Intro to Programming with Python Practical Session 12
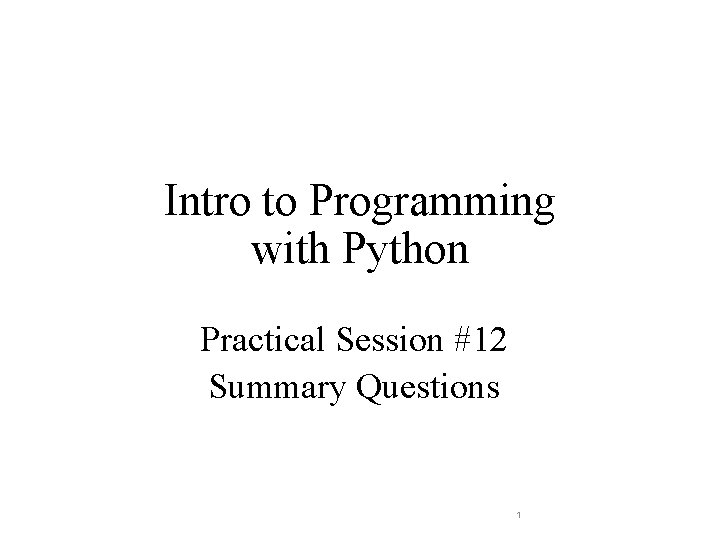
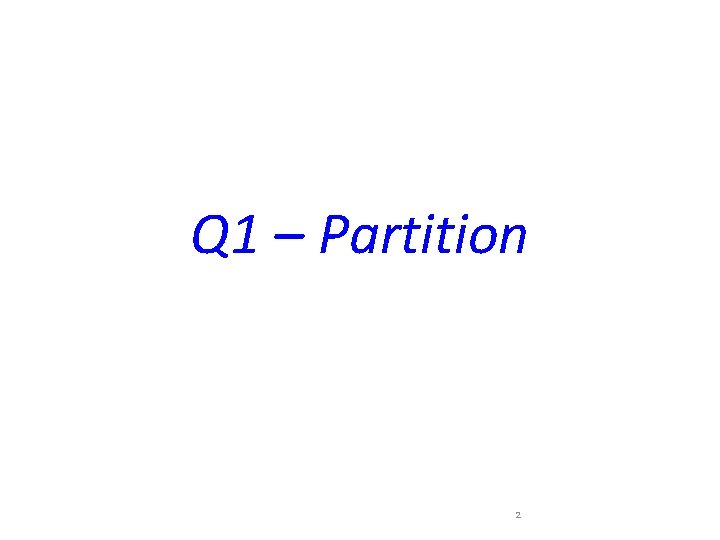
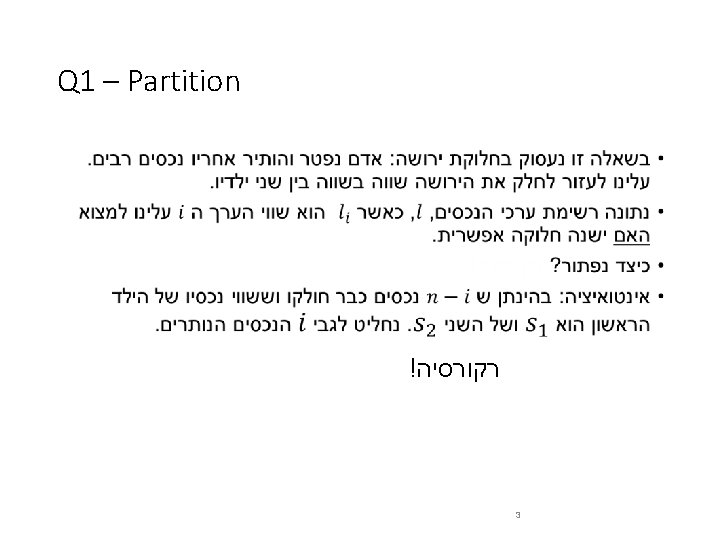
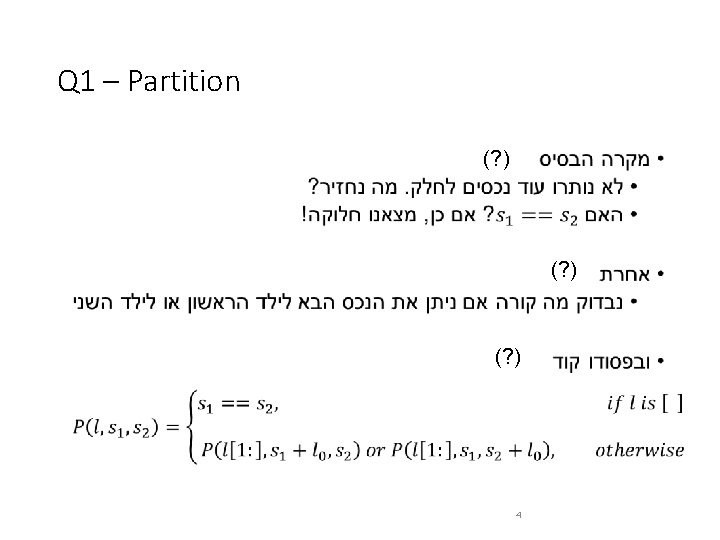
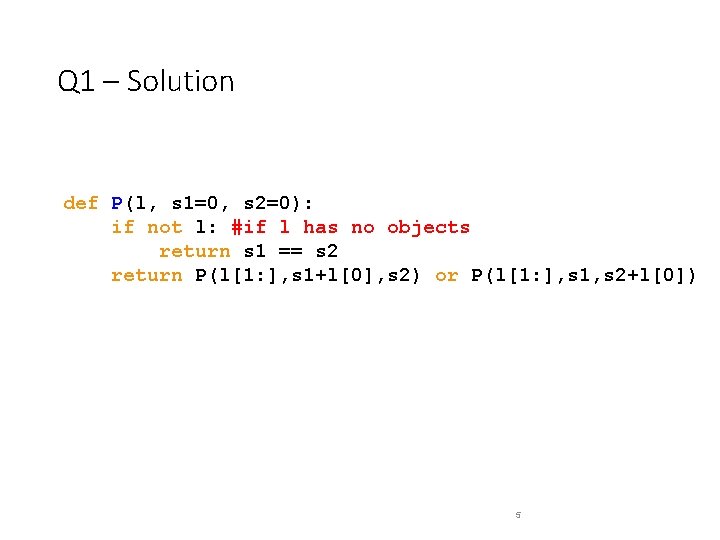
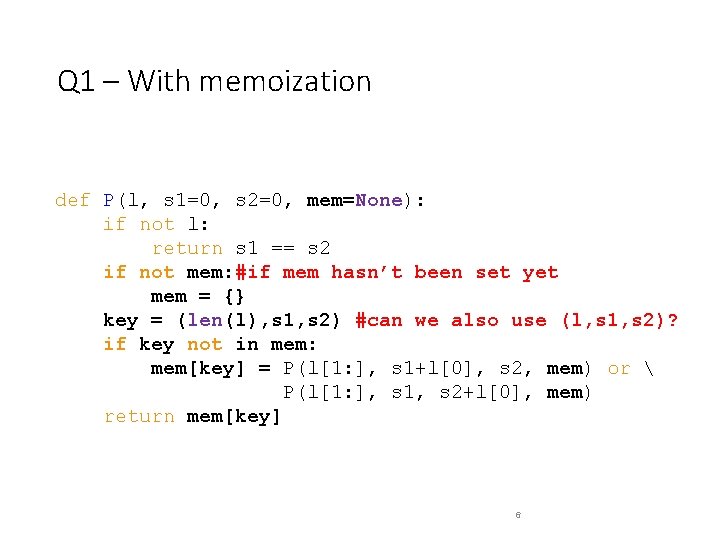
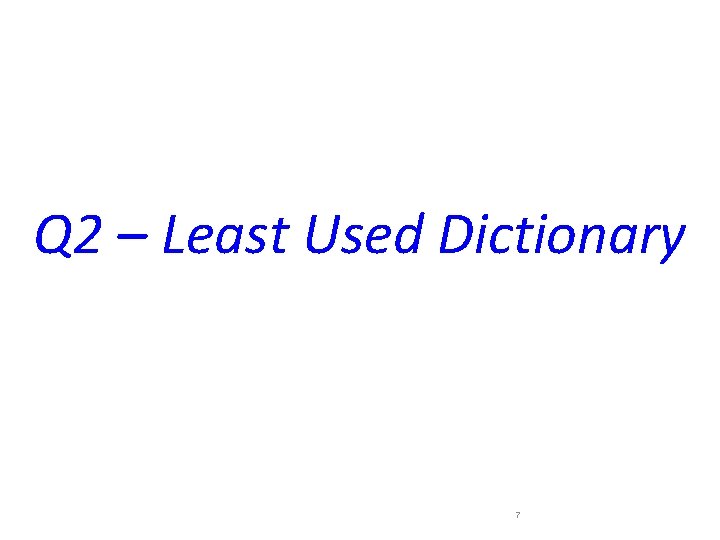
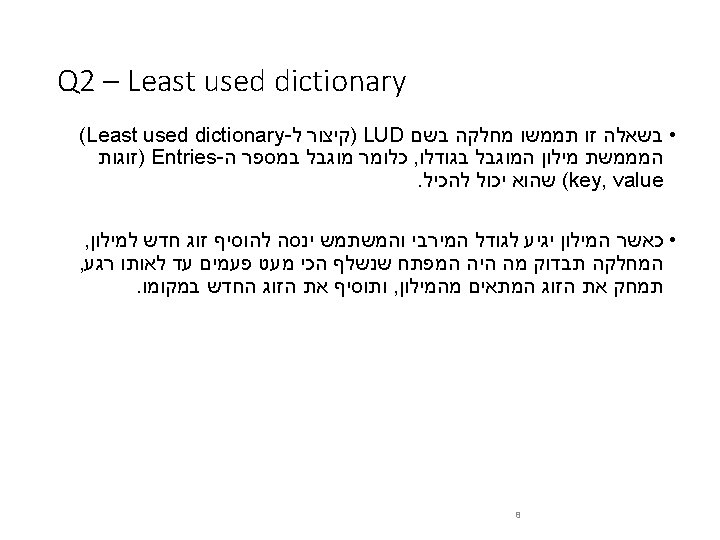
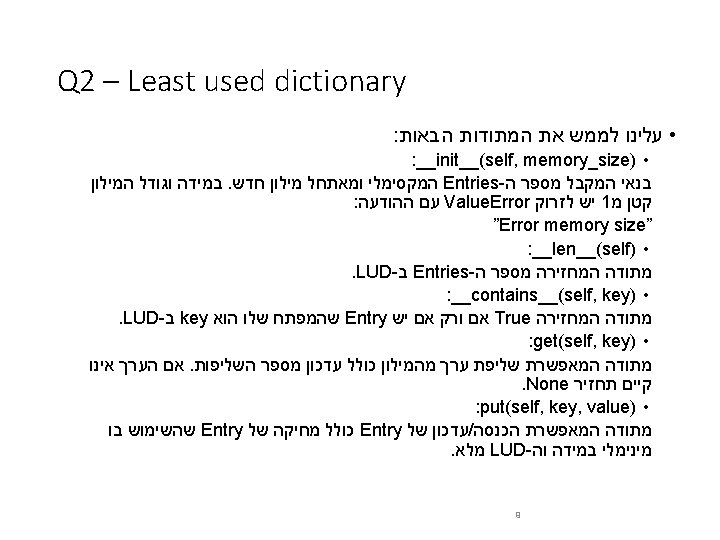
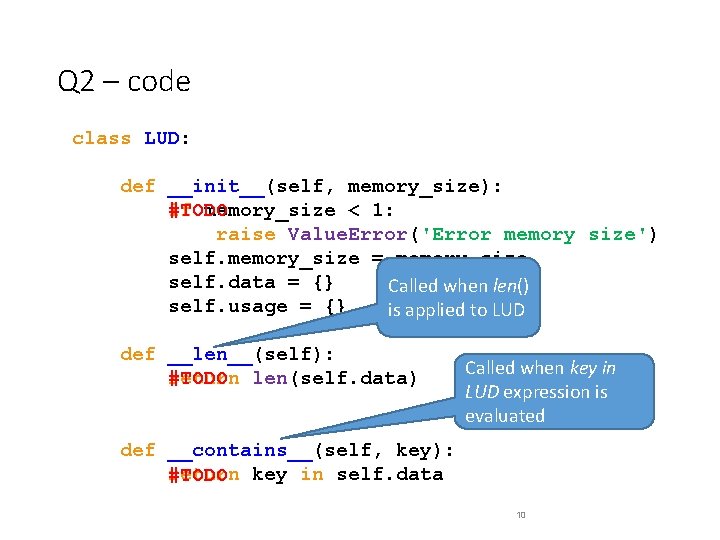
![Q 2 – code def extract_value(key_value): return key_value[1] class LUD: . . . Outside Q 2 – code def extract_value(key_value): return key_value[1] class LUD: . . . Outside](https://slidetodoc.com/presentation_image_h2/72dfd19d16aca756bf20c6c6edd6f229/image-11.jpg)
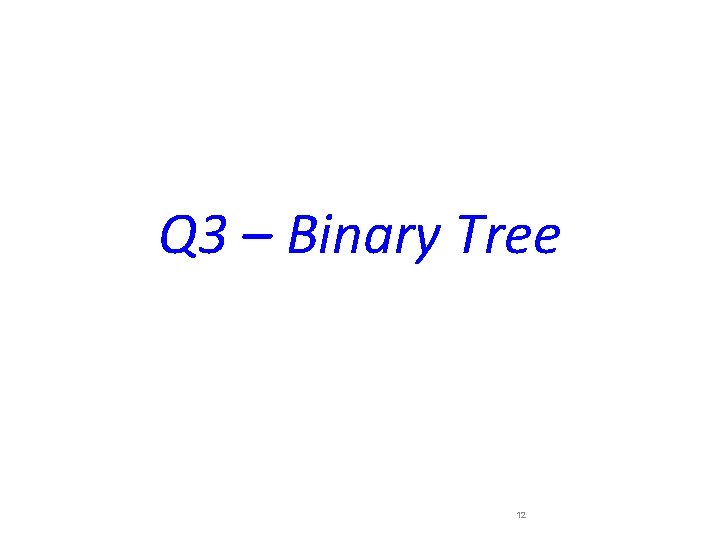
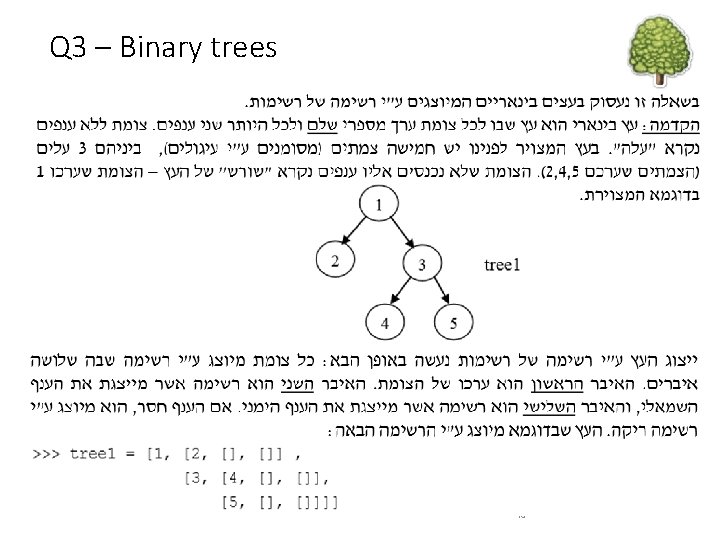
![Q 3 – Binary trees def is_leaf(tree): #TODO return not tree[1] and not tree[2] Q 3 – Binary trees def is_leaf(tree): #TODO return not tree[1] and not tree[2]](https://slidetodoc.com/presentation_image_h2/72dfd19d16aca756bf20c6c6edd6f229/image-14.jpg)
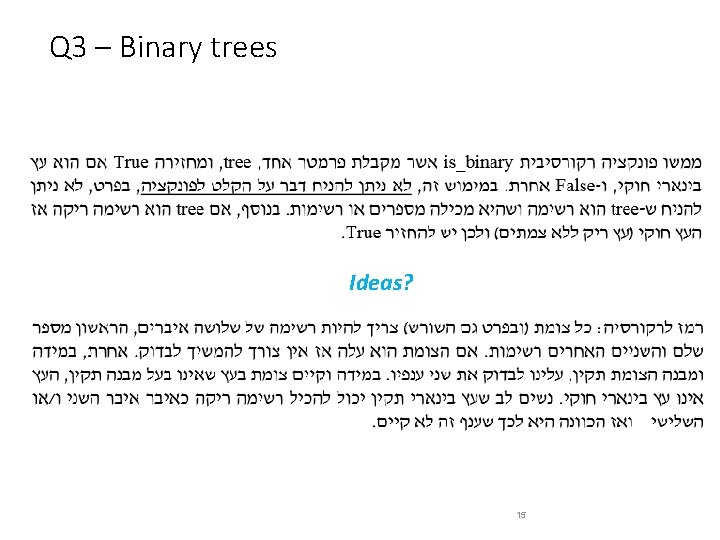
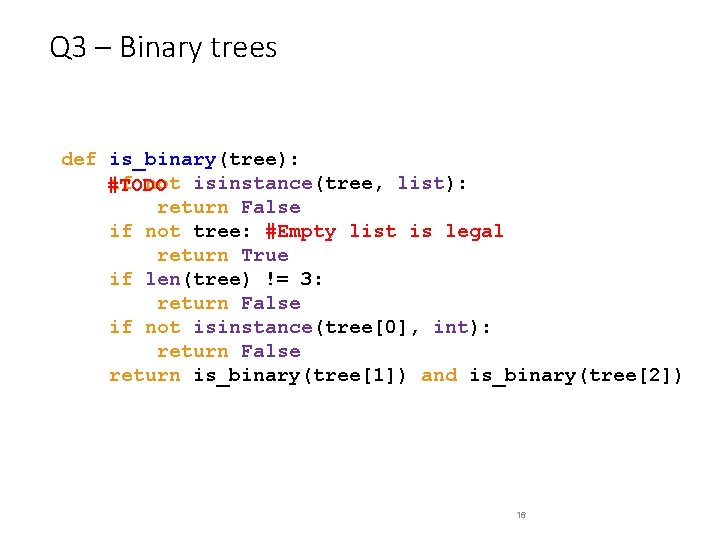
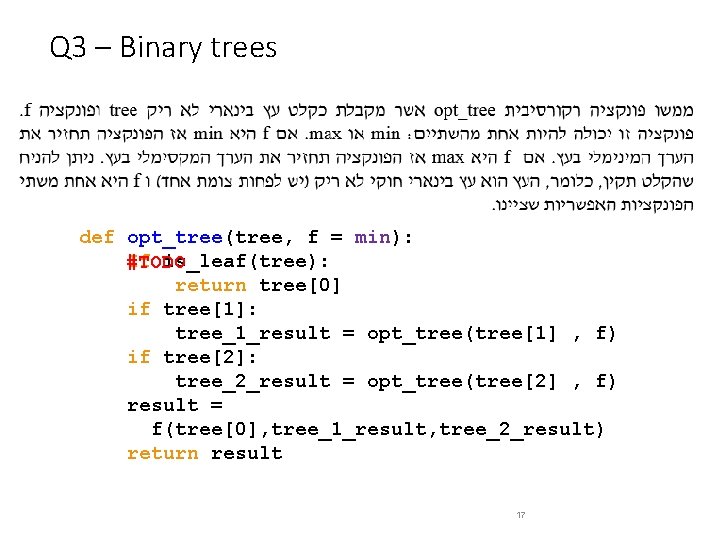
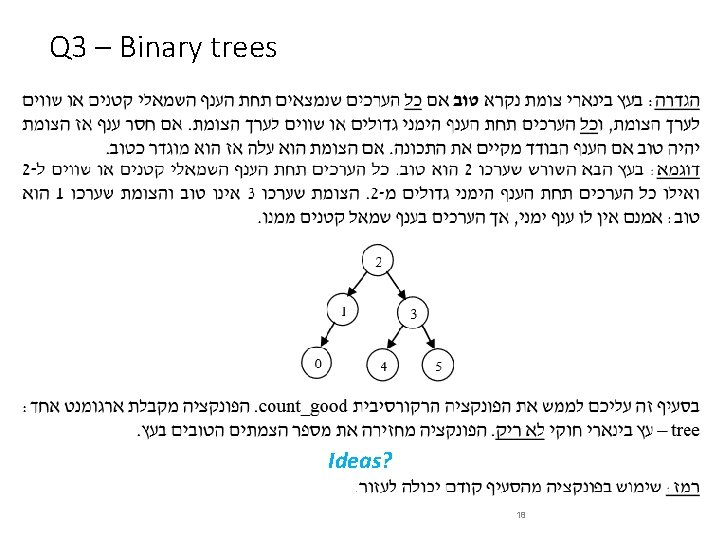
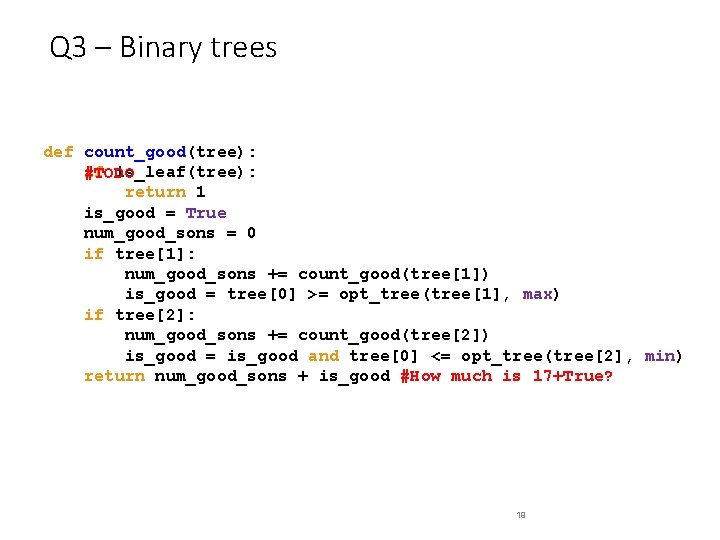
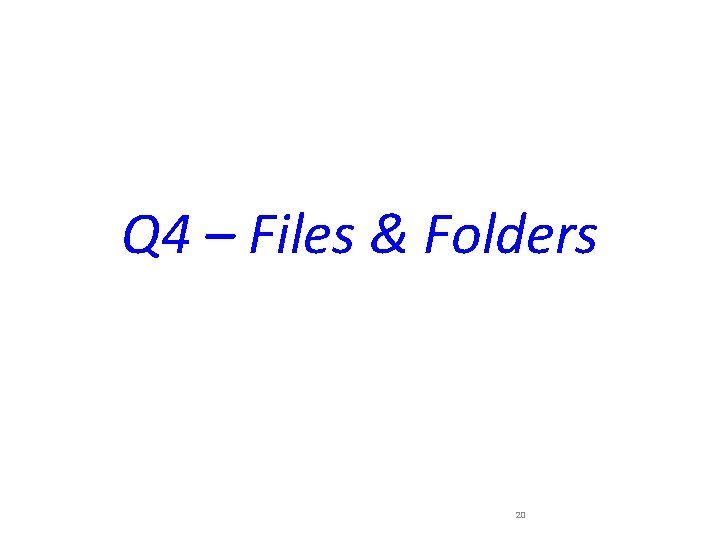
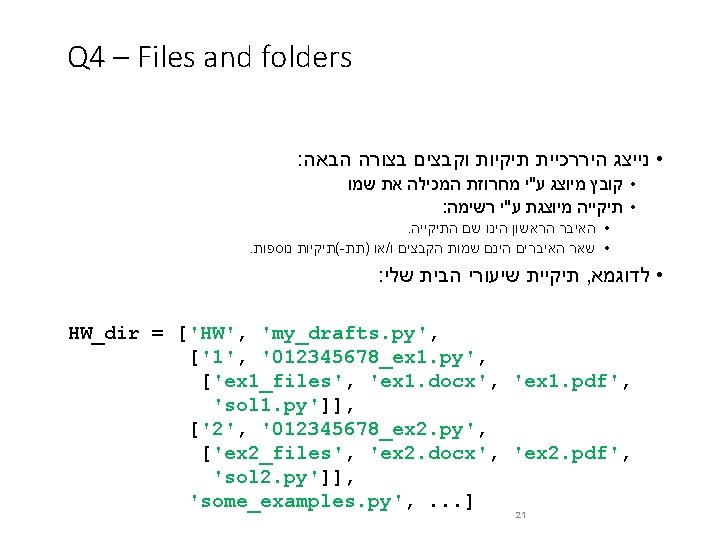
