Development Steps for a C Program The XCode
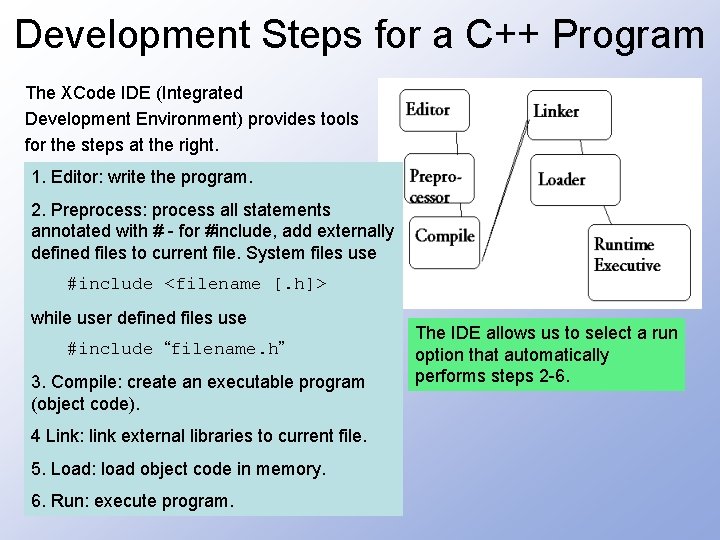
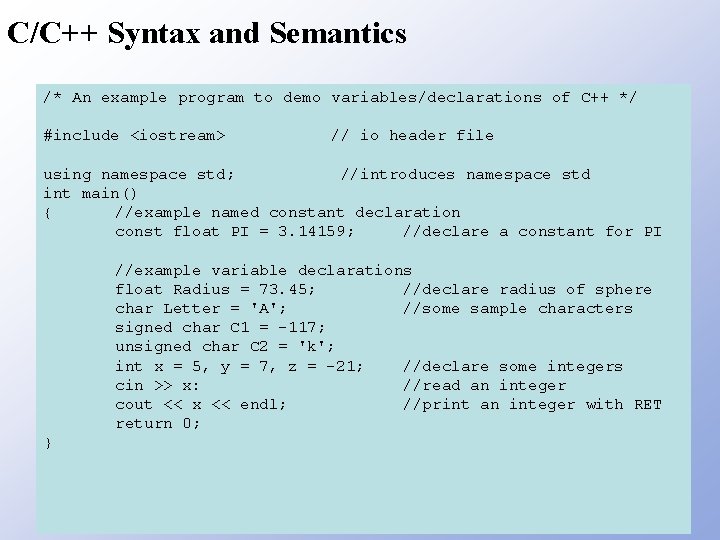
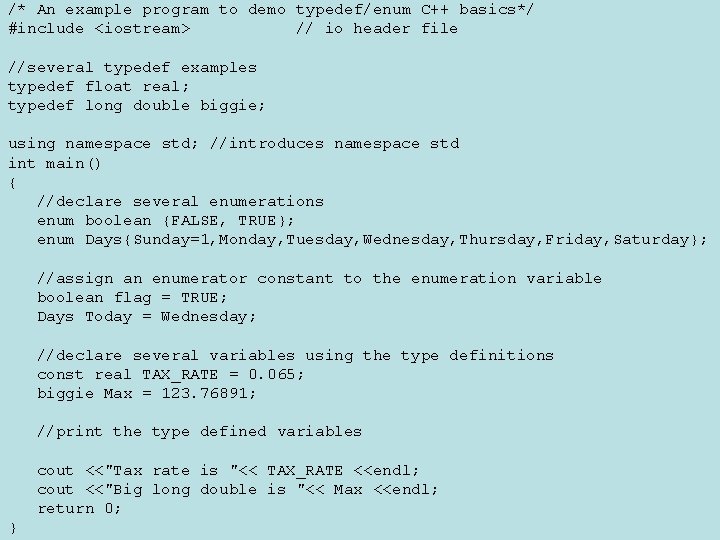
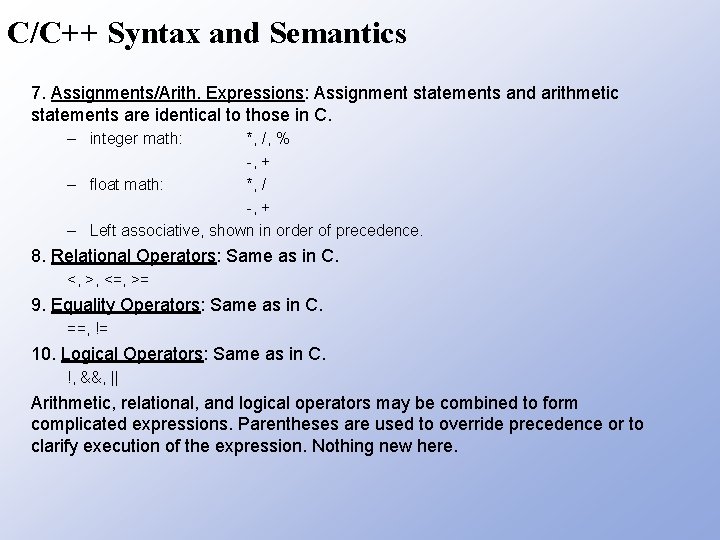
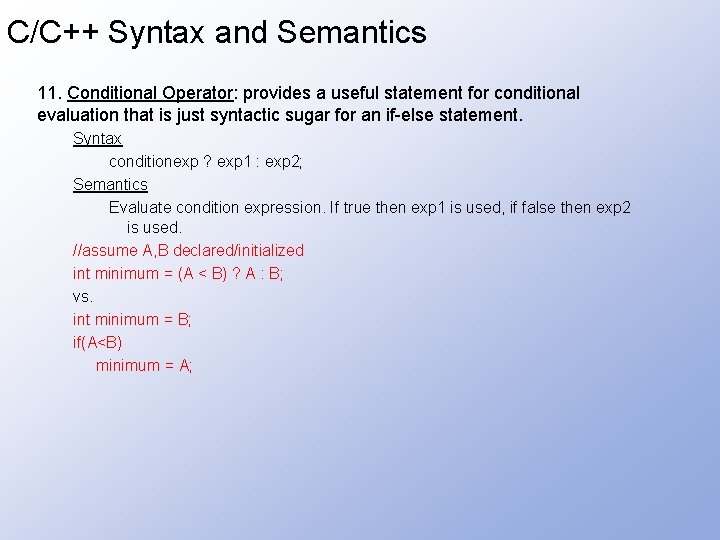
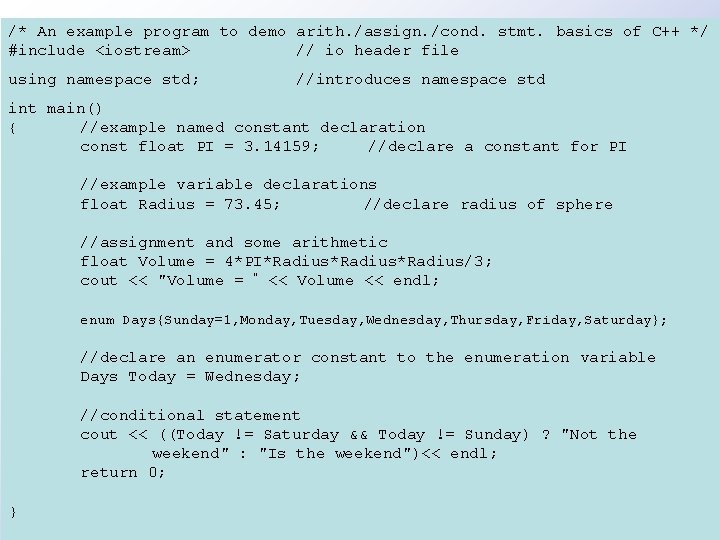
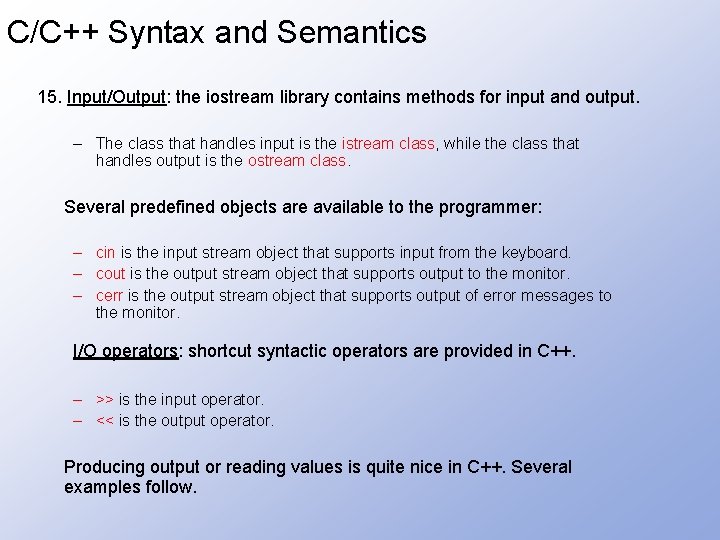
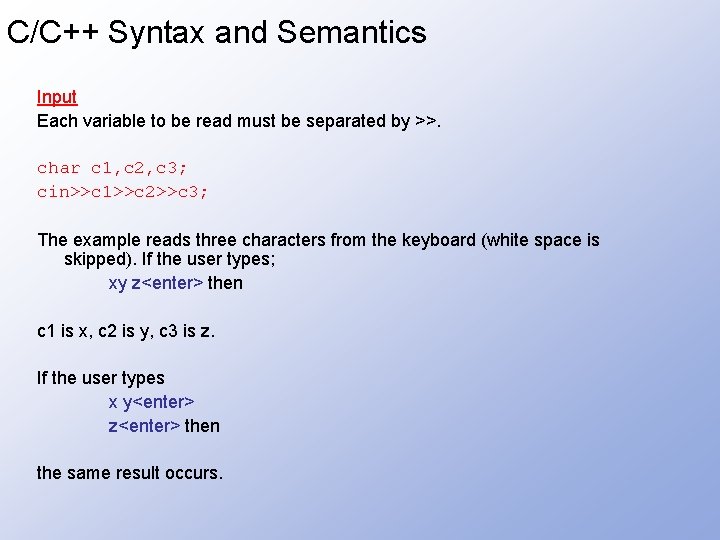
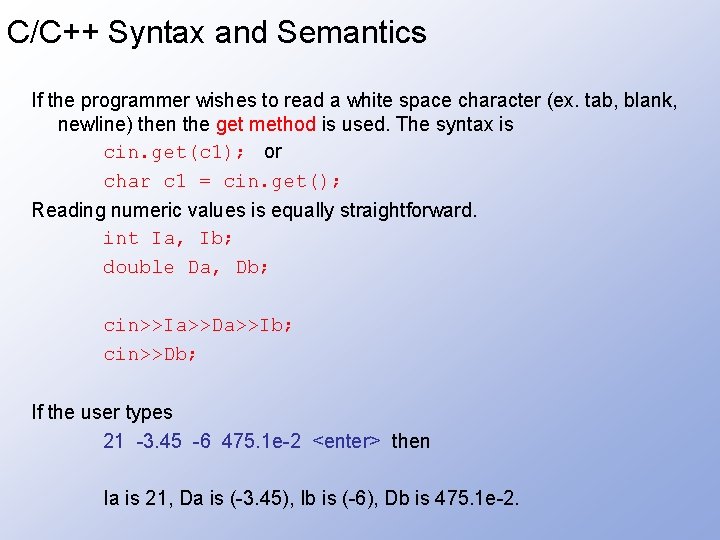
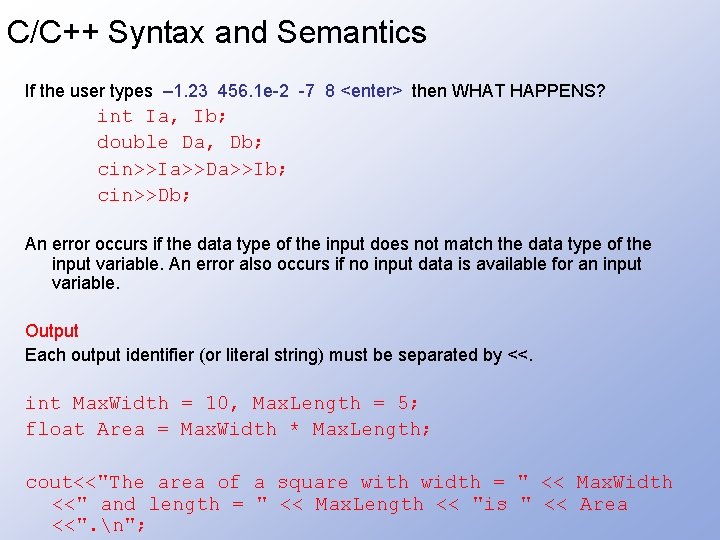
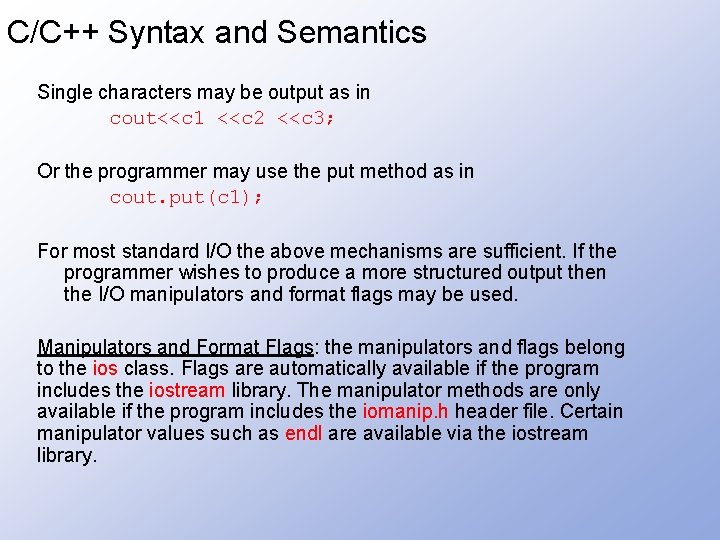
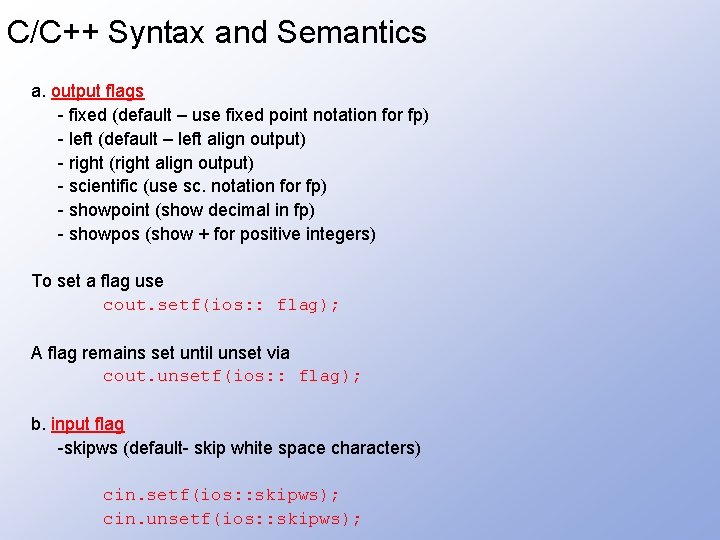
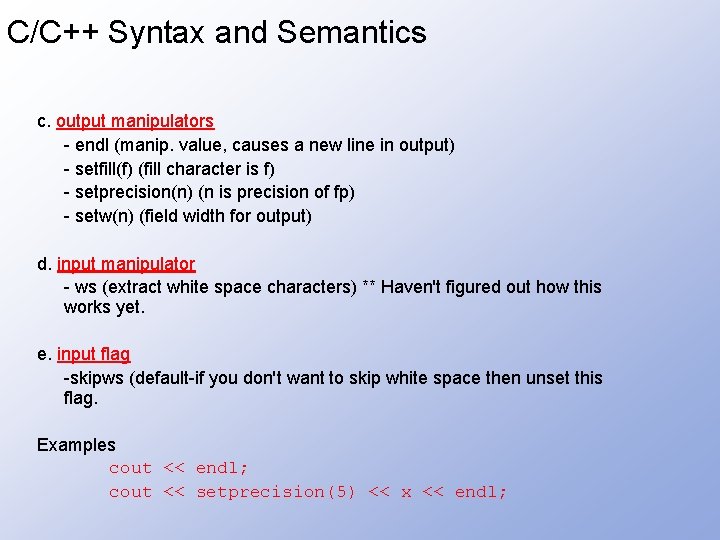
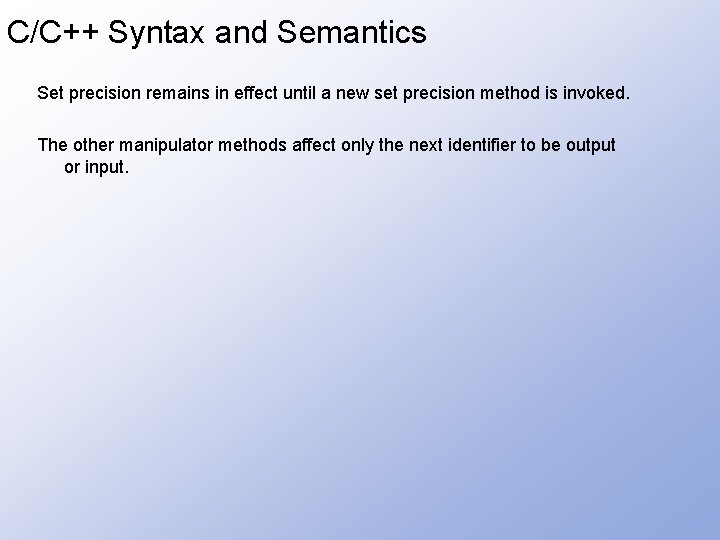
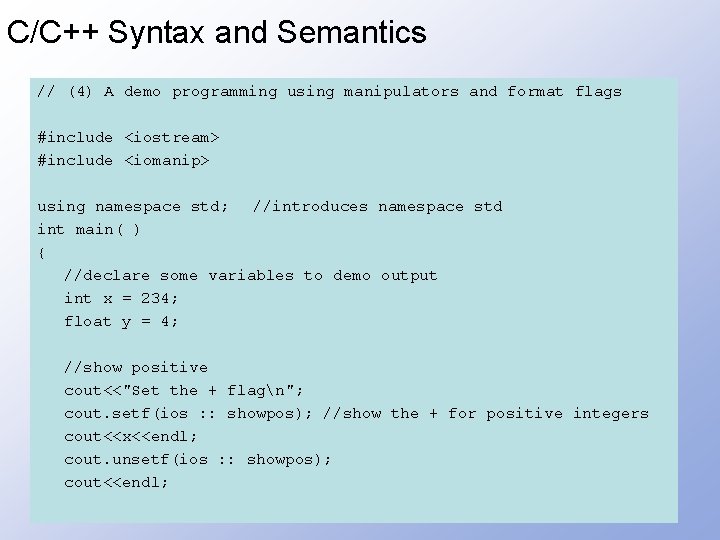
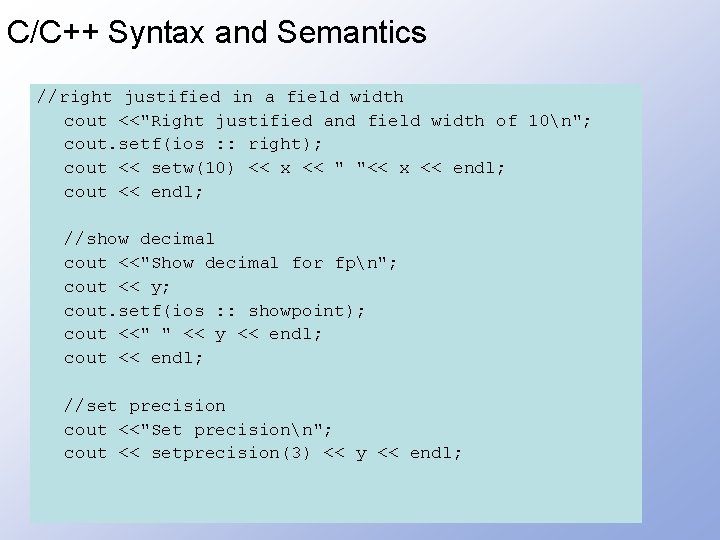
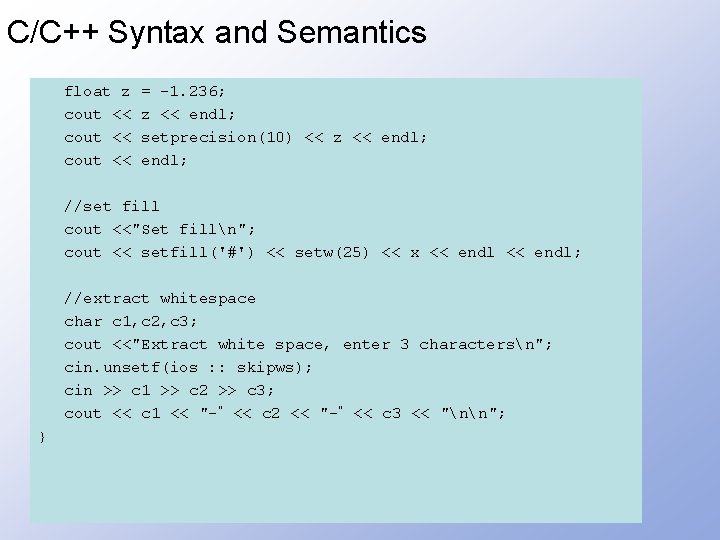
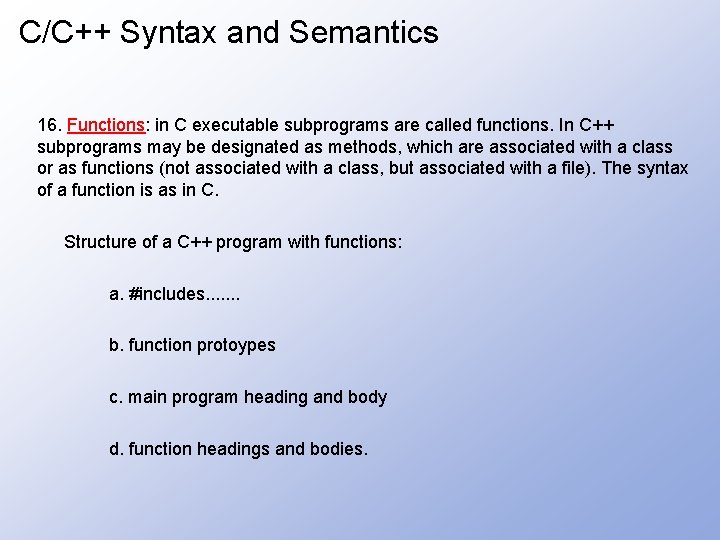
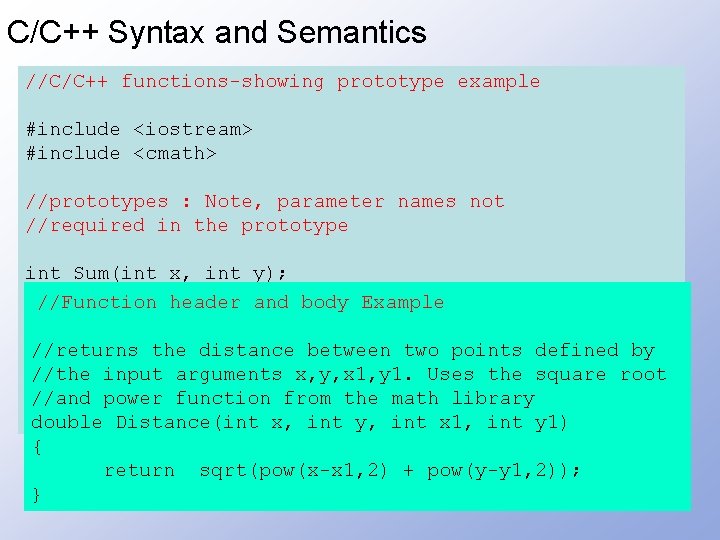
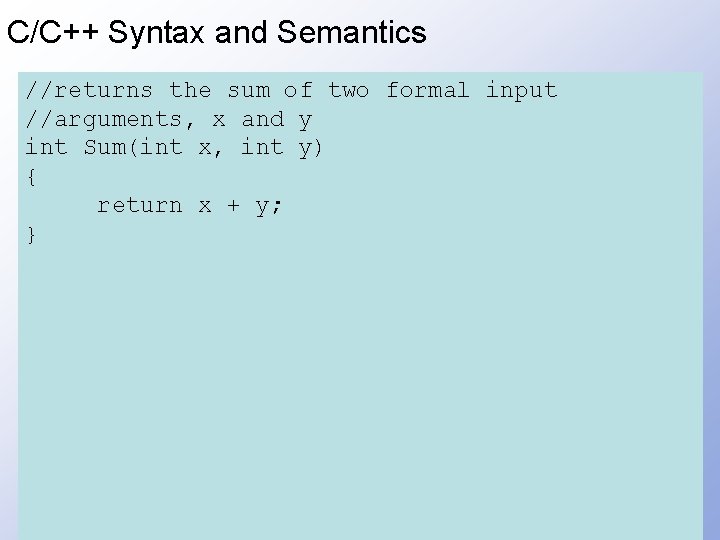
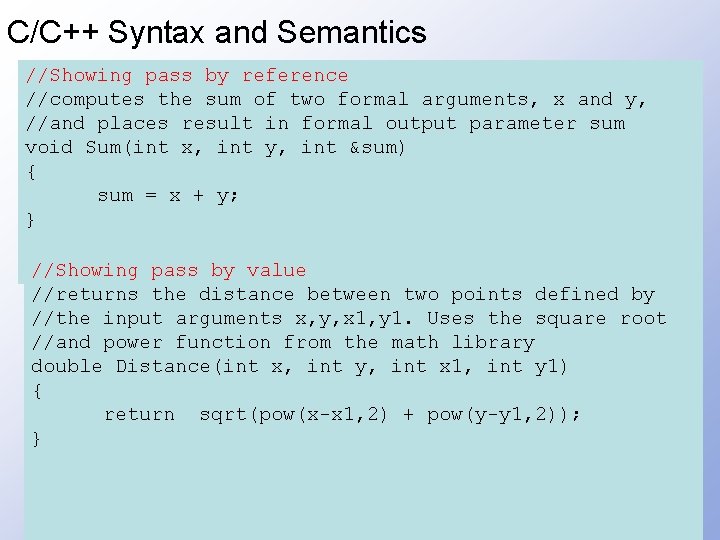
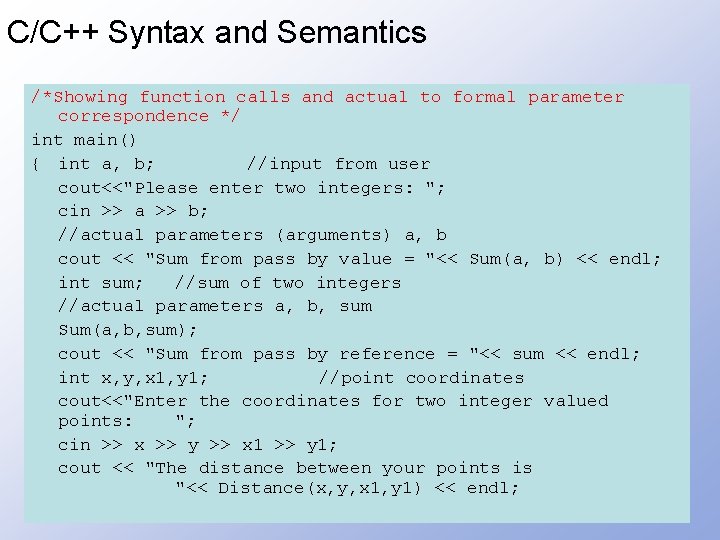
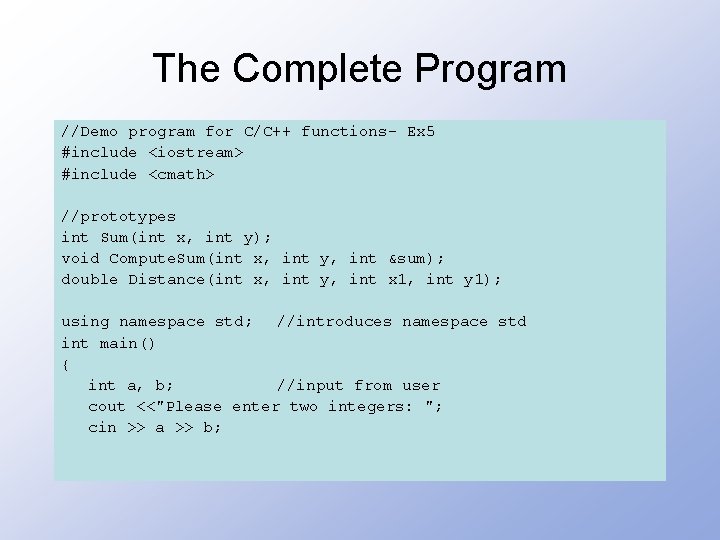
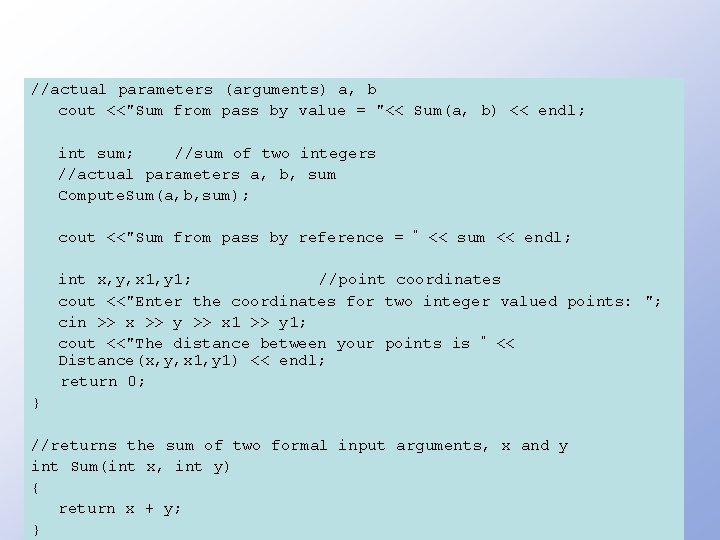
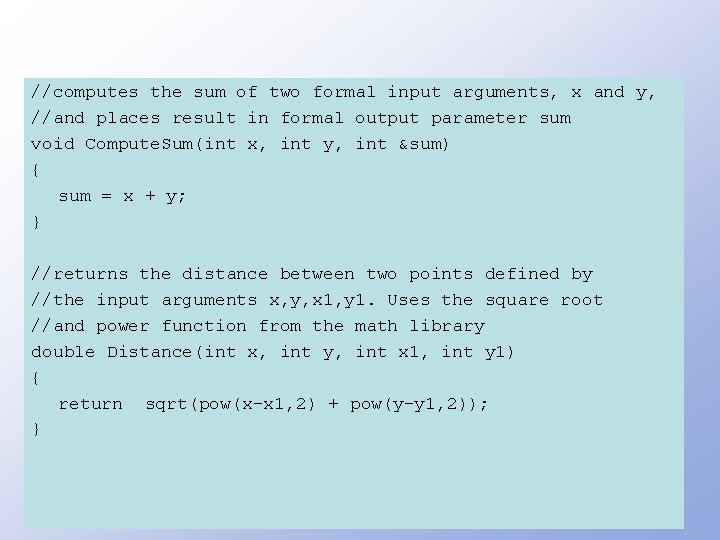
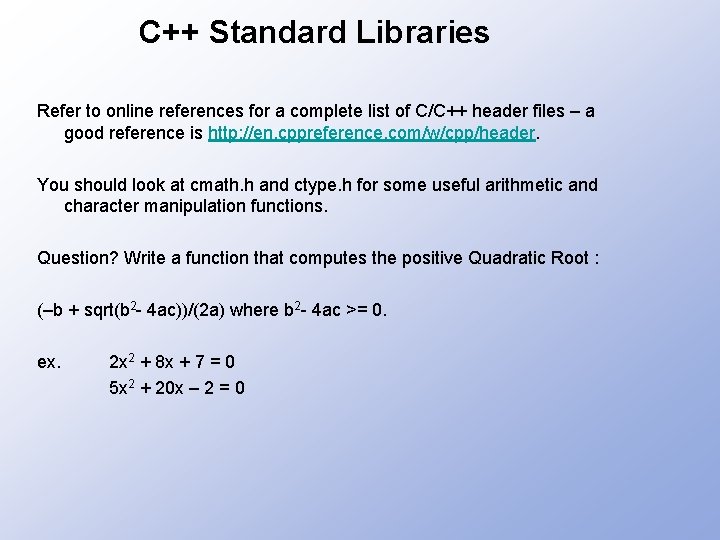
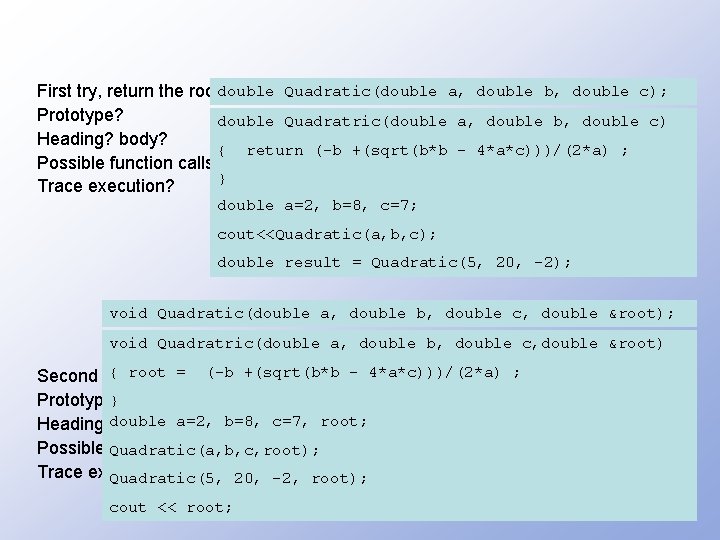
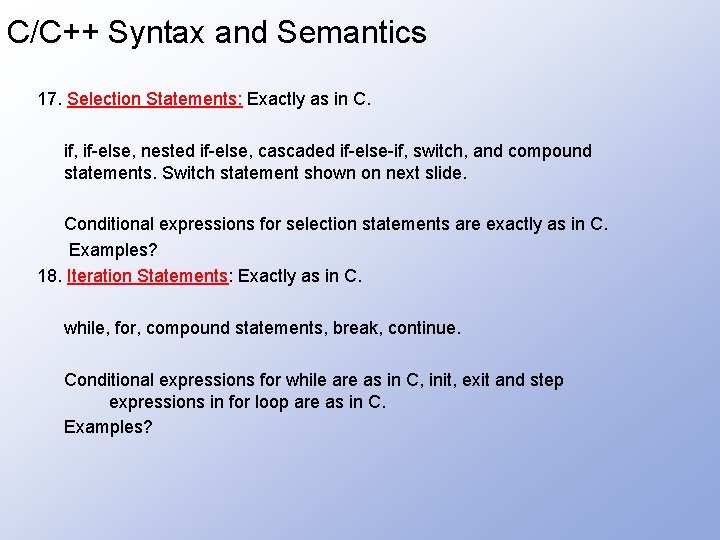
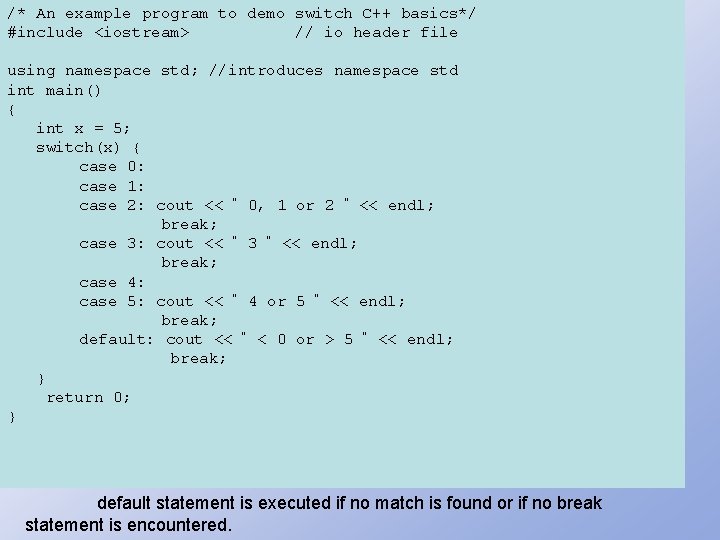
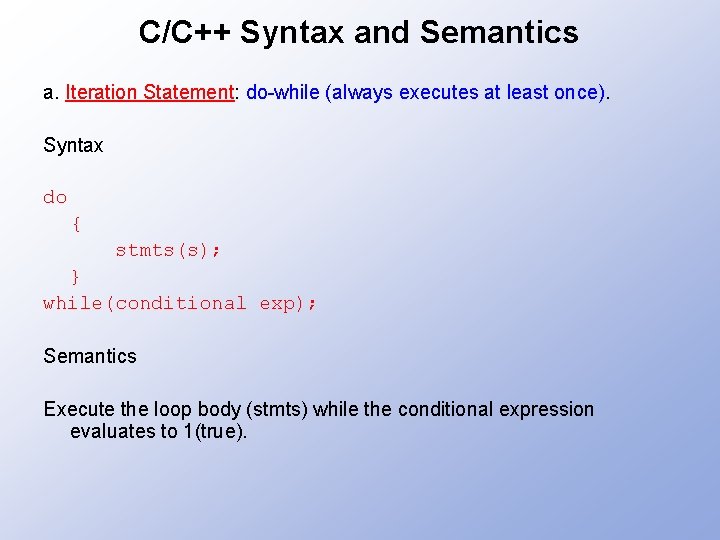
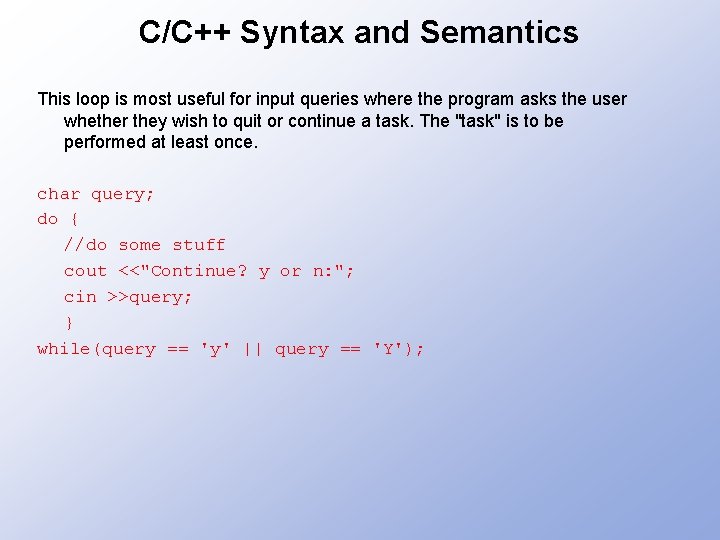
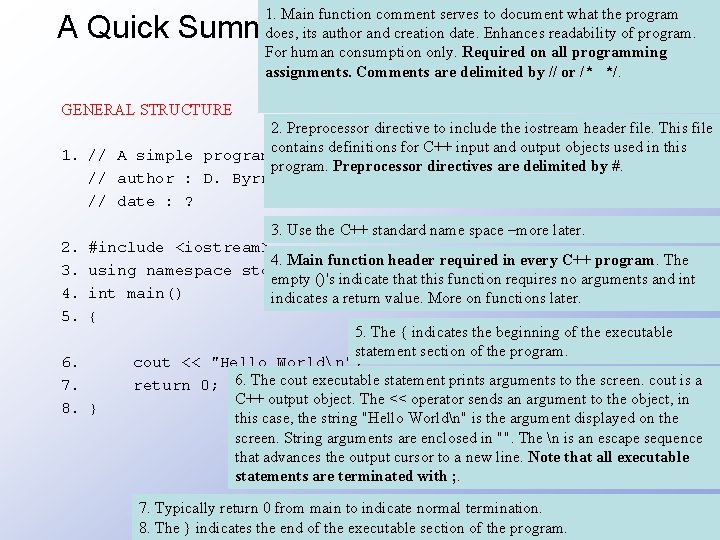
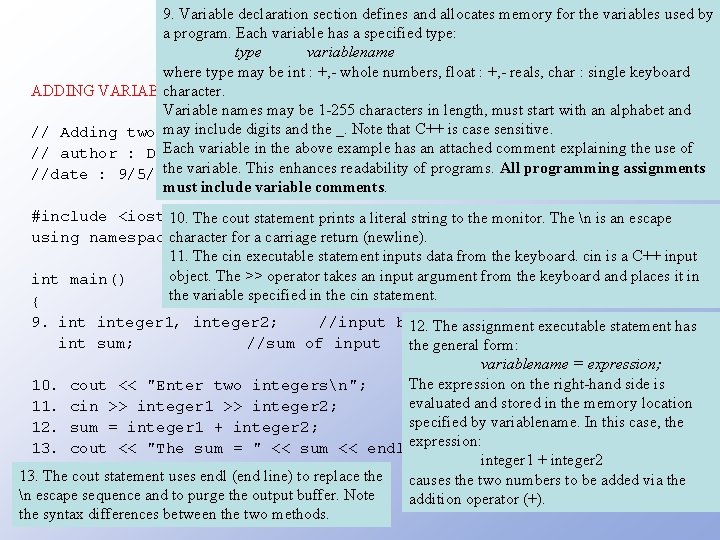
- Slides: 33
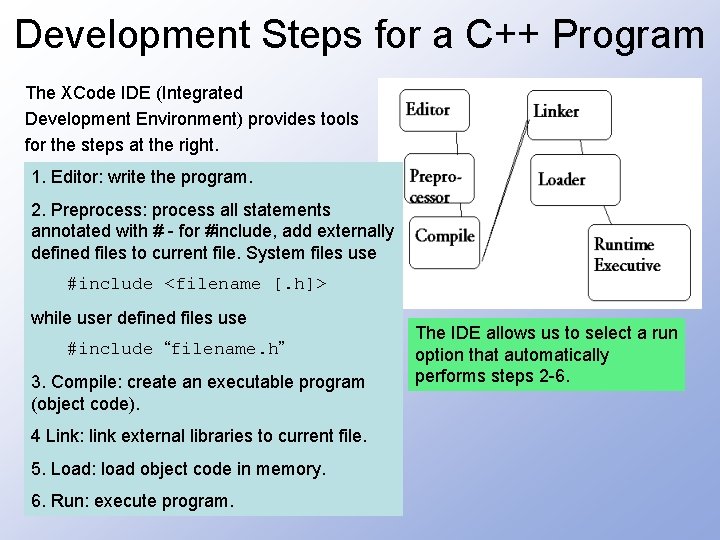
