Flow Control and Looping 1 Conditionals if statement
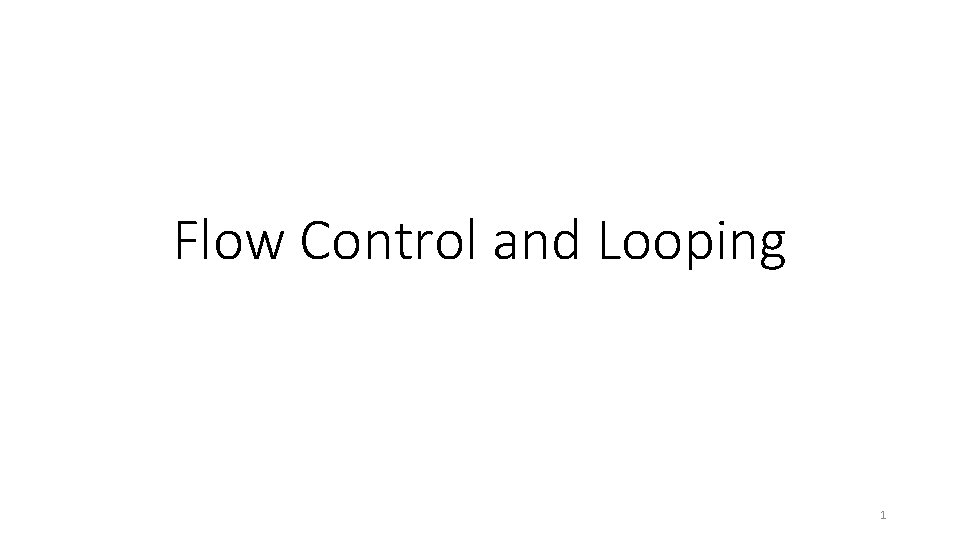
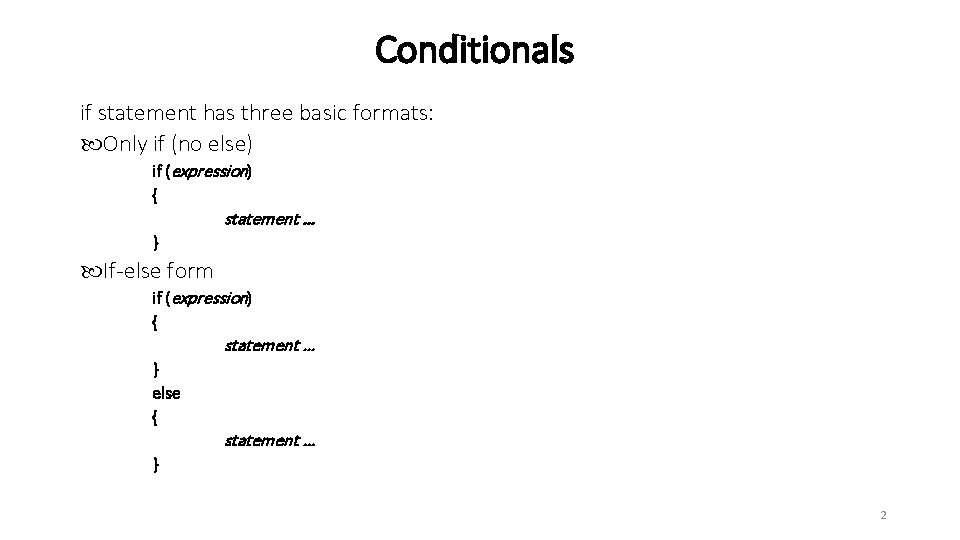
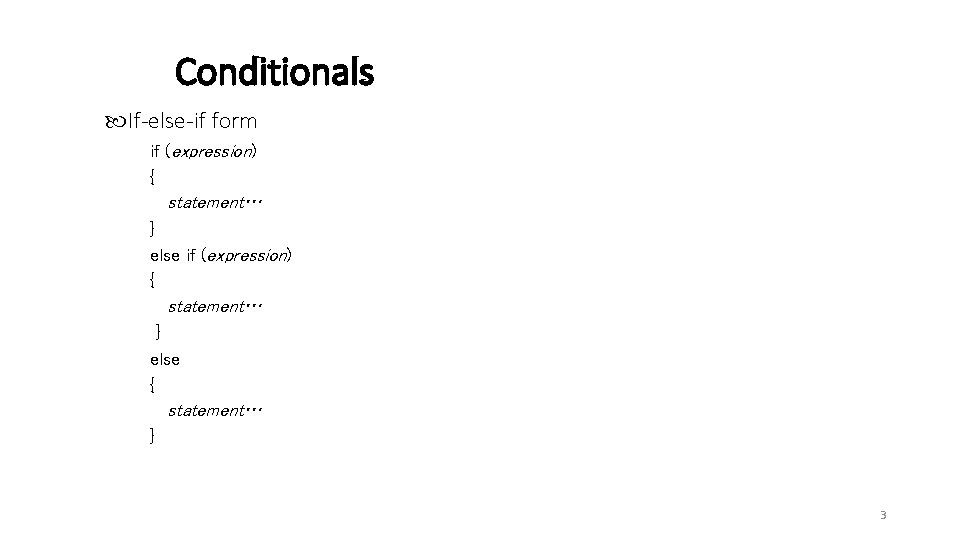
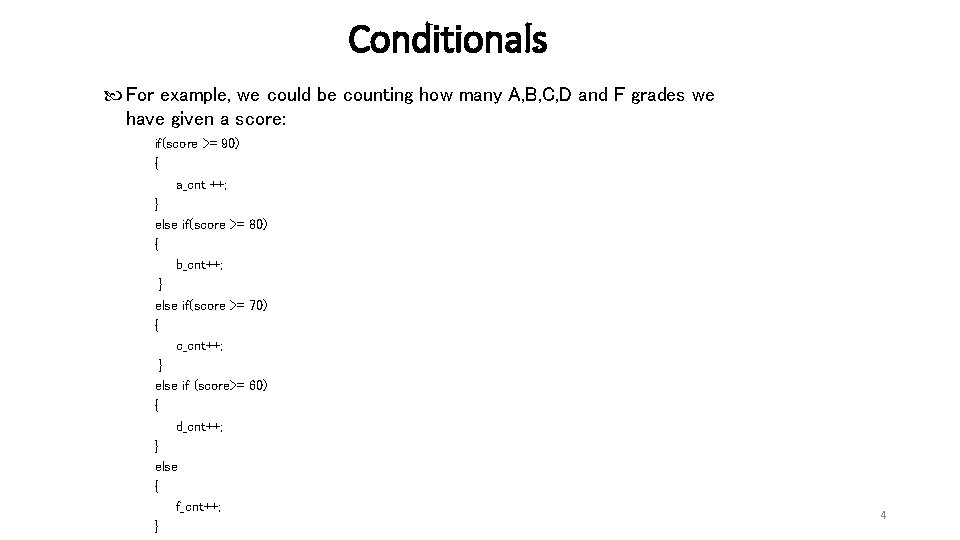
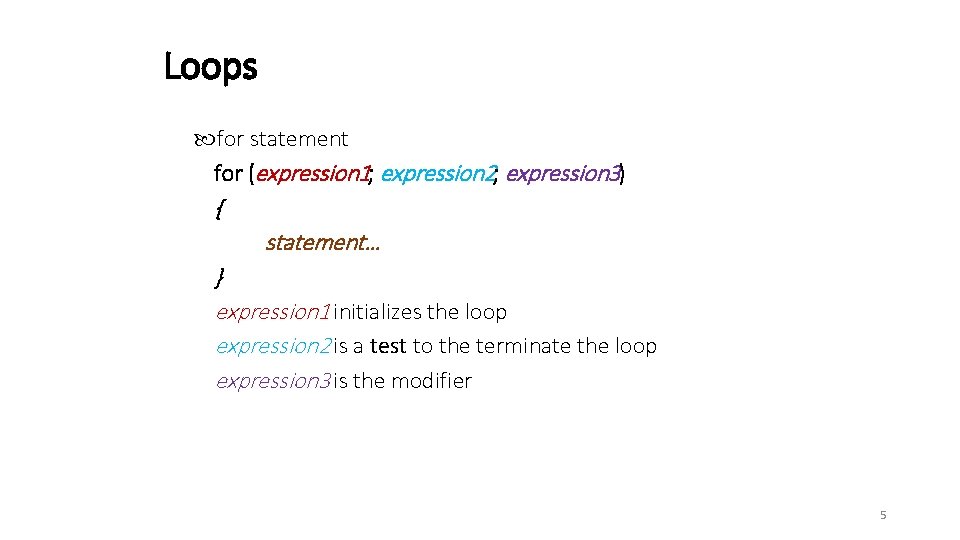
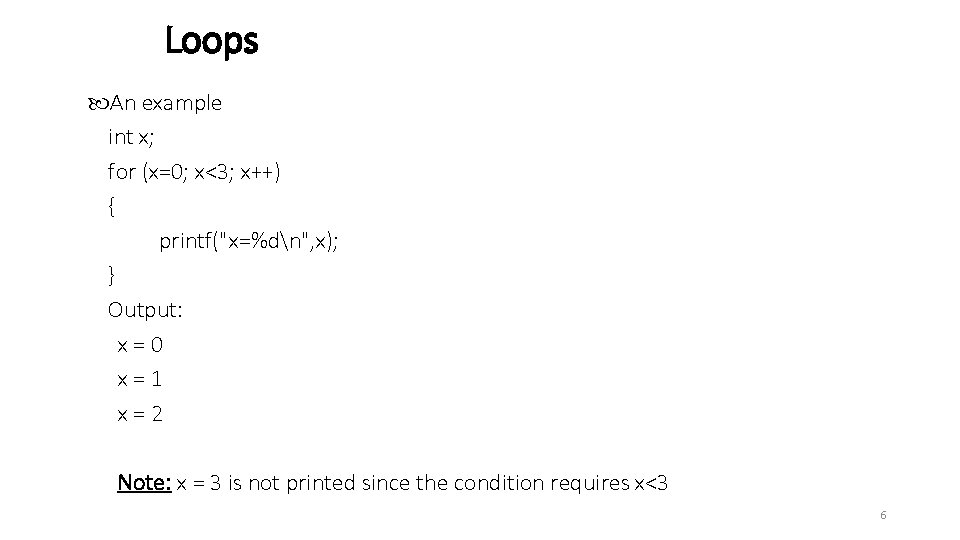
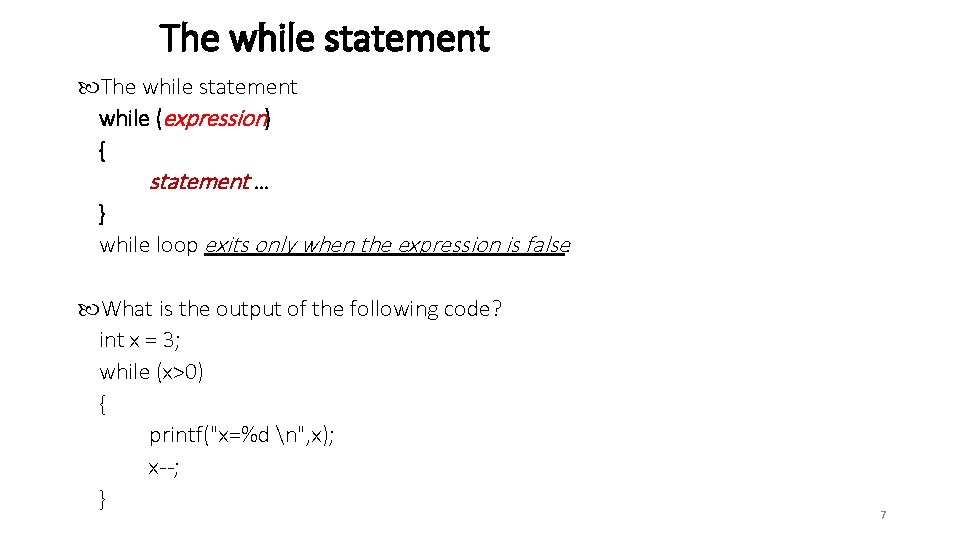
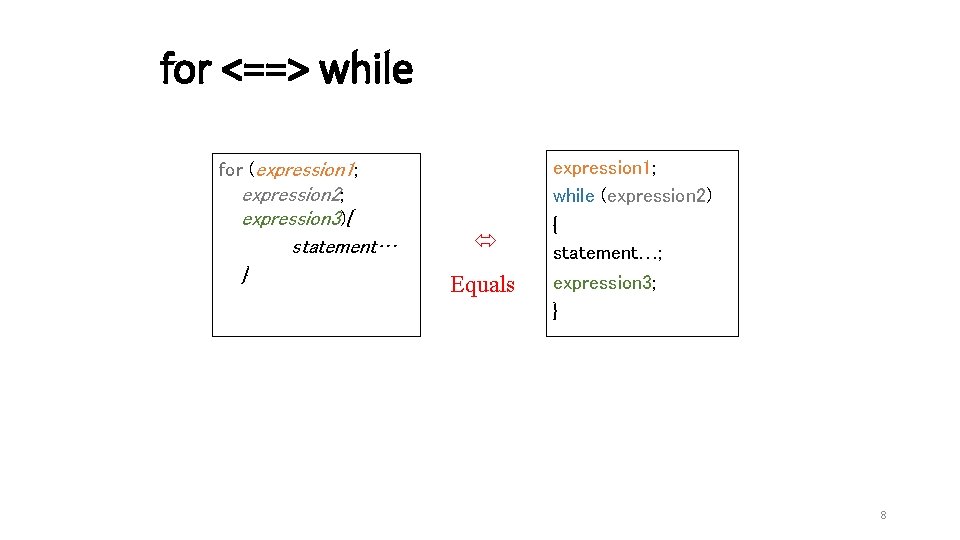
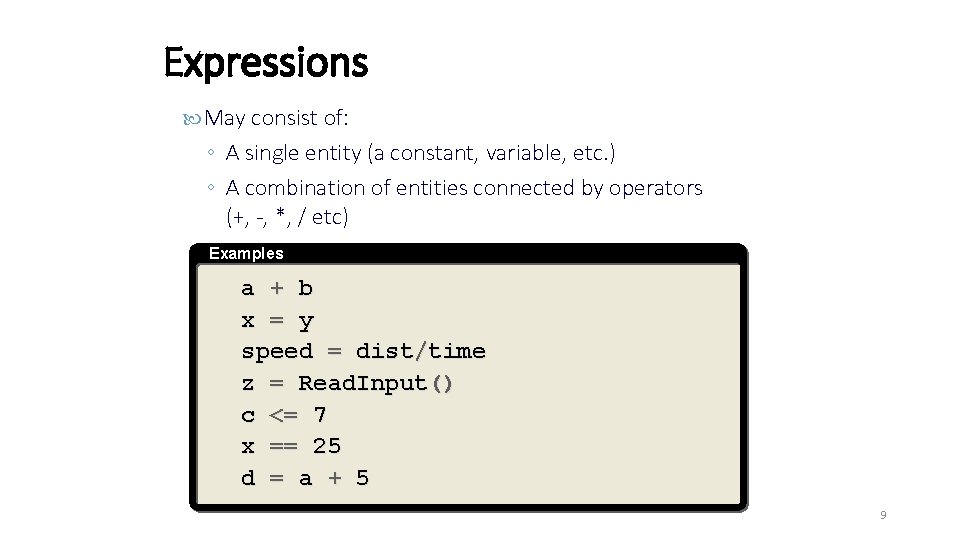
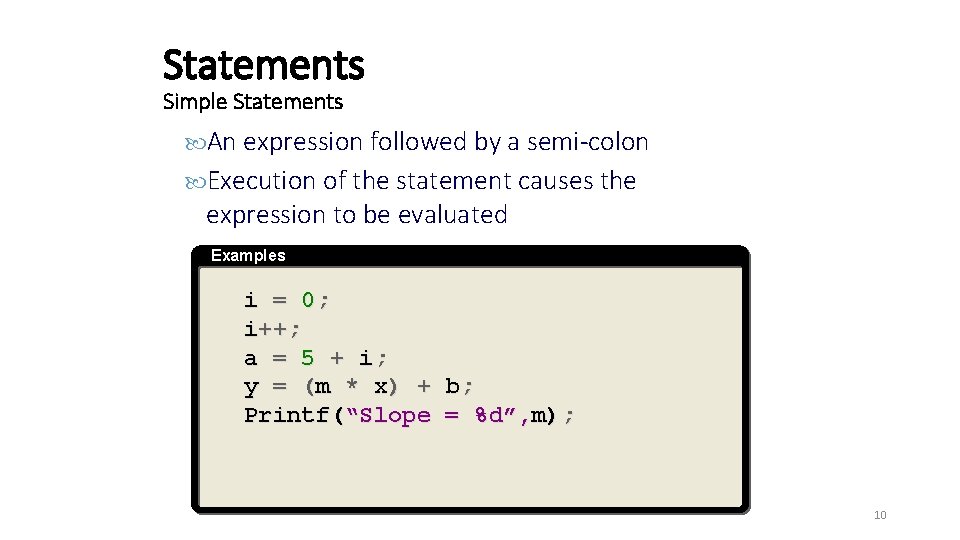
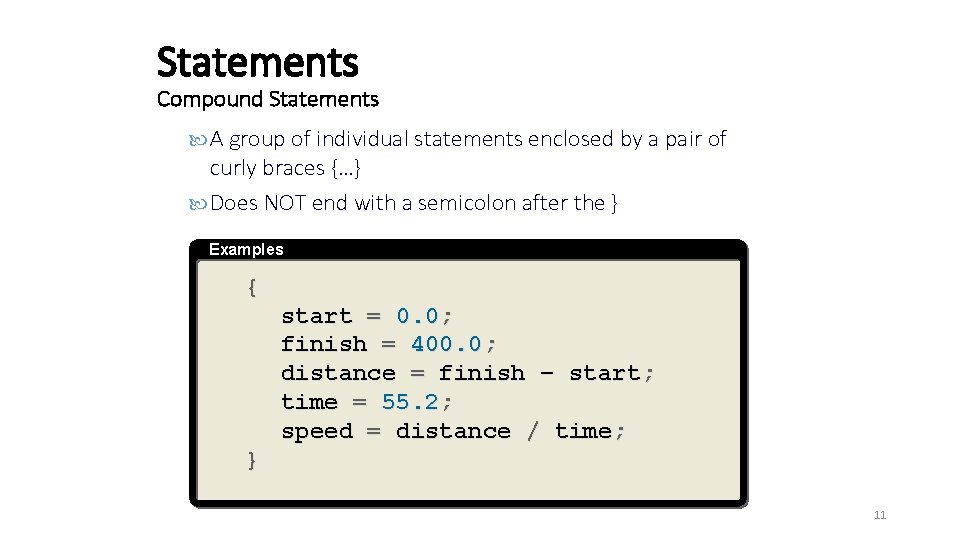
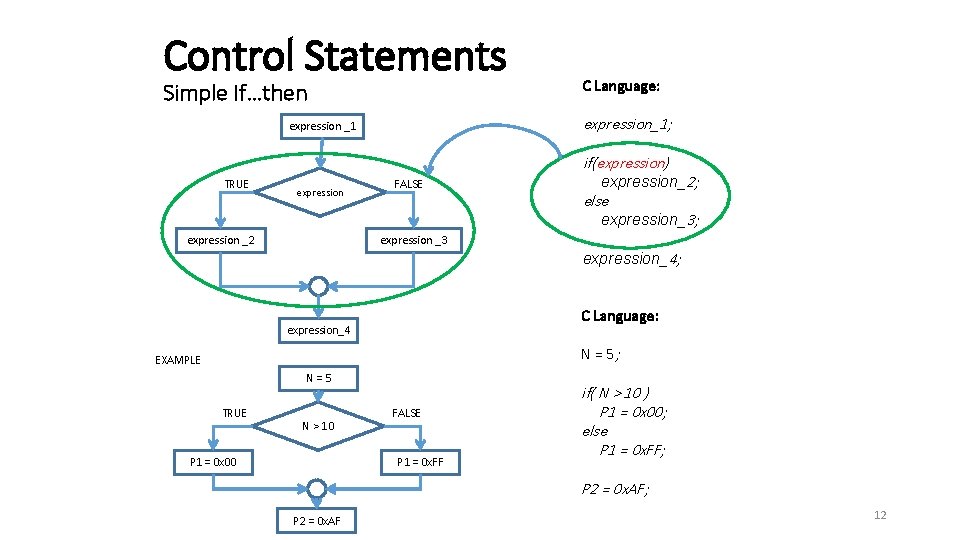
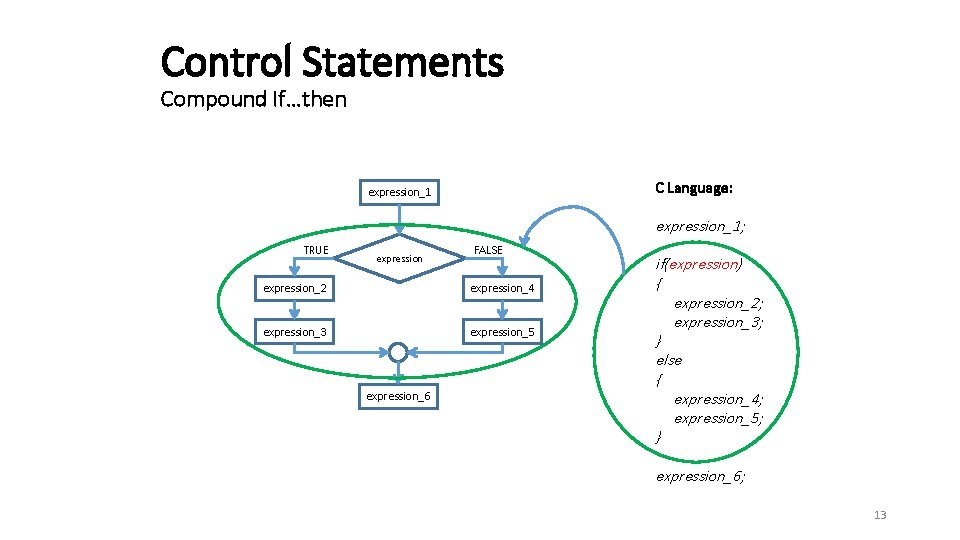
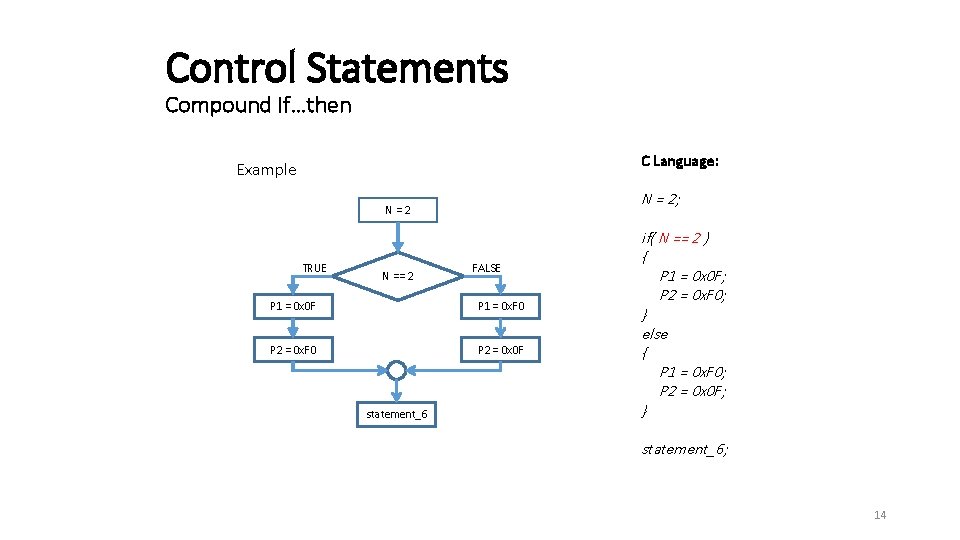
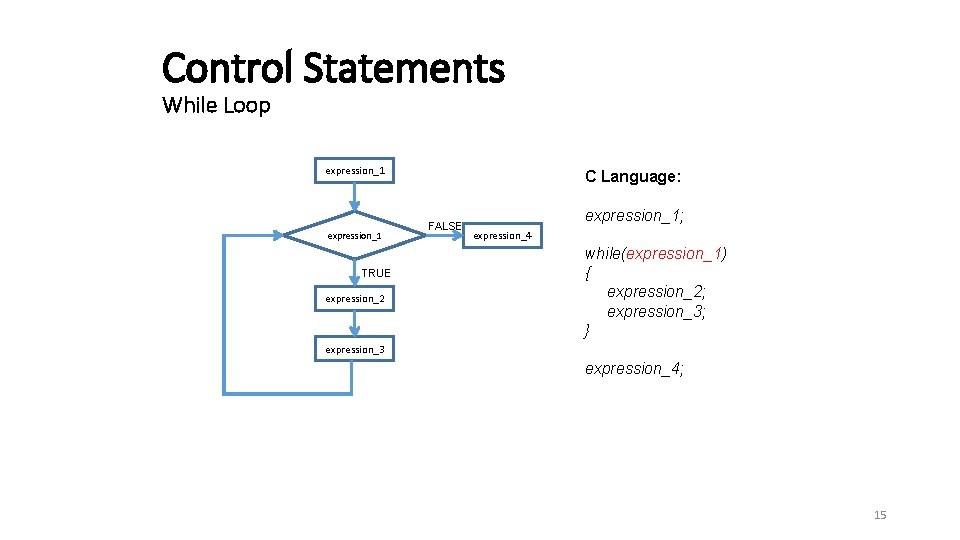
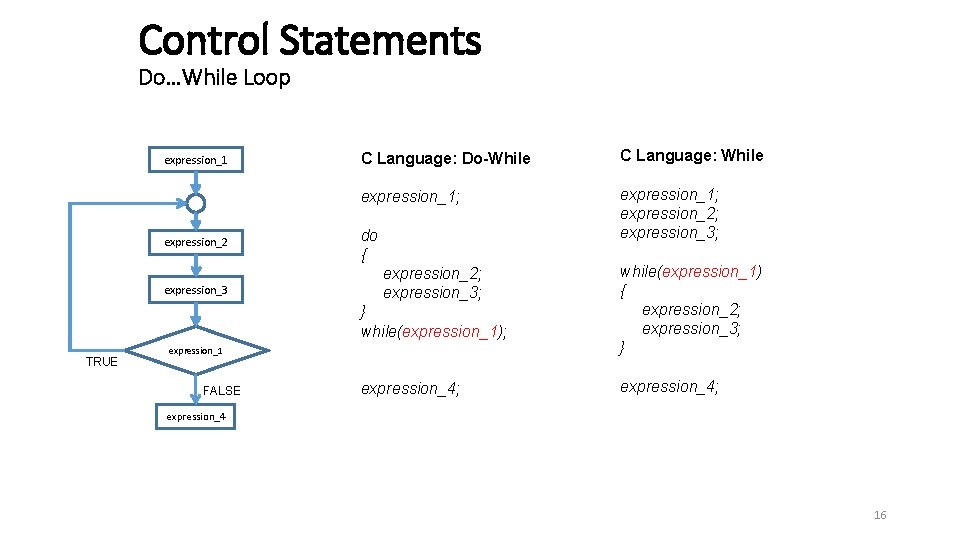
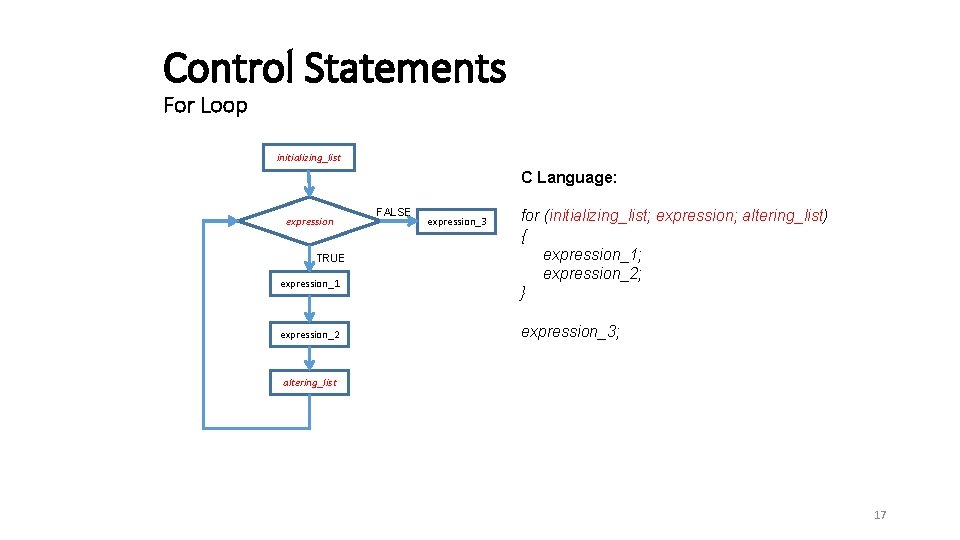
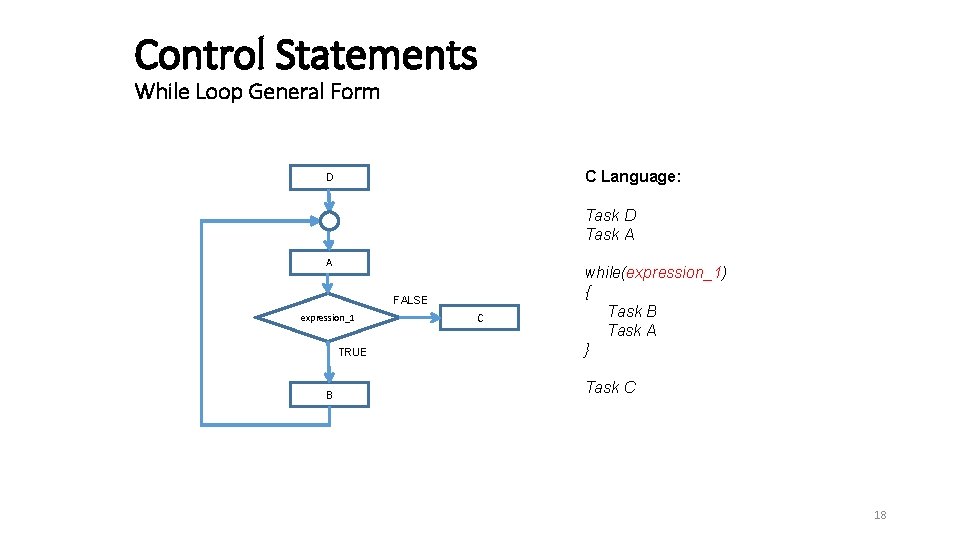
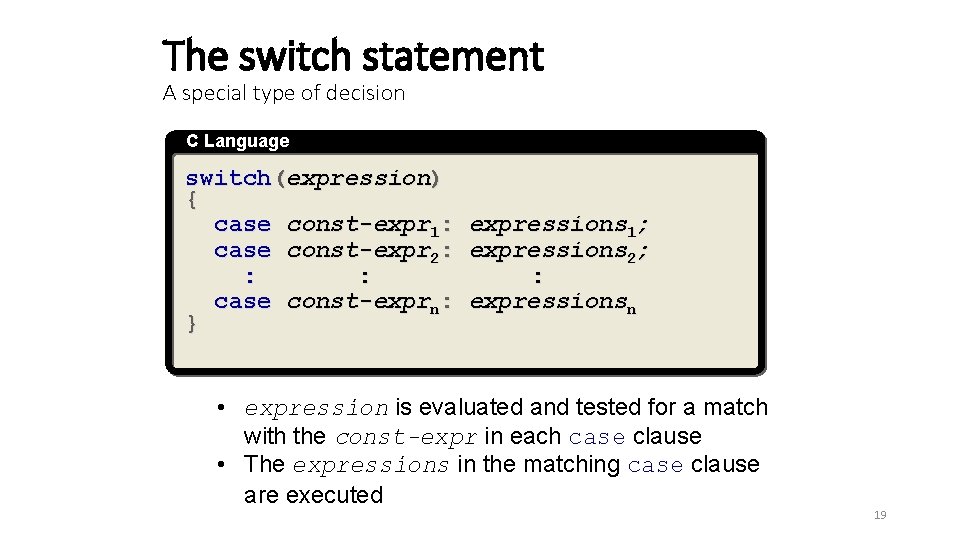
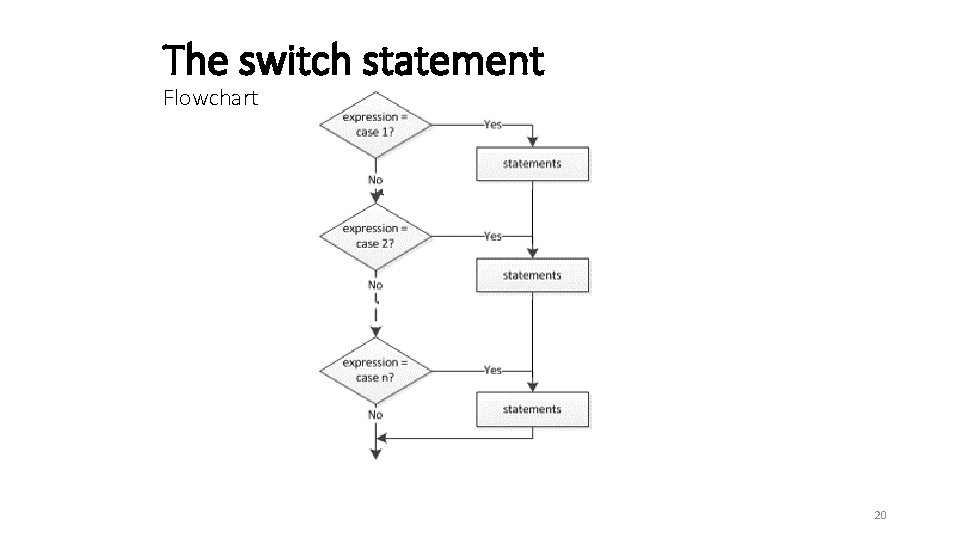
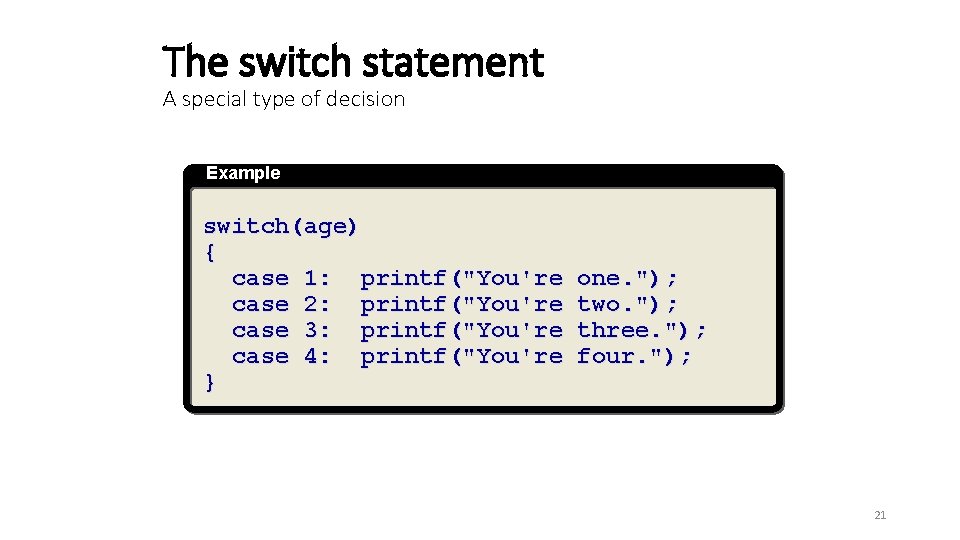
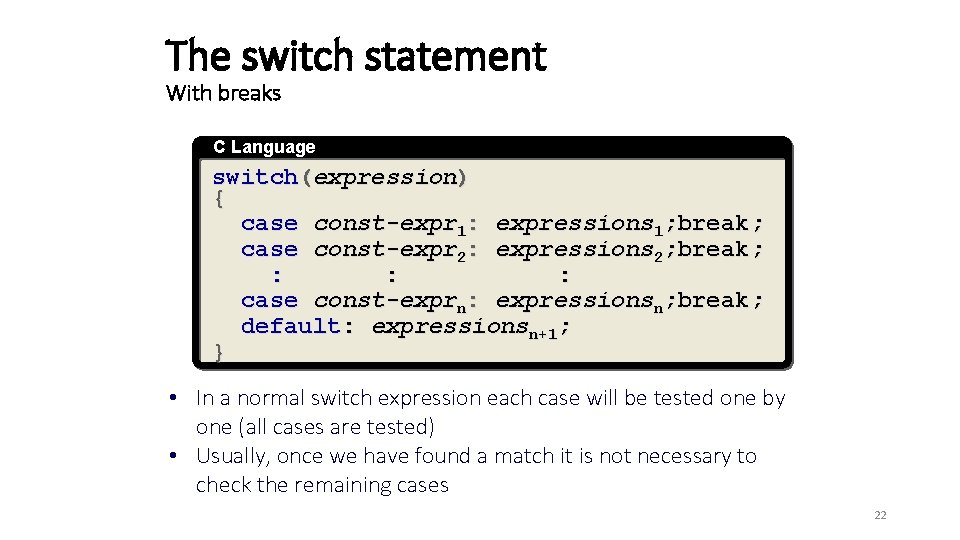
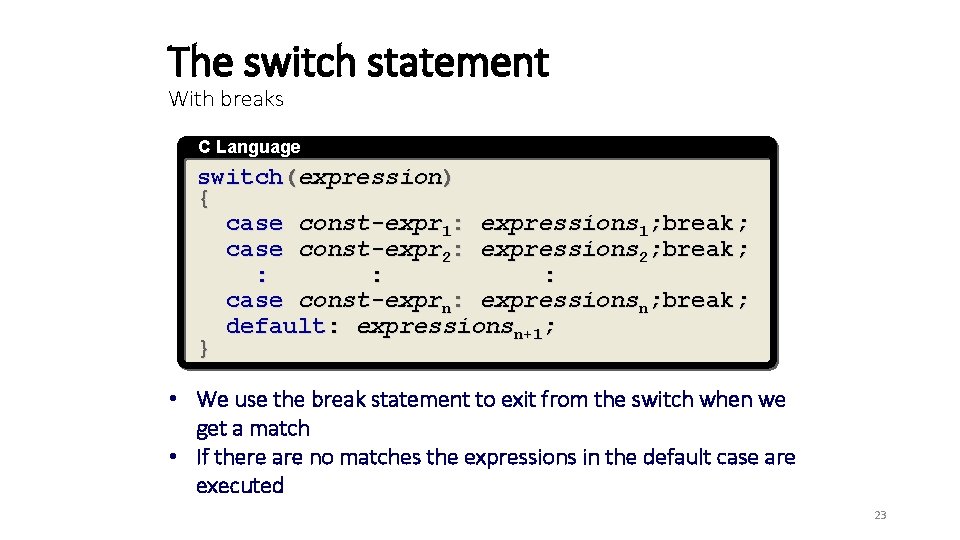