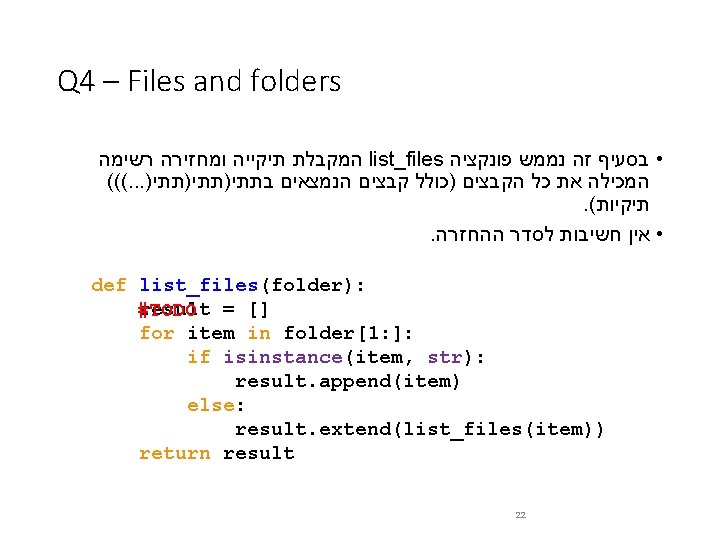
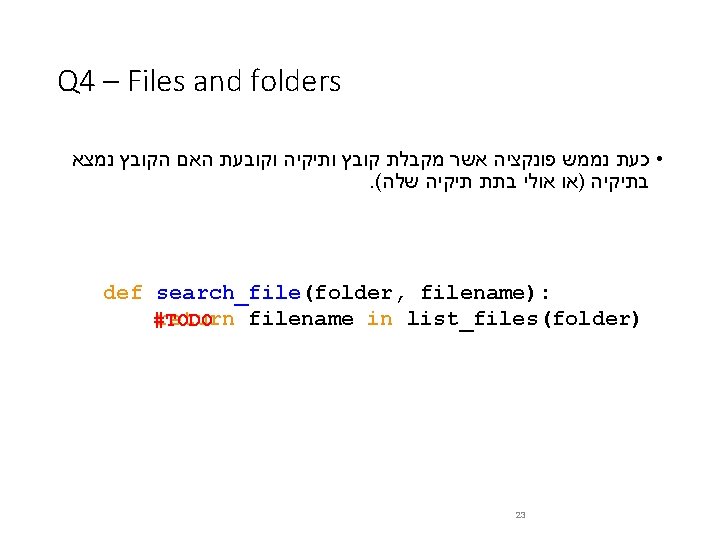
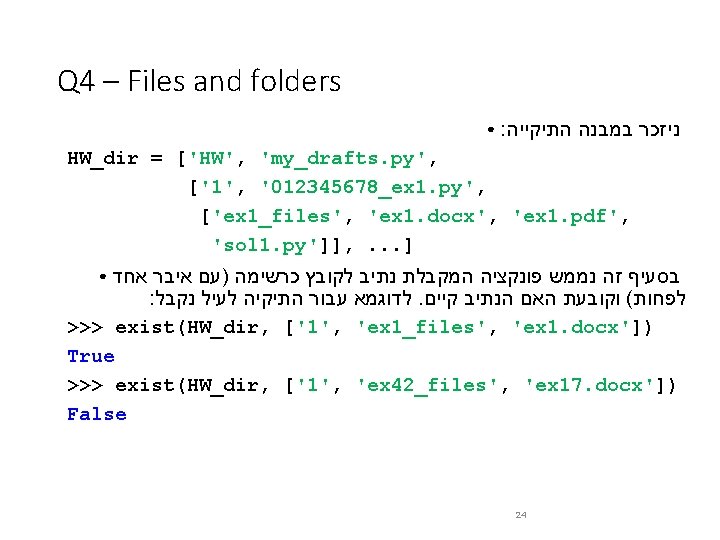
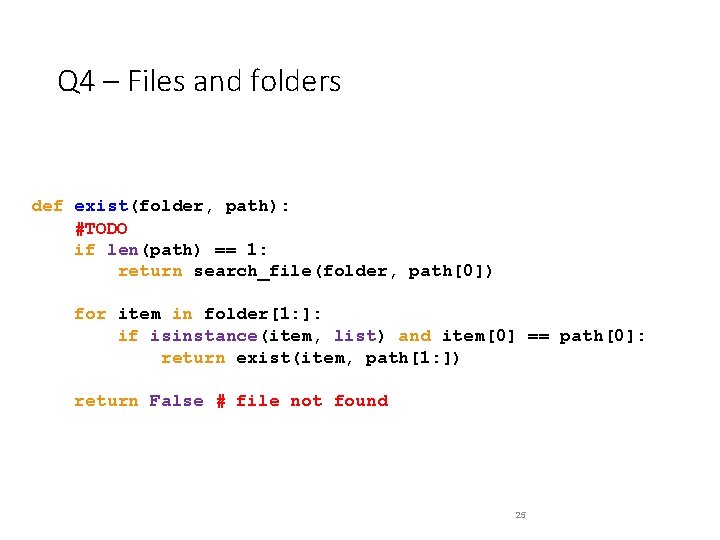
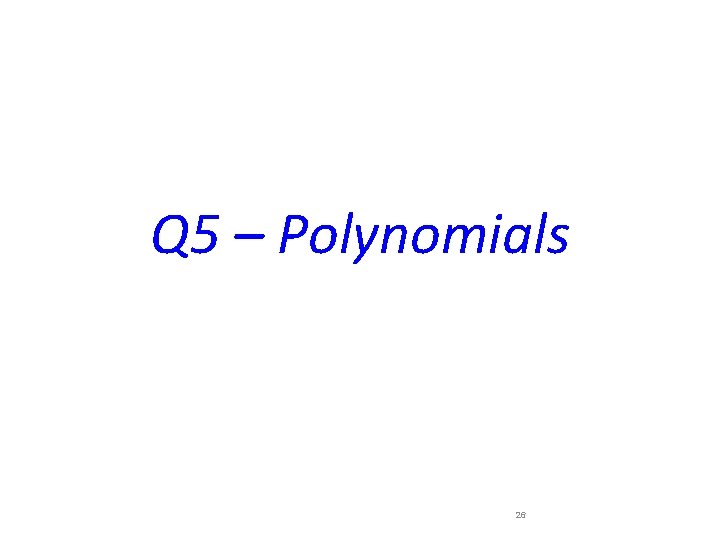
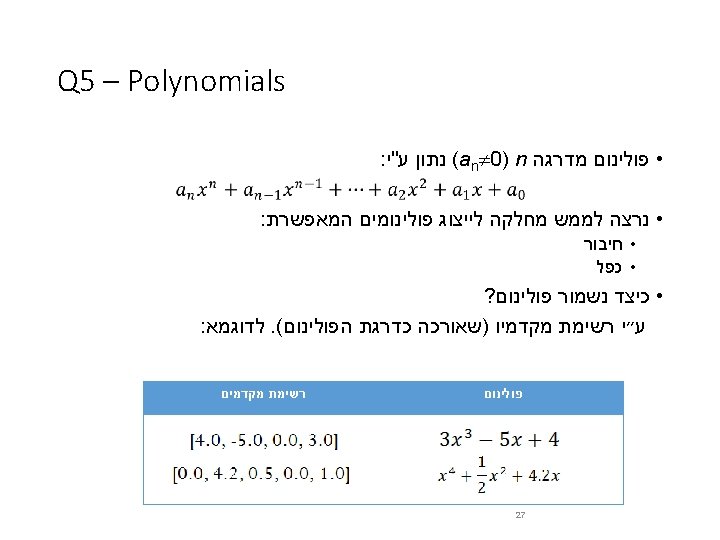
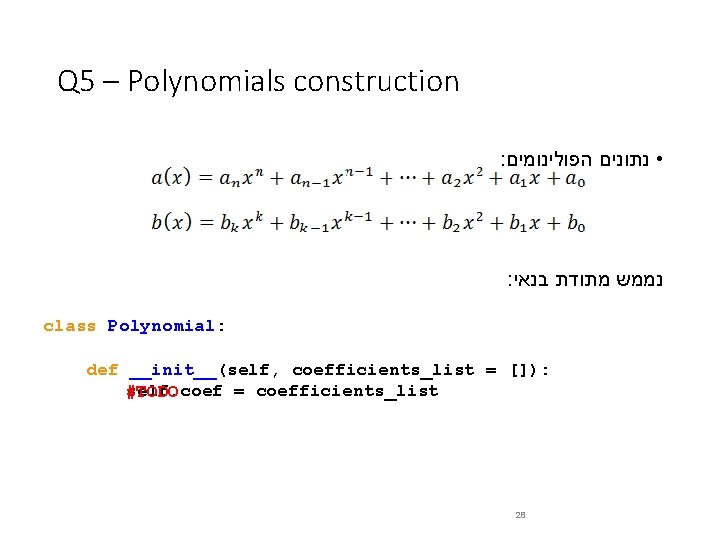
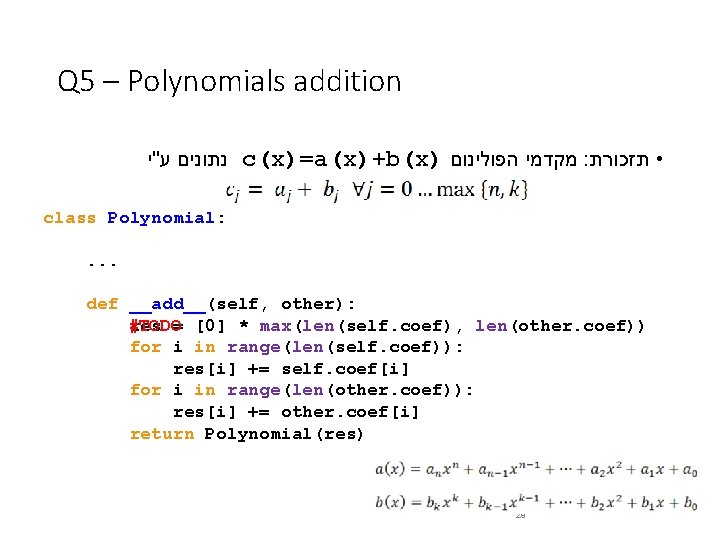
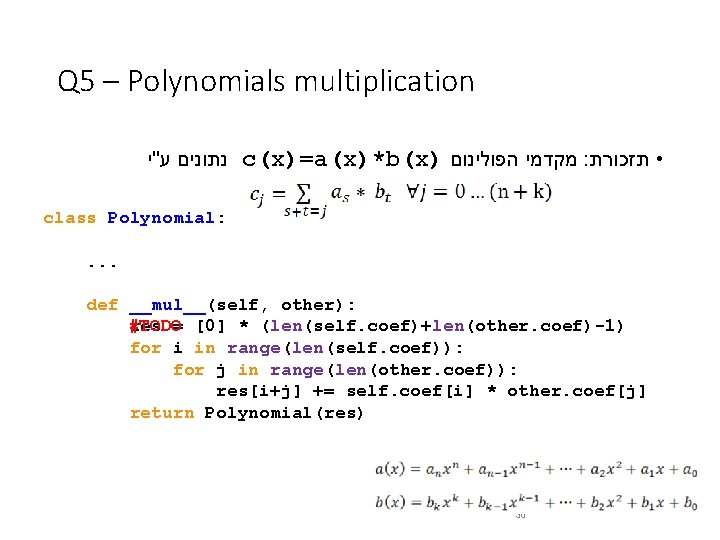
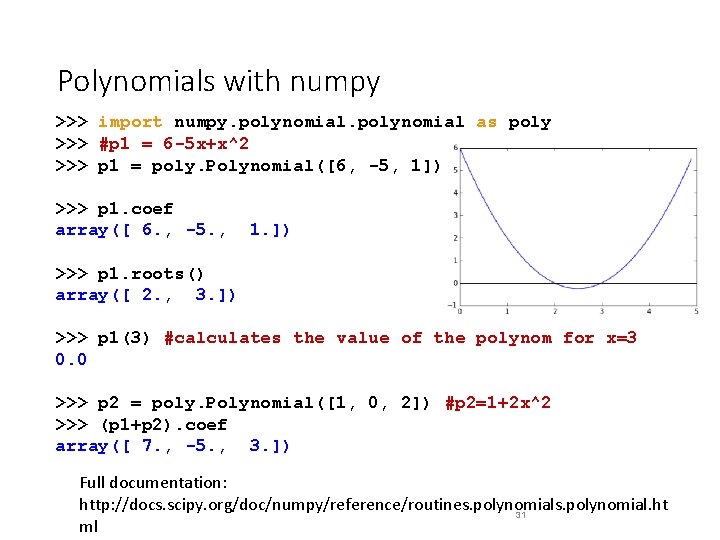
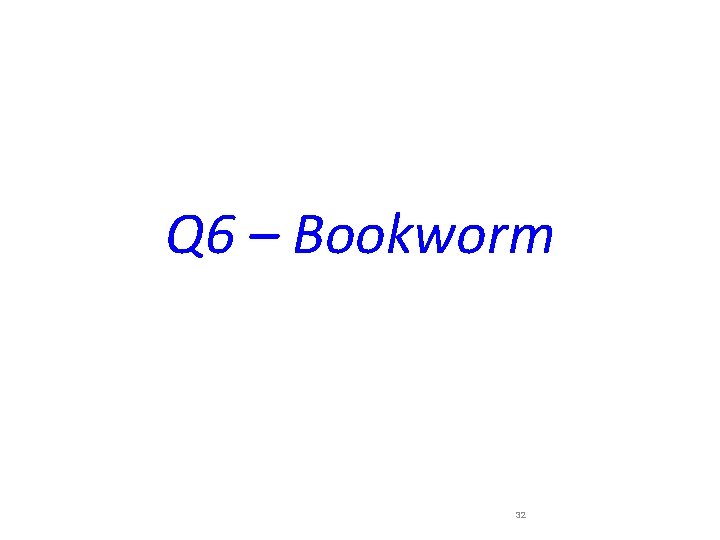
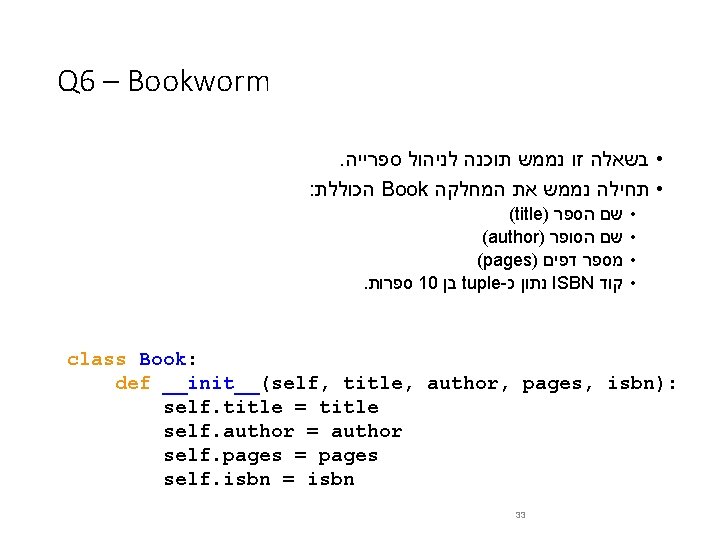
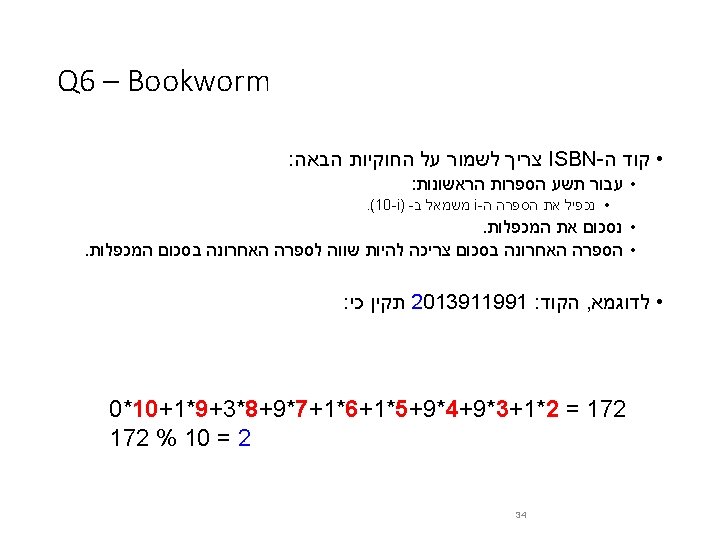
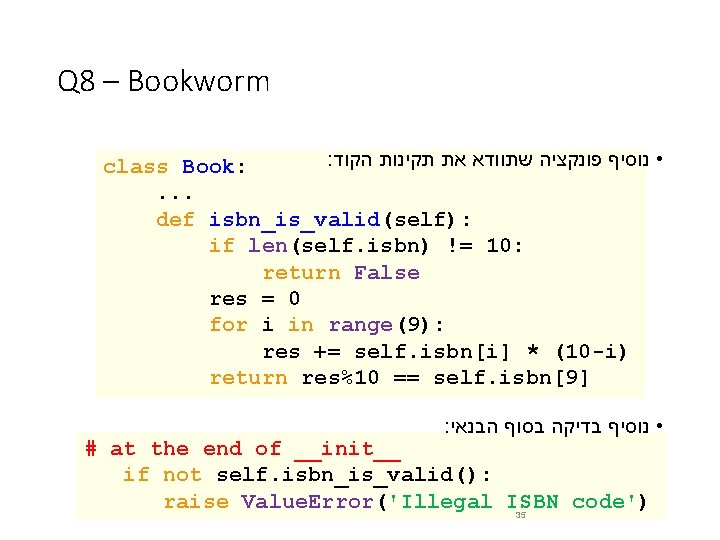
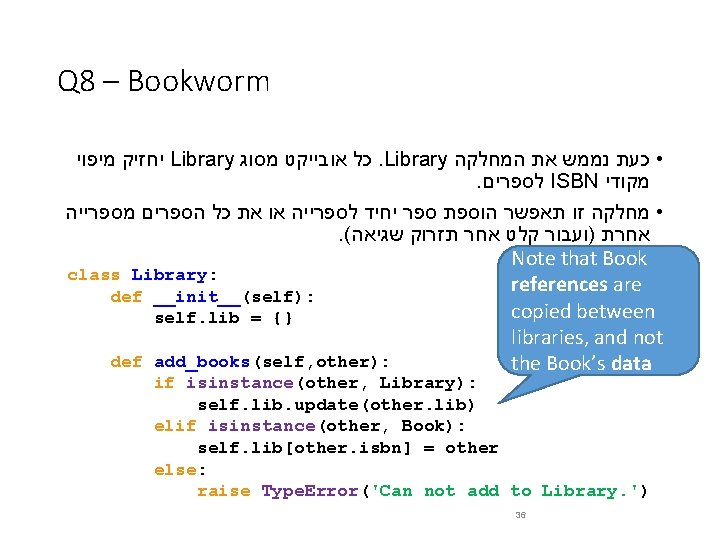
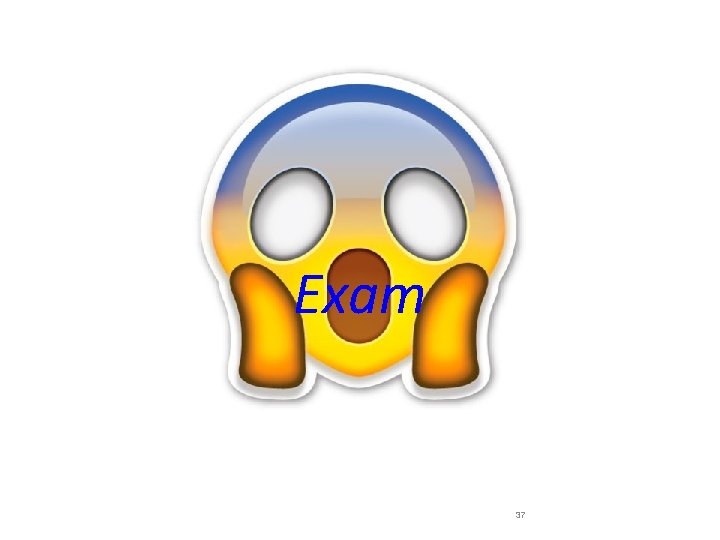
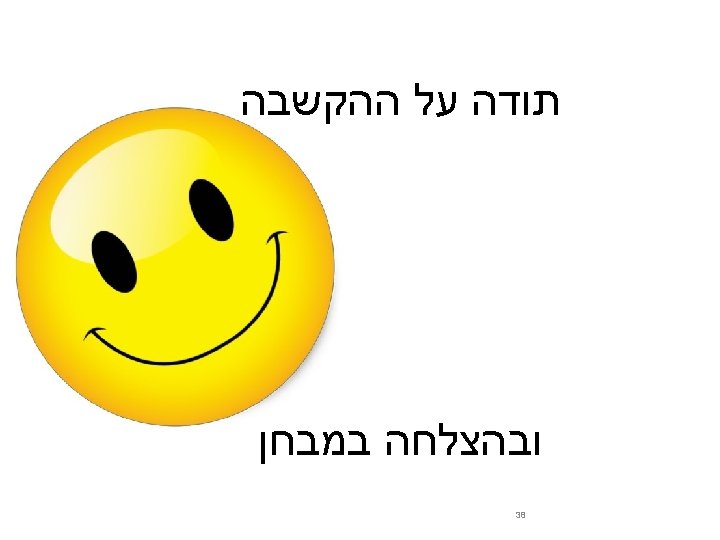
- Slides: 38
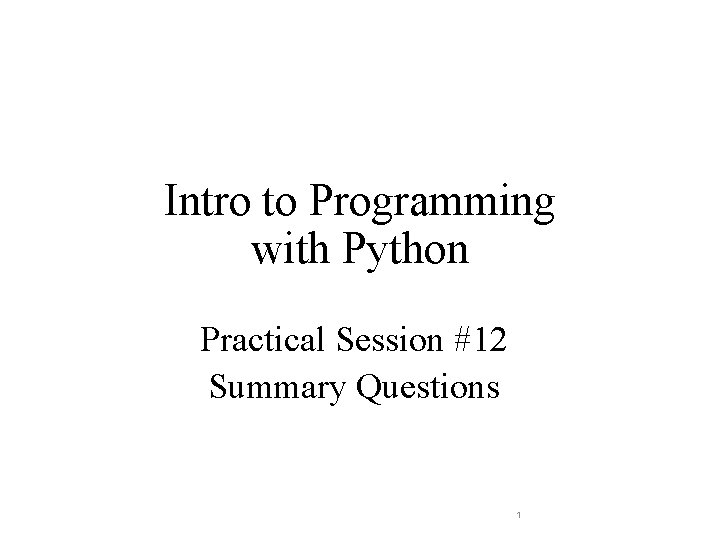
Intro to Programming with Python Practical Session #12 Summary Questions 1
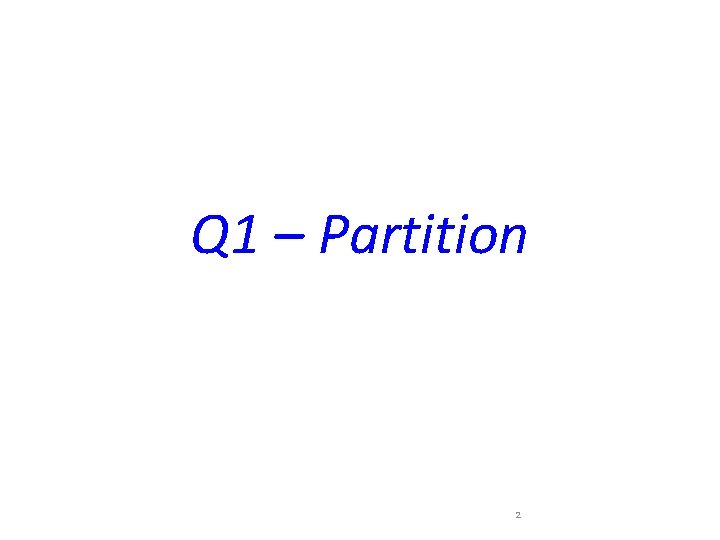
Q 1 – Partition 2
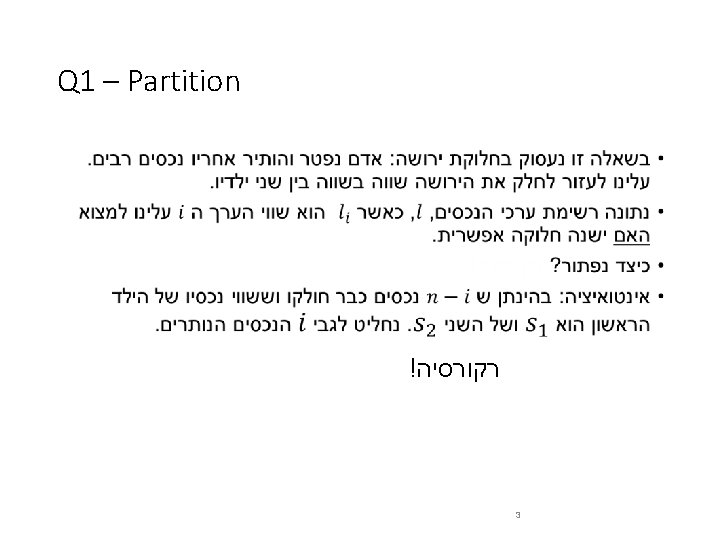
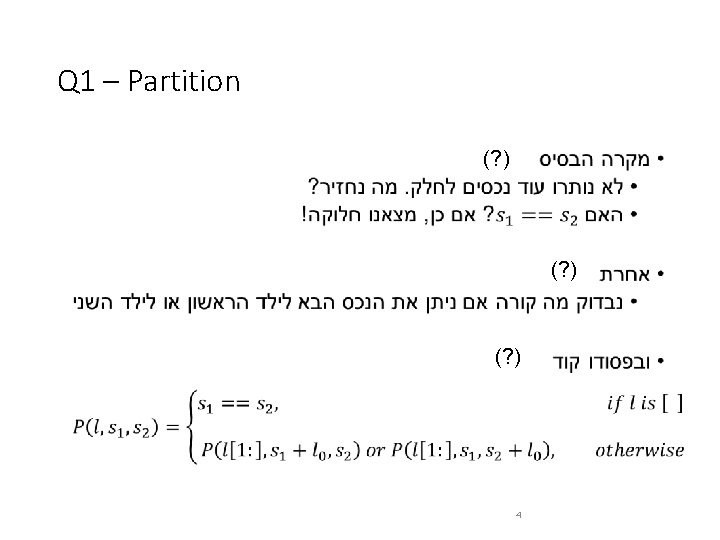
Q 1 – Partition • (? ) 4
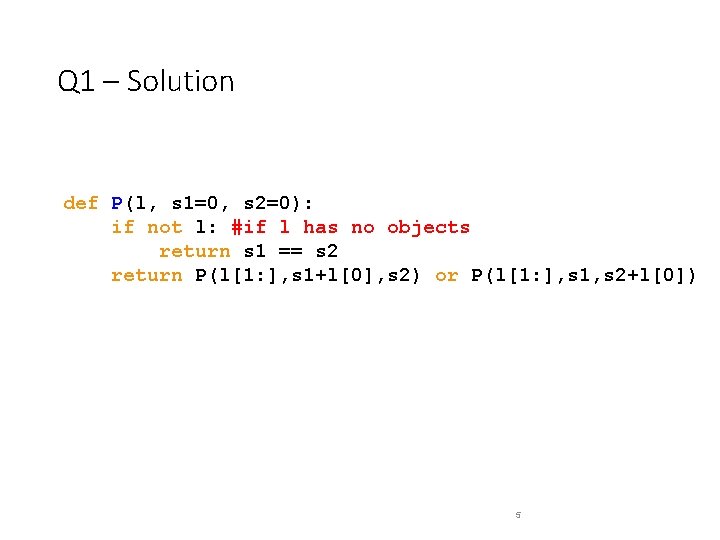
Q 1 – Solution def P(l, s 1=0, s 2=0): if not l: #if l has no objects return s 1 == s 2 return P(l[1: ], s 1+l[0], s 2) or P(l[1: ], s 1, s 2+l[0]) 5
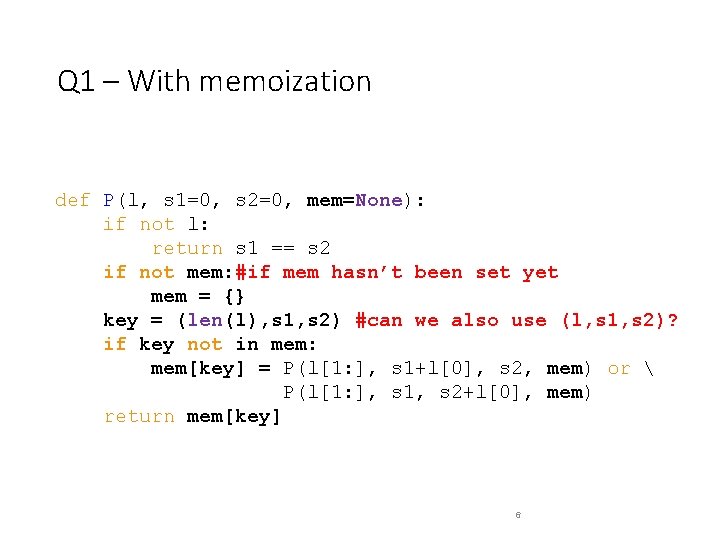