Development Steps for a C++ Program The XCode IDE (Integrated Development Environment) provides tools for the steps at the right. 1. Editor: write the program. 2. Preprocess: process all statements annotated with # - for #include, add externally defined files to current file. System files use #include <filename [. h]> while user defined files use #include “filename. h” 3. Compile: create an executable program (object code). 4 Link: link external libraries to current file. 5. Load: load object code in memory. 6. Run: execute program. The IDE allows us to select a run option that automatically performs steps 2 -6.
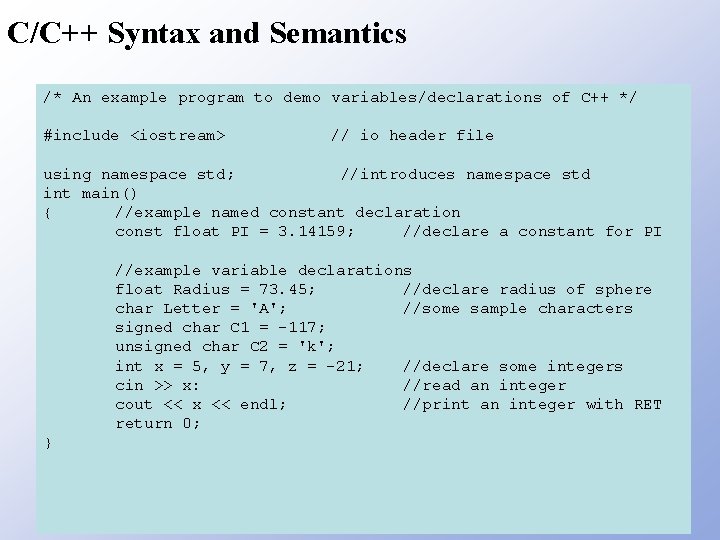
C/C++ Syntax and Semantics /* An example program to demo variables/declarations of C++ */ • Variables: a C++ #include <iostream> (case sensitive) associated with a // io identifier header file data type-starts with a letter, followed by a sequence of alphausing namespace std numerics andstd; the underscore. //introduces The value of namespace a variable may int main() change during named program execution. Each variable is given a { //example constant declaration memory through a variable declaration. constlocation float PI = 3. 14159; //declare a constant for • • } PI //example variable declarations Variable syntax (see Ex. cpp). Syntax is identical to floatdeclaration: Radius = 73. 