Chapter 7 Multiway Trees Data Structures and Algorithms
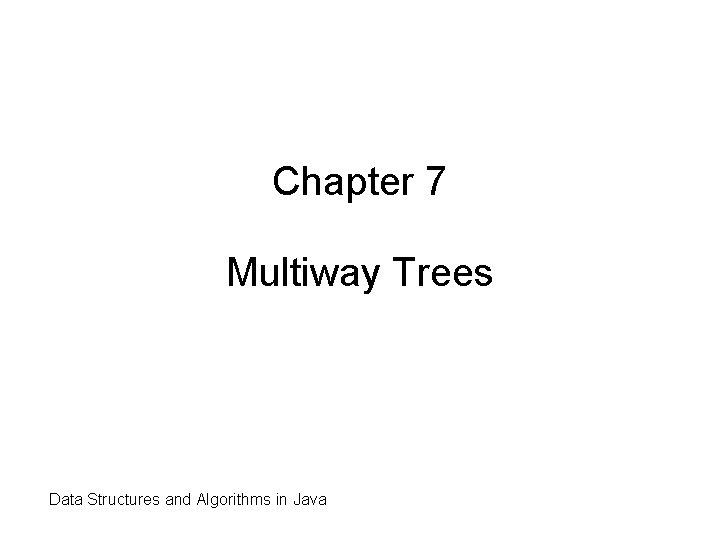
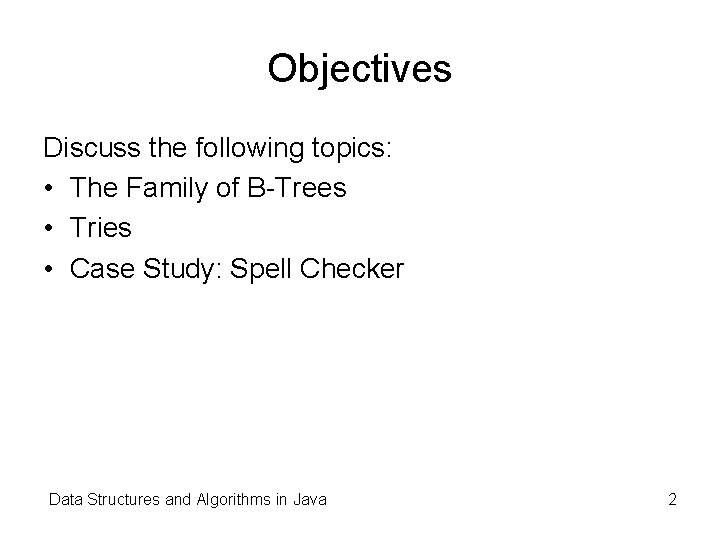
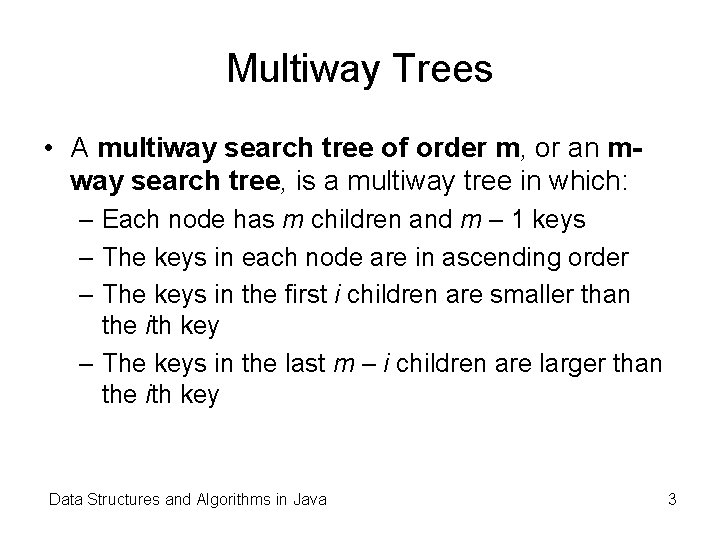
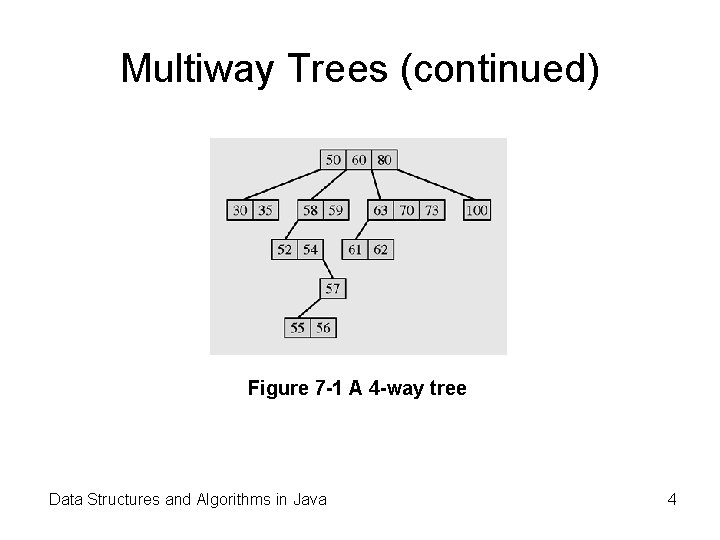
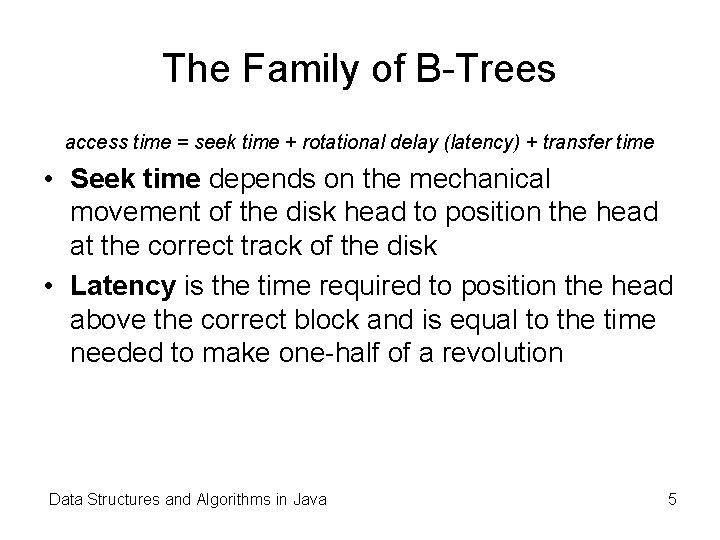
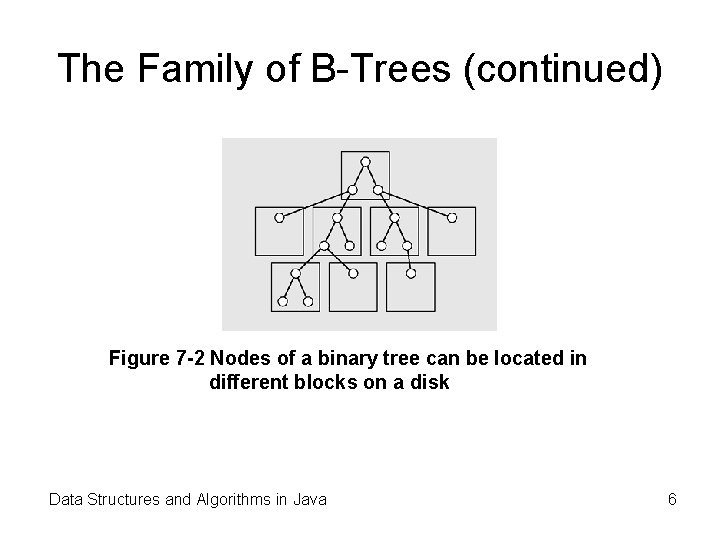
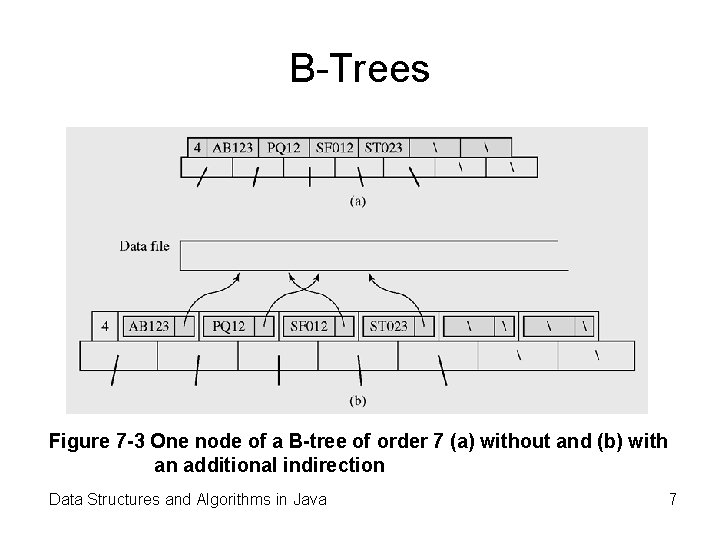
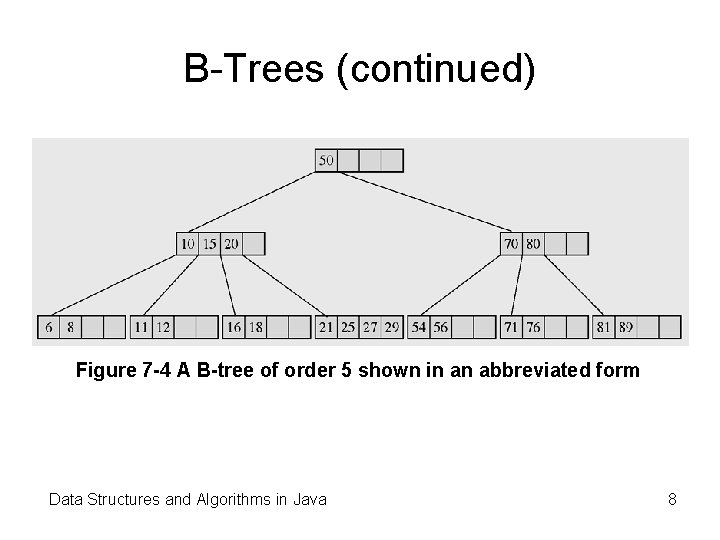
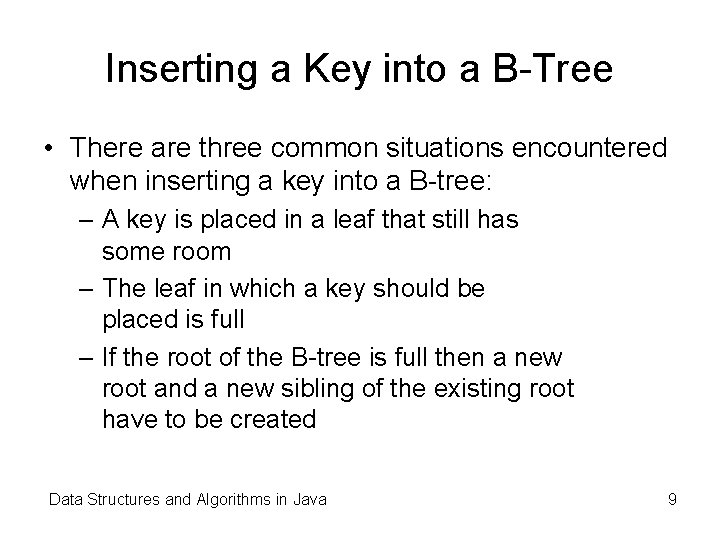
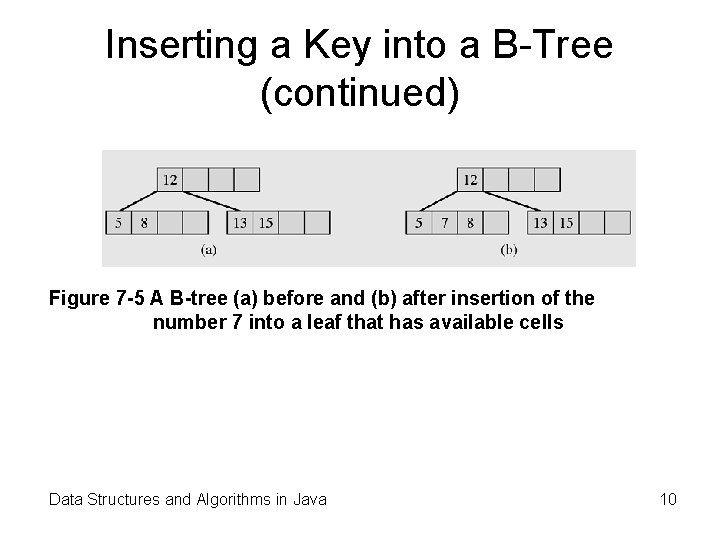
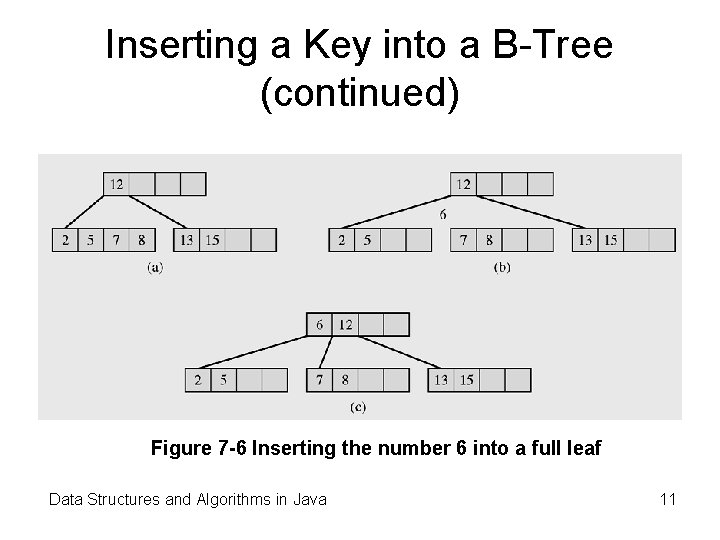
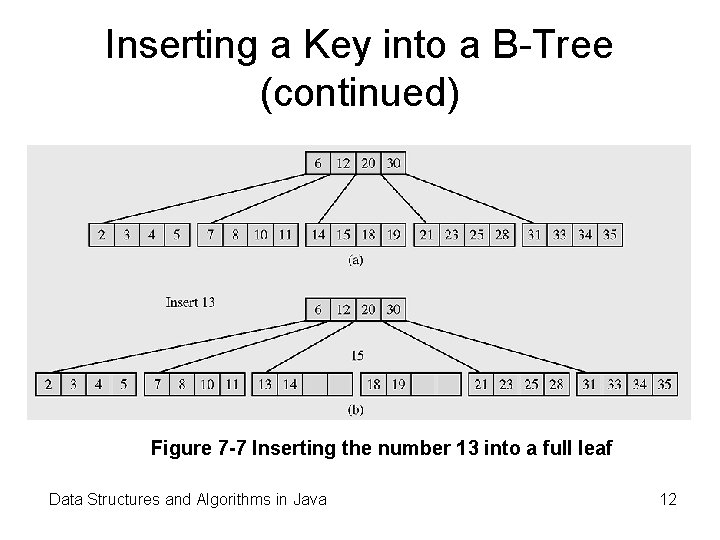
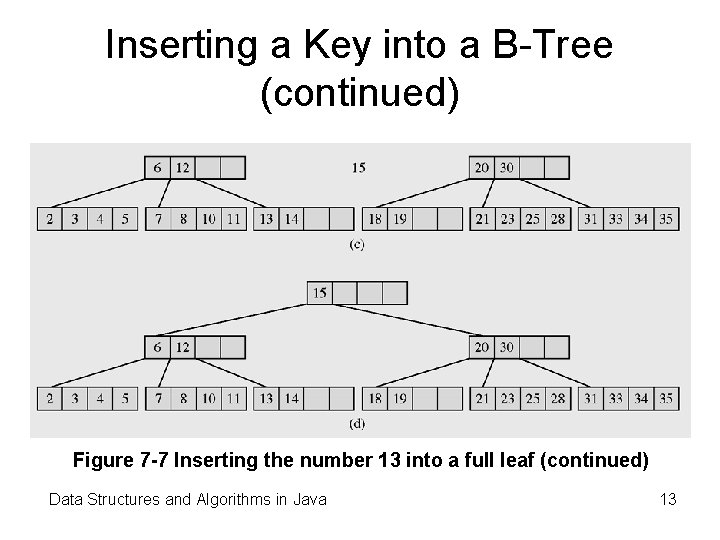
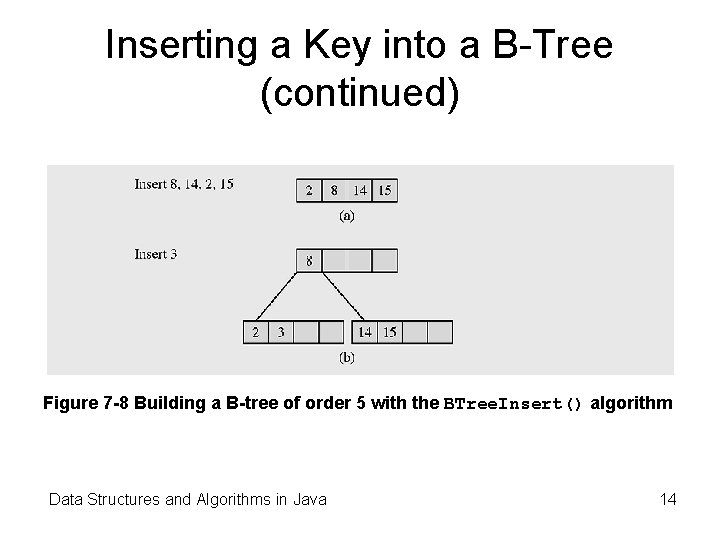
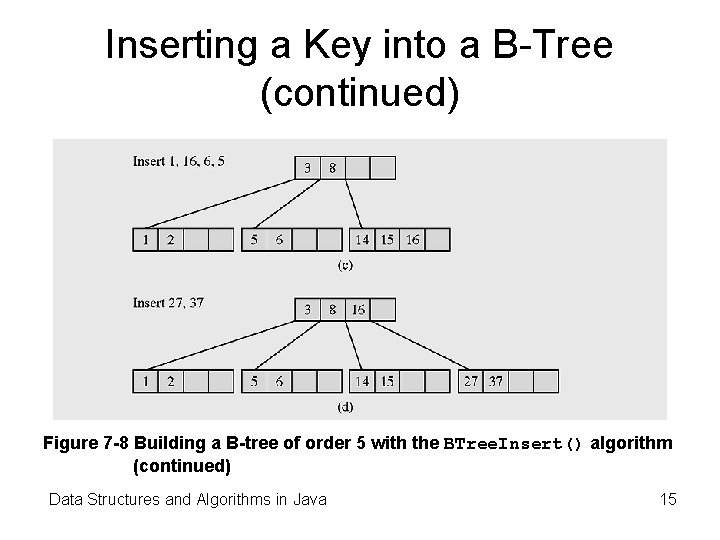
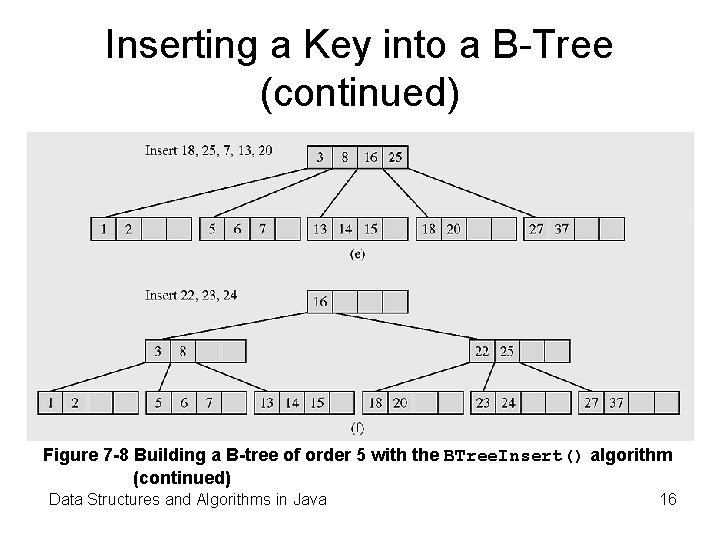
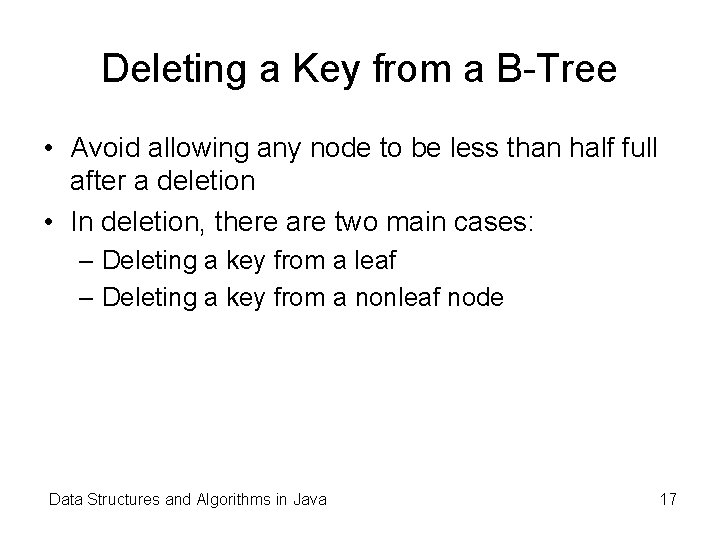
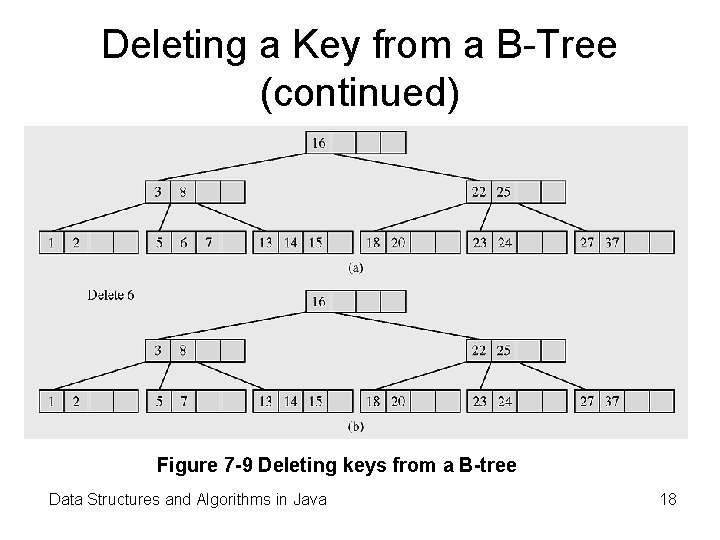
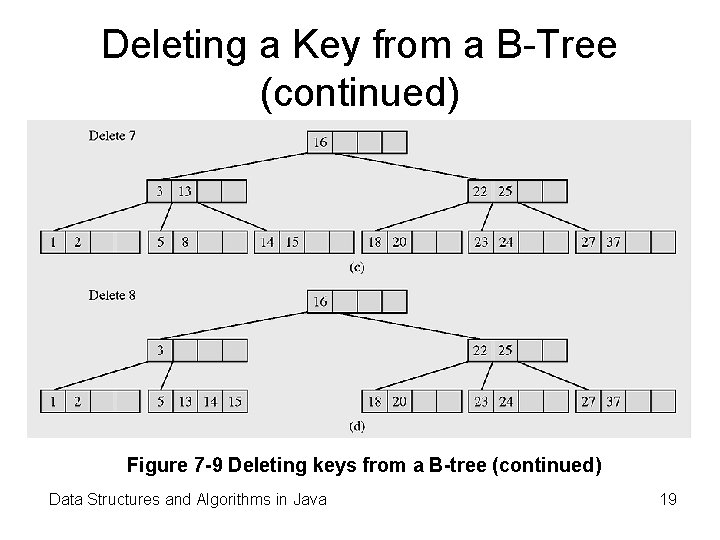
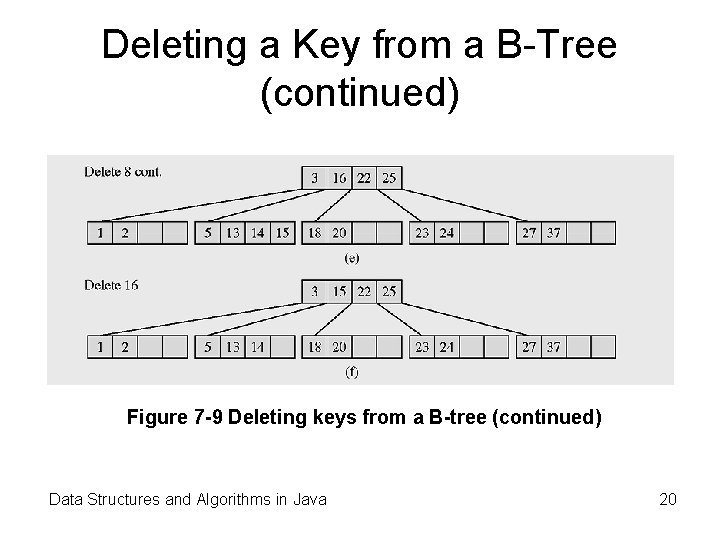
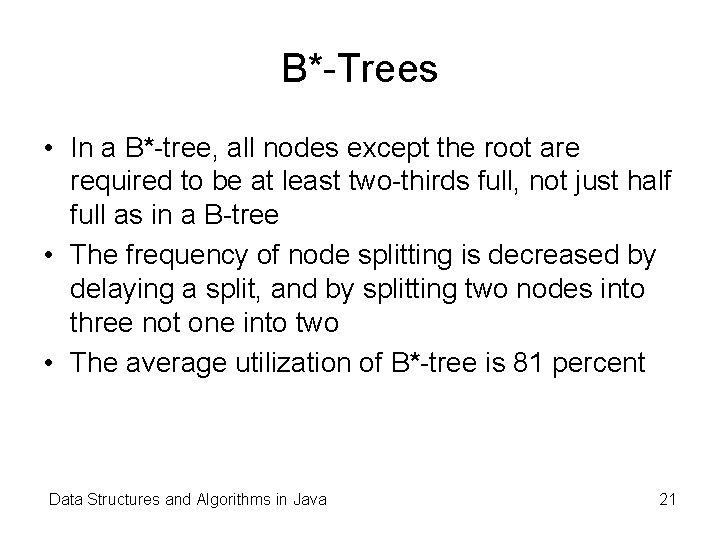
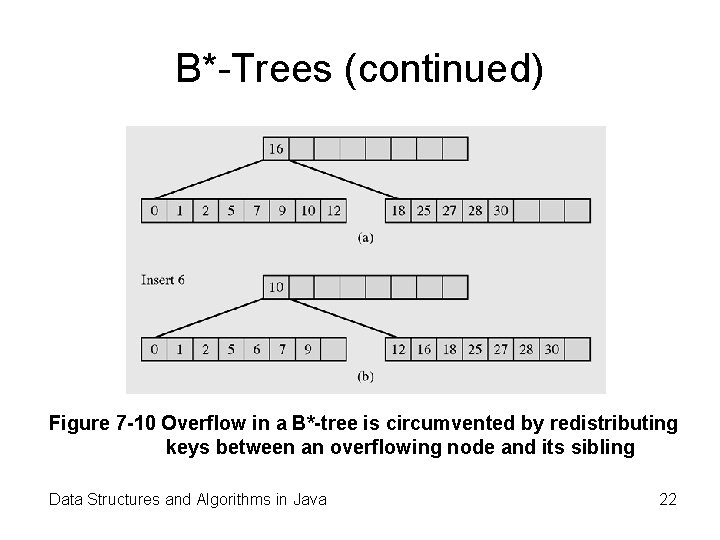
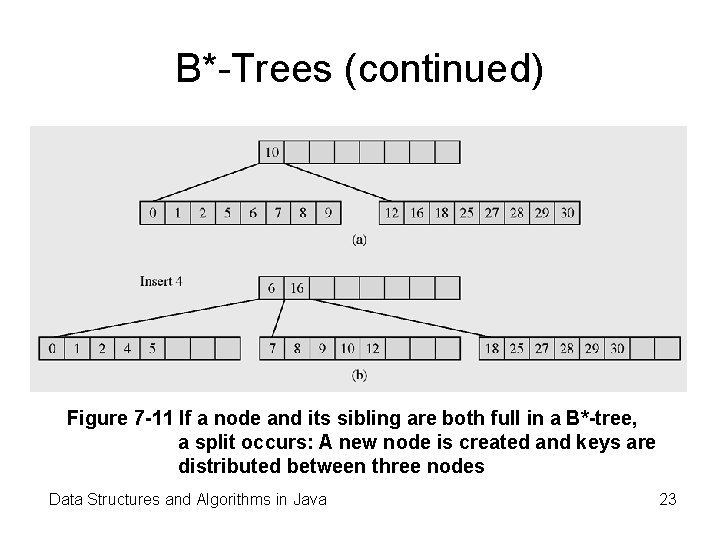
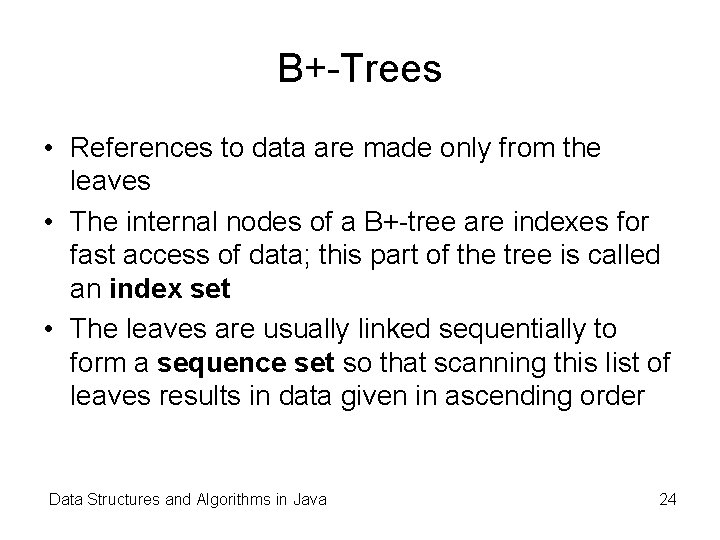
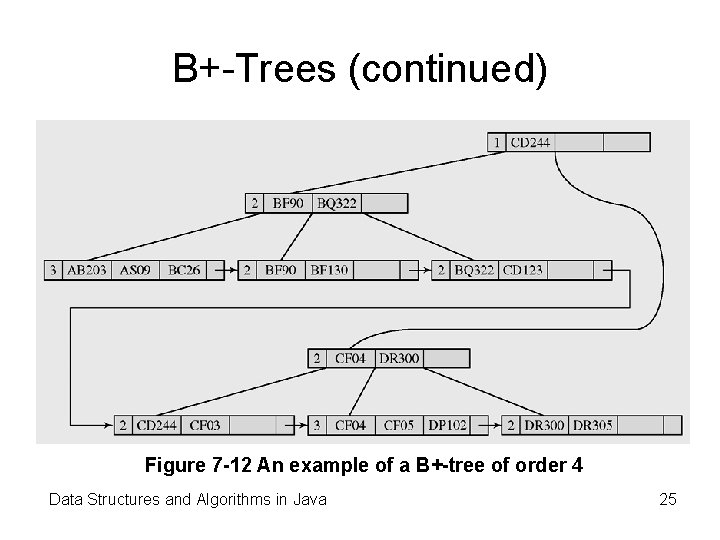
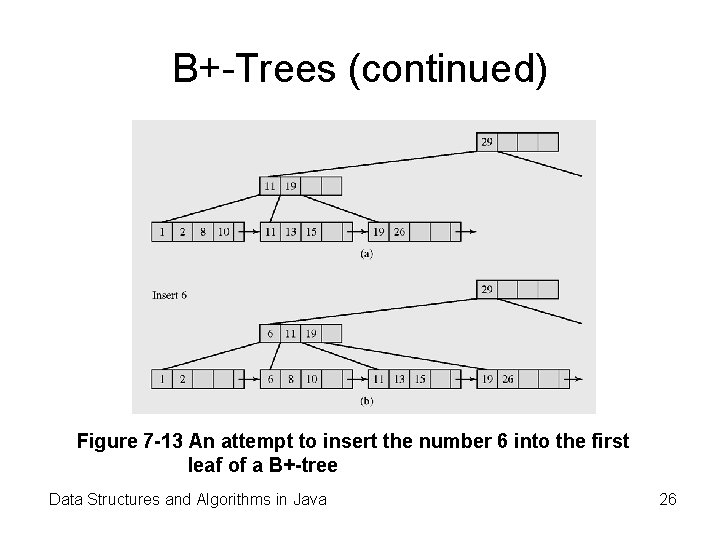
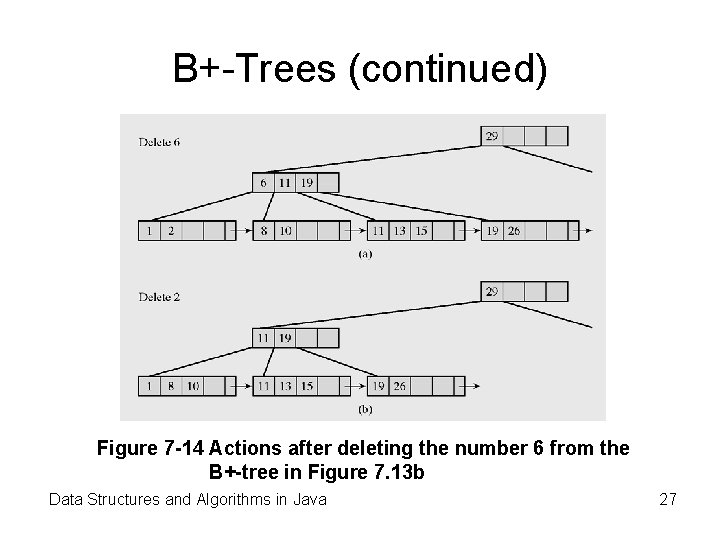
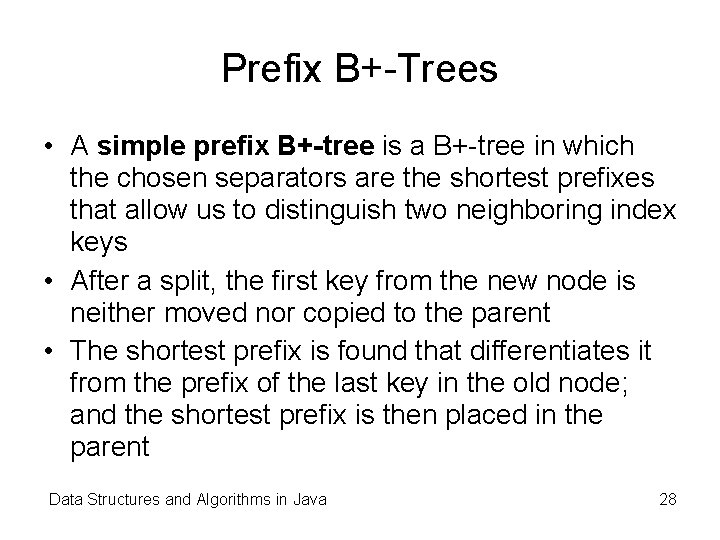
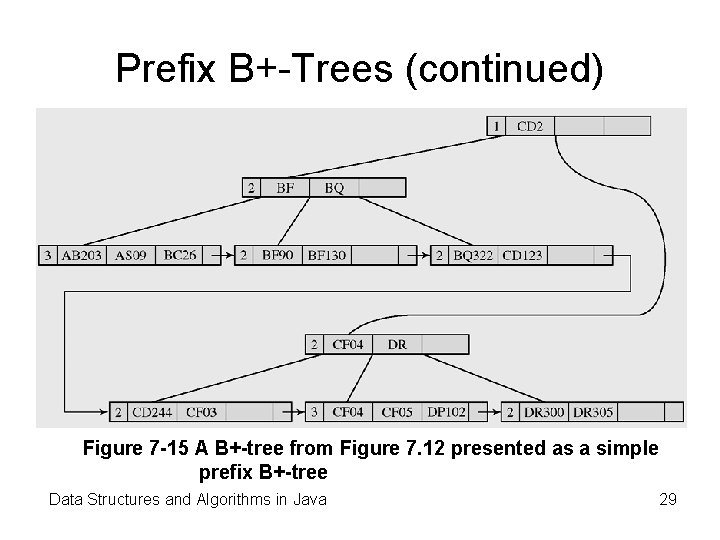
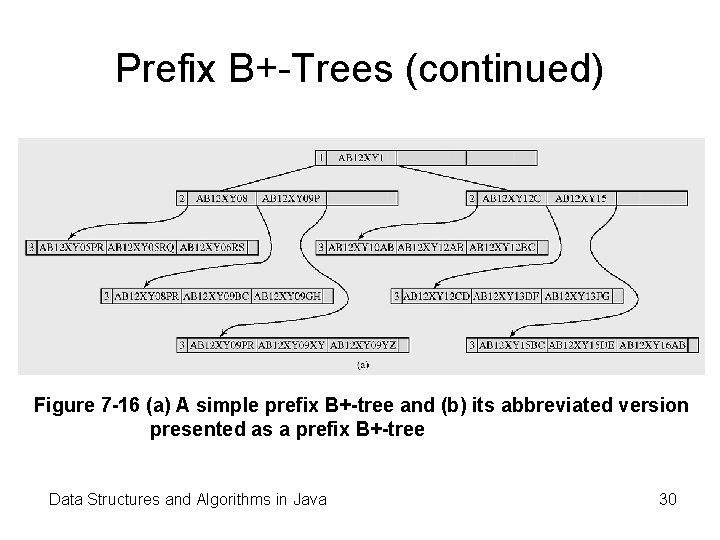
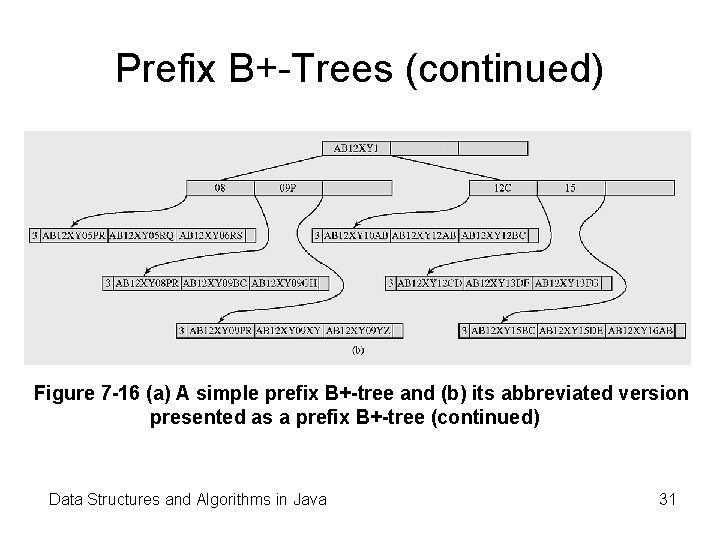
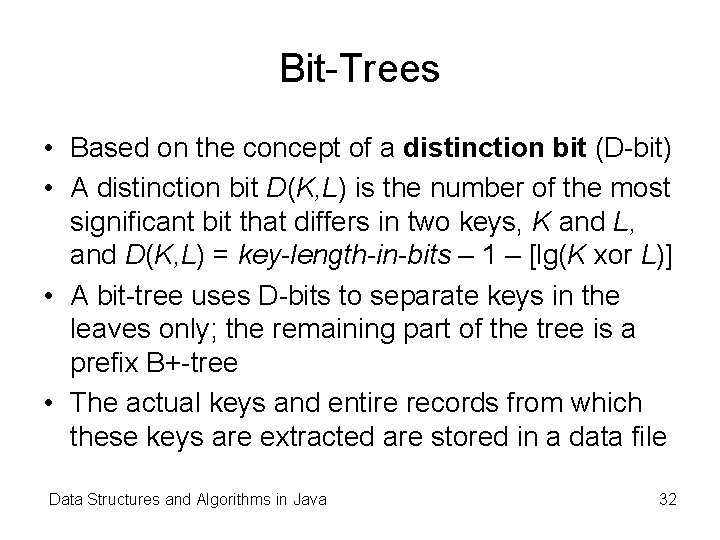
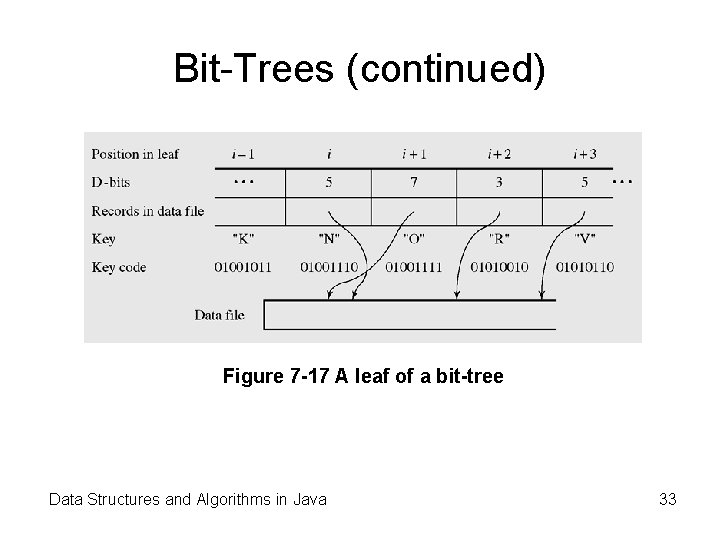
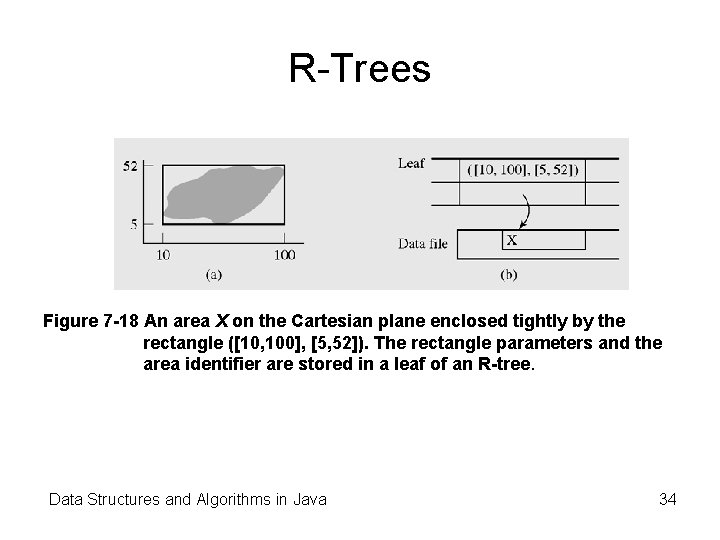
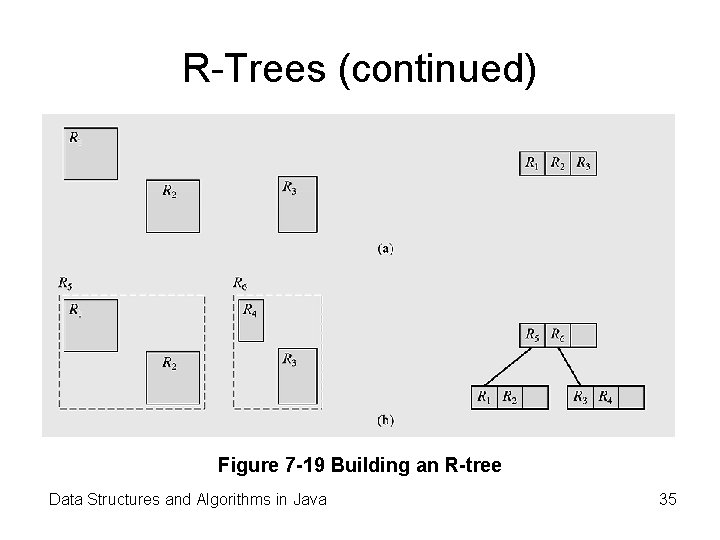
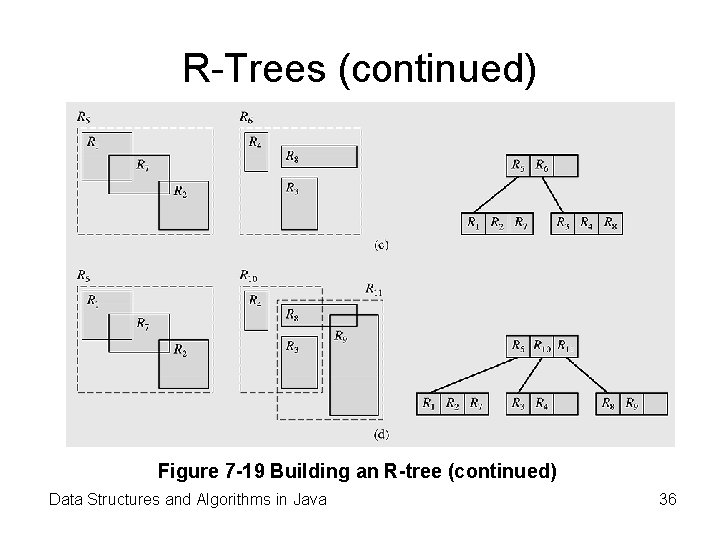
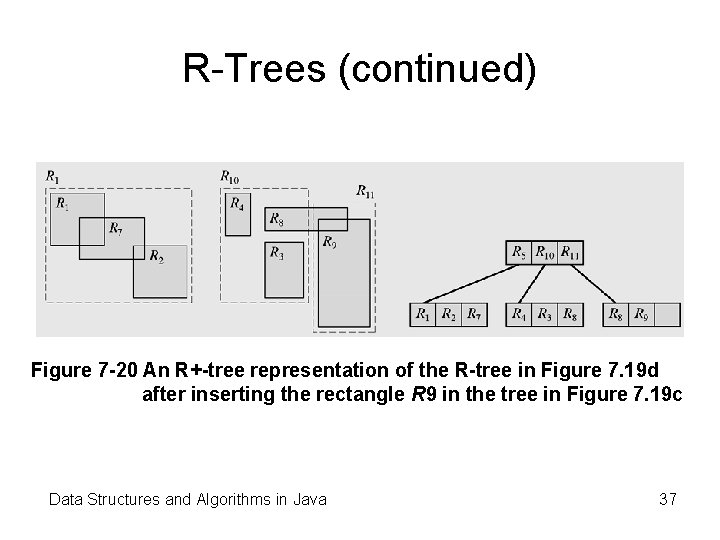
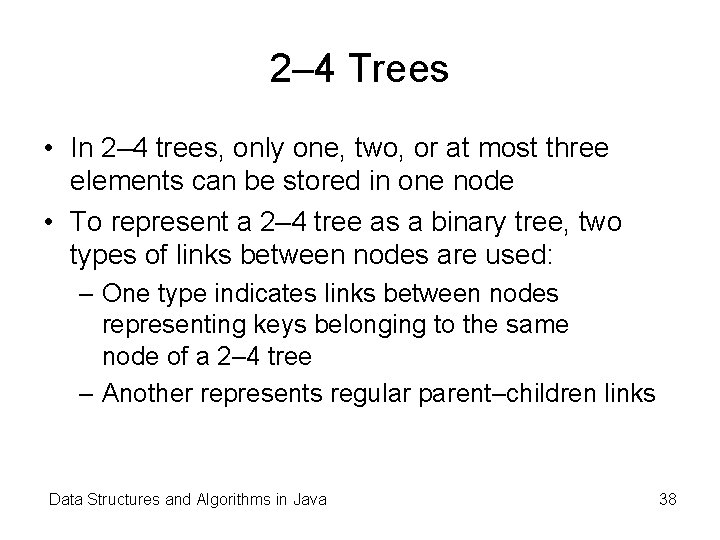
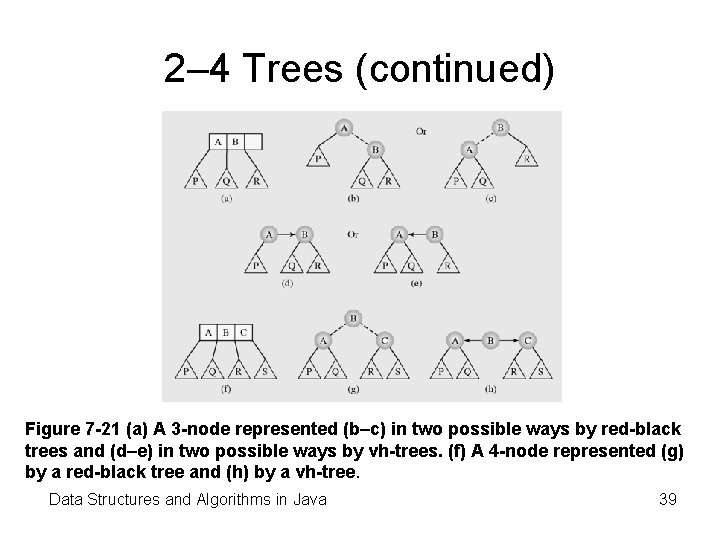
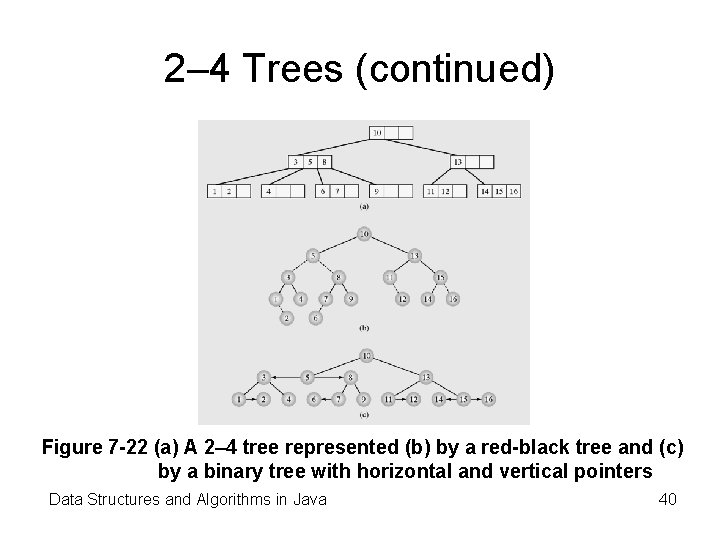
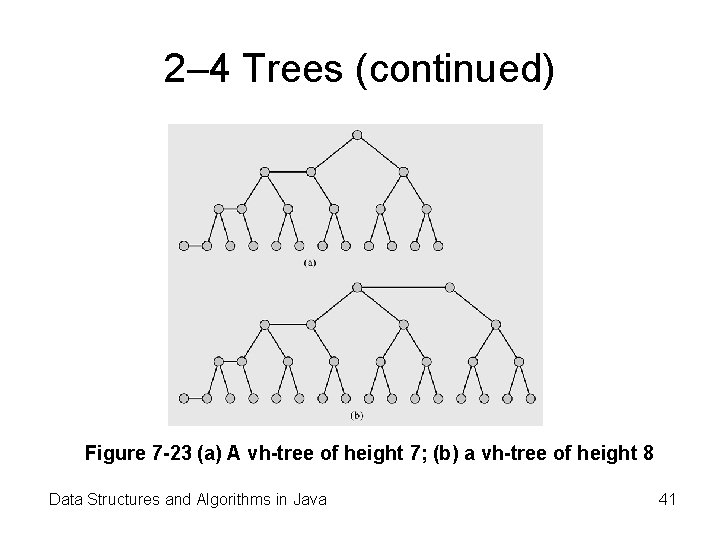
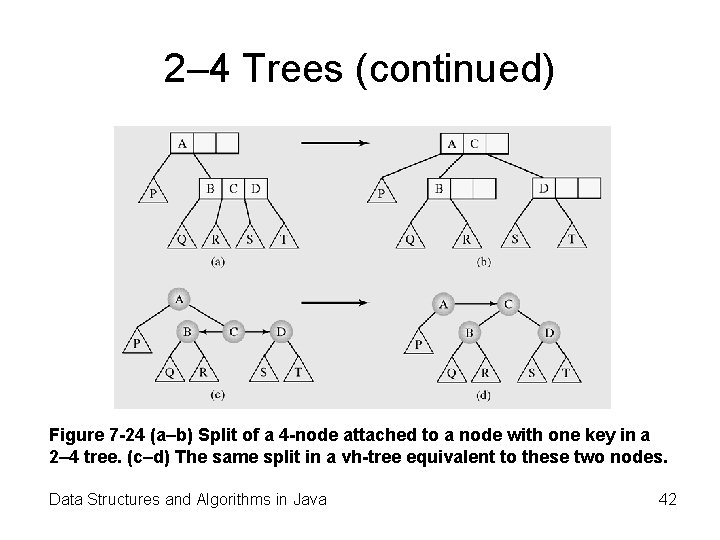
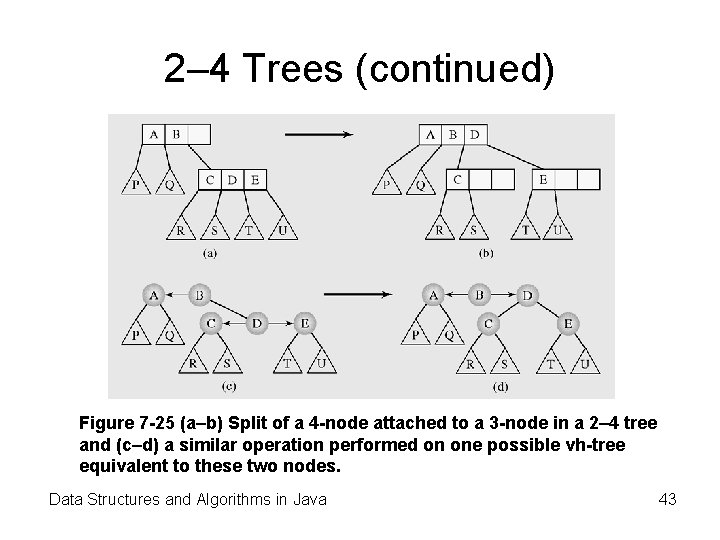
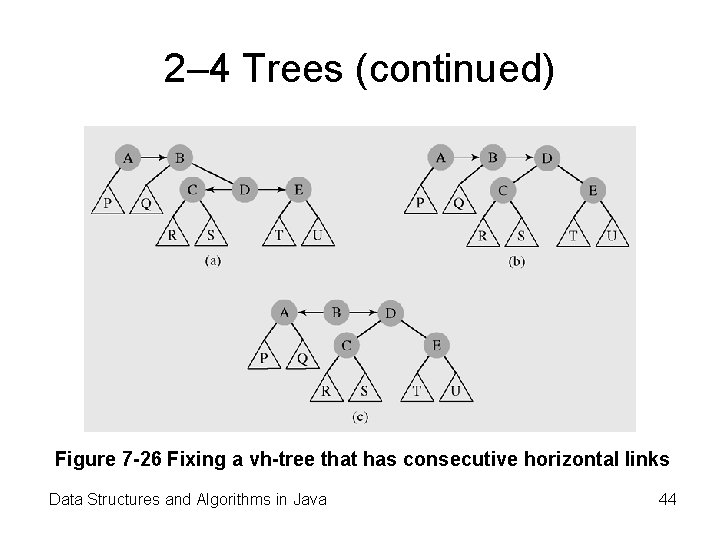
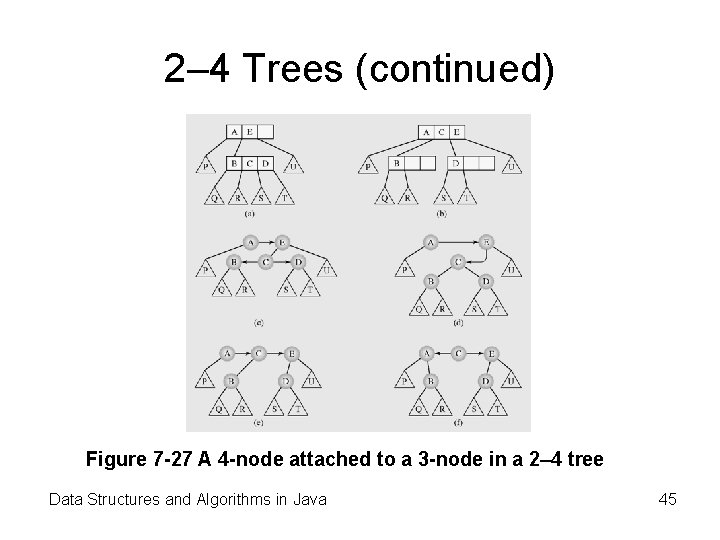
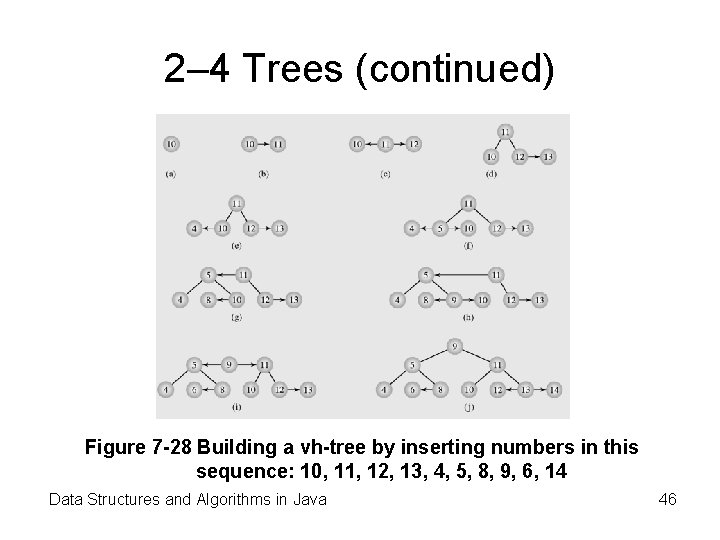
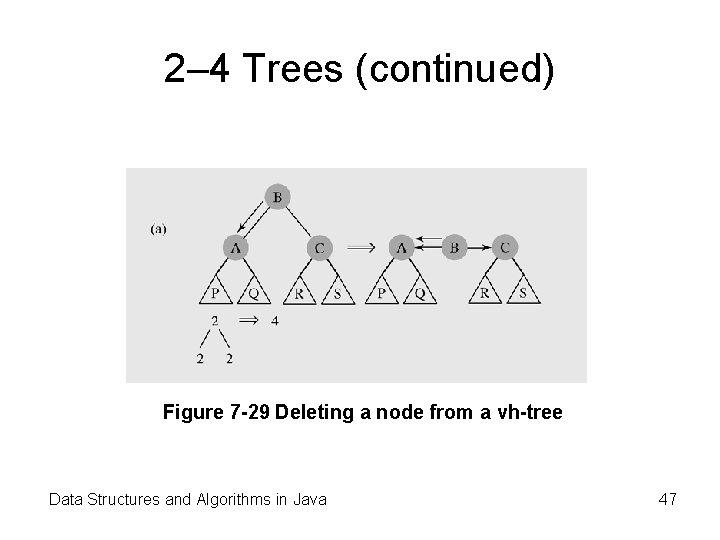
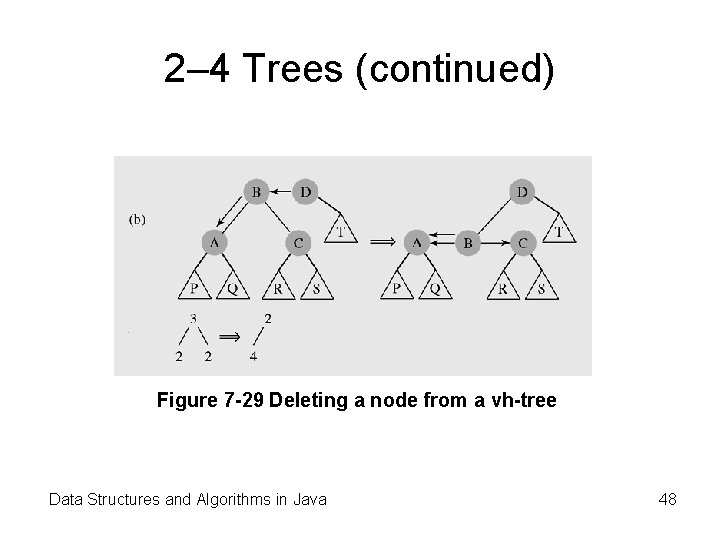
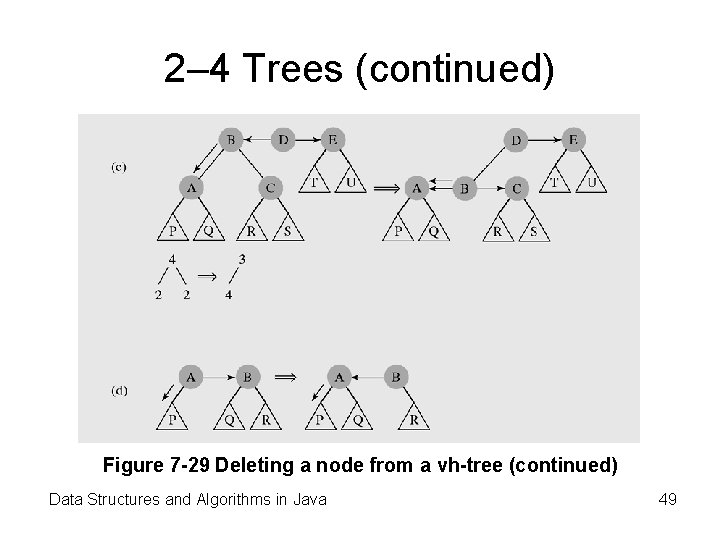
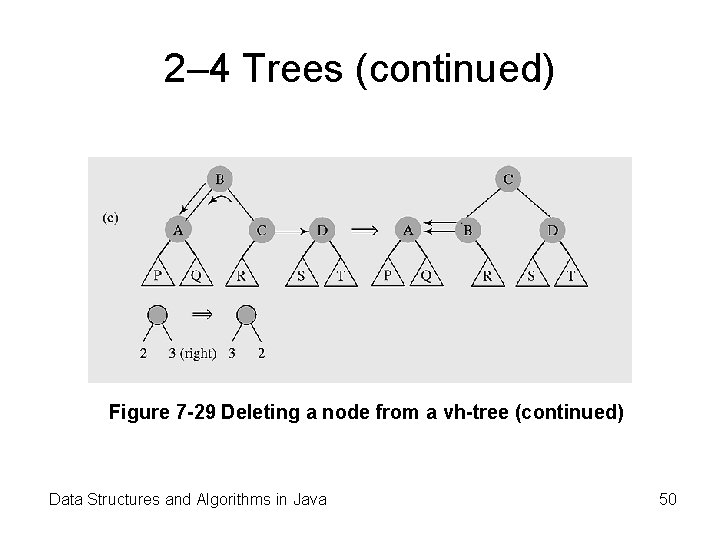
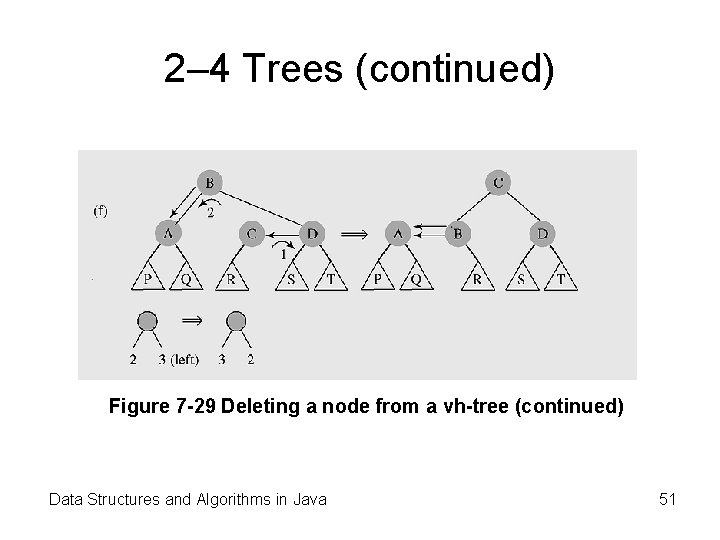
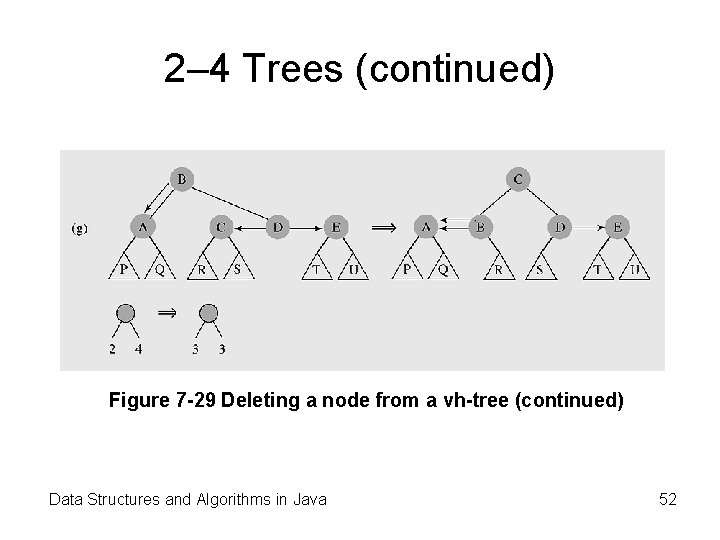