Q 1 – With memoization def P(l, s 1=0, s 2=0, mem=None): if not l: return s 1 == s 2 if not mem: #if mem hasn’t been set yet mem = {} key = (len(l), s 1, s 2) #can we also use (l, s 1, s 2)? if key not in mem: mem[key] = P(l[1: ], s 1+l[0], s 2, mem) or P(l[1: ], s 1, s 2+l[0], mem) return mem[key] 6
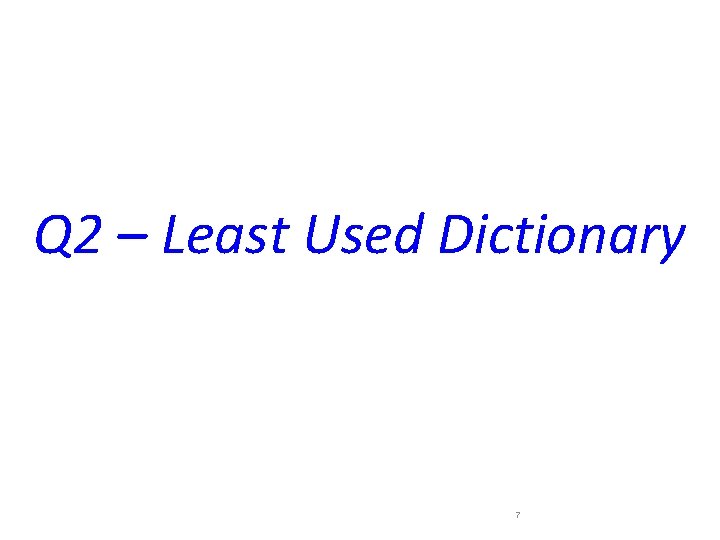
Q 2 – Least Used Dictionary 7
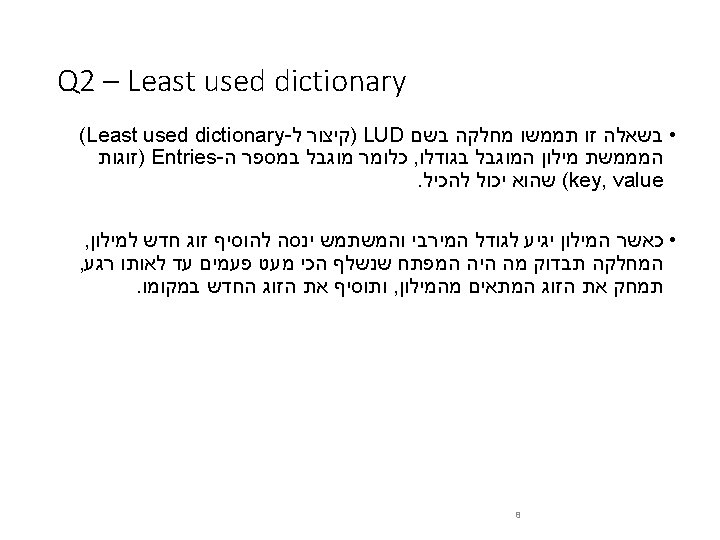
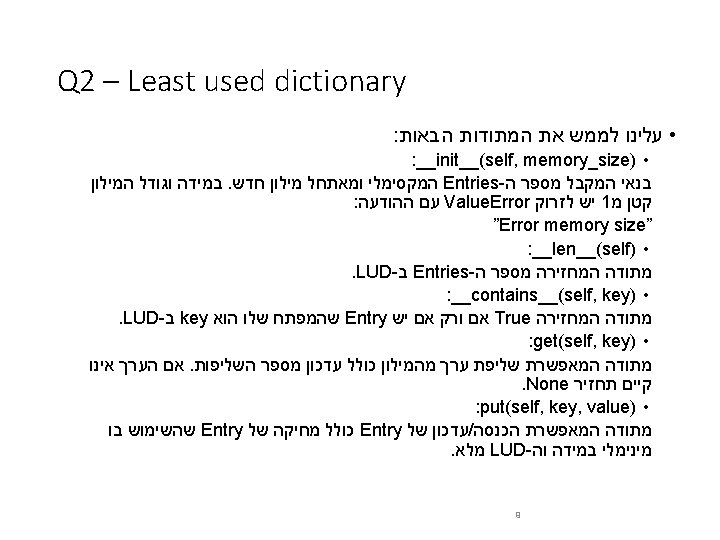
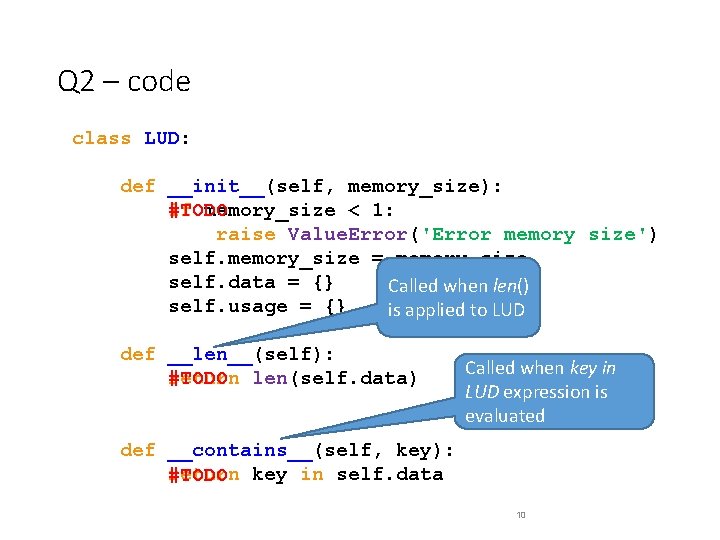
Q 2 – code class LUD: def __init__(self, memory_size): if memory_size < 1: #TODO raise Value. Error('Error memory size') self. memory_size = memory_size self. data = {} Called when len() self. usage = {} is applied to LUD def __len__(self): return len(self. data) #TODO Called when key in LUD expression is evaluated def __contains__(self, key): return key in self. data #TODO 10
![Q 2 code def extractvaluekeyvalue return keyvalue1 class LUD Outside Q 2 – code def extract_value(key_value): return key_value[1] class LUD: . . . Outside](https://slidetodoc.com/presentation_image_h2/72dfd19d16aca756bf20c6c6edd6f229/image-11.jpg)
Q 2 – code def extract_value(key_value): return key_value[1] class LUD: . . . Outside class definition. Doesn’t get “self” def get(self, key): val = self. data. get(key) #TODO if val != None: self. usage[key] += 1 return val def put(self, key, value): if len(self) == self. memory_size and key not in in self: #TODO mkey, mval = min(self. usage. items(), key=extract_value) self. data. pop(mkey) self. usage. pop(mkey) self. data[key] = value self. usage[key] = 0 11