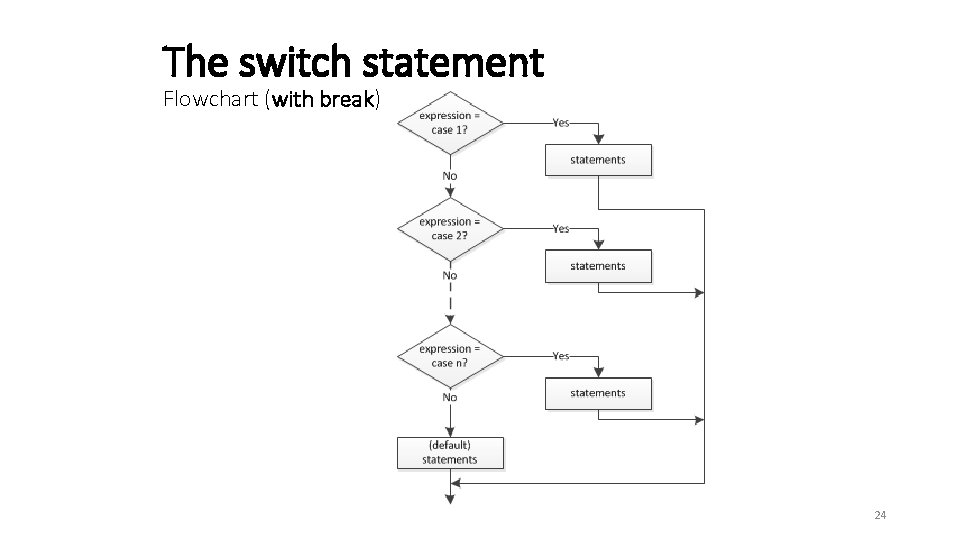
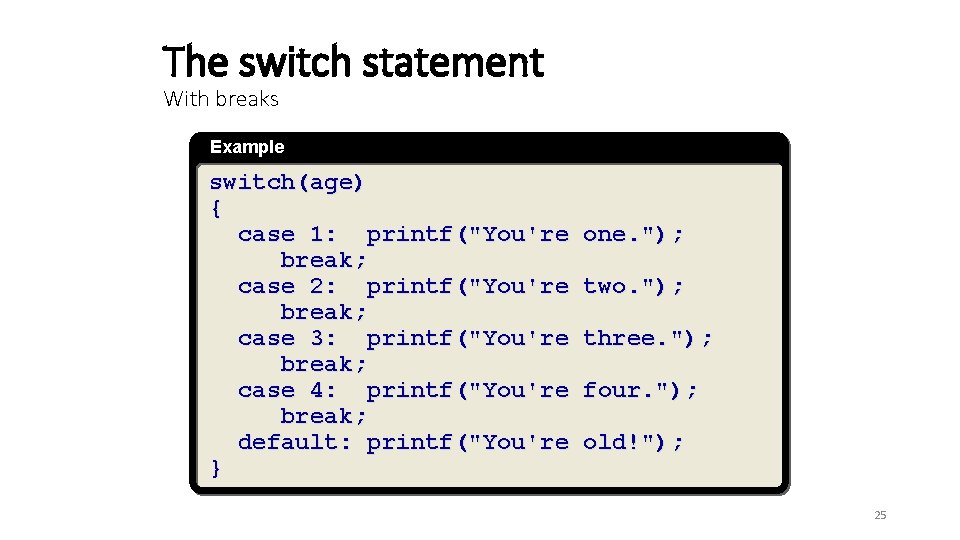
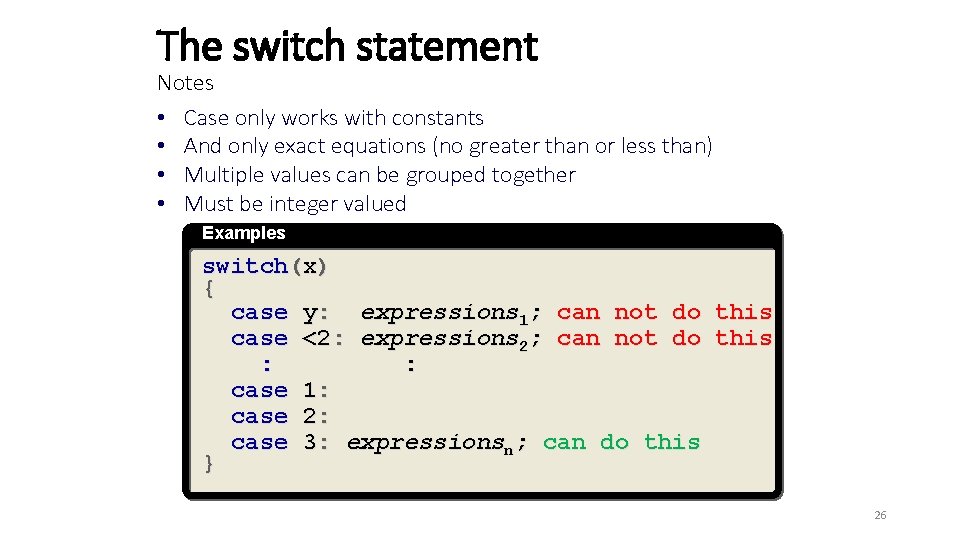
- Slides: 26
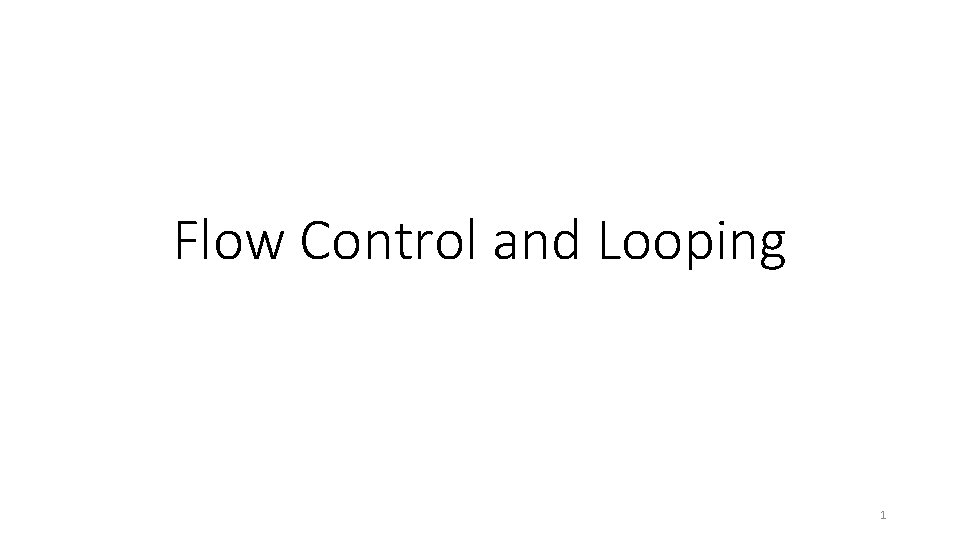
Flow Control and Looping 1
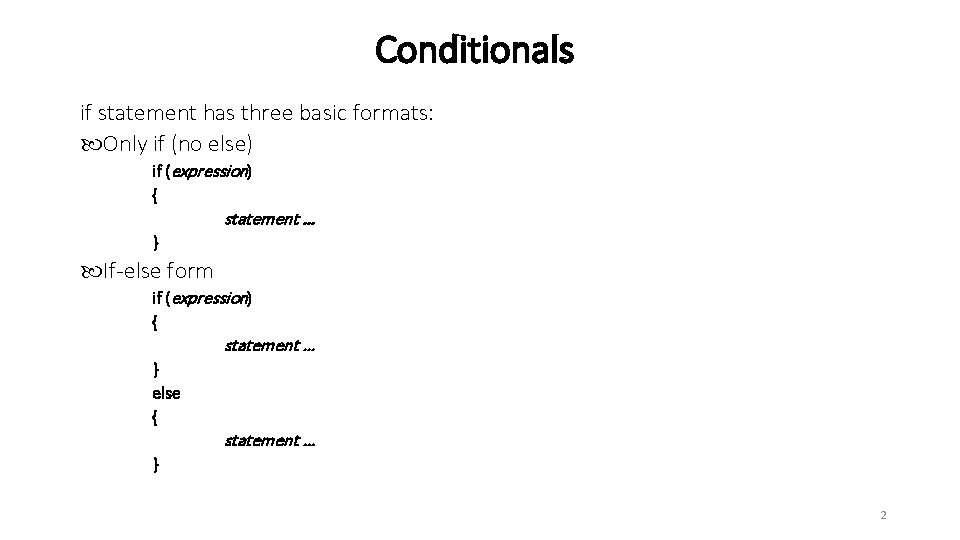
Conditionals if statement has three basic formats: Only if (no else) if (expression) { statement … } If-else form if (expression) { statement … } else { statement … } 2
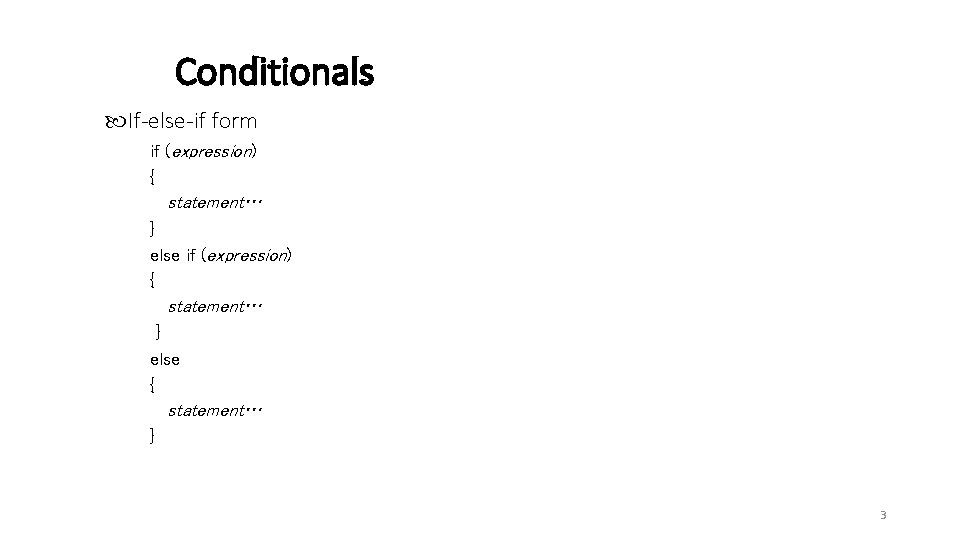
Conditionals If-else-if form if (expression) { statement… } else { statement… } 3
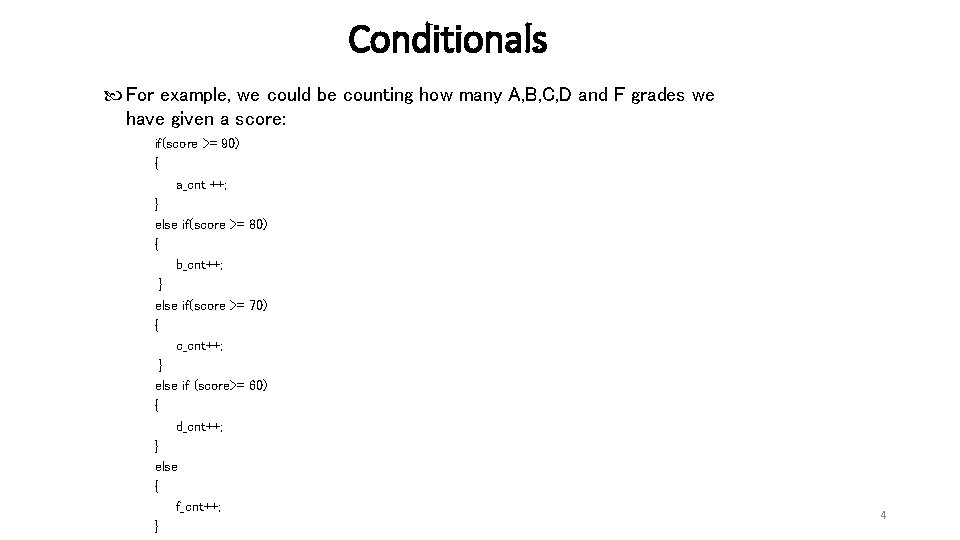
Conditionals For example, we could be counting how many A, B, C, D and F grades we have given a score: if(score >= 90) { a_cnt ++; } else if(score >= 80) { b_cnt++; } else if(score >= 70) { c_cnt++; } else if (score>= 60) { d_cnt++; } else { f_cnt++; } 4
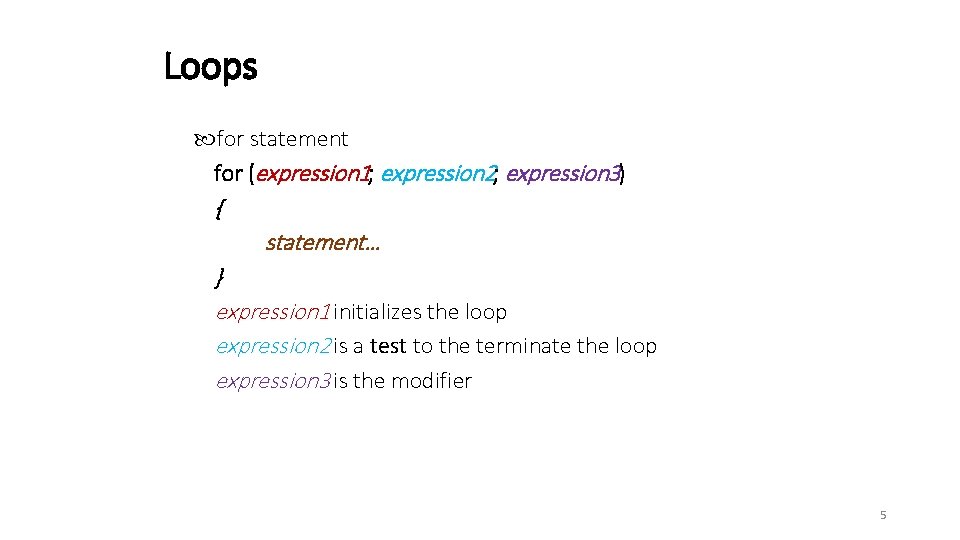
