GUI Swing Class Hierarchy Swing Components Swing Conatiners
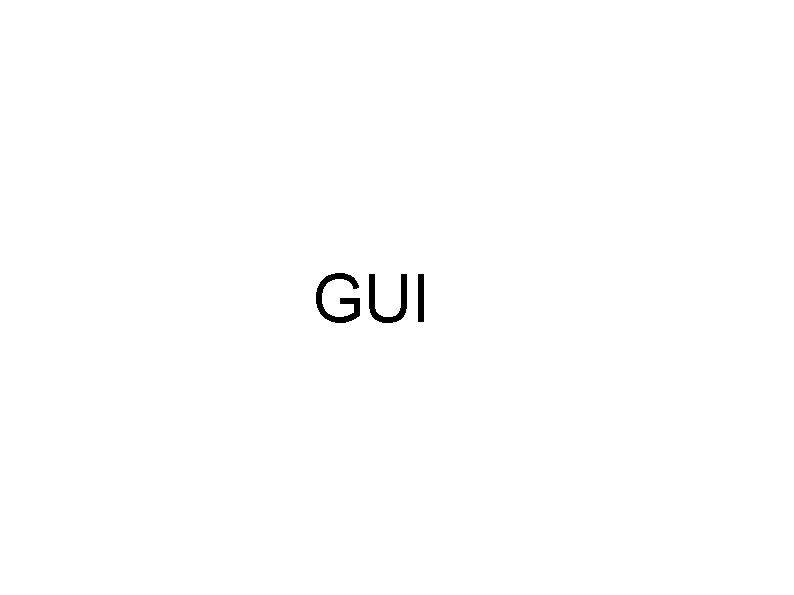
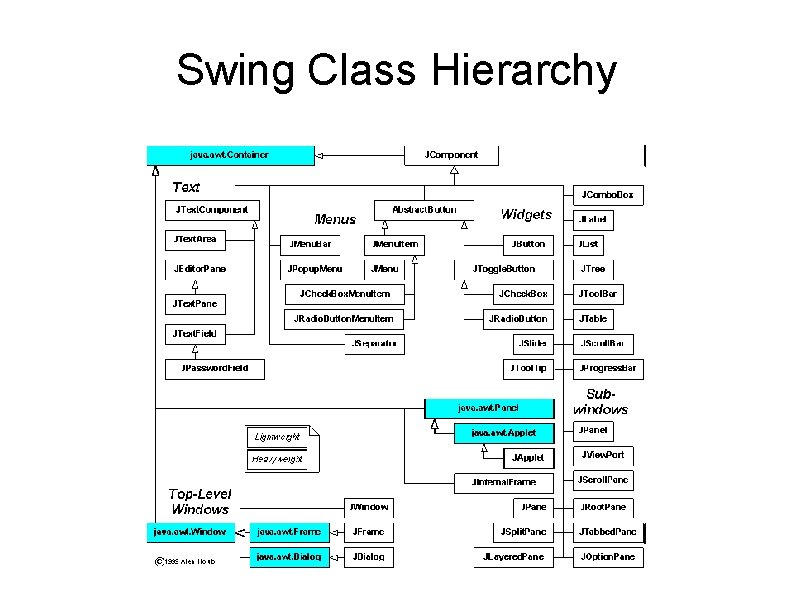
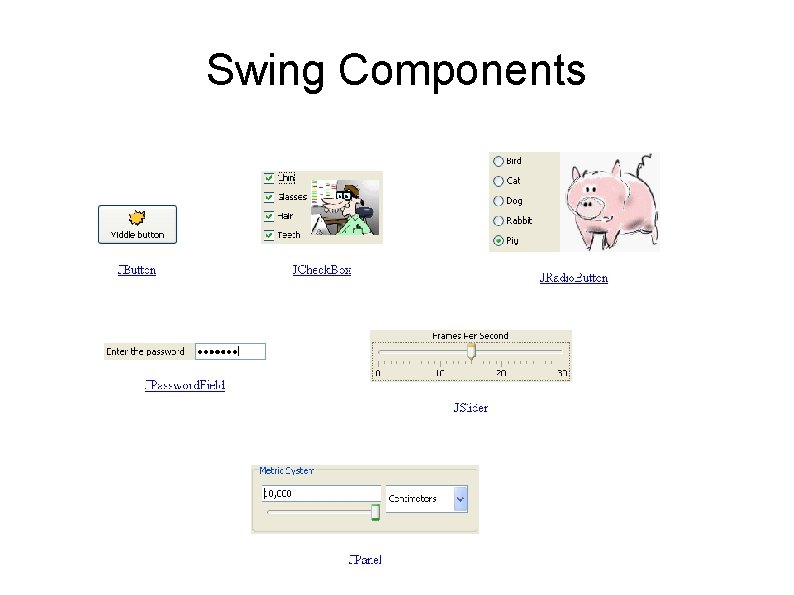
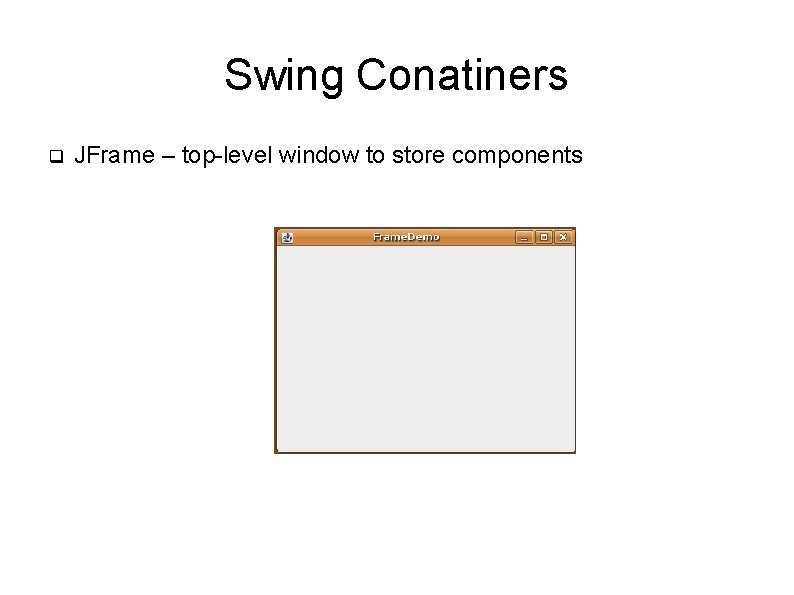
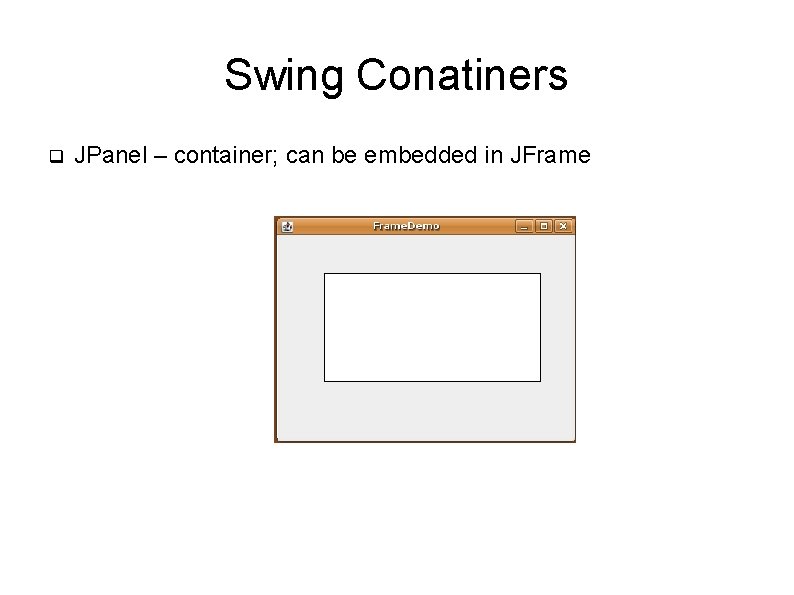
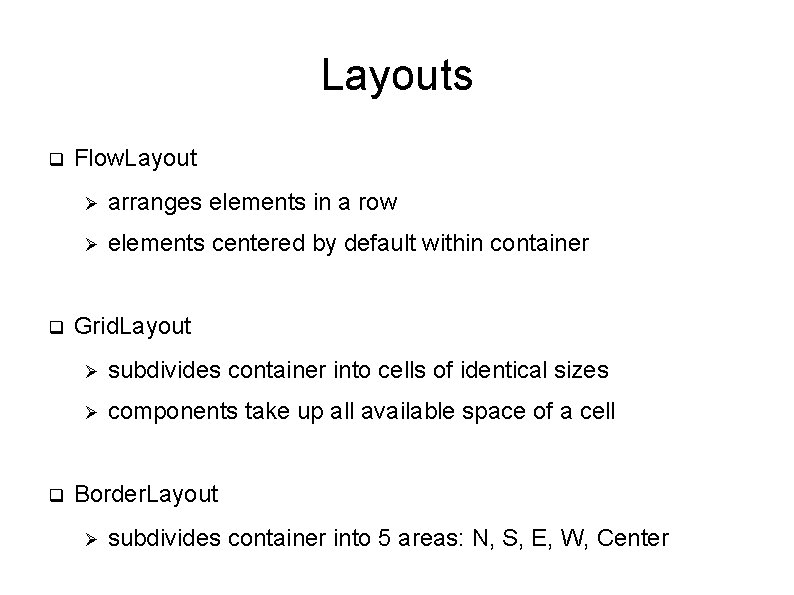
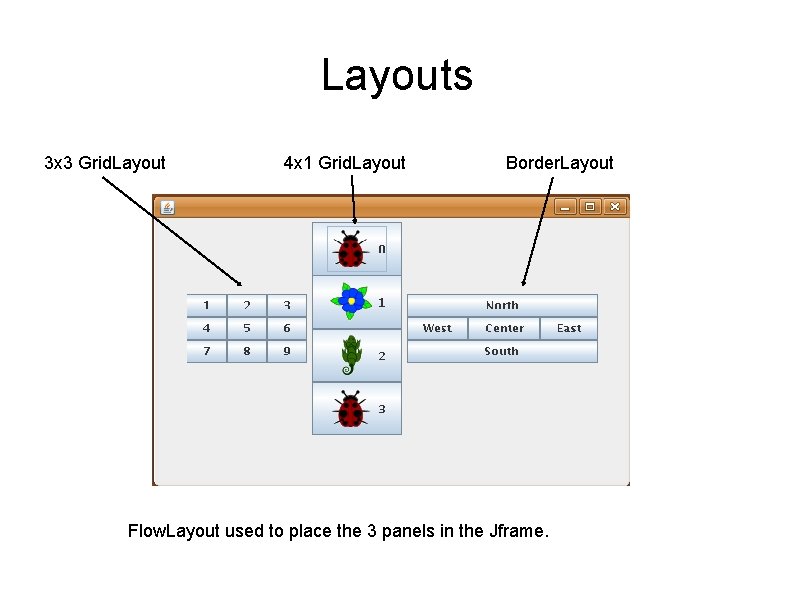
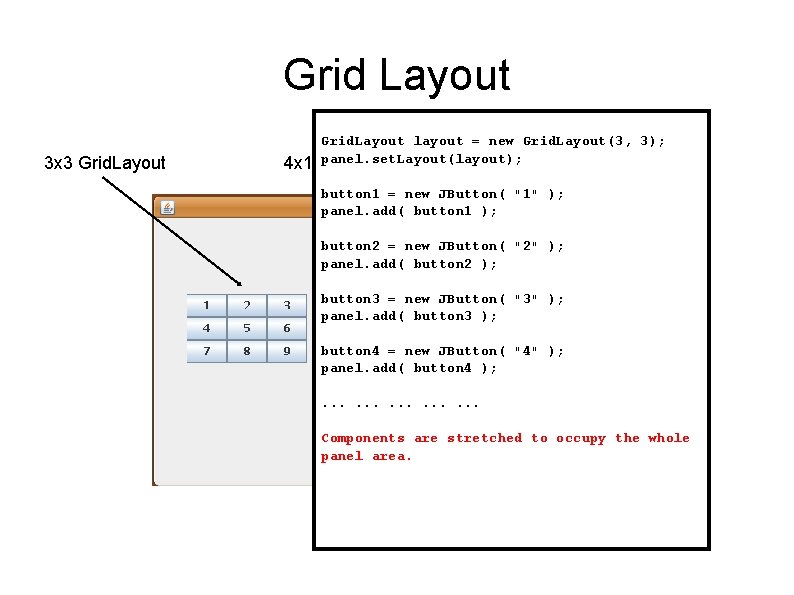
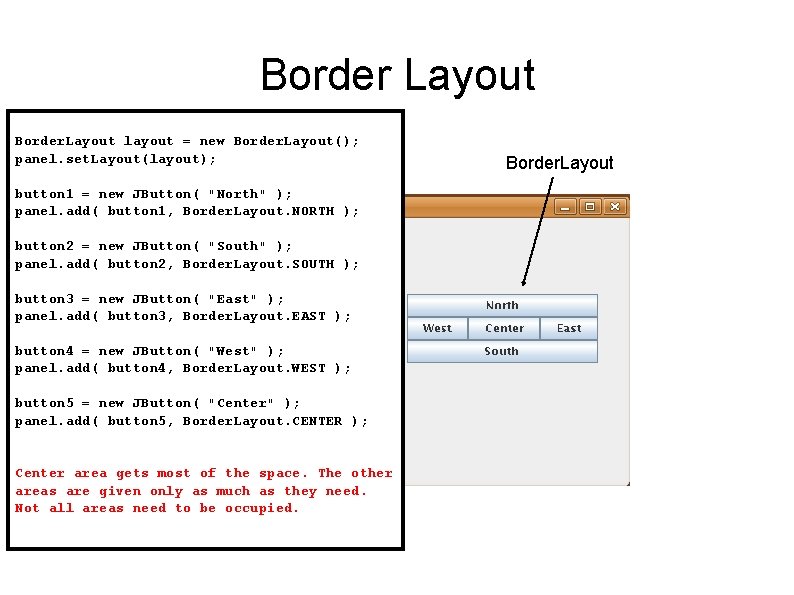
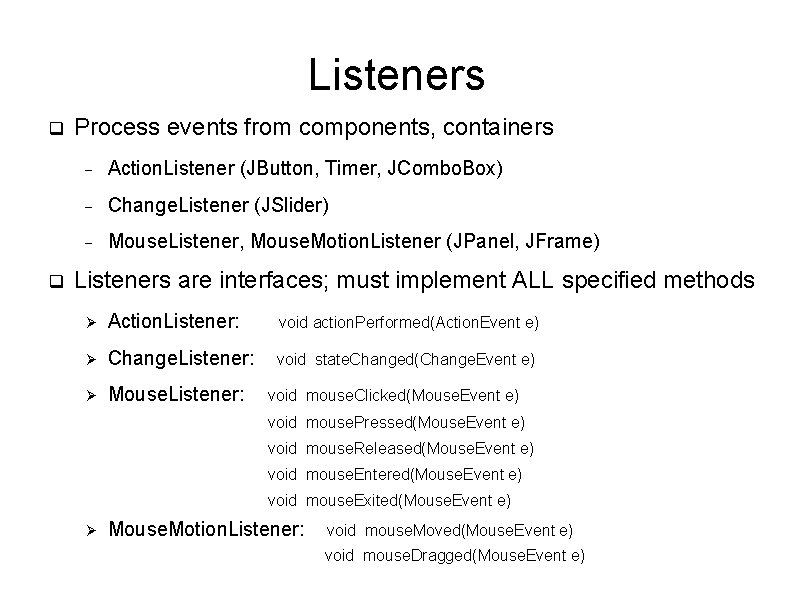
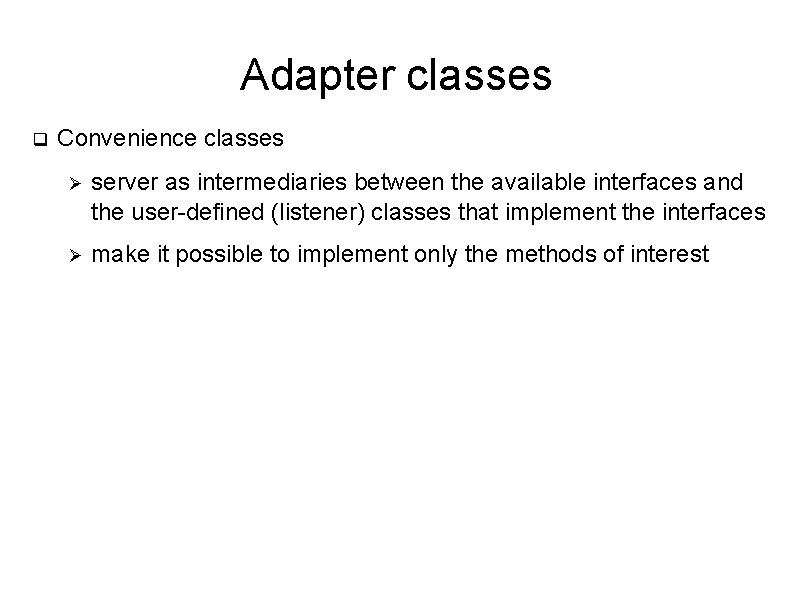
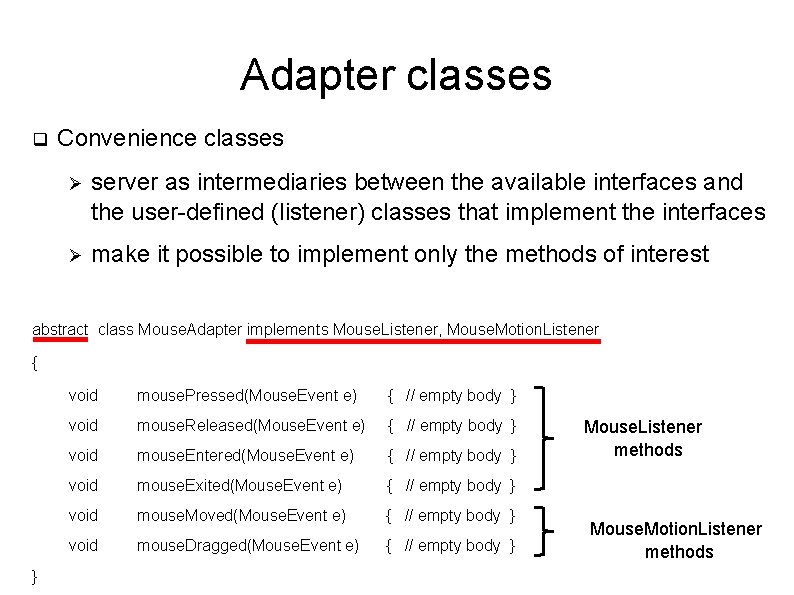
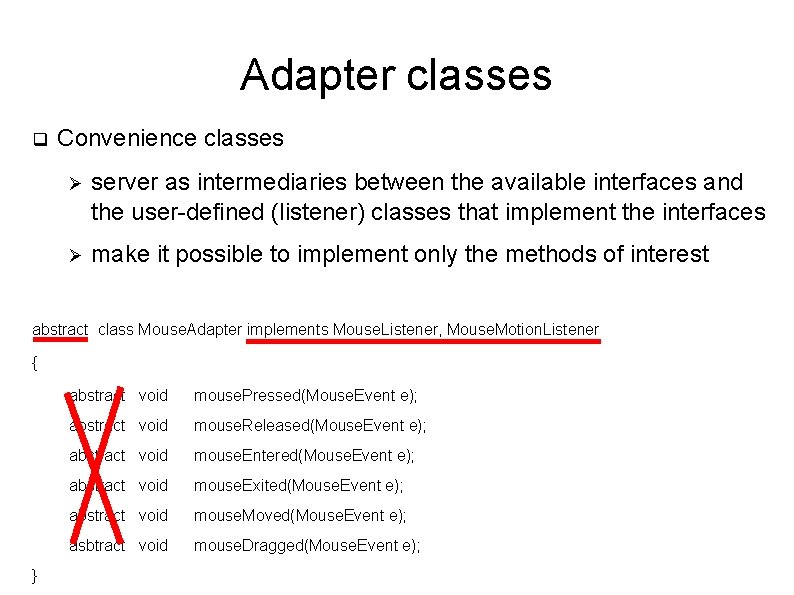
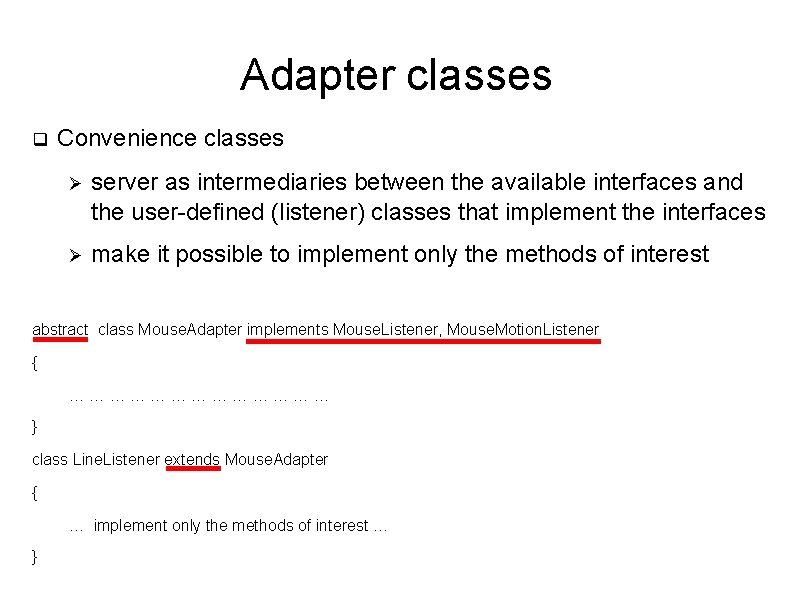
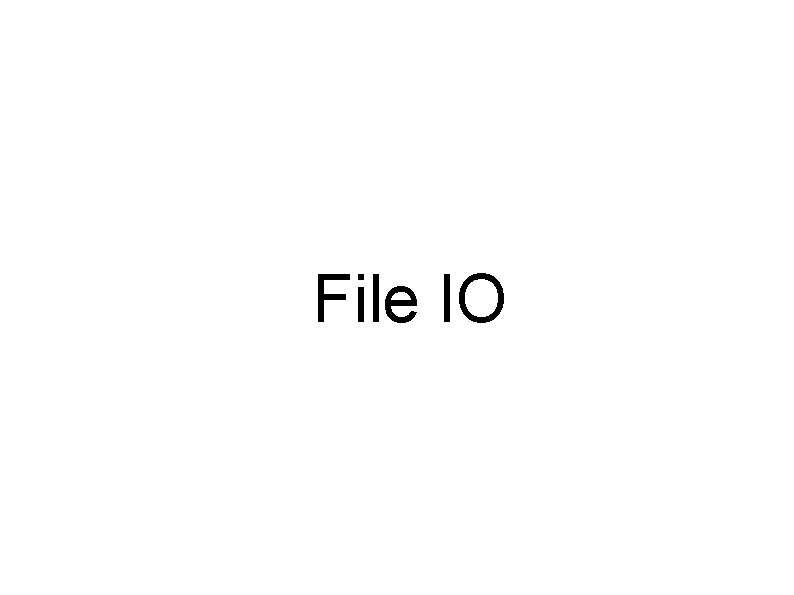
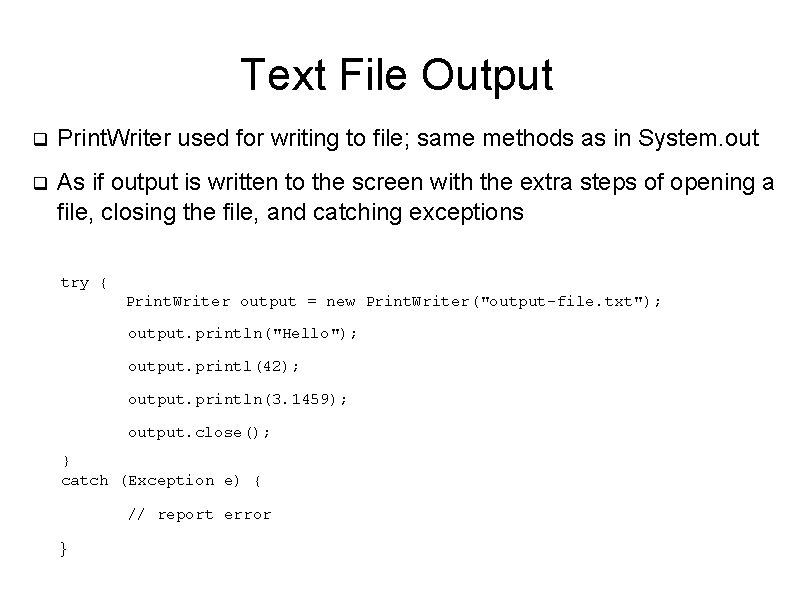
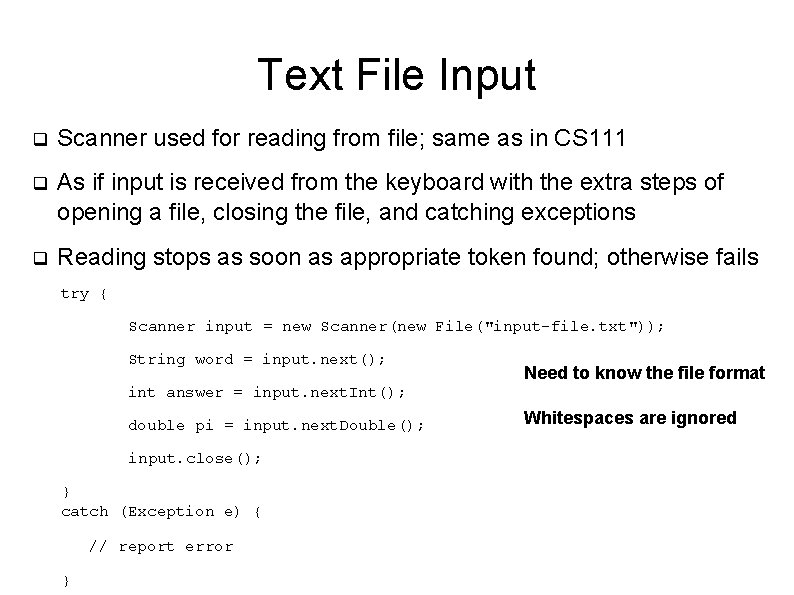
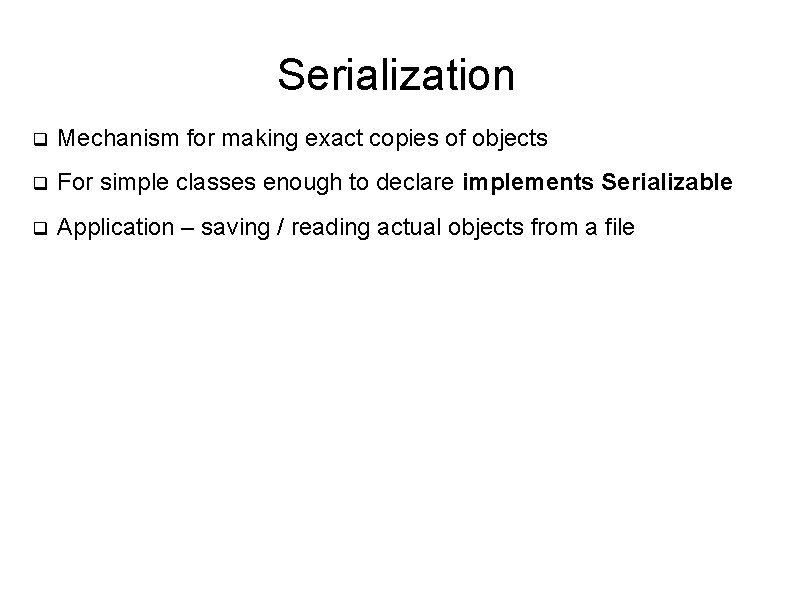
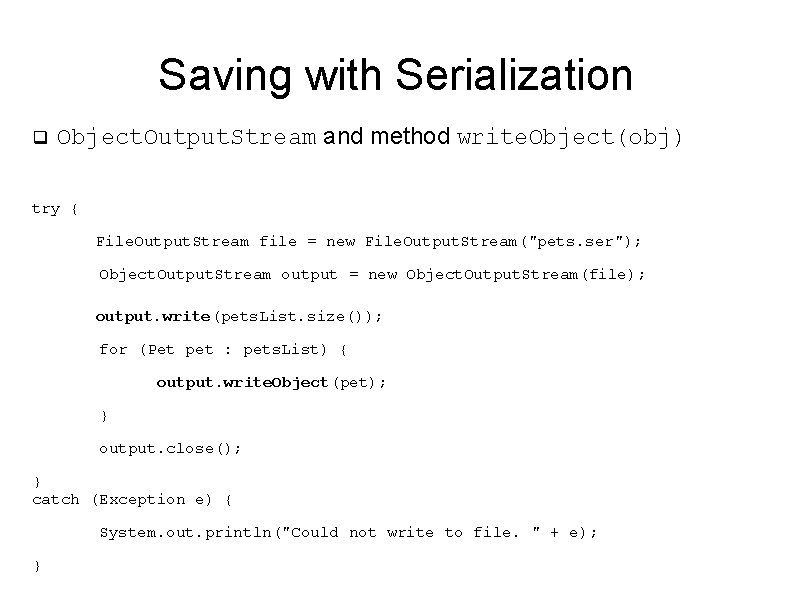
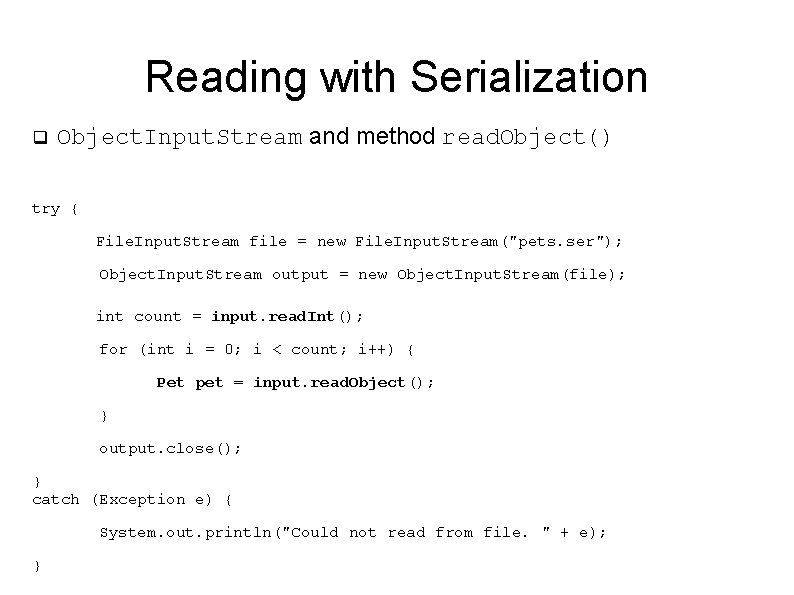
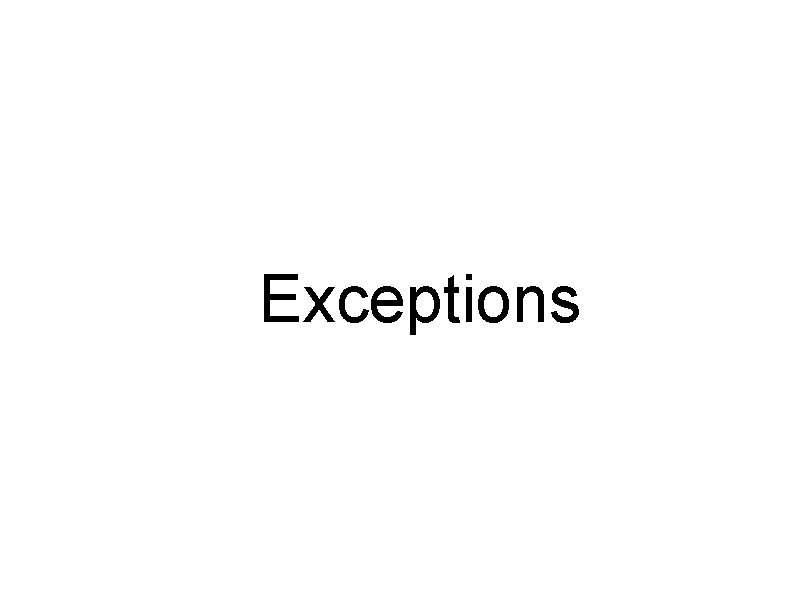
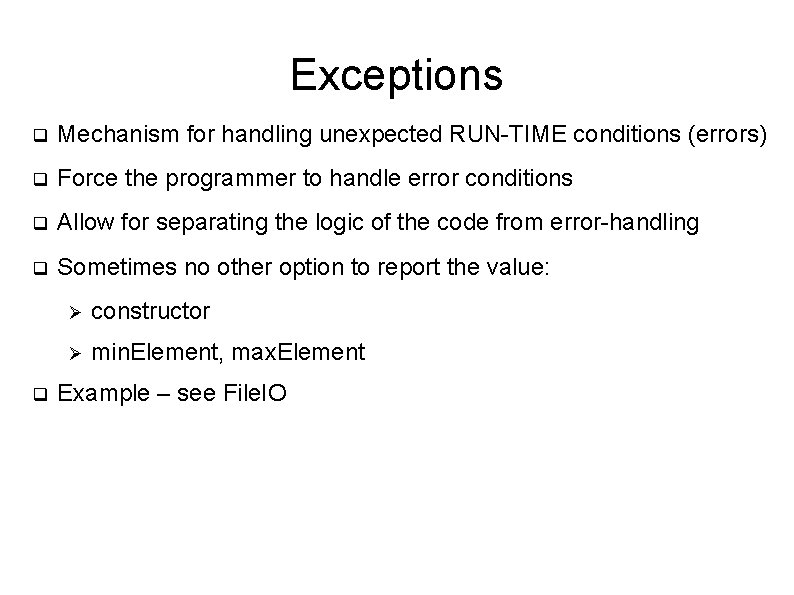
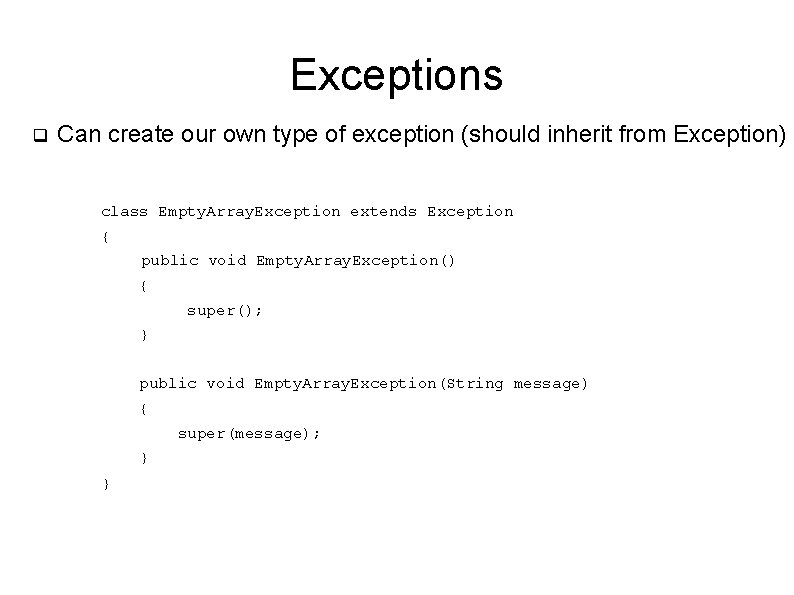
![Exceptions q Example of our own Exception --- throw/throws int min. Element(int[] numbers) throws Exceptions q Example of our own Exception --- throw/throws int min. Element(int[] numbers) throws](https://slidetodoc.com/presentation_image/3471b939acc5389f0b130cd057600e53/image-24.jpg)
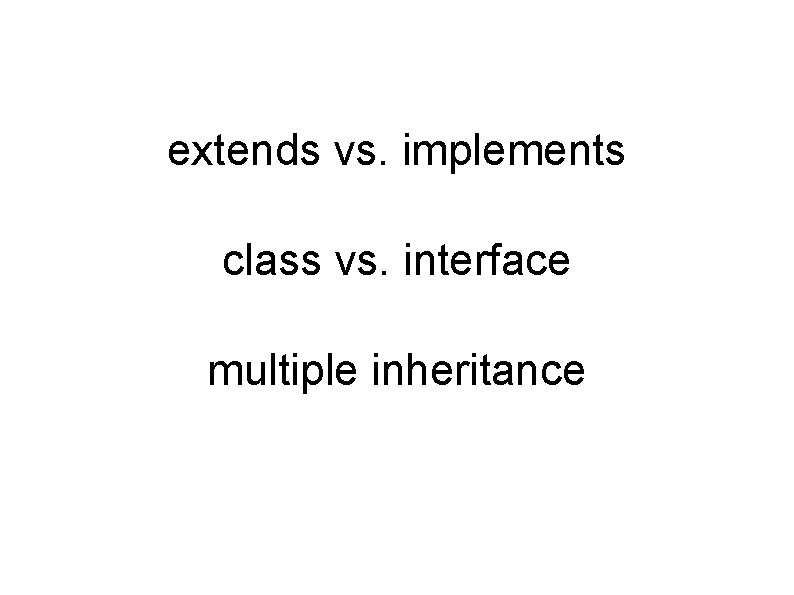
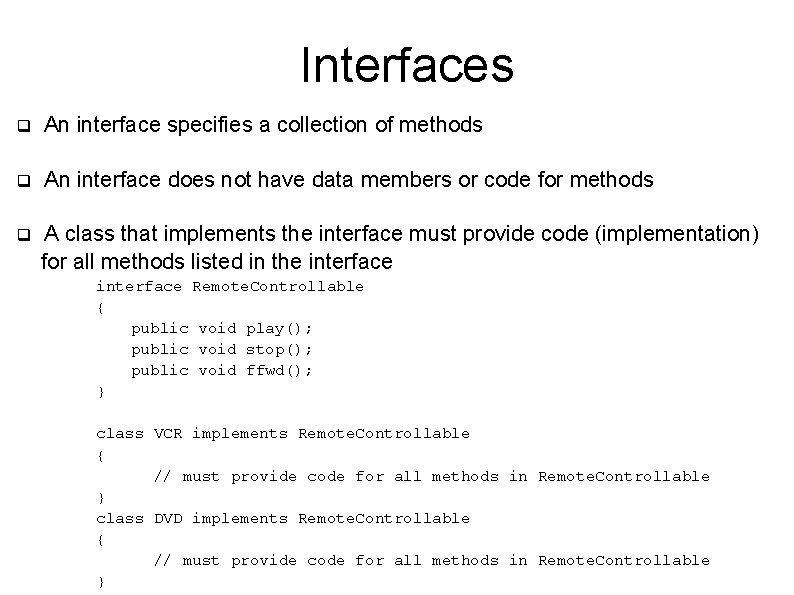
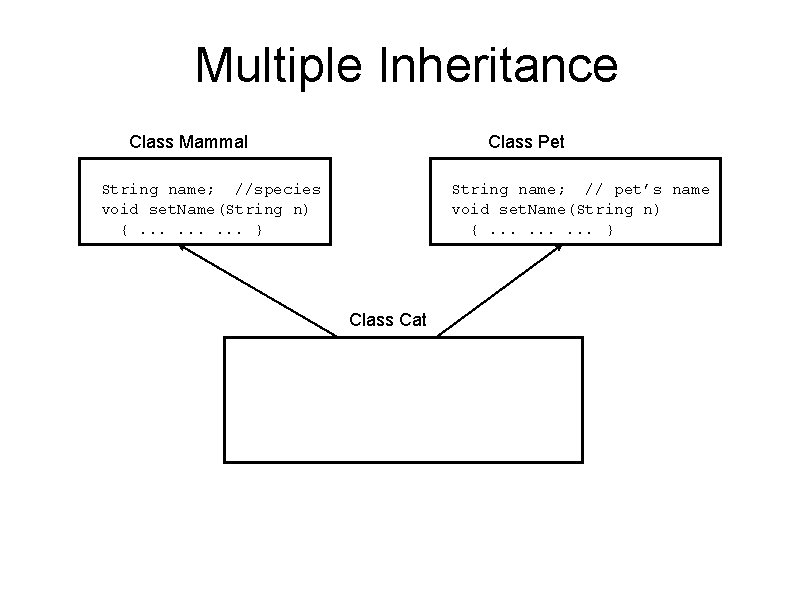
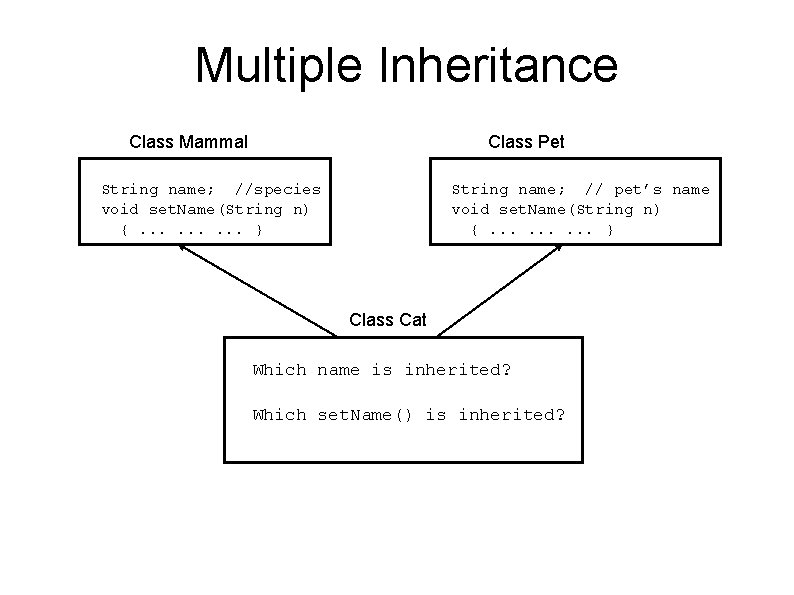
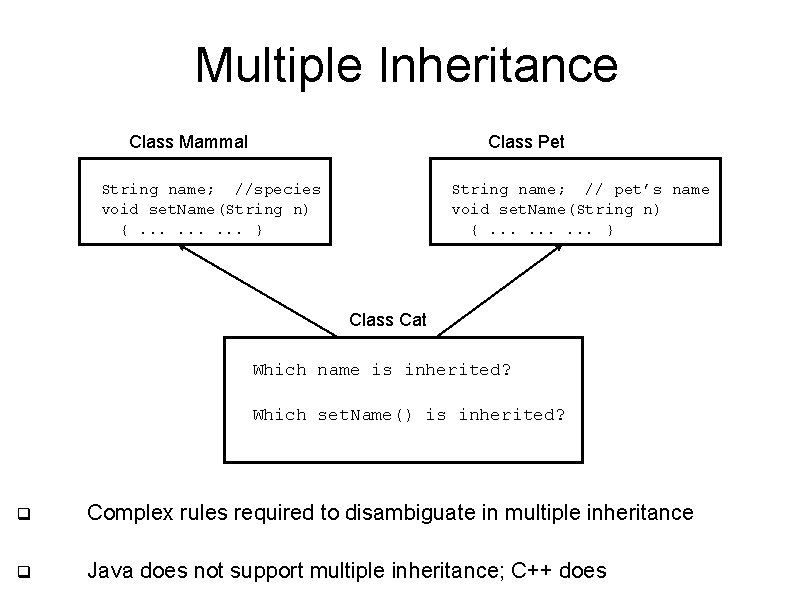
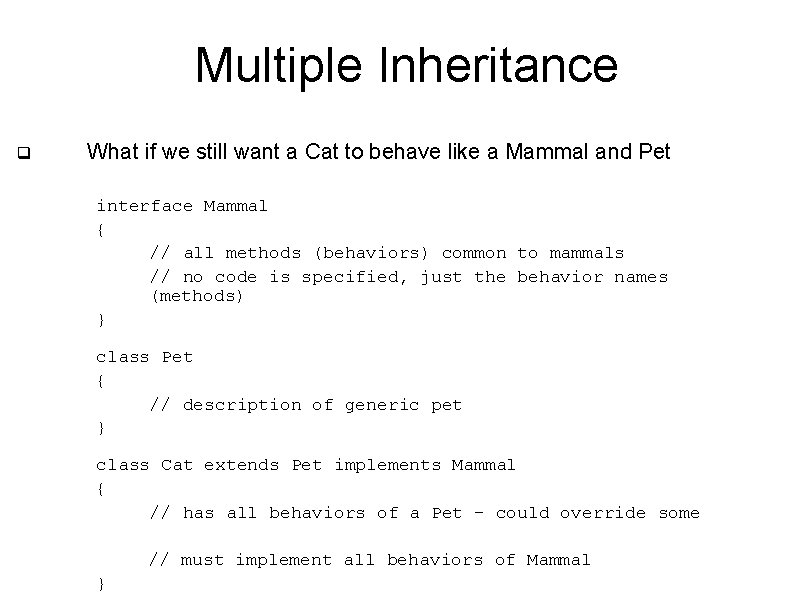
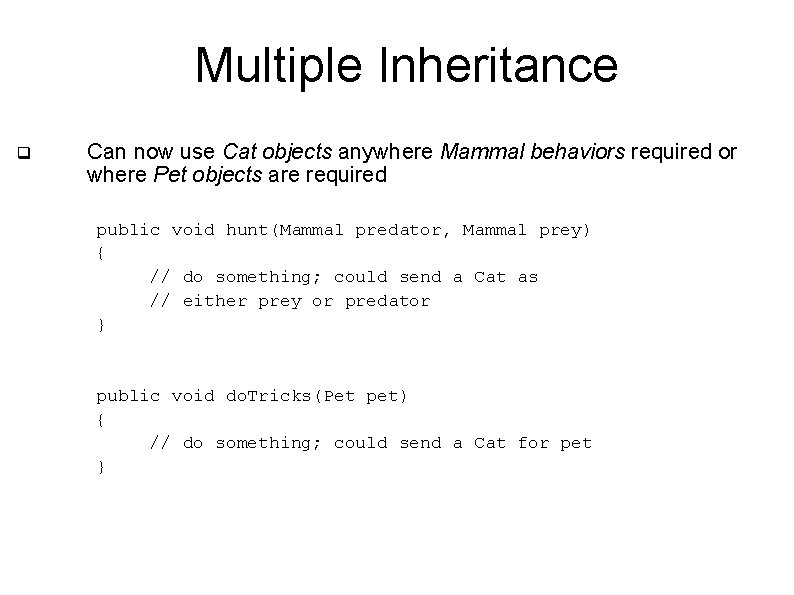
