Sorting Algorithms CSCI 385 Data Structures Analysis of
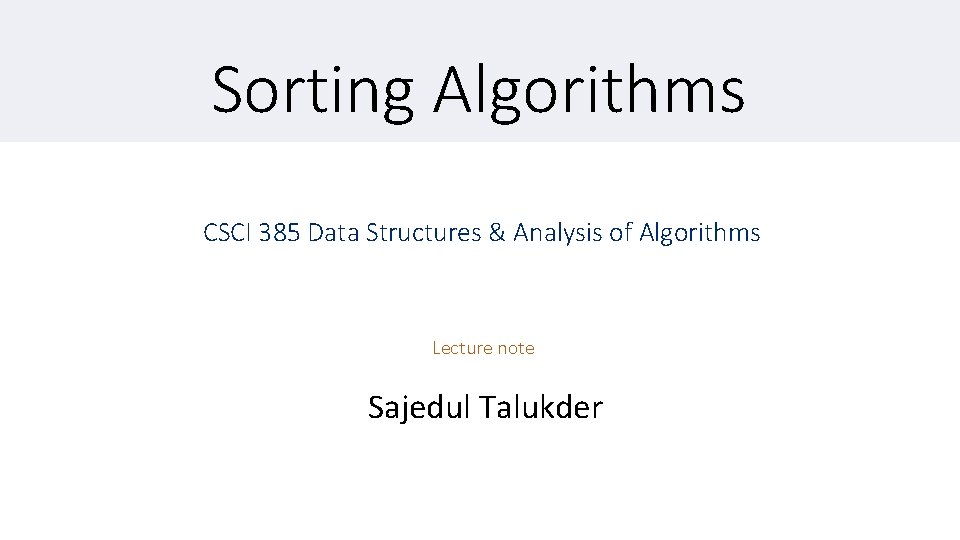
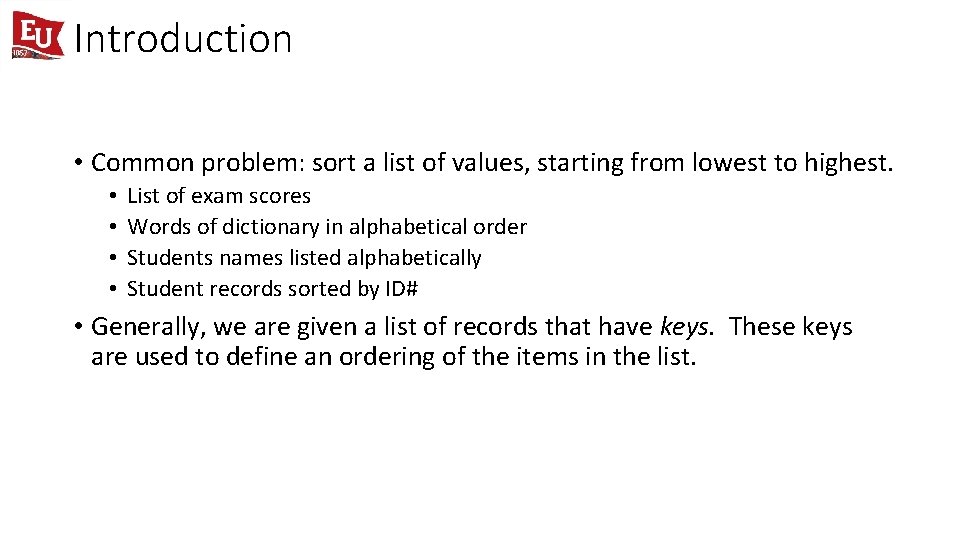
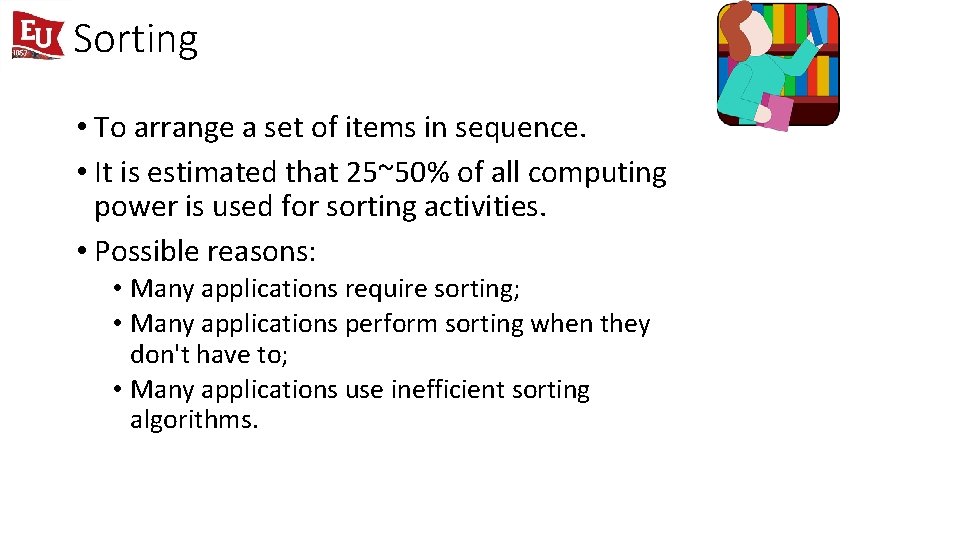
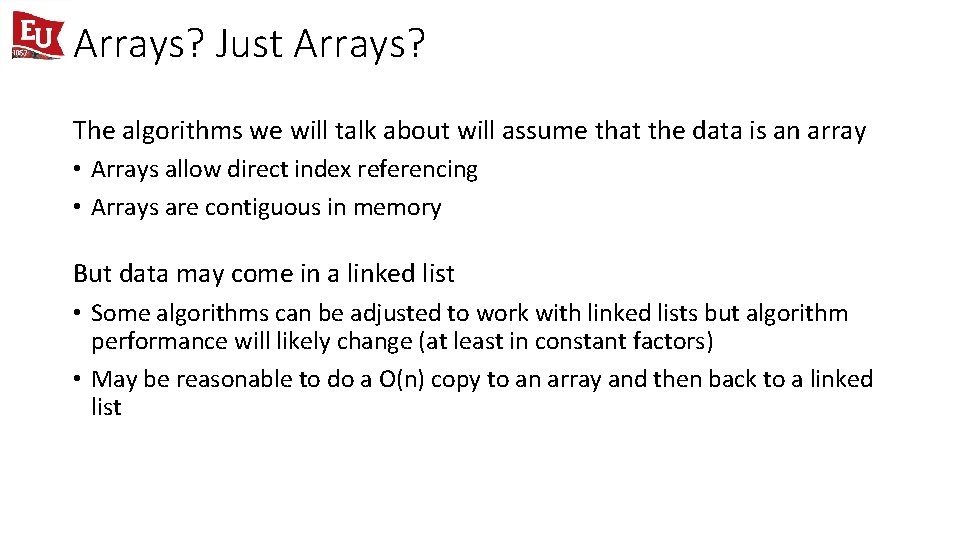
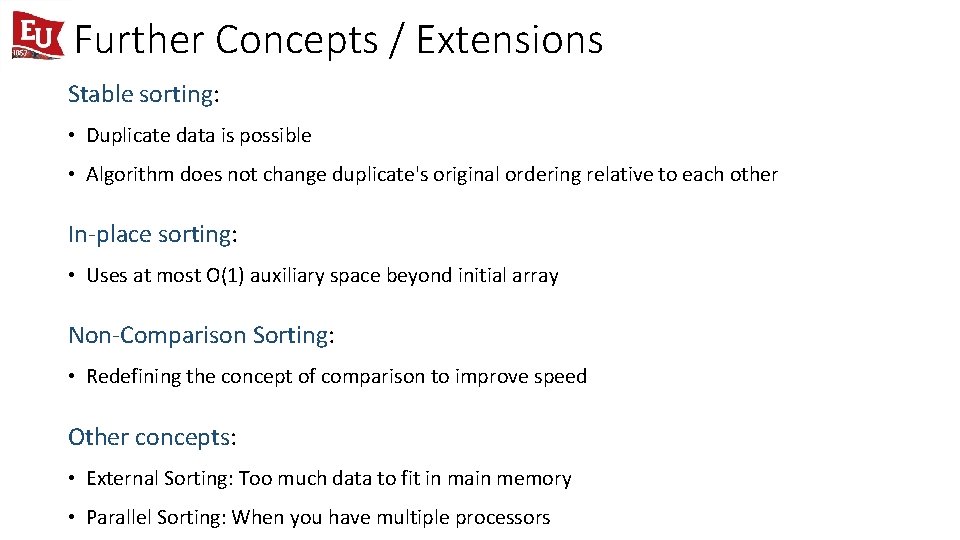
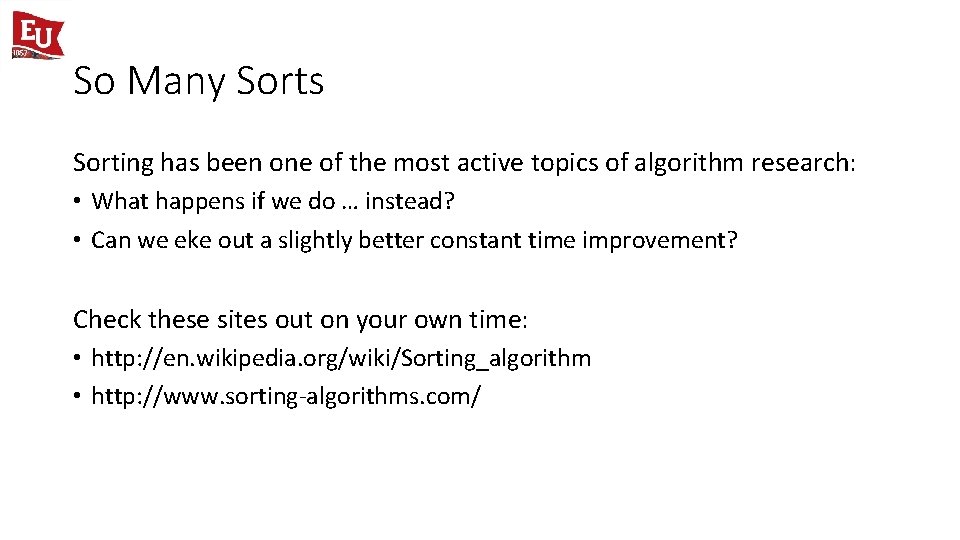
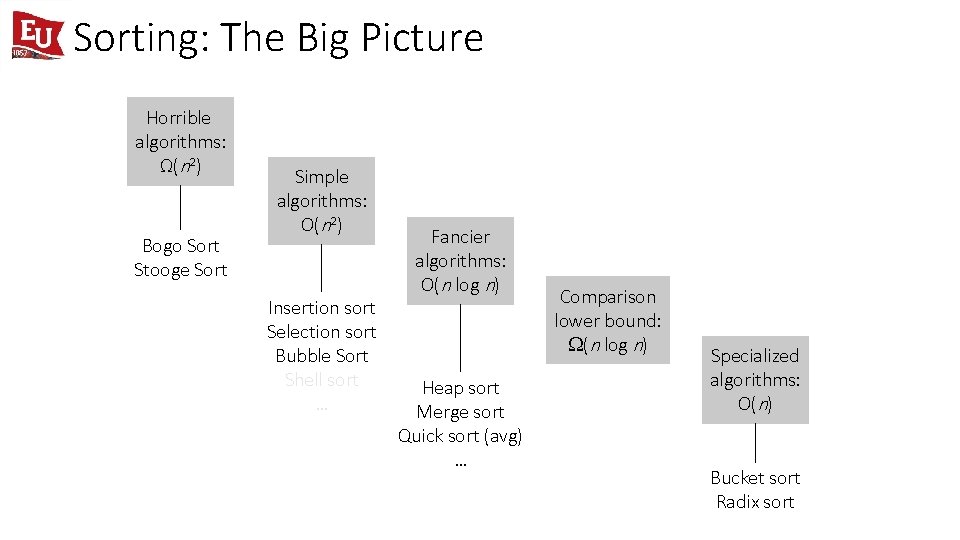
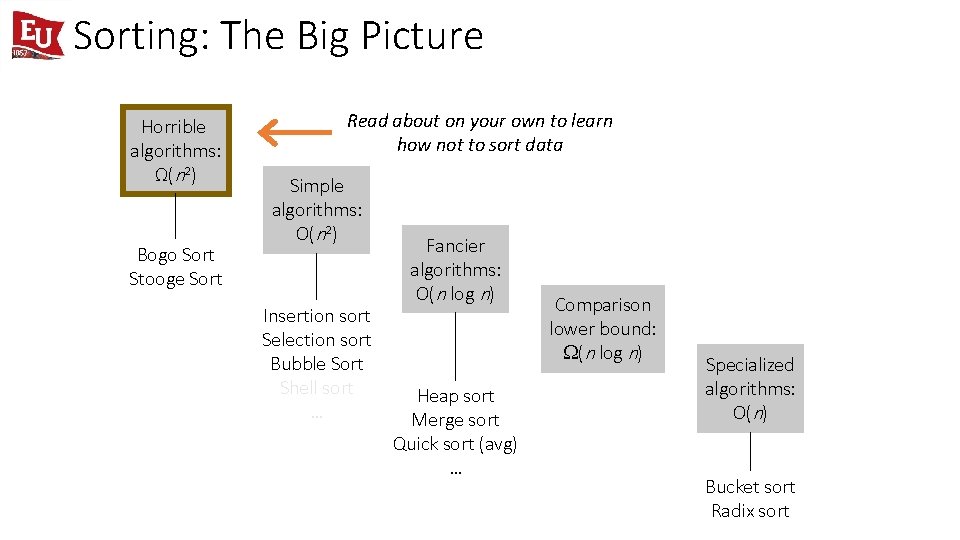
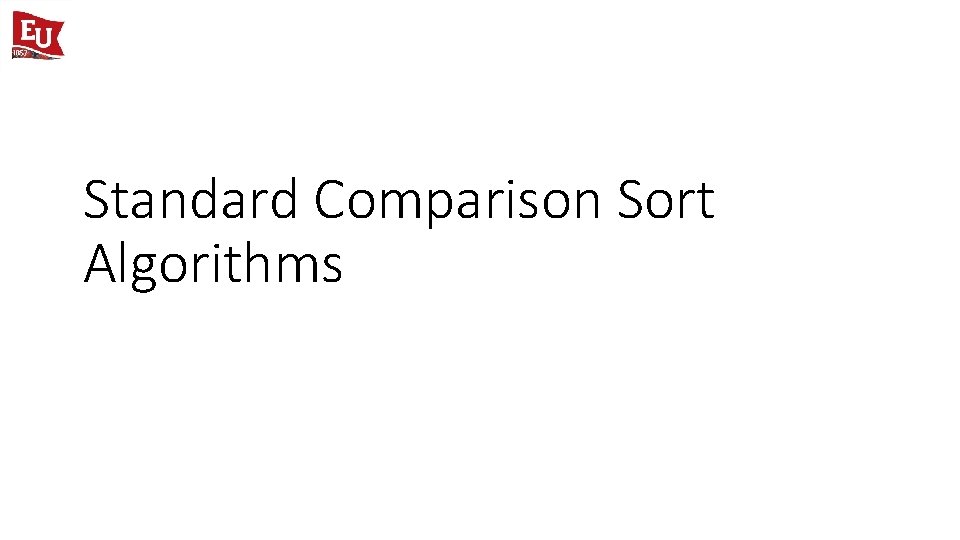
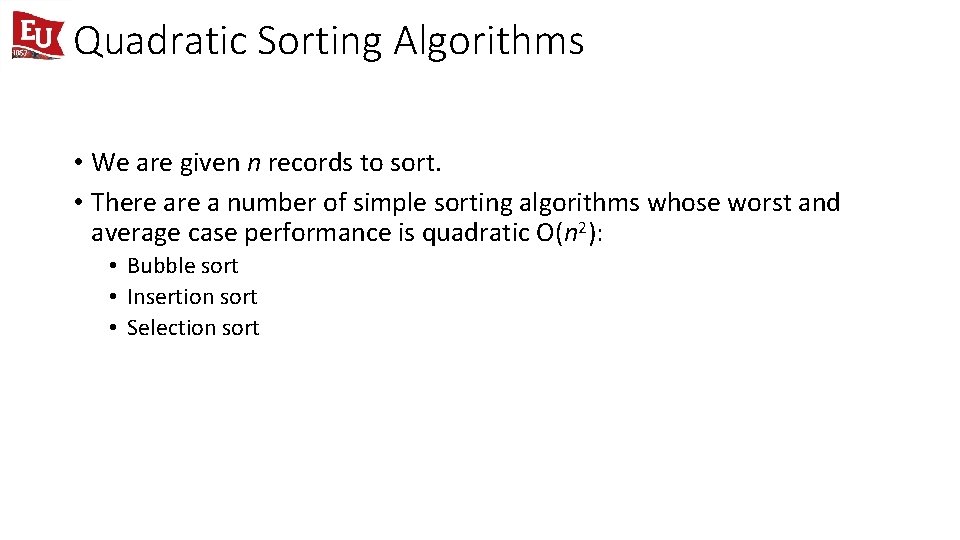
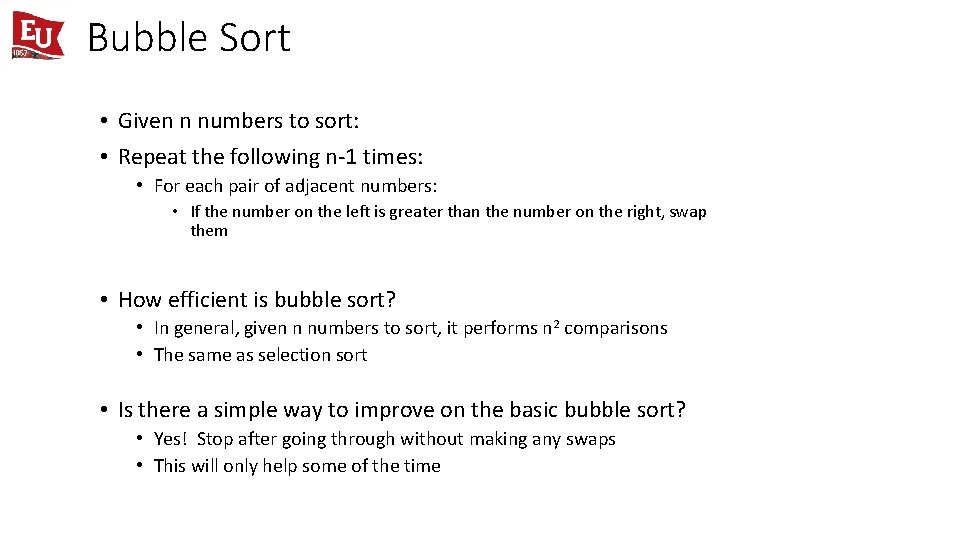
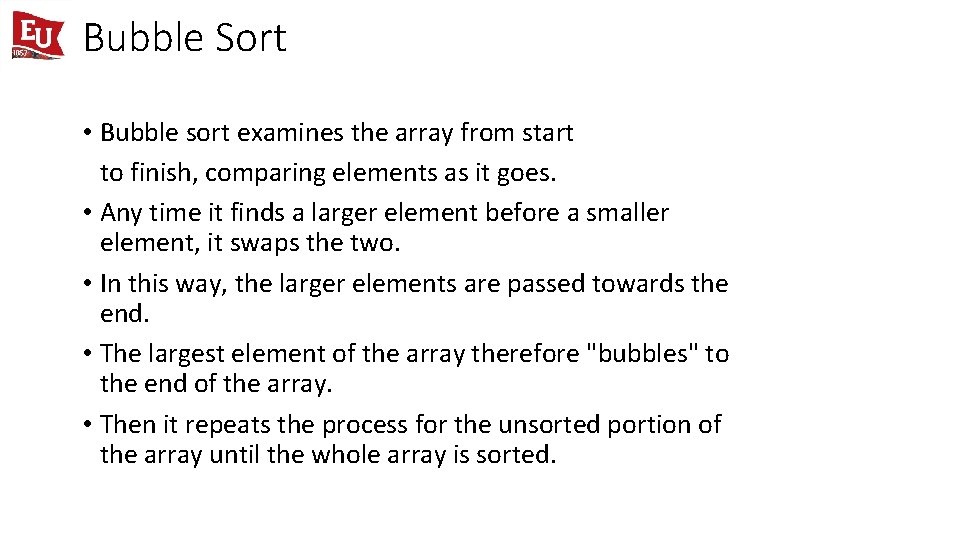
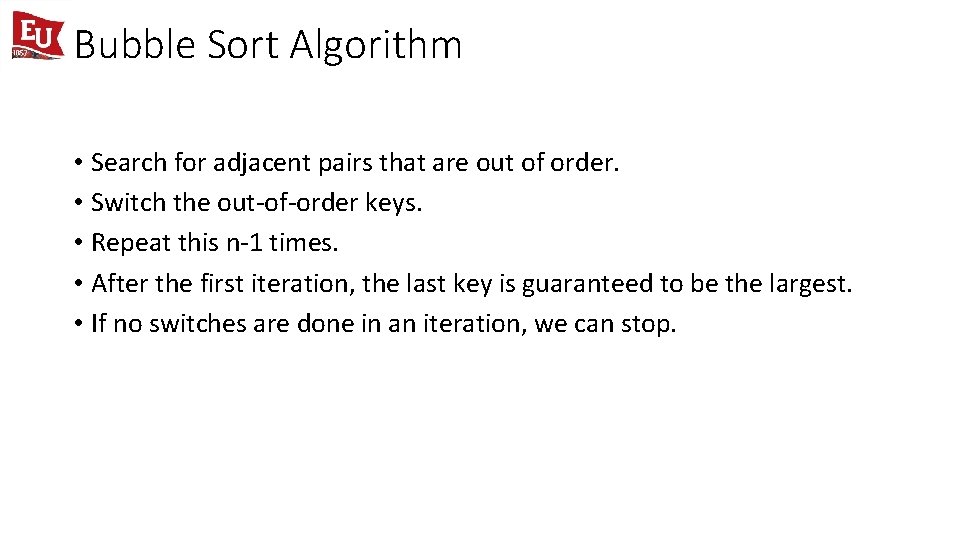
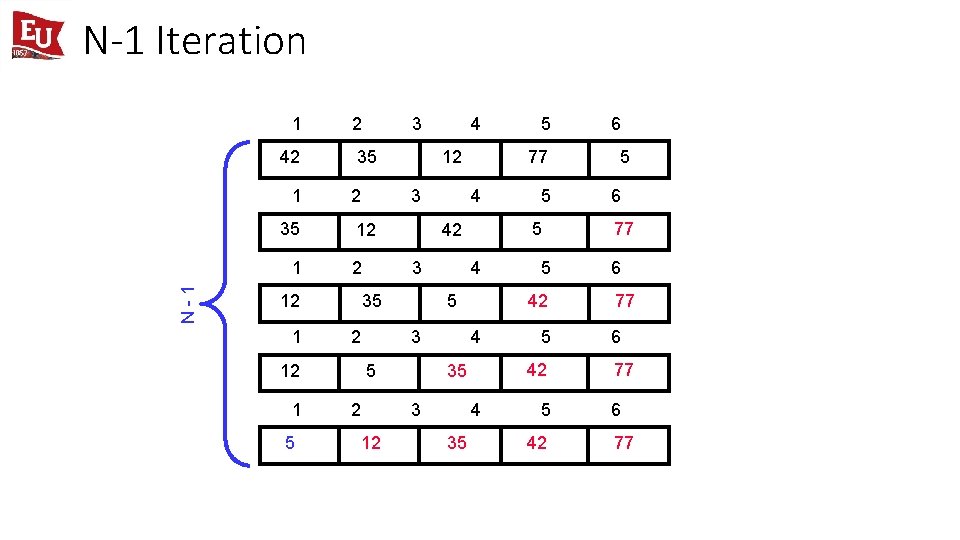
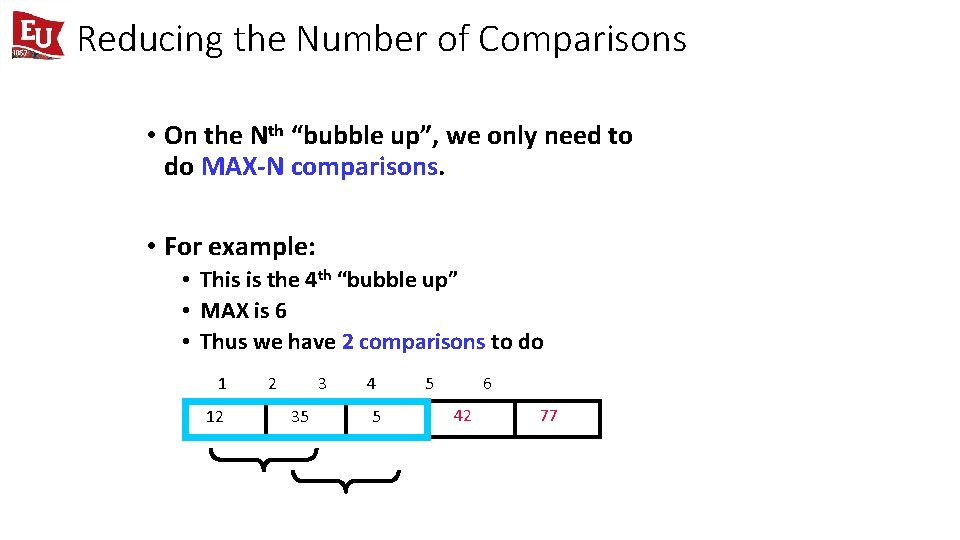
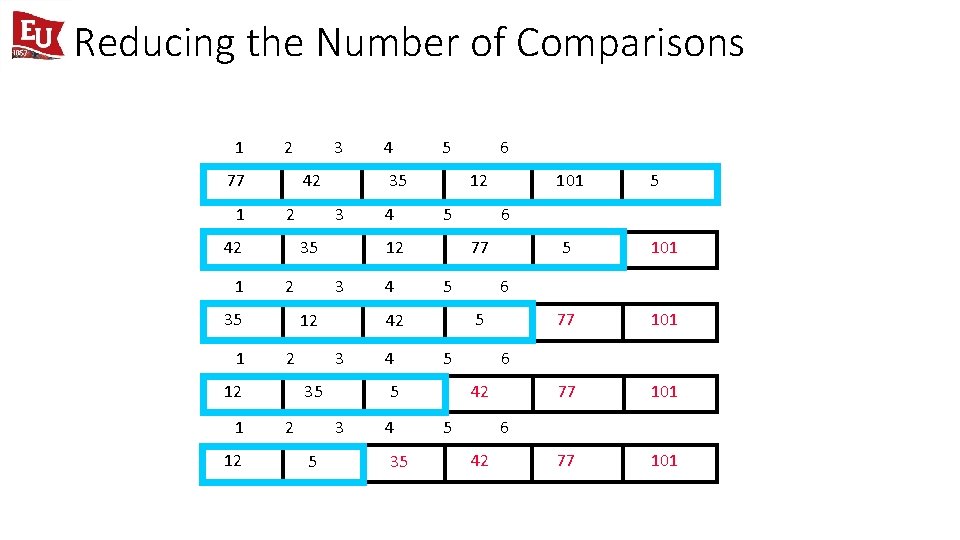
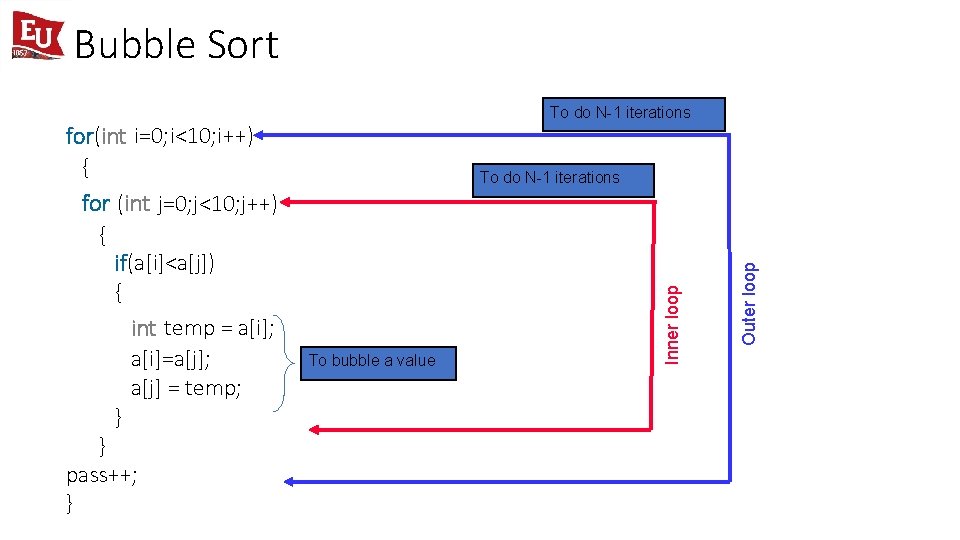
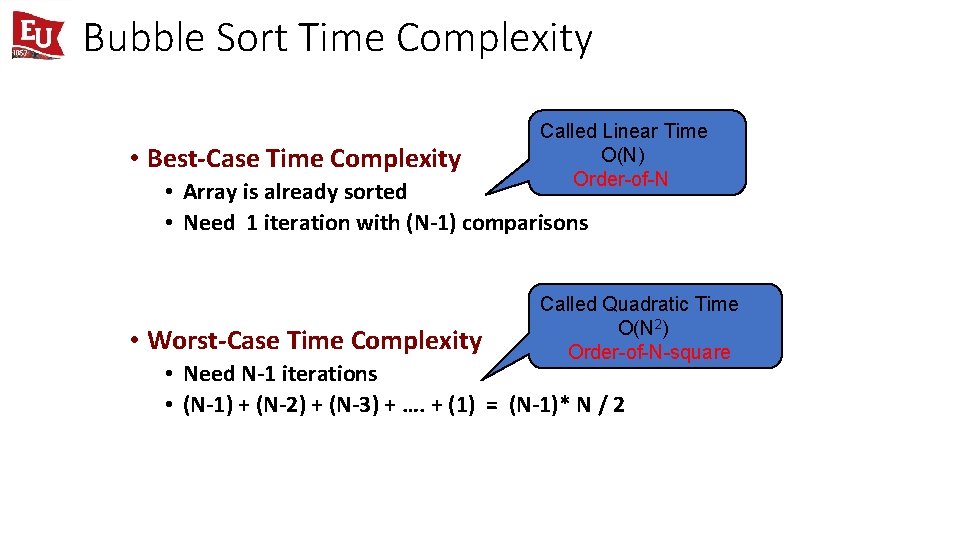
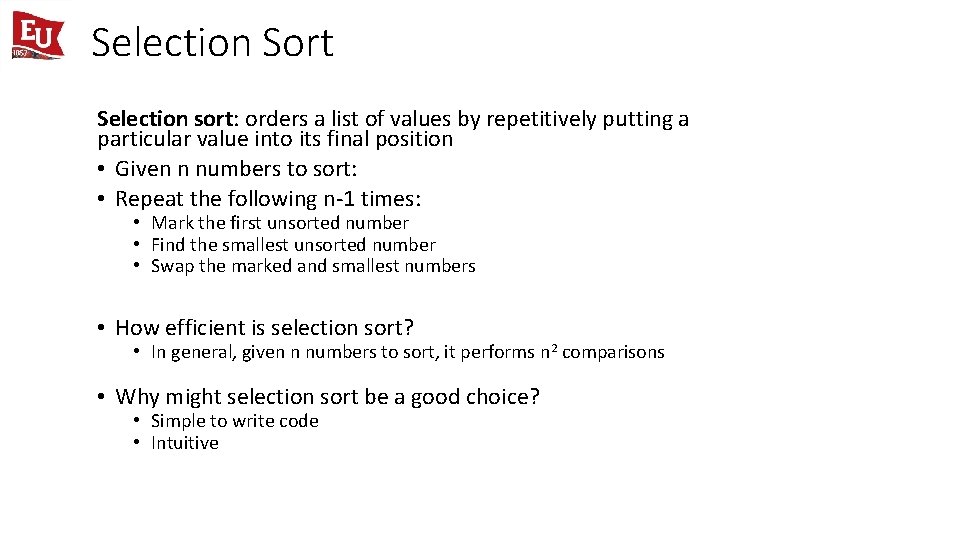
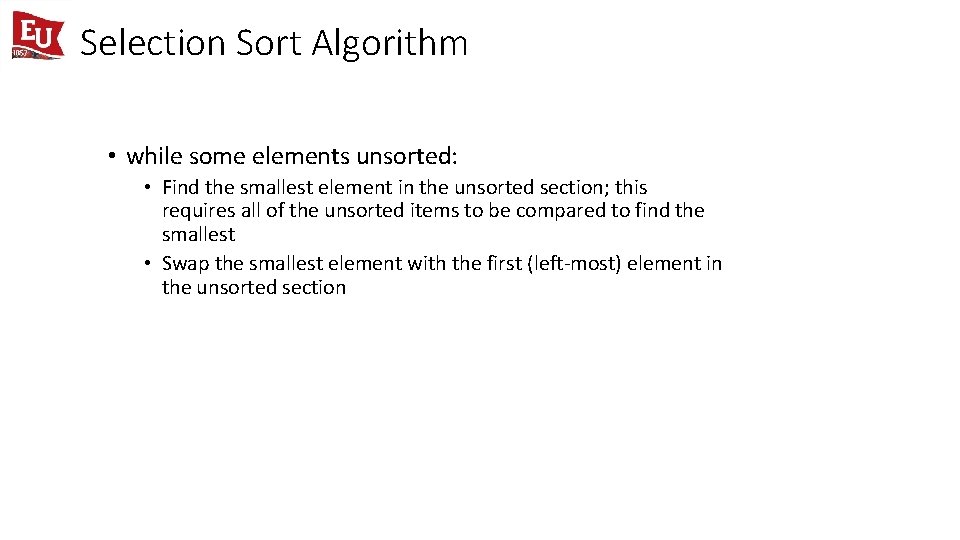
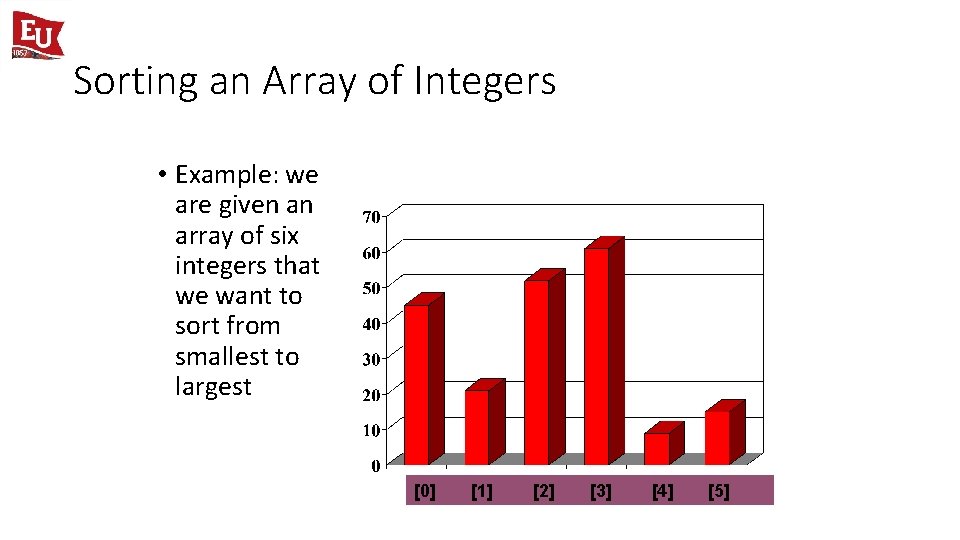
![The Selection Sort Algorithm • Start by finding the smallest entry. [0] [1] [2] The Selection Sort Algorithm • Start by finding the smallest entry. [0] [1] [2]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-22.jpg)