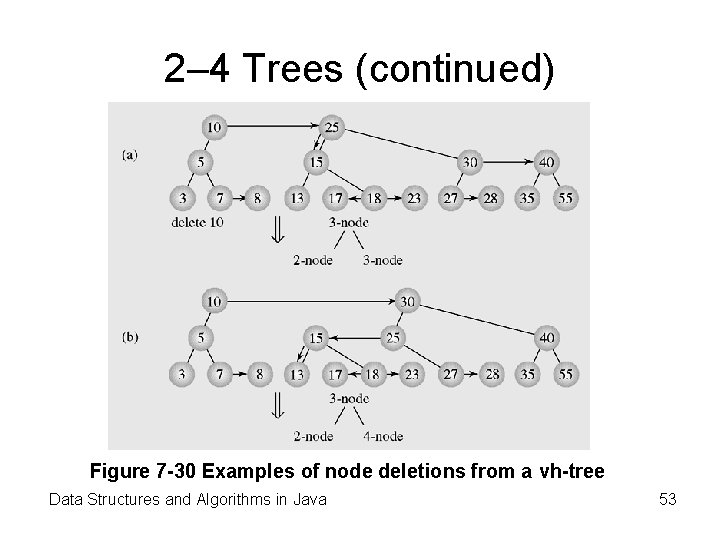
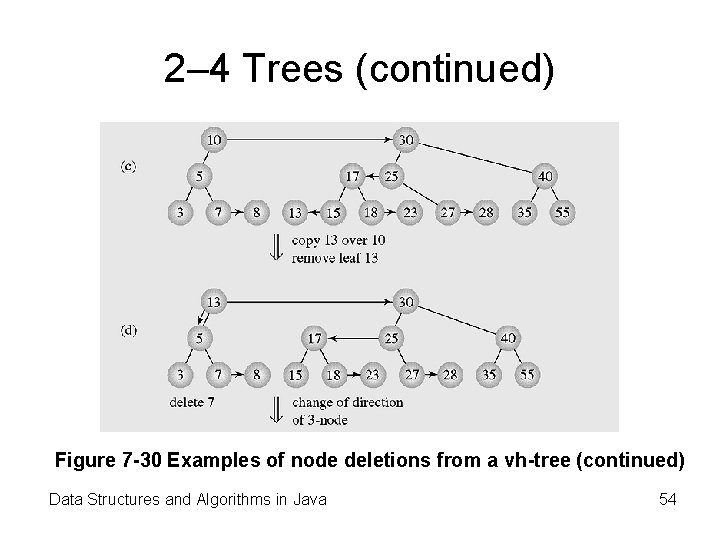
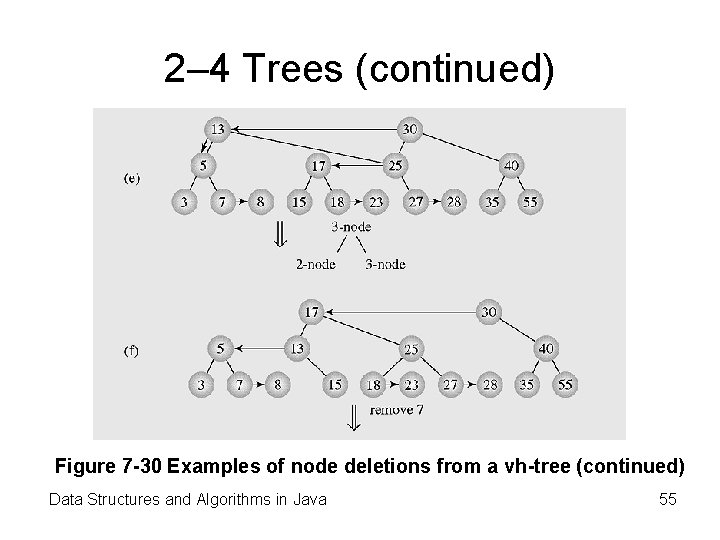
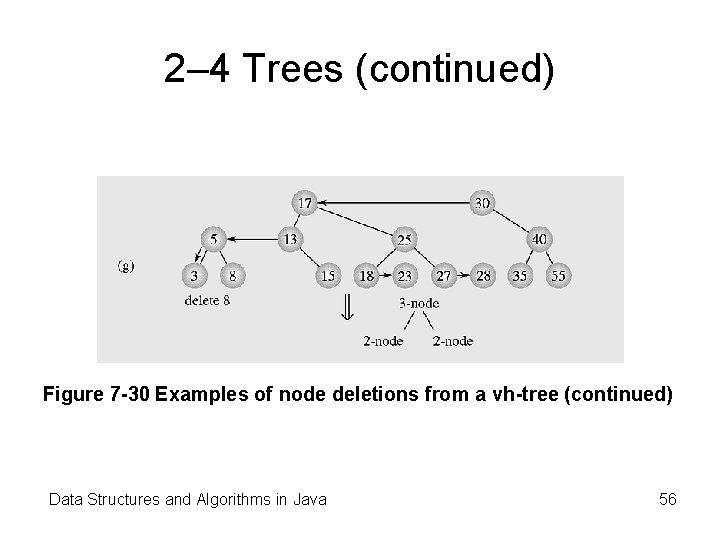
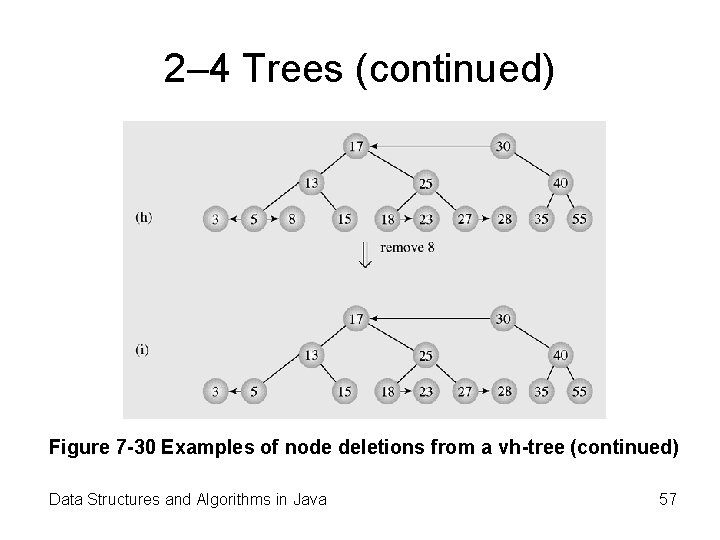
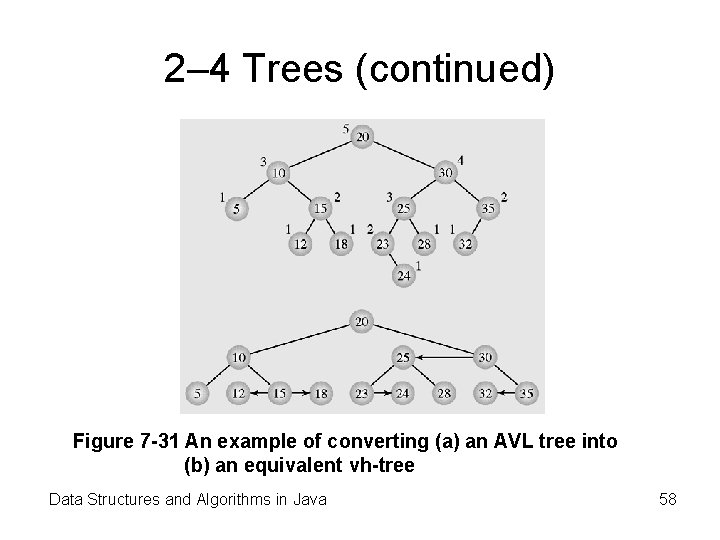
- Slides: 58
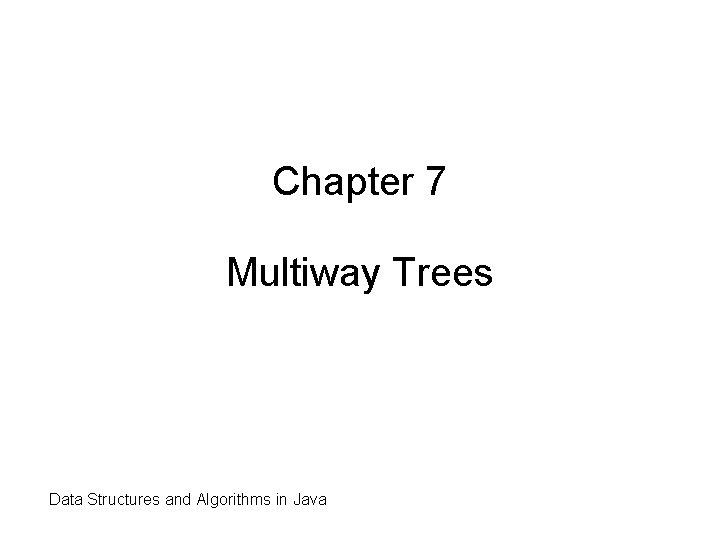
Chapter 7 Multiway Trees Data Structures and Algorithms in Java
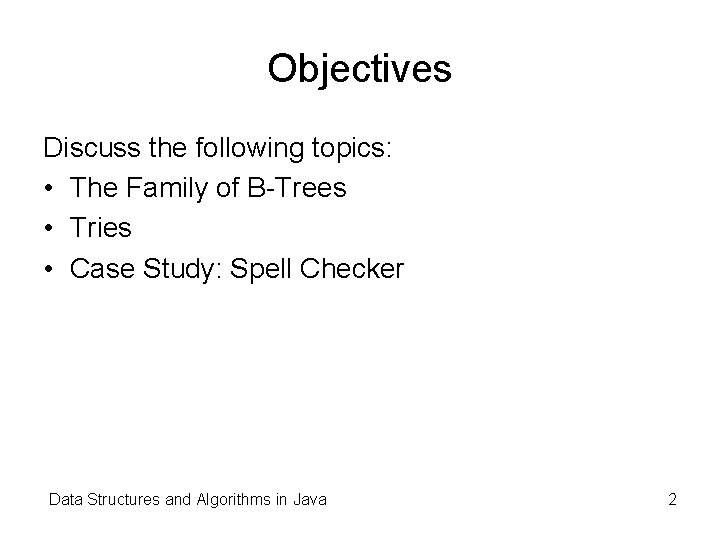
Objectives Discuss the following topics: • The Family of B-Trees • Tries • Case Study: Spell Checker Data Structures and Algorithms in Java 2
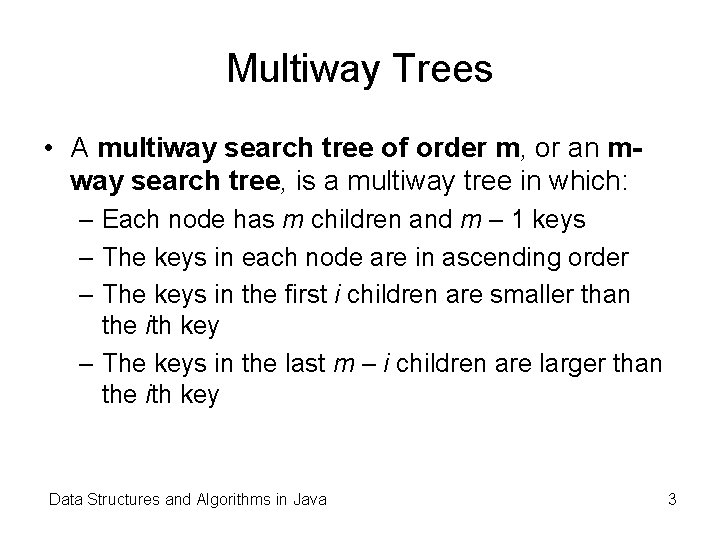
Multiway Trees • A multiway search tree of order m, or an mway search tree, is a multiway tree in which: – Each node has m children and m – 1 keys – The keys in each node are in ascending order – The keys in the first i children are smaller than the ith key – The keys in the last m – i children are larger than the ith key Data Structures and Algorithms in Java 3
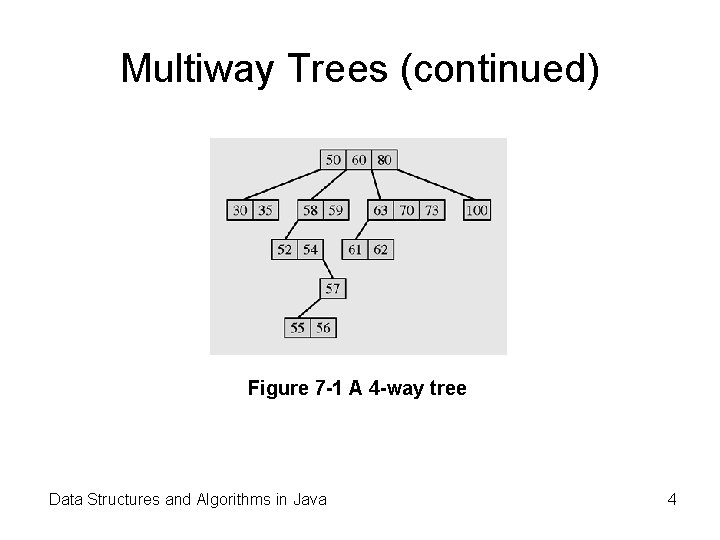
Multiway Trees (continued) Figure 7 -1 A 4 -way tree Data Structures and Algorithms in Java 4
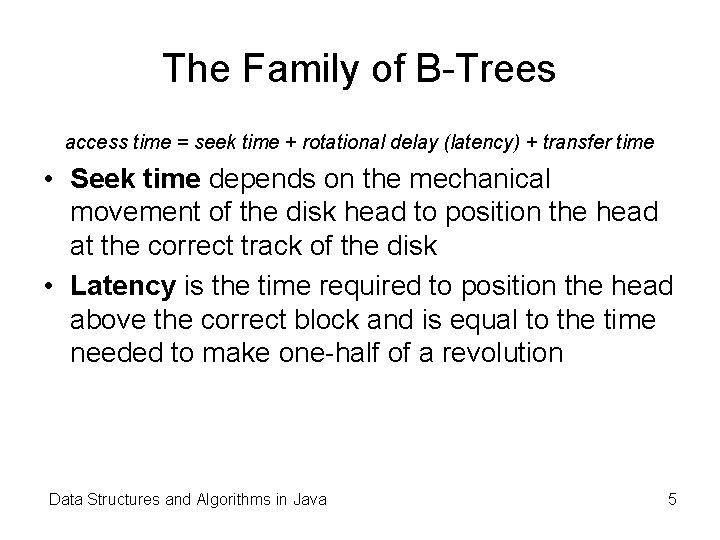
The Family of B-Trees access time = seek time + rotational delay (latency) + transfer time • Seek time depends on the mechanical movement of the disk head to position the head at the correct track of the disk • Latency is the time required to position the head above the correct block and is equal to the time needed to make one-half of a revolution Data Structures and Algorithms in Java 5
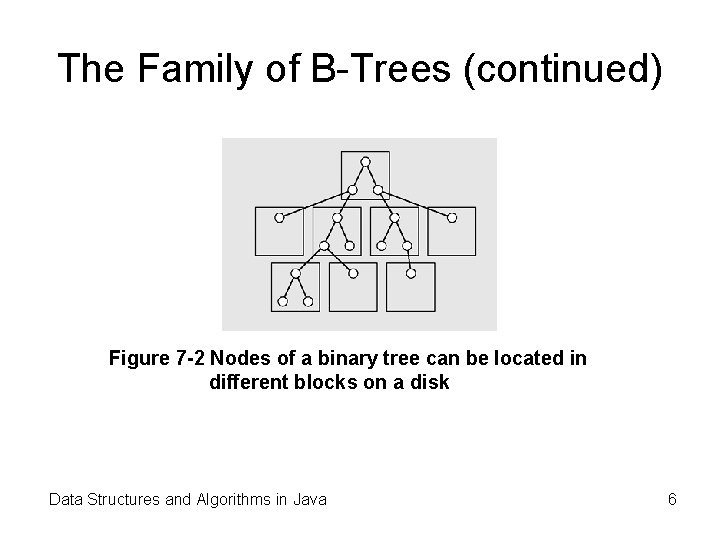
The Family of B-Trees (continued) Figure 7 -2 Nodes of a binary tree can be located in different blocks on a disk Data Structures and Algorithms in Java 6
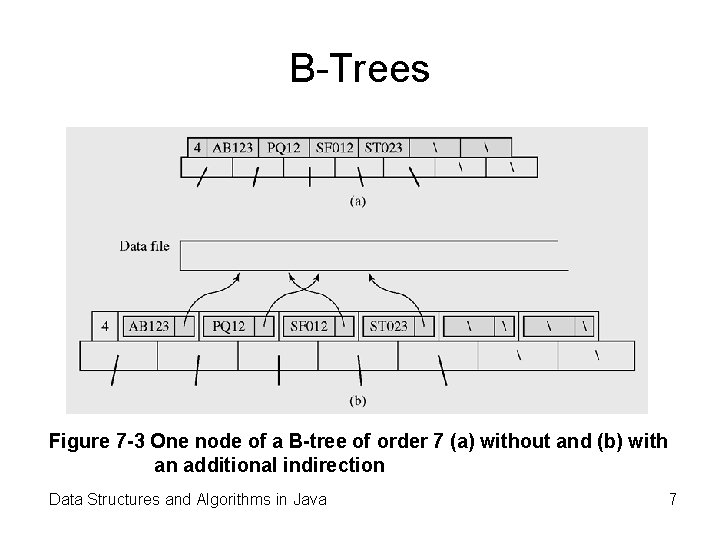
B-Trees Figure 7 -3 One node of a B-tree of order 7 (a) without and (b) with an additional indirection Data Structures and Algorithms in Java 7
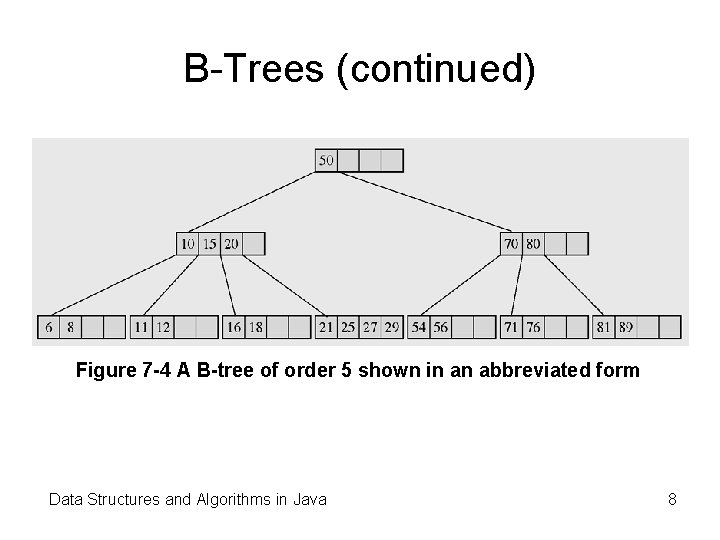
B-Trees (continued) Figure 7 -4 A B-tree of order 5 shown in an abbreviated form Data Structures and Algorithms in Java 8
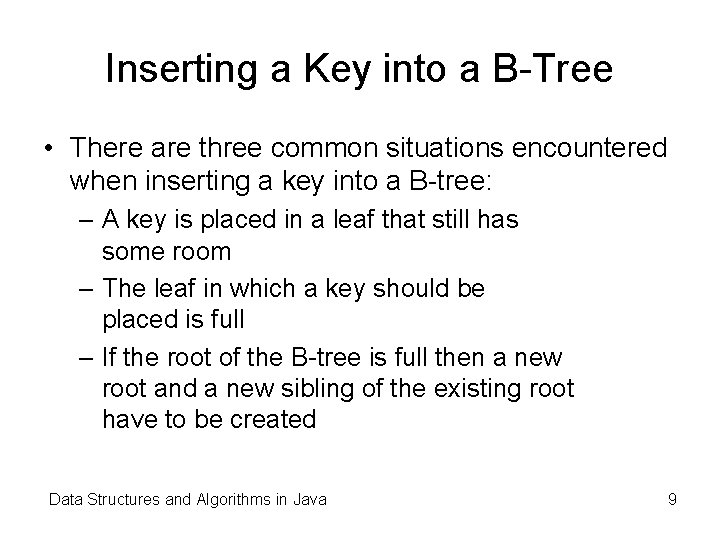
