GUI Programming I Practice Session Exercise Please Log
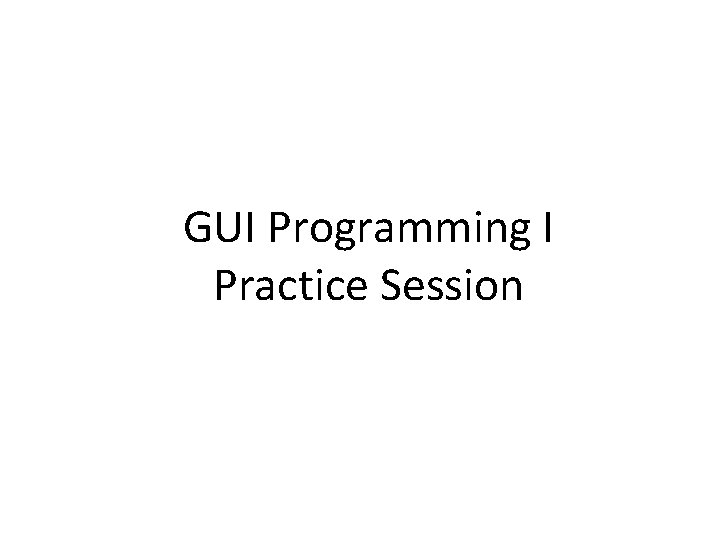
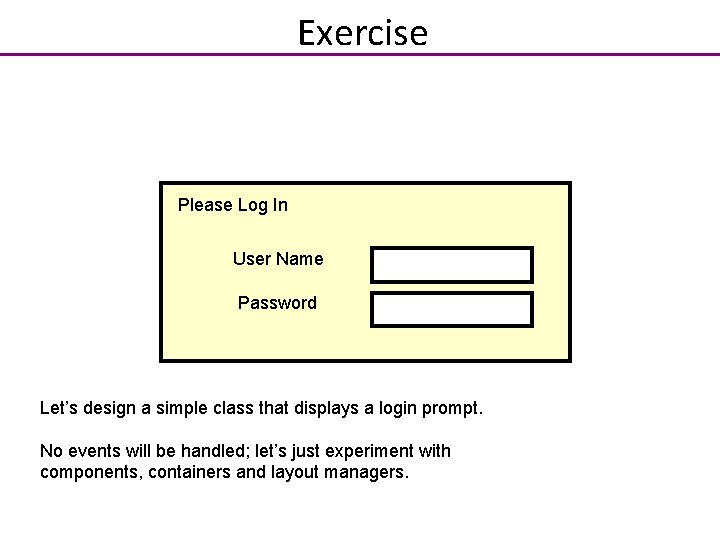
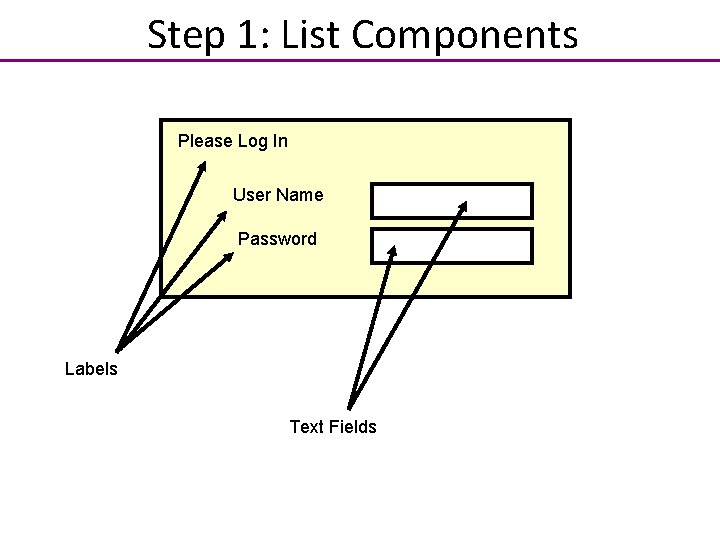
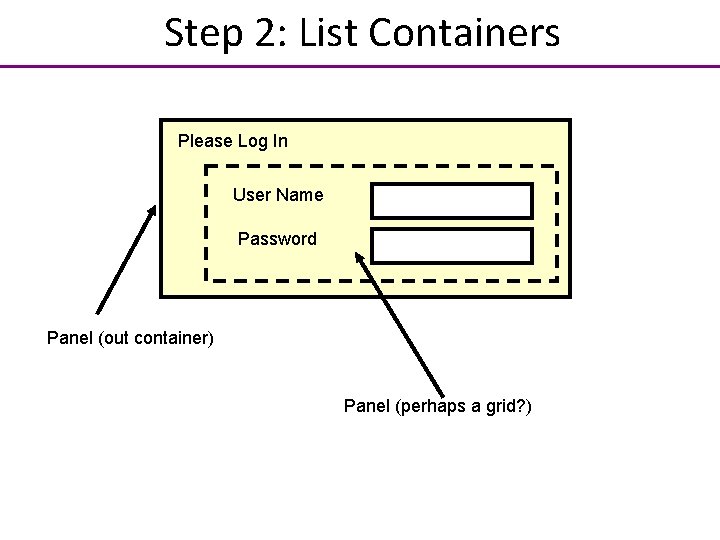
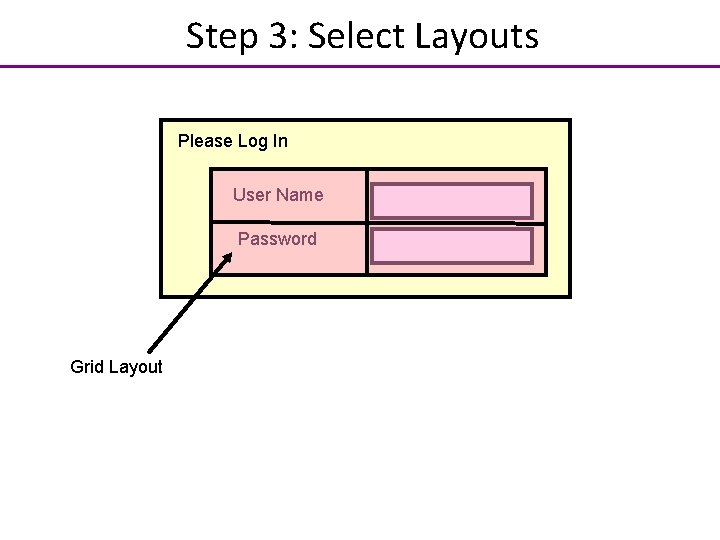
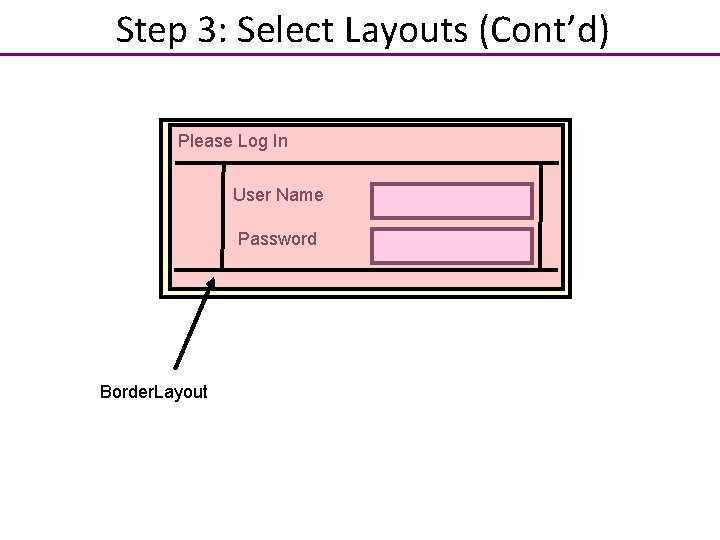
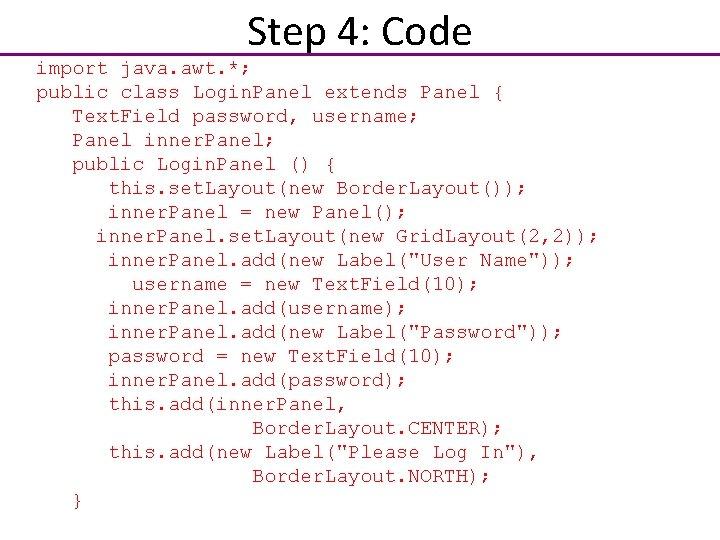
![Step 4: Code (Cont’d) // class Login. Panel (con’td)… public static void main(String[] args) Step 4: Code (Cont’d) // class Login. Panel (con’td)… public static void main(String[] args)](https://slidetodoc.com/presentation_image/b7a144612b392bcdda39f8ef4dcb93ab/image-8.jpg)
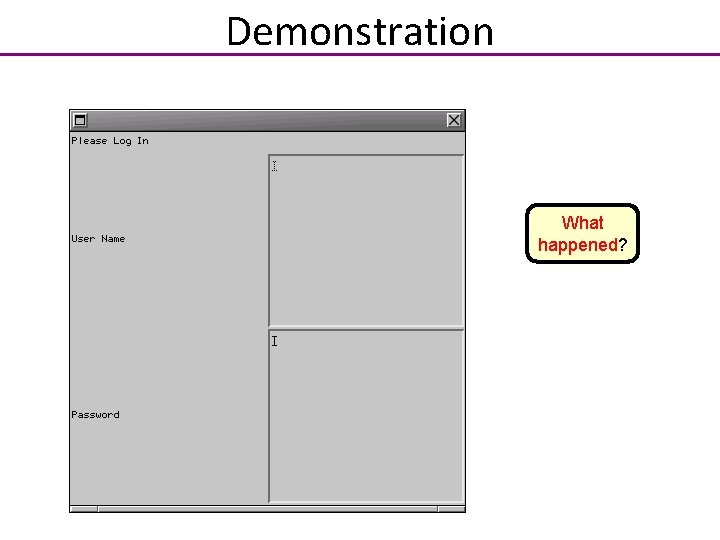
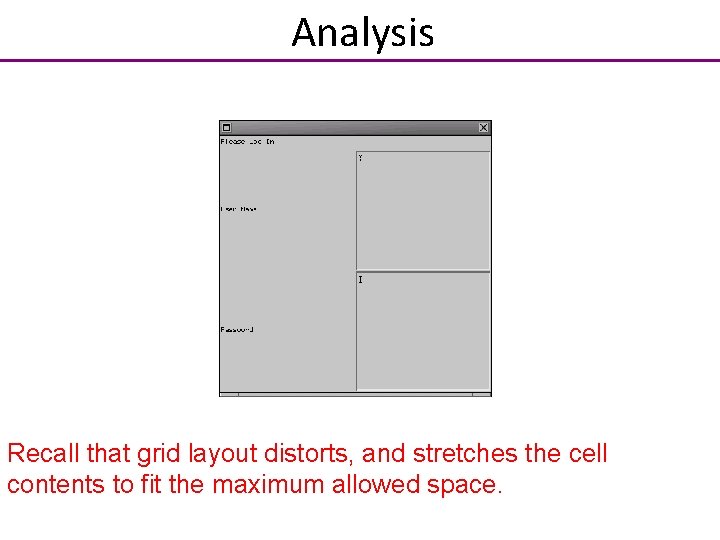
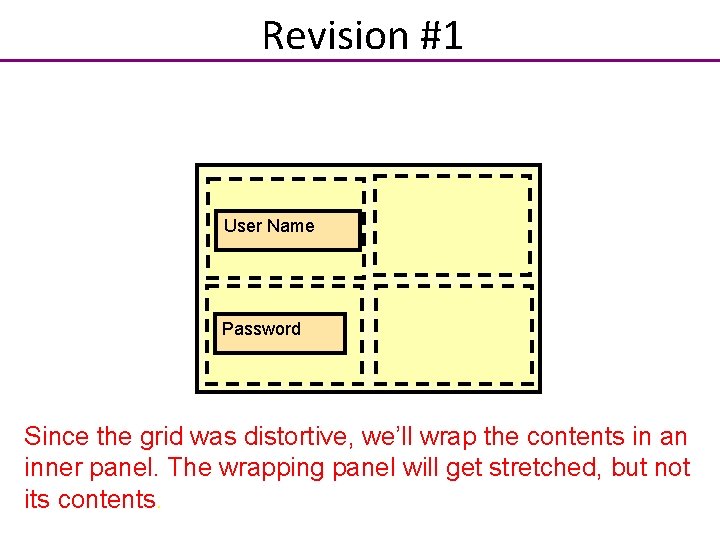
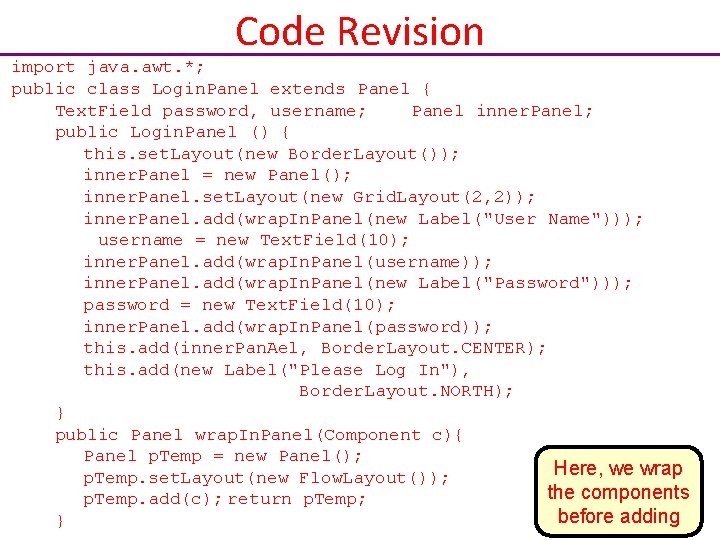
![Code Revision (Cont’d) /* Revised Login. Panel class (cont’d) */ public static void main(String[] Code Revision (Cont’d) /* Revised Login. Panel class (cont’d) */ public static void