LOOPING What are the 3 structures of writing
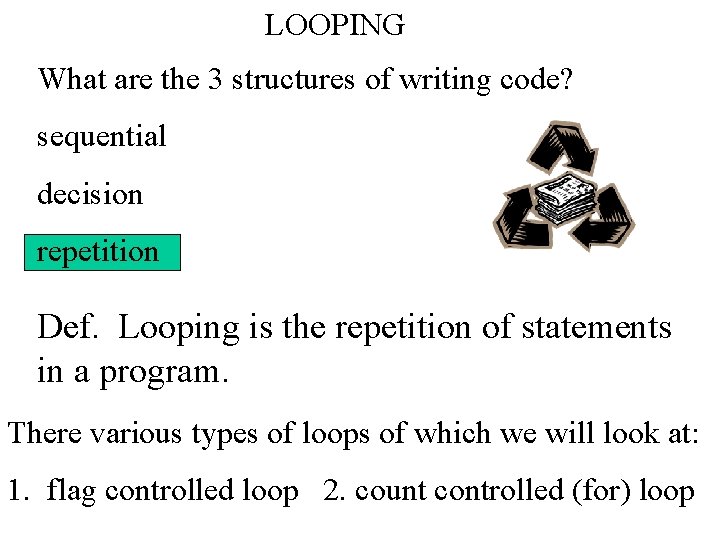
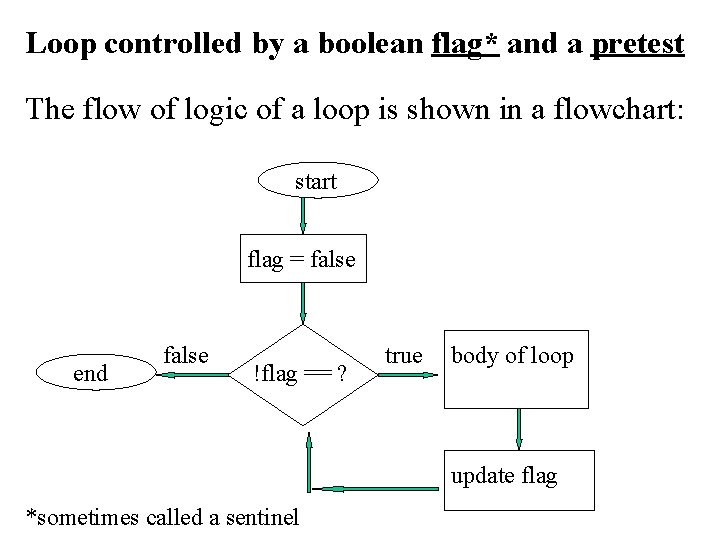
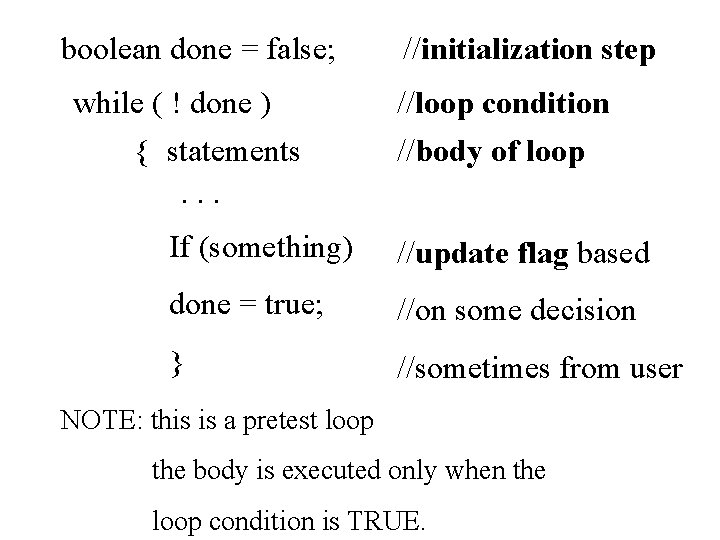
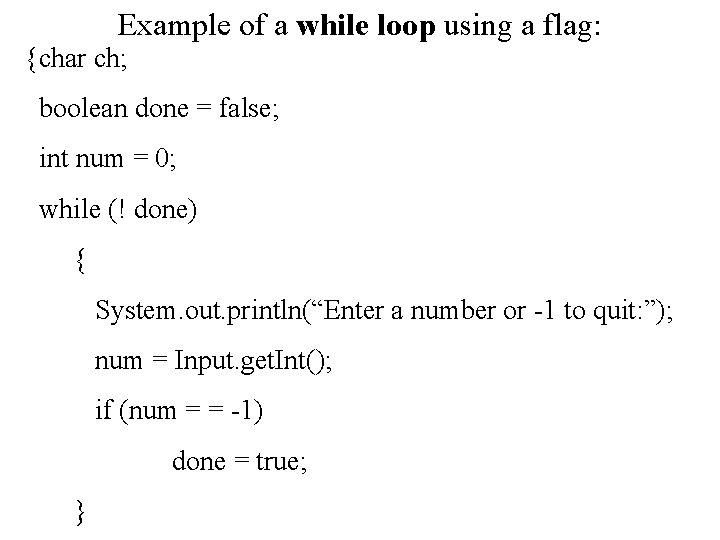
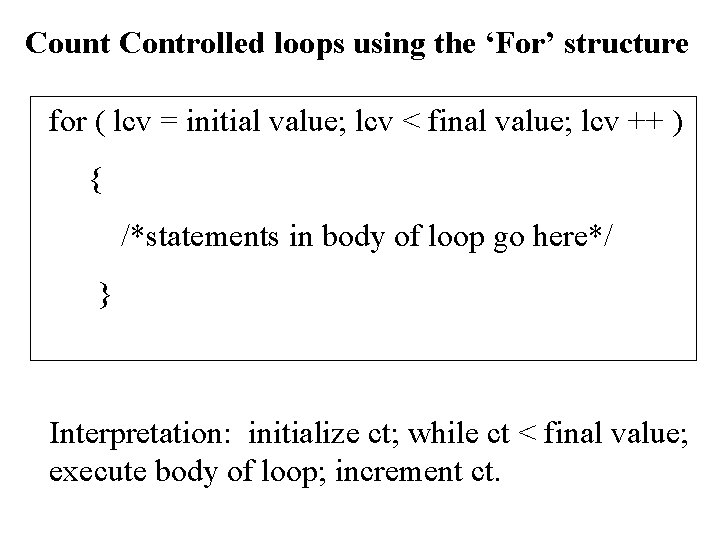
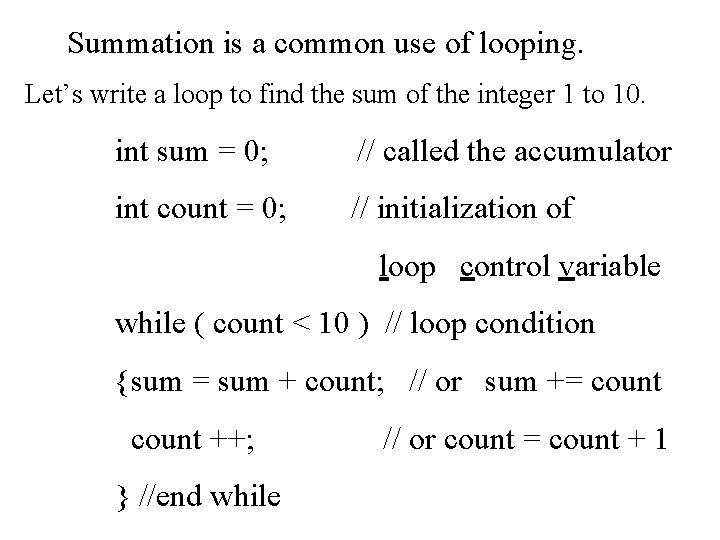
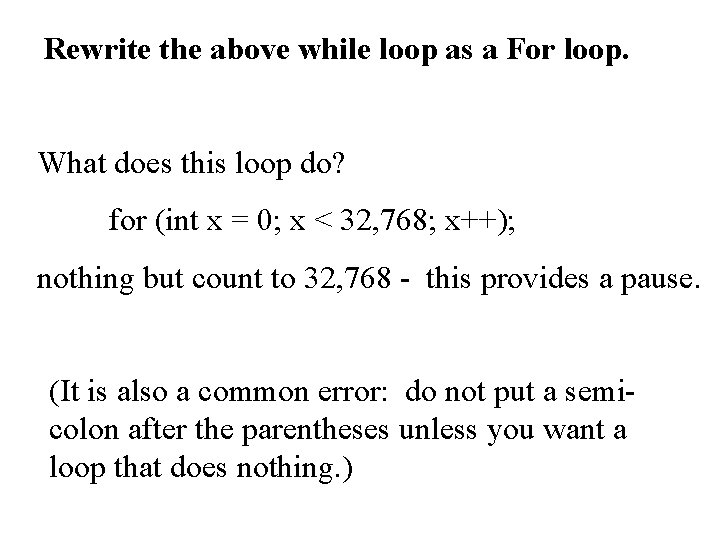
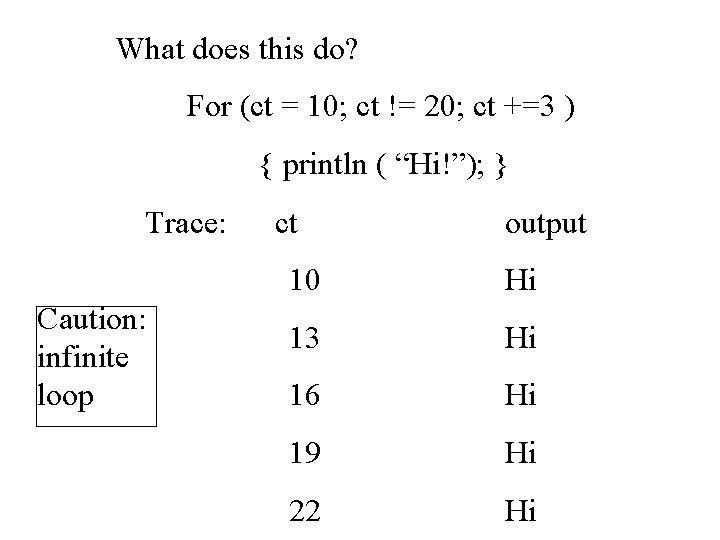