Inserting a Key into a B-Tree • There are three common situations encountered when inserting a key into a B-tree: – A key is placed in a leaf that still has some room – The leaf in which a key should be placed is full – If the root of the B-tree is full then a new root and a new sibling of the existing root have to be created Data Structures and Algorithms in Java 9
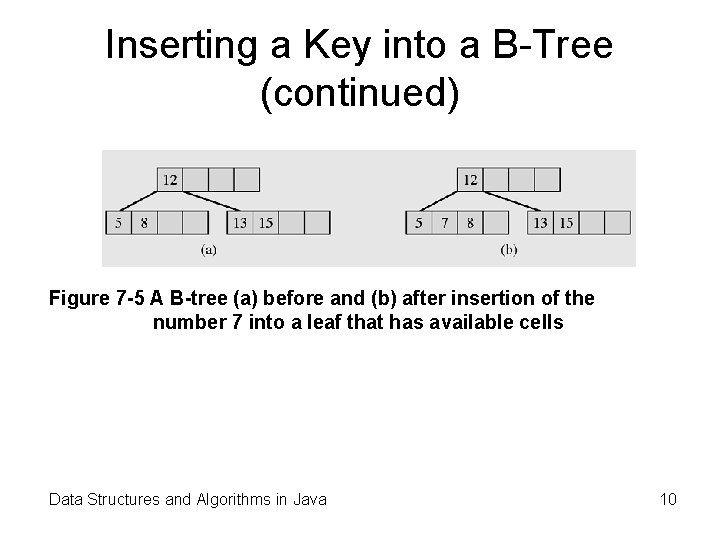
Inserting a Key into a B-Tree (continued) Figure 7 -5 A B-tree (a) before and (b) after insertion of the number 7 into a leaf that has available cells Data Structures and Algorithms in Java 10
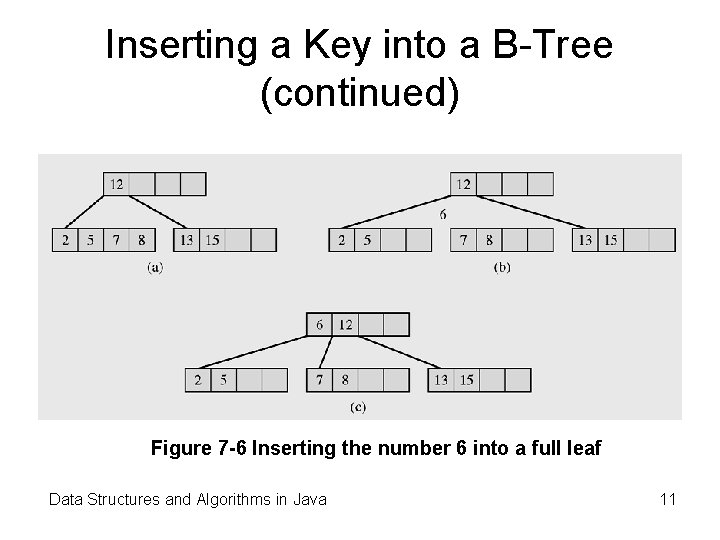
Inserting a Key into a B-Tree (continued) Figure 7 -6 Inserting the number 6 into a full leaf Data Structures and Algorithms in Java 11
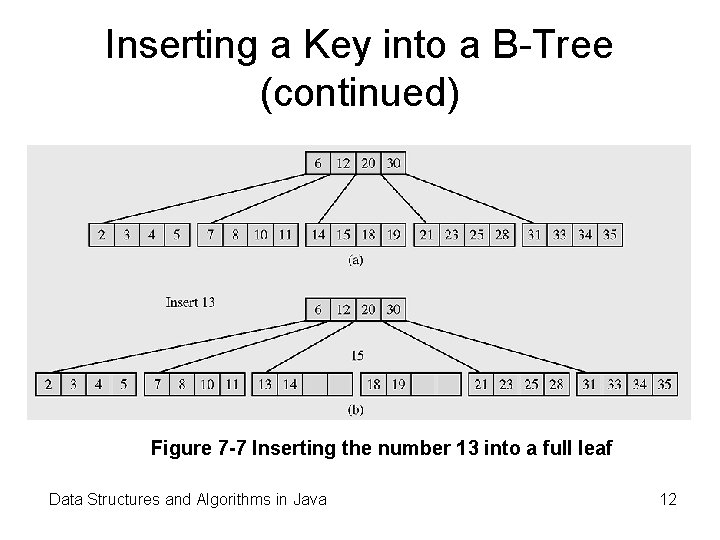
Inserting a Key into a B-Tree (continued) Figure 7 -7 Inserting the number 13 into a full leaf Data Structures and Algorithms in Java 12
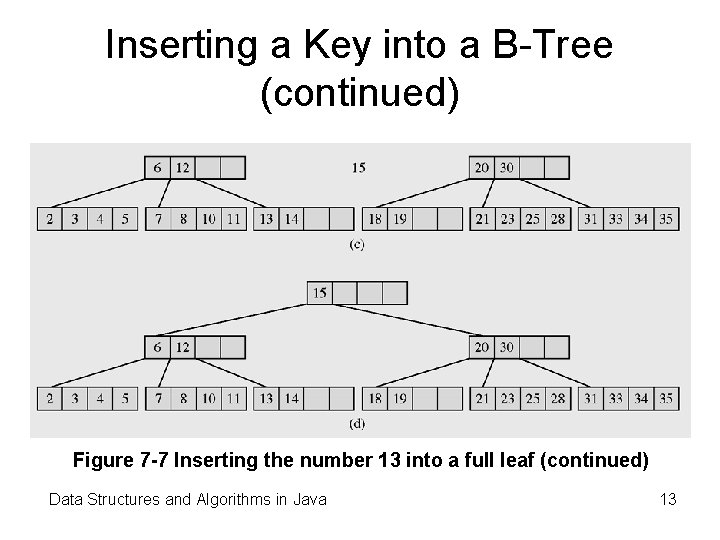
Inserting a Key into a B-Tree (continued) Figure 7 -7 Inserting the number 13 into a full leaf (continued) Data Structures and Algorithms in Java 13
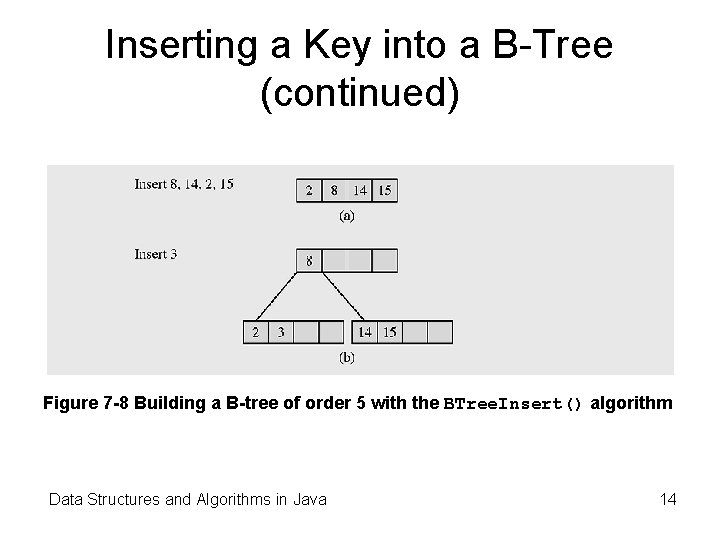
Inserting a Key into a B-Tree (continued) Figure 7 -8 Building a B-tree of order 5 with the BTree. Insert() algorithm Data Structures and Algorithms in Java 14
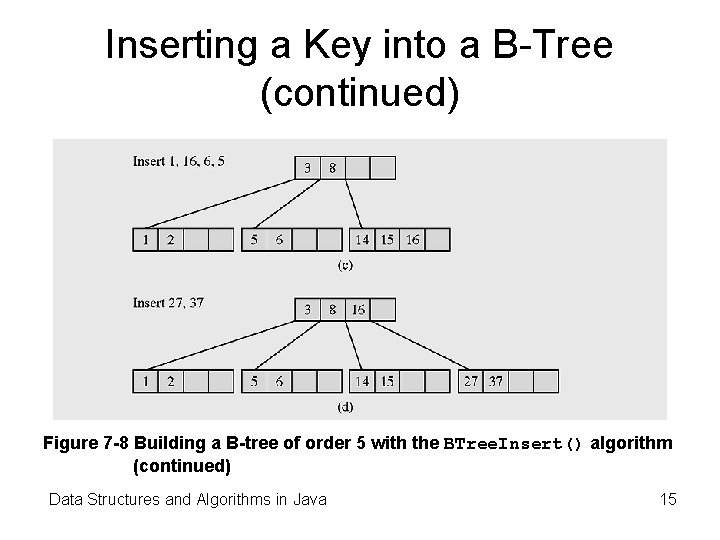
Inserting a Key into a B-Tree (continued) Figure 7 -8 Building a B-tree of order 5 with the BTree. Insert() algorithm (continued) Data Structures and Algorithms in Java 15
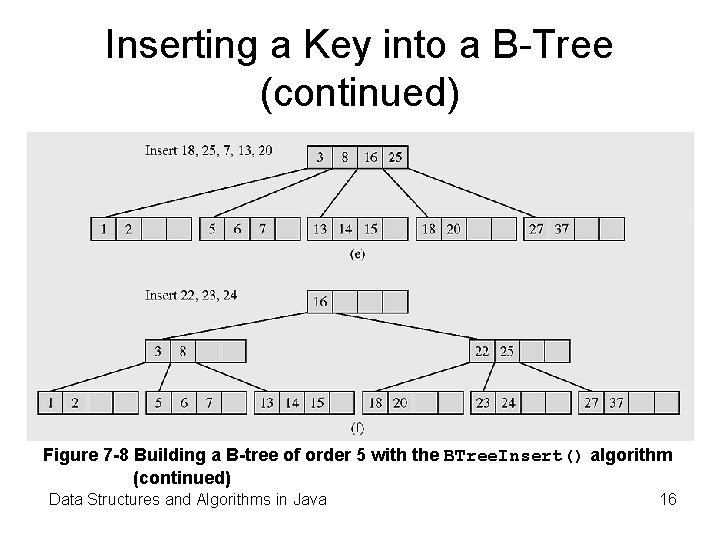
Inserting a Key into a B-Tree (continued) Figure 7 -8 Building a B-tree of order 5 with the BTree. Insert() algorithm (continued) Data Structures and Algorithms in Java 16
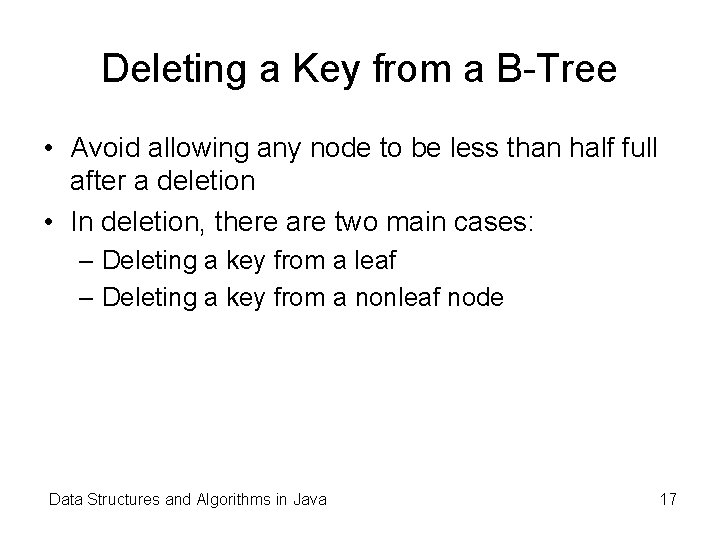
Deleting a Key from a B-Tree • Avoid allowing any node to be less than half full after a deletion • In deletion, there are two main cases: – Deleting a key from a leaf – Deleting a key from a nonleaf node Data Structures and Algorithms in Java 17
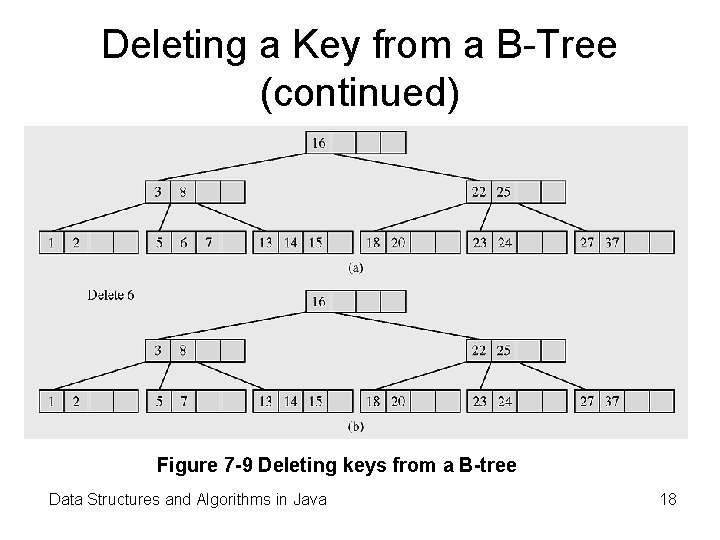
Deleting a Key from a B-Tree (continued) Figure 7 -9 Deleting keys from a B-tree Data Structures and Algorithms in Java 18
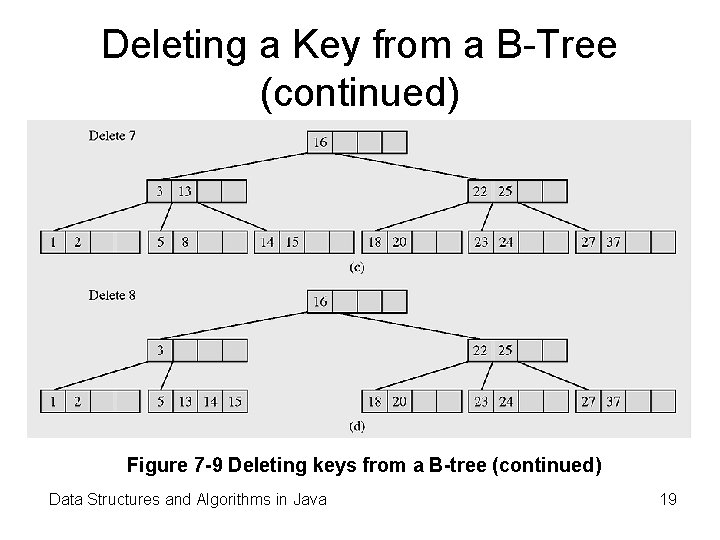
