Intro to Programming with Python Practical session 4
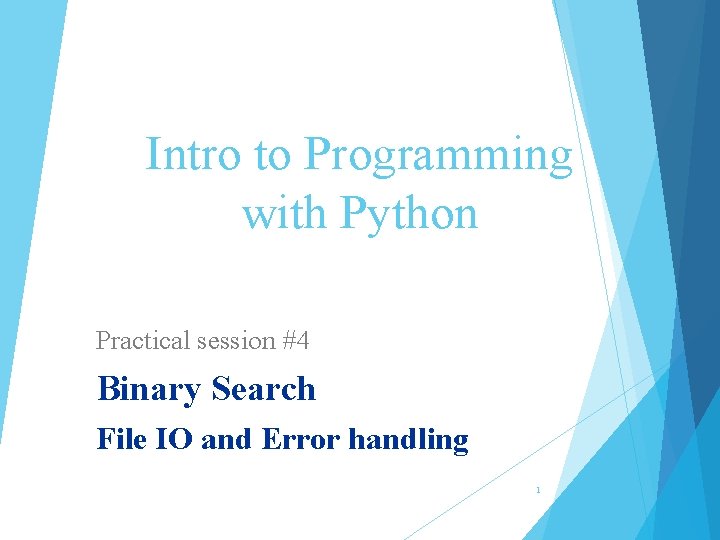
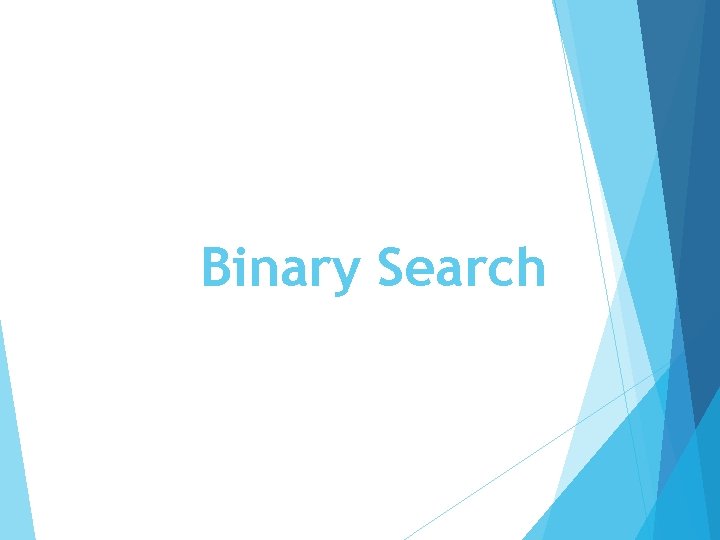
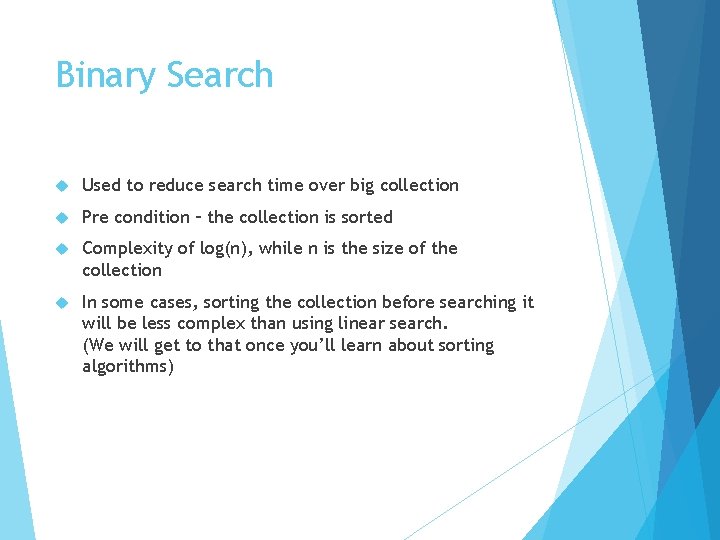
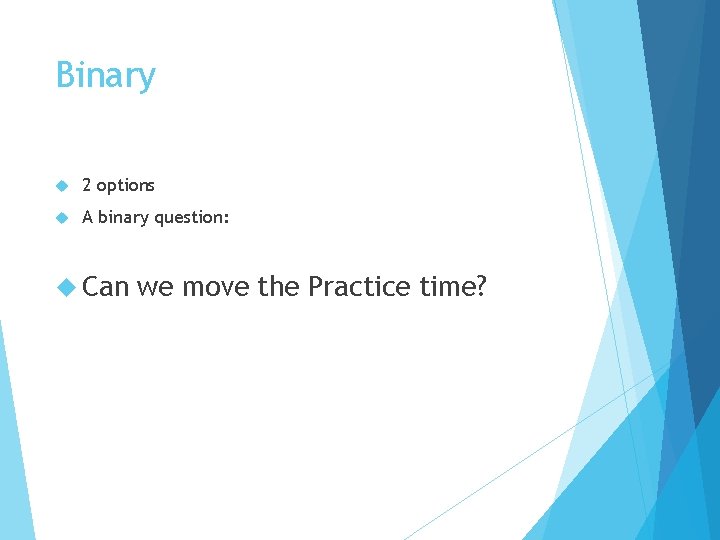
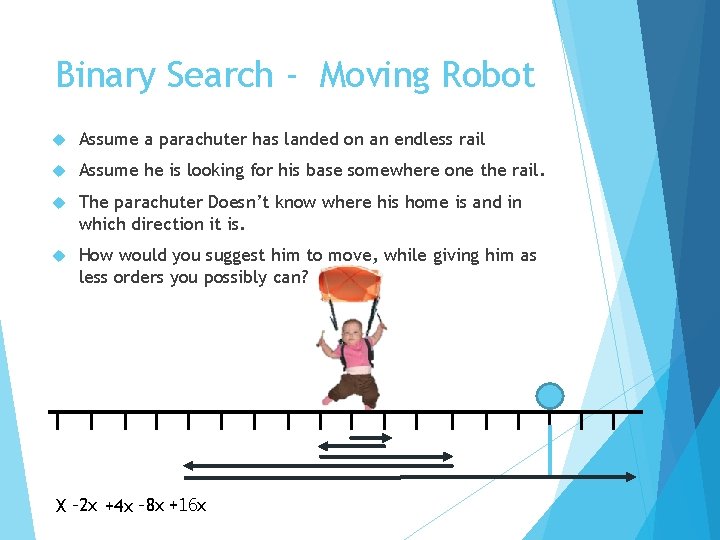
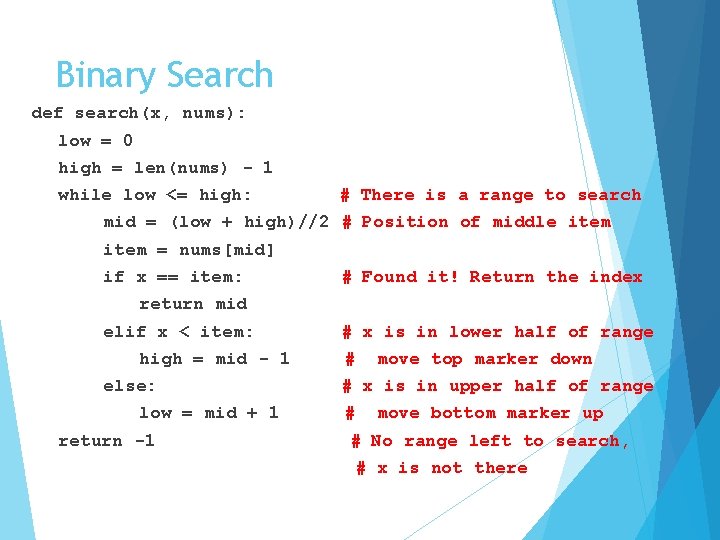
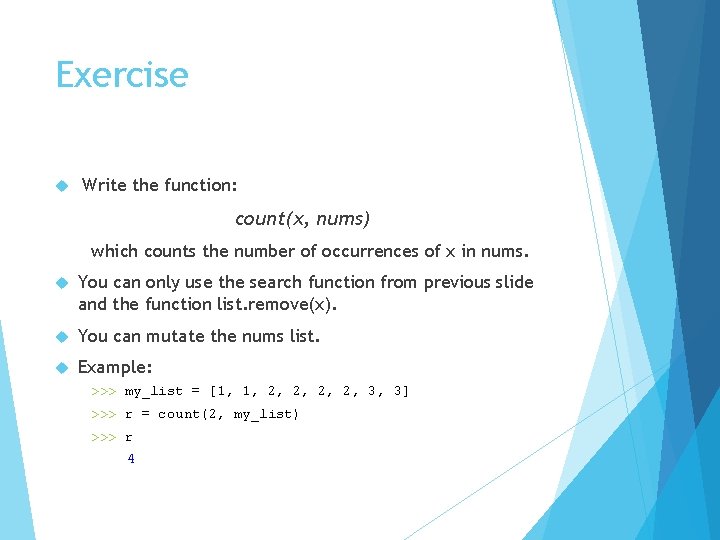
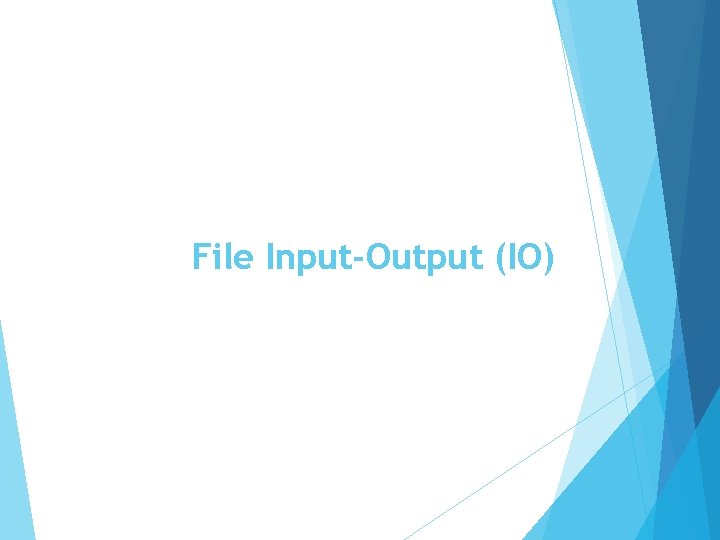
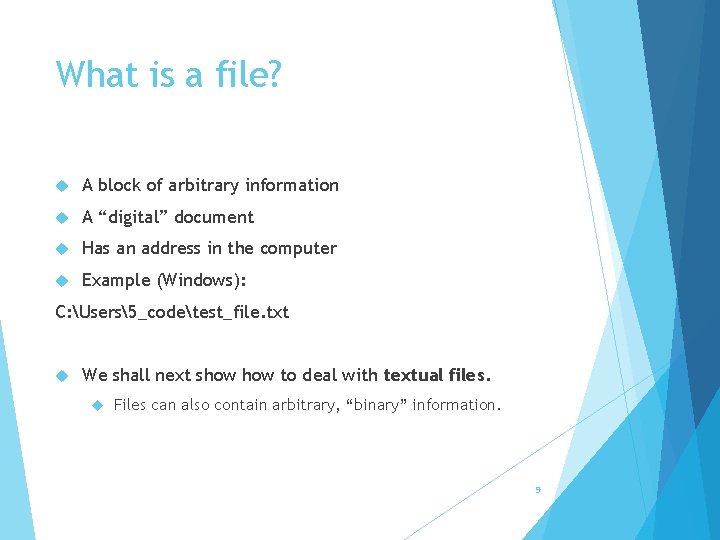
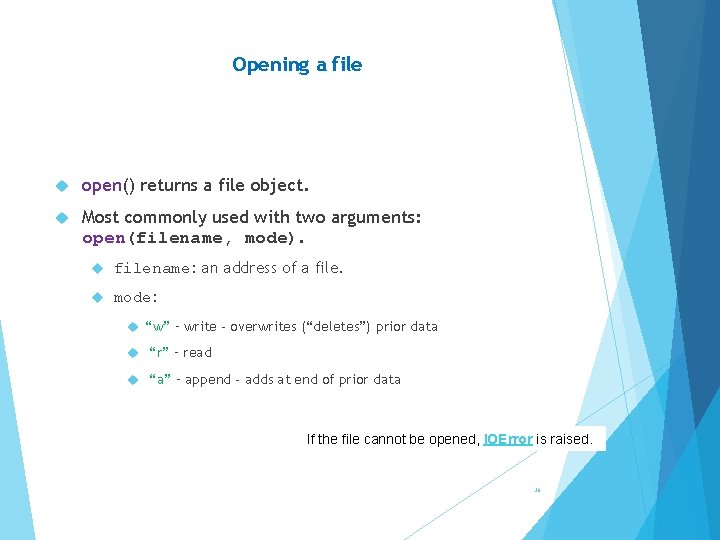
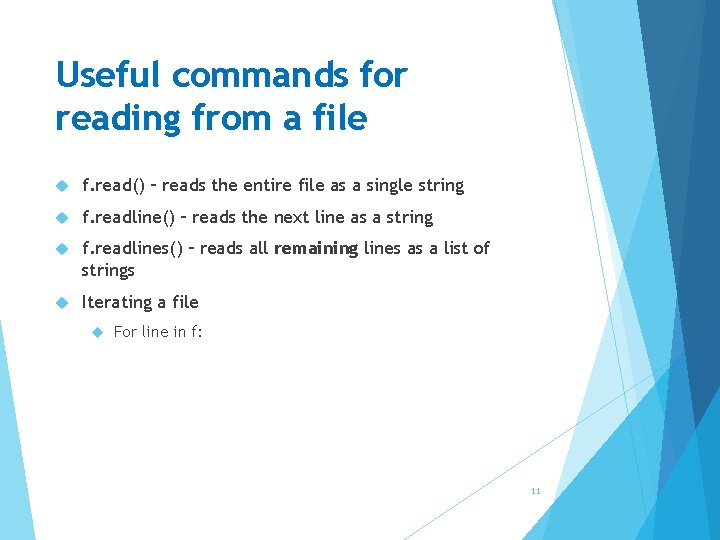
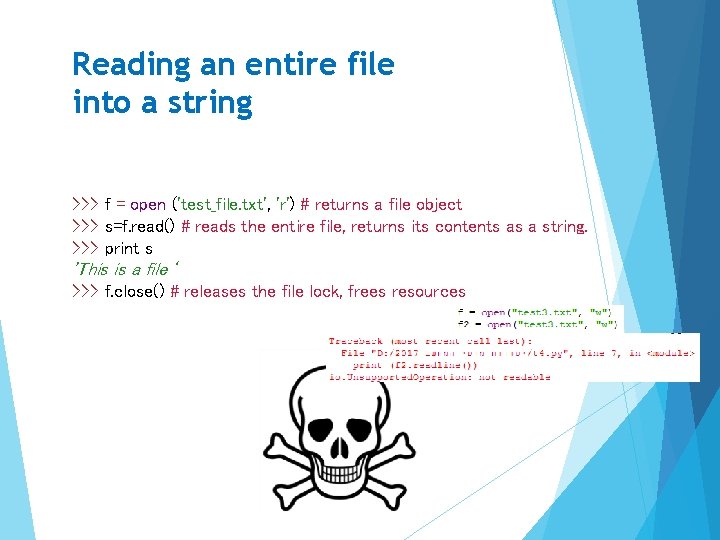
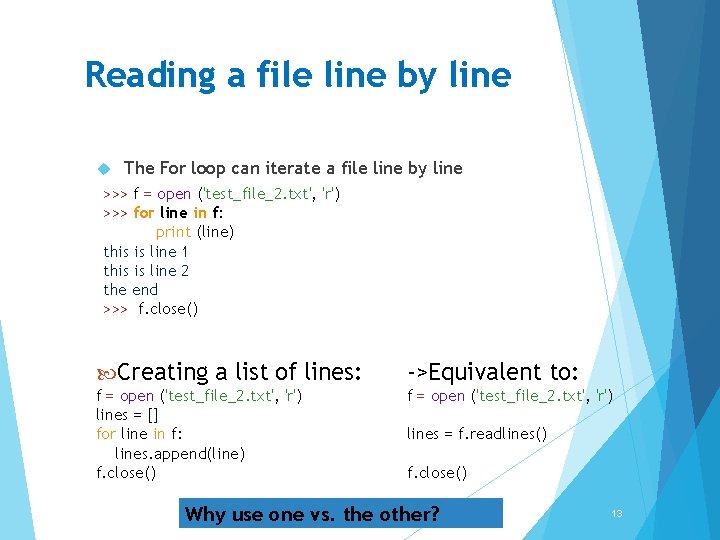
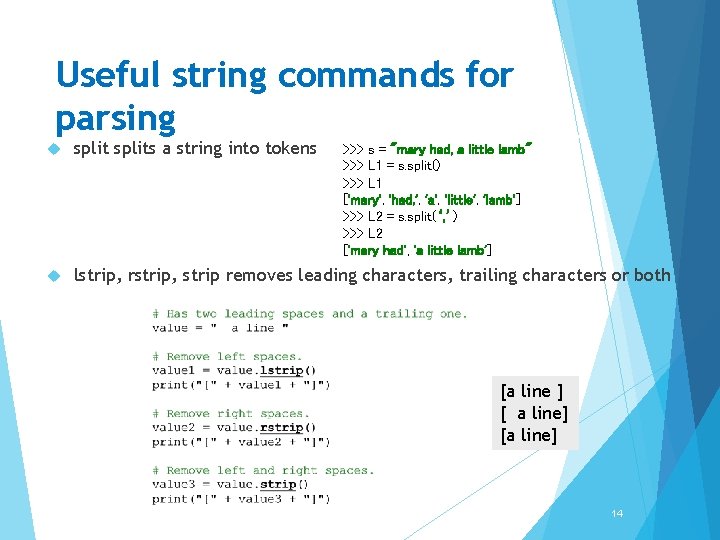
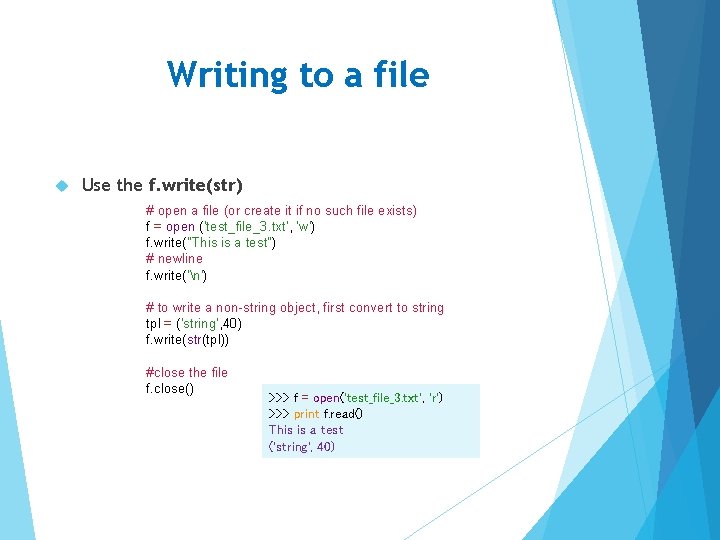
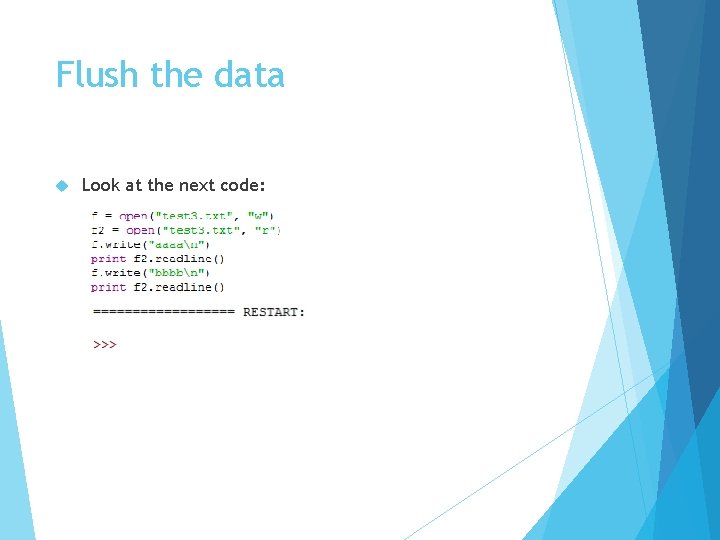
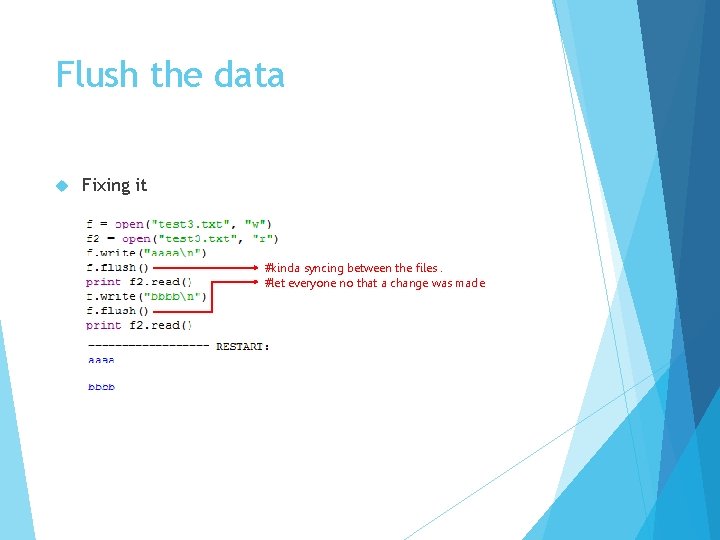
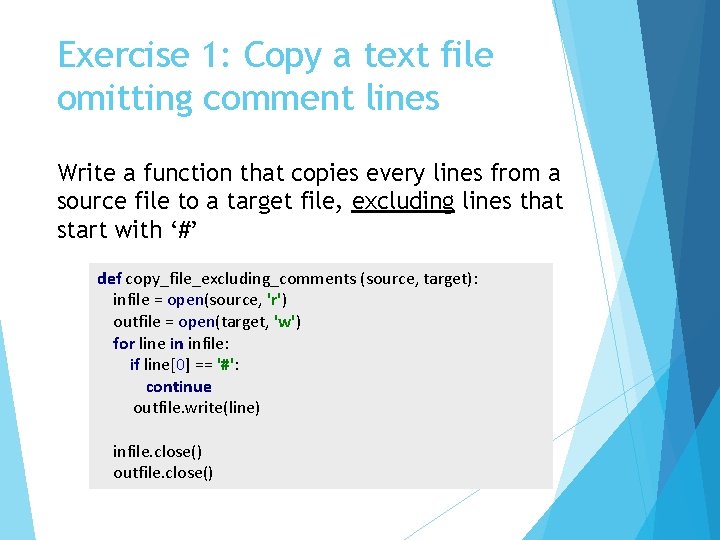
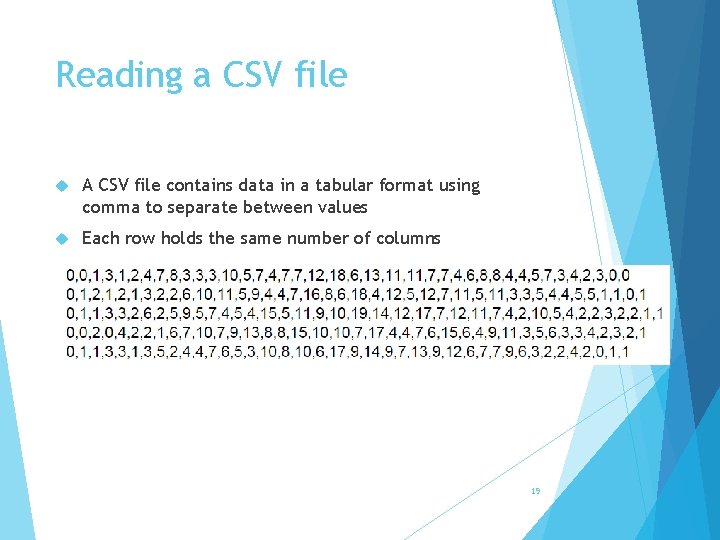
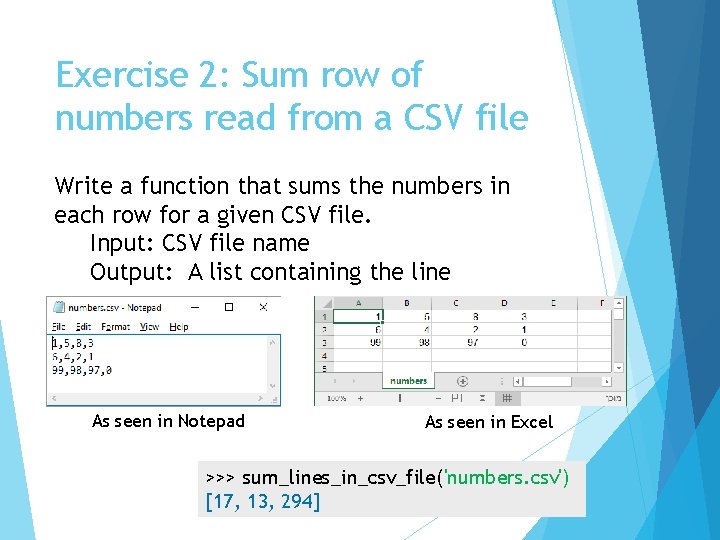
