GUI and EventHandling Programming Chapter Topics Eventdriven programming
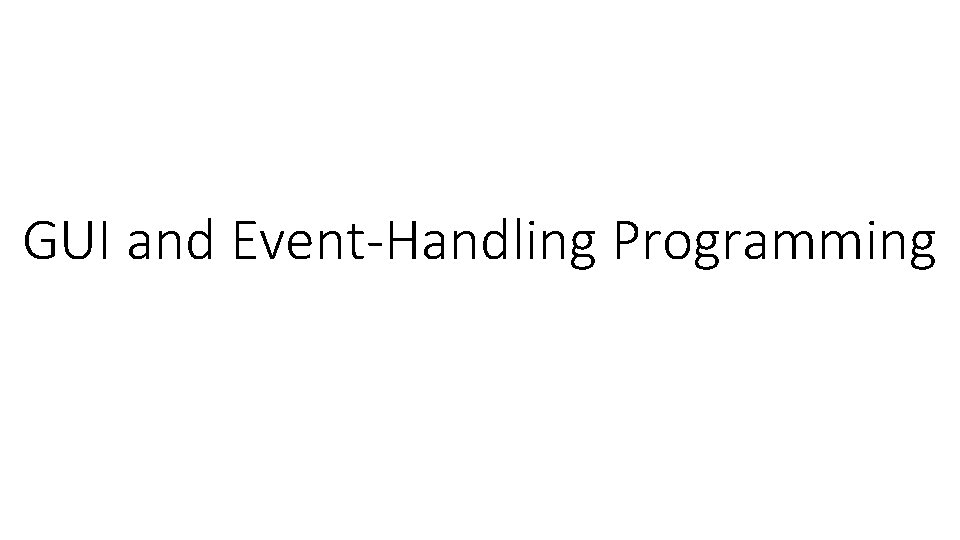
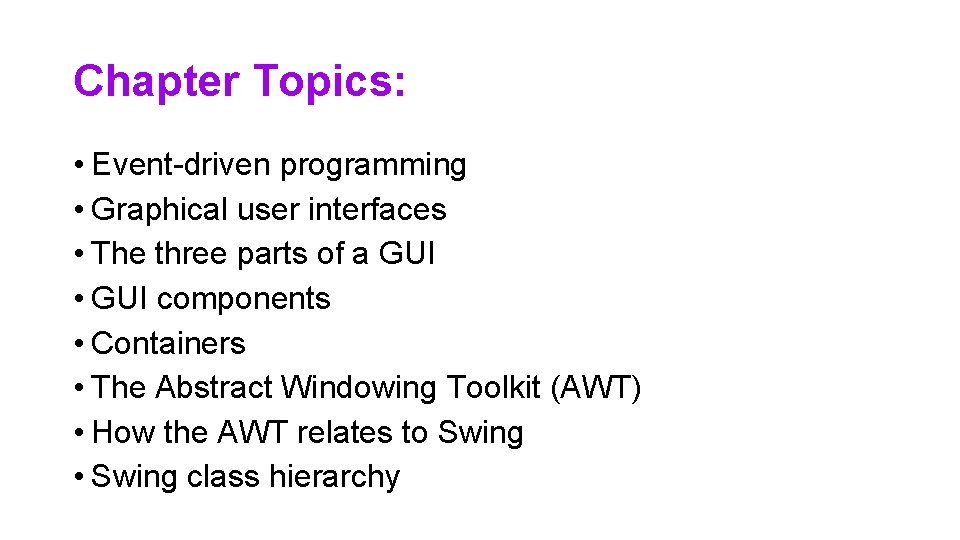
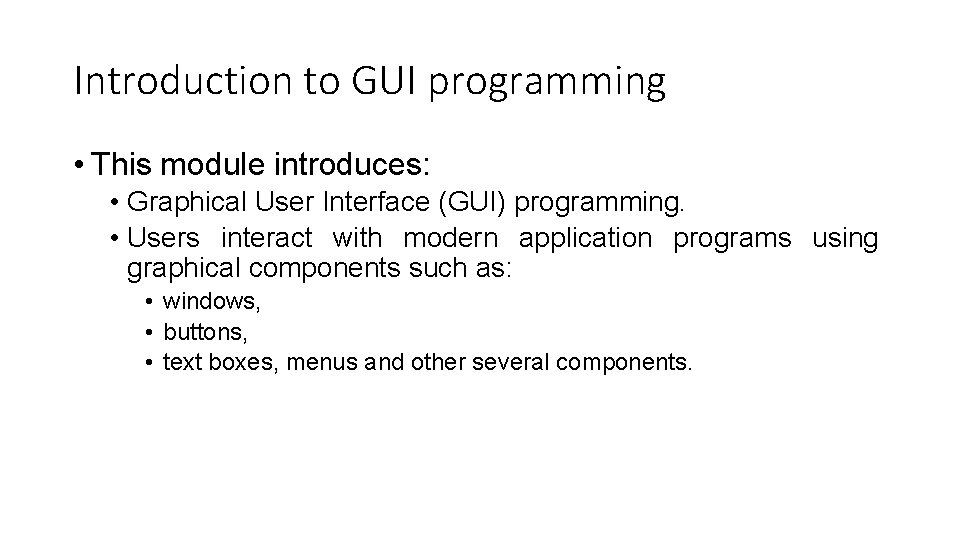
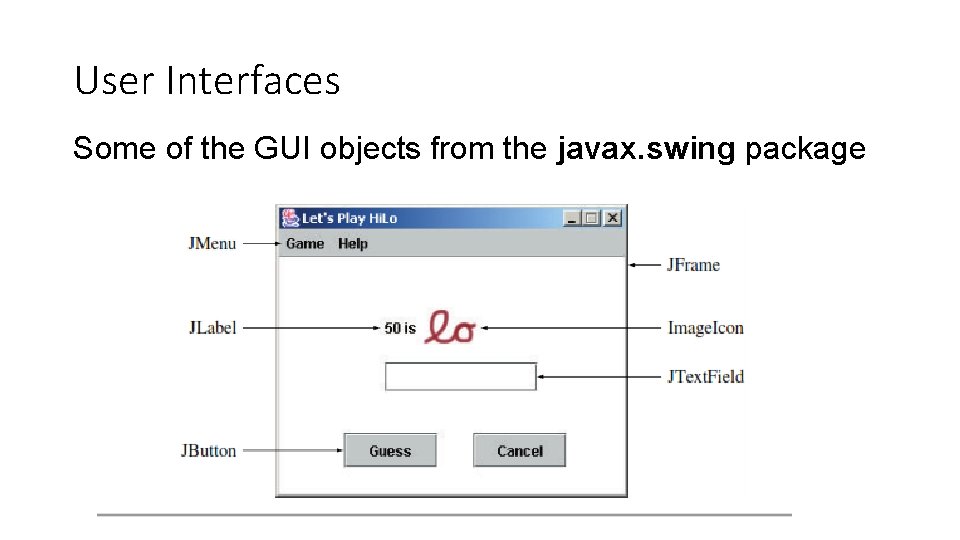
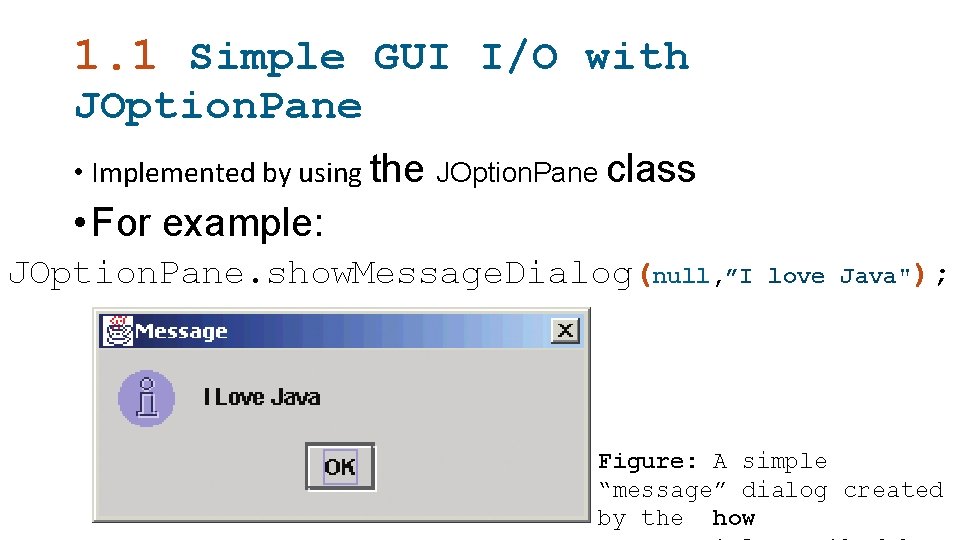
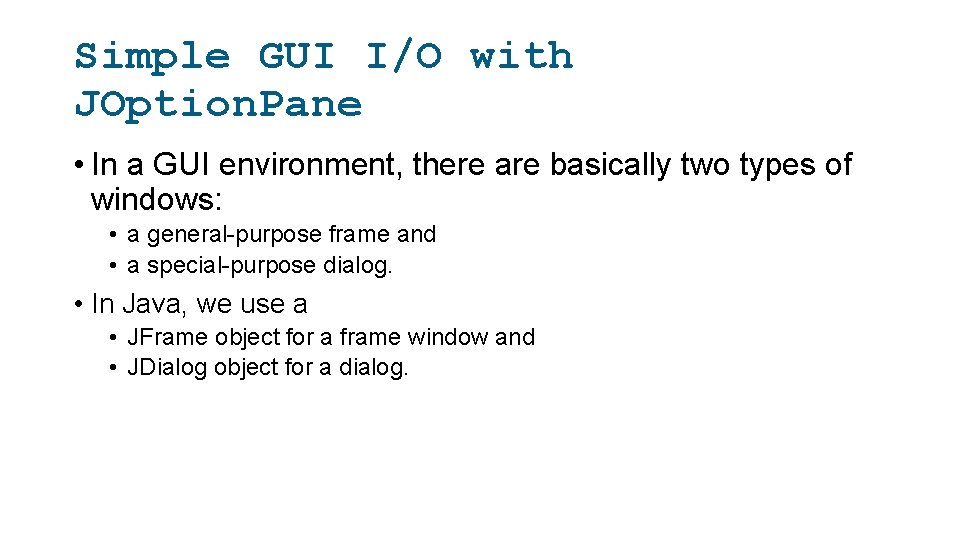
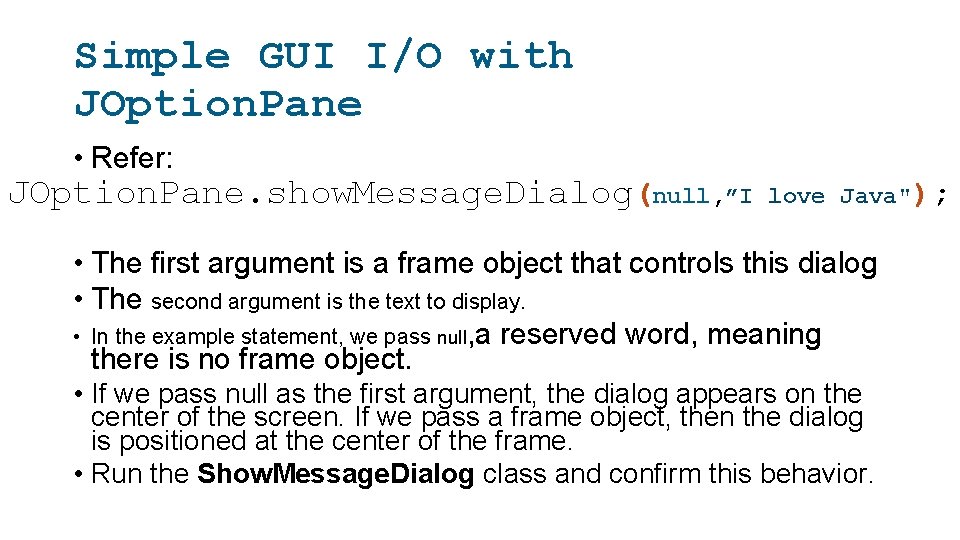
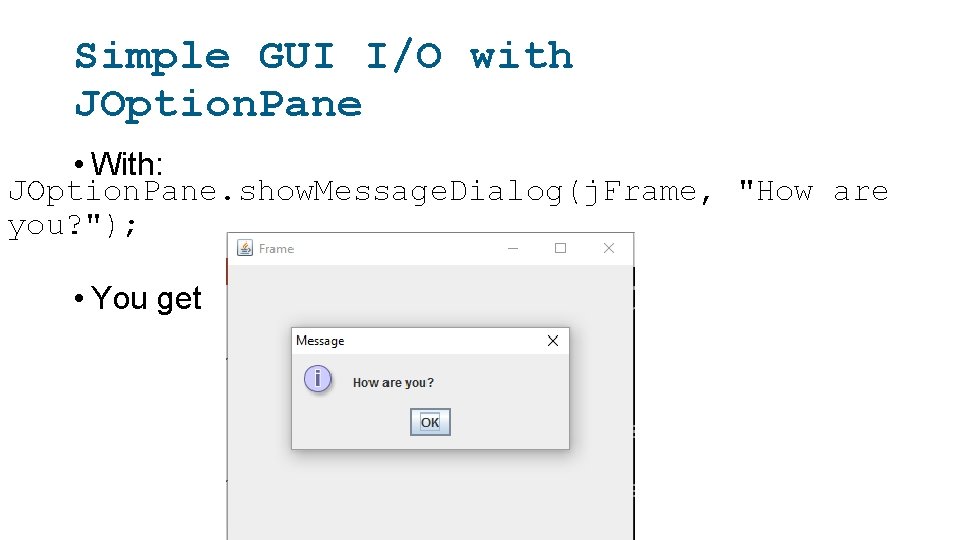
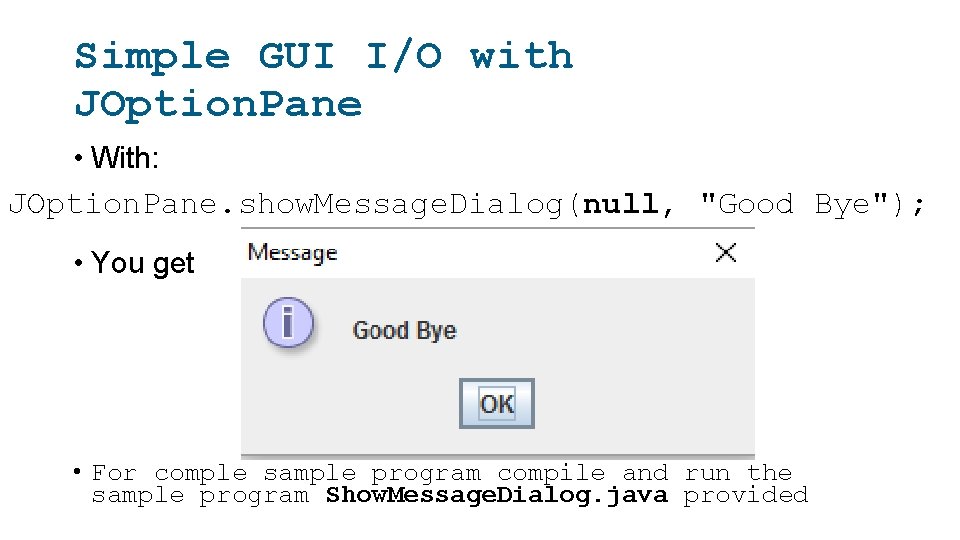
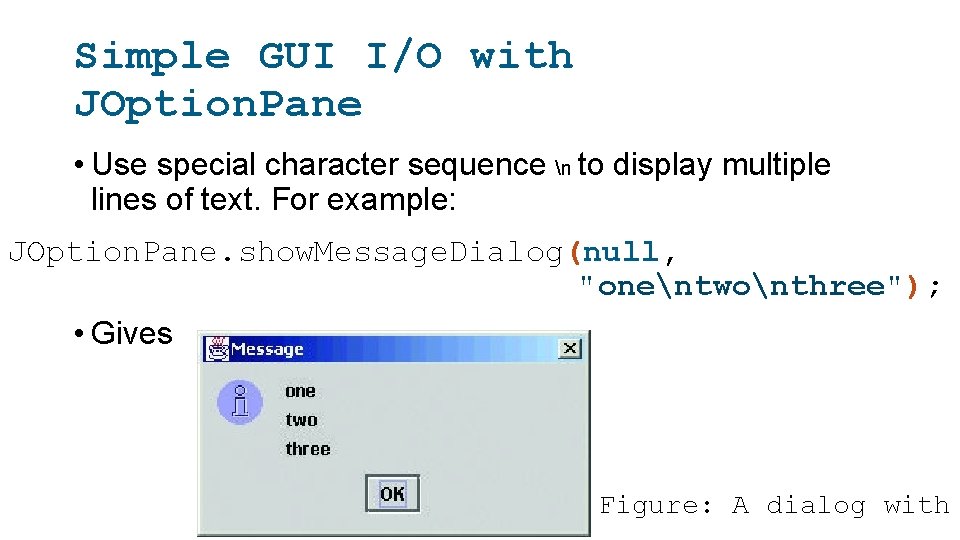
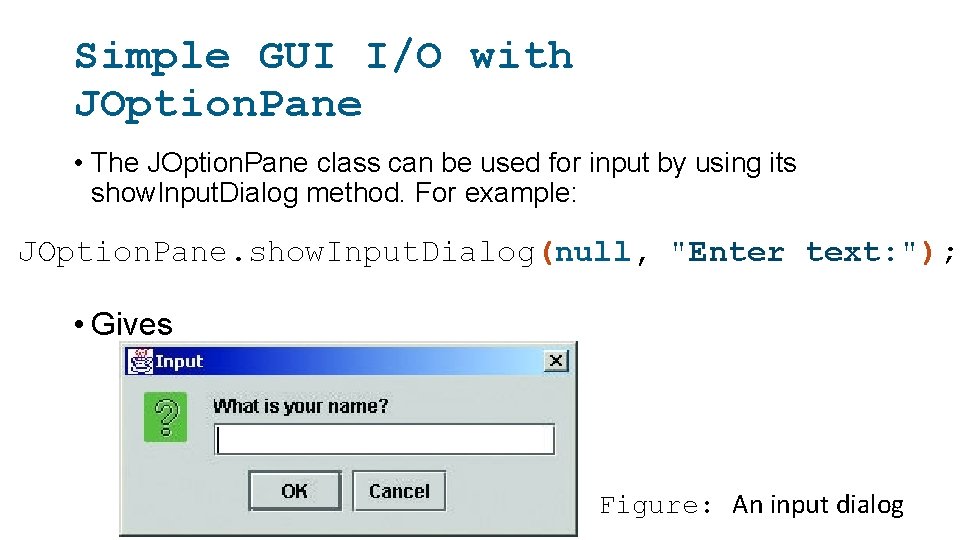
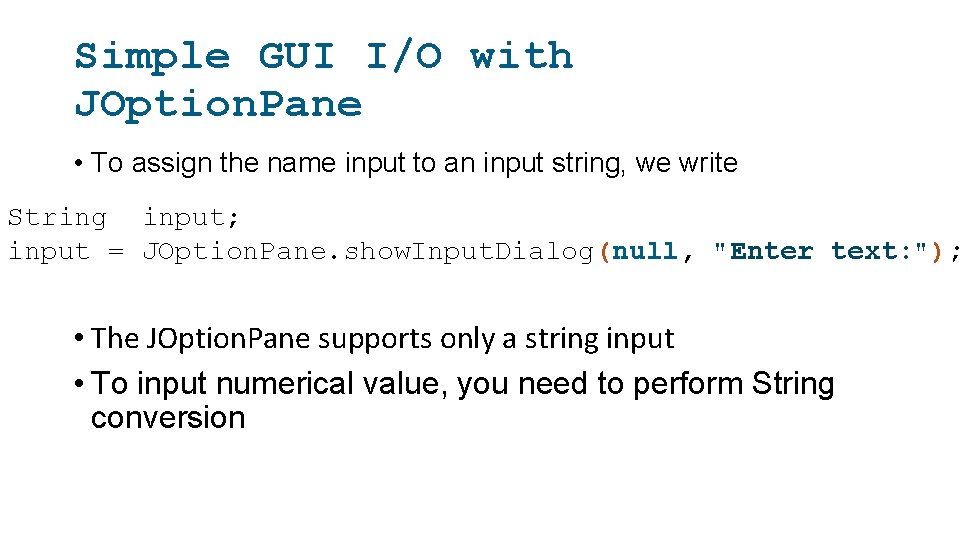
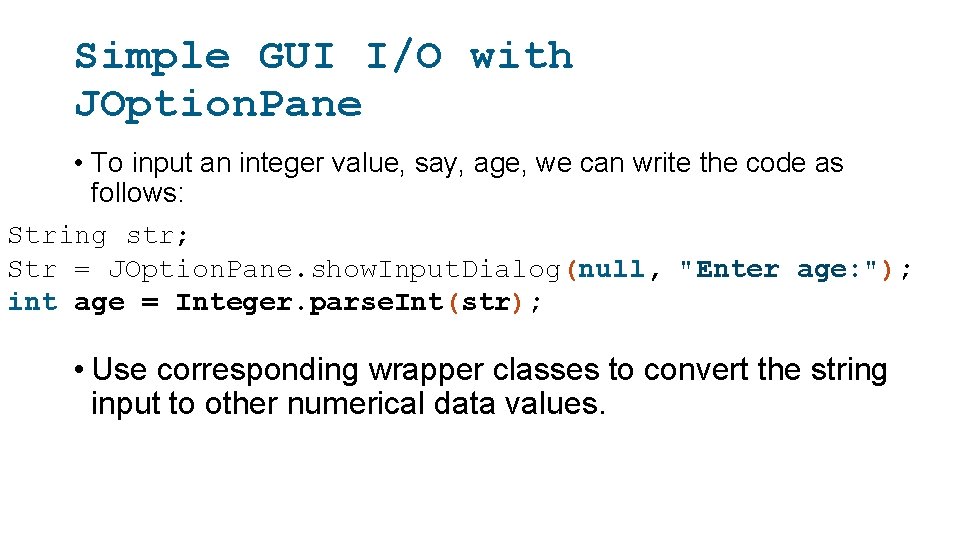
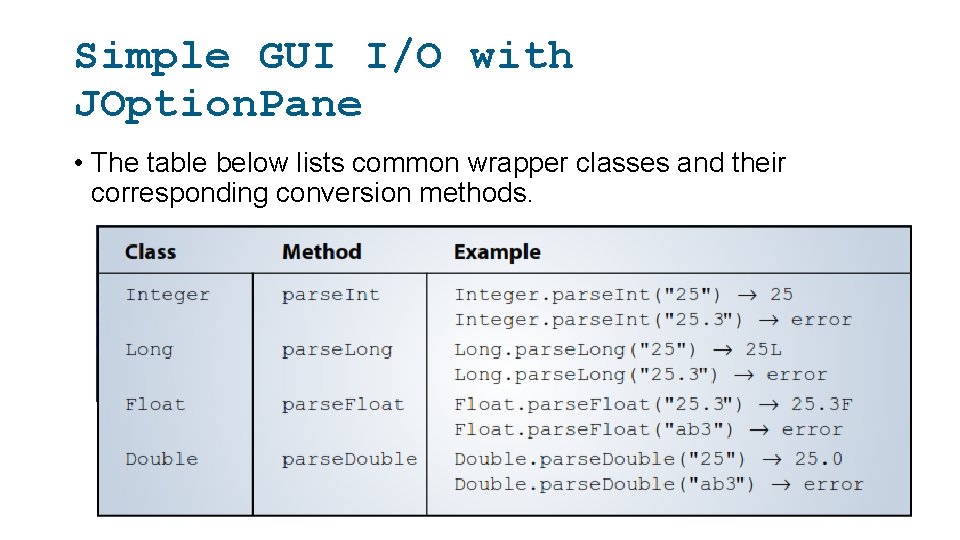
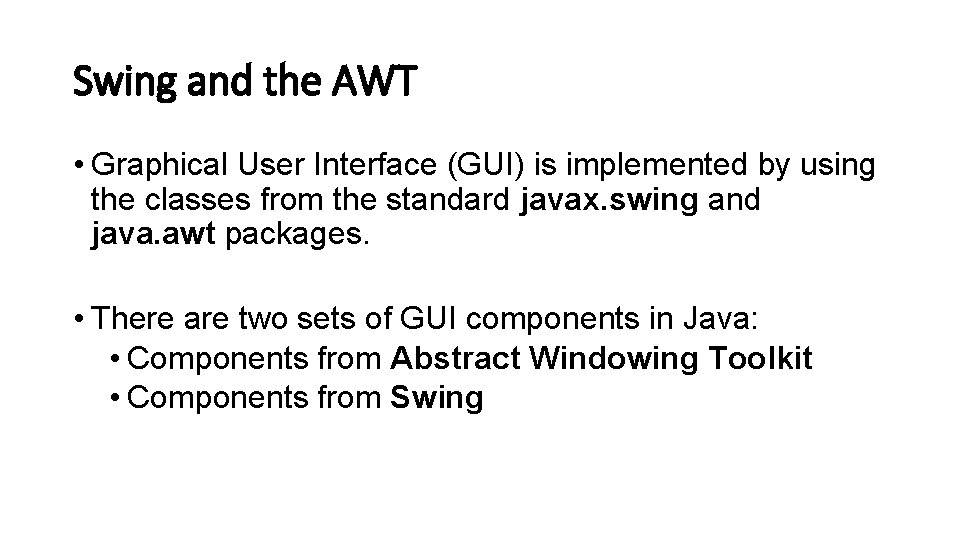
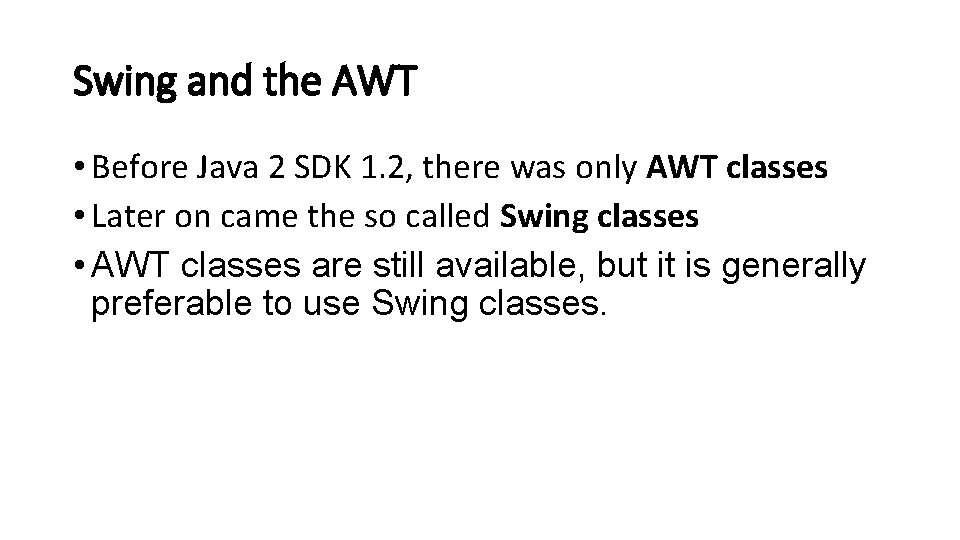
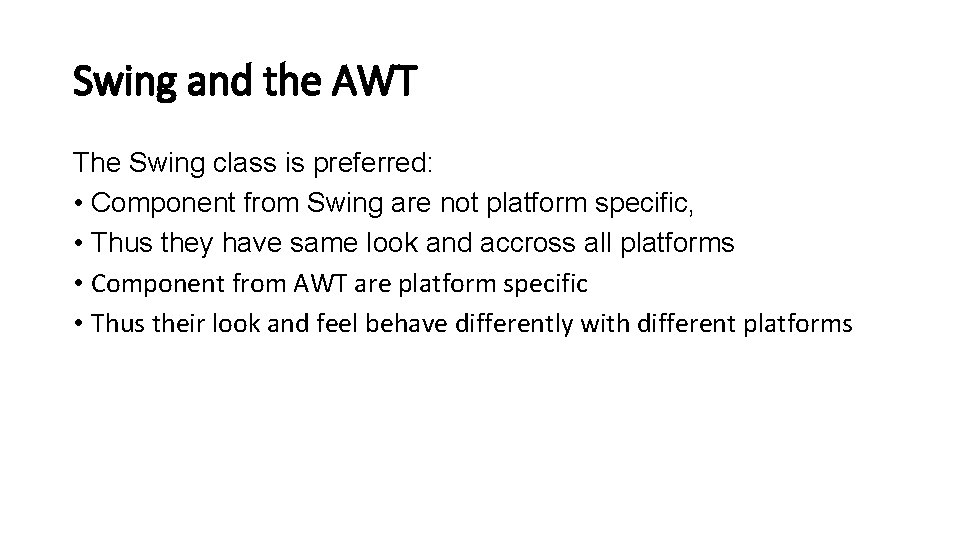
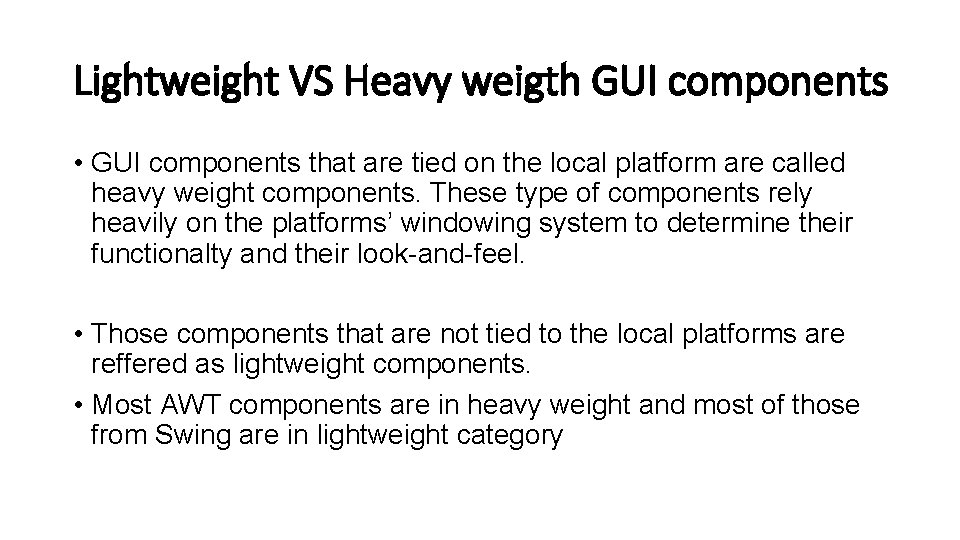
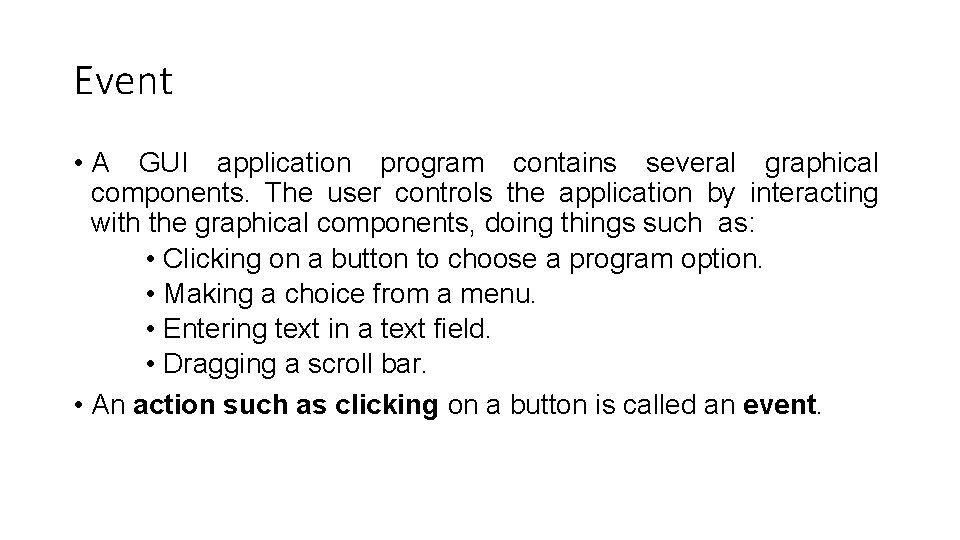
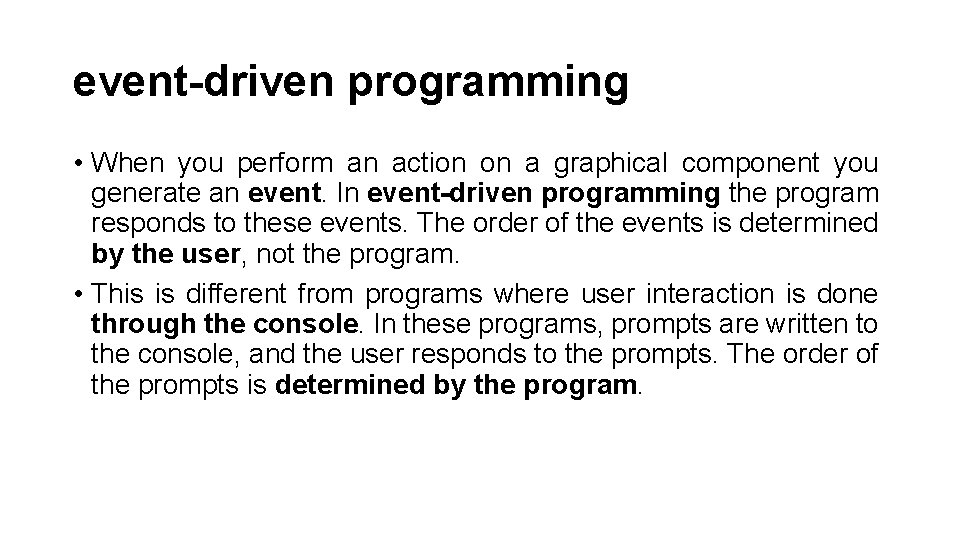
