a pointer to another node. 15 class node { public: typedef int value_type; . . . private value_type data_field; node *link_field; }; 10 data_field link_field 7 data_field null link_field
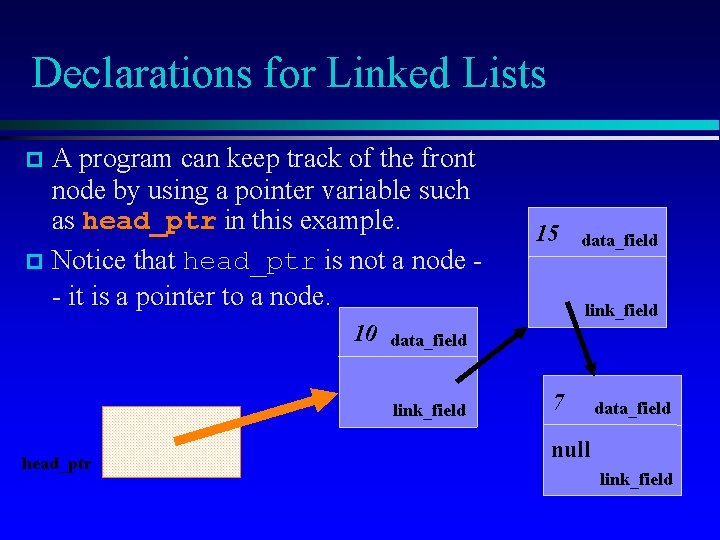
Declarations for Linked Lists A program can keep track of the front node by using a pointer variable such as head_ptr in this example. Notice that head_ptr is not a node - it is a pointer to a node. 10 data_field link_field head_ptr 15 7 data_field null link_field
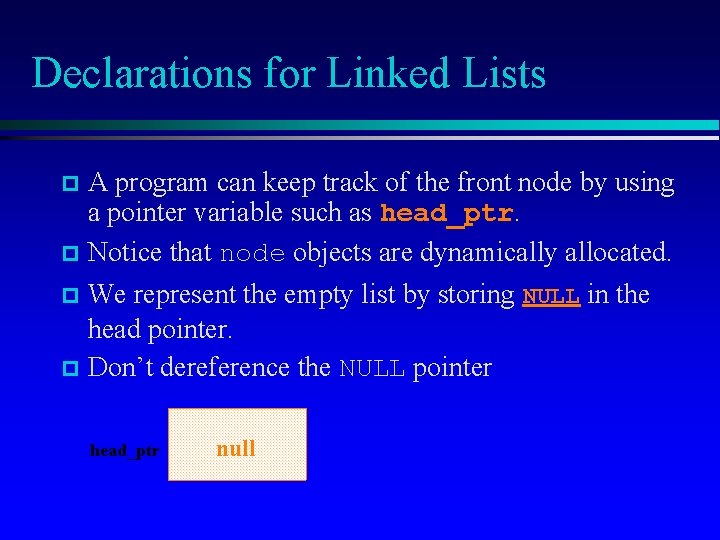
Declarations for Linked Lists A program can keep track of the front node by using a pointer variable such as head_ptr. Notice that node objects are dynamically allocated. We represent the empty list by storing NULL in the head pointer. Don’t dereference the NULL pointer head_ptr null
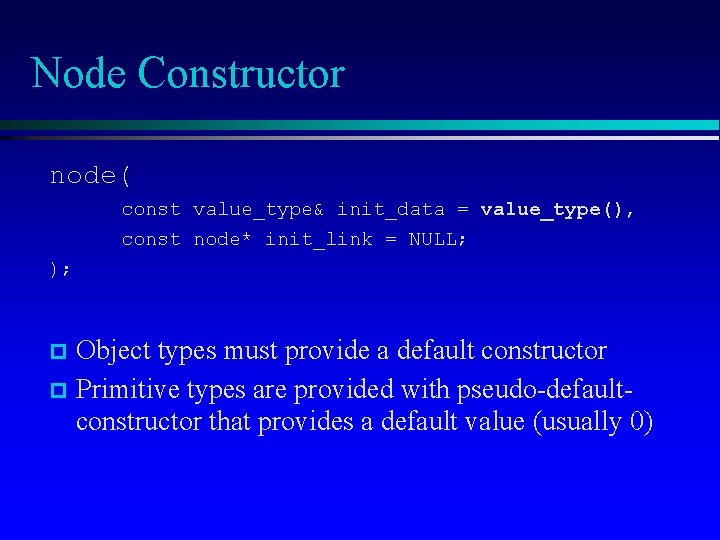
Node Constructor node( const value_type& init_data = value_type(), const node* init_link = NULL; ); Object types must provide a default constructor Primitive types are provided with pseudo-defaultconstructor that provides a default value (usually 0)
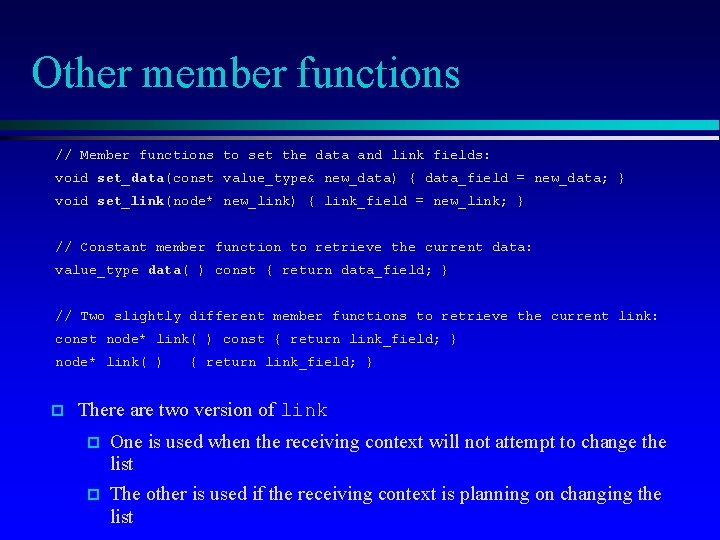
Other member functions // Member functions to set the data and link fields: void set_data(const value_type& new_data) { data_field = new_data; } void set_link(node* new_link) { link_field = new_link; } // Constant member function to retrieve the current data: value_type data( ) const { return data_field; } // Two slightly different member functions to retrieve the current link: const node* link( ) const { return link_field; } node* link( ) { return link_field; } There are two version of link One is used when the receiving context will not attempt to change the list The other is used if the receiving context is planning on changing the list