Deleting a Key from a B-Tree (continued) Figure 7 -9 Deleting keys from a B-tree (continued) Data Structures and Algorithms in Java 19
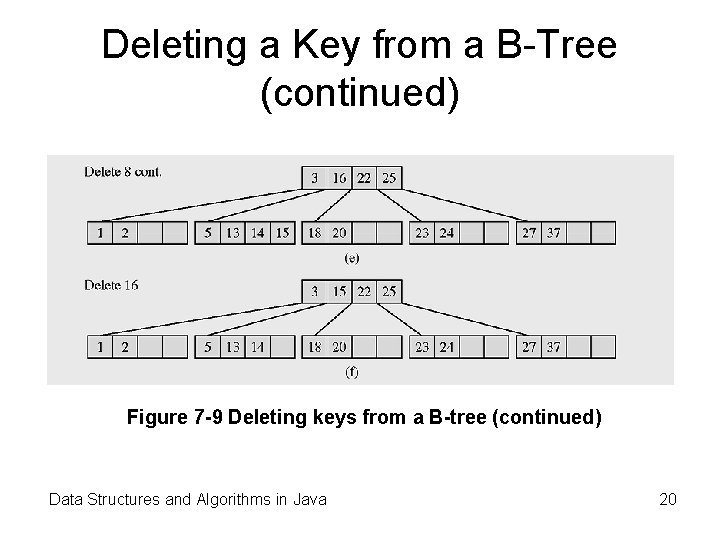
Deleting a Key from a B-Tree (continued) Figure 7 -9 Deleting keys from a B-tree (continued) Data Structures and Algorithms in Java 20
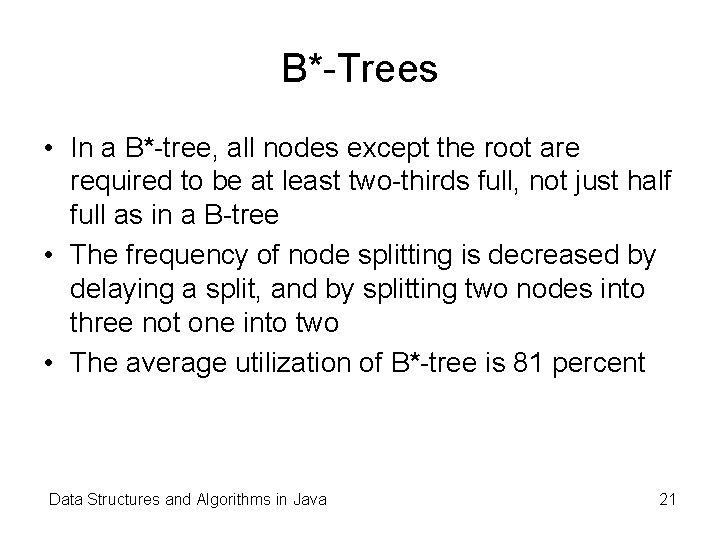
B*-Trees • In a B*-tree, all nodes except the root are required to be at least two-thirds full, not just half full as in a B-tree • The frequency of node splitting is decreased by delaying a split, and by splitting two nodes into three not one into two • The average utilization of B*-tree is 81 percent Data Structures and Algorithms in Java 21
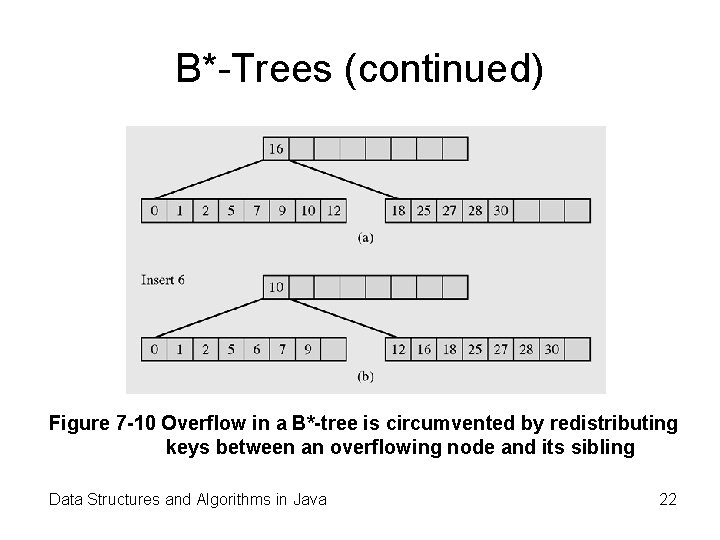
B*-Trees (continued) Figure 7 -10 Overflow in a B*-tree is circumvented by redistributing keys between an overflowing node and its sibling Data Structures and Algorithms in Java 22
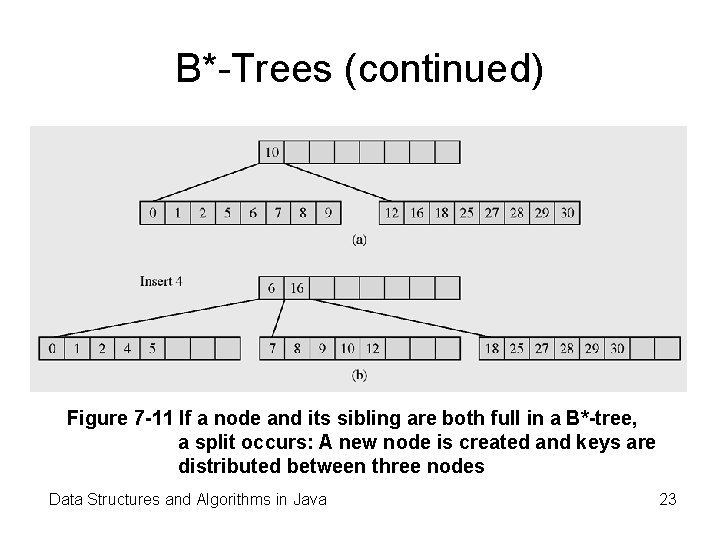
B*-Trees (continued) Figure 7 -11 If a node and its sibling are both full in a B*-tree, a split occurs: A new node is created and keys are distributed between three nodes Data Structures and Algorithms in Java 23
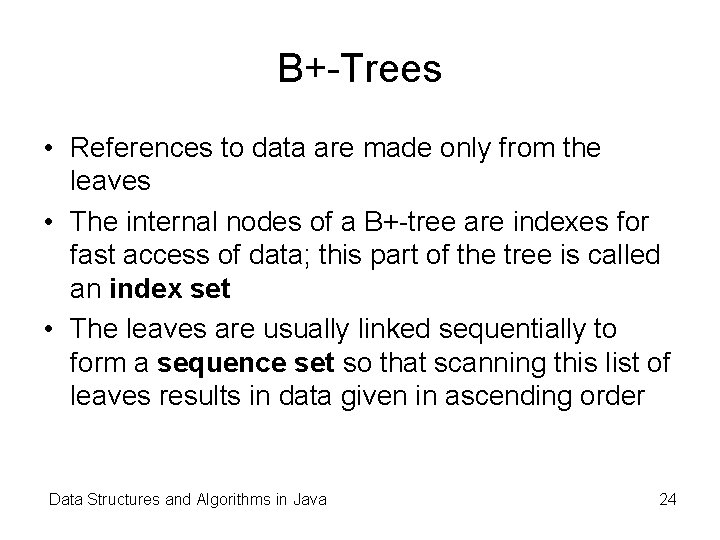
B+-Trees • References to data are made only from the leaves • The internal nodes of a B+-tree are indexes for fast access of data; this part of the tree is called an index set • The leaves are usually linked sequentially to form a sequence set so that scanning this list of leaves results in data given in ascending order Data Structures and Algorithms in Java 24
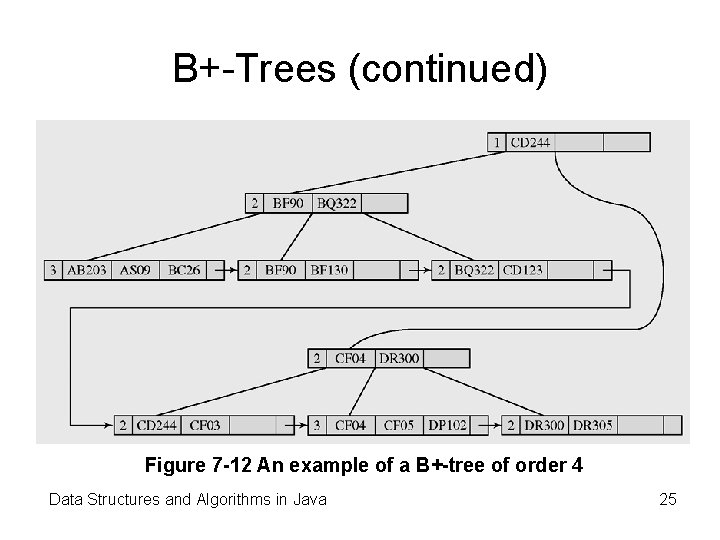
B+-Trees (continued) Figure 7 -12 An example of a B+-tree of order 4 Data Structures and Algorithms in Java 25
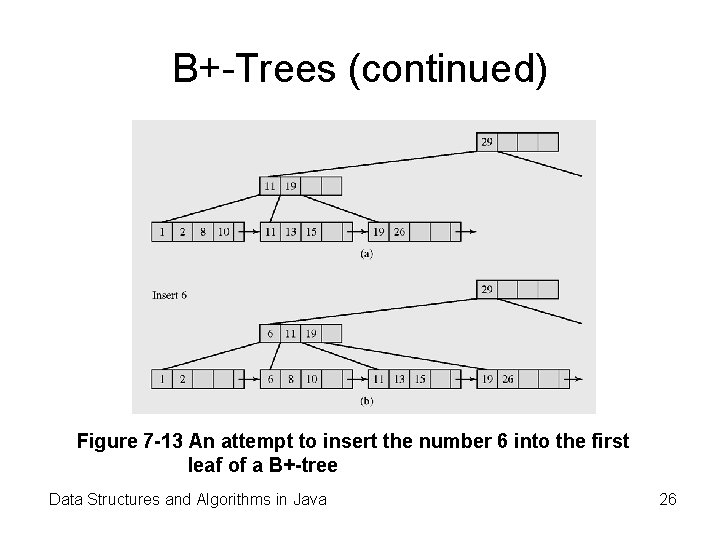
B+-Trees (continued) Figure 7 -13 An attempt to insert the number 6 into the first leaf of a B+-tree Data Structures and Algorithms in Java 26
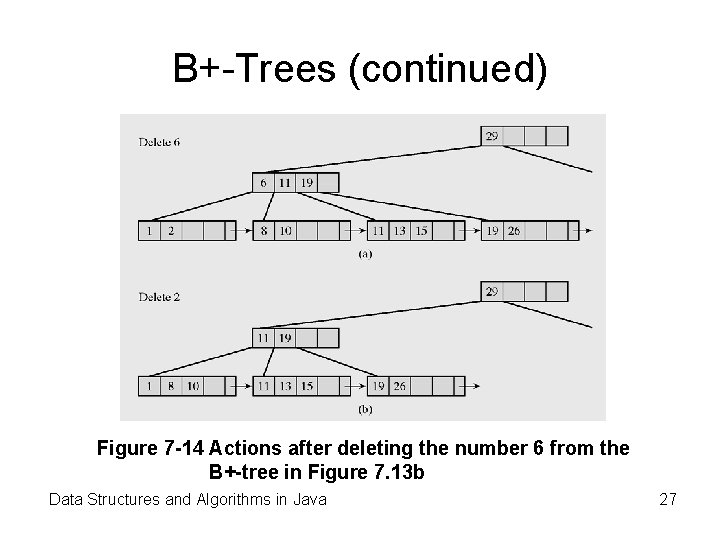
B+-Trees (continued) Figure 7 -14 Actions after deleting the number 6 from the B+-tree in Figure 7. 13 b Data Structures and Algorithms in Java 27
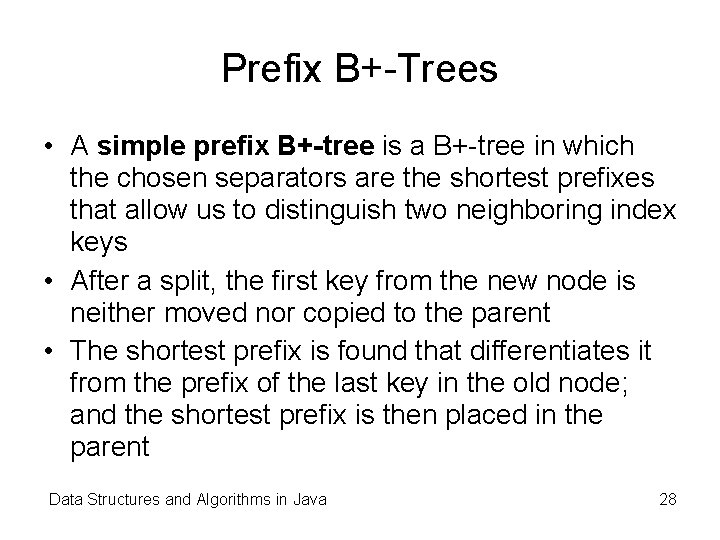