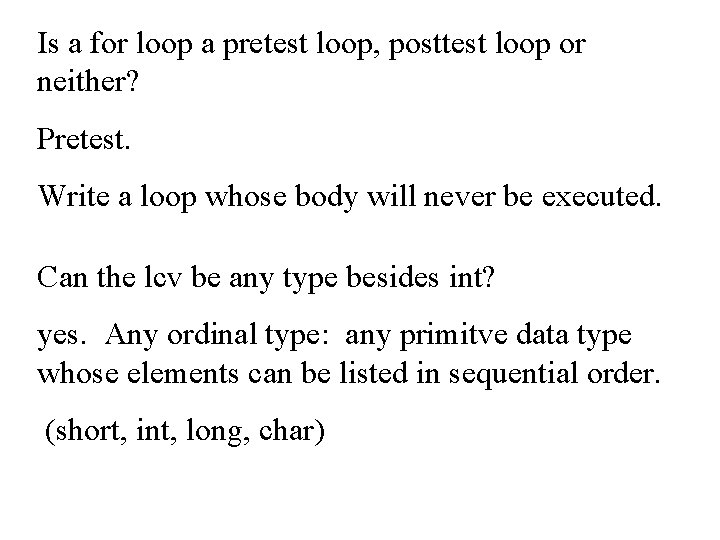
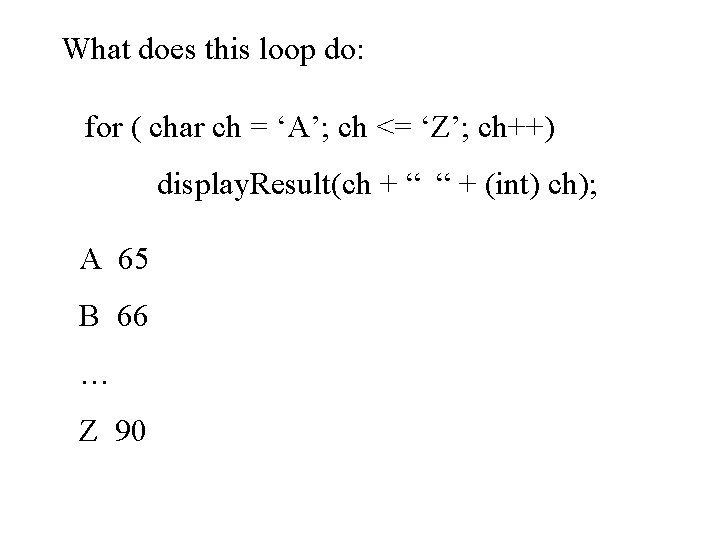
- Slides: 10
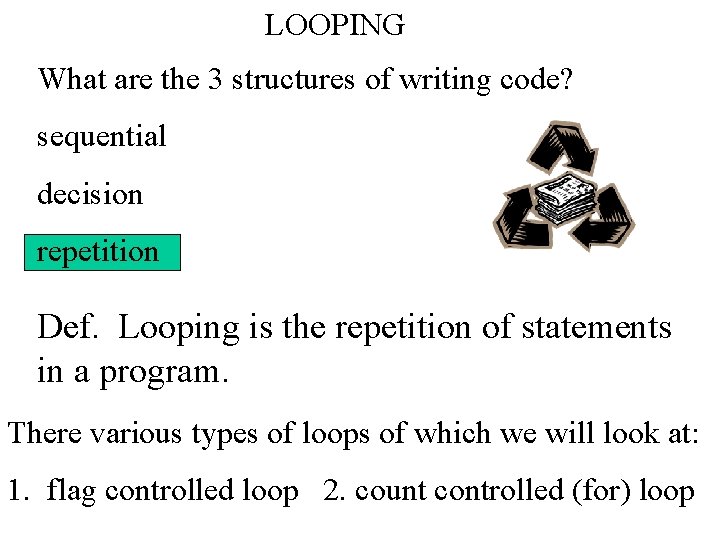
LOOPING What are the 3 structures of writing code? sequential decision repetition Def. Looping is the repetition of statements in a program. There various types of loops of which we will look at: 1. flag controlled loop 2. count controlled (for) loop
