Iteration 1 Java looping o o Options n
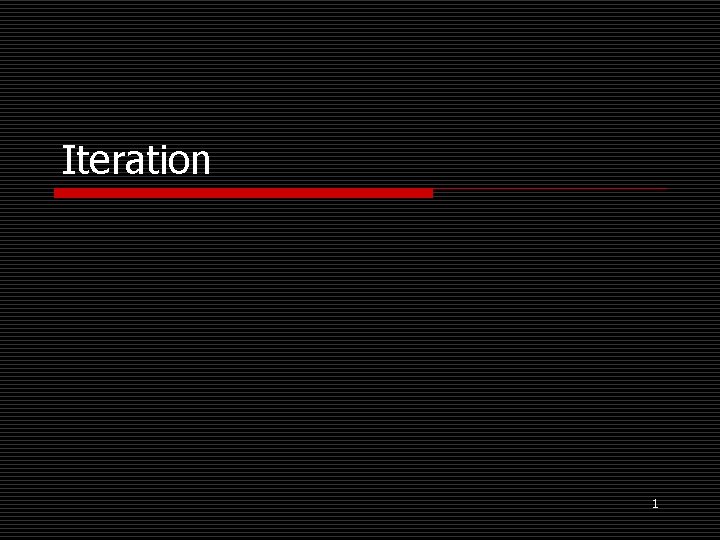
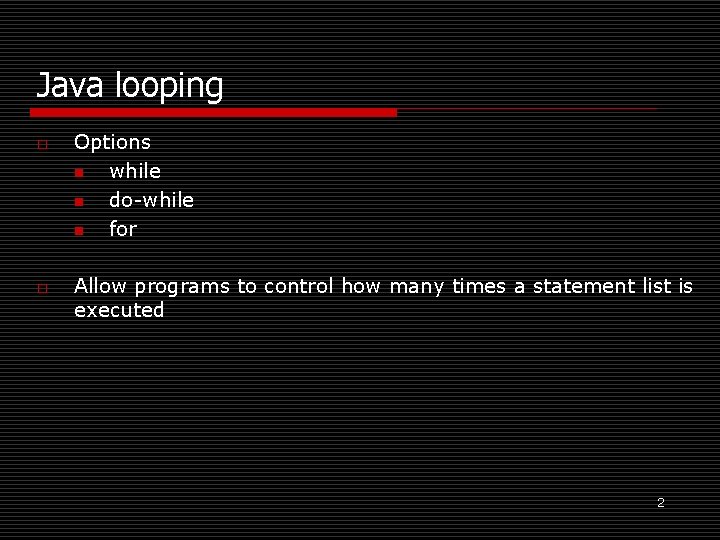
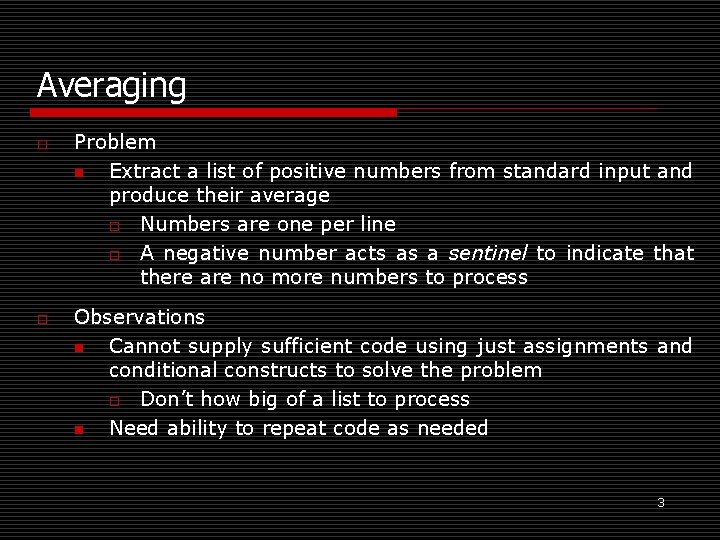
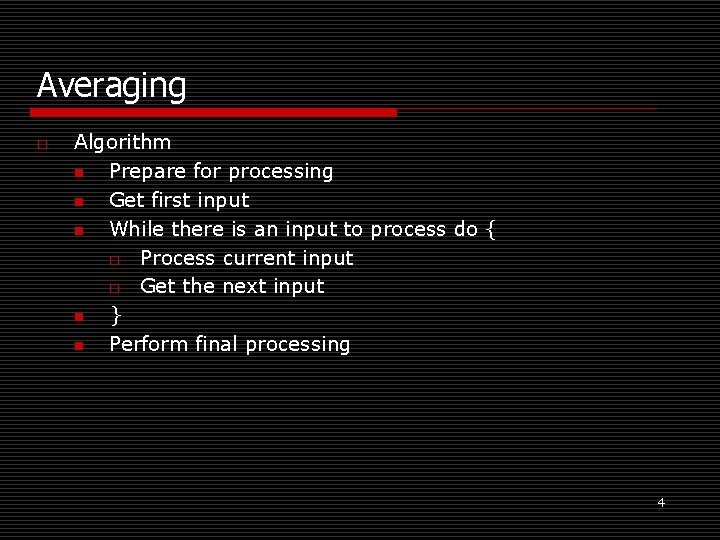
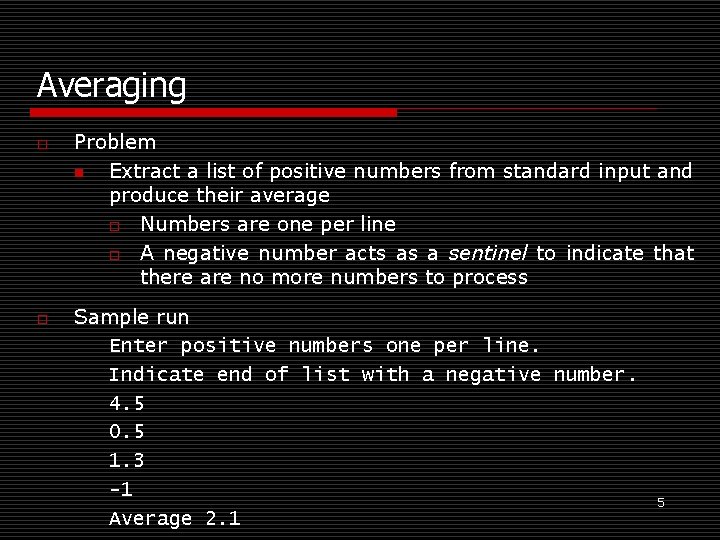
![public class Number. Average { // main(): application entry point public static void main(String[] public class Number. Average { // main(): application entry point public static void main(String[]](https://slidetodoc.com/presentation_image/a4cd213218a235e952dde5b7ca39c5ea/image-6.jpg)
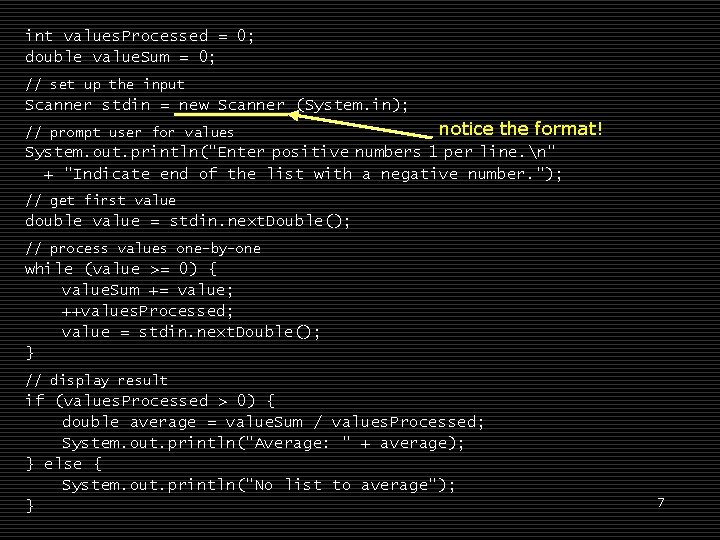
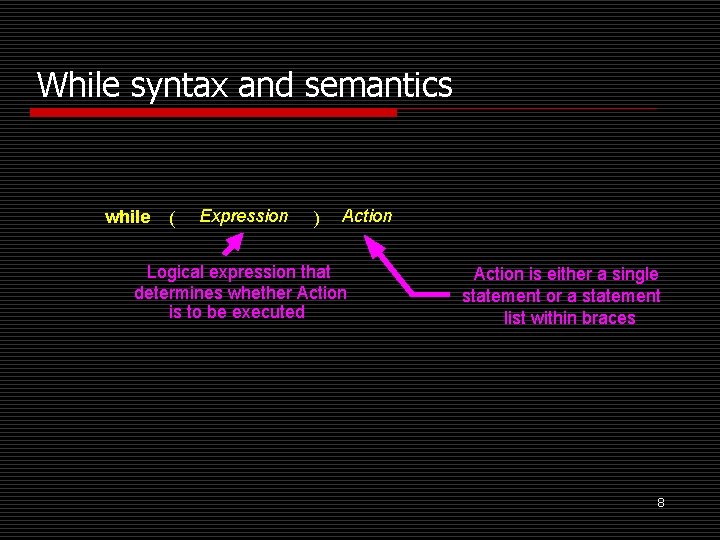
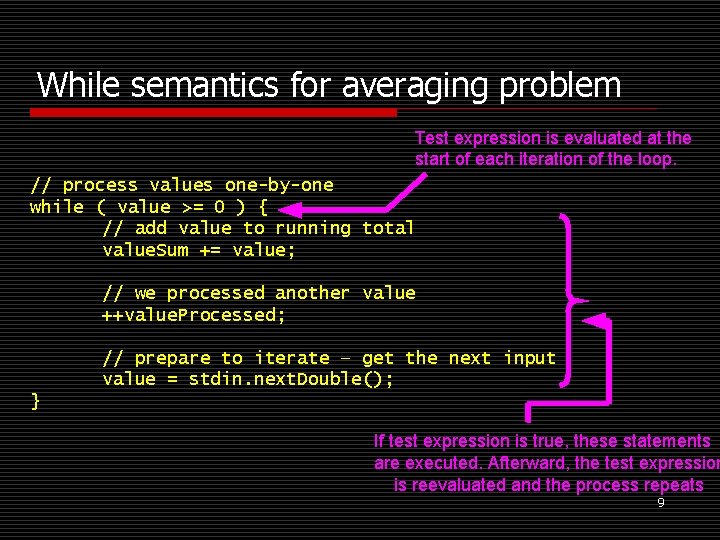
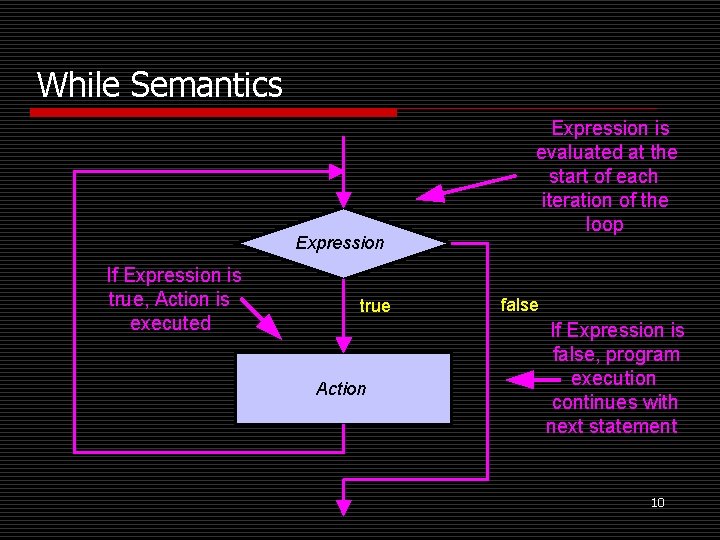
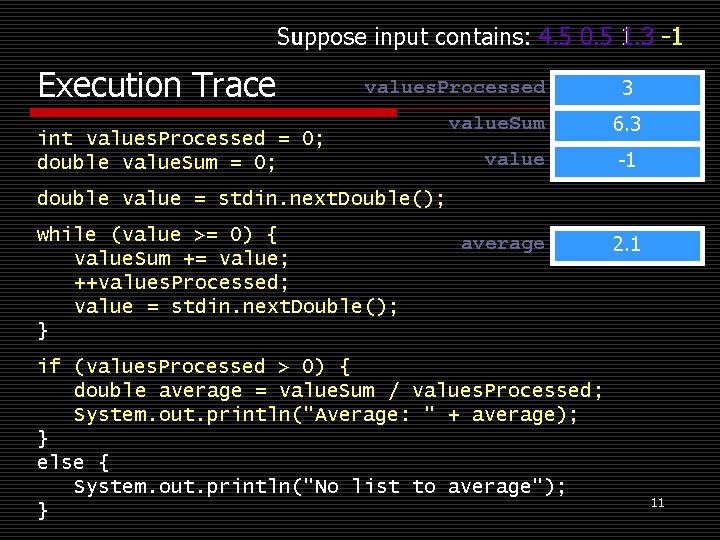
![Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin = Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin =](https://slidetodoc.com/presentation_image/a4cd213218a235e952dde5b7ca39c5ea/image-12.jpg)
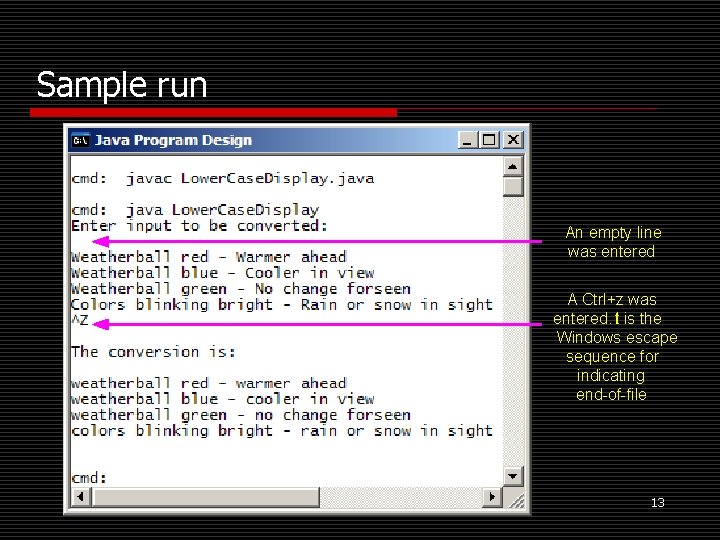
![Program trace public static void main(String[] args) { Scanner stdin = new Scanner (System. Program trace public static void main(String[] args) { Scanner stdin = new Scanner (System.](https://slidetodoc.com/presentation_image/a4cd213218a235e952dde5b7ca39c5ea/image-14.jpg)
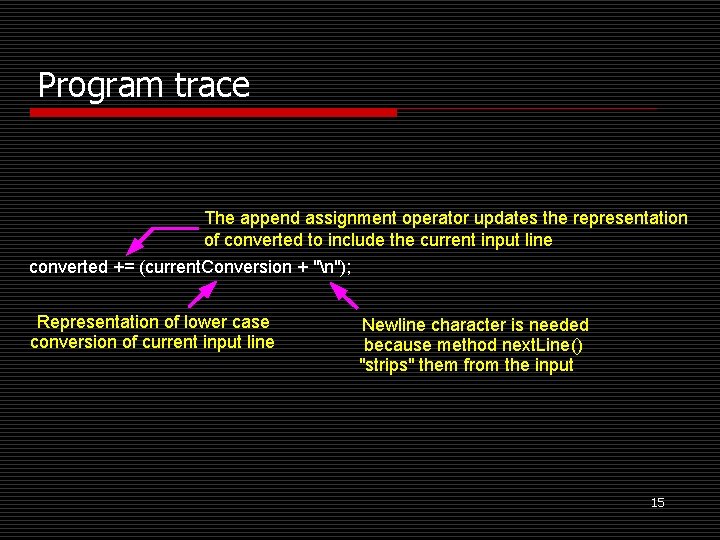
![Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin = Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin =](https://slidetodoc.com/presentation_image/a4cd213218a235e952dde5b7ca39c5ea/image-16.jpg)
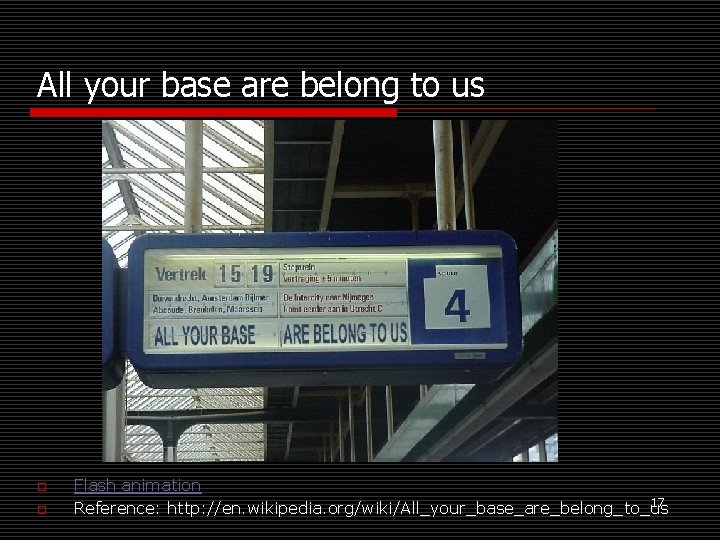
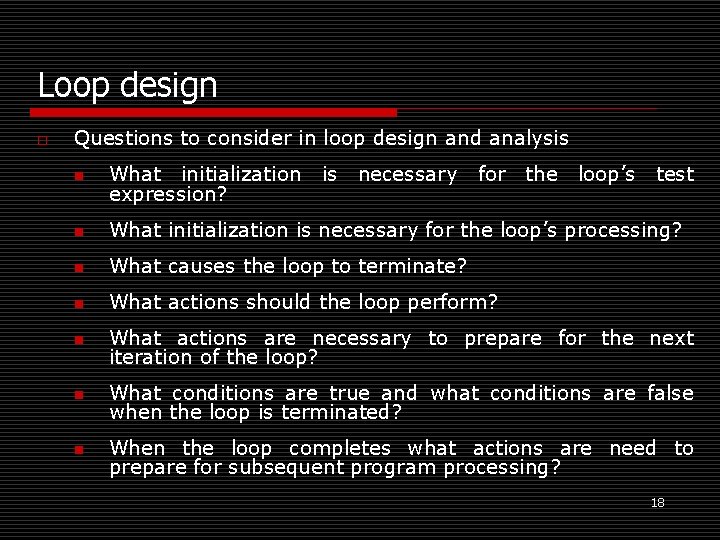
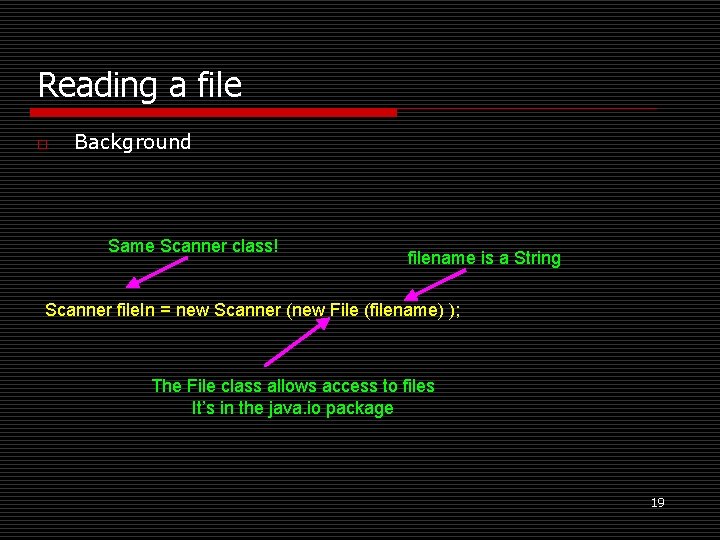
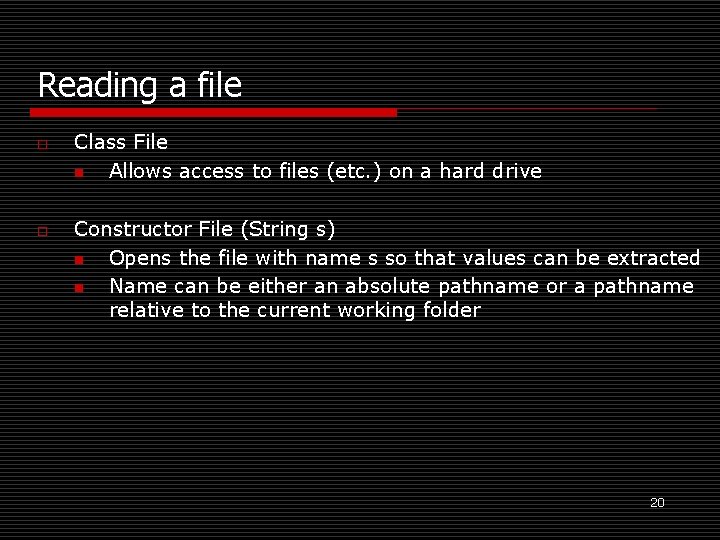
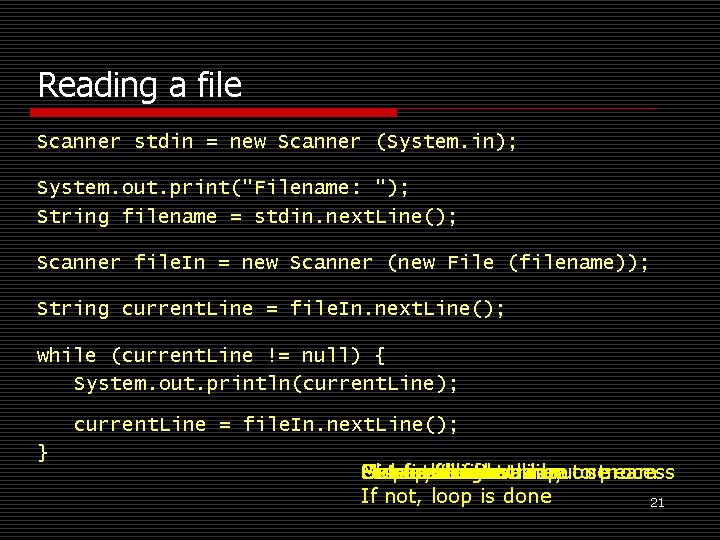
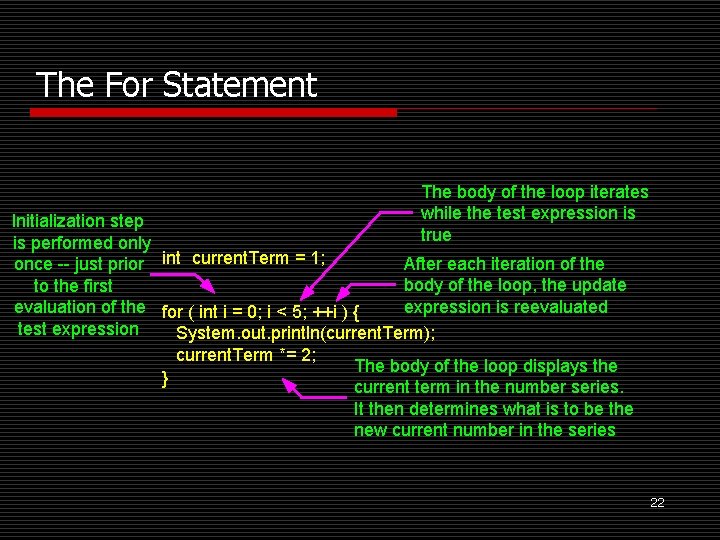
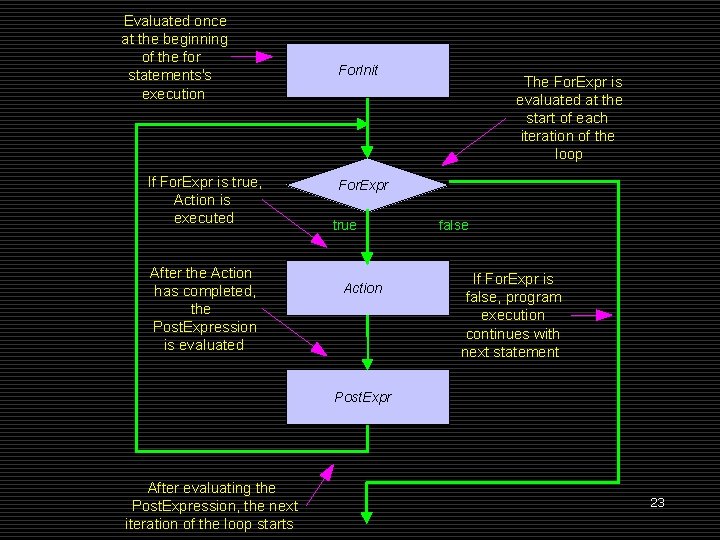
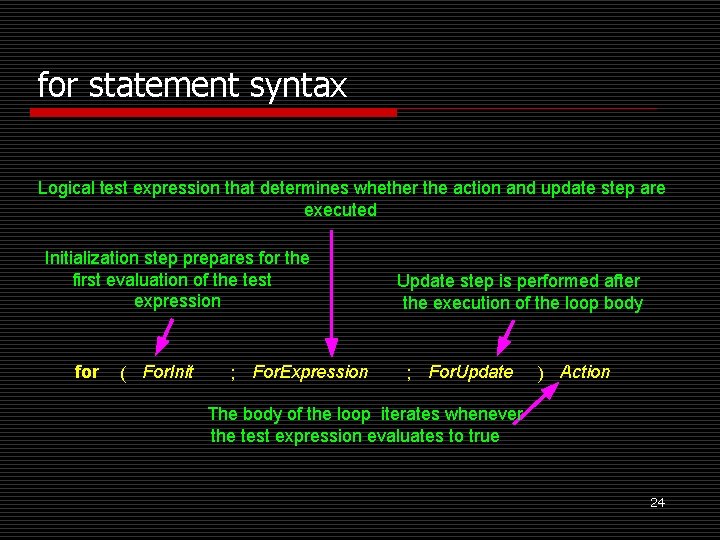
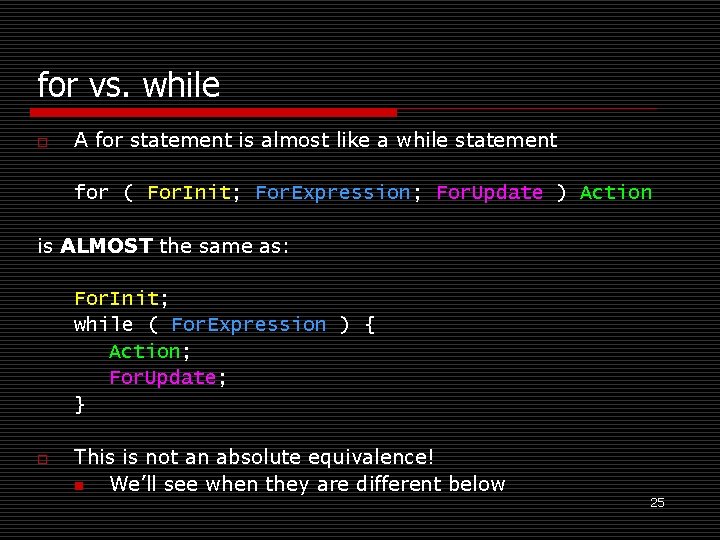
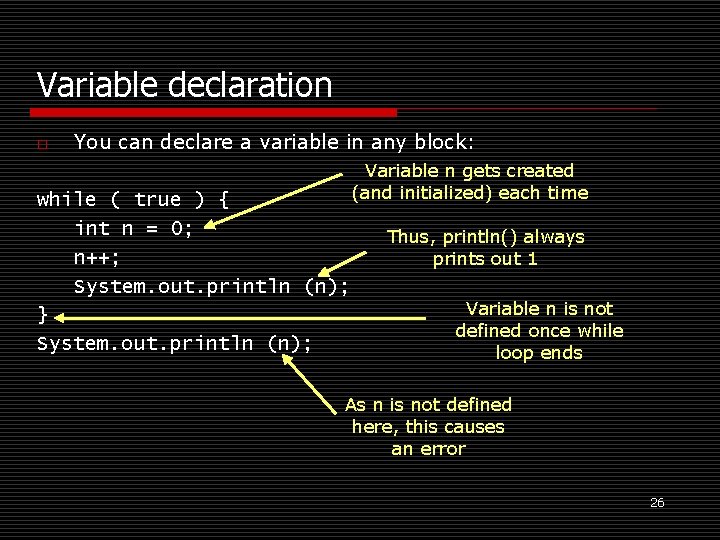
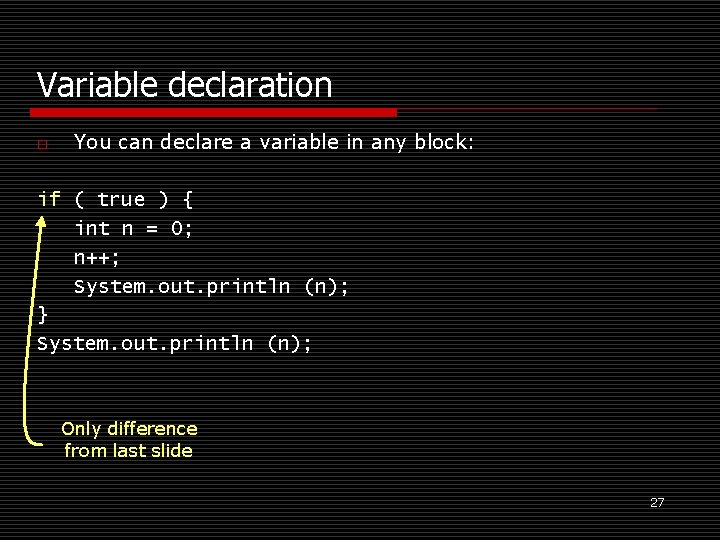
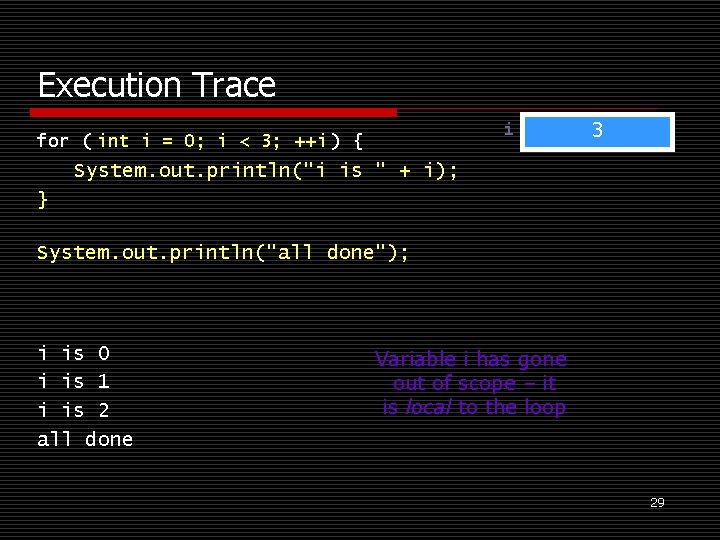
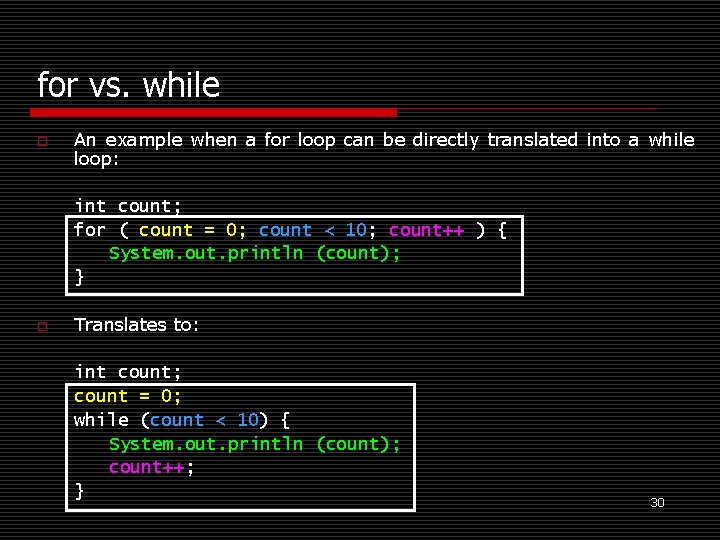
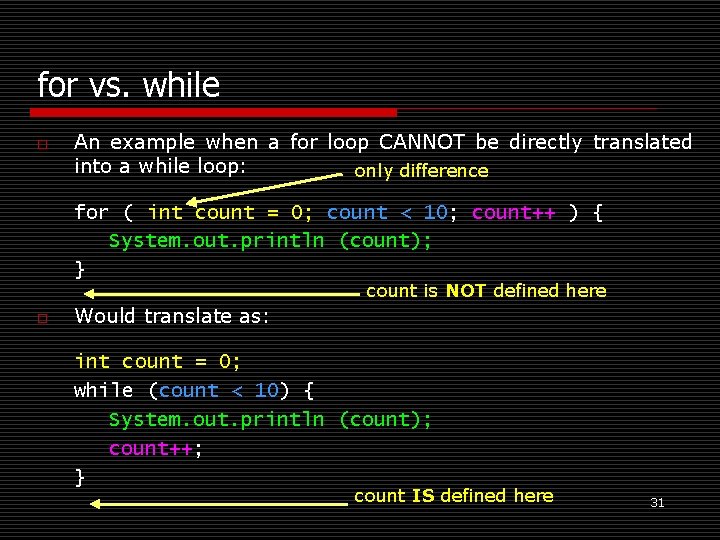
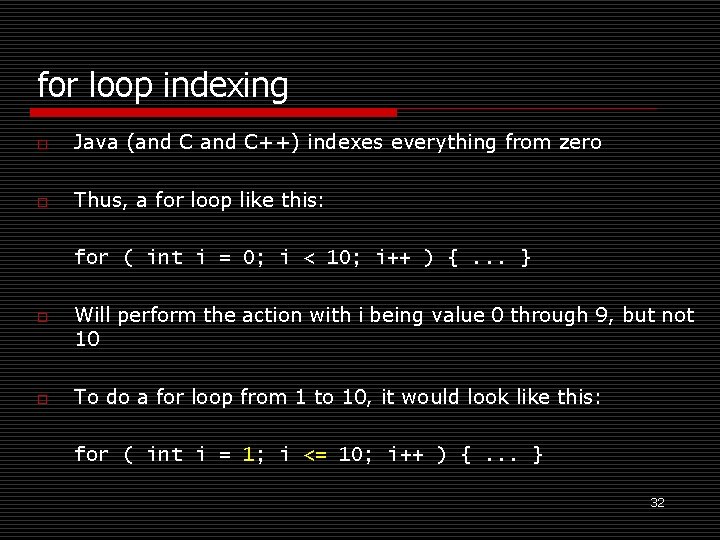
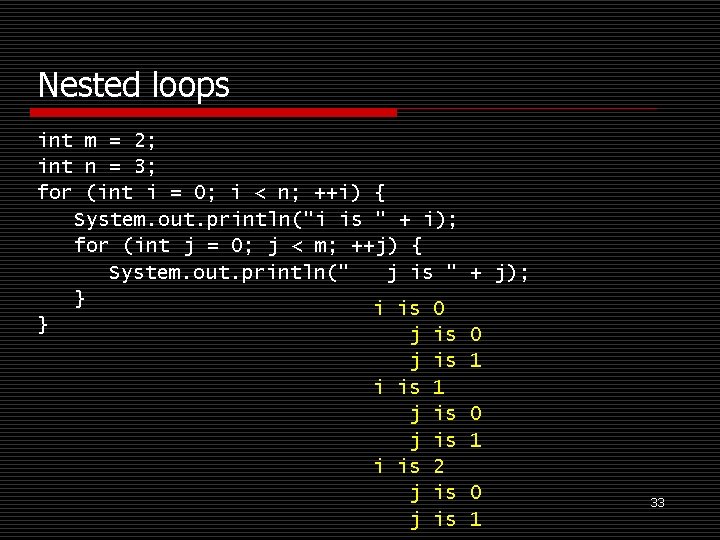
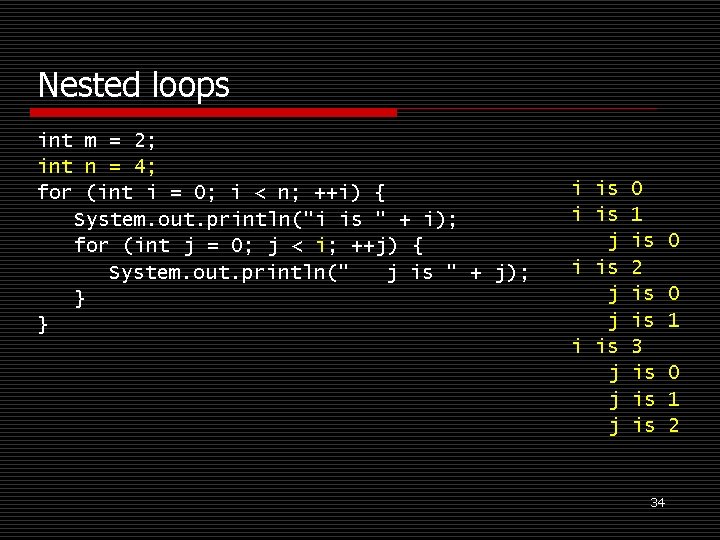
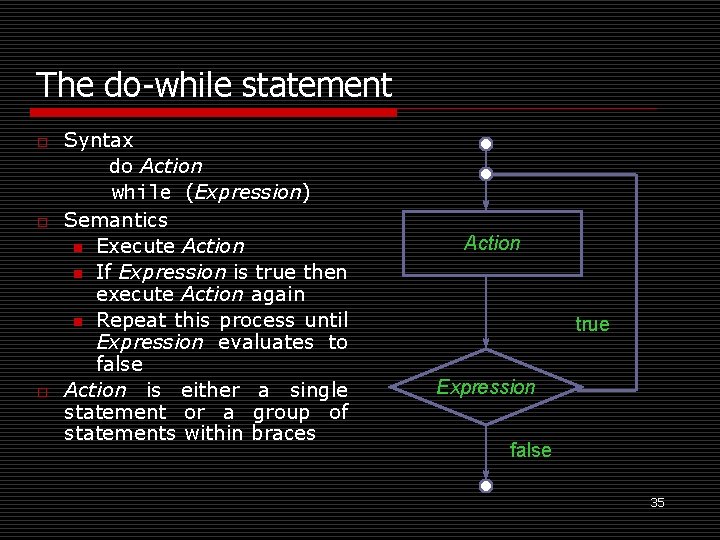
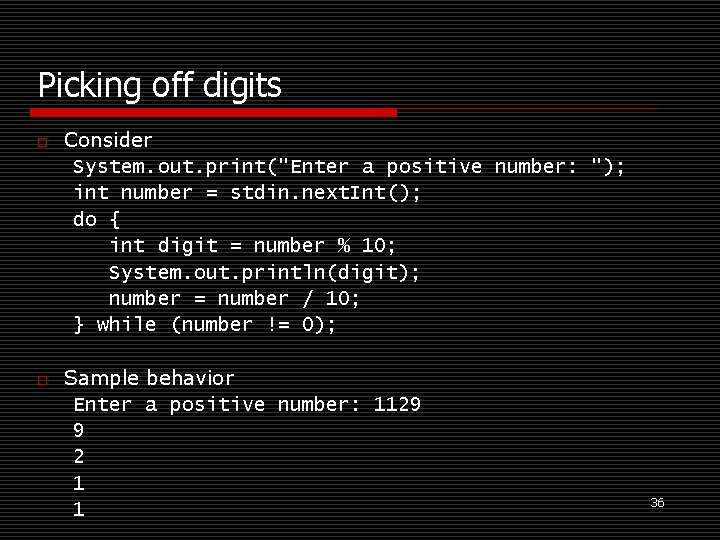
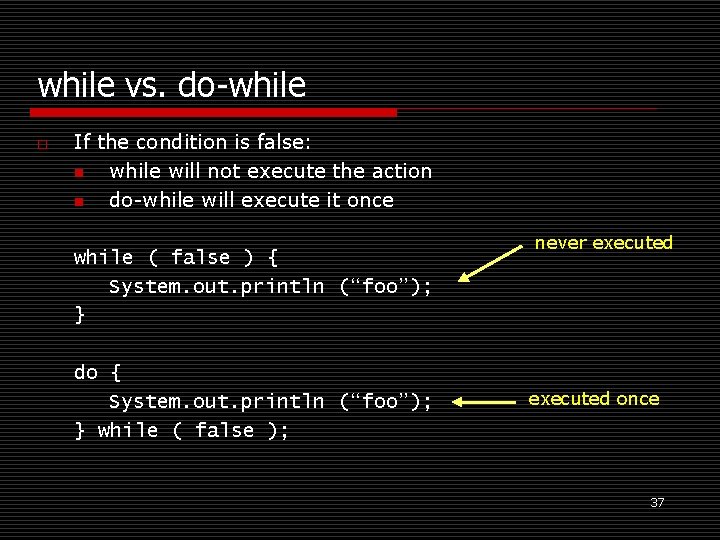
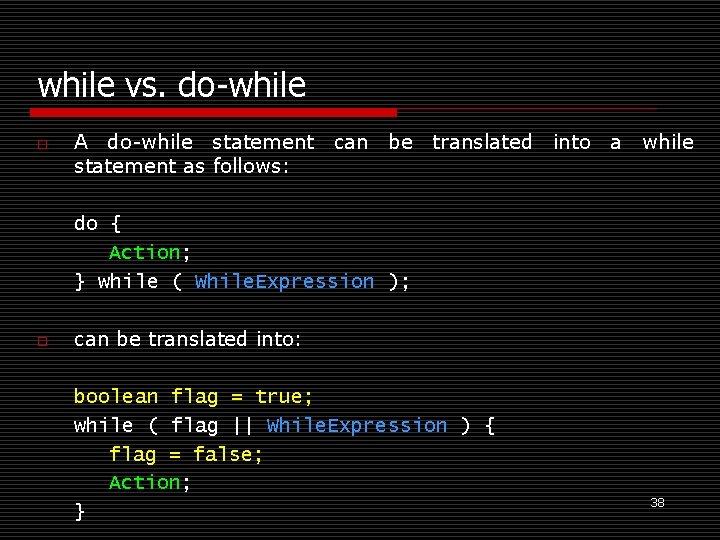
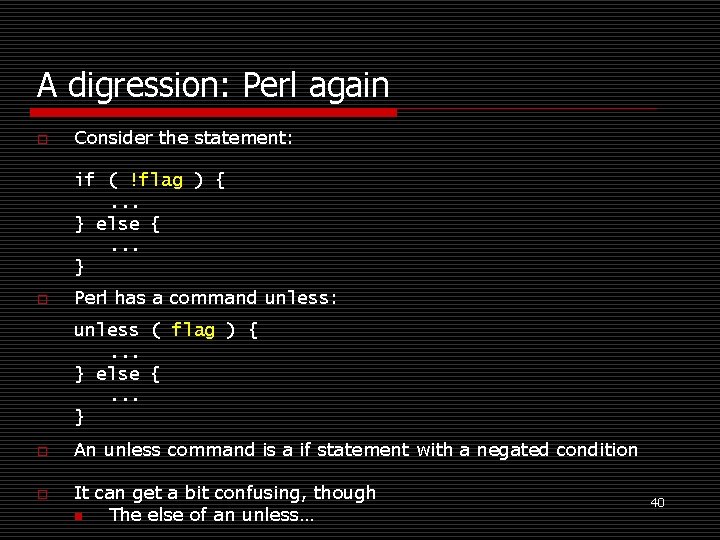
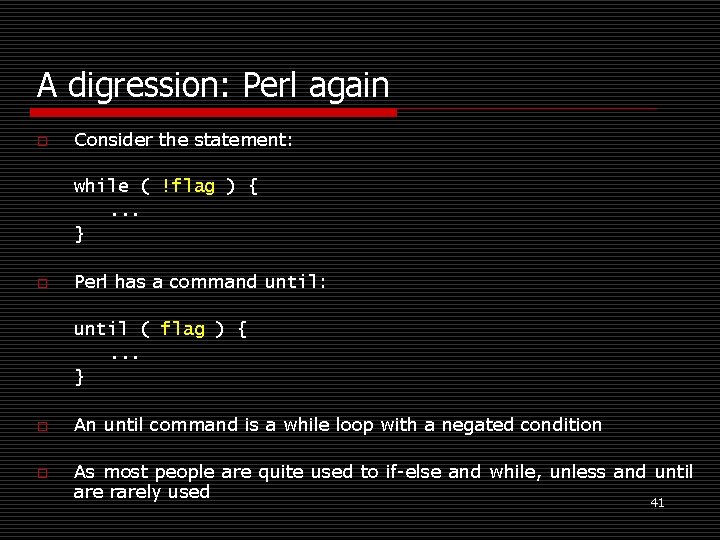
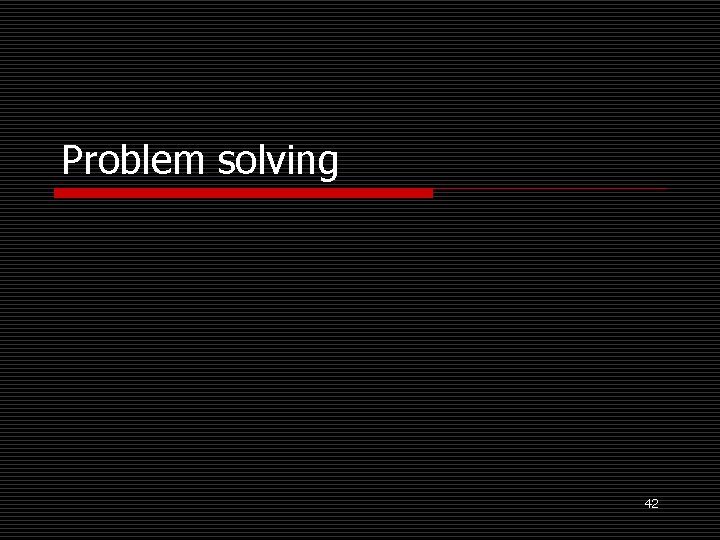
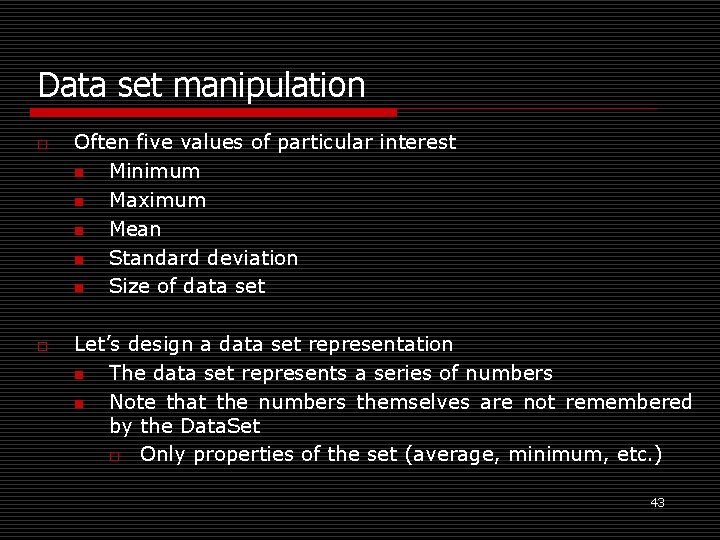
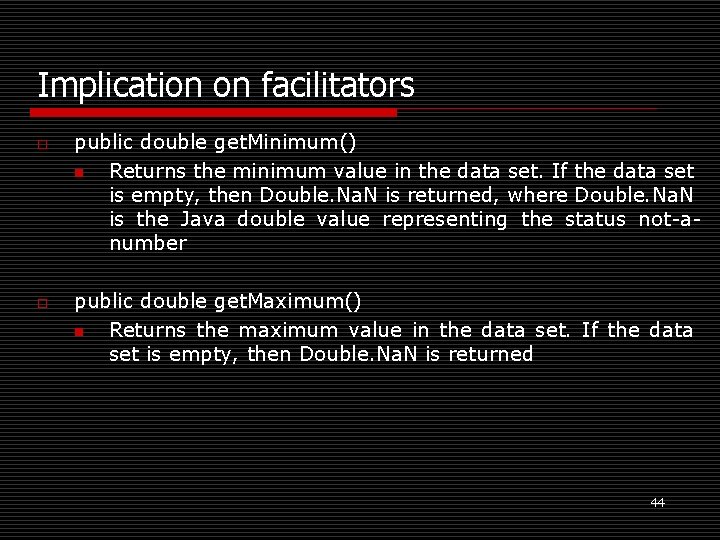
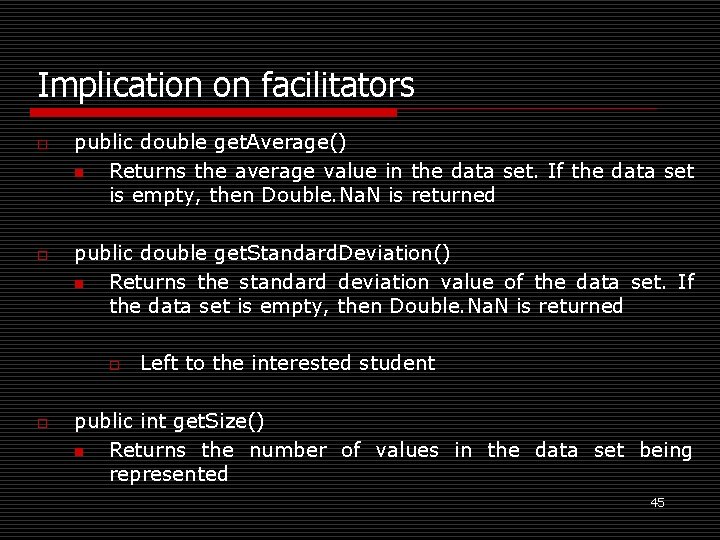
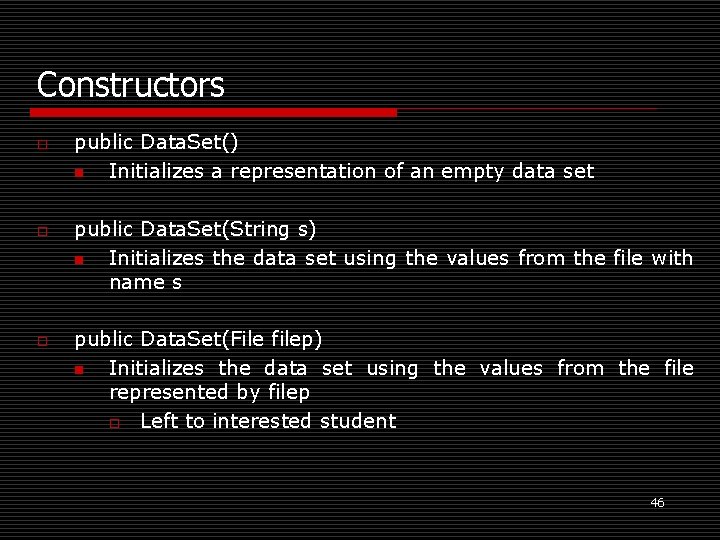
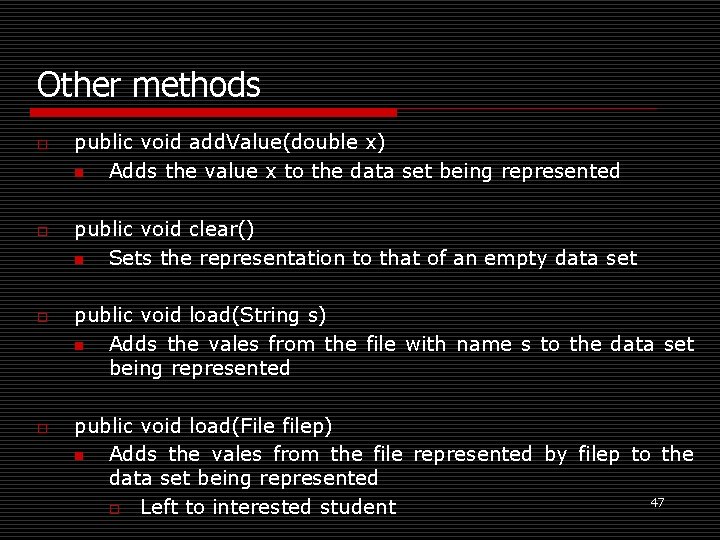
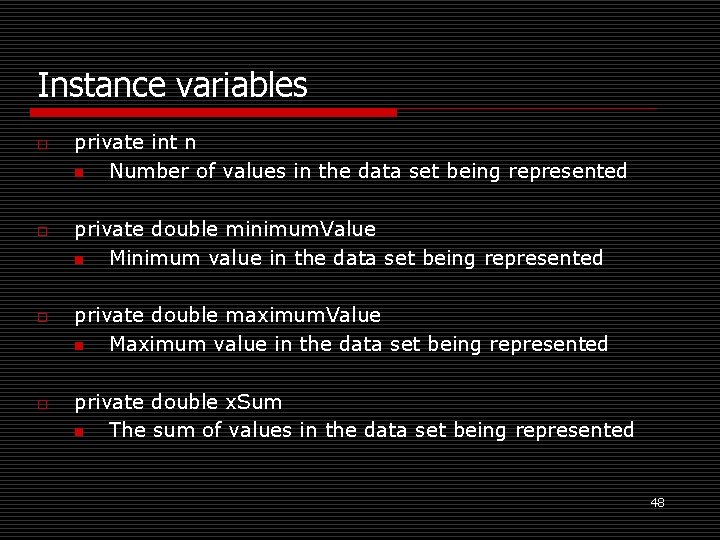
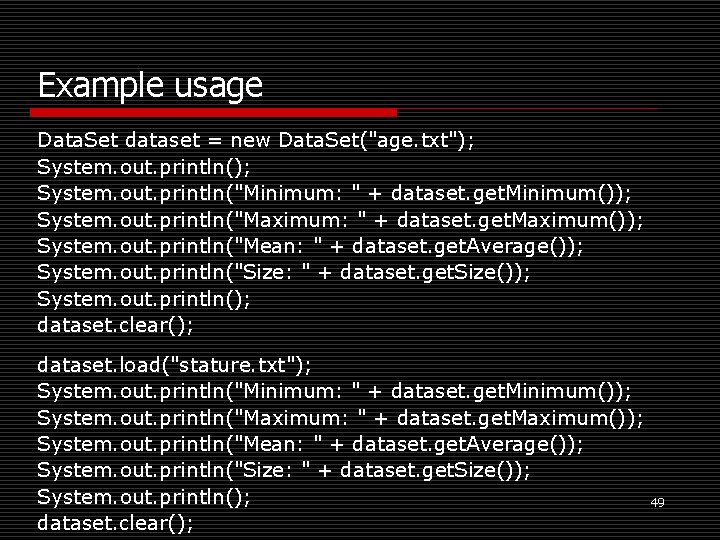
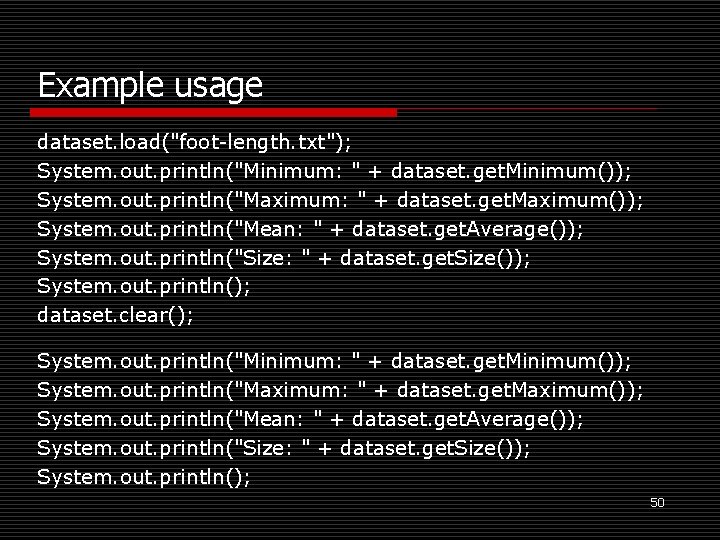
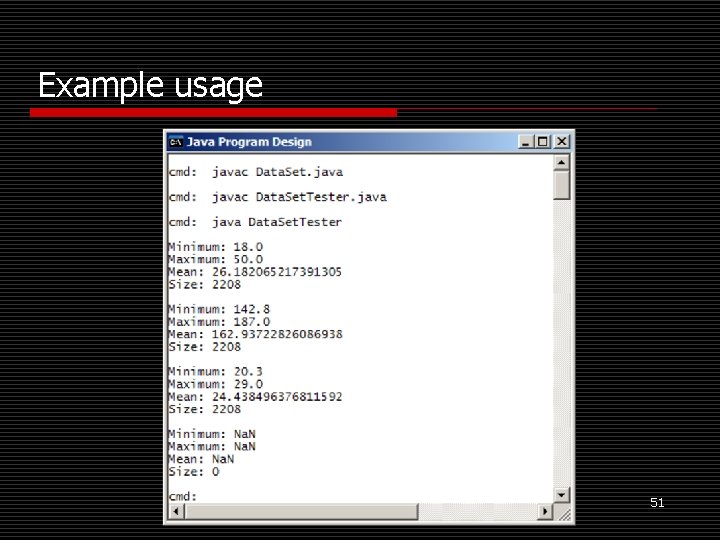
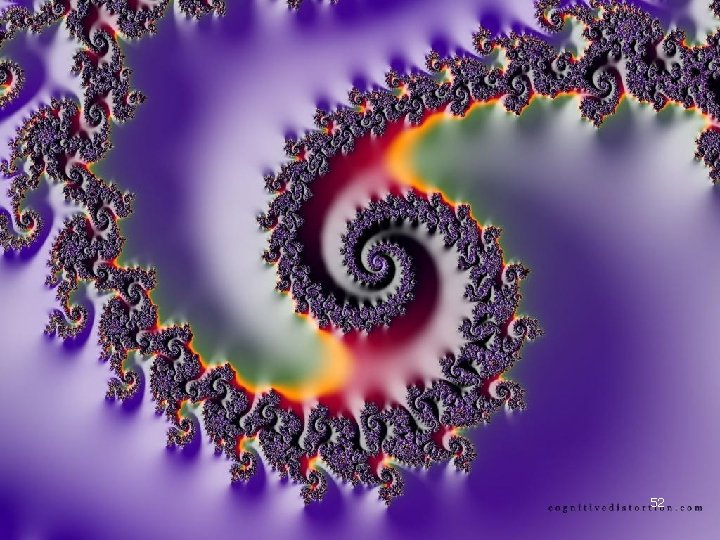
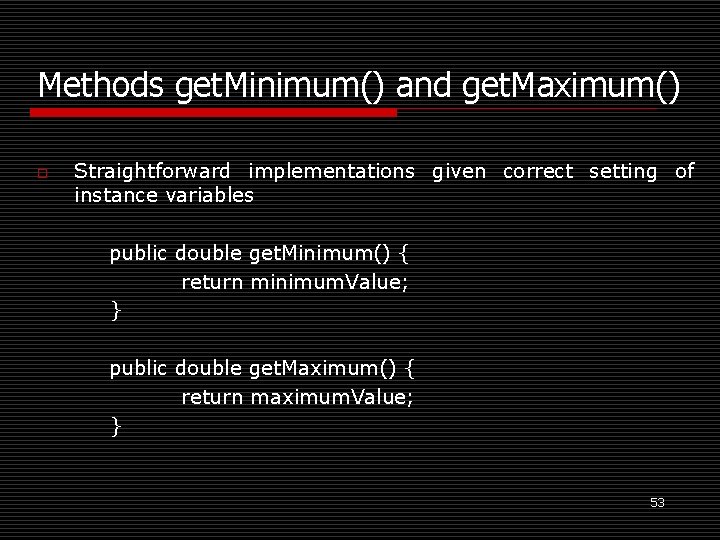
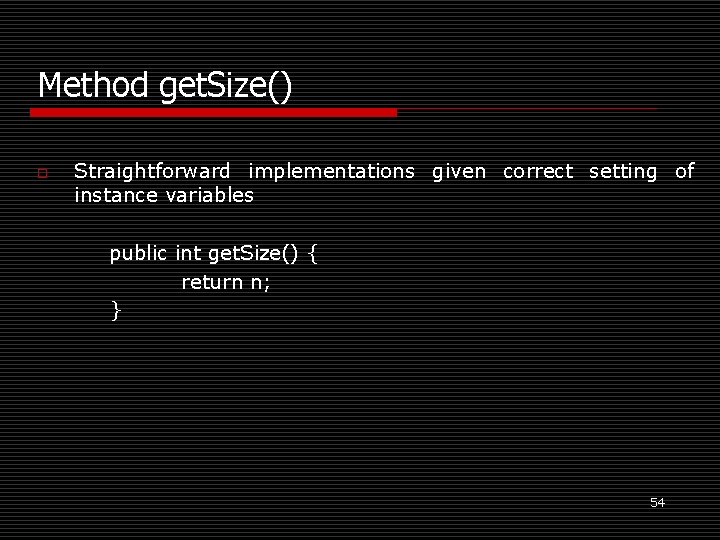
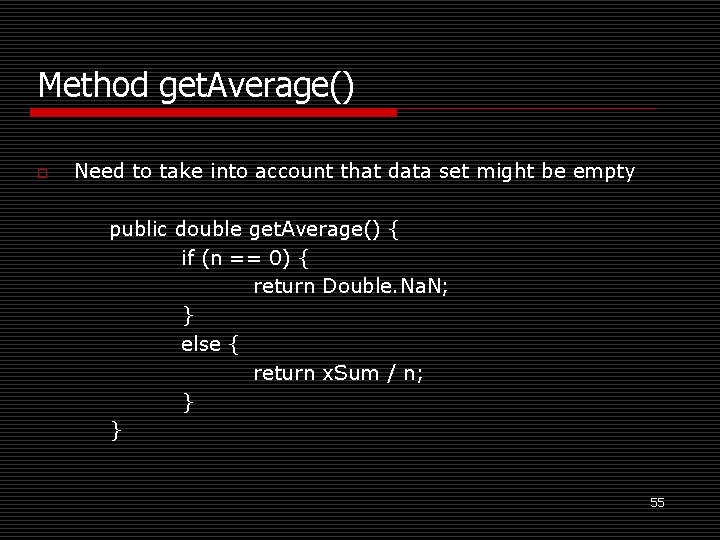
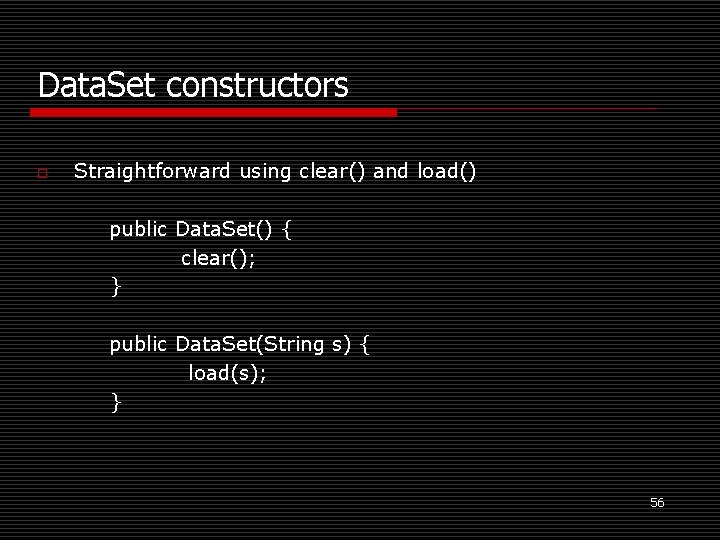
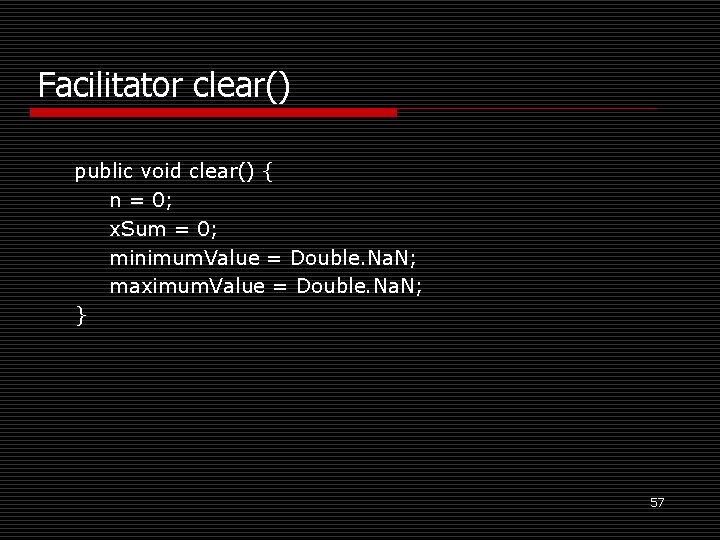
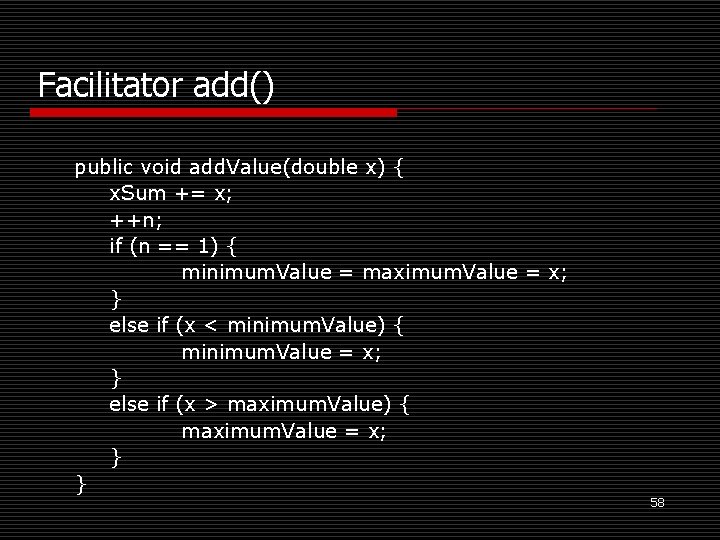