![The Selection Sort Algorithm • Swap the smallest entry with the first entry. [0] The Selection Sort Algorithm • Swap the smallest entry with the first entry. [0]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-23.jpg)
![The Selection Sort Algorithm • Swap the smallest entry with the first entry. [0] The Selection Sort Algorithm • Swap the smallest entry with the first entry. [0]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-24.jpg)
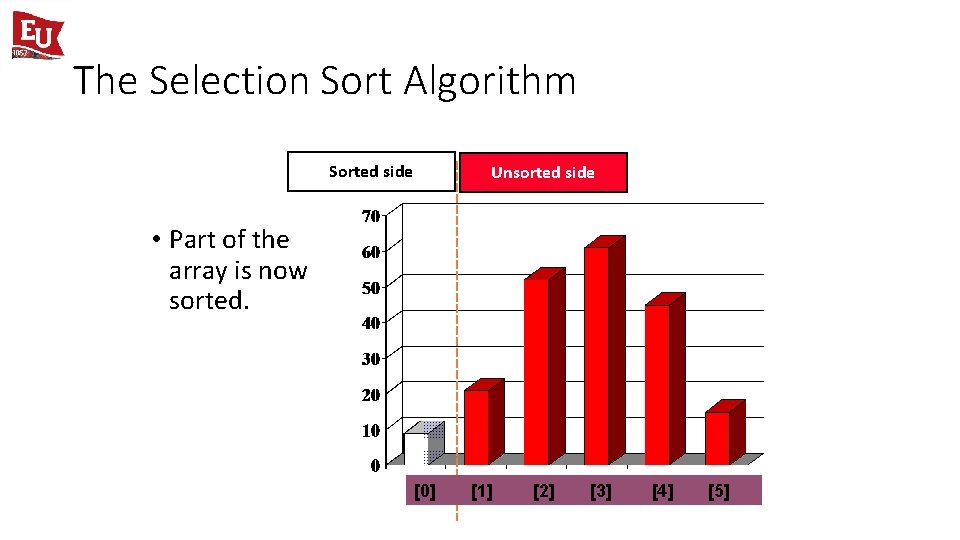
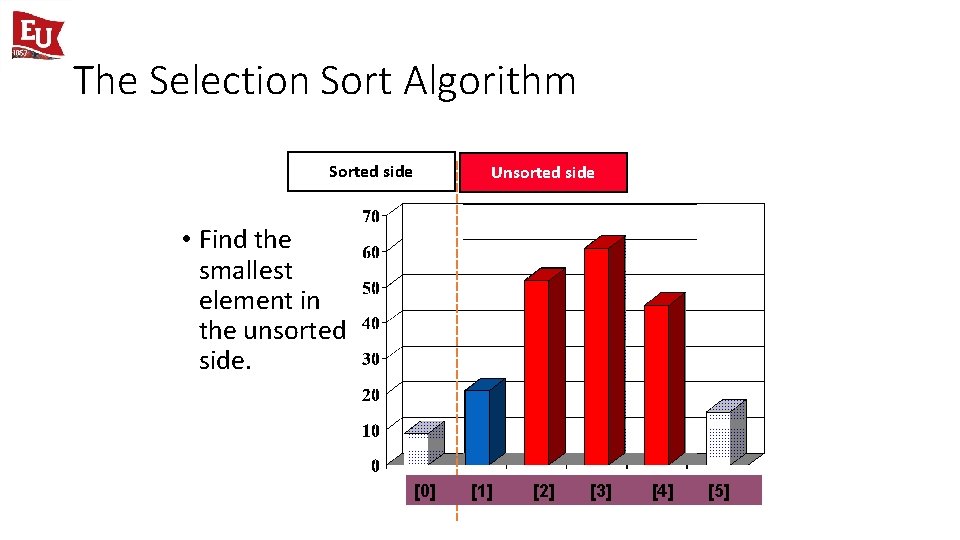
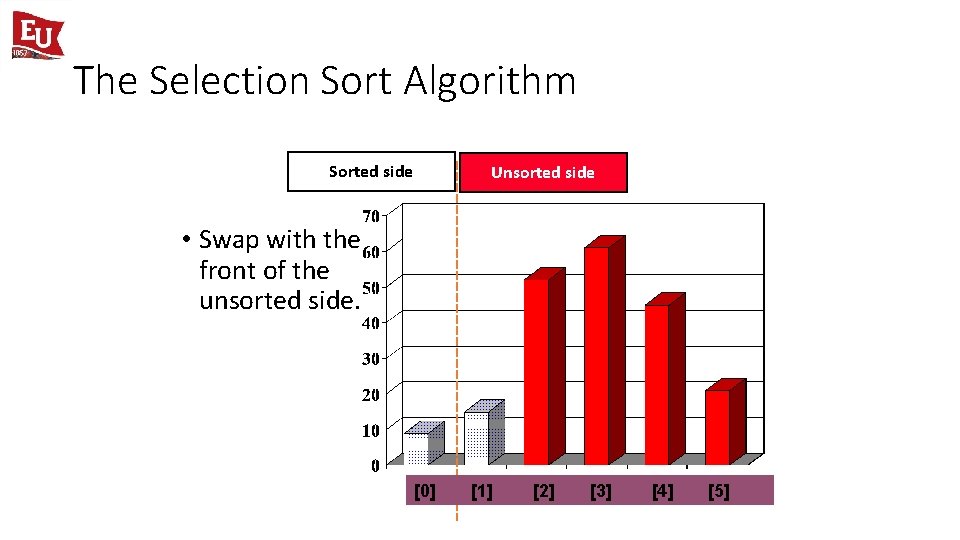
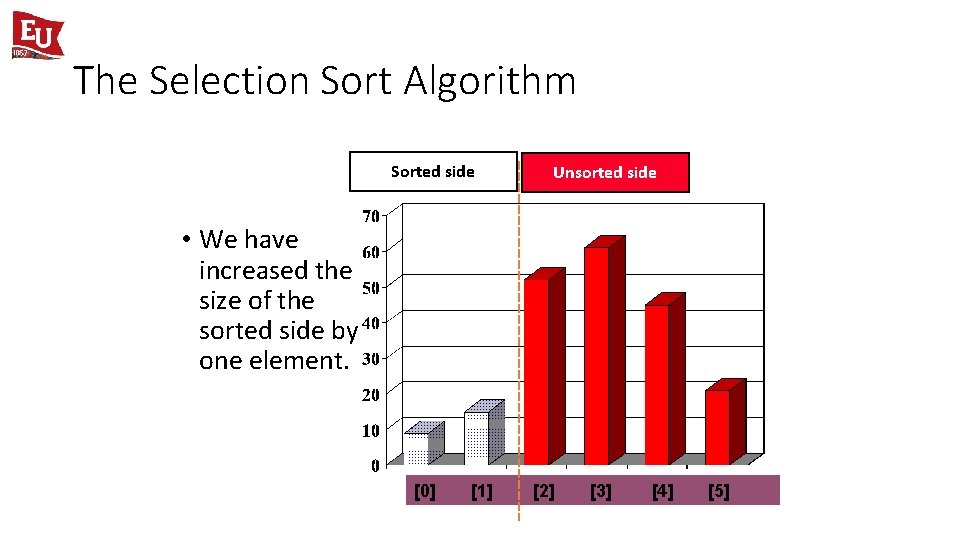
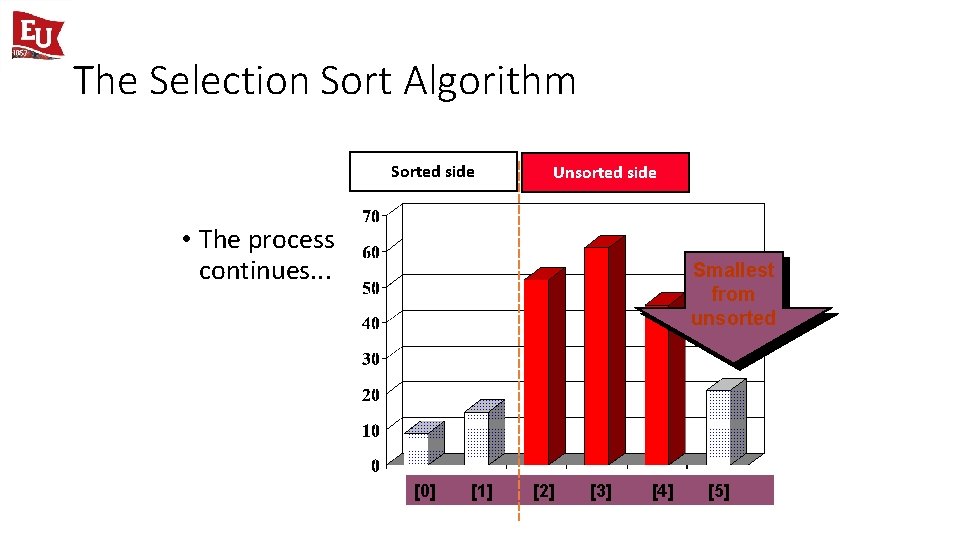
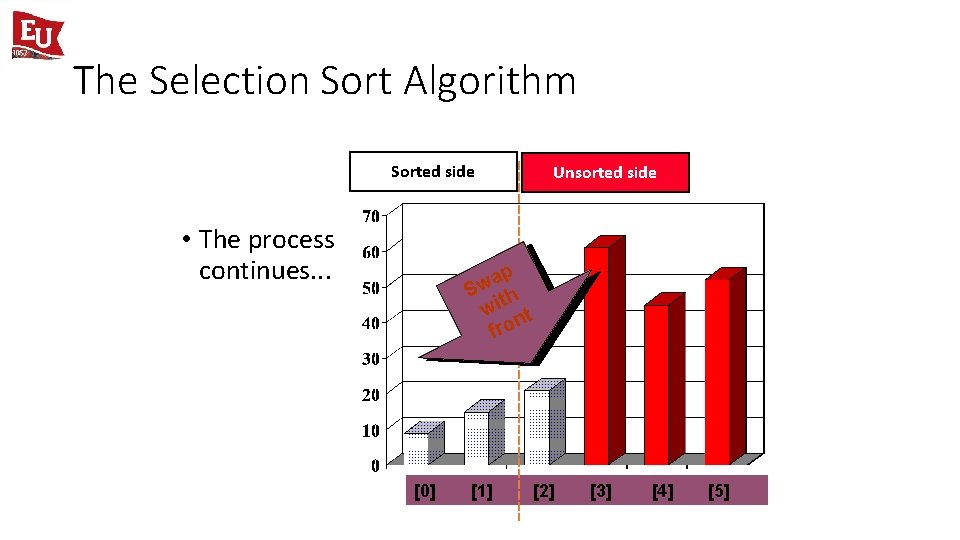
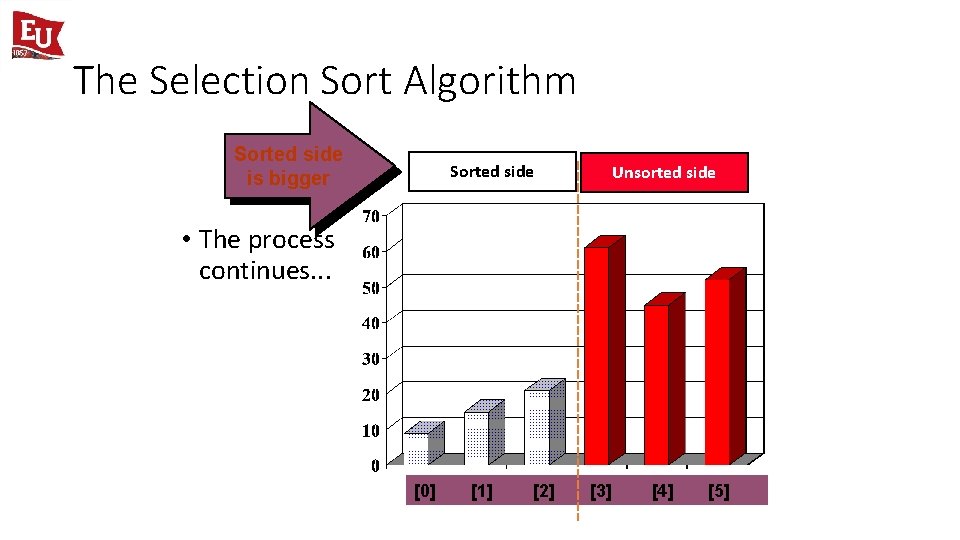
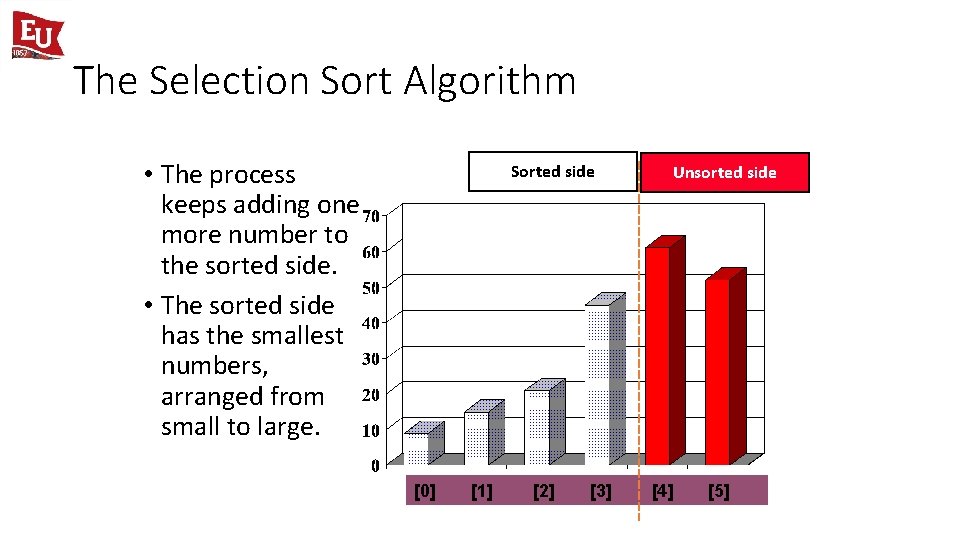
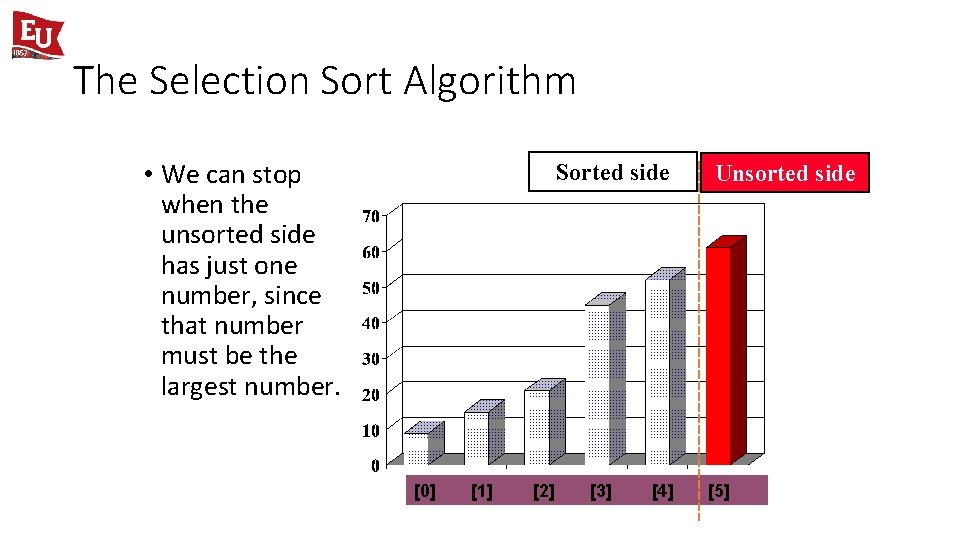
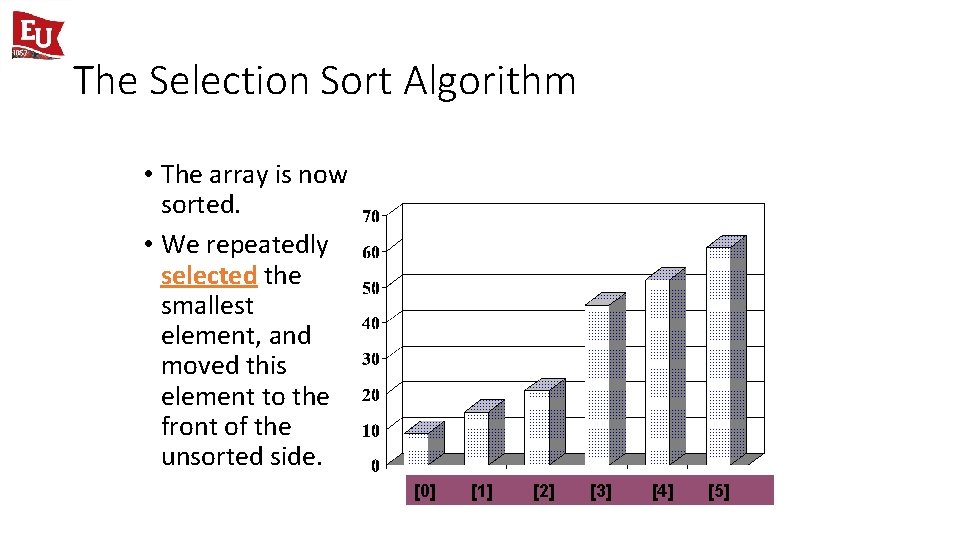
![Selection Sort Algorithm void selection. Sort(int arr[]) { int n = arr. length; int Selection Sort Algorithm void selection. Sort(int arr[]) { int n = arr. length; int](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-35.jpg)
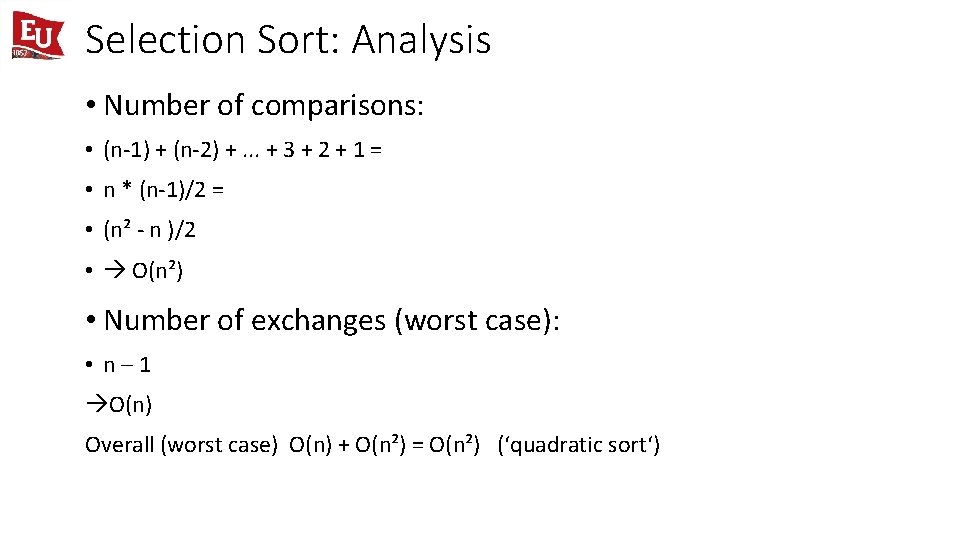
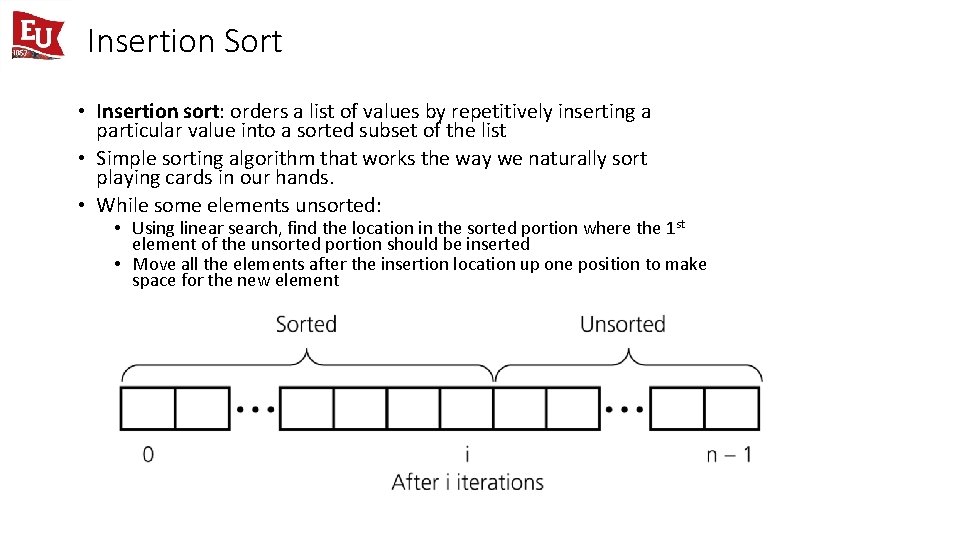
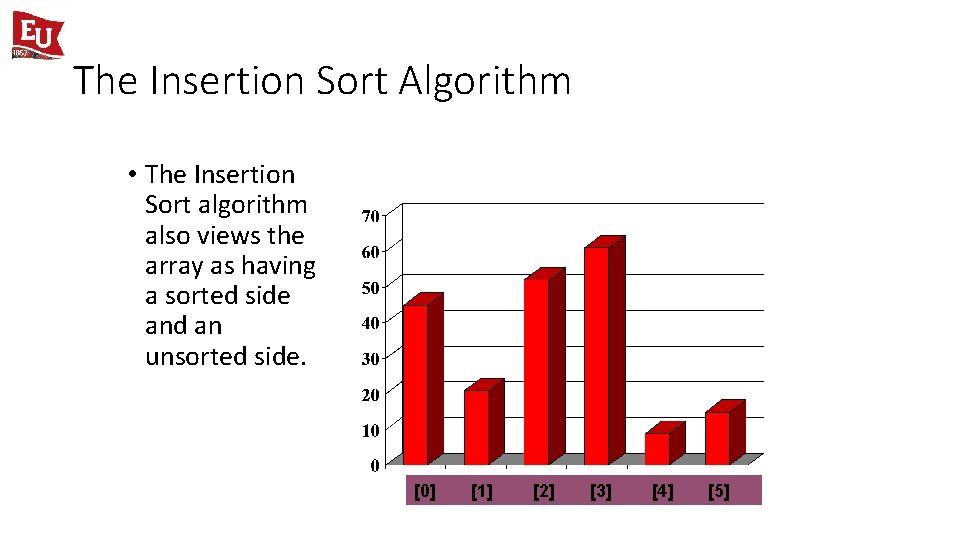
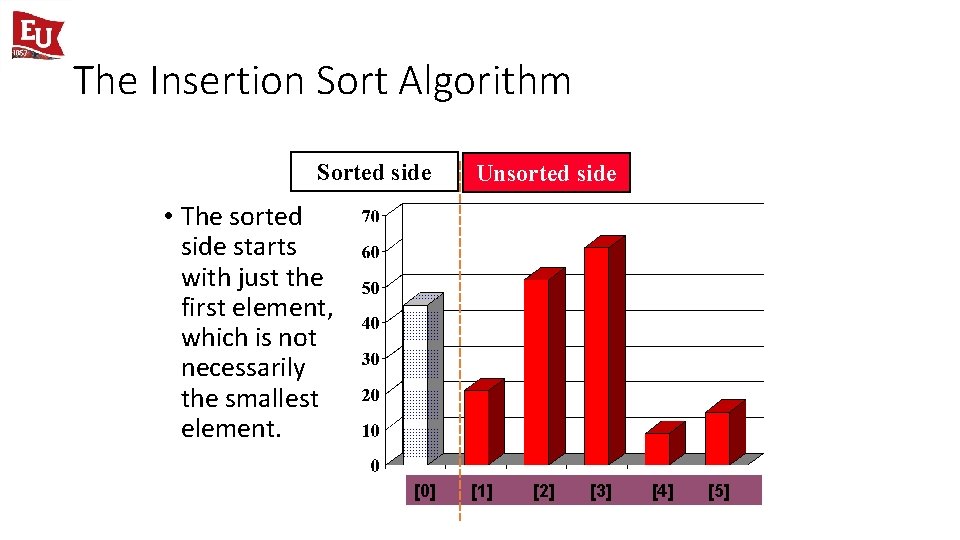