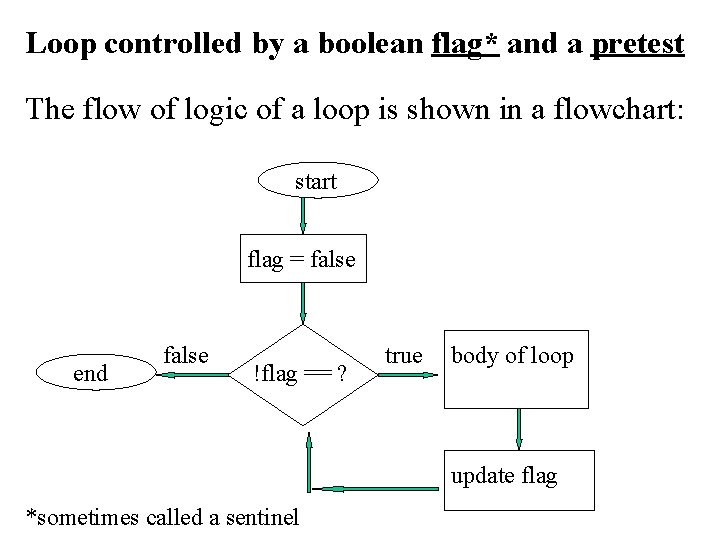
Loop controlled by a boolean flag* and a pretest The flow of logic of a loop is shown in a flowchart: start flag = false end false !flag == ? true body of loop update flag *sometimes called a sentinel
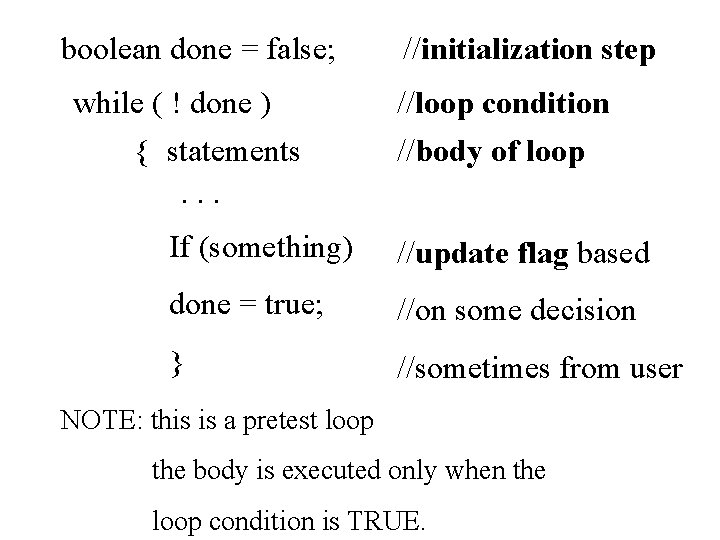
boolean done = false; while ( ! done ) { statements. . . //initialization