LOOPING What are the 3 structures of writing
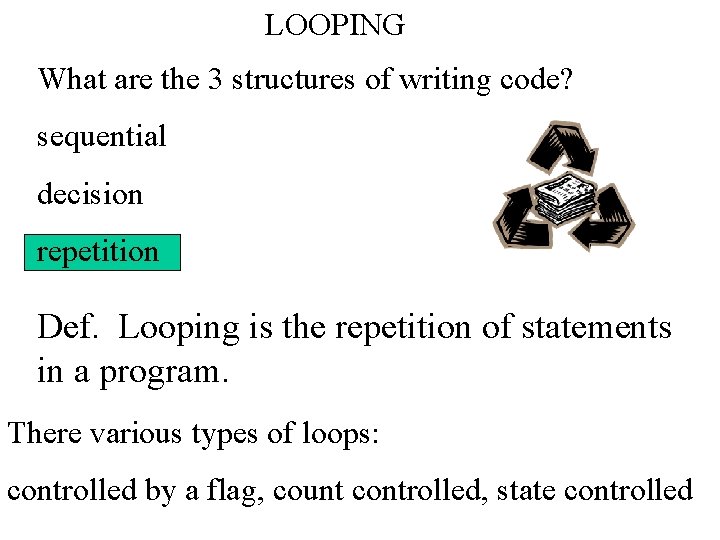
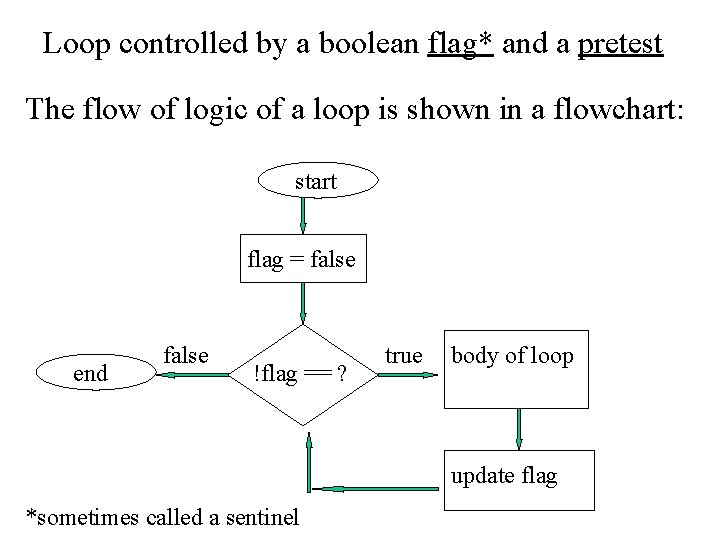
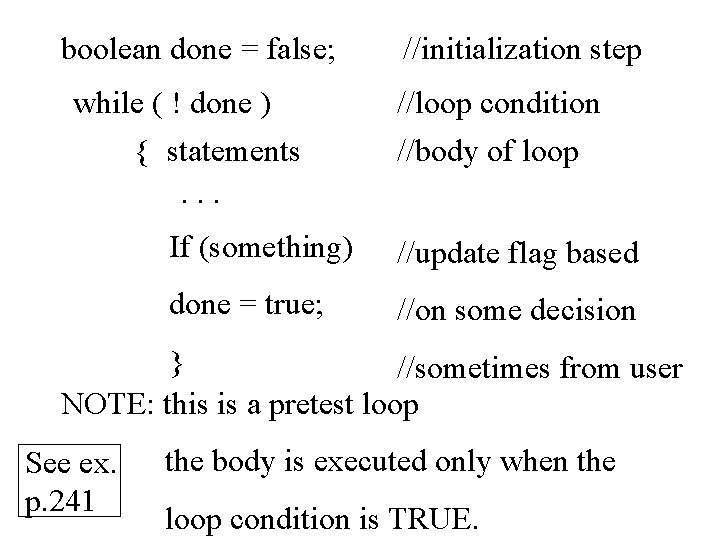
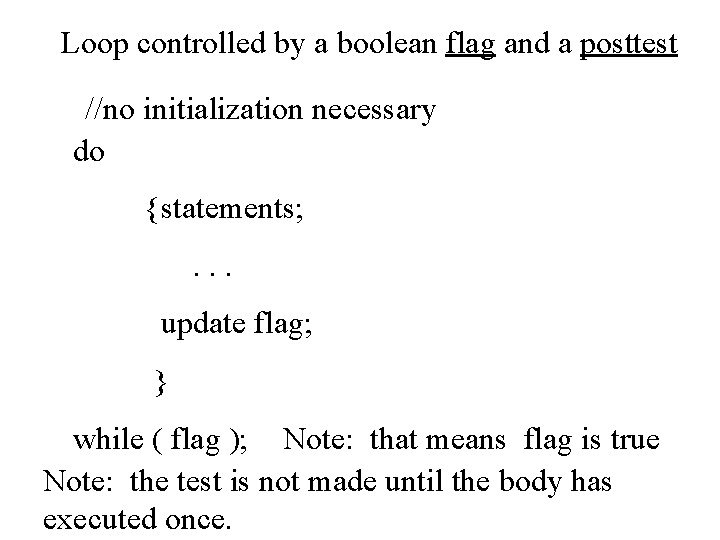
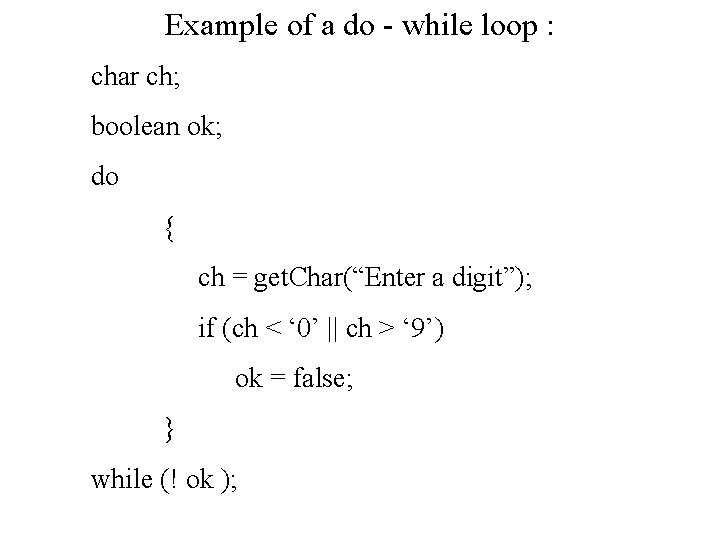
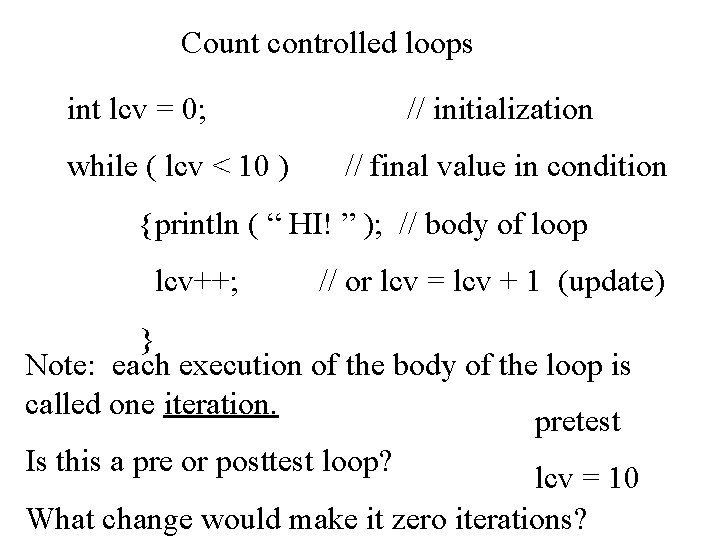
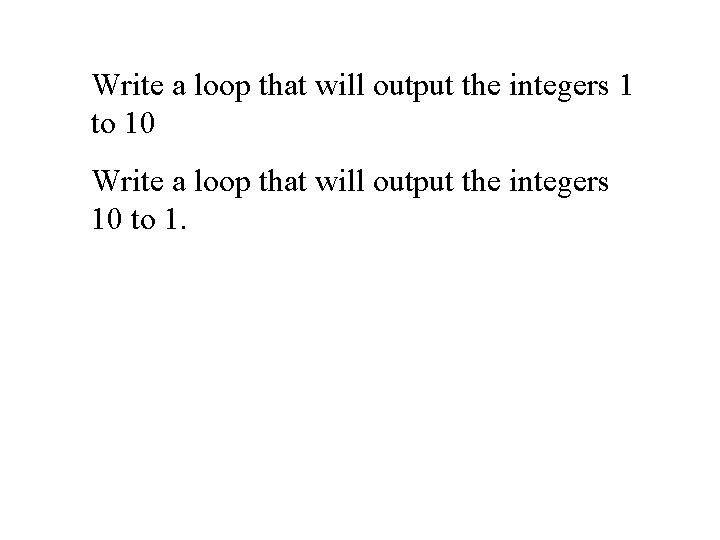