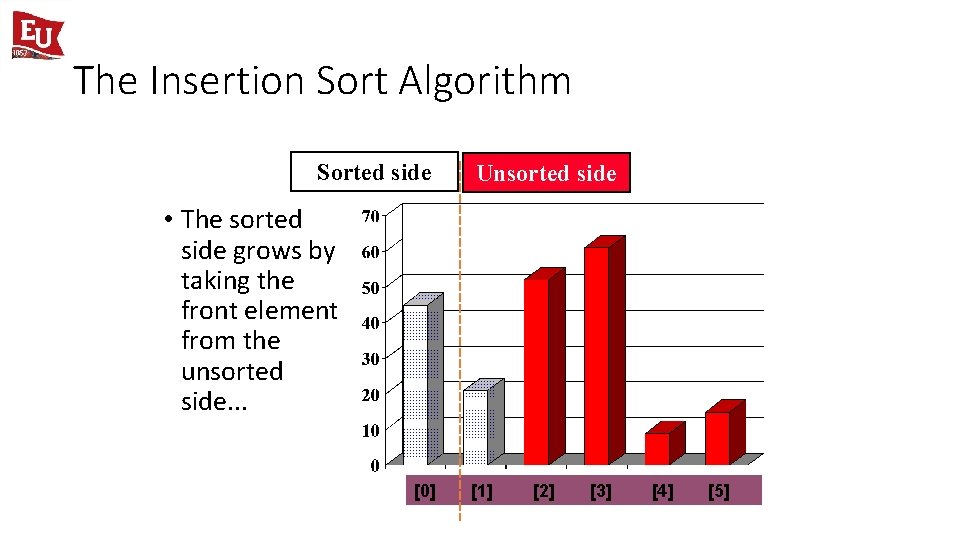
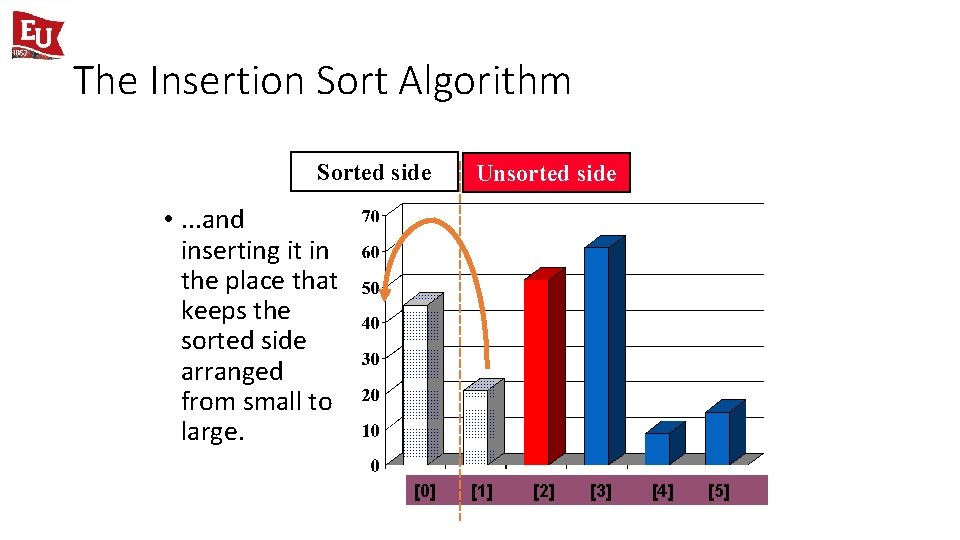
![The Insertion Sort Algorithm Sorted side [0] [1] Unsorted side [2] [3] [4] [5] The Insertion Sort Algorithm Sorted side [0] [1] Unsorted side [2] [3] [4] [5]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-42.jpg)
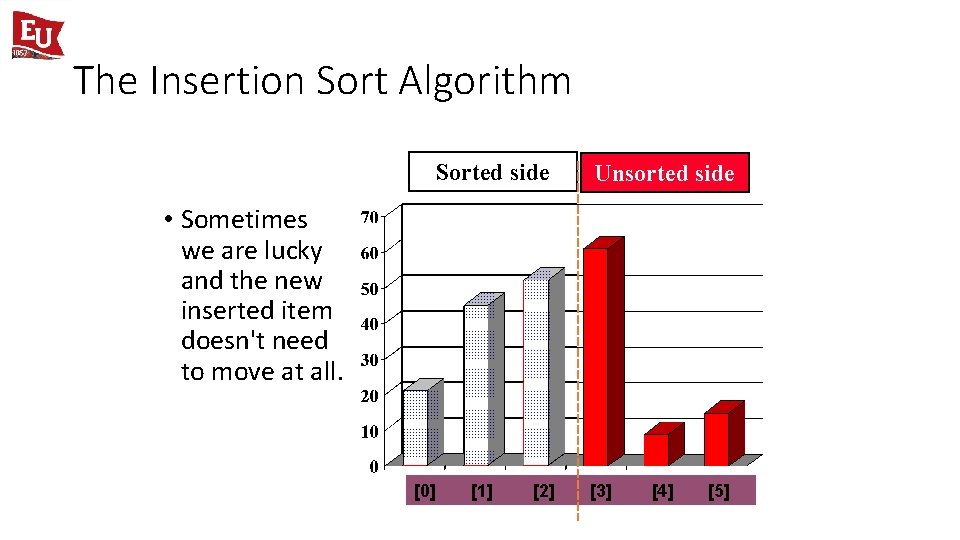
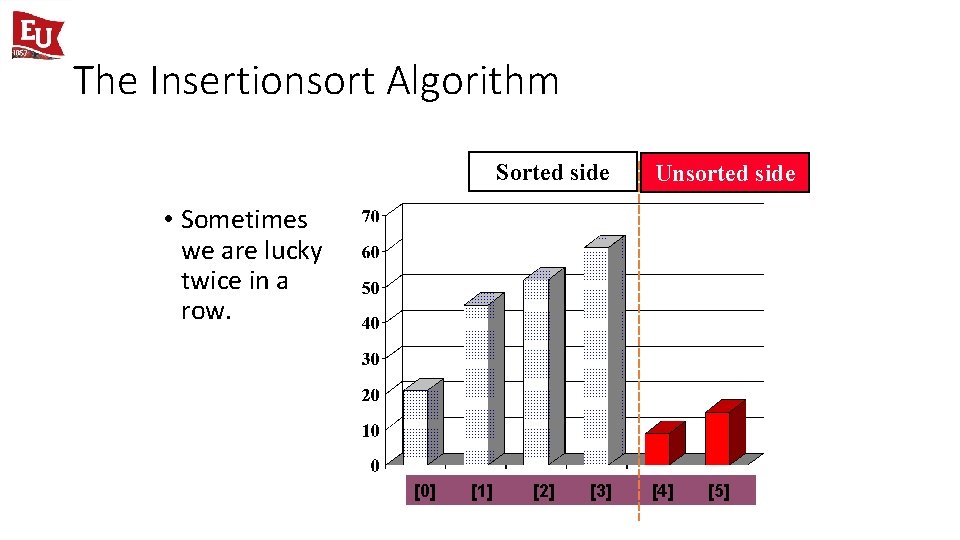
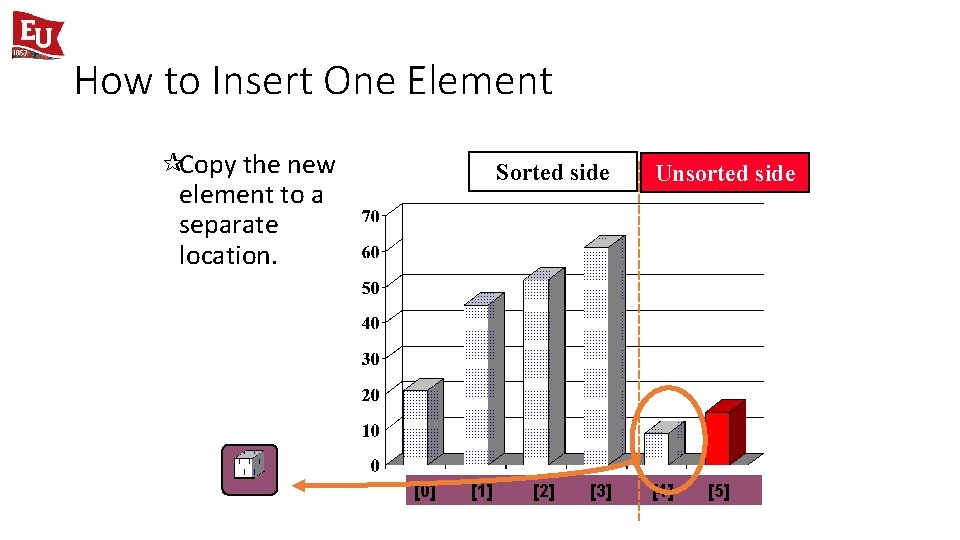
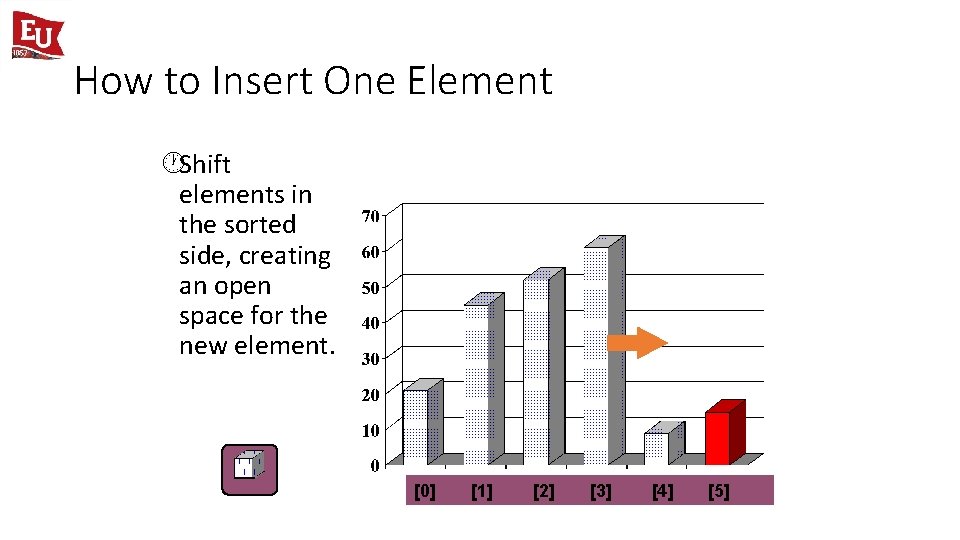
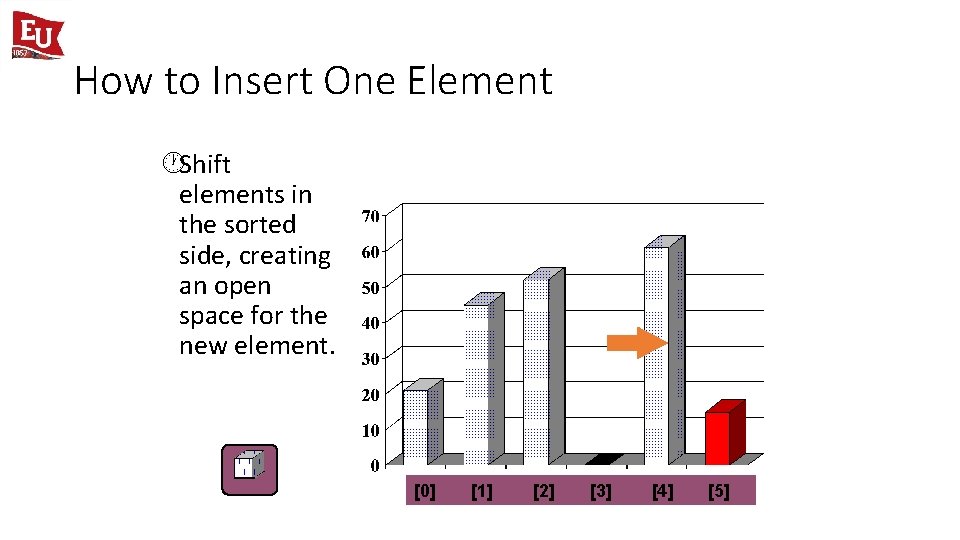
![How to Insert One Element ·Continue shifting elements. . . [0] [1] [2] [3] How to Insert One Element ·Continue shifting elements. . . [0] [1] [2] [3]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-48.jpg)
![How to Insert One Element ·Continue shifting elements. . . [0] [1] [2] [3] How to Insert One Element ·Continue shifting elements. . . [0] [1] [2] [3]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-49.jpg)
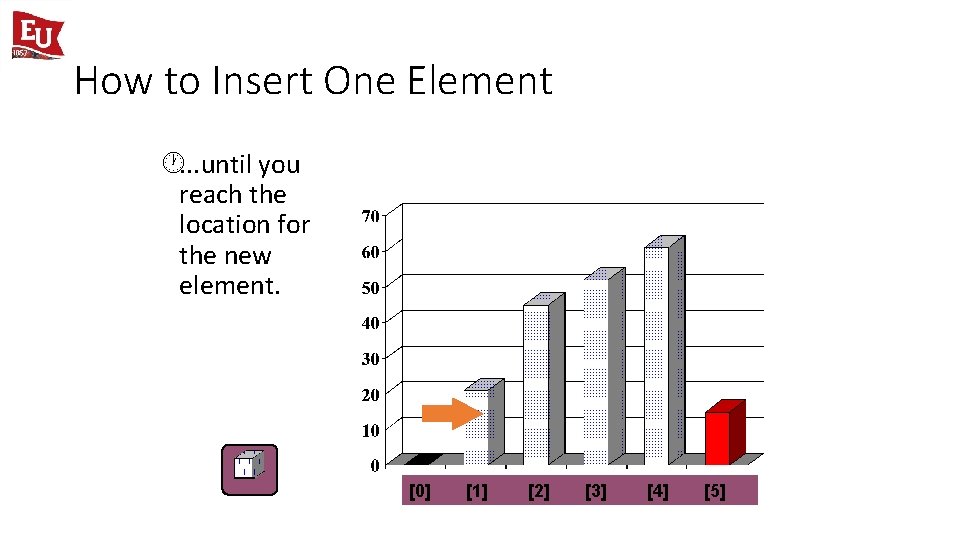
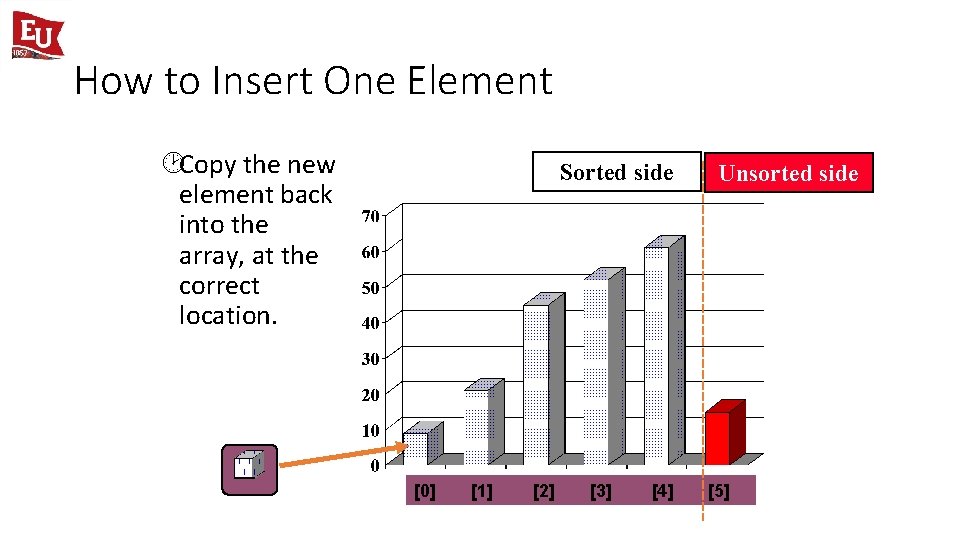
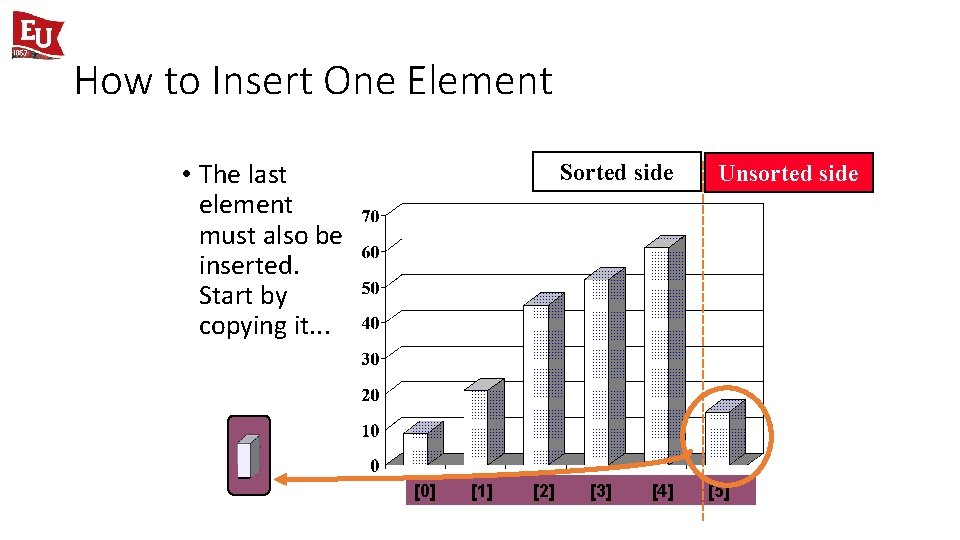
![Sorted Result [0] [1] [2] [3] [4] [5] Sorted Result [0] [1] [2] [3] [4] [5]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-53.jpg)
