Hadoop Programming Overview Map Reduce Types Input Formats
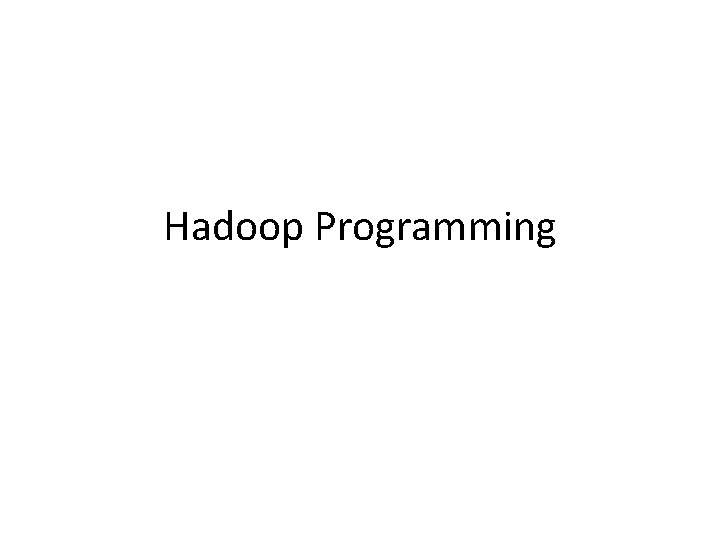
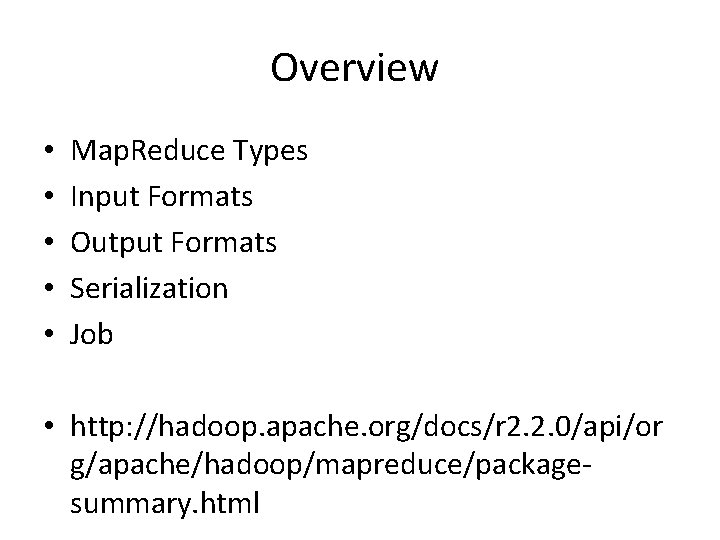
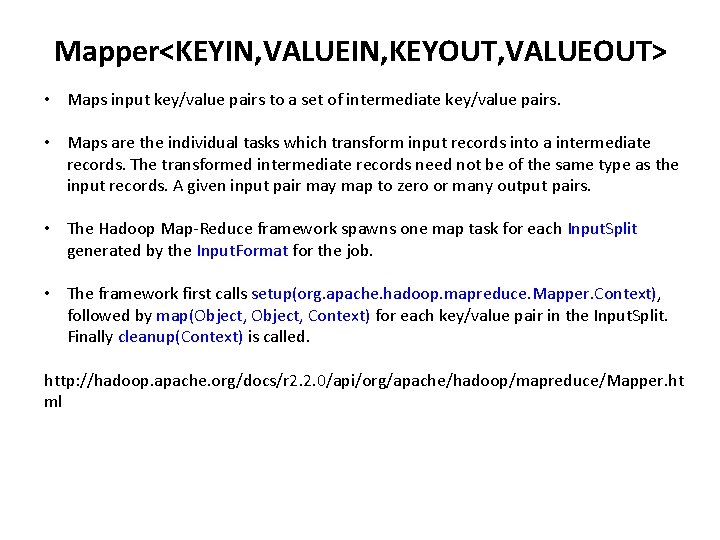
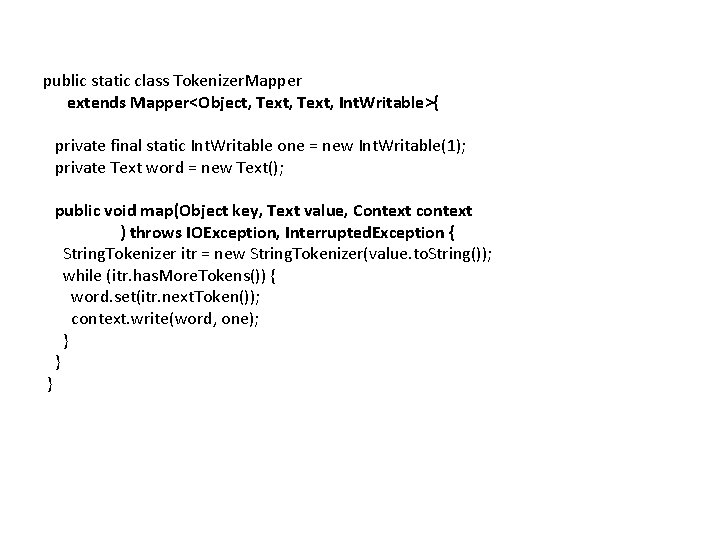
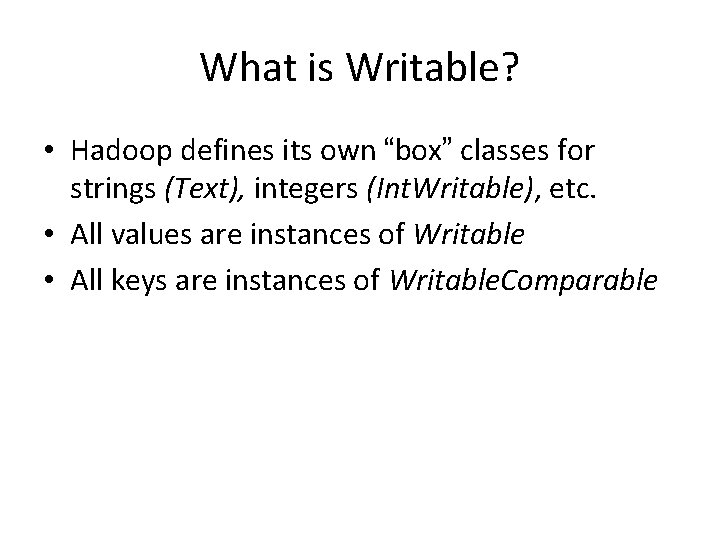
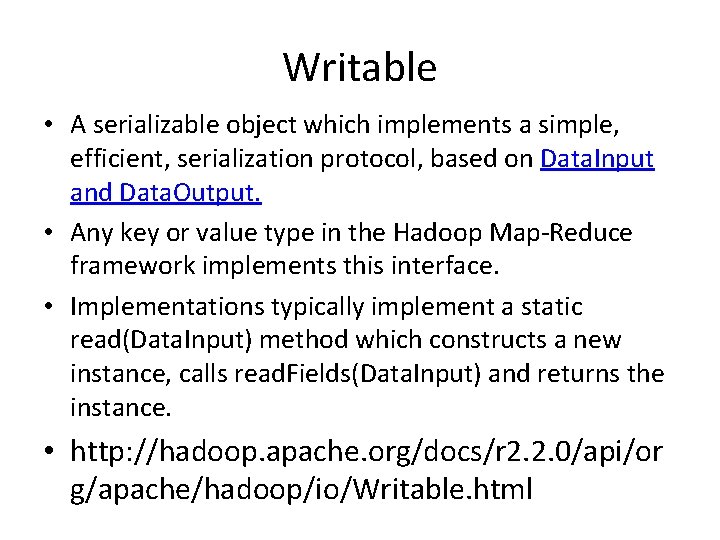
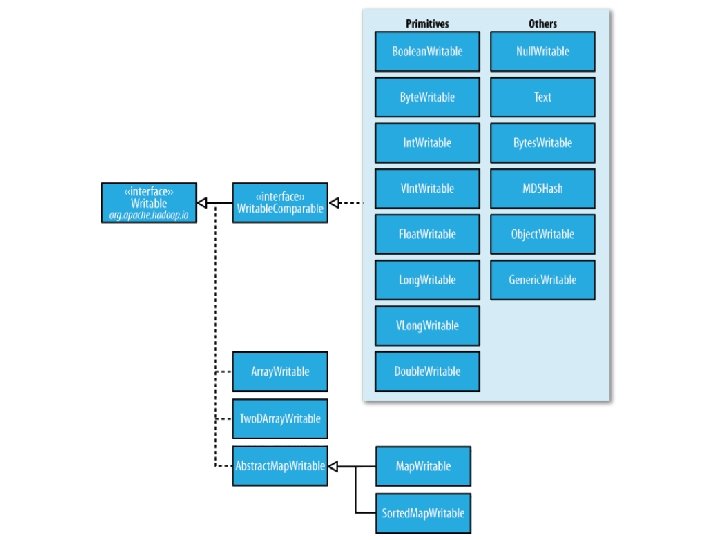
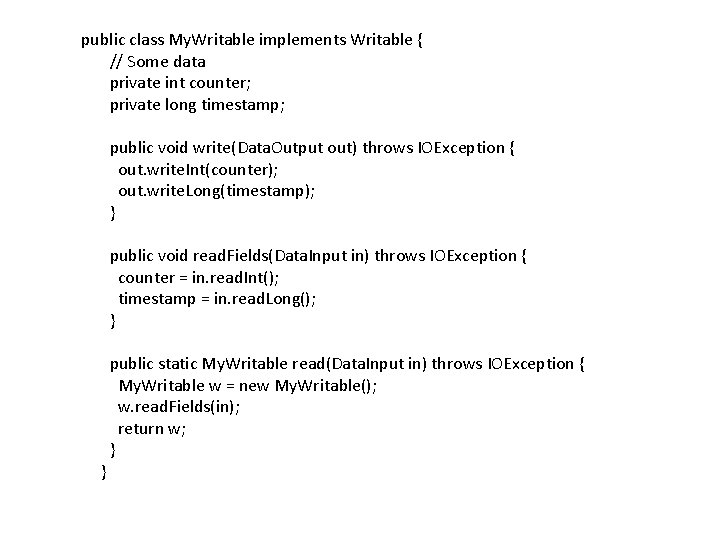
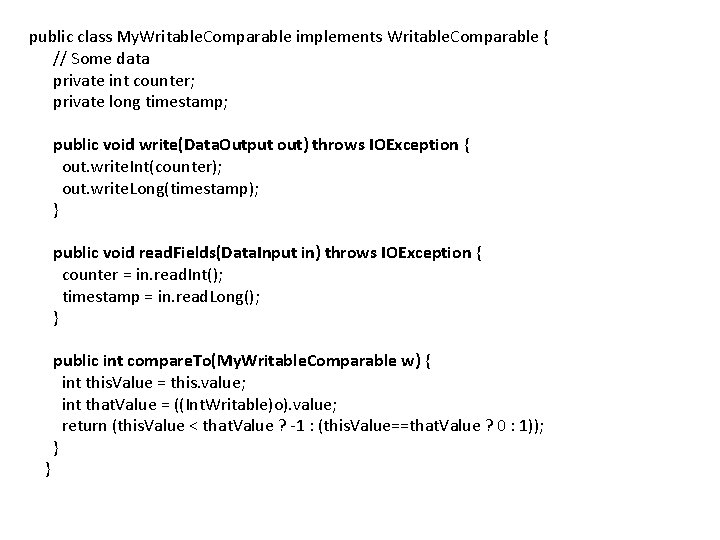
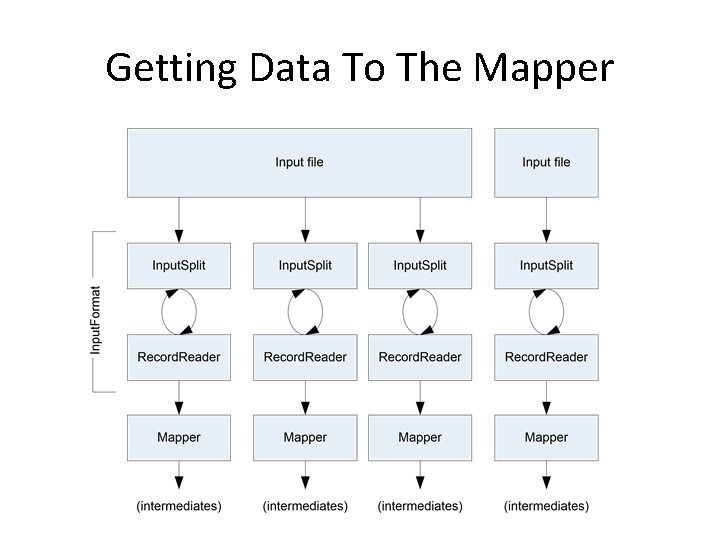
![public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); String[] public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); String[]](https://slidetodoc.com/presentation_image_h/e28c0d8263d24e66ba1dcacfc4fc5087/image-11.jpg)
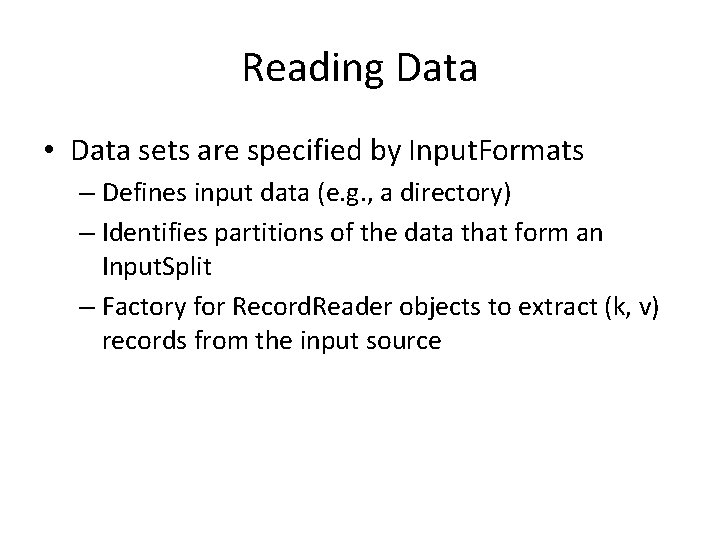
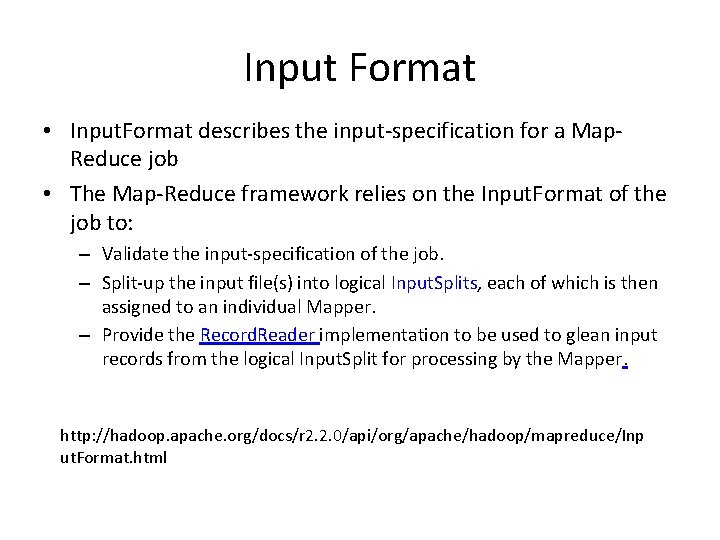
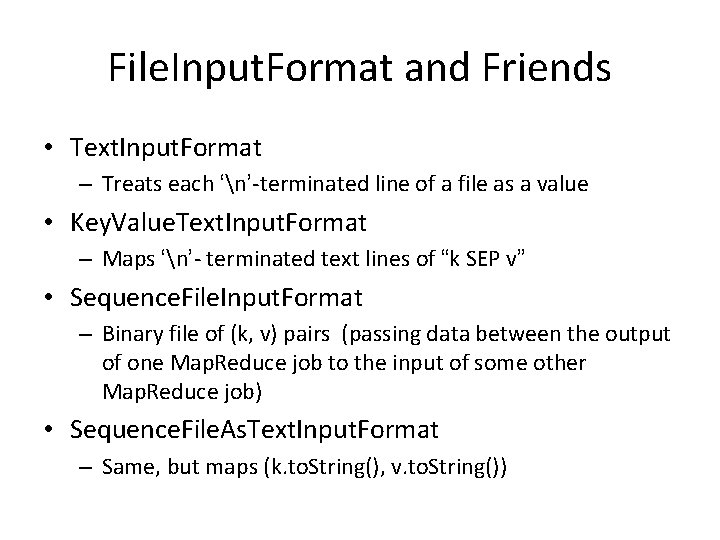
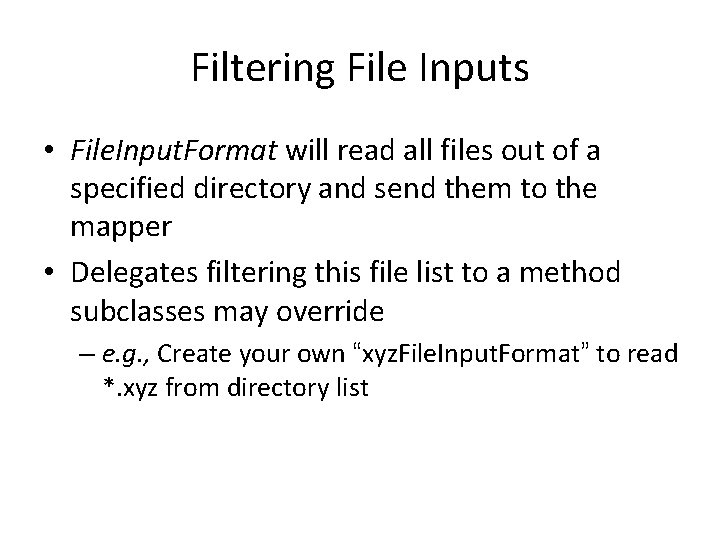
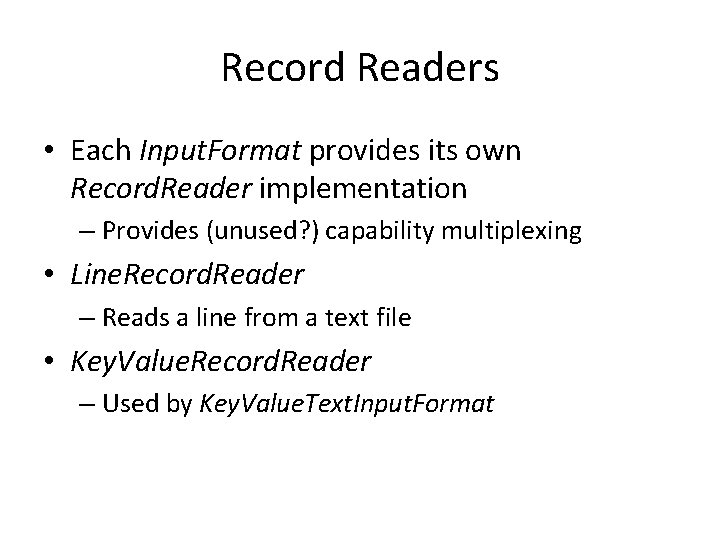
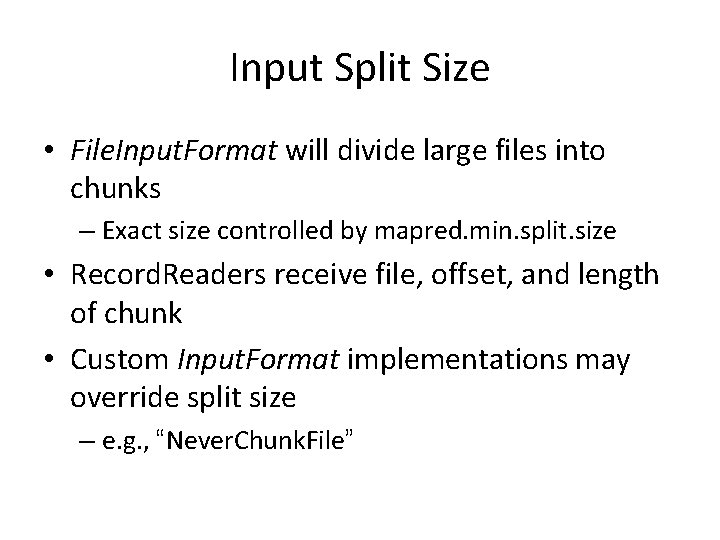
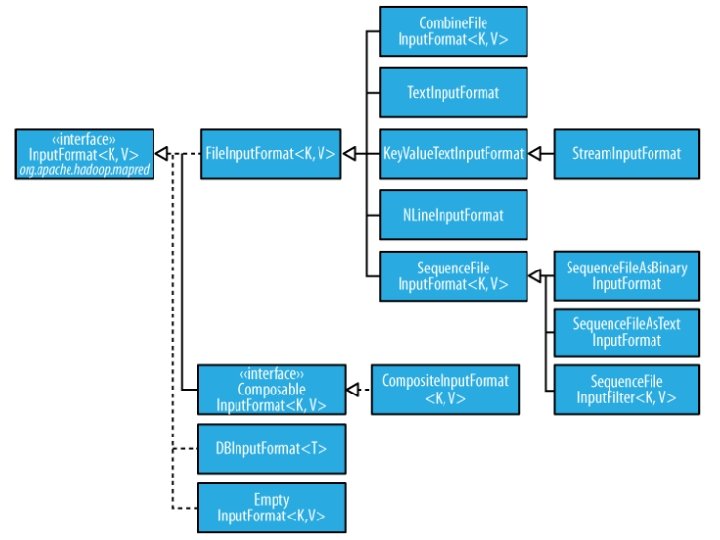
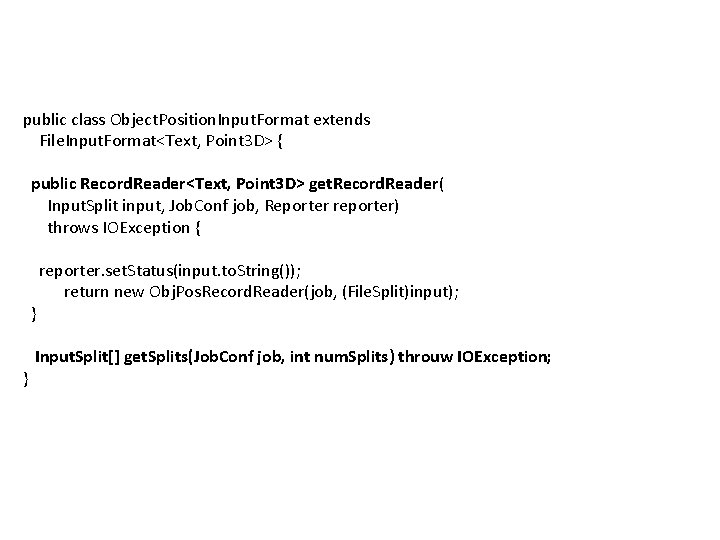
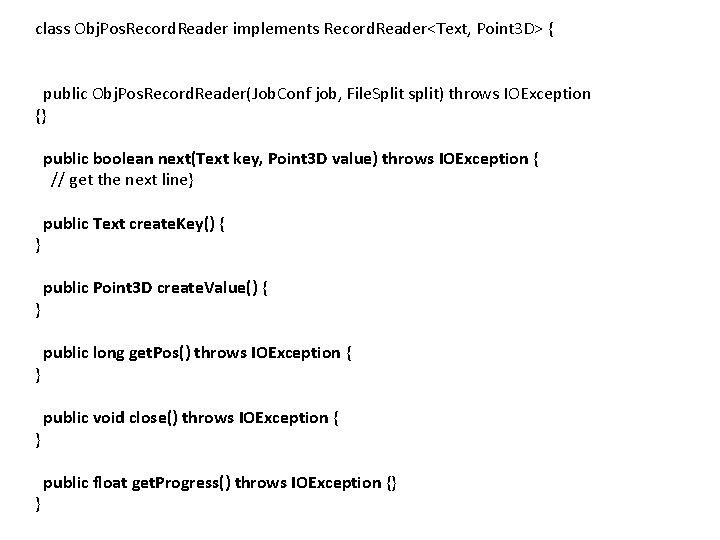
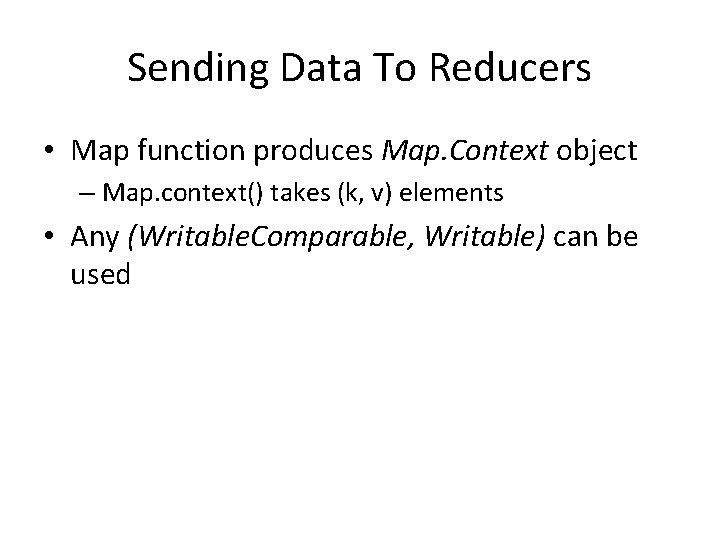
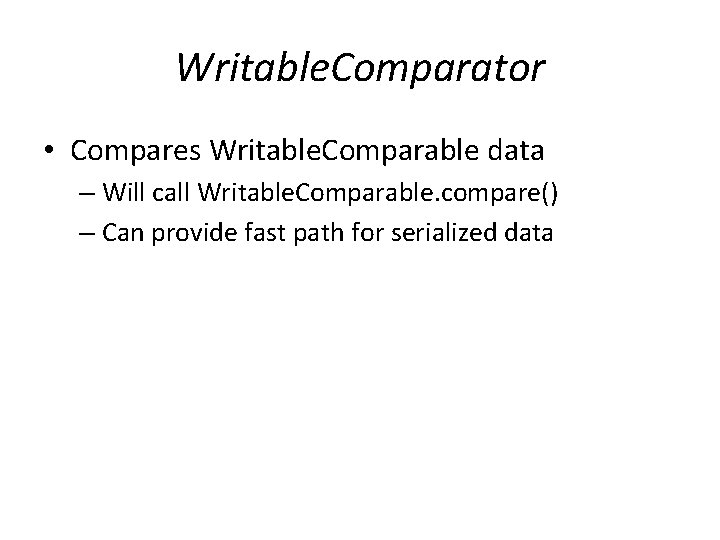
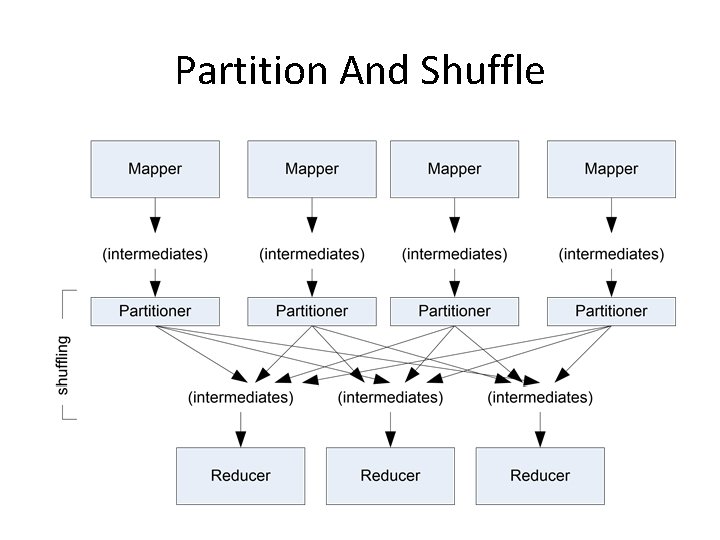
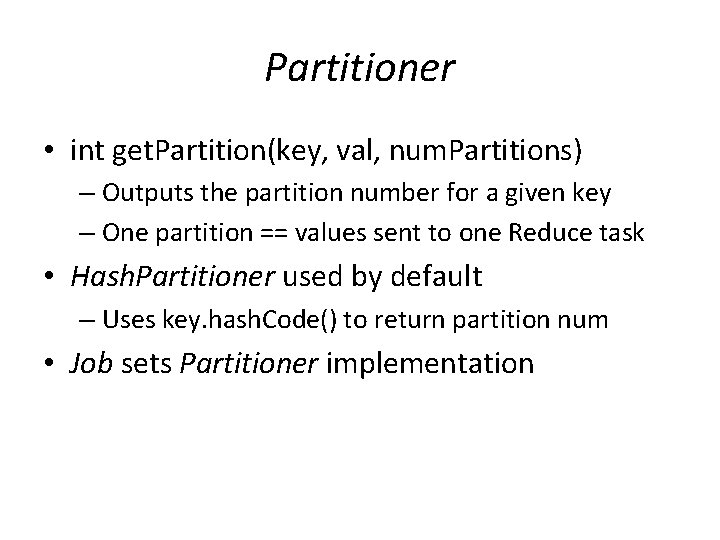
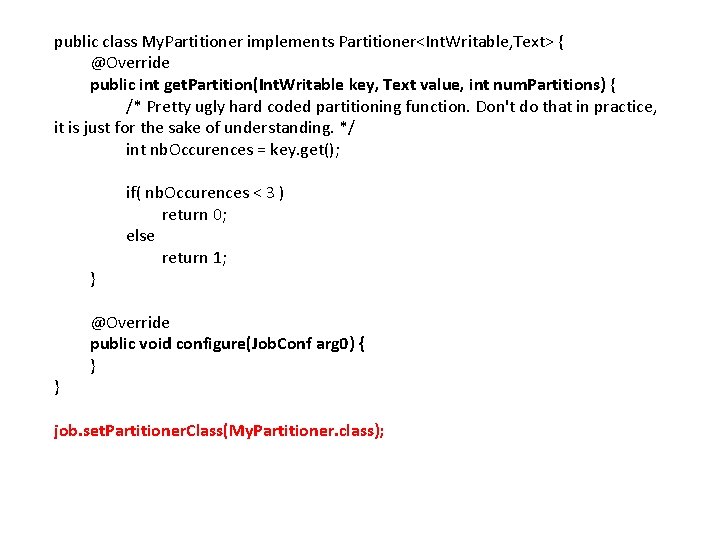
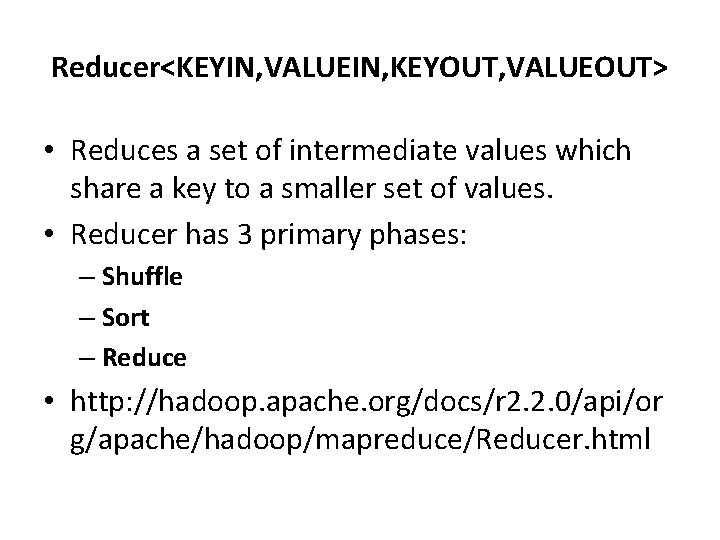
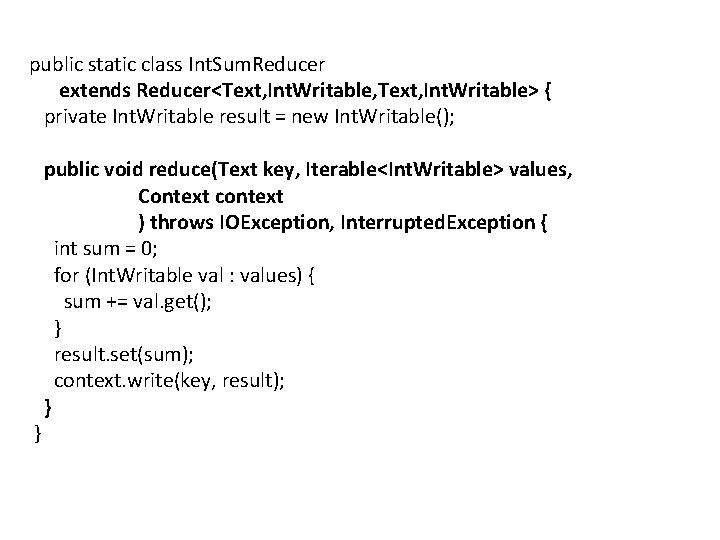
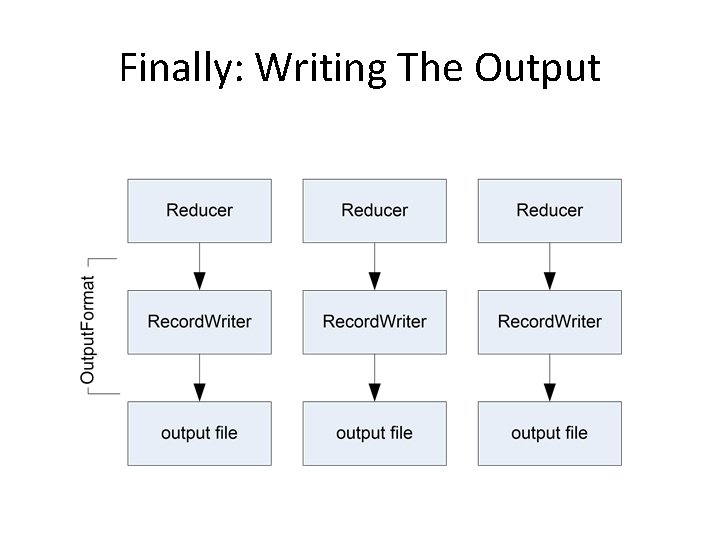
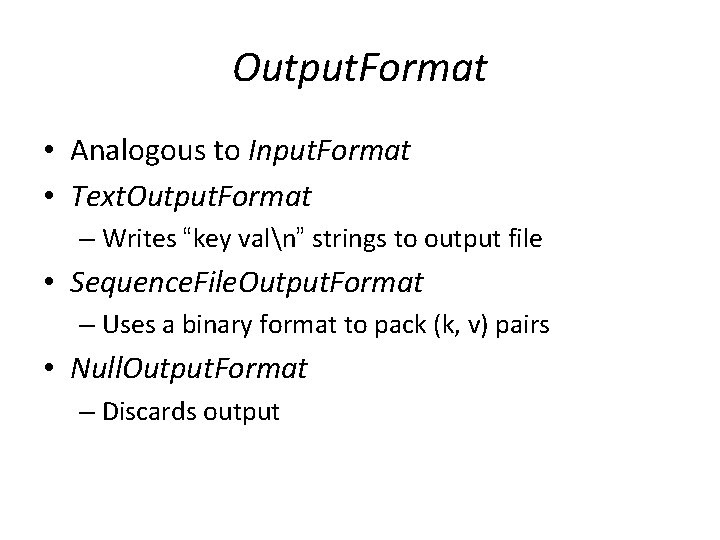
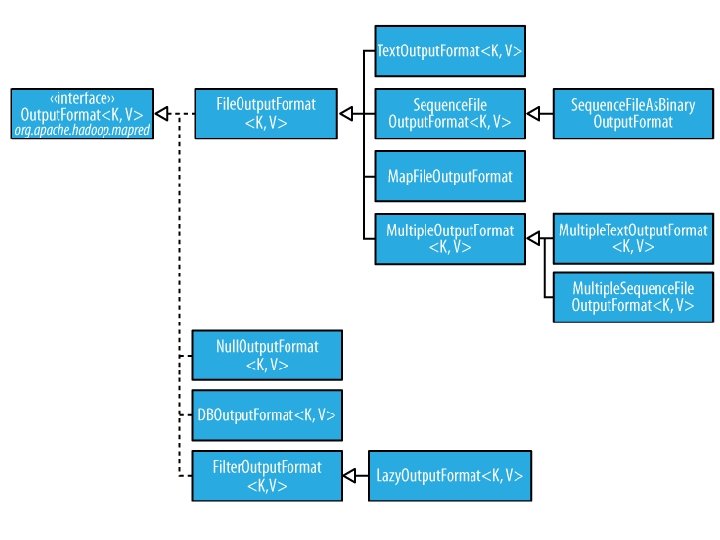
![public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); String[] public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); String[]](https://slidetodoc.com/presentation_image_h/e28c0d8263d24e66ba1dcacfc4fc5087/image-31.jpg)
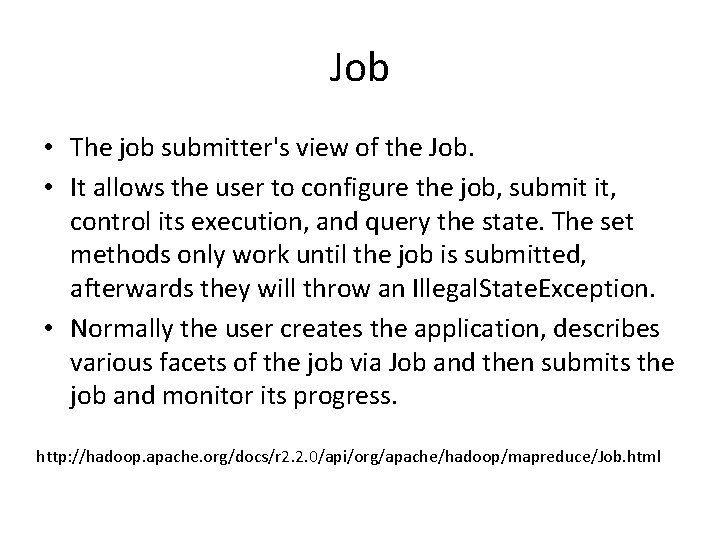
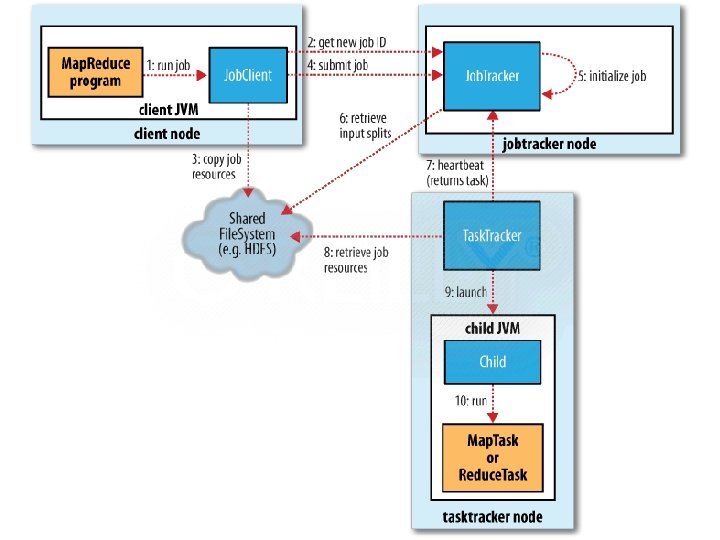
- Slides: 33