Loops for statement for (expression 1; expression 2; expression 3) { statement… } expression 1 initializes the loop expression 2 is a test to the terminate the loop expression 3 is the modifier 5
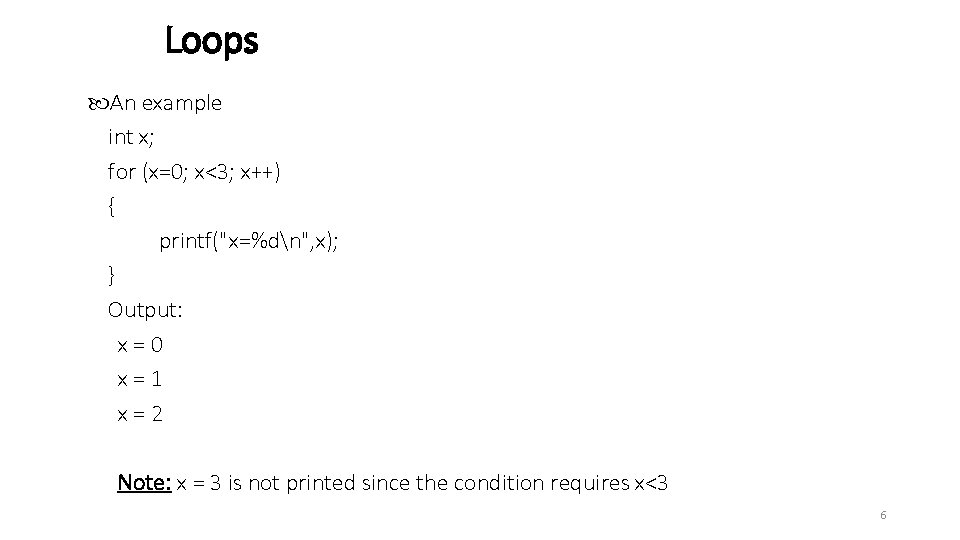
Loops An example int x; for (x=0; x<3; x++) { printf("x=%dn", x); } Output: x=0 x=1 x=2 Note: x = 3 is not printed since the condition requires x<3 6
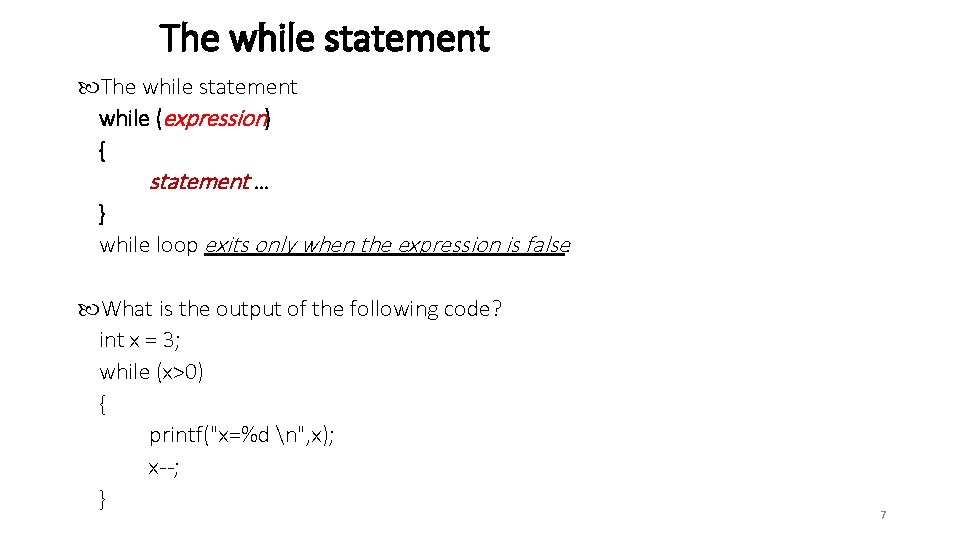
The while statement while (expression) { statement … } while loop exits only when the expression is false. What is the output of the following code? int x = 3; while (x>0) { printf("x=%d n", x); x--; } 7
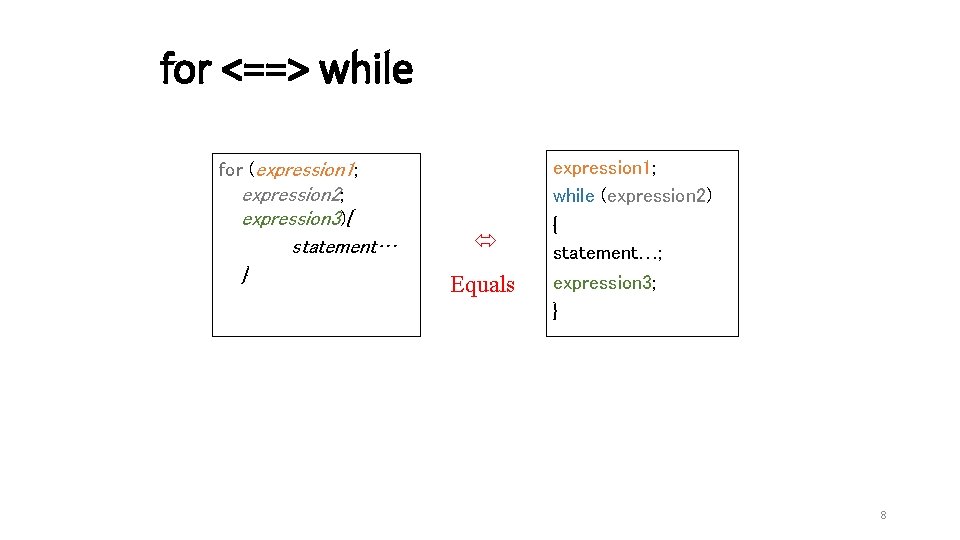
for <==> while for (expression 1; expression 2; expression 3){ statement… } Equals expression 1; while (expression 2) { statement…; expression 3; } 8
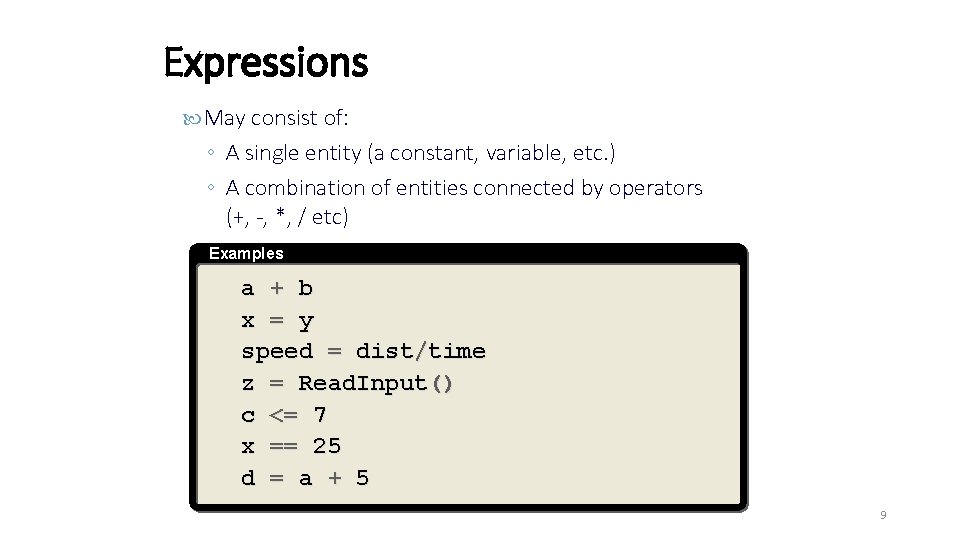
Expressions May consist of: ◦ A single entity (a constant, variable, etc. ) ◦ A combination of entities connected by operators (+, -, *, / etc) Examples a + b x = y speed = dist/time z = Read. Input() c <= 7 x == 25 d = a + 5 9