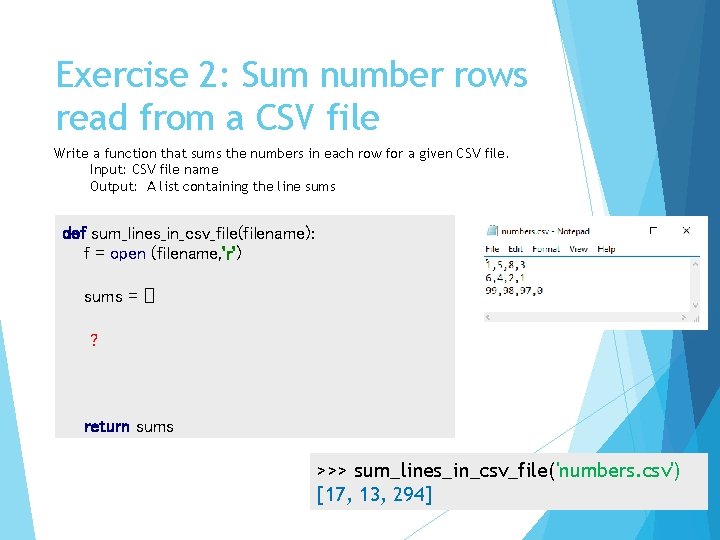
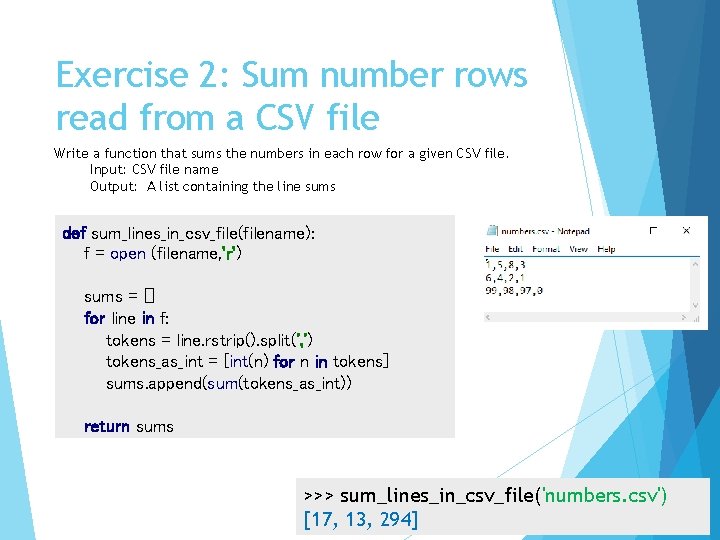
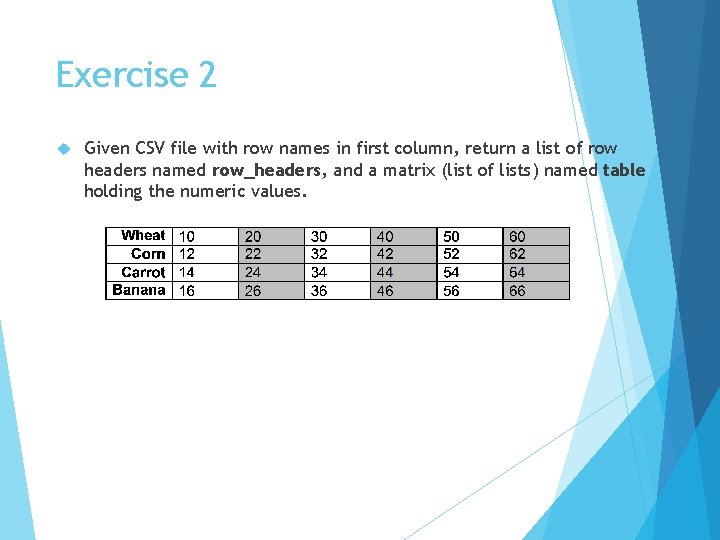
![def load_crops(filename): row_headers = [] table = [] f = open (filename, 'r’) for def load_crops(filename): row_headers = [] table = [] f = open (filename, 'r’) for](https://slidetodoc.com/presentation_image_h2/183bd65983ee3cd333695f897d6691f9/image-24.jpg)
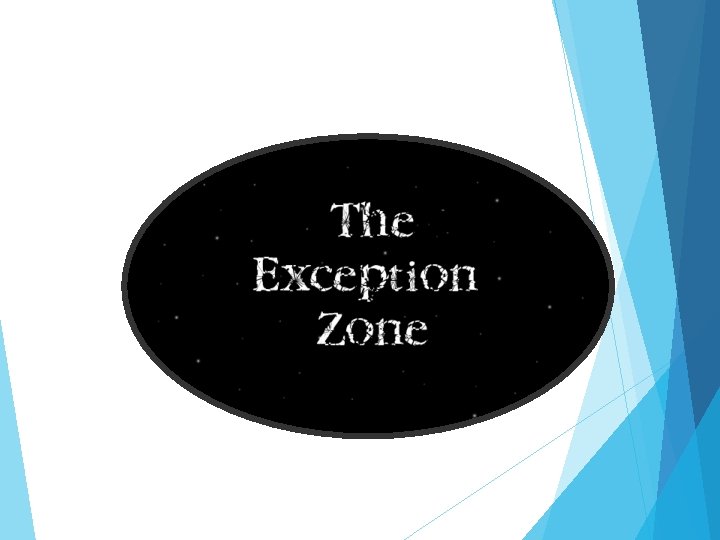
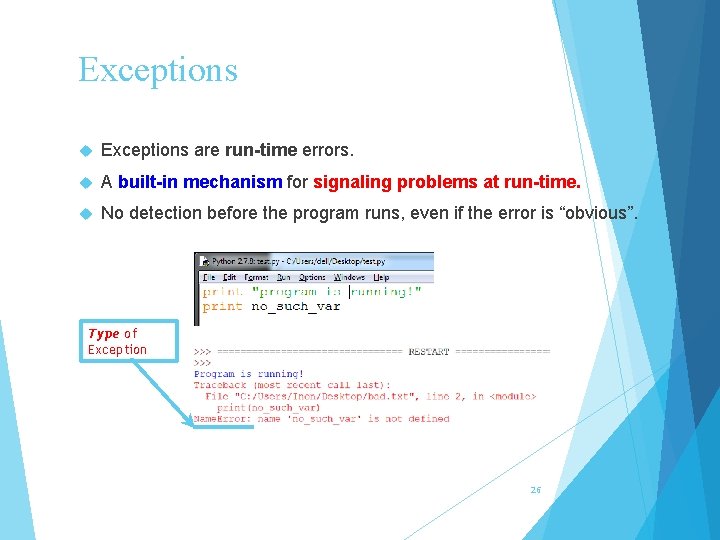
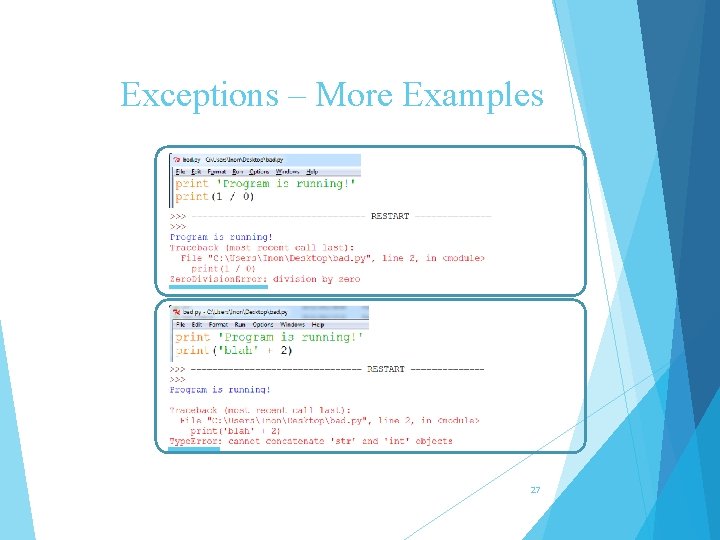
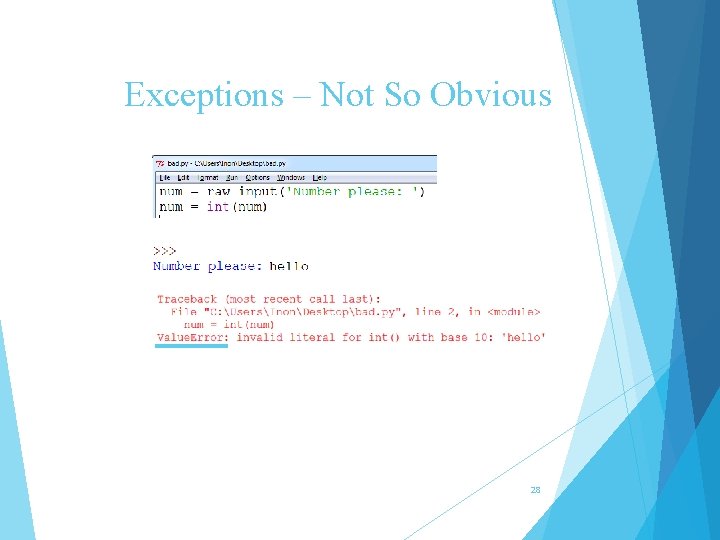
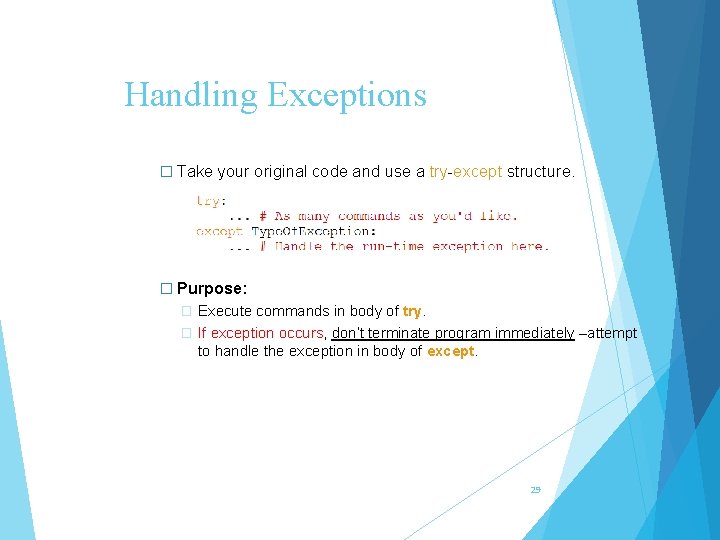
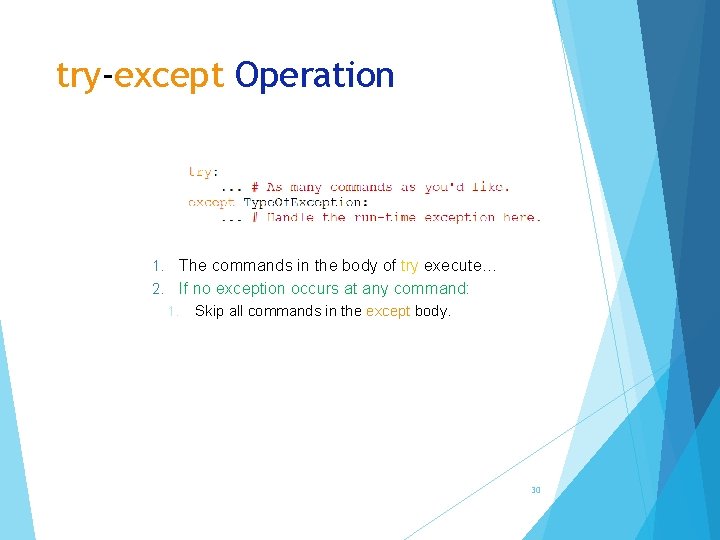
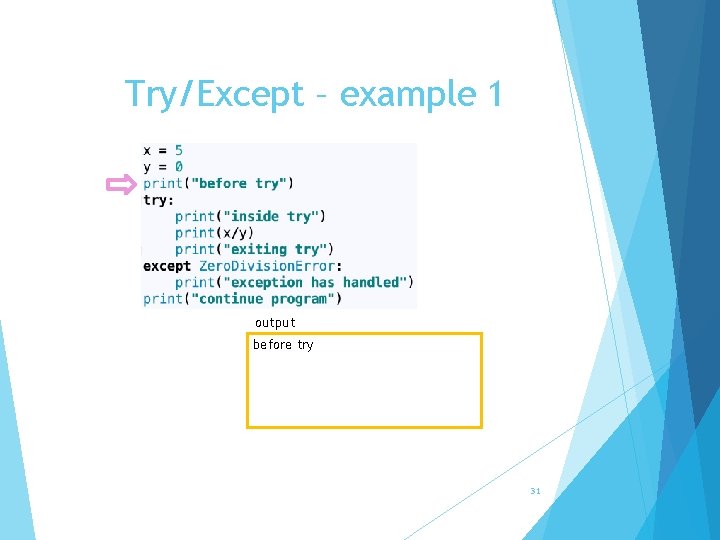
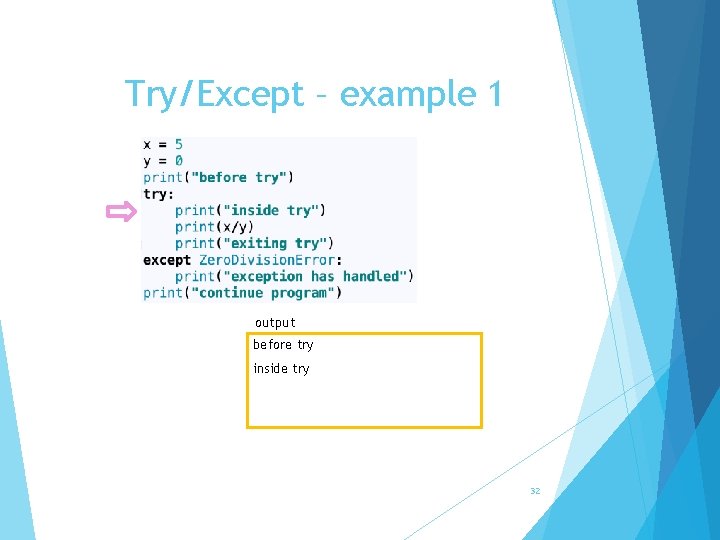
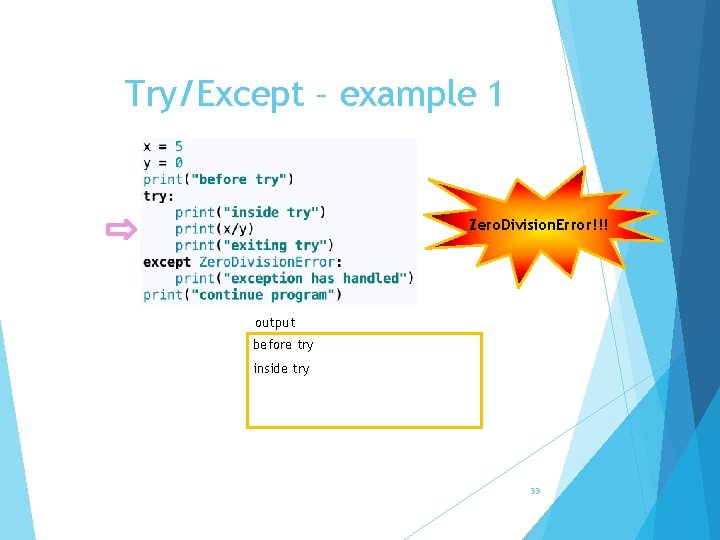
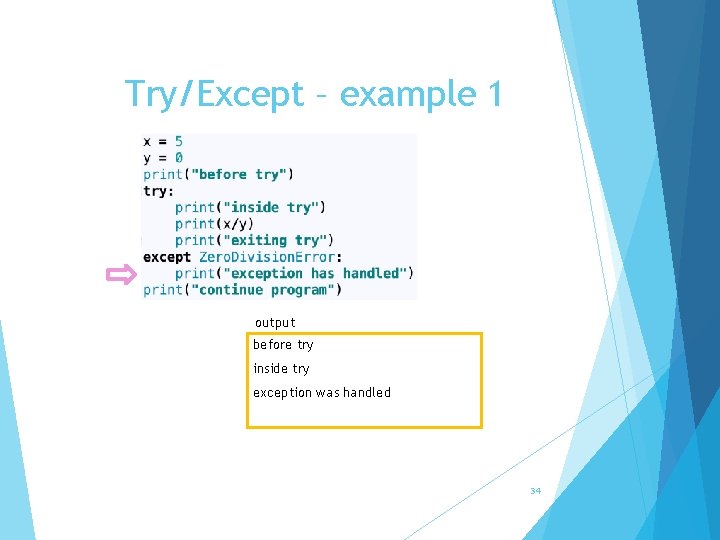
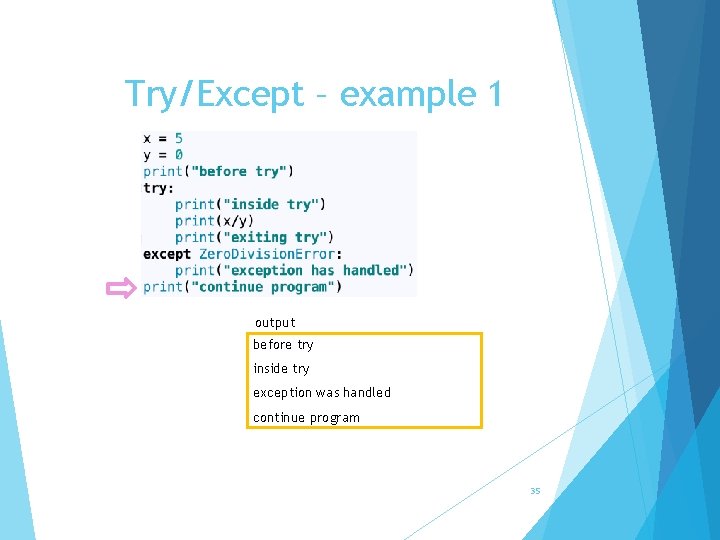