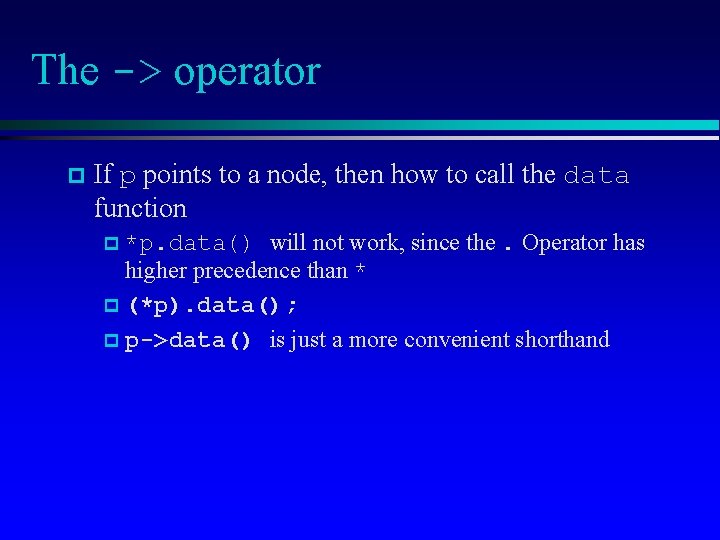
The -> operator If p points to a node, then how to call the data function *p. data() will not work, since the. Operator has higher precedence than * (*p). data(); p->data() is just a more convenient shorthand
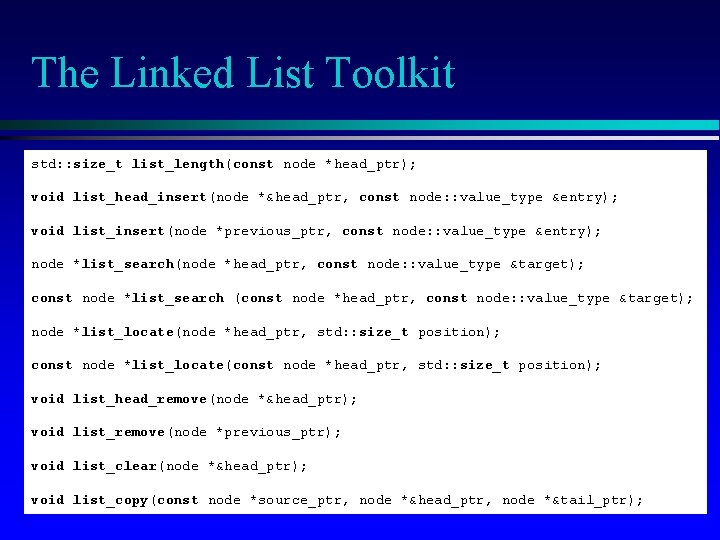
The Linked List Toolkit std: : size_t list_length(const node *head_ptr); void list_head_insert(node *&head_ptr, const node: : value_type &entry); void list_insert(node *previous_ptr, const node: : value_type &entry); node *list_search(node *head_ptr, const node: : value_type &target); const node *list_search (const node *head_ptr, const node: : value_type &target); node *list_locate(node *head_ptr, std: : size_t position); const node *list_locate(const node *head_ptr, std: : size_t position); void list_head_remove(node *&head_ptr); void list_remove(node *previous_ptr); void list_clear(node *&head_ptr); void list_copy(const node *source_ptr, node *&head_ptr, node *&tail_ptr);
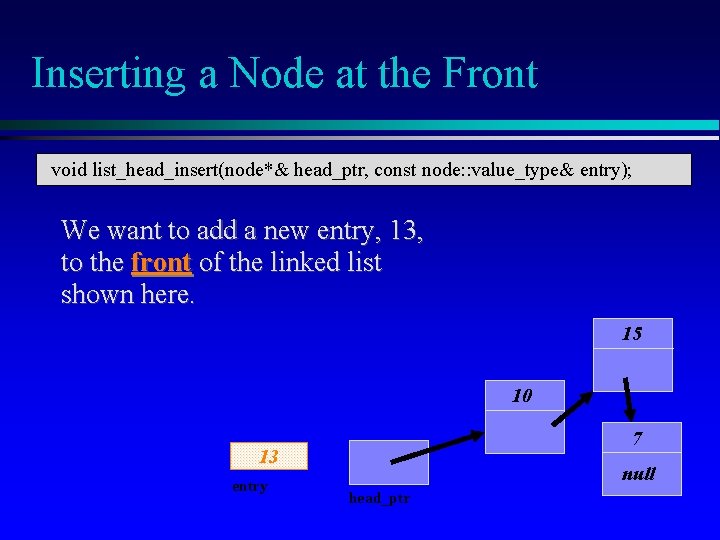
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry); We want to add a new entry, 13, to the front of the linked list shown here. 15 10 7 13 entry null head_ptr
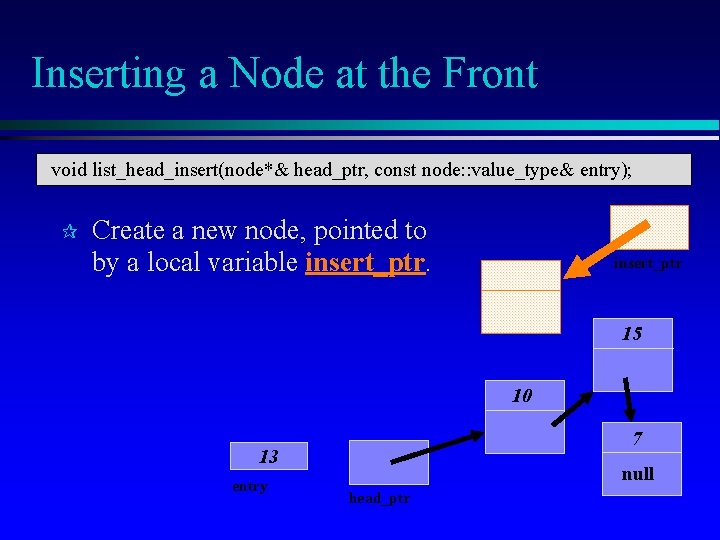
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry); Create a new node, pointed to by a local variable insert_ptr 15 10 7 13 entry null head_ptr
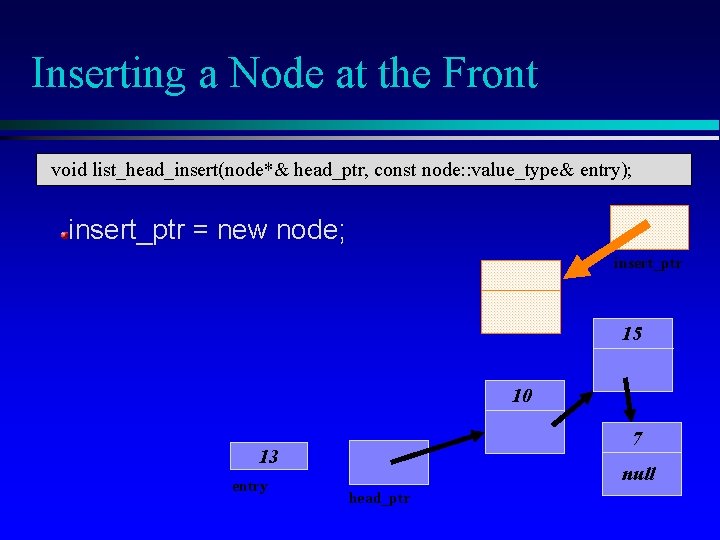
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry); insert_ptr = new node; insert_ptr 15 10 7 13 entry null head_ptr
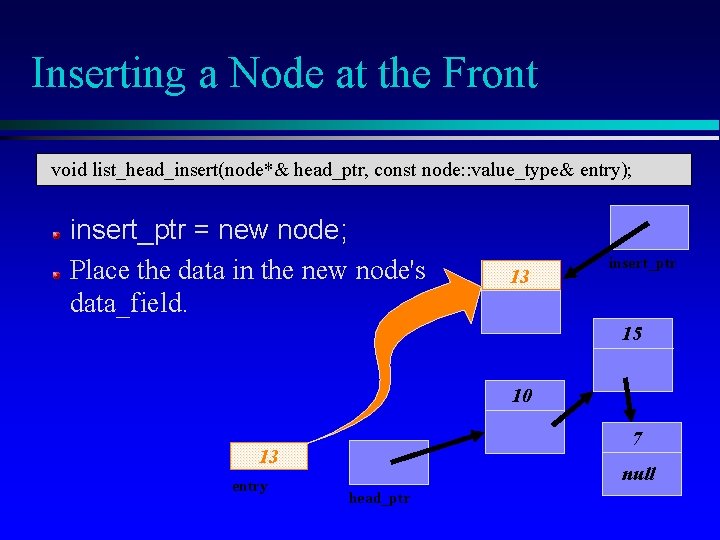
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry); insert_ptr = new node; Place the data in the new node's data_field. 13 insert_ptr 15 10 7 13 entry null head_ptr