step //loop condition //body of loop If (something) //update flag based done = true; //on some decision } //sometimes from user NOTE: this is a pretest loop the body is executed only when the loop condition is TRUE.
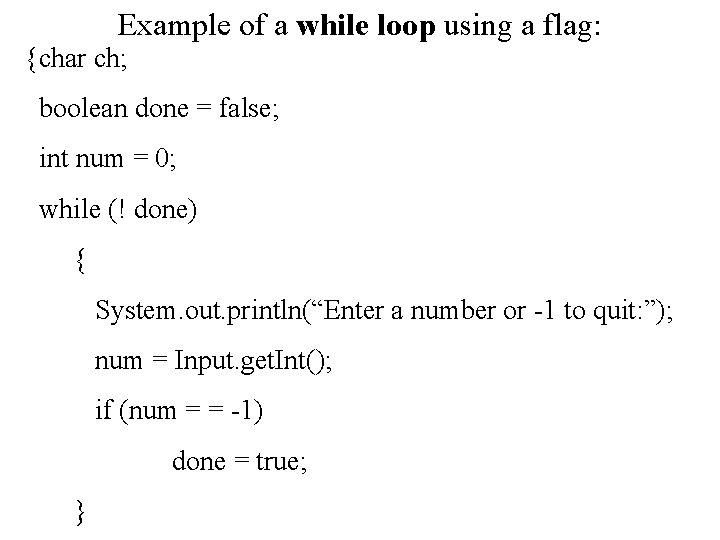
Example of a while loop using a flag: {char ch; boolean done = false; int num = 0; while (! done) { System. out. println(“Enter