Prefix B+-Trees • A simple prefix B+-tree is a B+-tree in which the chosen separators are the shortest prefixes that allow us to distinguish two neighboring index keys • After a split, the first key from the new node is neither moved nor copied to the parent • The shortest prefix is found that differentiates it from the prefix of the last key in the old node; and the shortest prefix is then placed in the parent Data Structures and Algorithms in Java 28
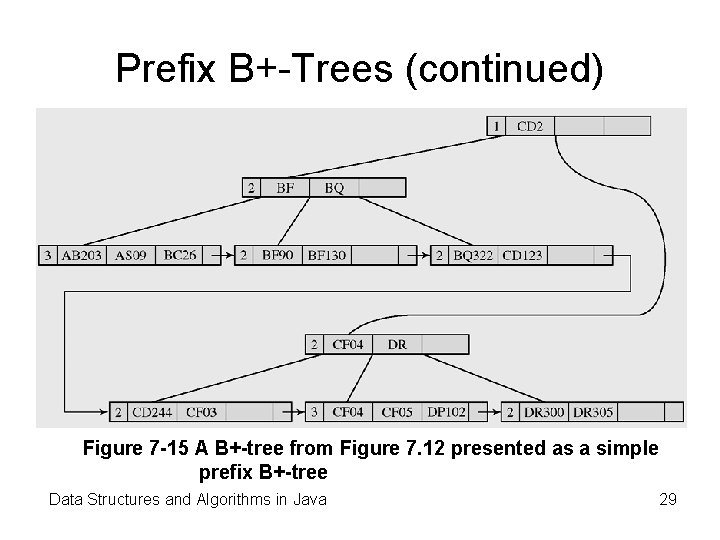
Prefix B+-Trees (continued) Figure 7 -15 A B+-tree from Figure 7. 12 presented as a simple prefix B+-tree Data Structures and Algorithms in Java 29
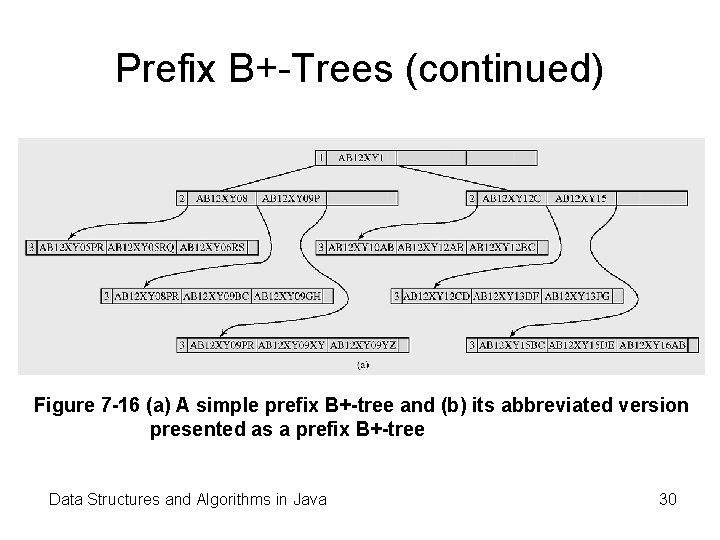
Prefix B+-Trees (continued) Figure 7 -16 (a) A simple prefix B+-tree and (b) its abbreviated version presented as a prefix B+-tree Data Structures and Algorithms in Java 30
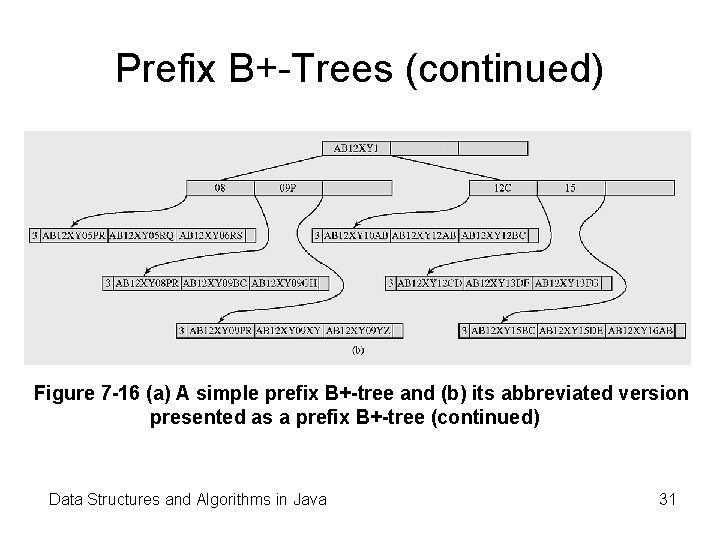
Prefix B+-Trees (continued) Figure 7 -16 (a) A simple prefix B+-tree and (b) its abbreviated version presented as a prefix B+-tree (continued) Data Structures and Algorithms in Java 31
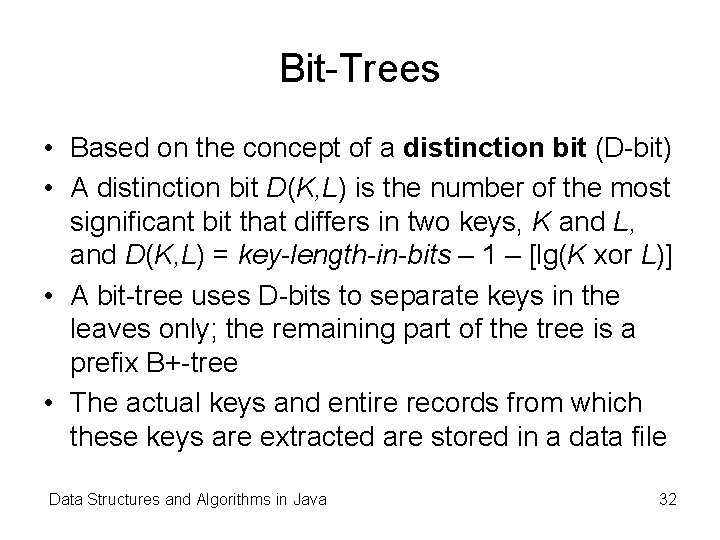
Bit-Trees • Based on the concept of a distinction bit (D-bit) • A distinction bit D(K, L) is the number of the most significant bit that differs in two keys, K and L, and D(K, L) = key-length-in-bits – 1 – [lg(K xor L)] • A bit-tree uses D-bits to separate keys in the leaves only; the remaining part of the tree is a prefix B+-tree • The actual keys and entire records from which these keys are extracted are stored in a data file Data Structures and Algorithms in Java 32
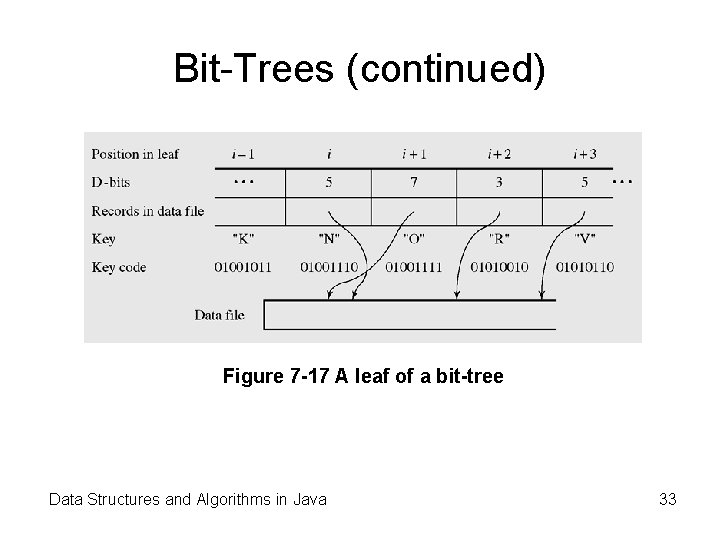
Bit-Trees (continued) Figure 7 -17 A leaf of a bit-tree Data Structures and Algorithms in Java 33
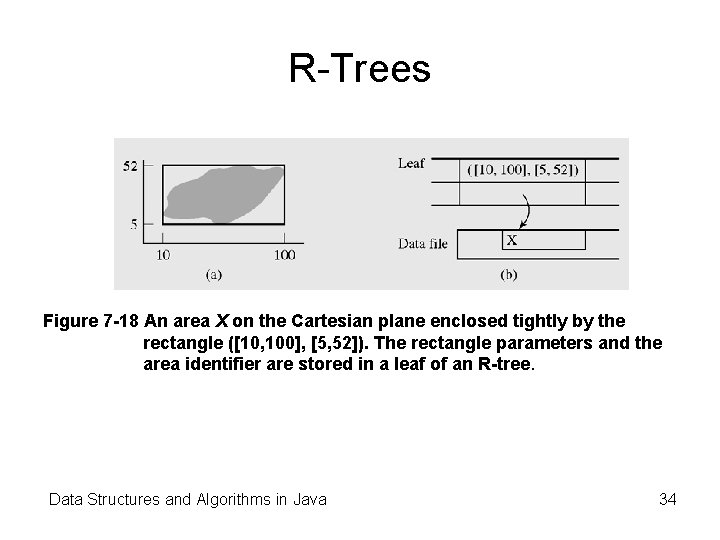
R-Trees Figure 7 -18 An area X on the Cartesian plane enclosed tightly by the rectangle ([10, 100], [5, 52]). The rectangle parameters and the area identifier are stored in a leaf of an R-tree. Data Structures and Algorithms in Java 34
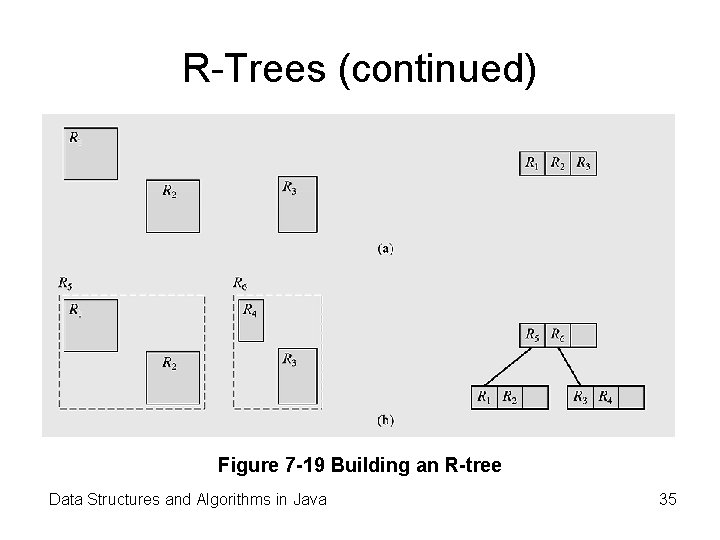
R-Trees (continued) Figure 7 -19 Building an R-tree Data Structures and Algorithms in Java 35
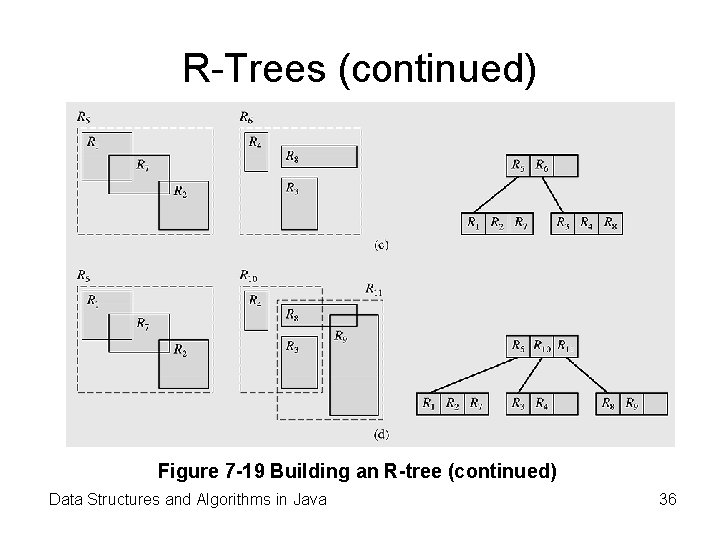