![Insertion Sort Code void insertion. Sort(int arr[]) { int n = arr. length; for Insertion Sort Code void insertion. Sort(int arr[]) { int n = arr. length; for](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-54.jpg)
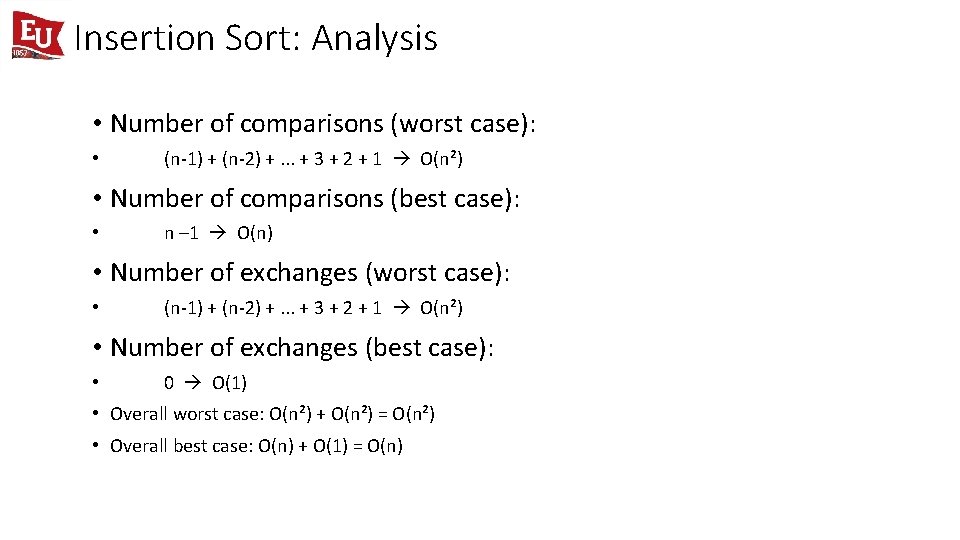
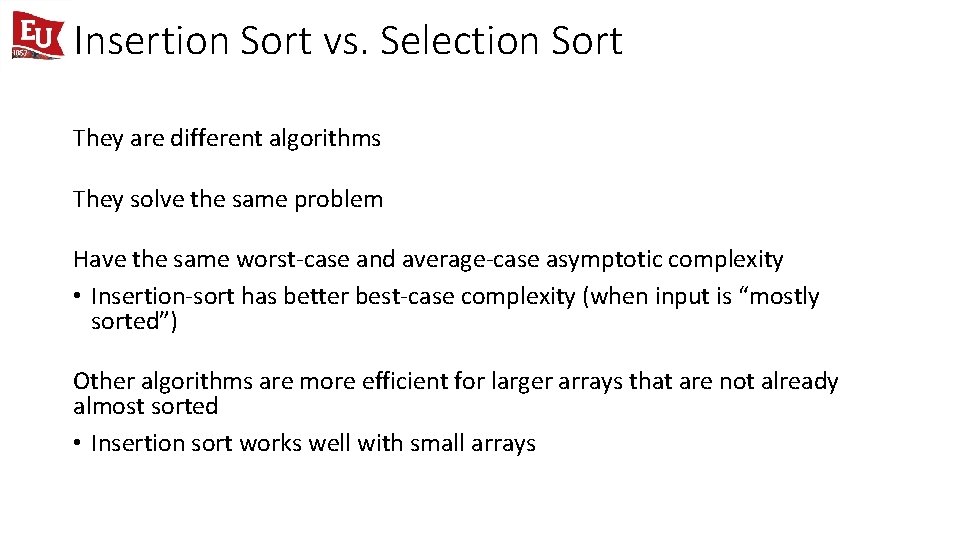
- Slides: 56
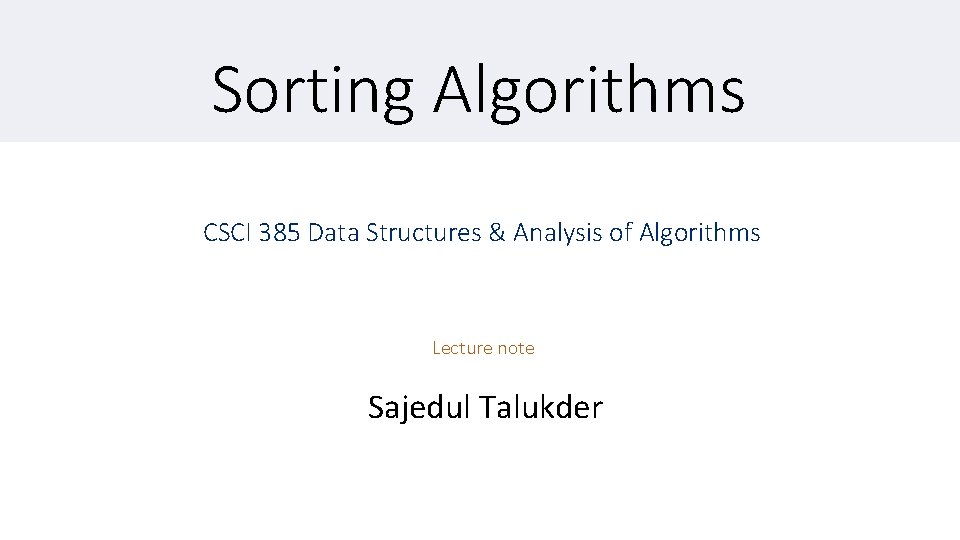
Sorting Algorithms CSCI 385 Data Structures & Analysis of Algorithms Lecture note Sajedul Talukder
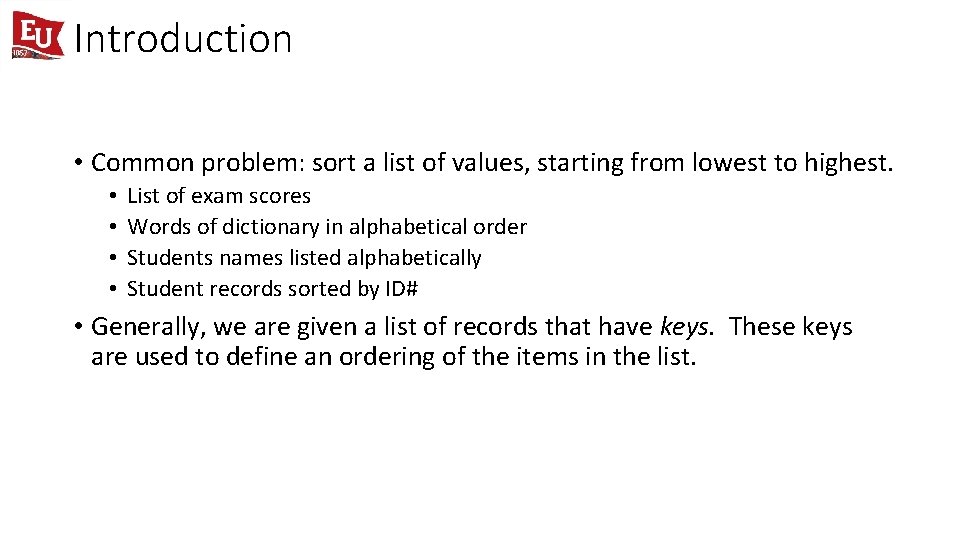
Introduction • Common problem: sort a list of values, starting from lowest to highest. • • List of exam scores Words of dictionary in alphabetical order Students names listed alphabetically Student records sorted by ID# • Generally, we are given a list of records that have keys. These keys are used to define an ordering of the items in the list.
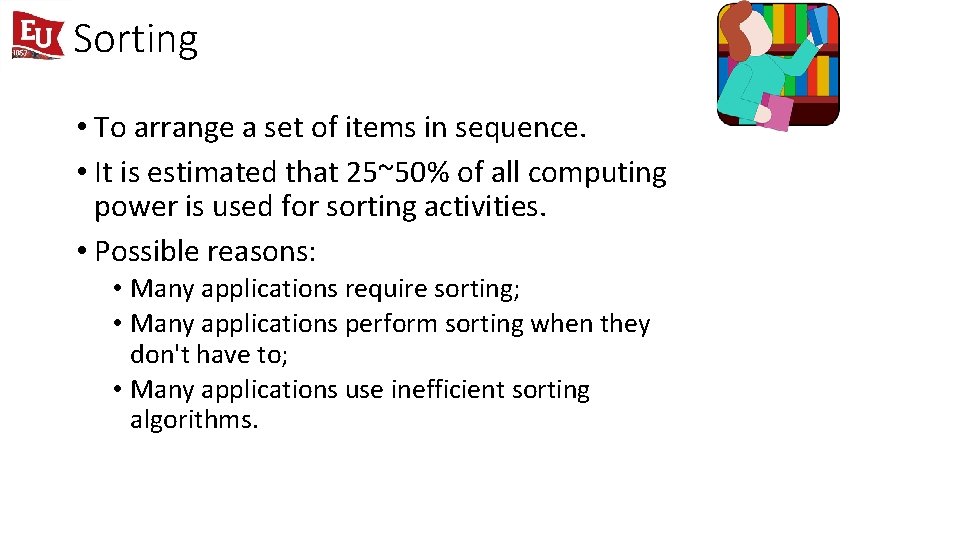
Sorting • To arrange a set of items in sequence. • It is estimated that 25~50% of all computing power is used for sorting activities. • Possible reasons: • Many applications require sorting; • Many applications perform sorting when they don't have to; • Many applications use inefficient sorting algorithms.