a number or -1 to quit: ”); num = Input. get. Int(); if (num = = -1) done = true; }
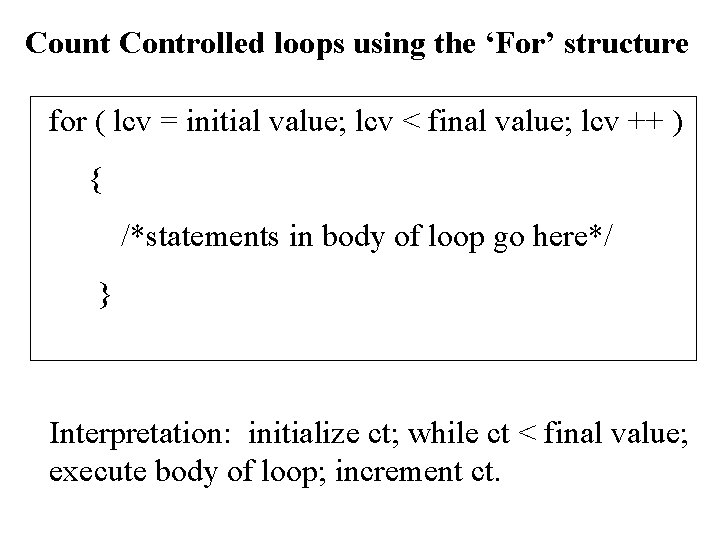
Count Controlled loops using the ‘For’ structure for ( lcv = initial value; lcv < final value; lcv ++ ) { /*statements in body of loop go here*/ } Interpretation: initialize ct; while ct < final value; execute body of loop; increment ct.