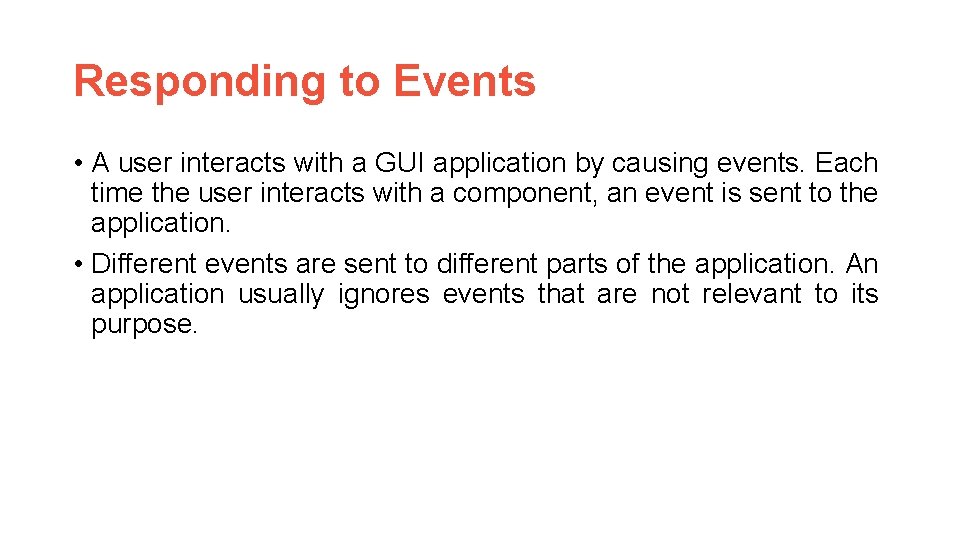
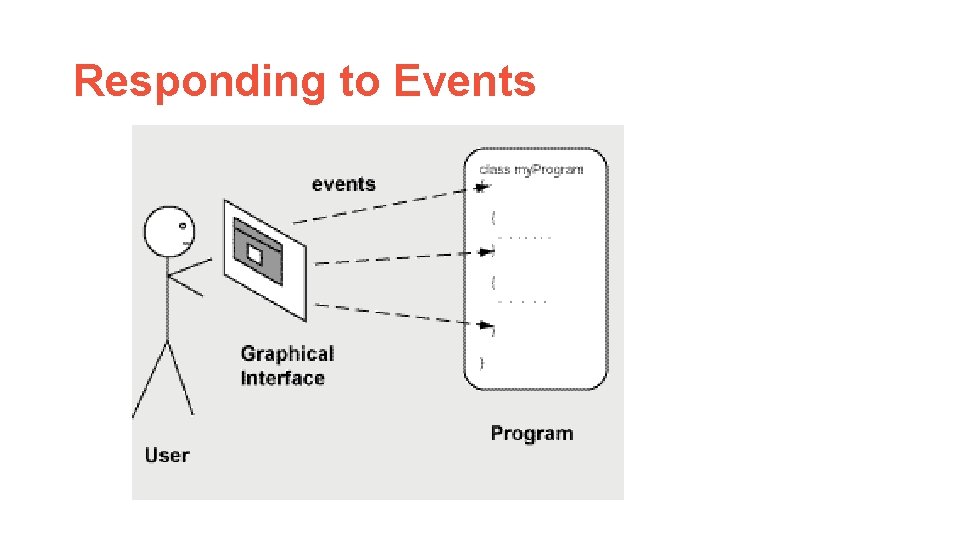
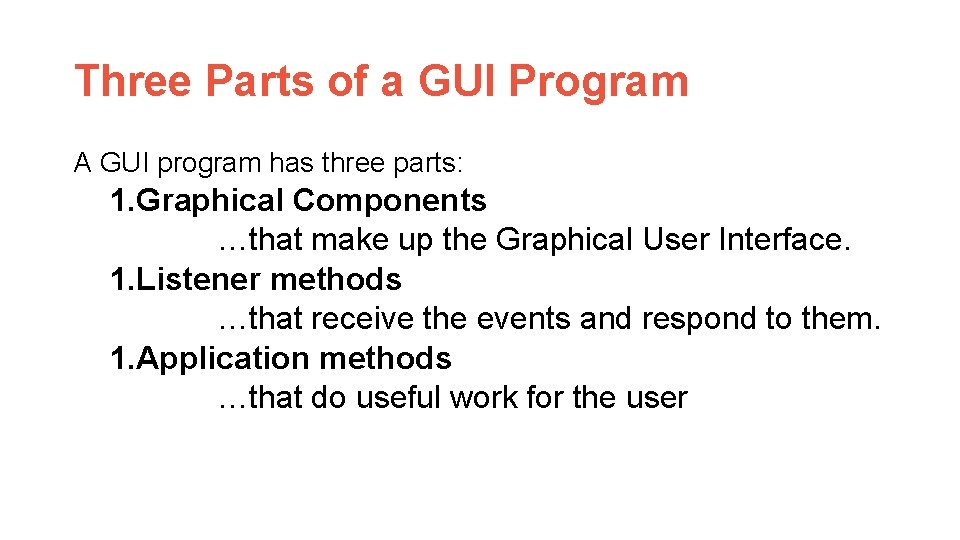
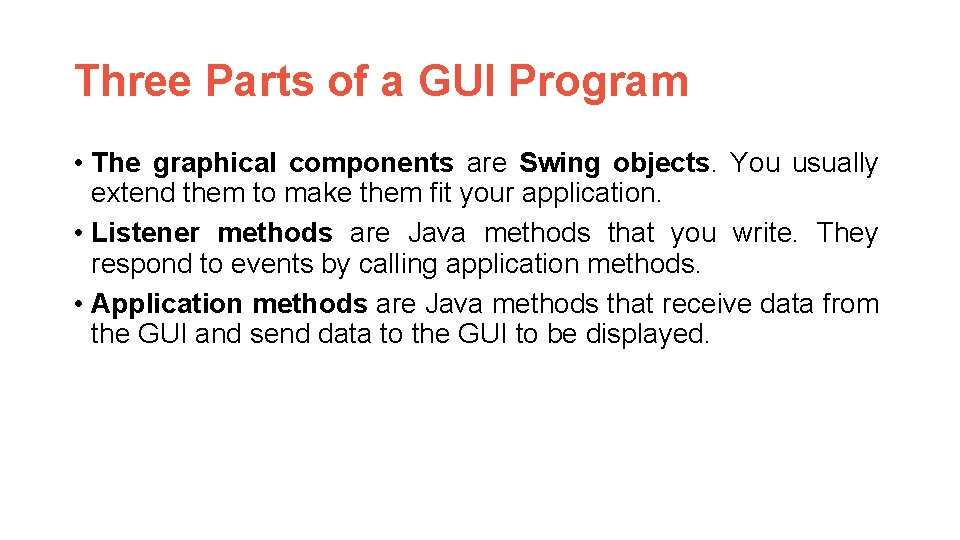
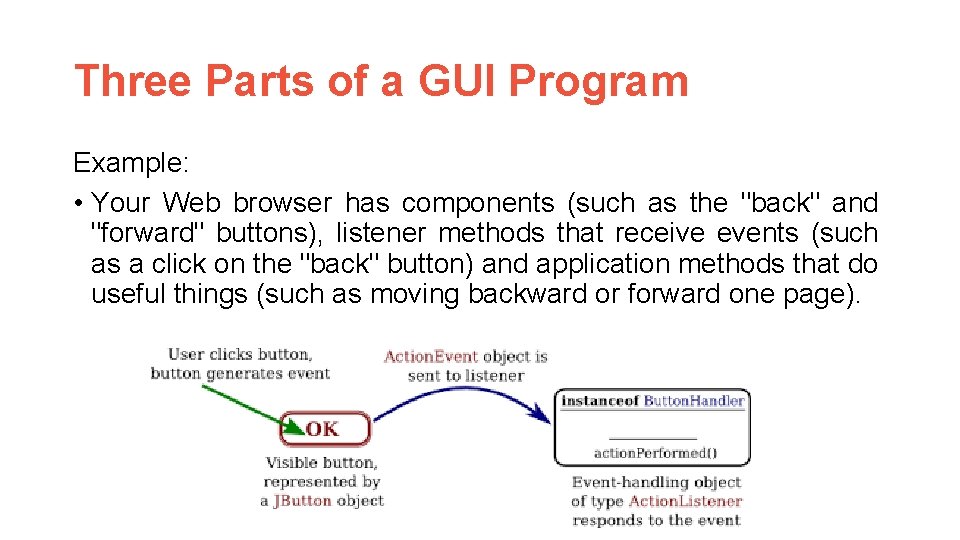
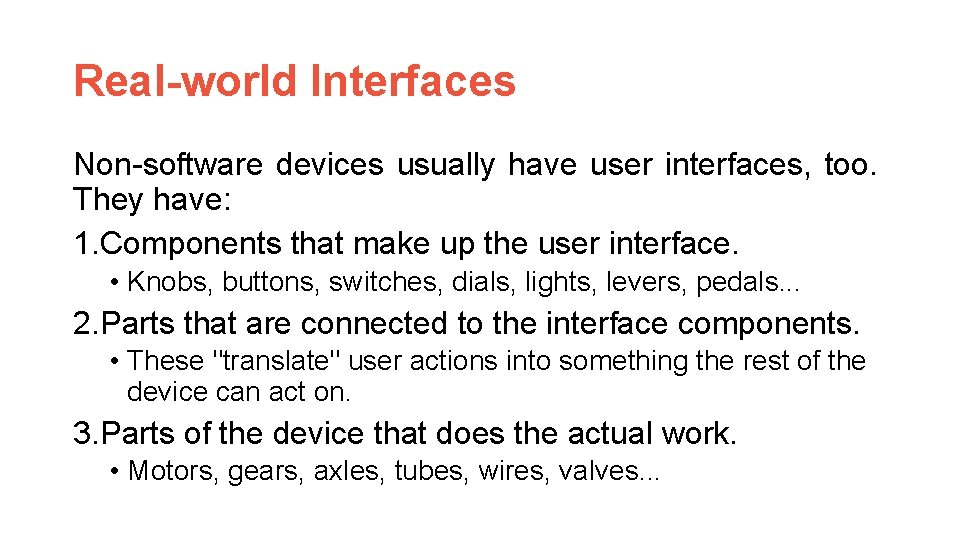
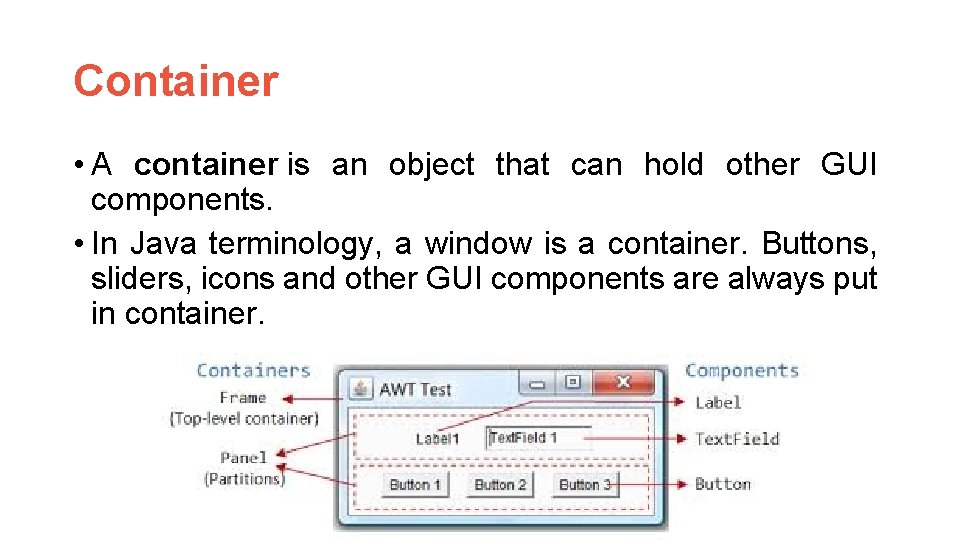
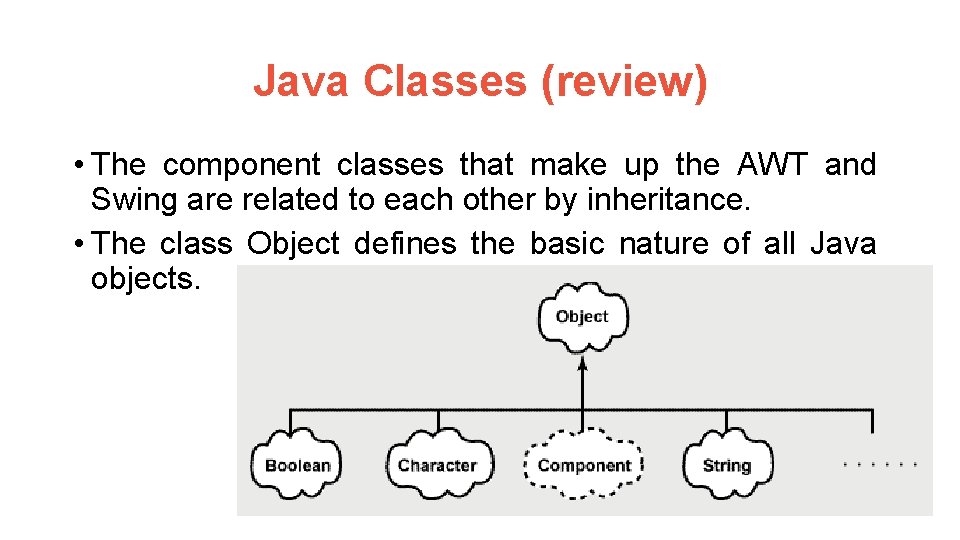
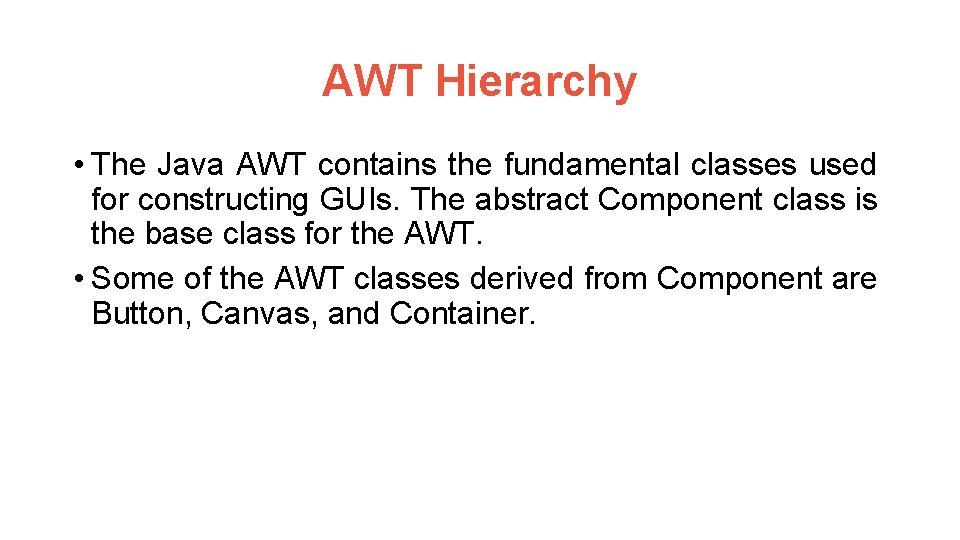
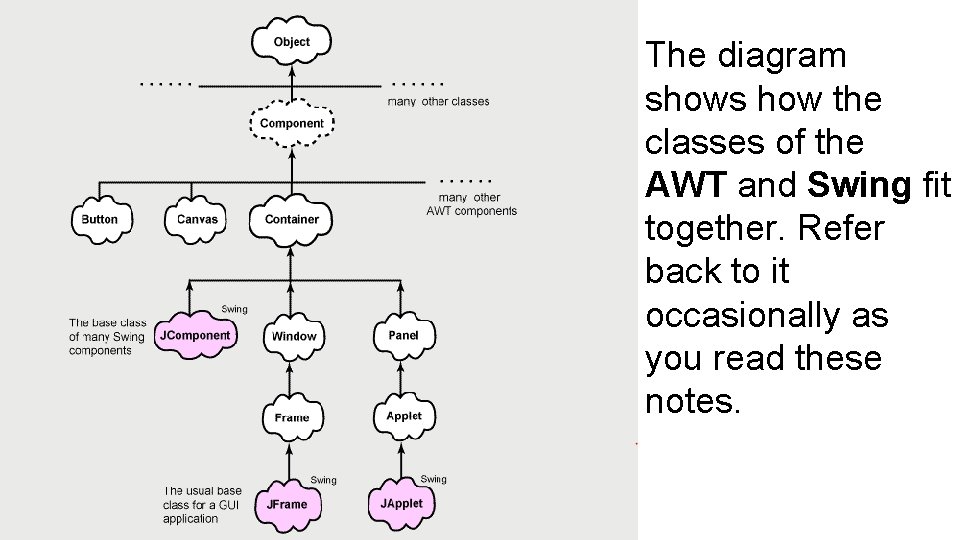
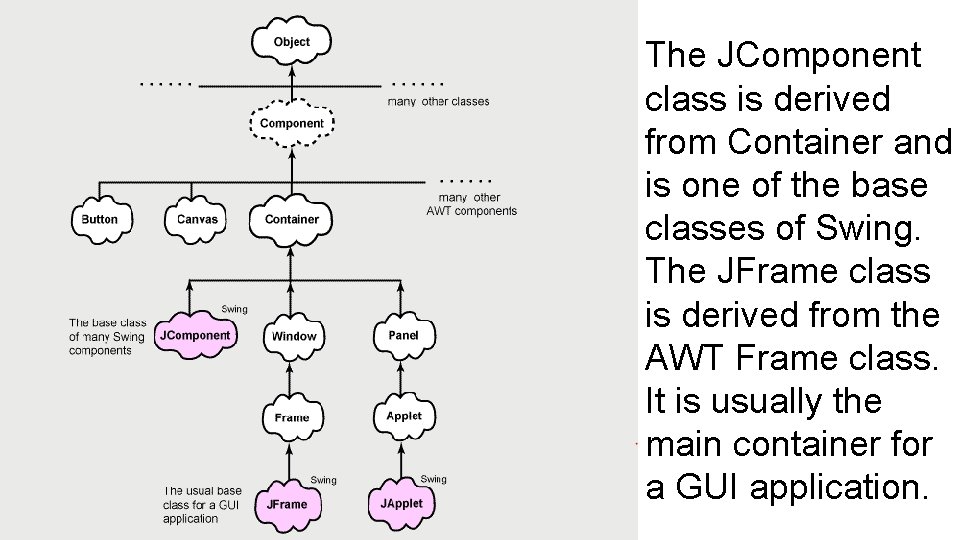
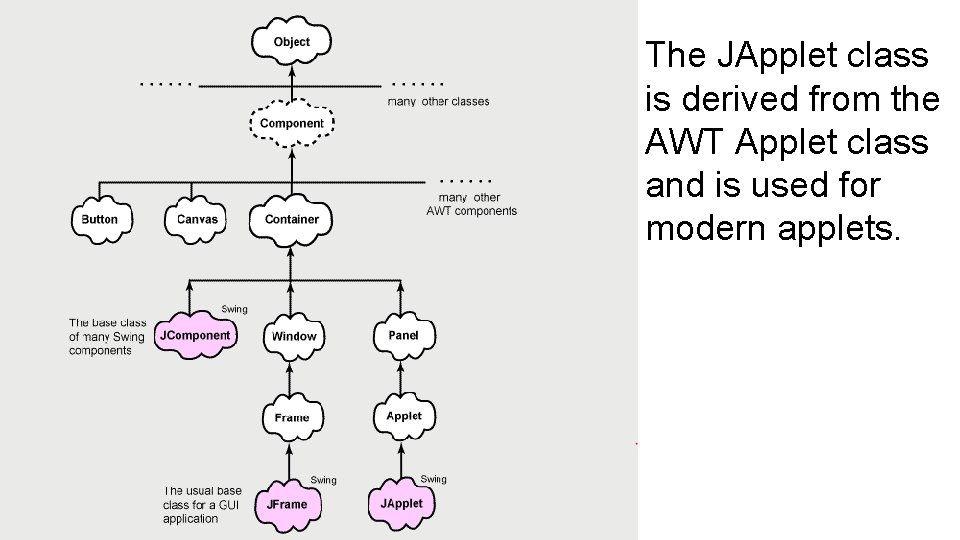
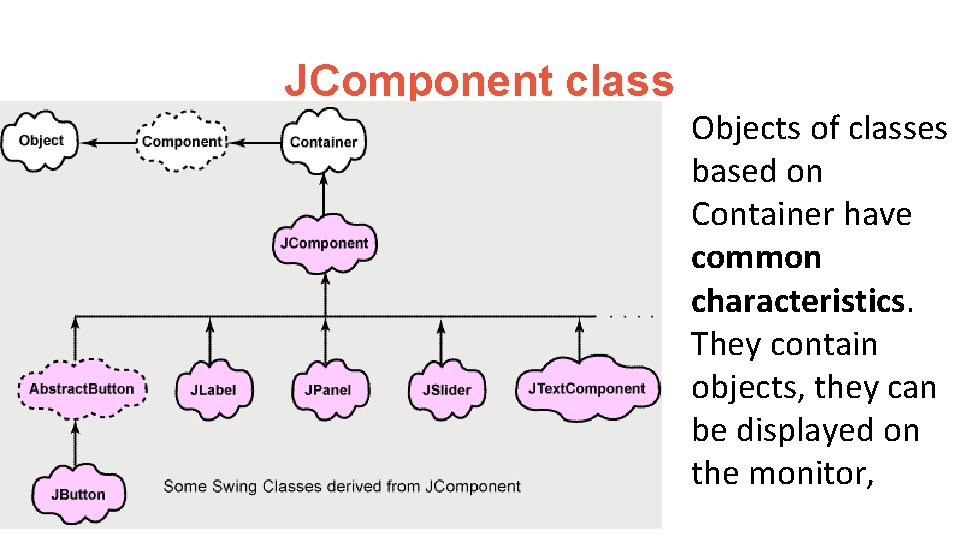
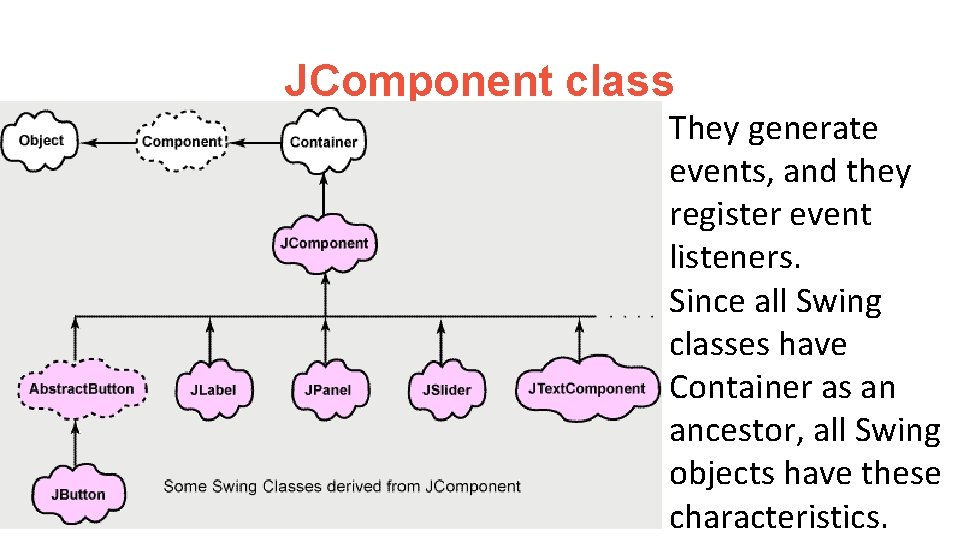
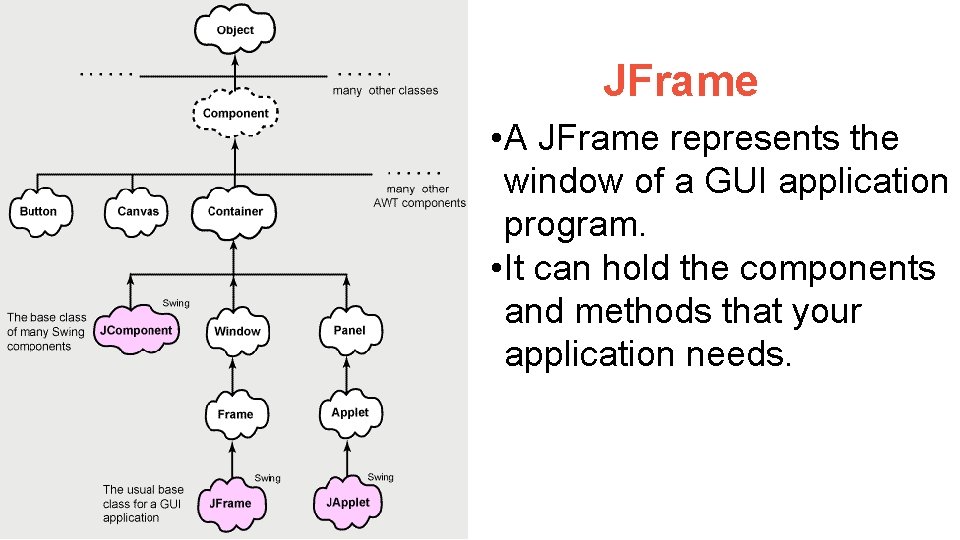
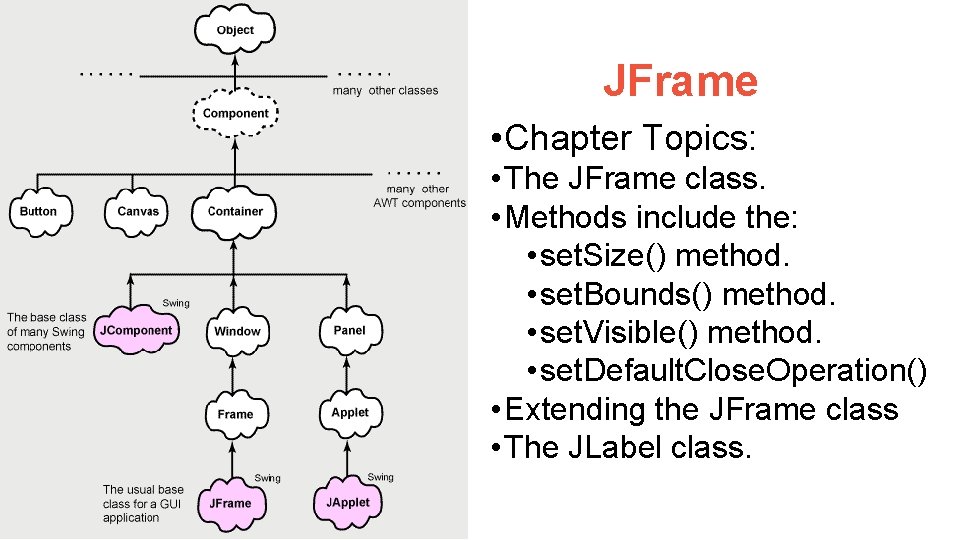
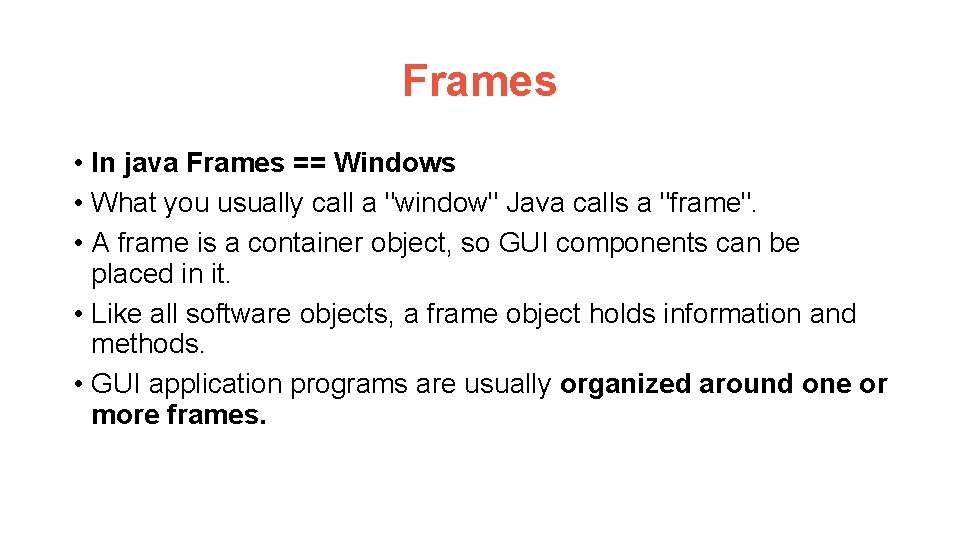
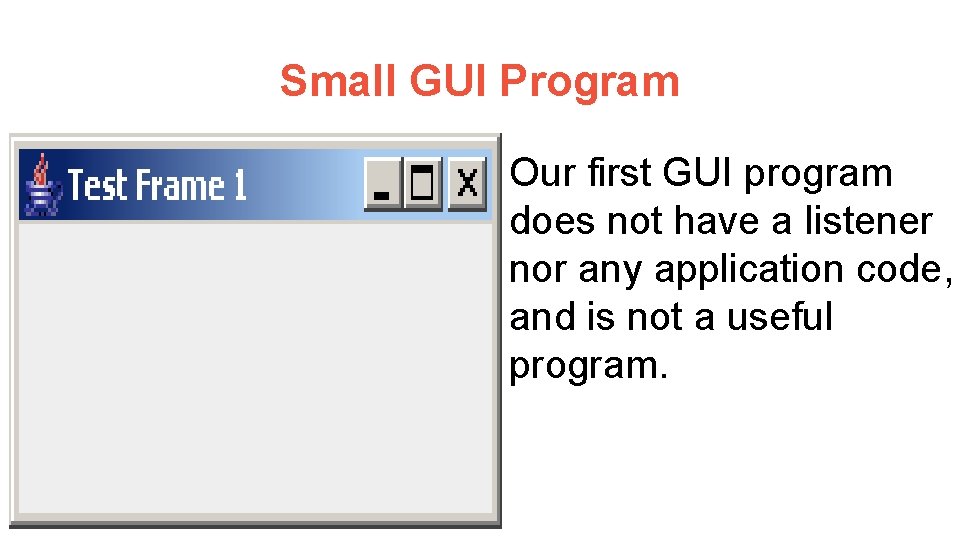
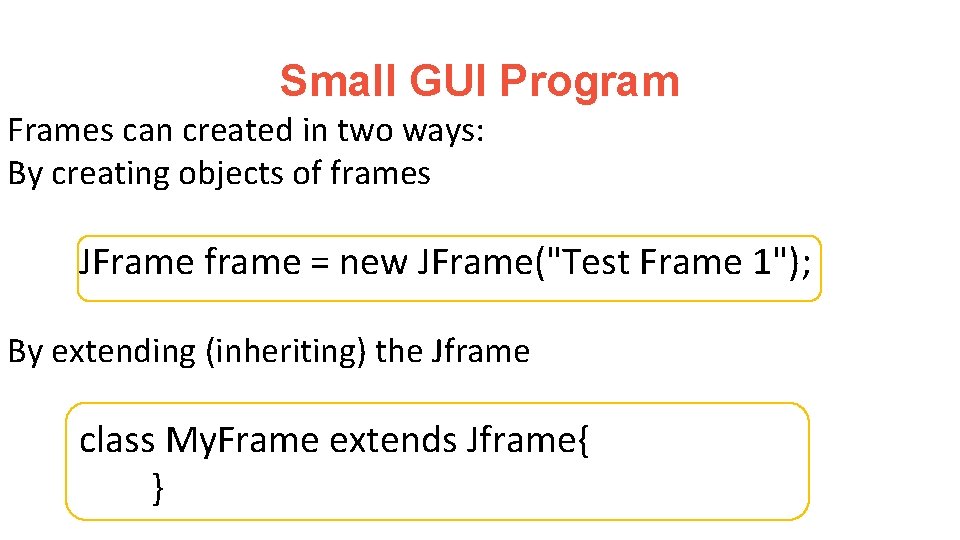
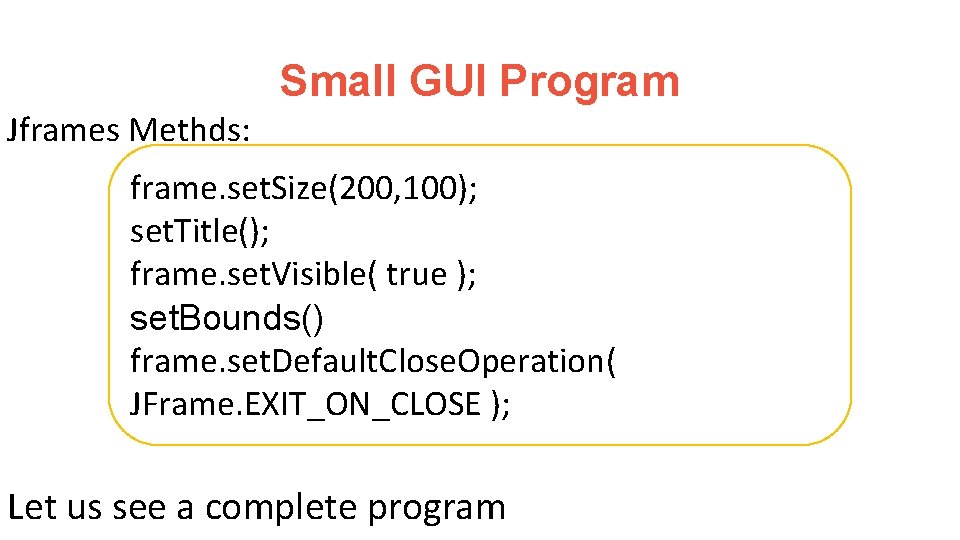
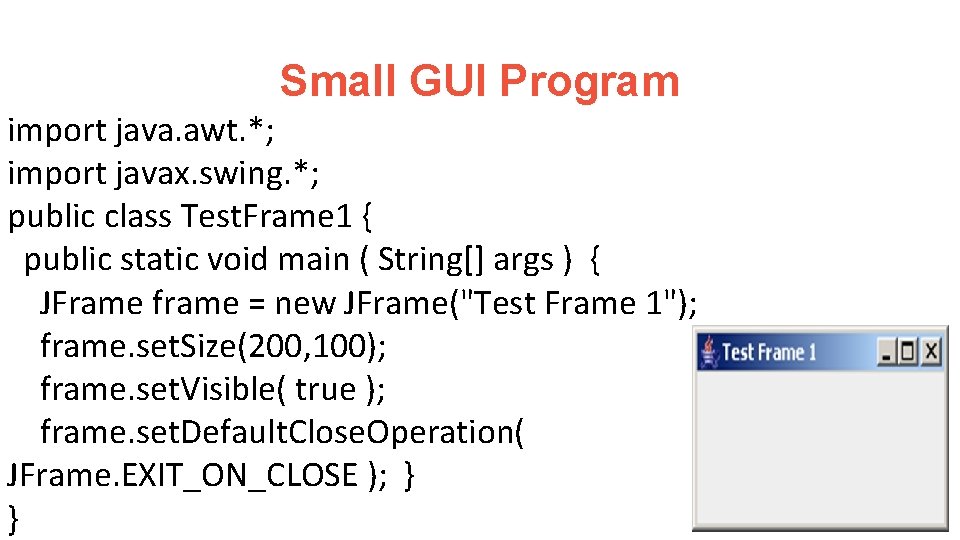