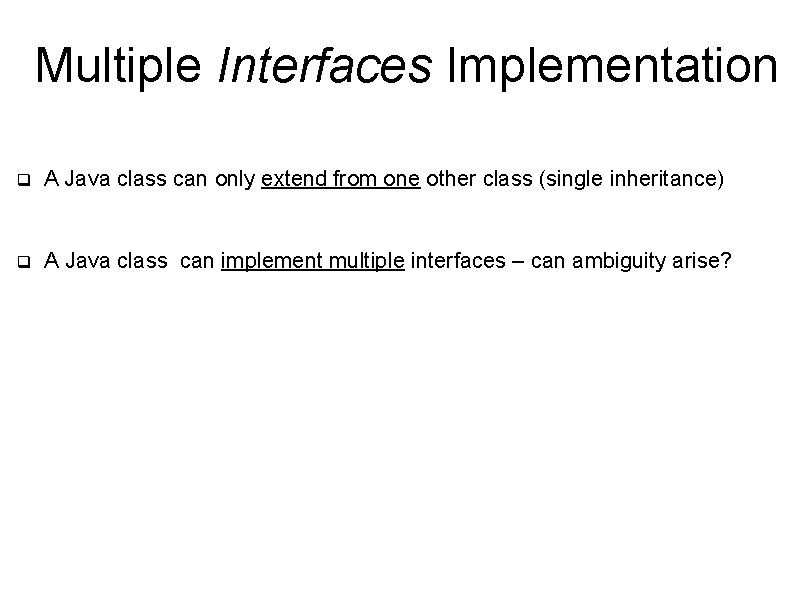
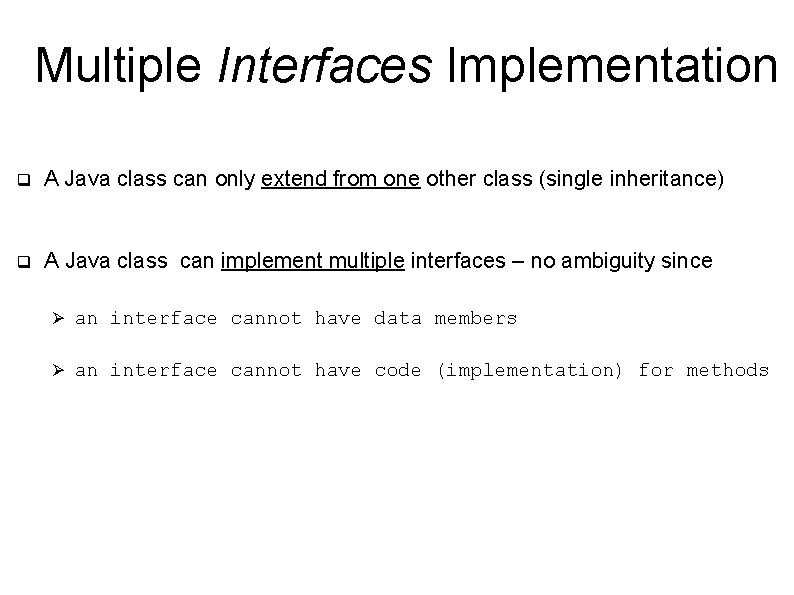
- Slides: 33
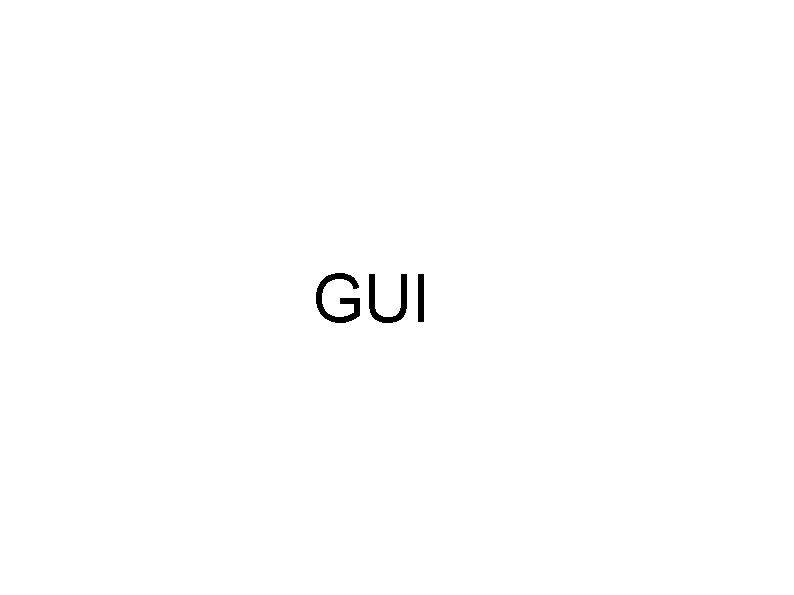
GUI
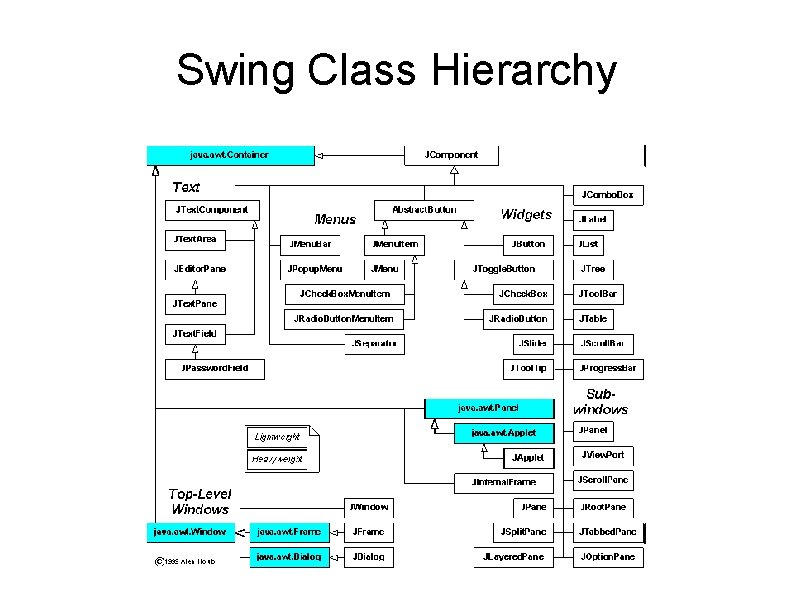
Swing Class Hierarchy
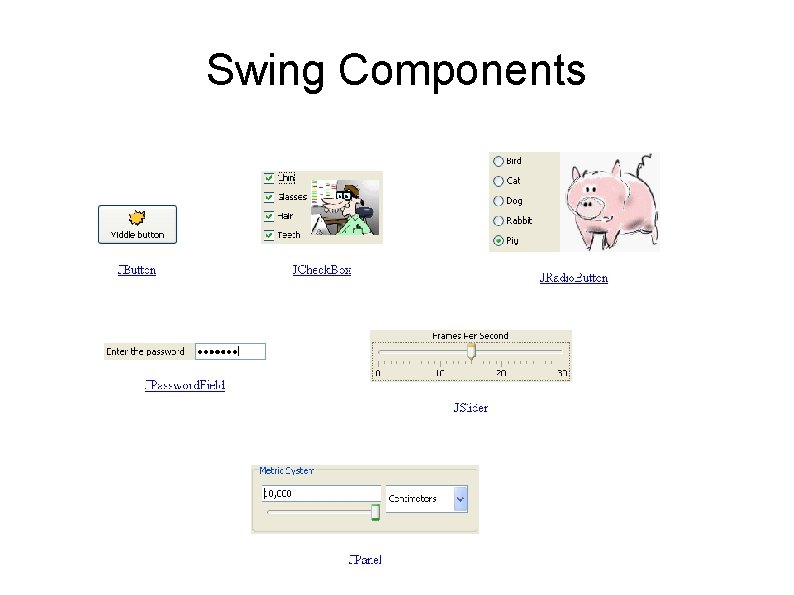
Swing Components
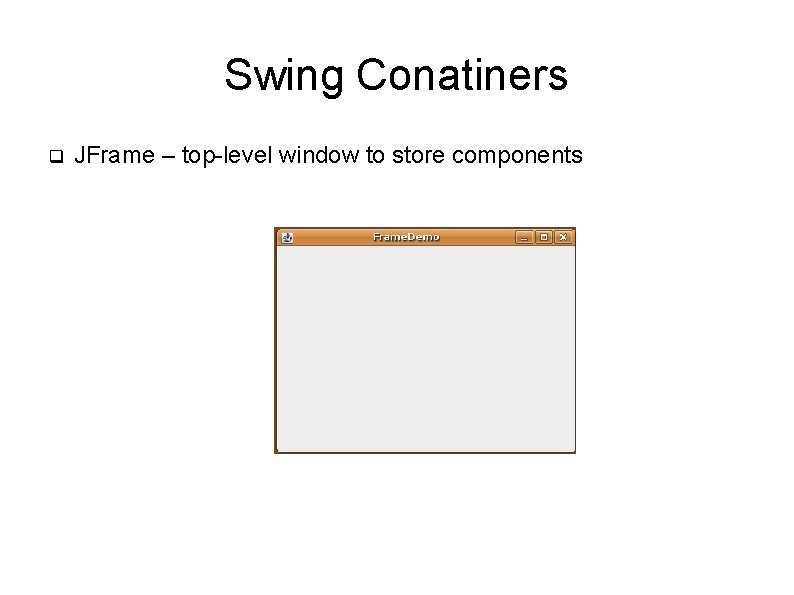
Swing Conatiners q JFrame – top-level window to store components
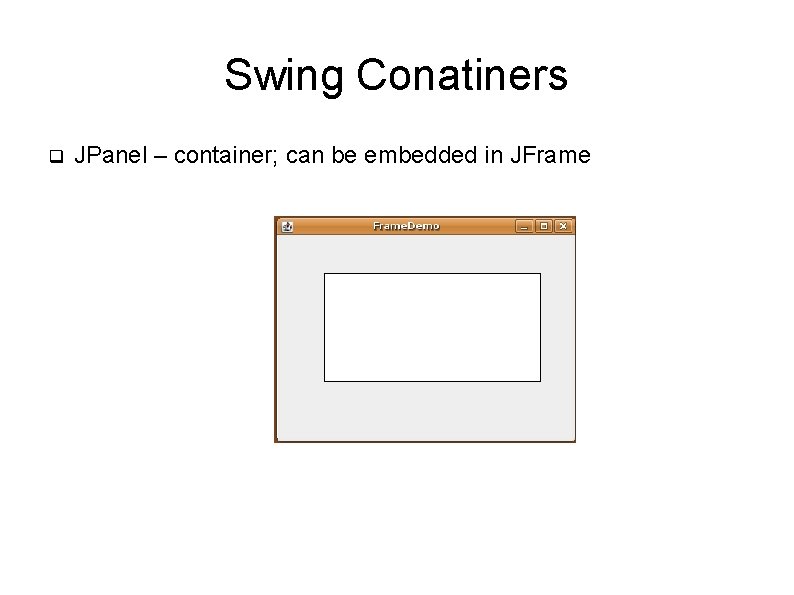
Swing Conatiners q JPanel – container; can be embedded in JFrame
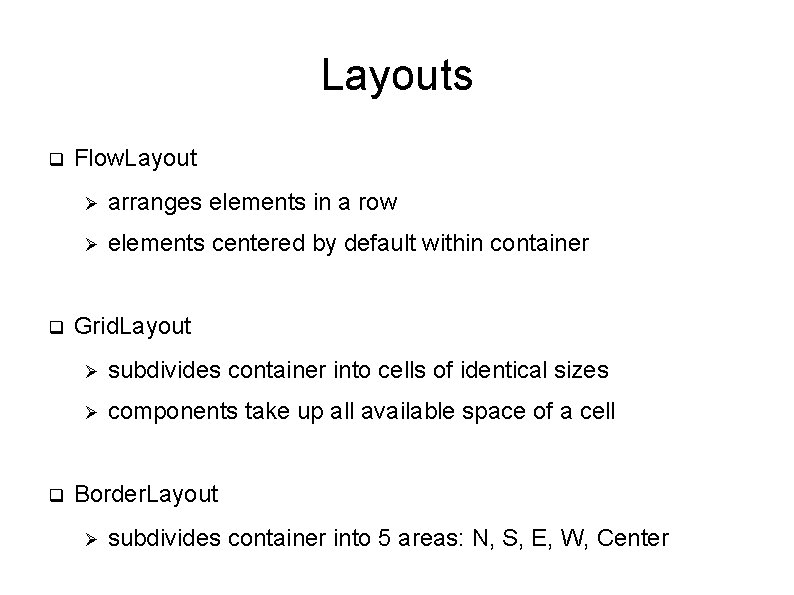
Layouts q q q Flow. Layout Ø arranges elements in a row Ø elements centered by default within container Grid. Layout Ø subdivides container into cells of identical sizes Ø components take up all available space of a cell Border. Layout Ø subdivides container into 5 areas: N, S, E, W, Center
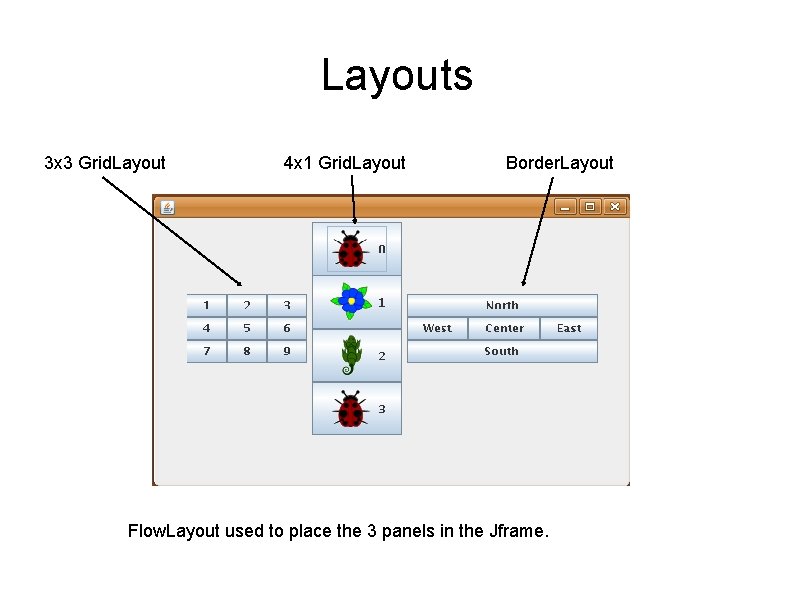
Layouts 3 x 3 Grid. Layout 4 x 1 Grid. Layout Border. Layout Flow. Layout used to place the 3 panels in the Jframe.
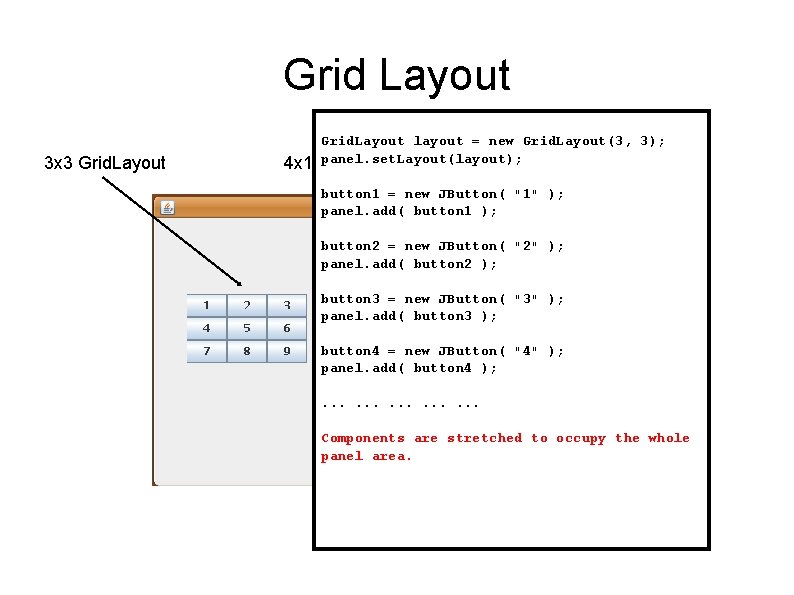
Grid Layout 3 x 3 Grid. Layout 4 x 1 Grid. Layout layout = new Grid. Layout(3, 3); panel. set. Layout(layout); Grid. Layout Border. Layout button 1 = new JButton( "1" ); panel. add( button 1 ); button 2 = new JButton( "2" ); panel. add( button 2 ); button 3 = new JButton( "3" ); panel. add( button 3 ); button 4 = new JButton( "4" ); panel. add( button 4 ); . . . . Components are stretched to occupy the whole panel area.
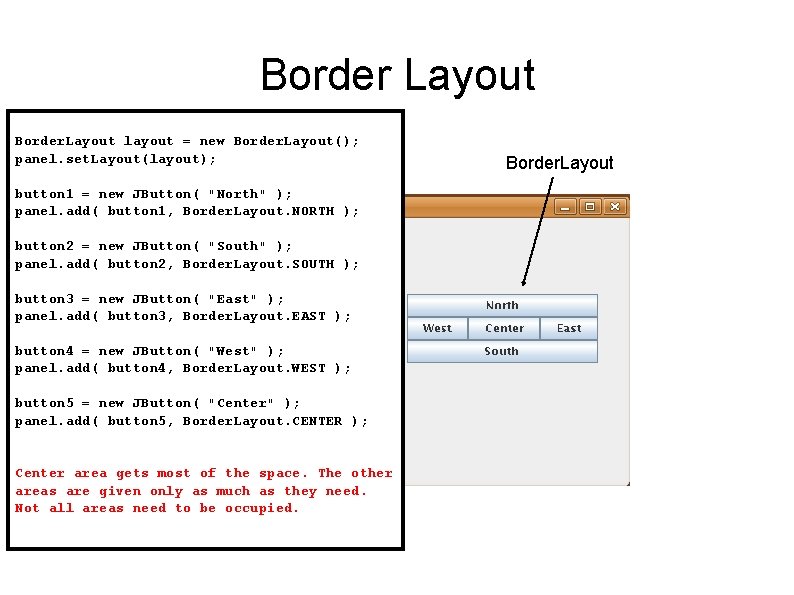