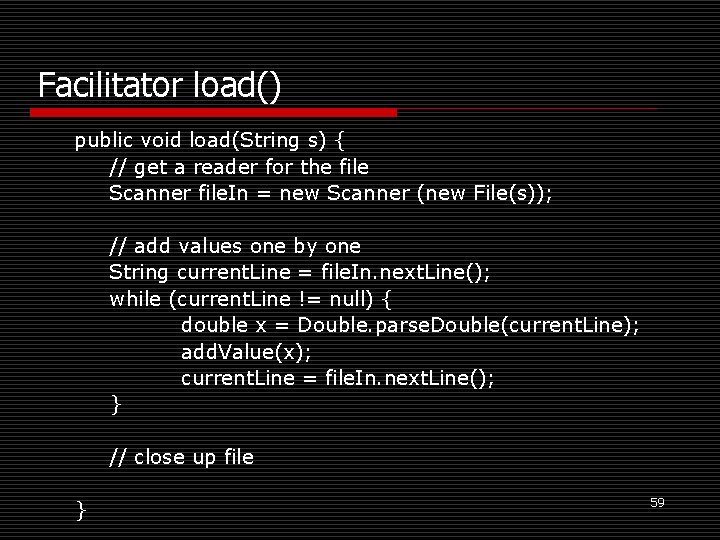
- Slides: 57
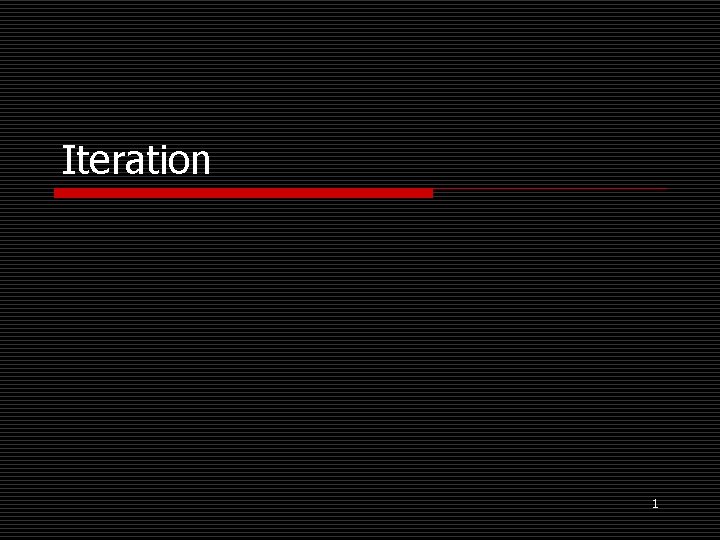
Iteration 1
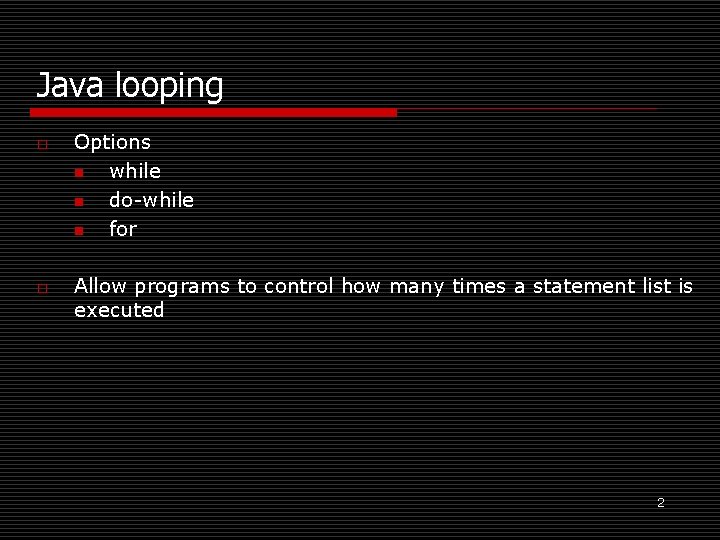
Java looping o o Options n while n do-while n for Allow programs to control how many times a statement list is executed 2
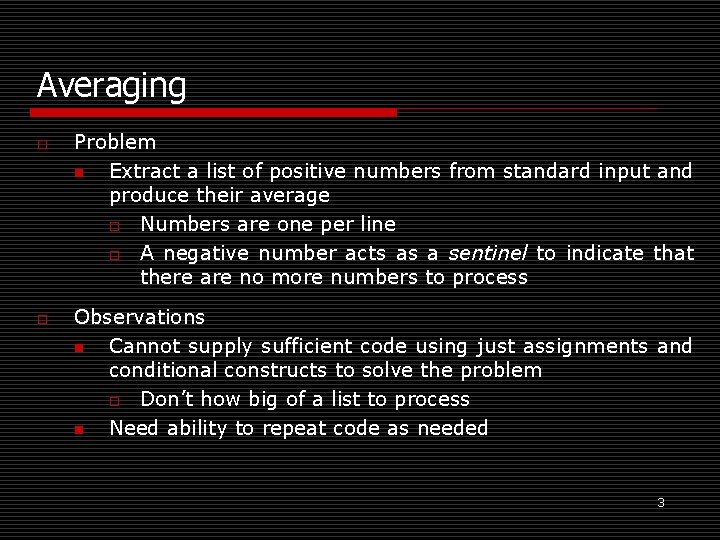
Averaging o o Problem n Extract a list of positive numbers from standard input and produce their average o Numbers are one per line o A negative number acts as a sentinel to indicate that there are no more numbers to process Observations n Cannot supply sufficient code using just assignments and conditional constructs to solve the problem o Don’t how big of a list to process n Need ability to repeat code as needed 3
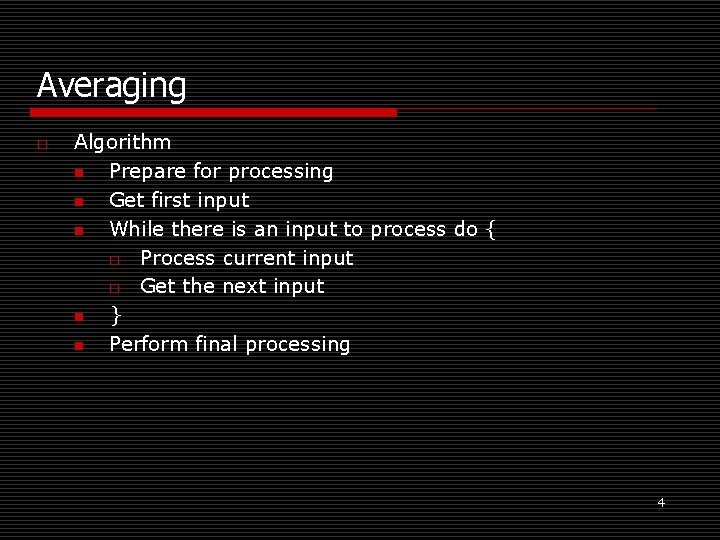
Averaging o Algorithm n Prepare for processing n Get first input n While there is an input to process do { o Process current input o Get the next input n } n Perform final processing 4
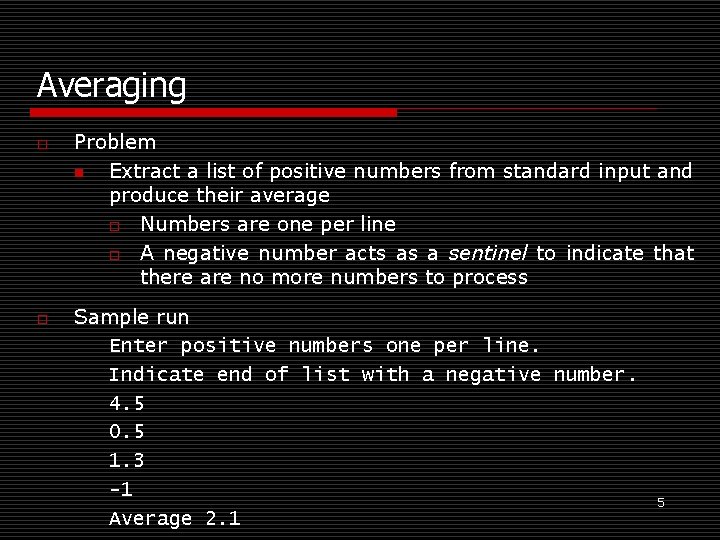
Averaging o o Problem n Extract a list of positive numbers from standard input and produce their average o Numbers are one per line o A negative number acts as a sentinel to indicate that there are no more numbers to process Sample run Enter positive numbers one per line. Indicate end of list with a negative number. 4. 5 0. 5 1. 3 -1 Average 2. 1 5
![public class Number Average main application entry point public static void mainString public class Number. Average { // main(): application entry point public static void main(String[]](https://slidetodoc.com/presentation_image/a4cd213218a235e952dde5b7ca39c5ea/image-6.jpg)
public class Number. Average { // main(): application entry point public static void main(String[] args) { // set up the input // prompt user for values // get first value // process values one-by-one while (value >= 0) { // add value to running total // processed another value // prepare next iteration - get next value } // display result if (values. Processed > 0) // compute and display average else // indicate no average to display } } 6
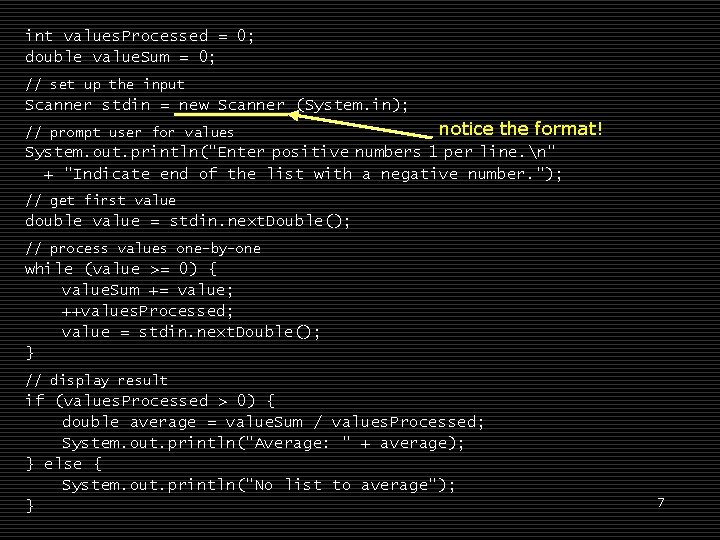
int values. Processed = 0; double value. Sum = 0; // set up the input Scanner stdin = new Scanner (System. in); // prompt user for values notice the format! System. out. println("Enter positive numbers 1 per line. n" + "Indicate end of the list with a negative number. "); // get first value double value = stdin. next. Double(); // process values one-by-one while (value >= 0) { value. Sum += value; ++values. Processed; value = stdin. next. Double(); } // display result if (values. Processed > 0) { double average = value. Sum / values. Processed; System. out. println("Average: " + average); } else { System. out. println("No list to average"); } 7
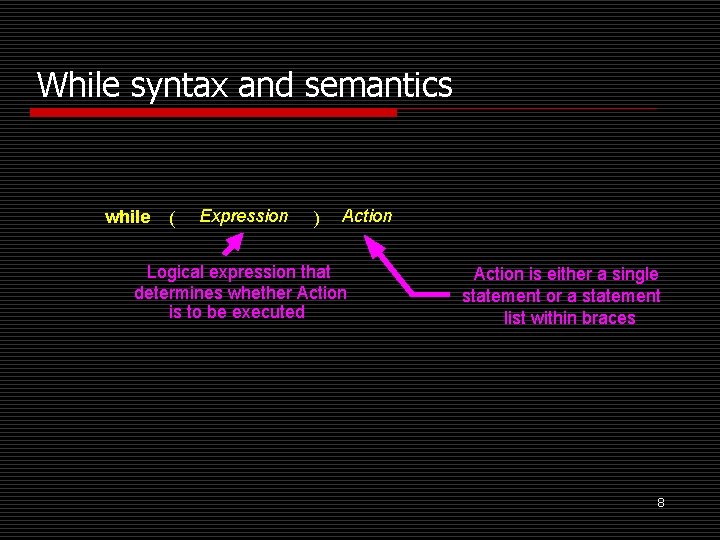
While syntax and semantics while ( Expression ) Action Logical expression that determines whether Action is to be executed Action is either a single statement or a statement list within braces 8
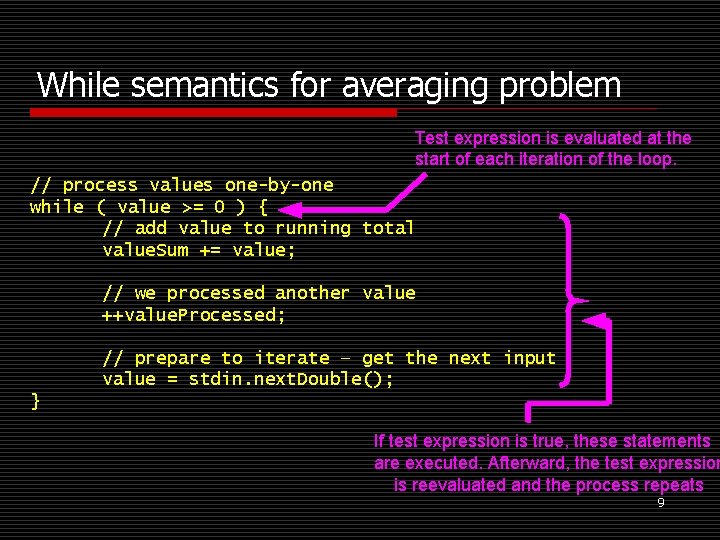
