Methods A Deeper Look 1 2 7 2
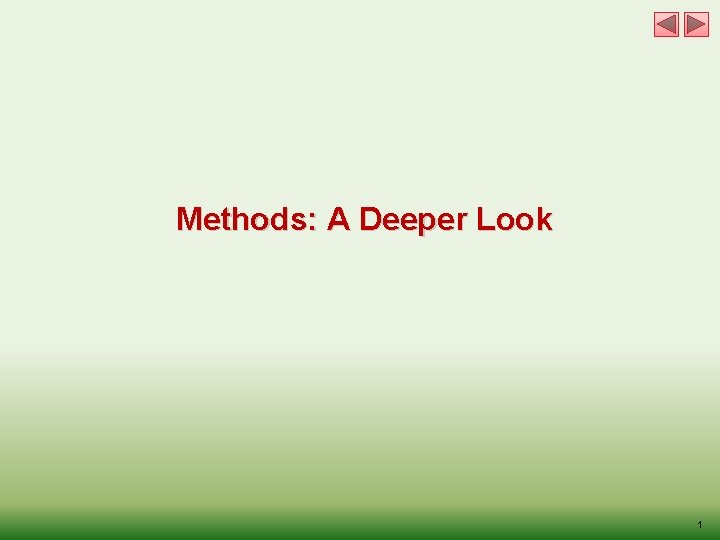
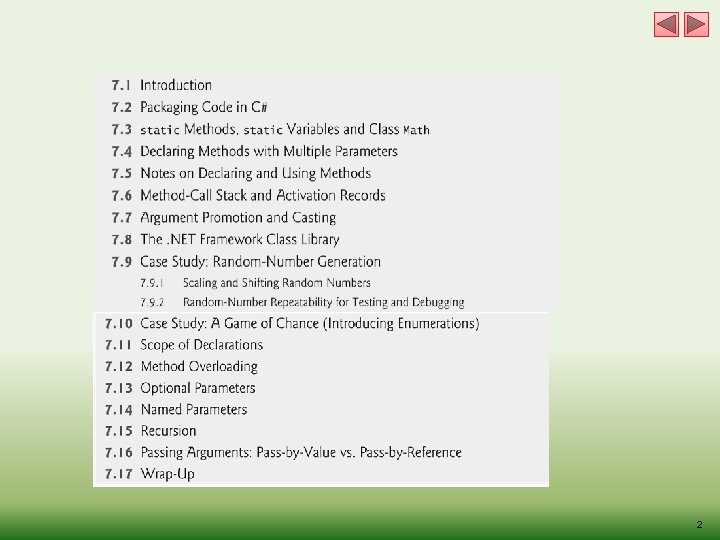
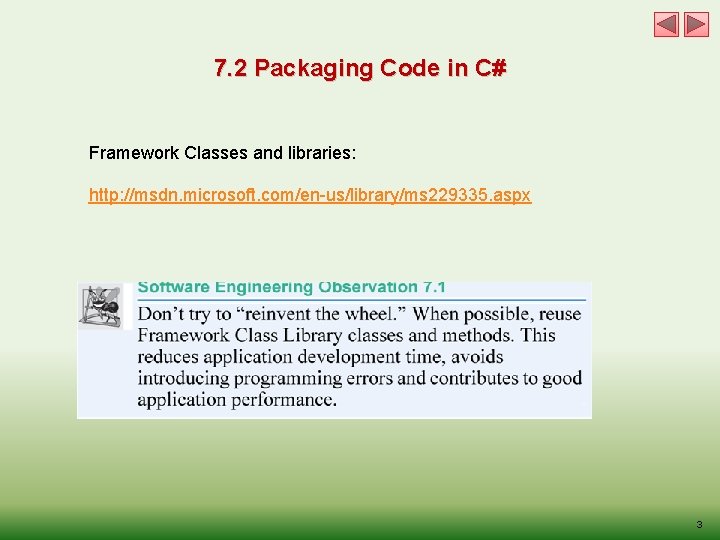
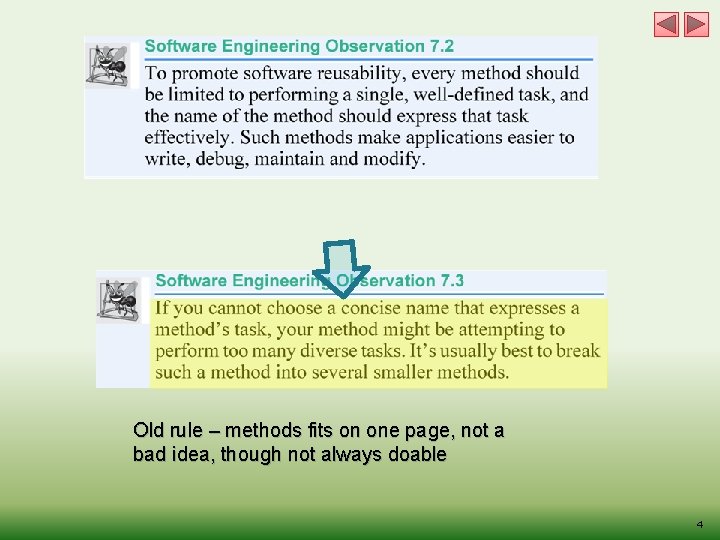
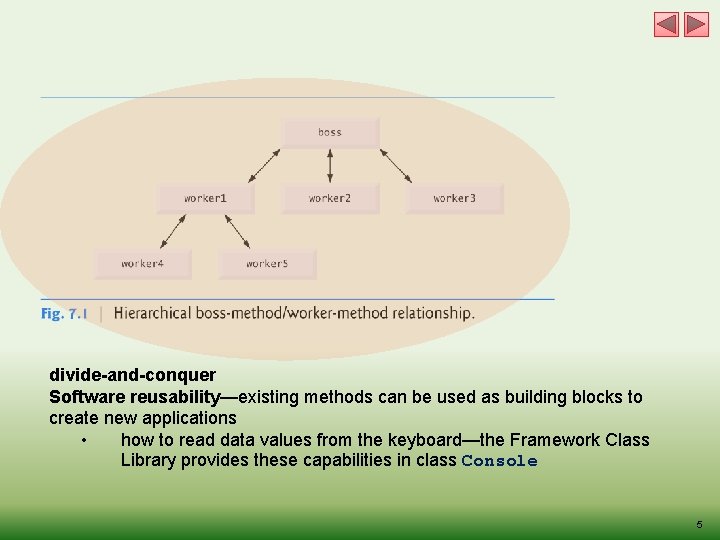
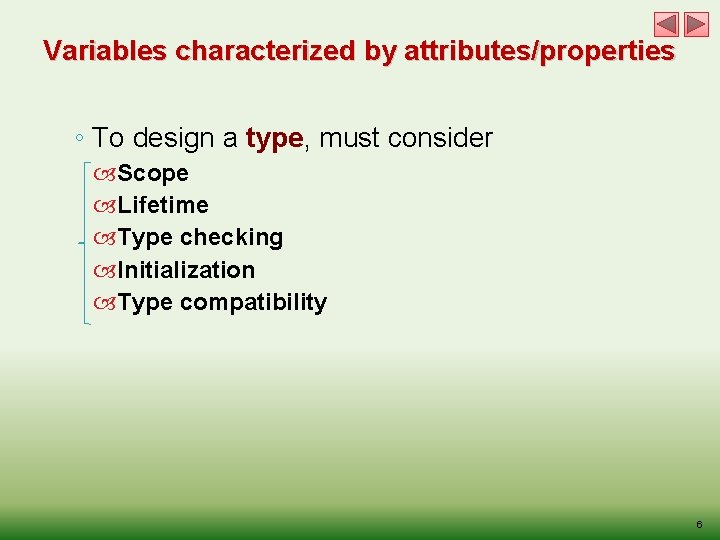
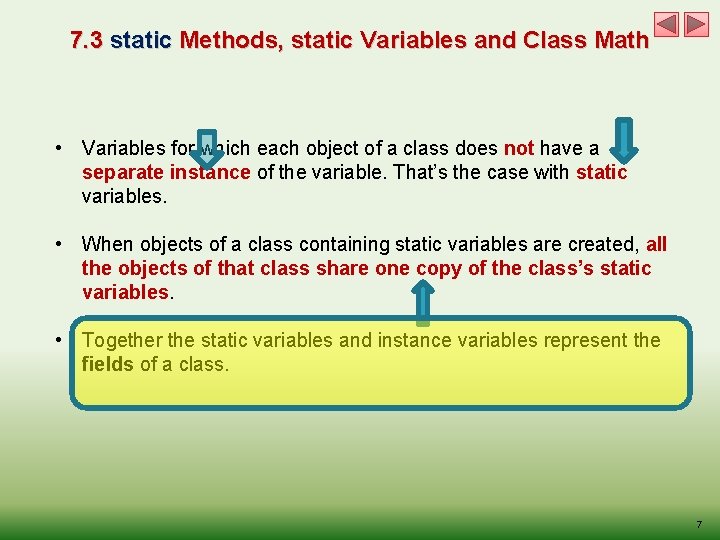
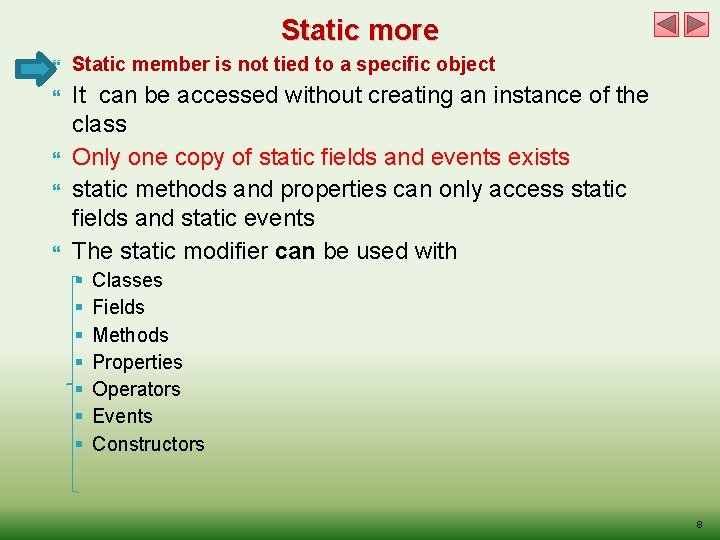
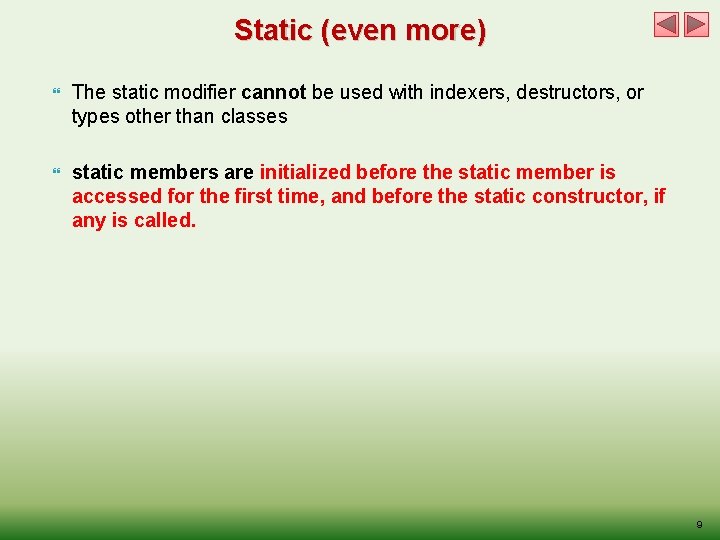
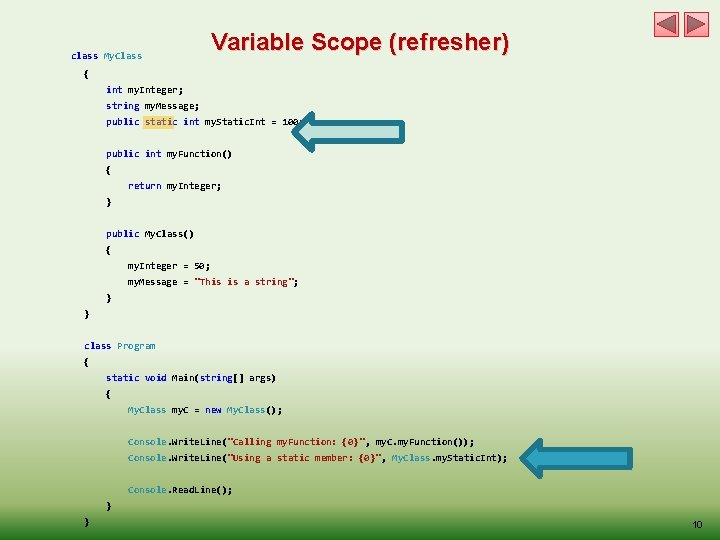
![Why Is Method Main Declared static? public static void Main(string args[]) Comm and-li ne Why Is Method Main Declared static? public static void Main(string args[]) Comm and-li ne](https://slidetodoc.com/presentation_image_h2/877659862373332b75950d0d38dd5fbf/image-11.jpg)
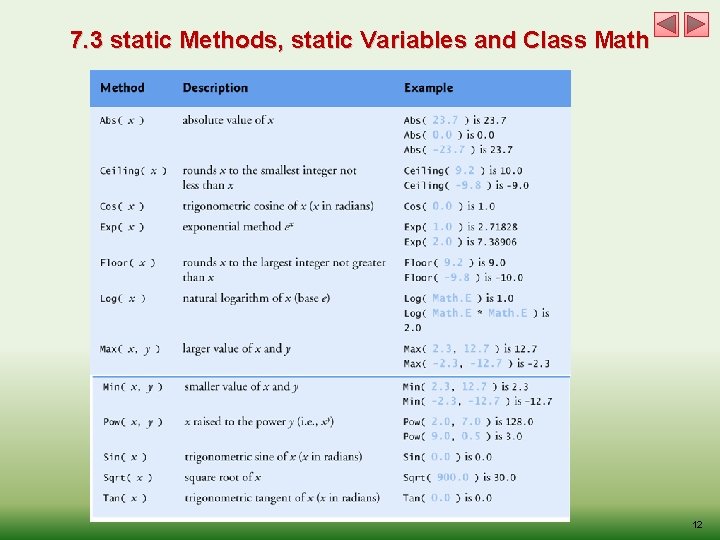
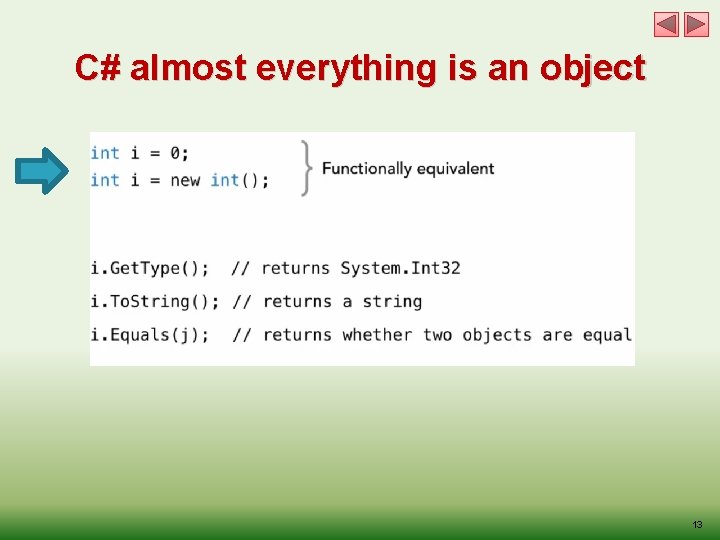
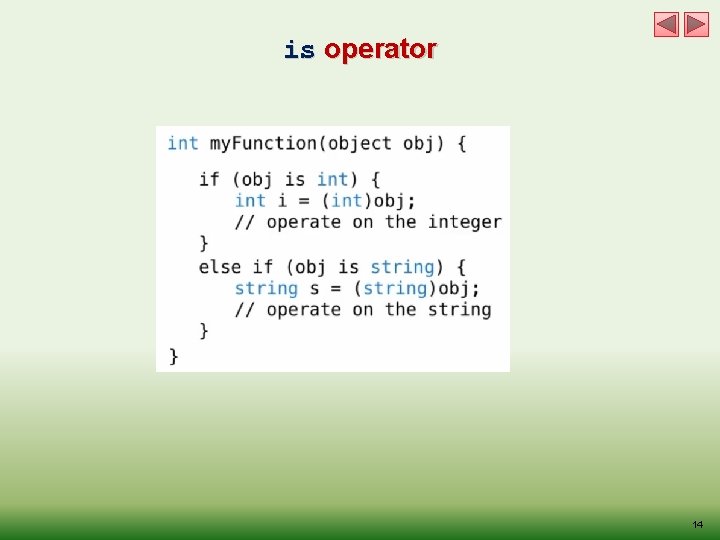
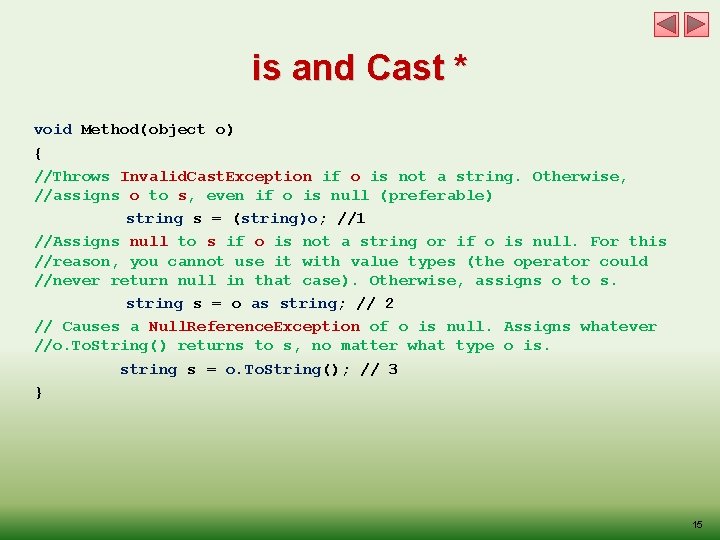
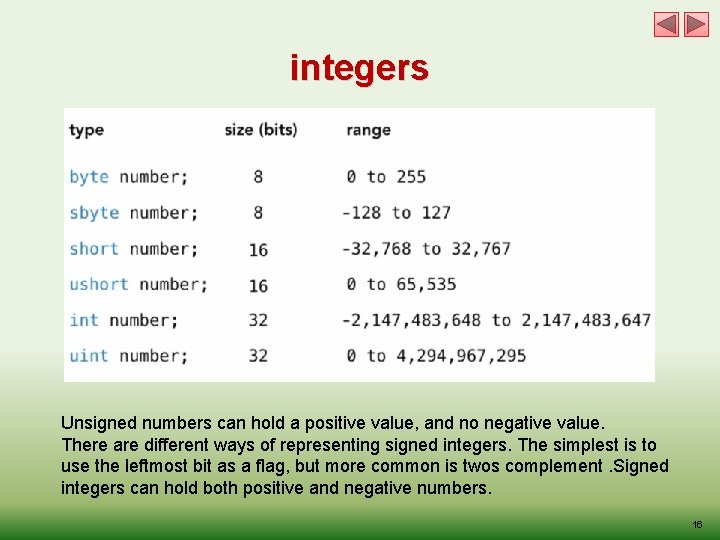
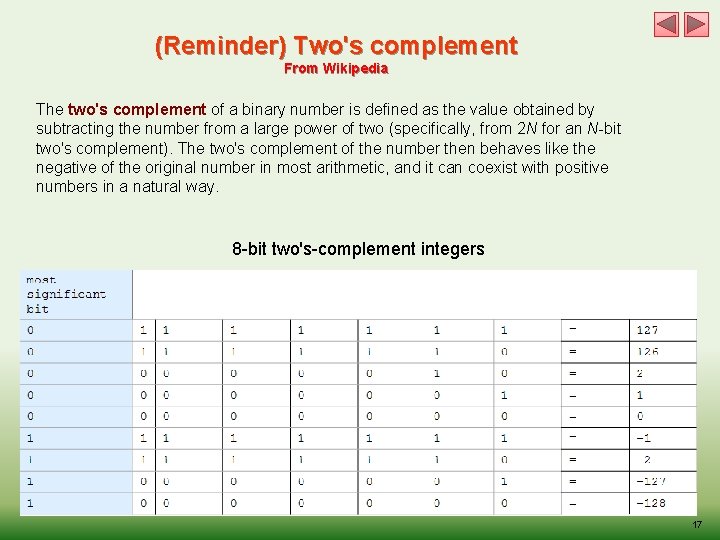
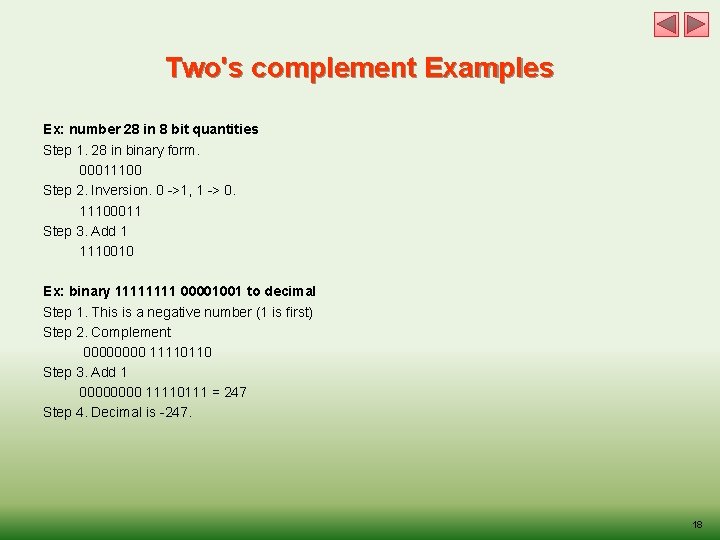
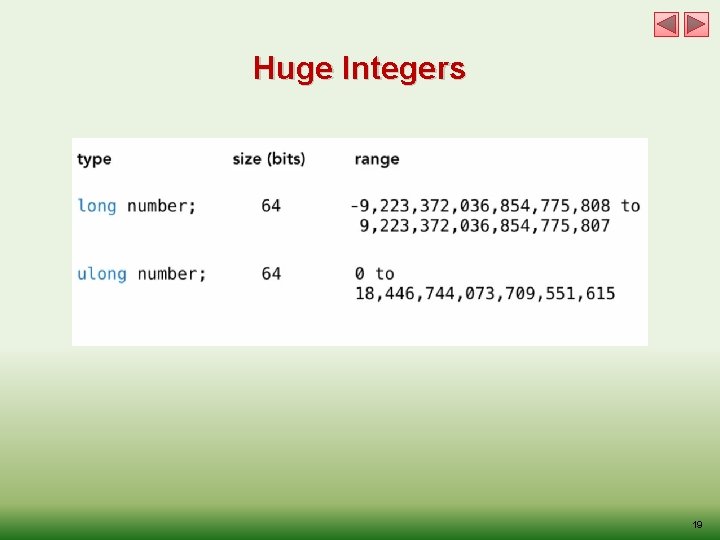
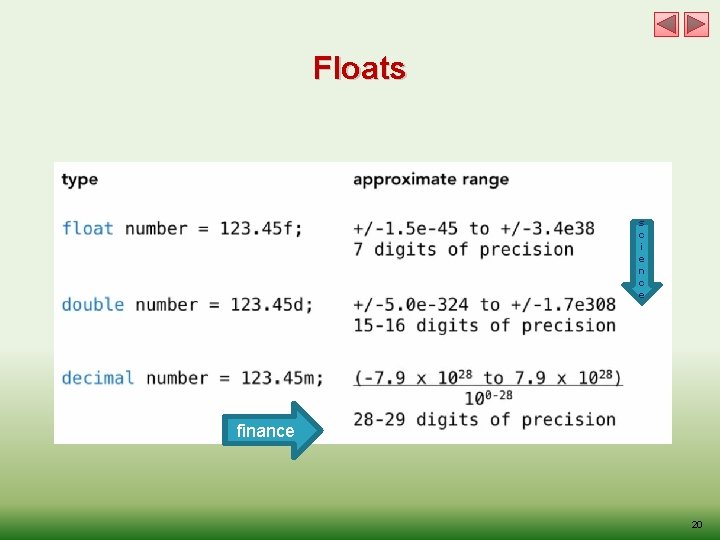
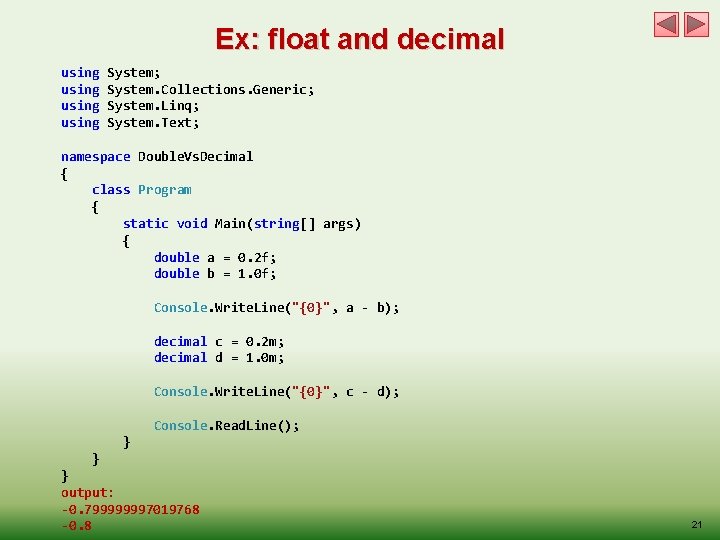
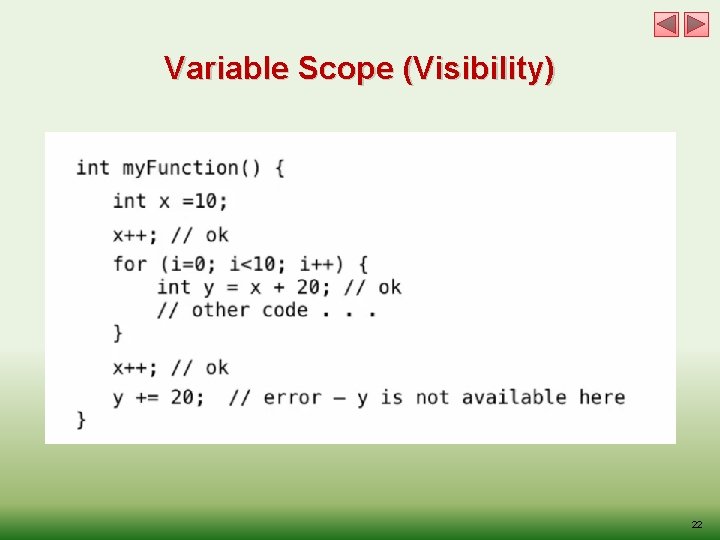
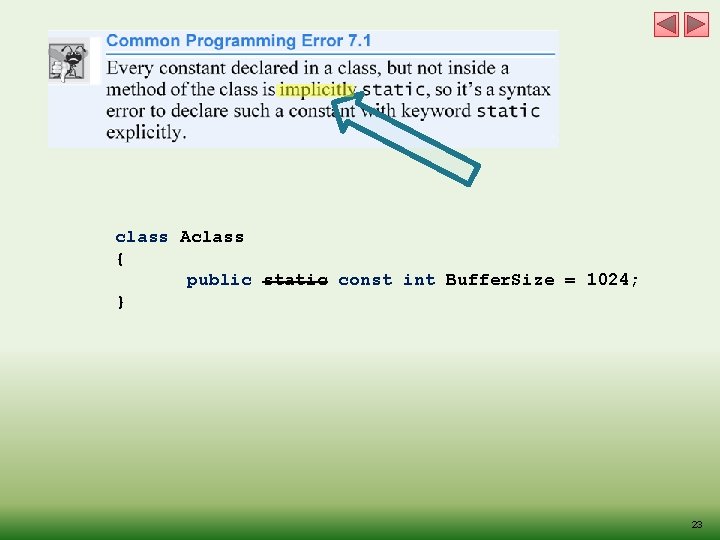
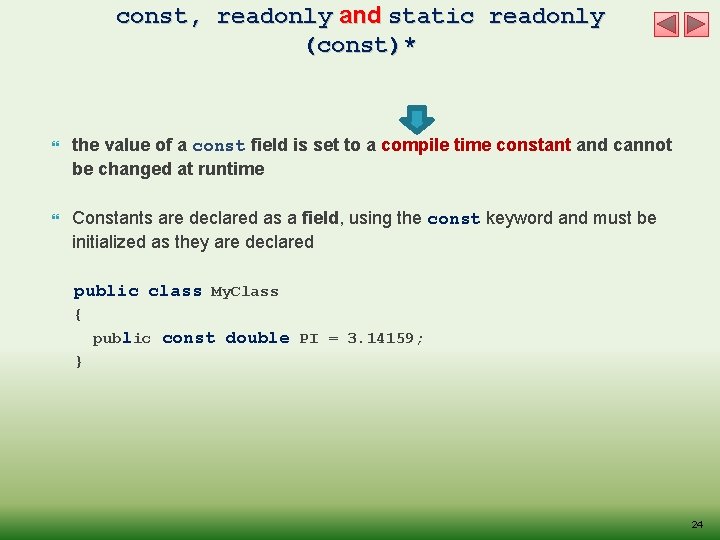
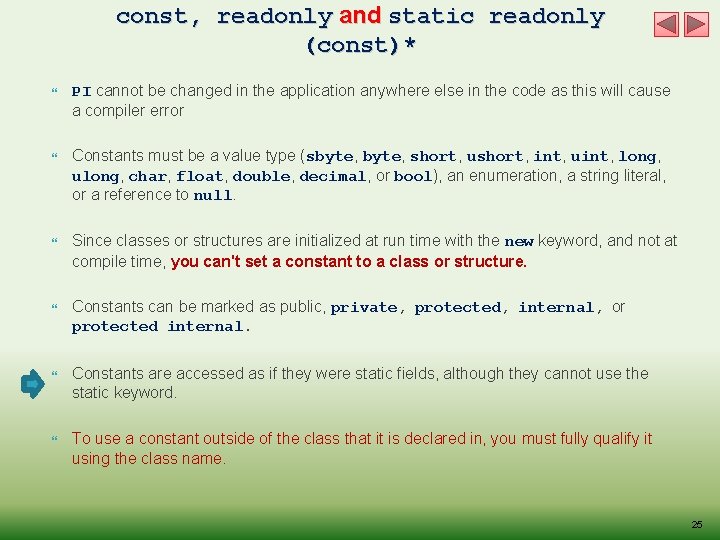
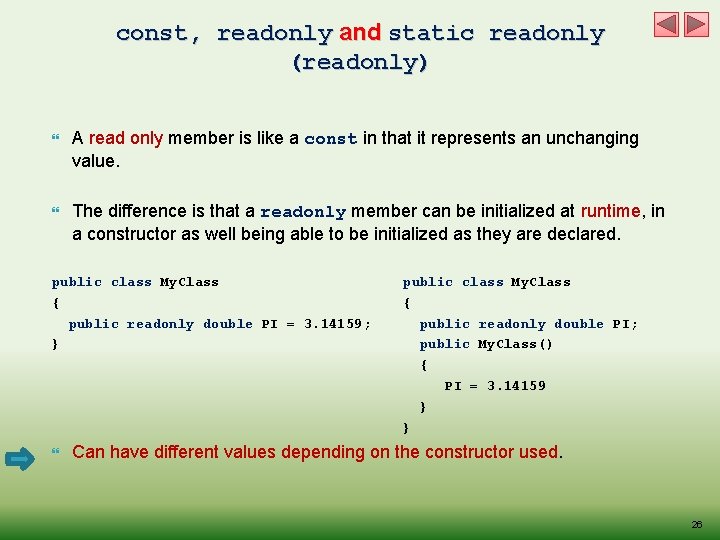
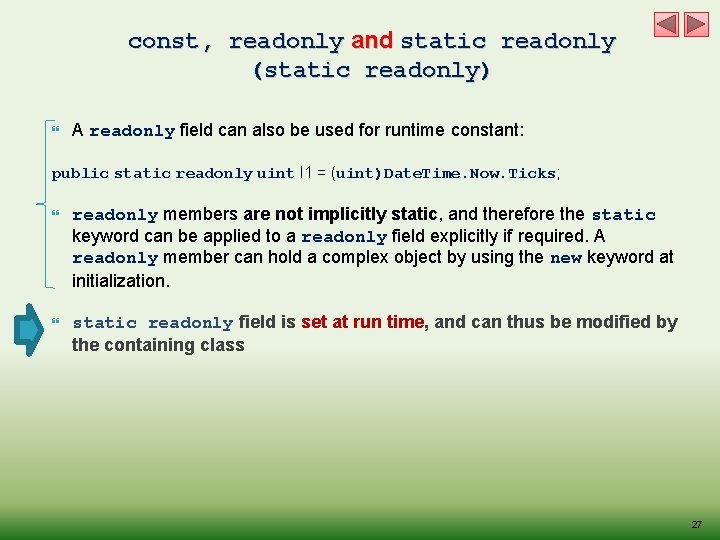
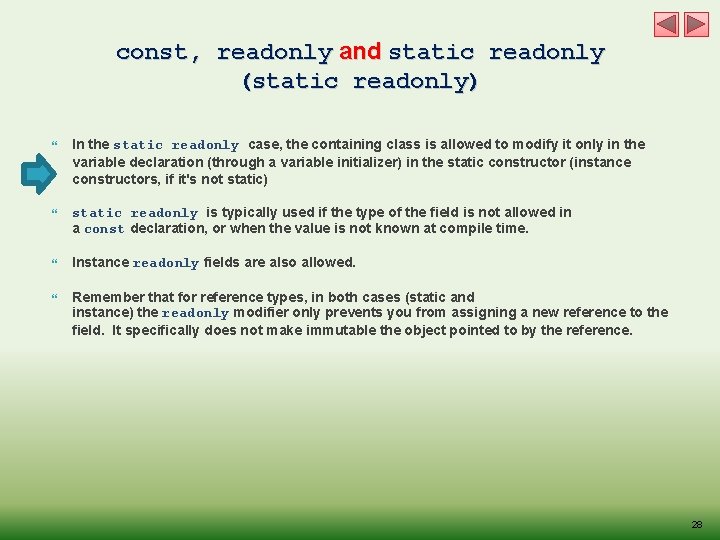
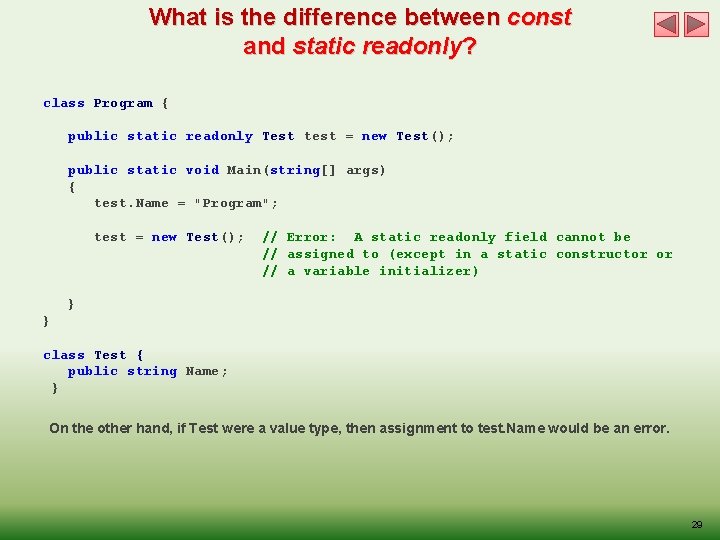
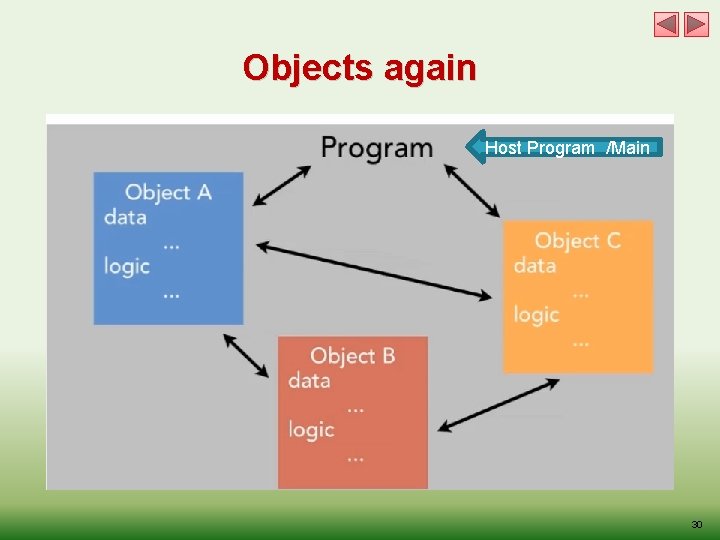
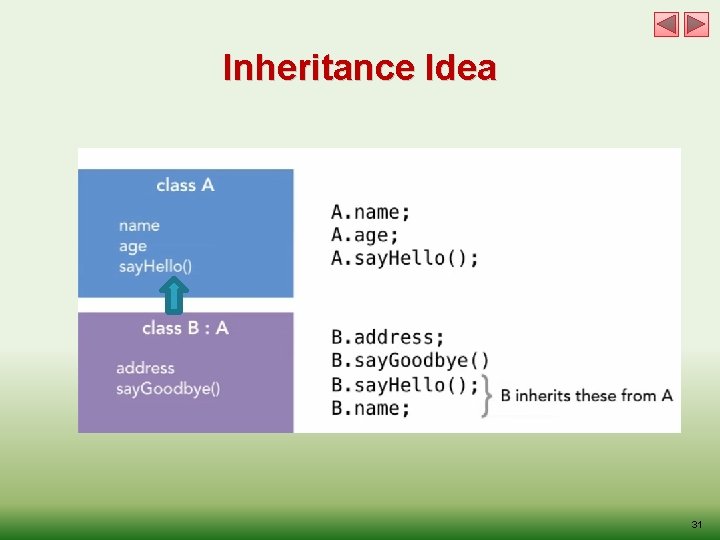
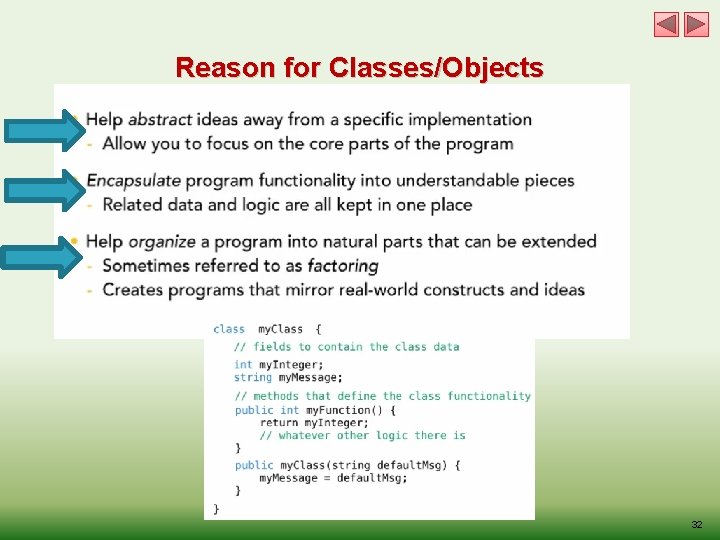
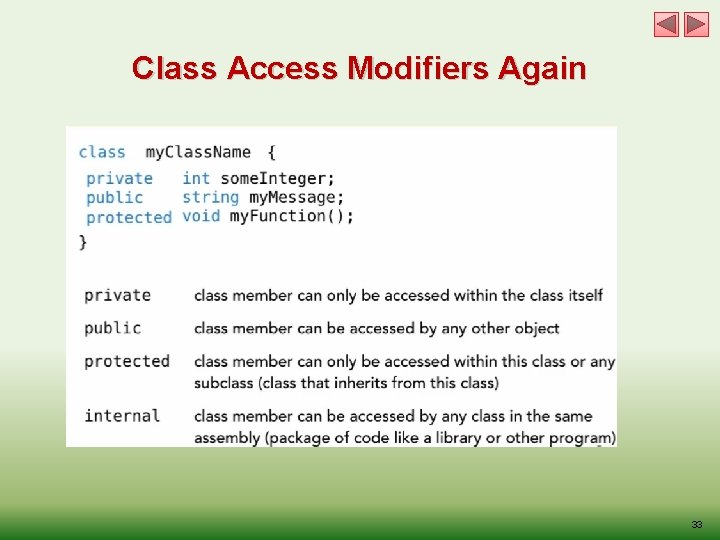
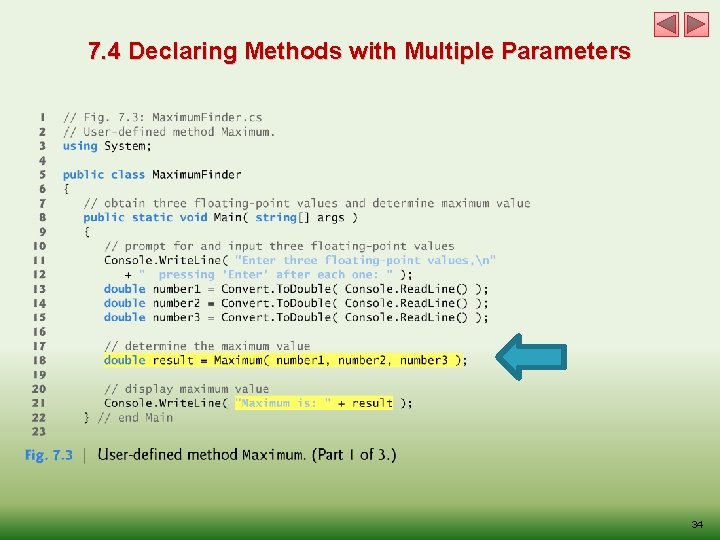
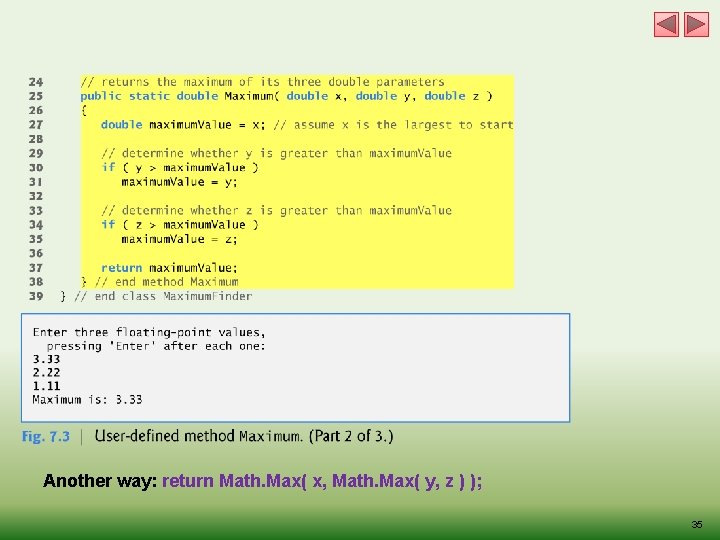
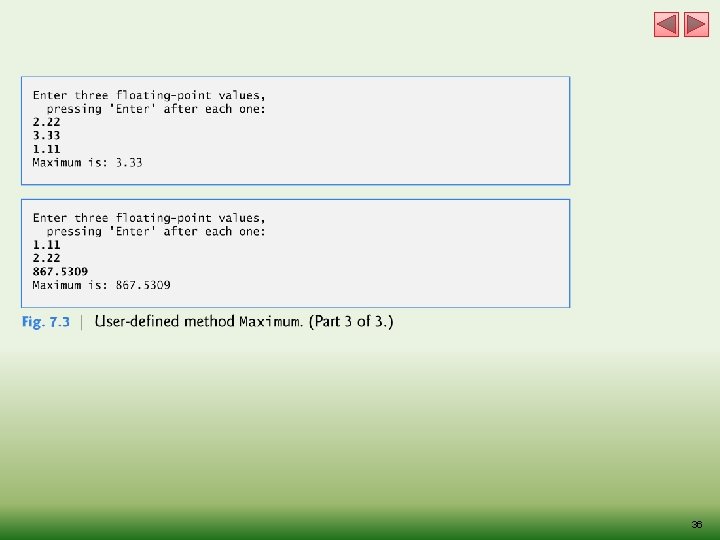
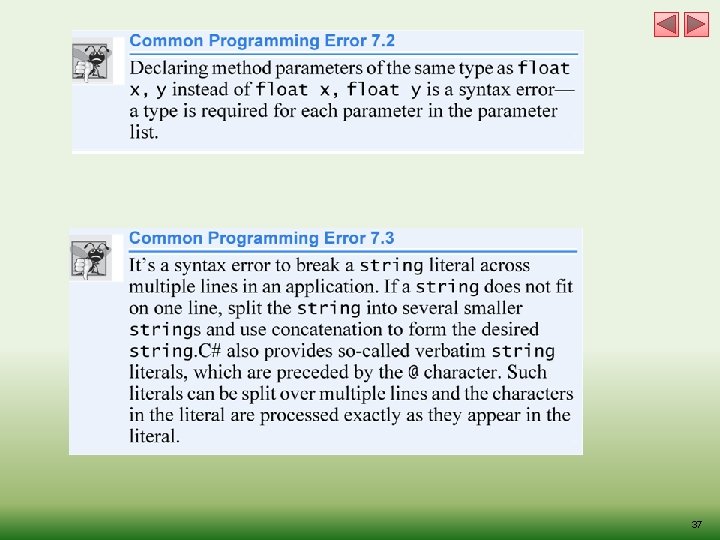
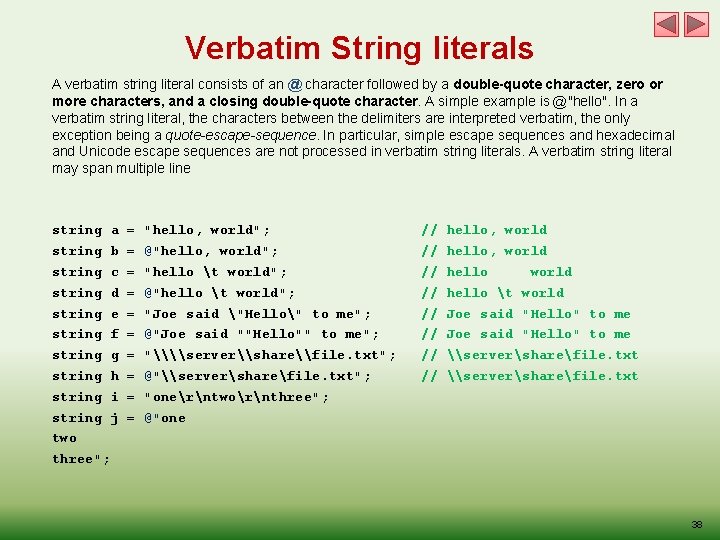
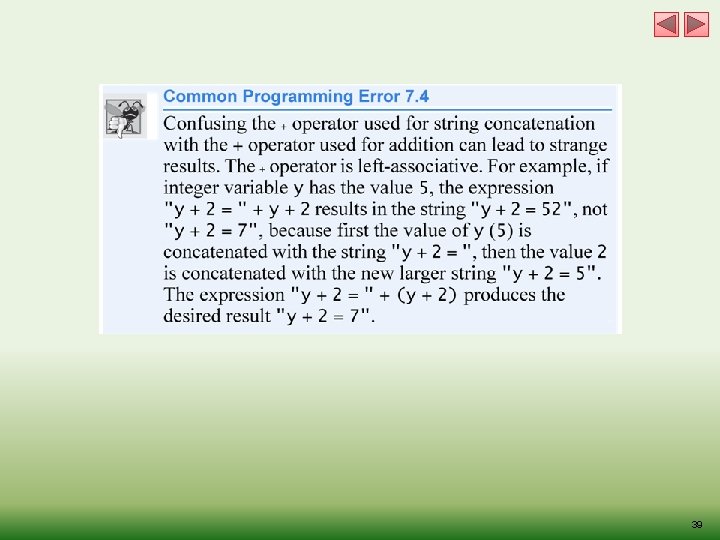
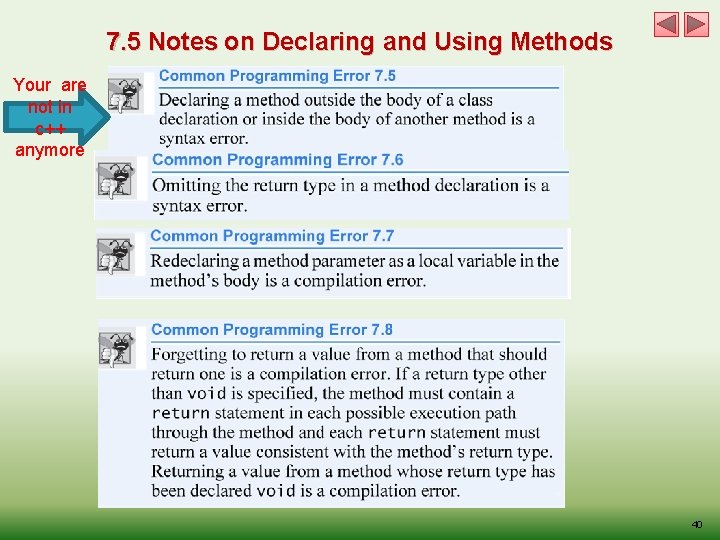
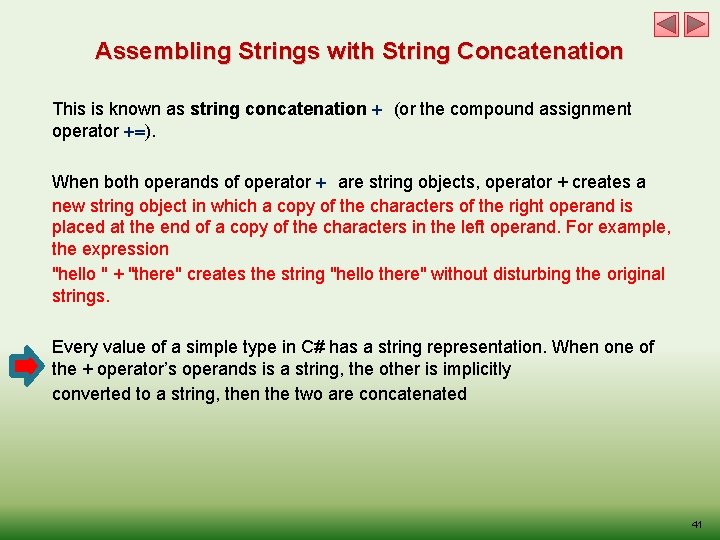
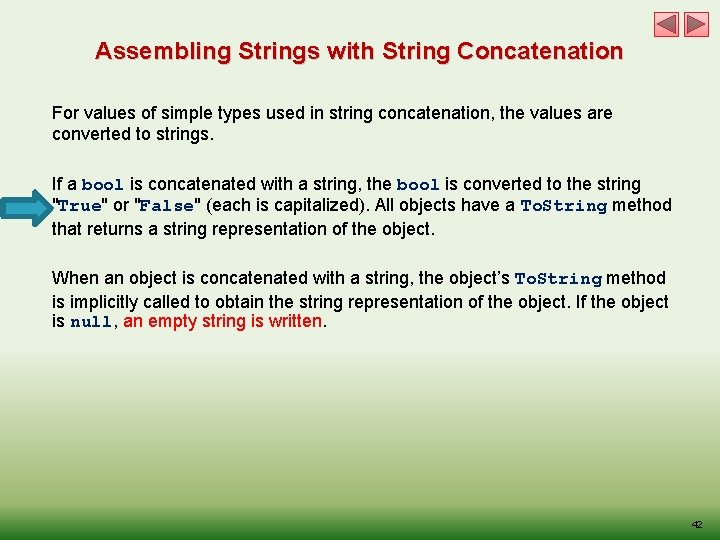
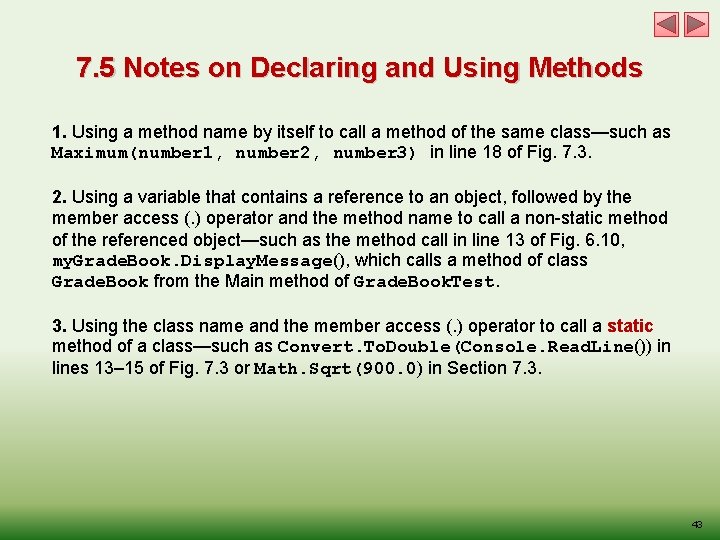
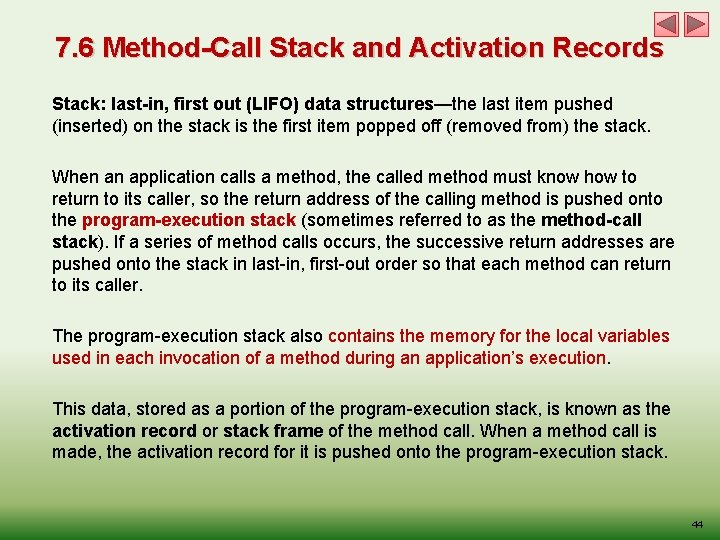
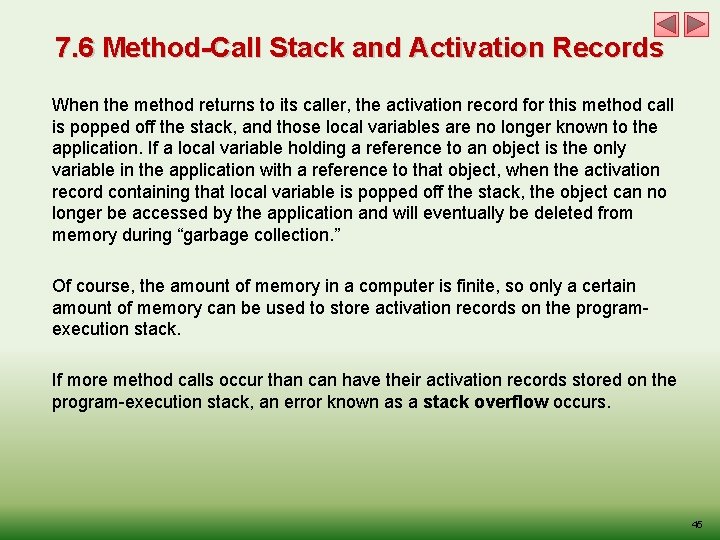
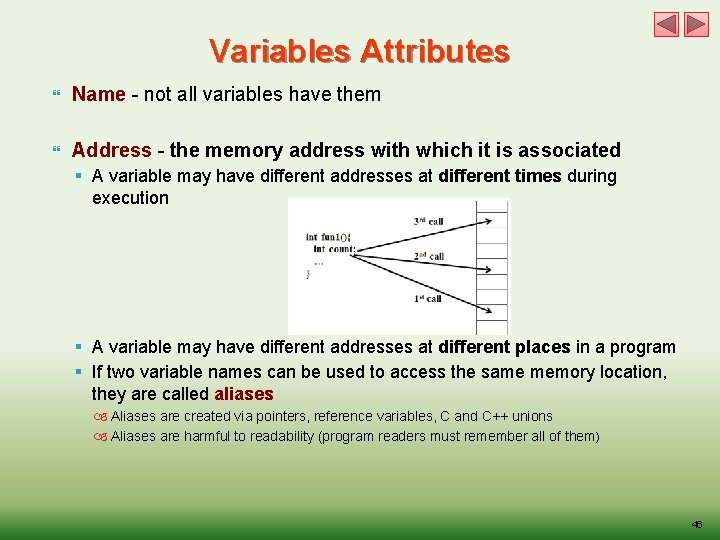
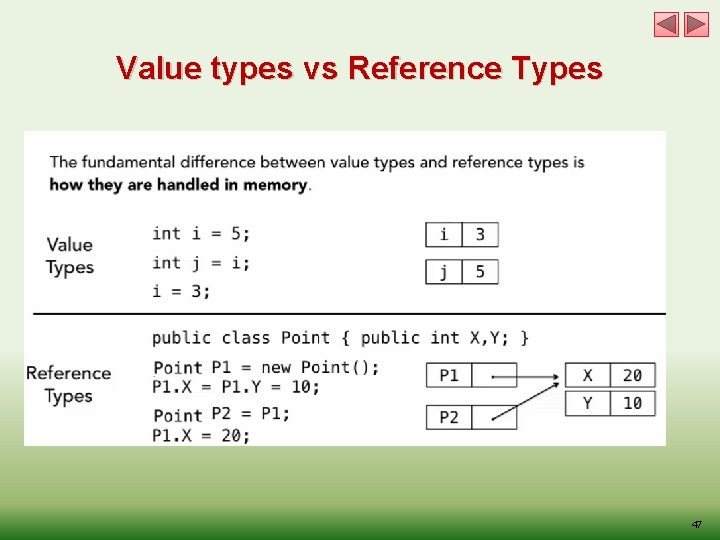
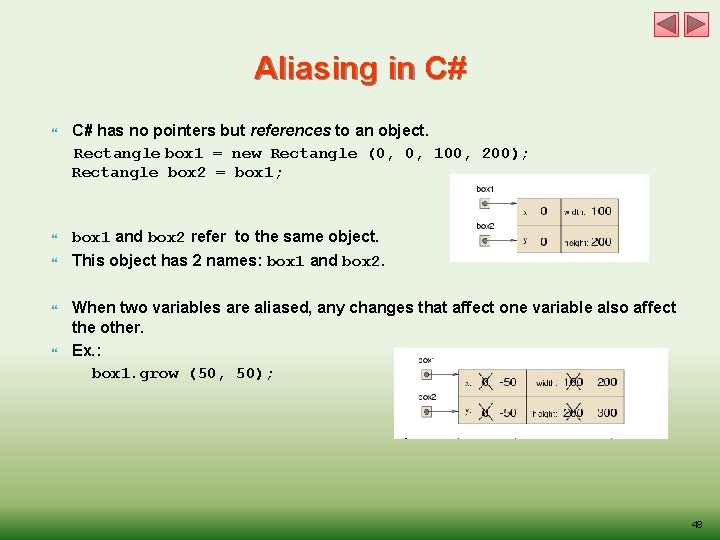
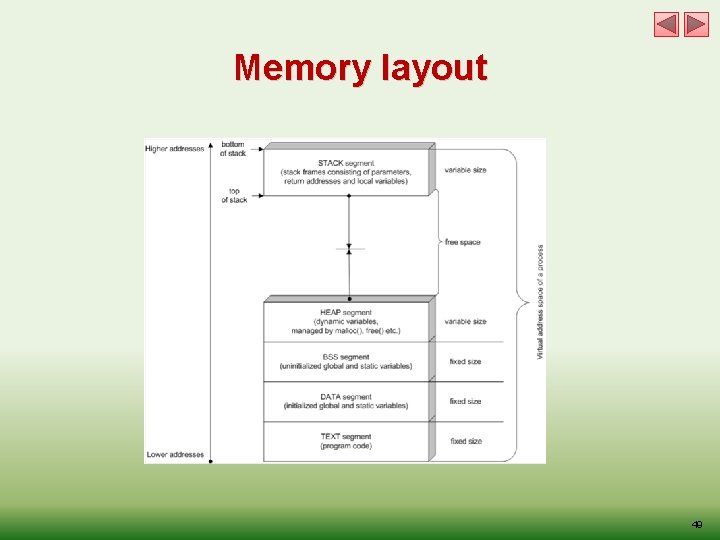
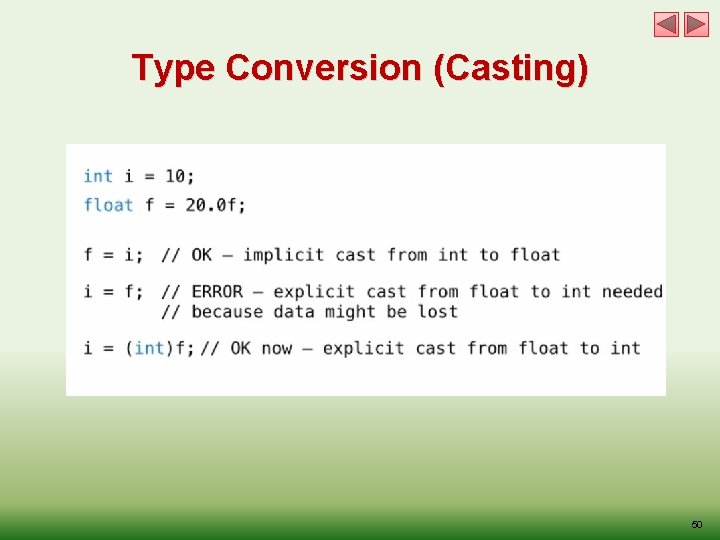
![Conversion Ex: namespace Conversion { class Program { static void Main(string[] args) { int Conversion Ex: namespace Conversion { class Program { static void Main(string[] args) { int](https://slidetodoc.com/presentation_image_h2/877659862373332b75950d0d38dd5fbf/image-51.jpg)
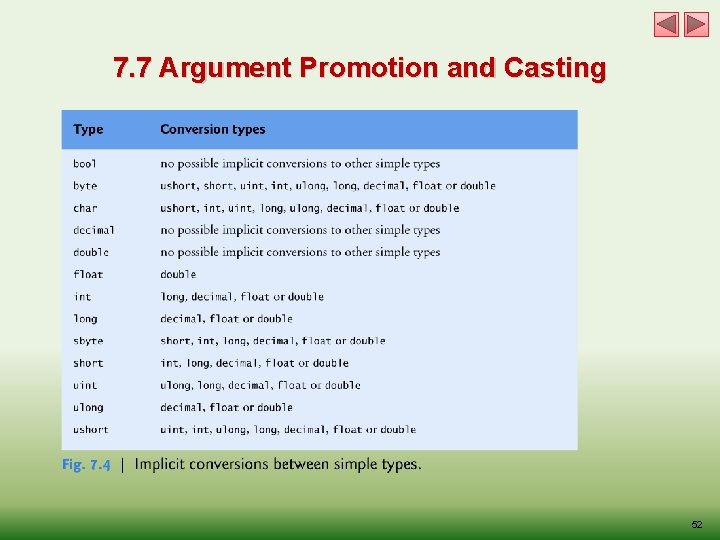
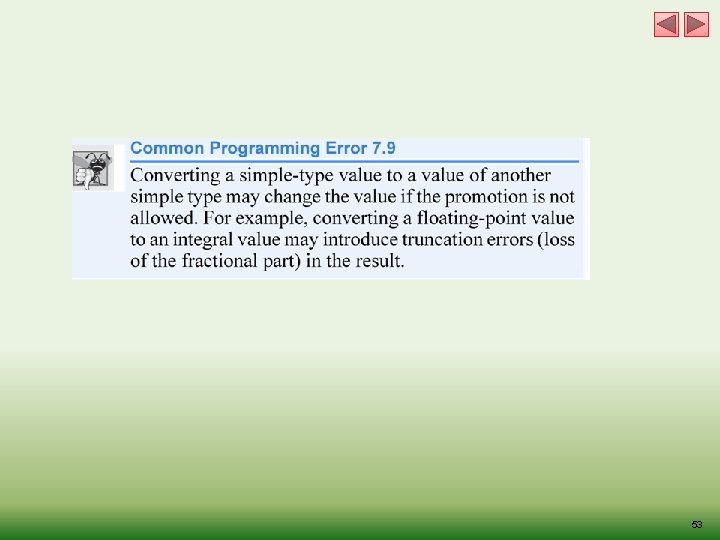
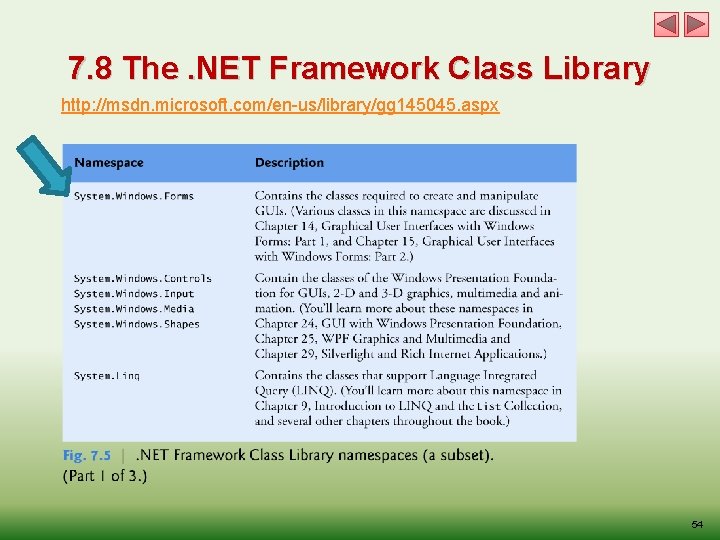
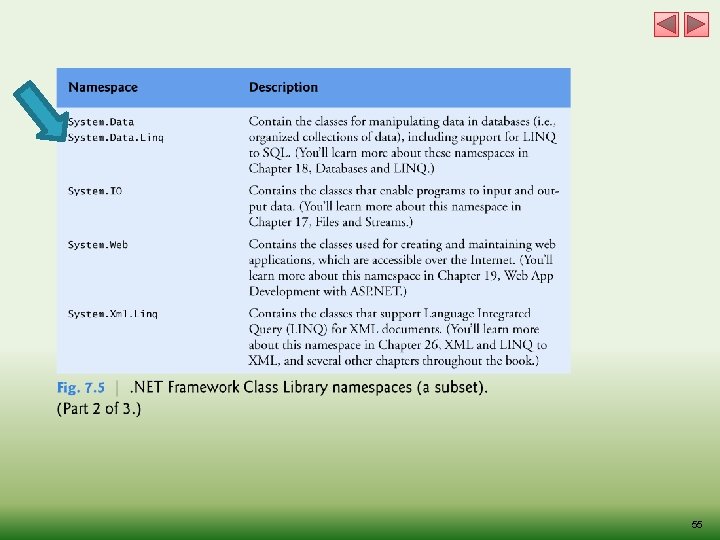
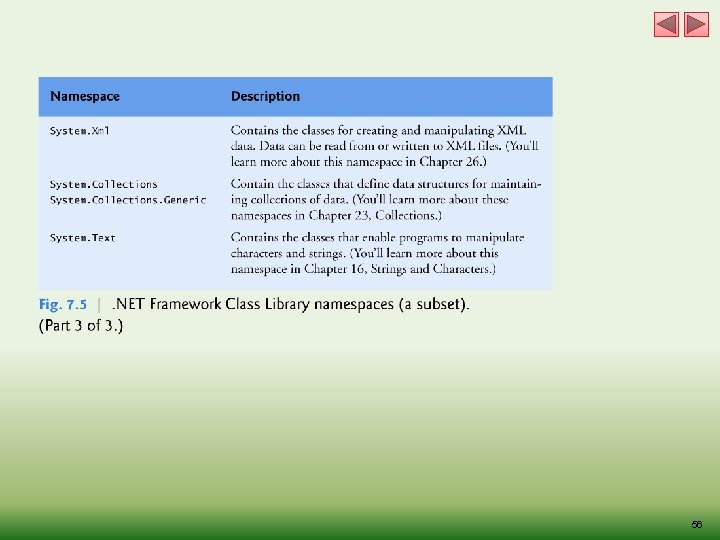
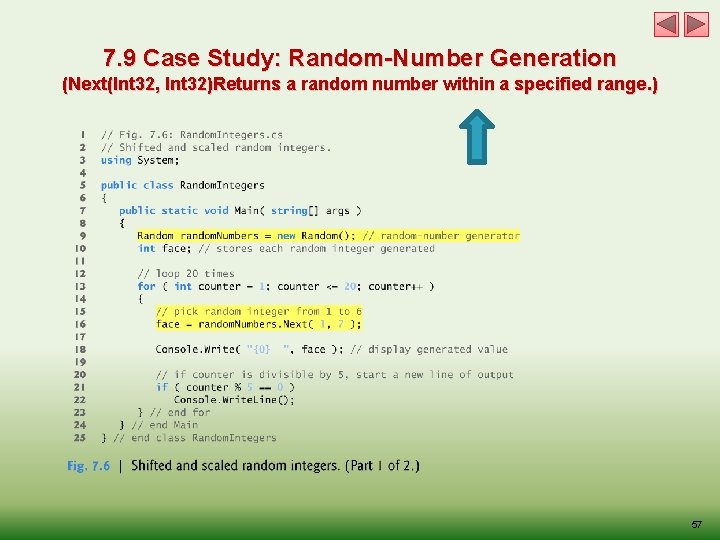
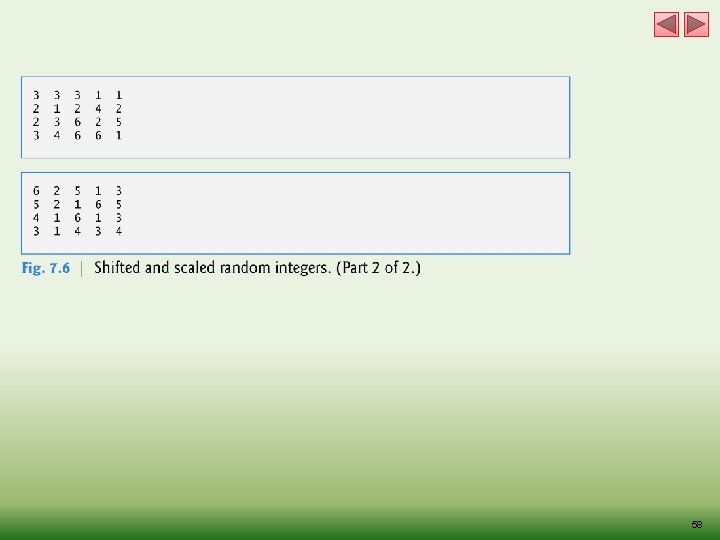
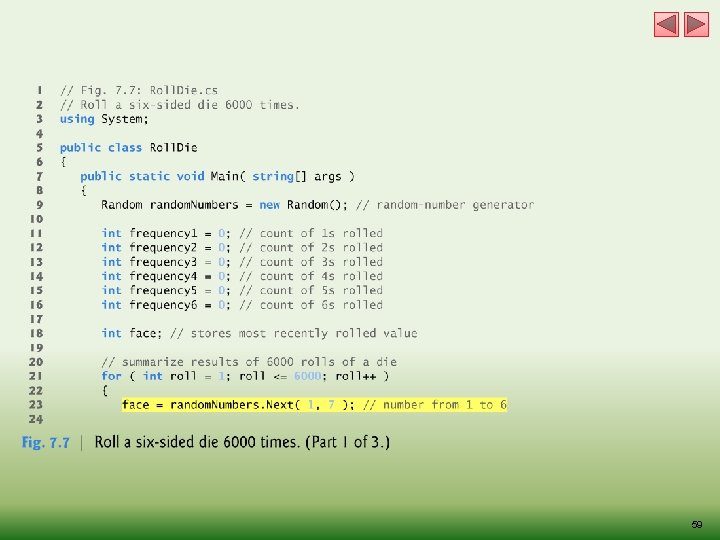
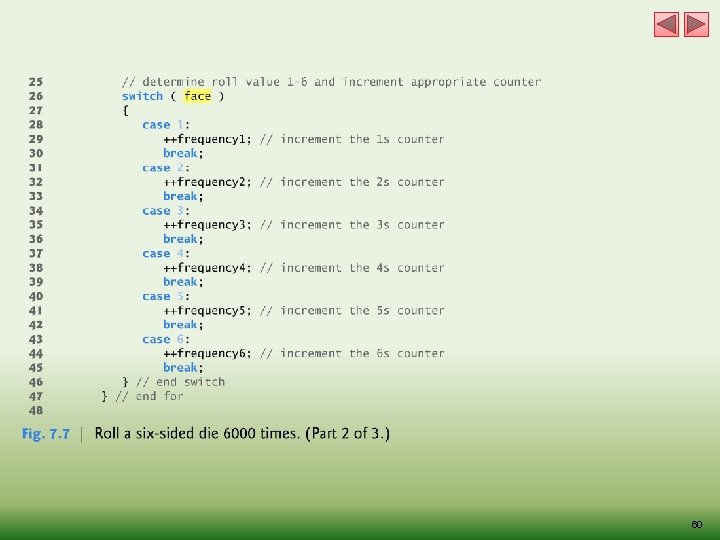
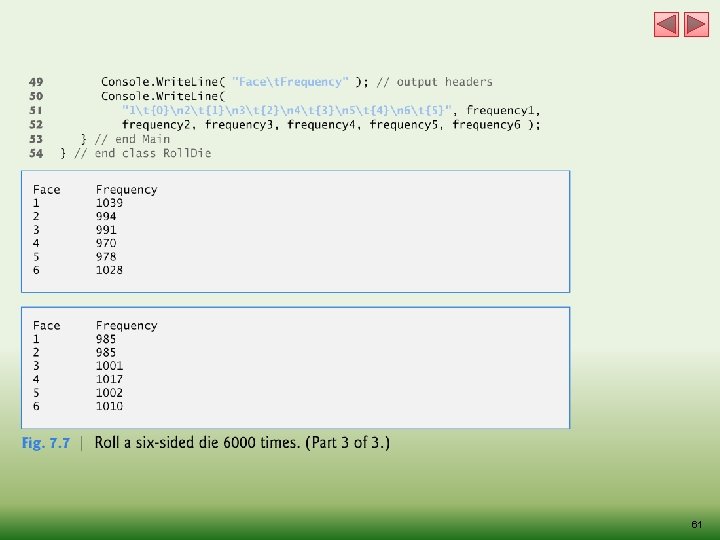
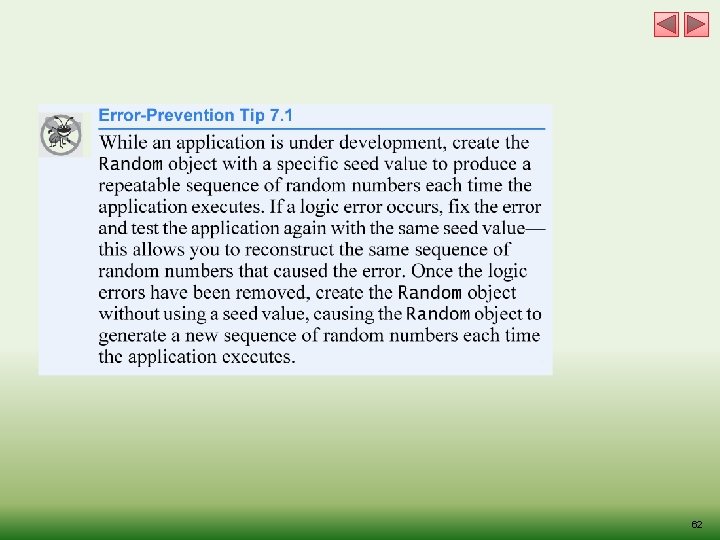
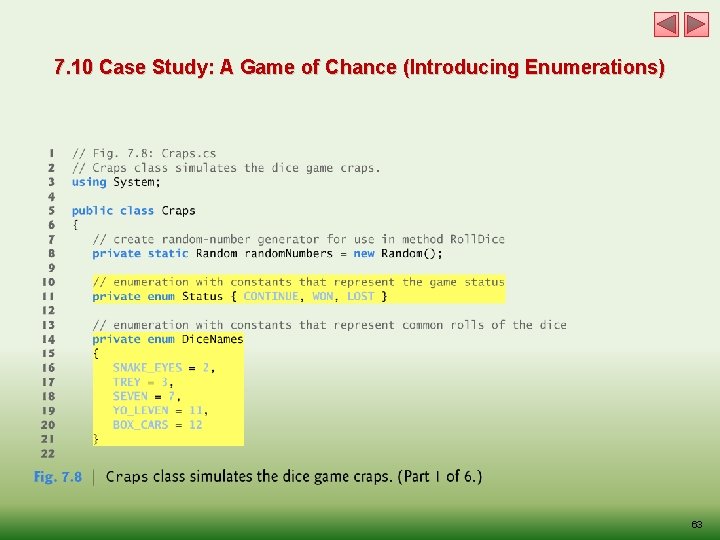
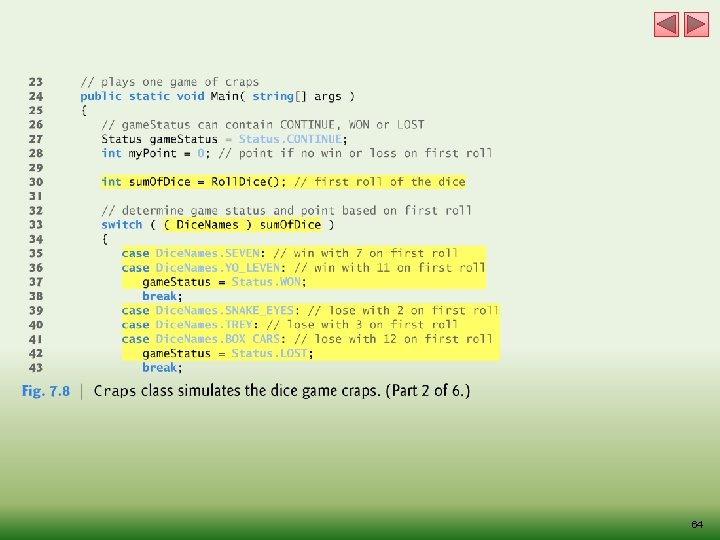
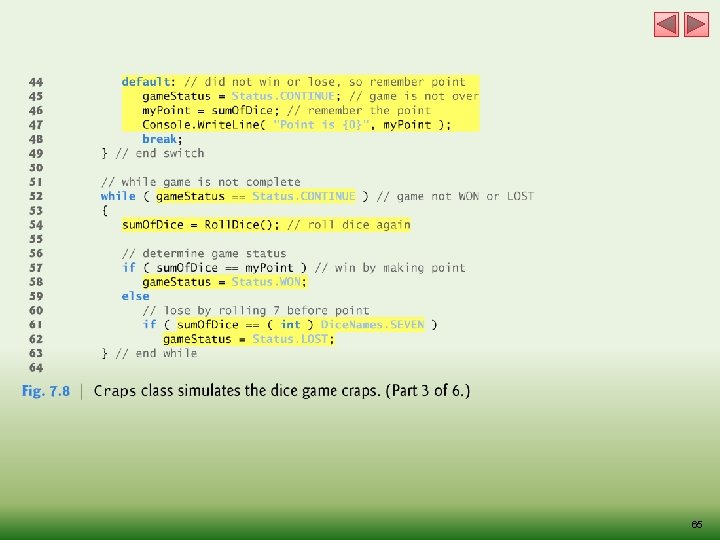
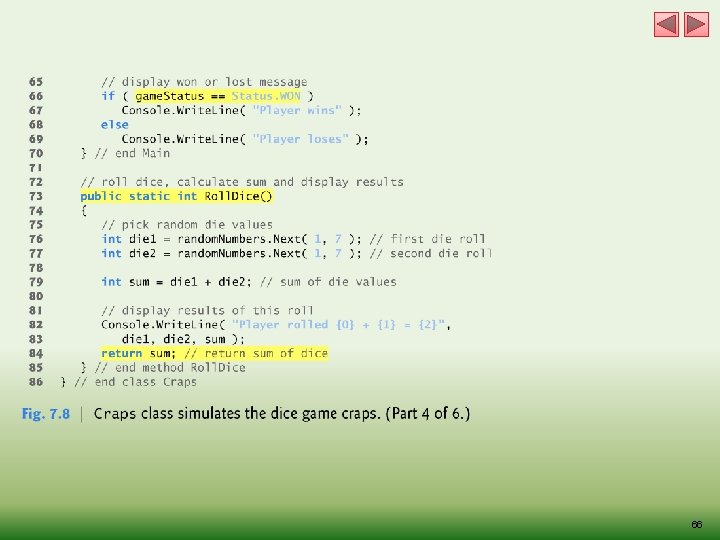
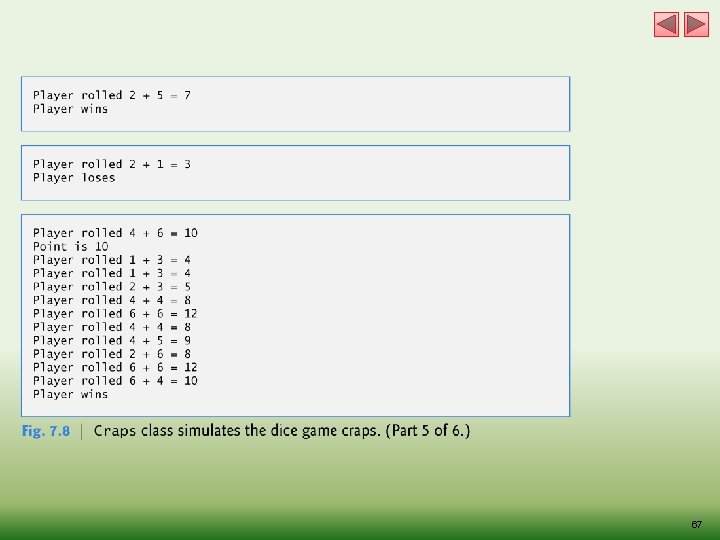
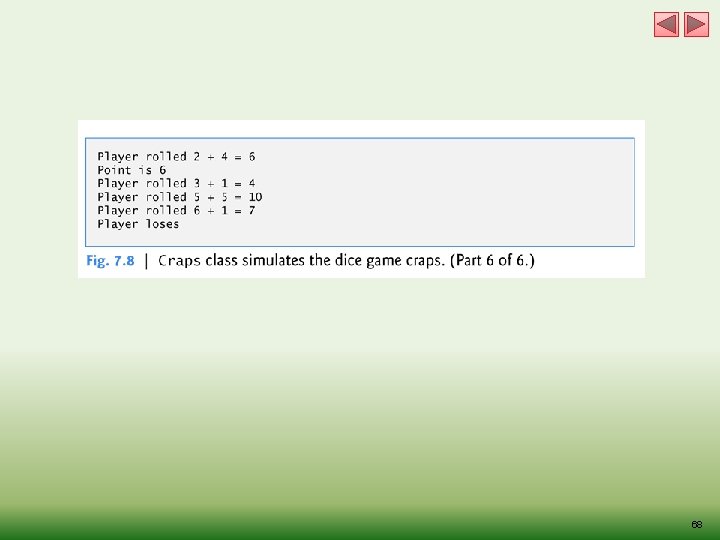
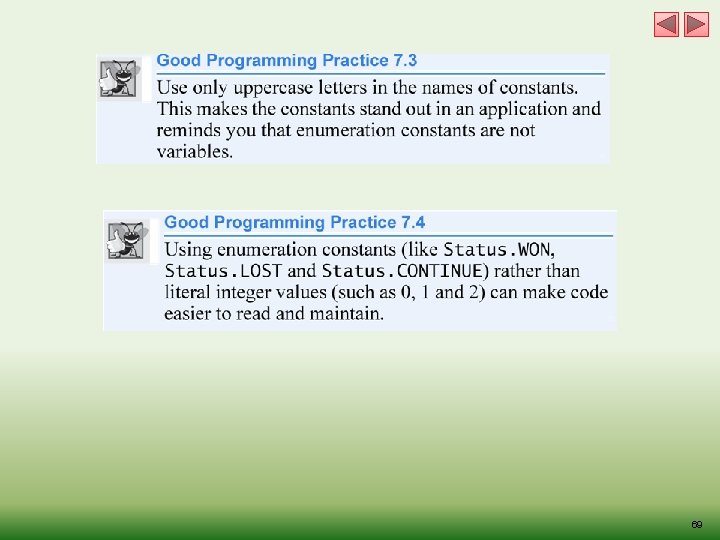
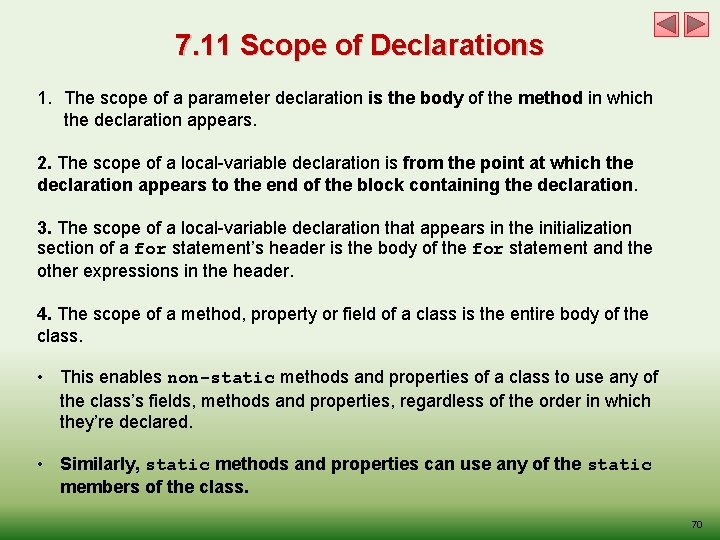
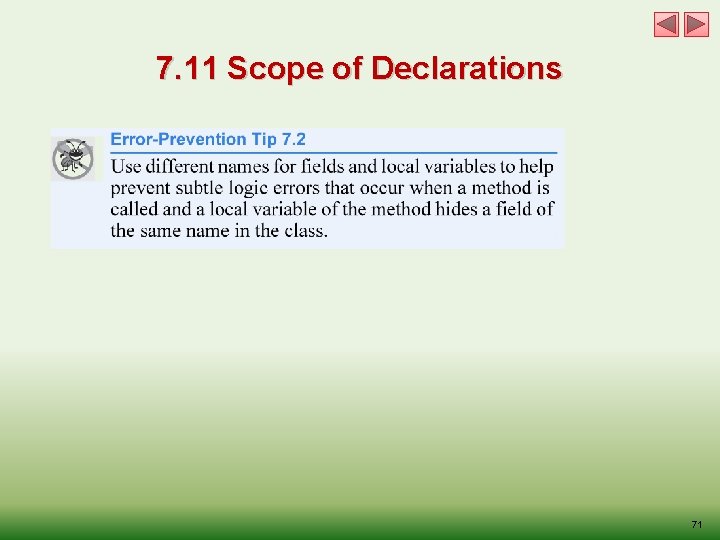
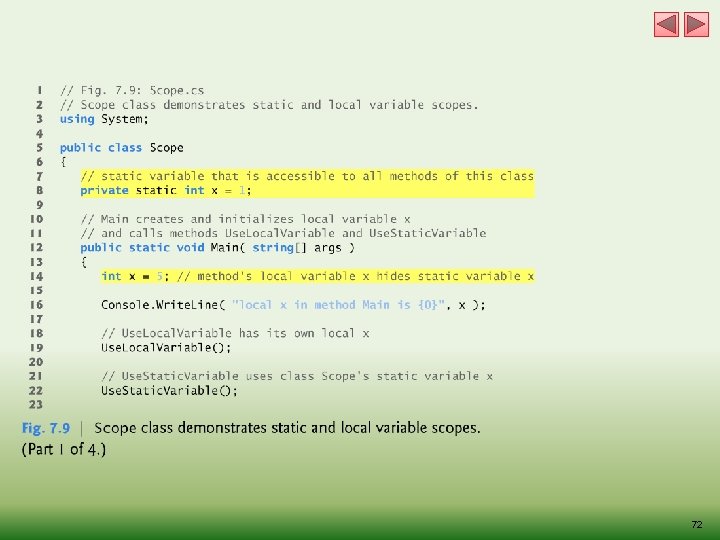
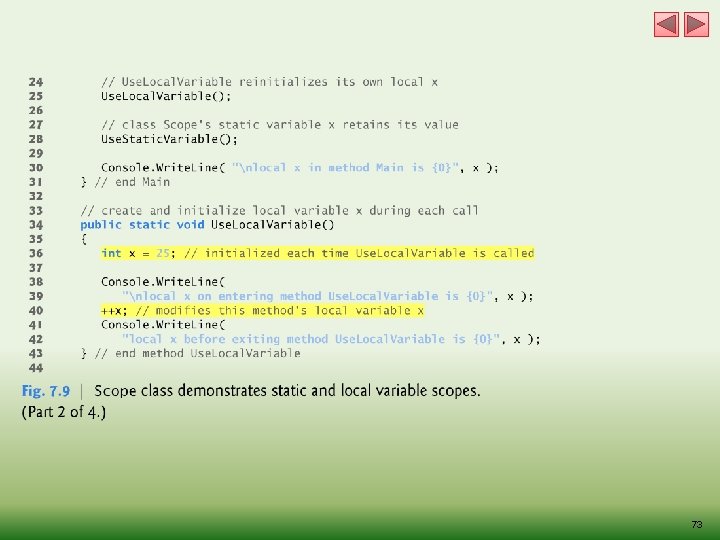
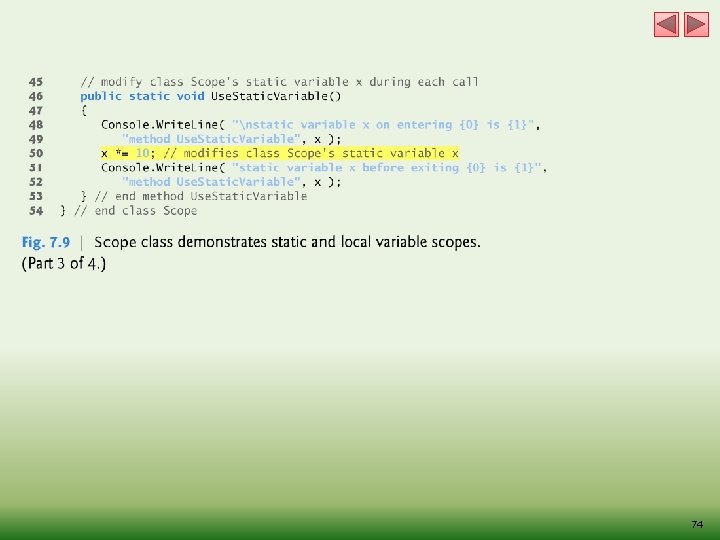
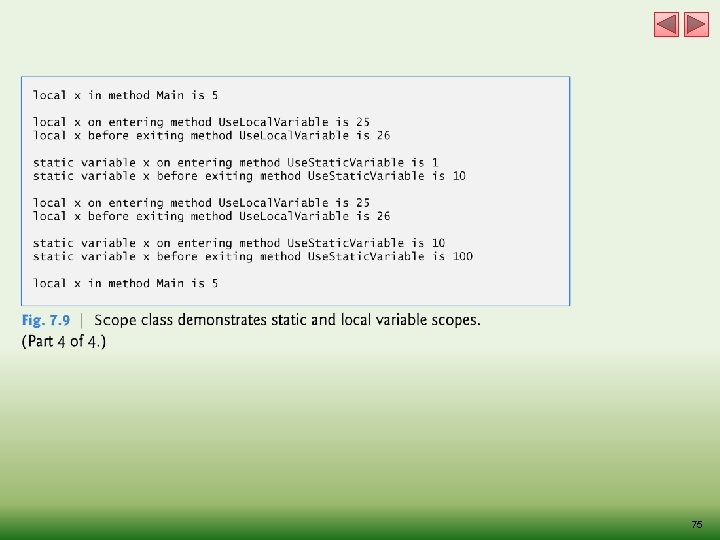
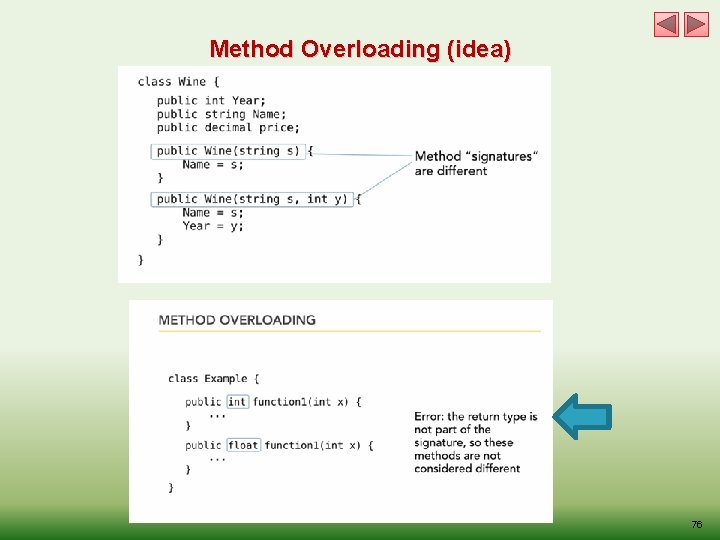
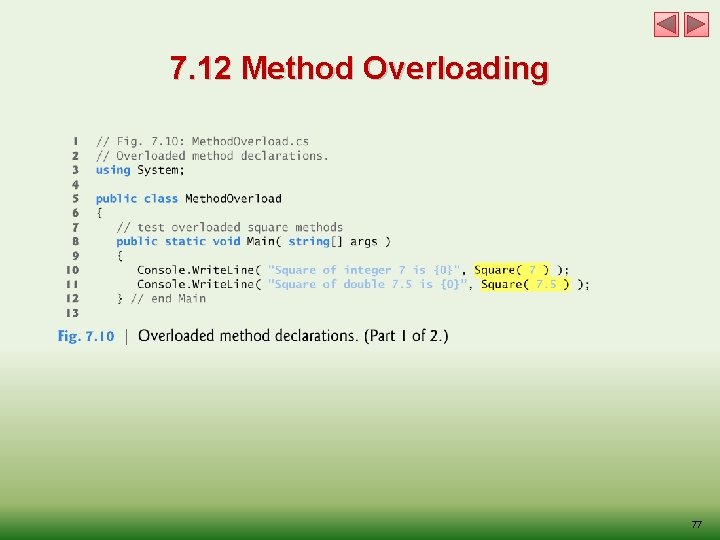