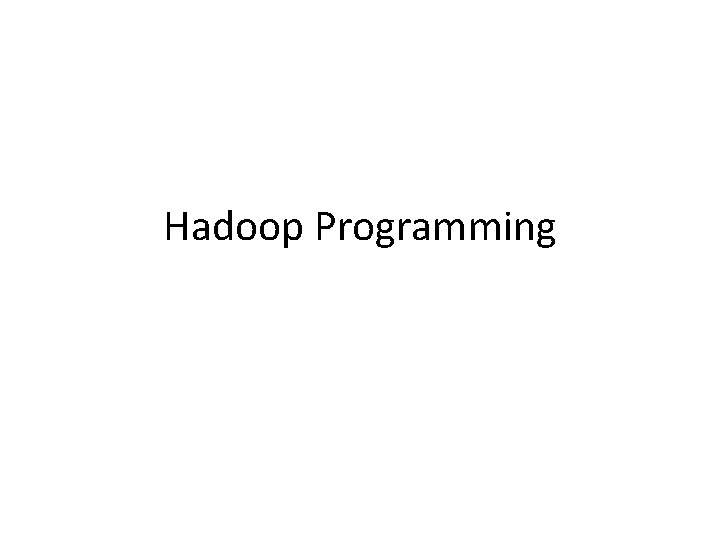
Hadoop Programming
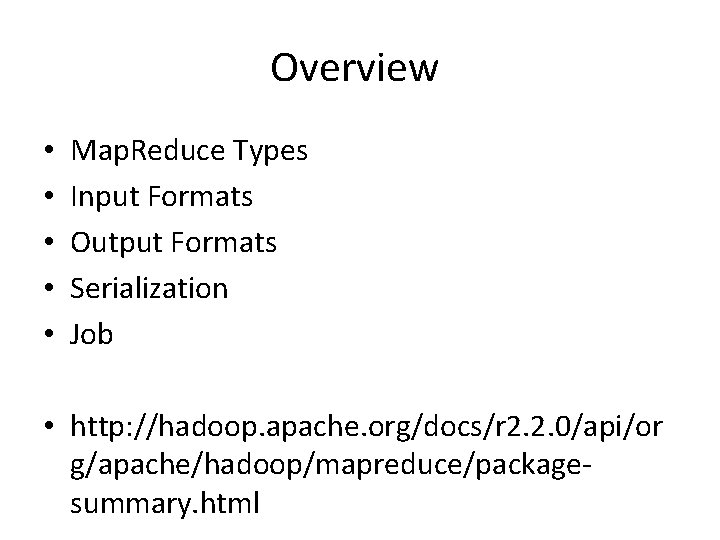
Overview • • • Map. Reduce Types Input Formats Output Formats Serialization Job • http: //hadoop. apache. org/docs/r 2. 2. 0/api/or g/apache/hadoop/mapreduce/packagesummary. html
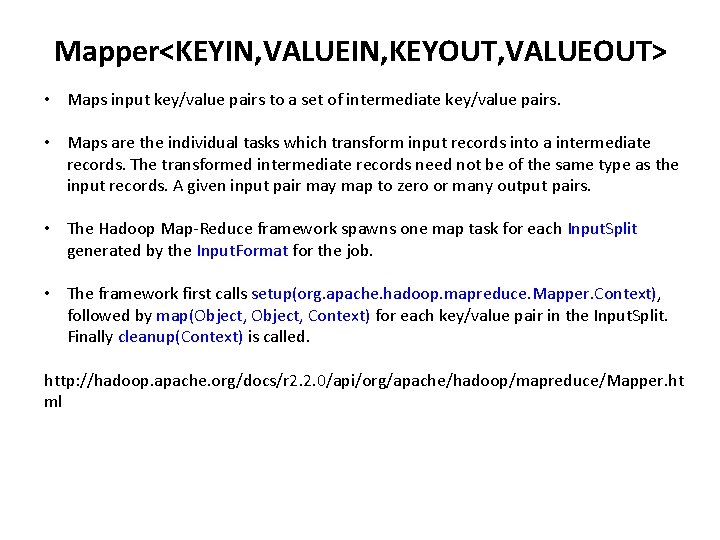
Mapper<KEYIN, VALUEIN, KEYOUT, VALUEOUT> • Maps input key/value pairs to a set of intermediate key/value pairs. • Maps are the individual tasks which transform input records into a intermediate records. The transformed intermediate records need not be of the same type as the input records. A given input pair may map to zero or many output pairs. • The Hadoop Map-Reduce framework spawns one map task for each Input. Split generated by the Input. Format for the job. • The framework first calls setup(org. apache. hadoop. mapreduce. Mapper. Context), followed by map(Object, Context) for each key/value pair in the Input. Split. Finally cleanup(Context) is called. http: //hadoop. apache. org/docs/r 2. 2. 0/api/org/apache/hadoop/mapreduce/Mapper. ht ml
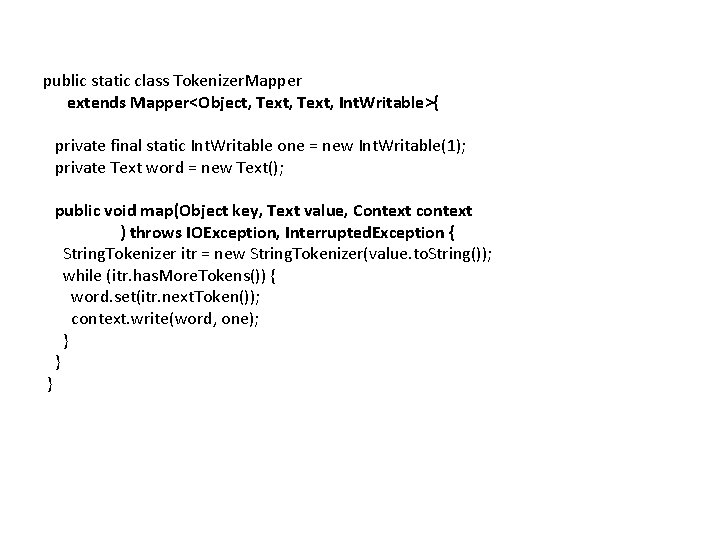
public static class Tokenizer. Mapper extends Mapper<Object, Text, Int. Writable>{ private final static Int. Writable one = new Int. Writable(1); private Text word = new Text(); } public void map(Object key, Text value, Context context ) throws IOException, Interrupted. Exception { String. Tokenizer itr = new String. Tokenizer(value. to. String()); while (itr. has. More. Tokens()) { word. set(itr. next. Token()); context. write(word, one); } }
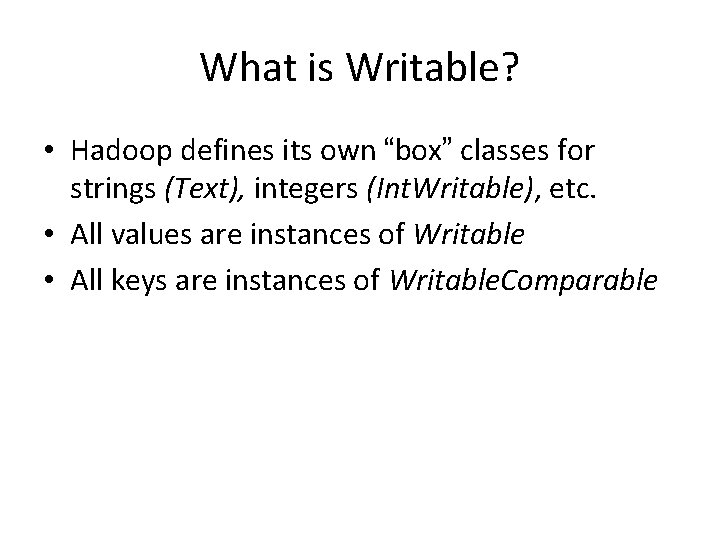
What is Writable? • Hadoop defines its own “box” classes for strings (Text), integers (Int. Writable), etc. • All values are instances of Writable • All keys are instances of Writable. Comparable
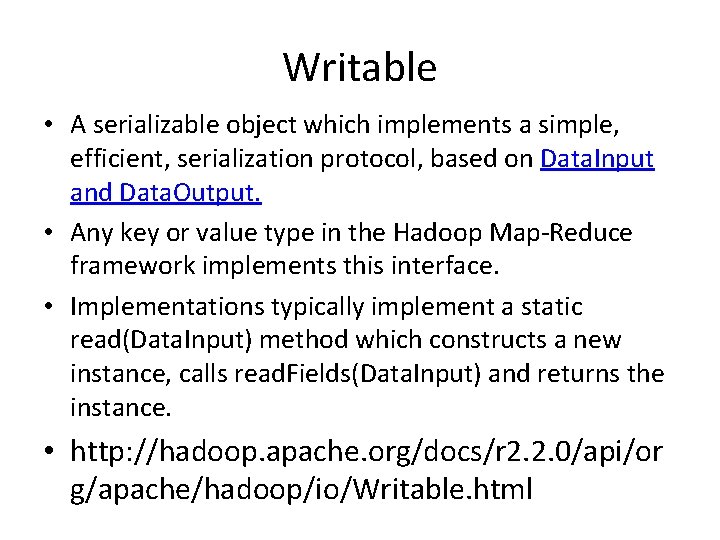