45; //declare radius of sphere char Letter. Variables = 'A'; may be declared //some in sample characters C declarations. a list using signed. Variables char C 1 may = -117; commas. be declared and assigned a value in a unsigned char C 2 = 'k'; declaration. be declared with a data int x = Variables 5, y = 7, must z = -21; //declare some type. integers cin >> x: //read an integer cout << x << endl; //print an integer with RET Named constants: a C++ identifier associated with a data type return 0; but whose value may not change during program execution. Why are named constants important?
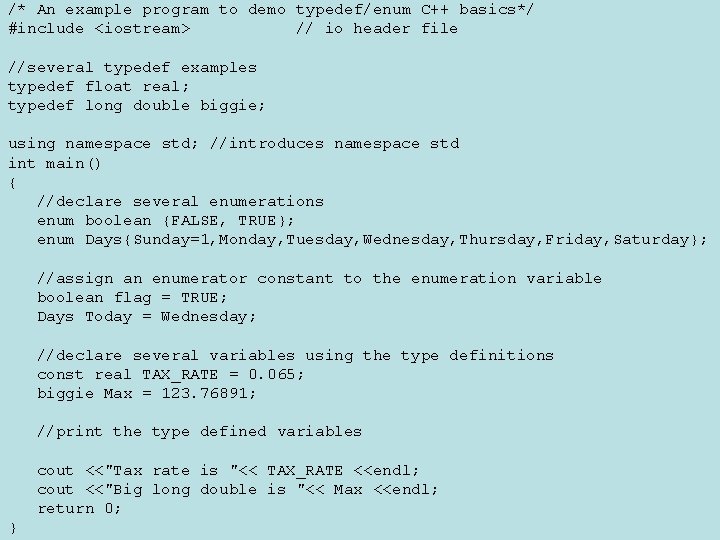
/* An example program to demo typedef/enum C++ basics*/ #include <iostream> // io file 5. Enumerations: an Enumerated data typeheader is merely syntactic sugar for an integer range of values. If we wish to manipulate the days of the week, months //several examples of the year, typedef a list of tree names; we could do so with a range of characters or typedef float real; integers but such a range is probably not meaningful to a reader. It is certainly typedef longtodouble biggie; more useful specify an enumerated type (see Ex. cpp)-more later. using namespace std; //introduces namespace std – The enumerator constants are specified in a list. The first constant is given the int main() value 0 (unless otherwise specified), the second the value 1, the third the value { 2, and so on. It is possible to associate a unique value (other than a sequence of //declare enumerations integers)several to each constant. enum boolean {FALSE, TRUE}; enum