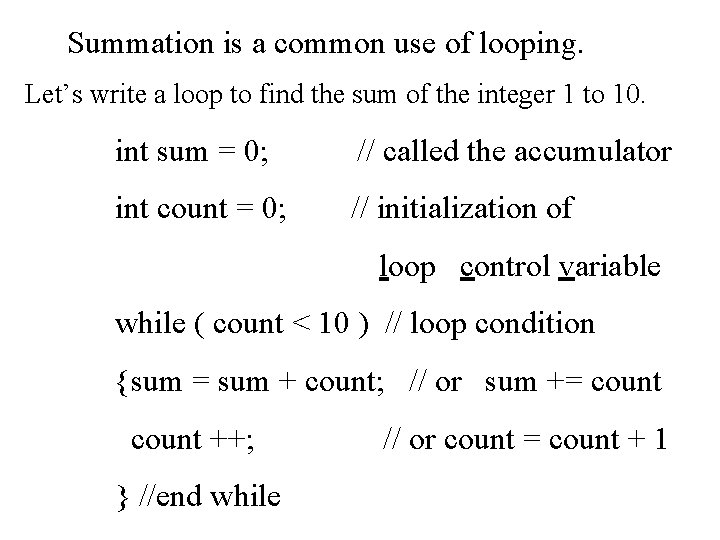
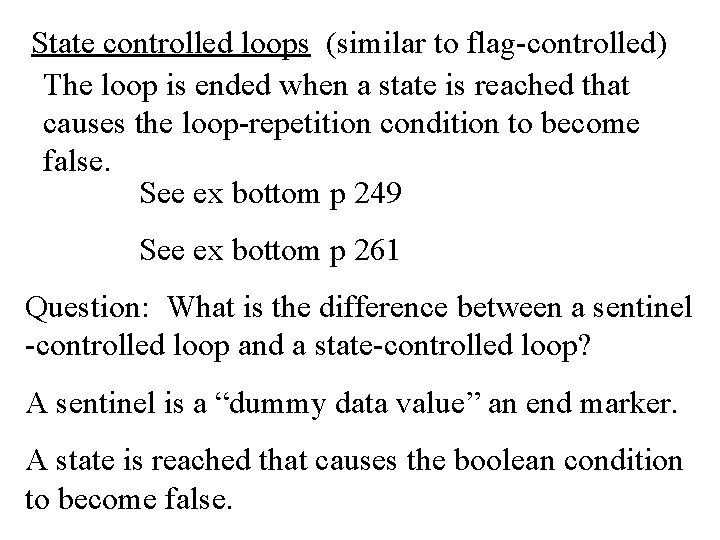
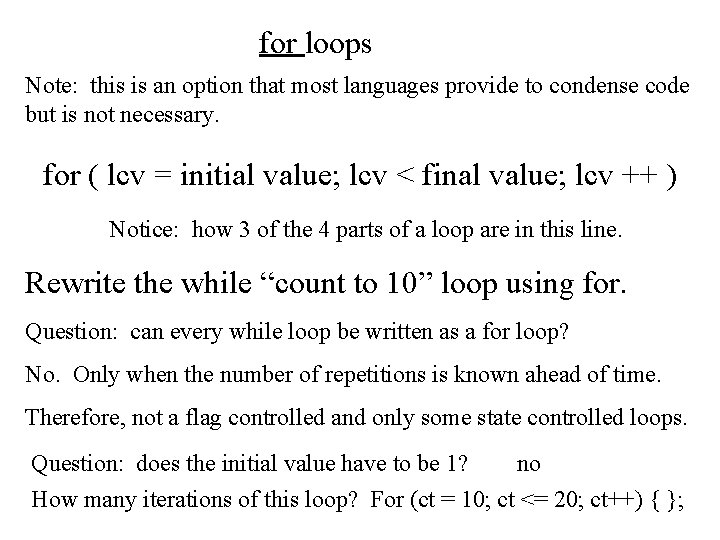
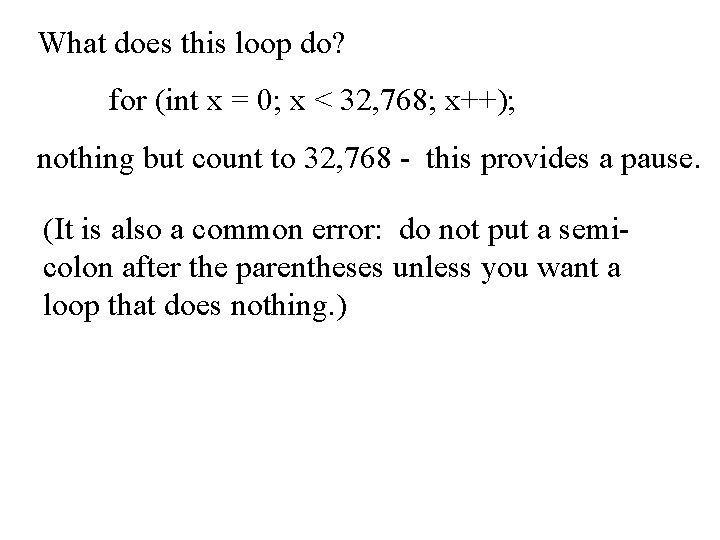
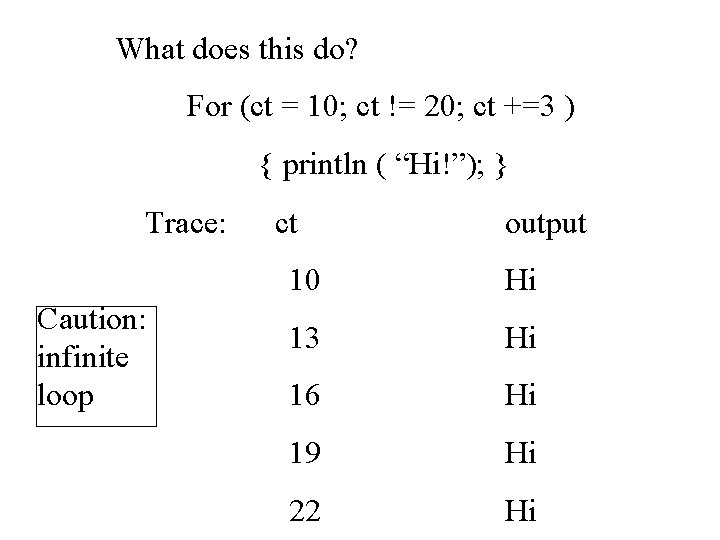
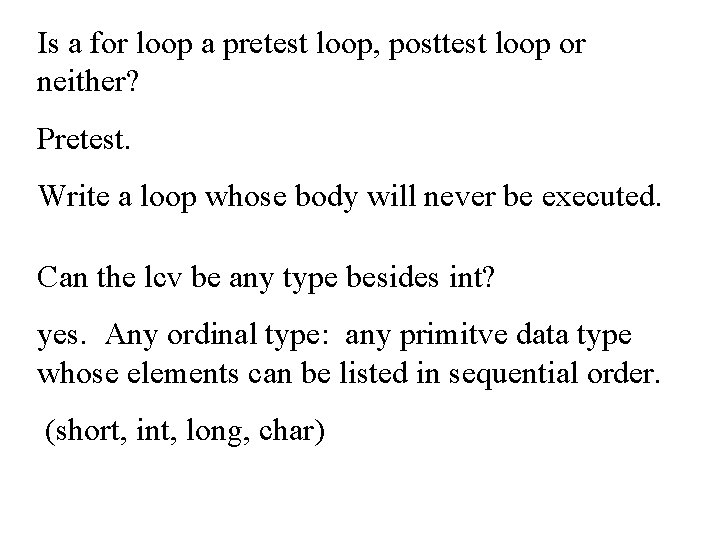
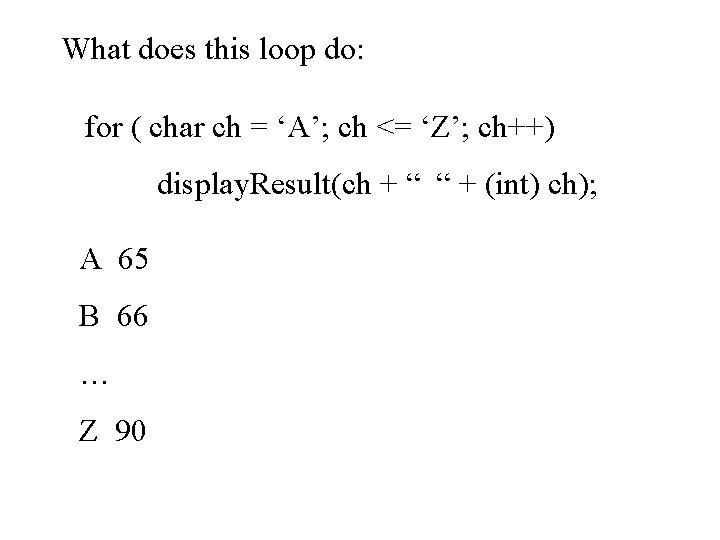
- Slides: 14
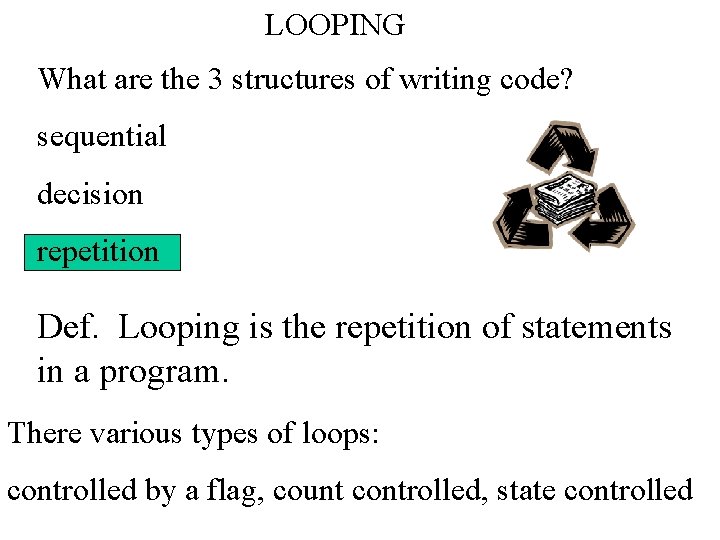