Writable • A serializable object which implements a simple, efficient, serialization protocol, based on Data. Input and Data. Output. • Any key or value type in the Hadoop Map-Reduce framework implements this interface. • Implementations typically implement a static read(Data. Input) method which constructs a new instance, calls read. Fields(Data. Input) and returns the instance. • http: //hadoop. apache. org/docs/r 2. 2. 0/api/or g/apache/hadoop/io/Writable. html
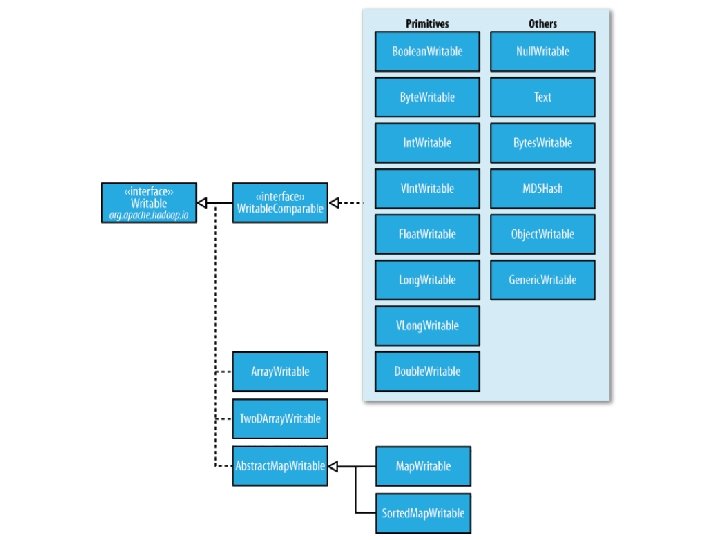
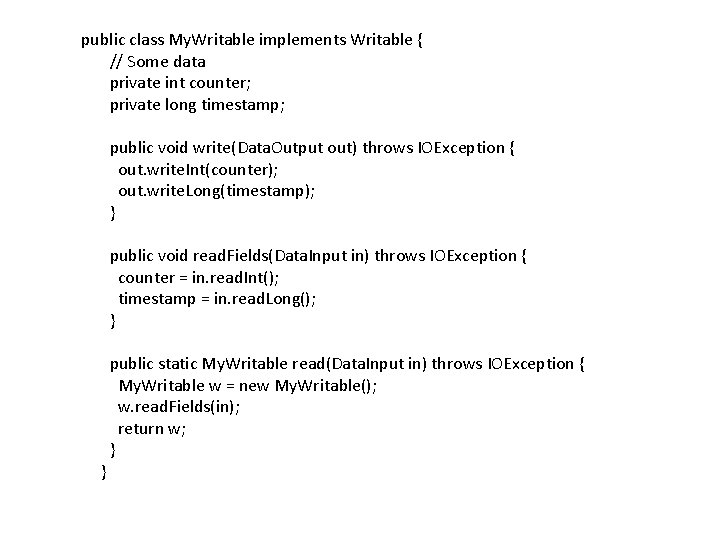
public class My. Writable implements Writable { // Some data private int counter; private long timestamp; public void write(Data. Output out) throws IOException { out. write. Int(counter); out. write. Long(timestamp); } public void read. Fields(Data. Input in) throws IOException { counter = in. read. Int(); timestamp = in. read. Long(); } } public static My. Writable read(Data. Input in) throws IOException { My. Writable w = new My. Writable(); w. read. Fields(in); return w; }
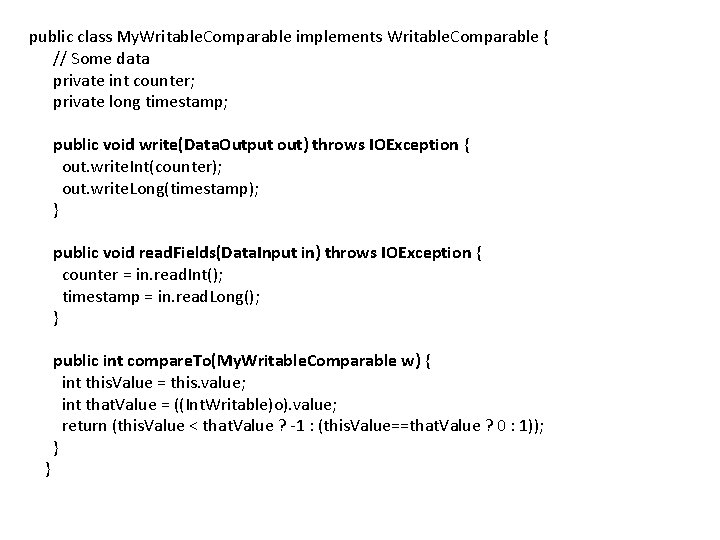
public class My. Writable. Comparable implements Writable. Comparable { // Some data private int counter; private long timestamp; public void write(Data. Output out) throws IOException { out. write. Int(counter); out. write. Long(timestamp); } public void read. Fields(Data. Input in) throws IOException { counter = in. read. Int(); timestamp = in. read. Long(); } } public int compare. To(My. Writable. Comparable w) { int this. Value = this. value; int that. Value = ((Int. Writable)o). value; return (this. Value < that. Value ? -1 : (this. Value==that. Value ? 0 : 1)); }
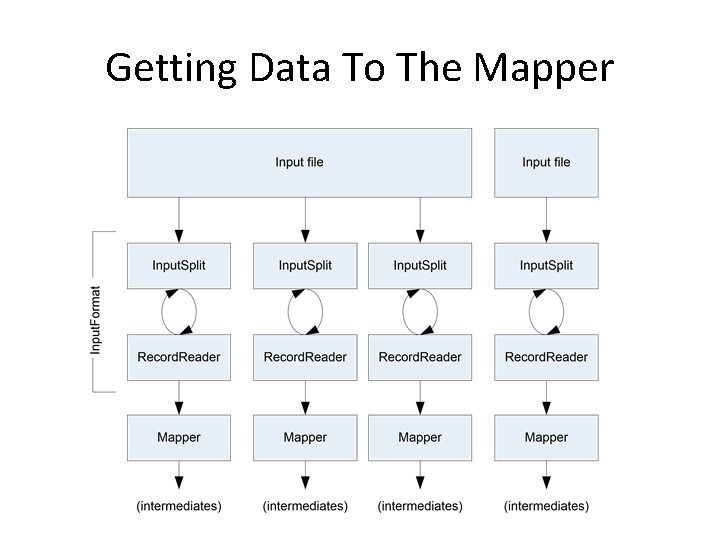
Getting Data To The Mapper