Border Layout Border. Layout layout = new Border. Layout(); panel. set. Layout(layout); 3 x 3 Grid. Layout 4 x 1 Grid. Layout button 1 = new JButton( "North" ); panel. add( button 1, Border. Layout. NORTH ); button 2 = new JButton( "South" ); panel. add( button 2, Border. Layout. SOUTH ); button 3 = new JButton( "East" ); panel. add( button 3, Border. Layout. EAST ); button 4 = new JButton( "West" ); panel. add( button 4, Border. Layout. WEST ); button 5 = new JButton( "Center" ); panel. add( button 5, Border. Layout. CENTER ); Center area gets most of the space. The other areas are given only as much as they need. Not all areas need to be occupied. Border. Layout
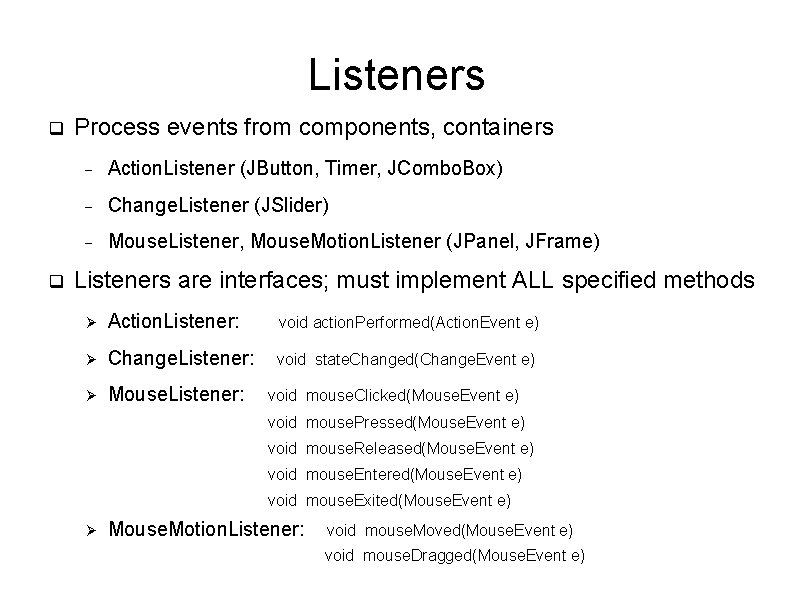
Listeners q q Process events from components, containers Action. Listener (JButton, Timer, JCombo. Box) Change. Listener (JSlider) Mouse. Listener, Mouse. Motion. Listener (JPanel, JFrame) Listeners are interfaces; must implement ALL specified methods Ø Action. Listener: void action. Performed(Action. Event e) Ø Change. Listener: void state. Changed(Change. Event e) Ø Mouse. Listener: void mouse. Clicked(Mouse. Event e) void mouse. Pressed(Mouse. Event e) void mouse. Released(Mouse. Event e) void mouse. Entered(Mouse. Event e) void mouse. Exited(Mouse. Event e) Ø Mouse. Motion. Listener: void mouse. Moved(Mouse. Event e) void mouse. Dragged(Mouse. Event e)
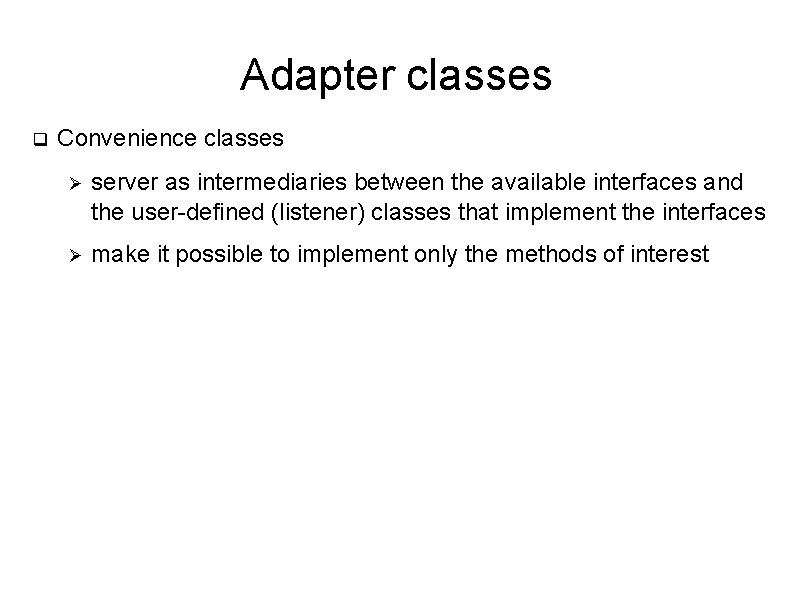
Adapter classes q Convenience classes Ø server as intermediaries between the available interfaces and the user-defined (listener) classes that implement the interfaces Ø make it possible to implement only the methods of interest
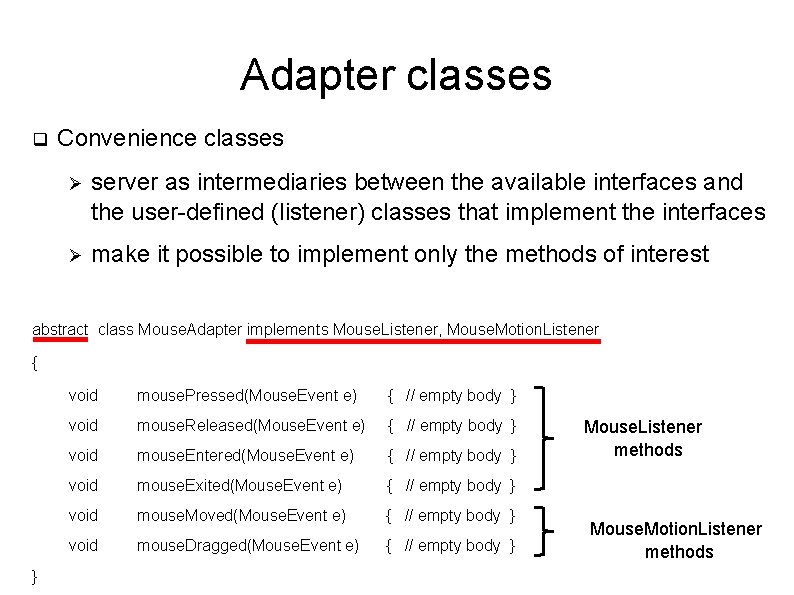