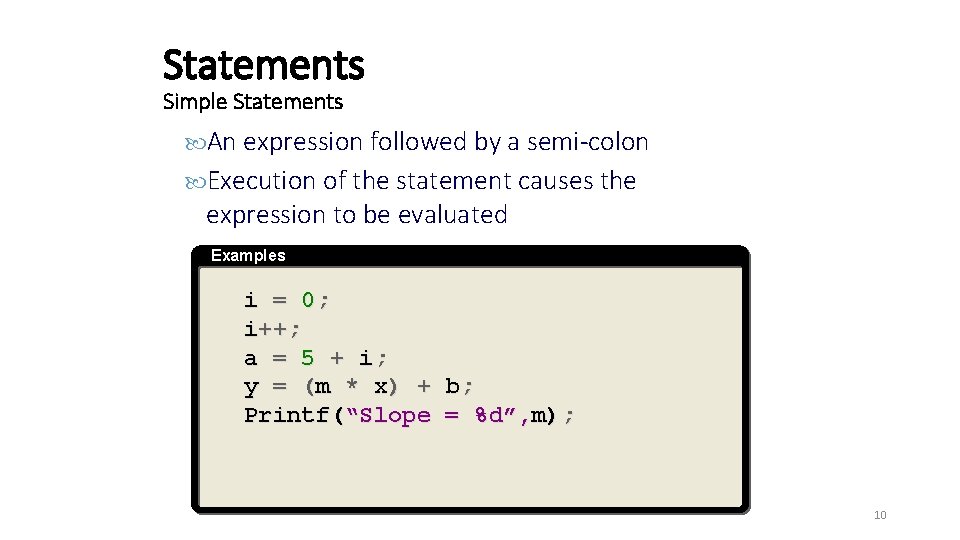
Statements Simple Statements An expression followed by a semi-colon Execution of the statement causes the expression to be evaluated Examples i = 0; i++; a = 5 + i; y = ( m * x ) + b; Printf(“Slope = %d”, m); 10
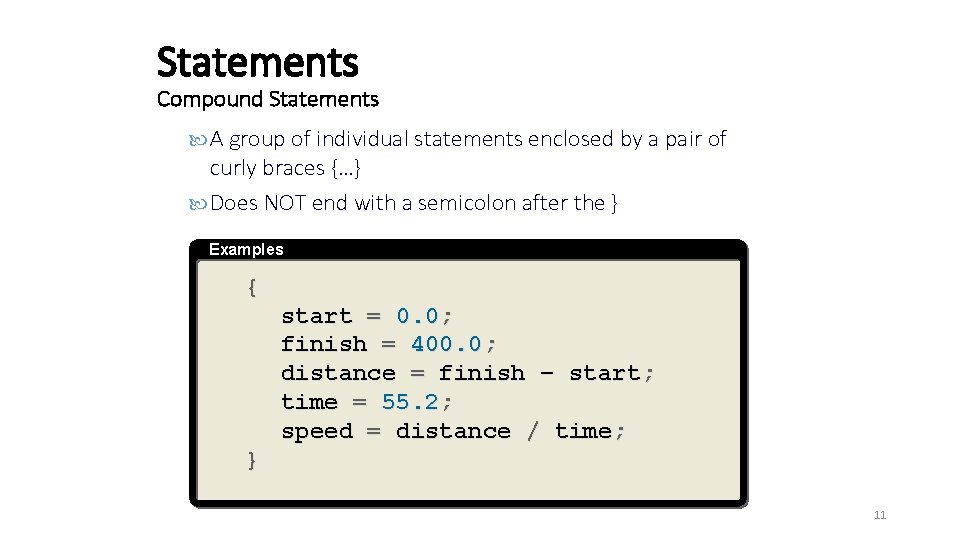
Statements Compound Statements A group of individual statements enclosed by a pair of curly braces {…} Does NOT end with a semicolon after the } Examples { start = 0. 0; finish = 400. 0; distance = finish – start; time = 55. 2; speed = distance / time; } 11
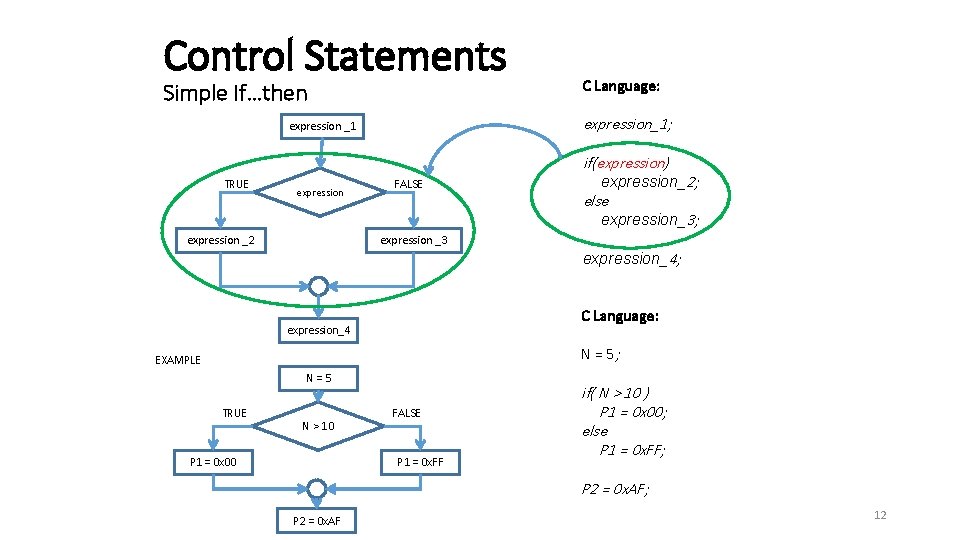
Control Statements Simple If…then C Language: expression_1; expression _1 if(expression) TRUE expression FALSE expression_2; else expression_3; expression _2 expression _3 expression_4; C Language: expression_4 N = 5; EXAMPLE N=5 TRUE N > 10 P 1 = 0 x 00 FALSE P 1 = 0 x. FF if( N > 10 ) P 1 = 0 x 00; else P 1 = 0 x. FF; P 2 = 0 x. AF; P 2 = 0 x. AF 12