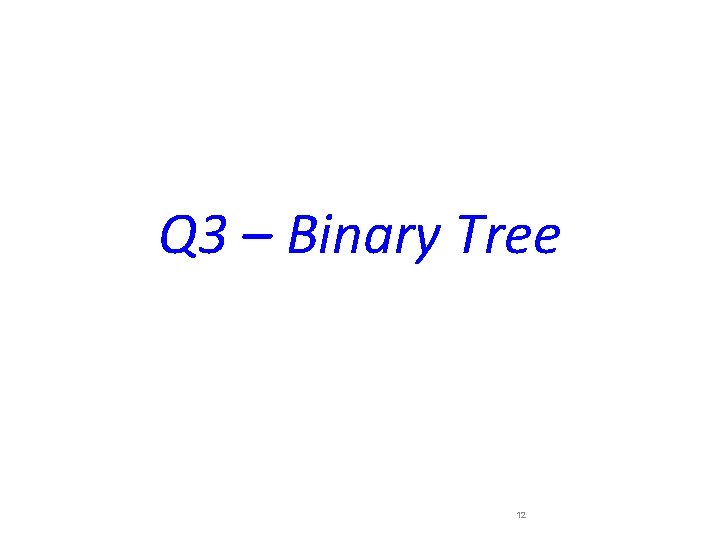
Q 3 – Binary Tree 12
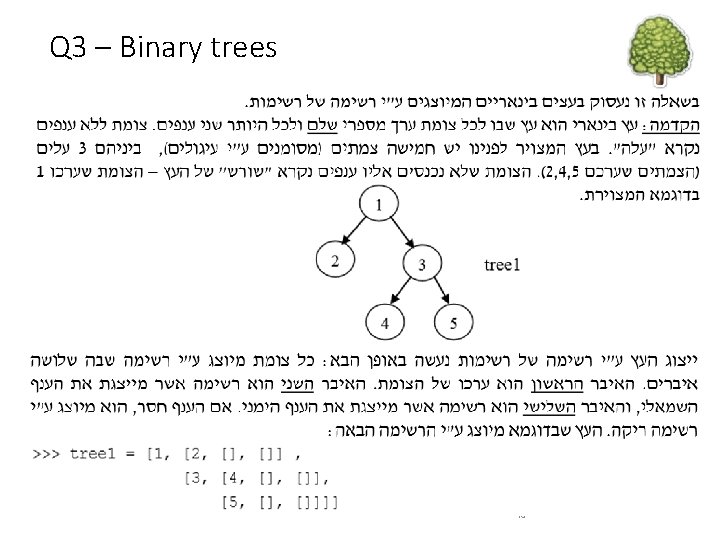
Q 3 – Binary trees 13
![Q 3 Binary trees def isleaftree TODO return not tree1 and not tree2 Q 3 – Binary trees def is_leaf(tree): #TODO return not tree[1] and not tree[2]](https://slidetodoc.com/presentation_image_h2/72dfd19d16aca756bf20c6c6edd6f229/image-14.jpg)
Q 3 – Binary trees def is_leaf(tree): #TODO return not tree[1] and not tree[2] #another option def is_leaf(tree): return len(tree[1])==0 and / len(tree[2])==0 14
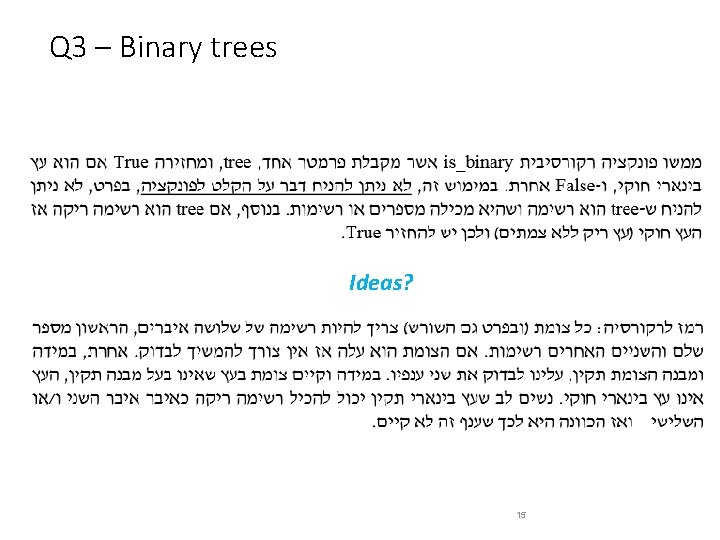
Q 3 – Binary trees Ideas? 15
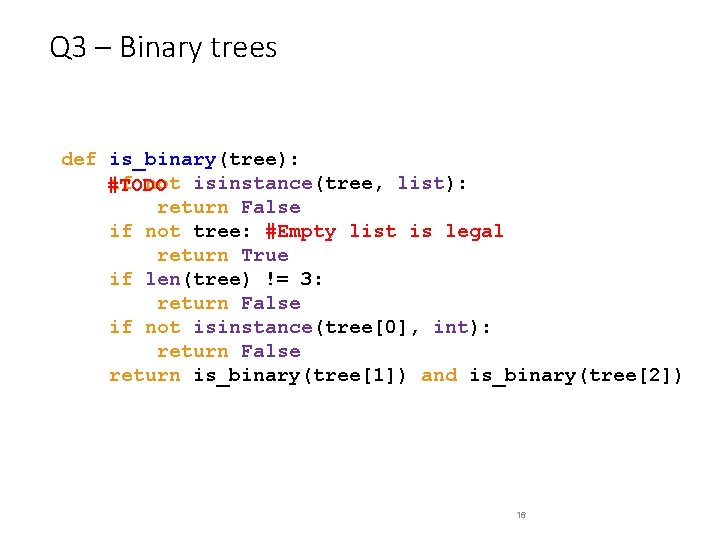
Q 3 – Binary trees def is_binary(tree): if not isinstance(tree, list): #TODO return False if not tree: #Empty list is legal return True if len(tree) != 3: return False if not isinstance(tree[0], int): return False return is_binary(tree[1]) and is_binary(tree[2]) When shall we return False? 16
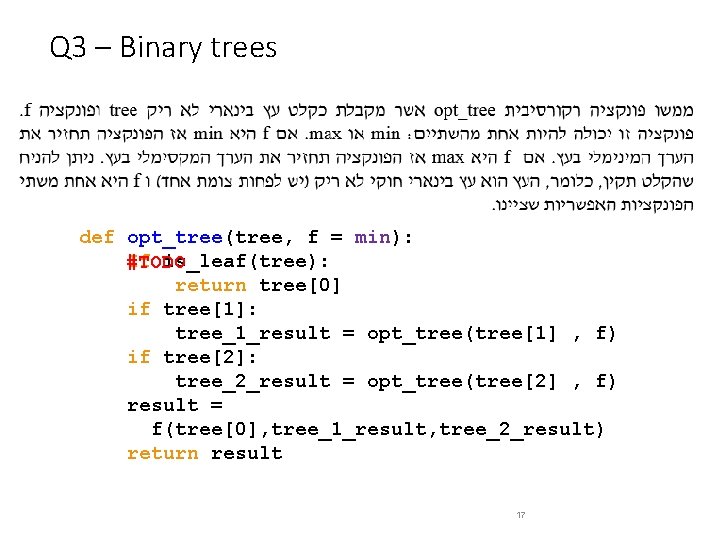
Q 3 – Binary trees def opt_tree(tree, f = min): If is_leaf(tree): #TODO return tree[0] if tree[1]: tree_1_result = opt_tree(tree[1] , f) if tree[2]: tree_2_result = opt_tree(tree[2] , f) result = f(tree[0], tree_1_result, tree_2_result) return result 17
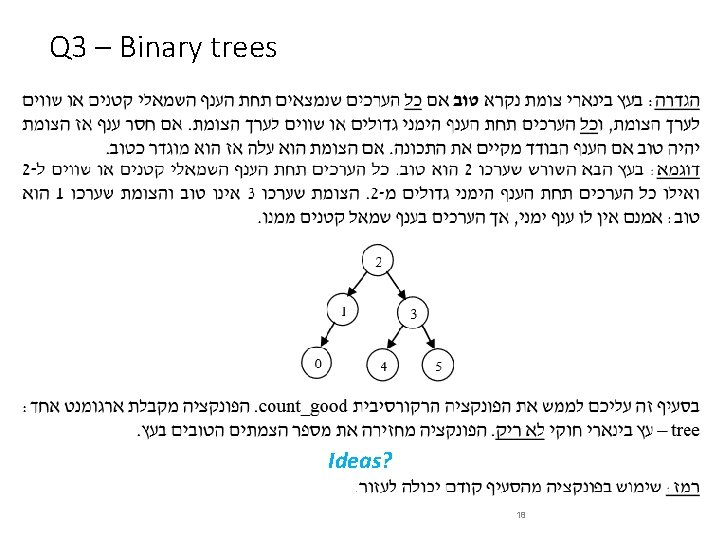