![public static void mainString args throws Exception Configuration conf new Configuration String public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); String[]](https://slidetodoc.com/presentation_image_h/e28c0d8263d24e66ba1dcacfc4fc5087/image-11.jpg)
public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); String[] other. Args = new Generic. Options. Parser(conf, args). get. Remaining. Args(); if (other. Args. length != 2) { System. err. println("Usage: wordcount <in> <out>"); System. exit(2); } Job job = new Job(conf, "word count"); job. set. Jar. By. Class(Word. Count. class); job. set. Mapper. Class(Tokenizer. Mapper. class); job. set. Combiner. Class(Int. Sum. Reducer. class); job. set. Reducer. Class(Int. Sum. Reducer. class); job. set. Output. Key. Class(Text. class); job. set. Output. Value. Class(Int. Writable. class); File. Input. Format. add. Input. Path(job, new Path(other. Args[0])); File. Output. Format. set. Output. Path(job, new Path(other. Args[1])); } System. exit(job. wait. For. Completion(true) ? 0 : 1);
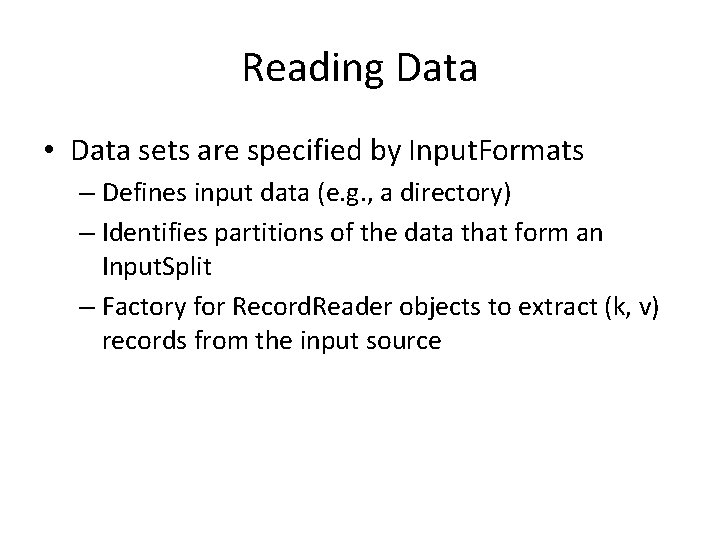
Reading Data • Data sets are specified by Input. Formats – Defines input data (e. g. , a directory) – Identifies partitions of the data that form an Input. Split – Factory for Record. Reader objects to extract (k, v) records from the input source
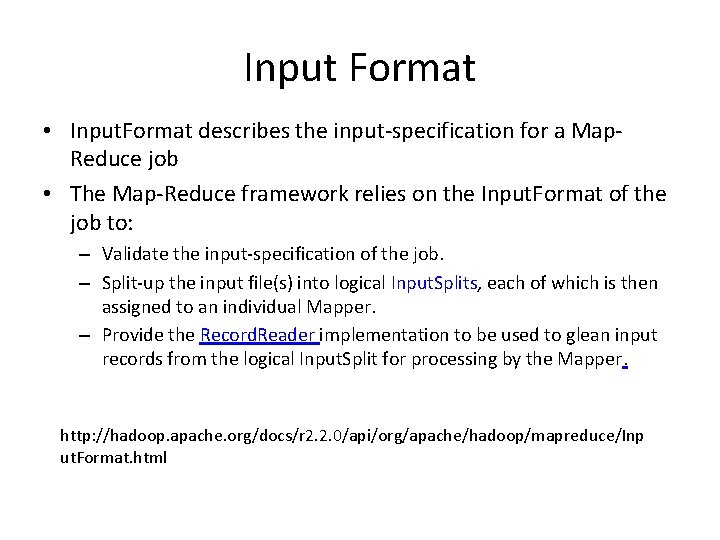
Input Format • Input. Format describes the input-specification for a Map. Reduce job • The Map-Reduce framework relies on the Input. Format of the job to: – Validate the input-specification of the job. – Split-up the input file(s) into logical Input. Splits, each of which is then assigned to an individual Mapper. – Provide the Record. Reader implementation to be used to glean input records from the logical Input. Split for processing by the Mapper. http: //hadoop. apache. org/docs/r 2. 2. 0/api/org/apache/hadoop/mapreduce/Inp ut. Format. html