While semantics for averaging problem Test expression is evaluated at the start of each iteration of the loop. // process values one-by-one while ( value >= 0 ) { // add value to running total value. Sum += value; // we processed another value ++value. Processed; // prepare to iterate – get the next input value = stdin. next. Double(); } If test expression is true, these statements are executed. Afterward, the test expression is reevaluated and the process repeats 9
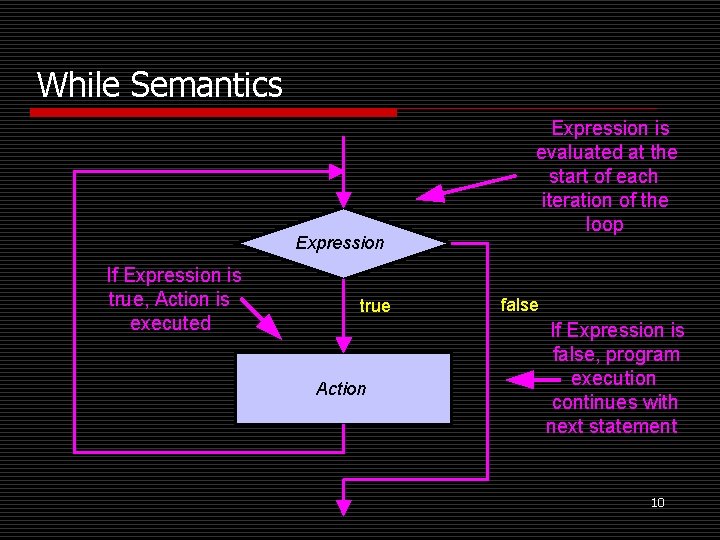
While Semantics Expression If Expression is true, Action is executed true Action Expression is evaluated at the start of each iteration of the loop false If Expression is false, program execution continues with next statement 10
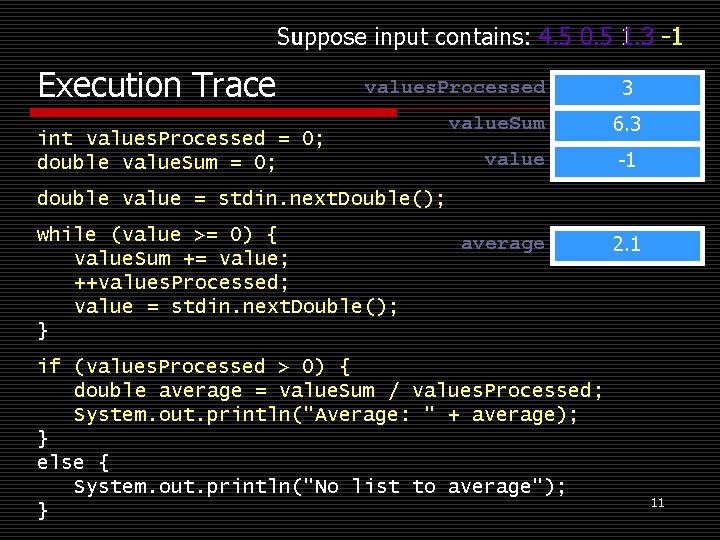
Suppose input contains: 4. 5 0. 5 1. 3 -1 Execution Trace values. Processed int values. Processed = 0; double value. Sum = 0; 0 1 2 3 value. Sum 4. 5 5. 0 6. 3 0 value 4. 5 0. 5 1. 3 -1 average 2. 1 double value = stdin. next. Double(); while (value >= 0) { value. Sum += value; ++values. Processed; value = stdin. next. Double(); } if (values. Processed > 0) { double average = value. Sum / values. Processed; System. out. println("Average: " + average); } else { System. out. println("No list to average"); } 11
![Converting text to strictly lowercase public static void mainString args Scanner stdin Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin =](https://slidetodoc.com/presentation_image/a4cd213218a235e952dde5b7ca39c5ea/image-12.jpg)
Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin = new Scanner (System. in); System. out. println("Enter input to be converted: "); String converted = ""; String current. Line = stdin. next. Line(); while (current. Line != null) { String current. Conversion = current. Line. to. Lower. Case(); converted += (current. Conversion + "n"); current. Line = stdin. next. Line(); } System. out. println("n. Conversion is: n" + converted); } 12
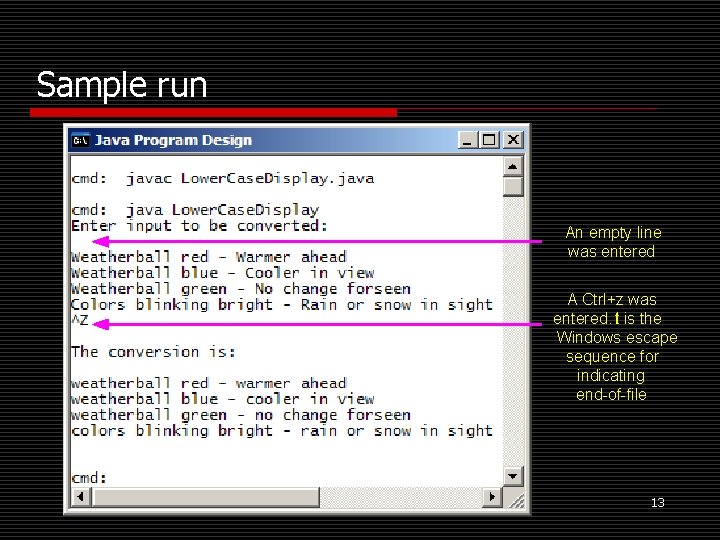
Sample run An empty line was entered A Ctrl+z was entered. t. I is the Windows escape sequence for indicating end-of-file 13
![Program trace public static void mainString args Scanner stdin new Scanner System Program trace public static void main(String[] args) { Scanner stdin = new Scanner (System.](https://slidetodoc.com/presentation_image/a4cd213218a235e952dde5b7ca39c5ea/image-14.jpg)
Program trace public static void main(String[] args) { Scanner stdin = new Scanner (System. in); System. out. println("Enter input to be converted: "); String converted = ""; String current. Line = stdin. next. Line(); while (current. Line != null) { String current. Conversion = current. Line. to. Lower. Case(); converted += (current. Conversion + "n"); current. Line = stdin. next. Line(); } System. out. println("n. Conversion is: n" + converted); } 14
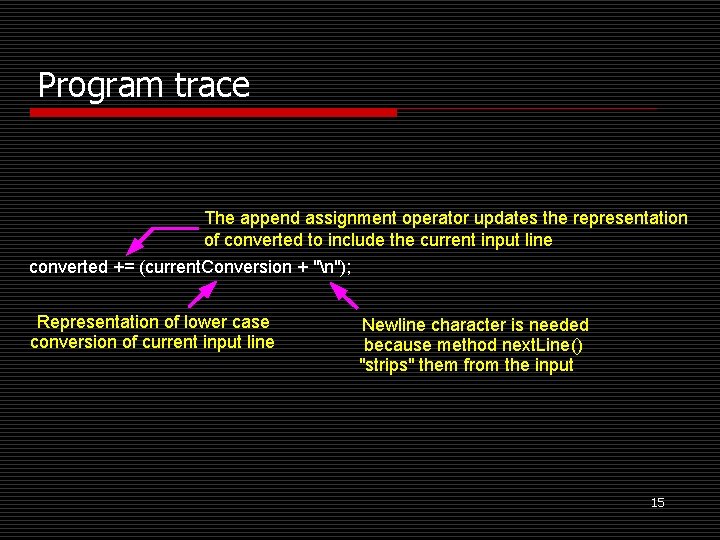
Program trace The append assignment operator updates the representation of converted to include the current input line converted += (current. Conversion + "n"); Representation of lower case conversion of current input line Newline character is needed because method next. Line() "strips" them from the input 15