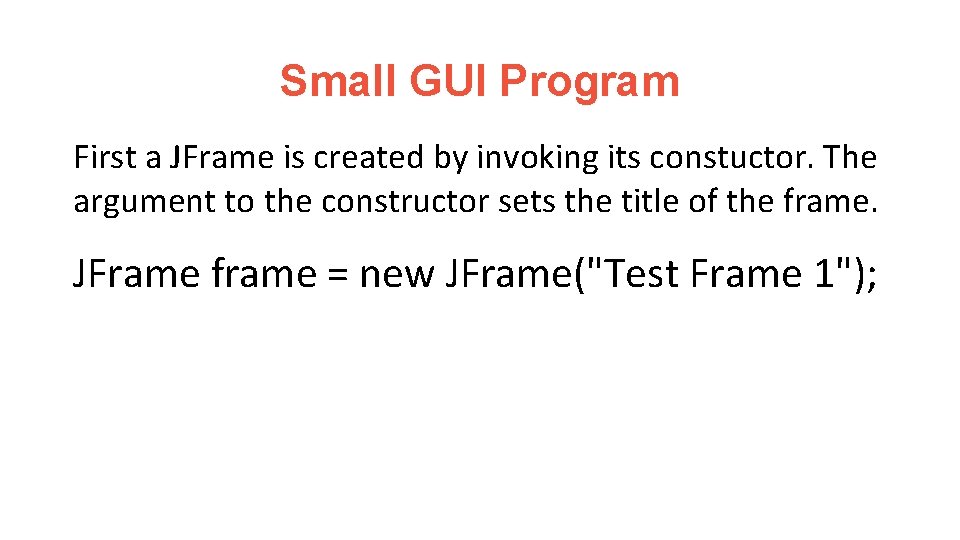
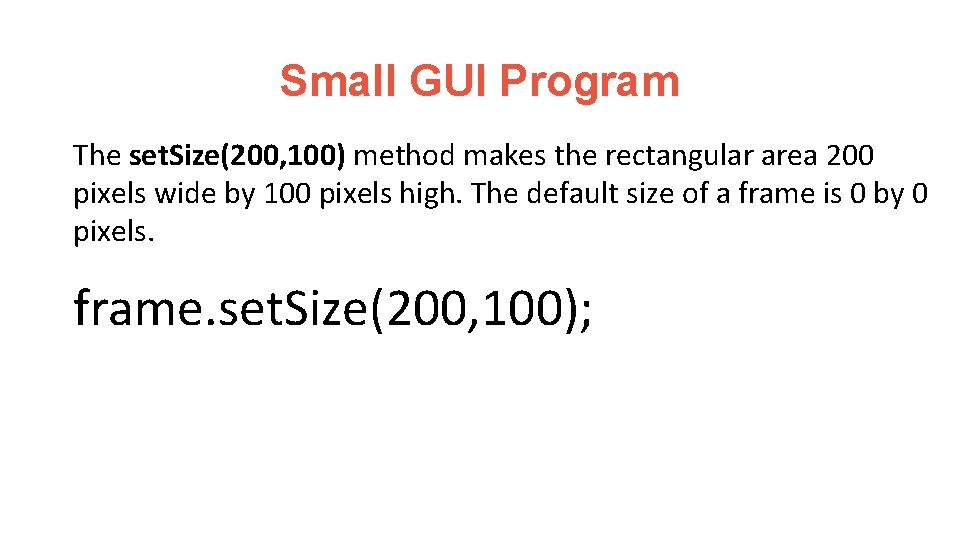
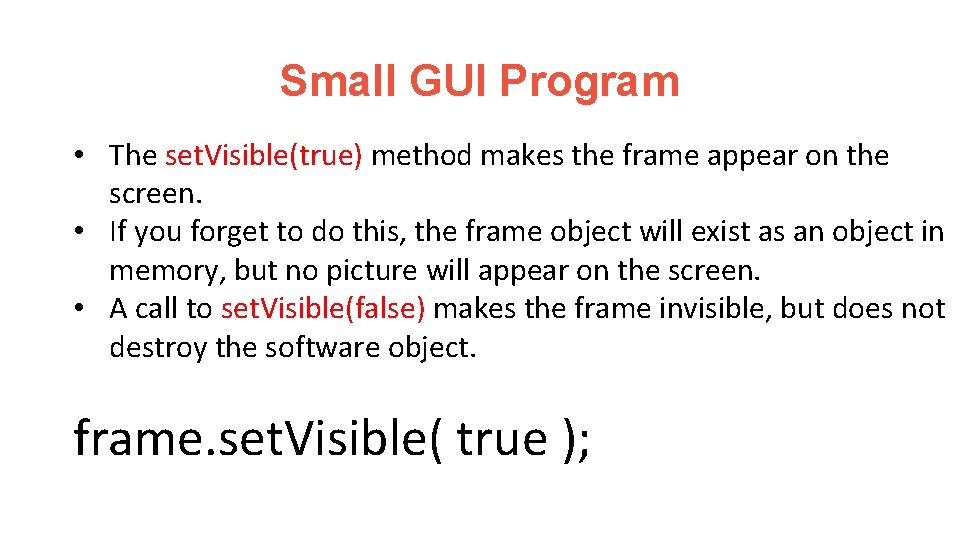
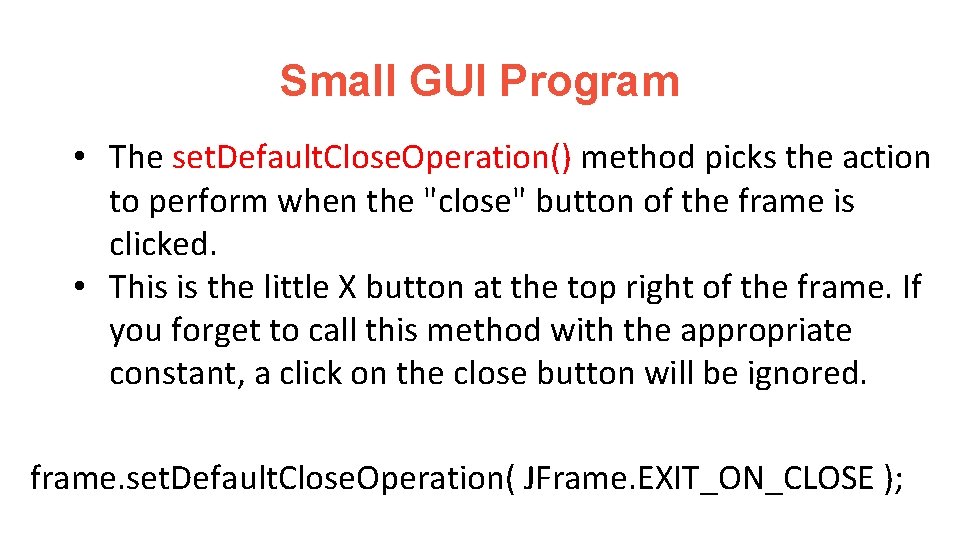
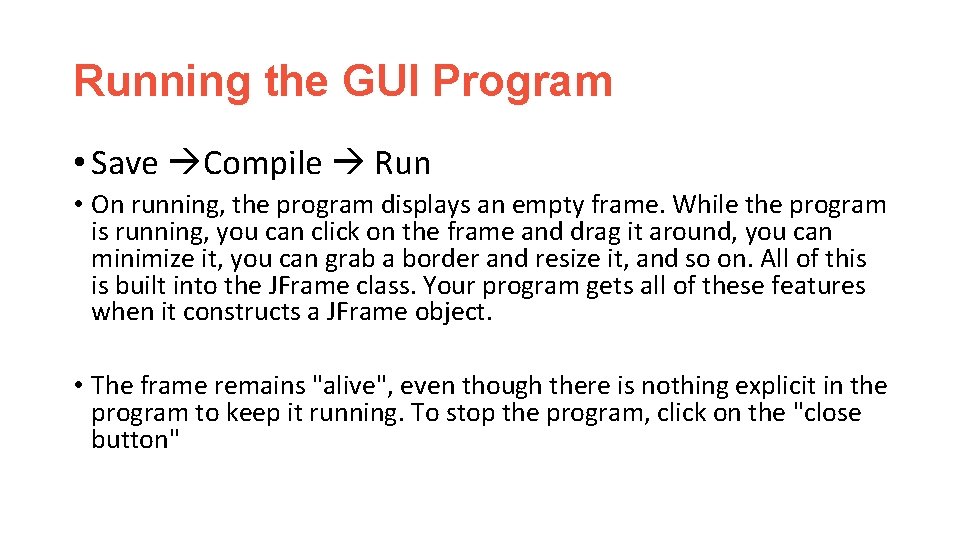
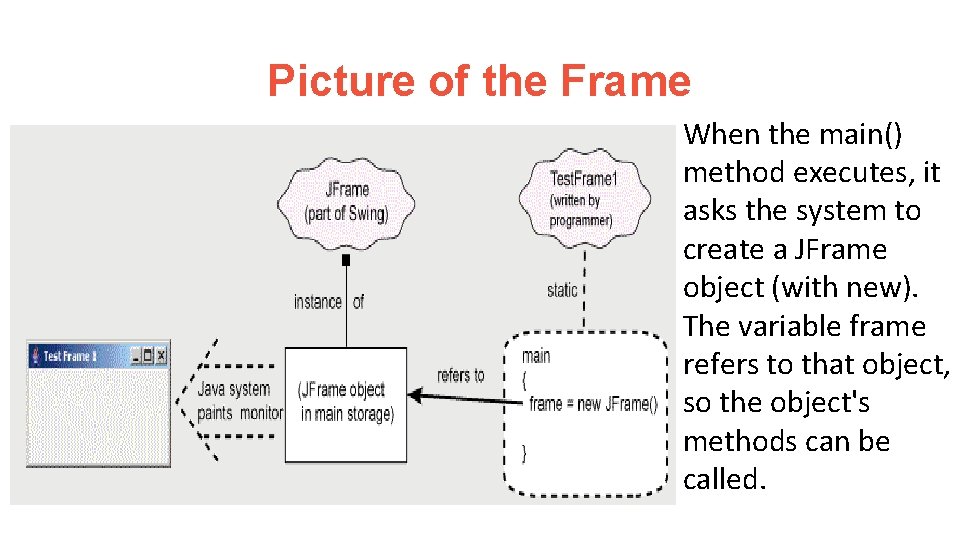
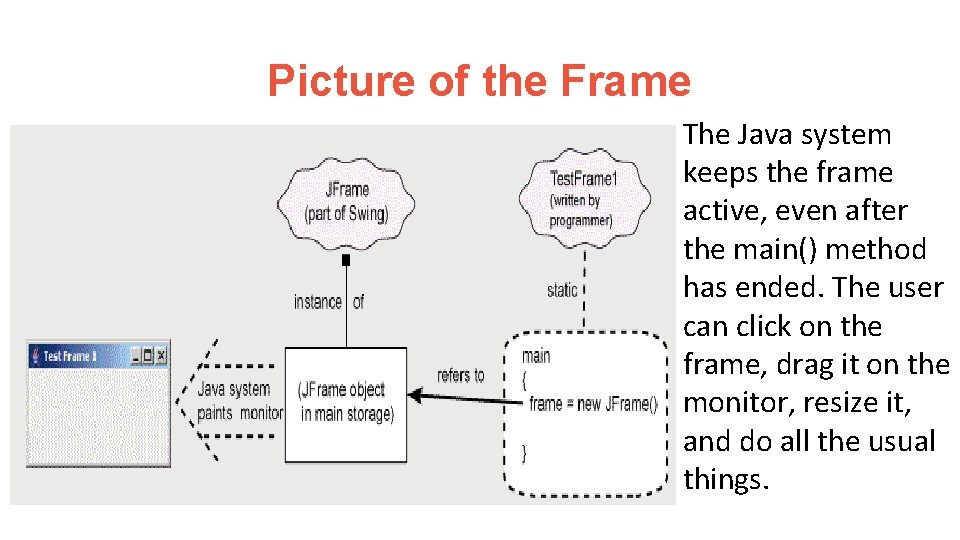
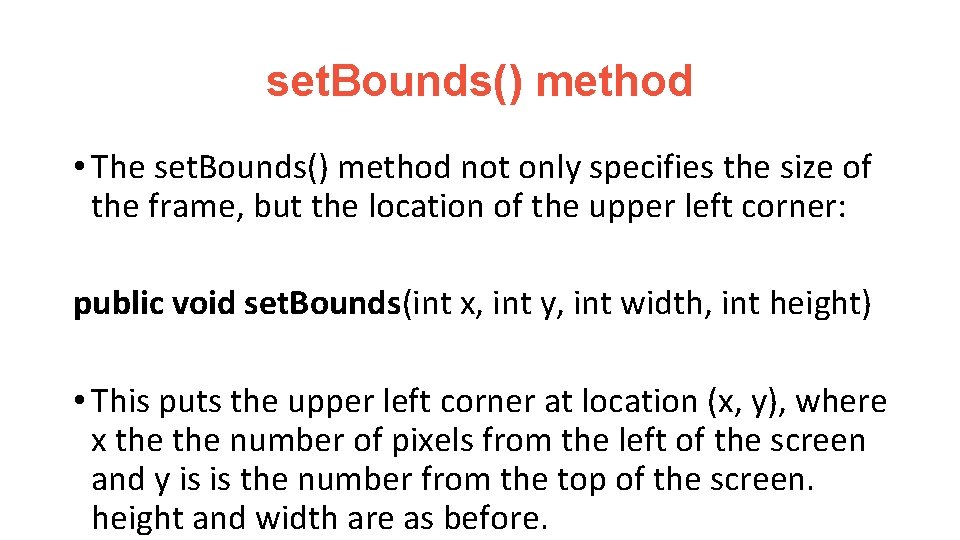
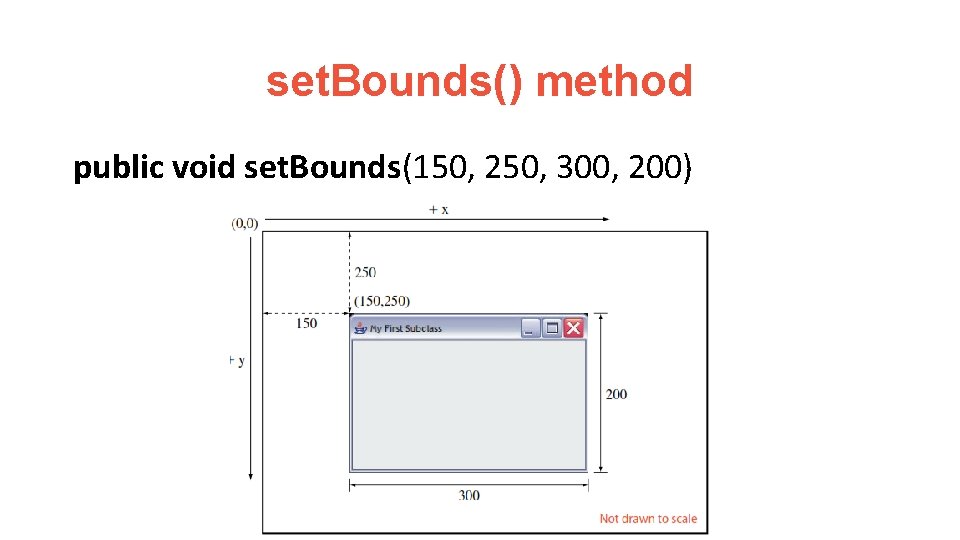
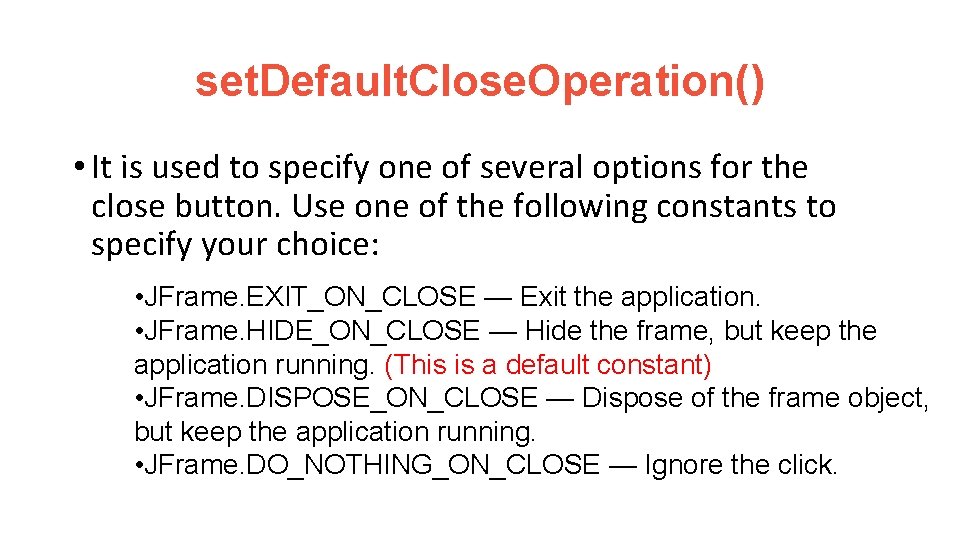
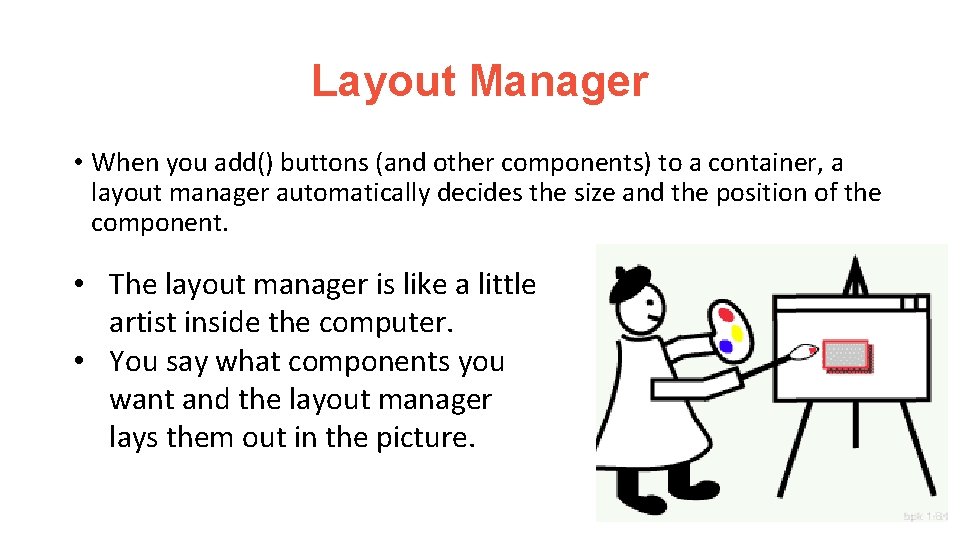
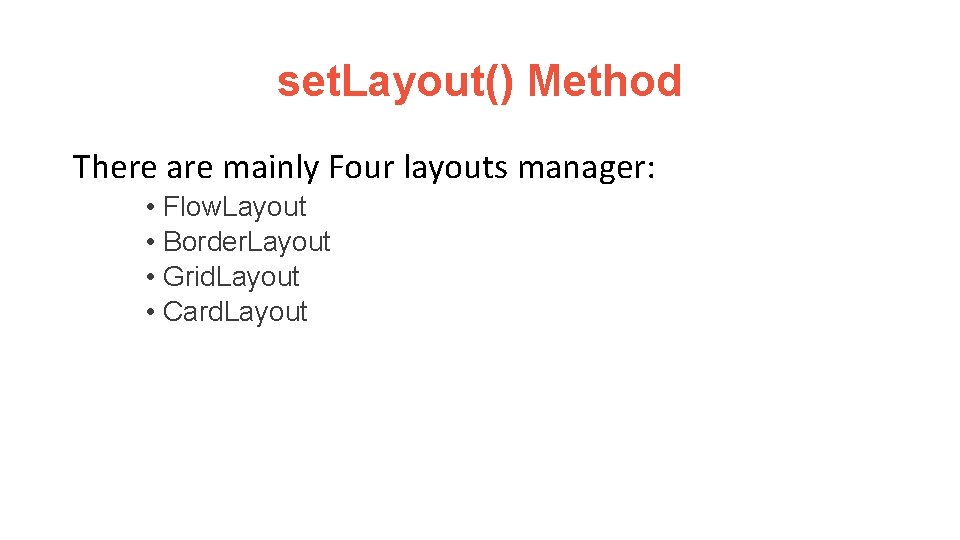
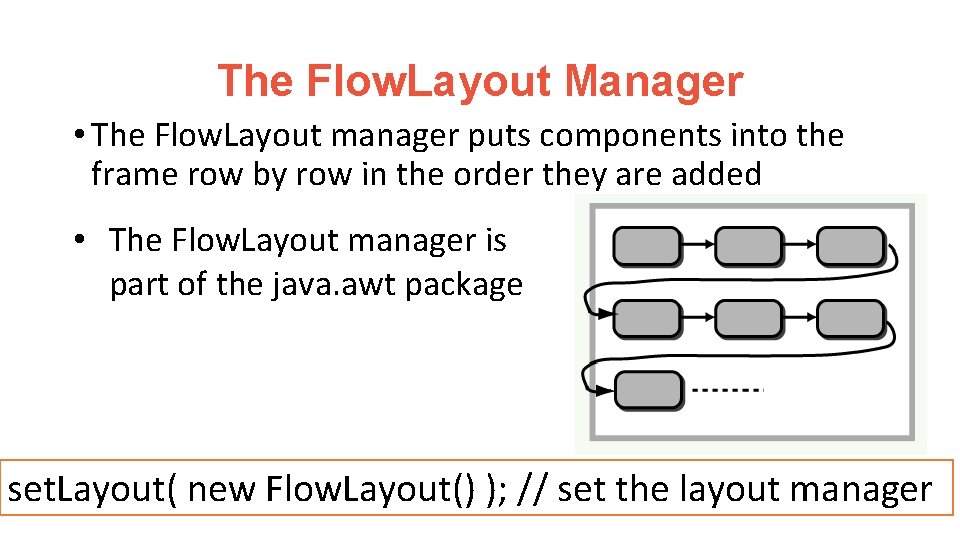
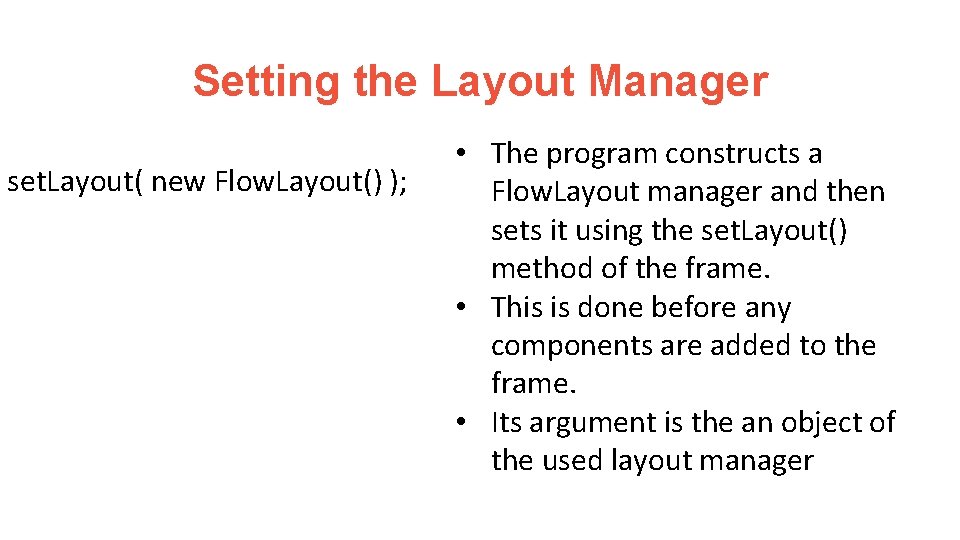
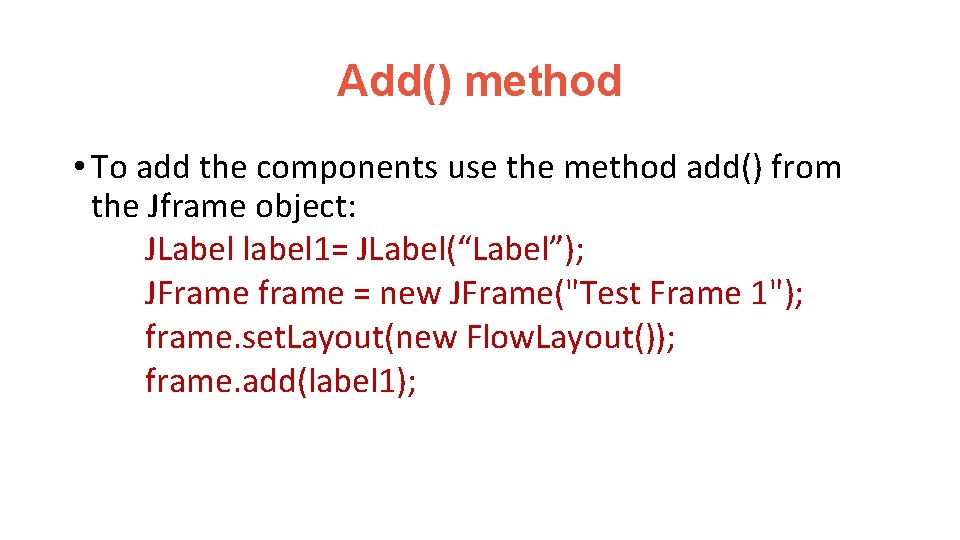
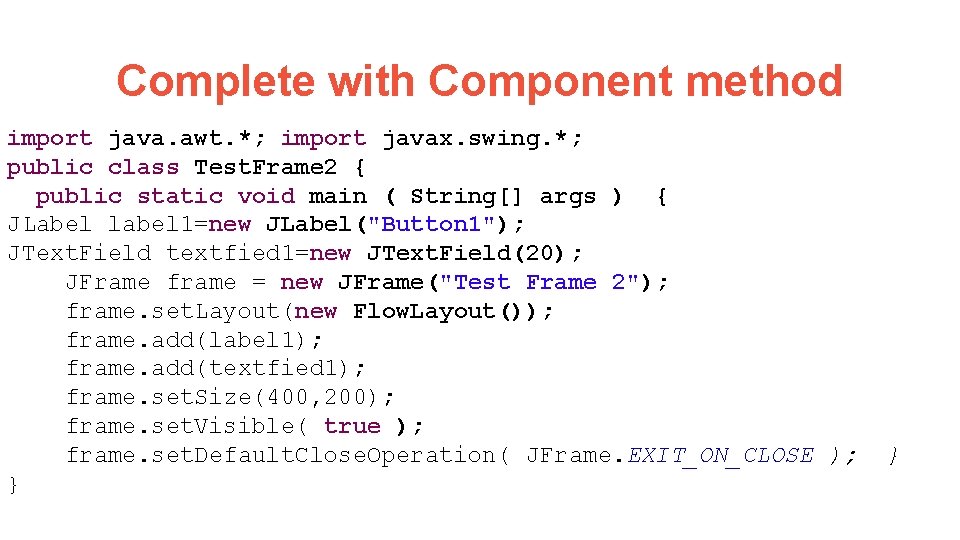
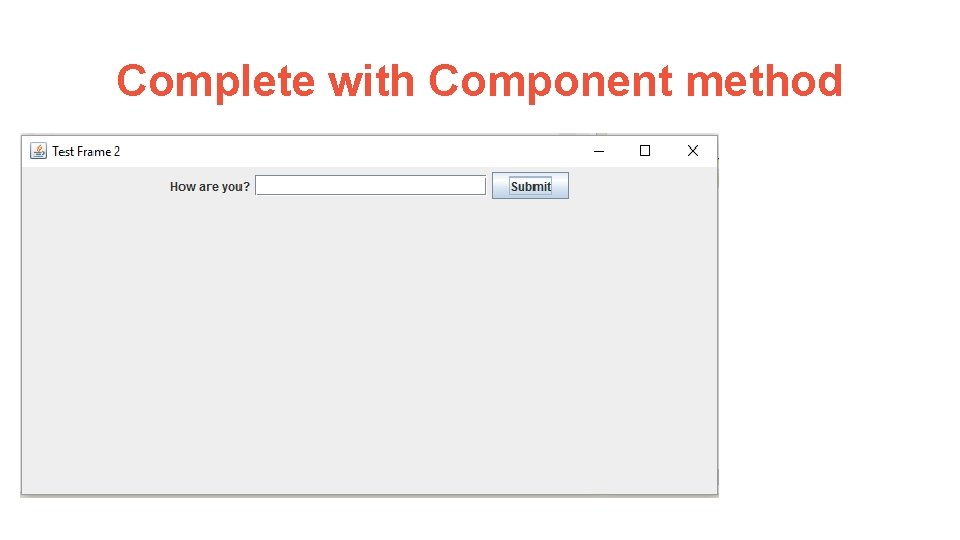
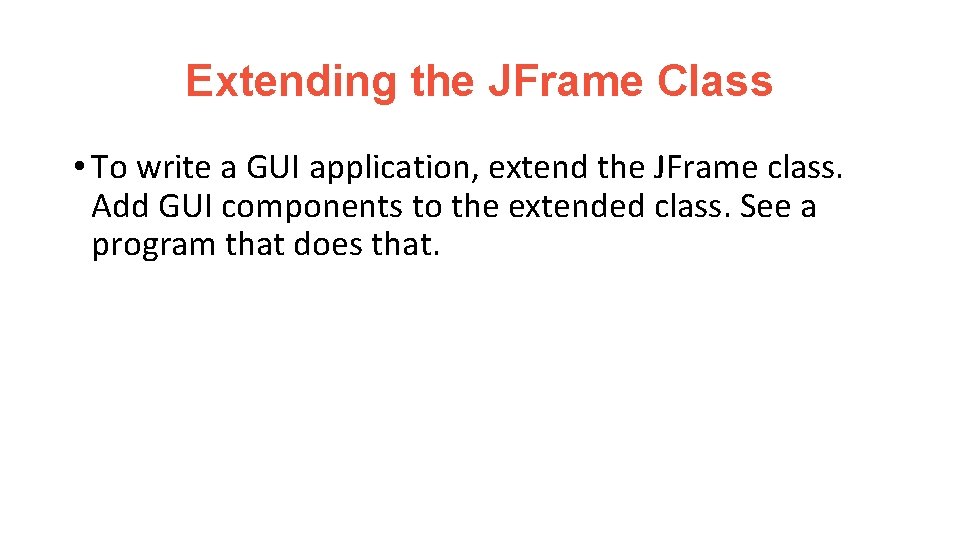
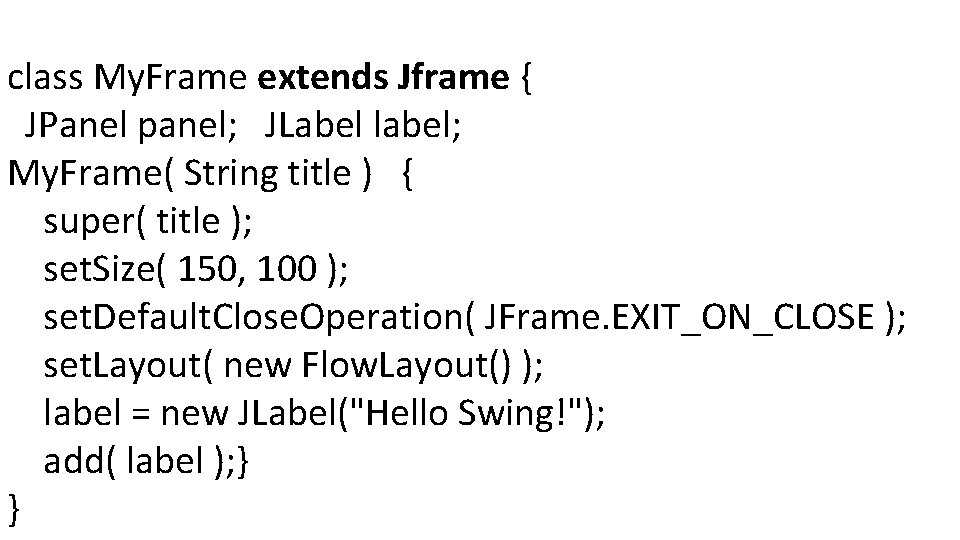
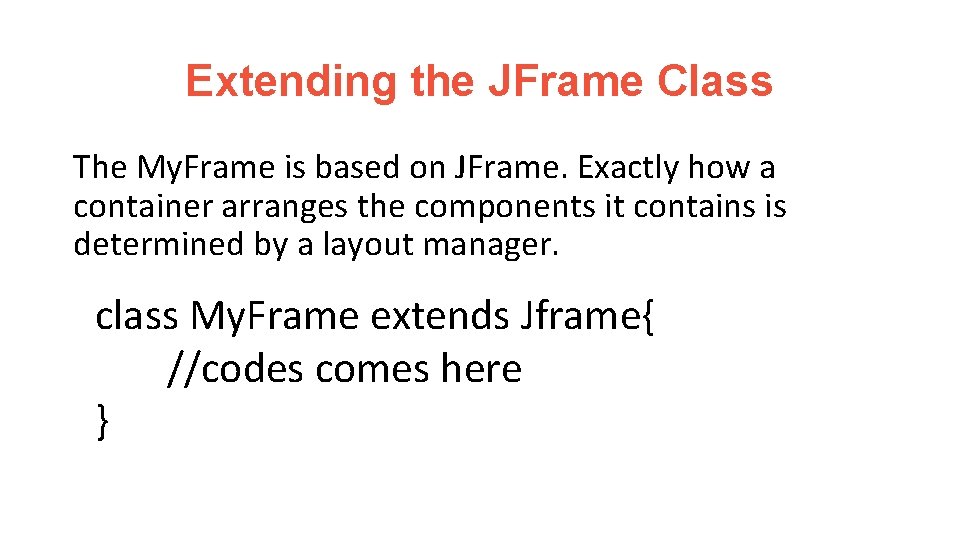
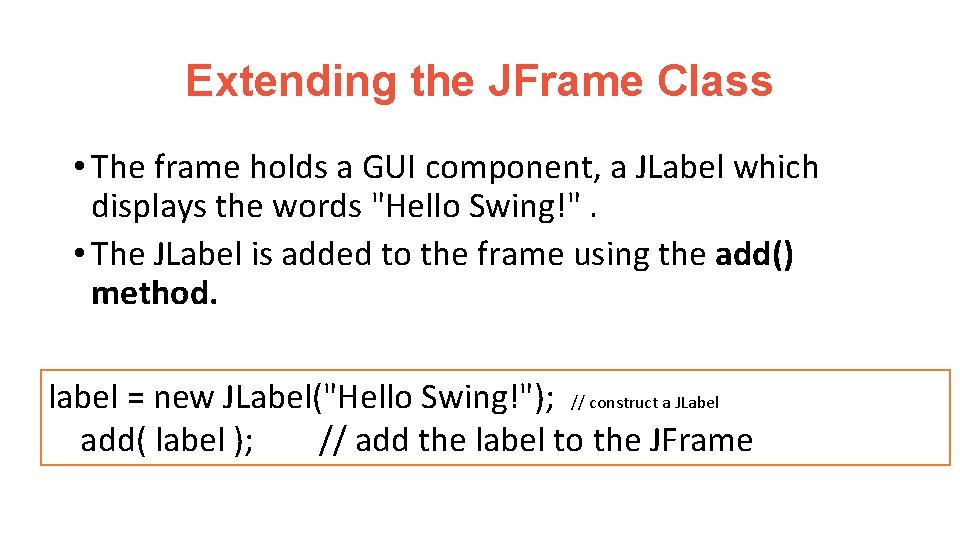
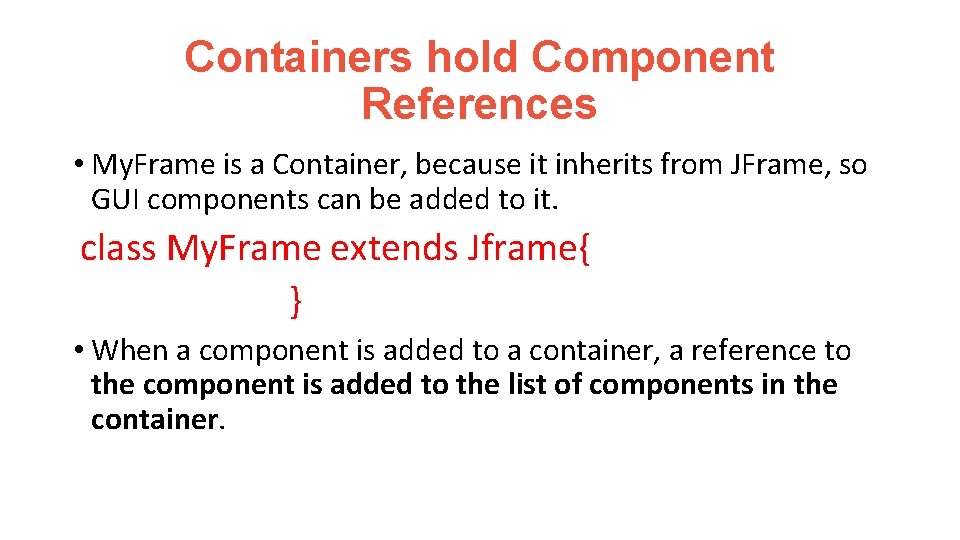