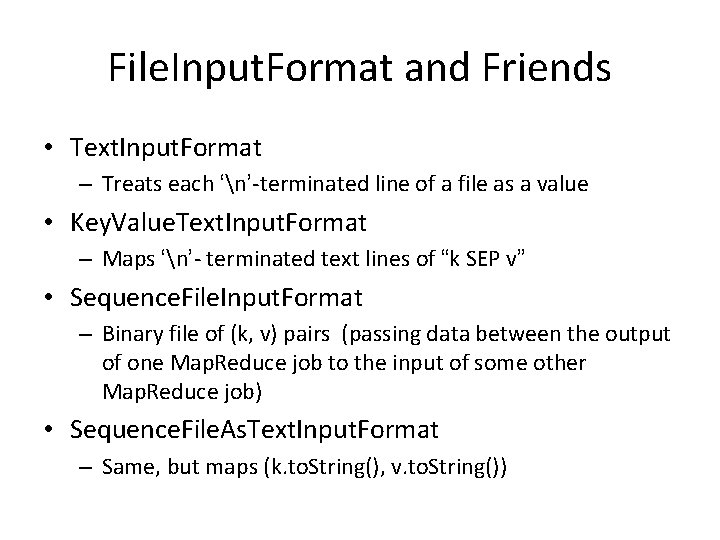
File. Input. Format and Friends • Text. Input. Format – Treats each ‘n’-terminated line of a file as a value • Key. Value. Text. Input. Format – Maps ‘n’- terminated text lines of “k SEP v” • Sequence. File. Input. Format – Binary file of (k, v) pairs (passing data between the output of one Map. Reduce job to the input of some other Map. Reduce job) • Sequence. File. As. Text. Input. Format – Same, but maps (k. to. String(), v. to. String())
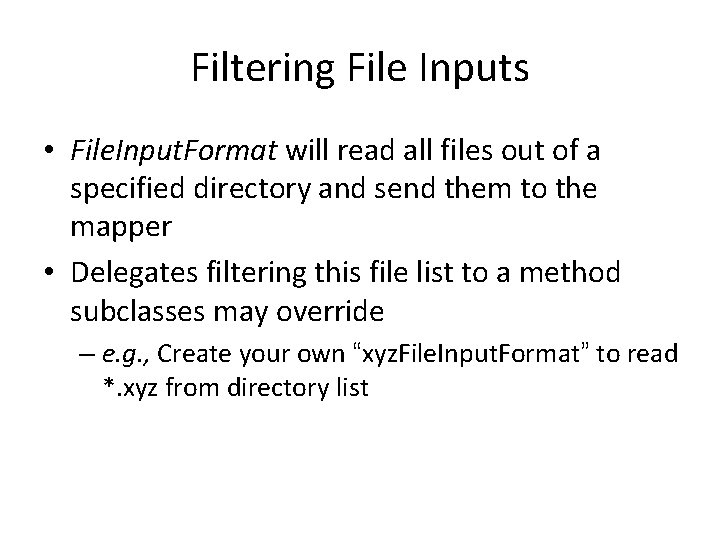
Filtering File Inputs • File. Input. Format will read all files out of a specified directory and send them to the mapper • Delegates filtering this file list to a method subclasses may override – e. g. , Create your own “xyz. File. Input. Format” to read *. xyz from directory list
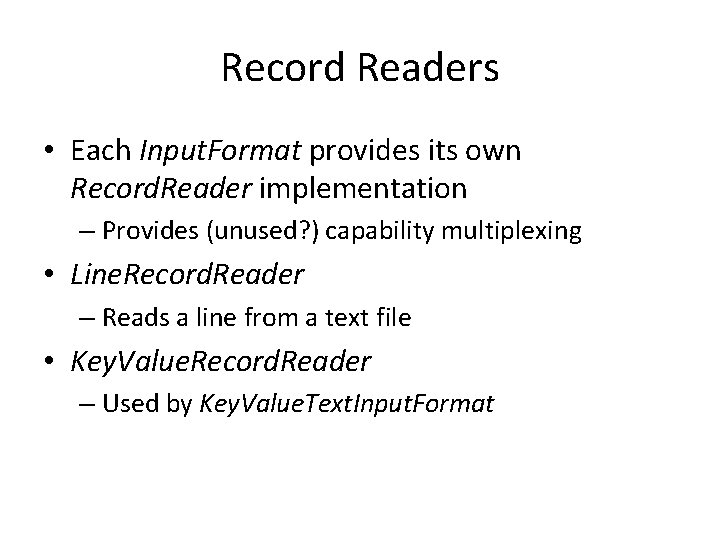
Record Readers • Each Input. Format provides its own Record. Reader implementation – Provides (unused? ) capability multiplexing • Line. Record. Reader – Reads a line from a text file • Key. Value. Record. Reader – Used by Key. Value. Text. Input. Format
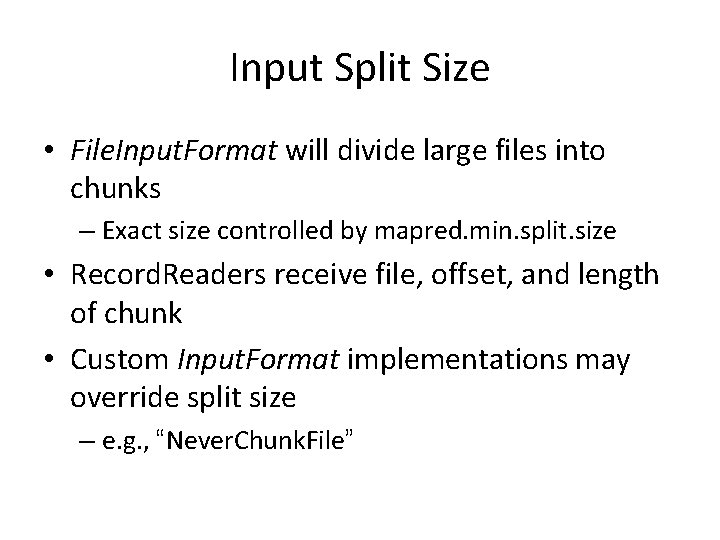
Input Split Size • File. Input. Format will divide large files into chunks – Exact size controlled by mapred. min. split. size • Record. Readers receive file, offset, and length of chunk • Custom Input. Format implementations may override split size – e. g. , “Never. Chunk. File”
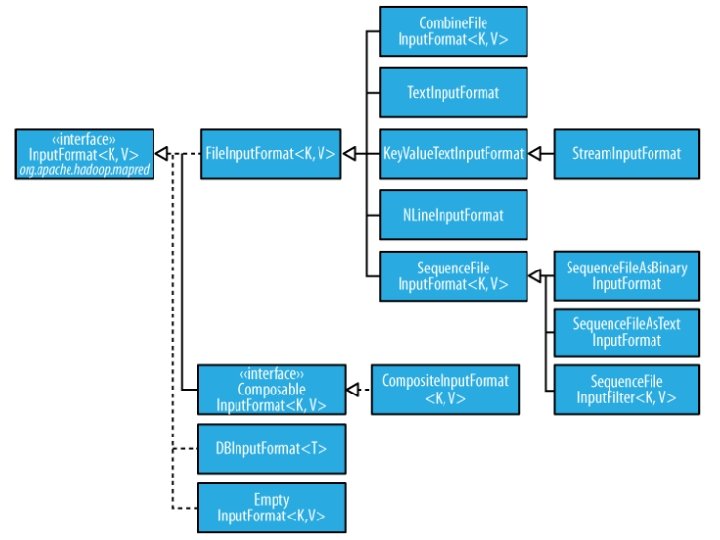
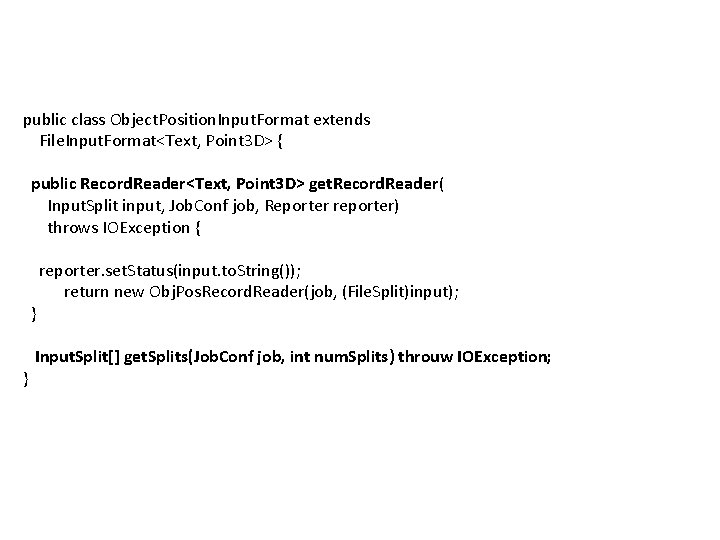
public class Object. Position. Input. Format extends File. Input. Format<Text, Point 3 D> { public Record. Reader<Text, Point 3 D> get. Record. Reader( Input. Split input, Job. Conf job, Reporter reporter) throws IOException { } } reporter. set. Status(input. to. String()); return new Obj. Pos. Record. Reader(job, (File. Split)input); Input. Split[] get. Splits(Job. Conf job, int num. Splits) throuw IOException;