![Converting text to strictly lowercase public static void mainString args Scanner stdin Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin =](https://slidetodoc.com/presentation_image/a4cd213218a235e952dde5b7ca39c5ea/image-16.jpg)
Converting text to strictly lowercase public static void main(String[] args) { Scanner stdin = new Scanner (System. in); System. out. println("Enter input to be converted: "); String converted = ""; String current. Line = stdin. next. Line(); while (current. Line != null) { String current. Conversion = current. Line. to. Lower. Case(); converted += (current. Conversion + "n"); current. Line = stdin. next. Line(); } System. out. println("n. Conversion is: n" + converted); } 16
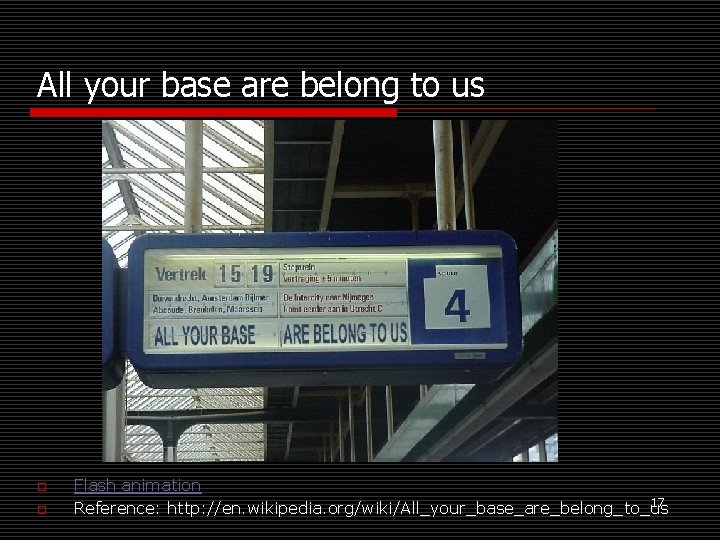
All your base are belong to us o o Flash animation 17 Reference: http: //en. wikipedia. org/wiki/All_your_base_are_belong_to_us
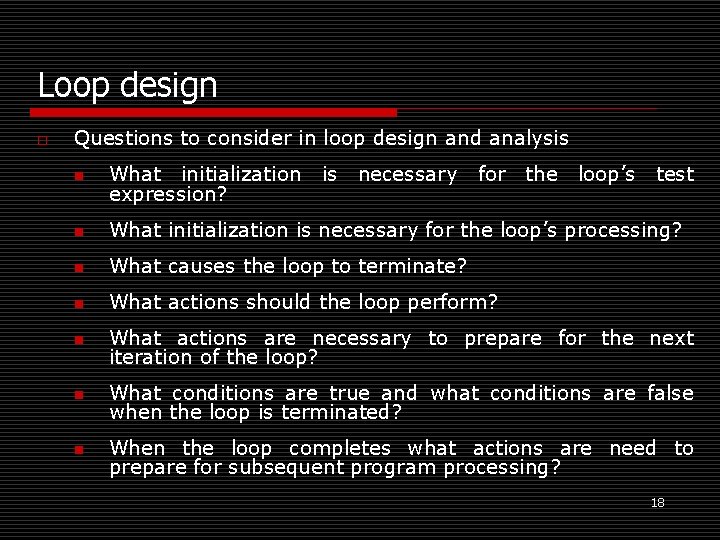
Loop design o Questions to consider in loop design and analysis n What initialization expression? is necessary for the loop’s test n What initialization is necessary for the loop’s processing? n What causes the loop to terminate? n What actions should the loop perform? n What actions are necessary to prepare for the next iteration of the loop? n What conditions are true and what conditions are false when the loop is terminated? n When the loop completes what actions are need to prepare for subsequent program processing? 18
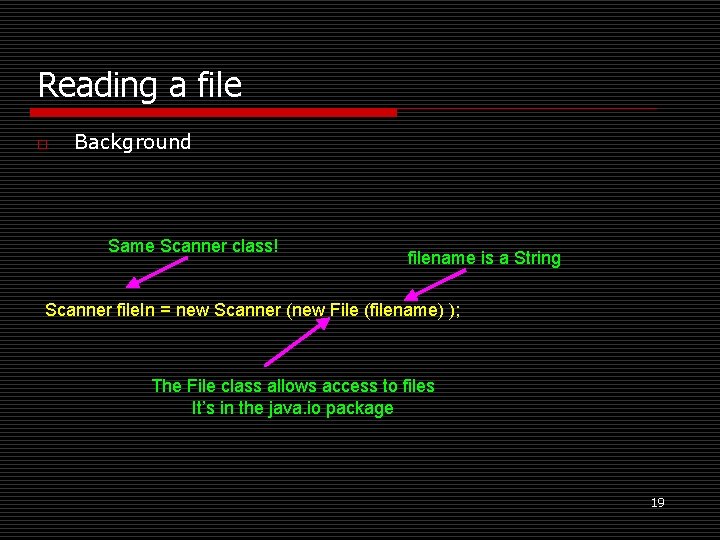
Reading a file o Background Same Scanner class! filename is a String Scanner file. In = new Scanner (new File (filename) ); The File class allows access to files It’s in the java. io package 19
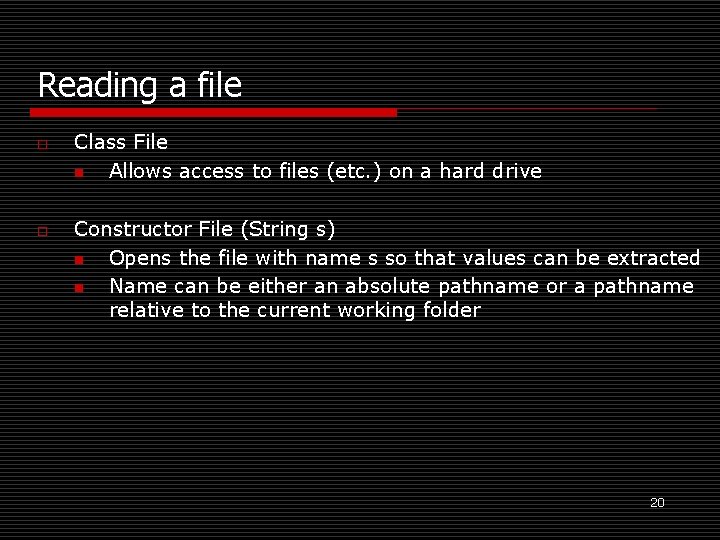
Reading a file o o Class File n Allows access to files (etc. ) on a hard drive Constructor File (String s) n Opens the file with name s so that values can be extracted n Name can be either an absolute pathname or a pathname relative to the current working folder 20
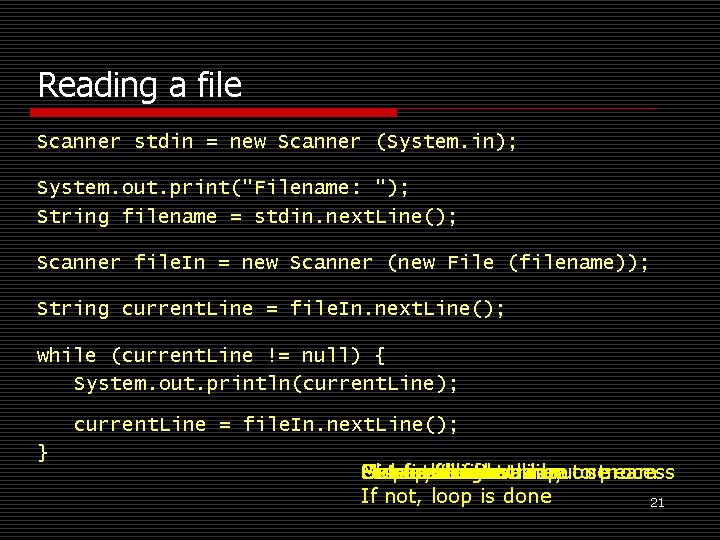
Reading a file Scanner stdin = new Scanner (System. in); System. out. print("Filename: "); String filename = stdin. next. Line(); Scanner file. In = new Scanner (new File (filename)); String current. Line = file. In. next. Line(); while (current. Line != null) { System. out. println(current. Line); current. Line = file. In. next. Line(); } Close Determine Set Process Display Get Make up first next sure the standard file current lines line file stream got fileone stream name aline input line by one tostream process If not, loop is done 21
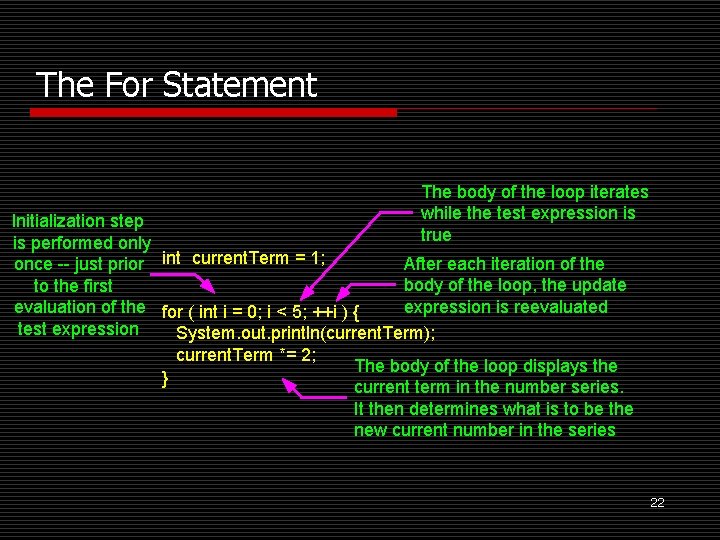
The For Statement The body of the loop iterates while the test expression is true Initialization step is performed only After each iteration of the once -- just prior int current. Term = 1; body of the loop, the update to the first expression is reevaluated evaluation of the for ( int i = 0; i < 5; ++i ) { test expression System. out. println(current. Term); current. Term *= 2; The body of the loop displays the } current term in the number series. It then determines what is to be the new current number in the series 22