LOOPING What are the 3 structures of writing code? sequential decision repetition Def. Looping is the repetition of statements in a program. There various types of loops: controlled by a flag, count controlled, state controlled
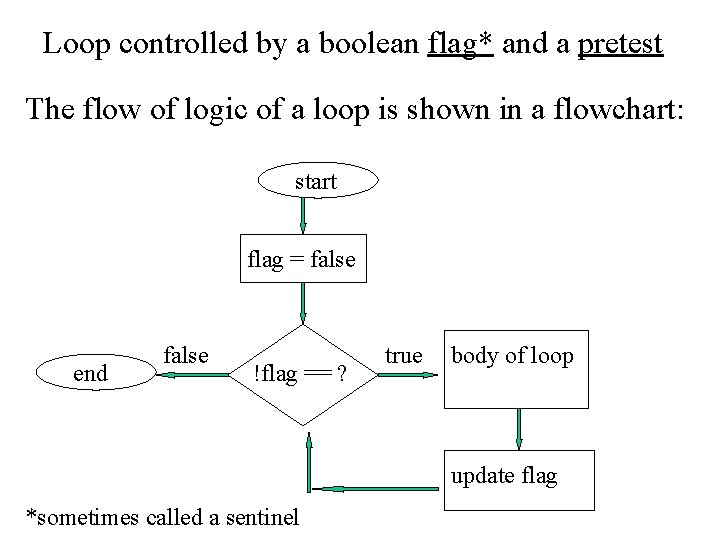
Loop controlled by a boolean flag* and a pretest The flow of logic of a loop is shown in a flowchart: start