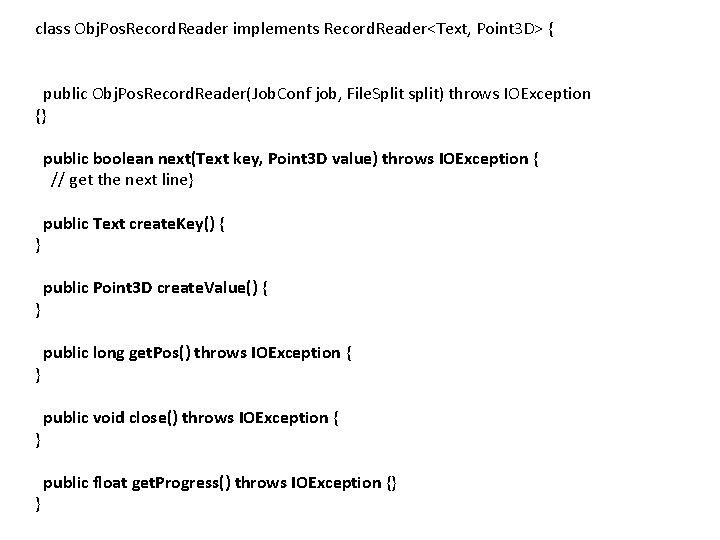
class Obj. Pos. Record. Reader implements Record. Reader<Text, Point 3 D> { public Obj. Pos. Record. Reader(Job. Conf job, File. Split split) throws IOException {} public boolean next(Text key, Point 3 D value) throws IOException { // get the next line} } } public Text create. Key() { public Point 3 D create. Value() { public long get. Pos() throws IOException { public void close() throws IOException { public float get. Progress() throws IOException {}
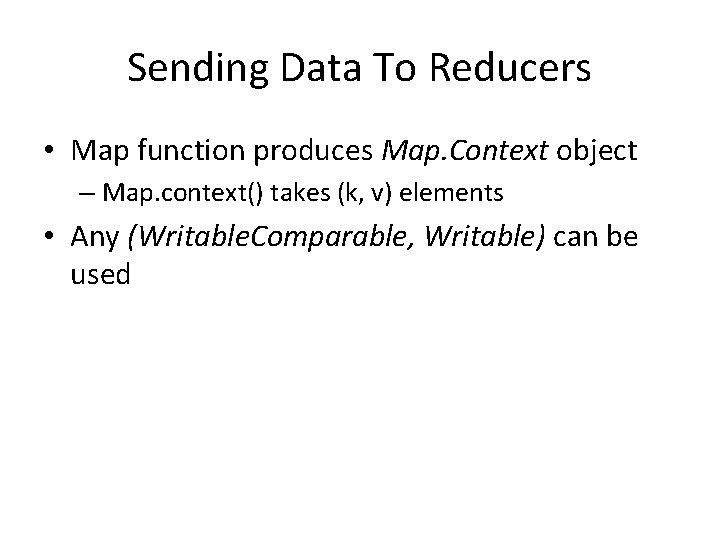
Sending Data To Reducers • Map function produces Map. Context object – Map. context() takes (k, v) elements • Any (Writable. Comparable, Writable) can be used
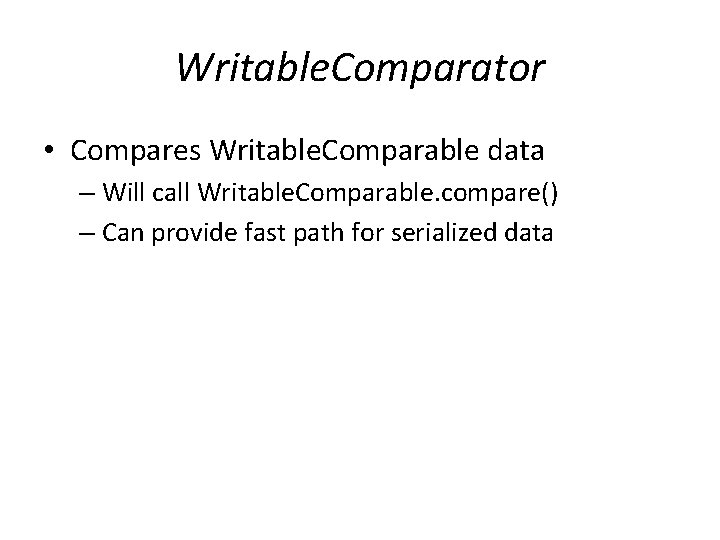
Writable. Comparator • Compares Writable. Comparable data – Will call Writable. Comparable. compare() – Can provide fast path for serialized data
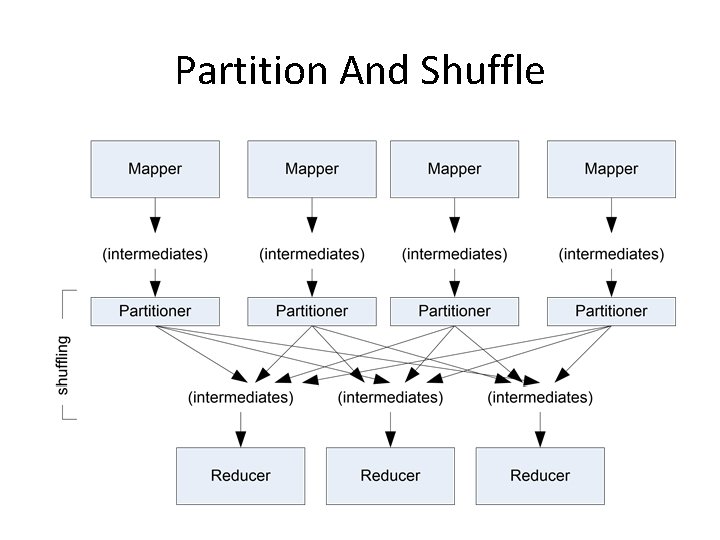
Partition And Shuffle
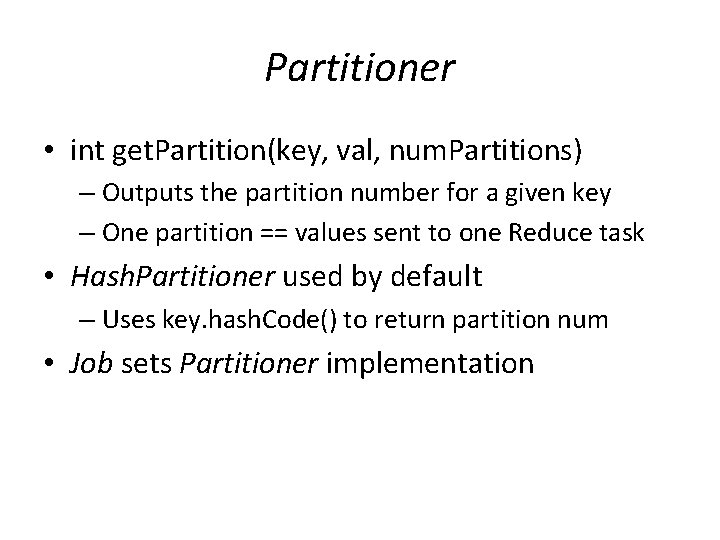
Partitioner • int get. Partition(key, val, num. Partitions) – Outputs the partition number for a given key – One partition == values sent to one Reduce task • Hash. Partitioner used by default – Uses key. hash. Code() to return partition num • Job sets Partitioner implementation
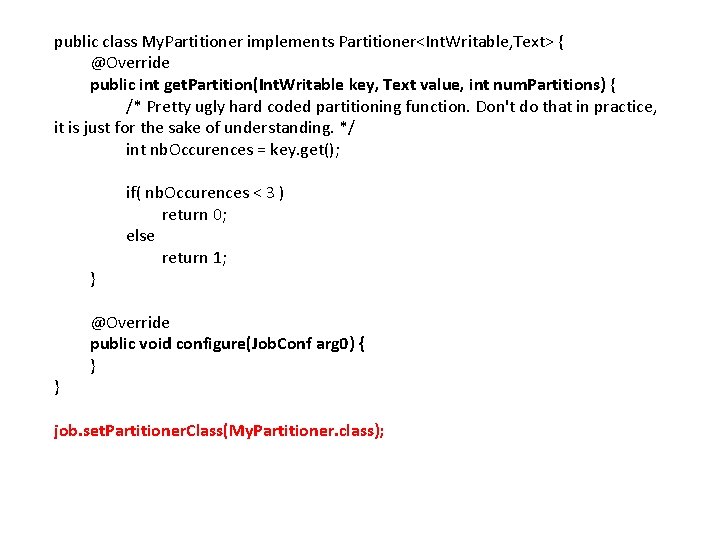
public class My. Partitioner implements Partitioner<Int. Writable, Text> { @Override public int get. Partition(Int. Writable key, Text value, int num. Partitions) { /* Pretty ugly hard coded partitioning function. Don't do that in practice, it is just for the sake of understanding. */ int nb. Occurences = key. get(); } } if( nb. Occurences < 3 ) return 0; else return 1; @Override public void configure(Job. Conf arg 0) { } job. set. Partitioner. Class(My. Partitioner. class);