R-Trees (continued) Figure 7 -19 Building an R-tree (continued) Data Structures and Algorithms in Java 36
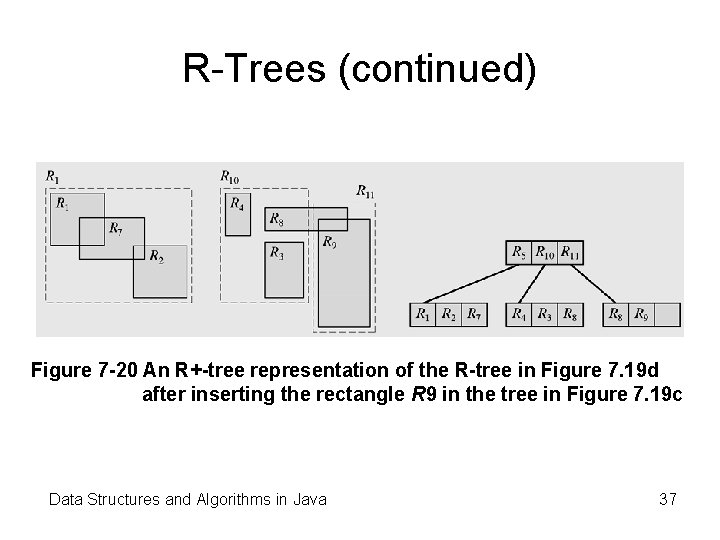
R-Trees (continued) Figure 7 -20 An R+-tree representation of the R-tree in Figure 7. 19 d after inserting the rectangle R 9 in the tree in Figure 7. 19 c Data Structures and Algorithms in Java 37
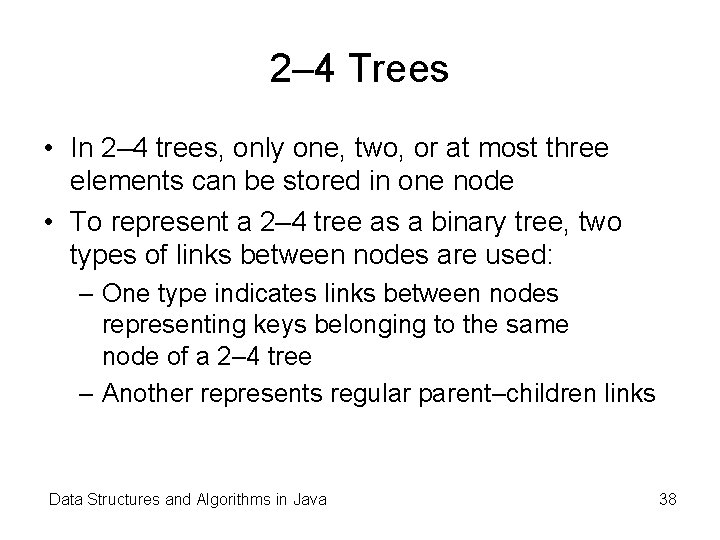
2– 4 Trees • In 2– 4 trees, only one, two, or at most three elements can be stored in one node • To represent a 2– 4 tree as a binary tree, two types of links between nodes are used: – One type indicates links between nodes representing keys belonging to the same node of a 2– 4 tree – Another represents regular parent–children links Data Structures and Algorithms in Java 38
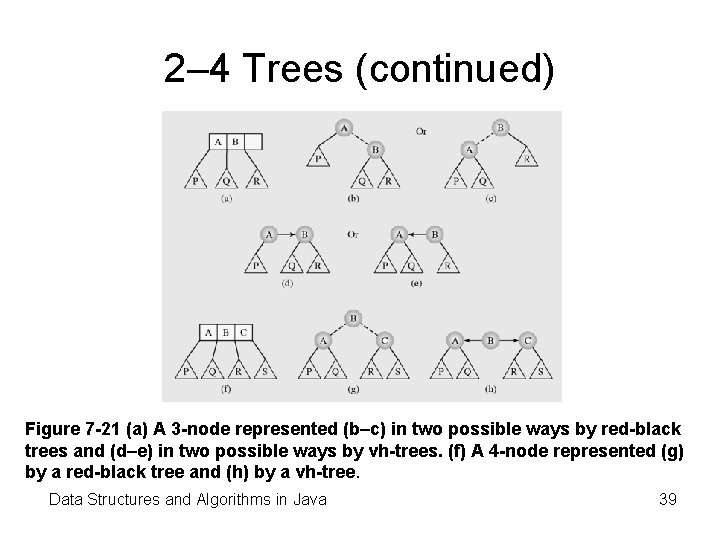
2– 4 Trees (continued) Figure 7 -21 (a) A 3 -node represented (b–c) in two possible ways by red-black trees and (d–e) in two possible ways by vh-trees. (f) A 4 -node represented (g) by a red-black tree and (h) by a vh-tree. Data Structures and Algorithms in Java 39
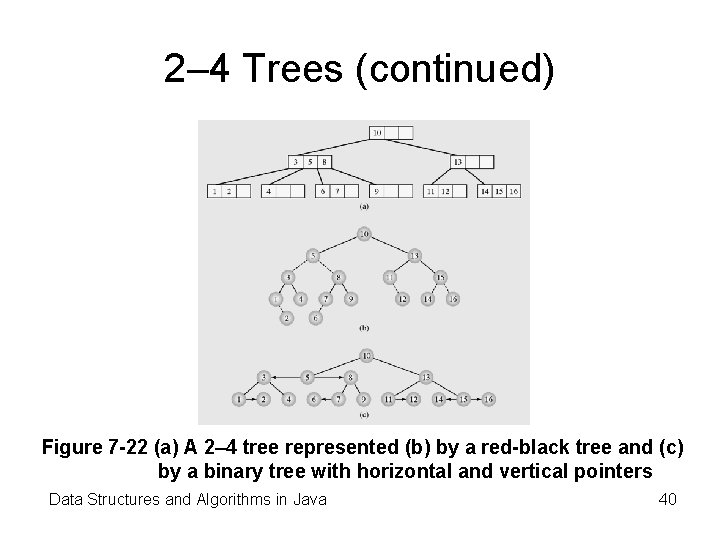
2– 4 Trees (continued) Figure 7 -22 (a) A 2– 4 tree represented (b) by a red-black tree and (c) by a binary tree with horizontal and vertical pointers Data Structures and Algorithms in Java 40
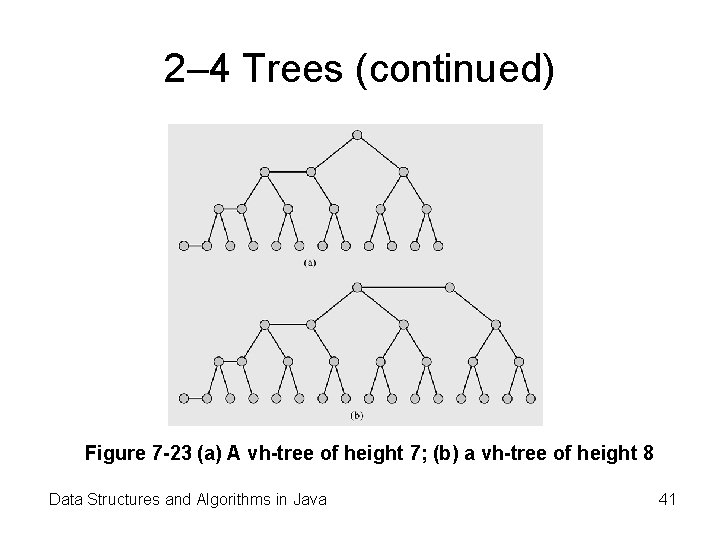
2– 4 Trees (continued) Figure 7 -23 (a) A vh-tree of height 7; (b) a vh-tree of height 8 Data Structures and Algorithms in Java 41
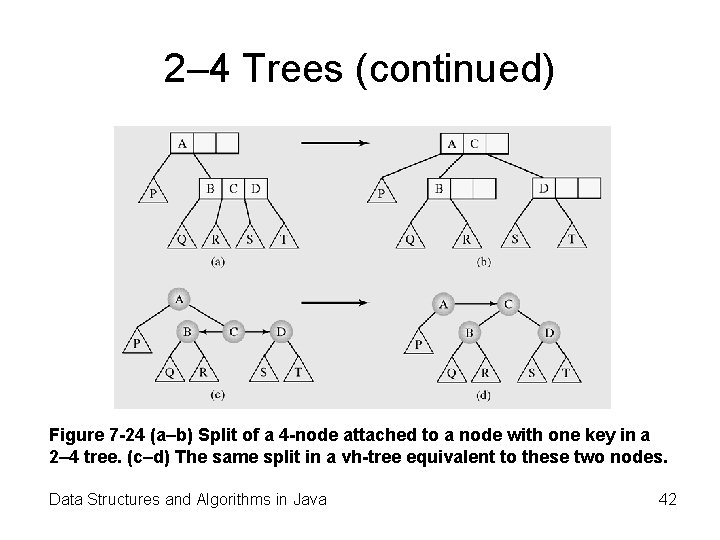
2– 4 Trees (continued) Figure 7 -24 (a–b) Split of a 4 -node attached to a node with one key in a 2– 4 tree. (c–d) The same split in a vh-tree equivalent to these two nodes. Data Structures and Algorithms in Java 42
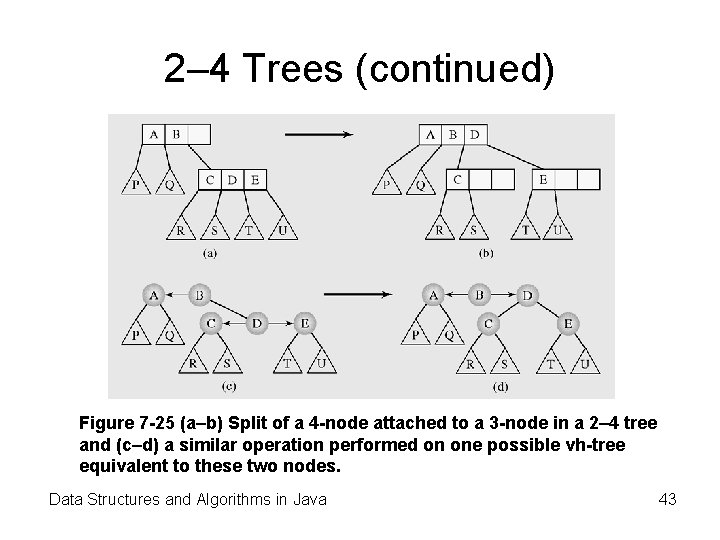
2– 4 Trees (continued) Figure 7 -25 (a–b) Split of a 4 -node attached to a 3 -node in a 2– 4 tree and (c–d) a similar operation performed on one possible vh-tree equivalent to these two nodes. Data Structures and Algorithms in Java 43
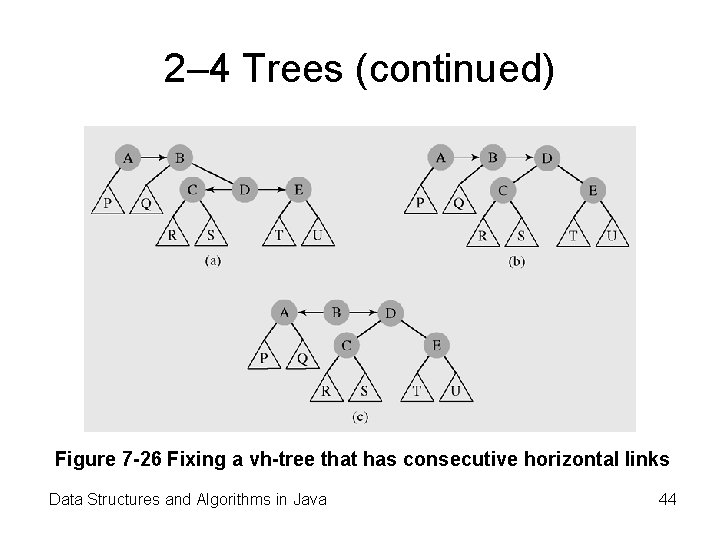
2– 4 Trees (continued) Figure 7 -26 Fixing a vh-tree that has consecutive horizontal links Data Structures and Algorithms in Java 44
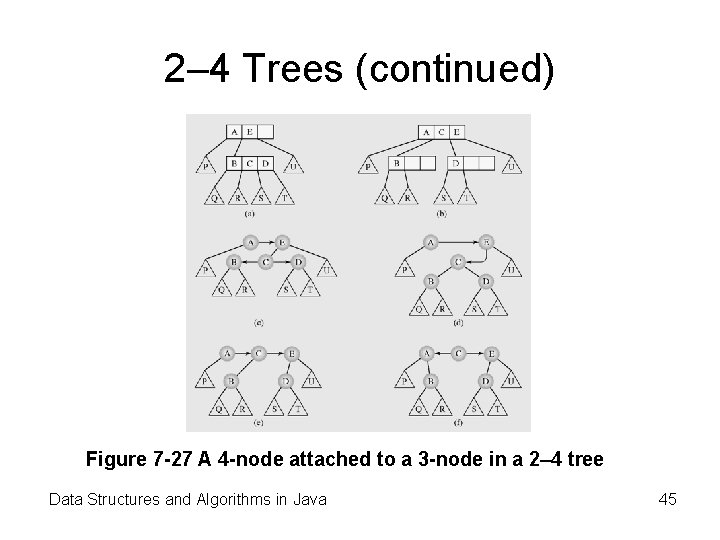