Adapter classes q Convenience classes Ø server as intermediaries between the available interfaces and the user-defined (listener) classes that implement the interfaces Ø make it possible to implement only the methods of interest abstract class Mouse. Adapter implements Mouse. Listener, Mouse. Motion. Listener { } void mouse. Pressed(Mouse. Event e) { // empty body } void mouse. Released(Mouse. Event e) { // empty body } void mouse. Entered(Mouse. Event e) { // empty body } void mouse. Exited(Mouse. Event e) { // empty body } void mouse. Moved(Mouse. Event e) { // empty body } void mouse. Dragged(Mouse. Event e) { // empty body } Mouse. Listener methods Mouse. Motion. Listener methods
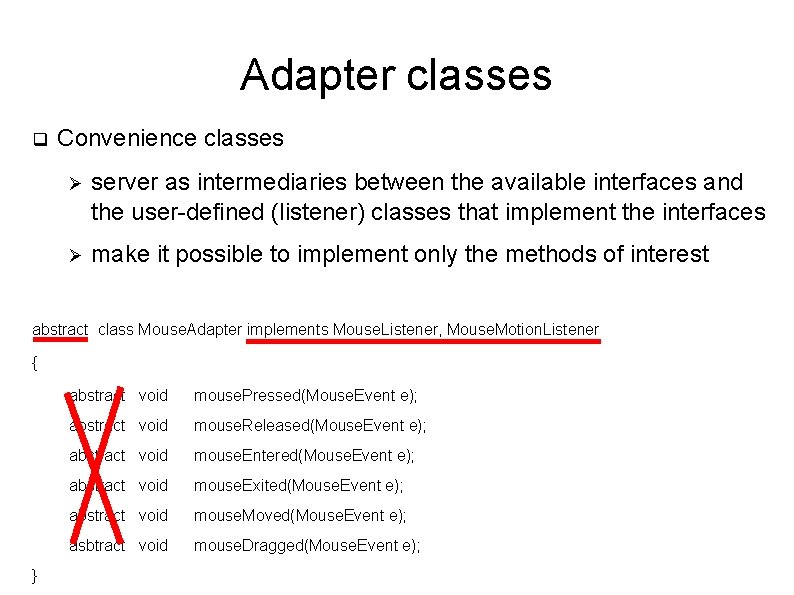
Adapter classes q Convenience classes Ø server as intermediaries between the available interfaces and the user-defined (listener) classes that implement the interfaces Ø make it possible to implement only the methods of interest abstract class Mouse. Adapter implements Mouse. Listener, Mouse. Motion. Listener { } abstract void mouse. Pressed(Mouse. Event e); abstract void mouse. Released(Mouse. Event e); abstract void mouse. Entered(Mouse. Event e); abstract void mouse. Exited(Mouse. Event e); abstract void mouse. Moved(Mouse. Event e); asbtract void mouse. Dragged(Mouse. Event e);
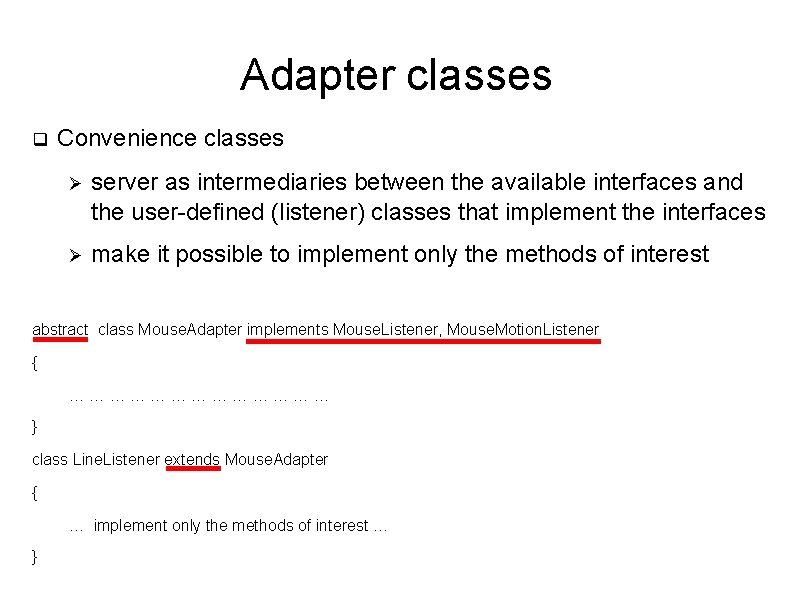
Adapter classes q Convenience classes Ø server as intermediaries between the available interfaces and the user-defined (listener) classes that implement the interfaces Ø make it possible to implement only the methods of interest abstract class Mouse. Adapter implements Mouse. Listener, Mouse. Motion. Listener { ………………… } class Line. Listener extends Mouse. Adapter { … implement only the methods of interest … }