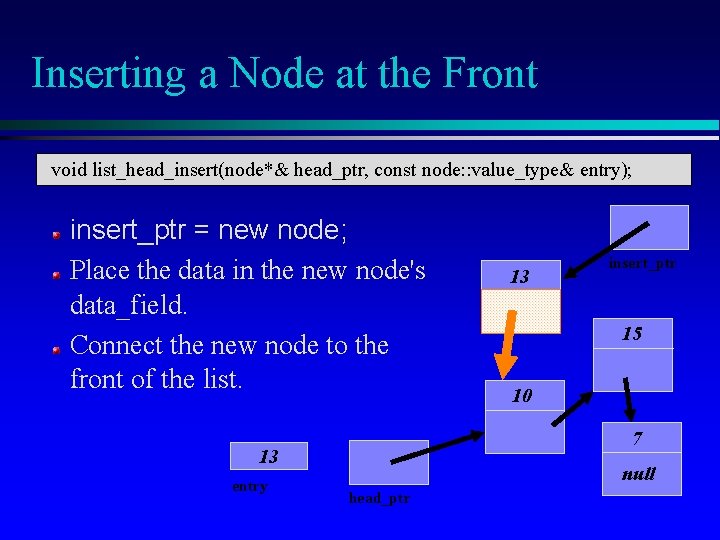
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry); insert_ptr = new node; Place the data in the new node's data_field. Connect the new node to the front of the list. insert_ptr 15 10 7 13 entry 13 null head_ptr
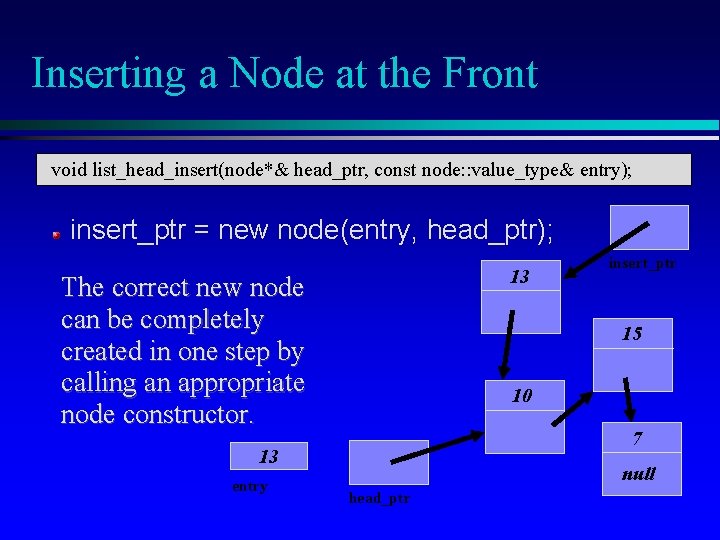
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry); insert_ptr = new node(entry, head_ptr); 13 The correct new node can be completely created in one step by calling an appropriate node constructor. 15 10 7 13 entry insert_ptr null head_ptr
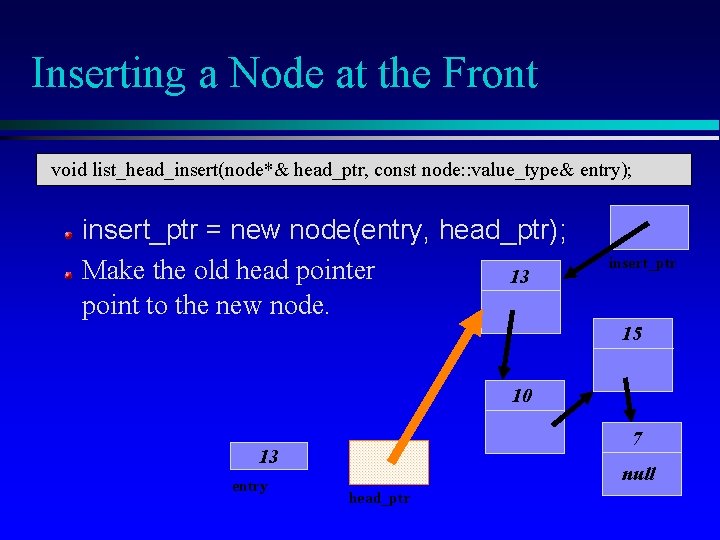
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry); insert_ptr = new node(entry, head_ptr); Make the old head pointer 13 point to the new node. insert_ptr 15 10 7 13 entry null head_ptr
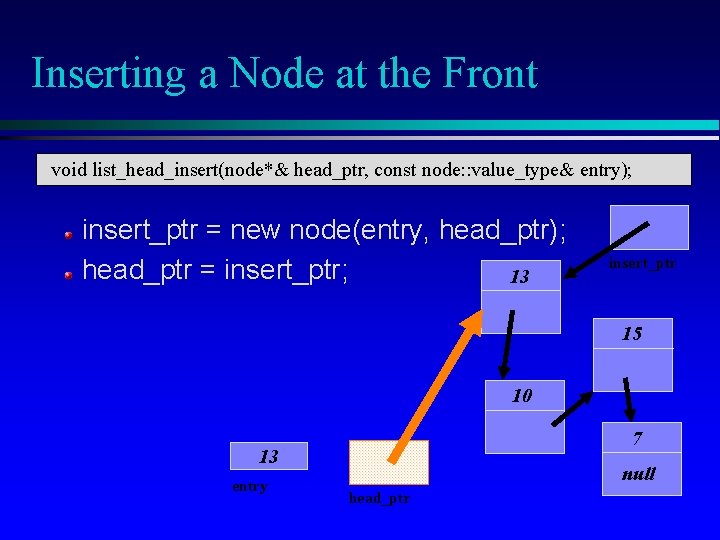
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry); insert_ptr = new node(entry, head_ptr); head_ptr = insert_ptr; 13 insert_ptr 15 10 7 13 entry null head_ptr
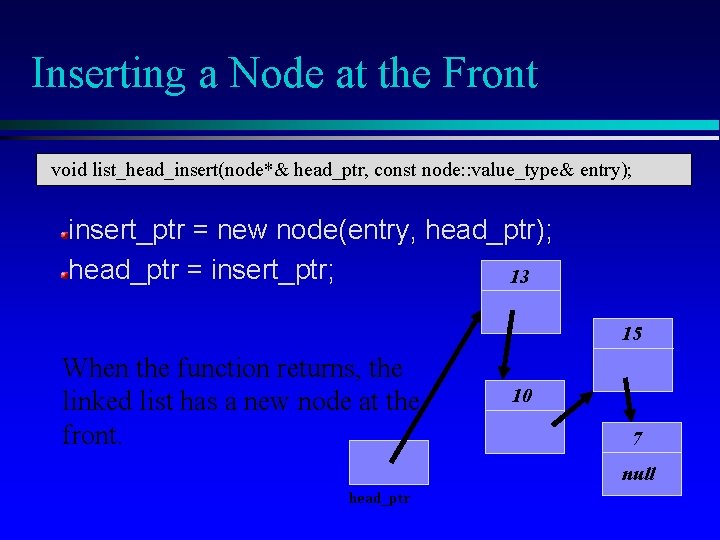
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry); insert_ptr = new node(entry, head_ptr); head_ptr = insert_ptr; 13 15 When the function returns, the linked list has a new node at the front. 10 7 null head_ptr
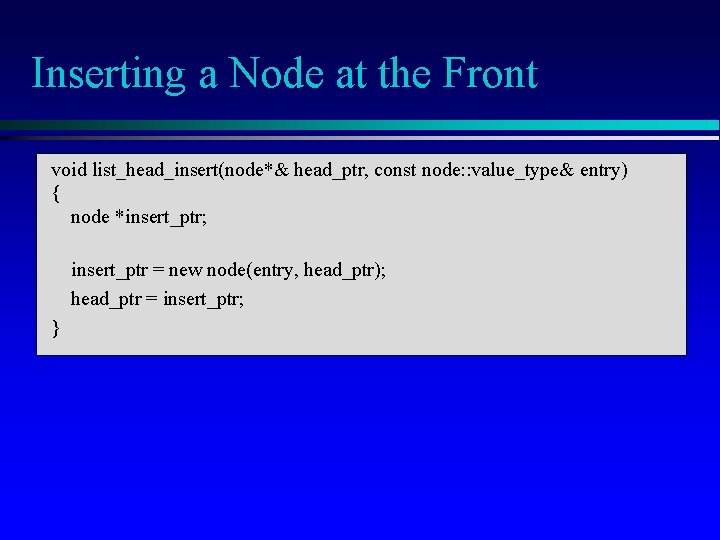
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry) { node *insert_ptr; insert_ptr = new node(entry, head_ptr); head_ptr = insert_ptr; }
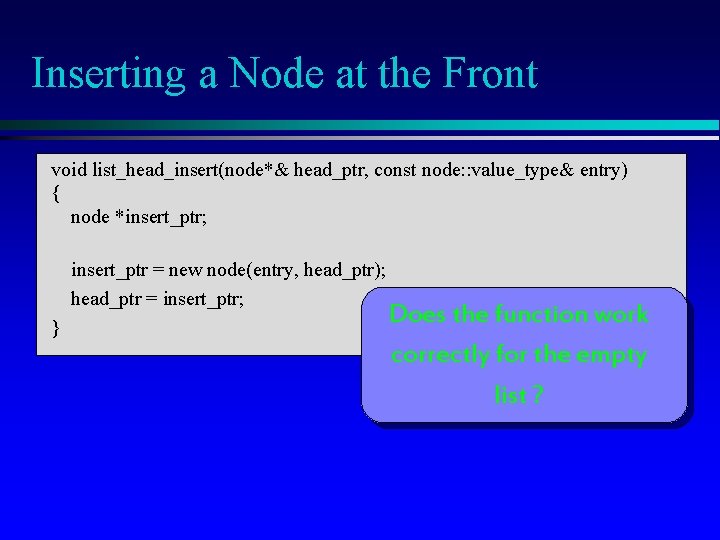
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry) { node *insert_ptr; insert_ptr = new node(entry, head_ptr); head_ptr = insert_ptr; } Does the function work correctly for the empty list ?