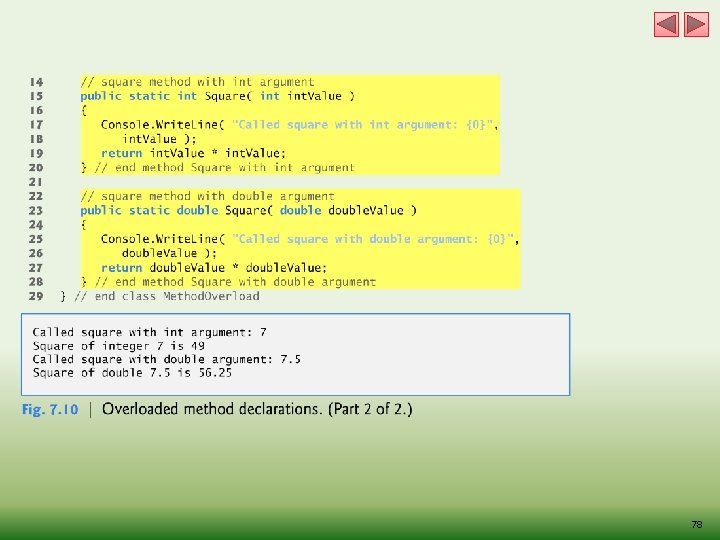
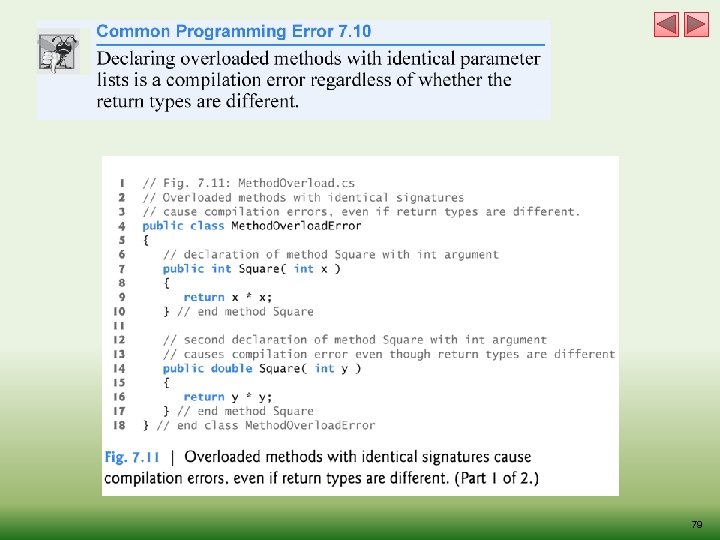
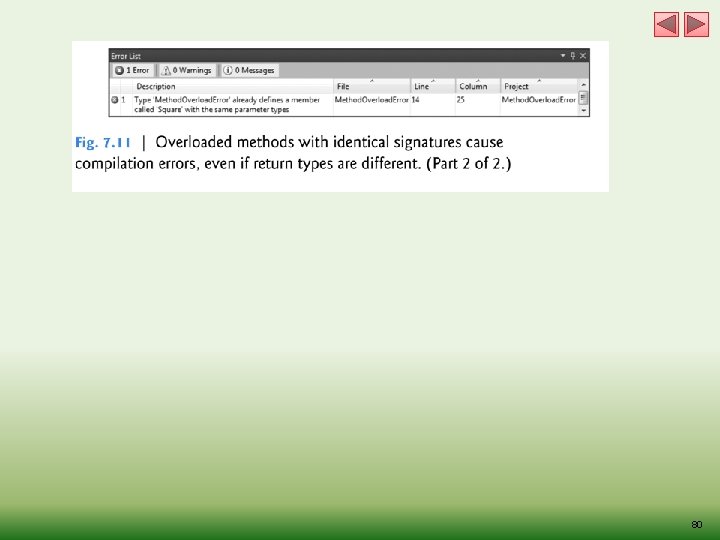
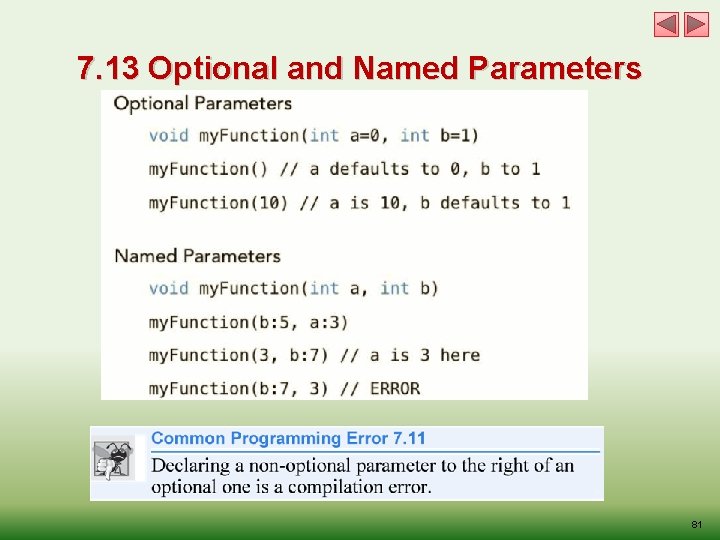
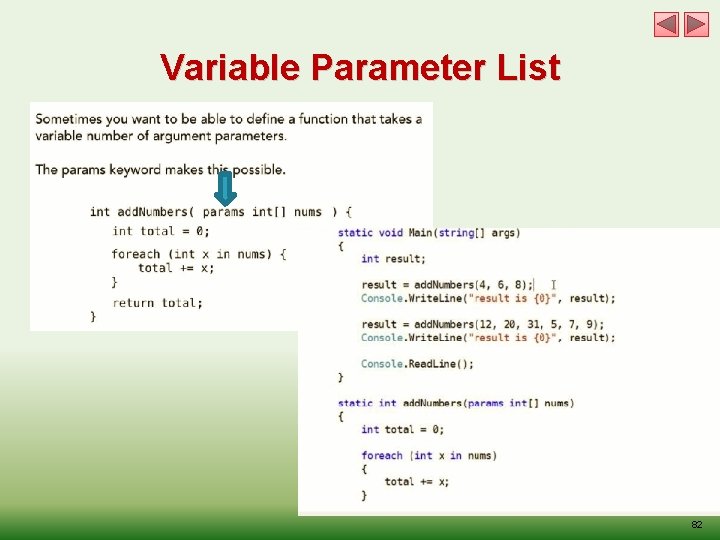
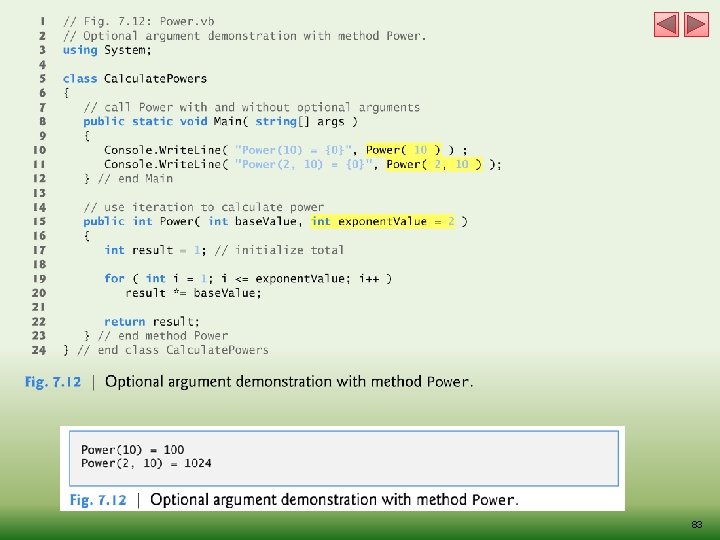
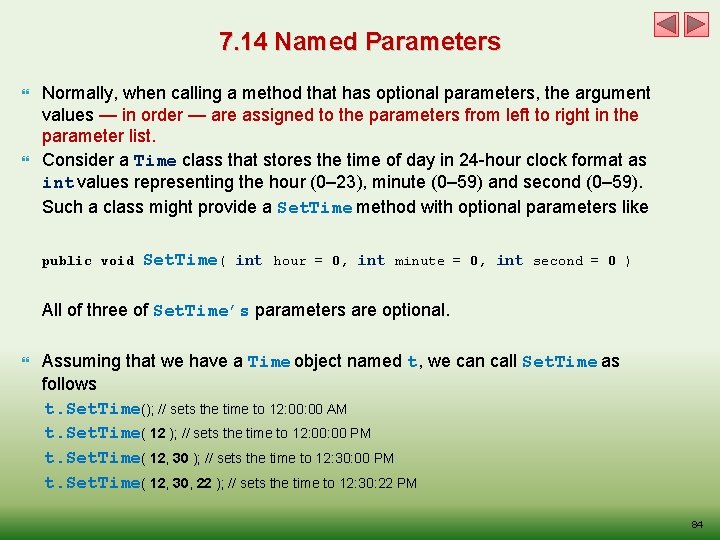
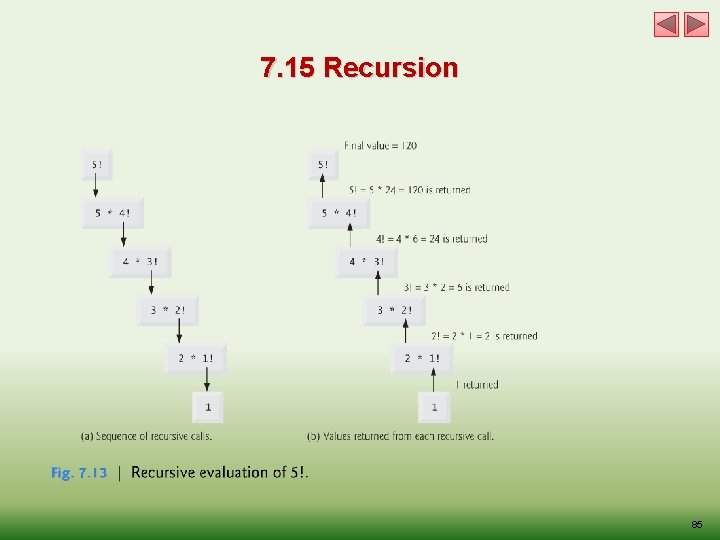
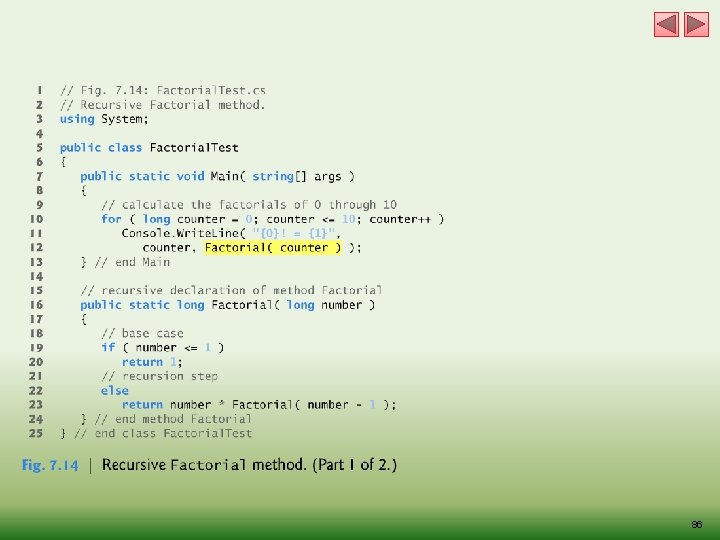
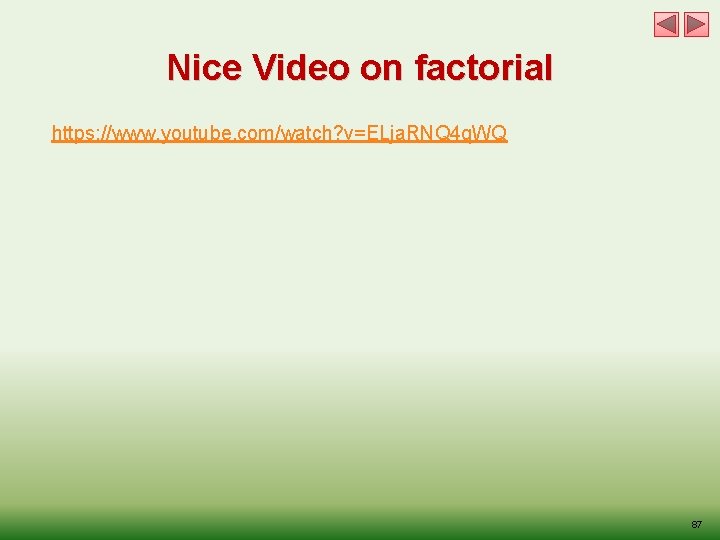
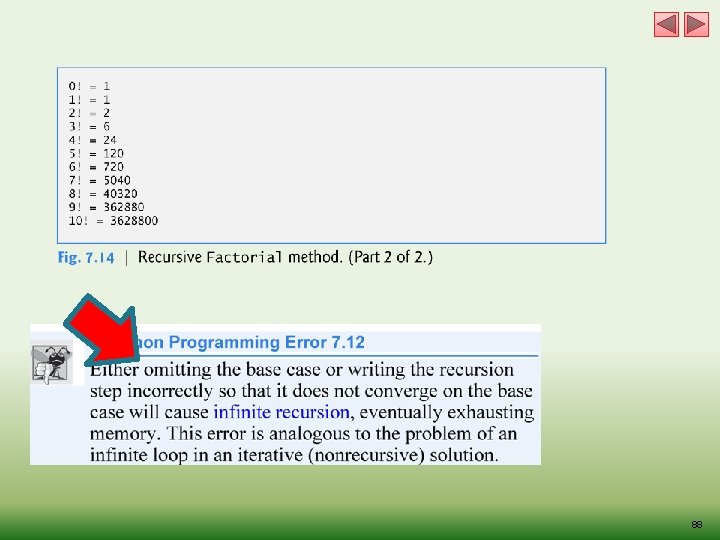
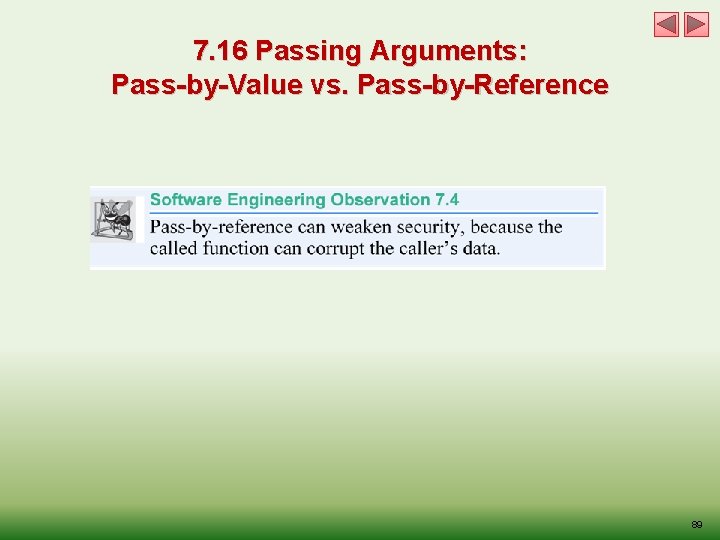
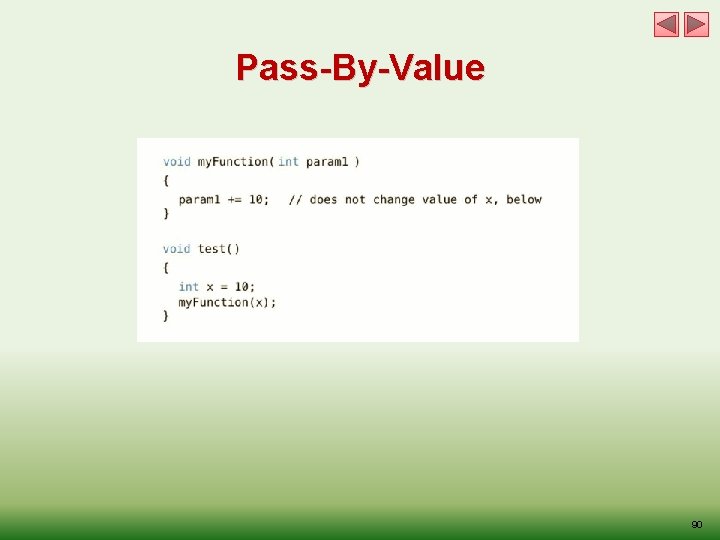
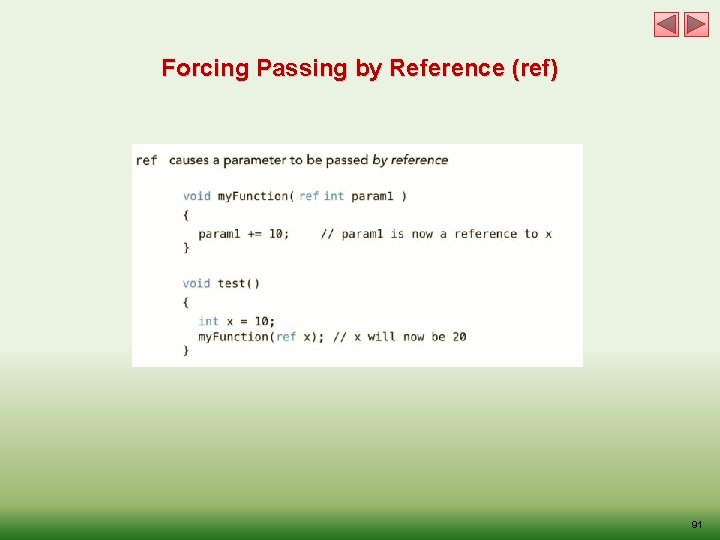
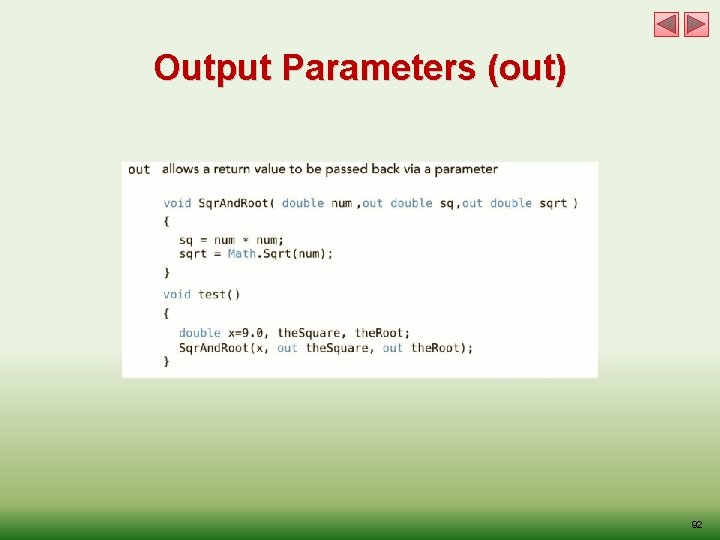
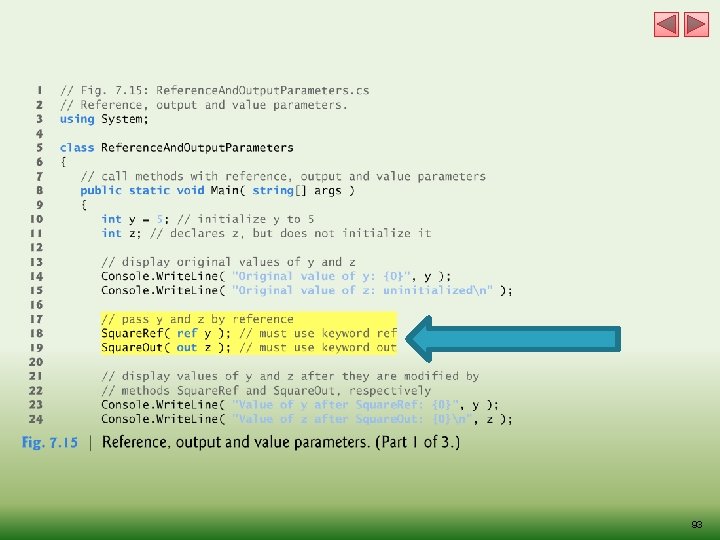
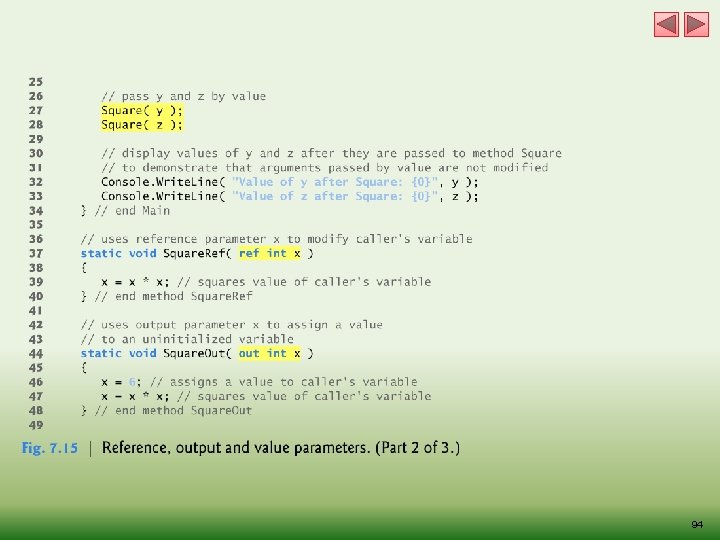
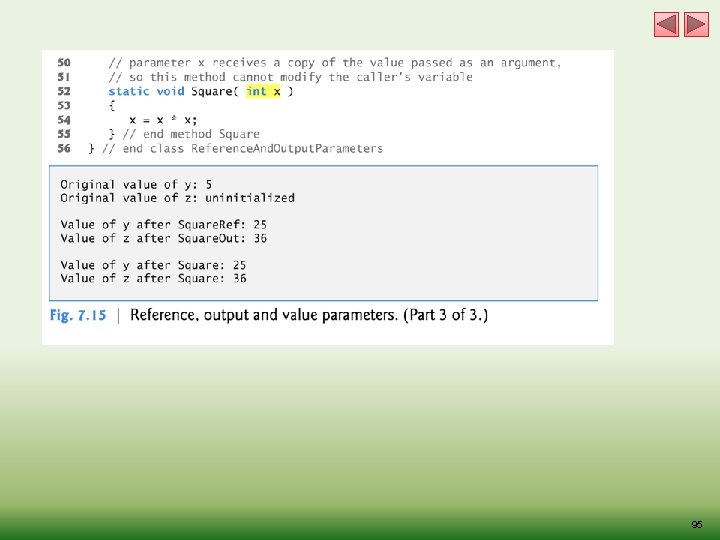
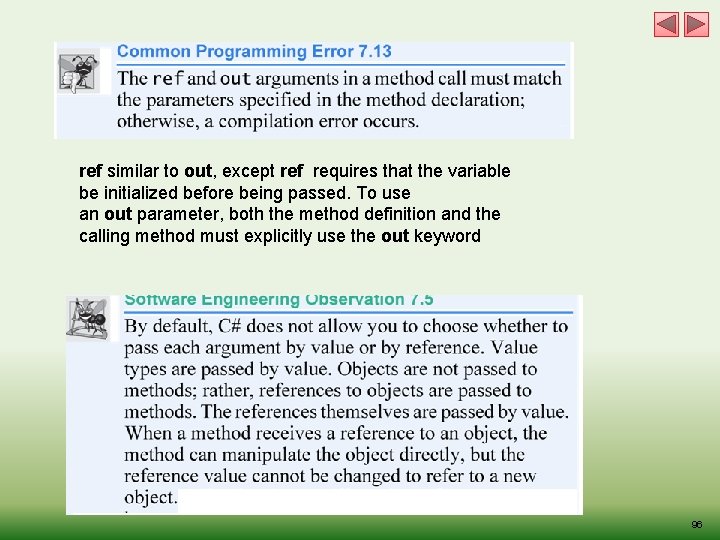
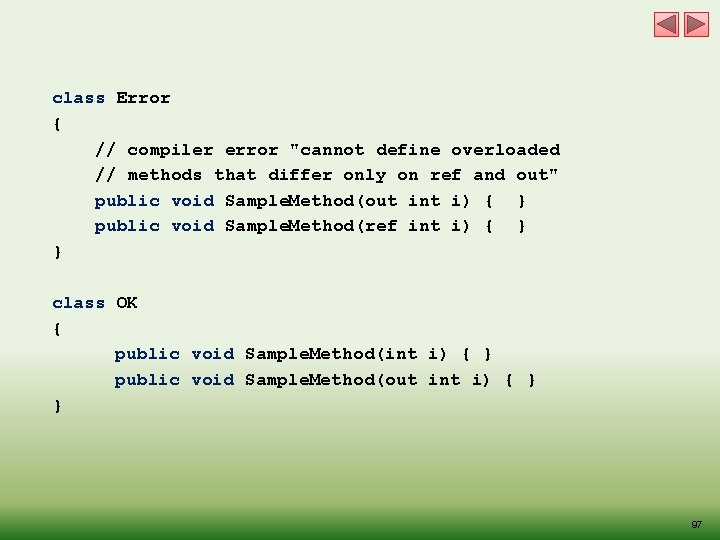
- Slides: 97
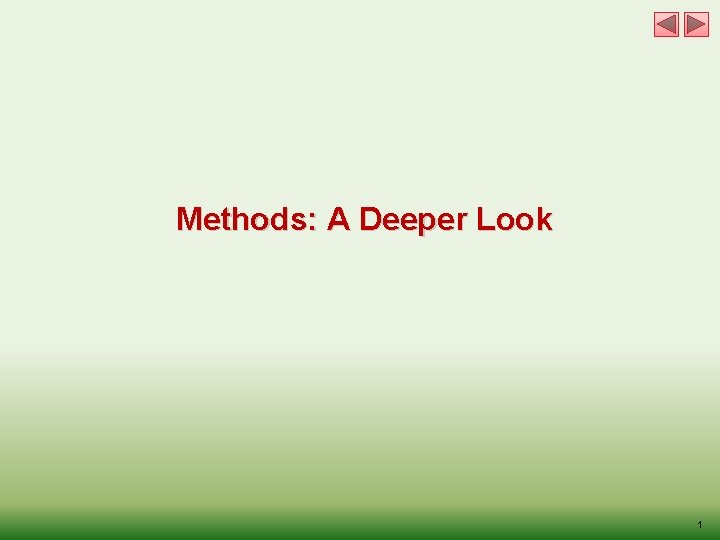
Methods: A Deeper Look 1
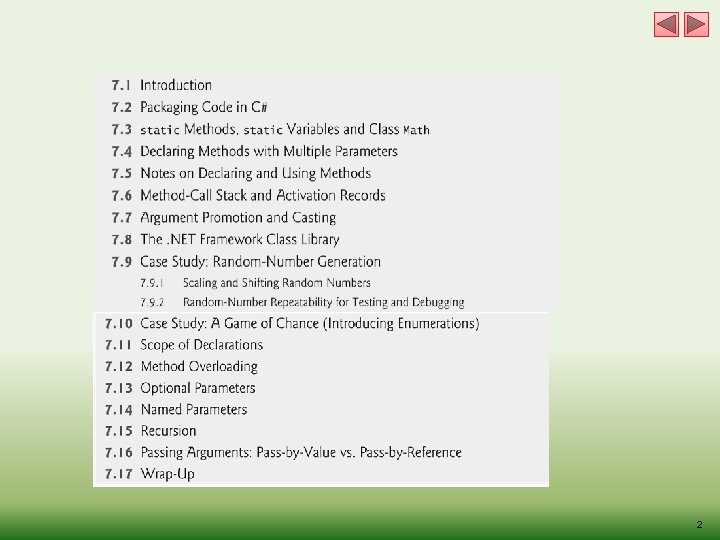
2
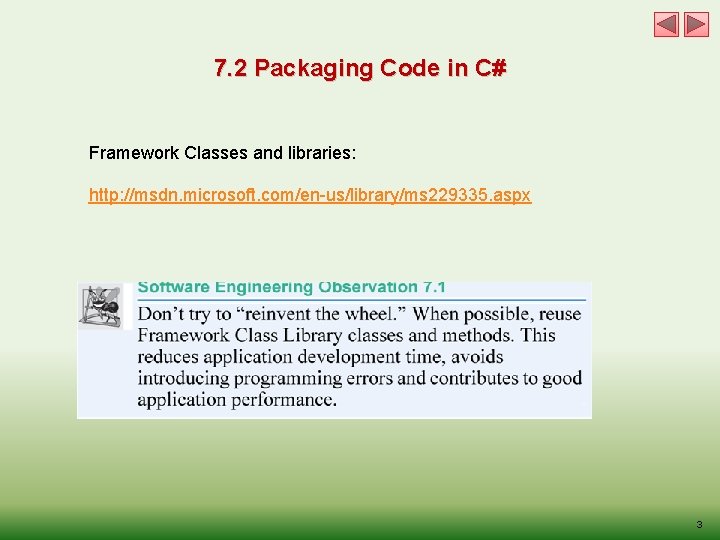
7. 2 Packaging Code in C# Framework Classes and libraries: http: //msdn. microsoft. com/en-us/library/ms 229335. aspx 3
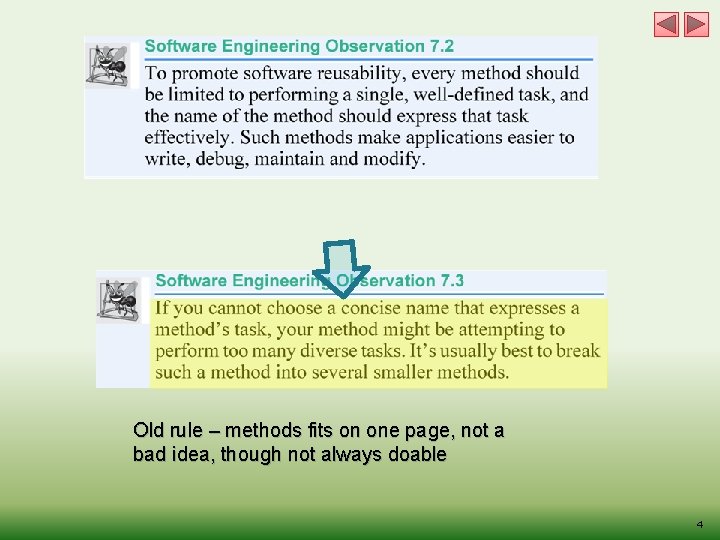
Old rule – methods fits on one page, not a bad idea, though not always doable 4
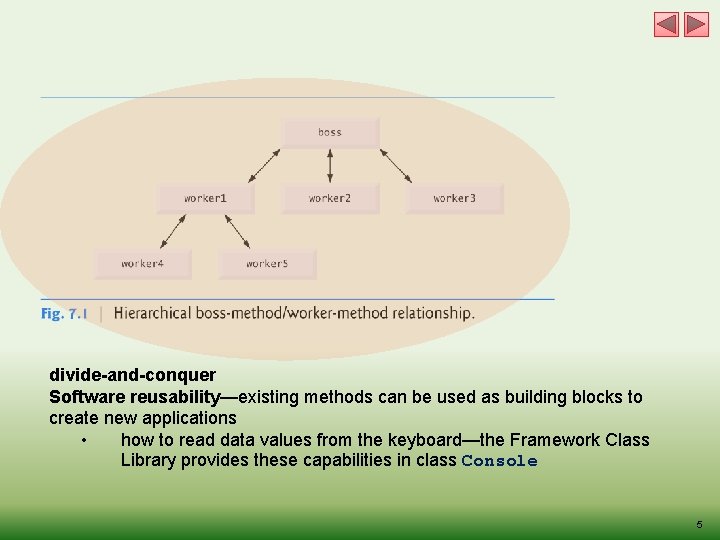
divide-and-conquer Software reusability—existing methods can be used as building blocks to create new applications • how to read data values from the keyboard—the Framework Class Library provides these capabilities in class Console 5
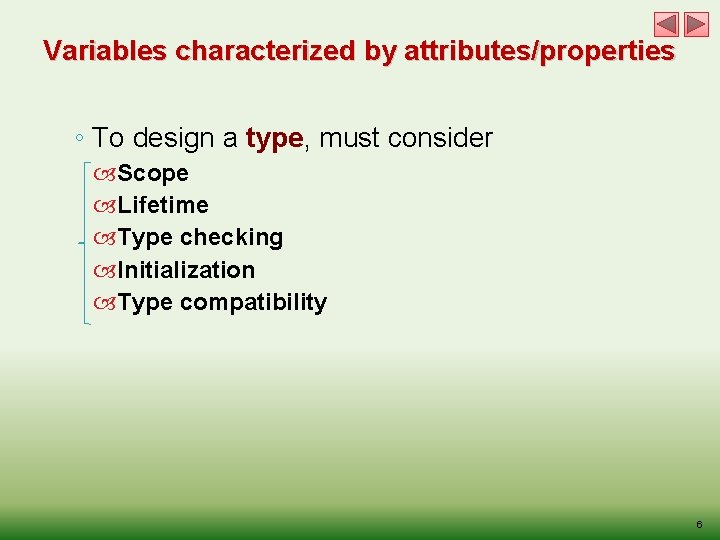
Variables characterized by attributes/properties ◦ To design a type, must consider Scope Lifetime Type checking Initialization Type compatibility 6
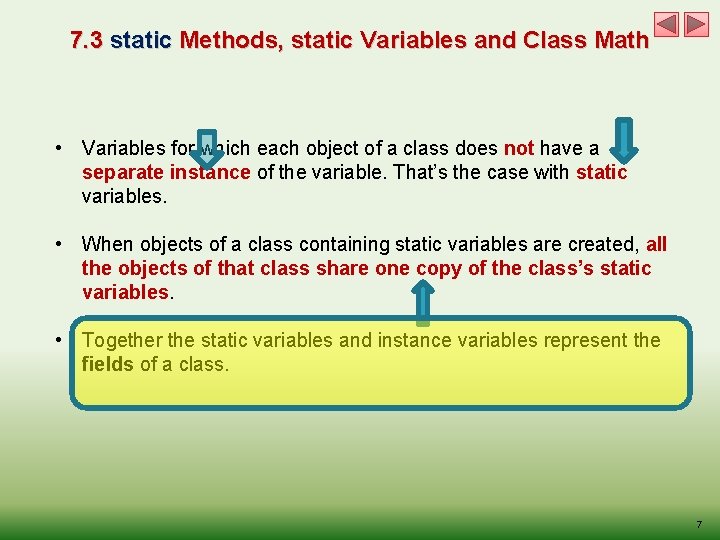
7. 3 static Methods, static Variables and Class Math • Variables for which each object of a class does not have a separate instance of the variable. That’s the case with static variables. • When objects of a class containing static variables are created, all the objects of that class share one copy of the class’s static variables. • Together the static variables and instance variables represent the fields of a class. 7
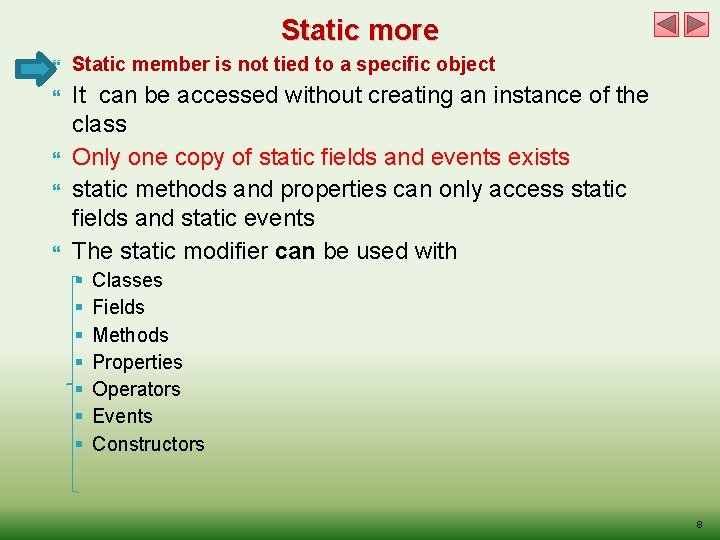
Static more Static member is not tied to a specific object It can be accessed without creating an instance of the class Only one copy of static fields and events exists static methods and properties can only access static fields and static events The static modifier can be used with § § § § Classes Fields Methods Properties Operators Events Constructors 8
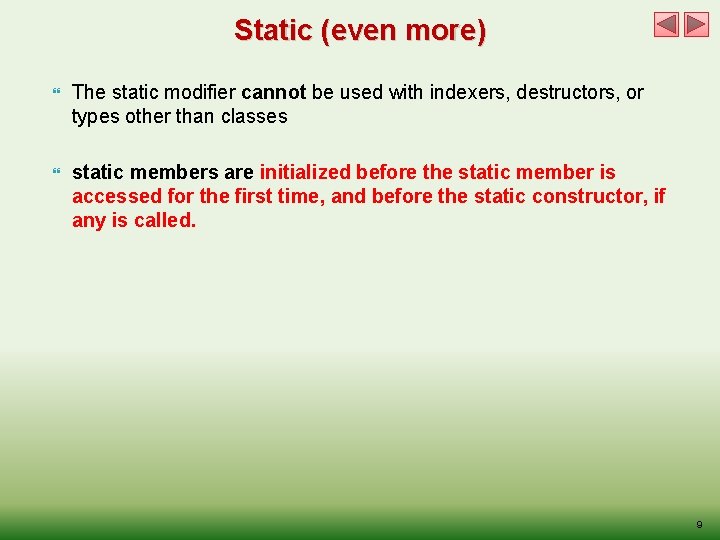
Static (even more) The static modifier cannot be used with indexers, destructors, or types other than classes static members are initialized before the static member is accessed for the first time, and before the static constructor, if any is called. 9
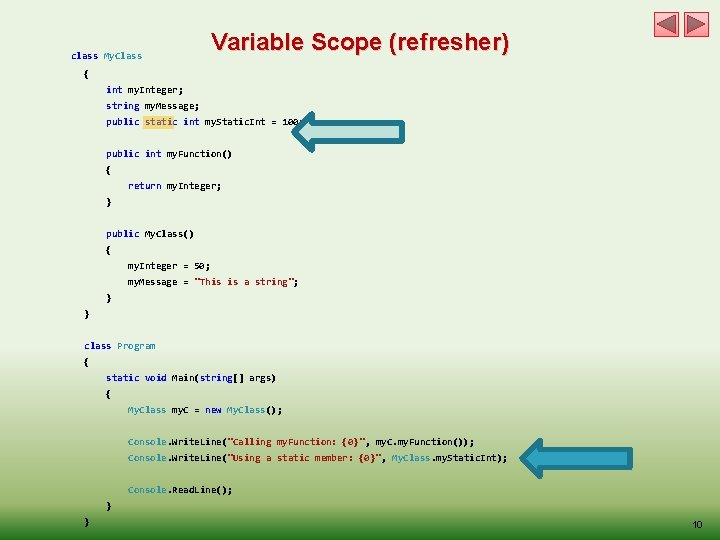
class My. Class Variable Scope (refresher) { int my. Integer; string my. Message; public static int my. Static. Int = 100; public int my. Function() { return my. Integer; } public My. Class() { my. Integer = 50; my. Message = "This is a string"; } } class Program { static void Main(string[] args) { My. Class my. C = new My. Class(); Console. Write. Line("Calling my. Function: {0}", my. C. my. Function()); Console. Write. Line("Using a static member: {0}", My. Class. my. Static. Int); Console. Read. Line(); } } 10
![Why Is Method Main Declared static public static void Mainstring args Comm andli ne Why Is Method Main Declared static? public static void Main(string args[]) Comm and-li ne](https://slidetodoc.com/presentation_image_h2/877659862373332b75950d0d38dd5fbf/image-11.jpg)