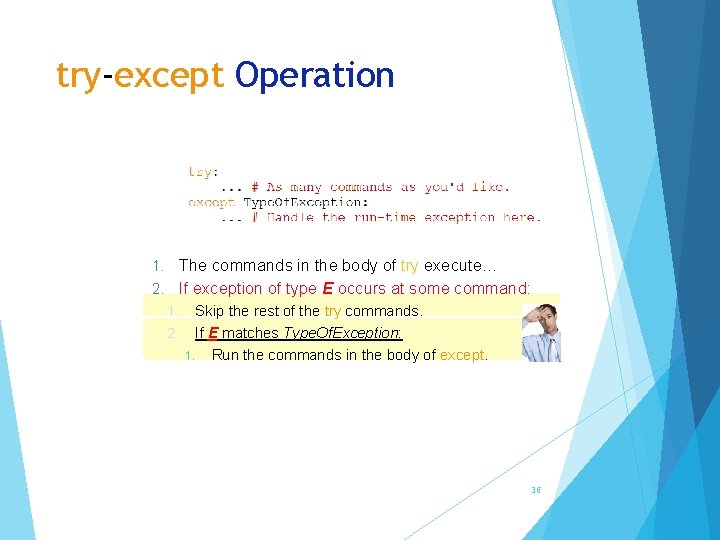
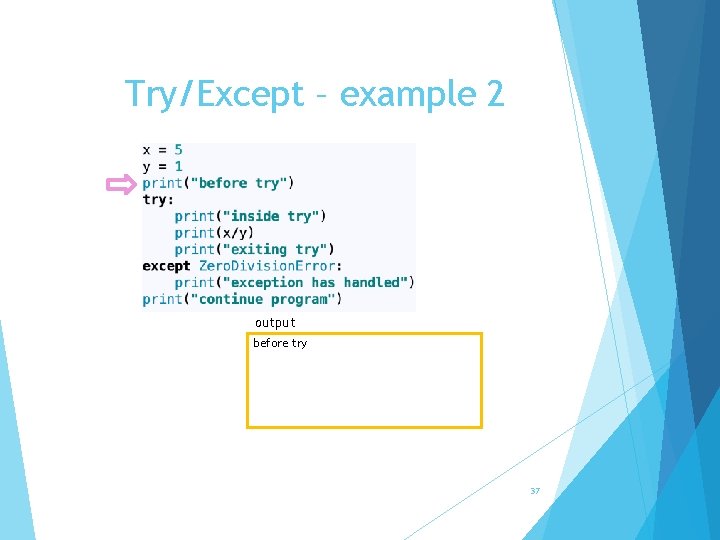
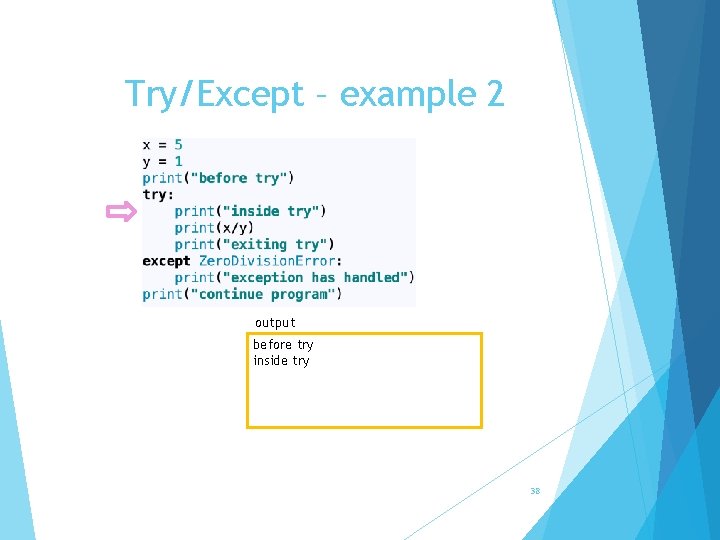
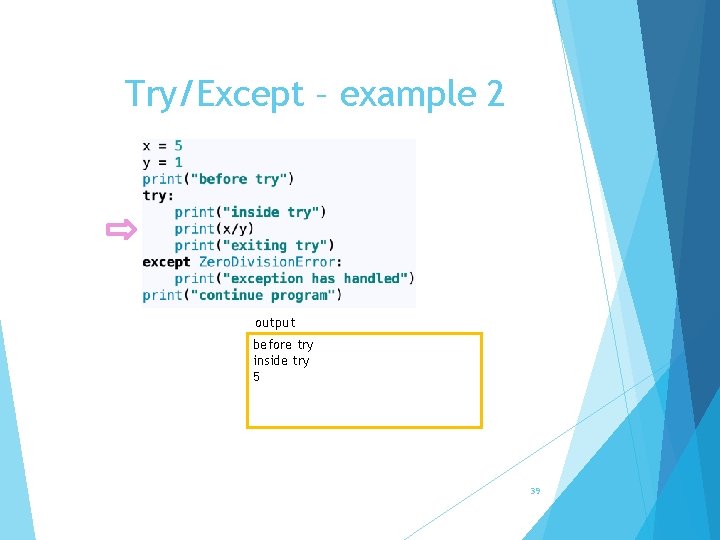
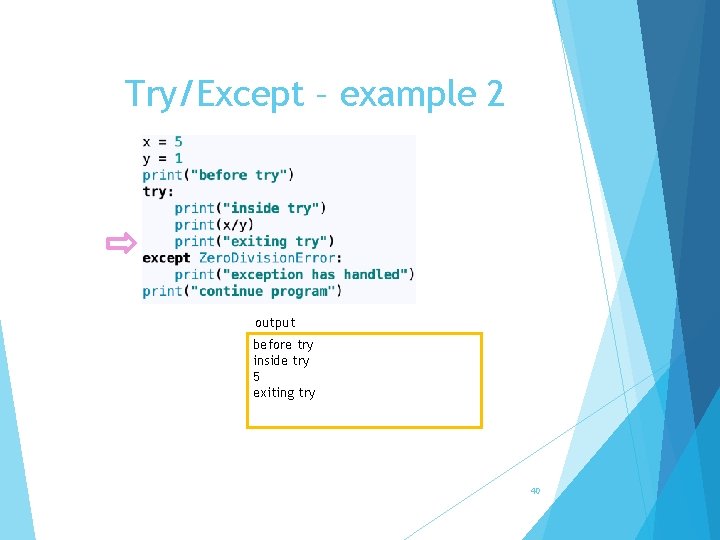
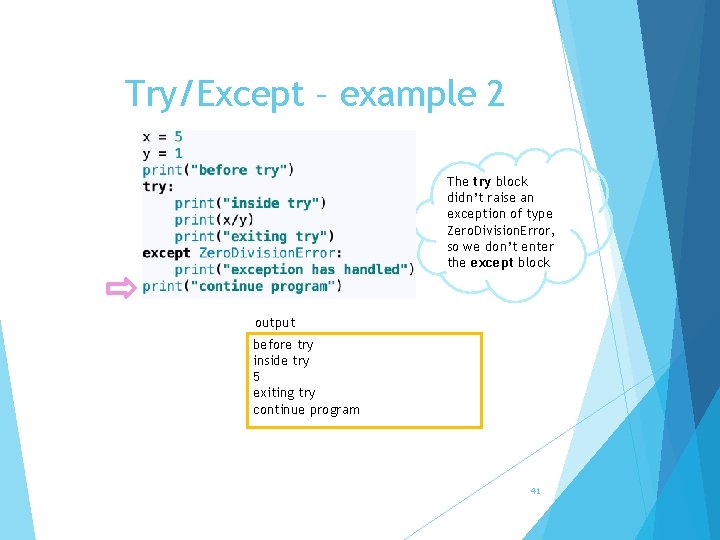
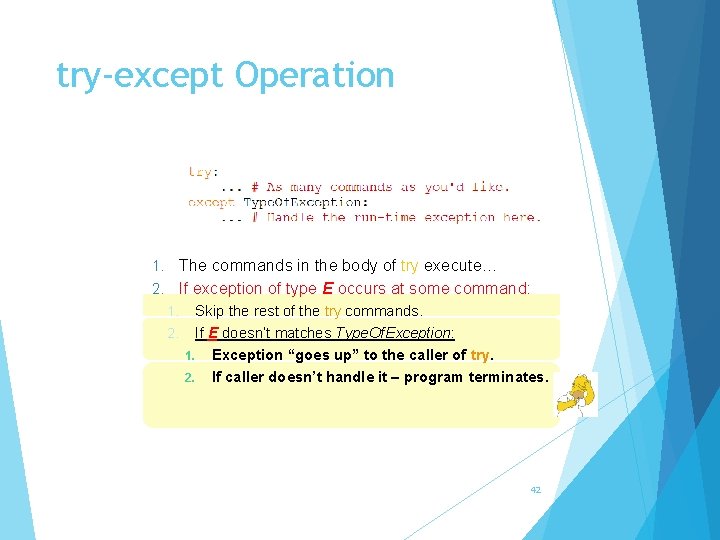
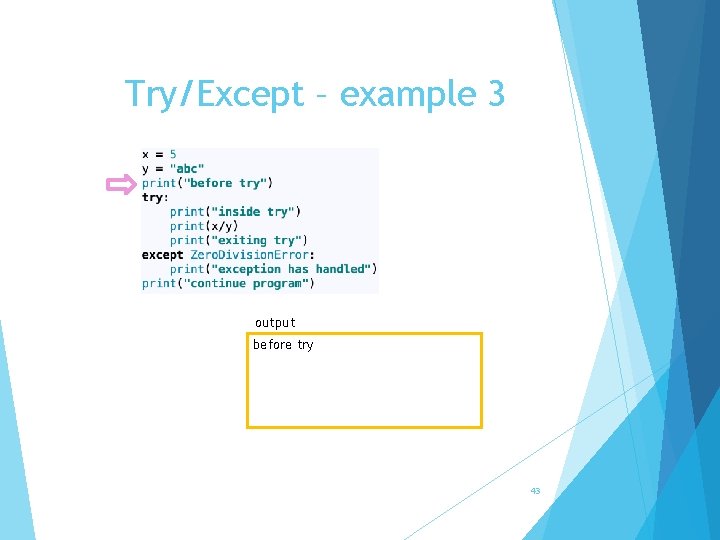
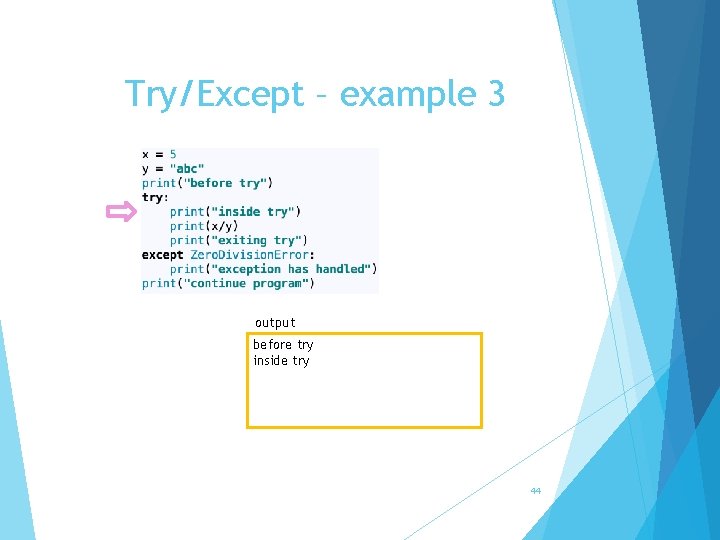
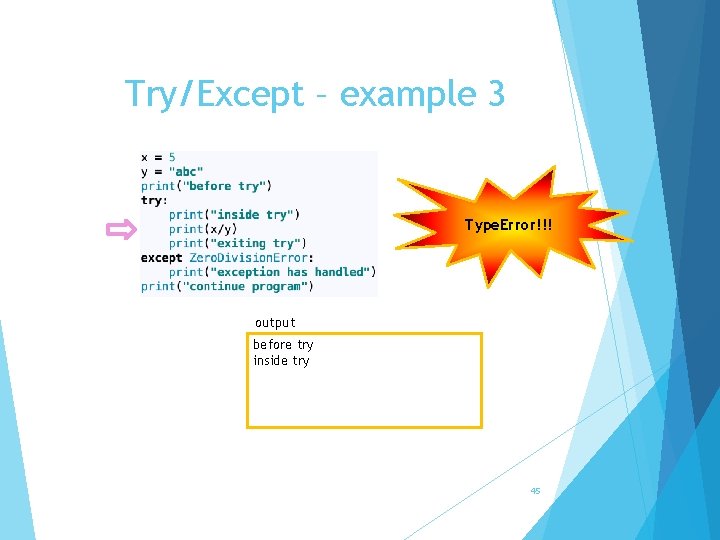
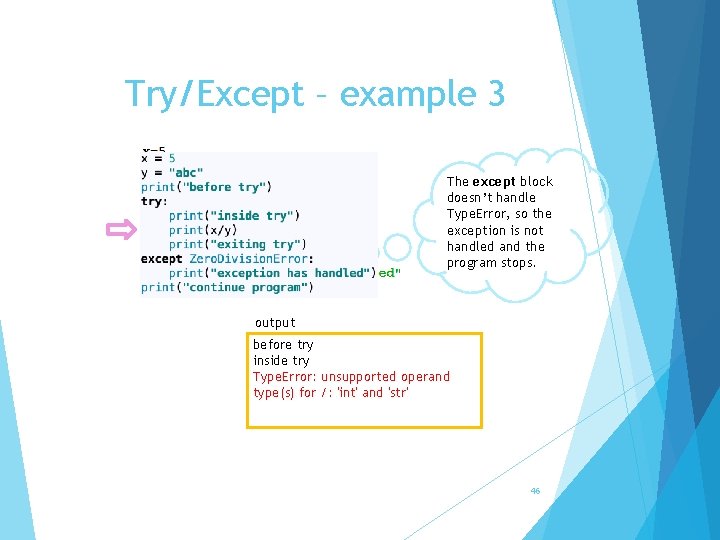
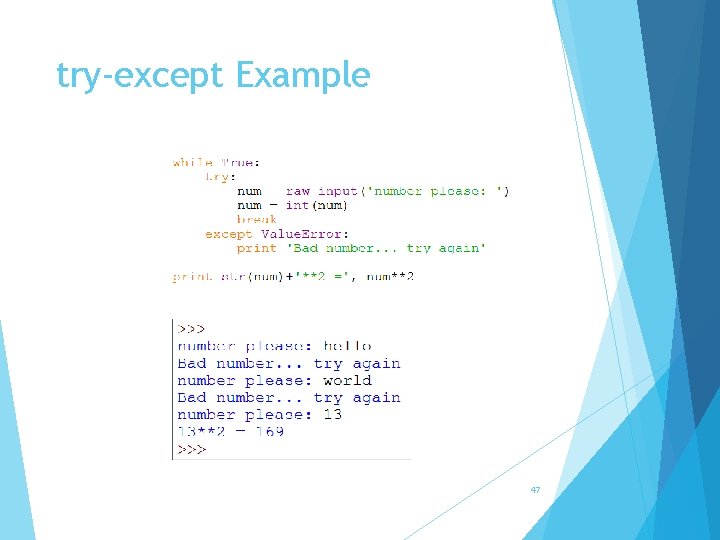
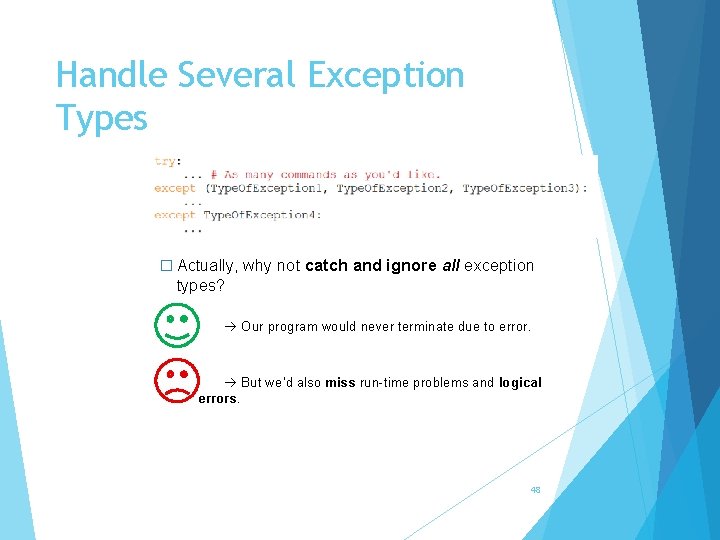
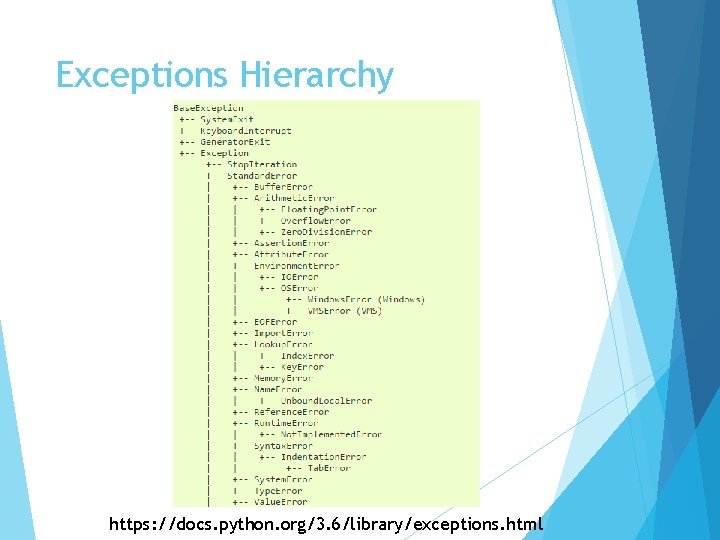
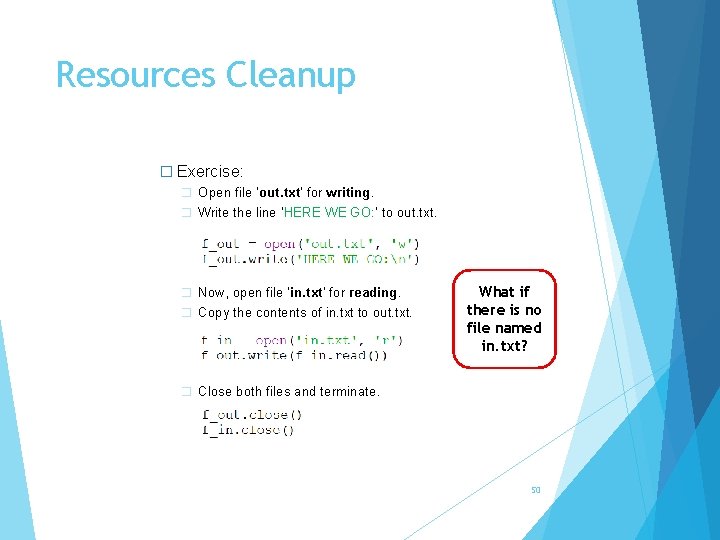
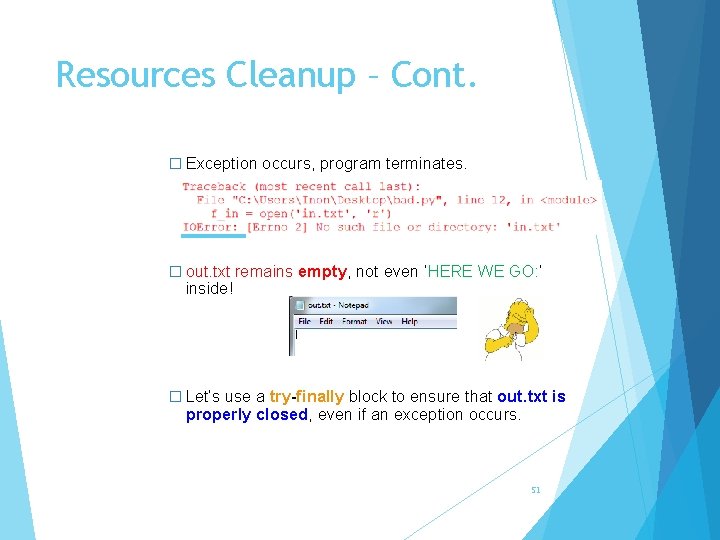
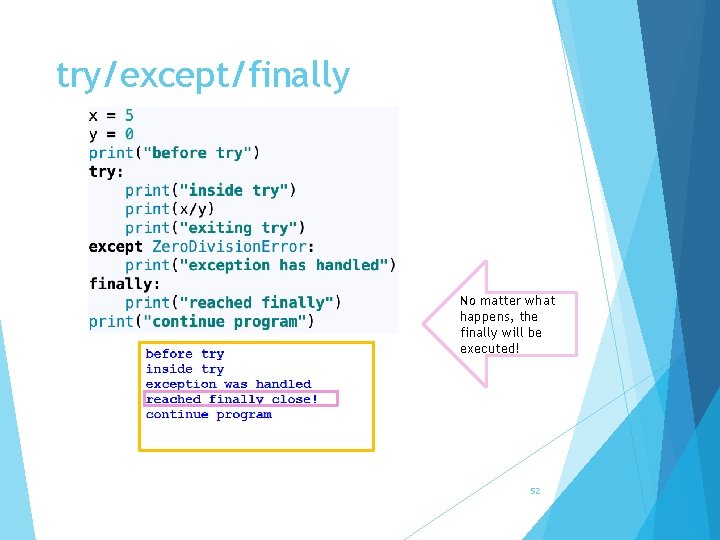
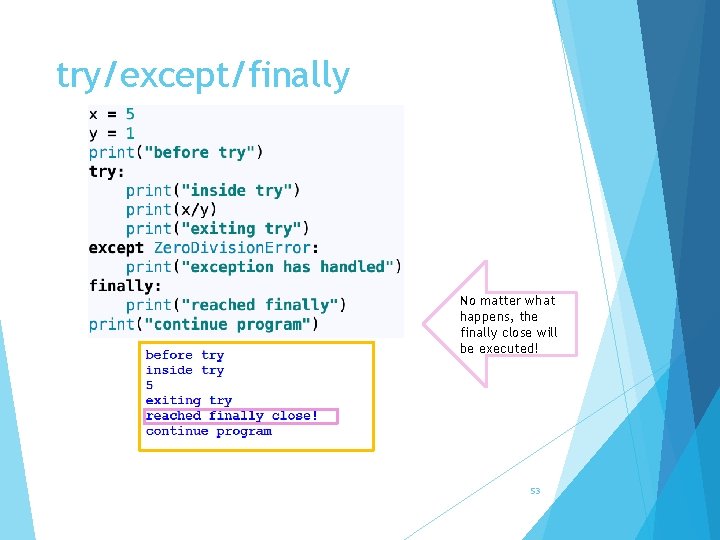
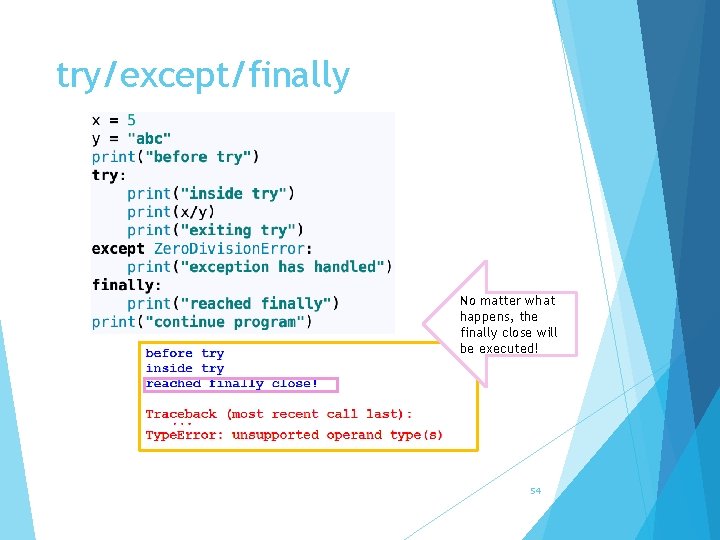
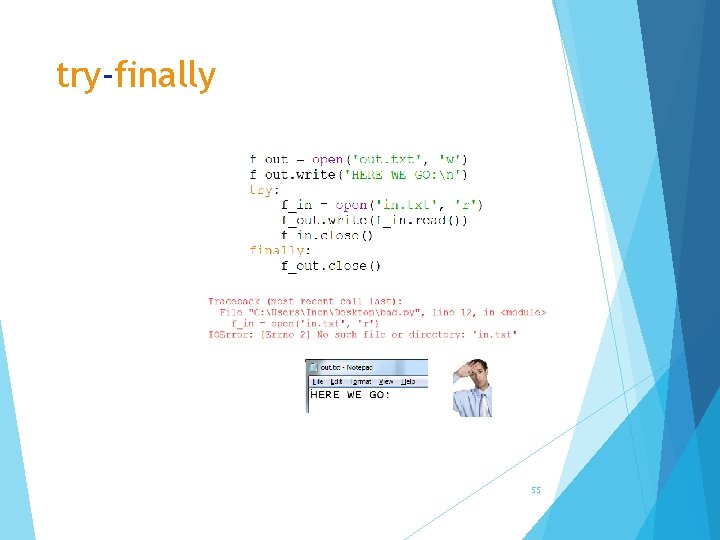
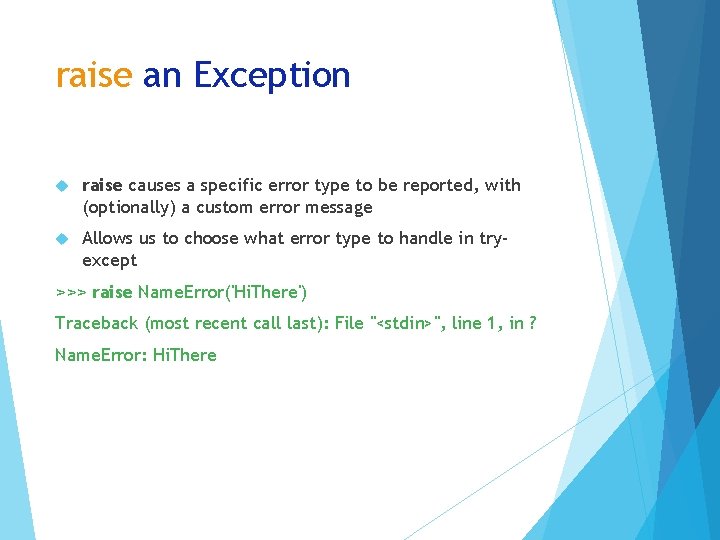