flag = false end false !flag == ? true body of loop update flag *sometimes called a sentinel
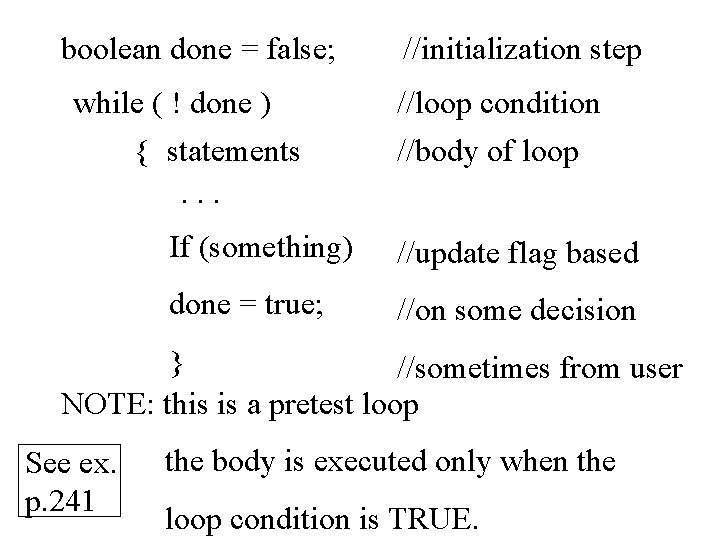
boolean done = false; while ( ! done ) { statements. . . //initialization step //loop condition //body of loop If (something) //update flag based done = true; //on some decision } //sometimes from user NOTE: this is a