2– 4 Trees (continued) Figure 7 -27 A 4 -node attached to a 3 -node in a 2– 4 tree Data Structures and Algorithms in Java 45
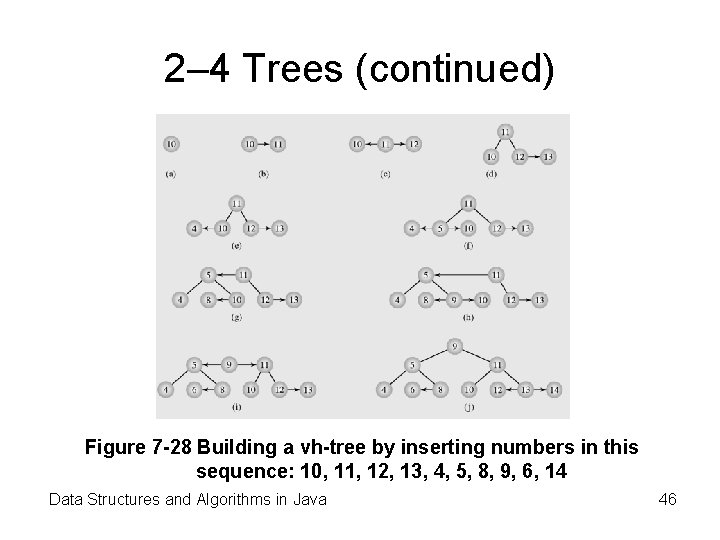
2– 4 Trees (continued) Figure 7 -28 Building a vh-tree by inserting numbers in this sequence: 10, 11, 12, 13, 4, 5, 8, 9, 6, 14 Data Structures and Algorithms in Java 46
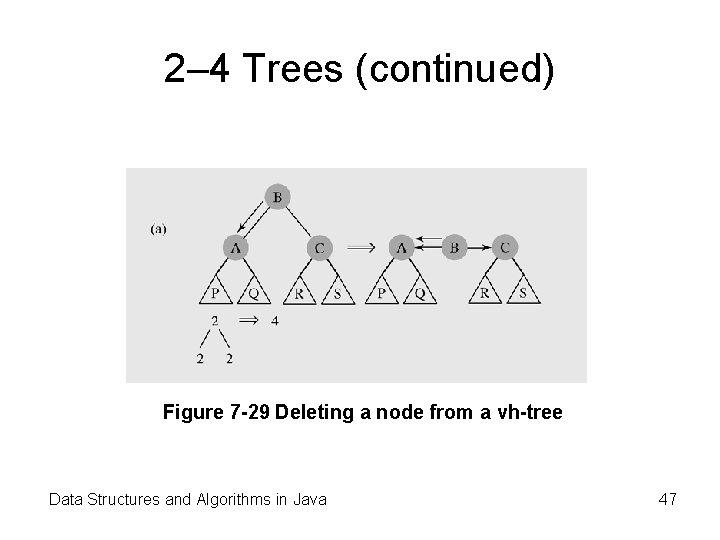
2– 4 Trees (continued) Figure 7 -29 Deleting a node from a vh-tree Data Structures and Algorithms in Java 47
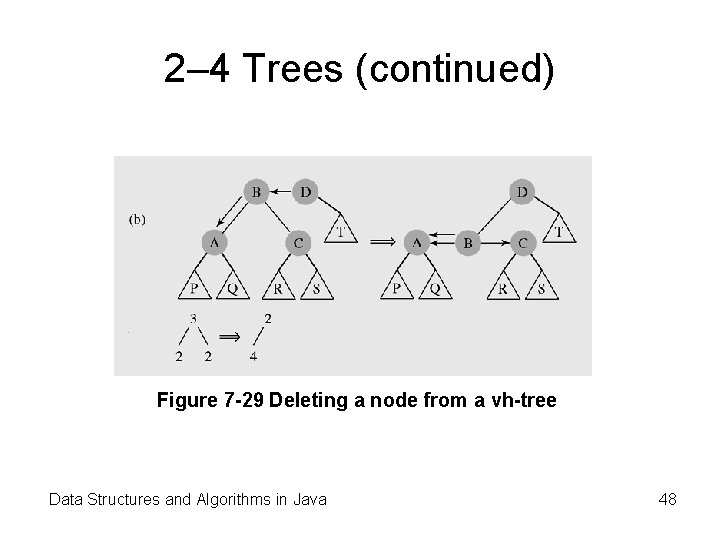
2– 4 Trees (continued) Figure 7 -29 Deleting a node from a vh-tree Data Structures and Algorithms in Java 48
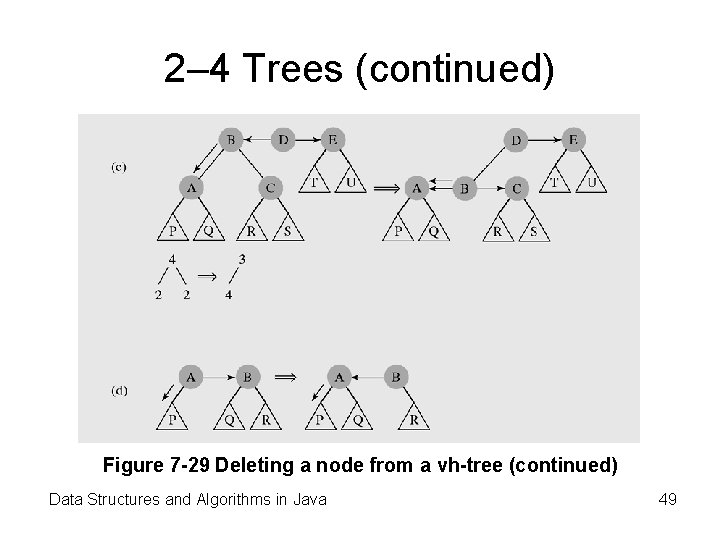
2– 4 Trees (continued) Figure 7 -29 Deleting a node from a vh-tree (continued) Data Structures and Algorithms in Java 49
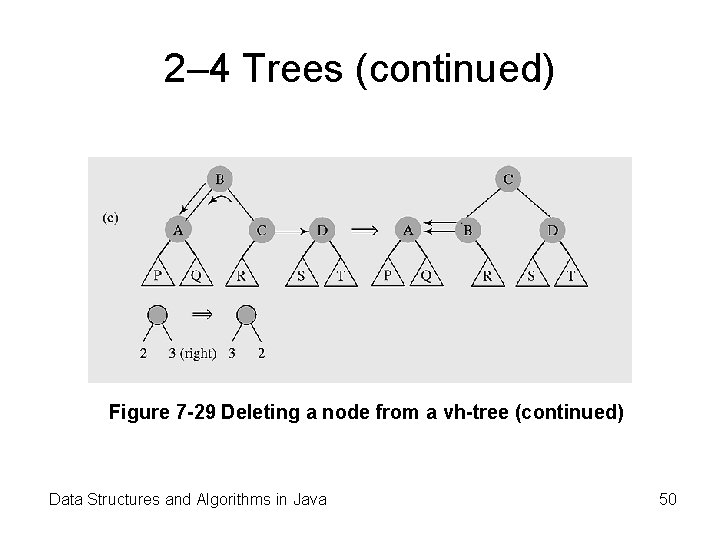
2– 4 Trees (continued) Figure 7 -29 Deleting a node from a vh-tree (continued) Data Structures and Algorithms in Java 50
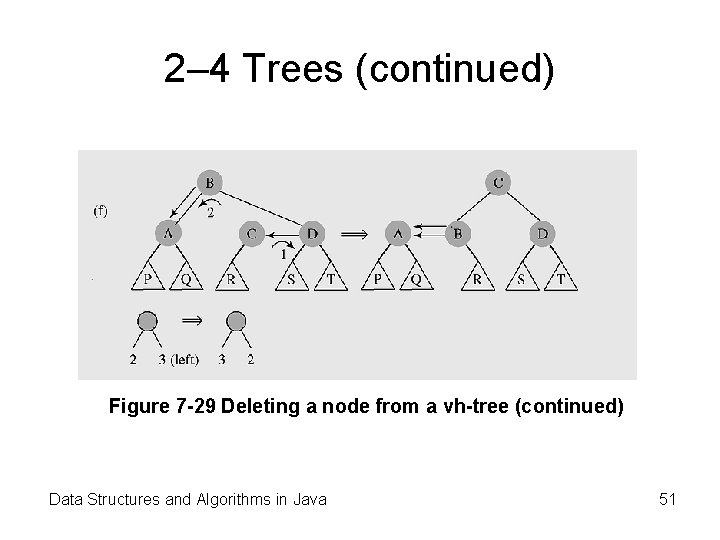
2– 4 Trees (continued) Figure 7 -29 Deleting a node from a vh-tree (continued) Data Structures and Algorithms in Java 51
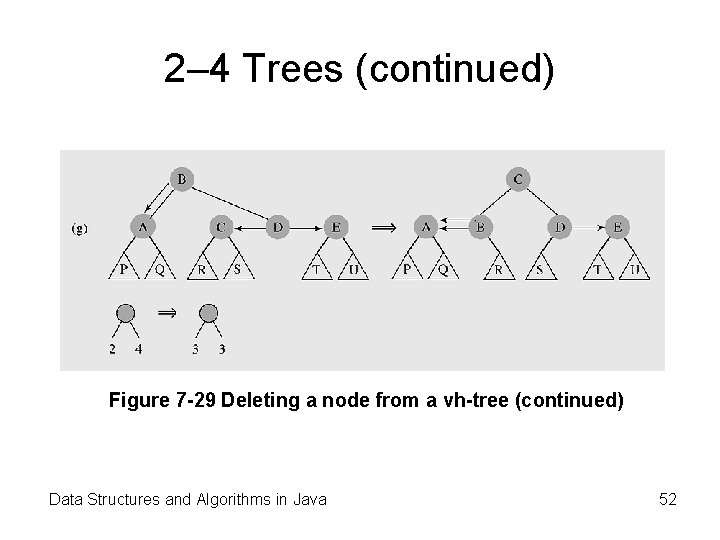
2– 4 Trees (continued) Figure 7 -29 Deleting a node from a vh-tree (continued) Data Structures and Algorithms in Java 52
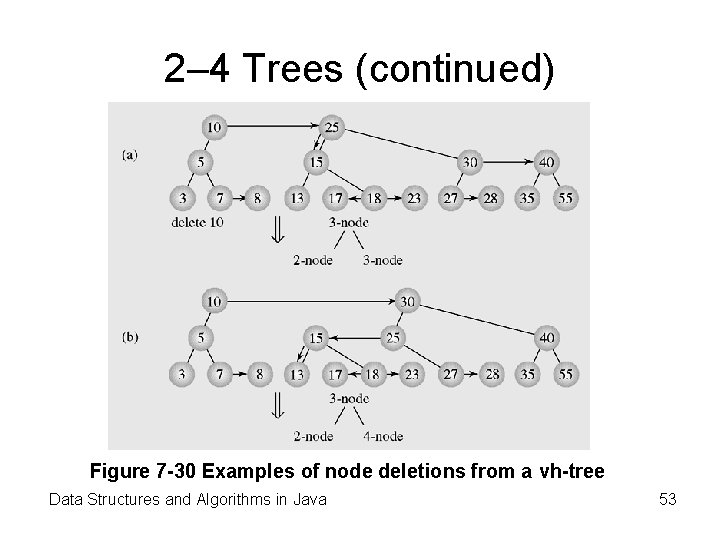
2– 4 Trees (continued) Figure 7 -30 Examples of node deletions from a vh-tree Data Structures and Algorithms in Java 53
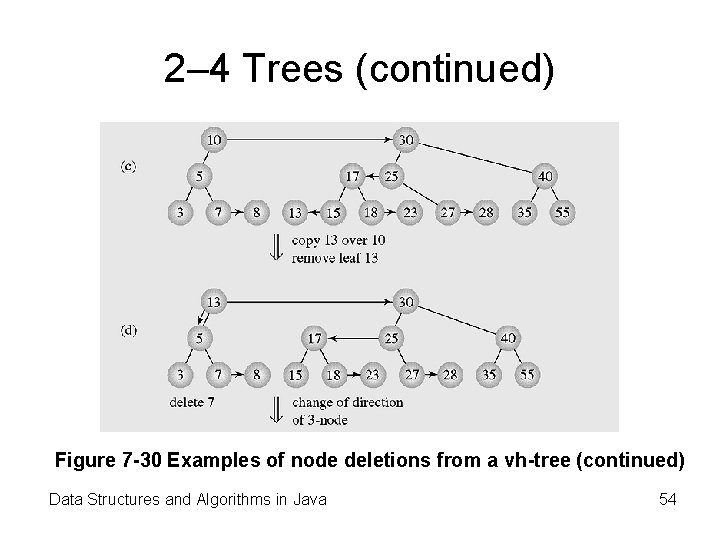
2– 4 Trees (continued) Figure 7 -30 Examples of node deletions from a vh-tree (continued) Data Structures and Algorithms in Java 54
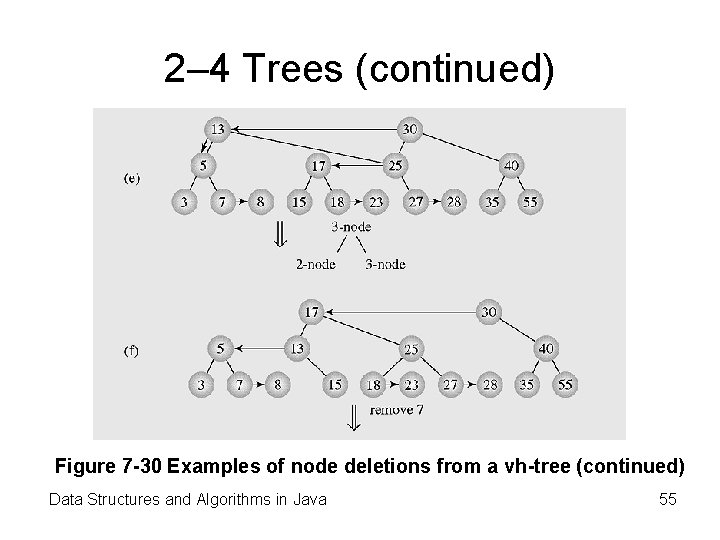
2– 4 Trees (continued) Figure 7 -30 Examples of node deletions from a vh-tree (continued) Data Structures and Algorithms in Java 55
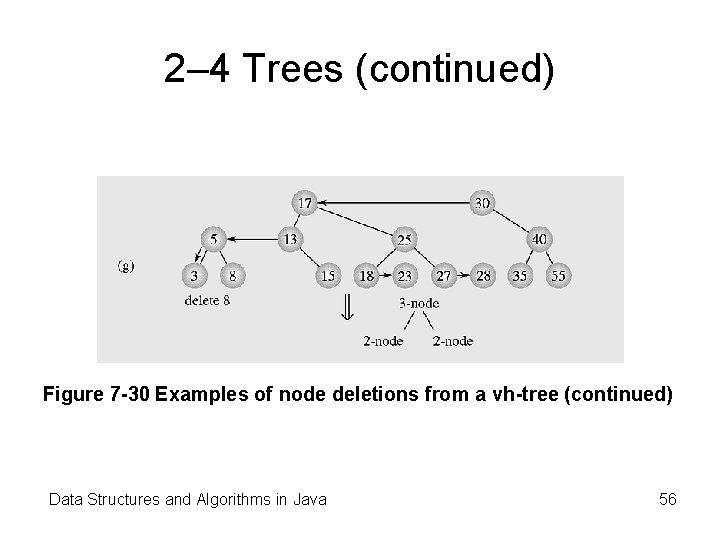
2– 4 Trees (continued) Figure 7 -30 Examples of node deletions from a vh-tree (continued) Data Structures and Algorithms in Java 56
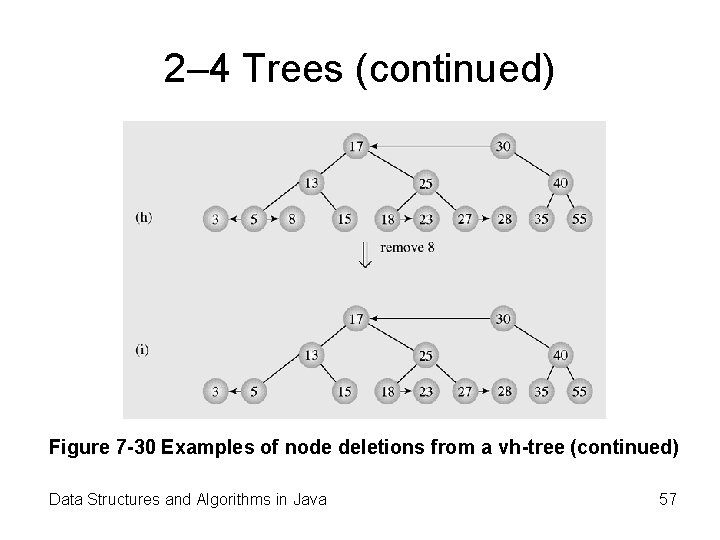
2– 4 Trees (continued) Figure 7 -30 Examples of node deletions from a vh-tree (continued) Data Structures and Algorithms in Java 57
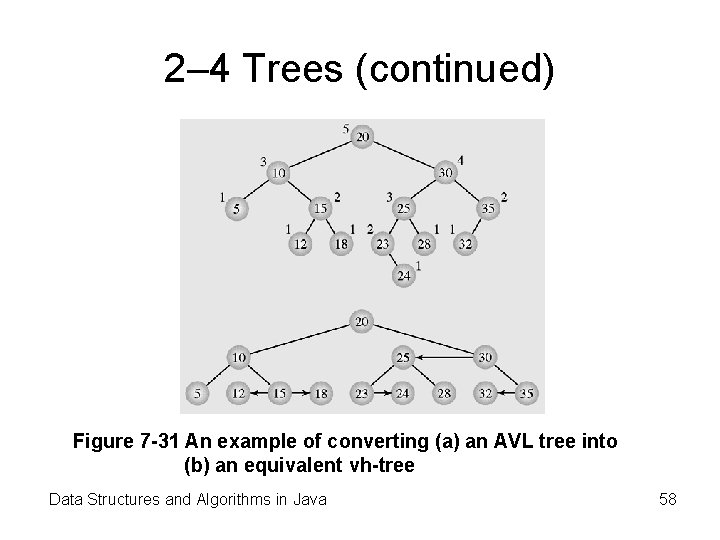