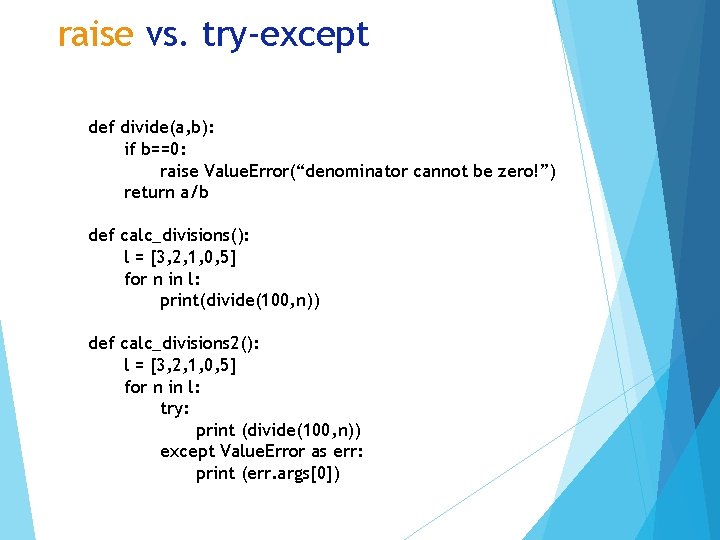
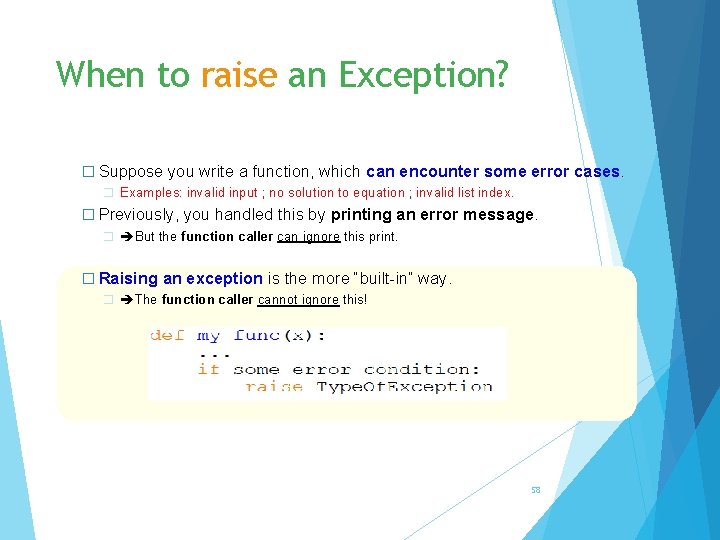
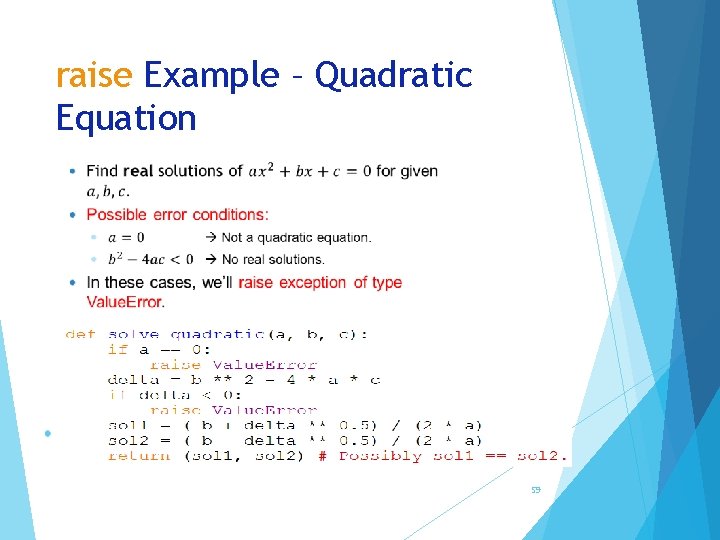
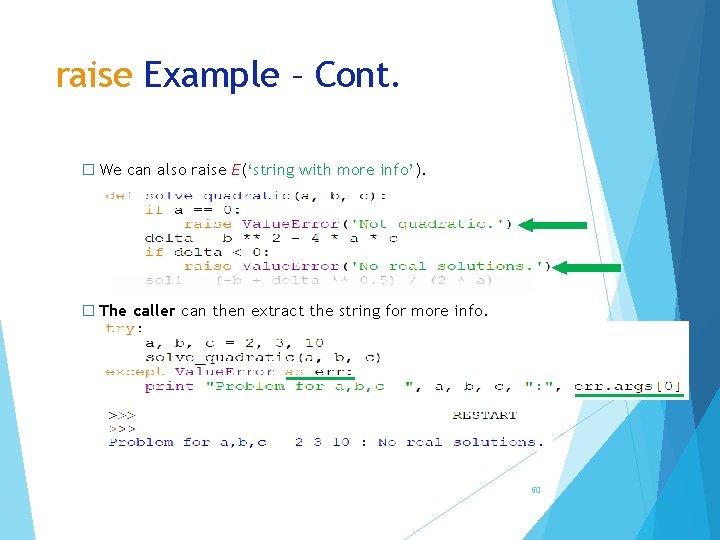
- Slides: 60
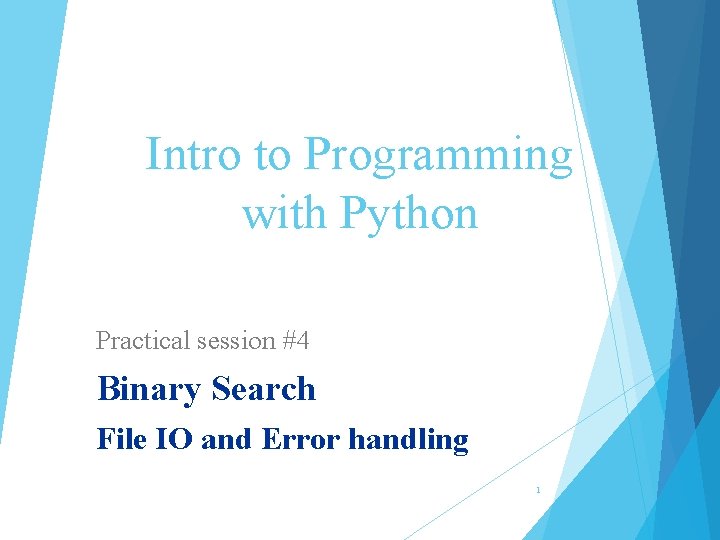
Intro to Programming with Python Practical session #4 Binary Search File IO and Error handling 1
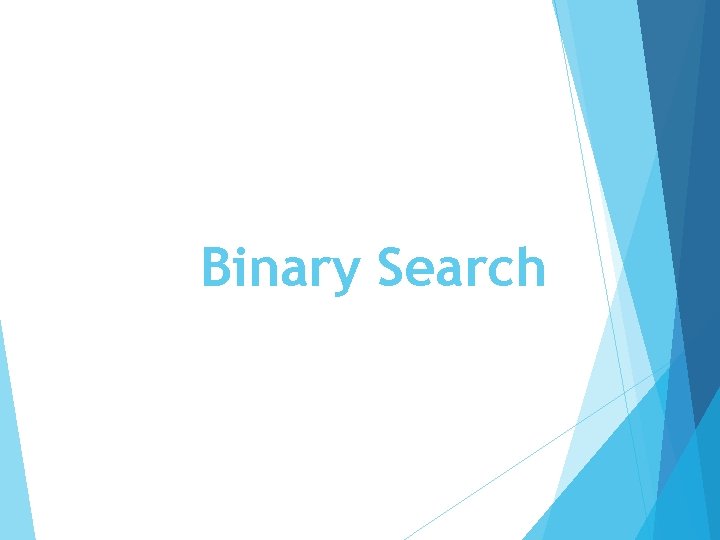
Binary Search
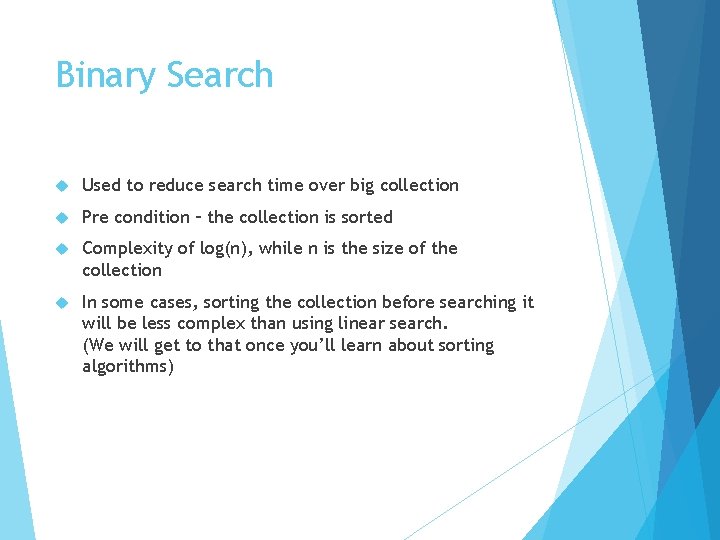
Binary Search Used to reduce search time over big collection Pre condition – the collection is sorted Complexity of log(n), while n is the size of the collection In some cases, sorting the collection before searching it will be less complex than using linear search. (We will get to that once you’ll learn about sorting algorithms)
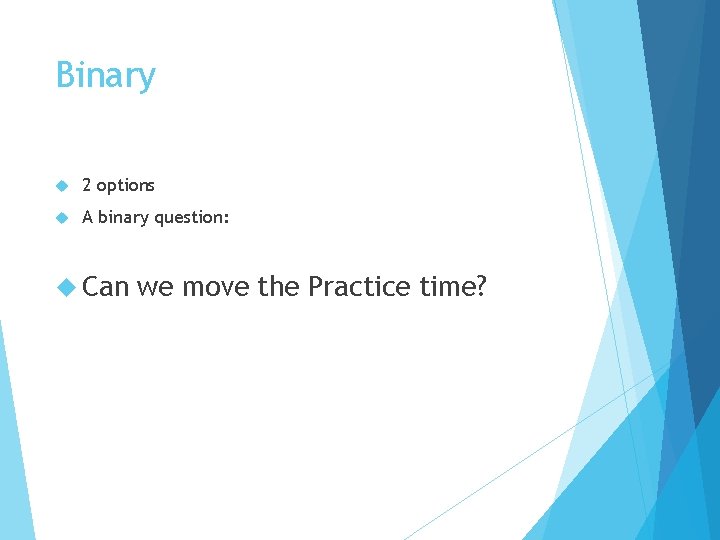
Binary 2 options A binary question: Can we move the Practice time?