pretest loop See ex. p. 241 the body is executed only when the loop condition is TRUE.
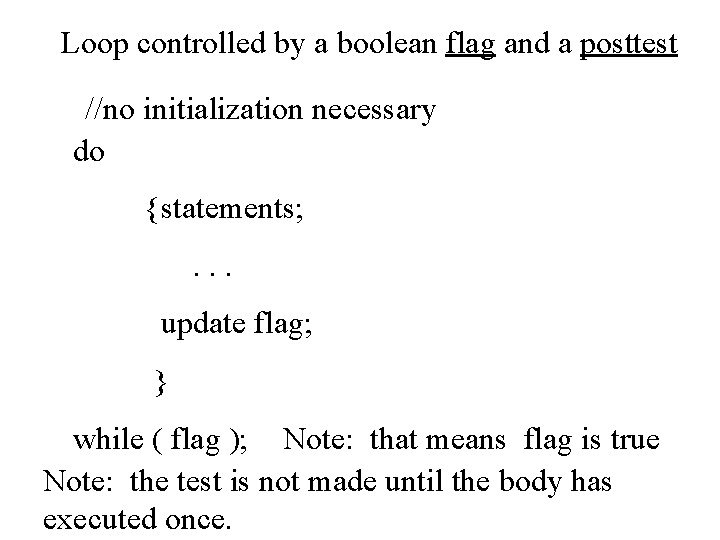
Loop controlled by a boolean flag and a posttest //no initialization necessary do {statements; . . . update flag; } while ( flag ); Note: that means flag is true Note: the test is not made until the body has executed once.