Days{Sunday=1, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday}; – Enumerated variables cannot be printed nor may values be read into enumerations. //assign an enumerator constant to the enumeration variable boolean flag = TRUE; Todayallow = Wednesday; 6. Days Typedefs: users to specify an alias (nickname) for an existing data type. At first glance this seems silly, but it is very useful for array types, and is //declare several variables usingatthe type definitions quite handy if the type has to be modified a later date (see Ex. cpp). Typedefs const TAX_RATE = 0. 065; If you wish the typedef to be visible to an may occurreal anywhere in a program. biggie entire file it. Max can =be 123. 76891; placed before the main program heading. //print the type defined variables cout <<"Tax rate is "<< TAX_RATE <<endl; cout <<"Big long double is "<< Max <<endl; return 0; }
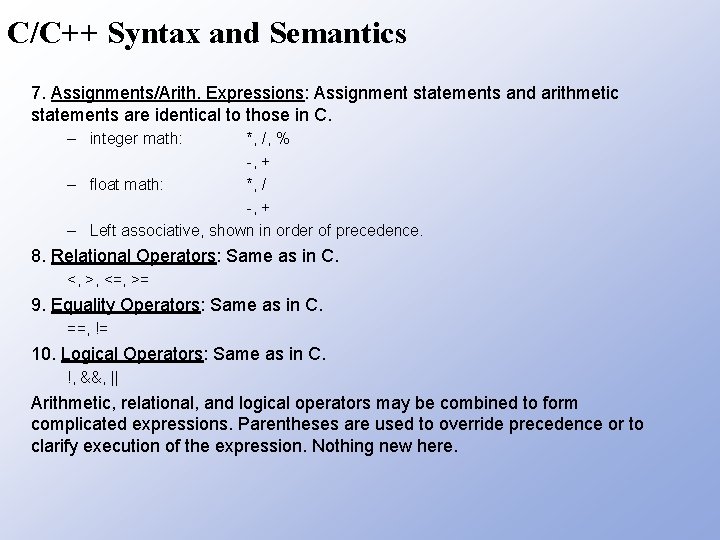
C/C++ Syntax and Semantics 7. Assignments/Arith. Expressions: Assignment statements and arithmetic statements are identical to those in C. – integer math: *, /, % -, + – float math: *, / -, + – Left associative, shown in order of precedence. 8. Relational Operators: Same as in C. <, >, <=, >= 9. Equality Operators: Same as in C. ==, != 10. Logical Operators: Same as in C. !, &&, || Arithmetic, relational, and logical operators may be combined to form complicated expressions. Parentheses are used to override precedence or to clarify execution of the expression. Nothing new here.