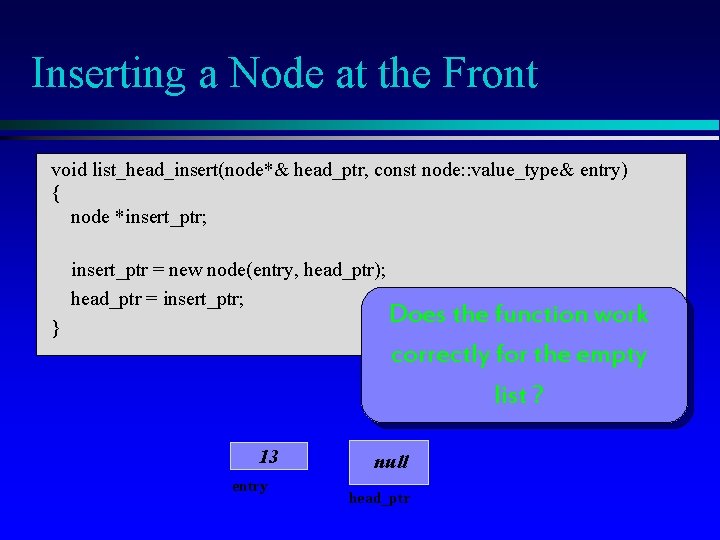
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry) { node *insert_ptr; insert_ptr = new node(entry, head_ptr); head_ptr = insert_ptr; } 13 entry Does the function work correctly for the empty list ? null head_ptr
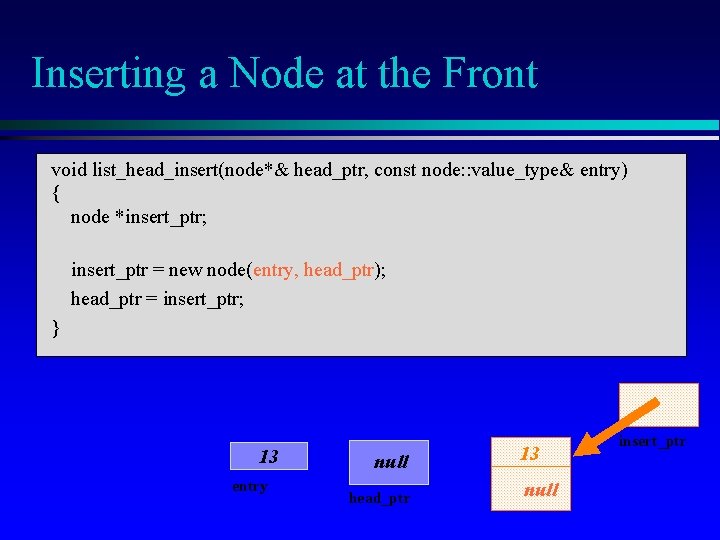
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry) { node *insert_ptr; insert_ptr = new node(entry, head_ptr); head_ptr = insert_ptr; } 13 entry null head_ptr 13 null insert_ptr
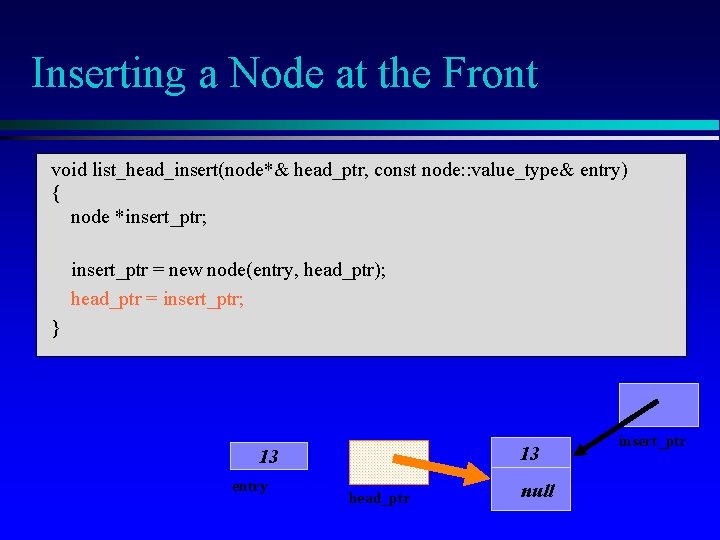
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry) { node *insert_ptr; insert_ptr = new node(entry, head_ptr); head_ptr = insert_ptr; } 13 13 entry head_ptr null insert_ptr
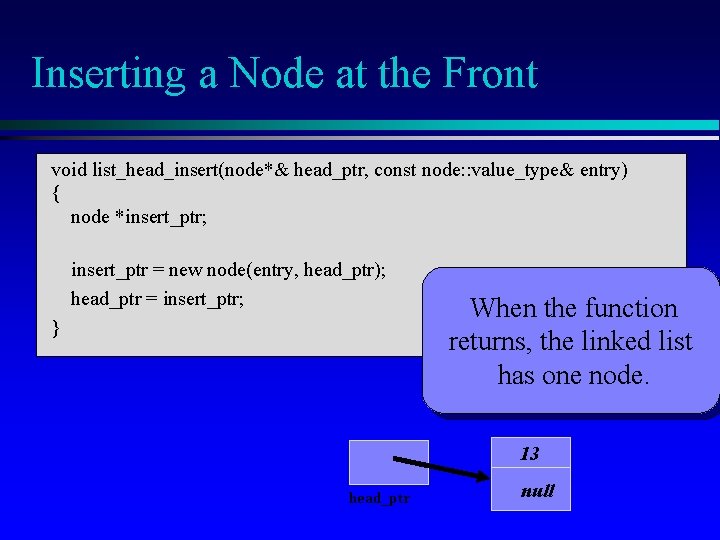
Inserting a Node at the Front void list_head_insert(node*& head_ptr, const node: : value_type& entry) { node *insert_ptr; insert_ptr = new node(entry, head_ptr); head_ptr = insert_ptr; } When the function returns, the linked list has one node. 13 head_ptr null
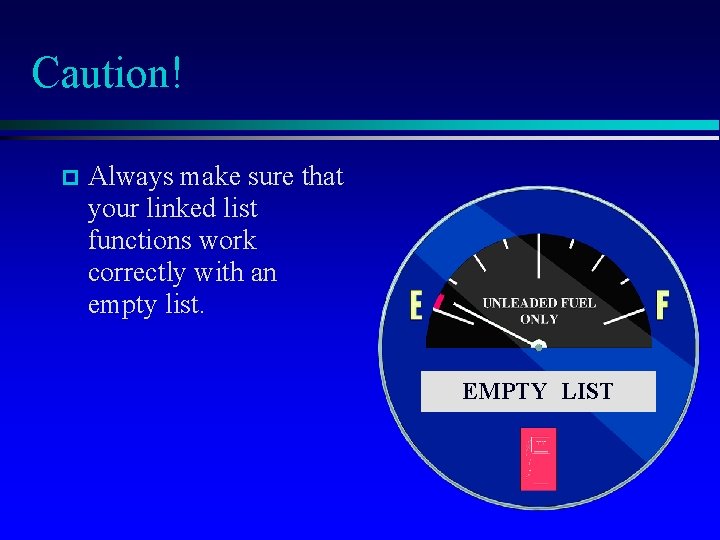
Caution! Always make sure that your linked list functions work correctly with an empty list. EMPTY LIST
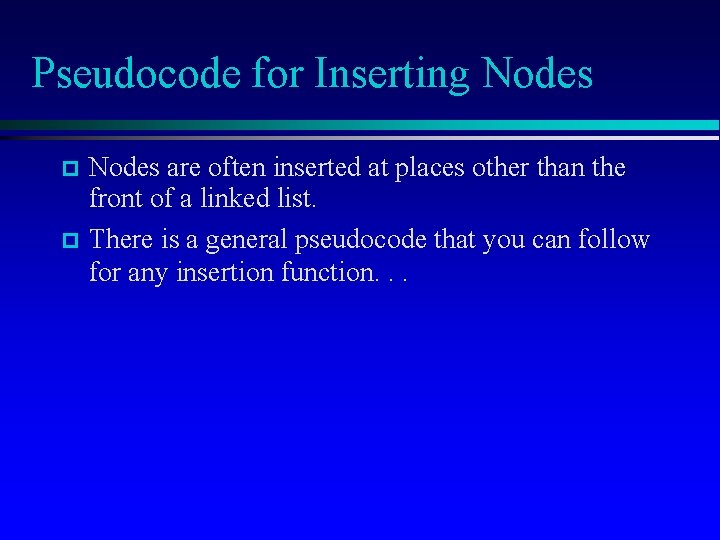
Pseudocode for Inserting Nodes are often inserted at places other than the front of a linked list. There is a general pseudocode that you can follow for any insertion function. . .