Why Is Method Main Declared static? public static void Main(string args[]) Comm and-li ne During application startup, when no objects of the class have been created, the Main method must be called to begin program execution. The Main method is sometimes called the application’s entry point. Declaring Main as static allows the execution environment to invoke Main without creating an instance of the class. Any class can have Main (sometimes used for testing) ◦ Project > [Project. Name] Properties. . . select the class containing the Main method that should be the entry point from the Startup object list box 11
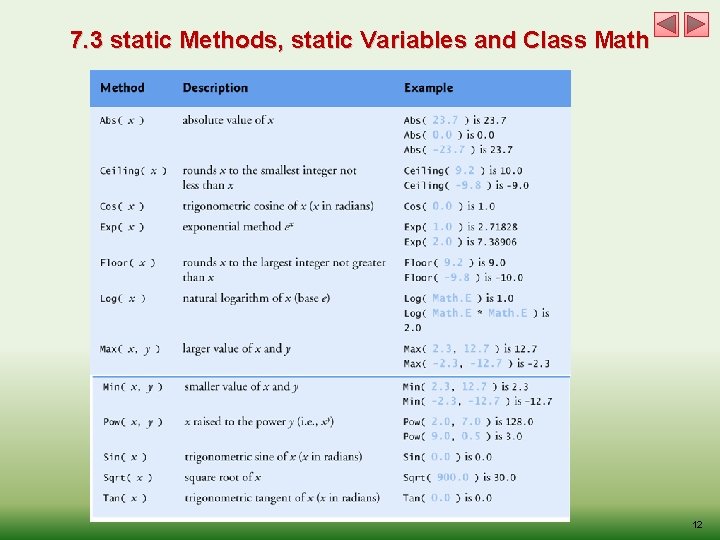
7. 3 static Methods, static Variables and Class Math 12
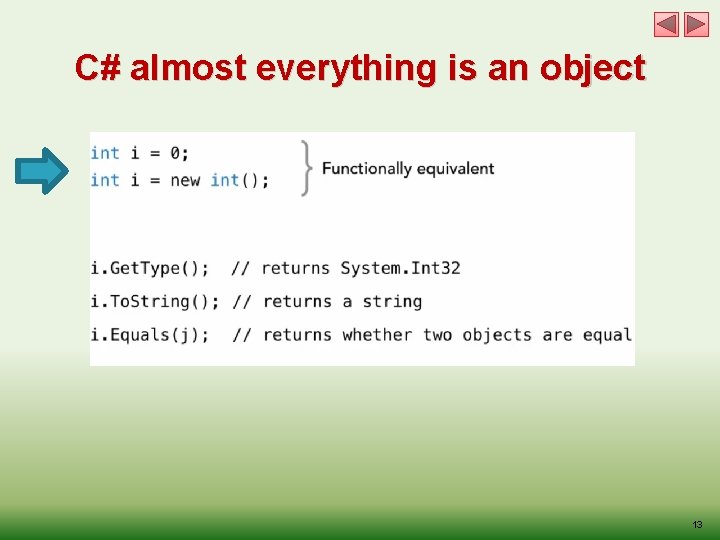
C# almost everything is an object 13
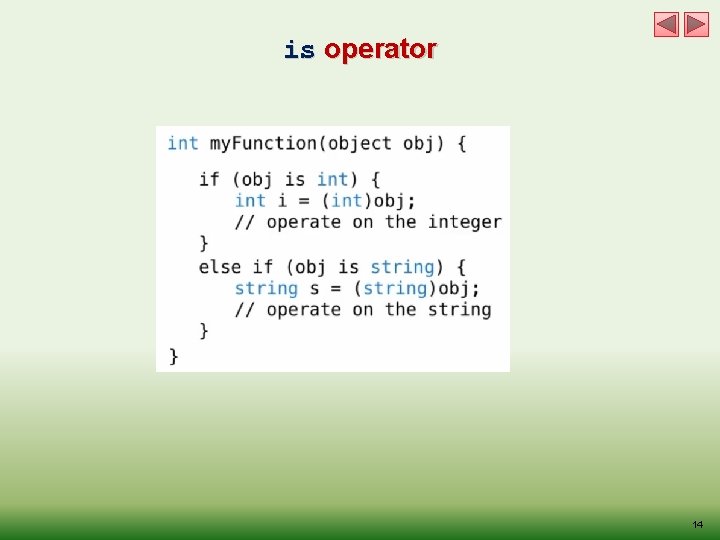
is operator 14
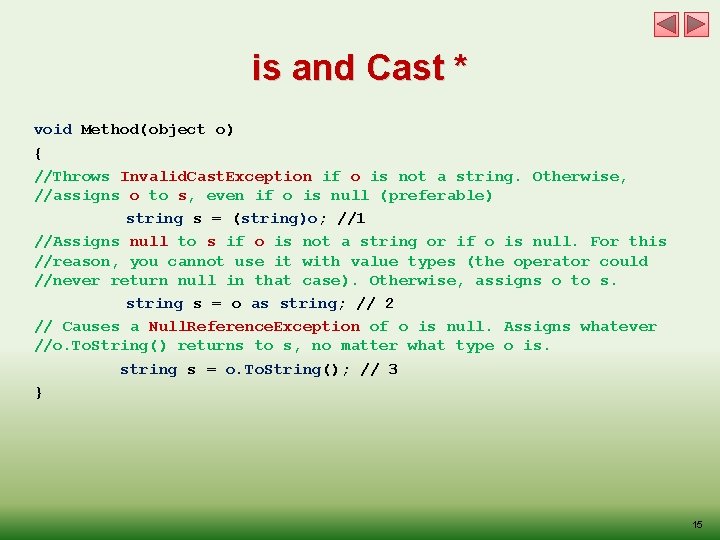
is and Cast * void Method(object o) { //Throws Invalid. Cast. Exception if o is not a string. Otherwise, //assigns o to s, even if o is null (preferable) string s = (string)o; //1 //Assigns null to s if o is not a string or if o is null. For this //reason, you cannot use it with value types (the operator could //never return null in that case). Otherwise, assigns o to s. string s = o as string; // 2 // Causes a Null. Reference. Exception of o is null. Assigns whatever //o. To. String() returns to s, no matter what type o is. string s = o. To. String(); // 3 } 15
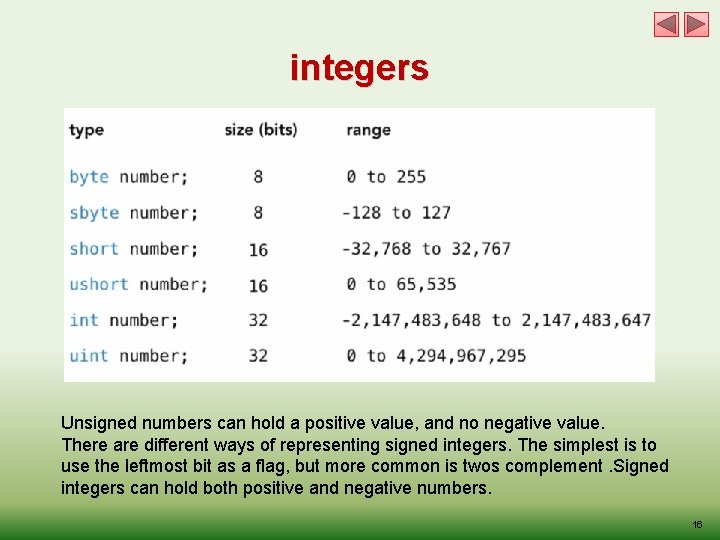
integers Unsigned numbers can hold a positive value, and no negative value. There are different ways of representing signed integers. The simplest is to use the leftmost bit as a flag, but more common is twos complement. Signed integers can hold both positive and negative numbers. 16
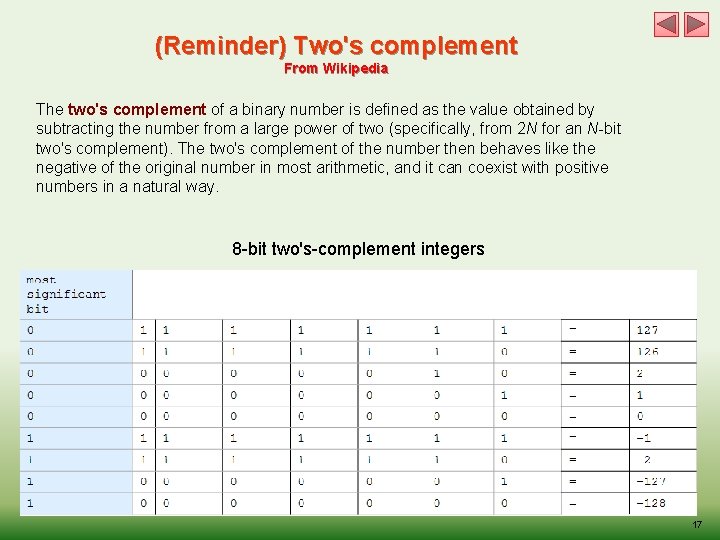
(Reminder) Two's complement From Wikipedia The two's complement of a binary number is defined as the value obtained by subtracting the number from a large power of two (specifically, from 2 N for an N-bit two's complement). The two's complement of the number then behaves like the negative of the original number in most arithmetic, and it can coexist with positive numbers in a natural way. 8 -bit two's-complement integers 17
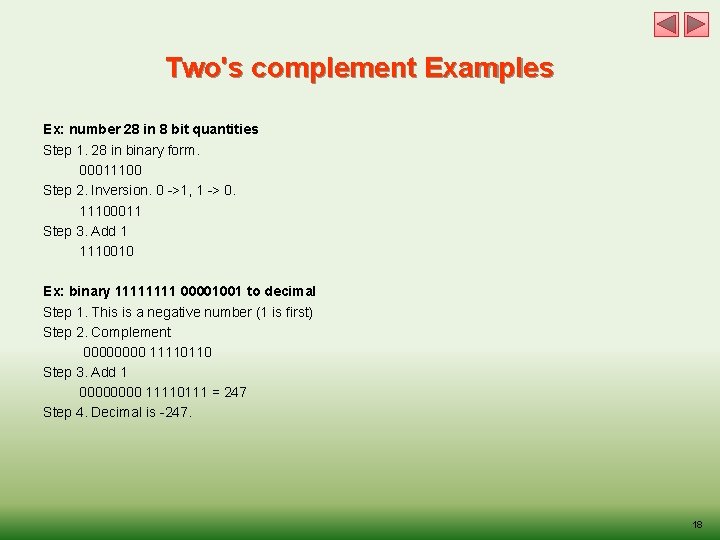
Two's complement Examples Ex: number 28 in 8 bit quantities Step 1. 28 in binary form. 00011100 Step 2. Inversion. 0 ->1, 1 -> 0. 11100011 Step 3. Add 1 1110010 Ex: binary 1111 00001001 to decimal Step 1. This is a negative number (1 is first) Step 2. Complement 0000 11110110 Step 3. Add 1 0000 11110111 = 247 Step 4. Decimal is -247. 18
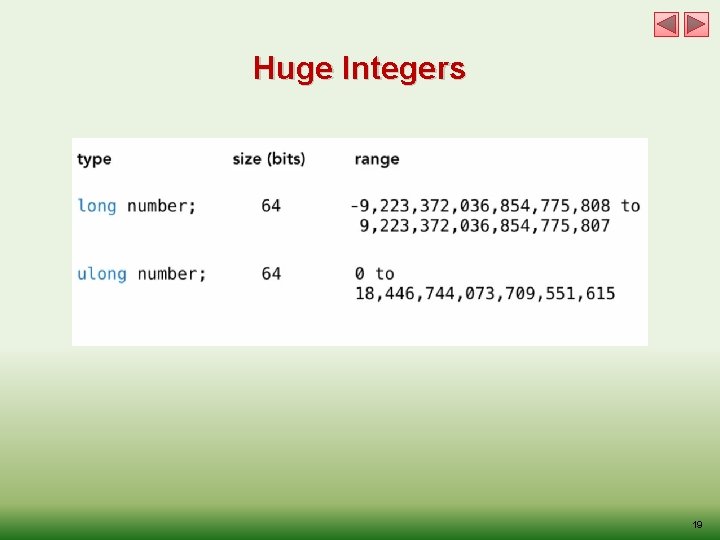
Huge Integers 19
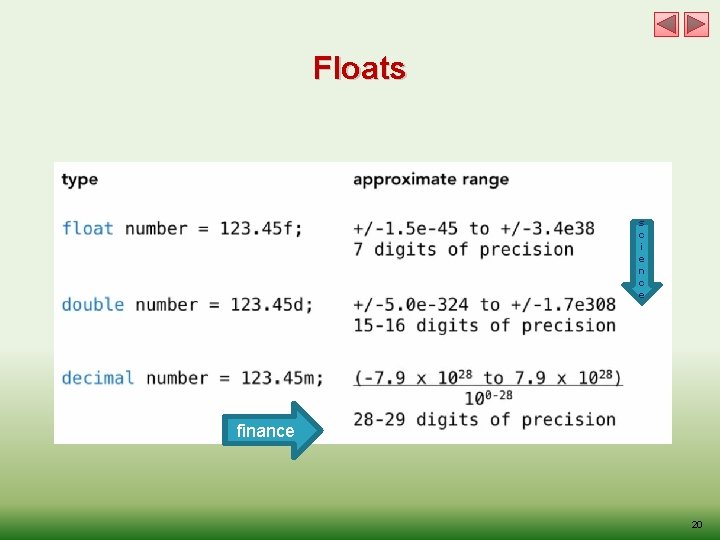
Floats s c i e n c e finance 20
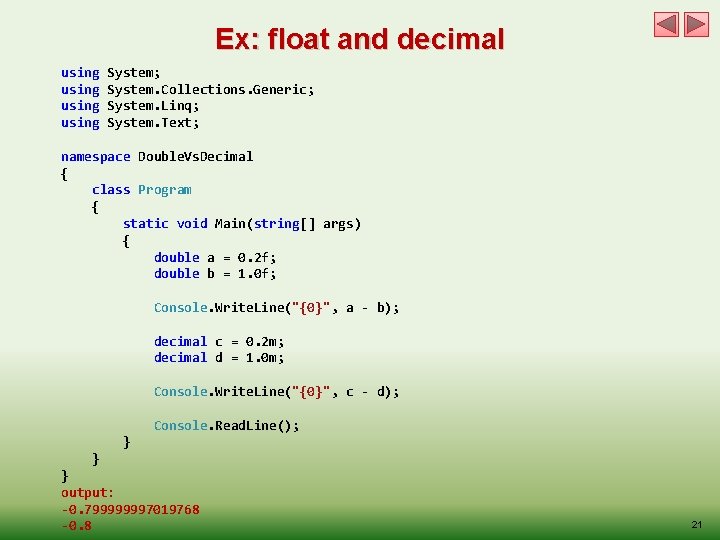
Ex: float and decimal using System; System. Collections. Generic; System. Linq; System. Text; namespace Double. Vs. Decimal { class Program { static void Main(string[] args) { double a = 0. 2 f; double b = 1. 0 f; Console. Write. Line("{0}", a - b); decimal c = 0. 2 m; decimal d = 1. 0 m; Console. Write. Line("{0}", c - d); Console. Read. Line(); } } } output: -0. 799999997019768 -0. 8 21
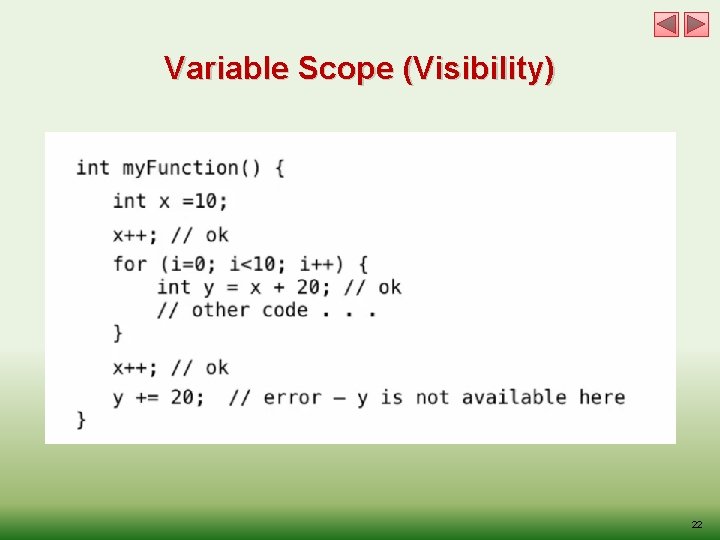
Variable Scope (Visibility) 22
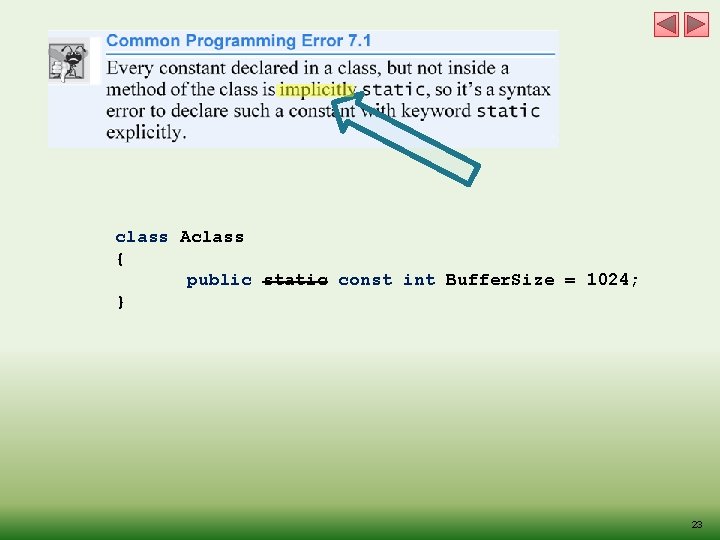
class Aclass { public static const int Buffer. Size = 1024; } 23
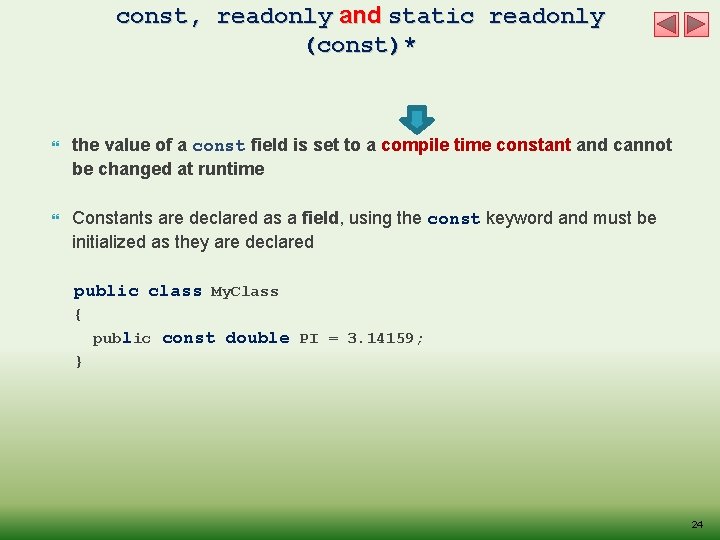
const, readonly and static readonly (const)* the value of a const field is set to a compile time constant and cannot be changed at runtime Constants are declared as a field, using the const keyword and must be initialized as they are declared public class My. Class { public const double PI = 3. 14159; } 24