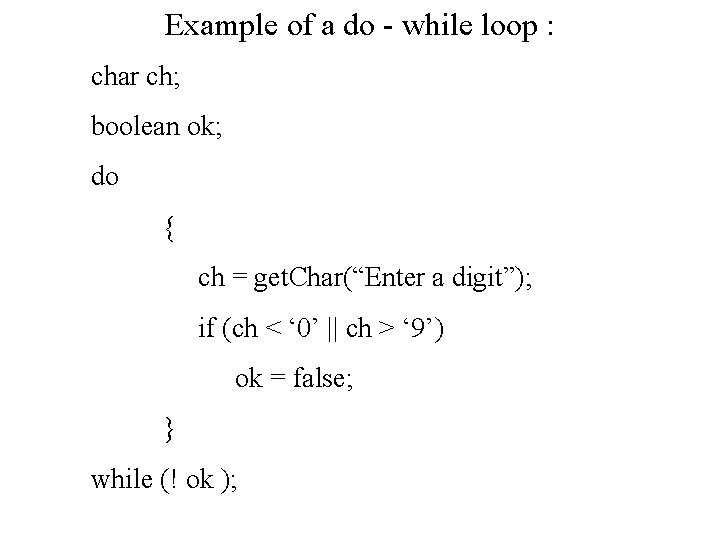
Example of a do - while loop : char ch; boolean ok; do { ch = get. Char(“Enter a digit”); if (ch < ‘ 0’ || ch > ‘ 9’) ok = false; } while (! ok );
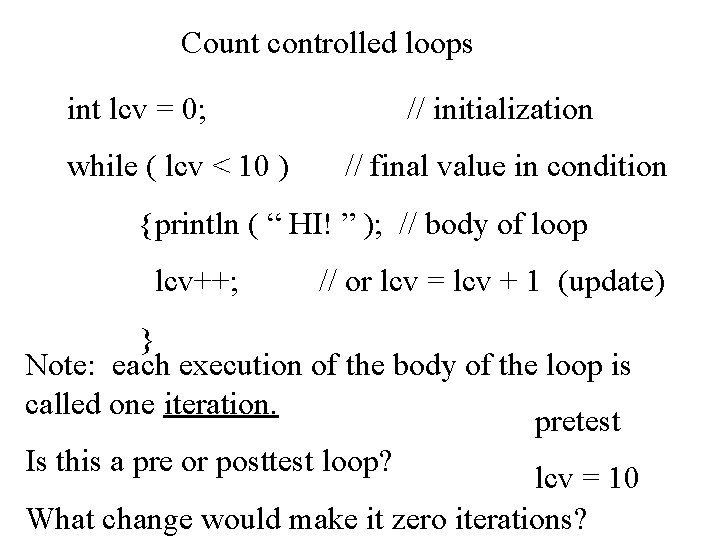
Count controlled loops int lcv = 0; while ( lcv < 10 ) // initialization // final value in condition {println ( “ HI! ” ); // body of loop lcv++; // or lcv = lcv + 1 (update) } Note: each execution of the body of the loop is called one iteration. pretest Is this a pre or posttest loop? lcv = 10 What change would make it zero iterations?
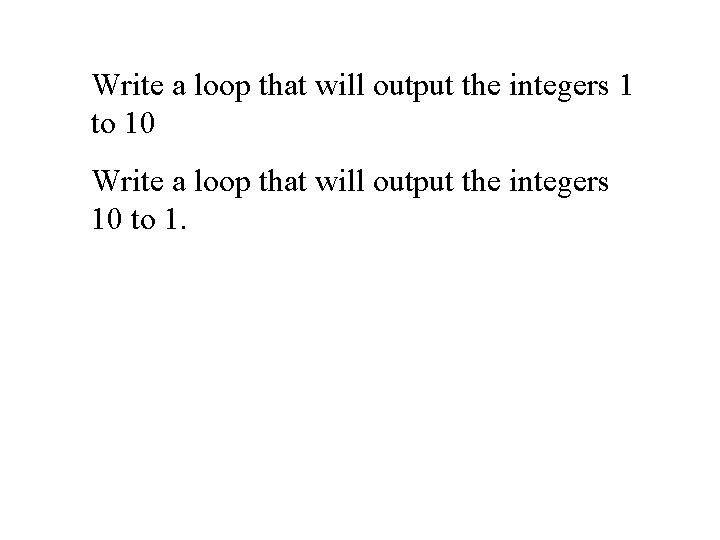
Write a loop that will output the integers 1 to 10 Write a loop that will output the integers 10 to 1.