main(String[]](https://slidetodoc.com/presentation_image/b7a144612b392bcdda39f8ef4dcb93ab/image-13.jpg)
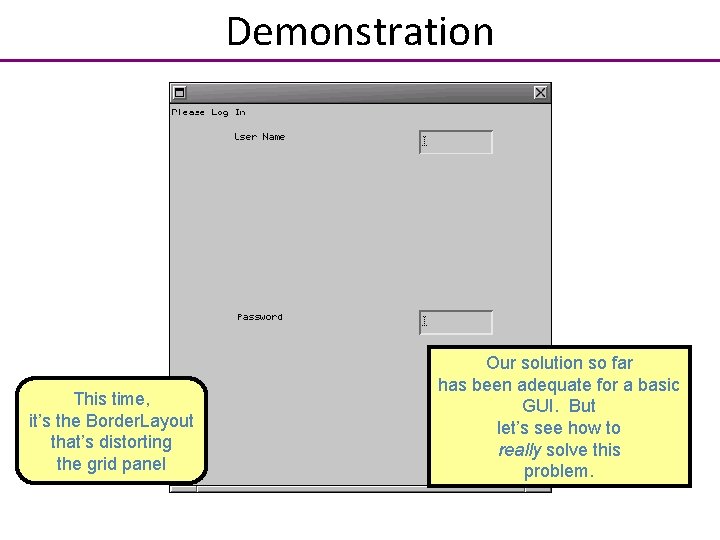
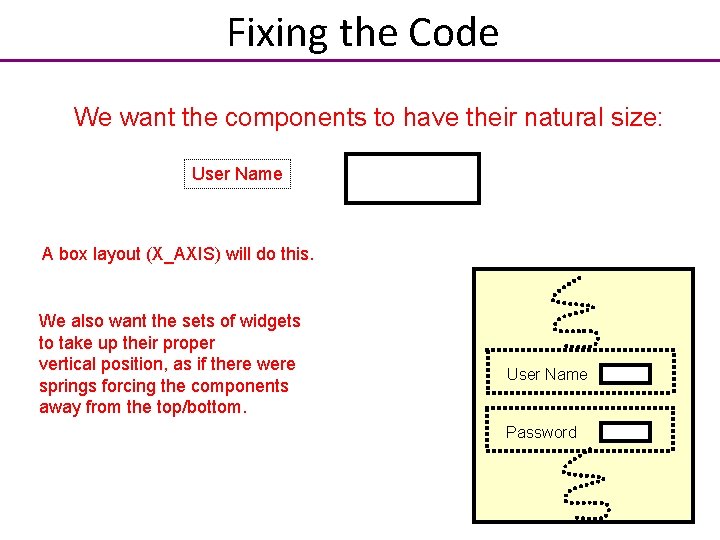
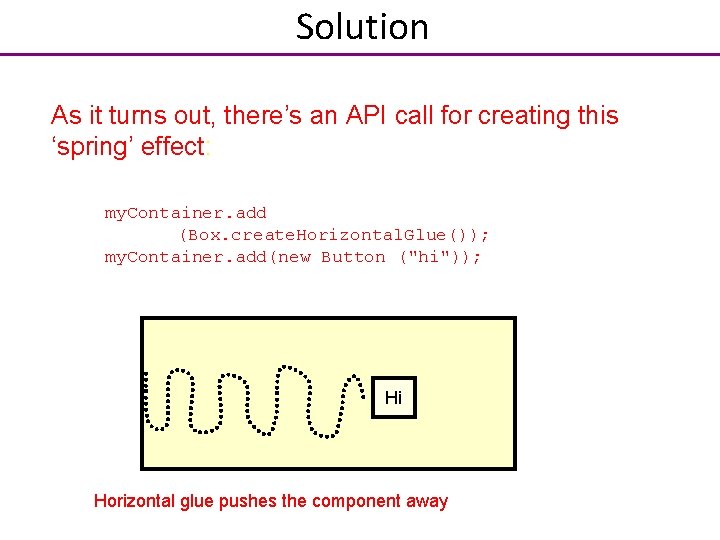
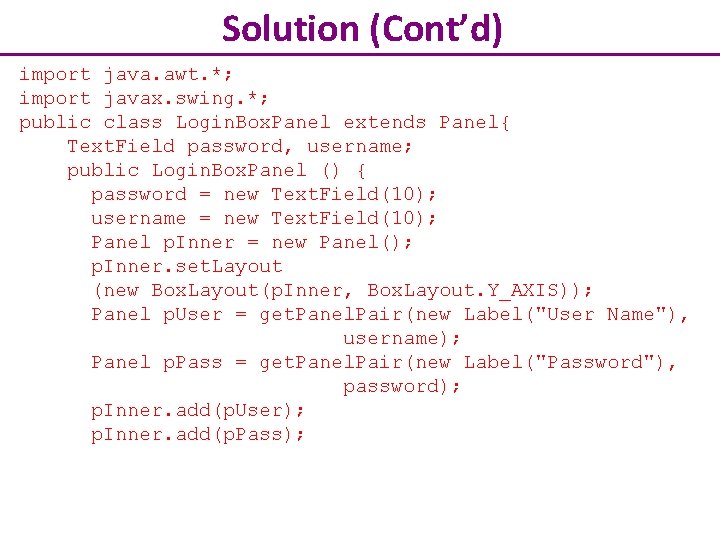
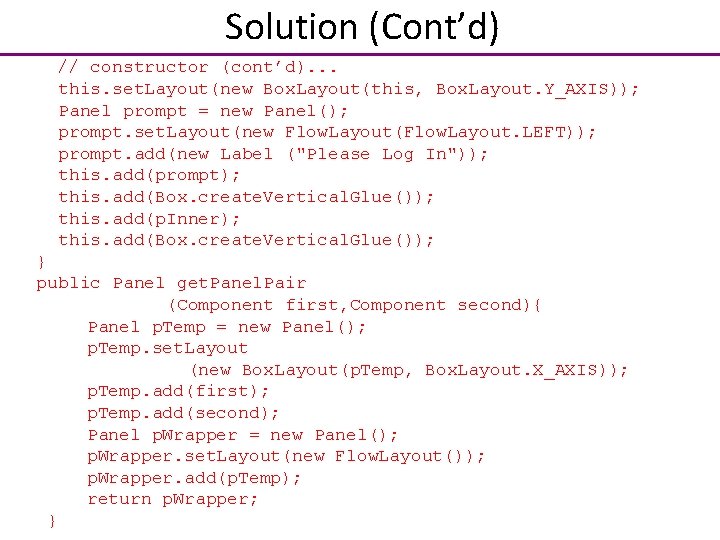
![Solution (Cont’d) public static void main(String[] args) { Frame f= new Frame(); f. set. Solution (Cont’d) public static void main(String[] args) { Frame f= new