Q 3 – Binary trees Ideas? 18
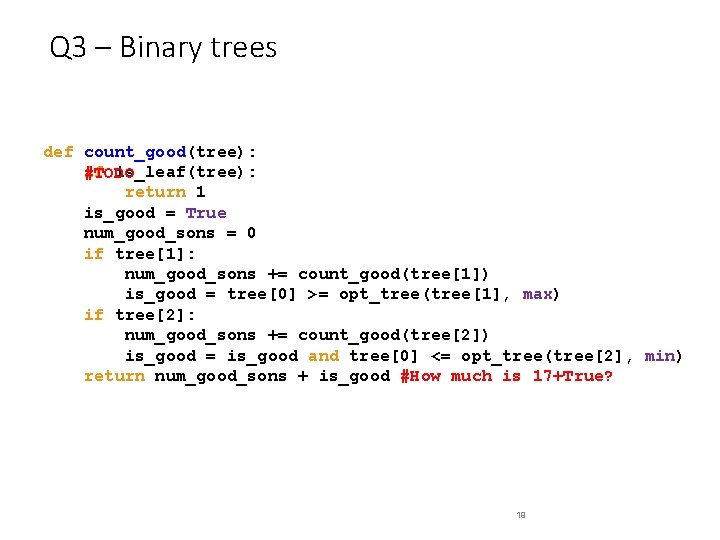
Q 3 – Binary trees def count_good(tree): if is_leaf(tree): #TODO return 1 is_good = True num_good_sons = 0 if tree[1]: num_good_sons += count_good(tree[1]) is_good = tree[0] >= opt_tree(tree[1], max) if tree[2]: num_good_sons += count_good(tree[2]) is_good = is_good and tree[0] <= opt_tree(tree[2], min) return num_good_sons + is_good #How much is 17+True? 19
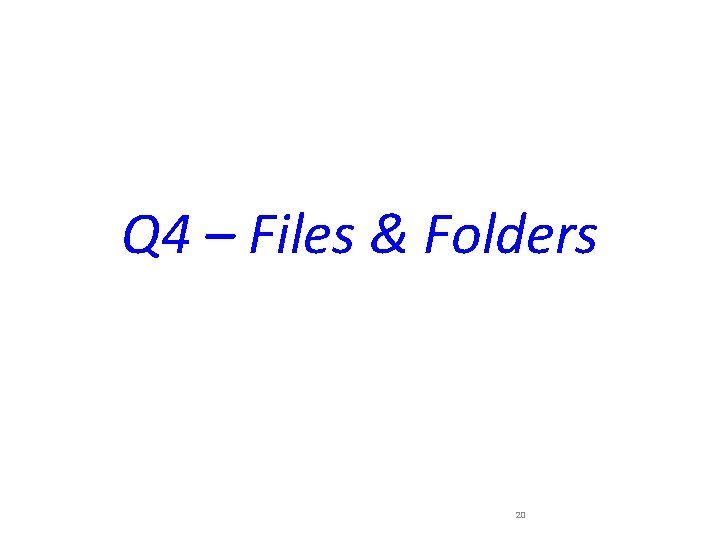
Q 4 – Files & Folders 20
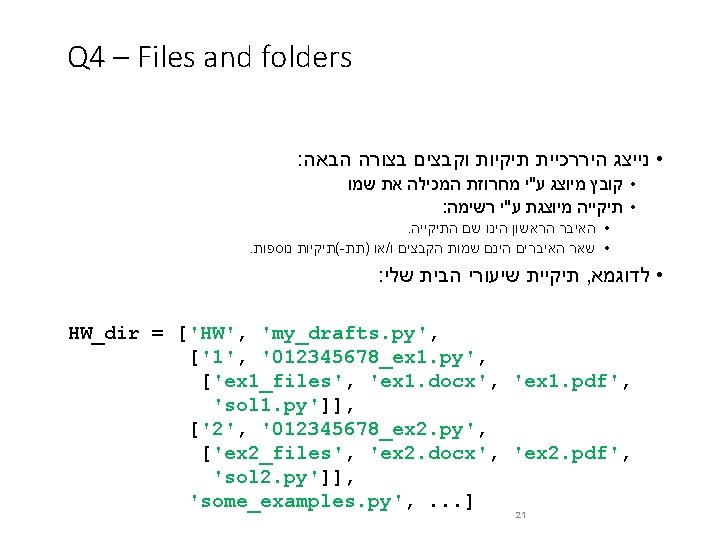
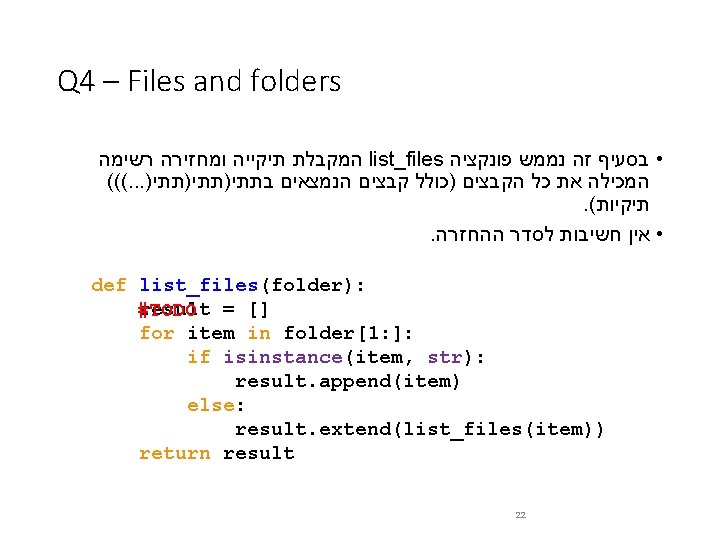
Q 4 – Files and folders המקבלת תיקייה ומחזירה רשימה list_files • בסעיף זה נממש פונקציה (((. . . ) המכילה את כל הקבצים )כולל קבצים הנמצאים בתתי)תתי . ( תיקיות . • אין חשיבות לסדר ההחזרה def list_files(folder): result = [] #TODO for item in folder[1: ]: [1: ] if isinstance(item, str): result. append(item) else: result. extend(list_files(item)) return result 22
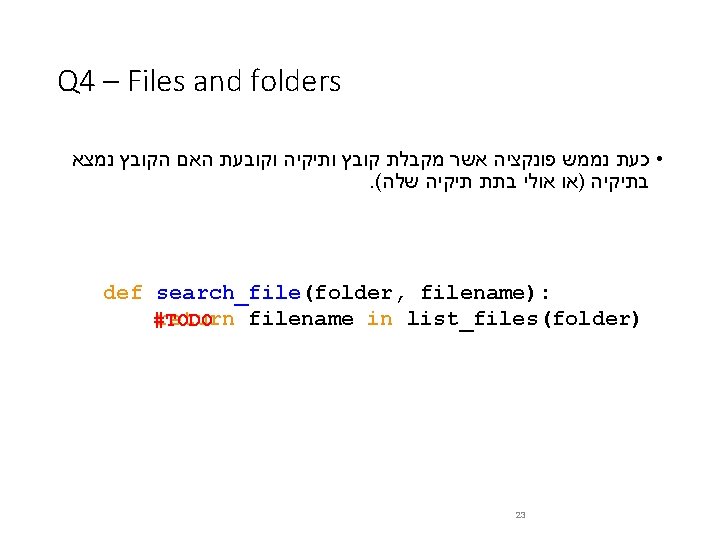
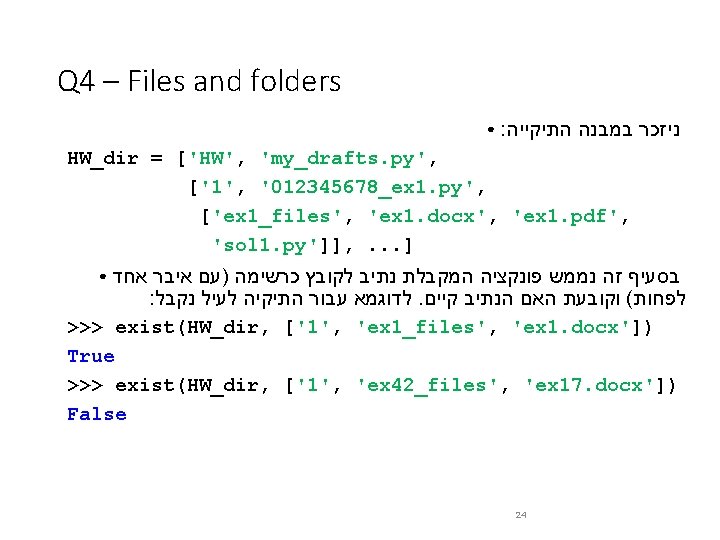
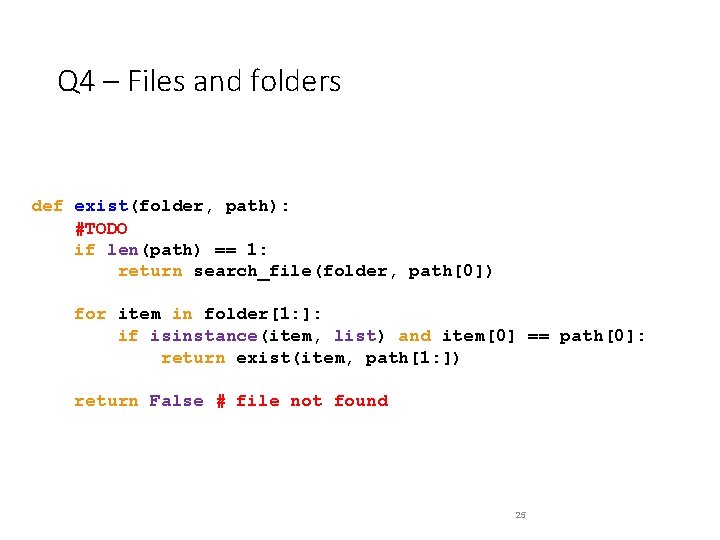
Q 4 – Files and folders def exist(folder, path): #TODO if len(path) == 1: return search_file(folder, path[0]) for item in folder[1: ]: if isinstance(item, list) and item[0] == path[0]: return exist(item, path[1: ]) return False # file not found 25
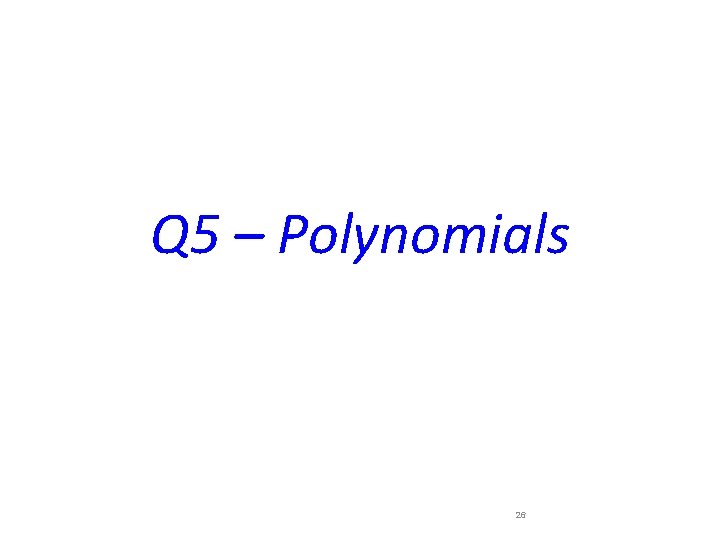
Q 5 – Polynomials 26