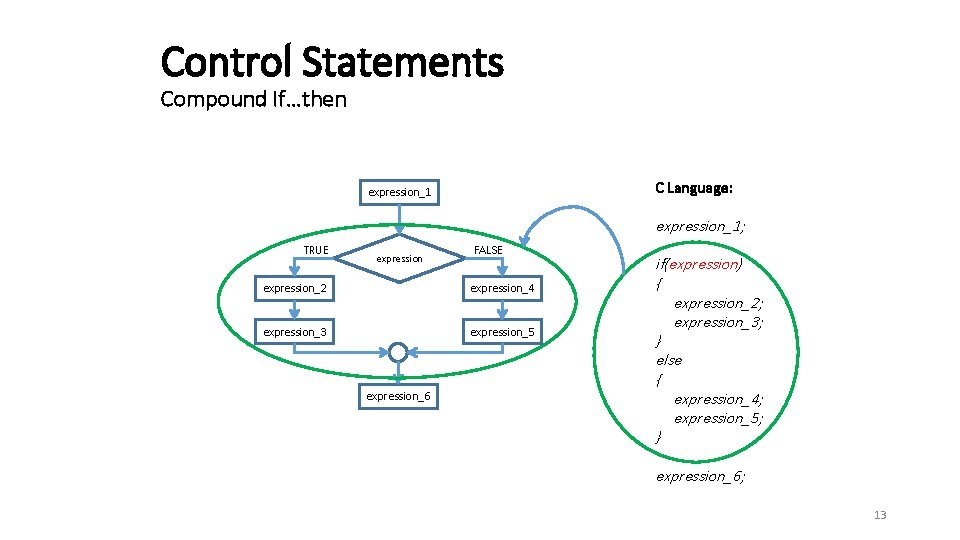
Control Statements Compound If…then C Language: expression_1; TRUE expression FALSE expression_2 expression_4 expression_3 expression_5 expression_6 if(expression) { expression_2; expression_3; } else { expression_4; expression_5; } expression_6; 13
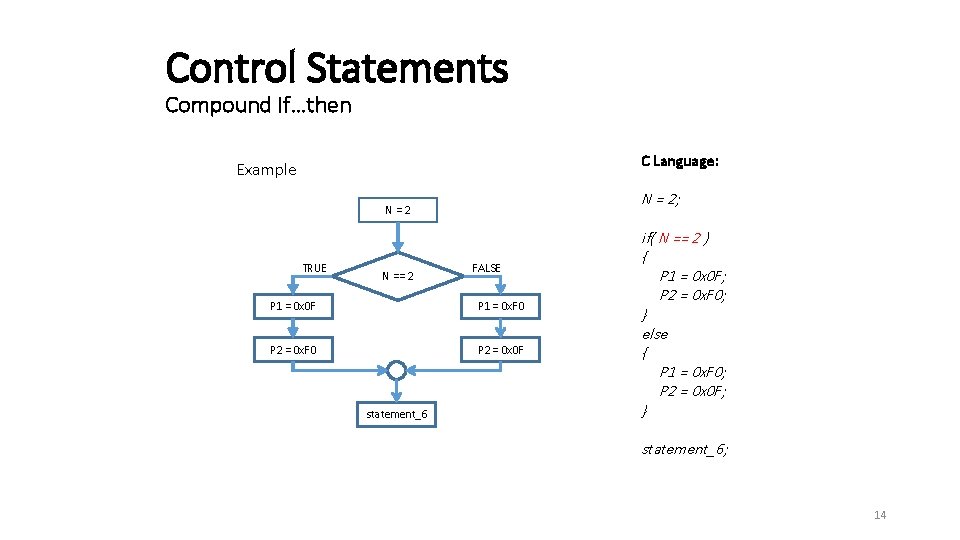
Control Statements Compound If…then C Language: Example N = 2; N=2 TRUE N == 2 FALSE P 1 = 0 x 0 F P 1 = 0 x. F 0 P 2 = 0 x 0 F statement_6 if( N == 2 ) { P 1 = 0 x 0 F; P 2 = 0 x. F 0; } else { P 1 = 0 x. F 0; P 2 = 0 x 0 F; } statement_6; 14
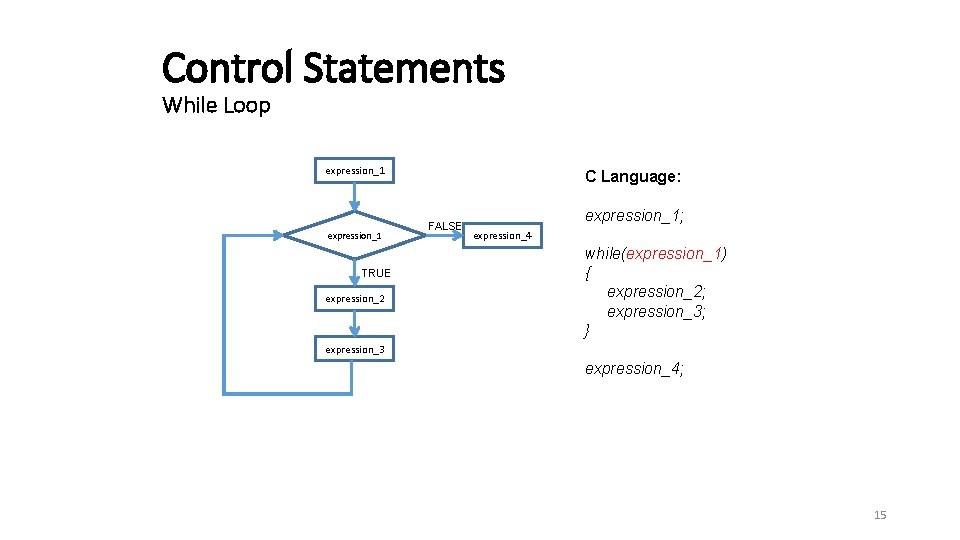
Control Statements While Loop expression_1 TRUE expression_2 C Language: FALSE expression_1; expression_4 while(expression_1) { expression_2; expression_3; } expression_3 expression_4; 15
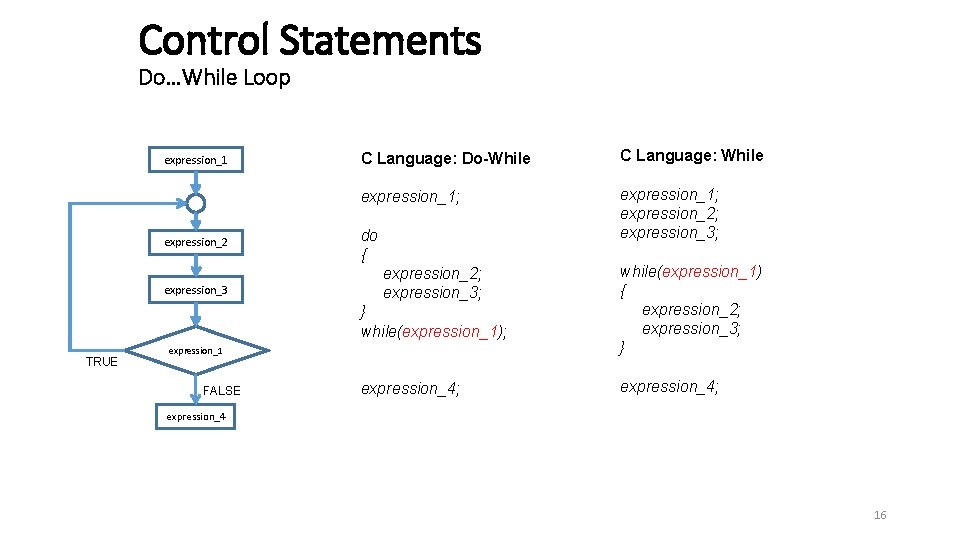
Control Statements Do…While Loop expression_1 expression_2 expression_3 C Language: Do-While C Language: While expression_1; expression_2; expression_3; do { expression_2; expression_3; } while(expression_1); TRUE expression_1 FALSE expression_4; while(expression_1) { expression_2; expression_3; } expression_4; expression_4 16
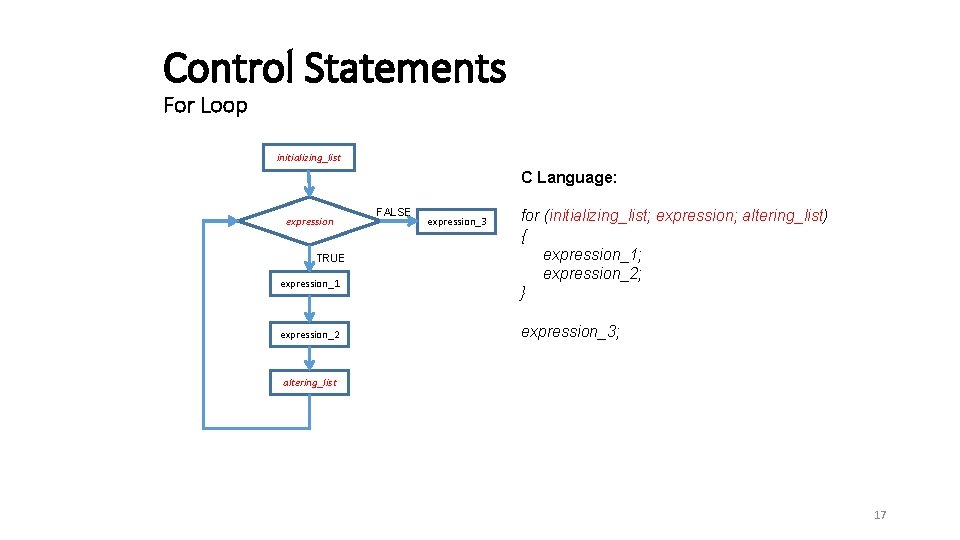
Control Statements For Loop initializing_list C Language: expression TRUE expression_1 expression_2 FALSE expression_3 for (initializing_list; expression; altering_list) { expression_1; expression_2; } expression_3; altering_list 17
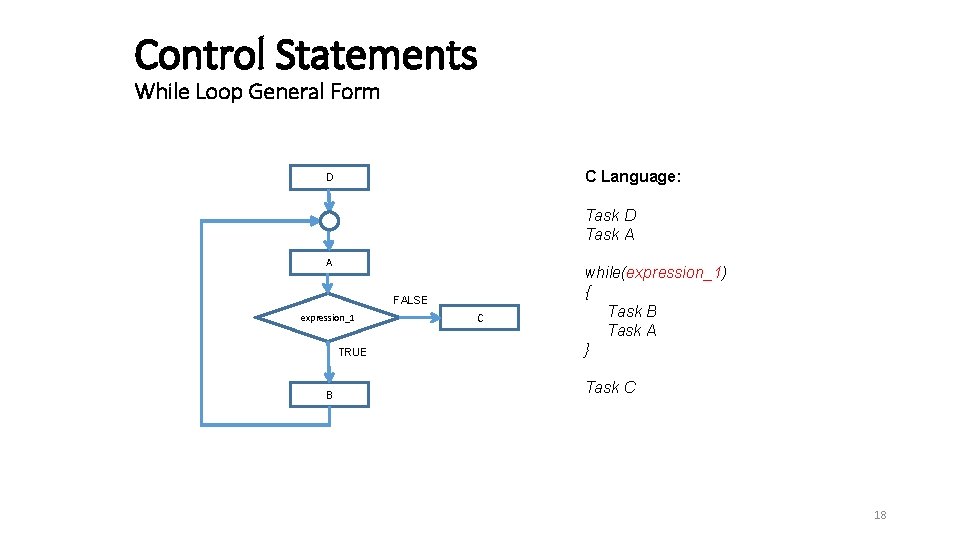
Control Statements While Loop General Form C Language: D Task A A FALSE expression_1 TRUE B C while(expression_1) { Task B Task A } Task C 18
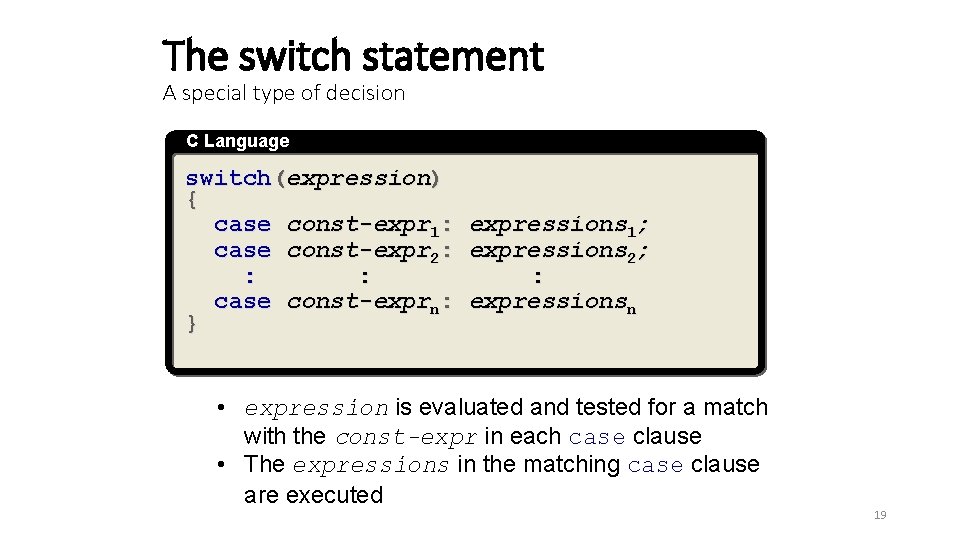
The switch statement A special type of decision C Language switch(expression) { case const-expr 1: expressions 1; case const-expr 2: expressions 2; : : : case const-exprn: expressionsn } • expression is evaluated and tested for a match with the const-expr in each case clause • The expressions in the matching case clause are executed 19