Frame(); f. set.](https://slidetodoc.com/presentation_image/b7a144612b392bcdda39f8ef4dcb93ab/image-19.jpg)
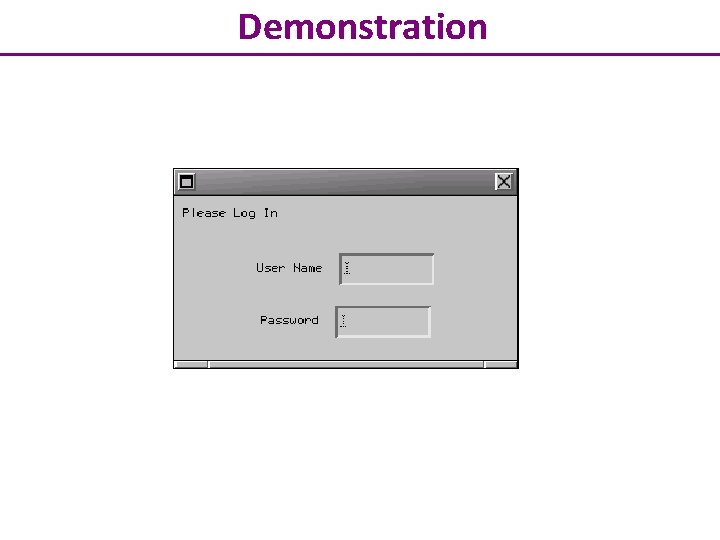
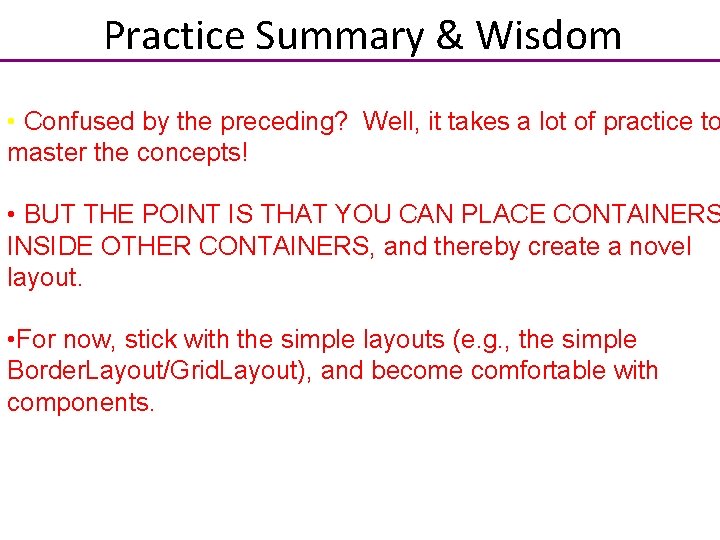
- Slides: 21
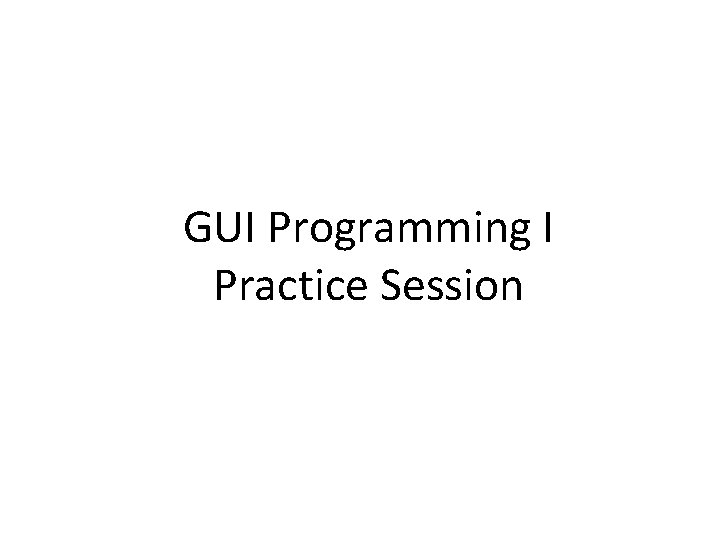
GUI Programming I Practice Session
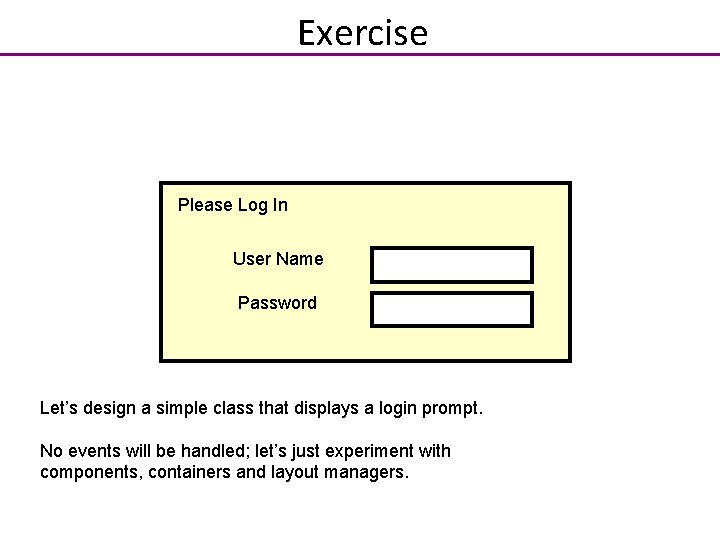
Exercise Please Log In User Name Password Let’s design a simple class that displays a login prompt. No events will be handled; let’s just experiment with components, containers and layout managers.