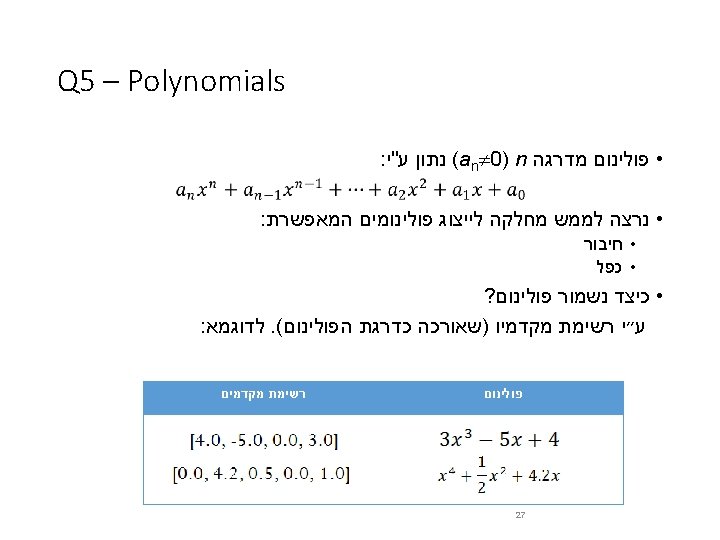
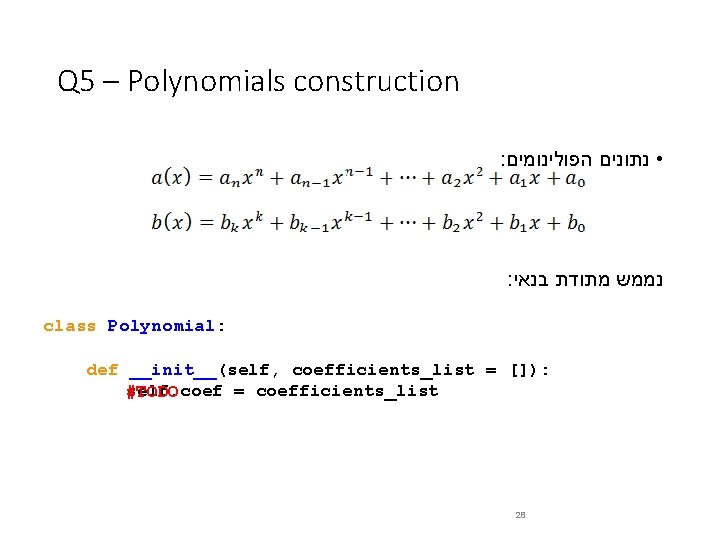
Q 5 – Polynomials construction : • נתונים הפולינומים : נממש מתודת בנאי class Polynomial: def __init__(self, coefficients_list = []): self. coef = coefficients_list #TODO 28
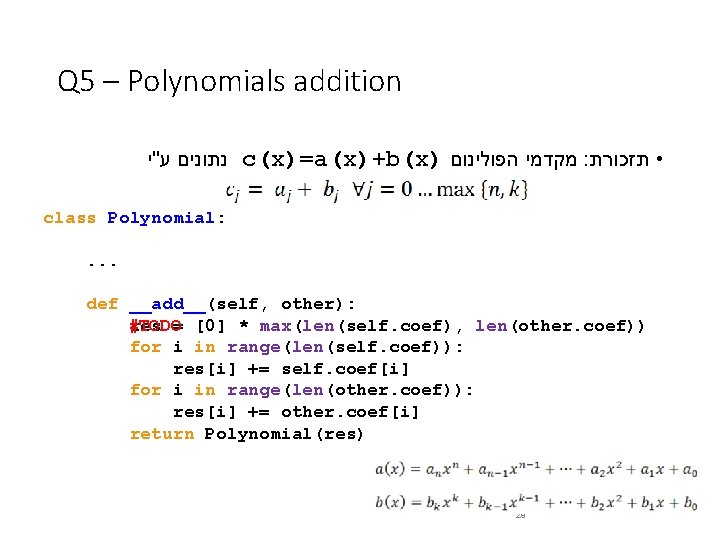
Q 5 – Polynomials addition נתונים ע"י c(x)=a(x)+b(x) מקדמי הפולינום : • תזכורת class Polynomial: . . . def __add__(self, other): res = [0] * max(len(self. coef), len(other. coef)) #TODO for i in range(len(self. coef)): res[i] += self. coef[i] for i in range(len(other. coef)): res[i] += other. coef[i] return Polynomial(res) 29
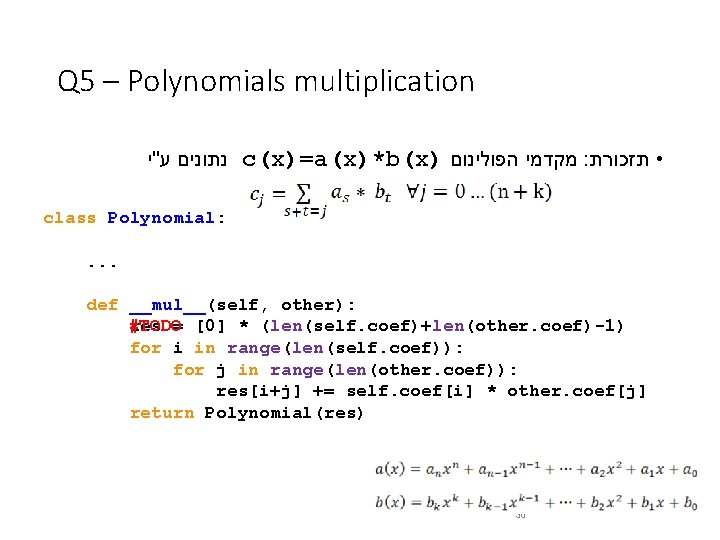
Q 5 – Polynomials multiplication נתונים ע"י c(x)=a(x)*b(x) מקדמי הפולינום : • תזכורת class Polynomial: . . . def __mul__(self, other): res = [0] * (len(self. coef)+len(other. coef)-1) #TODO for i in range(len(self. coef)): for j in range(len(other. coef)): res[i+j] += self. coef[i] * other. coef[j] return Polynomial(res) 30
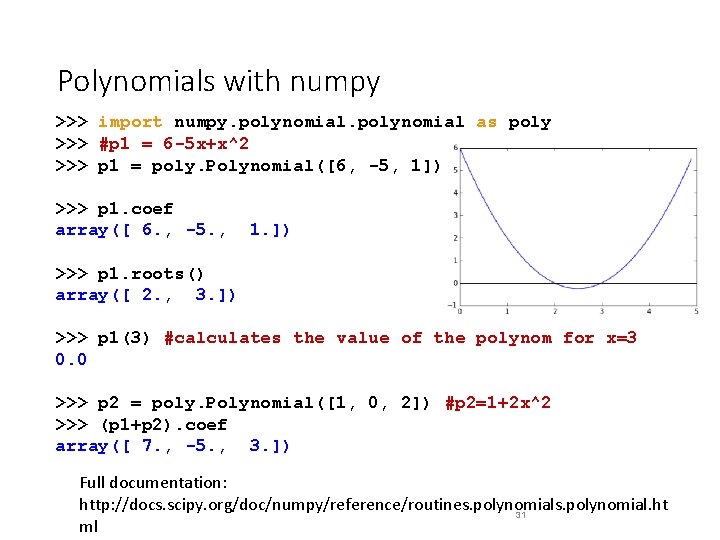
Polynomials with numpy >>> import numpy. polynomial as poly >>> #p 1 = 6 -5 x+x^2 >>> p 1 = poly. Polynomial([6, -5, 1]) >>> p 1. coef array([ 6. , -5. , 1. ]) >>> p 1. roots() array([ 2. , 3. ]) >>> p 1(3) #calculates the value of the polynom for x=3 0. 0 >>> p 2 = poly. Polynomial([1, 0, 2]) #p 2=1+2 x^2 >>> (p 1+p 2). coef array([ 7. , -5. , 3. ]) Full documentation: http: //docs. scipy. org/doc/numpy/reference/routines. polynomial. ht 31 ml