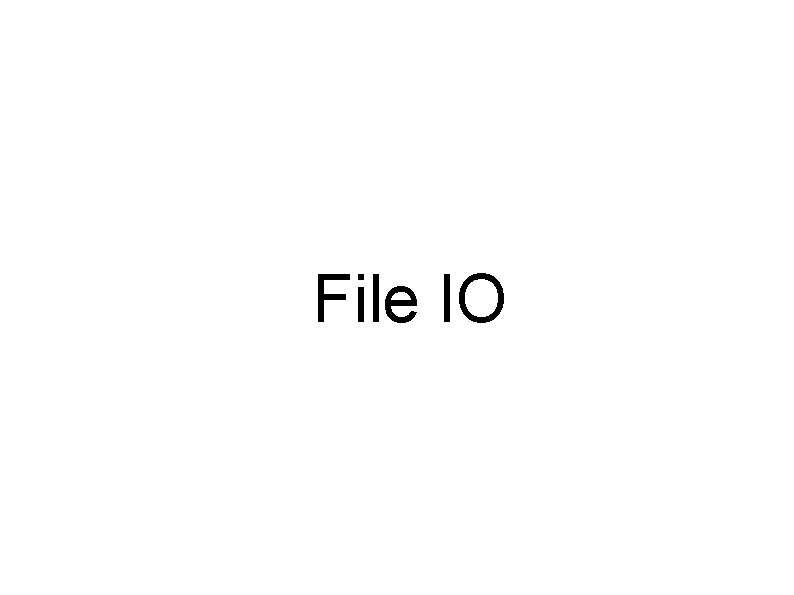
File IO
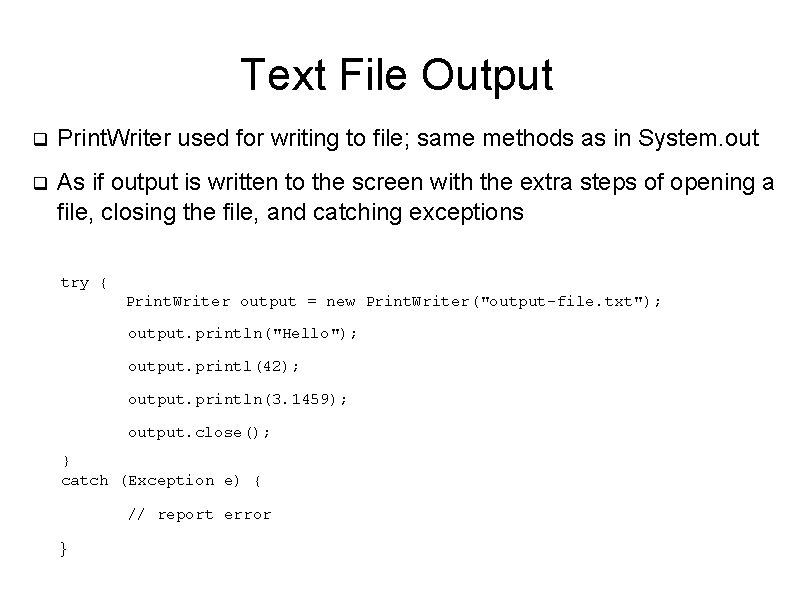
Text File Output q Print. Writer used for writing to file; same methods as in System. out q As if output is written to the screen with the extra steps of opening a file, closing the file, and catching exceptions try { Print. Writer output = new Print. Writer("output-file. txt"); output. println("Hello"); output. printl(42); output. println(3. 1459); output. close(); } catch (Exception e) { // report error }
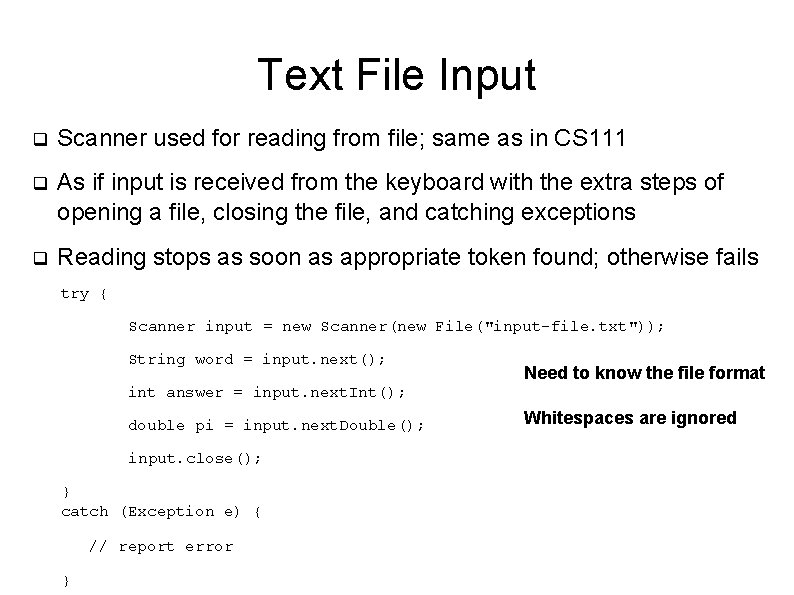
Text File Input q Scanner used for reading from file; same as in CS 111 q As if input is received from the keyboard with the extra steps of opening a file, closing the file, and catching exceptions q Reading stops as soon as appropriate token found; otherwise fails try { Scanner input = new Scanner(new File("input-file. txt")); String word = input. next(); Need to know the file format int answer = input. next. Int(); double pi = input. next. Double(); input. close(); } catch (Exception e) { // report error } Whitespaces are ignored
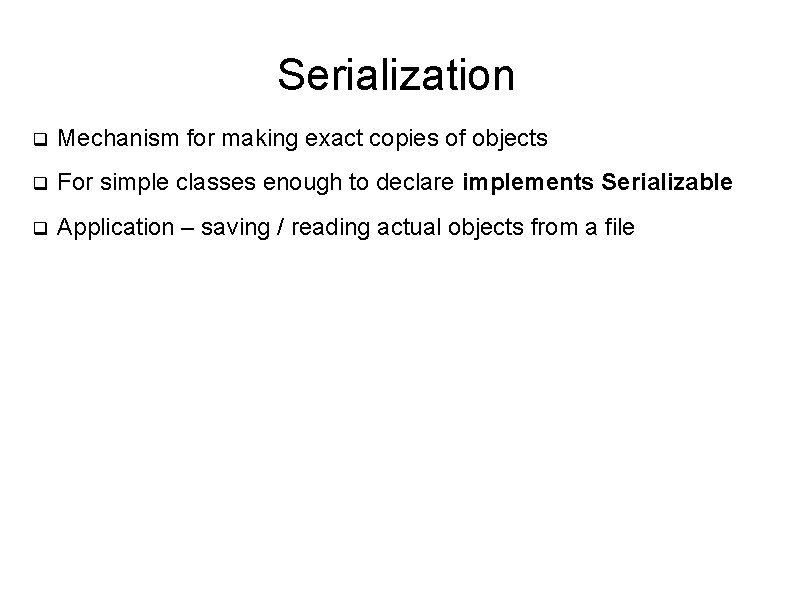
Serialization q Mechanism for making exact copies of objects q For simple classes enough to declare implements Serializable q Application – saving / reading actual objects from a file
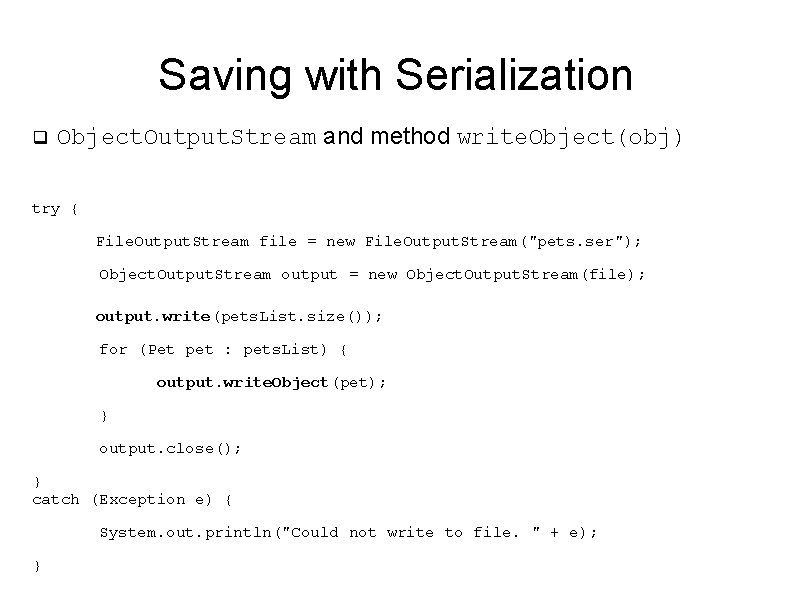