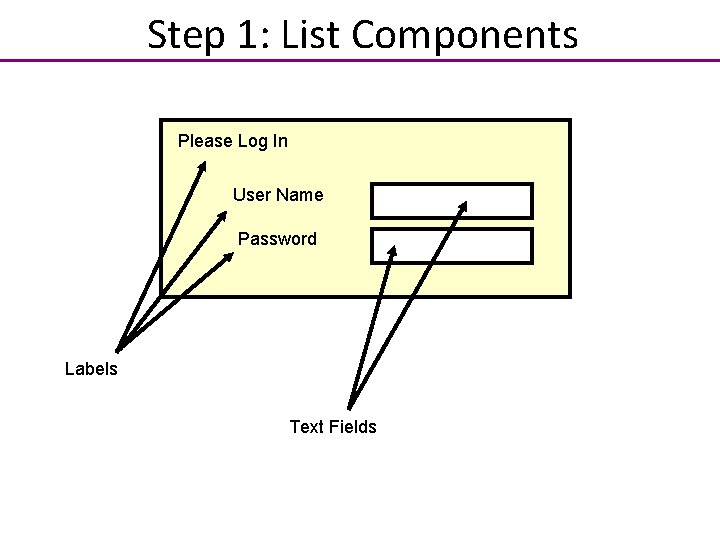
Step 1: List Components Please Log In User Name Password Labels Text Fields
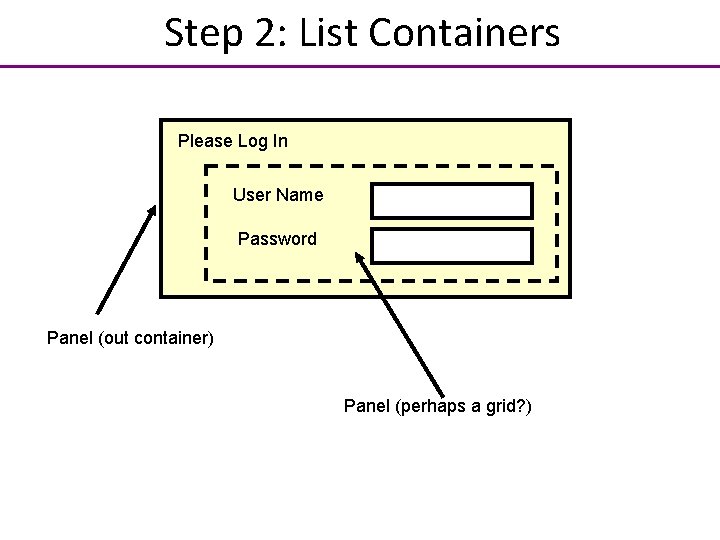
Step 2: List Containers Please Log In User Name Password Panel (out container) Panel (perhaps a grid? )
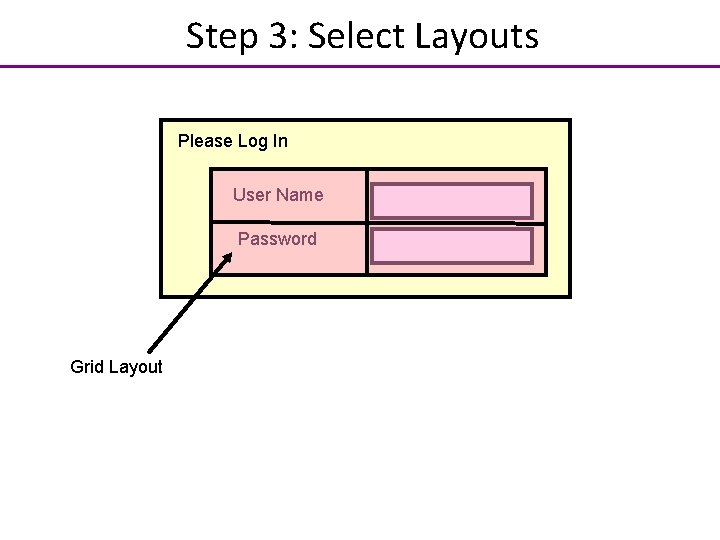
Step 3: Select Layouts Please Log In User Name Password Grid Layout
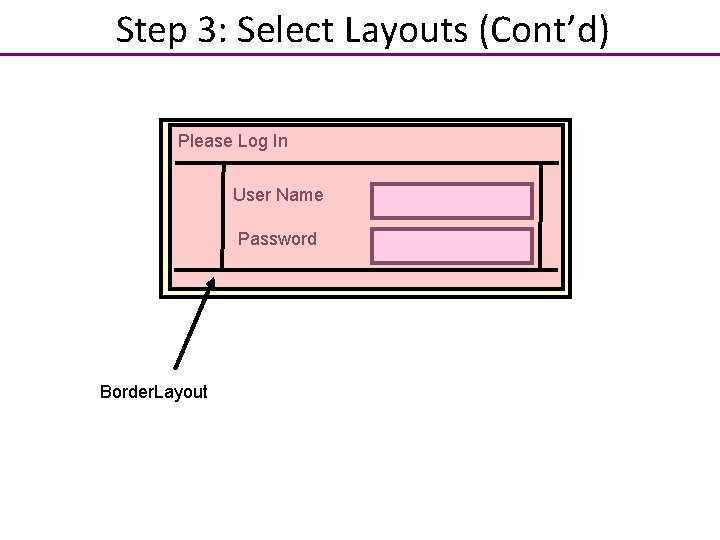
Step 3: Select Layouts (Cont’d) Please Log In User Name Password Border. Layout
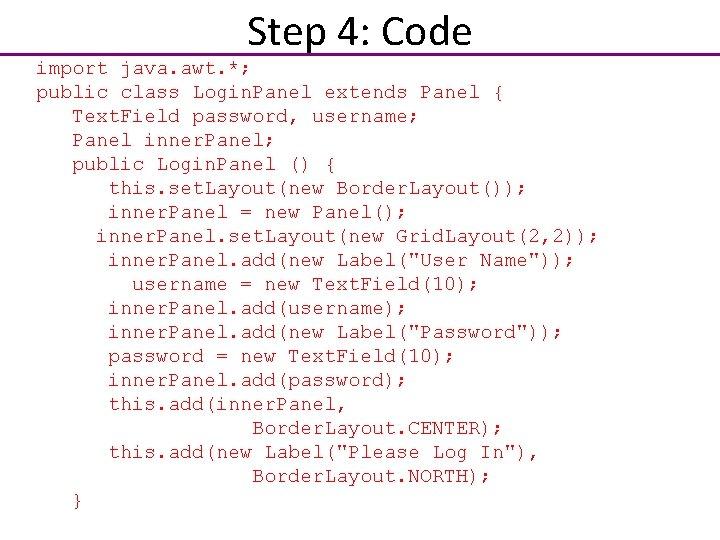
Step 4: Code import java. awt. *; public class Login. Panel extends Panel { Text. Field password, username; Panel inner. Panel; public Login. Panel () { this. set. Layout(new Border. Layout()); inner. Panel = new Panel(); inner. Panel. set. Layout(new Grid. Layout(2, 2)); inner. Panel. add(new Label("User Name")); username = new Text. Field(10); inner. Panel. add(username); inner. Panel. add(new Label("Password")); password = new Text. Field(10); inner. Panel. add(password); this. add(inner. Panel, Border. Layout. CENTER); this. add(new Label("Please Log In"), Border. Layout. NORTH); }