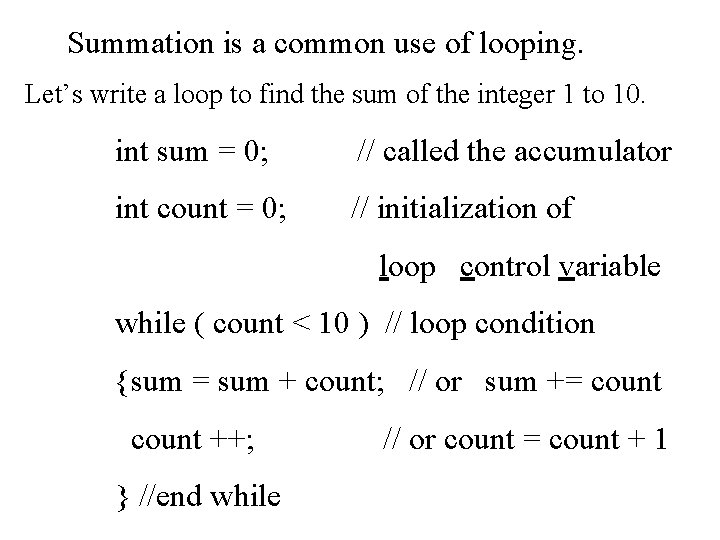
Summation is a common use of looping. Let’s write a loop to find the sum of the integer 1 to 10. int sum = 0; // called the accumulator int count = 0; // initialization of loop control variable while ( count < 10 ) // loop condition {sum = sum + count; // or sum += count ++; } //end while // or count = count + 1
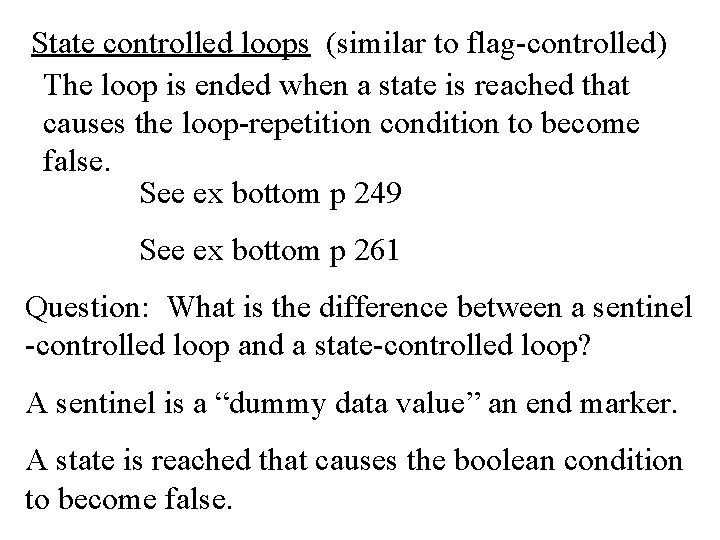
State controlled loops (similar to flag-controlled) The loop is ended when a state is reached that causes the loop-repetition condition to become false. See ex bottom p 249 See ex bottom p 261 Question: What is the difference between a sentinel -controlled loop and a state-controlled loop? A sentinel is a “dummy data value” an end marker. A state is reached that causes the boolean condition to become false.
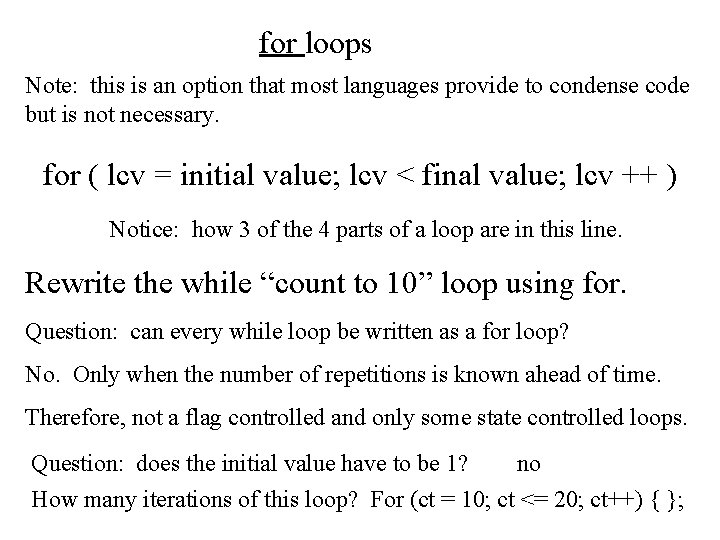
for loops Note: this is an option that most languages provide to condense code but is not necessary. for ( lcv = initial value; lcv < final value; lcv ++ ) Notice: how 3 of the 4 parts of a loop are in this line. Rewrite the while “count to 10” loop using for. Question: can every while loop be written as a for loop? No. Only when the number of repetitions is known ahead of time. Therefore, not a flag controlled and only some state controlled loops. Question: does the initial value have to be 1? no How many iterations of this loop? For (ct = 10; ct <= 20; ct++) { };