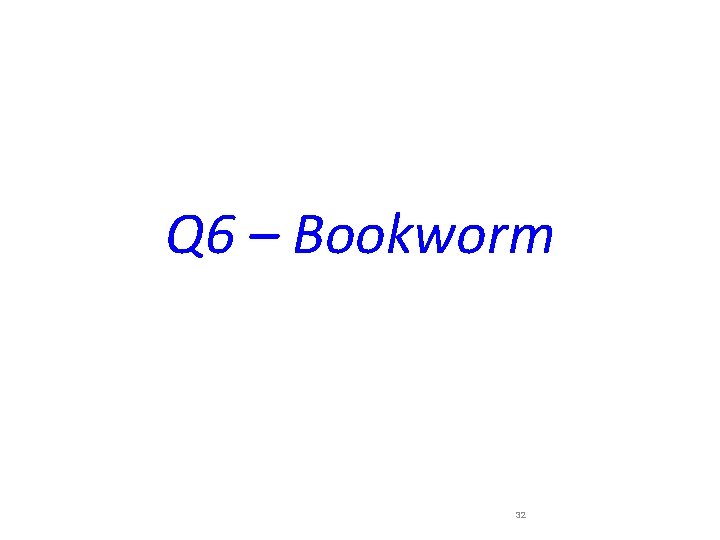
Q 6 – Bookworm 32
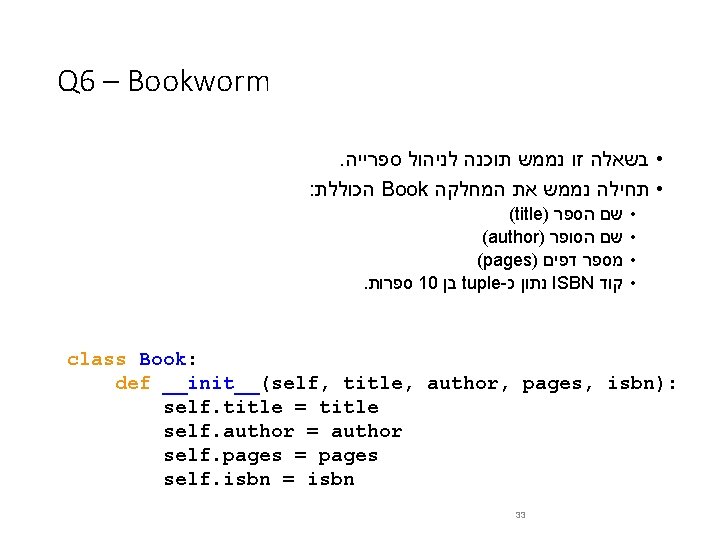
Q 6 – Bookworm. • בשאלה זו נממש תוכנה לניהול ספרייה : הכוללת Book • תחילה נממש את המחלקה (title) שם הספר (author) שם הסופר (pages) מספר דפים . ספרות 10 בן tuple- נתון כ ISBN קוד • • class Book: def __init__(self, title, author, pages, isbn): self. title = title self. author = author self. pages = pages self. isbn = isbn 33
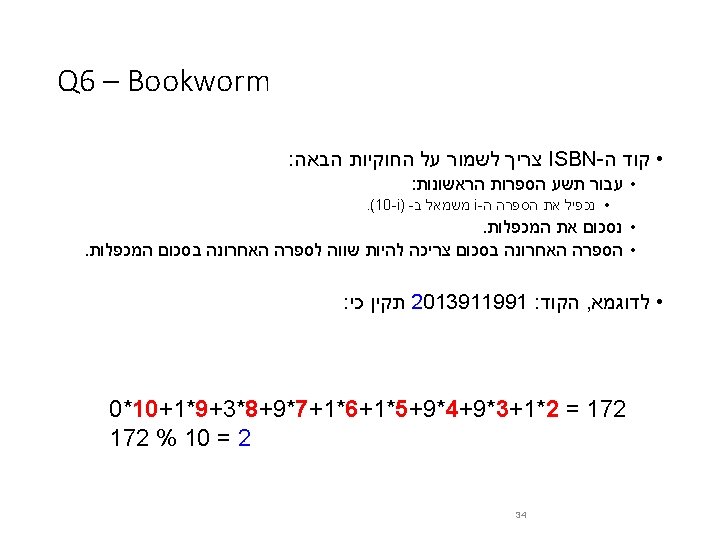
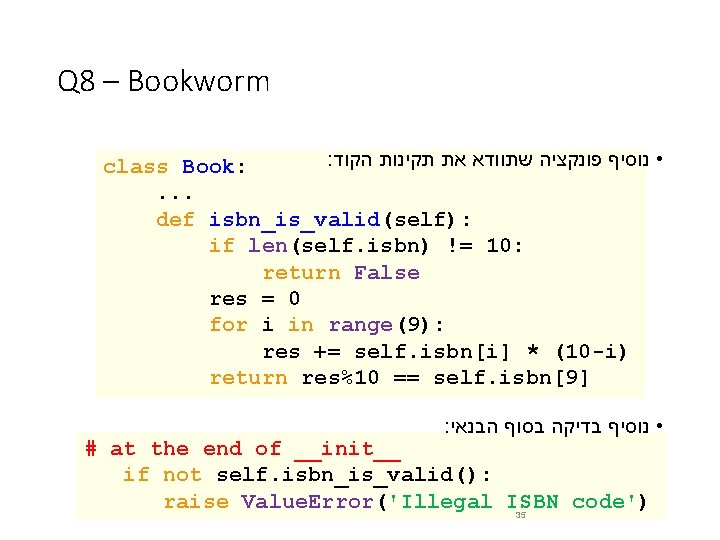
Q 8 – Bookworm : • נוסיף פונקציה שתוודא את תקינות הקוד class Book: . . . def isbn_is_valid(self): if len(self. isbn) != 10: return False res = 0 for i in range(9): res += self. isbn[i] * (10 -i) return res%10 == self. isbn[9] : • נוסיף בדיקה בסוף הבנאי # at the end of __init__ if not self. isbn_is_valid(): raise Value. Error('Illegal ISBN code') 35
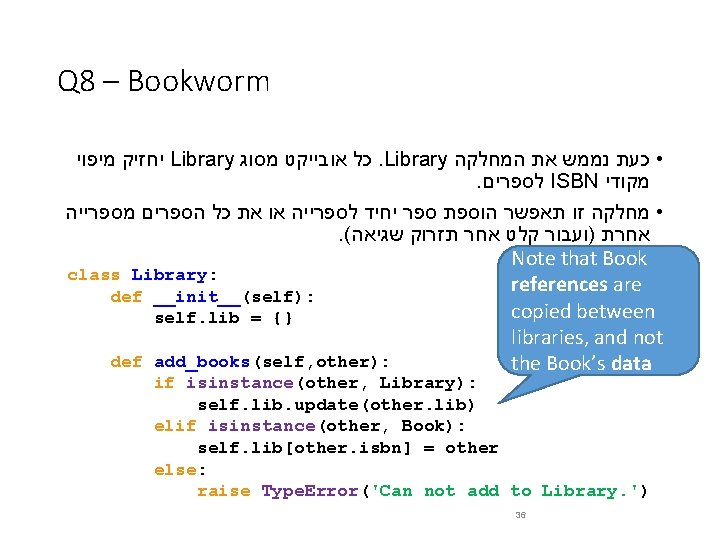
Q 8 – Bookworm יחזיק מיפוי Library כל אובייקט מסוג. Library • כעת נממש את המחלקה . לספרים ISBN מקודי • מחלקה זו תאפשר הוספת ספר יחיד לספרייה או את כל הספרים מספרייה . ( אחרת )ועבור קלט אחר תזרוק שגיאה Note that Book class Library: references are def __init__(self): copied between self. lib = {} libraries, and not def add_books(self, other): the Book’s data if isinstance(other, Library): self. lib. update(other. lib) elif isinstance(other, Book): self. lib[other. isbn] = other else: raise Type. Error('Can not add to Library. ') 36