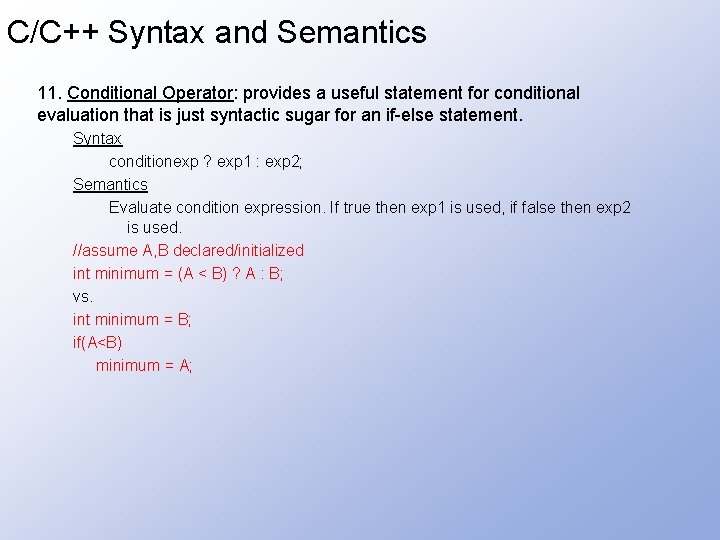
C/C++ Syntax and Semantics 11. Conditional Operator: provides a useful statement for conditional evaluation that is just syntactic sugar for an if-else statement. Syntax conditionexp ? exp 1 : exp 2; Semantics Evaluate condition expression. If true then exp 1 is used, if false then exp 2 is used. //assume A, B declared/initialized int minimum = (A < B) ? A : B; vs. int minimum = B; if(A<B) minimum = A;
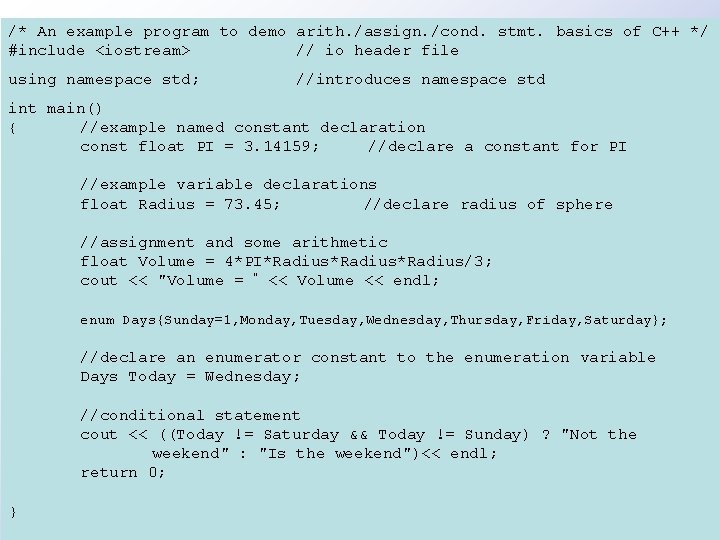
/* An example program to demo arith. /assign. /cond. stmt. basics of C++ */ #include <iostream> //integral io header file are mixed within an 12. Implicit type conversions: when data types expression, the result will be the integral promotion of the "largest data type" //introduces namespace std found in the expression. The conversion proceeds as; int main() char(short)->int->unsigned int->long->float->double->long double. using namespace std; { //example named constant declaration const float PI = 3. 14159; //declare a constant for PI 13. Explicit type conversions: as in Java, if information can be lost, the programmer must variable specify an declarations explicit type conversion. //example float Radius = 73. 45; //declare radius of sphere a. type(expression) //assignment and some arithmetic cout<< int(Volume); float Volume = 4*PI*Radius*Radius/3; cout << "Volume = ” << Volume << endl; b. casting; (type) expression enum Days{Sunday=1, Monday, Tuesday, Wednesday, Thursday, Friday, Saturday}; cout << (int) Volume; //declare an enumerator constant to the enumeration variable 14. Multiple Days assignment/Assignment Today = Wednesday; operators: assignment is right associative thus int A = B = C =statement 5; //valid in C++ //conditional cout << operators: ((Today != Saturday && Today != Sunday) ? "Not the Assignment Same as in Java. "Is-- the weekend")<< endl; +=, -=, weekend" %=, *=, /=, : ++, return 0; }
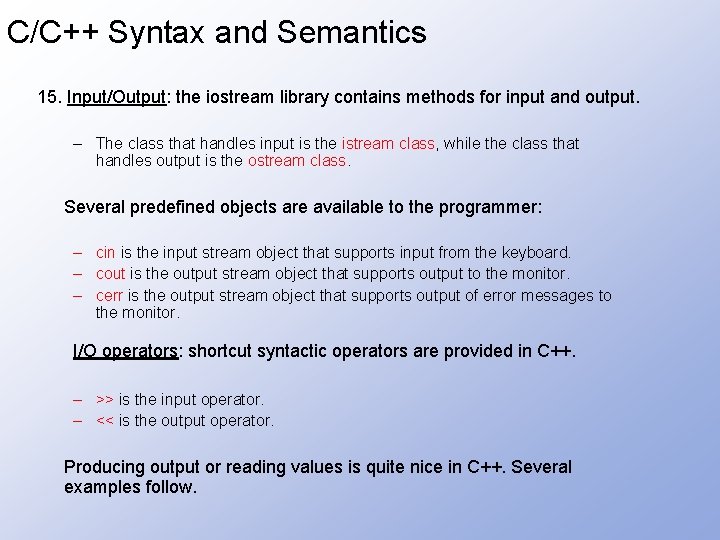
C/C++ Syntax and Semantics 15. Input/Output: the iostream library contains methods for input and output. – The class that handles input is the istream class, while the class that handles output is the ostream class. Several predefined objects are available to the programmer: – cin is the input stream object that supports input from the keyboard. – cout is the output stream object that supports output to the monitor. – cerr is the output stream object that supports output of error messages to the monitor. I/O operators: shortcut syntactic operators are provided in C++. – >> is the input operator. – << is the output operator. Producing output or reading values is quite nice in C++. Several examples follow.
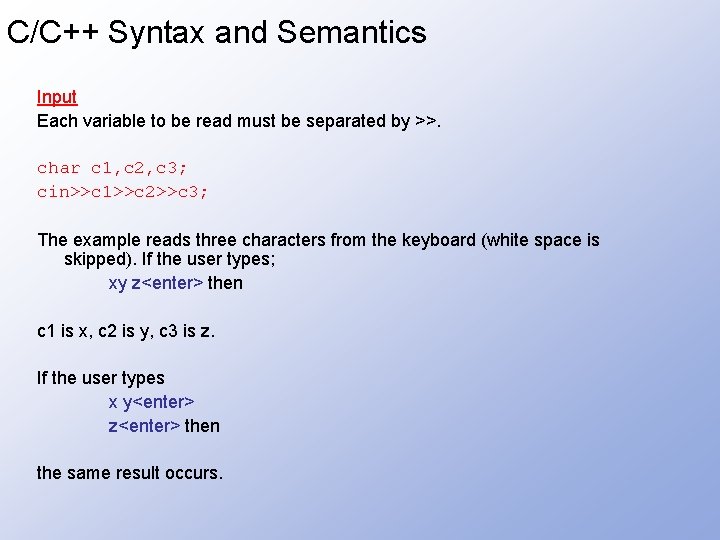
C/C++ Syntax and Semantics Input Each variable to be read must be separated by >>. char c 1, c 2, c 3; cin>>c 1>>c 2>>c 3; The example reads three characters from the keyboard (white space is skipped). If the user types; xy z<enter> then c 1 is x, c 2 is y, c 3 is z. If the user types x y<enter> z<enter> then the same result occurs.
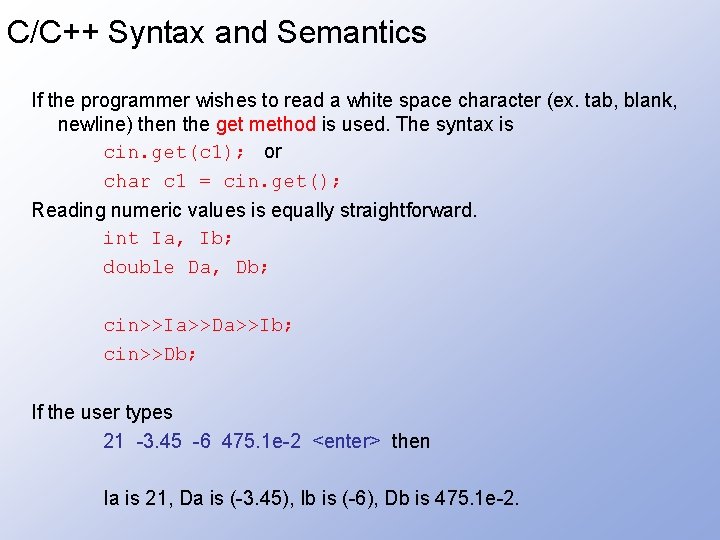
C/C++ Syntax and Semantics If the programmer wishes to read a white space character (ex. tab, blank, newline) then the get method is used. The syntax is cin. get(c 1); or char c 1 = cin. get(); Reading numeric values is equally straightforward. int Ia, Ib; double Da, Db; cin>>Ia>>Da>>Ib; cin>>Db; If the user types 21 -3. 45 -6 475. 1 e-2 <enter> then Ia is 21, Da is (-3. 45), Ib is (-6), Db is 475. 1 e-2.
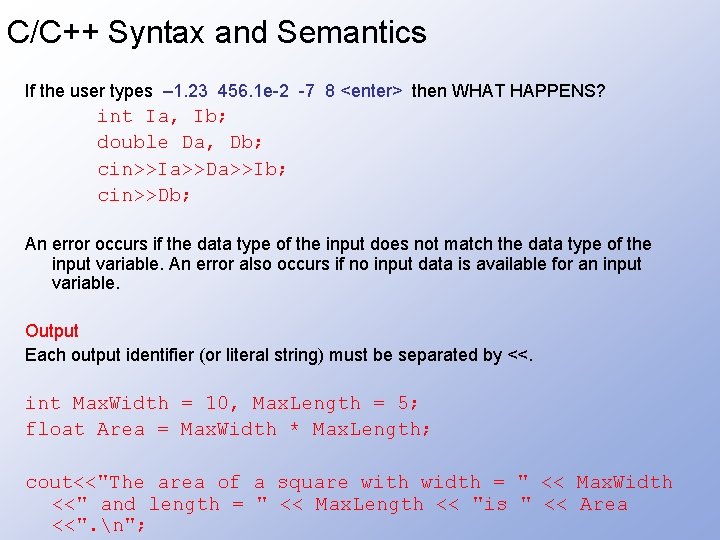
C/C++ Syntax and Semantics If the user types – 1. 23 456. 1 e-2 -7 8 <enter> then WHAT HAPPENS? int Ia, Ib; double Da, Db; cin>>Ia>>Da>>Ib; cin>>Db; An error occurs if the data type of the input does not match the data type of the input variable. An error also occurs if no input data is available for an input variable. Output Each output identifier (or literal string) must be separated by <<. int Max. Width = 10, Max. Length = 5; float Area = Max. Width * Max. Length; cout<<"The area of a square with width = " << Max. Width <<" and length = " << Max. Length << "is " << Area <<". n";
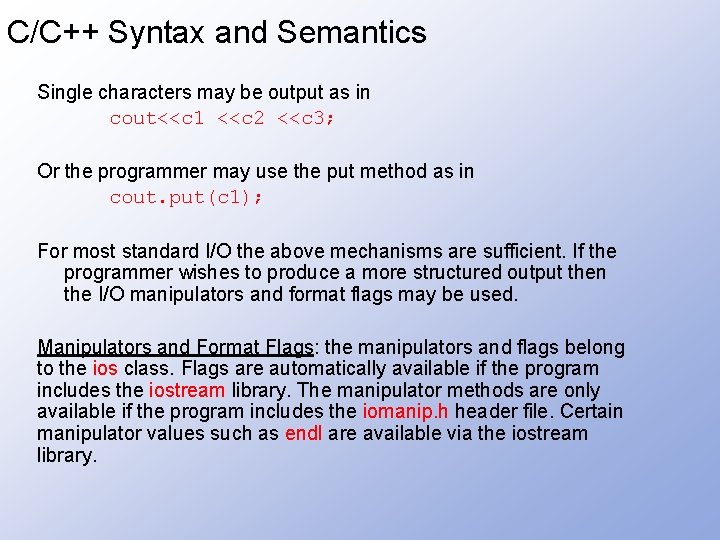
C/C++ Syntax and Semantics Single characters may be output as in cout<<c 1 <<c 2 <<c 3; Or the programmer may use the put method as in cout. put(c 1); For most standard I/O the above mechanisms are sufficient. If the programmer wishes to produce a more structured output then the I/O manipulators and format flags may be used. Manipulators and Format Flags: the manipulators and flags belong to the ios class. Flags are automatically available if the program includes the iostream library. The manipulator methods are only available if the program includes the iomanip. h header file. Certain manipulator values such as endl are available via the iostream library.