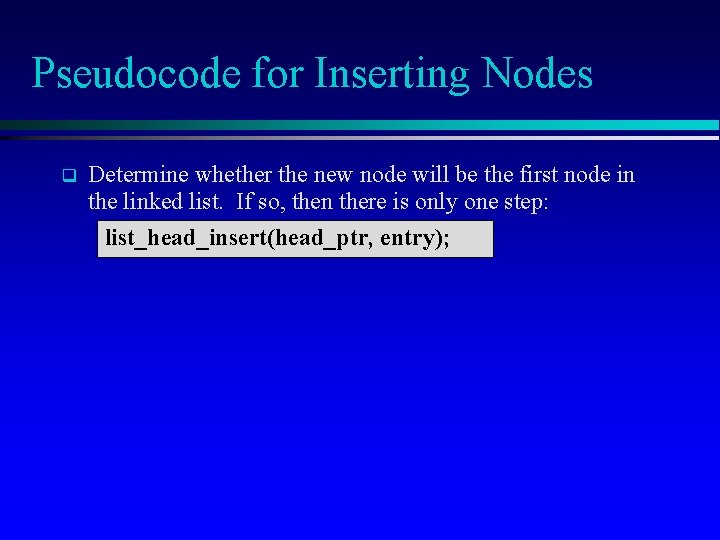
Pseudocode for Inserting Nodes q Determine whether the new node will be the first node in the linked list. If so, then there is only one step: list_head_insert(head_ptr, entry);
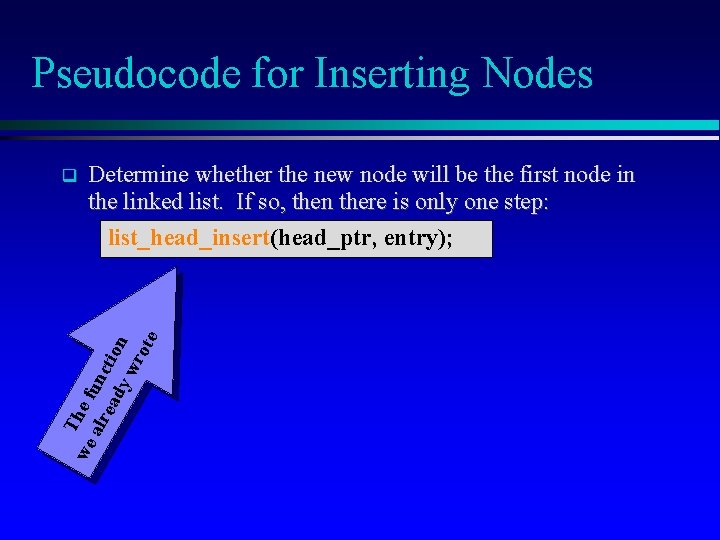
Pseudocode for Inserting Nodes Determine whether the new node will be the first node in the linked list. If so, then there is only one step: list_head_insert(head_ptr, entry); T we he f alr unc ead tio yw n rot e q
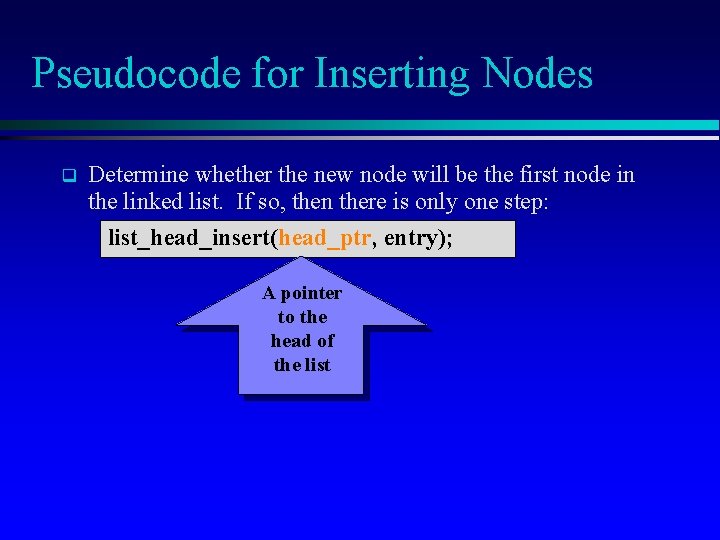
Pseudocode for Inserting Nodes q Determine whether the new node will be the first node in the linked list. If so, then there is only one step: list_head_insert(head_ptr, entry); A pointer to the head of the list
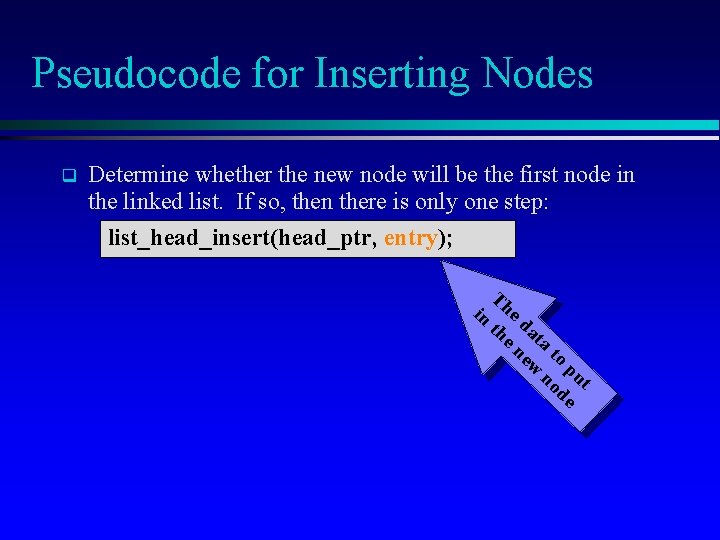
Pseudocode for Inserting Nodes q Determine whether the new node will be the first node in the linked list. If so, then there is only one step: list_head_insert(head_ptr, entry); Th in e th dat en a ew to no put de
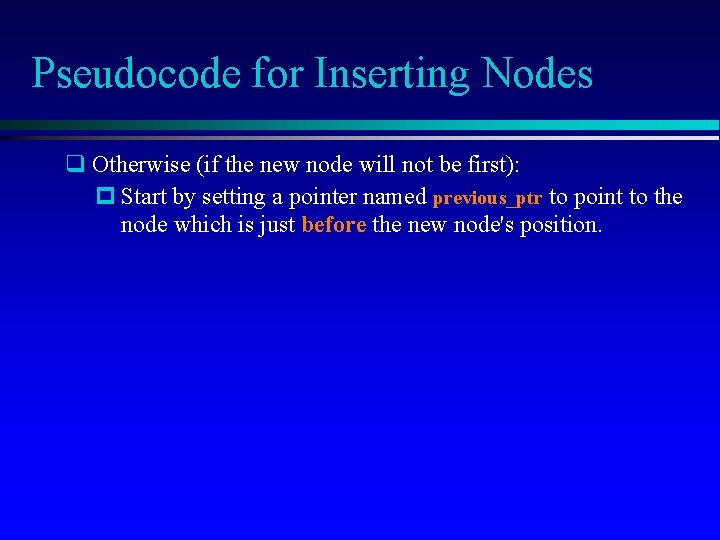
Pseudocode for Inserting Nodes q Otherwise (if the new node will not be first): Start by setting a pointer named previous_ptr to point to the node which is just before the new node's position.