Saving with Serialization q Object. Output. Stream and method write. Object(obj) try { File. Output. Stream file = new File. Output. Stream("pets. ser"); Object. Output. Stream output = new Object. Output. Stream(file); output. write(pets. List. size()); for (Pet pet : pets. List) { output. write. Object(pet); } output. close(); } catch (Exception e) { System. out. println("Could not write to file. " + e); }
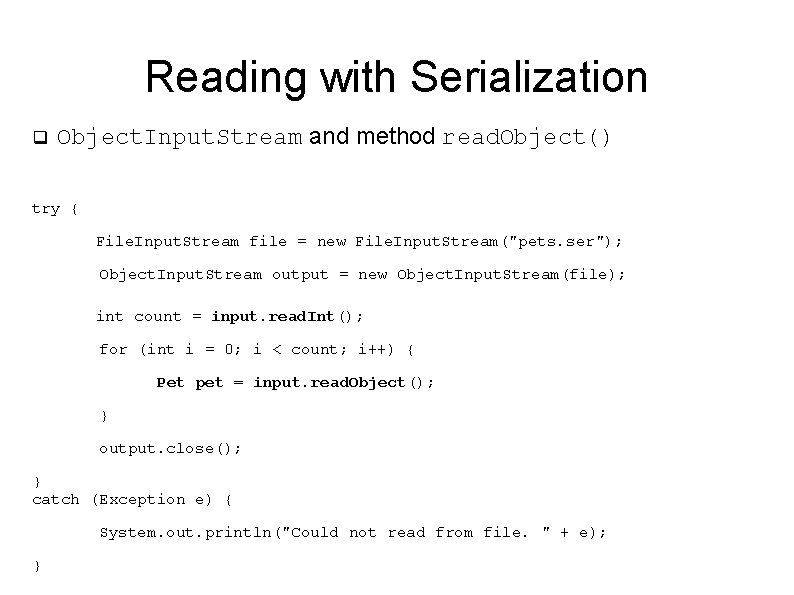
Reading with Serialization q Object. Input. Stream and method read. Object() try { File. Input. Stream file = new File. Input. Stream("pets. ser"); Object. Input. Stream output = new Object. Input. Stream(file); int count = input. read. Int(); for (int i = 0; i < count; i++) { Pet pet = input. read. Object(); } output. close(); } catch (Exception e) { System. out. println("Could not read from file. " + e); }
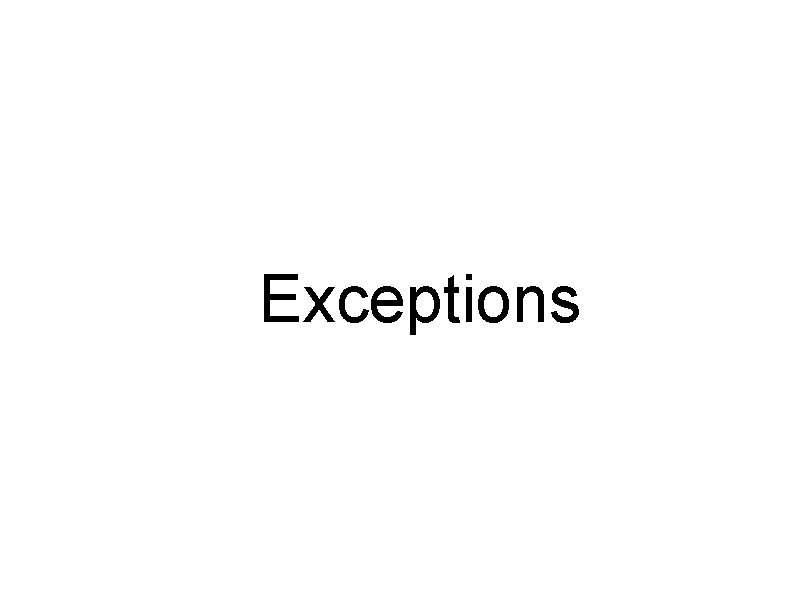
Exceptions
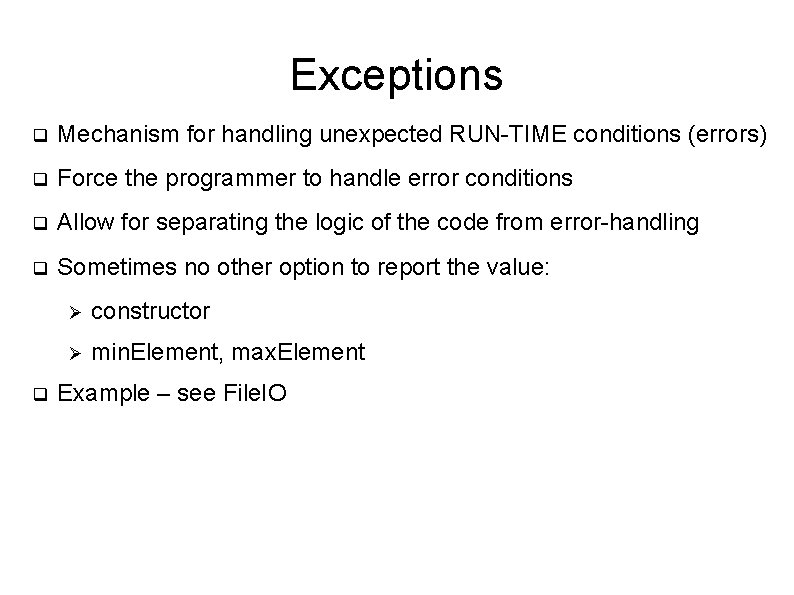
Exceptions q Mechanism for handling unexpected RUN-TIME conditions (errors) q Force the programmer to handle error conditions q Allow for separating the logic of the code from error-handling q Sometimes no other option to report the value: q Ø constructor Ø min. Element, max. Element Example – see File. IO
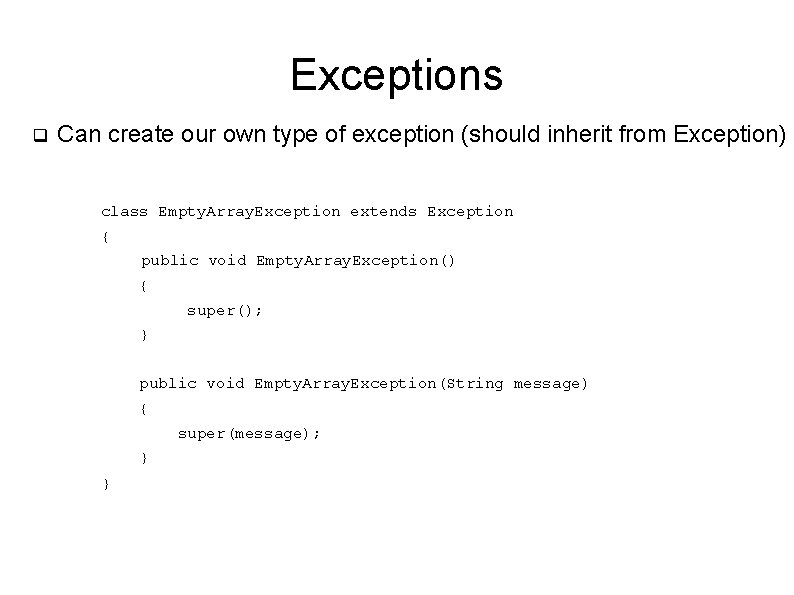
Exceptions q Can create our own type of exception (should inherit from Exception) class Empty. Array. Exception extends Exception { public void Empty. Array. Exception() { super(); } public void Empty. Array. Exception(String message) { super(message); } }