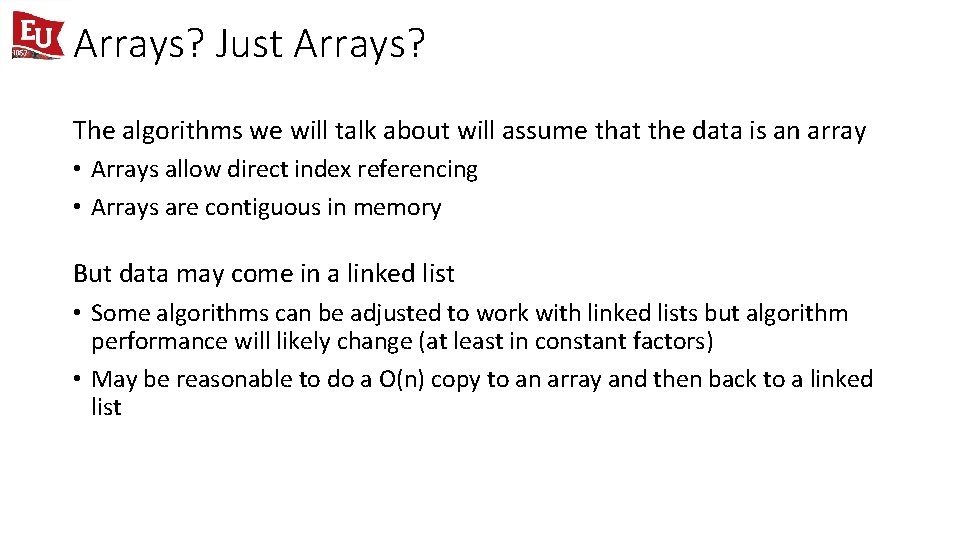
Arrays? Just Arrays? The algorithms we will talk about will assume that the data is an array • Arrays allow direct index referencing • Arrays are contiguous in memory But data may come in a linked list • Some algorithms can be adjusted to work with linked lists but algorithm performance will likely change (at least in constant factors) • May be reasonable to do a O(n) copy to an array and then back to a linked list
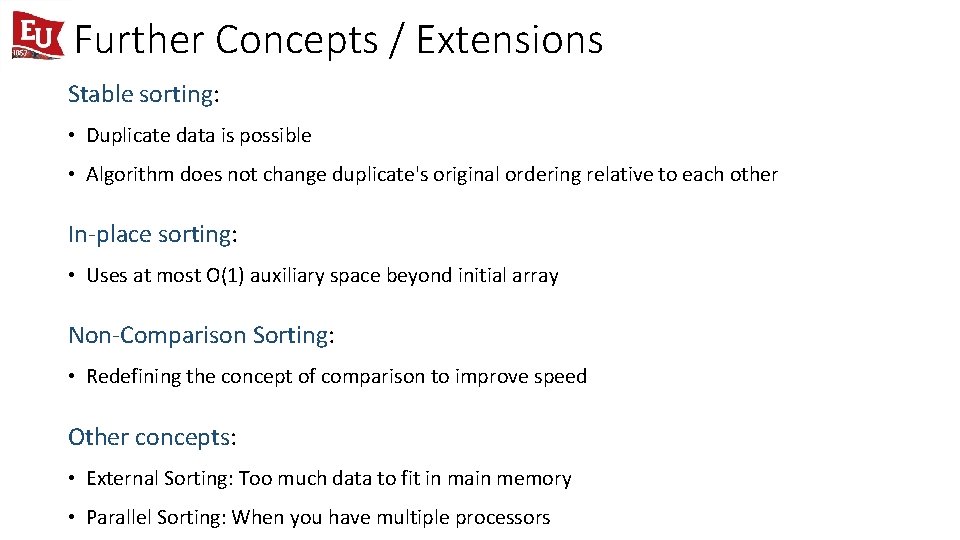
Further Concepts / Extensions Stable sorting: • Duplicate data is possible • Algorithm does not change duplicate's original ordering relative to each other In-place sorting: • Uses at most O(1) auxiliary space beyond initial array Non-Comparison Sorting: • Redefining the concept of comparison to improve speed Other concepts: • External Sorting: Too much data to fit in main memory • Parallel Sorting: When you have multiple processors
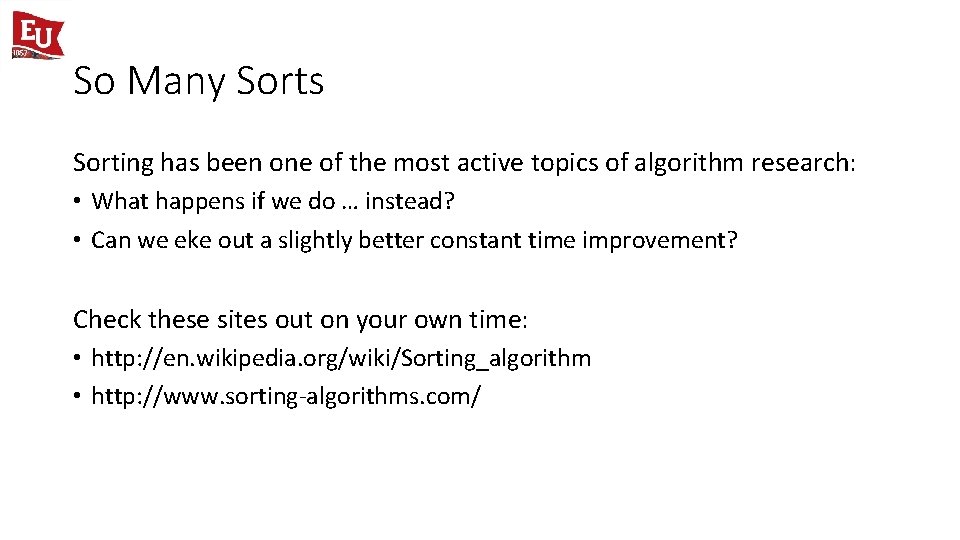
So Many Sorts Sorting has been one of the most active topics of algorithm research: • What happens if we do … instead? • Can we eke out a slightly better constant time improvement? Check these sites out on your own time: • http: //en. wikipedia. org/wiki/Sorting_algorithm • http: //www. sorting-algorithms. com/
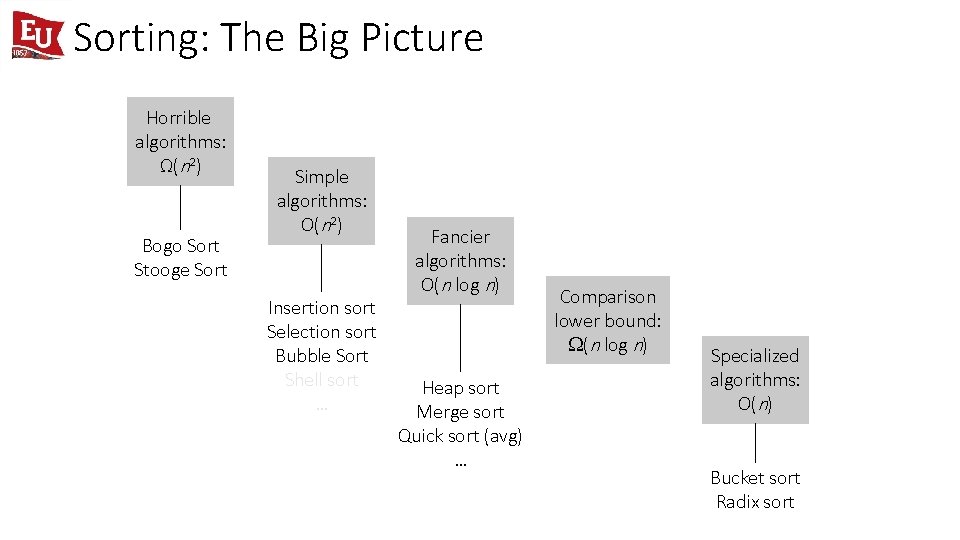
Sorting: The Big Picture Horrible algorithms: Ω(n 2) Bogo Sort Stooge Sort Simple algorithms: O(n 2) Insertion sort Selection sort Bubble Sort Shell sort … Fancier algorithms: O(n log n) Heap sort Merge sort Quick sort (avg) … Comparison lower bound: (n log n) Specialized algorithms: O(n) Bucket sort Radix sort
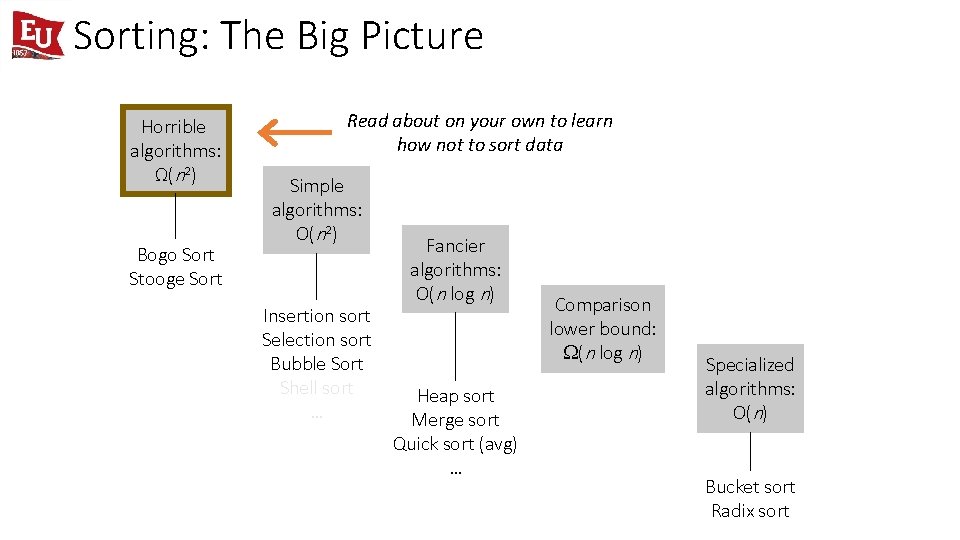
Sorting: The Big Picture Horrible algorithms: Ω(n 2) Bogo Sort Stooge Sort Read about on your own to learn how not to sort data Simple algorithms: O(n 2) Insertion sort Selection sort Bubble Sort Shell sort … Fancier algorithms: O(n log n) Heap sort Merge sort Quick sort (avg) … Comparison lower bound: (n log n) Specialized algorithms: O(n) Bucket sort Radix sort
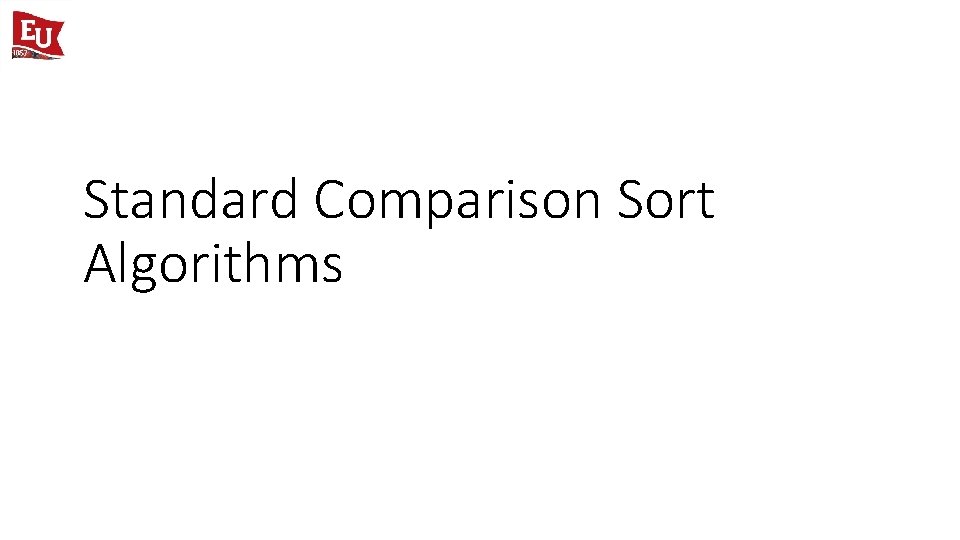
Standard Comparison Sort Algorithms
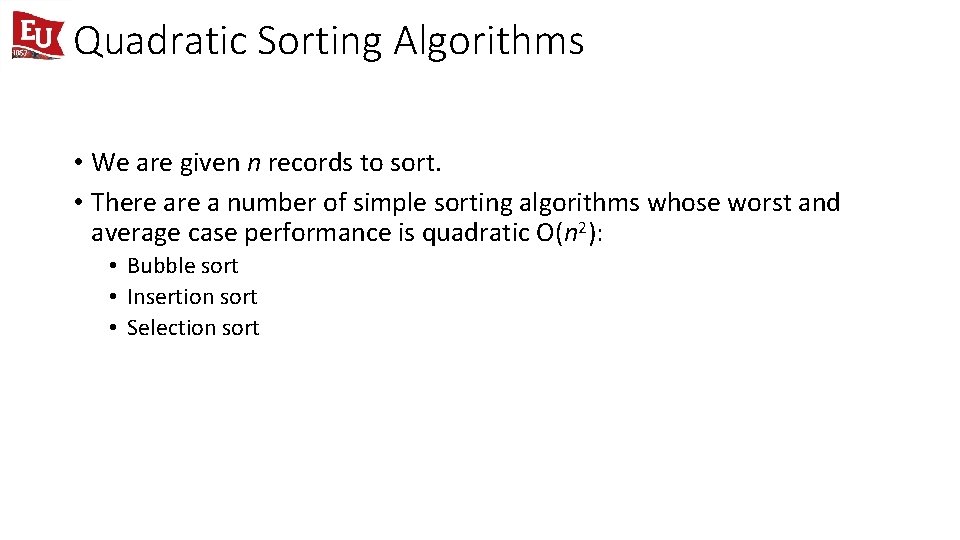
Quadratic Sorting Algorithms • We are given n records to sort. • There a number of simple sorting algorithms whose worst and average case performance is quadratic O(n 2): • Bubble sort • Insertion sort • Selection sort
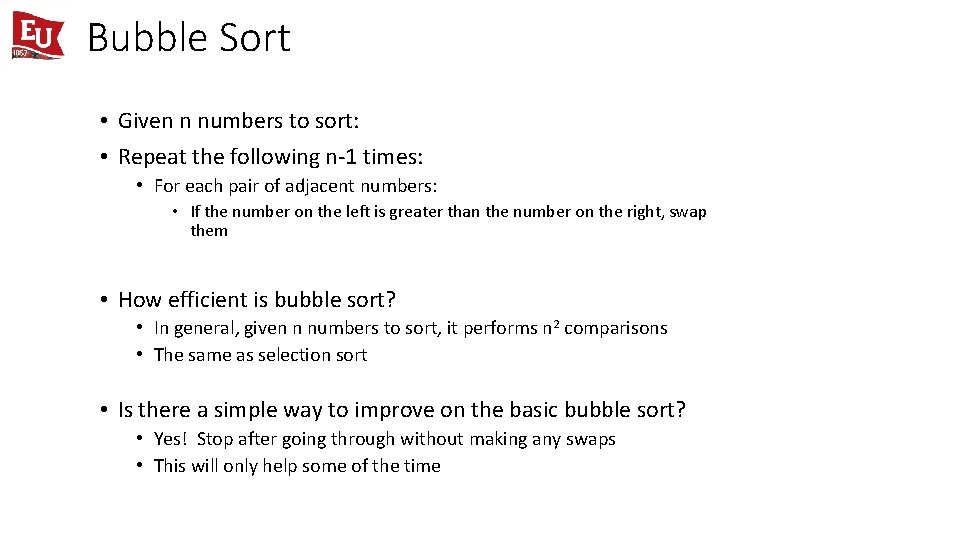
Bubble Sort • Given n numbers to sort: • Repeat the following n-1 times: • For each pair of adjacent numbers: • If the number on the left is greater than the number on the right, swap them • How efficient is bubble sort? • In general, given n numbers to sort, it performs n 2 comparisons • The same as selection sort • Is there a simple way to improve on the basic bubble sort? • Yes! Stop after going through without making any swaps • This will only help some of the time
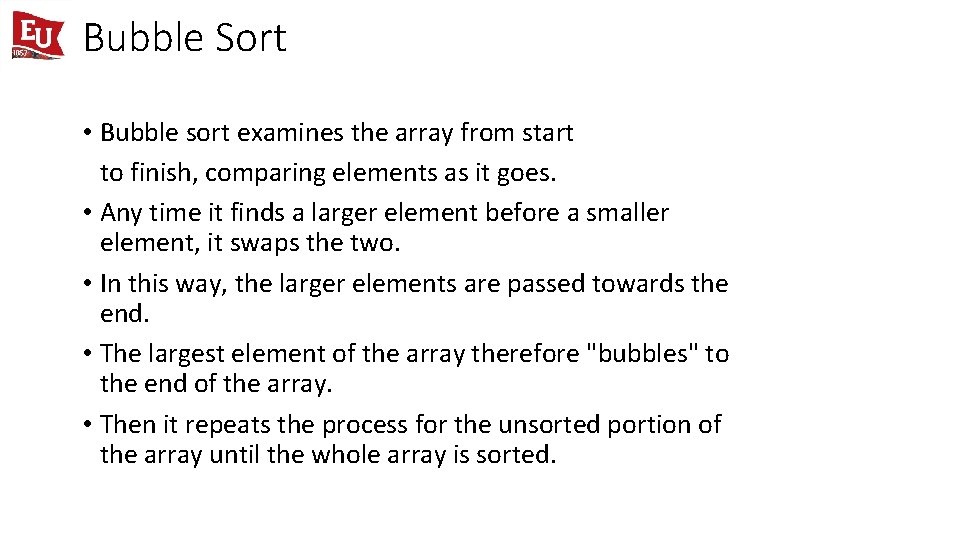
Bubble Sort • Bubble sort examines the array from start to finish, comparing elements as it goes. • Any time it finds a larger element before a smaller element, it swaps the two. • In this way, the larger elements are passed towards the end. • The largest element of the array therefore "bubbles" to the end of the array. • Then it repeats the process for the unsorted portion of the array until the whole array is sorted.
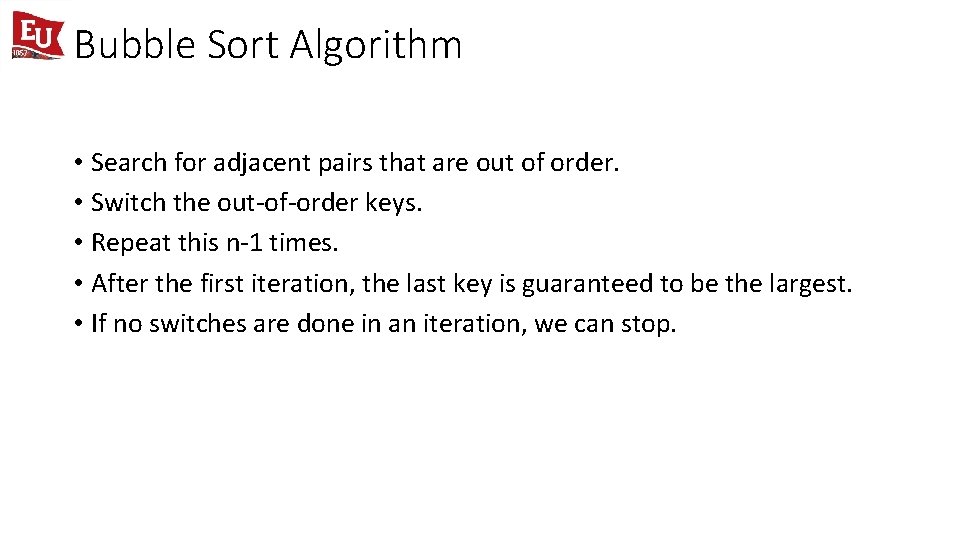
Bubble Sort Algorithm • Search for adjacent pairs that are out of order. • Switch the out-of-order keys. • Repeat this n-1 times. • After the first iteration, the last key is guaranteed to be the largest. • If no switches are done in an iteration, we can stop.