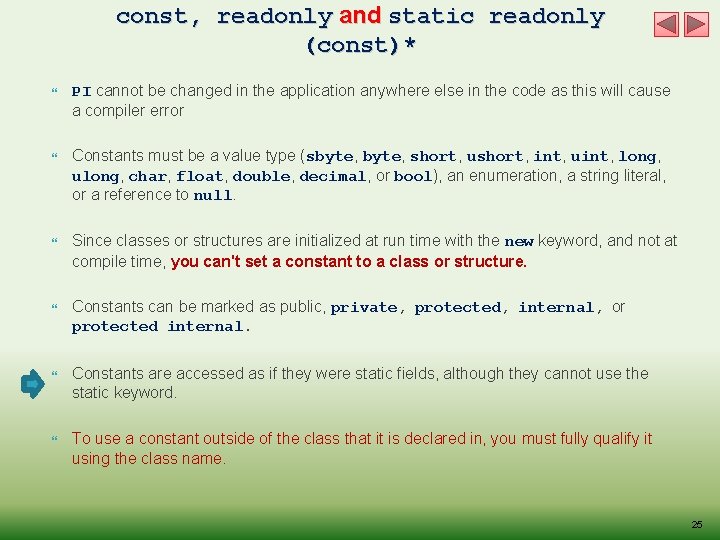
const, readonly and static readonly (const)* PI cannot be changed in the application anywhere else in the code as this will cause a compiler error Constants must be a value type (sbyte, short, ushort, int, uint, long, ulong, char, float, double, decimal, or bool), an enumeration, a string literal, or a reference to null. Since classes or structures are initialized at run time with the new keyword, and not at compile time, you can't set a constant to a class or structure. Constants can be marked as public, private, protected, internal, or protected internal. Constants are accessed as if they were static fields, although they cannot use the static keyword. To use a constant outside of the class that it is declared in, you must fully qualify it using the class name. 25
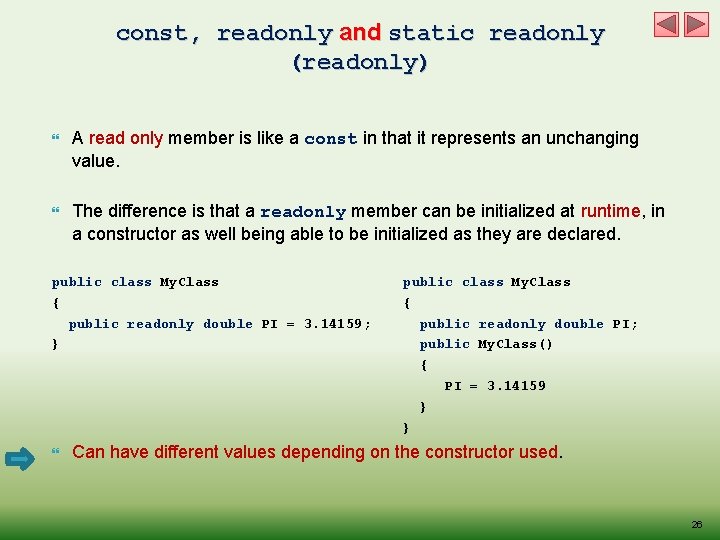
const, readonly and static readonly (readonly) A read only member is like a const in that it represents an unchanging value. The difference is that a readonly member can be initialized at runtime, in a constructor as well being able to be initialized as they are declared. public class My. Class { public readonly double PI = 3. 14159; } public class My. Class { public readonly double PI; public My. Class() { PI = 3. 14159 } } Can have different values depending on the constructor used. 26
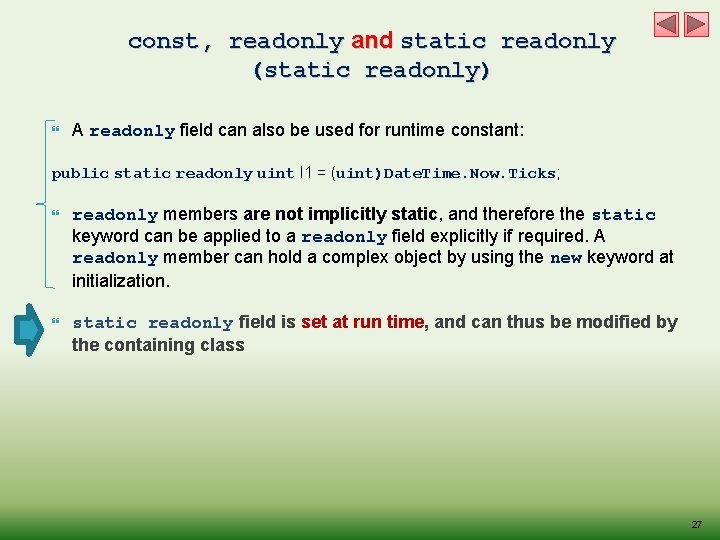
const, readonly and static readonly (static readonly) A readonly field can also be used for runtime constant: public static readonly uint l 1 = (uint)Date. Time. Now. Ticks; readonly members are not implicitly static, and therefore the static keyword can be applied to a readonly field explicitly if required. A readonly member can hold a complex object by using the new keyword at initialization. static readonly field is set at run time, and can thus be modified by the containing class 27
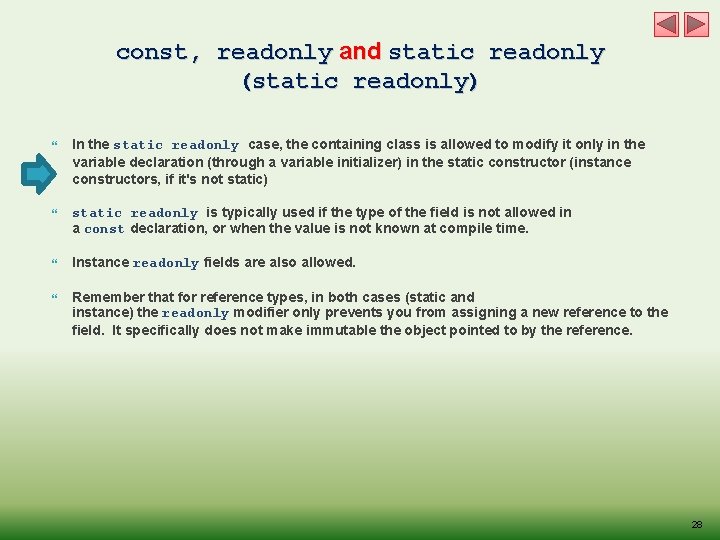
const, readonly and static readonly (static readonly) In the static readonly case, the containing class is allowed to modify it only in the variable declaration (through a variable initializer) in the static constructor (instance constructors, if it's not static) static readonly is typically used if the type of the field is not allowed in a const declaration, or when the value is not known at compile time. Instance readonly fields are also allowed. Remember that for reference types, in both cases (static and instance) the readonly modifier only prevents you from assigning a new reference to the field. It specifically does not make immutable the object pointed to by the reference. 28
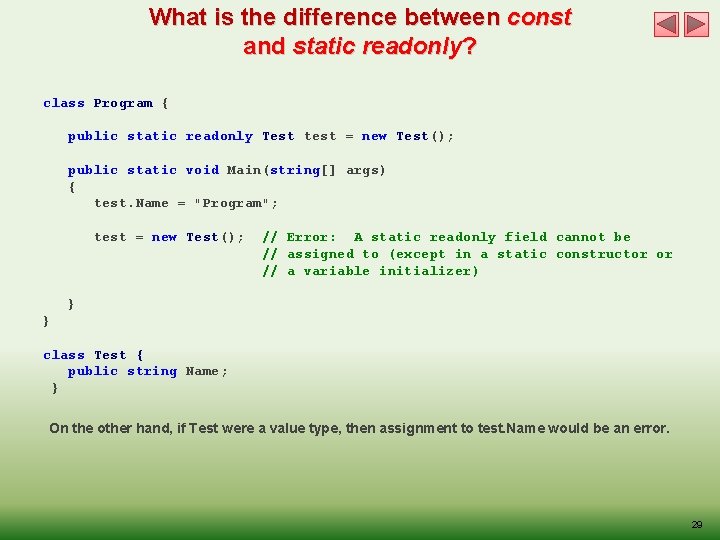
What is the difference between const and static readonly? class Program { public static readonly Test test = new Test(); public static void Main(string[] args) { test. Name = "Program"; test = new Test(); // Error: A static readonly field cannot be // assigned to (except in a static constructor or // a variable initializer) } } class Test { public string Name; } On the other hand, if Test were a value type, then assignment to test. Name would be an error. 29
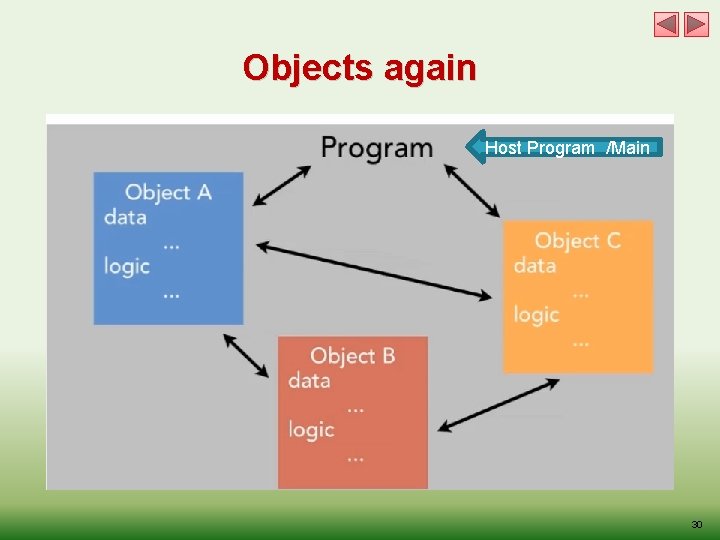
Objects again Host Program /Main 30
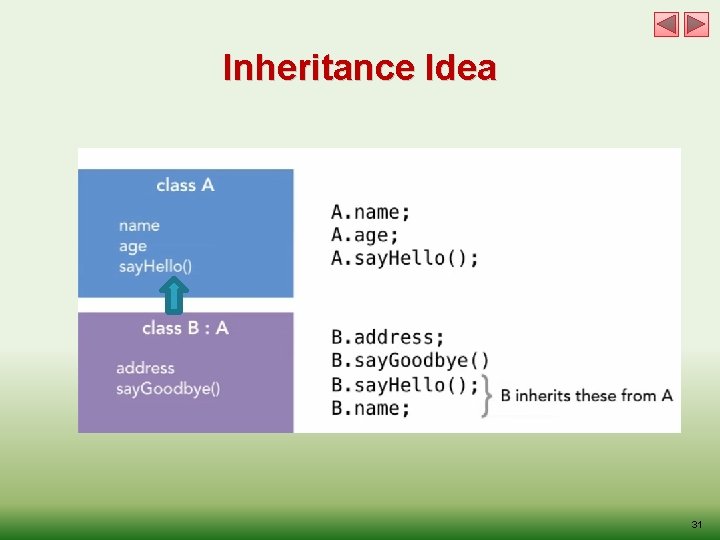
Inheritance Idea 31
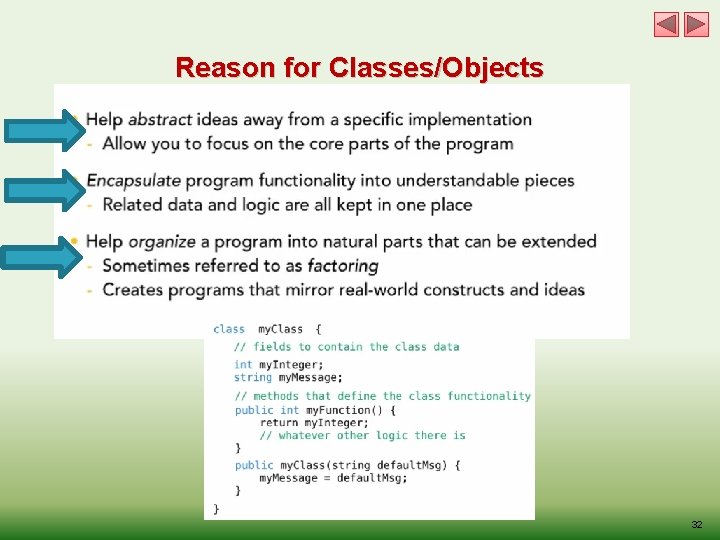
Reason for Classes/Objects 32
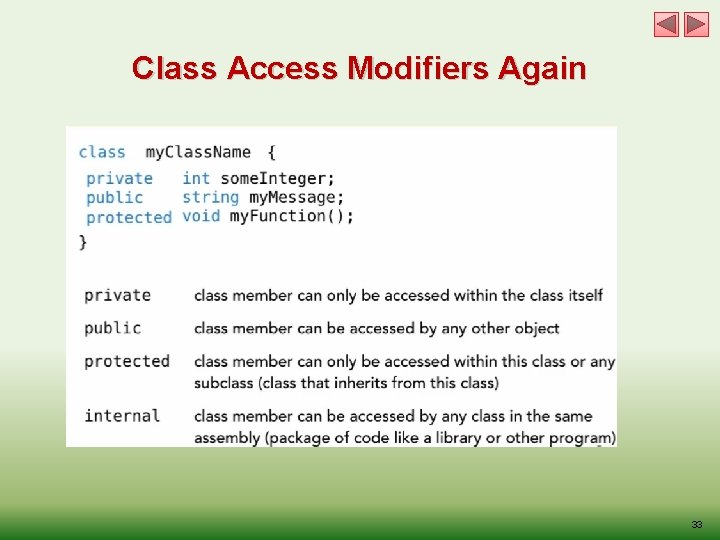
Class Access Modifiers Again 33
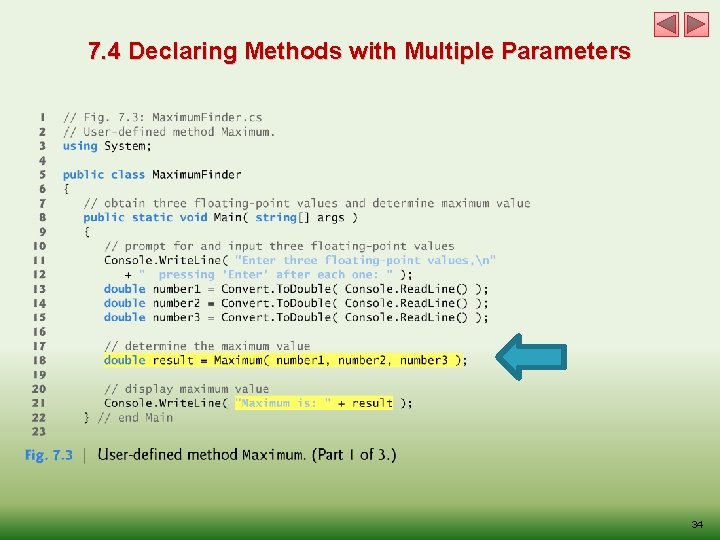
7. 4 Declaring Methods with Multiple Parameters 34
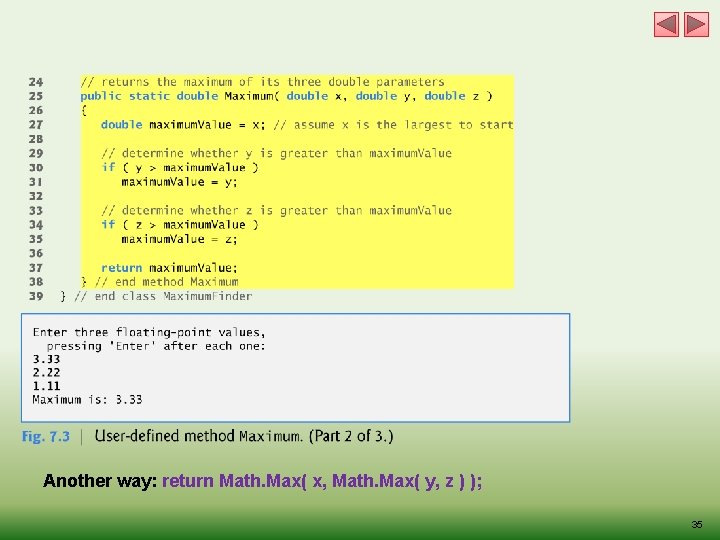
Another way: return Math. Max( x, Math. Max( y, z ) ); 35