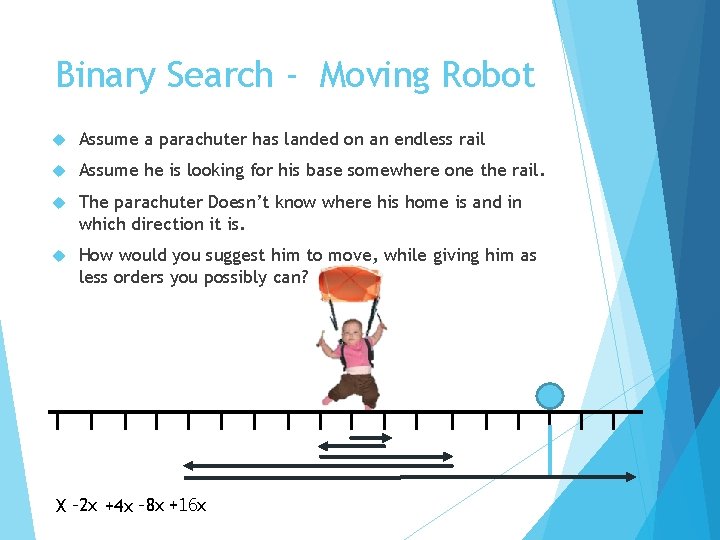
Binary Search - Moving Robot Assume a parachuter has landed on an endless rail Assume he is looking for his base somewhere one the rail. The parachuter Doesn’t know where his home is and in which direction it is. How would you suggest him to move, while giving him as less orders you possibly can? X -2 x +4 x -8 x +16 x
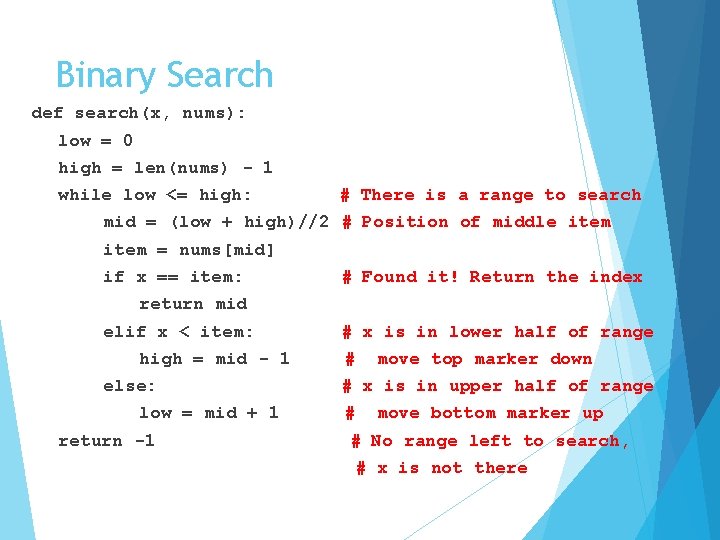
Binary Search def search(x, nums): low = 0 high = len(nums) - 1 while low <= high: # There is a range to search mid = (low + high)//2 # Position of middle item = nums[mid] if x == item: # Found it! Return the index return mid elif x < item: high = mid - 1 else: low = mid + 1 return -1 # x is in lower half of range # move top marker down # x is in upper half of range # move bottom marker up # No range left to search, # x is not there
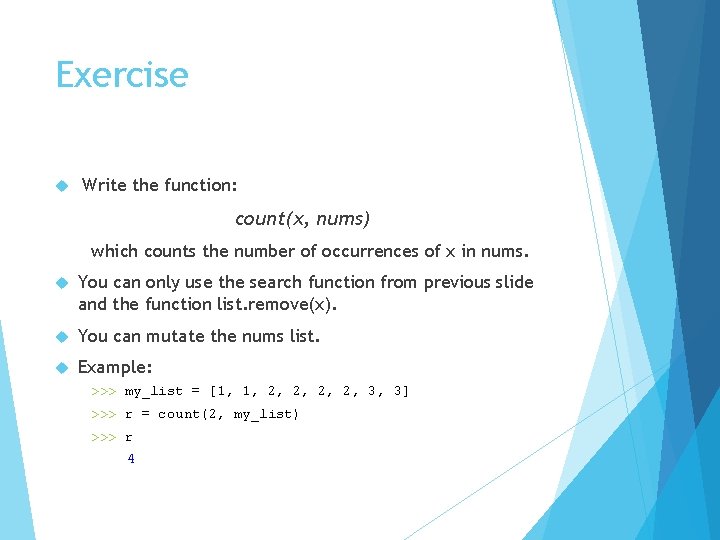
Exercise Write the function: count(x, nums) which counts the number of occurrences of x in nums. You can only use the search function from previous slide and the function list. remove(x). You can mutate the nums list. Example: >>> my_list = [1, 1, 2, 2, 3, 3] >>> r = count(2, my_list) >>> r 4
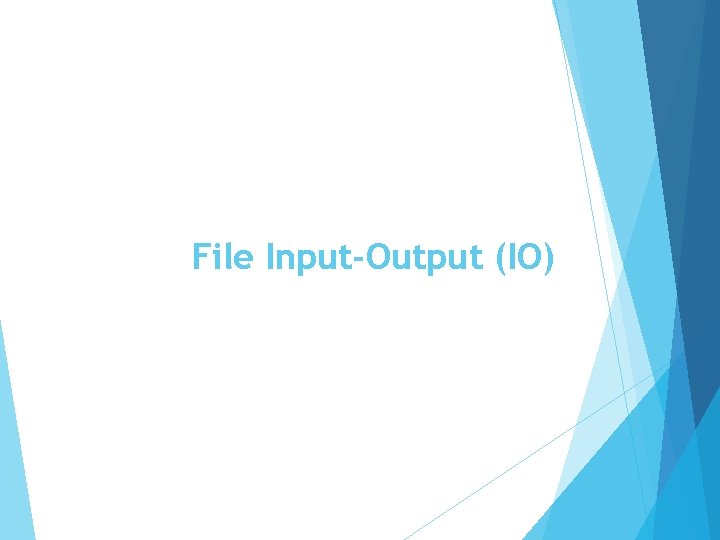
File Input-Output (IO)
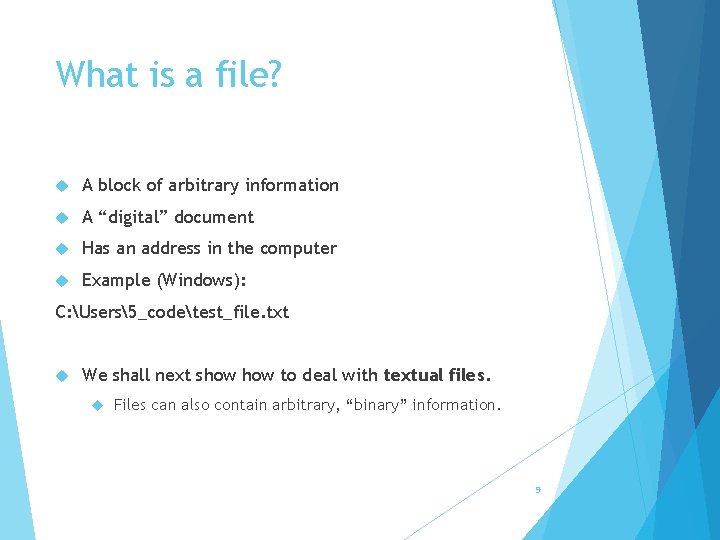
What is a file? A block of arbitrary information A “digital” document Has an address in the computer Example (Windows): C: Users5_codetest_file. txt We shall next show to deal with textual files. Files can also contain arbitrary, “binary” information. 9
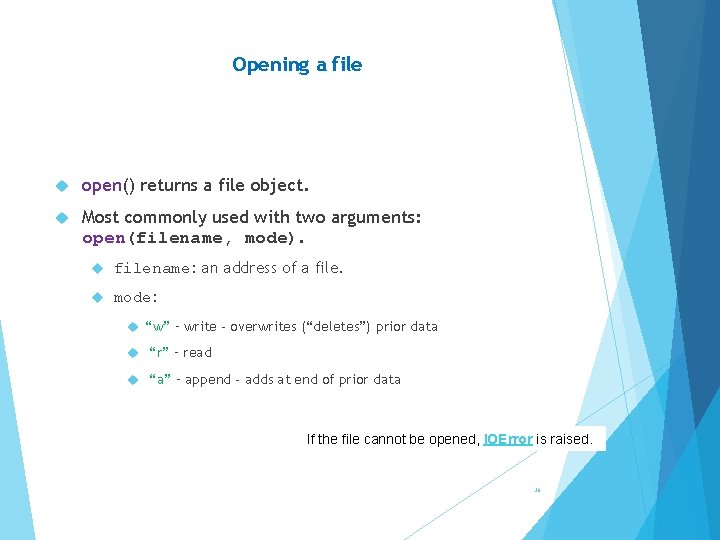
Opening a file open() returns a file object. Most commonly used with two arguments: open(filename, mode). filename: an address of a file. mode: “w” – write - overwrites (“deletes”) prior data “r” – read “a” – append - adds at end of prior data What happens the file does not exist ? If the file cannot beifopened, IOError is raised. 10
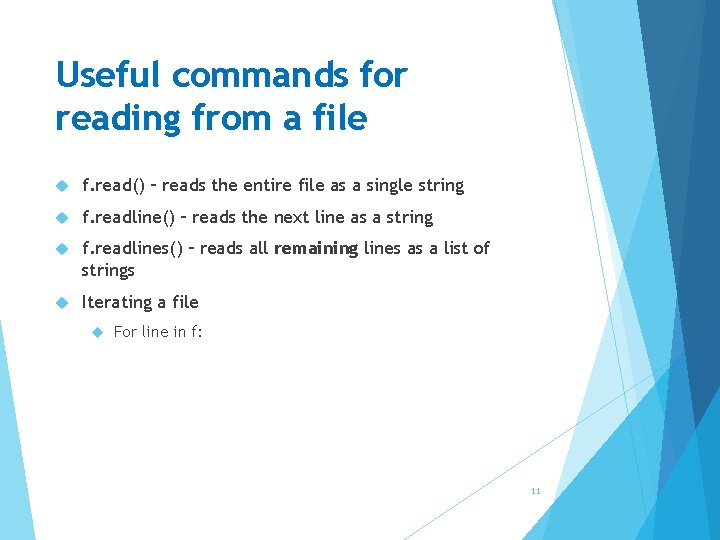
Useful commands for reading from a file f. read() – reads the entire file as a single string f. readline() – reads the next line as a string f. readlines() – reads all remaining lines as a list of strings Iterating a file For line in f: 11
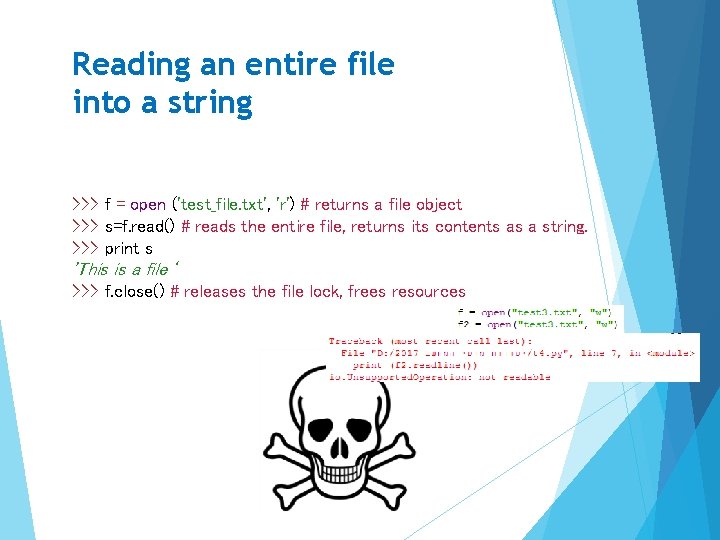
Reading an entire file into a string >>> f = open ('test_file. txt', 'r') # returns a file object >>> s=f. read() # reads the entire file, returns its contents as a string. >>> print s 'This is a file‘ >>> f. close() # releases the file lock, frees resources 12
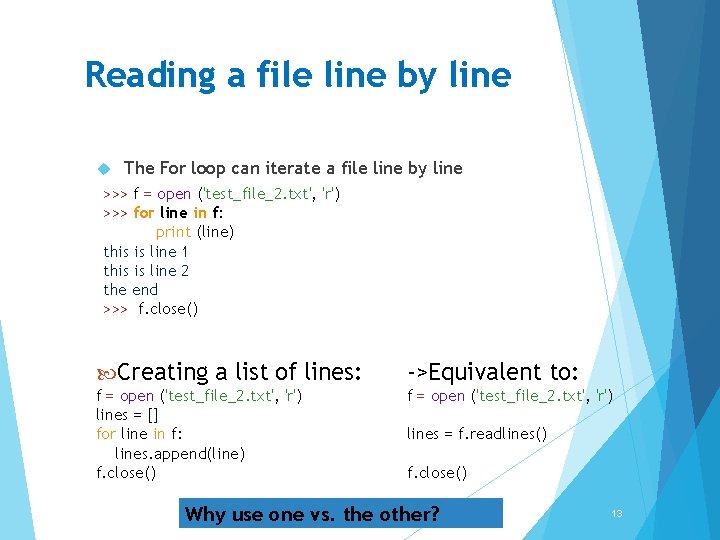
Reading a file line by line The For loop can iterate a file line by line >>> f = open ('test_file_2. txt', 'r') >>> for line in f: print (line) this is line 1 this is line 2 the end >>> f. close() Creating a list of lines: ->Equivalent to: f = open ('test_file_2. txt', 'r') lines = [] for line in f: lines. append(line) f. close() f = open ('test_file_2. txt', 'r') lines = f. readlines() f. close() Why use one vs. the other? 13
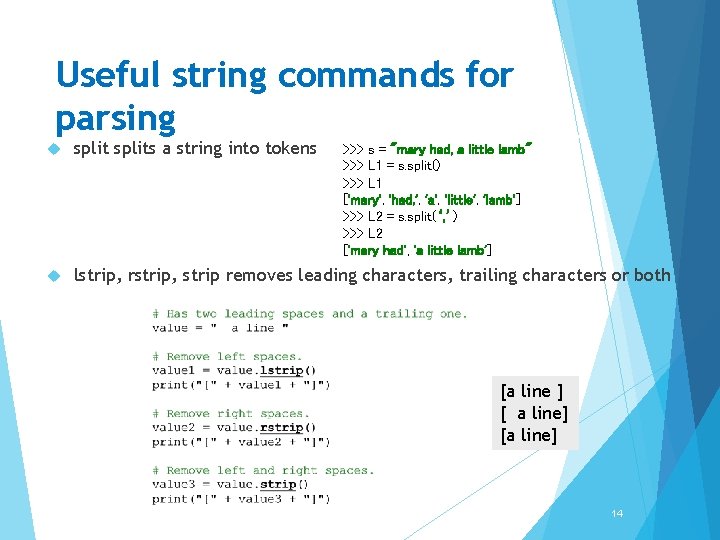
Useful string commands for parsing splits a string into tokens lstrip, rstrip, strip removes leading characters, trailing characters or both >>> s = "mary had, a little lamb" >>> L 1 = s. split() >>> L 1 ['mary', 'had, ', 'a', 'little', 'lamb'] >>> L 2 = s. split(‘, ’) >>> L 2 ['mary had', 'a little lamb'] [a line ] [ a line] [a line] 14
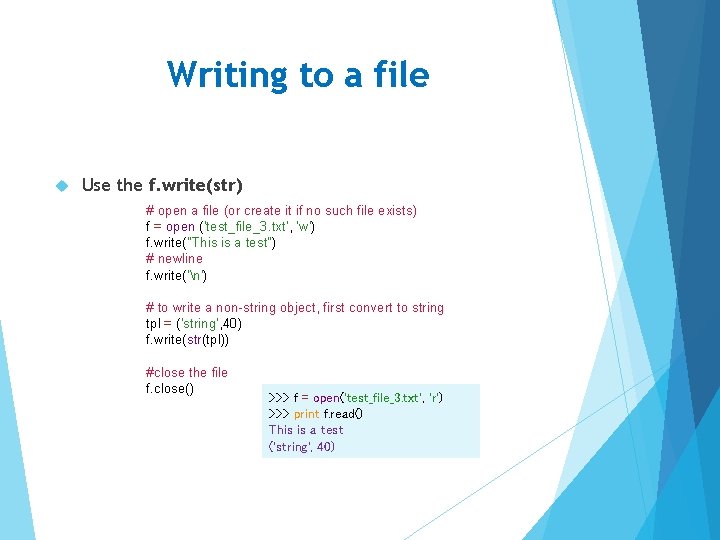