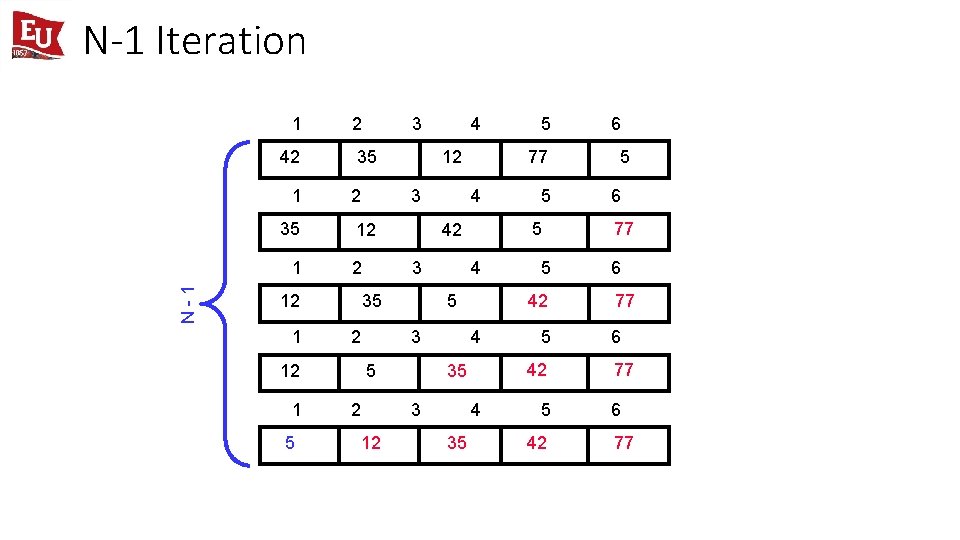
N-1 Iteration 1 42 1 35 N-1 1 2 35 2 1 5 3 2 4 3 2 2 12 4 4 35 5 42 35 3 5 42 5 5 4 3 5 77 42 35 12 4 12 12 12 1 3 5 42 6 5 6 77
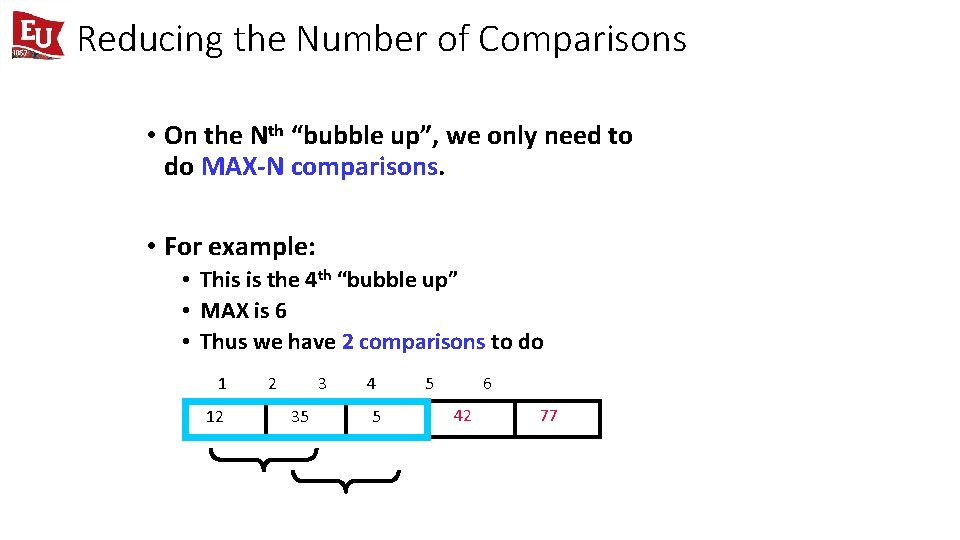
Reducing the Number of Comparisons • On the Nth “bubble up”, we only need to do MAX-N comparisons. • For example: • This is the 4 th “bubble up” • MAX is 6 • Thus we have 2 comparisons to do 1 12 2 3 35 4 5 5 6 42 77
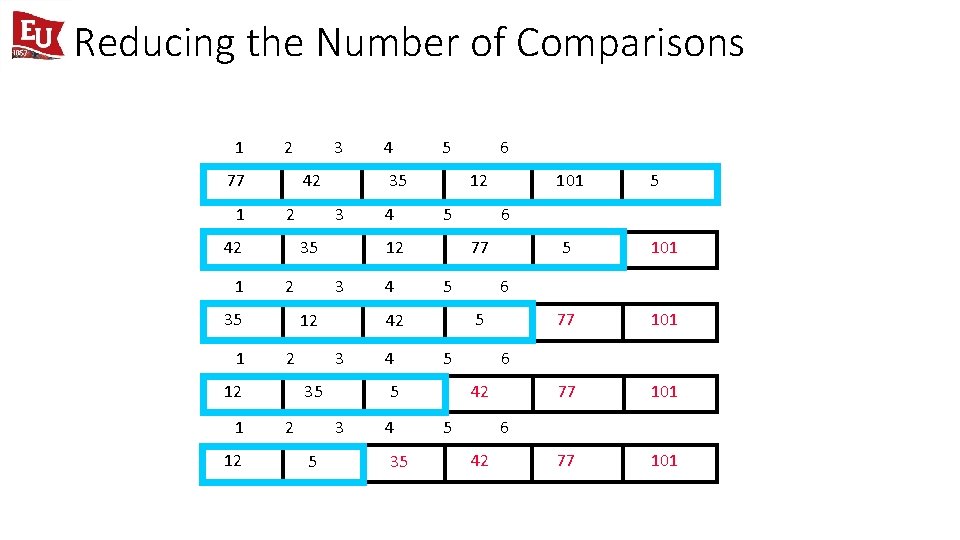
Reducing the Number of Comparisons 1 2 77 1 42 2 42 1 12 3 2 35 5 4 4 4 35 101 5 5 101 77 101 6 77 5 6 5 5 6 42 5 3 5 4 6 12 42 3 2 5 12 12 12 1 3 2 4 35 35 35 1 3 5 6 42
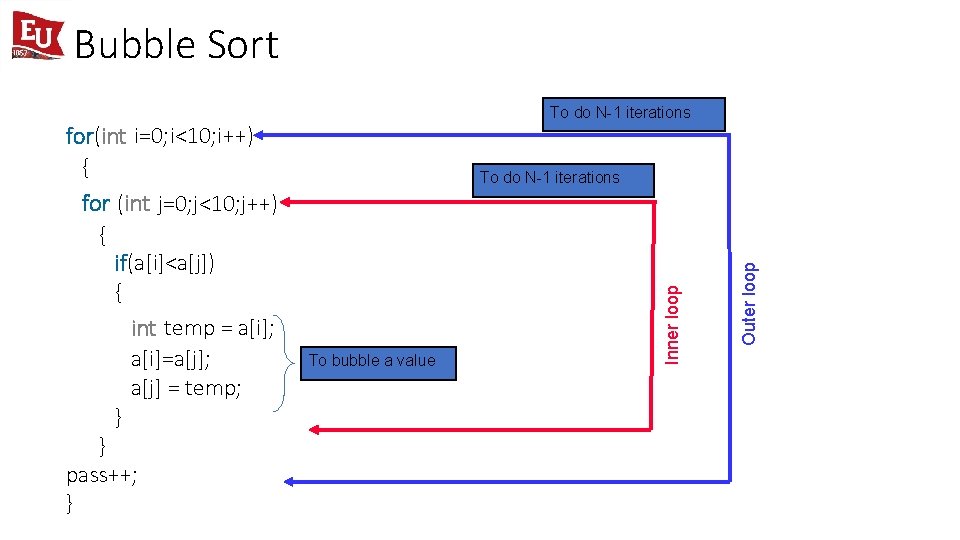
Bubble Sort To do N-1 iterations for(int i=0; i<10; i++) { int temp = a[i]; a[i]=a[j]; a[j] = temp; } } pass++; } To bubble a value Outer loop for (int j=0; j<10; j++) { if(a[i]<a[j]) { Inner loop To do N-1 iterations
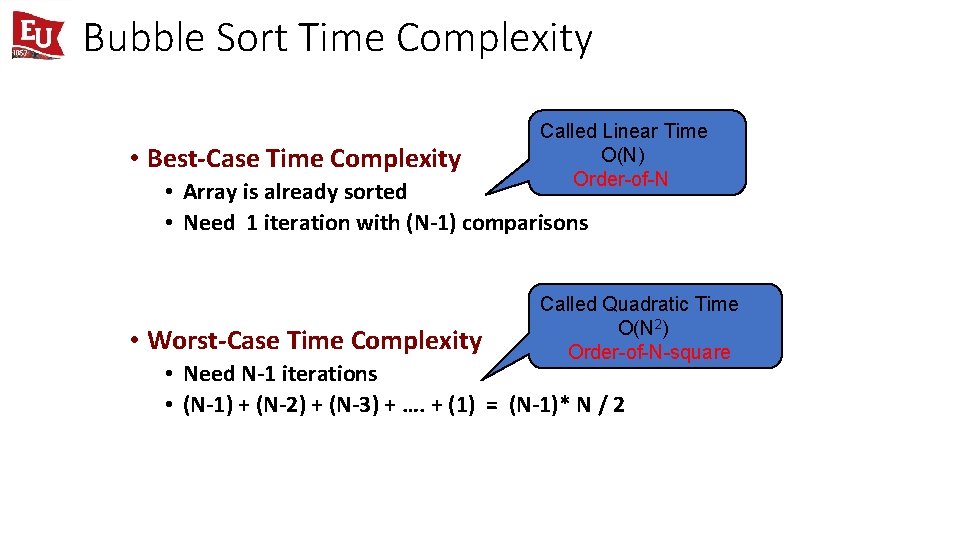
Bubble Sort Time Complexity • Best-Case Time Complexity Called Linear Time O(N) Order-of-N • Array is already sorted • Need 1 iteration with (N-1) comparisons • Worst-Case Time Complexity Called Quadratic Time O(N 2) Order-of-N-square • Need N-1 iterations • (N-1) + (N-2) + (N-3) + …. + (1) = (N-1)* N / 2
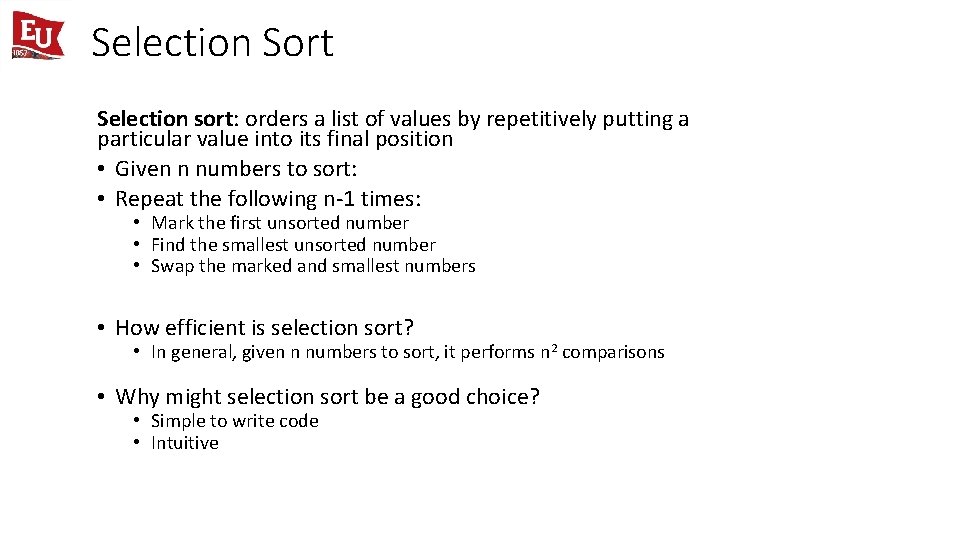
Selection Sort Selection sort: orders a list of values by repetitively putting a particular value into its final position • Given n numbers to sort: • Repeat the following n-1 times: • Mark the first unsorted number • Find the smallest unsorted number • Swap the marked and smallest numbers • How efficient is selection sort? • In general, given n numbers to sort, it performs n 2 comparisons • Why might selection sort be a good choice? • Simple to write code • Intuitive
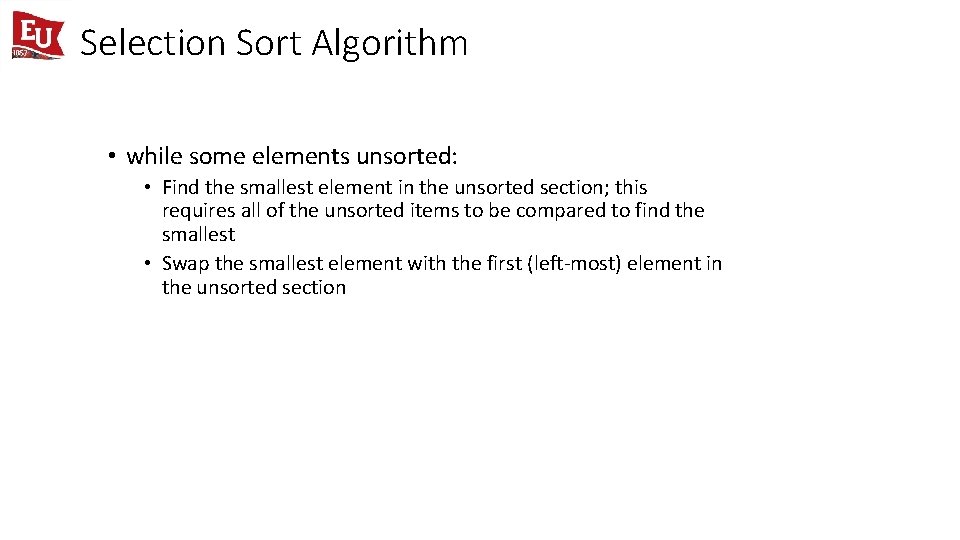
Selection Sort Algorithm • while some elements unsorted: • Find the smallest element in the unsorted section; this requires all of the unsorted items to be compared to find the smallest • Swap the smallest element with the first (left-most) element in the unsorted section
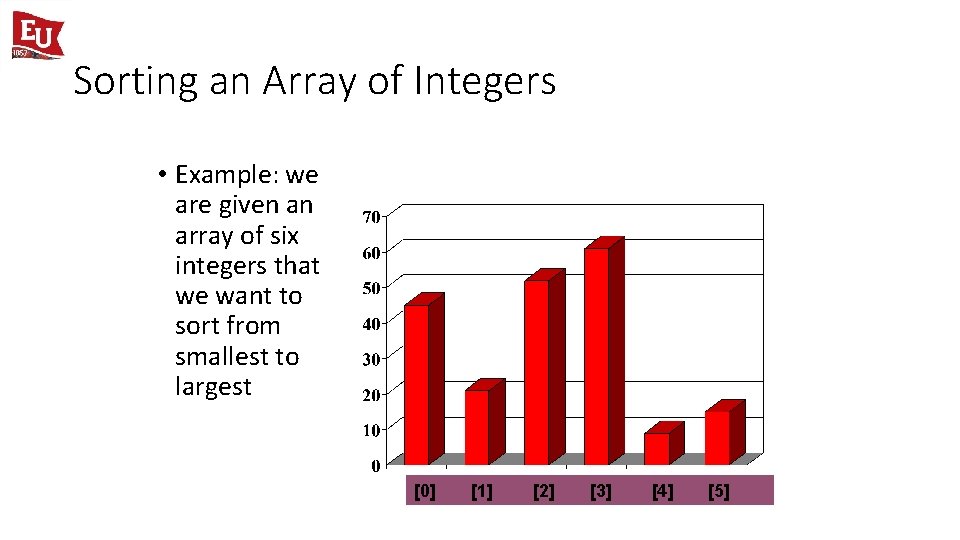
Sorting an Array of Integers • Example: we are given an array of six integers that we want to sort from smallest to largest [0] [1] [2] [3] [4] [5]
![The Selection Sort Algorithm Start by finding the smallest entry 0 1 2 The Selection Sort Algorithm • Start by finding the smallest entry. [0] [1] [2]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-22.jpg)
The Selection Sort Algorithm • Start by finding the smallest entry. [0] [1] [2] [3] [4] [5]
![The Selection Sort Algorithm Swap the smallest entry with the first entry 0 The Selection Sort Algorithm • Swap the smallest entry with the first entry. [0]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-23.jpg)
The Selection Sort Algorithm • Swap the smallest entry with the first entry. [0] [1] [2] [3] [4] [5]
![The Selection Sort Algorithm Swap the smallest entry with the first entry 0 The Selection Sort Algorithm • Swap the smallest entry with the first entry. [0]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-24.jpg)
The Selection Sort Algorithm • Swap the smallest entry with the first entry. [0] [1] [2] [3] [4] [5]
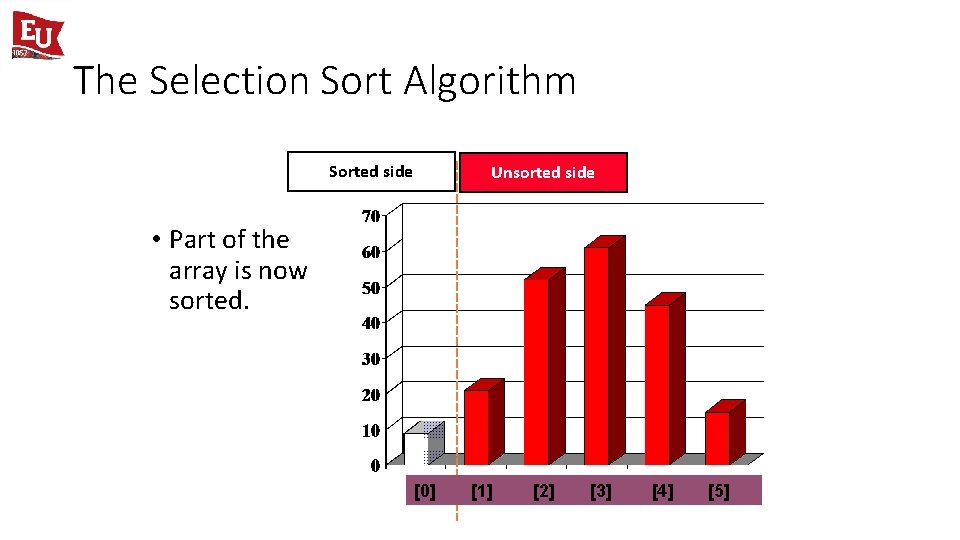
The Selection Sort Algorithm Sorted side Unsorted side • Part of the array is now sorted. [0] [1] [2] [3] [4] [5]
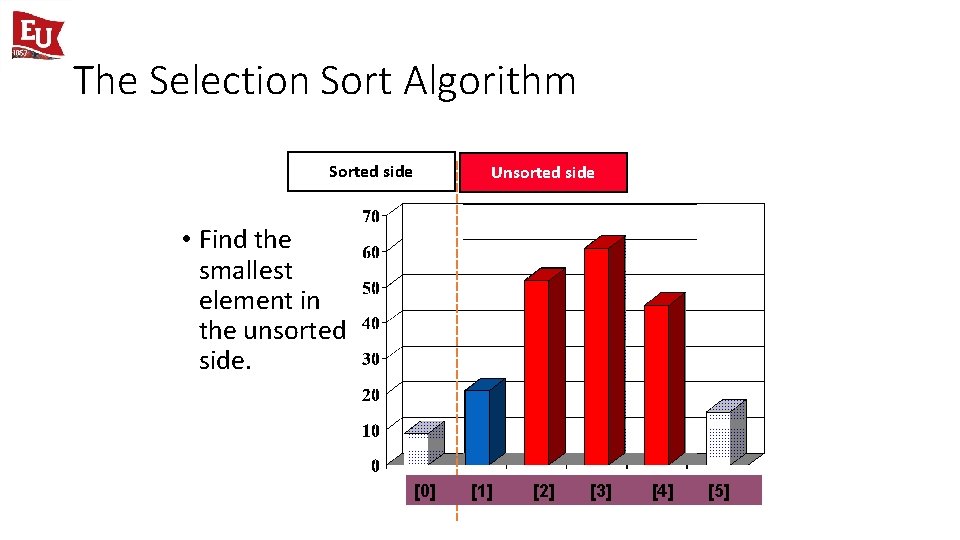
The Selection Sort Algorithm Sorted side Unsorted side • Find the smallest element in the unsorted side. [0] [1] [2] [3] [4] [5]
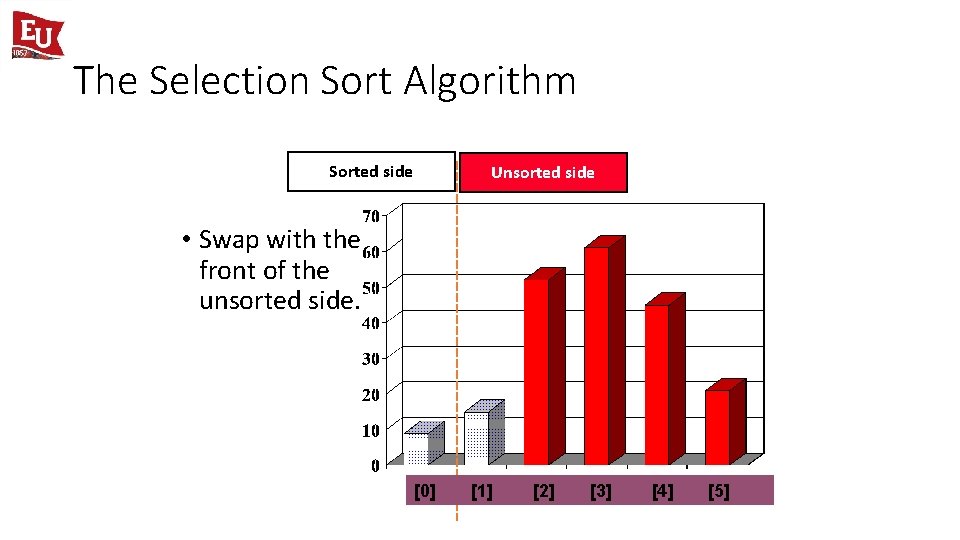
The Selection Sort Algorithm Sorted side Unsorted side • Swap with the front of the unsorted side. [0] [1] [2] [3] [4] [5]
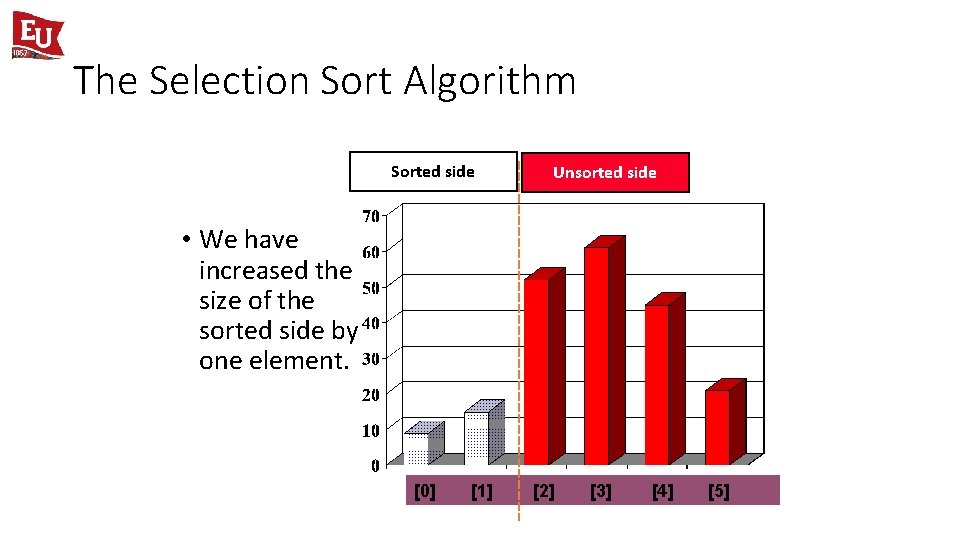
The Selection Sort Algorithm Sorted side Unsorted side • We have increased the size of the sorted side by one element. [0] [1] [2] [3] [4] [5]
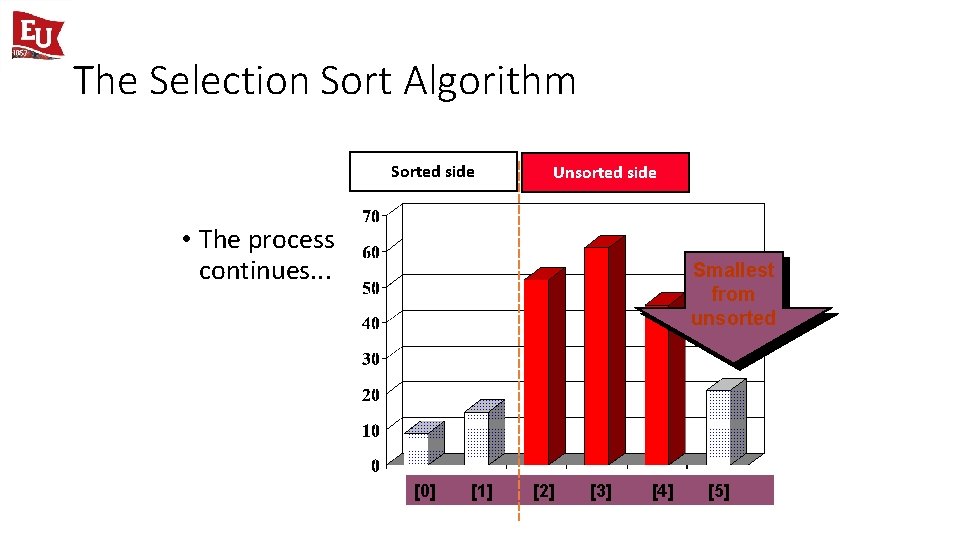
The Selection Sort Algorithm Sorted side Unsorted side • The process continues. . . Smallest from unsorted [0] [1] [2] [3] [4] [5]
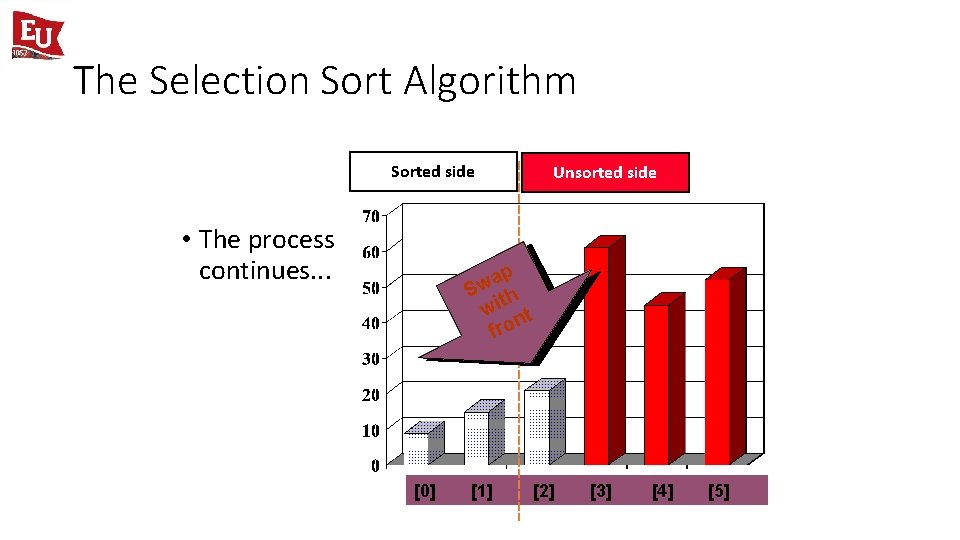
The Selection Sort Algorithm Sorted side • The process continues. . . Unsorted side p a S w th wi t n fr o [0] [1] [2] [3] [4] [5]