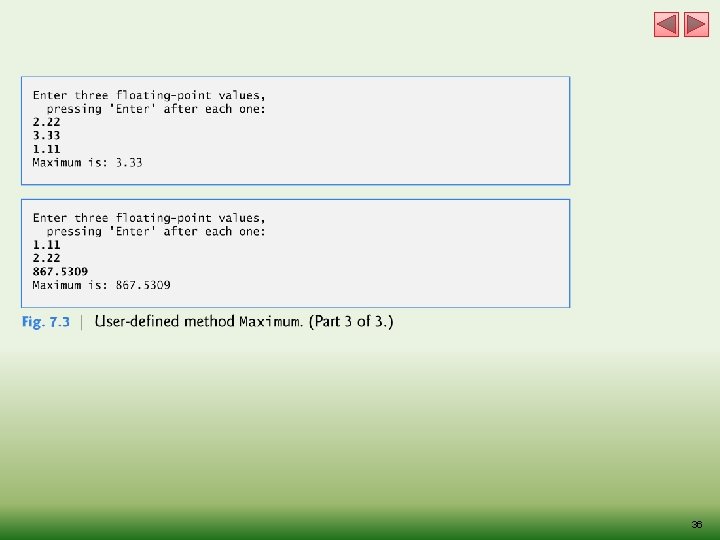
36
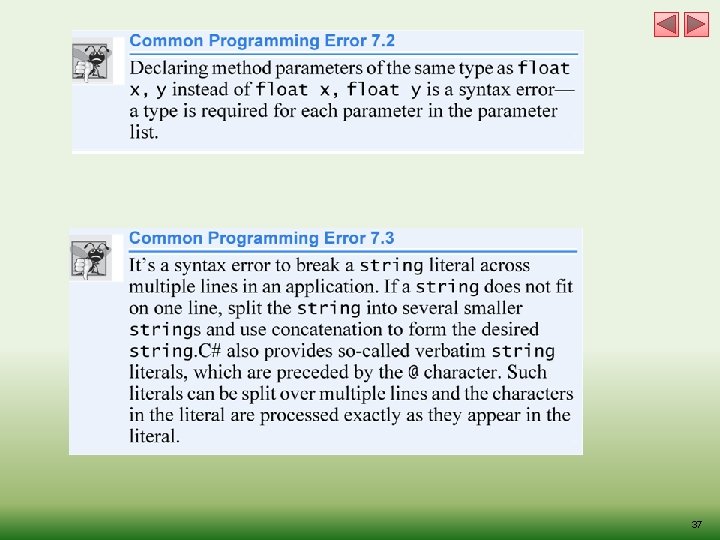
37
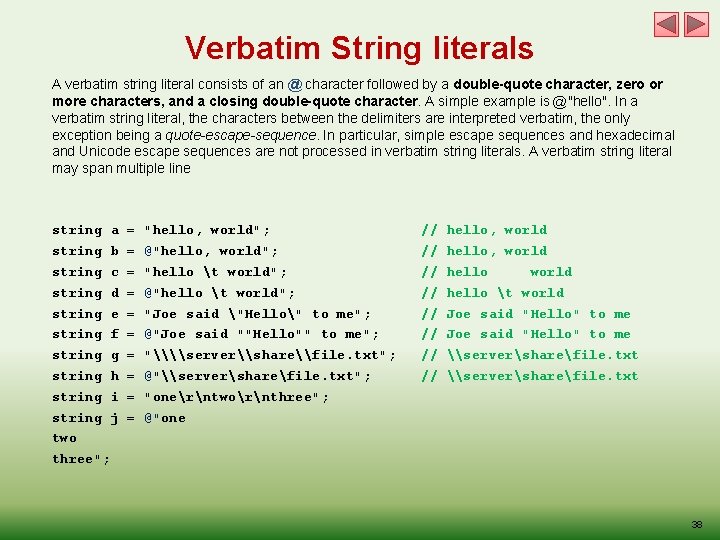
Verbatim String literals A verbatim string literal consists of an @ character followed by a double-quote character, zero or more characters, and a closing double-quote character. A simple example is @"hello". In a verbatim string literal, the characters between the delimiters are interpreted verbatim, the only exception being a quote-escape-sequence. In particular, simple escape sequences and hexadecimal and Unicode escape sequences are not processed in verbatim string literals. A verbatim string literal may span multiple line string a string b string c string d string e string f string g string h string i string j two three"; = = = = = "hello, world"; @"hello, world"; "hello t world"; @"hello t world"; "Joe said "Hello" to me"; @"Joe said ""Hello"" to me"; "\\server\share\file. txt"; @"\serversharefile. txt"; "onerntwornthree"; @"one // // hello, world hello t world Joe said "Hello" to me \serversharefile. txt 38
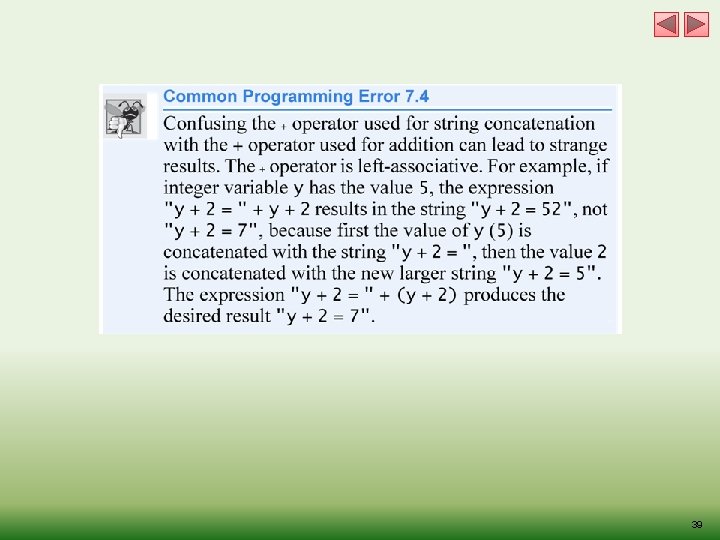
39
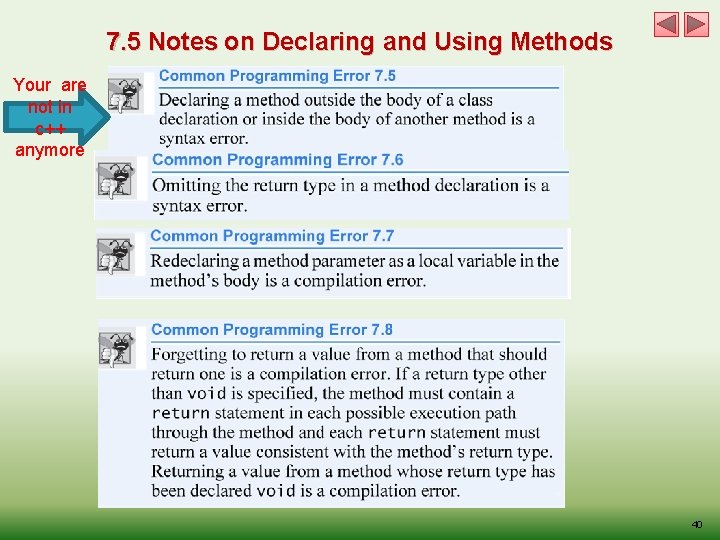
7. 5 Notes on Declaring and Using Methods Your are not in c++ anymore 40
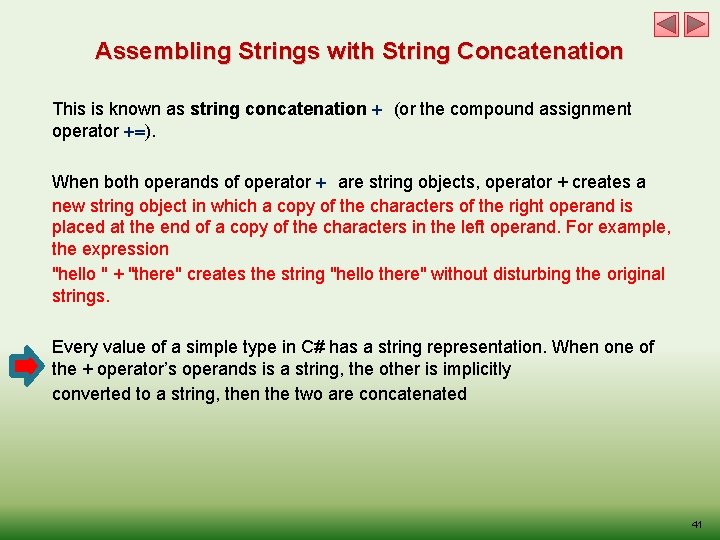
Assembling Strings with String Concatenation This is known as string concatenation + (or the compound assignment operator +=). When both operands of operator + are string objects, operator + creates a new string object in which a copy of the characters of the right operand is placed at the end of a copy of the characters in the left operand. For example, the expression "hello " + "there" creates the string "hello there" without disturbing the original strings. Every value of a simple type in C# has a string representation. When one of the + operator’s operands is a string, the other is implicitly converted to a string, then the two are concatenated 41
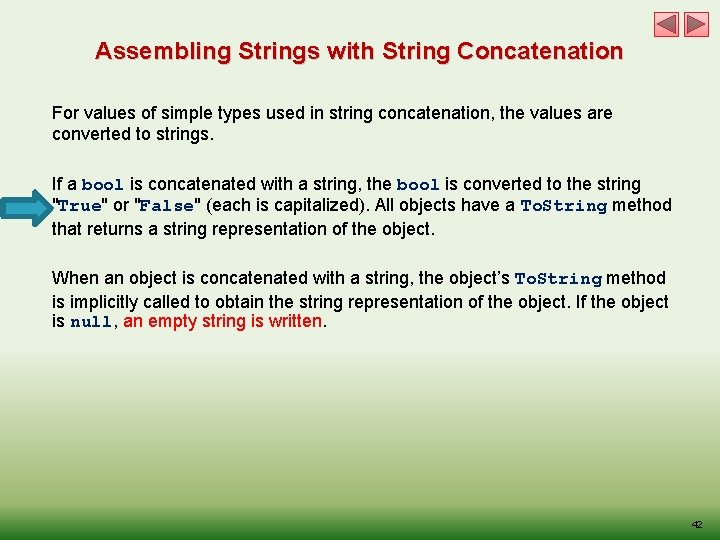
Assembling Strings with String Concatenation For values of simple types used in string concatenation, the values are converted to strings. If a bool is concatenated with a string, the bool is converted to the string "True" or "False" (each is capitalized). All objects have a To. String method that returns a string representation of the object. When an object is concatenated with a string, the object’s To. String method is implicitly called to obtain the string representation of the object. If the object is null, an empty string is written. 42
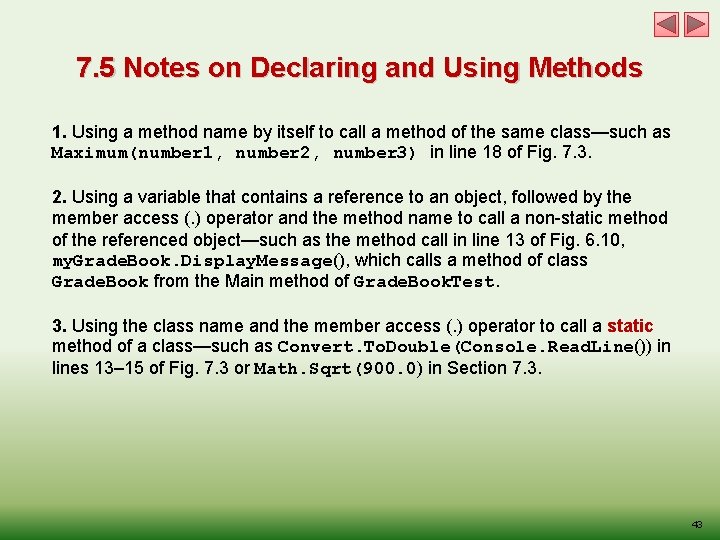
7. 5 Notes on Declaring and Using Methods 1. Using a method name by itself to call a method of the same class—such as Maximum(number 1, number 2, number 3) in line 18 of Fig. 7. 3. 2. Using a variable that contains a reference to an object, followed by the member access (. ) operator and the method name to call a non-static method of the referenced object—such as the method call in line 13 of Fig. 6. 10, my. Grade. Book. Display. Message(), which calls a method of class Grade. Book from the Main method of Grade. Book. Test. 3. Using the class name and the member access (. ) operator to call a static method of a class—such as Convert. To. Double(Console. Read. Line()) in lines 13– 15 of Fig. 7. 3 or Math. Sqrt(900. 0) in Section 7. 3. 43
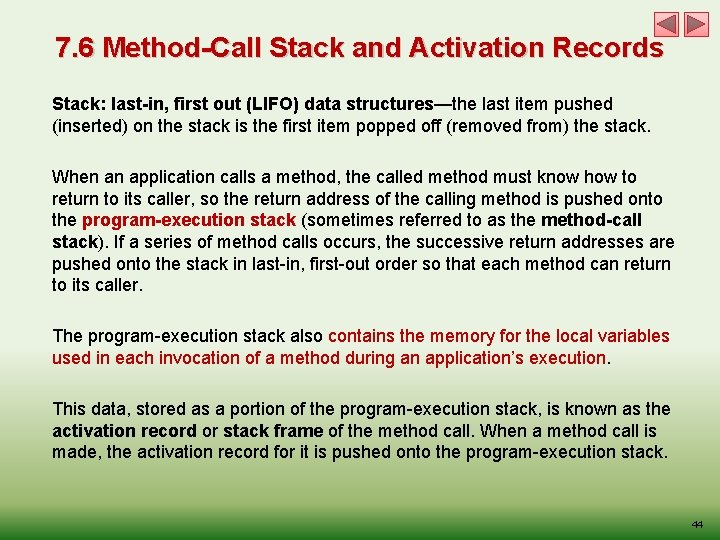