Writing to a file Use the f. write(str) # open a file (or create it if no such file exists) f = open ('test_file_3. txt', ‘w') f. write("This is a test") # newline f. write('n') # to write a non-string object, first convert to string tpl = ('string', 40) f. write(str(tpl)) #close the file f. close() >>> f = open('test_file_3. txt', 'r') >>> print f. read() This is a test ('string', 40) 15
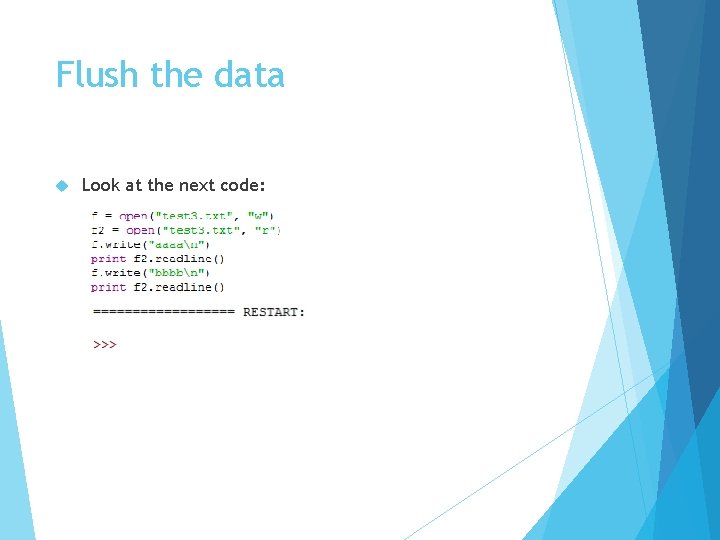
Flush the data Look at the next code:
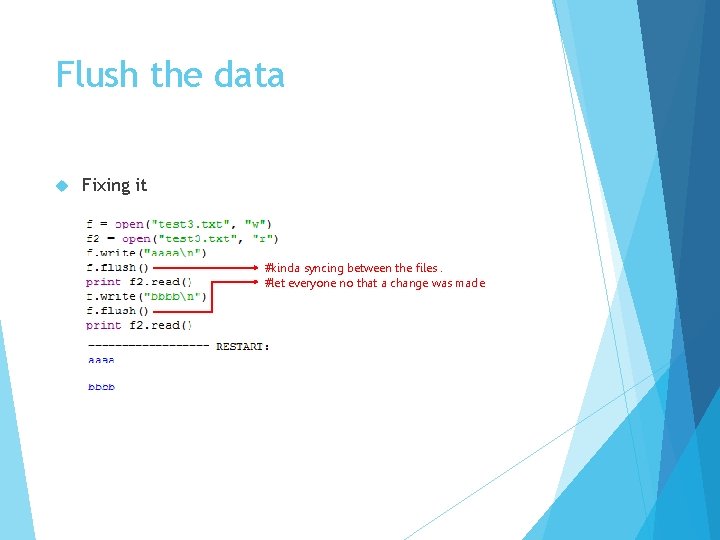
Flush the data Fixing it #kinda syncing between the files. #let everyone no that a change was made
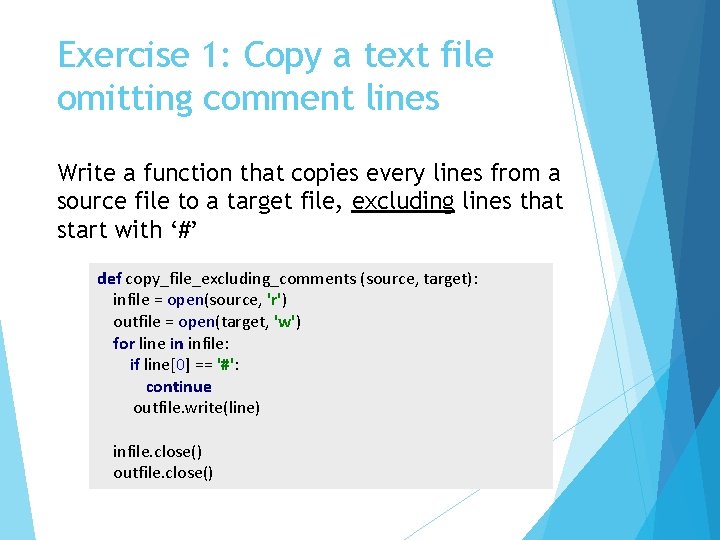
Exercise 1: Copy a text file omitting comment lines Write a function that copies every lines from a source file to a target file, excluding lines that start with ‘#’ def copy_file_excluding_comments (source, target): infile = open(source, 'r') outfile = open(target, 'w') for line in infile: if line[0] == '#': continue outfile. write(line) infile. close() outfile. close()
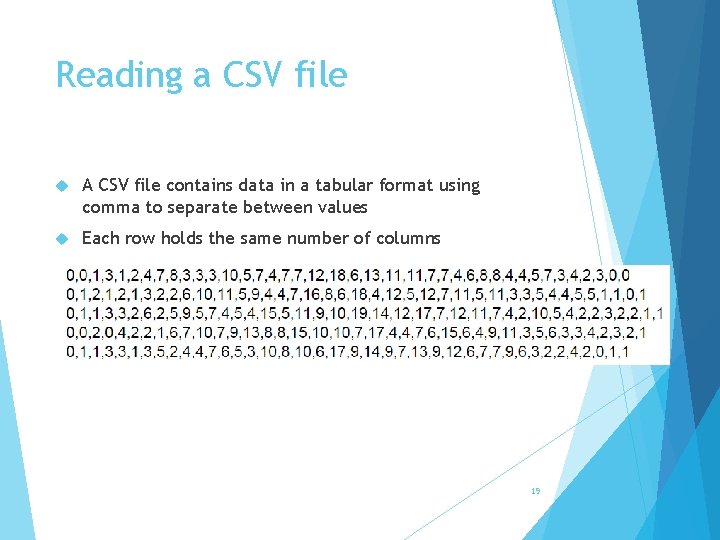
Reading a CSV file A CSV file contains data in a tabular format using comma to separate between values Each row holds the same number of columns 19
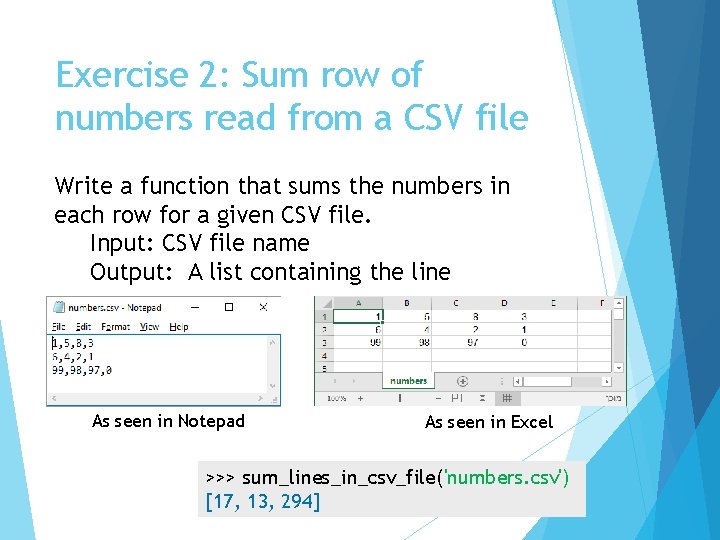
Exercise 2: Sum row of numbers read from a CSV file Write a function that sums the numbers in each row for a given CSV file. Input: CSV file name Output: A list containing the line sums As seen in Notepad As seen in Excel >>> sum_lines_in_csv_file('numbers. csv') [17, 13, 294]
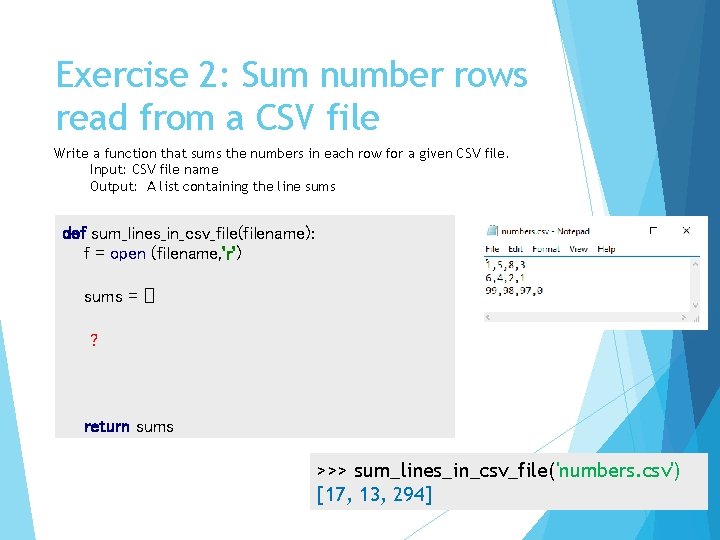
Exercise 2: Sum number rows read from a CSV file Write a function that sums the numbers in each row for a given CSV file. Input: CSV file name Output: A list containing the line sums def sum_lines_in_csv_file(filename): f = open (filename, 'r') sums = [] ? return sums >>> sum_lines_in_csv_file('numbers. csv') [17, 13, 294]
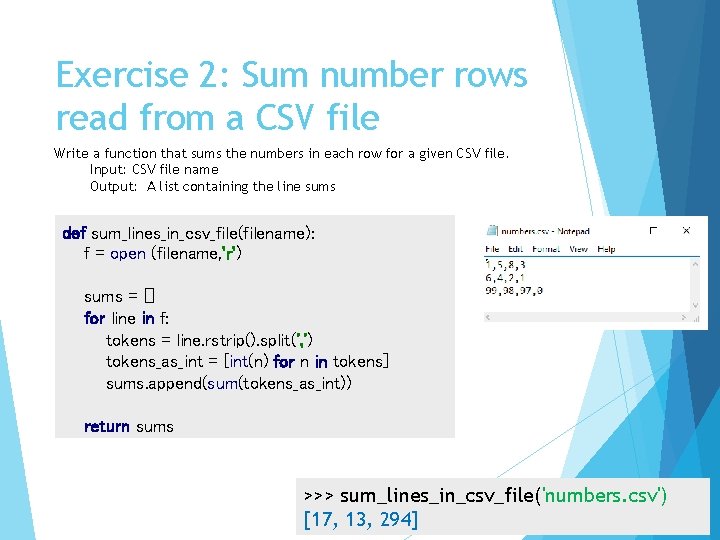
Exercise 2: Sum number rows read from a CSV file Write a function that sums the numbers in each row for a given CSV file. Input: CSV file name Output: A list containing the line sums def sum_lines_in_csv_file(filename): f = open (filename, 'r') sums = [] for line in f: tokens = line. rstrip(). split(', ') tokens_as_int = [int(n) for n in tokens] sums. append(sum(tokens_as_int)) return sums >>> sum_lines_in_csv_file('numbers. csv') [17, 13, 294]
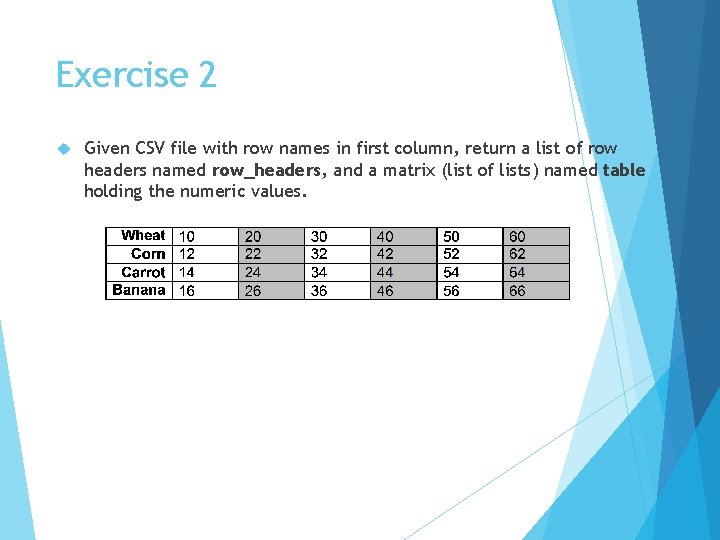
Exercise 2 Given CSV file with row names in first column, return a list of row headers named row_headers, and a matrix (list of lists) named table holding the numeric values.
![def loadcropsfilename rowheaders table f open filename r for def load_crops(filename): row_headers = [] table = [] f = open (filename, 'r’) for](https://slidetodoc.com/presentation_image_h2/183bd65983ee3cd333695f897d6691f9/image-24.jpg)
def load_crops(filename): row_headers = [] table = [] f = open (filename, 'r’) for line in f: tokens = line. strip('n'). split(', ’) row_headers. append(tokens[0]) table. append([int(x) for x in tokens[1: ]]) f. close() return row_headers, table
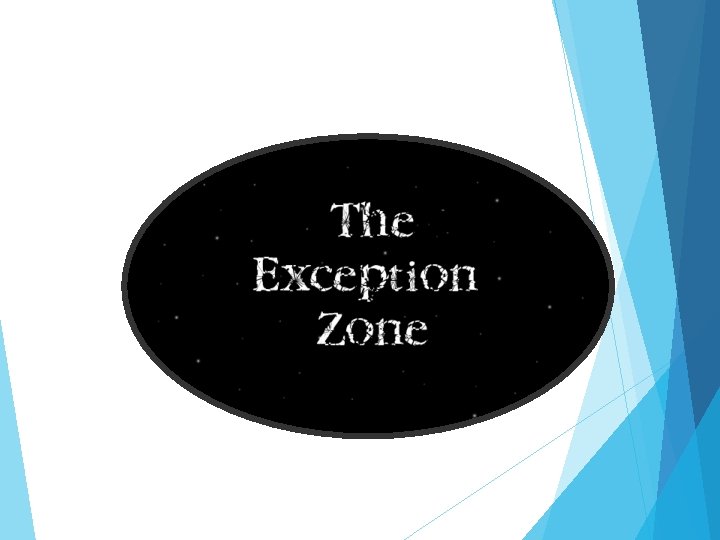
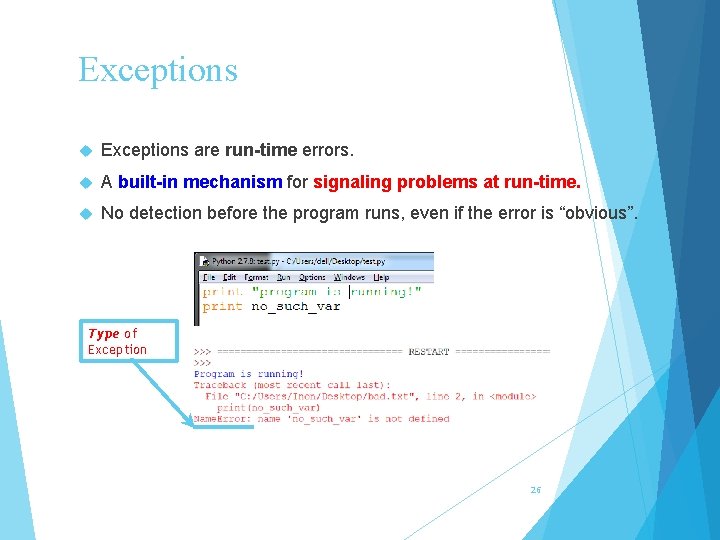
Exceptions are run-time errors. A built-in mechanism for signaling problems at run-time. No detection before the program runs, even if the error is “obvious”. Type of Exception 26
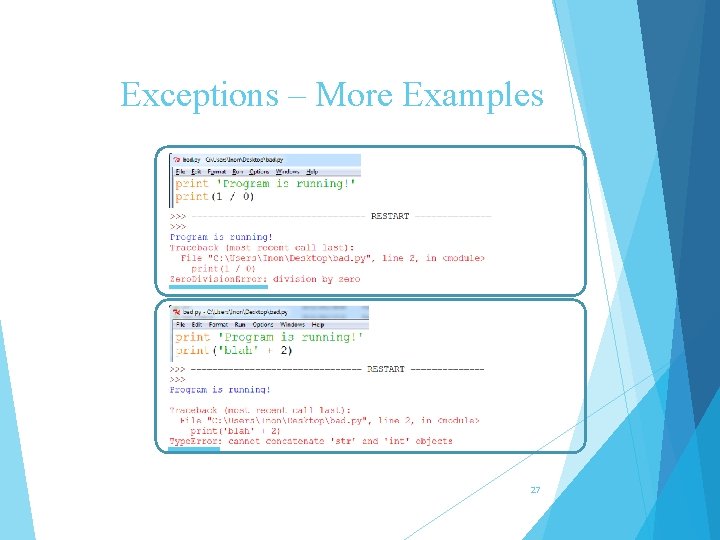
Exceptions – More Examples 27
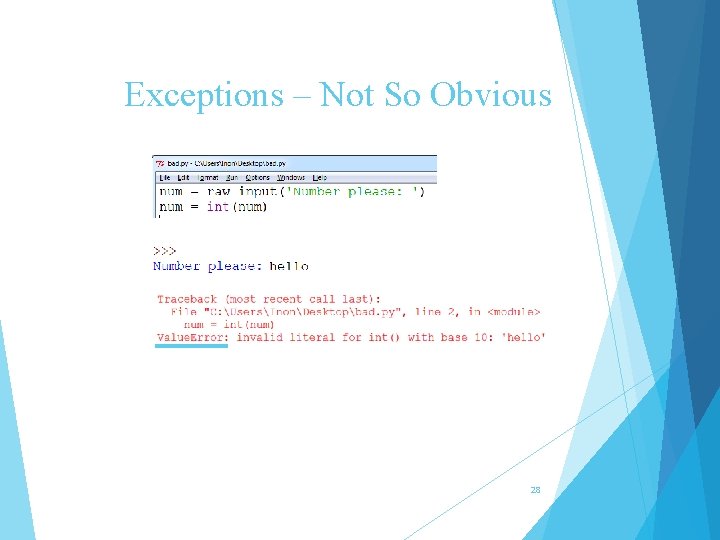
Exceptions – Not So Obvious 28
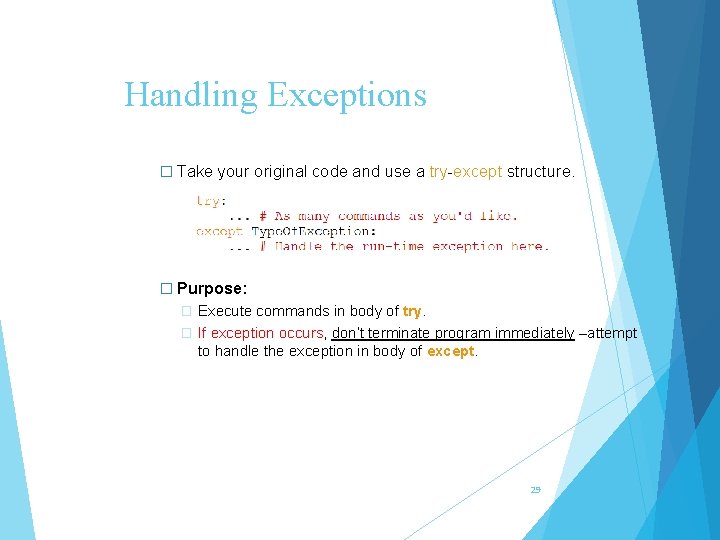