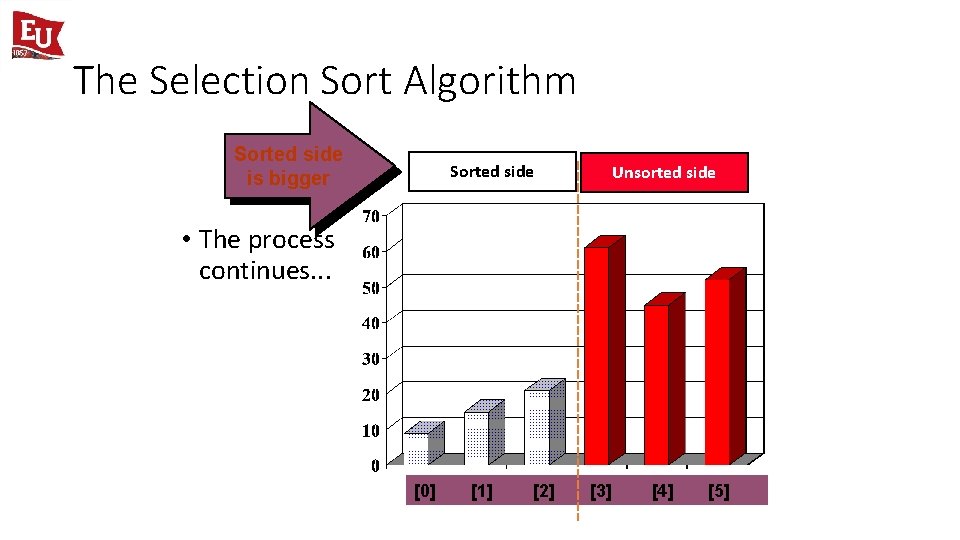
The Selection Sort Algorithm Sorted side is bigger Sorted side Unsorted side • The process continues. . . [0] [1] [2] [3] [4] [5]
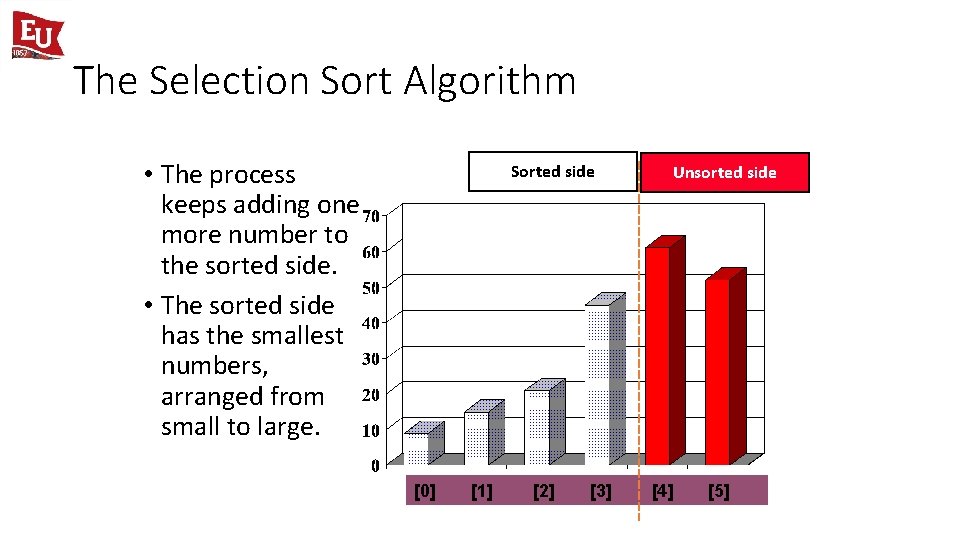
The Selection Sort Algorithm • The process keeps adding one more number to the sorted side. • The sorted side has the smallest numbers, arranged from small to large. Sorted side [0] [1] [2] [3] Unsorted side [4] [5]
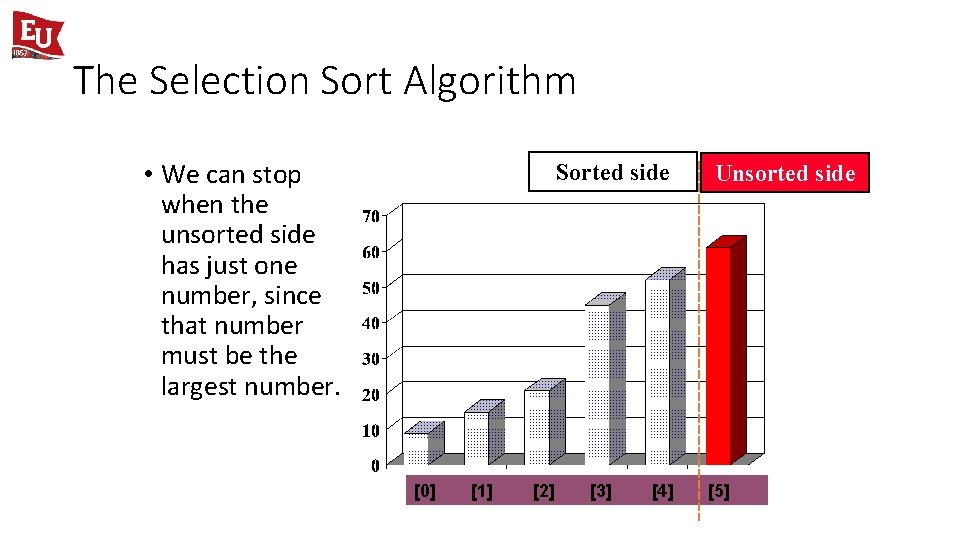
The Selection Sort Algorithm • We can stop when the unsorted side has just one number, since that number must be the largest number. Sorted side [0] [1] [2] [3] [4] Unsorted side [5]
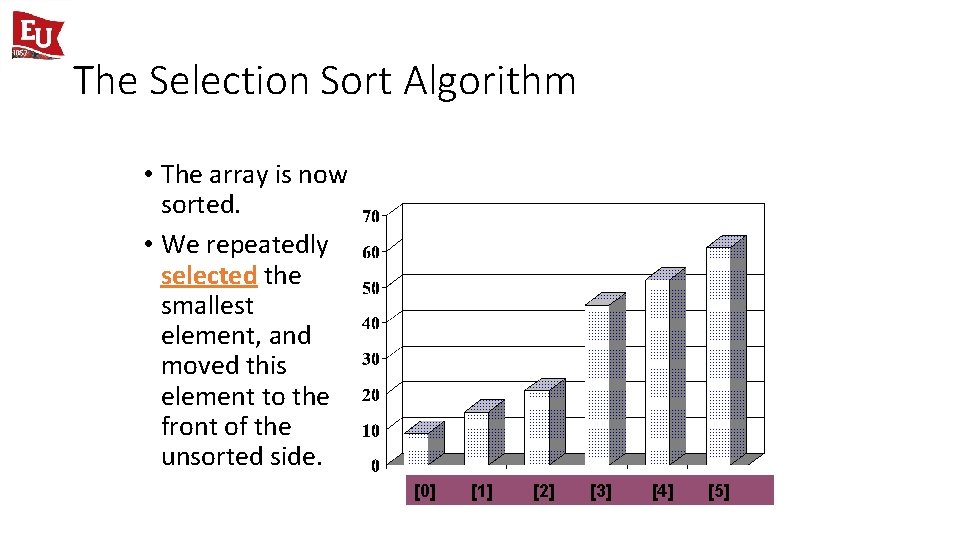
The Selection Sort Algorithm • The array is now sorted. • We repeatedly selected the smallest element, and moved this element to the front of the unsorted side. [0] [1] [2] [3] [4] [5]
![Selection Sort Algorithm void selection Sortint arr int n arr length int Selection Sort Algorithm void selection. Sort(int arr[]) { int n = arr. length; int](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-35.jpg)
Selection Sort Algorithm void selection. Sort(int arr[]) { int n = arr. length; int i, j, min. Index, tmp; for (i = 0; i < n - 1; i++) { min. Index = i; for (j = i + 1; j < n; j++) if (arr[j] < arr[min. Index]) min. Index = j; if (min. Index != i) { tmp = arr[i]; arr[i] = arr[min. Index]; arr[min. Index] = tmp; } } }
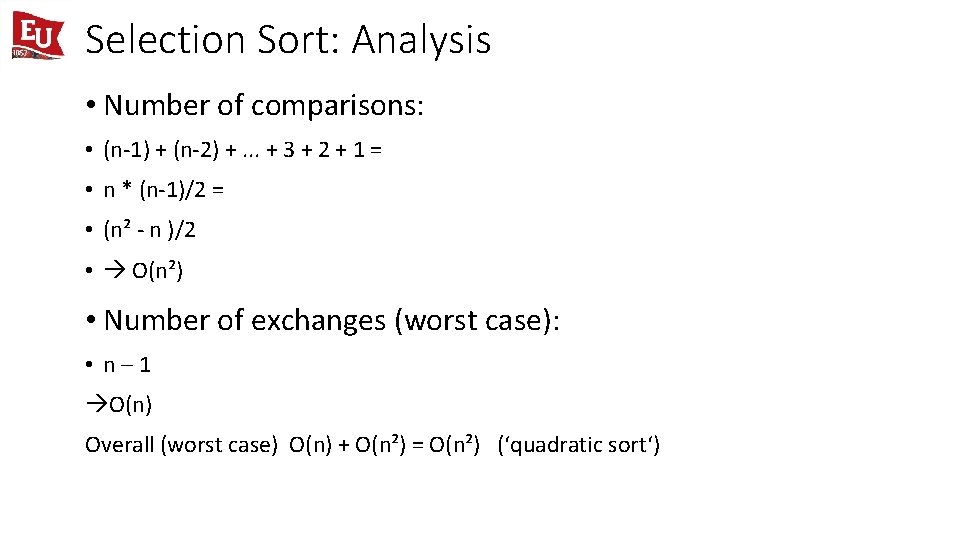
Selection Sort: Analysis • Number of comparisons: • (n-1) + (n-2) +. . . + 3 + 2 + 1 = • n * (n-1)/2 = • (n² - n )/2 • O(n²) • Number of exchanges (worst case): • n– 1 O(n) Overall (worst case) O(n) + O(n²) = O(n²) (‘quadratic sort‘)
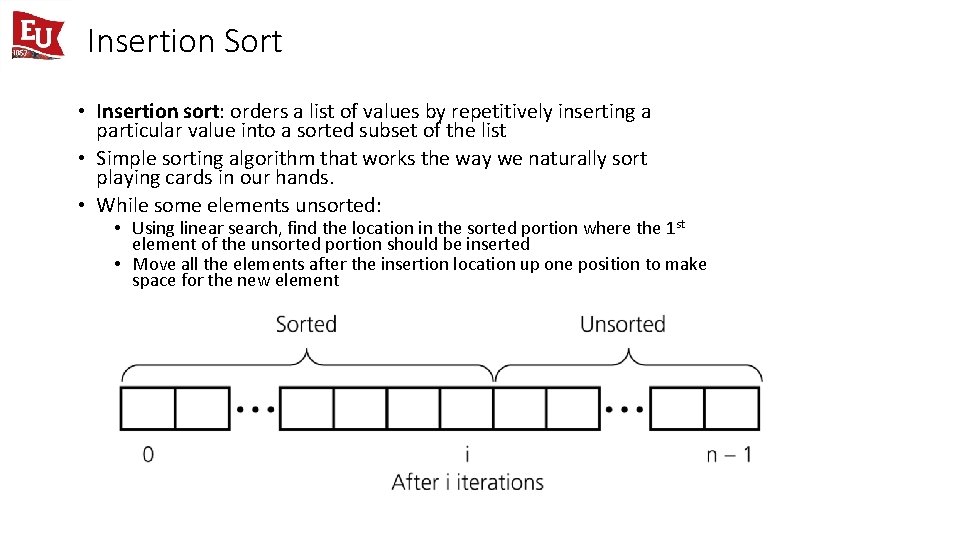
Insertion Sort • Insertion sort: orders a list of values by repetitively inserting a particular value into a sorted subset of the list • Simple sorting algorithm that works the way we naturally sort playing cards in our hands. • While some elements unsorted: • Using linear search, find the location in the sorted portion where the 1 st element of the unsorted portion should be inserted • Move all the elements after the insertion location up one position to make space for the new element
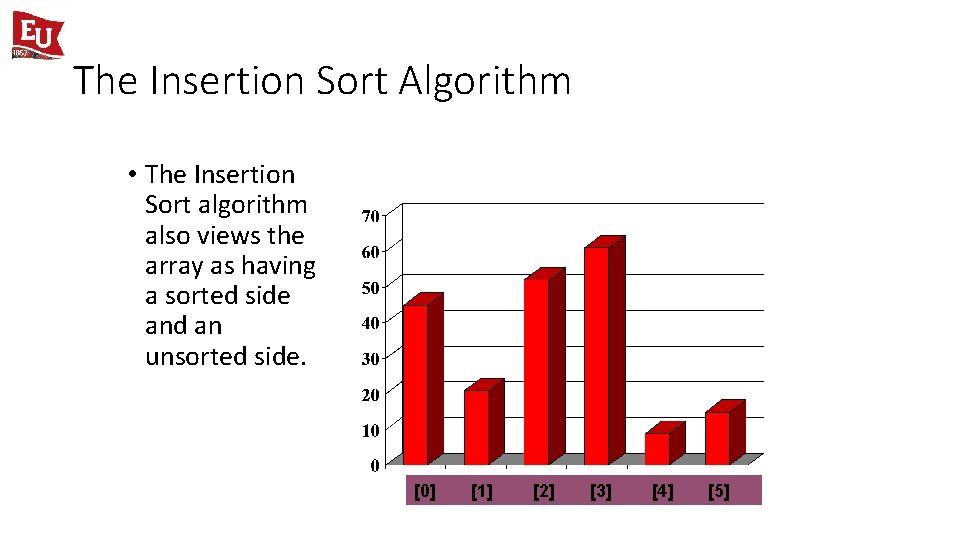
The Insertion Sort Algorithm • The Insertion Sort algorithm also views the array as having a sorted side and an unsorted side. [0] [1] [2] [3] [4] [5]
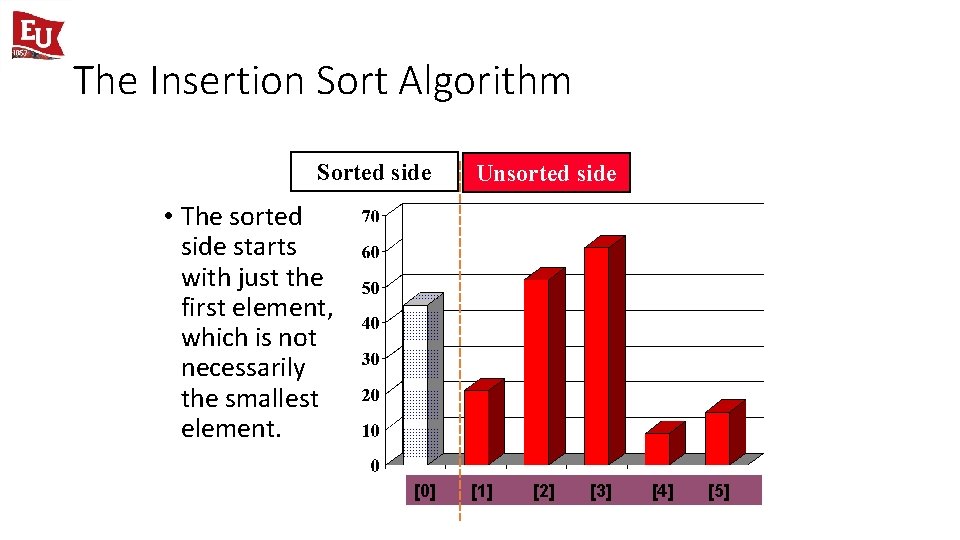
The Insertion Sort Algorithm Sorted side Unsorted side • The sorted side starts with just the first element, which is not necessarily the smallest element. [0] [1] [2] [3] [4] [5]
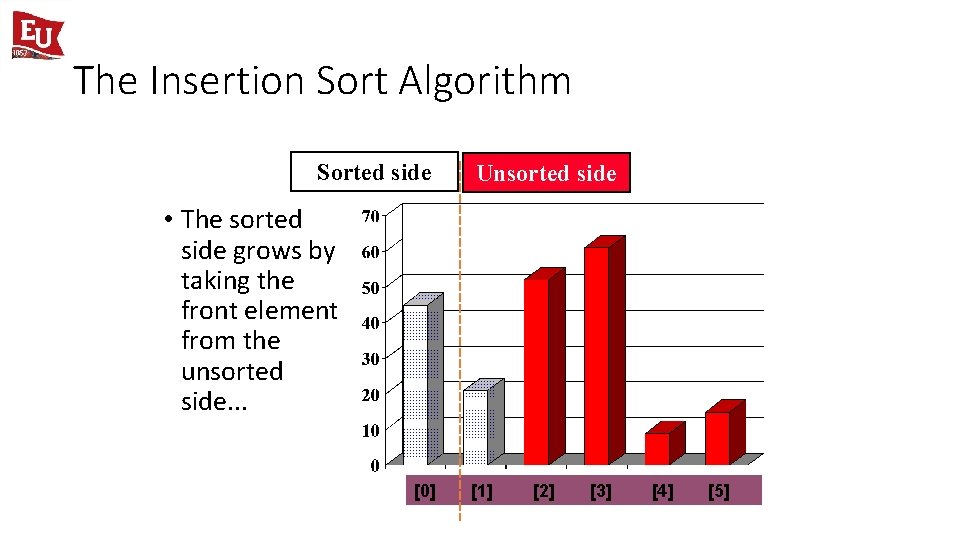
The Insertion Sort Algorithm Sorted side Unsorted side • The sorted side grows by taking the front element from the unsorted side. . . [0] [1] [2] [3] [4] [5]
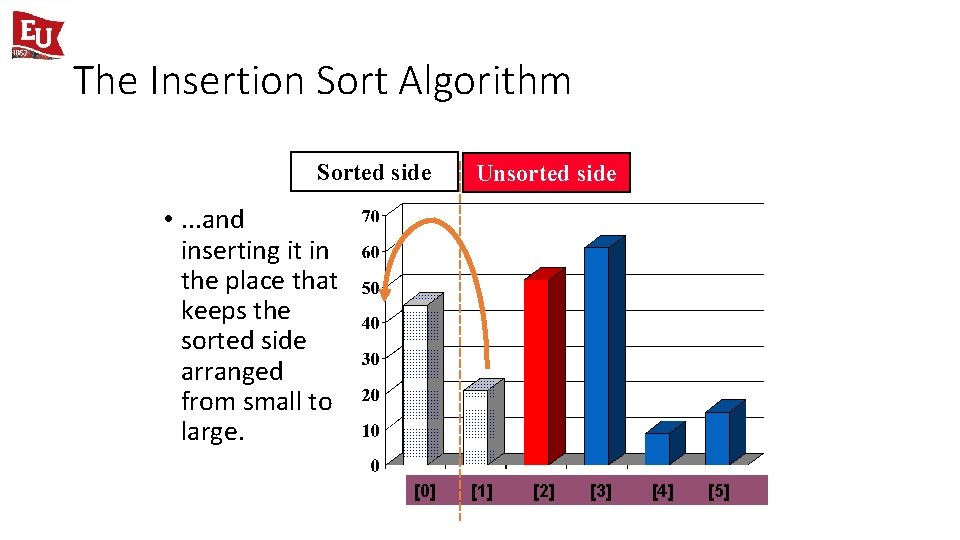
The Insertion Sort Algorithm Sorted side Unsorted side • . . . and inserting it in the place that keeps the sorted side arranged from small to large. [0] [1] [2] [3] [4] [5]
![The Insertion Sort Algorithm Sorted side 0 1 Unsorted side 2 3 4 5 The Insertion Sort Algorithm Sorted side [0] [1] Unsorted side [2] [3] [4] [5]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-42.jpg)
The Insertion Sort Algorithm Sorted side [0] [1] Unsorted side [2] [3] [4] [5]
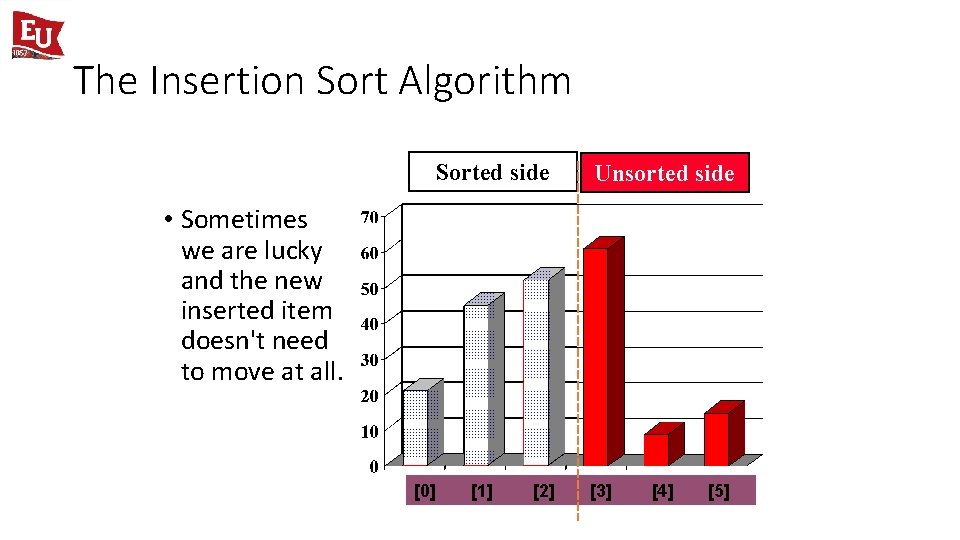
The Insertion Sort Algorithm Sorted side Unsorted side • Sometimes we are lucky and the new inserted item doesn't need to move at all. [0] [1] [2] [3] [4] [5]
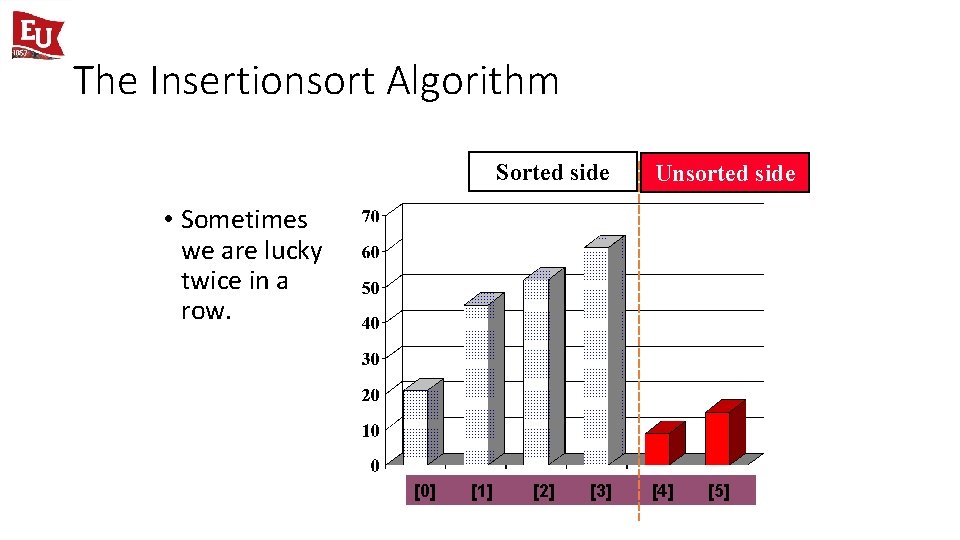
The Insertionsort Algorithm Sorted side Unsorted side • Sometimes we are lucky twice in a row. [0] [1] [2] [3] [4] [5]
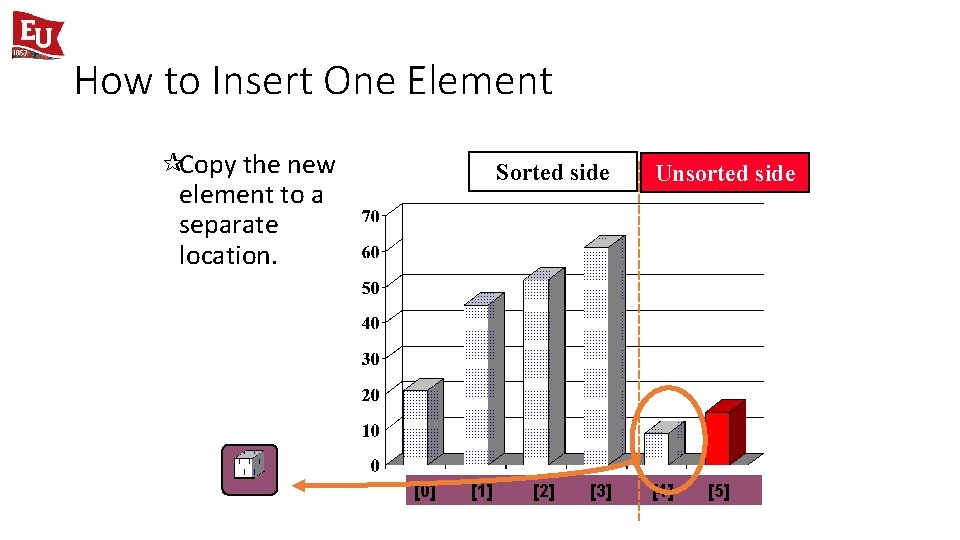
How to Insert One Element ¶Copy the new element to a separate location. Sorted side [0] [1] [2] [3] Unsorted side [4] [5]
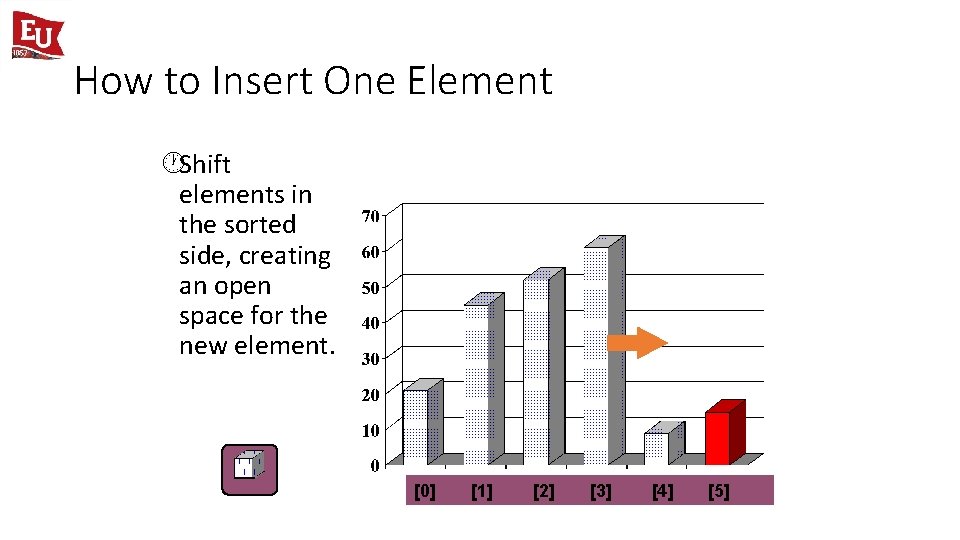
How to Insert One Element ·Shift elements in the sorted side, creating an open space for the new element. [0] [1] [2] [3] [4] [5]
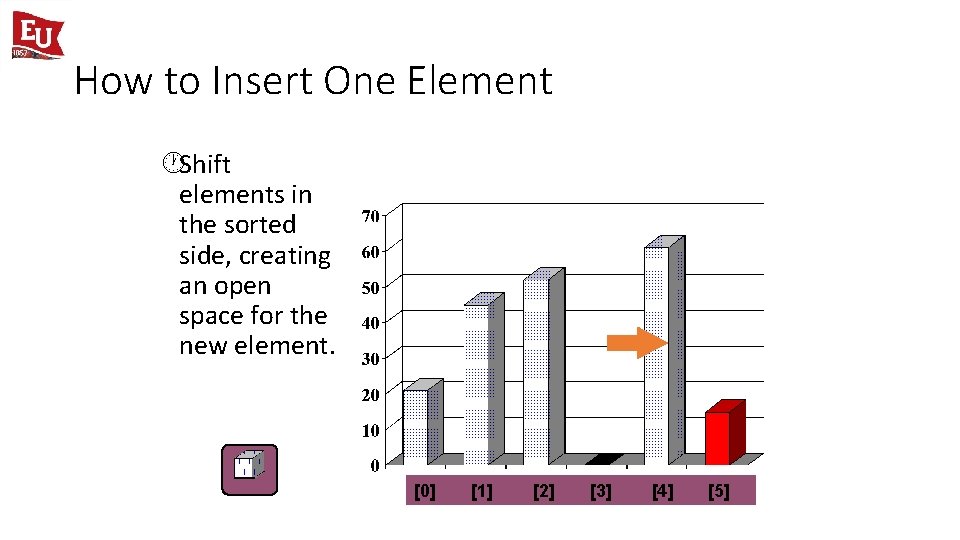
How to Insert One Element ·Shift elements in the sorted side, creating an open space for the new element. [0] [1] [2] [3] [4] [5]