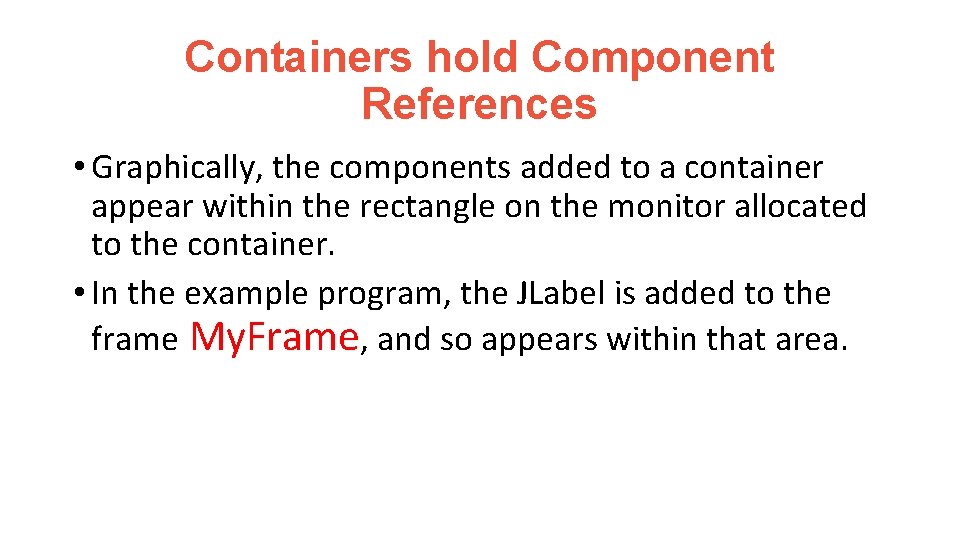
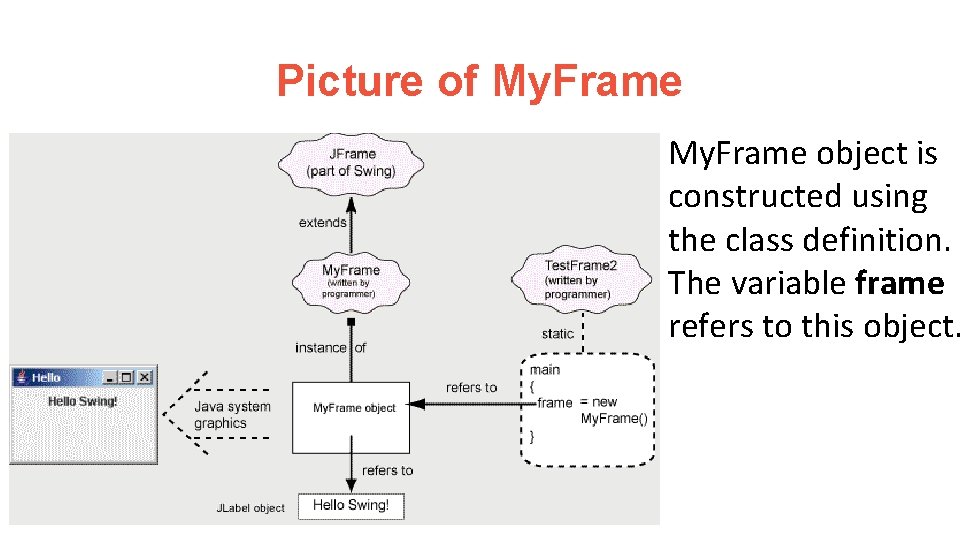
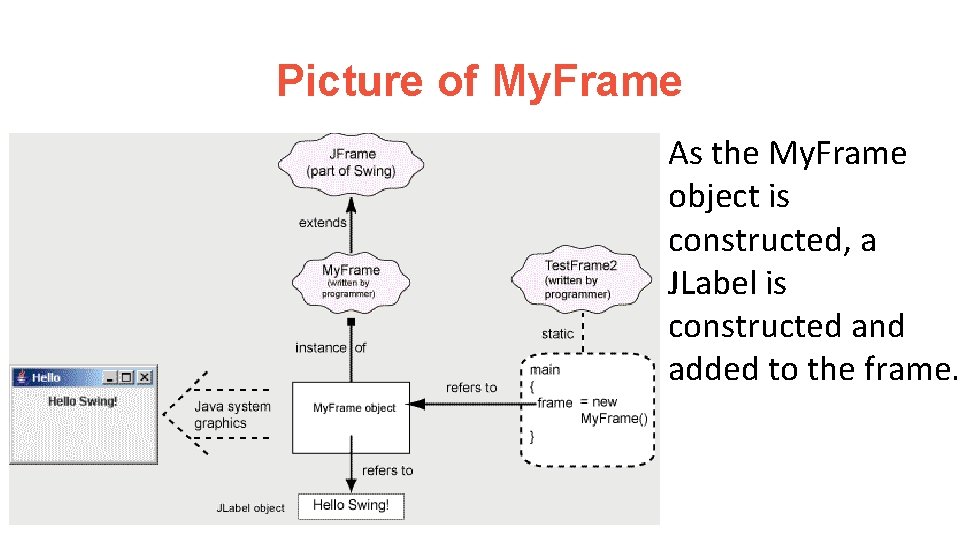
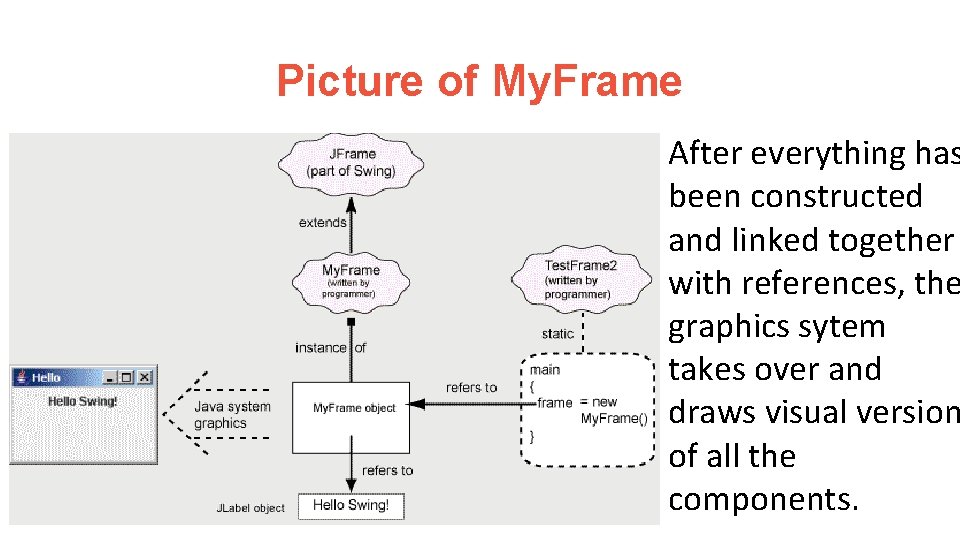
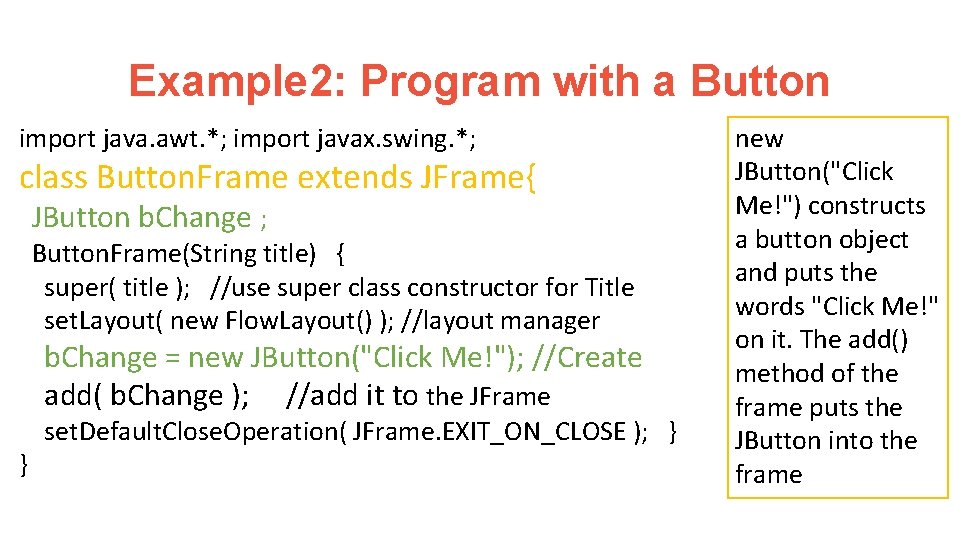
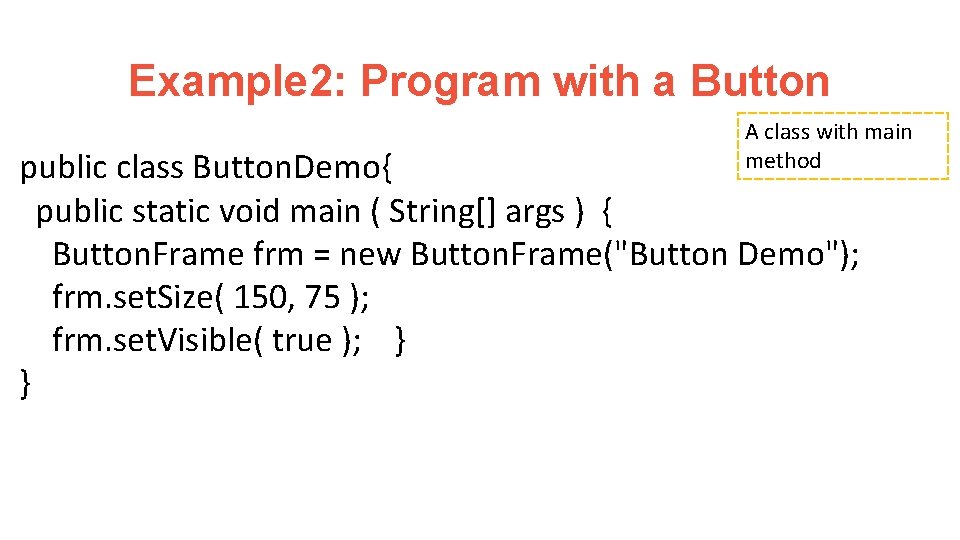
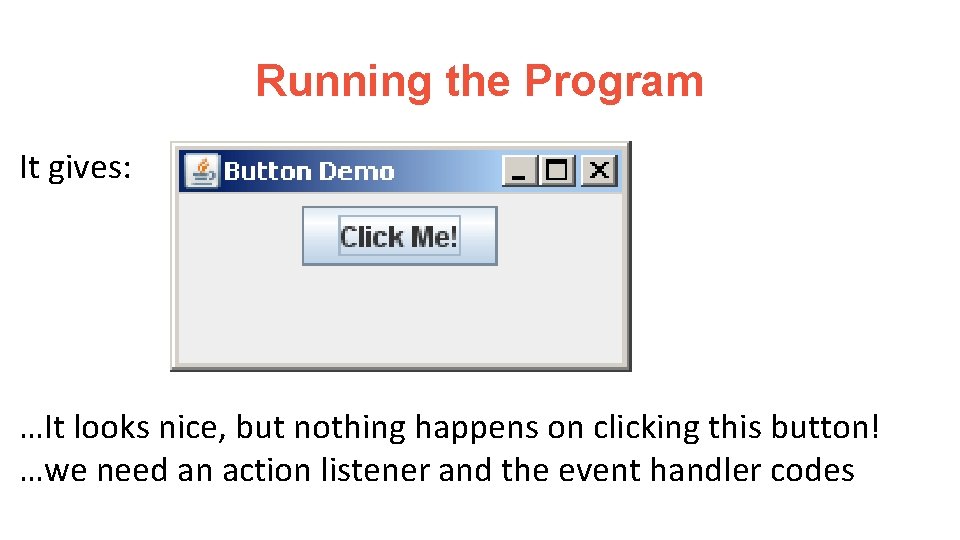
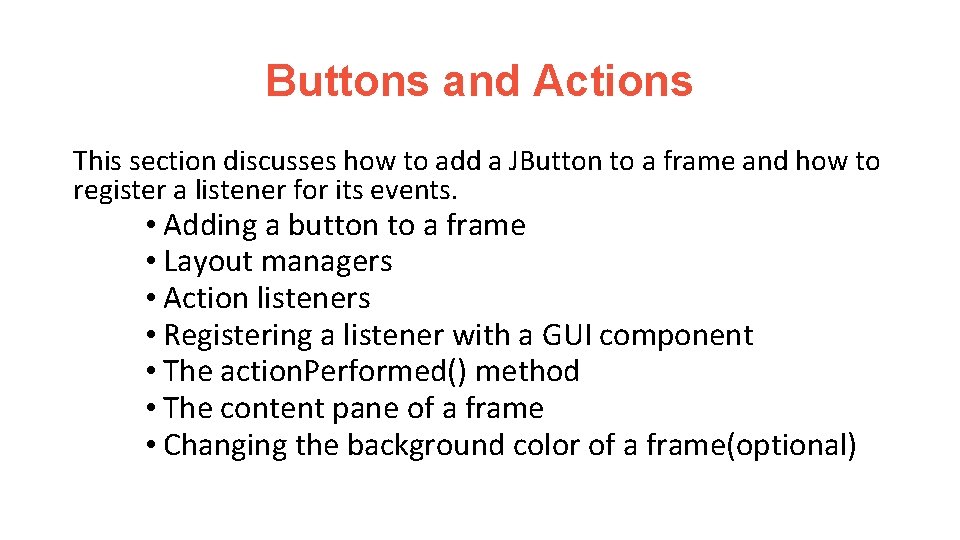
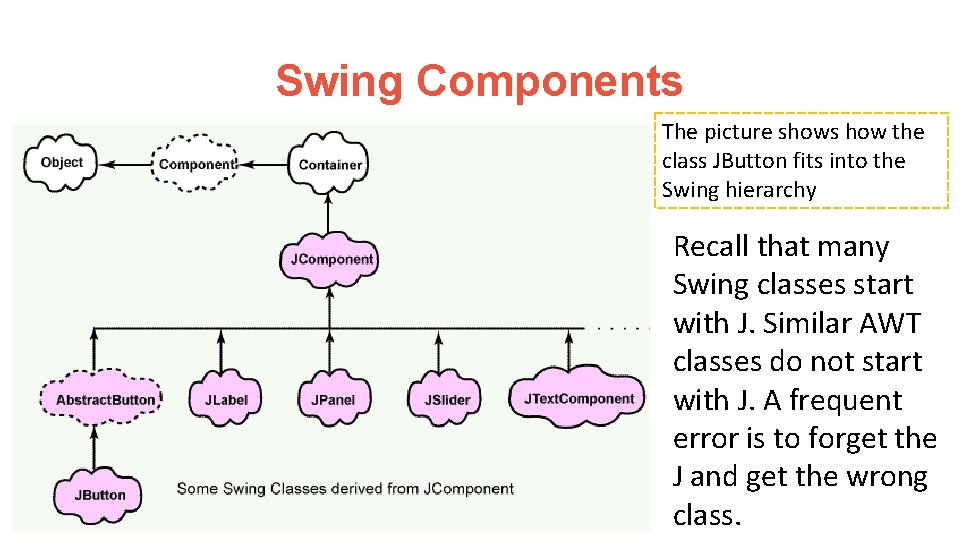
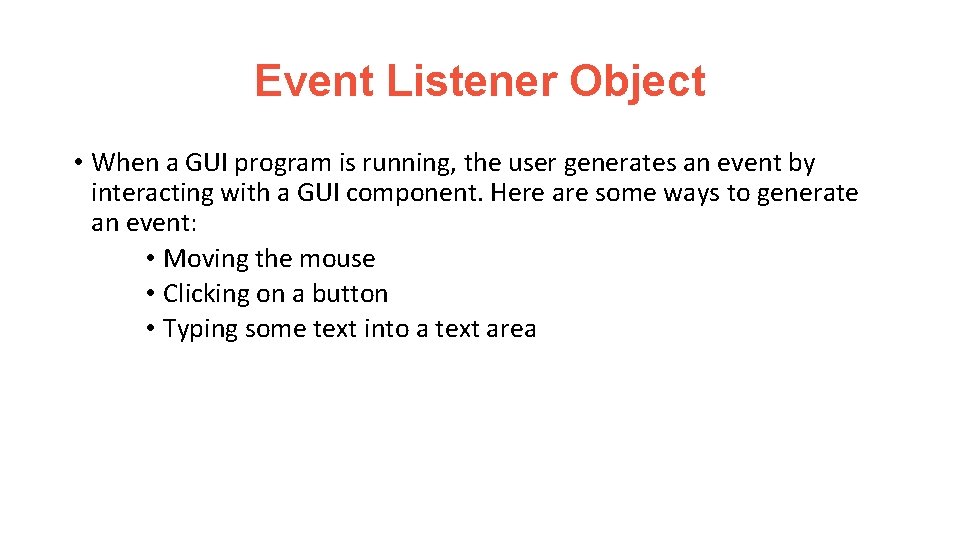
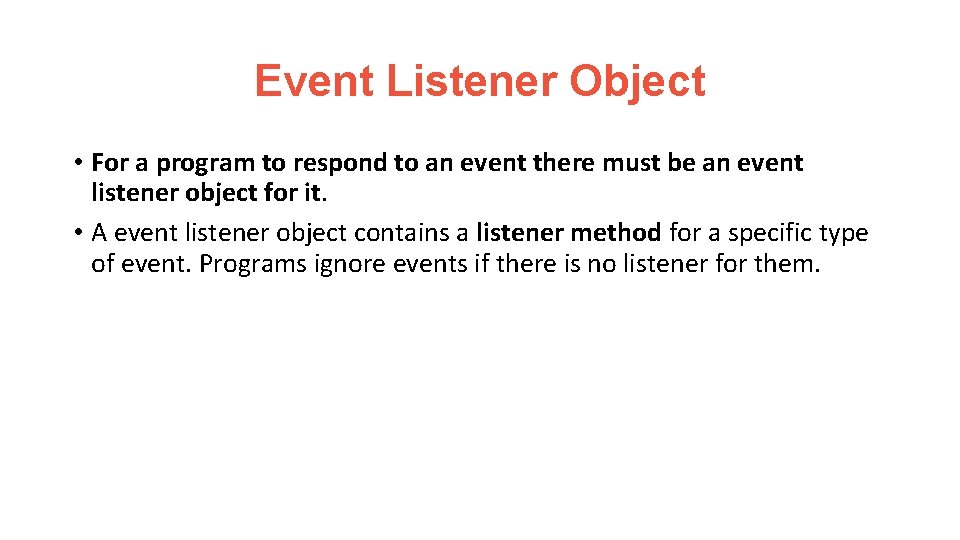
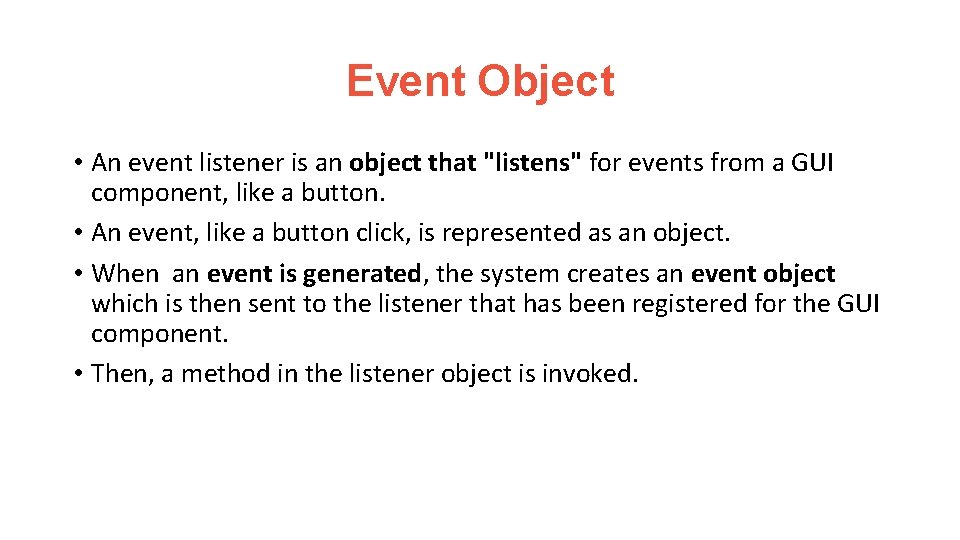
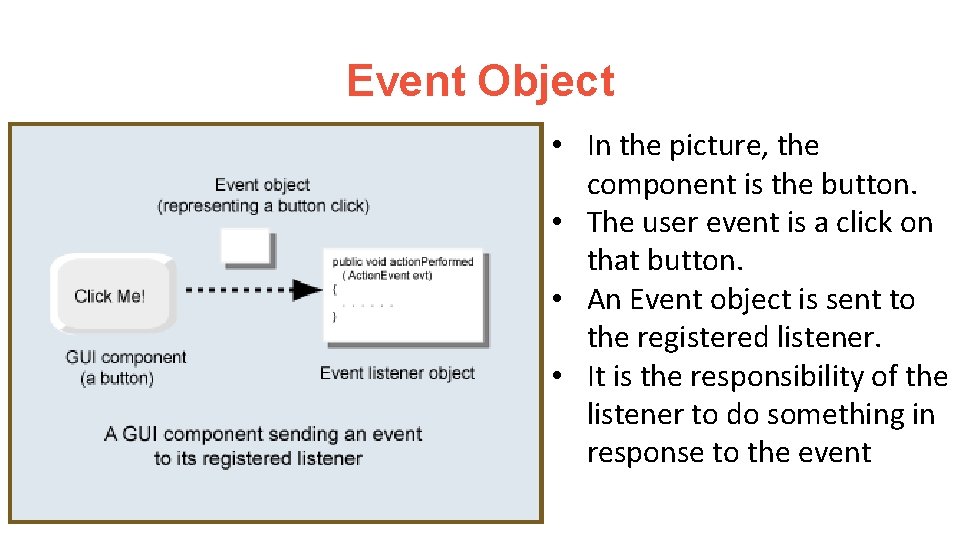
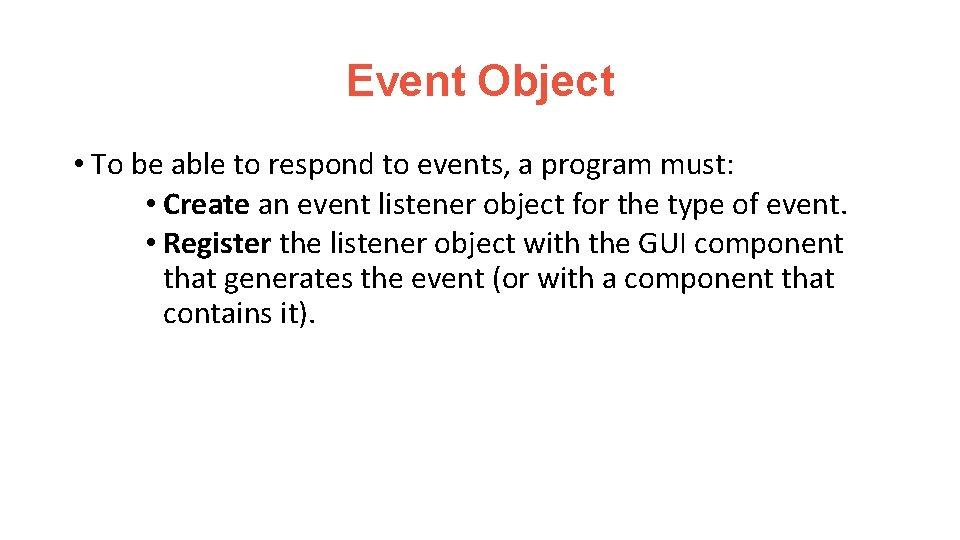
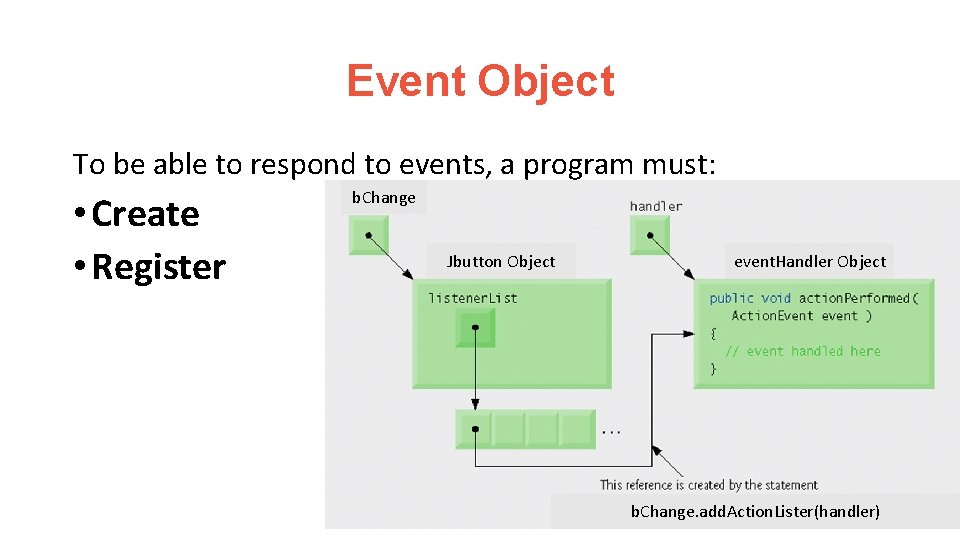
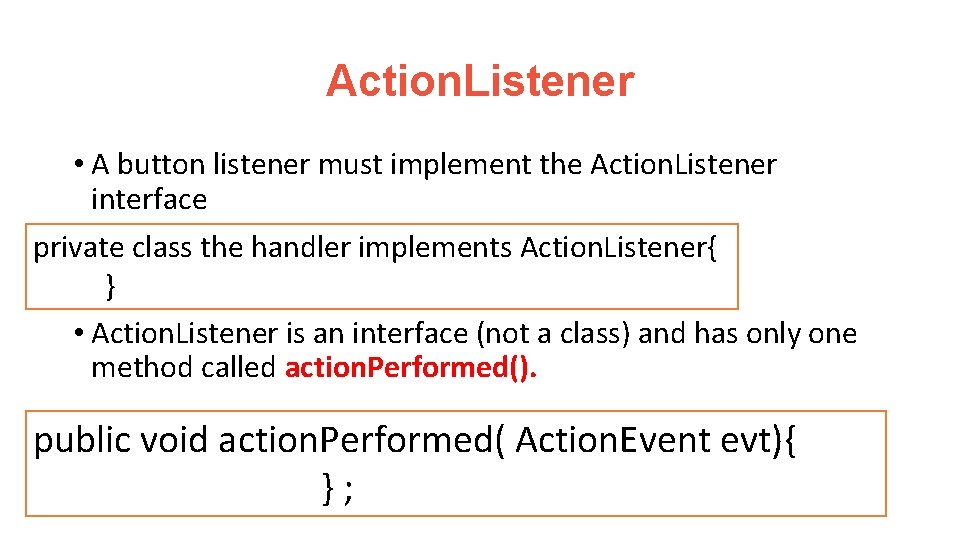
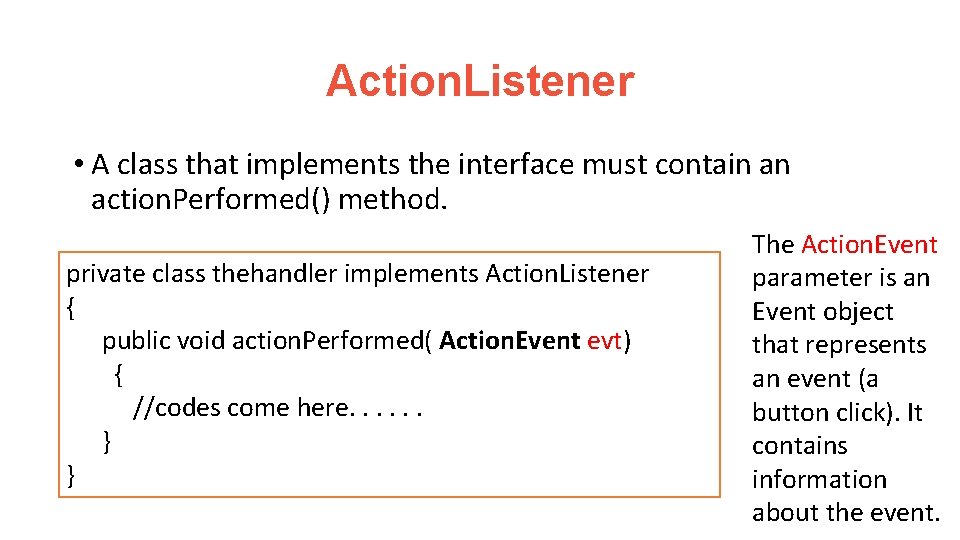
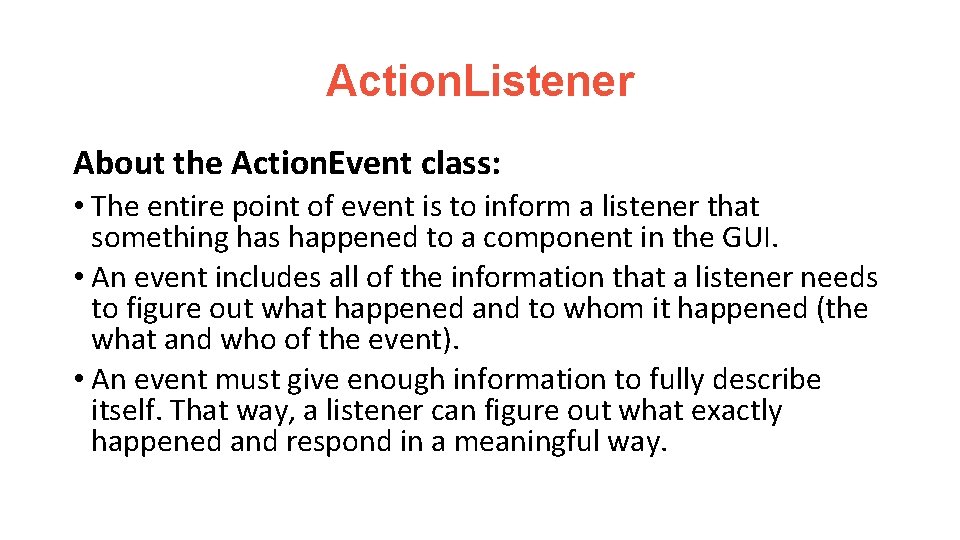
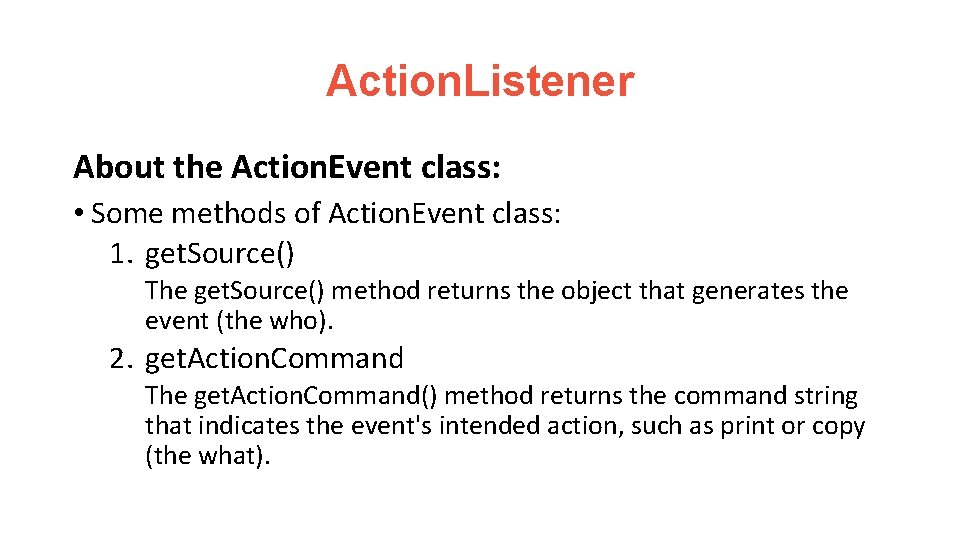
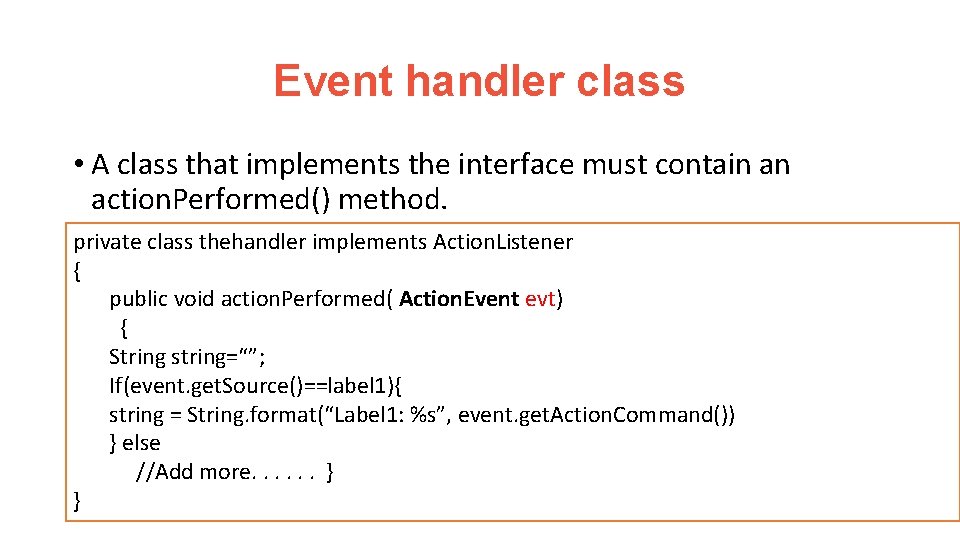
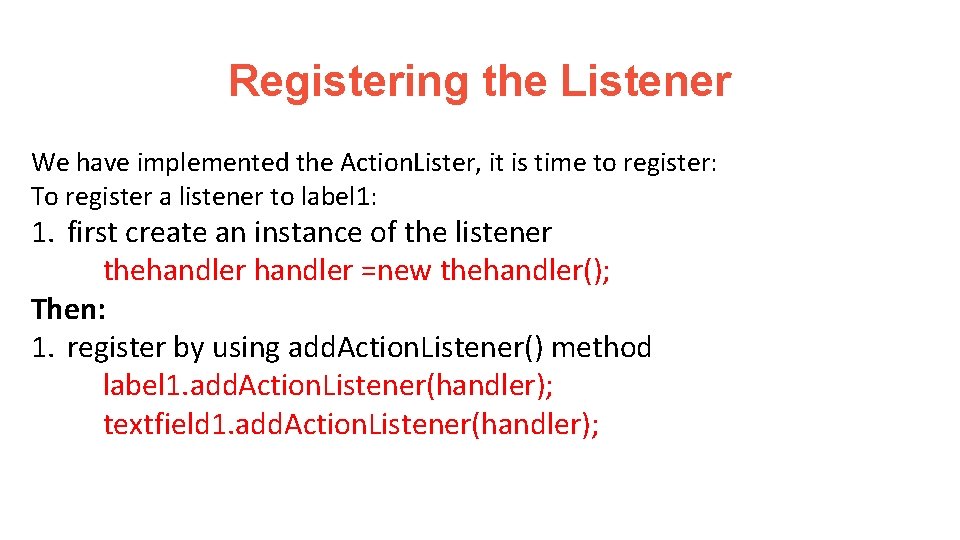
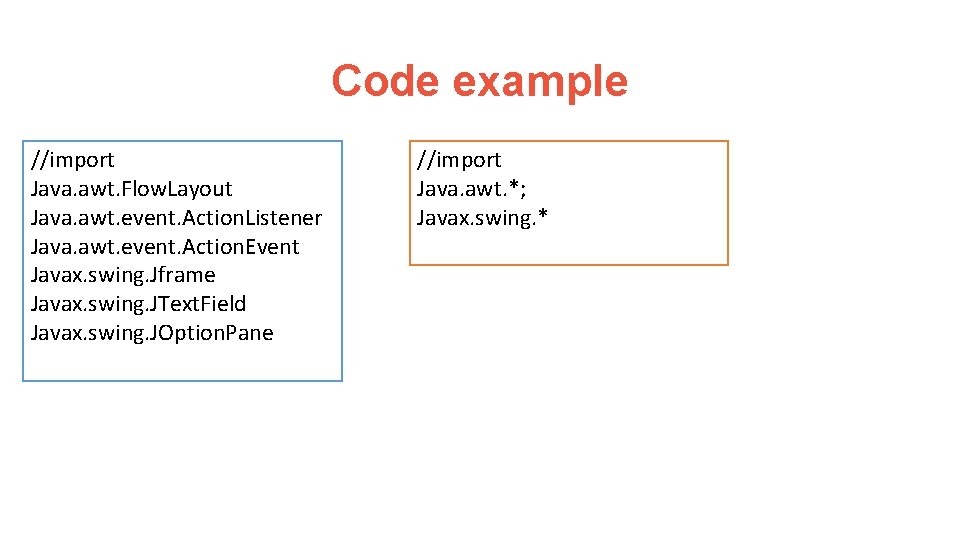