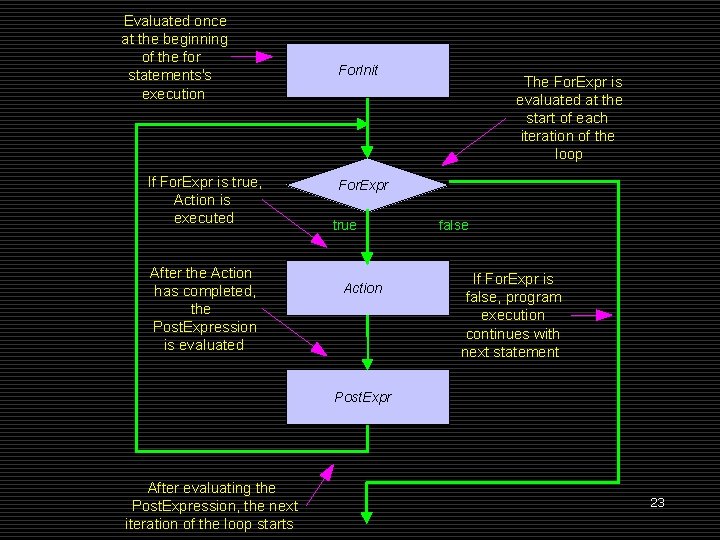
Evaluated once at the beginning of the for statements's execution If For. Expr is true, Action is executed After the Action has completed, the Post. Expression is evaluated For. Init The For. Expr is evaluated at the start of each iteration of the loop For. Expr true Action false If For. Expr is false, program execution continues with next statement Post. Expr After evaluating the Post. Expression, the next iteration of the loop starts 23
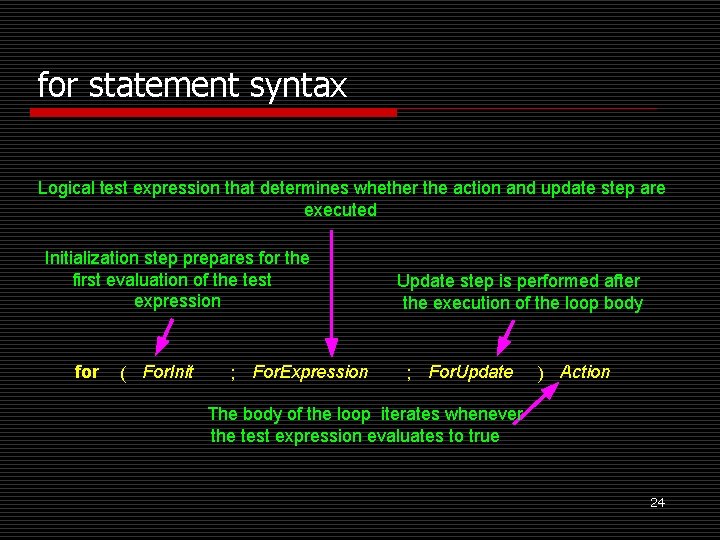
for statement syntax Logical test expression that determines whether the action and update step are executed Initialization step prepares for the first evaluation of the test expression for ( For. Init ; For. Expression Update step is performed after the execution of the loop body ; For. Update ) Action The body of the loop iterates whenever the test expression evaluates to true 24
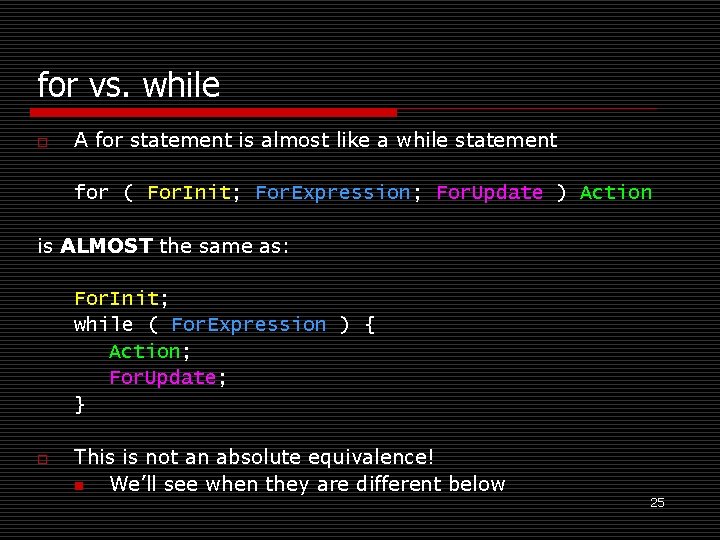
for vs. while o A for statement is almost like a while statement for ( For. Init; For. Expression; For. Update ) Action is ALMOST the same as: For. Init; while ( For. Expression ) { Action; For. Update; } o This is not an absolute equivalence! n We’ll see when they are different below 25
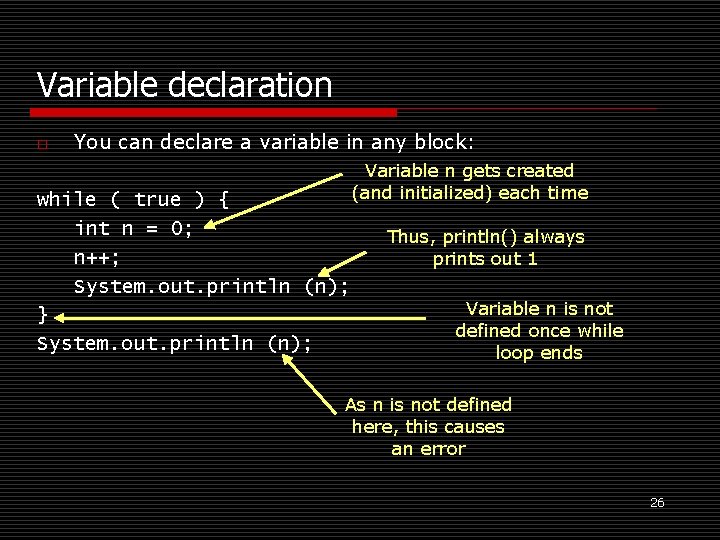
Variable declaration o You can declare a variable in any block: while ( true ) { int n = 0; n++; System. out. println (n); } System. out. println (n); Variable n gets created (and initialized) each time Thus, println() always prints out 1 Variable n is not defined once while loop ends As n is not defined here, this causes an error 26
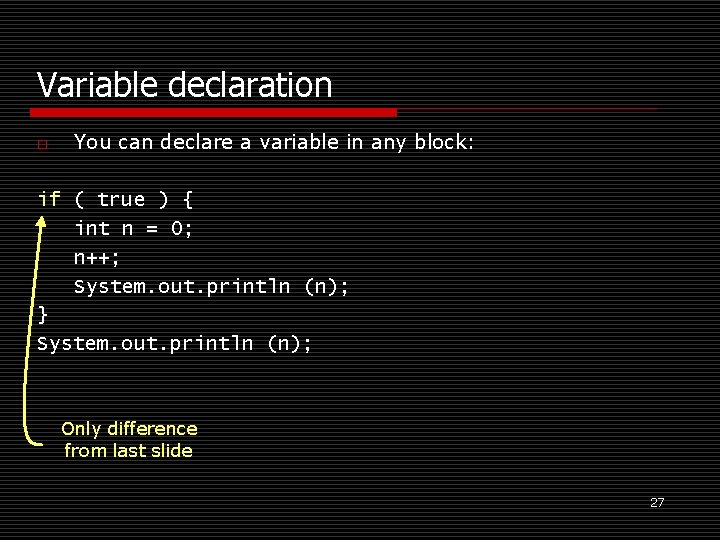
Variable declaration o You can declare a variable in any block: if ( true ) { int n = 0; n++; System. out. println (n); } System. out. println (n); Only difference from last slide 27
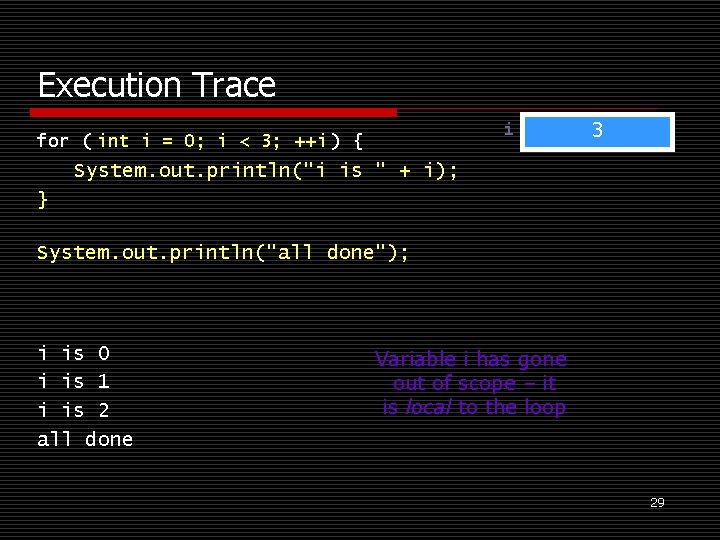
Execution Trace i for ( int i = 0; i < 3; ++i ) { 0 3 2 1 System. out. println("i is " + i); } System. out. println("all done"); i is 0 i is 1 i is 2 all done Variable i has gone out of scope – it is local to the loop 29
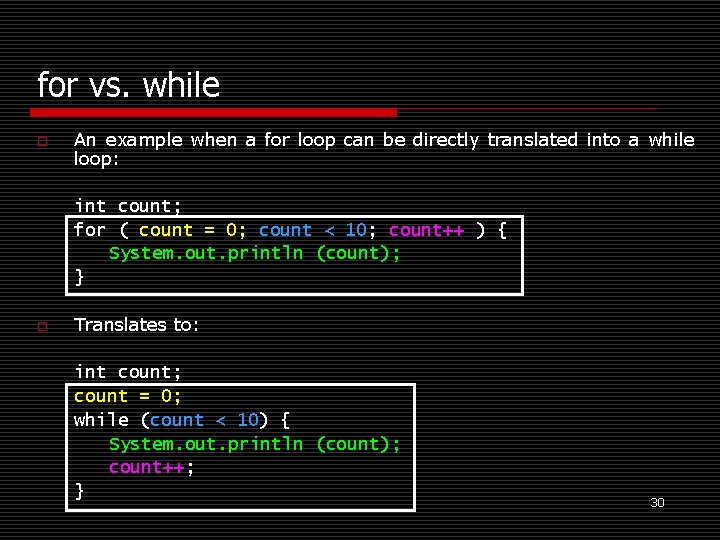
for vs. while o An example when a for loop can be directly translated into a while loop: int count; for ( count = 0; count < 10; count++ ) { System. out. println (count); } o Translates to: int count; count = 0; while (count < 10) { System. out. println (count); count++; } 30
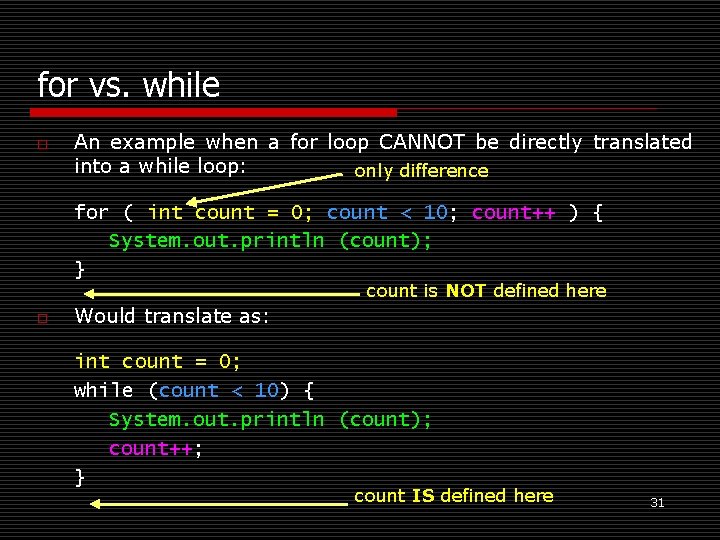
for vs. while o An example when a for loop CANNOT be directly translated into a while loop: only difference for ( int count = 0; count < 10; count++ ) { System. out. println (count); } count is NOT defined here o Would translate as: int count = 0; while (count < 10) { System. out. println (count); count++; } count IS defined here 31
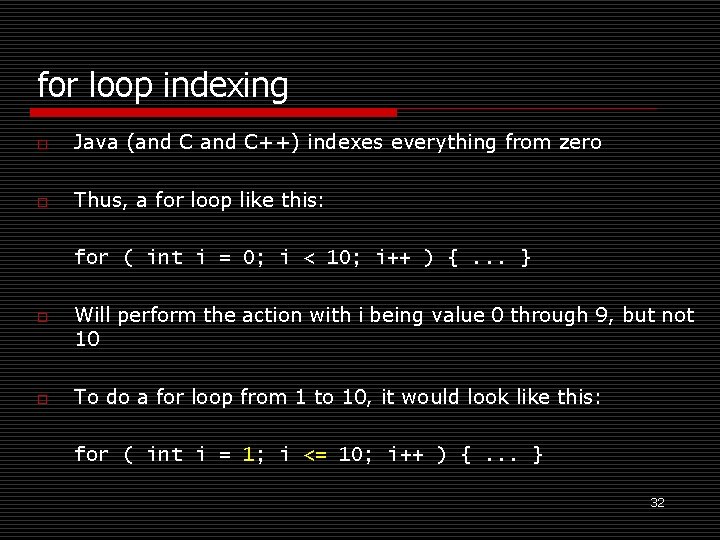
for loop indexing o Java (and C++) indexes everything from zero o Thus, a for loop like this: for ( int i = 0; i < 10; i++ ) {. . . } o o Will perform the action with i being value 0 through 9, but not 10 To do a for loop from 1 to 10, it would look like this: for ( int i = 1; i <= 10; i++ ) {. . . } 32