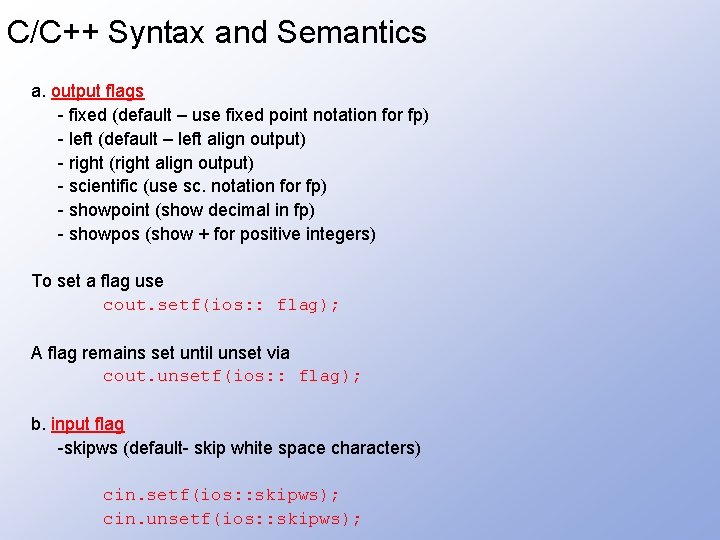
C/C++ Syntax and Semantics a. output flags - fixed (default – use fixed point notation for fp) - left (default – left align output) - right (right align output) - scientific (use sc. notation for fp) - showpoint (show decimal in fp) - showpos (show + for positive integers) To set a flag use cout. setf(ios: : flag); A flag remains set until unset via cout. unsetf(ios: : flag); b. input flag -skipws (default- skip white space characters) cin. setf(ios: : skipws); cin. unsetf(ios: : skipws);
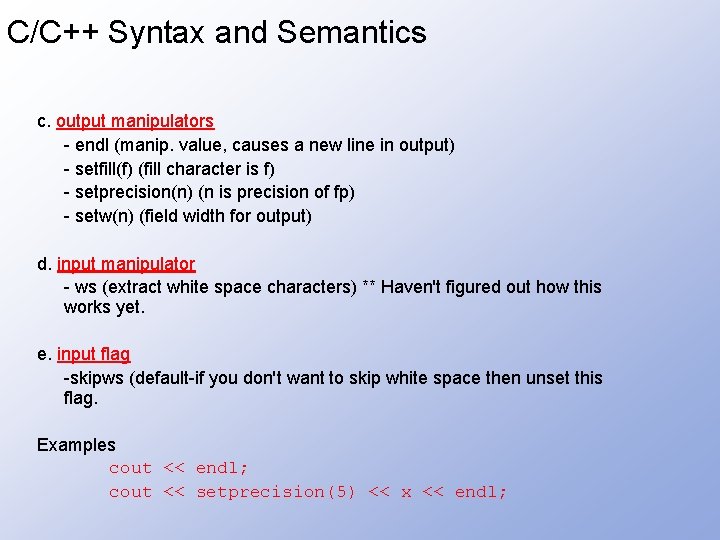
C/C++ Syntax and Semantics c. output manipulators - endl (manip. value, causes a new line in output) - setfill(f) (fill character is f) - setprecision(n) (n is precision of fp) - setw(n) (field width for output) d. input manipulator - ws (extract white space characters) ** Haven't figured out how this works yet. e. input flag -skipws (default-if you don't want to skip white space then unset this flag. Examples cout << endl; cout << setprecision(5) << x << endl;
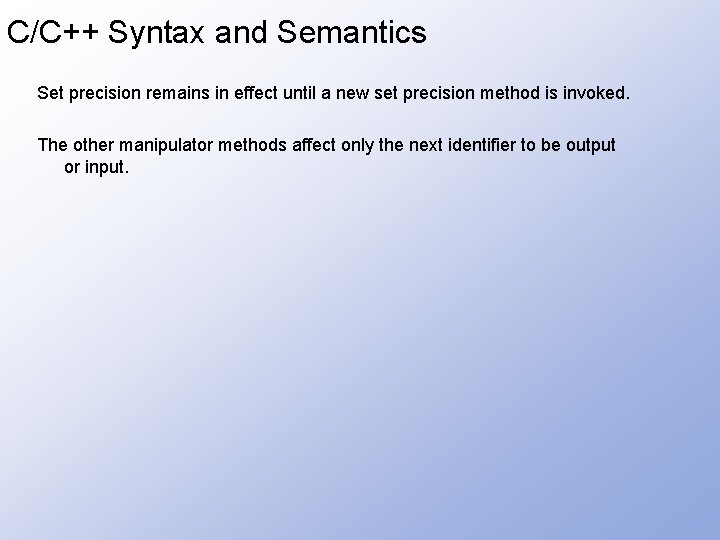
C/C++ Syntax and Semantics Set precision remains in effect until a new set precision method is invoked. The other manipulator methods affect only the next identifier to be output or input.
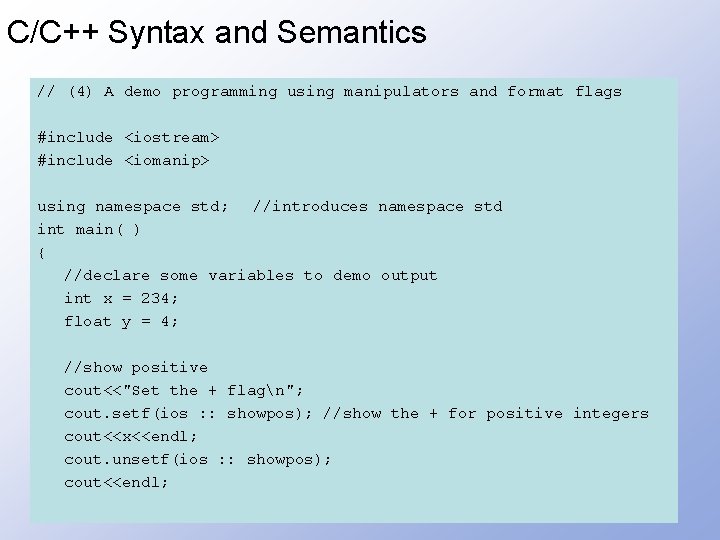
C/C++ Syntax and Semantics // (4) A demo programming using manipulators and format flags #include <iostream> #include <iomanip> using namespace std; //introduces namespace std int main( ) { //declare some variables to demo output int x = 234; float y = 4; //show positive cout<<"Set the + flagn"; cout. setf(ios : : showpos); //show the + for positive integers cout<<x<<endl; cout. unsetf(ios : : showpos); cout<<endl;
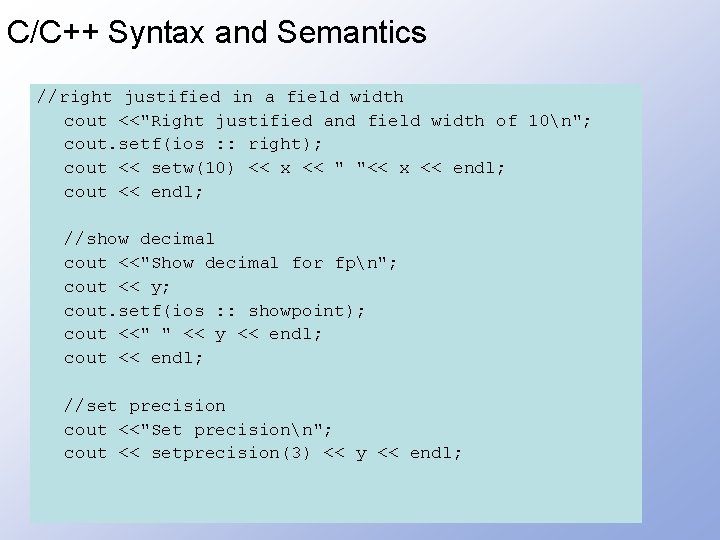
C/C++ Syntax and Semantics //right justified in a field width cout <<"Right justified and field width of 10n"; cout. setf(ios : : right); cout << setw(10) << x << " "<< x << endl; cout << endl; //show decimal cout <<"Show decimal for fpn"; cout << y; cout. setf(ios : : showpoint); cout <<" " << y << endl; cout << endl; //set precision cout <<"Set precisionn"; cout << setprecision(3) << y << endl;
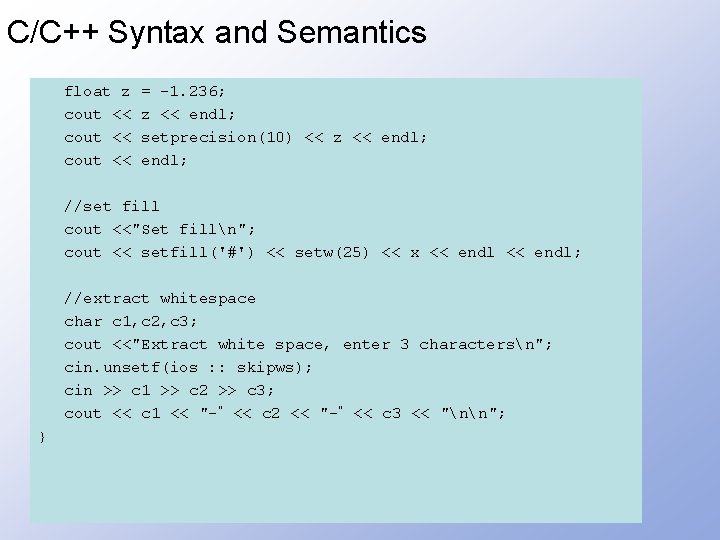
C/C++ Syntax and Semantics float z cout << = -1. 236; z << endl; setprecision(10) << z << endl; //set fill cout <<"Set filln"; cout << setfill('#') << setw(25) << x << endl; //extract whitespace char c 1, c 2, c 3; cout <<"Extract white space, enter 3 charactersn"; cin. unsetf(ios : : skipws); cin >> c 1 >> c 2 >> c 3; cout << c 1 << "-” << c 2 << "-” << c 3 << "nn"; }
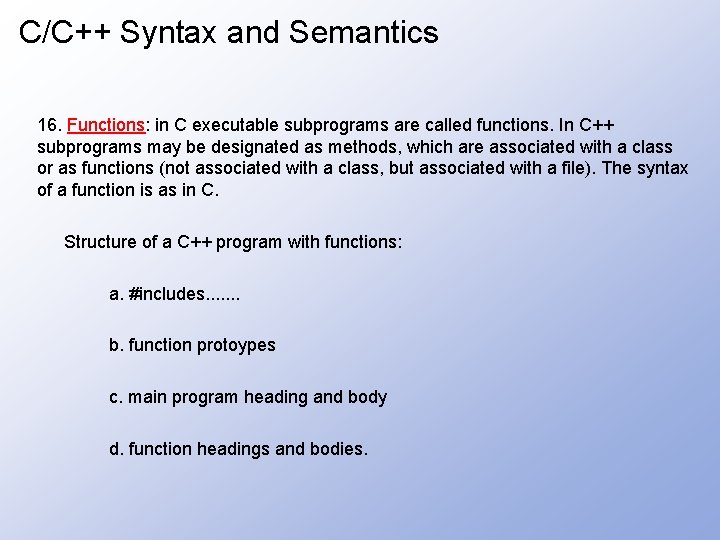
C/C++ Syntax and Semantics 16. Functions: in C executable subprograms are called functions. In C++ subprograms may be designated as methods, which are associated with a class or as functions (not associated with a class, but associated with a file). The syntax of a function is as in C. Structure of a C++ program with functions: a. #includes. . . . b. function protoypes c. main program heading and body d. function headings and bodies.
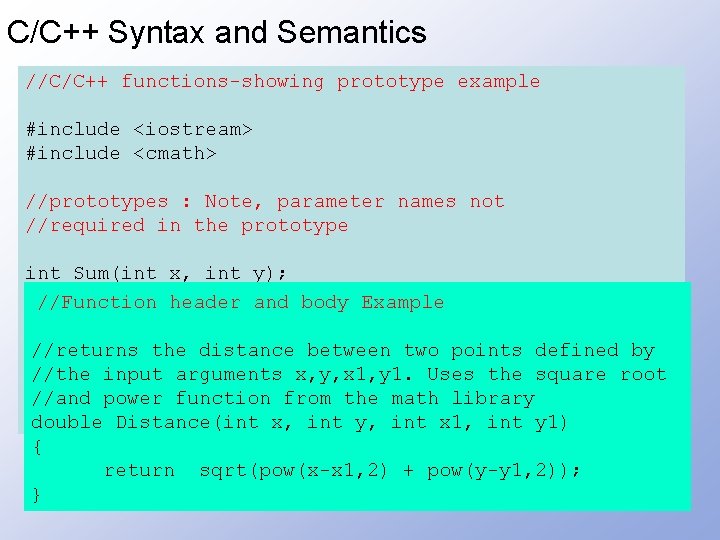
C/C++ Syntax and Semantics //C/C++ functions-showing prototype example Function Prototype Syntax: required for compilation and linking. #include <iostream> type function name(formal parameter list); #includereturn <cmath> //prototypes Note, parameter names not Function Heading: and Body Syntax //required in the prototype return type function name(formal parameter list) int Sum(int x, int y); { void Sum(intheader x, int y, int &sum); //overloaded w. ref. //Function body and body Example double Distance(int x, int y, int x 1, int y 1); } //returns the distance between two points defined by using std; x, y, x 1, y 1. //introduces stdroot //the namespace input arguments Usesnamespace the square Formal Parameter List Syntax int main() //and power function from the math library type 1 varname 1, type 2 varname 2, . . . double Distance(int x, int y, int x 1, int y 1) { return sqrt(pow(x-x 1, 2) + pow(y-y 1, 2)); }
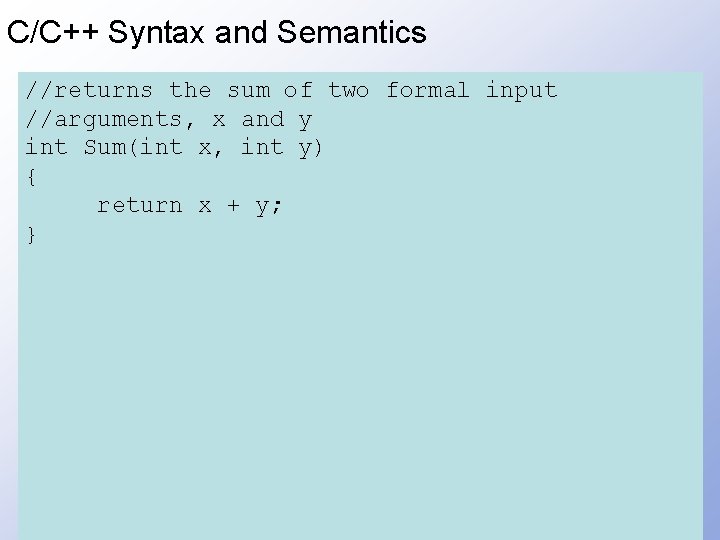
C/C++ Syntax and Semantics //returns the sum of two formal The body of a function may contain locally declaredinput constants, variables, and any valid C/C++ statement. //arguments, x and y If the function has a non-void return type then the function must return a value through a return statement. int Sum(int x, int y) { return exp; return x + y; }Parameter Passing Modes a. Pass by value (pass by copy): in C/C++, formal parameters without (&) are passed by value. This means that the value of the actual parameter (in the function call) is copied to its corresponding formal parameter. Sometimes value parameters are referred to as input parameters=> the value is used by a function but any modification to the value by the function is not visible outside of the function. Use when a parameter value should not be changed (or if changes should not be visible outside the function).