![Exceptions q Example of our own Exception throwthrows int min Elementint numbers throws Exceptions q Example of our own Exception --- throw/throws int min. Element(int[] numbers) throws](https://slidetodoc.com/presentation_image/3471b939acc5389f0b130cd057600e53/image-24.jpg)
Exceptions q Example of our own Exception --- throw/throws int min. Element(int[] numbers) throws Empty. Array. Exception { // empty array --- throw an exception if (numbers. length == 0) { throw Empty. Array. Exception(“Empty array given”); } // //. . . compute smallest element. . . // }
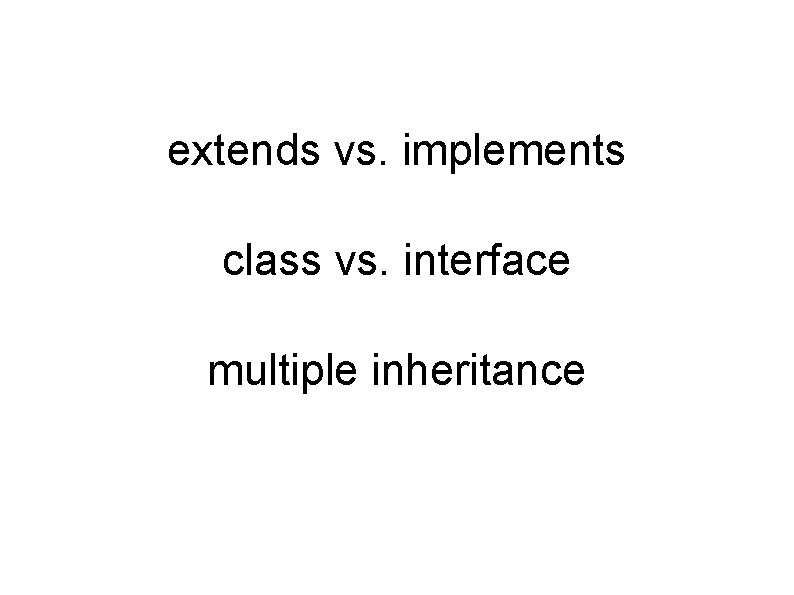
extends vs. implements class vs. interface multiple inheritance
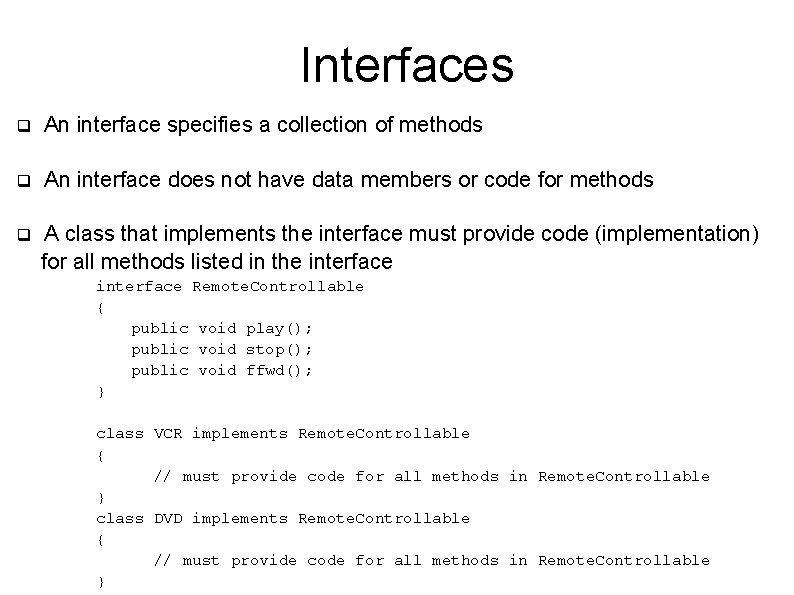
Interfaces q An interface specifies a collection of methods q An interface does not have data members or code for methods q A class that implements the interface must provide code (implementation) for all methods listed in the interface Remote. Controllable { public void play(); public void stop(); public void ffwd(); } class VCR implements Remote. Controllable { // must provide code for all methods in Remote. Controllable } class DVD implements Remote. Controllable { // must provide code for all methods in Remote. Controllable }
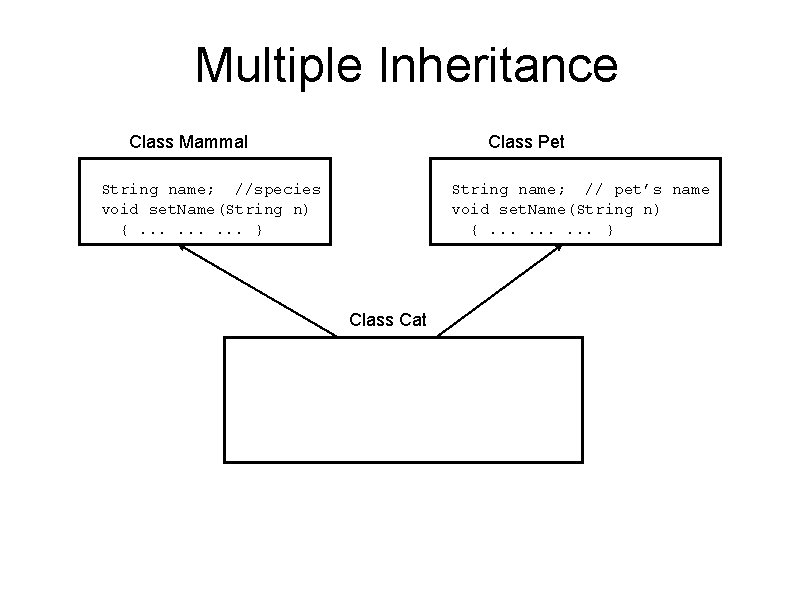
Multiple Inheritance Class Mammal Class Pet String name; //species void set. Name(String n) {. . } String name; // pet’s name void set. Name(String n) {. . } Class Cat
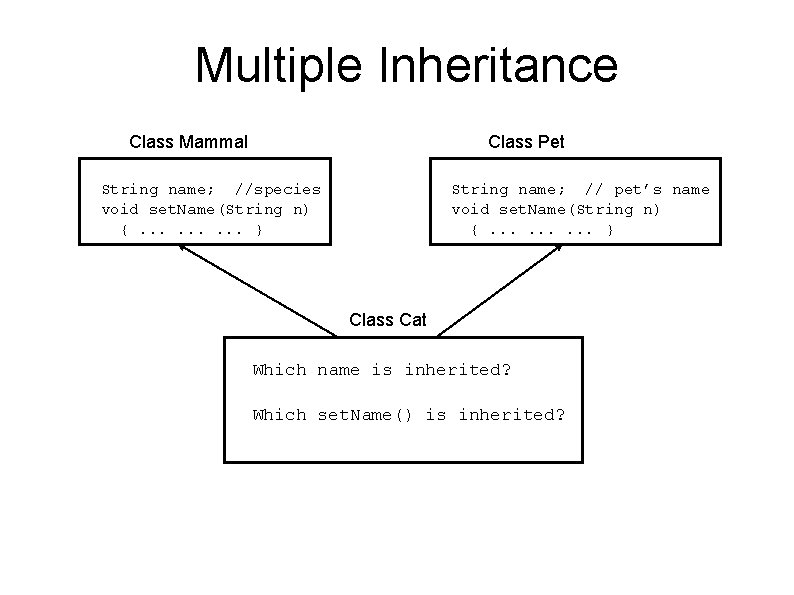
Multiple Inheritance Class Mammal Class Pet String name; //species void set. Name(String n) {. . } String name; // pet’s name void set. Name(String n) {. . } Class Cat Which name is inherited? Which set. Name() is inherited?