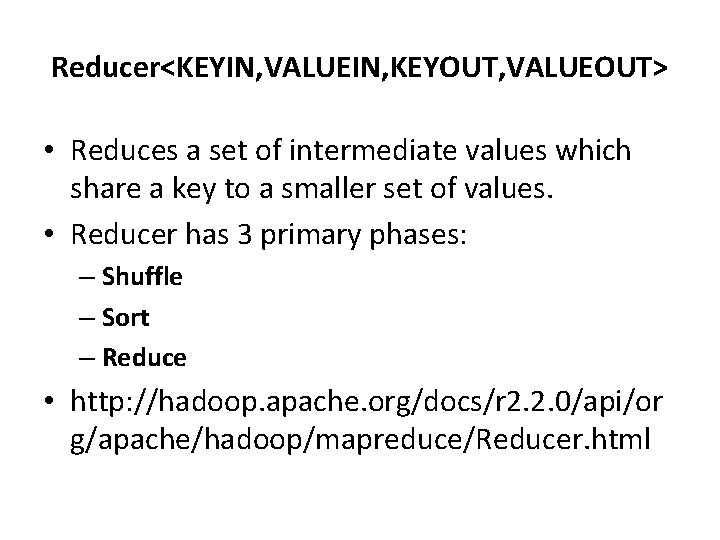
Reducer<KEYIN, VALUEIN, KEYOUT, VALUEOUT> • Reduces a set of intermediate values which share a key to a smaller set of values. • Reducer has 3 primary phases: – Shuffle – Sort – Reduce • http: //hadoop. apache. org/docs/r 2. 2. 0/api/or g/apache/hadoop/mapreduce/Reducer. html
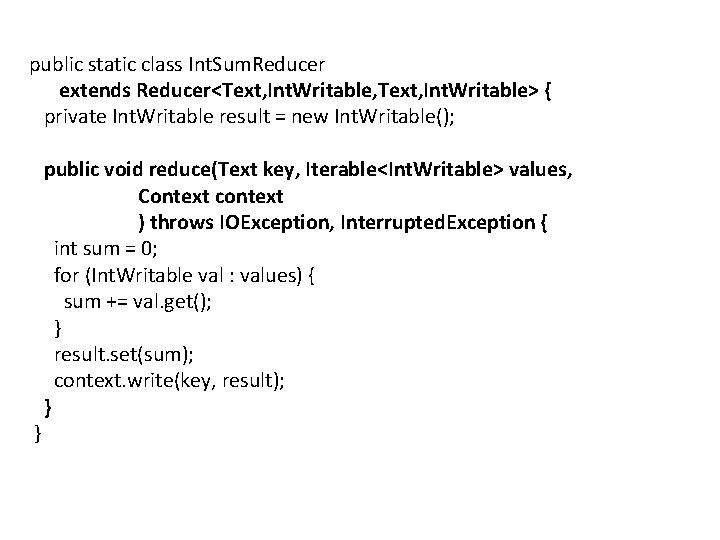
public static class Int. Sum. Reducer extends Reducer<Text, Int. Writable, Text, Int. Writable> { private Int. Writable result = new Int. Writable(); } public void reduce(Text key, Iterable<Int. Writable> values, Context context ) throws IOException, Interrupted. Exception { int sum = 0; for (Int. Writable val : values) { sum += val. get(); } result. set(sum); context. write(key, result); }
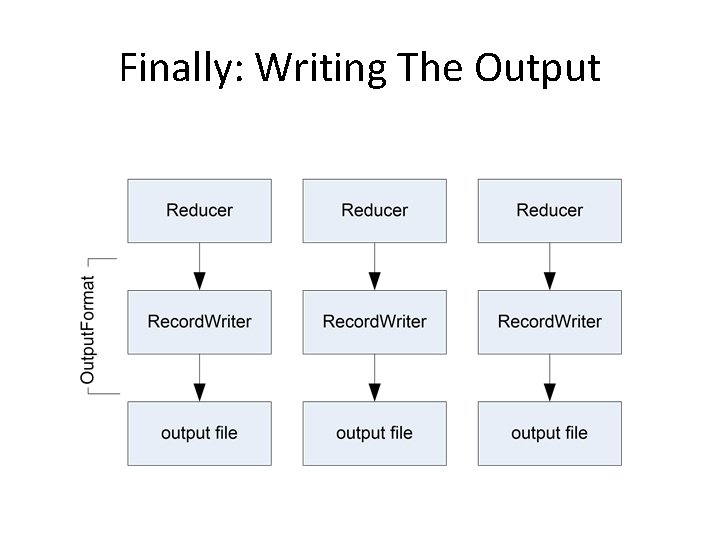
Finally: Writing The Output
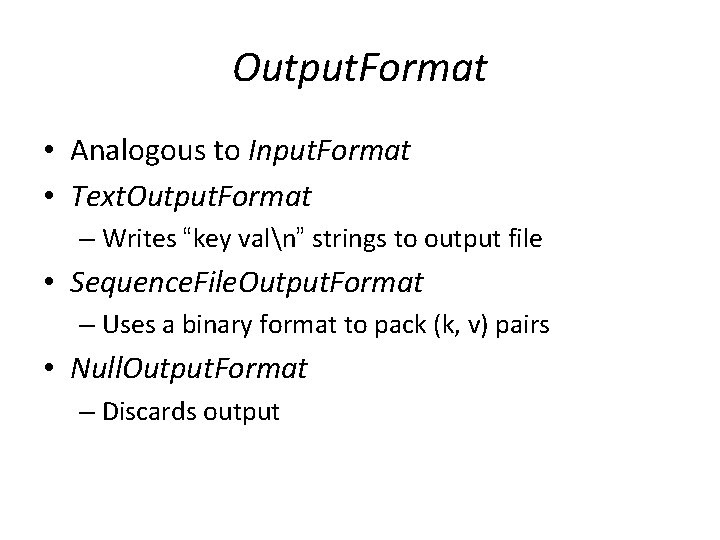
Output. Format • Analogous to Input. Format • Text. Output. Format – Writes “key valn” strings to output file • Sequence. File. Output. Format – Uses a binary format to pack (k, v) pairs • Null. Output. Format – Discards output
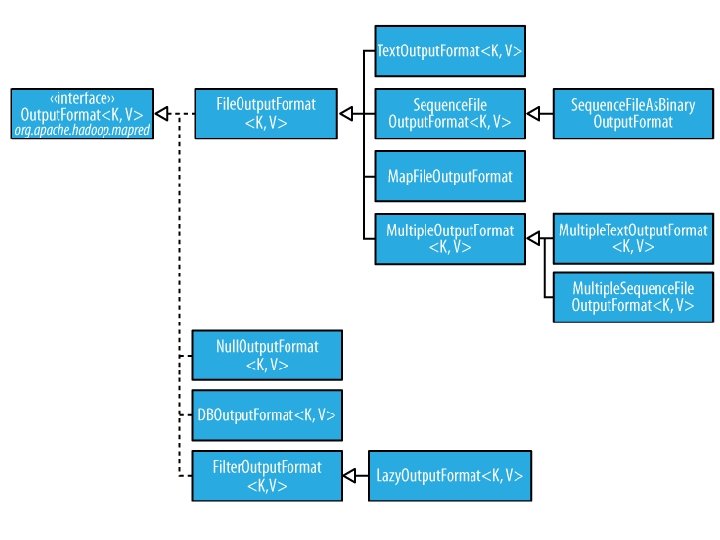
![public static void mainString args throws Exception Configuration conf new Configuration String public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); String[]](https://slidetodoc.com/presentation_image_h/e28c0d8263d24e66ba1dcacfc4fc5087/image-31.jpg)
public static void main(String[] args) throws Exception { Configuration conf = new Configuration(); String[] other. Args = new Generic. Options. Parser(conf, args). get. Remaining. Args(); if (other. Args. length != 2) { System. err. println("Usage: wordcount <in> <out>"); System. exit(2); } Job job = new Job(conf, "word