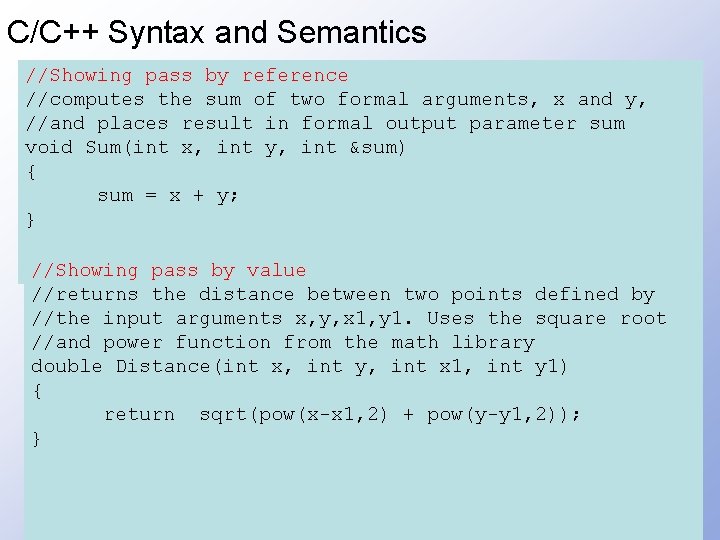
C/C++ Syntax and Semantics //Showing pass by reference //computes the sum of two formal arguments, x and y, b. Passplaces by reference (pass by in C/C++, formal parameters sum with //and result inaddress): formal output parameter (&) are passed by reference. This means that the address of the actual voidparameter Sum(int x, int &sum) is copied to itsy, corresponding formal parameter. Sometimes { reference parameters are referred to as output parameters => value is computed sum =by xfunction + y; and visible outside the function, or as input/output } parameters => value is used and modified by the function and modification is visible outside the function. //Showing pass by value Use when modifications should be visible outside the function, when //returns the values distance two or more need tobetween be returnedtwo from points a function, defined by default by //the => input x, y, x 1, y 1. Uses square alwaysarguments used with arrays, and => always used the with the object ofroot method function invocation. from the math library //and apower double Distance(int x, int y, int x 1, int y 1) {c. Constraints on actual parameters: when passing by value an argument may be a literal constant, variable, named constant, or an expression. return sqrt(pow(x-x 1, 2) + pow(y-y 1, 2)); When passing by reference, only a variable is allowed as an actual } argument. Number and type of actual and formal parameters must match. A one-toone positional correspondence exists between actual and formal.
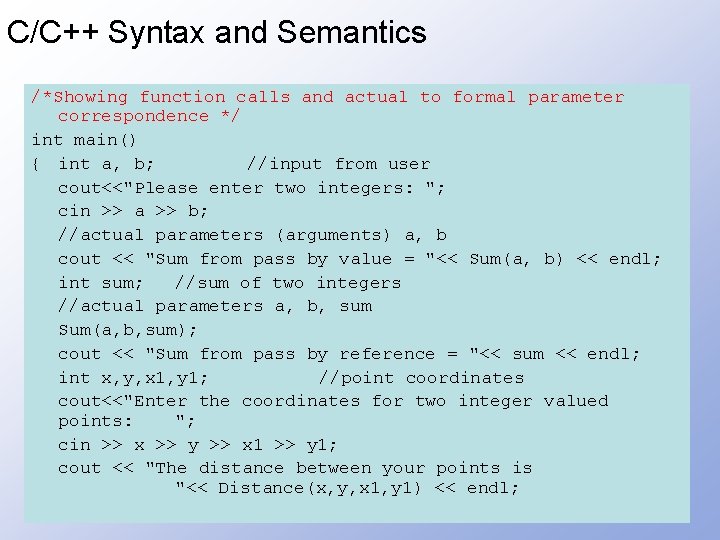
C/C++ Syntax and Semantics /*Showing function calls and actual to formal parameter correspondence */ int main() { int a, b; //input from user cout<<"Please enter two integers: "; cin >> a >> b; //actual parameters (arguments) a, b cout << "Sum from pass by value = "<< Sum(a, b) << endl; int sum; //sum of two integers //actual parameters a, b, sum Sum(a, b, sum); cout << "Sum from pass by reference = "<< sum << endl; int x, y, x 1, y 1; //point coordinates cout<<"Enter the coordinates for two integer valued points: "; cin >> x >> y >> x 1 >> y 1; cout << "The distance between your points is "<< Distance(x, y, x 1, y 1) << endl;
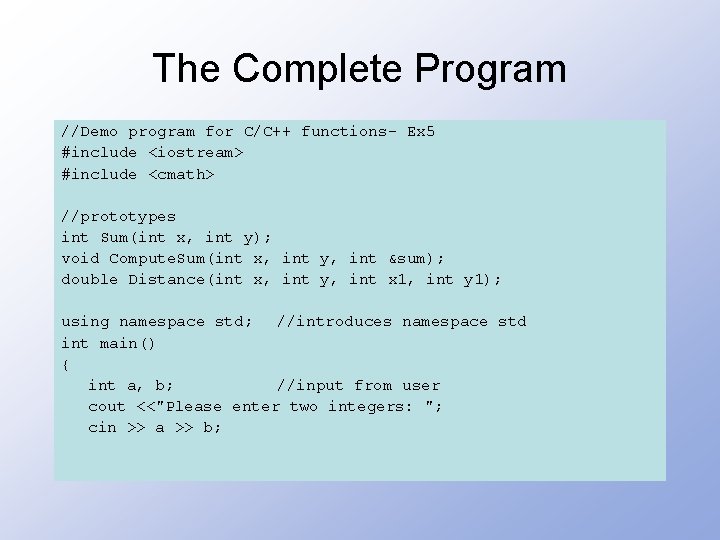
The Complete Program //Demo program for C/C++ functions- Ex 5 #include <iostream> #include <cmath> //prototypes int Sum(int x, int y); void Compute. Sum(int x, int y, int &sum); double Distance(int x, int y, int x 1, int y 1); using namespace std; //introduces namespace std int main() { int a, b; //input from user cout <<"Please enter two integers: "; cin >> a >> b;
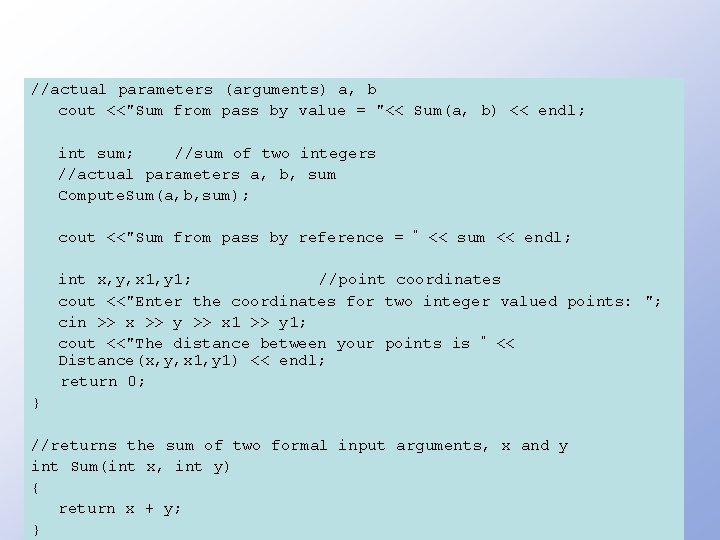
//actual parameters (arguments) a, b cout <<"Sum from pass by value = "<< Sum(a, b) << endl; int sum; //sum of two integers //actual parameters a, b, sum Compute. Sum(a, b, sum); cout <<"Sum from pass by reference = ” << sum << endl; int x, y, x 1, y 1; //point coordinates cout <<"Enter the coordinates for two integer valued points: "; cin >> x >> y >> x 1 >> y 1; cout <<"The distance between your points is ” << Distance(x, y, x 1, y 1) << endl; return 0; } //returns the sum of two formal input arguments, x and y int Sum(int x, int y) { return x + y; }
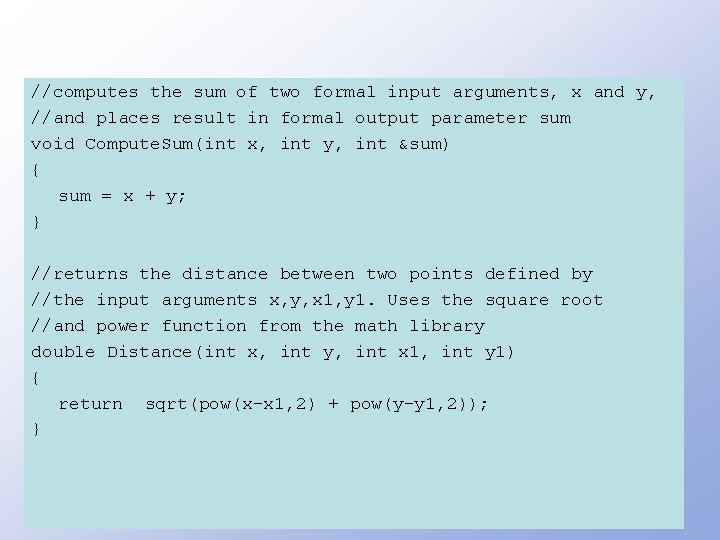
//computes the sum of two formal input arguments, x and y, //and places result in formal output parameter sum void Compute. Sum(int x, int y, int &sum) { sum = x + y; } //returns the distance between two points defined by //the input arguments x, y, x 1, y 1. Uses the square root //and power function from the math library double Distance(int x, int y, int x 1, int y 1) { return sqrt(pow(x-x 1, 2) + pow(y-y 1, 2)); }
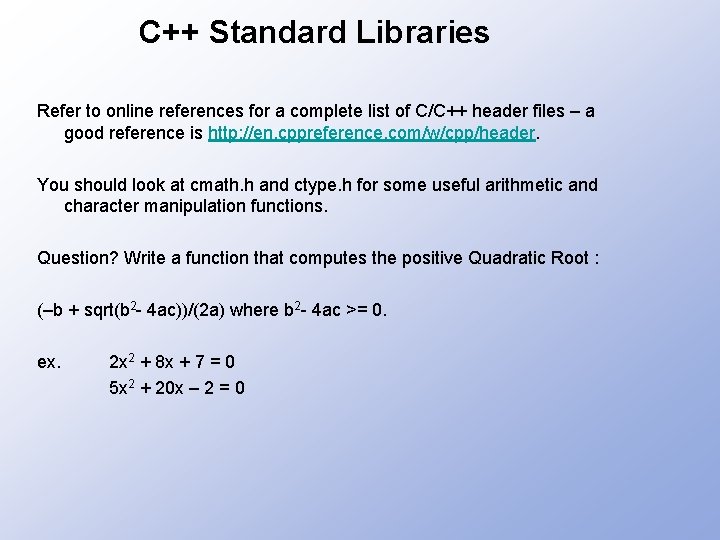
C++ Standard Libraries Refer to online references for a complete list of C/C++ header files – a good reference is http: //en. cppreference. com/w/cpp/header. You should look at cmath. h and ctype. h for some useful arithmetic and character manipulation functions. Question? Write a function that computes the positive Quadratic Root : (–b + sqrt(b 2 - 4 ac))/(2 a) where b 2 - 4 ac >= 0. ex. 2 x 2 + 8 x + 7 = 0 5 x 2 + 20 x – 2 = 0
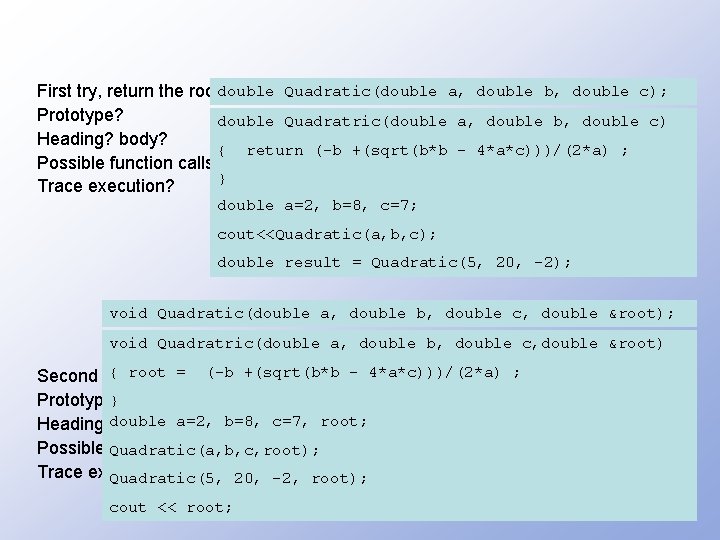
First try, return the rootdouble through. Quadratic(double a return statement. a, double b, double c); Prototype? double Quadratric(double a, double b, double c) Heading? body? { return (-b +(sqrt(b*b - 4*a*c)))/(2*a) ; Possible function calls? } Trace execution? double a=2, b=8, c=7; cout<<Quadratic(a, b, c); double result = Quadratic(5, 20, -2); void Quadratic(double a, double b, double c, double &root); void Quadratric(double a, double b, double c, double &root) { root (-b +(sqrt(b*b ; Second try, use a=reference parameter -for 4*a*c)))/(2*a) the root. } Prototype? Heading? double body? a=2, b=8, c=7, root; Possible Quadratic(a, b, c, root); function calls? Trace execution Quadratic(5, 20, -2, root); cout << root;
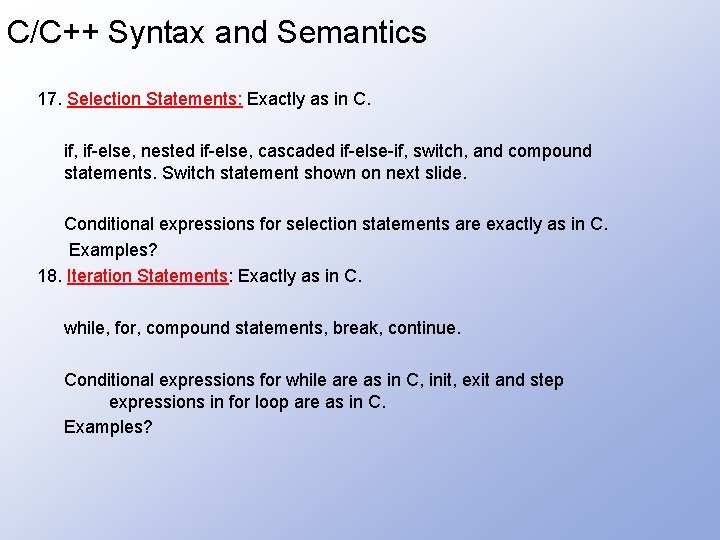
C/C++ Syntax and Semantics 17. Selection Statements: Exactly as in C. if, if-else, nested if-else, cascaded if-else-if, switch, and compound statements. Switch statement shown on next slide. Conditional expressions for selection statements are exactly as in C. Examples? 18. Iteration Statements: Exactly as in C. while, for, compound statements, break, continue. Conditional expressions for while are as in C, init, exit and step expressions in for loop are as in C. Examples?