![Step 4 Code Contd class Login Panel contd public static void mainString args Step 4: Code (Cont’d) // class Login. Panel (con’td)… public static void main(String[] args)](https://slidetodoc.com/presentation_image/b7a144612b392bcdda39f8ef4dcb93ab/image-8.jpg)
Step 4: Code (Cont’d) // class Login. Panel (con’td)… public static void main(String[] args) { Frame f = new Frame(); f. set. Size(400, 400); f. add(new Login. Panel()); f. show(); } } // Login. Panel
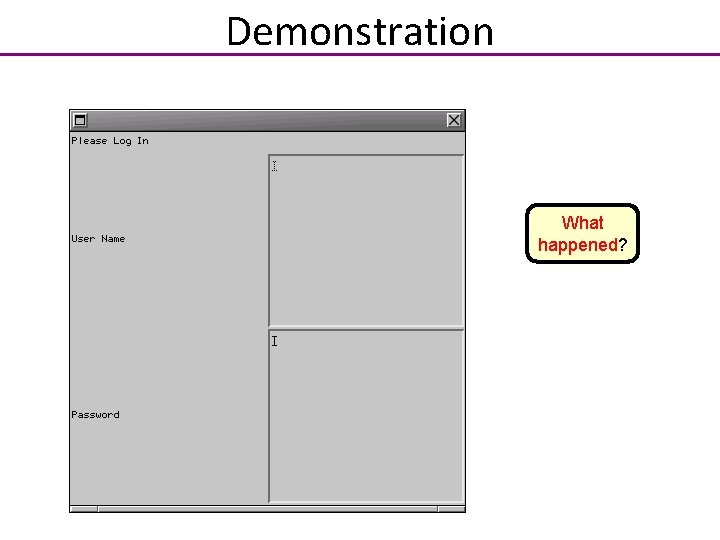
Demonstration What happened?
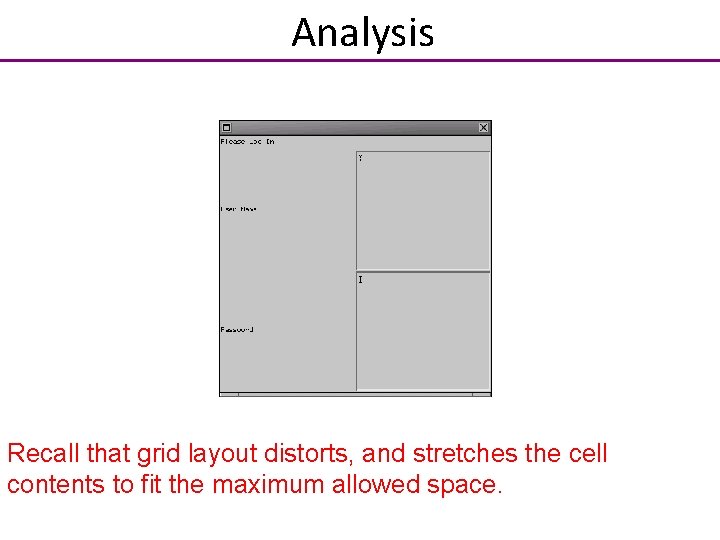
Analysis Recall that grid layout distorts, and stretches the cell contents to fit the maximum allowed space.
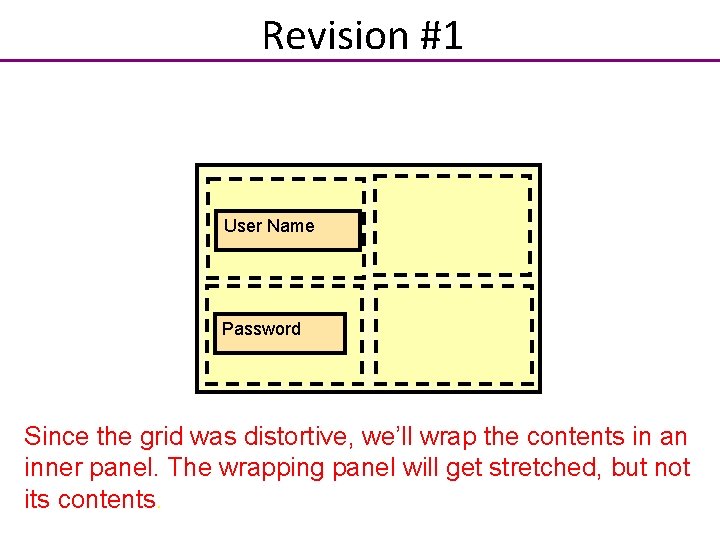
Revision #1 User Name Password Since the grid was distortive, we’ll wrap the contents in an inner panel. The wrapping panel will get stretched, but not its contents.