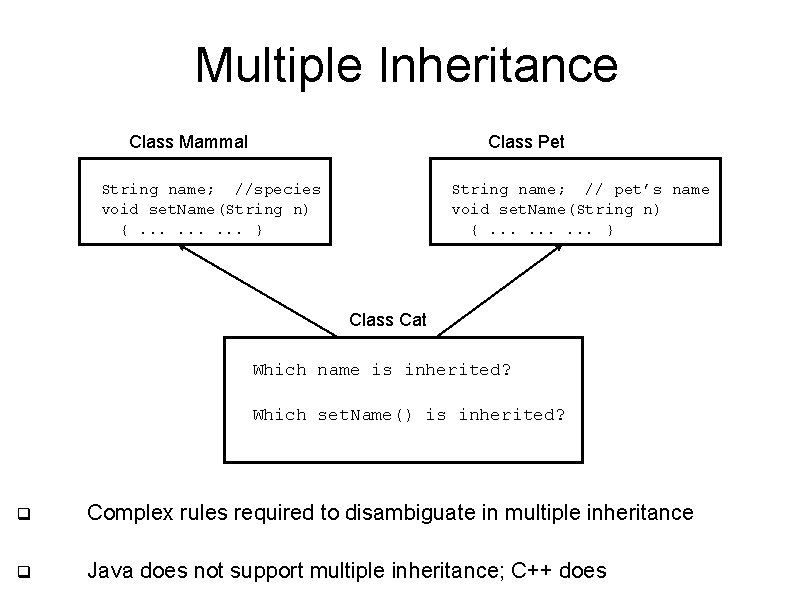
Multiple Inheritance Class Mammal Class Pet String name; //species void set. Name(String n) {. . } String name; // pet’s name void set. Name(String n) {. . } Class Cat Which name is inherited? Which set. Name() is inherited? q Complex rules required to disambiguate in multiple inheritance q Java does not support multiple inheritance; C++ does
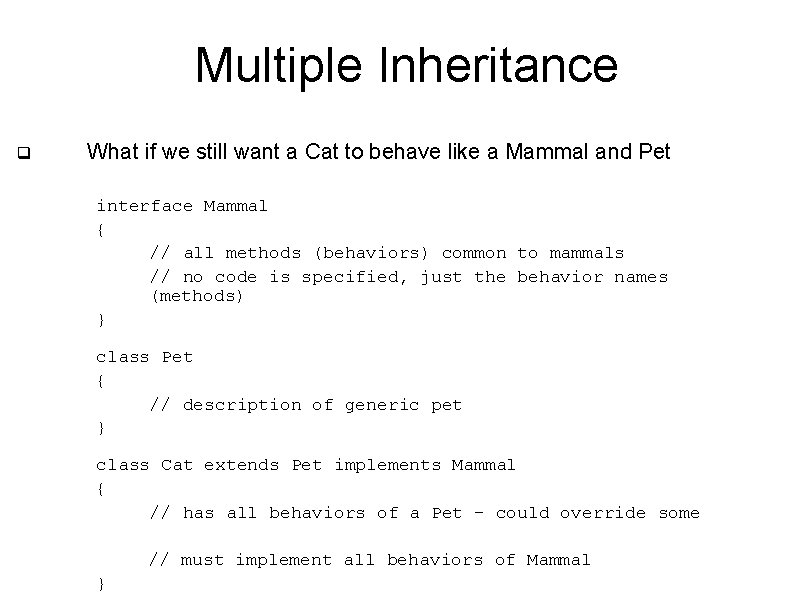
Multiple Inheritance q What if we still want a Cat to behave like a Mammal and Pet interface Mammal { // all methods (behaviors) common to mammals // no code is specified, just the behavior names (methods) } class Pet { // description of generic pet } class Cat extends Pet implements Mammal { // has all behaviors of a Pet – could override some // must implement all behaviors of Mammal }
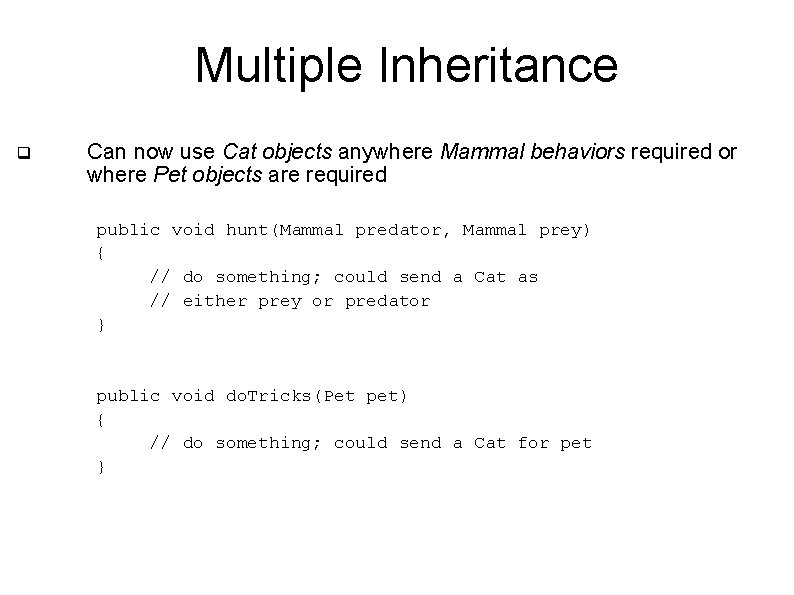
Multiple Inheritance q Can now use Cat objects anywhere Mammal behaviors required or where Pet objects are required public void hunt(Mammal predator, Mammal prey) { // do something; could send a Cat as // either prey or predator } public void do. Tricks(Pet pet) { // do something; could send a Cat for pet }
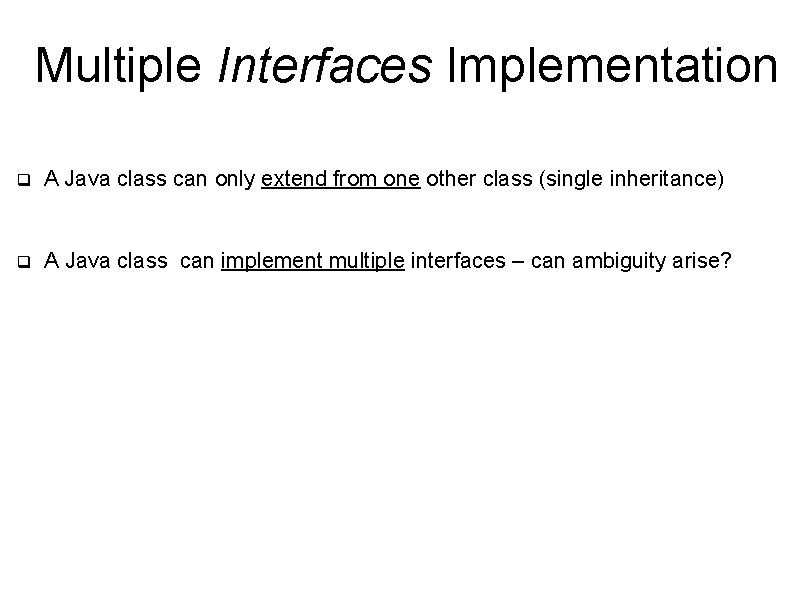
Multiple Interfaces Implementation q A Java class can only extend from one other class (single inheritance) q A Java class can implement multiple interfaces – can ambiguity arise?
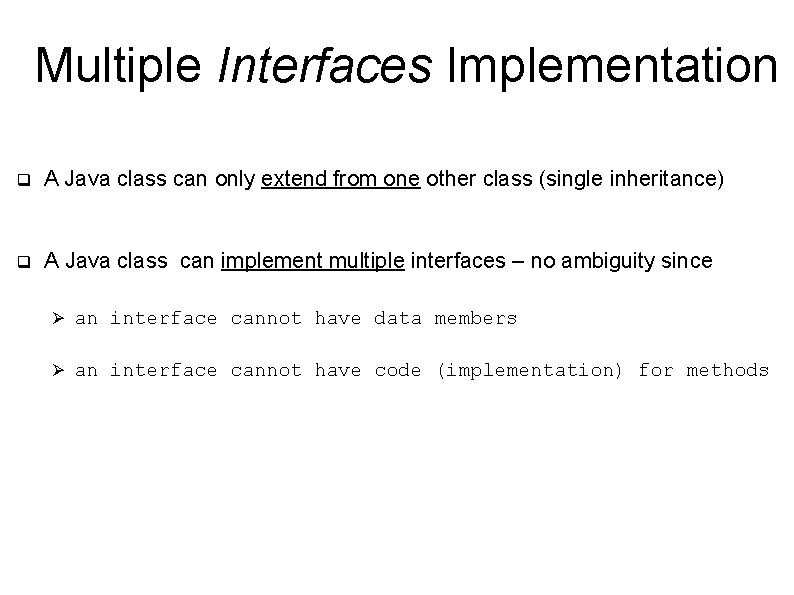
Multiple Interfaces Implementation q A Java class can only extend from one other class (single inheritance) q A Java class can implement multiple interfaces – no ambiguity since Ø an interface cannot have data members Ø an interface cannot have code (implementation) for methods
Swing hierarchy
Ge gi gue gui güe güi
Swing gui designer
Java swing responsive
Containment hierarchy
Gui components
Gui components
Components of swing
Boxlayout swing
Gui programmierung java
Thread class hierarchy in java
8wpf
Java exception hierarchy
Holy roman empire social structure
Haskell class hierarchy
Ileana ober
In today's class
Putting a package together
Abstract class vs concrete class
Lower boundary of modal class
Class i vs class ii mhc
Difference between abstract class and concrete class
Class width
Response class vs stimulus class
Response class vs stimulus class
Therapeutic class and pharmacologic class
Class maths student student1 class student string name
Categorical frequency distribution example
In greenfoot, you can cast an actor class to a world class?
Static vs dynamic class loading in java
Class 0 esd
Static uml diagrams
Class 2 class 3
Public class test subject extends test class