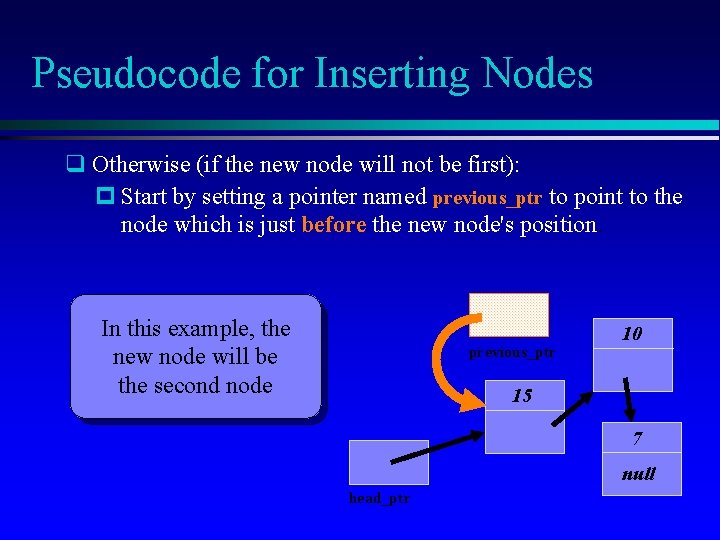
Pseudocode for Inserting Nodes q Otherwise (if the new node will not be first): Start by setting a pointer named previous_ptr to point to the node which is just before the new node's position. In this example, the new node will be the second node previous_ptr 10 15 7 null head_ptr
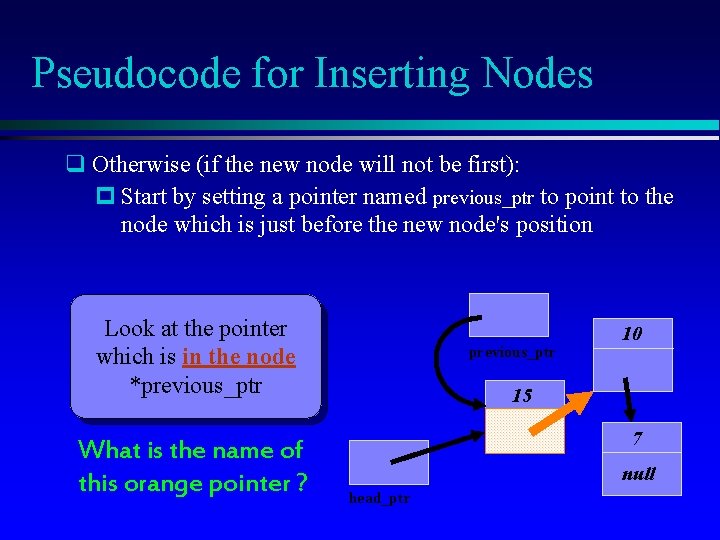
Pseudocode for Inserting Nodes q Otherwise (if the new node will not be first): Start by setting a pointer named previous_ptr to point to the node which is just before the new node's position Look at the pointer which is in the node *previous_ptr What is the name of this orange pointer ? previous_ptr 10 15 7 null head_ptr
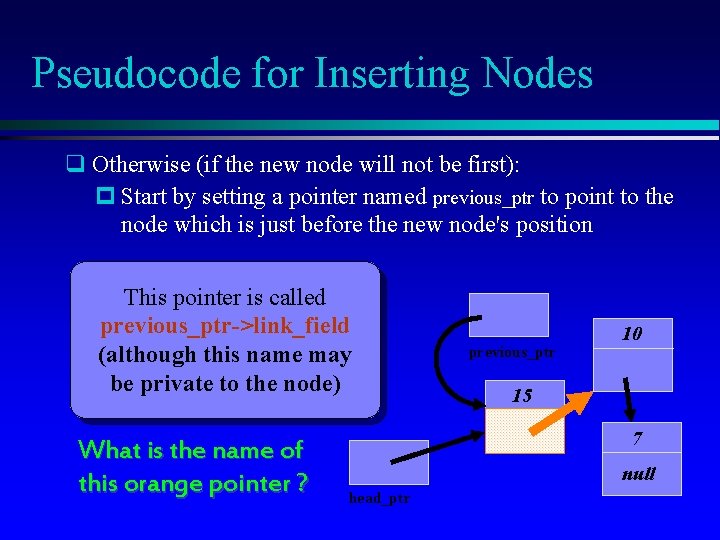
Pseudocode for Inserting Nodes q Otherwise (if the new node will not be first): Start by setting a pointer named previous_ptr to point to the node which is just before the new node's position This pointer is called previous_ptr->link_field (although this name may be private to the node) What is the name of this orange pointer ? previous_ptr 10 15 7 null head_ptr
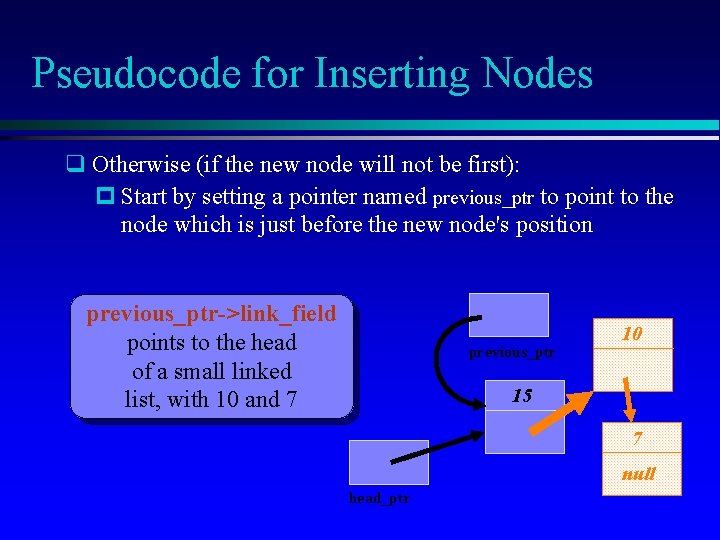
Pseudocode for Inserting Nodes q Otherwise (if the new node will not be first): Start by setting a pointer named previous_ptr to point to the node which is just before the new node's position previous_ptr->link_field points to the head of a small linked list, with 10 and 7 previous_ptr 10 15 7 null head_ptr
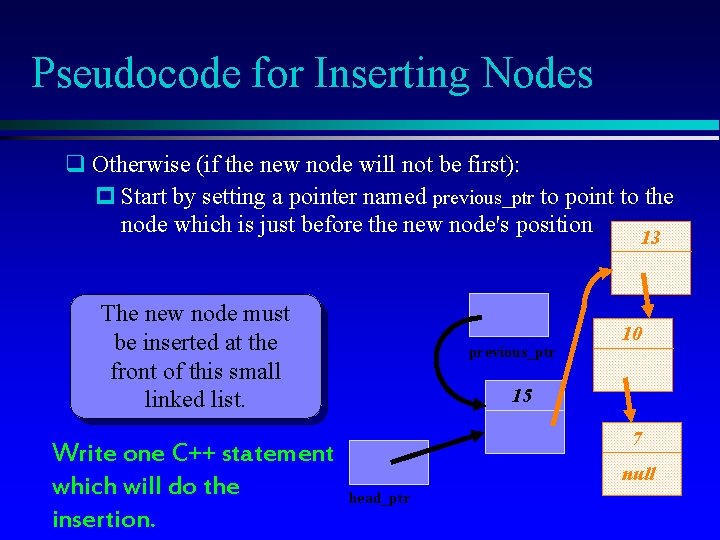
Pseudocode for Inserting Nodes q Otherwise (if the new node will not be first): Start by setting a pointer named previous_ptr to point to the node which is just before the new node's position. 13 The new node must be inserted at the front of this small linked list. Write one C++ statement which will do the insertion. previous_ptr 10 15 7 null head_ptr