7. 6 Method-Call Stack and Activation Records Stack: last-in, first out (LIFO) data structures—the last item pushed (inserted) on the stack is the first item popped off (removed from) the stack. When an application calls a method, the called method must know how to return to its caller, so the return address of the calling method is pushed onto the program-execution stack (sometimes referred to as the method-call stack). If a series of method calls occurs, the successive return addresses are pushed onto the stack in last-in, first-out order so that each method can return to its caller. The program-execution stack also contains the memory for the local variables used in each invocation of a method during an application’s execution. This data, stored as a portion of the program-execution stack, is known as the activation record or stack frame of the method call. When a method call is made, the activation record for it is pushed onto the program-execution stack. 44
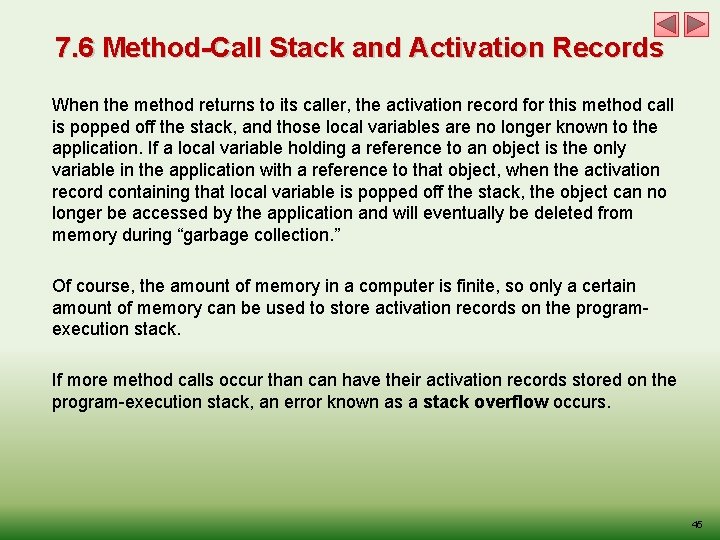
7. 6 Method-Call Stack and Activation Records When the method returns to its caller, the activation record for this method call is popped off the stack, and those local variables are no longer known to the application. If a local variable holding a reference to an object is the only variable in the application with a reference to that object, when the activation record containing that local variable is popped off the stack, the object can no longer be accessed by the application and will eventually be deleted from memory during “garbage collection. ” Of course, the amount of memory in a computer is finite, so only a certain amount of memory can be used to store activation records on the programexecution stack. If more method calls occur than can have their activation records stored on the program-execution stack, an error known as a stack overflow occurs. 45
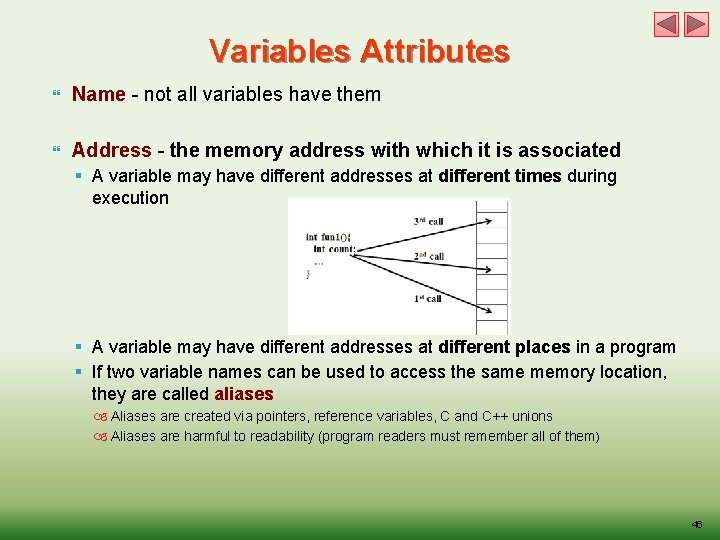
Variables Attributes Name - not all variables have them Address - the memory address with which it is associated § A variable may have different addresses at different times during execution § A variable may have different addresses at different places in a program § If two variable names can be used to access the same memory location, they are called aliases Aliases are created via pointers, reference variables, C and C++ unions Aliases are harmful to readability (program readers must remember all of them) 46
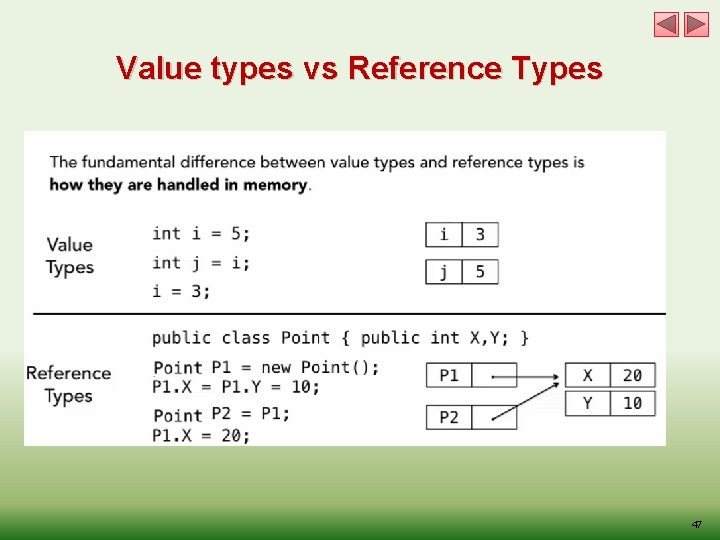
Value types vs Reference Types 47
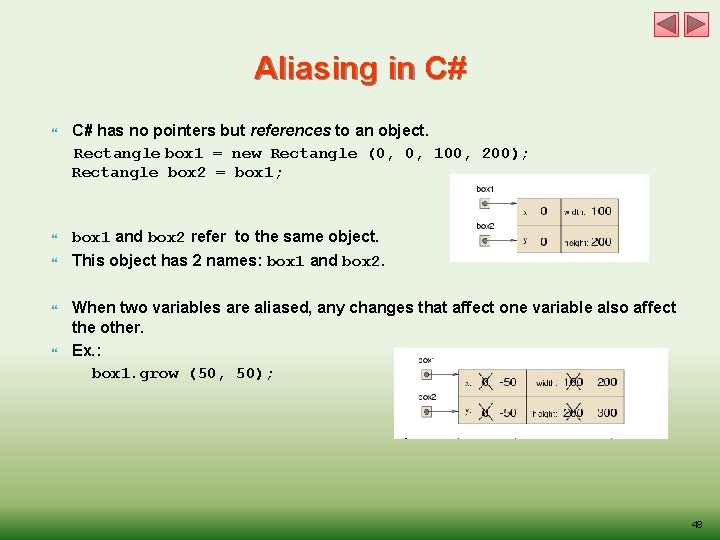
Aliasing in C# has no pointers but references to an object. Rectangle box 1 = new Rectangle (0, 0, 100, 200); Rectangle box 2 = box 1; box 1 and box 2 refer to the same object. This object has 2 names: box 1 and box 2. When two variables are aliased, any changes that affect one variable also affect the other. Ex. : box 1. grow (50, 50); 48
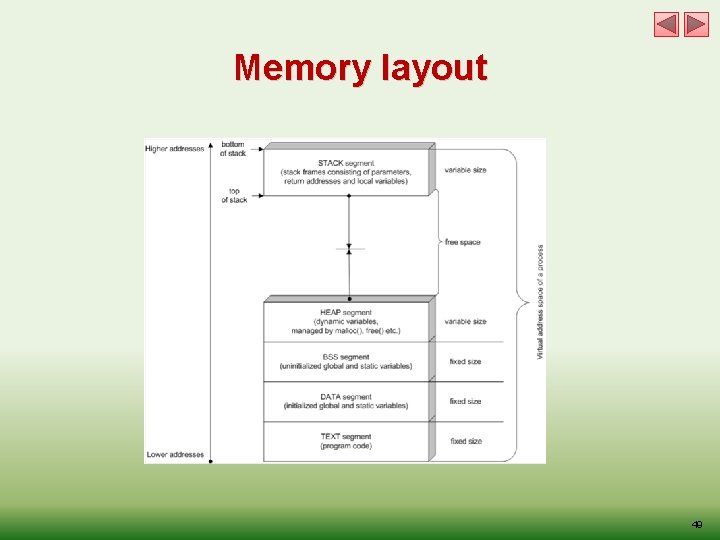
Memory layout 49
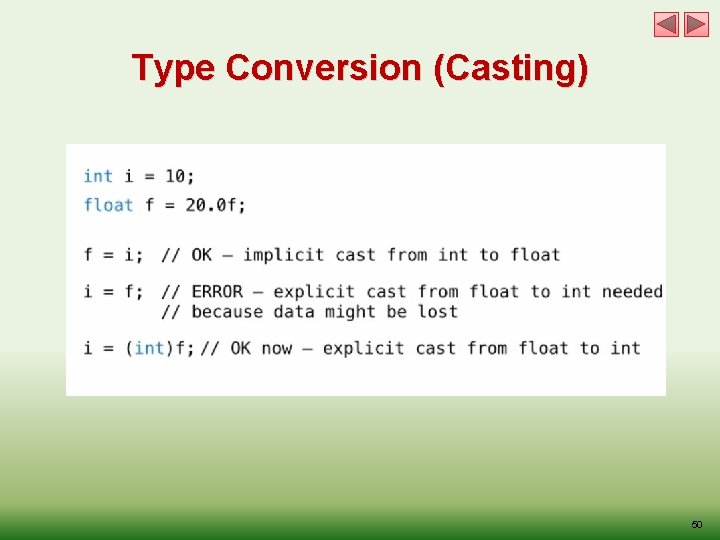
Type Conversion (Casting) 50
![Conversion Ex namespace Conversion class Program static void Mainstring args int Conversion Ex: namespace Conversion { class Program { static void Main(string[] args) { int](https://slidetodoc.com/presentation_image_h2/877659862373332b75950d0d38dd5fbf/image-51.jpg)
Conversion Ex: namespace Conversion { class Program { static void Main(string[] args) { int i = 10; short x = 5; float f = 20. 0 f; //i = x; //x = i; // f = i; // i = f; } } } 51
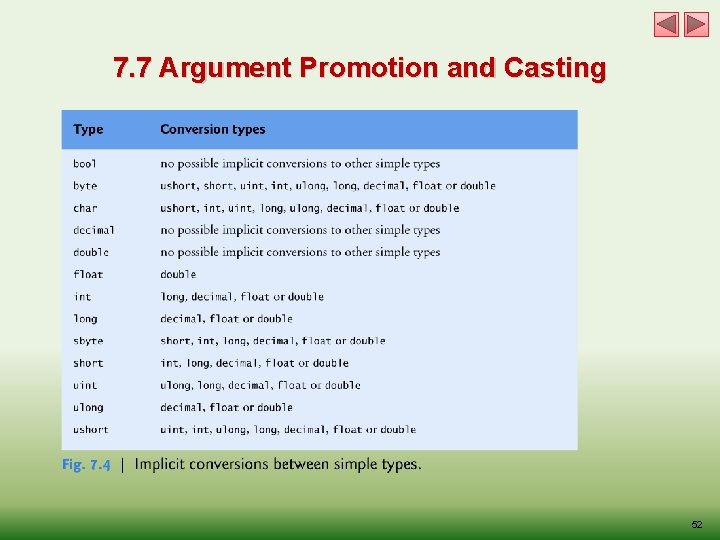
7. 7 Argument Promotion and Casting 52
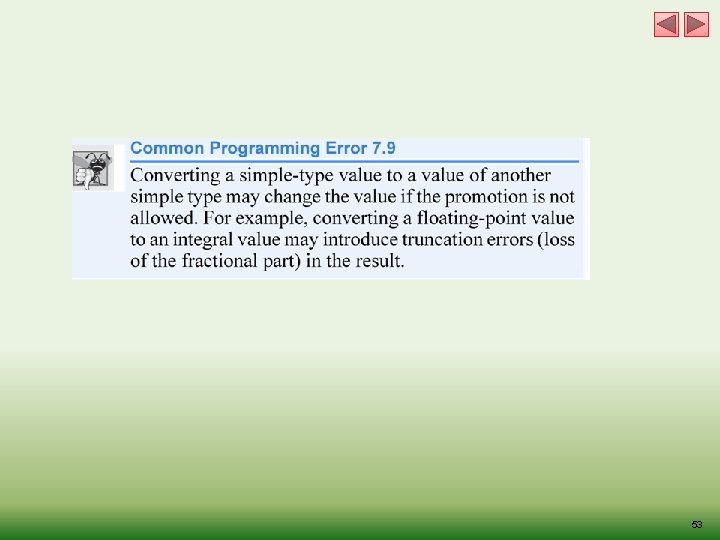
53
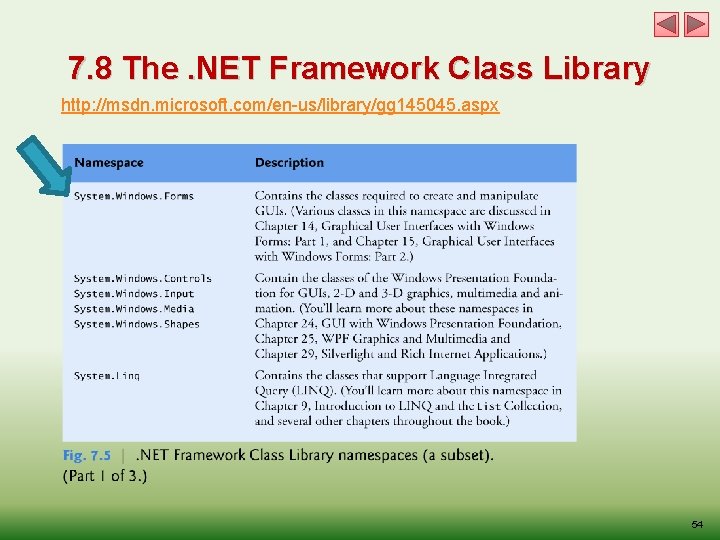
7. 8 The. NET Framework Class Library http: //msdn. microsoft. com/en-us/library/gg 145045. aspx 54
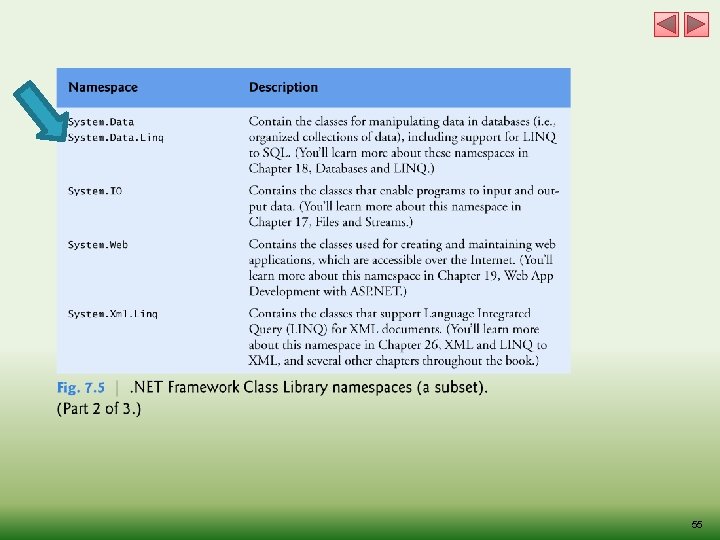
55
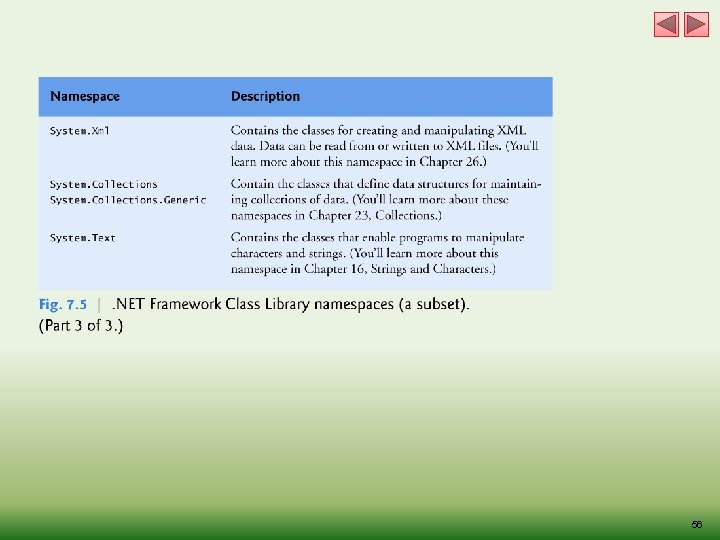
56
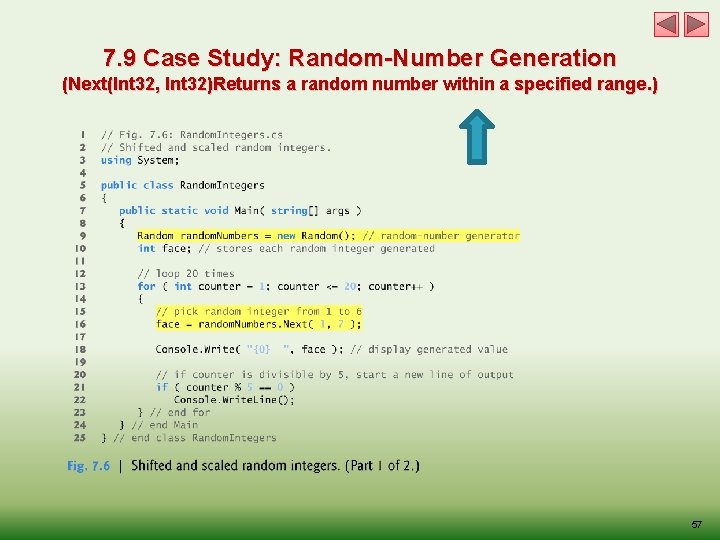
7. 9 Case Study: Random-Number Generation (Next(Int 32, Int 32)Returns a random number within a specified range. ) 57