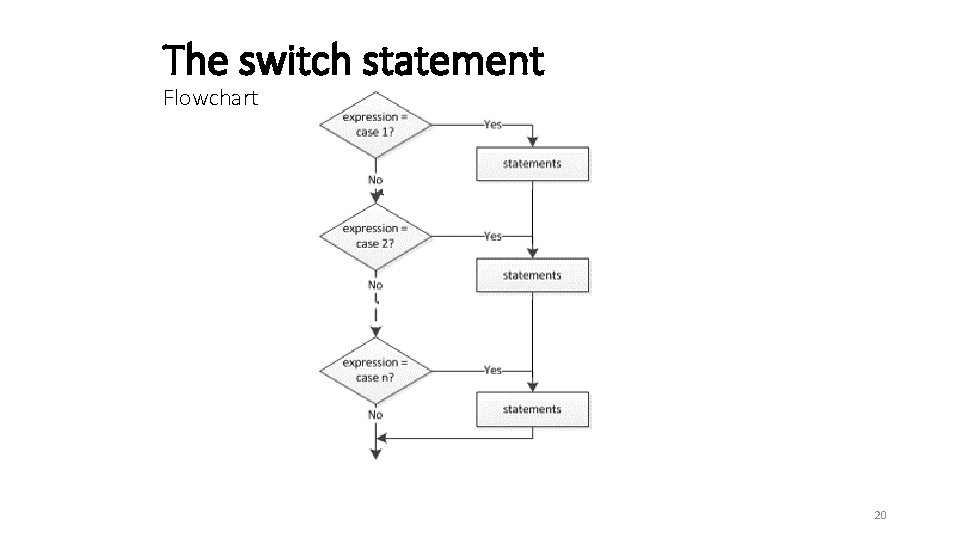
The switch statement Flowchart 20
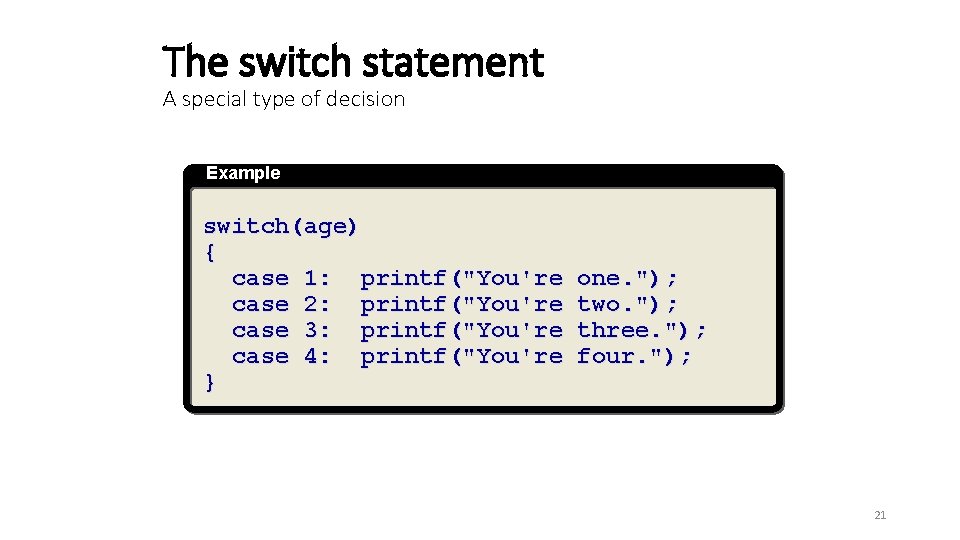
The switch statement A special type of decision Example switch(age) { case 1: printf("You're case 2: printf("You're case 3: printf("You're case 4: printf("You're } one. "); two. "); three. "); four. "); 21
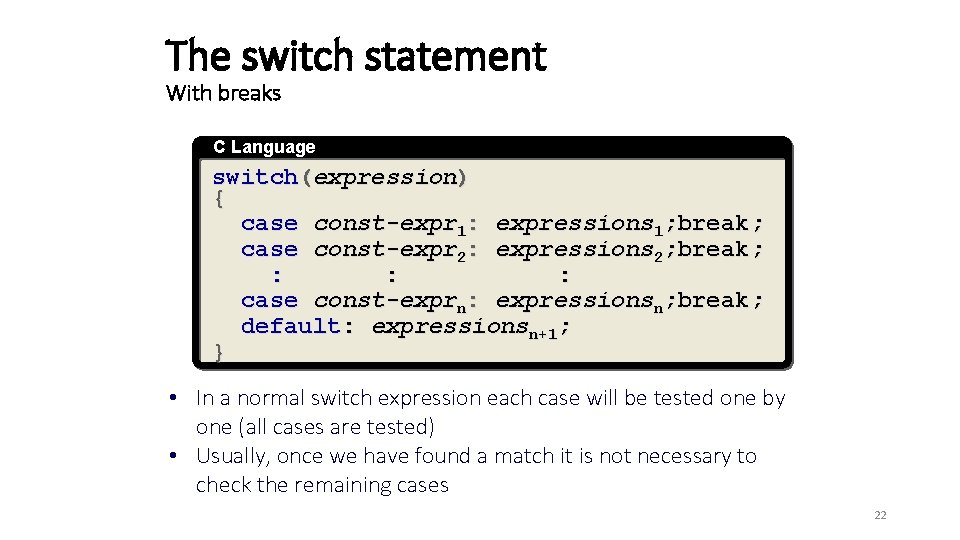
The switch statement With breaks C Language switch(expression) { case const-expr 1: expressions 1; break; case const-expr 2: expressions 2; break; : : : case const-exprn: expressionsn; break; default: expressionsn+1; } • In a normal switch expression each case will be tested one by one (all cases are tested) • Usually, once we have found a match it is not necessary to check the remaining cases 22
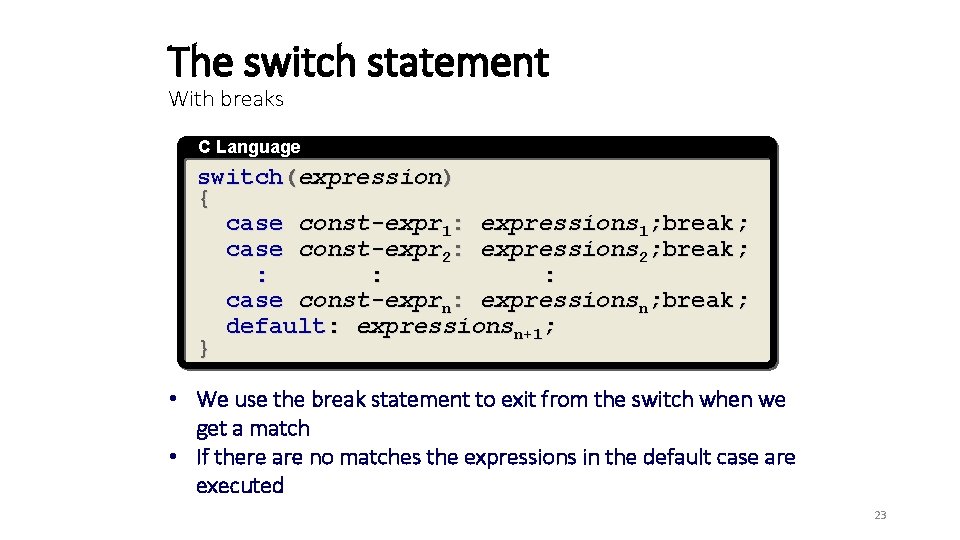
The switch statement With breaks C Language switch(expression) { case const-expr 1: expressions 1; break; case const-expr 2: expressions 2; break; : : : case