2– 4 Trees (continued) Figure 7 -31 An example of converting (a) an AVL tree into (b) an equivalent vh-tree Data Structures and Algorithms in Java 58
Multiway search tree
Data structures and algorithms iit bombay
Princeton data structures and algorithms
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Ajit diwan iit bombay
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Signature file structure in information retrieval system
Data structures and algorithms
Algorithms + data structures = programs
Two way selection and multiway selection
Two way selection and multiway selection in c
3 way search tree
Repetition flowchart examples
Multiway trie
105 ×106
Map reduce join
Two phase multiway merge sort
Multiway merge join pentaho
Examples of homologous
Data structures chapter 1
Data stream
Macro expansion in system software
Assembler algorithm and data structures
Data structures and abstractions with java
Adts, data structures, and problem solving with c++
Data structures and algorithm
Persistent and ephemeral data structures
Lesson 1 competition and market structures
Chapter 7 section 3 structures and organelles
Knee anatomy chapter 16 worksheet 1
Chapter 16 worksheet the knee and related structures
Chapter 7 section 3 structures and organelles
Bean trees chapter 9 summary
Why evergreen trees never lose their leaves story
Computational thinking algorithms and programming
1001 design
Association analysis: basic concepts and algorithms
Computer arithmetic: algorithms and hardware designs
Algorithms for select and join operations
Algorithms and flowcharts
Undecidable problems and unreasonable time algorithms.
Cluster analysis basic concepts and algorithms
Probabilistic analysis and randomized algorithms
Introduction of design and analysis of algorithms
Algorithms for query processing and optimization
Synchronization algorithms and concurrent programming
Parallel and distributed algorithms
Game programming algorithms and techniques
Cluster analysis basic concepts and algorithms
Cluster analysis basic concepts and algorithms
Aries algorithm
Dsp algorithms and architecture
Parametric and non parametric algorithms
Exercise 24
Binary search in design and analysis of algorithms