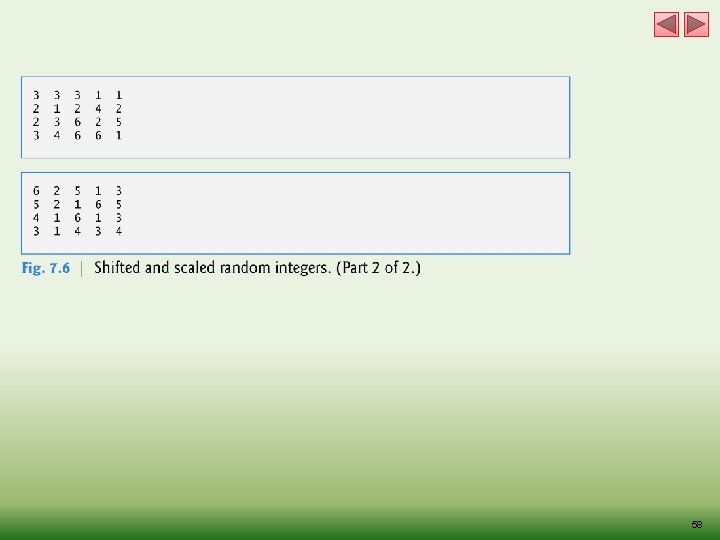
58
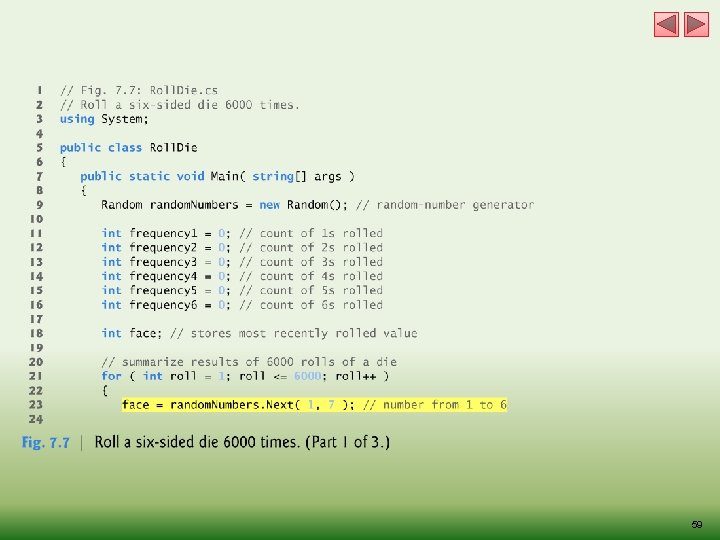
59
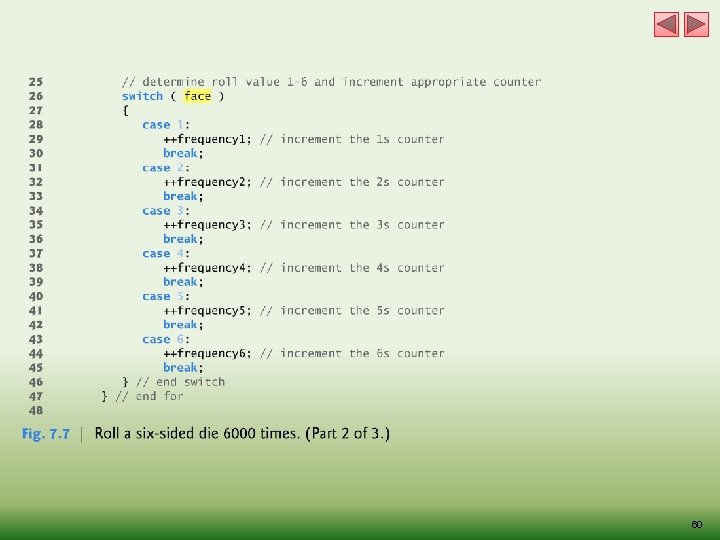
60
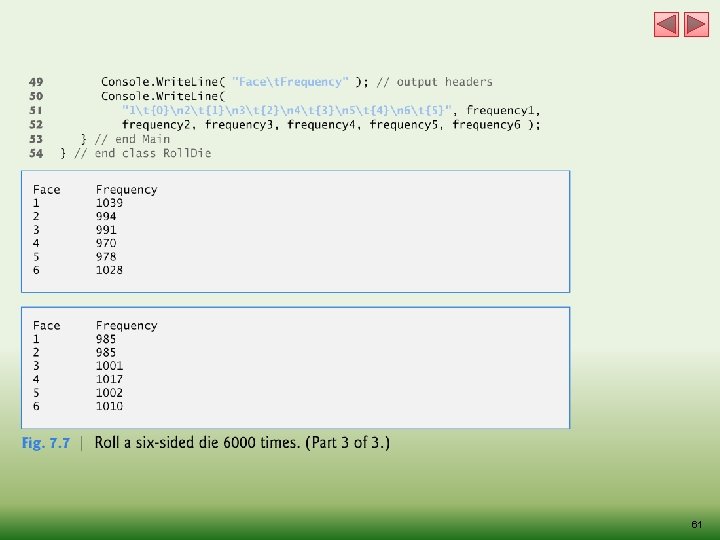
61
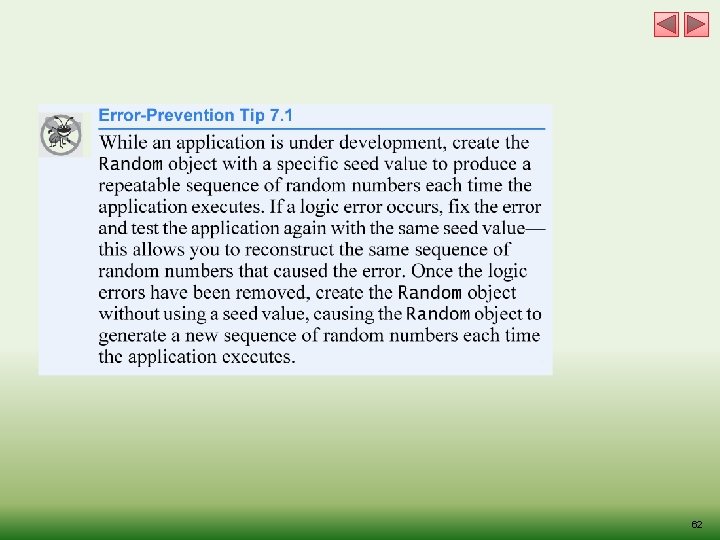
62
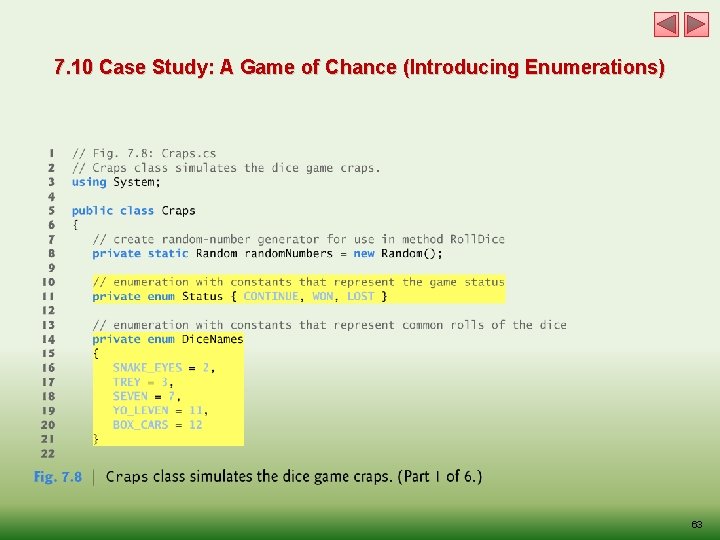
7. 10 Case Study: A Game of Chance (Introducing Enumerations) 63
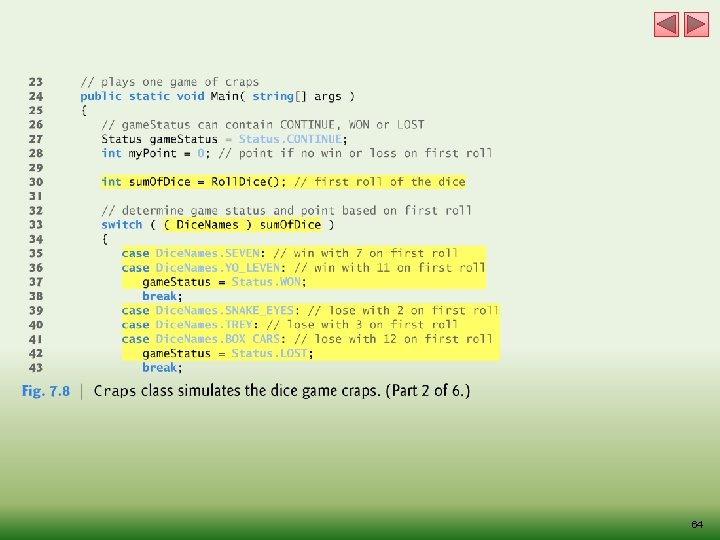
64
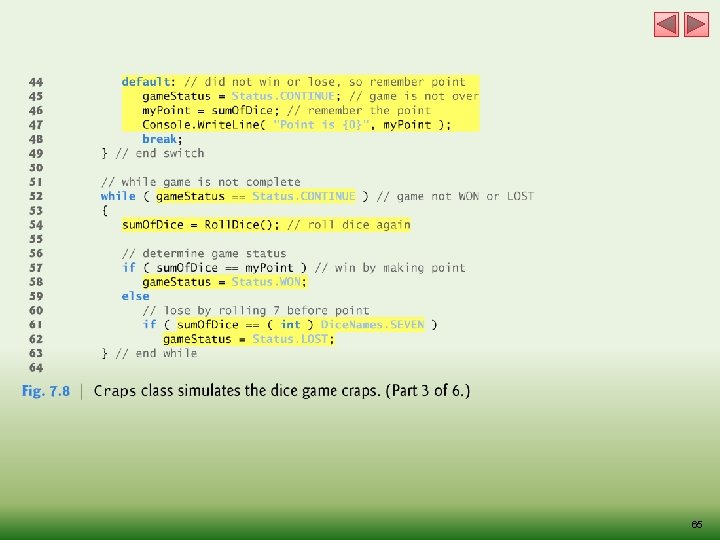
65
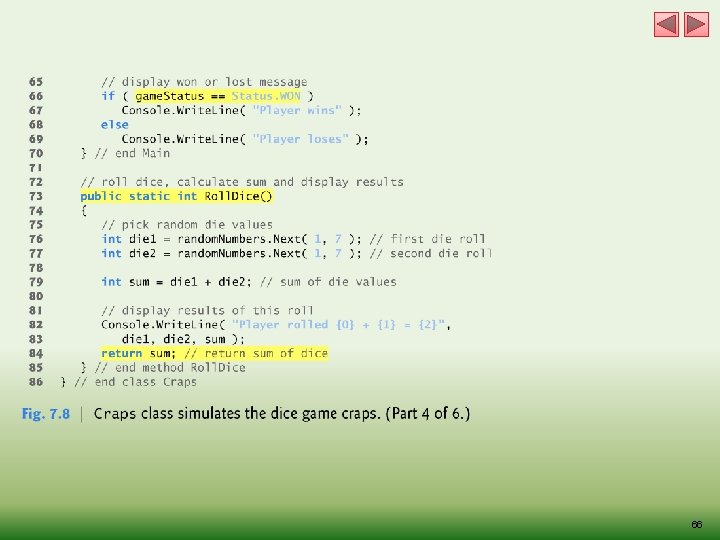
66
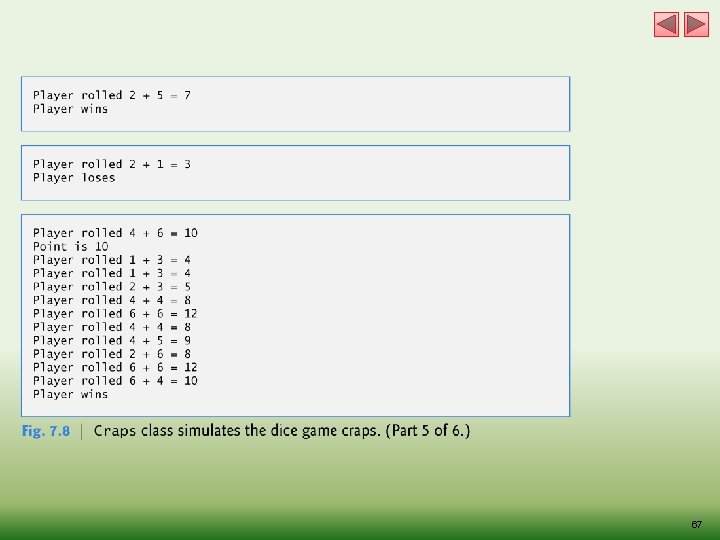
67
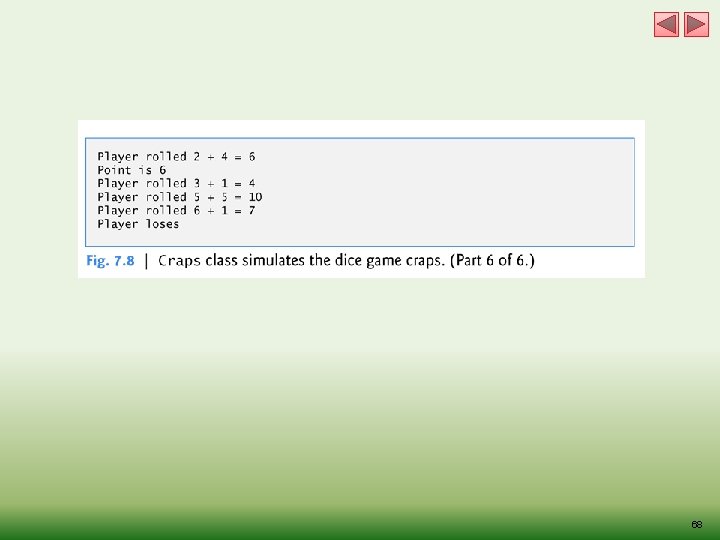
68
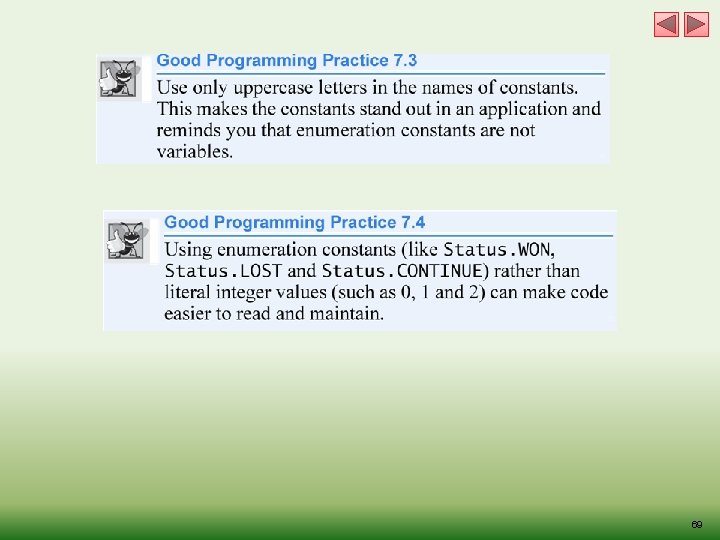
69
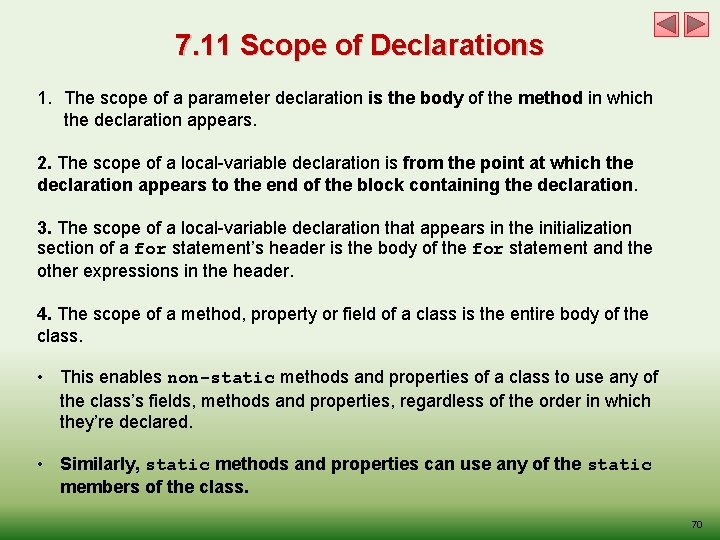
7. 11 Scope of Declarations 1. The scope of a parameter declaration is the body of the method in which the declaration appears. 2. The scope of a local-variable declaration is from the point at which the declaration appears to the end of the block containing the declaration. 3. The scope of a local-variable declaration that appears in the initialization section of a for statement’s header is the body of the for statement and the other expressions in the header. 4. The scope of a method, property or field of a class is the entire body of the class. • This enables non-static methods and properties of a class to use any of the class’s fields, methods and properties, regardless of the order in which they’re declared. • Similarly, static methods and properties can use any of the static members of the class. 70
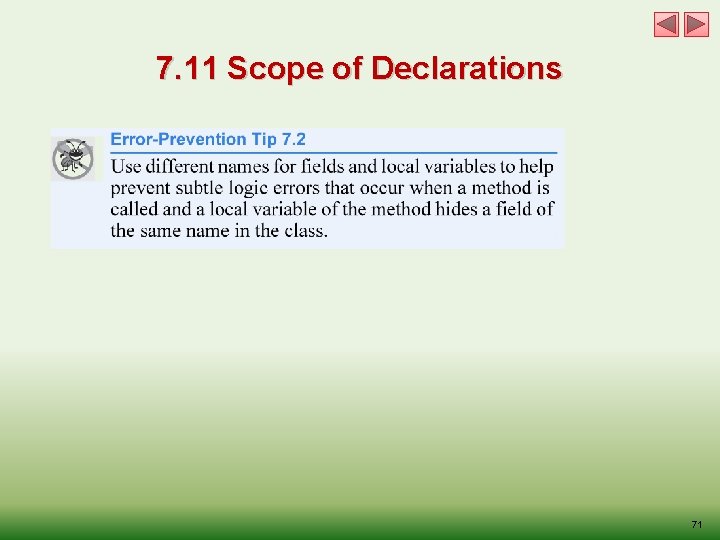
7. 11 Scope of Declarations 71
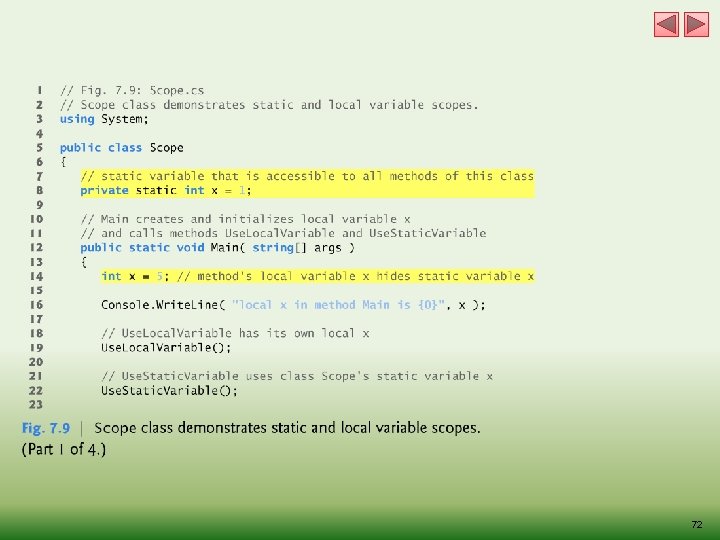
72
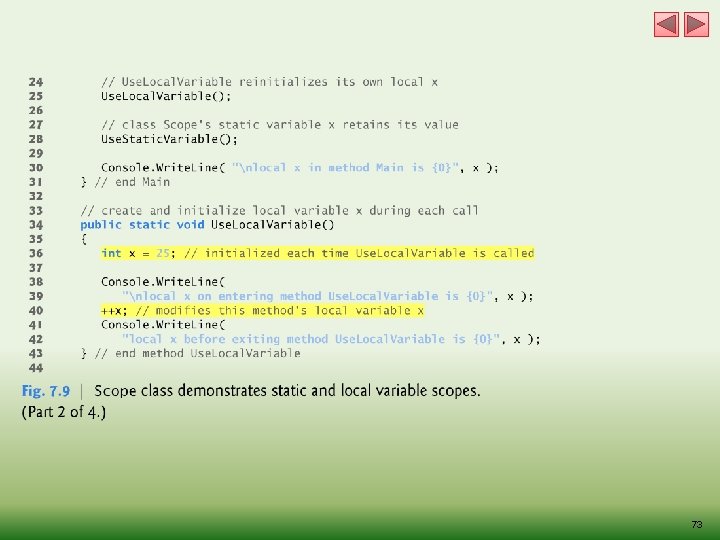
73
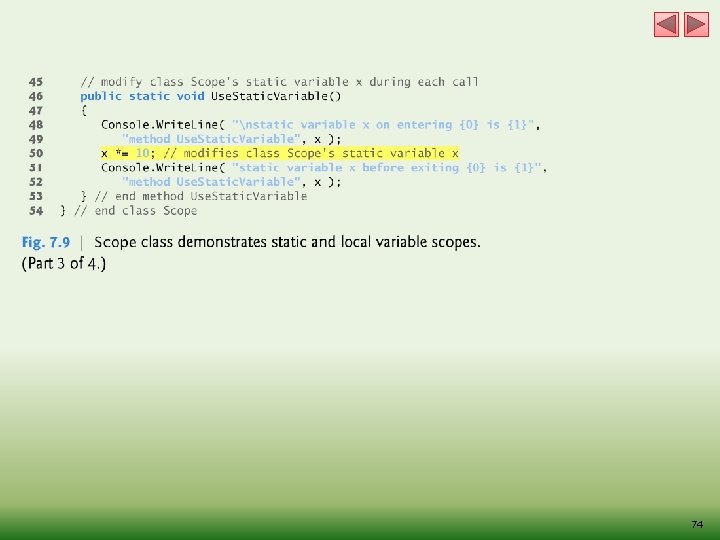
74
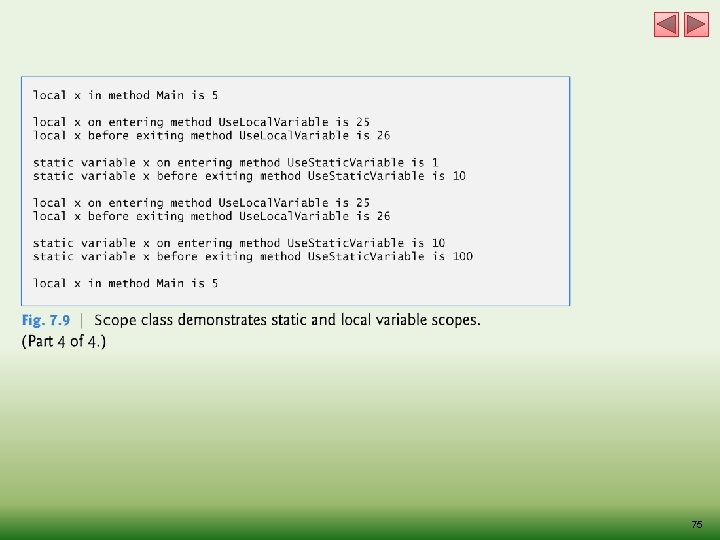
75
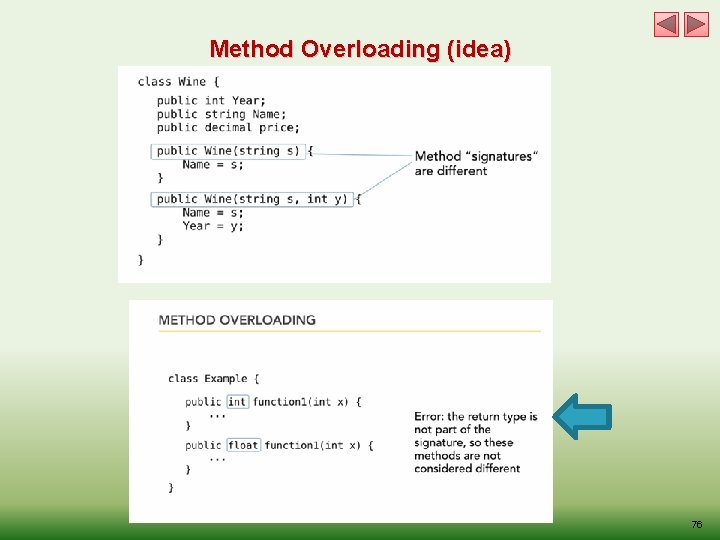
Method Overloading (idea) 76
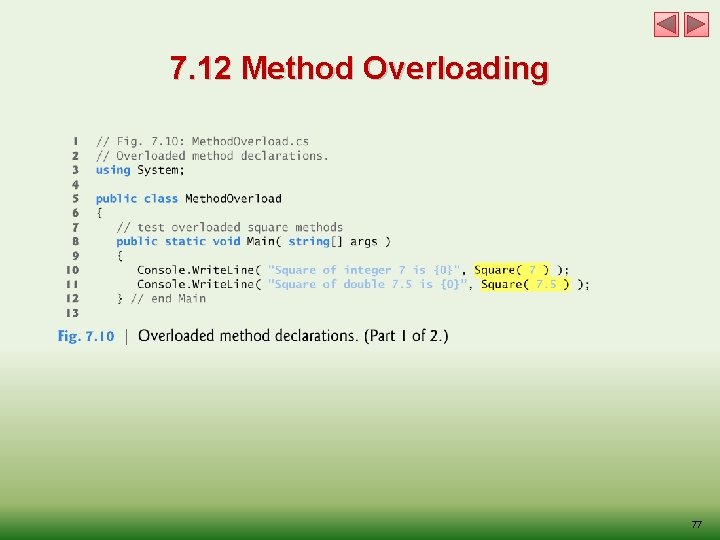
7. 12 Method Overloading 77
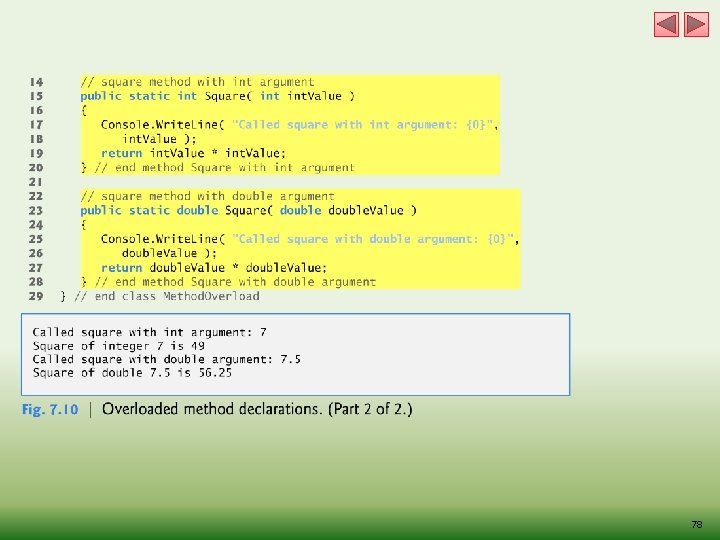
78
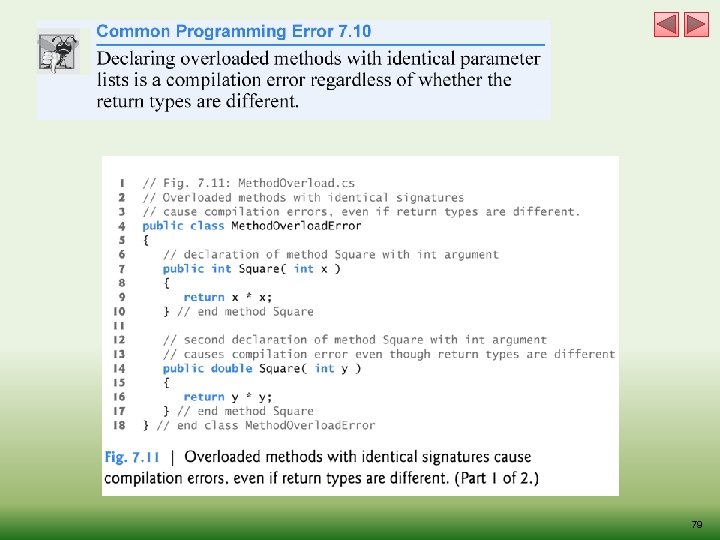
79
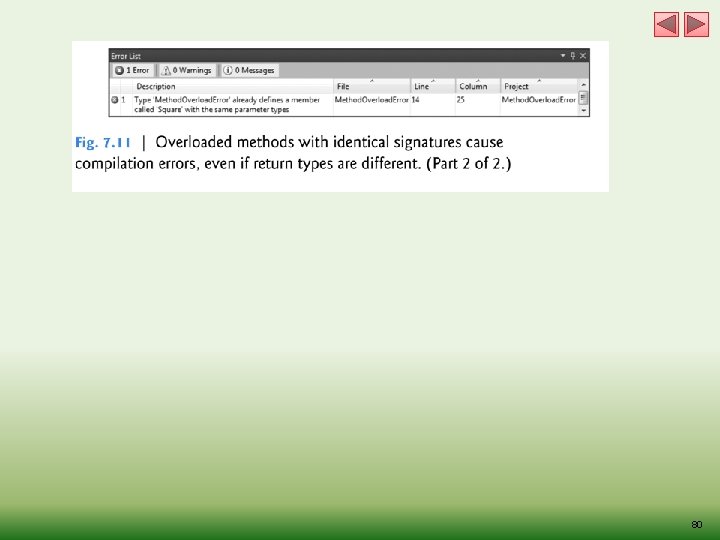
80
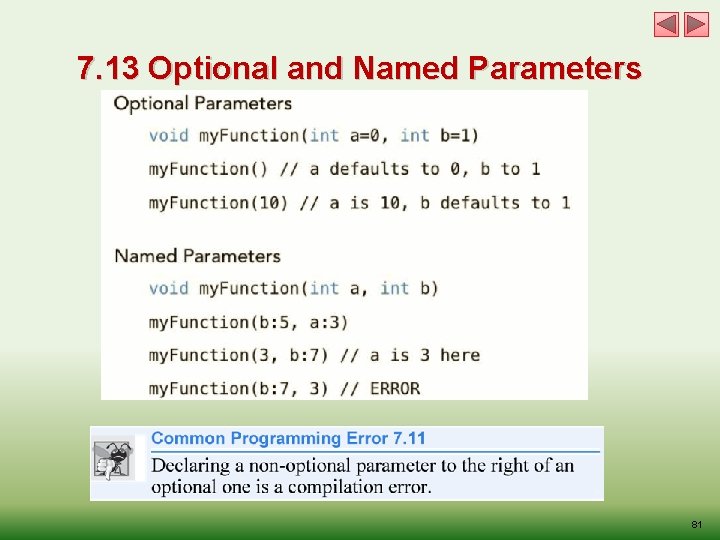
7. 13 Optional and Named Parameters 81
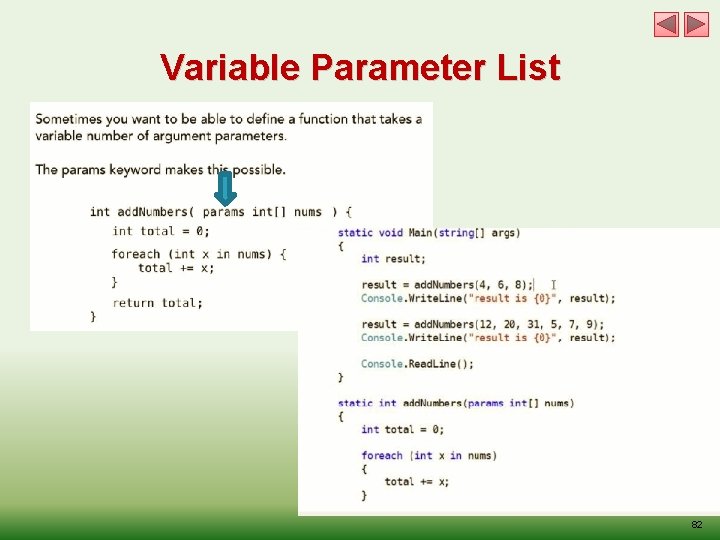
Variable Parameter List 82
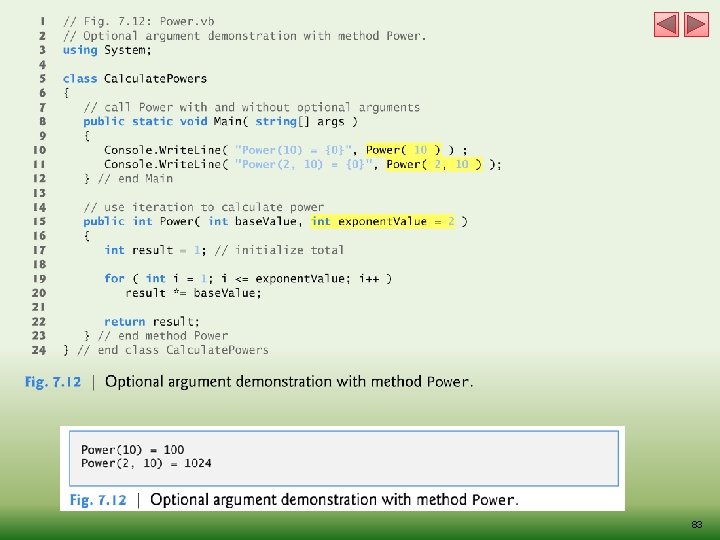
83
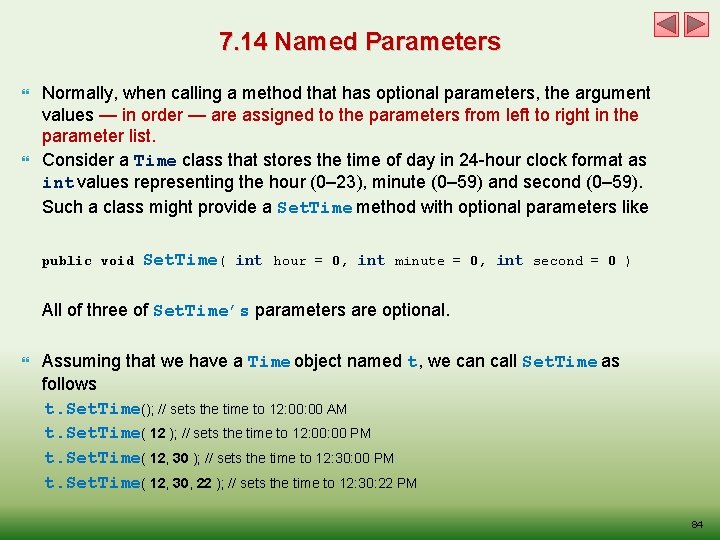
7. 14 Named Parameters Normally, when calling a method that has optional parameters, the argument values — in order — are assigned to the parameters from left to right in the parameter list. Consider a Time class that stores the time of day in 24 -hour clock format as int values representing the hour (0– 23), minute (0– 59) and second (0– 59). Such a class might provide a Set. Time method with optional parameters like public void Set. Time( int hour = 0, int minute = 0, int second = 0 ) All of three of Set. Time’s parameters are optional. Assuming that we have a Time object named t, we can call Set. Time as follows t. Set. Time(); // sets the time to 12: 00 AM t. Set. Time( 12 ); // sets the time to 12: 00 PM t. Set. Time( 12, 30 ); // sets the time to 12: 30: 00 PM t. Set. Time( 12, 30, 22 ); // sets the time to 12: 30: 22 PM 84
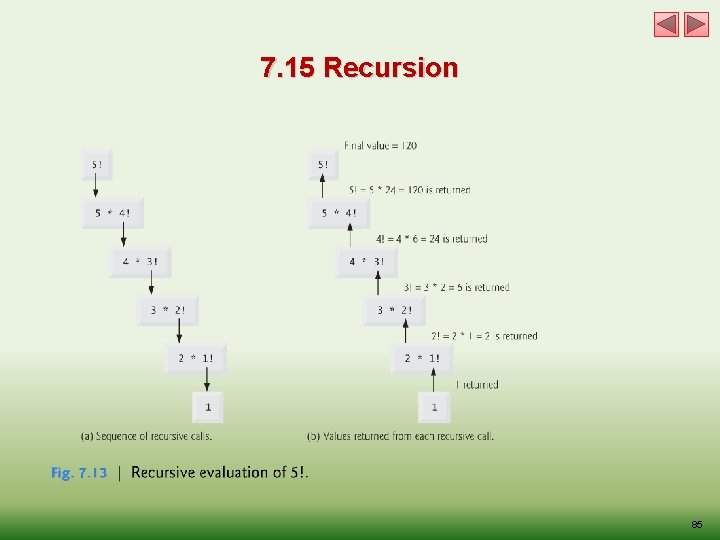
7. 15 Recursion 85
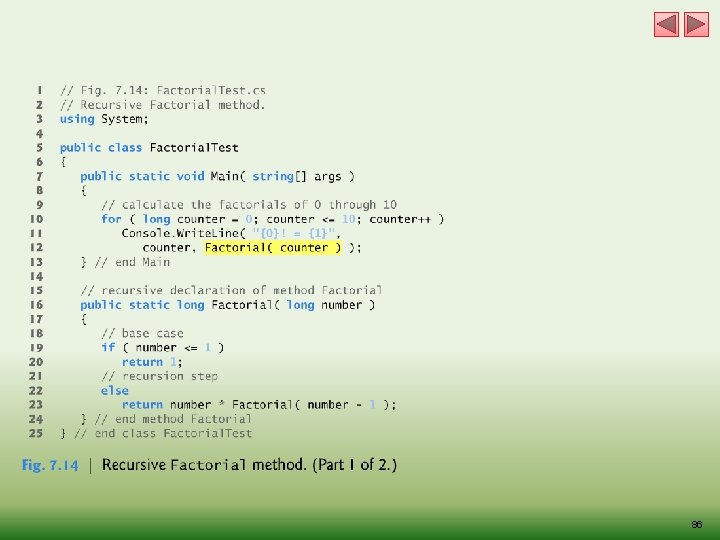
86
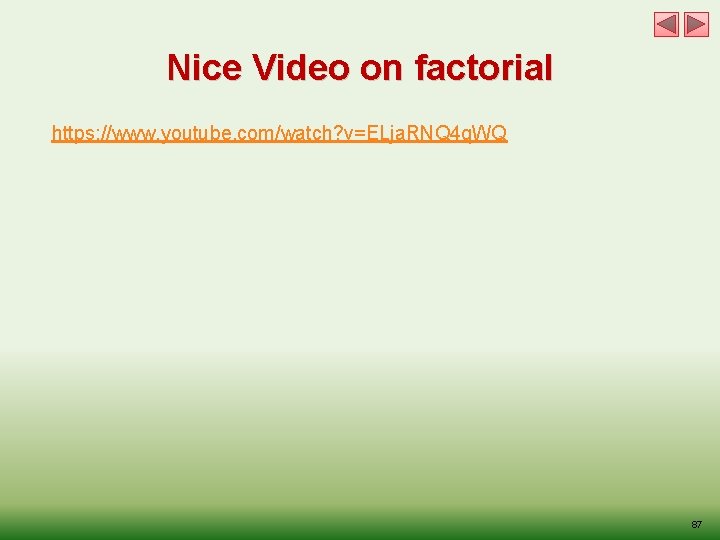
Nice Video on factorial https: //www. youtube. com/watch? v=ELja. RNQ 4 q. WQ 87
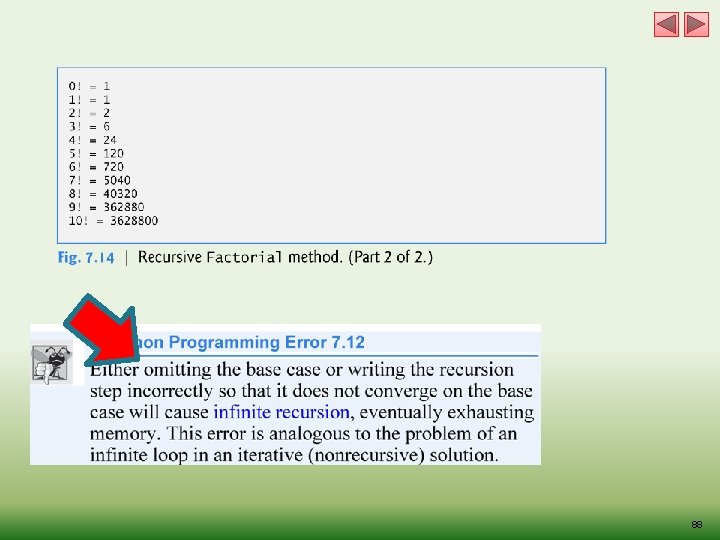
88
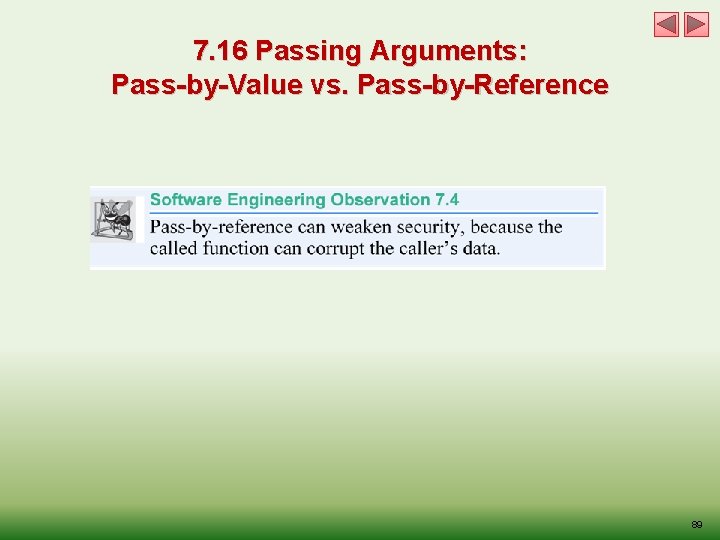
7. 16 Passing Arguments: Pass-by-Value vs. Pass-by-Reference 89
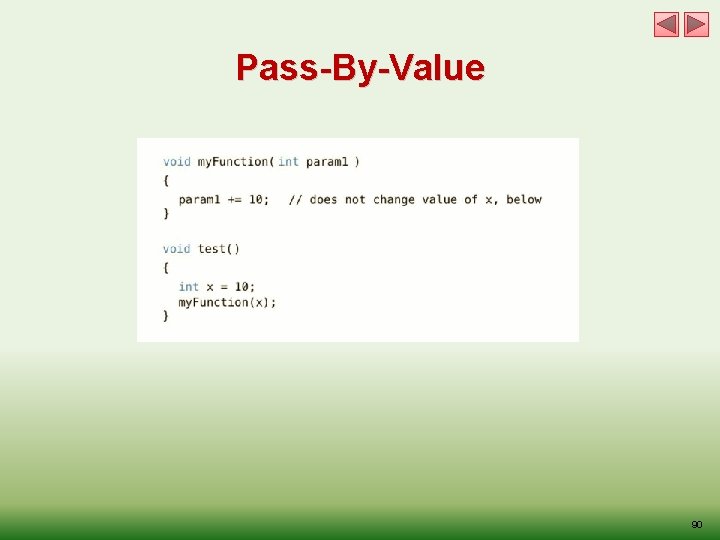
Pass-By-Value 90
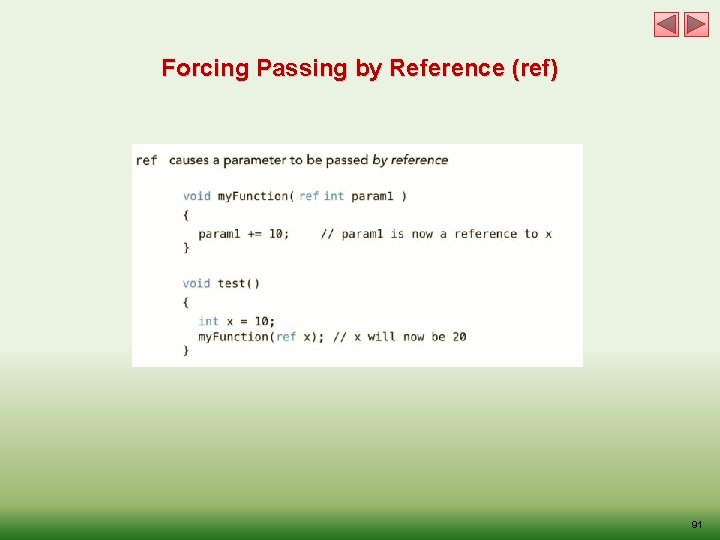
Forcing Passing by Reference (ref) 91
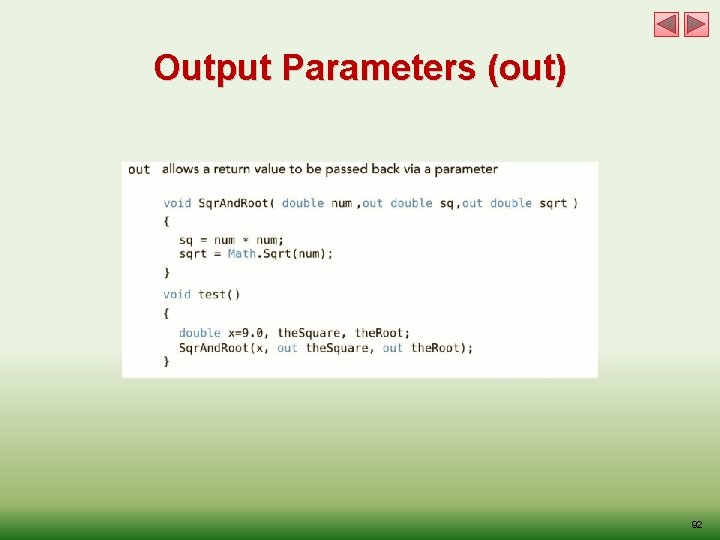
Output Parameters (out) 92