Handling Exceptions � Take your original code and use a try-except structure. � Purpose: � Execute commands in body of try. � If exception occurs, don’t terminate program immediately –attempt to handle the exception in body of except. 29
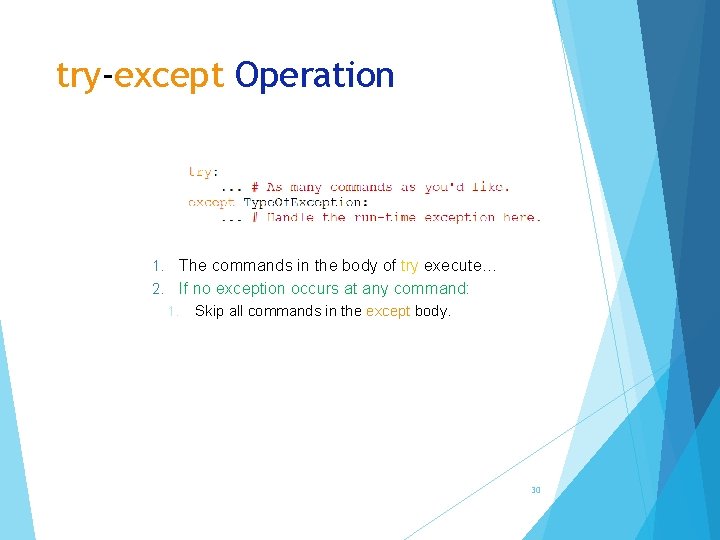
try-except Operation The commands in the body of try execute… 2. If no exception occurs at any command: 1. Skip all commands in the except body. 30
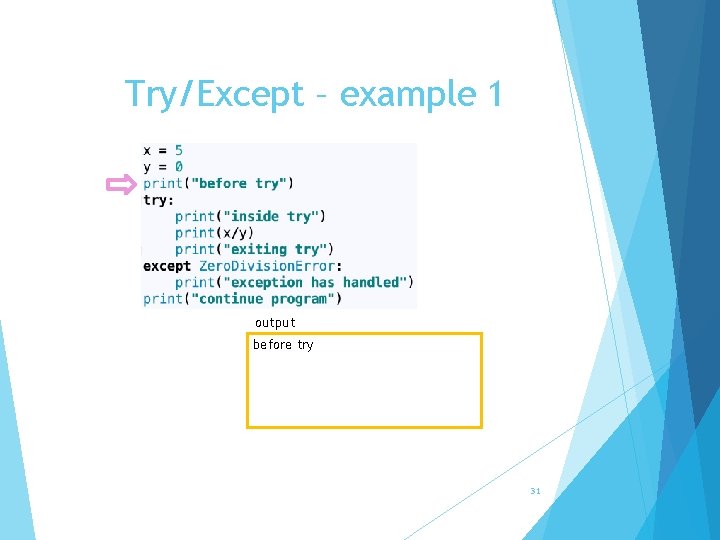
Try/Except – example 1 output before try 31
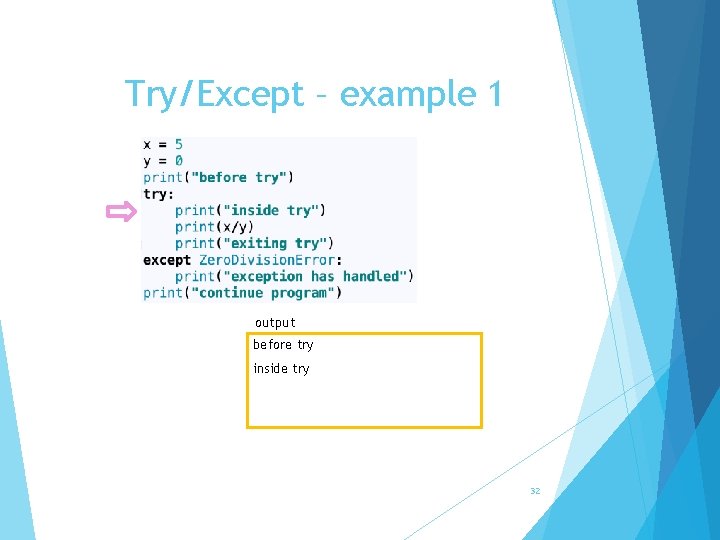
Try/Except – example 1 output before try inside try 32
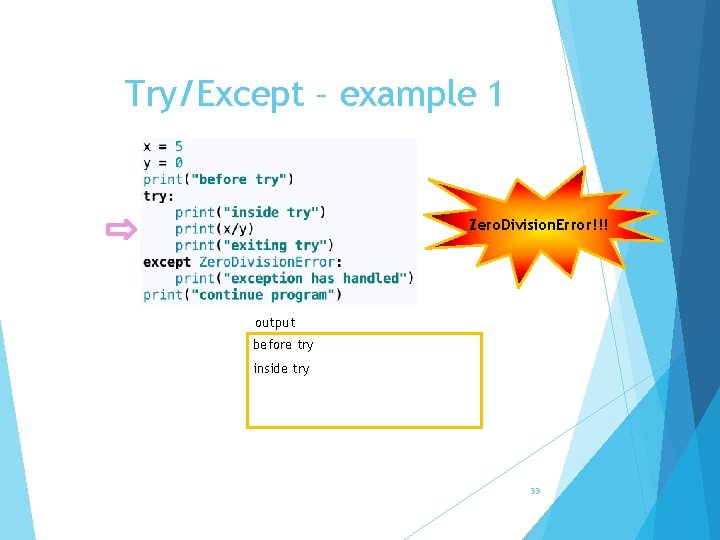
Try/Except – example 1 Zero. Division. Error!!! output before try inside try 33
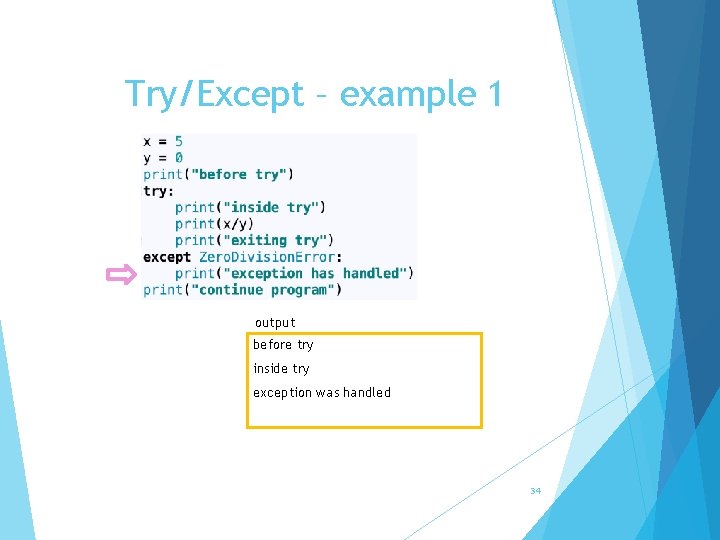
Try/Except – example 1 output before try inside try exception was handled 34
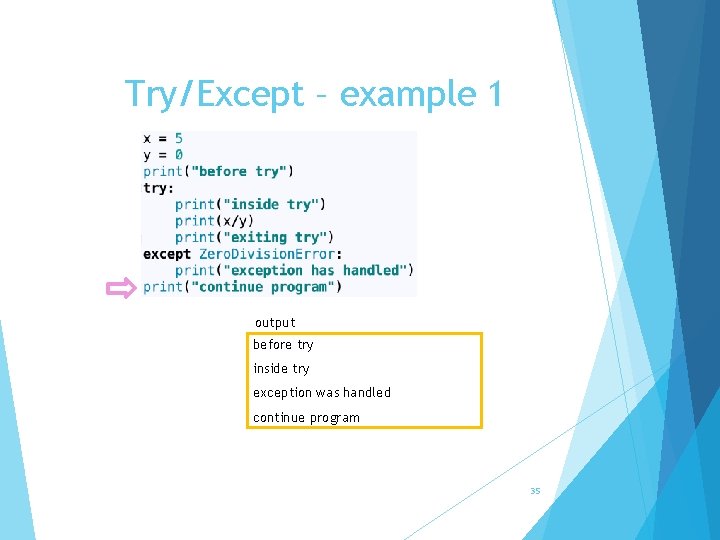
Try/Except – example 1 output before try inside try exception was handled continue program 35
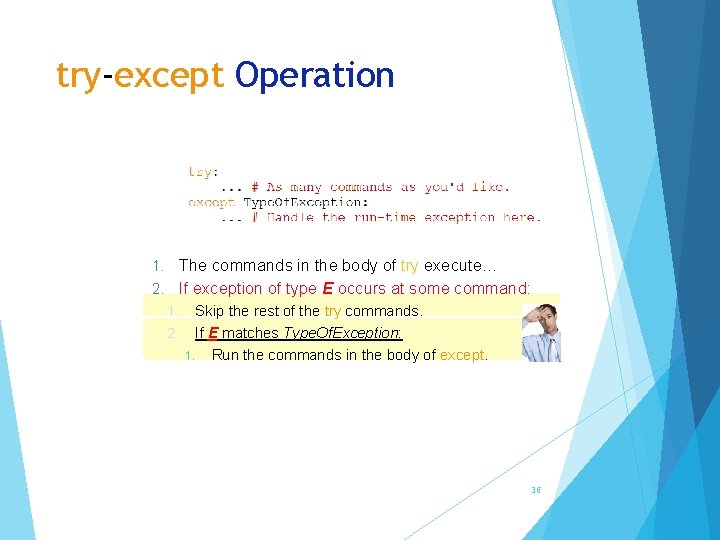
try-except Operation The commands in the body of try execute… 2. If exception of type E occurs at some command: 1. Skip the rest of the try commands. 2. If E matches Type. Of. Exception: 1. Run the commands in the body of except. 1. 36
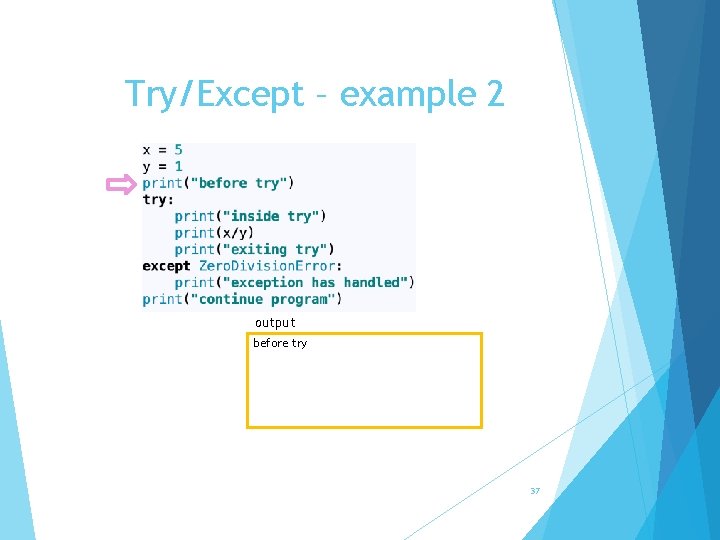
Try/Except – example 2 output before try 37
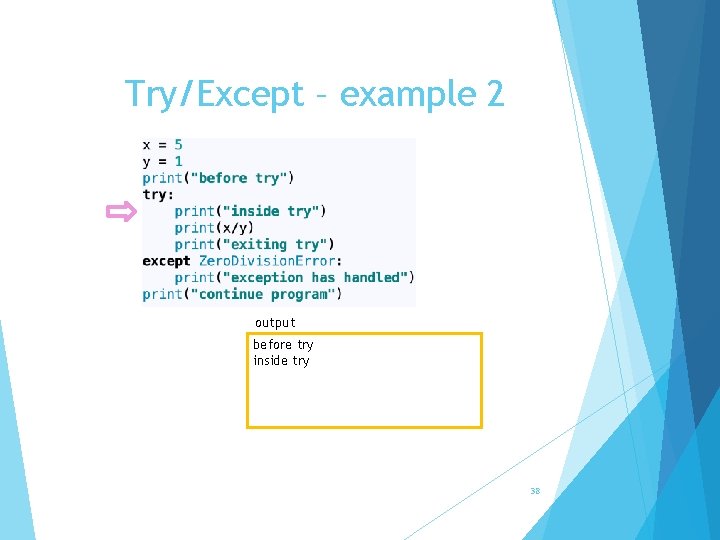
Try/Except – example 2 output before try inside try 38
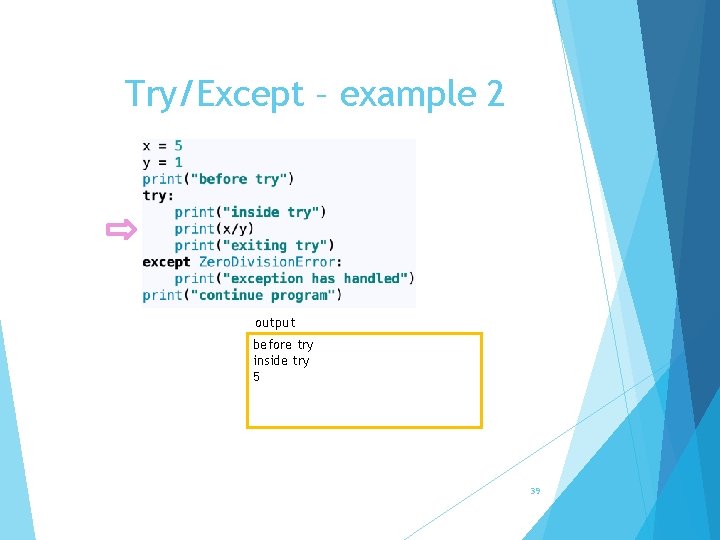
Try/Except – example 2 output before try inside try 5 39
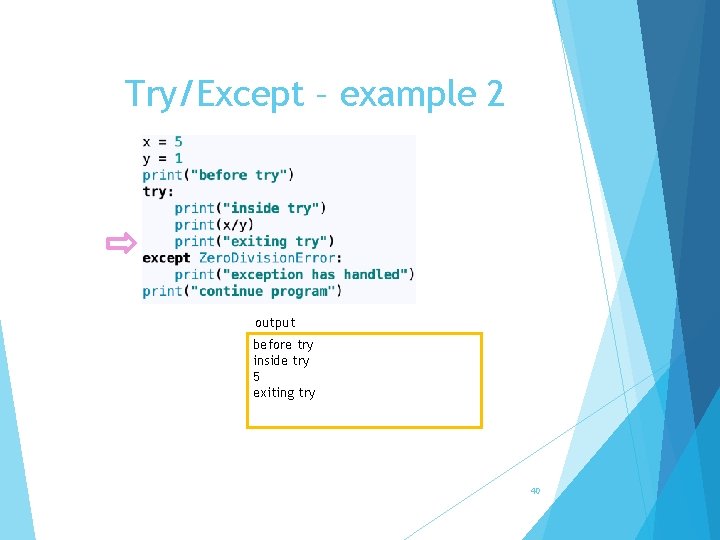
Try/Except – example 2 output before try inside try 5 exiting try 40
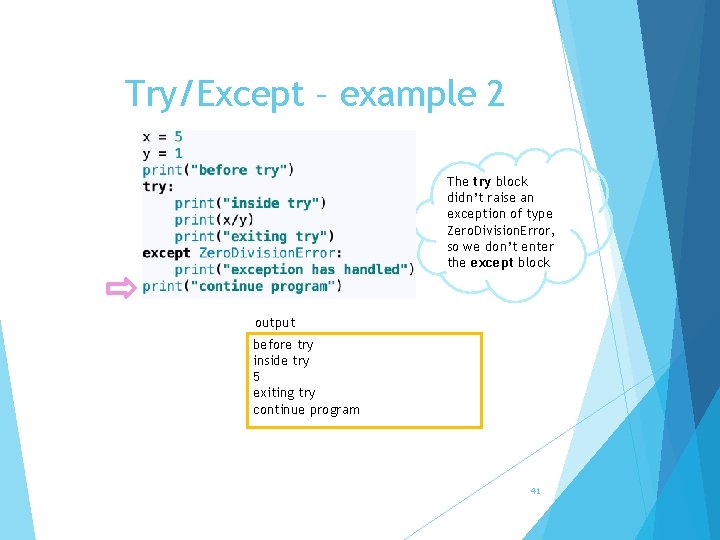
Try/Except – example 2 The try block didn’t raise an exception of type Zero. Division. Error, so we don’t enter the except block output before try inside try 5 exiting try continue program 41
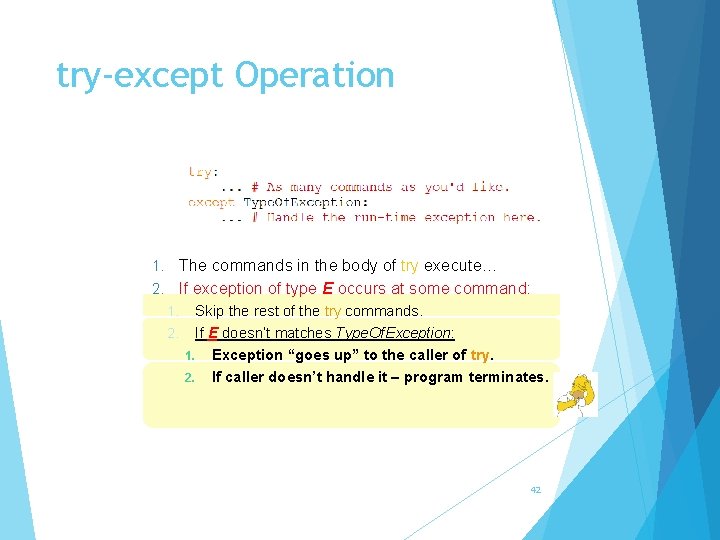
try-except Operation The commands in the body of try execute… 2. If exception of type E occurs at some command: 1. Skip the rest of the try commands. 2. If E doesn’t matches Type. Of. Exception: 1. Exception “goes up” to the caller of try. 2. If caller doesn’t handle it – program terminates. 1. 42
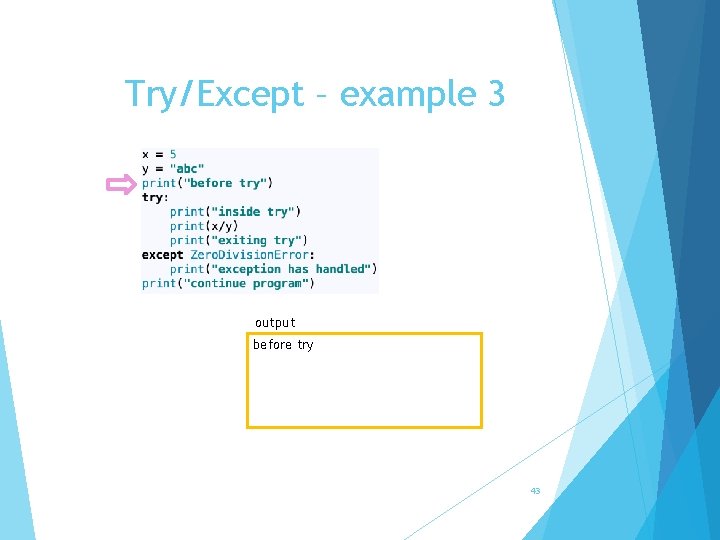
Try/Except – example 3 output before try 43
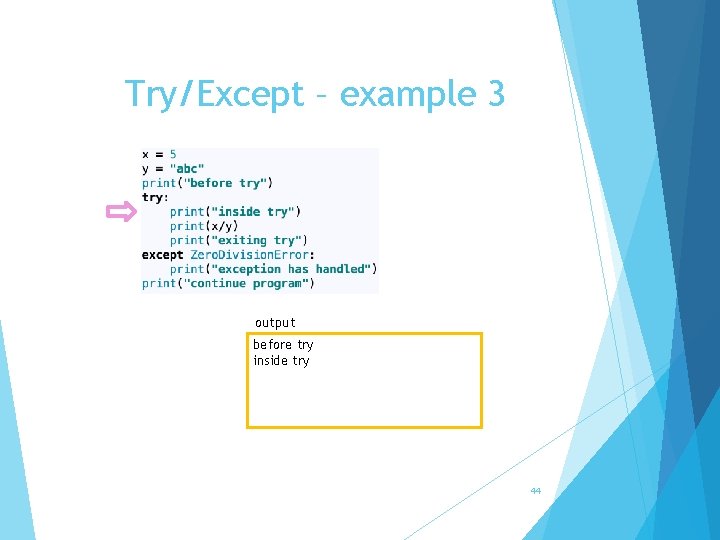
Try/Except – example 3 output before try inside try 44
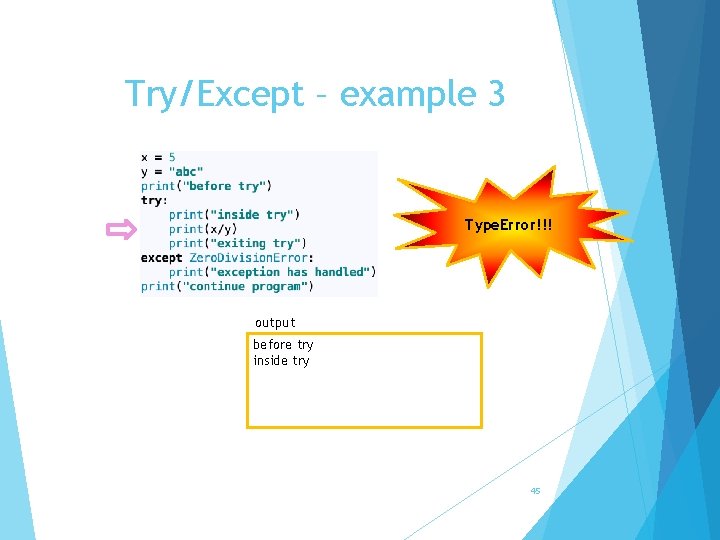
Try/Except – example 3 Type. Error!!! output before try inside try 45
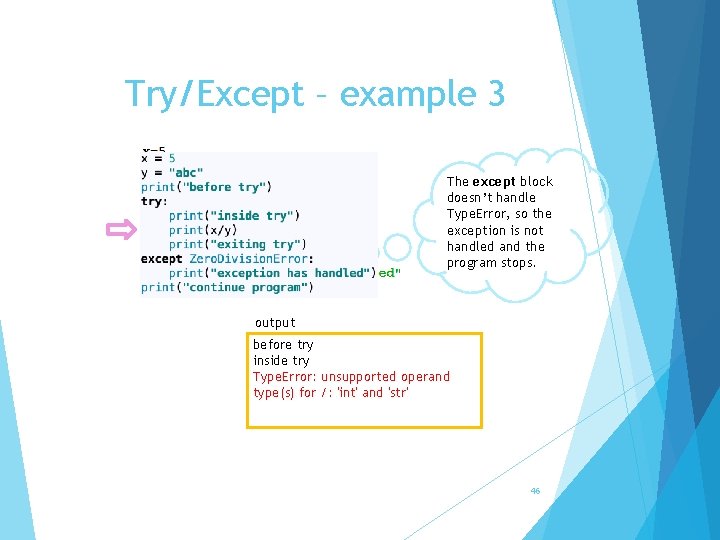
Try/Except – example 3 The except block doesn’t handle Type. Error, so the exception is not handled and the program stops. output before try inside try Type. Error: unsupported operand type(s) for /: 'int' and 'str' 46
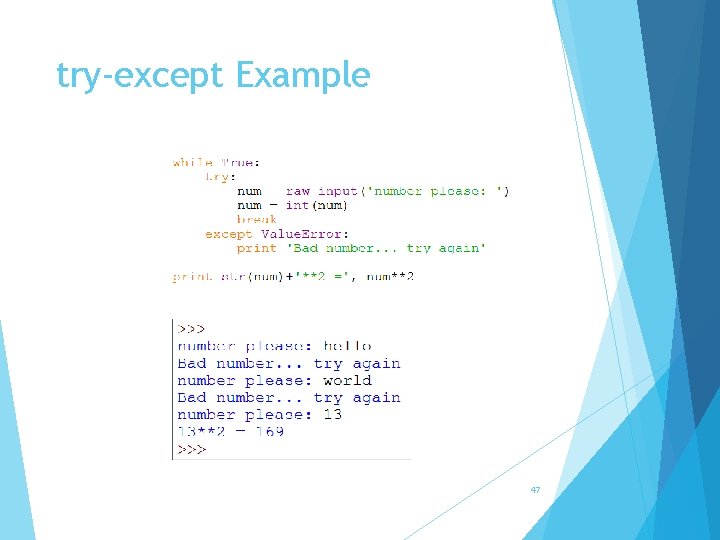
try-except Example 47
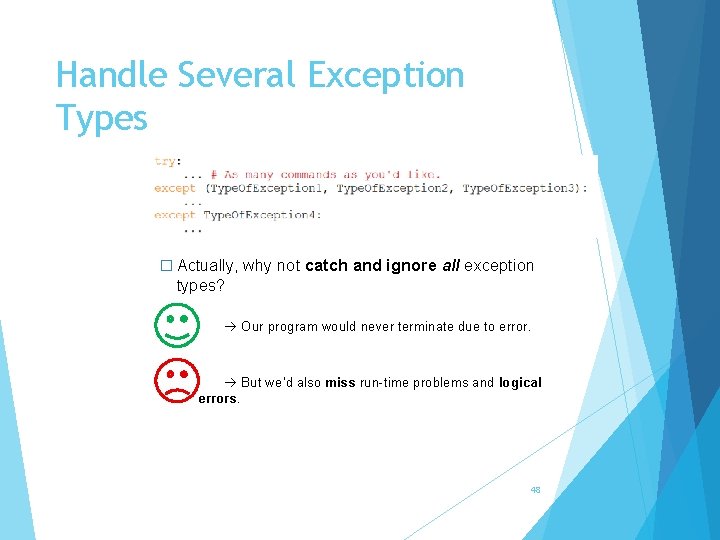
Handle Several Exception Types � Actually, why not catch and ignore all exception types? � � Our program would never terminate due to error. But we’d also miss run-time problems and logical errors. 48
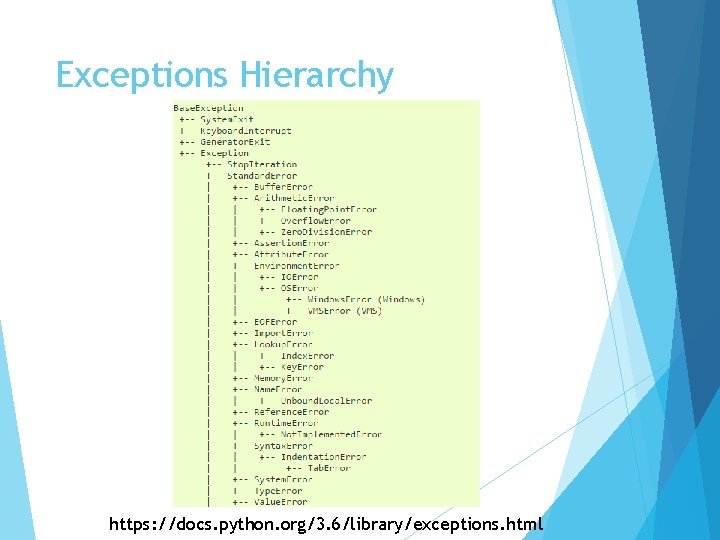
Exceptions Hierarchy https: //docs. python. org/3. 6/library/exceptions. html
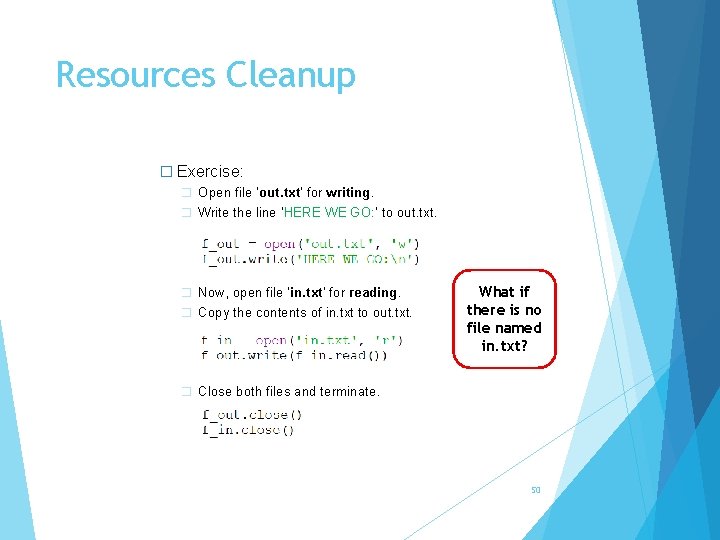
Resources Cleanup � Exercise: � Open file ‘out. txt’ for writing. � Write the line ‘HERE WE GO: ’ to out. txt. � Now, open file ‘in. txt’ for reading. � Copy the contents of in. txt to out. txt. What if there is no file named in. txt? � Close both files and terminate. 50
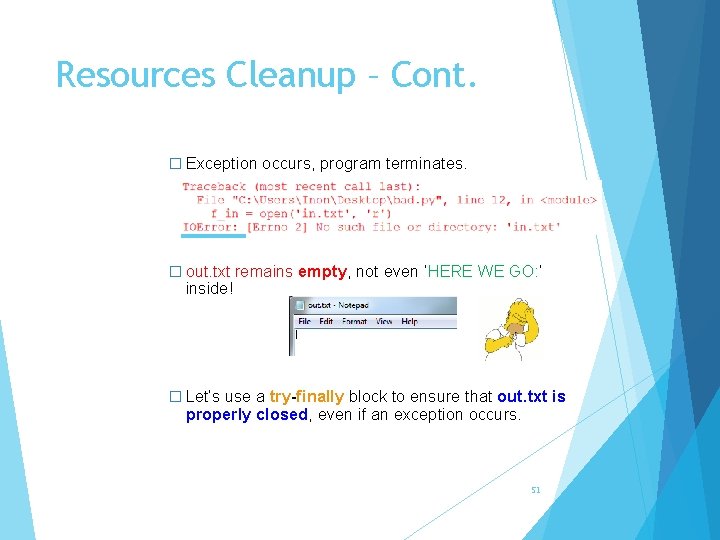