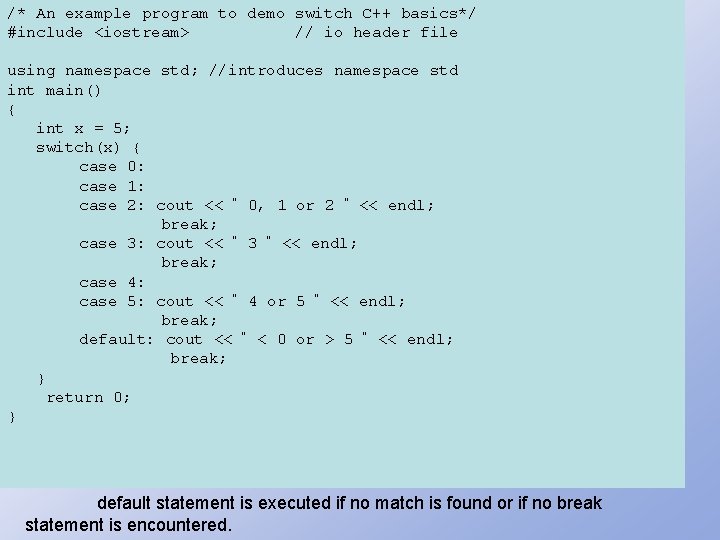
/* An example program to demo switch C++ basics*/ #include <iostream> // io header file Switch Statement using namespace std; //introduces namespace std int main() Switch Statements: Exactly as in C. { int x = 5; switch(selector) { switch(x) { case 0: label 1 : [stmt; ] case 1: [break; ] case 2: cout << “ 0, 1 or 2 ” << endl; ……………. . break; case 3: labeln cout << : “ 3 “ << endl; case [stmt; ] break; [break; ] case 4: default : << “ 4 or[stmt; ] case 5: cout 5 “ << endl; break; [break; ] default: cout << “ < 0 or > 5 “ << endl; } break; } selector is a scalar (int, char, boolean) return 0; each label is a constant } if a match is found between selector and label then statements associated with label are executed. execution falls thru each label unless a break is encountered default statement is executed if no match is found or if no break statement is encountered.
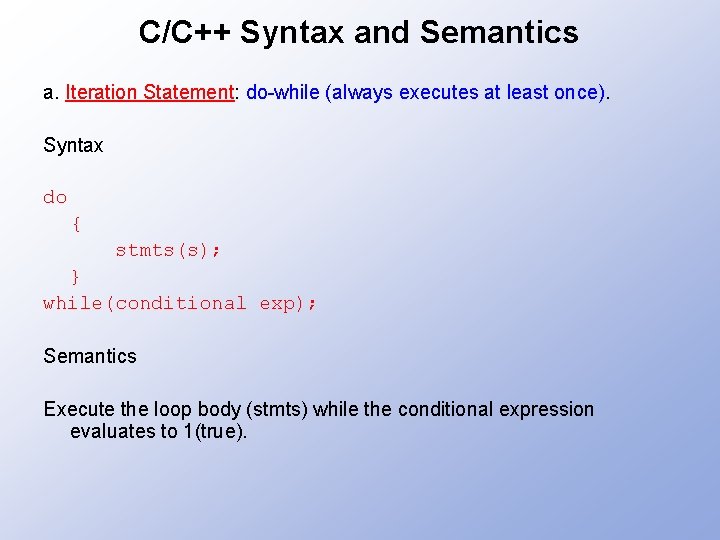
C/C++ Syntax and Semantics a. Iteration Statement: do-while (always executes at least once). Syntax do { stmts(s); } while(conditional exp); Semantics Execute the loop body (stmts) while the conditional expression evaluates to 1(true).
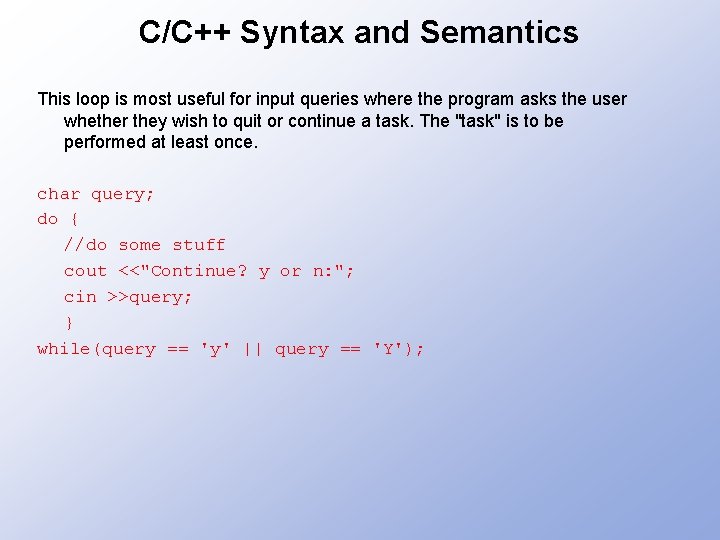
C/C++ Syntax and Semantics This loop is most useful for input queries where the program asks the user whether they wish to quit or continue a task. The "task" is to be performed at least once. char query; do { //do some stuff cout <<"Continue? y or n: "; cin >>query; } while(query == 'y' || query == 'Y');
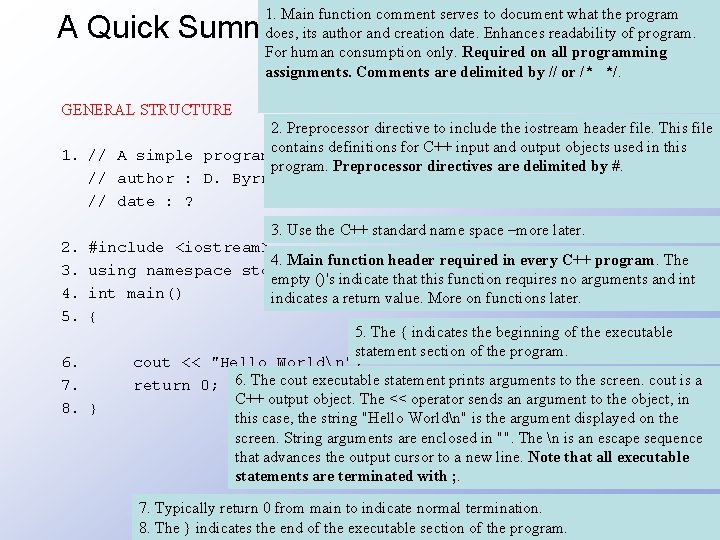
1. Main function comment serves to document what the program does, its author and creation date. Enhances readability of program. For human consumption only. Required on all programming assignments. Comments are delimited by // or /* */. A Quick Summary of Basics and Issues for Labs GENERAL STRUCTURE 2. Preprocessor directive to include the iostream header file. This file definitions for C++ output input and output objects used in this 1. // A simple program contains that demonstrates program. Preprocessor directives are delimited by #. // author : D. Byrnes // date : ? 3. Use the C++ standard name space –more later. 2. 3. 4. 5. #include <iostream> 4. Main function header required in every C++ program. The using namespace std; //introduces namespace std empty ()'s indicate that this function requires no arguments and int main() indicates a return value. More on functions later. { 5. The { indicates the beginning of the executable statement section of the program. 6. cout << "Hello Worldn"; statement prints arguments to the screen. cout is a 7. return 0; 6. The cout executable //normal termination C++ output object. The << operator sends an argument to the object, in 8. } this case, the string "Hello Worldn" is the argument displayed on the screen. String arguments are enclosed in "". The n is an escape sequence that advances the output cursor to a new line. Note that all executable statements are terminated with ; . 7. Typically return 0 from main to indicate normal termination. 8. The } indicates the end of the executable section of the program.
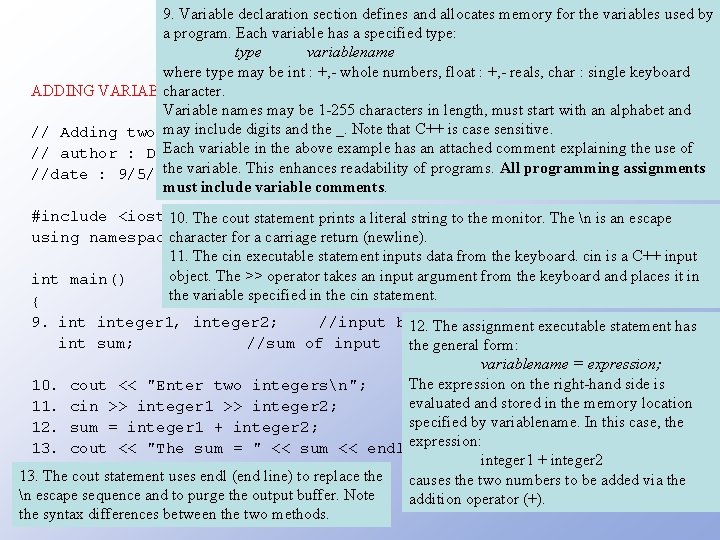
9. Variable declaration section defines and allocates memory for the variables used by a program. Each variable has a specified type: type variablename where type may be int : +, - whole numbers, float : +, - reals, char : single keyboard ADDING VARIABLES AND INPUT character. Variable names may be 1 -255 characters in length, must start with an alphabet and include digits the _. Notetheir that C++ is case sensitive. // Adding two may integers and printing sum variable in the above example has an attached comment explaining the use of // author : D. Each Byrnes the variable. This enhances readability of programs. All programming assignments //date : 9/5/94 must include variable comments. #include <iostream. h> 10. The cout statement prints a literal string to the monitor. The n is an escape using namespacecharacter std; for//introduces namespace std a carriage return (newline). 11. The cin executable statement inputs data from the keyboard. cin is a C++ input object. The >> operator takes an input argument from the keyboard and places it in int main() the variable specified in the cin statement. { 9. integer 1, integer 2; //input by 12. user The assignment executable statement has int sum; //sum of input the general form: variablename = expression; The expression on the right-hand side is 10. cout << "Enter two integersn"; evaluated and stored in the memory location 11. cin >> integer 1 >> integer 2; specified by variablename. In this case, the 12. sum = integer 1 + integer 2; 13. cout << "The sum = " << sum << endl; expression: integer 1 + integer 2 } 13. The cout statement uses endl (end line) to replace the causes the two numbers to be added via the n escape sequence and to purge the output buffer. Note addition operator (+). the syntax differences between the two methods.
Xcode 10 tutorial for beginners
Xcode change app icon
Assembly language programming in 8086 microprocessor
Program development steps in c
Axial skills
Iso 22301 utbildning
Typiska drag för en novell
Nationell inriktning för artificiell intelligens
Ekologiskt fotavtryck
Varför kallas perioden 1918-1939 för mellankrigstiden
En lathund för arbete med kontinuitetshantering
Adressändring ideell förening
Personlig tidbok fylla i
Anatomi organ reproduksi
Förklara densitet för barn
Datorkunskap för nybörjare
Boverket ka
Mall debattartikel
Autokratiskt ledarskap
Nyckelkompetenser för livslångt lärande
Påbyggnader för flakfordon
Kraft per area
Publik sektor
Urban torhamn
Presentera för publik crossboss
Vad är ett minoritetsspråk
Bat mitza
Treserva lathund
Mjälthilus
Claes martinsson
Cks
Verifikationsplan
Mat för idrottare
Verktyg för automatisering av utbetalningar