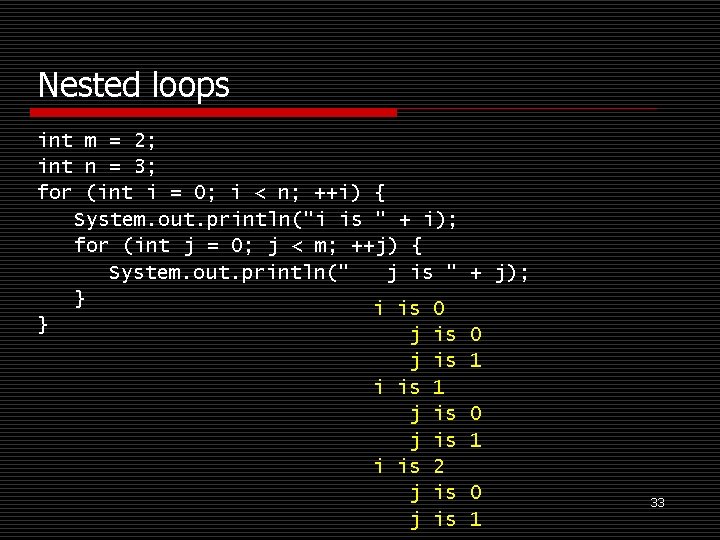
Nested loops int m = 2; int n = 3; for (int i = 0; i < n; ++i) { System. out. println("i is " + i); for (int j = 0; j < m; ++j) { System. out. println(" j is " + j); } i is 0 } j is 0 j is 1 i is 1 j is 0 j is 1 i is 2 j is 0 j is 1 33
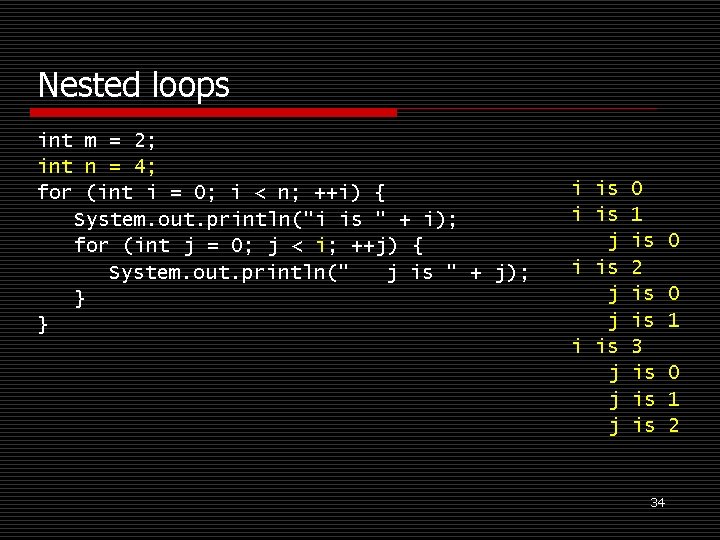
Nested loops int m = 2; int n = 4; for (int i = 0; i < n; ++i) { System. out. println("i is " + i); for (int j = 0; j < i; ++j) { System. out. println(" j is " + j); } } i is 0 i is 1 j is i is 2 j is i is 3 j is 34 0 0 1 2
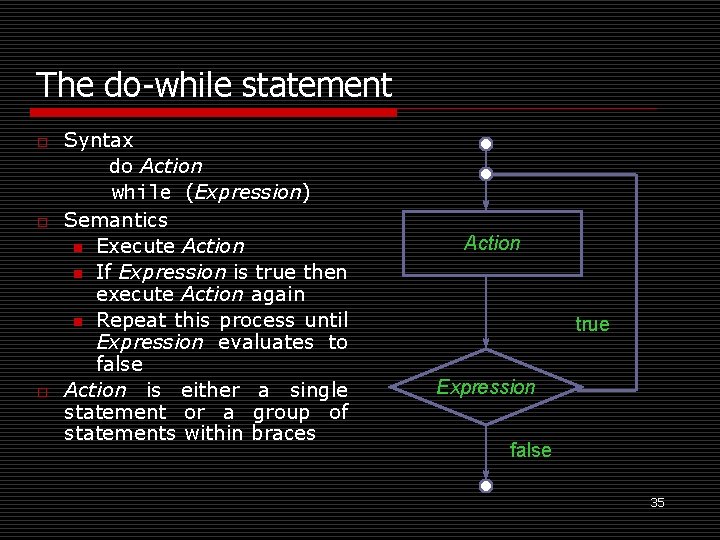
The do-while statement o o o Syntax do Action while (Expression) Semantics n Execute Action n If Expression is true then execute Action again n Repeat this process until Expression evaluates to false Action is either a single statement or a group of statements within braces Action true Expression false 35
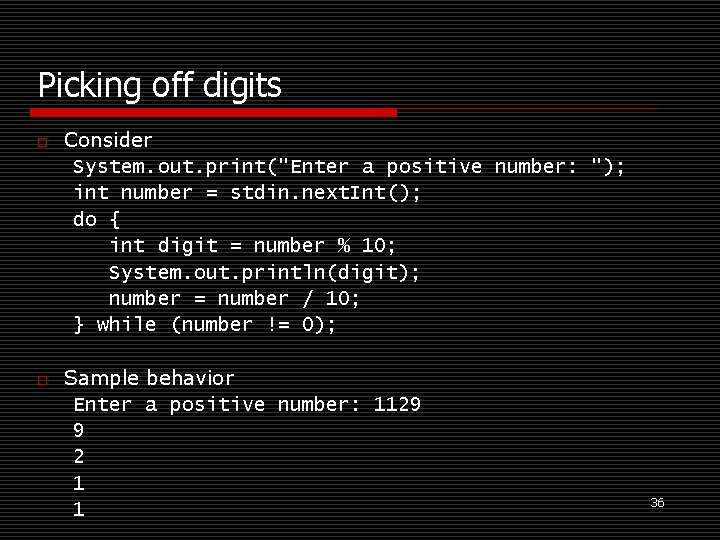
Picking off digits o o Consider System. out. print("Enter a positive number: "); int number = stdin. next. Int(); do { int digit = number % 10; System. out. println(digit); number = number / 10; } while (number != 0); Sample behavior Enter a positive number: 1129 9 2 1 1 36
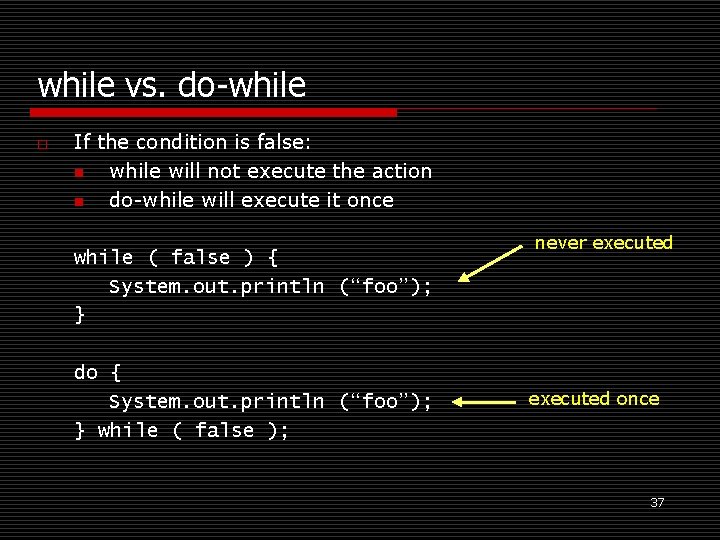
while vs. do-while o If the condition is false: n while will not execute the action n do-while will execute it once while ( false ) { System. out. println (“foo”); } do { System. out. println (“foo”); } while ( false ); never executed once 37
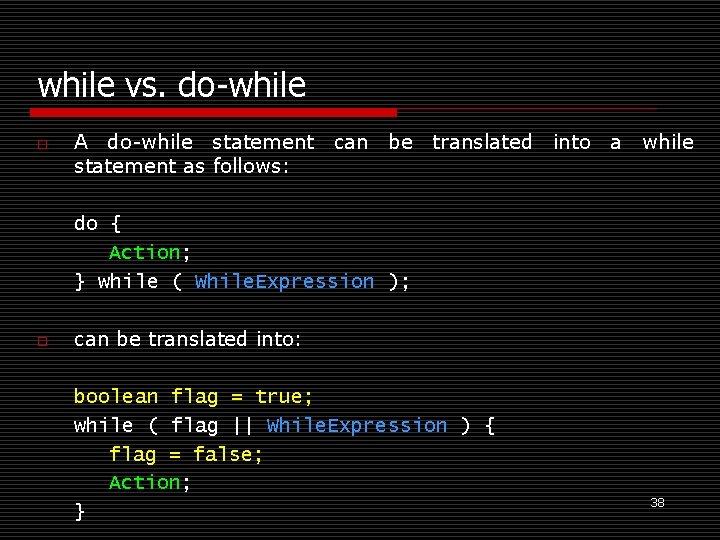
while vs. do-while o A do-while statement can be translated into a while statement as follows: do { Action; } while ( While. Expression ); o can be translated into: boolean flag = true; while ( flag || While. Expression ) { flag = false; Action; } 38
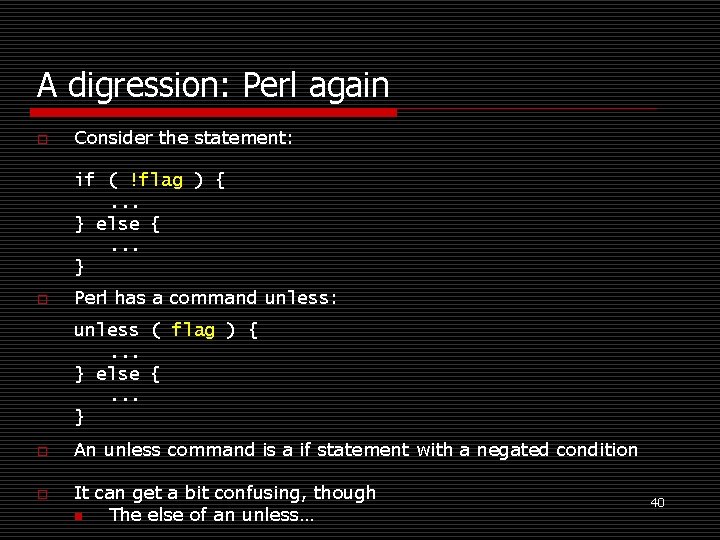
A digression: Perl again o Consider the statement: if ( !flag ) {. . . } else {. . . } o Perl has a command unless: unless ( flag ) {. . . } else {. . . } o o An unless command is a if statement with a negated condition It can get a bit confusing, though n The else of an unless… 40
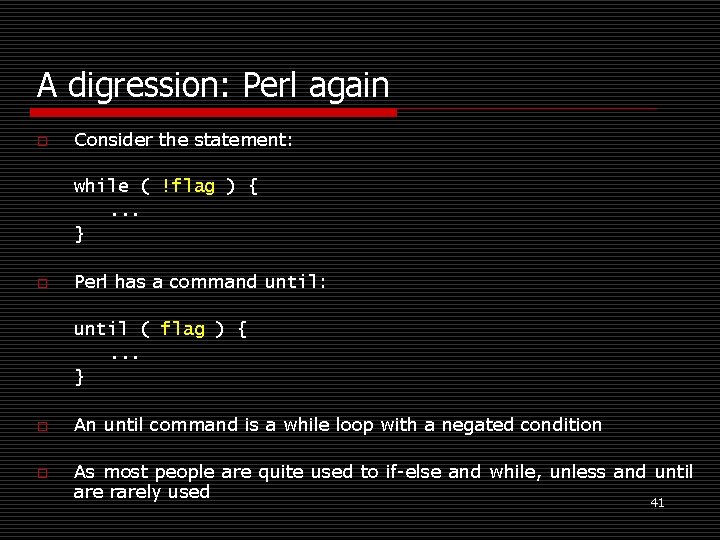
A digression: Perl again o Consider the statement: while ( !flag ) {. . . } o Perl has a command until: until ( flag ) {. . . } o o An until command is a while loop with a negated condition As most people are quite used to if-else and while, unless and until are rarely used 41
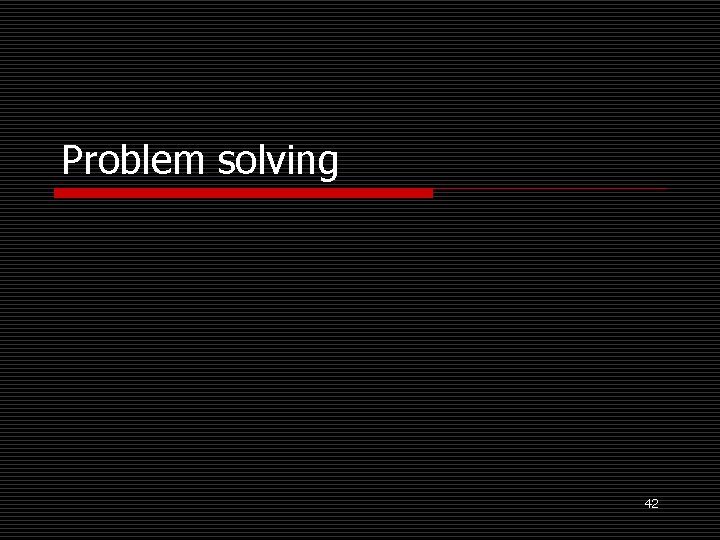
Problem solving 42
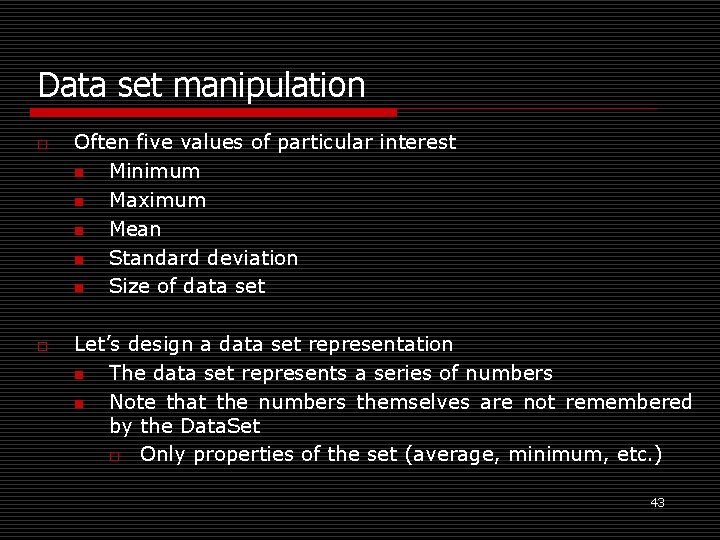
Data set manipulation o o Often five values of particular interest n Minimum n Maximum n Mean n Standard deviation n Size of data set Let’s design a data set representation n The data set represents a series of numbers n Note that the numbers themselves are not remembered by the Data. Set o Only properties of the set (average, minimum, etc. ) 43
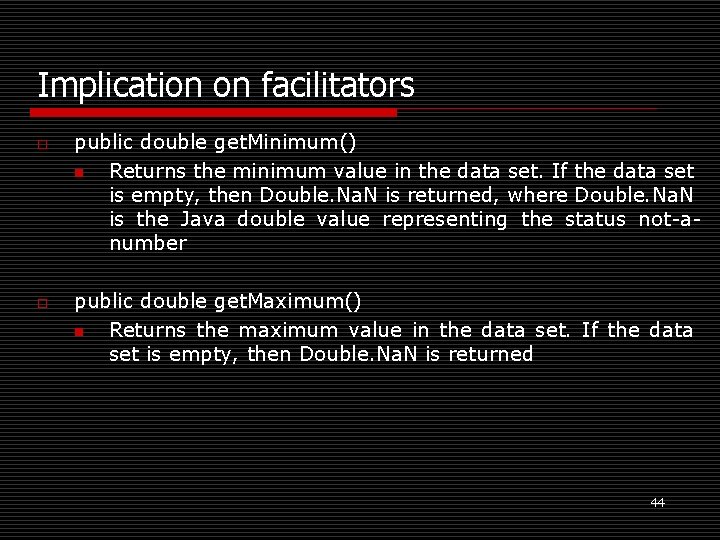
Implication on facilitators o o public double get. Minimum() n Returns the minimum value in the data set. If the data set is empty, then Double. Na. N is returned, where Double. Na. N is the Java double value representing the status not-anumber public double get. Maximum() n Returns the maximum value in the data set. If the data set is empty, then Double. Na. N is returned 44