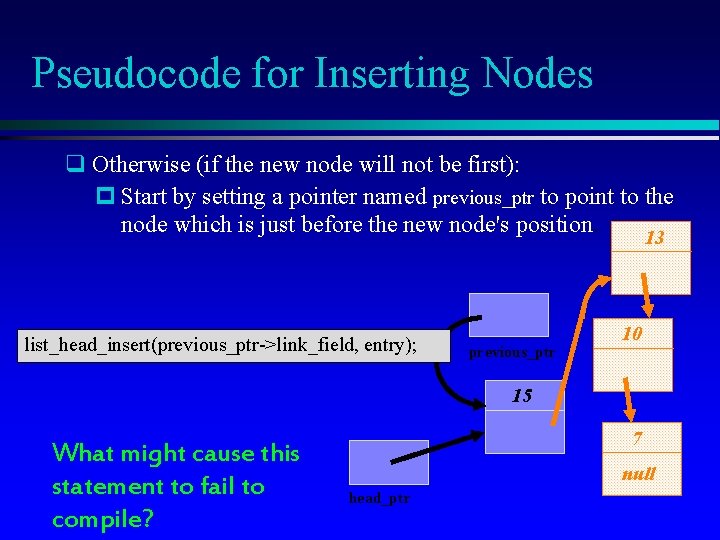
Pseudocode for Inserting Nodes q Otherwise (if the new node will not be first): Start by setting a pointer named previous_ptr to point to the node which is just before the new node's position. 13 list_head_insert(previous_ptr->link_field, entry); previous_ptr 10 15 What might cause this statement to fail to compile? 7 null head_ptr
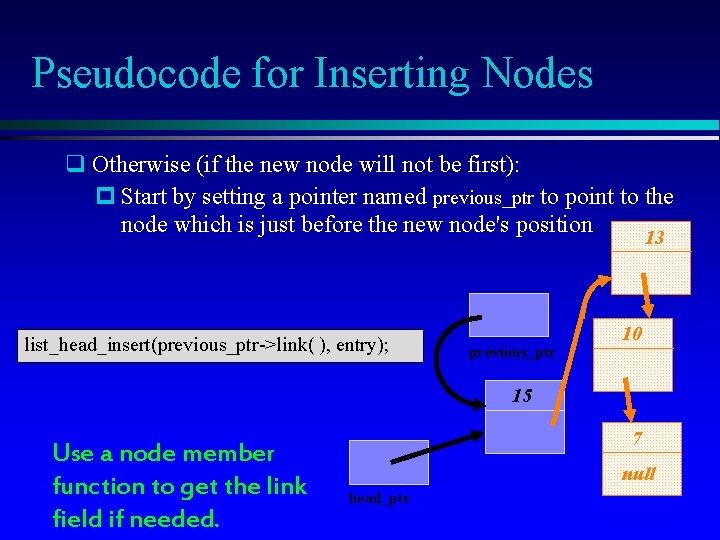
Pseudocode for Inserting Nodes q Otherwise (if the new node will not be first): Start by setting a pointer named previous_ptr to point to the node which is just before the new node's position. 13 list_head_insert(previous_ptr->link( ), entry); previous_ptr 10 15 Use a node member function to get the link field if needed. 7 null head_ptr
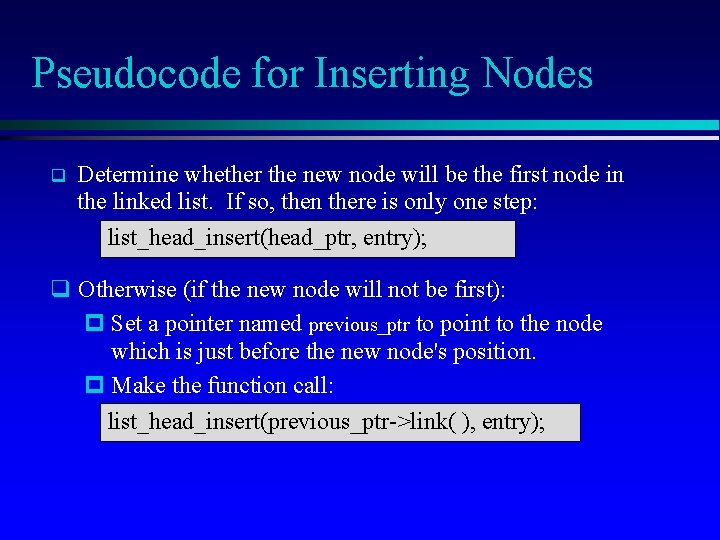
Pseudocode for Inserting Nodes q Determine whether the new node will be the first node in the linked list. If so, then there is only one step: list_head_insert(head_ptr, entry); q Otherwise (if the new node will not be first): Set a pointer named previous_ptr to point to the node which is just before the new node's position. Make the function call: list_head_insert(previous_ptr->link( ), entry);
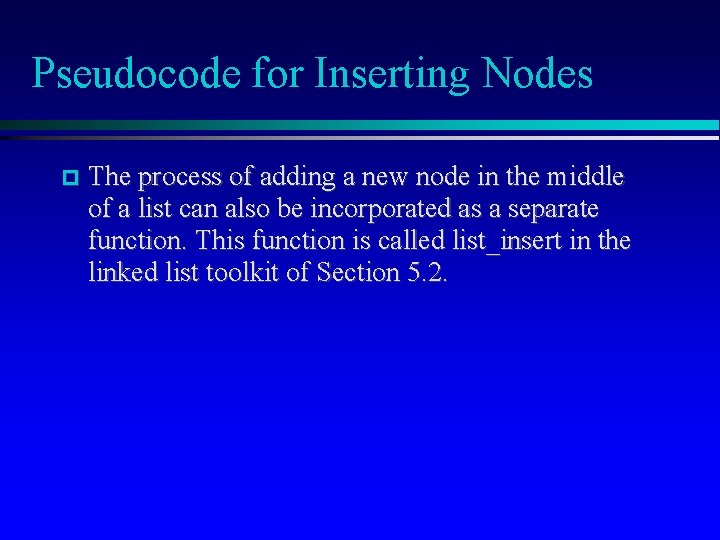
Pseudocode for Inserting Nodes The process of adding a new node in the middle of a list can also be incorporated as a separate function. This function is called list_insert in the linked list toolkit of Section 5. 2.
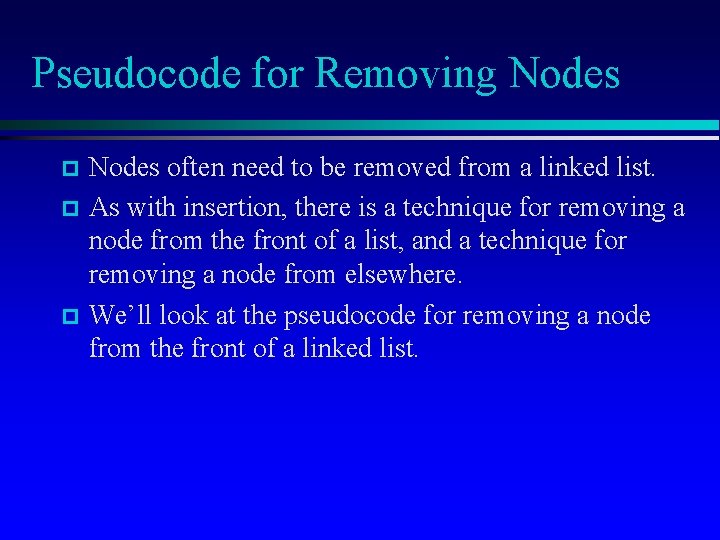
Pseudocode for Removing Nodes often need to be removed from a linked list. As with insertion, there is a technique for removing a node from the front of a list, and a technique for removing a node from elsewhere. We’ll look at the pseudocode for removing a node from the front of a linked list.