Resources Cleanup – Cont. � Exception occurs, program terminates. � out. txt remains empty, not even ‘HERE WE GO: ’ inside! � Let’s use a try-finally block to ensure that out. txt is properly closed, even if an exception occurs. 51
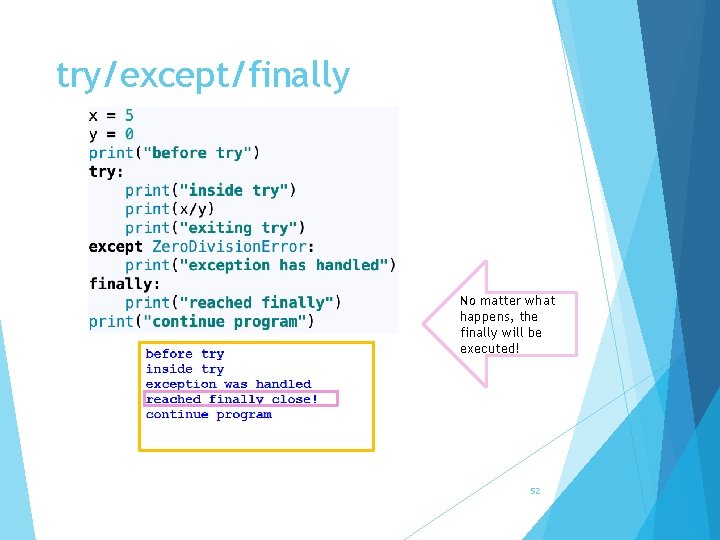
try/except/finally No matter what happens, the finally will be executed! 52
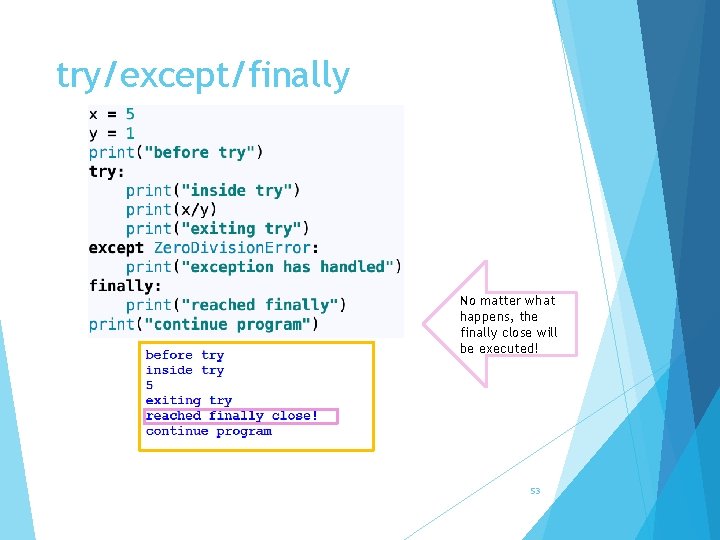
try/except/finally No matter what happens, the finally close will be executed! 53
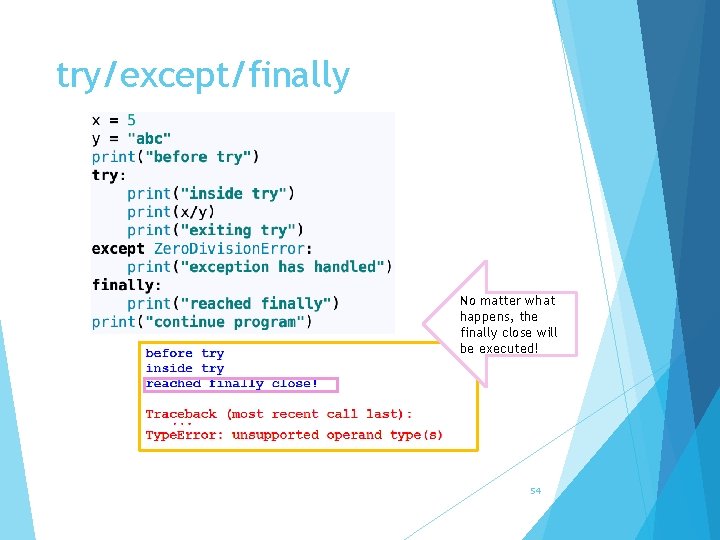
try/except/finally No matter what happens, the finally close will be executed! 54
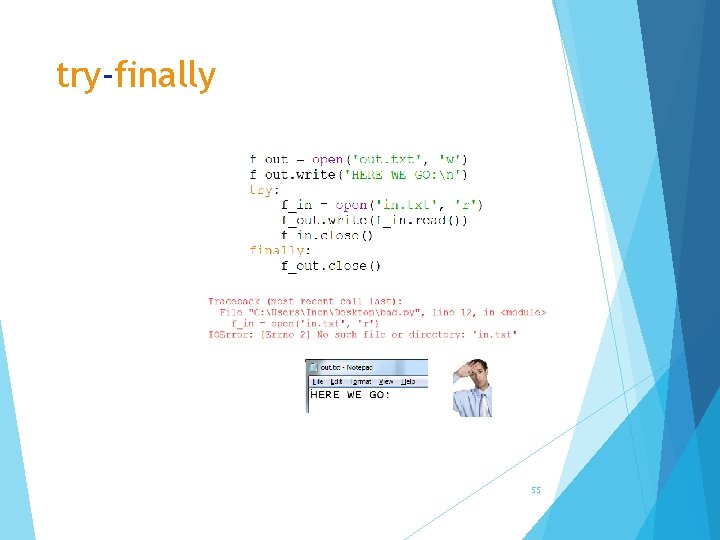
try-finally 55
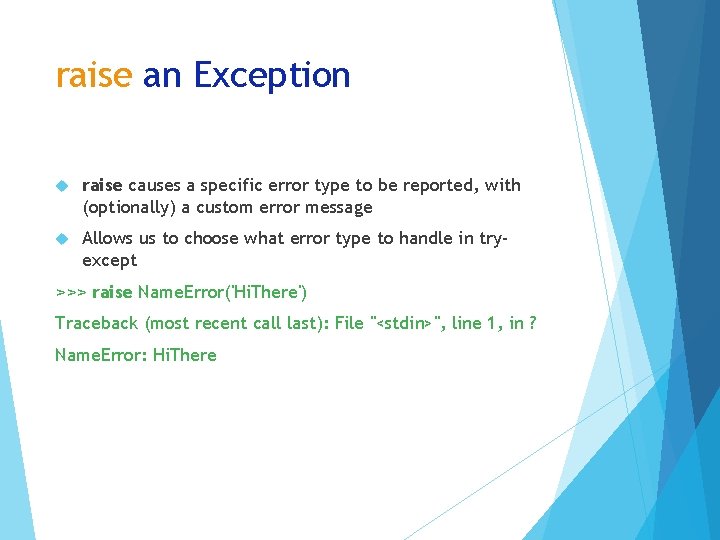
raise an Exception raise causes a specific error type to be reported, with (optionally) a custom error message Allows us to choose what error type to handle in tryexcept >>> raise Name. Error('Hi. There') Traceback (most recent call last): File "<stdin>", line 1, in ? Name. Error: Hi. There
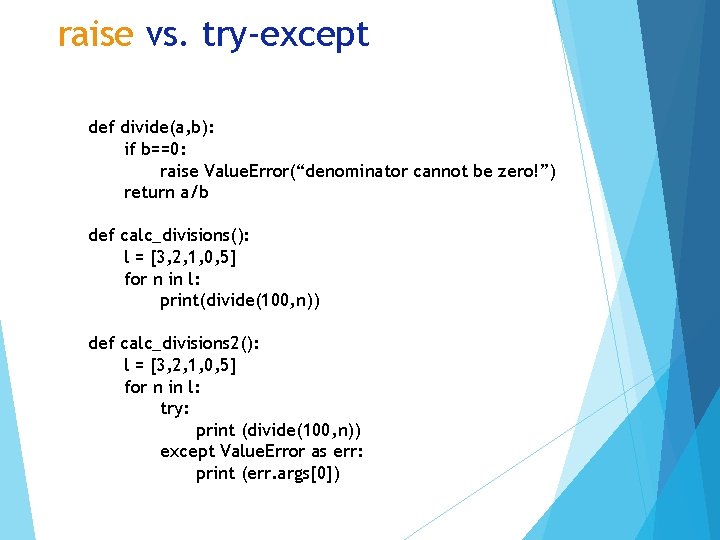
raise vs. try-except def divide(a, b): if b==0: raise Value. Error(“denominator cannot be zero!”) return a/b def calc_divisions(): l = [3, 2, 1, 0, 5] for n in l: print(divide(100, n)) def calc_divisions 2(): l = [3, 2, 1, 0, 5] for n in l: try: print (divide(100, n)) except Value. Error as err: print (err. args[0])
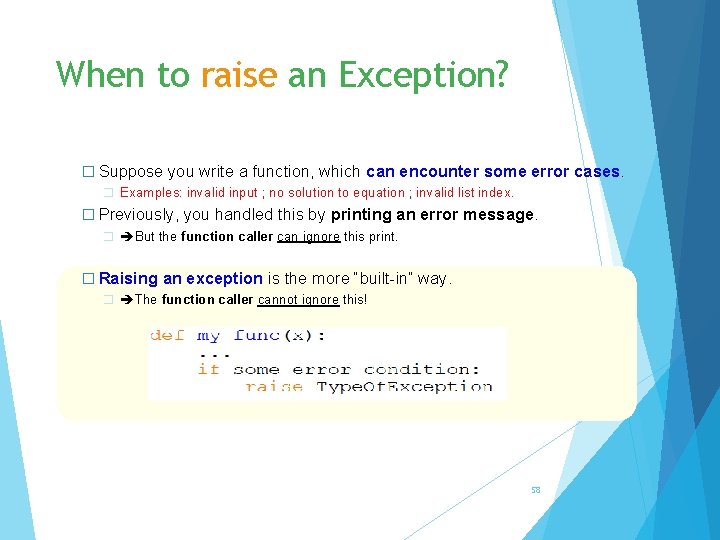
When to raise an Exception? � Suppose you write a function, which can encounter some error cases. � Examples: invalid input ; no solution to equation ; invalid list index. � Previously, you handled this by printing an error message. � But the function caller can ignore this print. � Raising an exception is the more “built-in” way. � The function caller cannot ignore this! 58
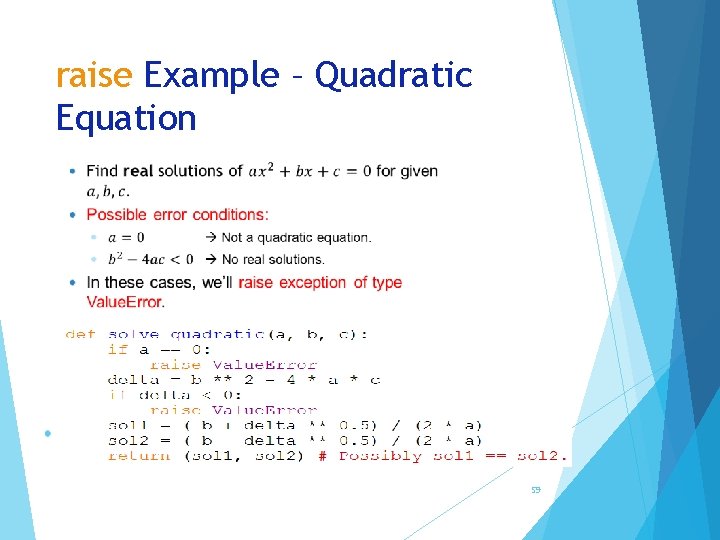
raise Example – Quadratic Equation 59
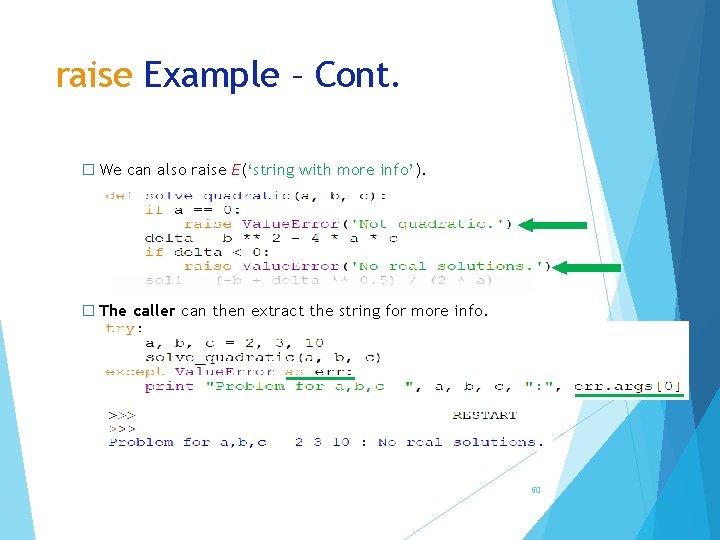
raise Example – Cont. � We can also raise E(‘string with more info’). � The caller can then extract the string for more info. 60
Multipicand
Factor label definition
Multipicand
Practical session definition
Python programming in context
Python blackjack oop
Programming essentials in python
Python programming in context
Python cgi form example
Procedural programming python
Python programming an introduction to computer science
Constraint programming python
Audiolab python
Python chapter 5 programming exercises
Rapid gui programming with python and qt
Python programming
Second order cone programming python
Definition of system programming
Linear vs integer programming
Perbedaan linear programming dan integer programming
Programing adalah
Greedy vs dynamic
Gradle intro
Math ia checklist
Example of introduction paragraph
Motifs in fahrenheit 451
Estados de la materia intro
Introduction to hrm
Dns in computer networks
Mgm intro
Introduction to mice
Logo pengantar bisnis
Floor hockey positions
Slidetodoc.com
Intro to cryptography
Bridge in introduction paragraph
Alter introduction templates
Introduction body conclusion example
The image characteristics are ____.
Ap us government and politics unit 1 study guide
Intro to polyamory
States of matter
Plan de dissertation
Intro body conclusion
Intro to ecology
Essay structure
Intro to vlsi
Intro section on facebook
What is a thematic essay
Intro to vlsi
Vanitas intro
Intro to business chapter 7
Piecewise function generator
Introduction to business chapter 8
Random forest intro
Makeup intro
Intro to ecosystems
Cengage intro to business
Severance intro
Intro to parabolas
Andrew ng intro machine learning