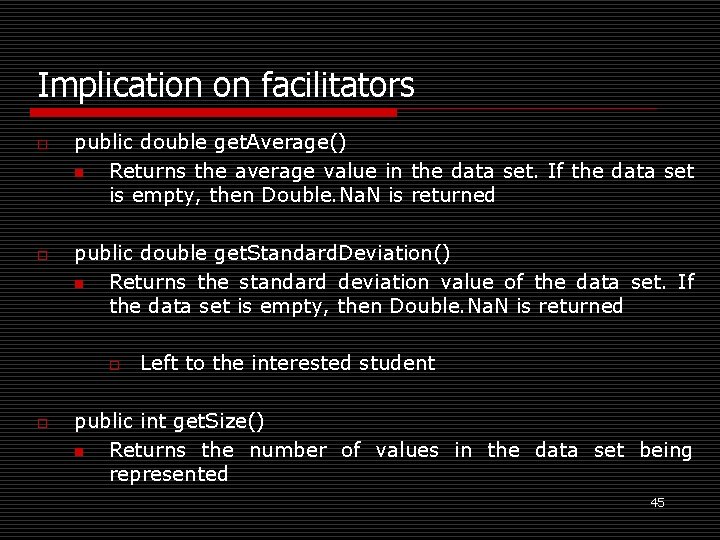
Implication on facilitators o o public double get. Average() n Returns the average value in the data set. If the data set is empty, then Double. Na. N is returned public double get. Standard. Deviation() n Returns the standard deviation value of the data set. If the data set is empty, then Double. Na. N is returned o o Left to the interested student public int get. Size() n Returns the number of values in the data set being represented 45
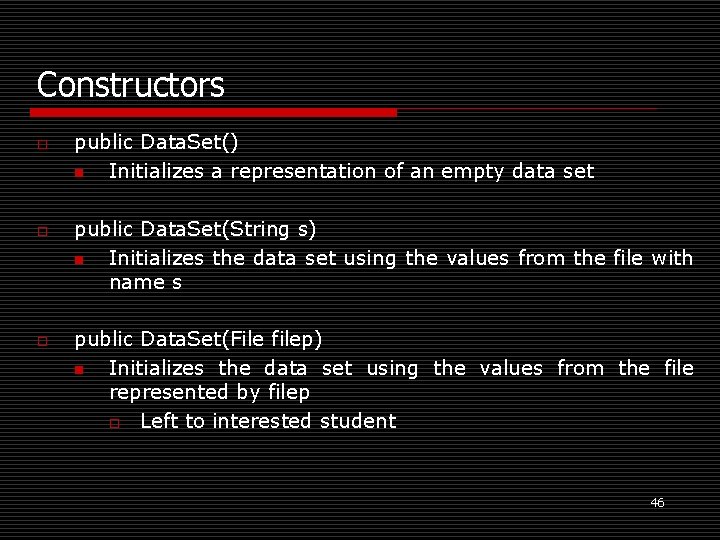
Constructors o o o public Data. Set() n Initializes a representation of an empty data set public Data. Set(String s) n Initializes the data set using the values from the file with name s public Data. Set(File filep) n Initializes the data set using the values from the file represented by filep o Left to interested student 46
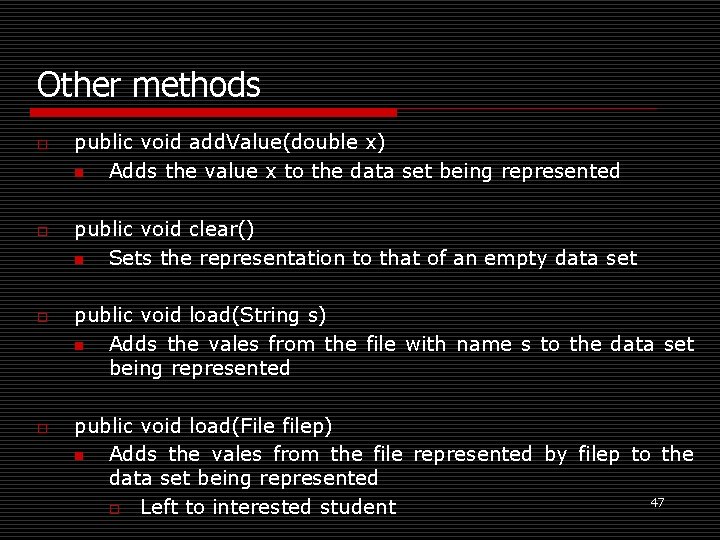
Other methods o o public void add. Value(double x) n Adds the value x to the data set being represented public void clear() n Sets the representation to that of an empty data set public void load(String s) n Adds the vales from the file with name s to the data set being represented public void load(File filep) n Adds the vales from the file represented by filep to the data set being represented 47 o Left to interested student
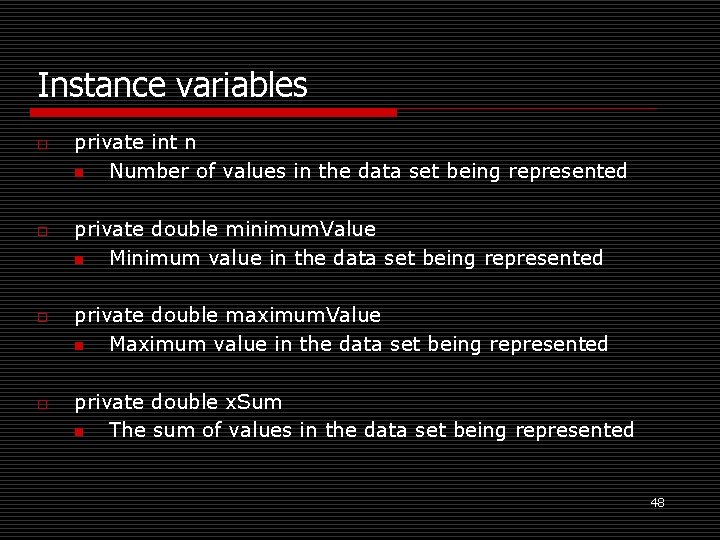
Instance variables o o private int n n Number of values in the data set being represented private double minimum. Value n Minimum value in the data set being represented private double maximum. Value n Maximum value in the data set being represented private double x. Sum n The sum of values in the data set being represented 48
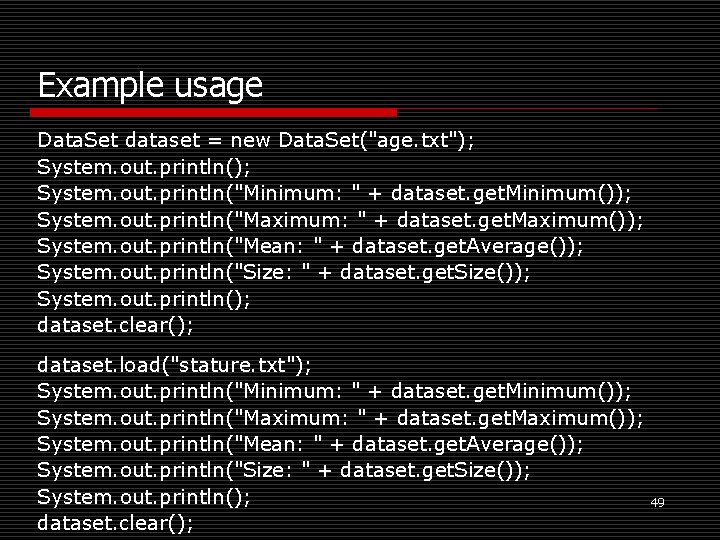
Example usage Data. Set dataset = new Data. Set("age. txt"); System. out. println("Minimum: " + dataset. get. Minimum()); System. out. println("Maximum: " + dataset. get. Maximum()); System. out. println("Mean: " + dataset. get. Average()); System. out. println("Size: " + dataset. get. Size()); System. out. println(); dataset. clear(); dataset. load("stature. txt"); System. out. println("Minimum: " + dataset. get. Minimum()); System. out. println("Maximum: " + dataset. get. Maximum()); System. out. println("Mean: " + dataset. get. Average()); System. out. println("Size: " + dataset. get. Size()); System. out. println(); 49 dataset. clear();
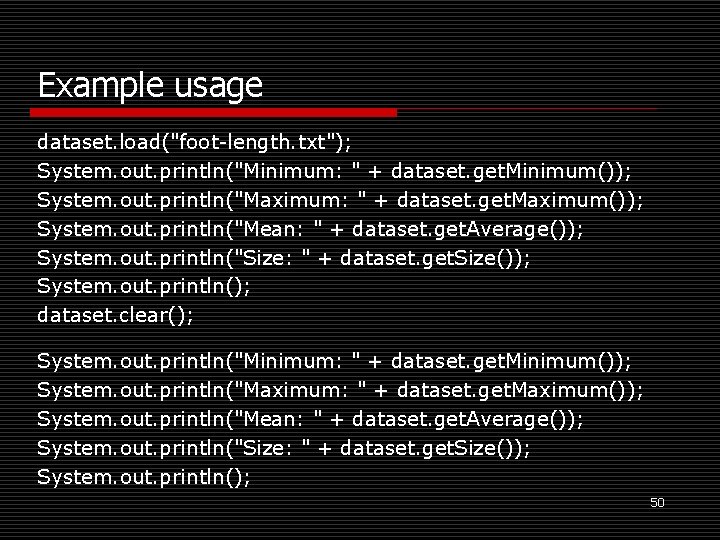
Example usage dataset. load("foot-length. txt"); System. out. println("Minimum: " + dataset. get. Minimum()); System. out. println("Maximum: " + dataset. get. Maximum()); System. out. println("Mean: " + dataset. get. Average()); System. out. println("Size: " + dataset. get. Size()); System. out. println(); dataset. clear(); System. out. println("Minimum: " + dataset. get. Minimum()); System. out. println("Maximum: " + dataset. get. Maximum()); System. out. println("Mean: " + dataset. get. Average()); System. out. println("Size: " + dataset. get. Size()); System. out. println(); 50