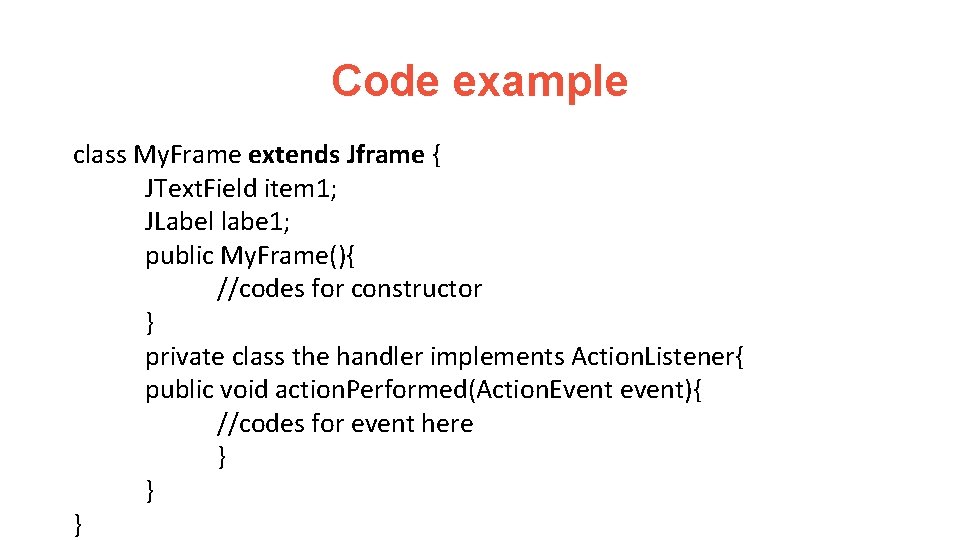
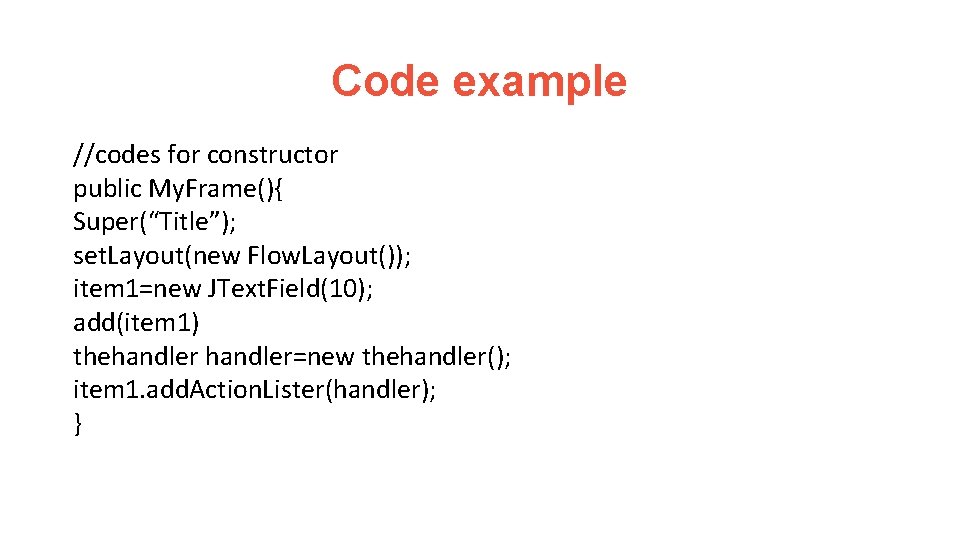
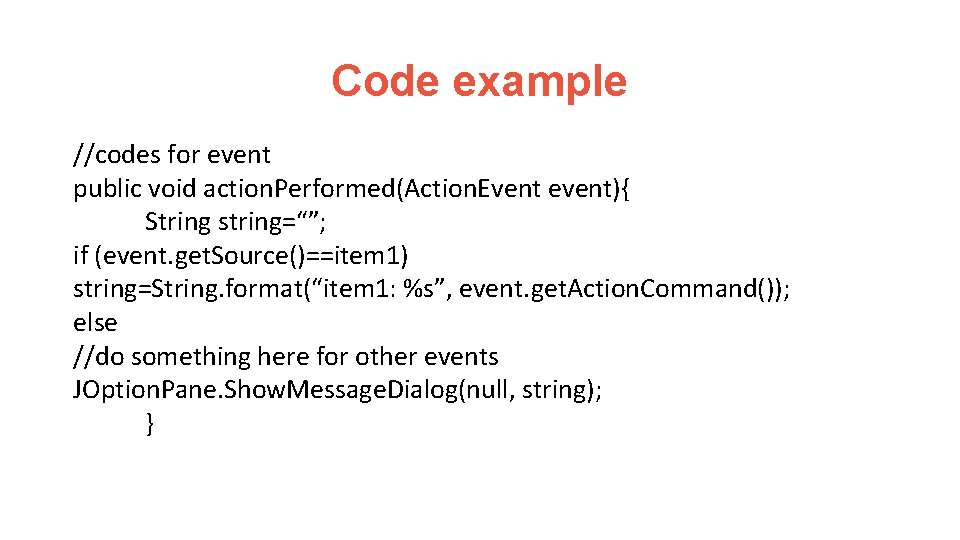
![Code example //main method public class My. Frame. Demo{ Public static void main(String[] args){ Code example //main method public class My. Frame. Demo{ Public static void main(String[] args){](https://slidetodoc.com/presentation_image_h/0c3d932d7ad923f768bb9733747892ff/image-89.jpg)
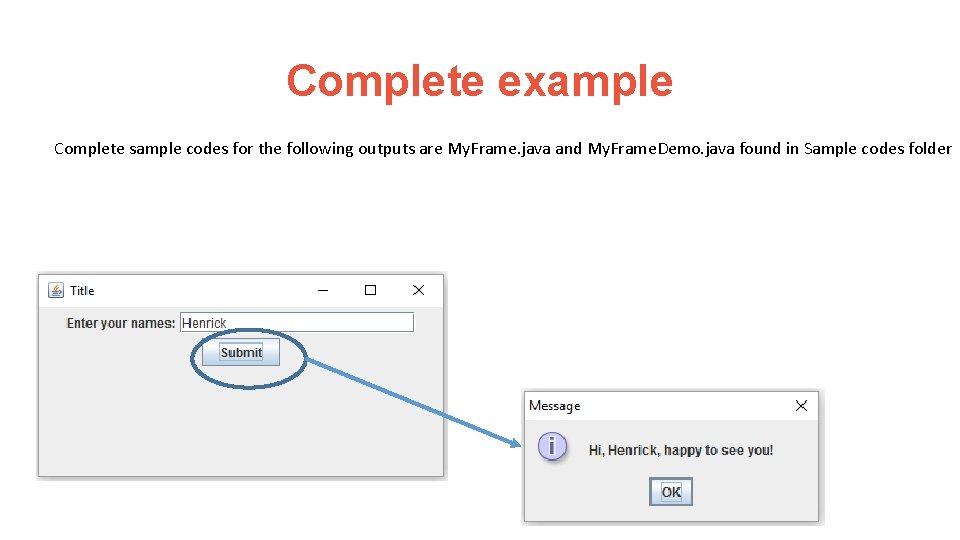
- Slides: 90
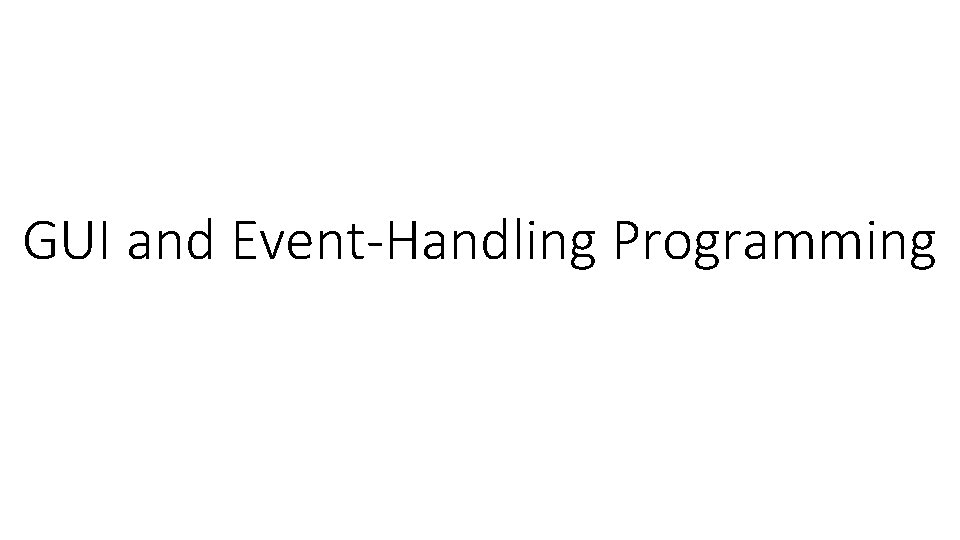
GUI and Event-Handling Programming
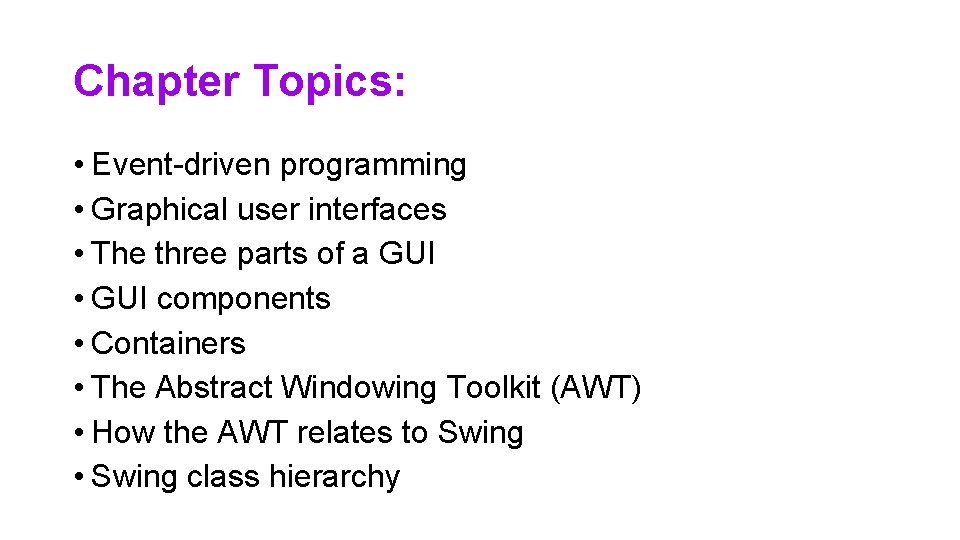
Chapter Topics: • Event-driven programming • Graphical user interfaces • The three parts of a GUI • GUI components • Containers • The Abstract Windowing Toolkit (AWT) • How the AWT relates to Swing • Swing class hierarchy
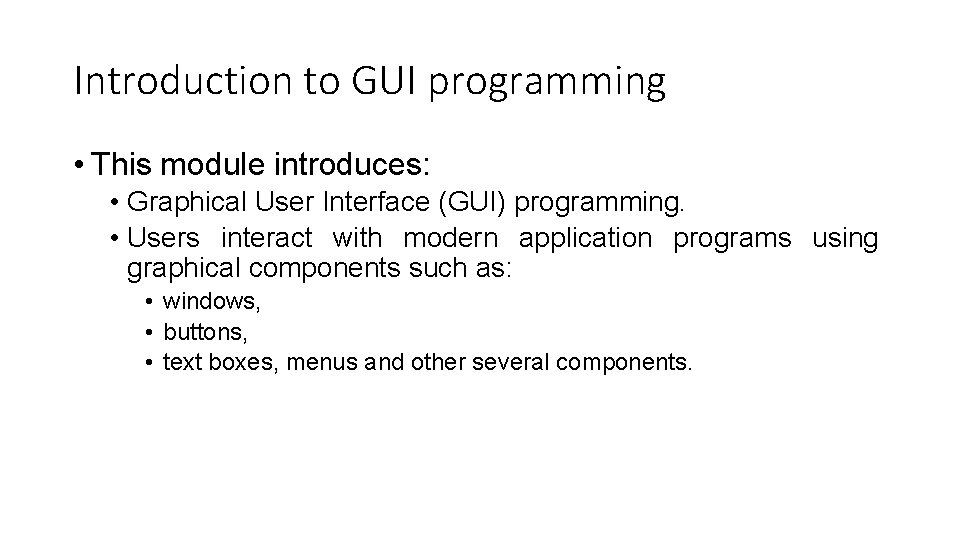