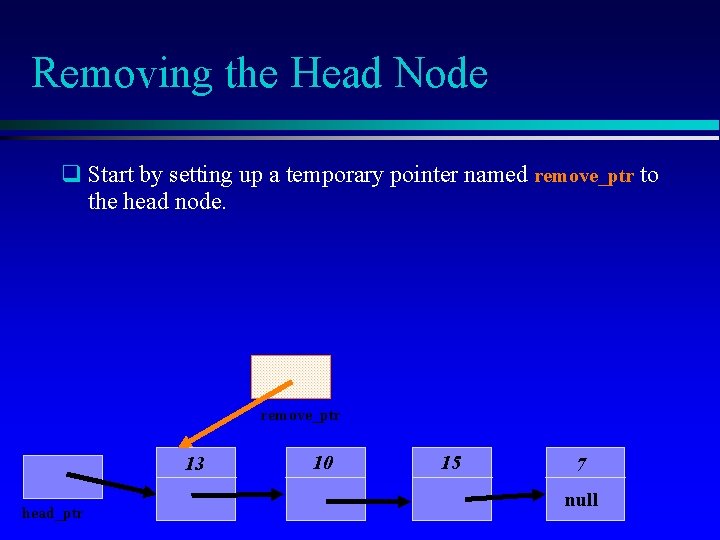
Removing the Head Node q Start by setting up a temporary pointer named remove_ptr to the head node. remove_ptr 13 head_ptr 10 15 7 null
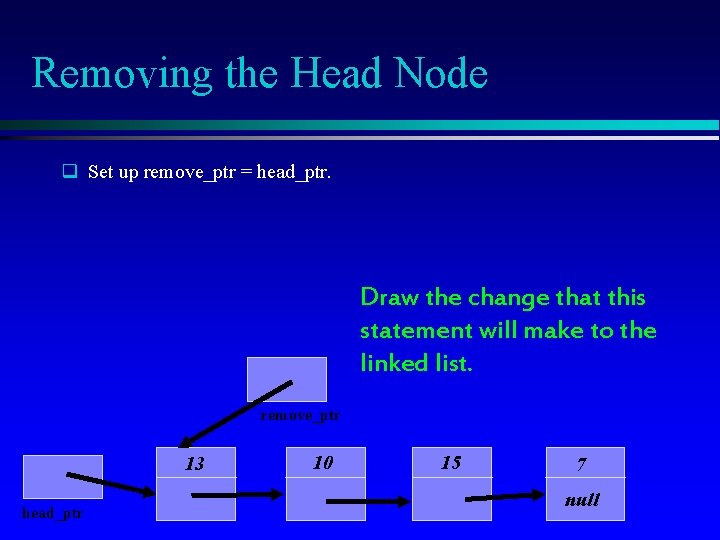
Removing the Head Node q Set up remove_ptr = head_ptr. Draw the change that this statement will make to the linked list. remove_ptr 13 head_ptr 10 15 7 null
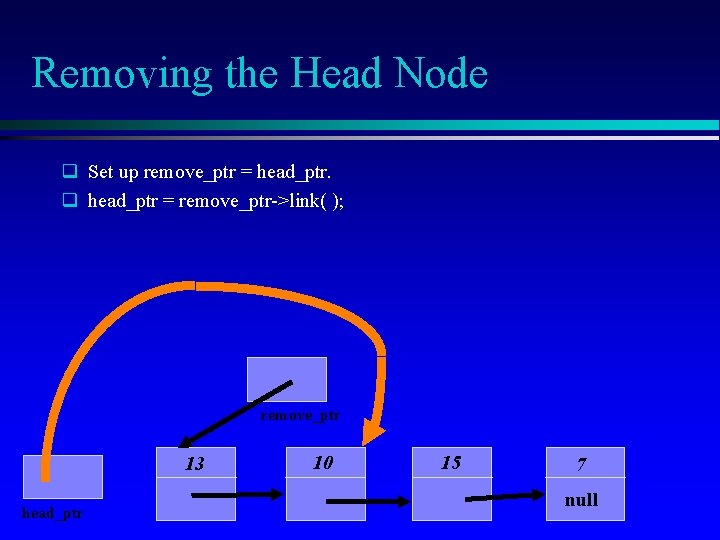
Removing the Head Node q Set up remove_ptr = head_ptr. q head_ptr = remove_ptr->link( ); remove_ptr 13 head_ptr 10 15 7 null
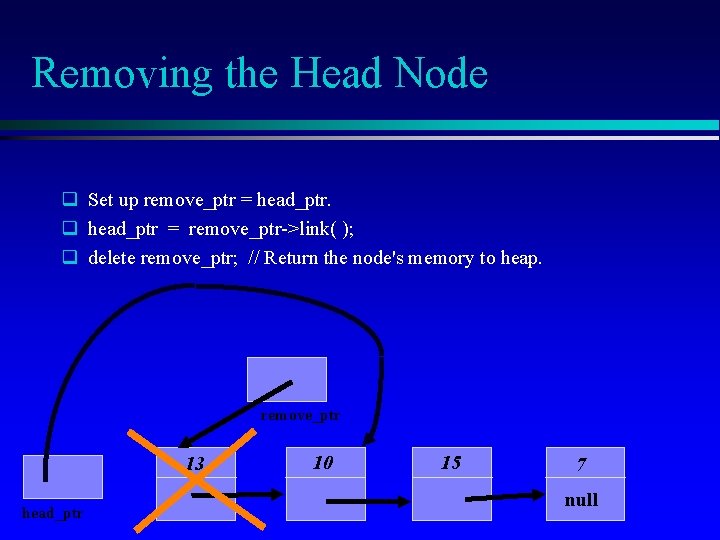
Removing the Head Node q Set up remove_ptr = head_ptr. q head_ptr = remove_ptr->link( ); q delete remove_ptr; // Return the node's memory to heap. remove_ptr 13 head_ptr 10 15 7 null
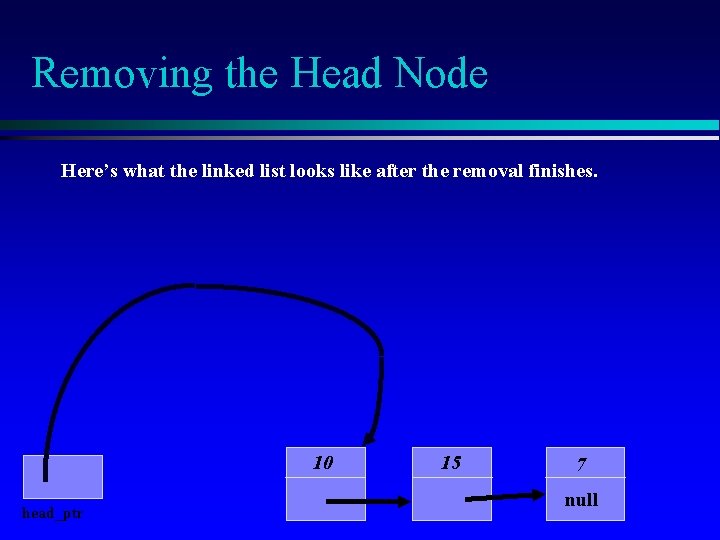
Removing the Head Node Here’s what the linked list looks like after the removal finishes. 10 head_ptr 15 7 null
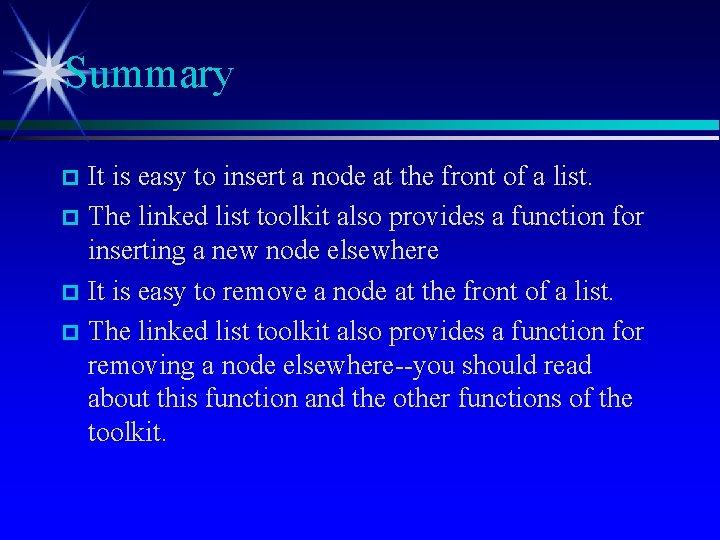
Summary It is easy to insert a node at the front of a list. The linked list toolkit also provides a function for inserting a new node elsewhere It is easy to remove a node at the front of a list. The linked list toolkit also provides a function for removing a node elsewhere--you should read about this function and the other functions of the toolkit.
Singly linked list vs doubly linked list
Singly vs doubly linked list
Perbedaan single linked list dan double linked list
The lion and the mouse plot elements
It introduces the characters and the setting
Cat morgan introduces himself
Language processing system in compiler design
Stalls
Jeep/eagle introduces a new product
Semicolon vs colon
Lesson 3: lists practice
Ow sound
Glwords
Swst spelling lists level 5
Resource lists edinburgh
Concatinates
Lists of tuples python
Lisp lists
Java types of lists
Words their way spelling tests
Read wrote inc
Prolog head tail
Parallel structure example
Python list functions
Inca sun temple of cuzco ap world history
Wish lists year
Political lists new
Qri word lists
No nonsense spelling lists
Wish lists year
"new bookmarking lists 2018"
What is diigo
Political wish lists
Ie words
Ucl library reading lists
Strengths lists
Blockly c
Chapter 22 genetics and genetically linked diseases
Hình ảnh bộ gõ cơ thể búng tay
Bổ thể
Tỉ lệ cơ thể trẻ em
Chó sói
Chụp tư thế worms-breton
Hát lên người ơi alleluia
Các môn thể thao bắt đầu bằng tiếng bóng
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Công thức tính thế năng