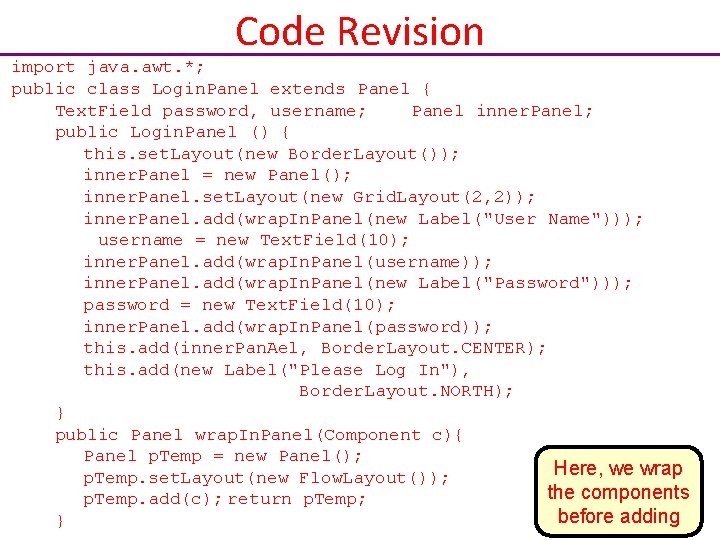
Code Revision import java. awt. *; public class Login. Panel extends Panel { Text. Field password, username; Panel inner. Panel; public Login. Panel () { this. set. Layout(new Border. Layout()); inner. Panel = new Panel(); inner. Panel. set. Layout(new Grid. Layout(2, 2)); inner. Panel. add(wrap. In. Panel(new Label("User Name"))); username = new Text. Field(10); inner. Panel. add(wrap. In. Panel(username)); inner. Panel. add(wrap. In. Panel(new Label("Password"))); password = new Text. Field(10); inner. Panel. add(wrap. In. Panel(password)); this. add(inner. Pan. Ael, Border. Layout. CENTER); this. add(new Label("Please Log In"), Border. Layout. NORTH); } public Panel wrap. In. Panel(Component c){ Panel p. Temp = new Panel(); Here, we wrap p. Temp. set. Layout(new Flow. Layout()); the components p. Temp. add(c); return p. Temp; before adding }
![Code Revision Contd Revised Login Panel class contd public static void mainString Code Revision (Cont’d) /* Revised Login. Panel class (cont’d) */ public static void main(String[]](https://slidetodoc.com/presentation_image/b7a144612b392bcdda39f8ef4dcb93ab/image-13.jpg)
Code Revision (Cont’d) /* Revised Login. Panel class (cont’d) */ public static void main(String[] args) { Frame f= new Frame(); f. set. Size(400, 400); f. add(new Login. Panel()); f. show(); } } // Login. Panel
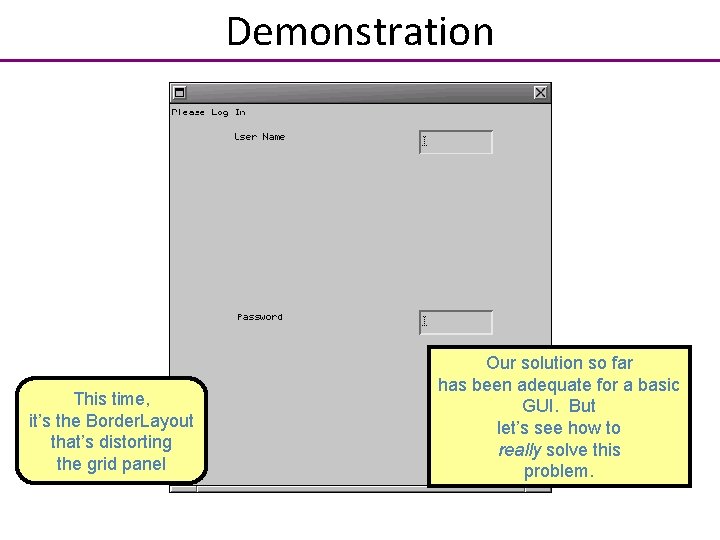
Demonstration This time, it’s the Border. Layout that’s distorting the grid panel Our solution so far has been adequate for a basic GUI. But let’s see how to really solve this problem.
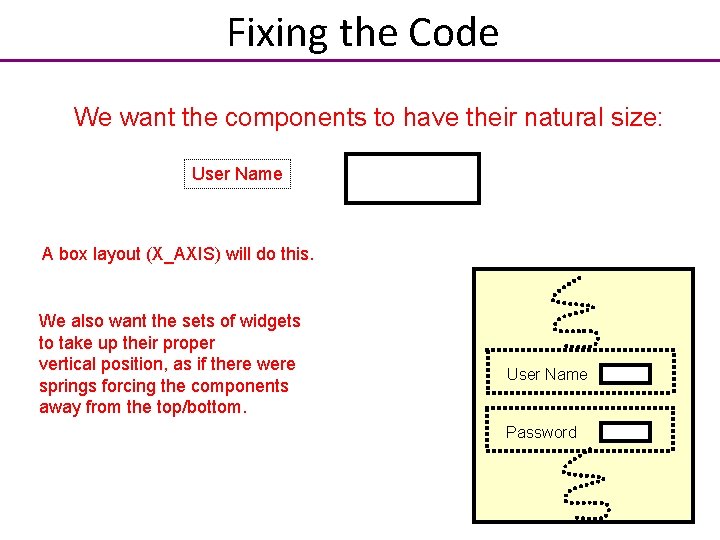
Fixing the Code We want the components to have their natural size: User Name A box layout (X_AXIS) will do this. We also want the sets of widgets to take up their proper vertical position, as if there were springs forcing the components away from the top/bottom. User Name Password
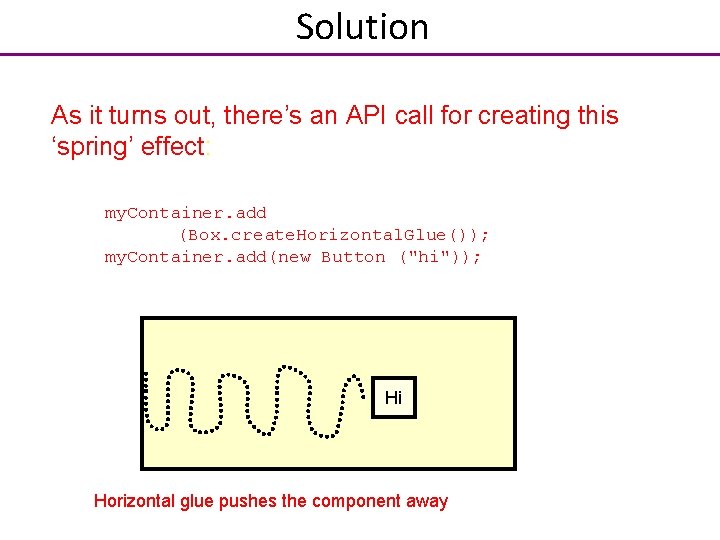
Solution As it turns out, there’s an API call for creating this ‘spring’ effect: my. Container. add (Box. create. Horizontal. Glue()); my. Container. add(new Button ("hi")); Hi Horizontal glue pushes the component away
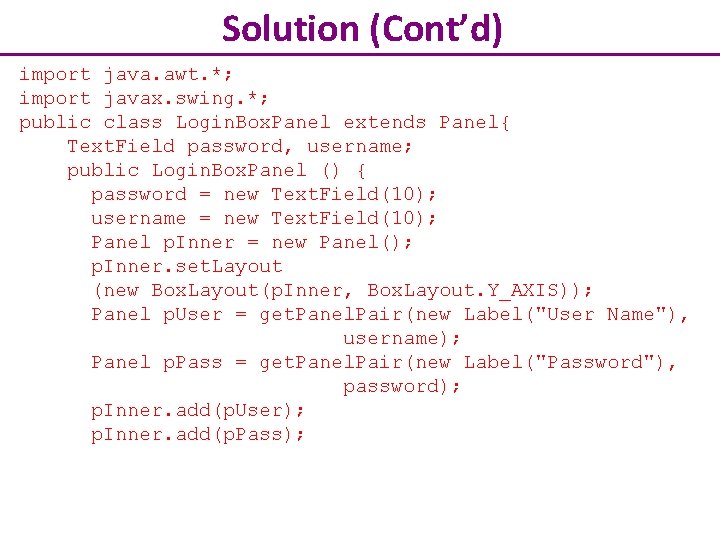
Solution (Cont’d) import java. awt. *; import javax. swing. *; public class Login. Box. Panel extends Panel{ Text. Field password, username; public Login. Box. Panel () { password = new Text. Field(10); username = new Text. Field(10); Panel p. Inner = new Panel(); p. Inner. set. Layout (new Box. Layout(p. Inner, Box. Layout. Y_AXIS)); Panel p. User = get. Panel. Pair(new Label("User Name"), username); Panel p. Pass = get. Panel. Pair(new Label("Password"), password); p. Inner. add(p. User); p. Inner. add(p. Pass);