Introduction to GUI programming • This module introduces: • Graphical User Interface (GUI) programming. • Users interact with modern application programs using graphical components such as: • windows, • buttons, • text boxes, menus and other several components.
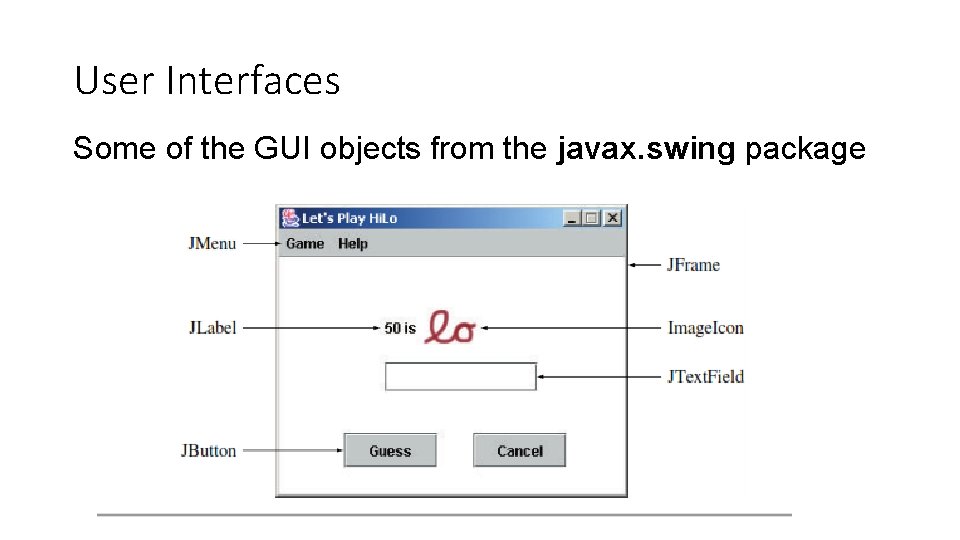
User Interfaces Some of the GUI objects from the javax. swing package
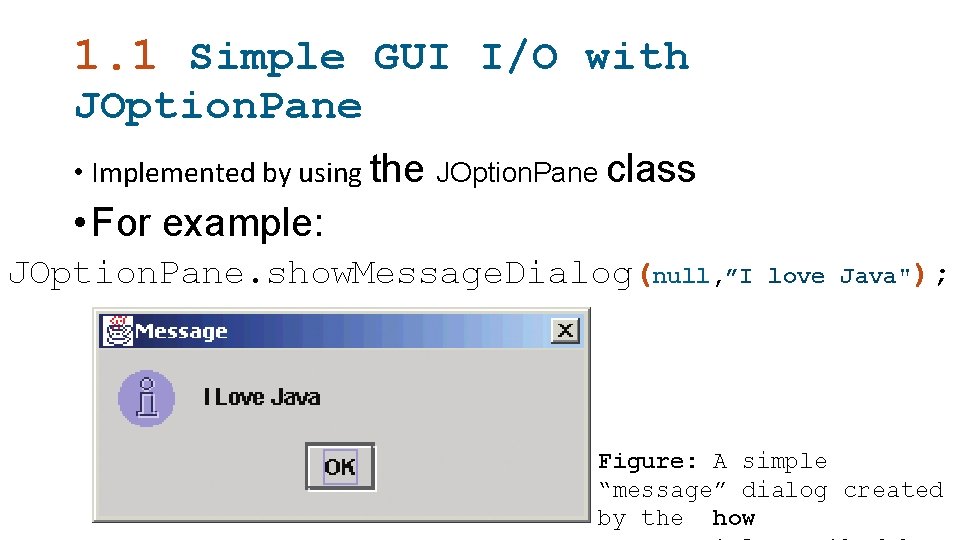
1. 1 Simple GUI I/O with JOption. Pane • Implemented by using the JOption. Pane class • For example: JOption. Pane. show. Message. Dialog(null, ”I love Java"); Figure: A simple “message” dialog created by the how
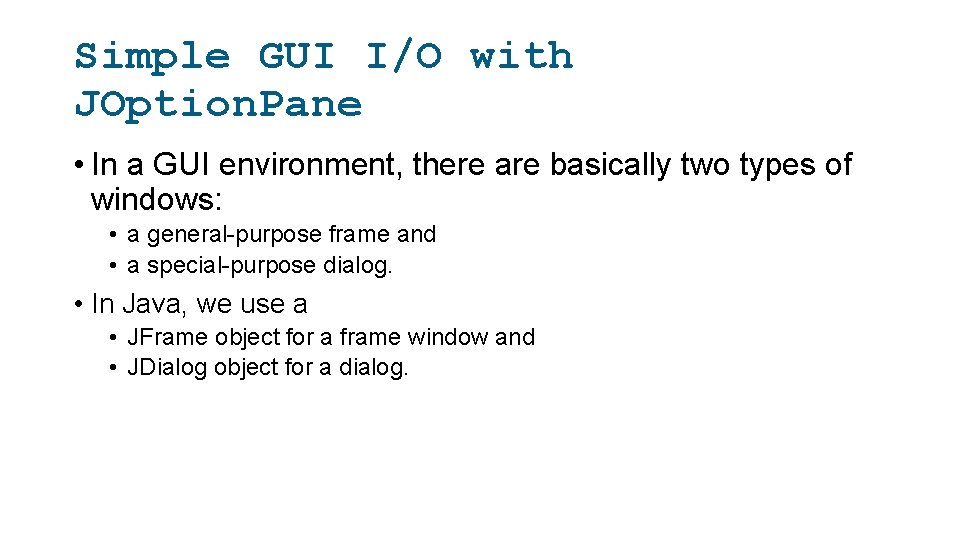
Simple GUI I/O with JOption. Pane • In a GUI environment, there are basically two types of windows: • a general-purpose frame and • a special-purpose dialog. • In Java, we use a • JFrame object for a frame window and • JDialog object for a dialog.