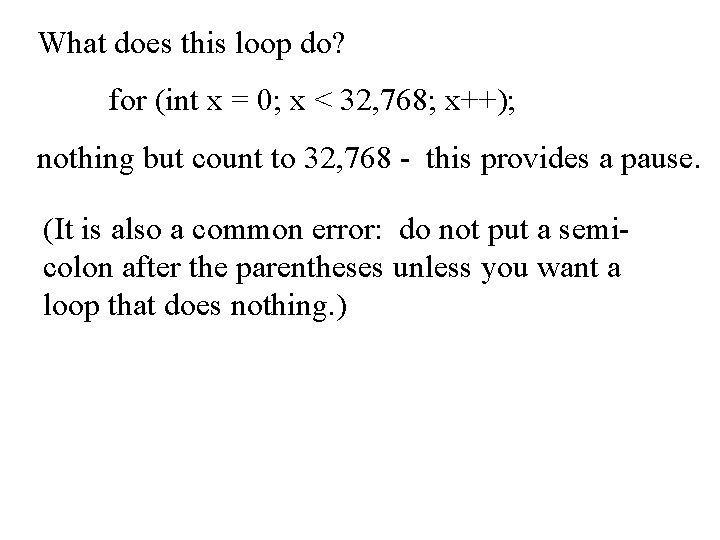
What does this loop do? for (int x = 0; x < 32, 768; x++); nothing but count to 32, 768 - this provides a pause. (It is also a common error: do not put a semicolon after the parentheses unless you want a loop that does nothing. )
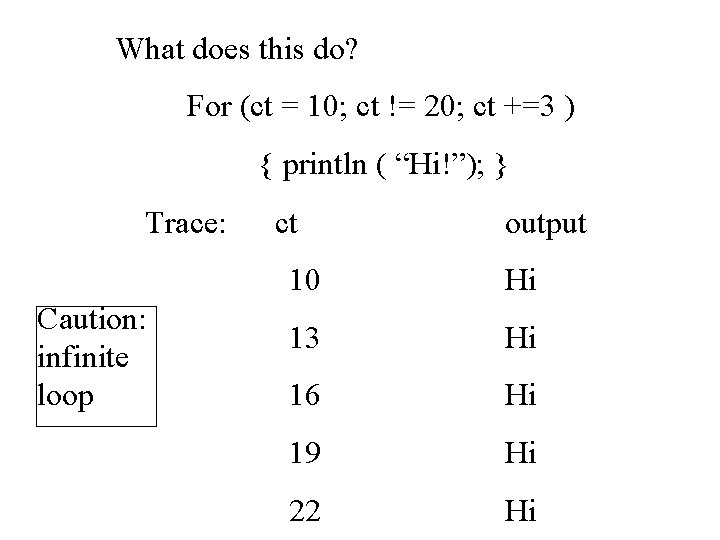
What does this do? For (ct = 10; ct != 20; ct +=3 ) { println ( “Hi!”); } Trace: Caution: infinite loop ct output 10 Hi 13 Hi 16 Hi 19 Hi 22 Hi
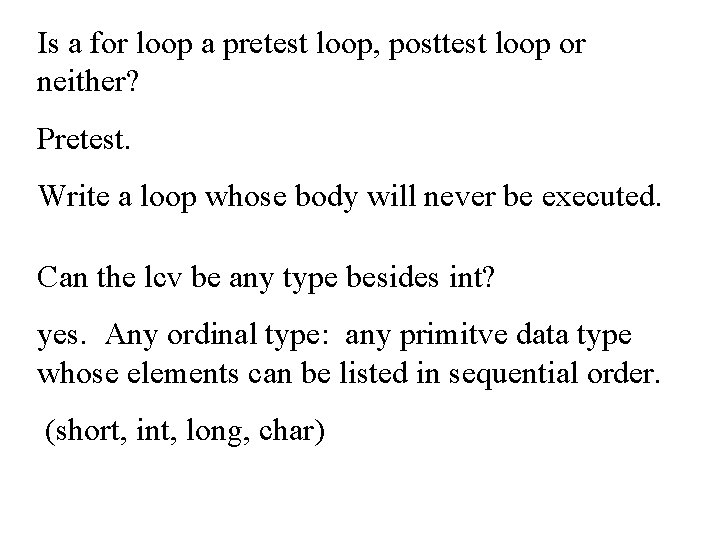
Is a for loop a pretest loop, posttest loop or neither? Pretest. Write a loop whose body will never be executed. Can the lcv be any type besides int? yes. Any ordinal type: any primitve data type whose elements can be listed in sequential order. (short, int, long, char)
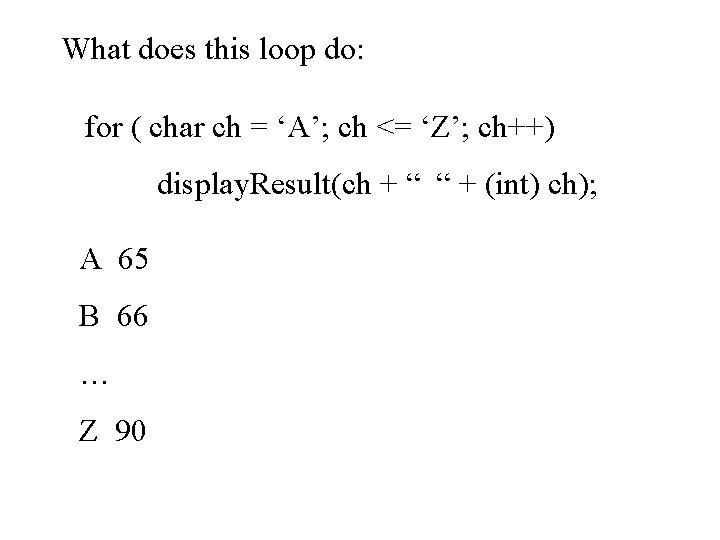
What does this loop do: for ( char ch = ‘A’; ch <= ‘Z’; ch++) display. Result(ch + “ “ + (int) ch); A 65 B 66 … Z 90
Insidan region jh
Looping in writing
Homologous structures examples
Loop adalah
Istilah "pengulangan" dalam pemrogram pascal dikenal dengan
Bentuk umum perulangan
Looping statement in qbasic
Looping bersarang
Perulangan bersarang java
Disadvantages of looping in education
Counter controlled
Dangling in network diagram
Automatic counter loop
Loop aircraft motion
Pengulangan merupakan struktur dasar