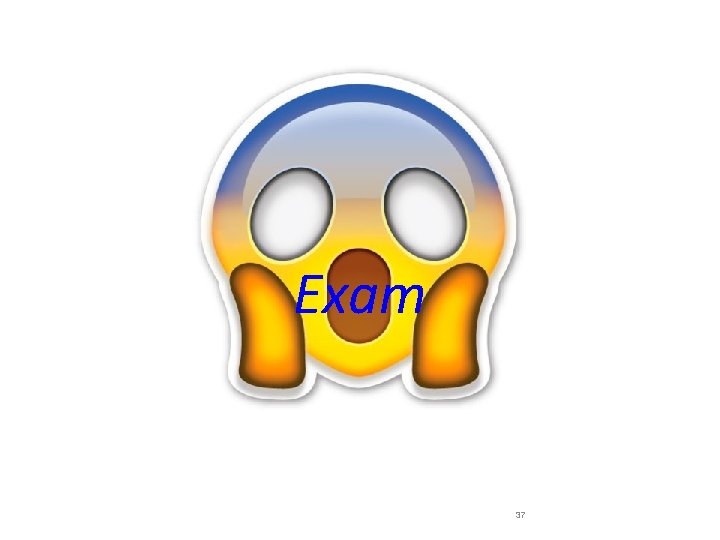
Exam 37
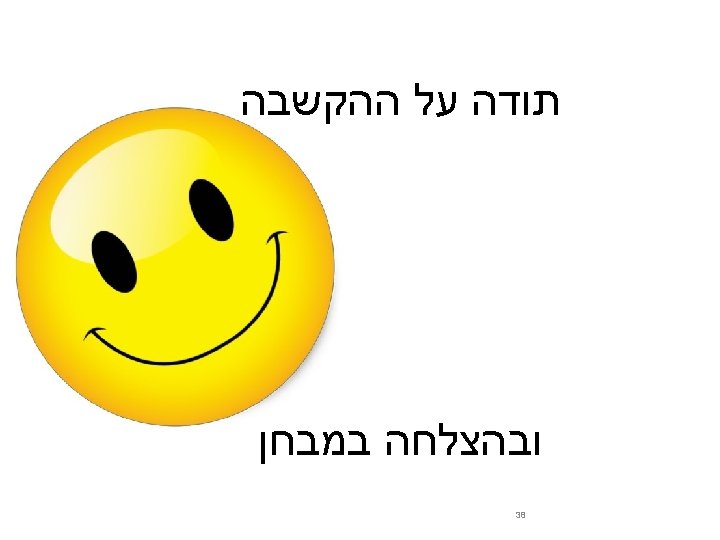
Practical session definition
Practical session definition
Practical session definition
Practical session meaning
Python programming in context
Python blackjack oop
Programming essentials in python
Python programming in context
Cgi programming in python
Python procedural
Python programming an introduction to computer science
Constraint programming python
Audiolab python
Python chapter 5 programming exercises
Rapid gui programming with python and qt
Python programming
Second order cone programming python
System programming definition
Linear vs integer programming
Perbedaan linear programming dan integer programming
Definisi integer
Greedy vs dynamic programming
Intro to assembly language
Act 3 lady macbeth
Personification in introduction to poetry by billy collins
Gerrc paragraph
Mumindalen intro
Performance task introduction
Lynda intro
Bivariate scatter plot
They say i say format
How to write a text response essay introduction
Intro to new media
Mgm intro
Logic intro
Chicken foot dbq
Daniel lars morten
States of matter
Objectives of environmental pollution