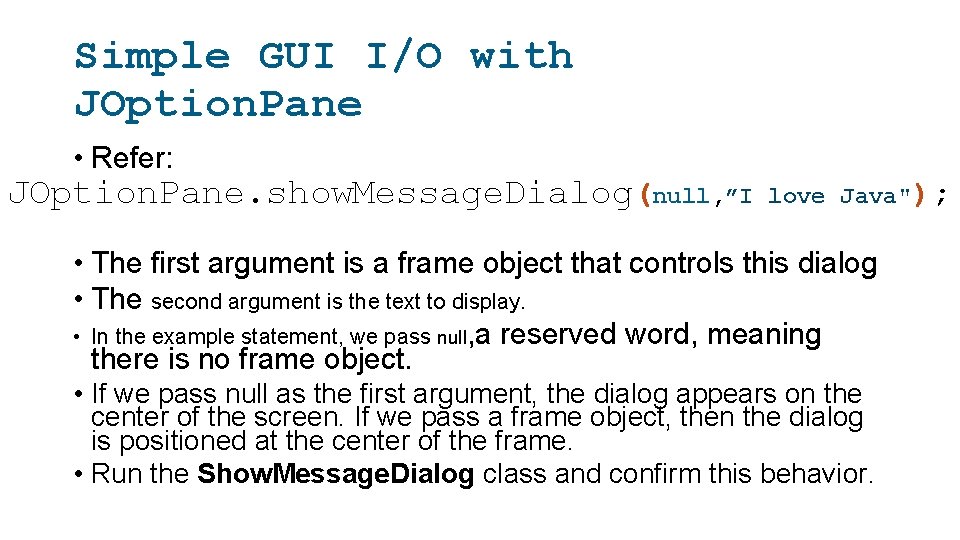
Simple GUI I/O with JOption. Pane • Refer: JOption. Pane. show. Message. Dialog(null, ”I love Java"); • The first argument is a frame object that controls this dialog • The second argument is the text to display. • In the example statement, we pass null, a reserved word, meaning there is no frame object. • If we pass null as the first argument, the dialog appears on the center of the screen. If we pass a frame object, then the dialog is positioned at the center of the frame. • Run the Show. Message. Dialog class and confirm this behavior.
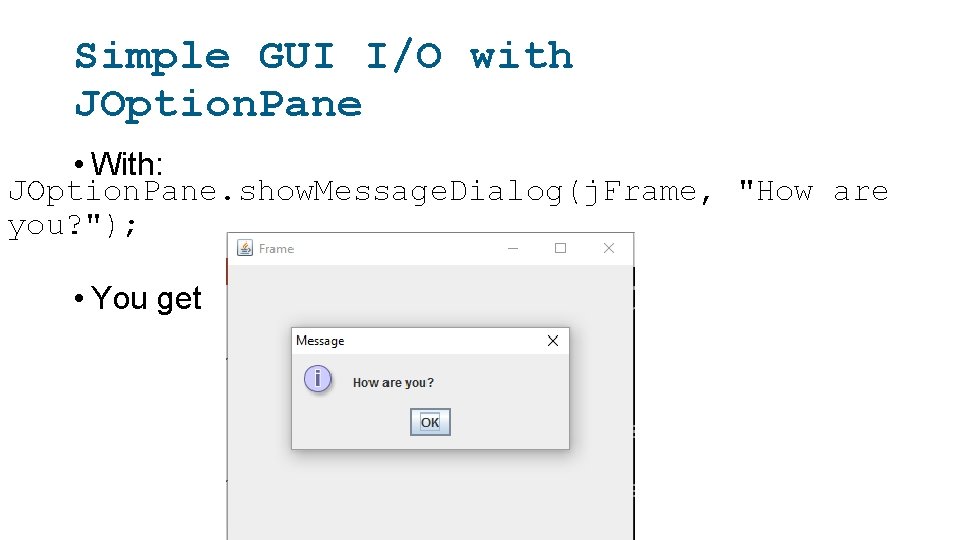
Simple GUI I/O with JOption. Pane • With: JOption. Pane. show. Message. Dialog(j. Frame, "How are you? "); • You get
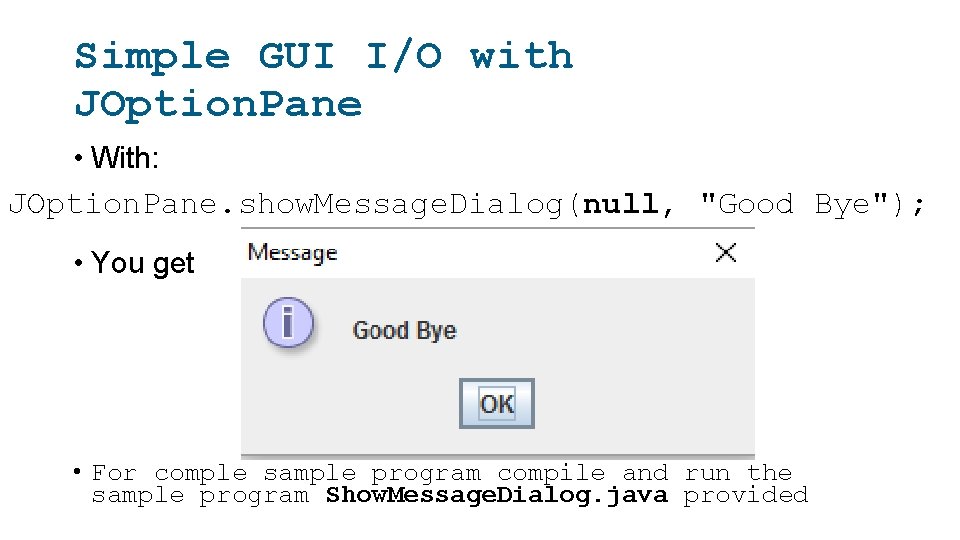
Simple GUI I/O with JOption. Pane • With: JOption. Pane. show. Message. Dialog(null, "Good Bye"); • You get • For comple sample program compile and run the sample program Show. Message. Dialog. java provided
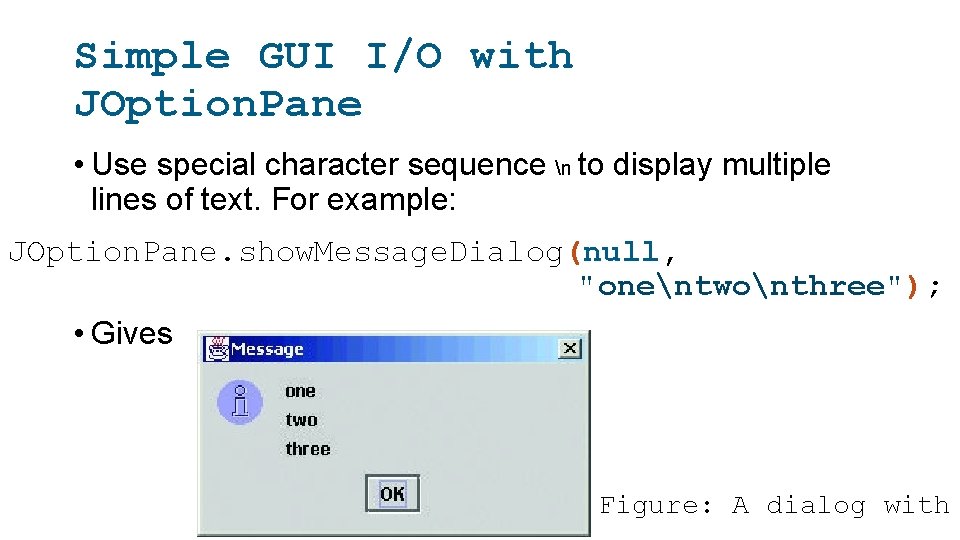
Simple GUI I/O with JOption. Pane • Use special character sequence n to display multiple lines of text. For example: JOption. Pane. show. Message. Dialog(null, "onentwonthree"); • Gives Figure: A dialog with
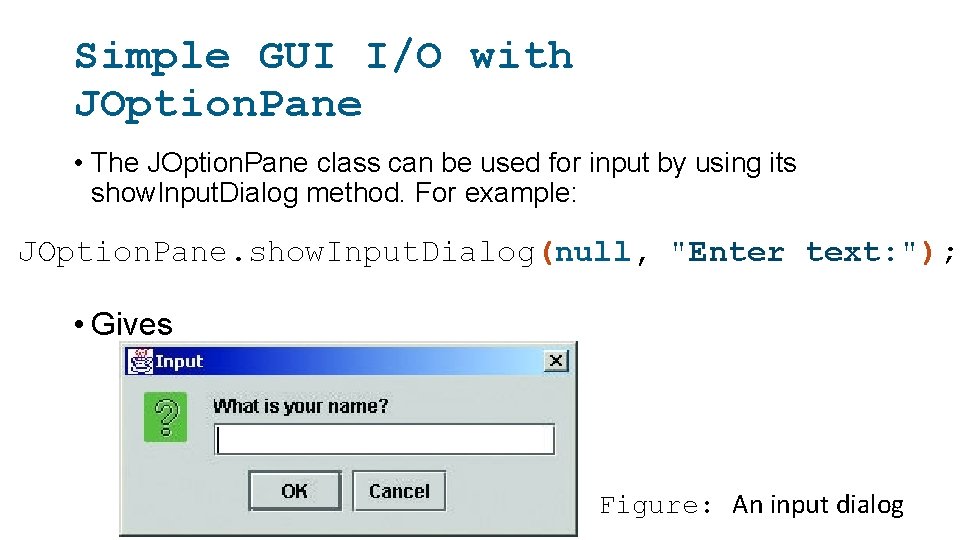
Simple GUI I/O with JOption. Pane • The JOption. Pane class can be used for input by using its show. Input. Dialog method. For example: JOption. Pane. show. Input. Dialog(null, "Enter text: "); • Gives Figure: An input dialog
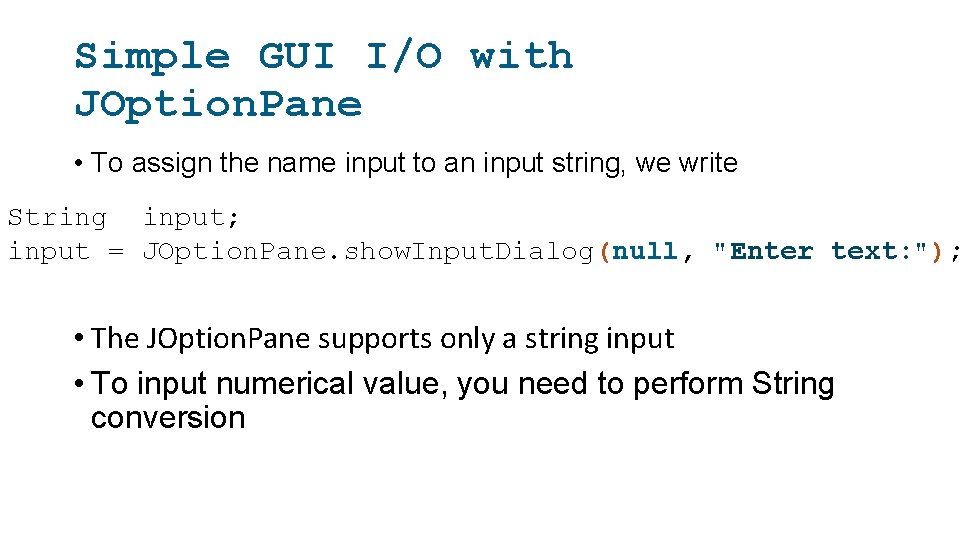
Simple GUI I/O with JOption. Pane • To assign the name input to an input string, we write String input; input = JOption. Pane. show. Input. Dialog(null, "Enter text: "); • The JOption. Pane supports only a string input • To input numerical value, you need to perform String conversion
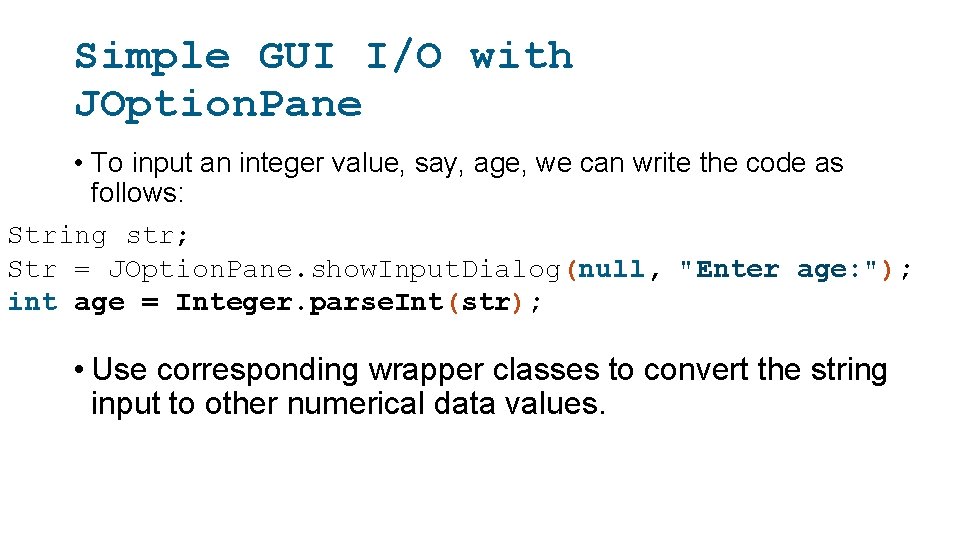
Simple GUI I/O with JOption. Pane • To input an integer value, say, age, we can write the code as follows: String str; Str = JOption. Pane. show. Input. Dialog(null, "Enter age: "); int age = Integer. parse. Int(str); • Use corresponding wrapper classes to convert the string input to other numerical data values.
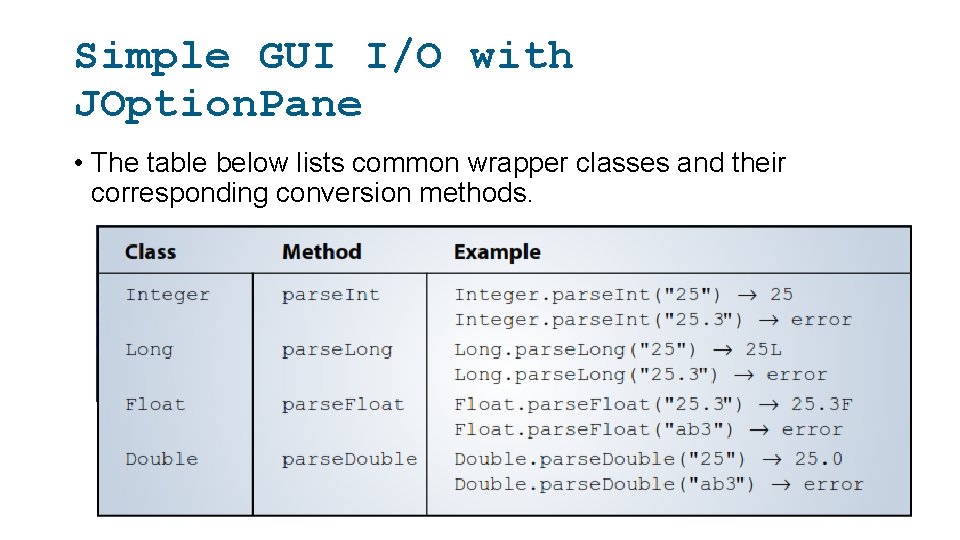
Simple GUI I/O with JOption. Pane • The table below lists common wrapper classes and their corresponding conversion methods.
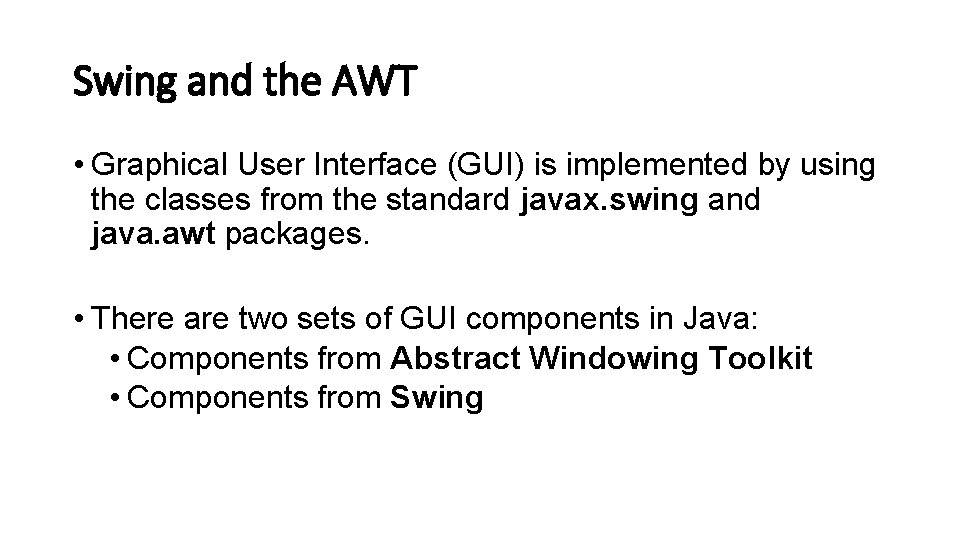
Swing and the AWT • Graphical User Interface (GUI) is implemented by using the classes from the standard javax. swing and java. awt packages. • There are two sets of GUI components in Java: • Components from Abstract Windowing Toolkit • Components from Swing
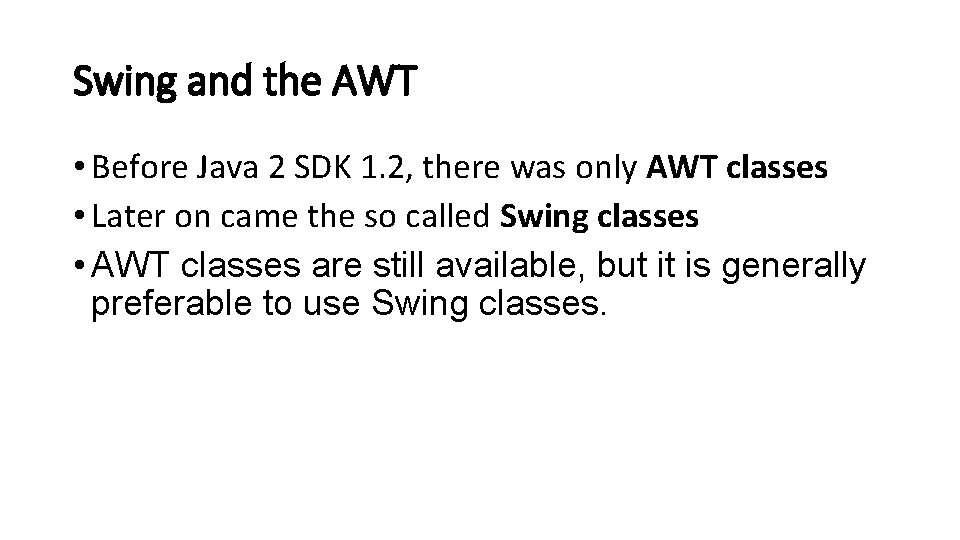
Swing and the AWT • Before Java 2 SDK 1. 2, there was only AWT classes • Later on came the so called Swing classes • AWT classes are still available, but it is generally preferable to use Swing classes.
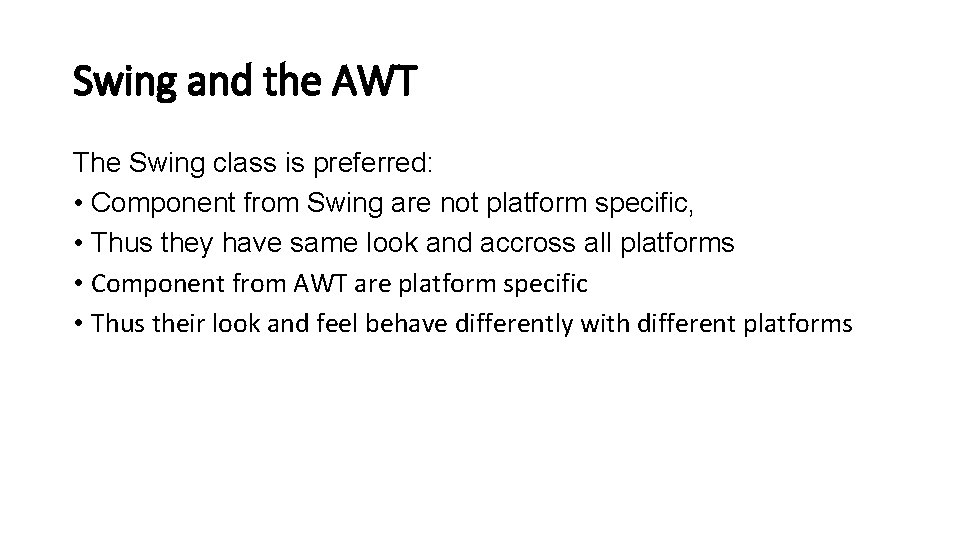
Swing and the AWT The Swing class is preferred: • Component from Swing are not platform specific, • Thus they have same look and accross all platforms • Component from AWT are platform specific • Thus their look and feel behave differently with different platforms
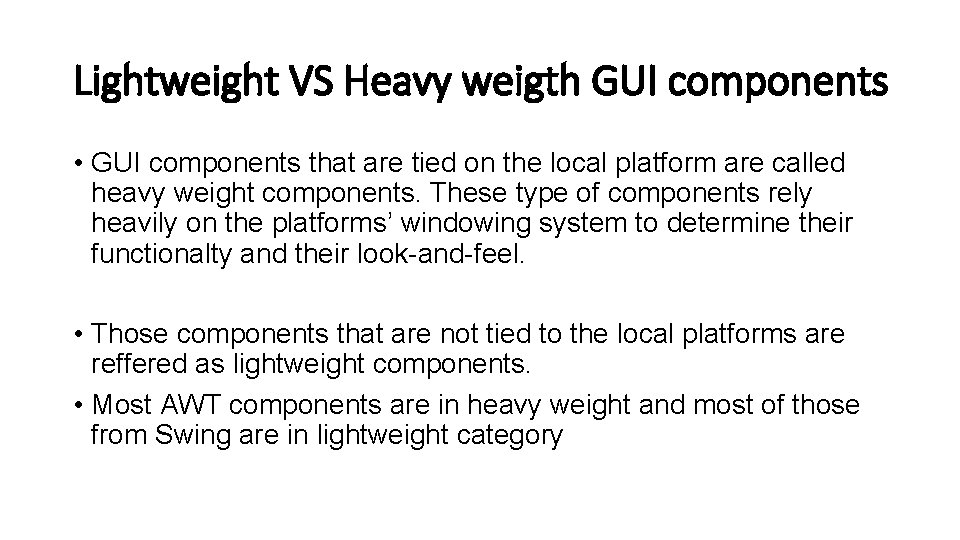
Lightweight VS Heavy weigth GUI components • GUI components that are tied on the local platform are called heavy weight components. These type of components rely heavily on the platforms’ windowing system to determine their functionalty and their look-and-feel. • Those components that are not tied to the local platforms are reffered as lightweight components. • Most AWT components are in heavy weight and most of those from Swing are in lightweight category
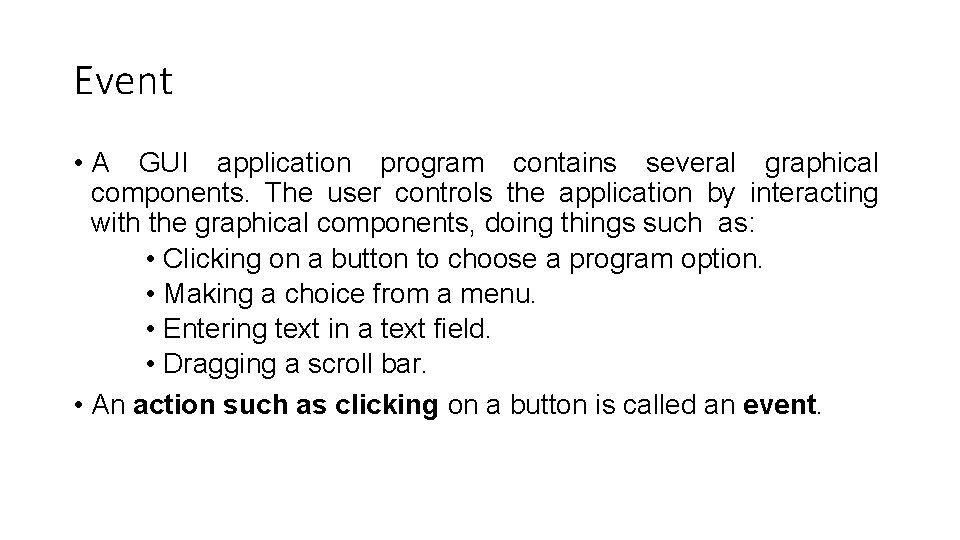
Event • A GUI application program contains several graphical components. The user controls the application by interacting with the graphical components, doing things such as: • Clicking on a button to choose a program option. • Making a choice from a menu. • Entering text in a text field. • Dragging a scroll bar. • An action such as clicking on a button is called an event.