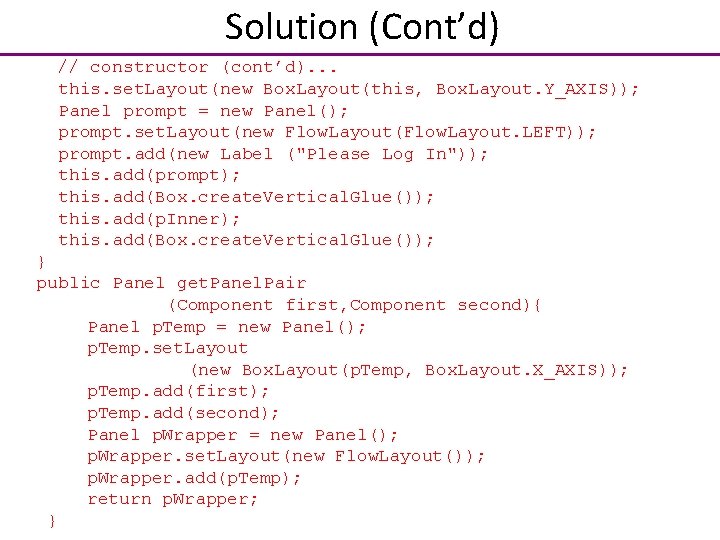
Solution (Cont’d) // constructor (cont’d). . . this. set. Layout(new Box. Layout(this, Box. Layout. Y_AXIS)); Panel prompt = new Panel(); prompt. set. Layout(new Flow. Layout(Flow. Layout. LEFT)); prompt. add(new Label ("Please Log In")); this. add(prompt); this. add(Box. create. Vertical. Glue()); this. add(p. Inner); this. add(Box. create. Vertical. Glue()); } public Panel get. Panel. Pair (Component first, Component second){ Panel p. Temp = new Panel(); p. Temp. set. Layout (new Box. Layout(p. Temp, Box. Layout. X_AXIS)); p. Temp. add(first); p. Temp. add(second); Panel p. Wrapper = new Panel(); p. Wrapper. set. Layout(new Flow. Layout()); p. Wrapper. add(p. Temp); return p. Wrapper; }
![Solution Contd public static void mainString args Frame f new Frame f set Solution (Cont’d) public static void main(String[] args) { Frame f= new Frame(); f. set.](https://slidetodoc.com/presentation_image/b7a144612b392bcdda39f8ef4dcb93ab/image-19.jpg)
Solution (Cont’d) public static void main(String[] args) { Frame f= new Frame(); f. set. Size(400, 200); f. add(new Login. Box. Panel()); f. show(); }// main } // Login. Box. Panel
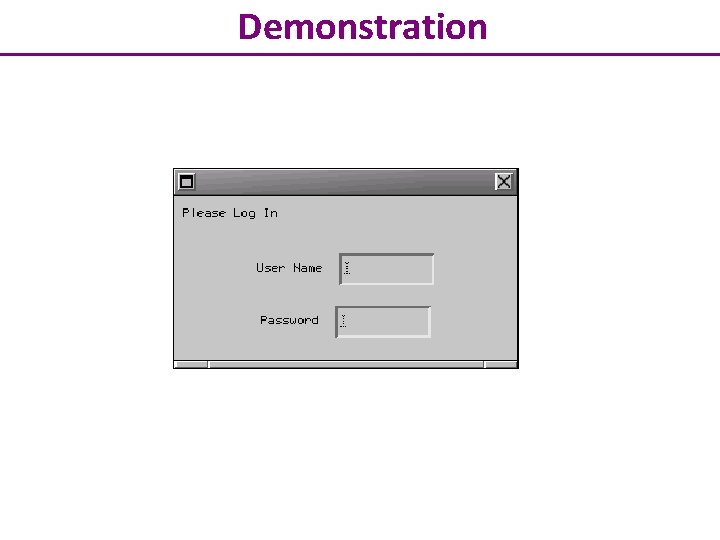
Demonstration
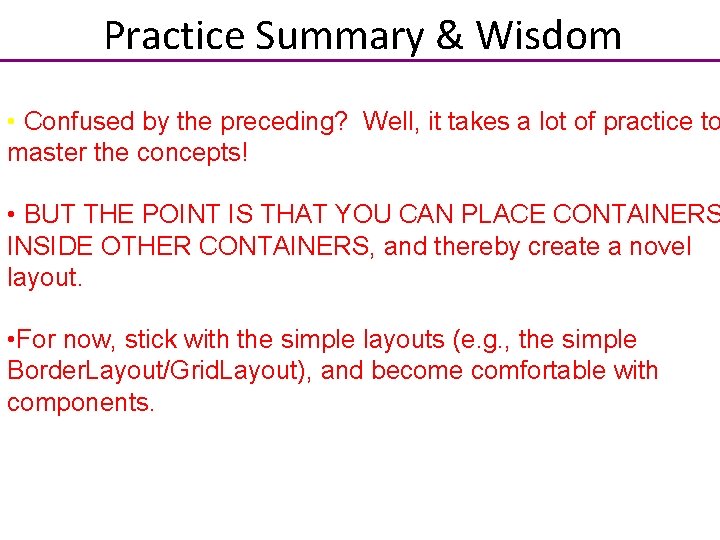
Practice Summary & Wisdom • Confused by the preceding? Well, it takes a lot of practice to master the concepts! • BUT THE POINT IS THAT YOU CAN PLACE CONTAINERS INSIDE OTHER CONTAINERS, and thereby create a novel layout. • For now, stick with the simple layouts (e. g. , the simple Border. Layout/Grid. Layout), and become comfortable with components.
Akar pangkat 674
Tentukan nilai p dari 3 log 243=p
1+3,3 log 30
Diketahui log 3 = a dan log 2 = b maka log 18 =
Log 3 = 0 477 dan log 2 = 0 301 nilai log 18 =
³log243
Jika log 3=0 477 dan log 5=0 699 maka log 45 adalah
Fungsi persamaan dan pertidaksamaan logaritma
Ge gi gue gui güe güi
An exercise session has three stages
Ruby on rails gui
Linux gui interface
Rapid gui programming with python and qt
Will you please be quiet please summary
Power law log log plot
Power law log log plot
How to get rid of logs
-18 ÷2 gives
Log k = log a - ea/rt
Exponential
Persamaan 7 log 217 + 7 log 31 ialah
5 properties of logarithms