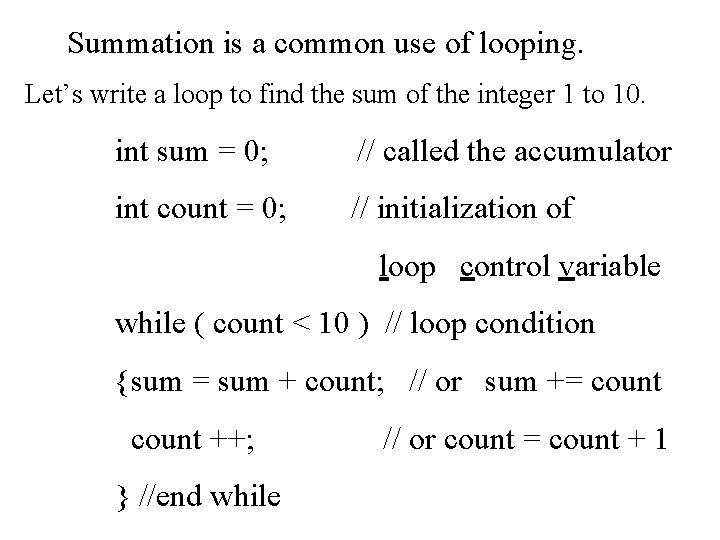
Summation is a common use of looping. Let’s write a loop to find the sum of the integer 1 to 10. int sum = 0; // called the accumulator int count = 0; // initialization of loop control variable while ( count < 10 ) // loop condition {sum = sum + count; // or sum += count ++; } //end while // or count = count + 1
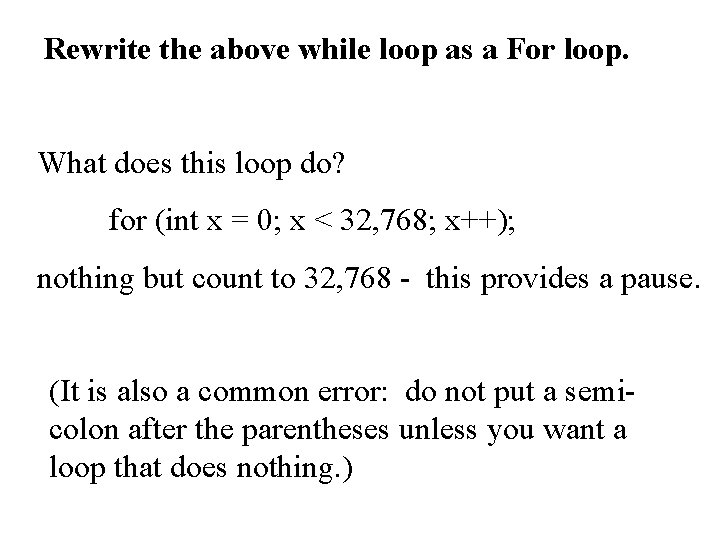
Rewrite the above while loop as a For loop. What does this loop do? for (int x = 0; x < 32, 768; x++); nothing but count to 32, 768 - this provides a pause. (It is also a common error: do not put a semicolon after the parentheses unless you want a loop that does nothing. )