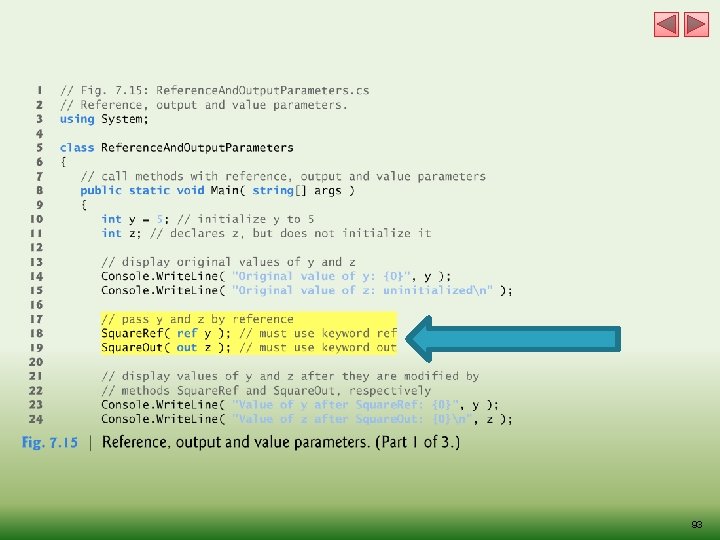
93
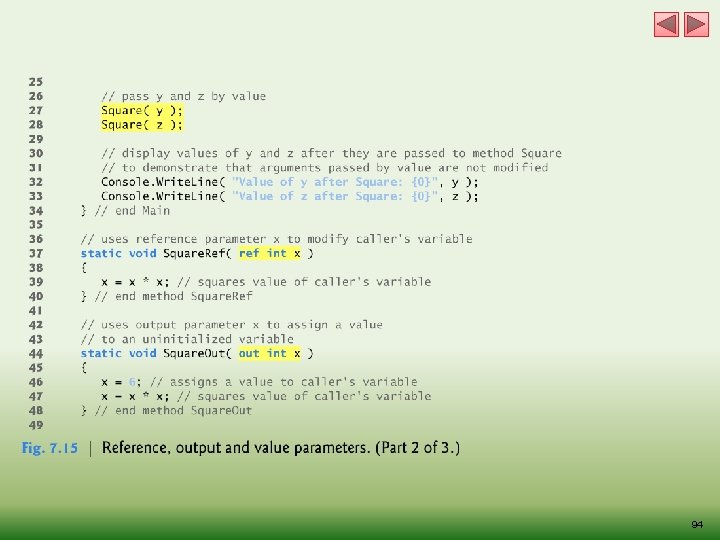
94
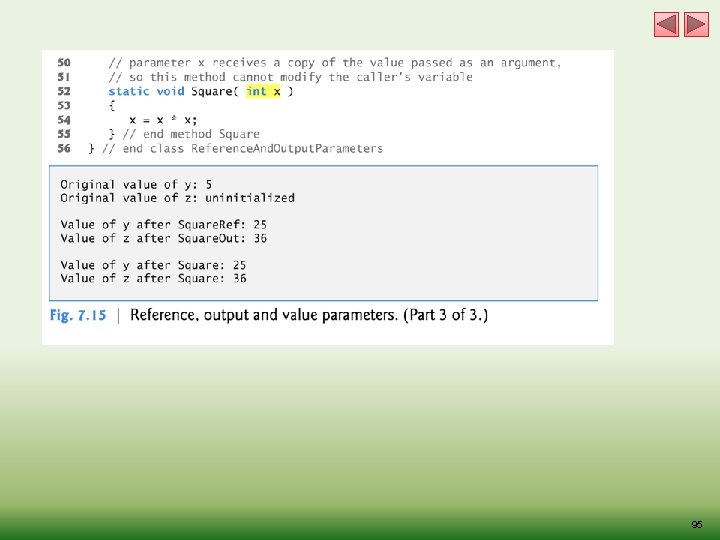
95
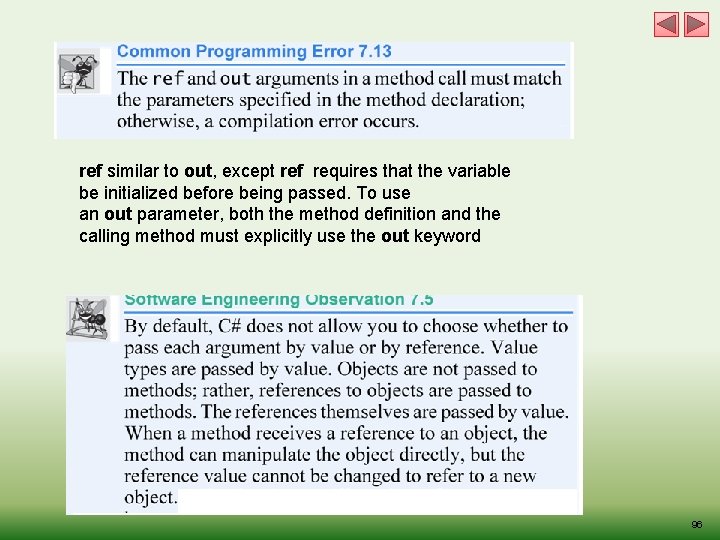
ref similar to out, except ref requires that the variable be initialized before being passed. To use an out parameter, both the method definition and the calling method must explicitly use the out keyword 96
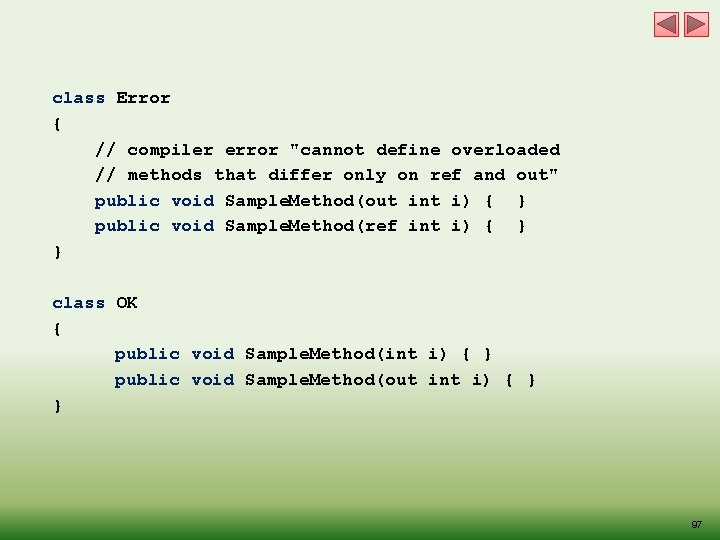
class Error { // compiler error "cannot define overloaded // methods that differ only on ref and out" public void Sample. Method(out int i) { } public void Sample. Method(ref int i) { } } class OK { public void Sample. Method(int i) { } public void Sample. Method(out int i) { } } 97
Look up look down look left look right
Into the heart of jesus deeper and deeper i go
A-wax pattern recognition
1 look at the picture
Look at the image in activity 1
Activity 1 picture analysis
Deep dive synonym
Dclm netherlands
Deeper life netherlands
Deeper life search the scriptures volume 78
Deeper life bible church messages
Deeper life bible church netherlands search the scriptures
Deeper life canada
Growing deeper in god
Promote together
Deeper christian life ministry live
Deeper life netherlands
Netherlands
Dclm netherlands
Deeper life netherlands
Go deeper in christ
Your love is deeper than the ocean higher than the heavens
Deeper life bible church netherlands
Deeper life netherlands
Deeper life netherlands
Deeper life netherlands
Deeper life netherlands
Deeper life bible church
Vdoe teacher direct
Deeperph
Deeper life netherlands
Deeper life netherlands
Deeper life bible church netherlands search the scriptures
What is deeper exam
Vietnam war at home webquest answers
Deeper life youth search the scripture
Inside the floating cloak he was tall
Isotonicity adjustment methods slideshare
Importance of data in legal research
Business research methods by zikmund
Newton forward interpolation formula
Binning methods in data mining
What is instrument processing
Methods of community organization
Purpose of teaching method
Autoclave principle ppt
Observation methods of data collection
Sales breakdown method
Rso test
Project screening example
Advanced higher modern studies
Indirect method of linear measurement
Od survey methods
Dac testing methods
Sampling methods in qualitative and quantitative research
Labor induction methods
Metal identification methods
Need satisfaction presentation
Uninformed search algorithm
Plain milling can be otherwise called as
Differential equations formula
Demand estimation and forecasting
Ck metrics suite
Erp selection methods and criteria
Sterilization by mechanical methods
Ranking method of job evaluation
Clandestine methods of entry cmoe
A thousand teachers a thousand methods means
Purpose of direct method
Management by objectives performance appraisal
Basic human aspirations are -
Examples of sifting
Methods of gathering data national 5 pe
Livestock valuation methods
Which of the market forms of meat does not undergo chilling
Enzymatic method of blood glucose estimation
Mining frequent itemsets using vertical data format
Departmental accounts format
Need satisfaction presentation
Quality business a level
Methods of investigating behaviour
Transfer pricing methods with examples
Data input methods
Single blind study
Methods for determining time of death
Error detection methods
Qualitative research methods
Benefits sought segmentation
Ways of presenting information
Advanced business research
On optimistic methods for concurrency control
Linear convolution using dft
Array methods
Mechanical disinfection
Variational principle
What is circuit training method
Jaws
抽樣分配