count"); job. set. Jar. By. Class(Word. Count. class); job. set. Mapper. Class(Tokenizer. Mapper. class); job. set. Combiner. Class(Int. Sum. Reducer. class); job. set. Reducer. Class(Int. Sum. Reducer. class); job. set. Output. Key. Class(Text. class); job. set. Output. Value. Class(Int. Writable. class); File. Input. Format. add. Input. Path(job, new Path(other. Args[0])); File. Output. Format. set. Output. Path(job, new Path(other. Args[1])); } System. exit(job. wait. For. Completion(true) ? 0 : 1);
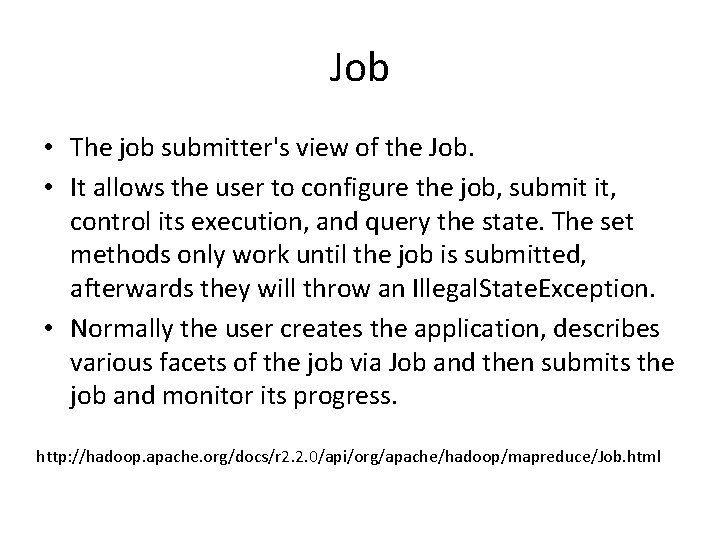
Job • The job submitter's view of the Job. • It allows the user to configure the job, submit it, control its execution, and query the state. The set methods only work until the job is submitted, afterwards they will throw an Illegal. State. Exception. • Normally the user creates the application, describes various facets of the job via Job and then submits the job and monitor its progress. http: //hadoop. apache. org/docs/r 2. 2. 0/api/org/apache/hadoop/mapreduce/Job. html
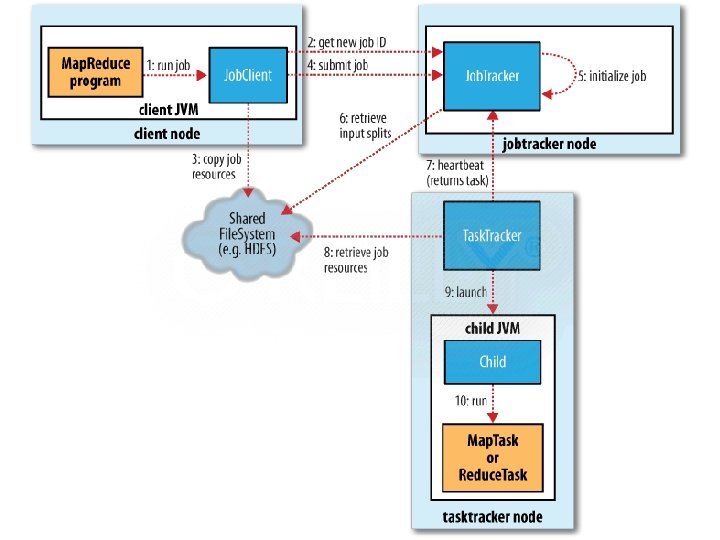
Input formats in hadoop
Hadoop file formats
Hadoop i/o compression and serialization
Ibm 360 machine structure
Map reduce types
+hadoop programming
Android programming overview
Mapreduce شرح
Map reduce word count
Google map reduce
Map reduce combine
Mapreduce combiner
Map reduce algorithm
Map reduce paper
Map-reduce
Map reduce program
Map-reduce
Sherpamap
Sanjay ghemawat
Java map reduce
Lisp map reduce
Google map reduce
Peripheral input (perangkat input) adalah…
Finely tuned input and roughly tuned input
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
System programming definition
Linear vs integer programming
Perbedaan linear programming dan integer programming
Montaj maksud
List various rapid prototyping data formats
Rtf in multimedia
File formats in multimedia
File formats in multimedia