const-exprn: expressionsn; break; default: expressionsn+1; } • We use the break statement to exit from the switch when we get a match • If there are no matches the expressions in the default case are executed 23
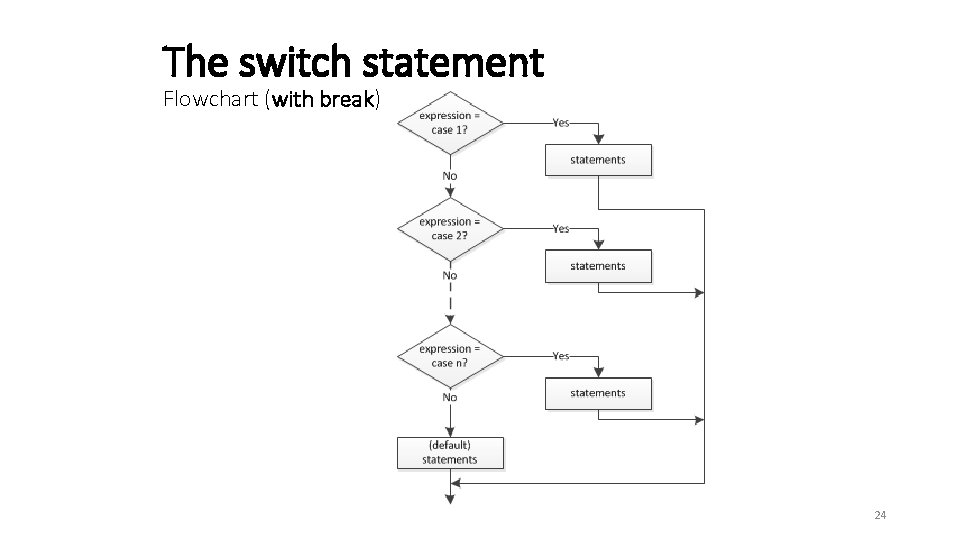
The switch statement Flowchart (with break) 24
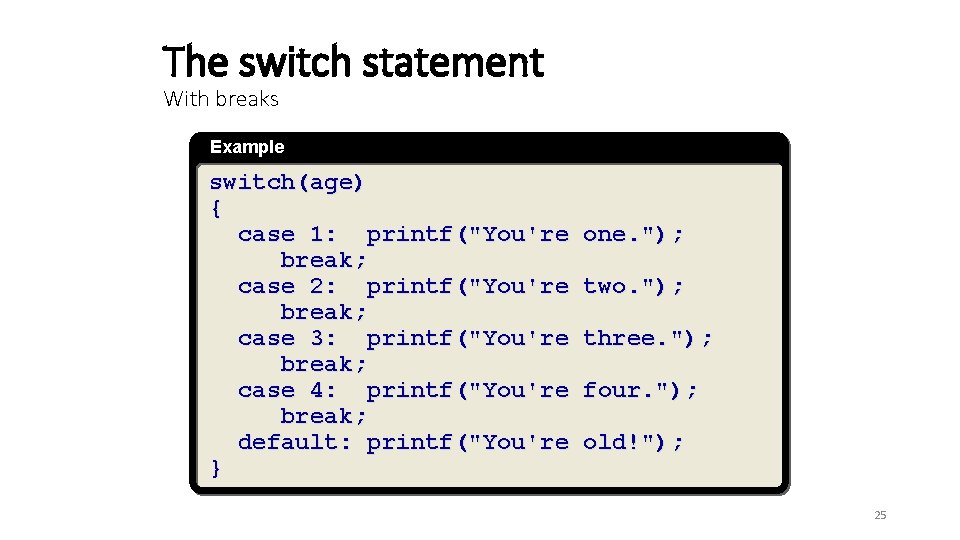
The switch statement With breaks Example switch(age) { case 1: printf("You're break; case 2: printf("You're break; case 3: printf("You're break; case 4: printf("You're break; default: printf("You're } one. "); two. "); three. "); four. "); old!"); 25
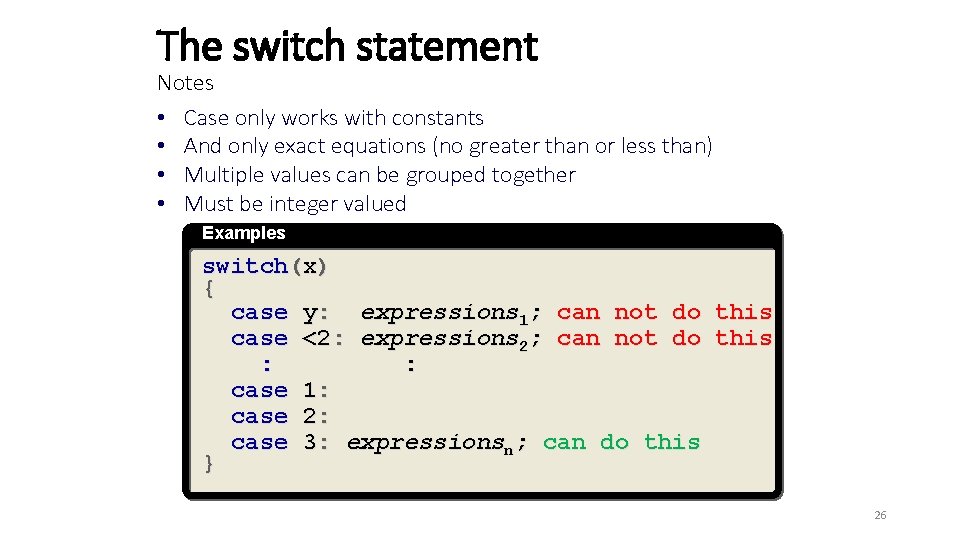
The switch statement Notes • Case only works with constants • And only exact equations (no greater than or less than) • Multiple values can be grouped together • Must be integer valued Examples switch(x) { case y: expressions 1; can not do this case <2: expressions 2; can not do this : : case 1: case 2: case 3: expressionsn; can do this } 26