![How to Insert One Element Continue shifting elements 0 1 2 3 How to Insert One Element ·Continue shifting elements. . . [0] [1] [2] [3]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-48.jpg)
How to Insert One Element ·Continue shifting elements. . . [0] [1] [2] [3] [4] [5]
![How to Insert One Element Continue shifting elements 0 1 2 3 How to Insert One Element ·Continue shifting elements. . . [0] [1] [2] [3]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-49.jpg)
How to Insert One Element ·Continue shifting elements. . . [0] [1] [2] [3] [4] [5]
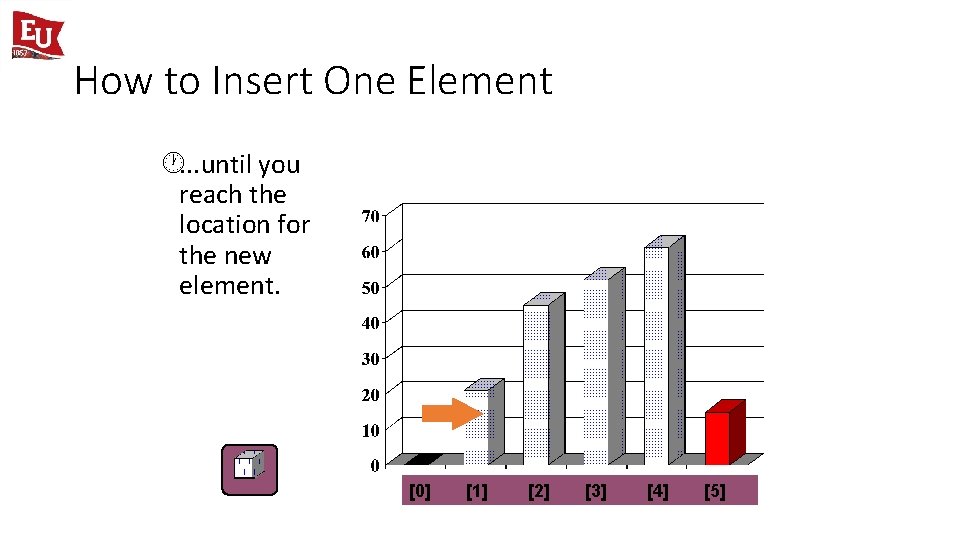
How to Insert One Element ·. . . until you reach the location for the new element. [0] [1] [2] [3] [4] [5]
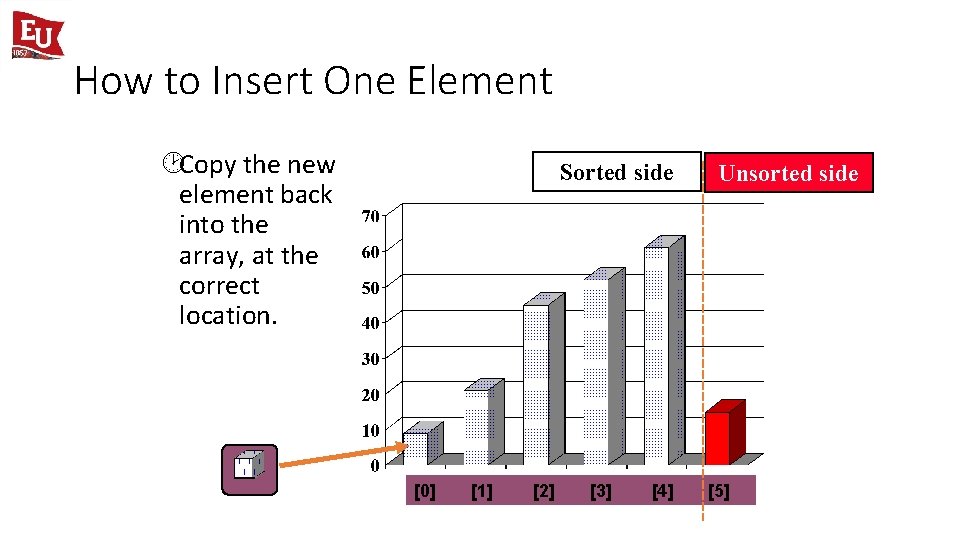
How to Insert One Element ¸Copy the new element back into the array, at the correct location. Sorted side [0] [1] [2] [3] [4] Unsorted side [5]
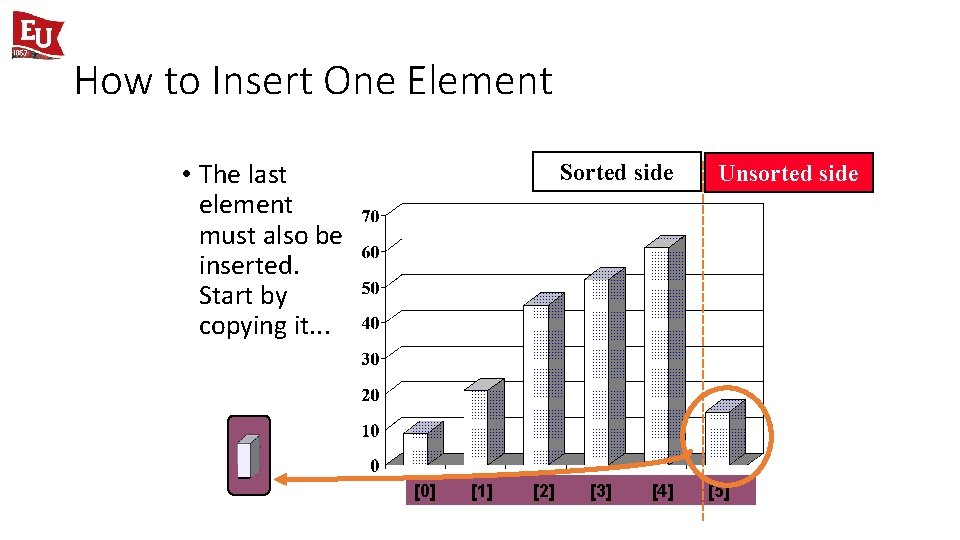
How to Insert One Element • The last element must also be inserted. Start by copying it. . . Sorted side [0] [1] [2] [3] [4] Unsorted side [5]
![Sorted Result 0 1 2 3 4 5 Sorted Result [0] [1] [2] [3] [4] [5]](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-53.jpg)
Sorted Result [0] [1] [2] [3] [4] [5]
![Insertion Sort Code void insertion Sortint arr int n arr length for Insertion Sort Code void insertion. Sort(int arr[]) { int n = arr. length; for](https://slidetodoc.com/presentation_image_h/9553347887e9355e279db80ed19a69d2/image-54.jpg)
Insertion Sort Code void insertion. Sort(int arr[]) { int n = arr. length; for (int i=1; i<n; ++i) { int key = arr[i]; int j = i-1; /* Move elements of arr[0. . i-1], that are greater than key, to one position ahead of their current position */ while (j>=0 && arr[j] > key) { arr[j+1] = arr[j]; j = j-1; } arr[j+1] = key; } }
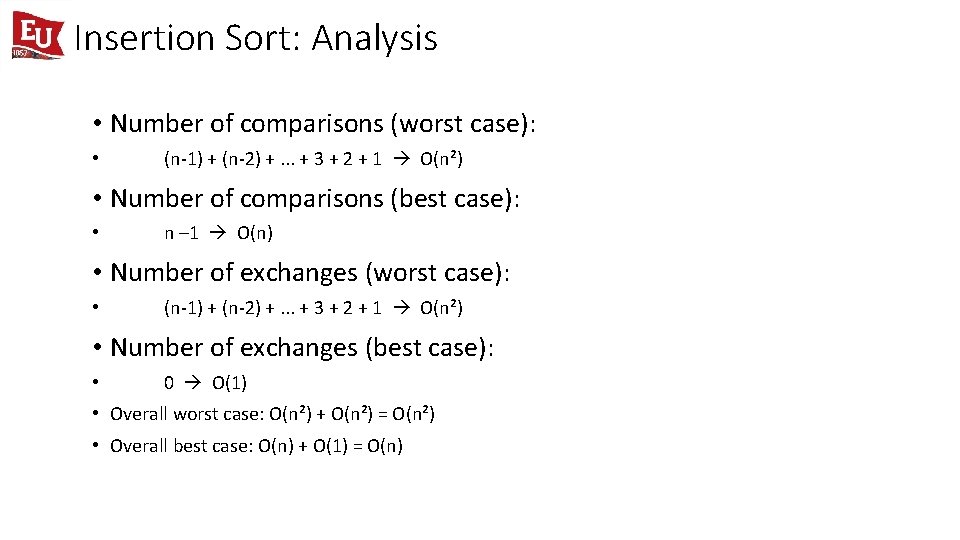
Insertion Sort: Analysis • Number of comparisons (worst case): • (n-1) + (n-2) +. . . + 3 + 2 + 1 O(n²) • Number of comparisons (best case): • n – 1 O(n) • Number of exchanges (worst case): • (n-1) + (n-2) +. . . + 3 + 2 + 1 O(n²) • Number of exchanges (best case): • 0 O(1) • Overall worst case: O(n²) + O(n²) = O(n²) • Overall best case: O(n) + O(1) = O(n)
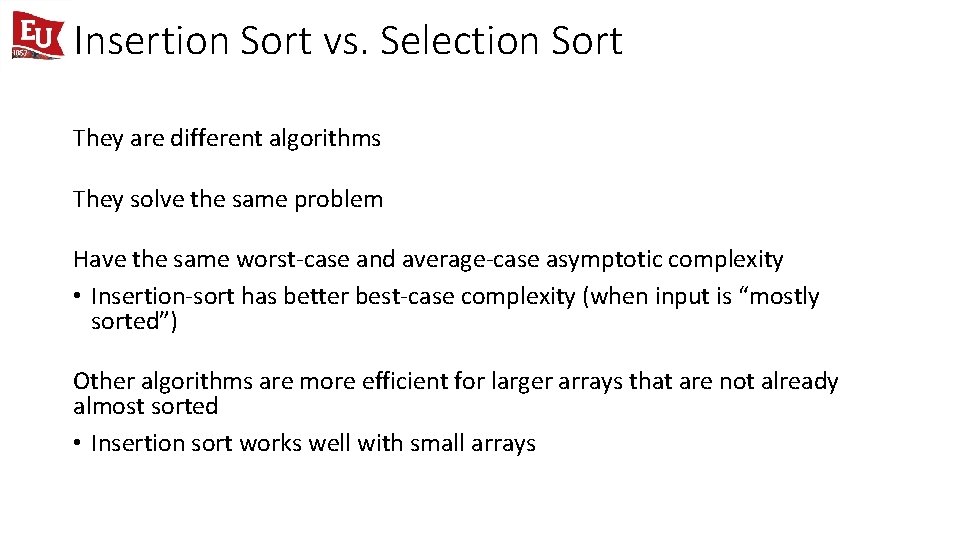
Insertion Sort vs. Selection Sort They are different algorithms They solve the same problem Have the same worst-case and average-case asymptotic complexity • Insertion-sort has better best-case complexity (when input is “mostly sorted”) Other algorithms are more efficient for larger arrays that are not already almost sorted • Insertion sort works well with small arrays
Difference between external and internal sorting
Recursive sorting algorithms
Quadratic sorting algorithms
Efficiency of sorting algorithms
C sorting algorithms
Efficiency of sorting algorithms
Place:sort=8&redirectsmode=2&maxresults=10/
Sorting algorithms with examples
External sorting algorithms
Most common sorting algorithms
Introduction to sorting algorithms
Insertion sort decision tree 4 elements
Data structures and algorithms iit bombay
Cos 423
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Ajit diwan iit bombay
Algorithms + data structures = programs
Data structures and algorithms
Data structures and algorithms
Waterloo data structures and algorithms
Signature file structure in information retrieval system
Data structures and algorithms
Homologous structure
Berikut ini beberapa tujuan dari sorting
Orale charakterstruktur
Tujuan melakukan sorting data di dokumen adalah
Searching dan sorting
Pengertian exchange sort
Cmu 16-385
Bentuk bilangan oktal dari bilangan desimal 385
Mgt 385
Em 385 1 1 2014
Ece 385
Army class b accident
Soen 385
Ece 385 lab 8
Xkcd 385
Fort polk dmv
385 f to c
Mgt 385
Soen 343
459-393-385
Design and analysis of algorithms syllabus
An introduction to the analysis of algorithms
Analyze algorithm
Association analysis: basic concepts and algorithms
What is input and output in algorithm
Analysis of algorithms
Analysis of algorithms
Measuring algorithm efficiency
Cluster analysis basic concepts and algorithms
Randomized algorithms and probabilistic analysis
Design and analysis of algorithms introduction
Analysis of algorithms lecture notes
Cluster analysis basic concepts and algorithms