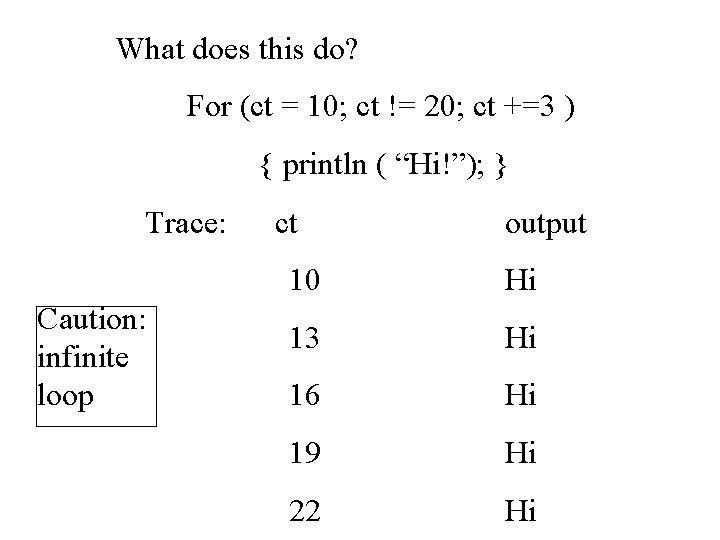
What does this do? For (ct = 10; ct != 20; ct +=3 ) { println ( “Hi!”); } Trace: Caution: infinite loop ct output 10 Hi 13 Hi 16 Hi 19 Hi 22 Hi
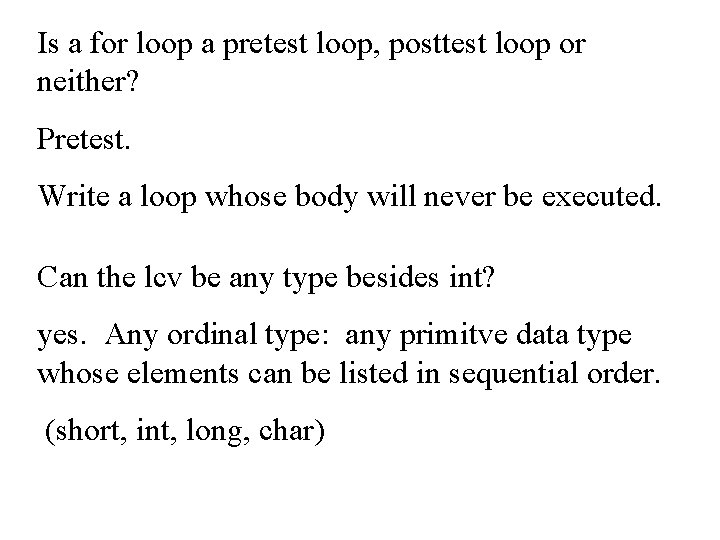
Is a for loop a pretest loop, posttest loop or neither? Pretest. Write a loop whose body will never be executed. Can the lcv be any type besides int? yes. Any ordinal type: any primitve data type whose elements can be listed in sequential order. (short, int, long, char)
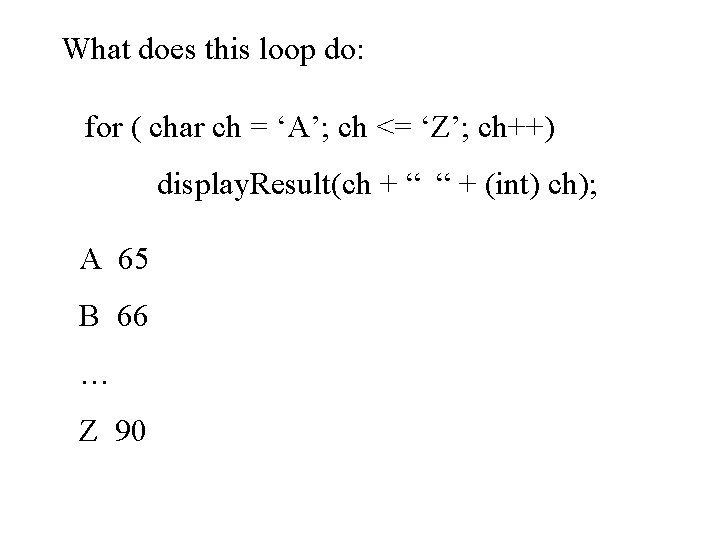
What does this loop do: for ( char ch = ‘A’; ch <= ‘Z’; ch++) display. Result(ch + “ “ + (int) ch); A 65 B 66 … Z 90