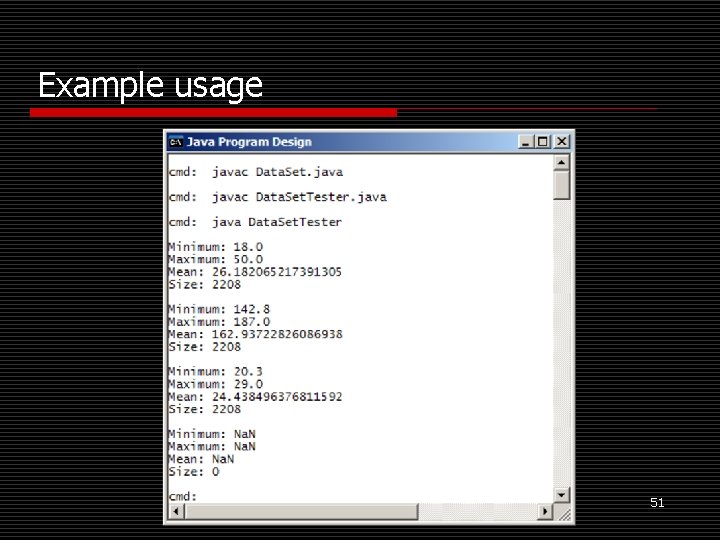
Example usage 51
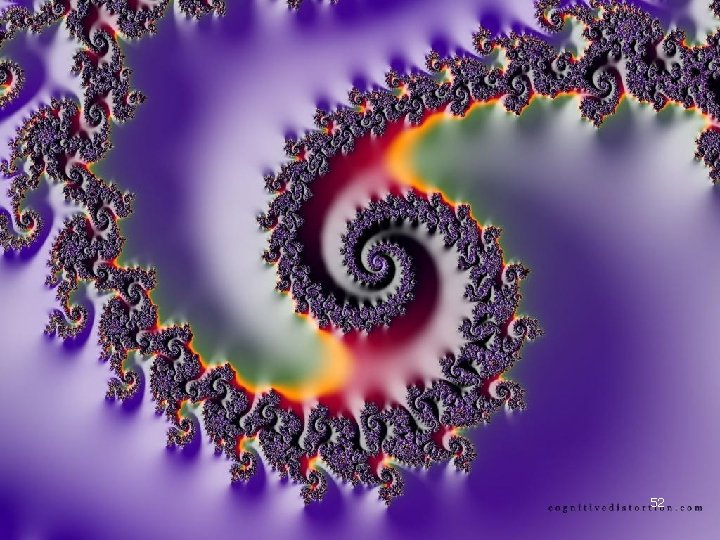
Fractals 52
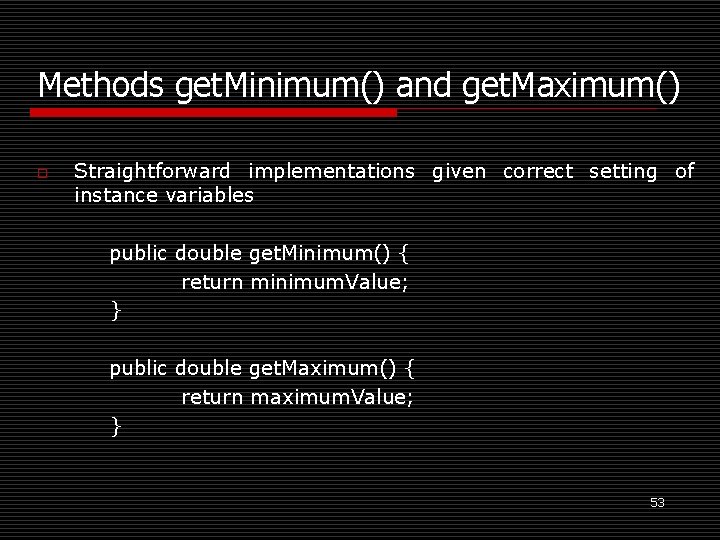
Methods get. Minimum() and get. Maximum() o Straightforward implementations given correct setting of instance variables public double get. Minimum() { return minimum. Value; } public double get. Maximum() { return maximum. Value; } 53
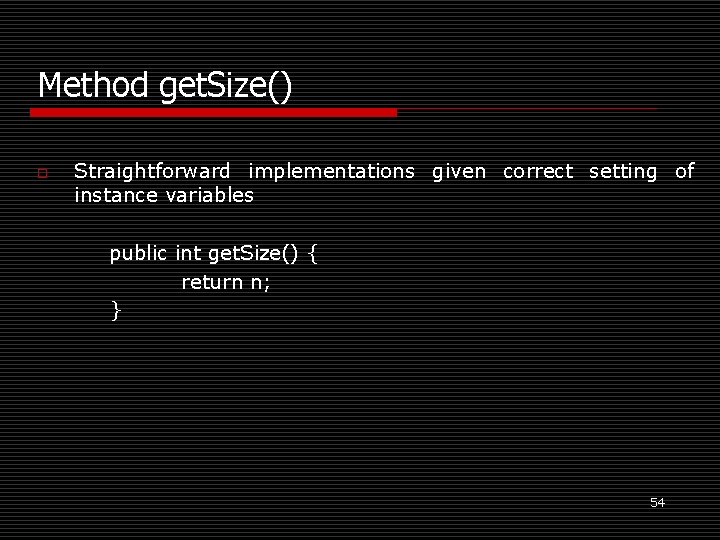
Method get. Size() o Straightforward implementations given correct setting of instance variables public int get. Size() { return n; } 54
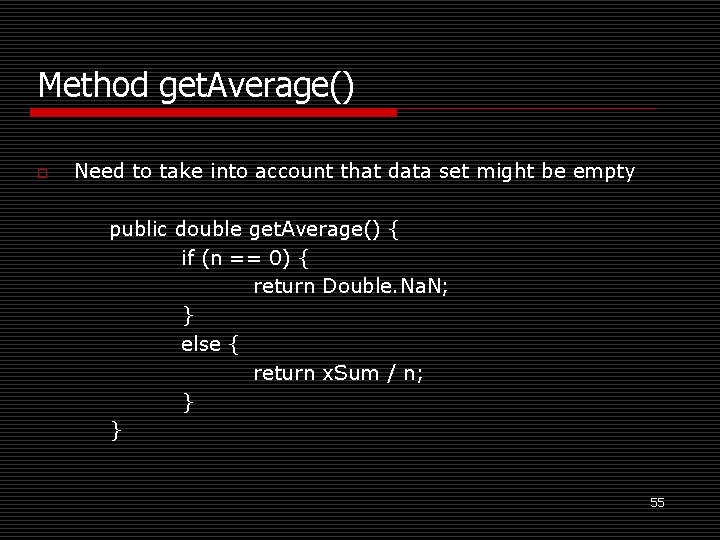
Method get. Average() o Need to take into account that data set might be empty public double get. Average() { if (n == 0) { return Double. Na. N; } else { return x. Sum / n; } } 55
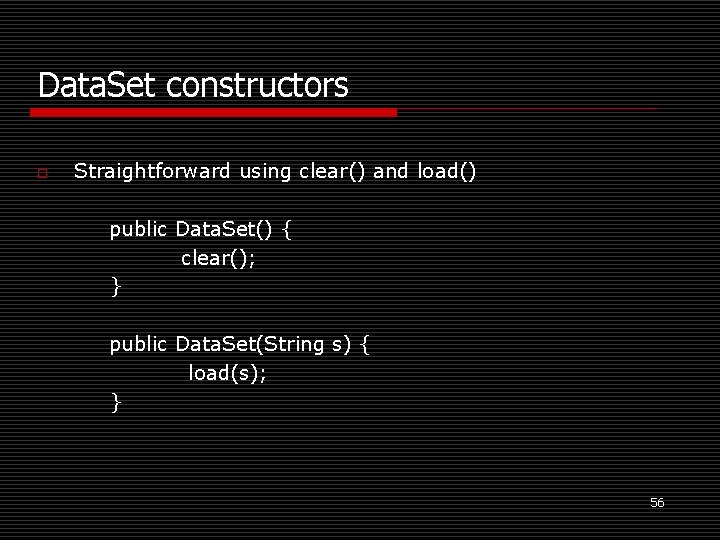
Data. Set constructors o Straightforward using clear() and load() public Data. Set() { clear(); } public Data. Set(String s) { load(s); } 56
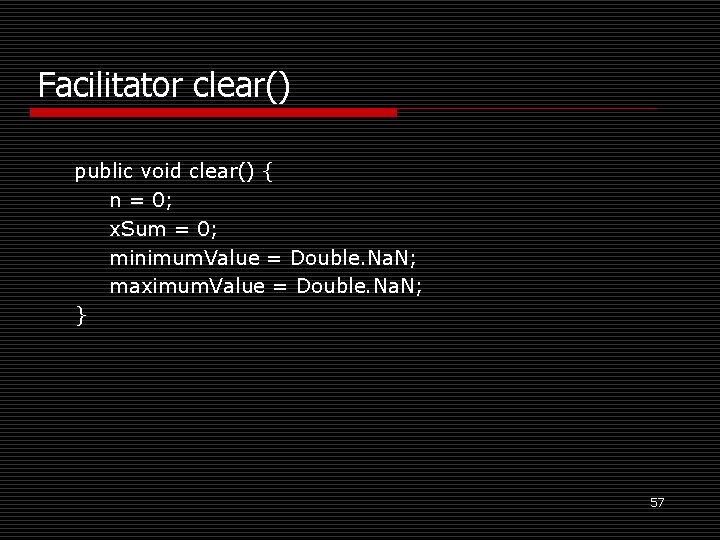
Facilitator clear() public void clear() { n = 0; x. Sum = 0; minimum. Value = Double. Na. N; maximum. Value = Double. Na. N; } 57
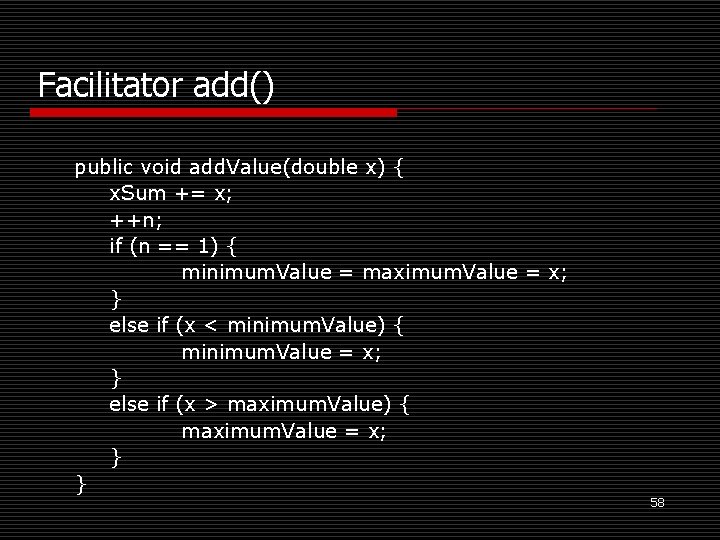
Facilitator add() public void add. Value(double x) { x. Sum += x; ++n; if (n == 1) { minimum. Value = maximum. Value = x; } else if (x < minimum. Value) { minimum. Value = x; } else if (x > maximum. Value) { maximum. Value = x; } } 58
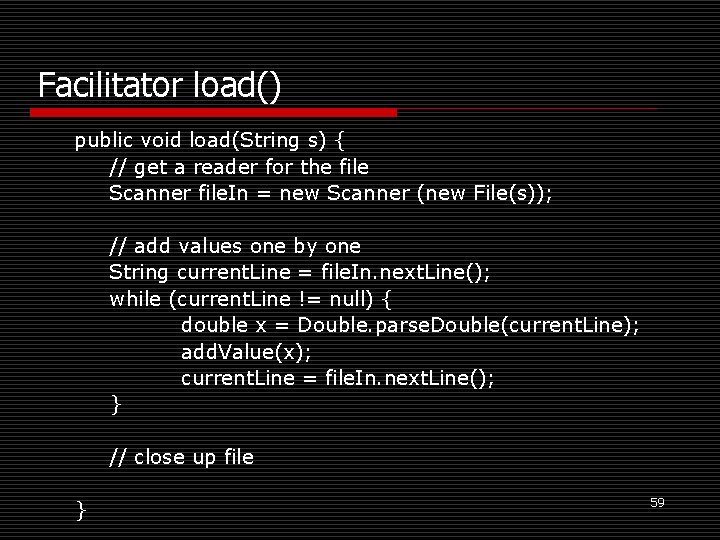
Facilitator load() public void load(String s) { // get a reader for the file Scanner file. In = new Scanner (new File(s)); // add values one by one String current. Line = file. In. next. Line(); while (current. Line != null) { double x = Double. parse. Double(current. Line); add. Value(x); current. Line = file. In. next. Line(); } // close up file } 59
Perulangan bersarang java
Iteration statements in java
Int x = 0; for (x=1; x<4; x++); printf("x=%d ", x);
Looping statement in qbasic
Struktur
Pert vs cpm
Array
What is a workaround
Pascal looping
Digunakan untuk mengerjakan
Nested for loop alternative
Looping
Counter controlled loop flowchart
Looping writing
Perulangan looping
Small basic turtle graphics
Disadvantages of looping in education
Apple core
Loop aircraft motion
Perulangan looping
Bentuk umum statemen for adalah
Looping algoritma
Counter controlled
Looping structure
Contoh flowchart perulangan for
Metode pengulangan
Value iteration
Lambda iteration
Embarrassingly parallel meaning
Recursion vs iteration
Fixed point iteration method
Value iteration
5 levels of agile planning
Iteration vs recursion
Entity life history
Jurassic park chaos theory
Value iteration
Scratch iteration
Lambda iteration
Tail recursion vs iteration
What is the purpose of an iteration recap?
Iteration structure
Iteration
Newton raphson method
Lambda iteration
What is the purpose of iteration goals?
Iteration abstraction
Iteration definition computer science
Iteration.n5
Orthogonal iteration
Gauss seidel method example
Iteration workflows in software project management
Bbc iteration
Value iteration
Fitted q iteration
Final iteration definition
Ncut
Iterating in math