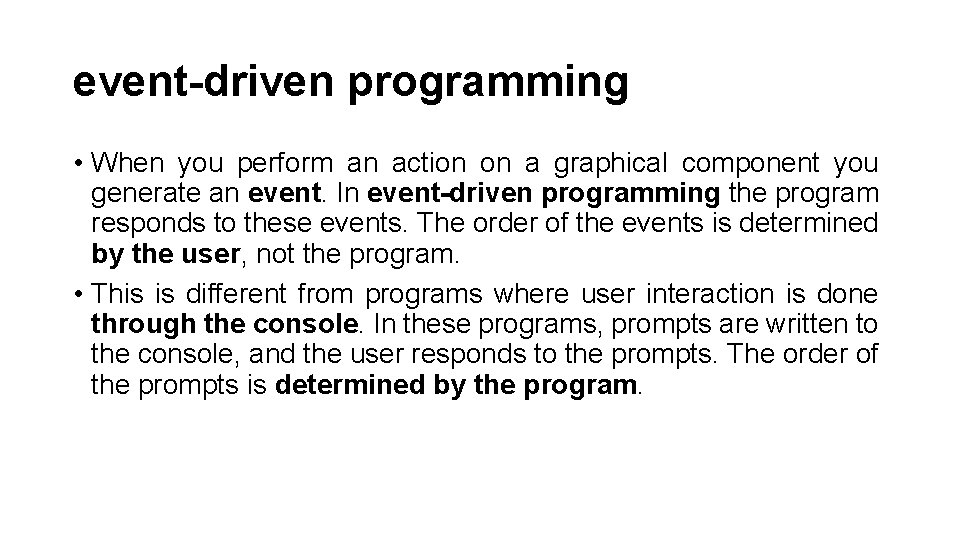
event-driven programming • When you perform an action on a graphical component you generate an event. In event-driven programming the program responds to these events. The order of the events is determined by the user, not the program. • This is different from programs where user interaction is done through the console. In these programs, prompts are written to the console, and the user responds to the prompts. The order of the prompts is determined by the program.
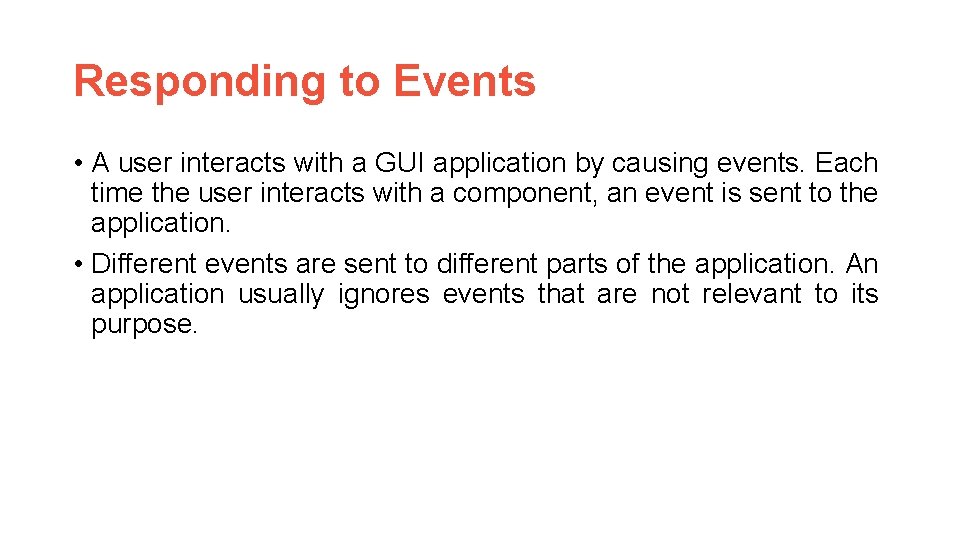
Responding to Events • A user interacts with a GUI application by causing events. Each time the user interacts with a component, an event is sent to the application. • Different events are sent to different parts of the application. An application usually ignores events that are not relevant to its purpose.
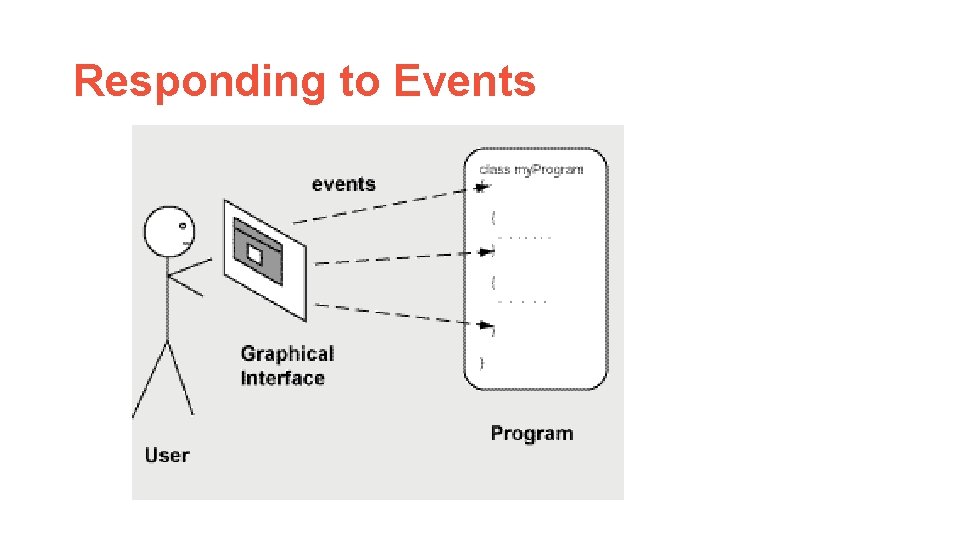
Responding to Events
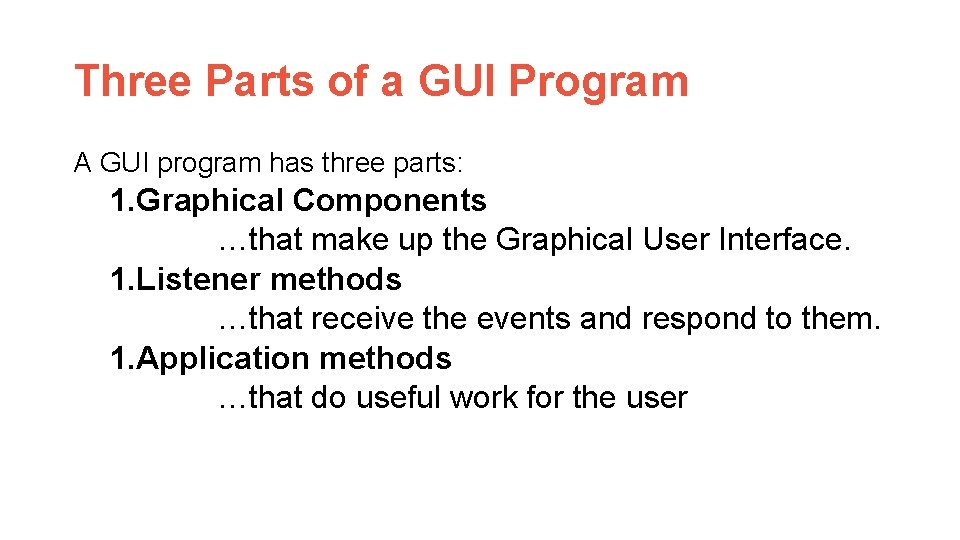
Three Parts of a GUI Program A GUI program has three parts: 1. Graphical Components …that make up the Graphical User Interface. 1. Listener methods …that receive the events and respond to them. 1. Application methods …that do useful work for the user
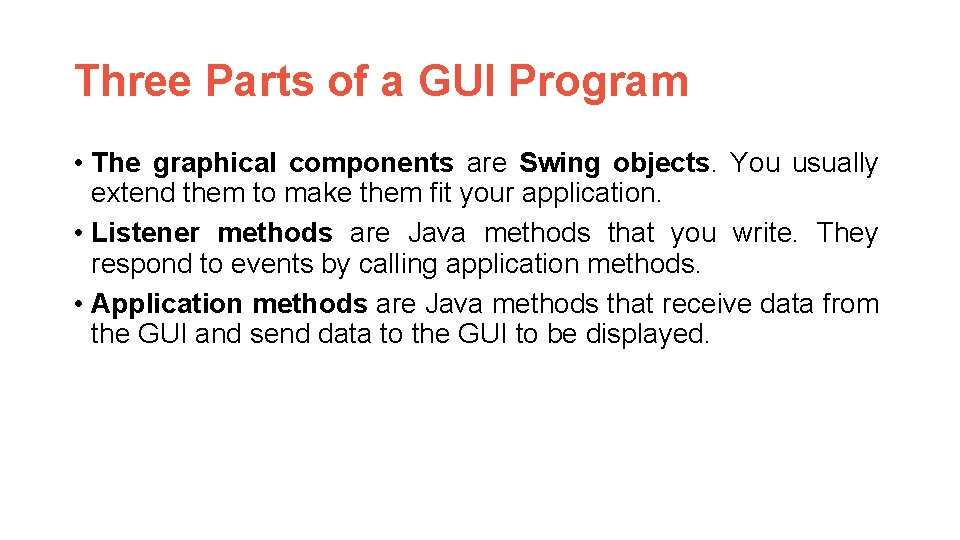
Three Parts of a GUI Program • The graphical components are Swing objects. You usually extend them to make them fit your application. • Listener methods are Java methods that you write. They respond to events by calling application methods. • Application methods are Java methods that receive data from the GUI and send data to the GUI to be displayed.
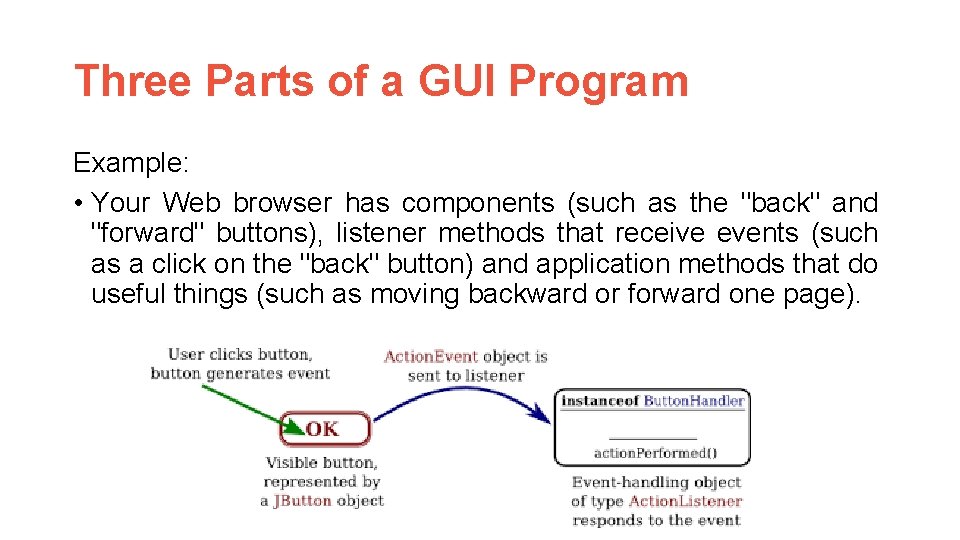
Three Parts of a GUI Program Example: • Your Web browser has components (such as the "back" and "forward" buttons), listener methods that receive events (such as a click on the "back" button) and application methods that do useful things (such as moving backward or forward one page).
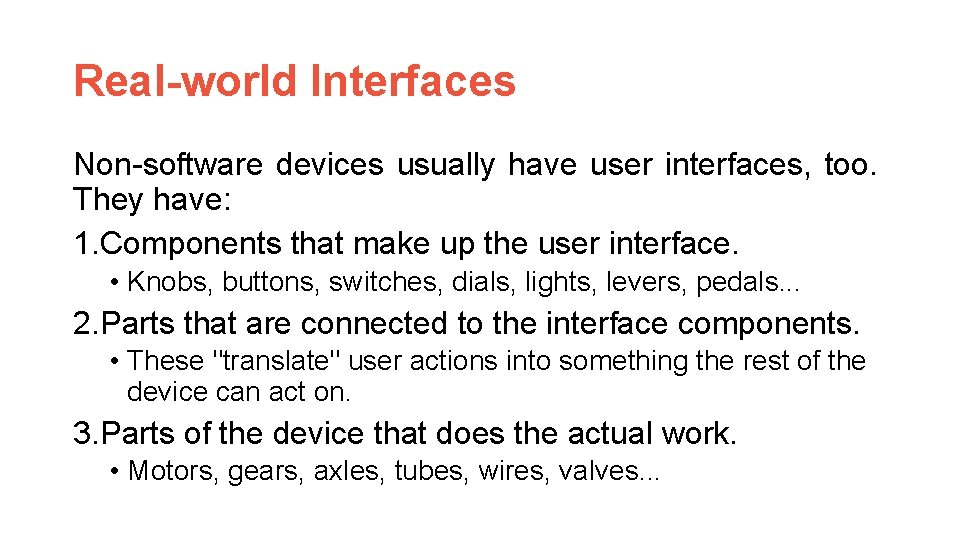
Real-world Interfaces Non-software devices usually have user interfaces, too. They have: 1. Components that make up the user interface. • Knobs, buttons, switches, dials, lights, levers, pedals. . . 2. Parts that are connected to the interface components. • These "translate" user actions into something the rest of the device can act on. 3. Parts of the device that does the actual work. • Motors, gears, axles, tubes, wires, valves. . .
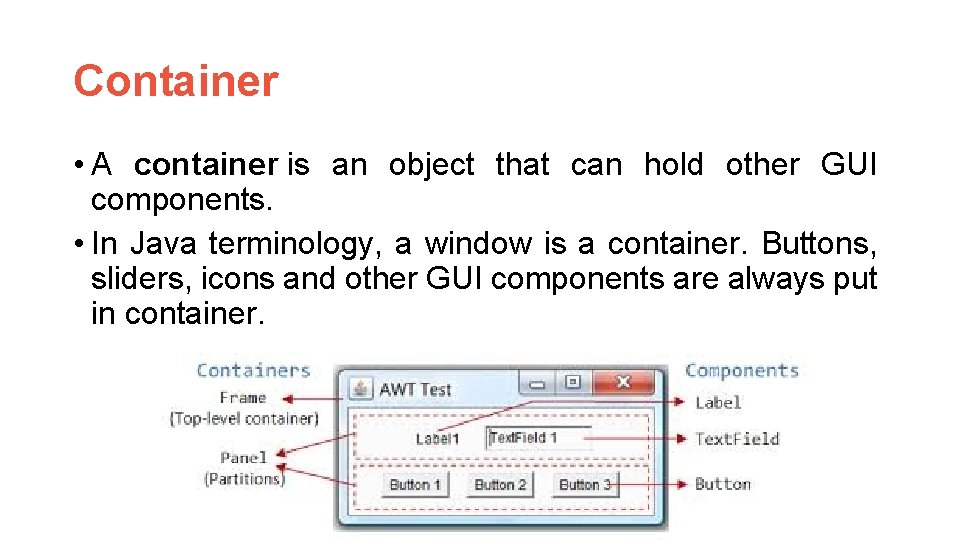
Container • A container is an object that can hold other GUI components. • In Java terminology, a window is a container. Buttons, sliders, icons and other GUI components are always put in container.
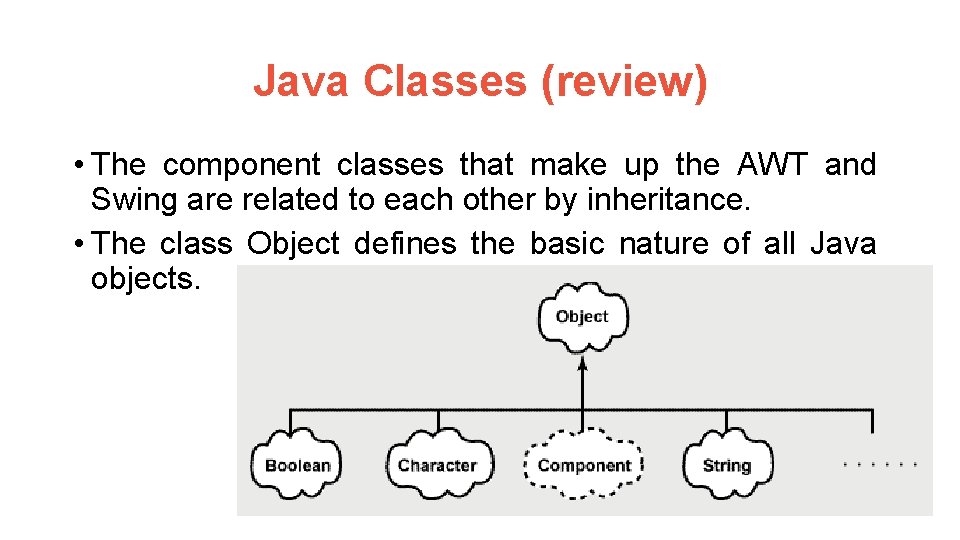
Java Classes (review) • The component classes that make up the AWT and Swing are related to each other by inheritance. • The class Object defines the basic nature of all Java objects.
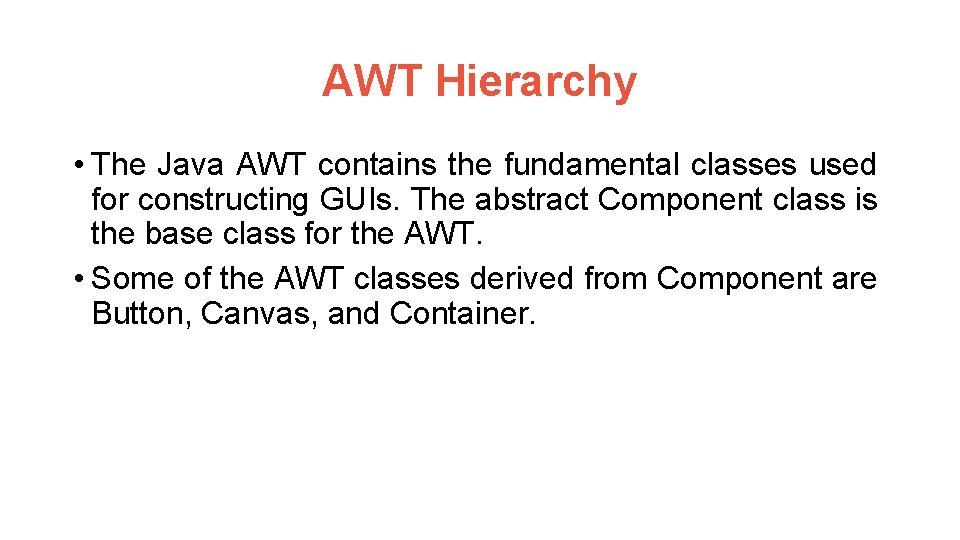
AWT Hierarchy • The Java AWT contains the fundamental classes used for constructing GUIs. The abstract Component class is the base class for the AWT. • Some of the AWT classes derived from Component are Button, Canvas, and Container.
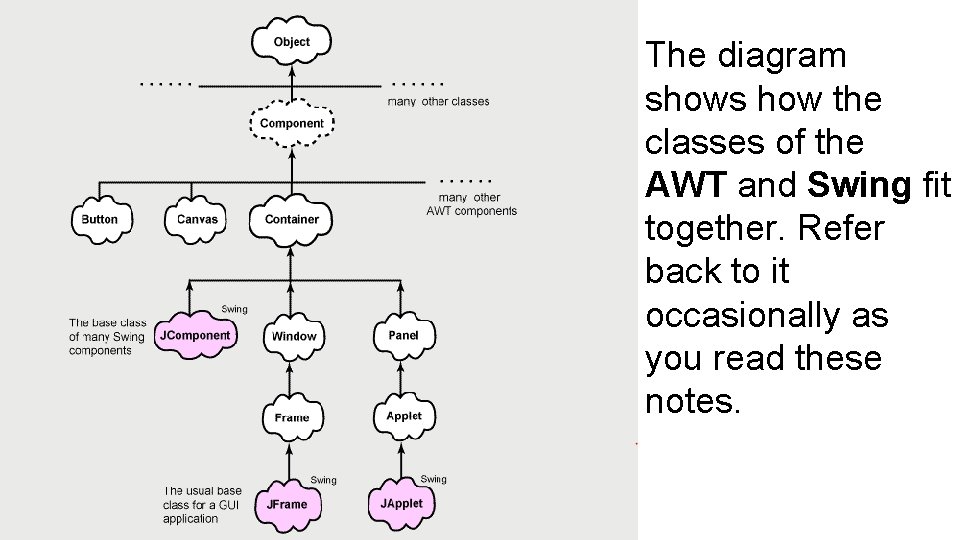
AWT The diagram Hierarchy shows how the classes of the AWT and Swing fit together. Refer back to it occasionally as you read these notes.
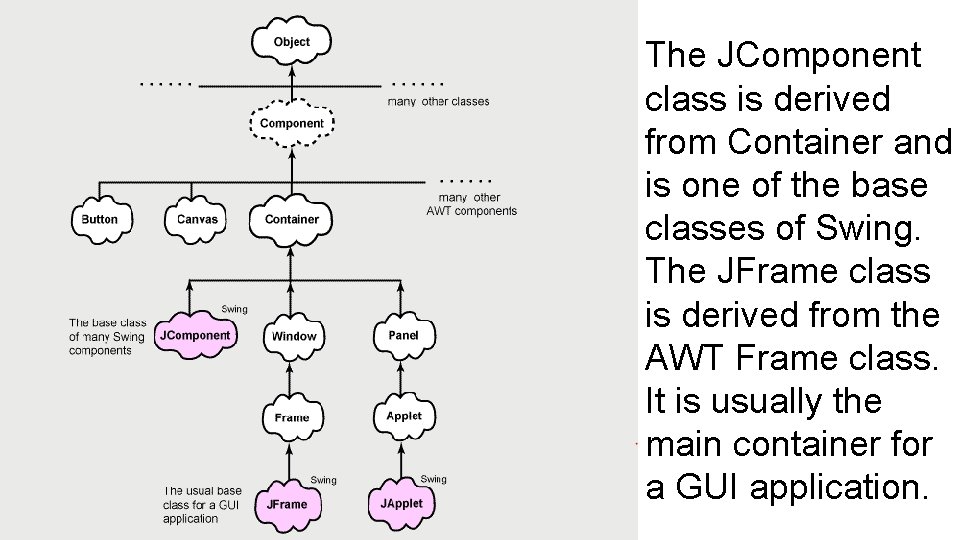
AWT The JComponent Hierarchy class is derived from Container and is one of the base classes of Swing. The JFrame class is derived from the AWT Frame class. It is usually the main container for a GUI application.
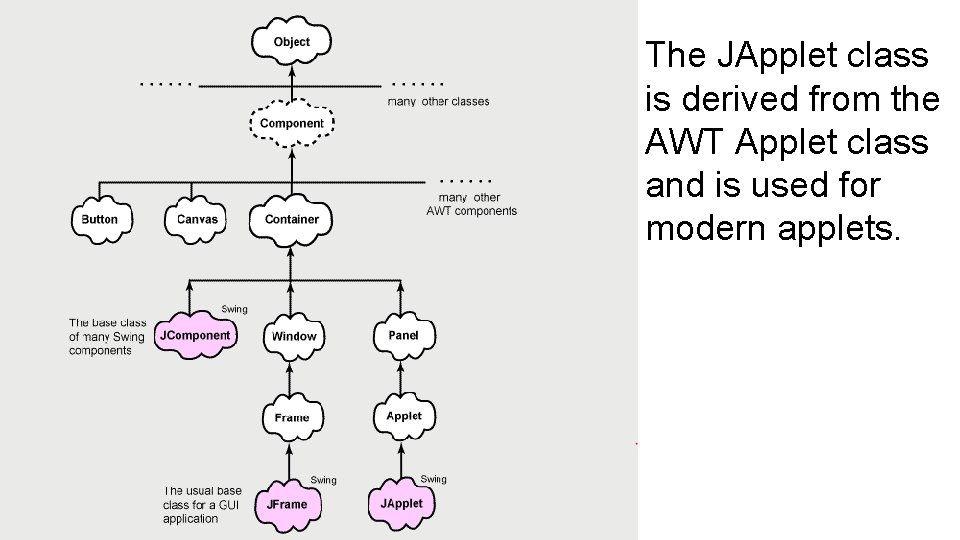
AWT The JApplet class Hierarchy is derived from the AWT Applet class and is used for modern applets.
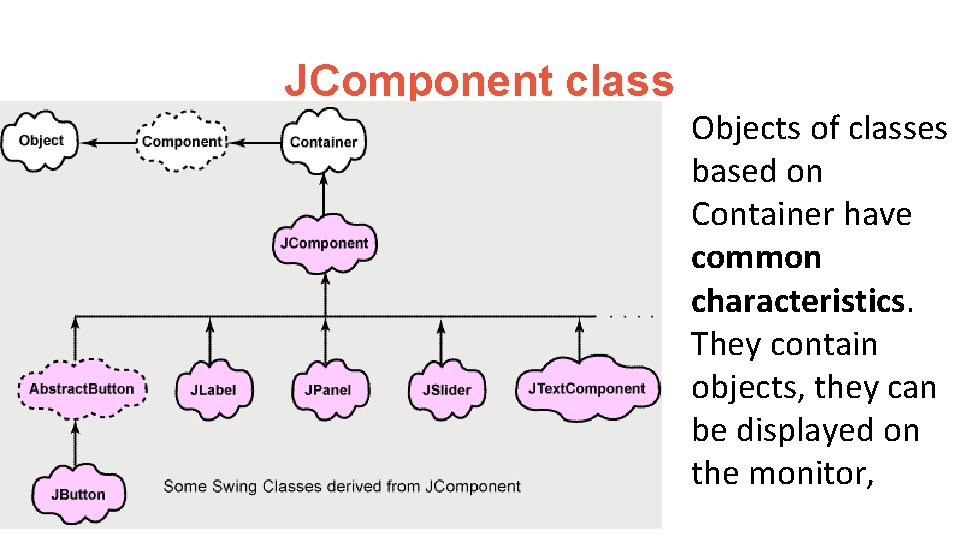
JComponent class Objects of classes based on Container have common characteristics. They contain objects, they can be displayed on the monitor,
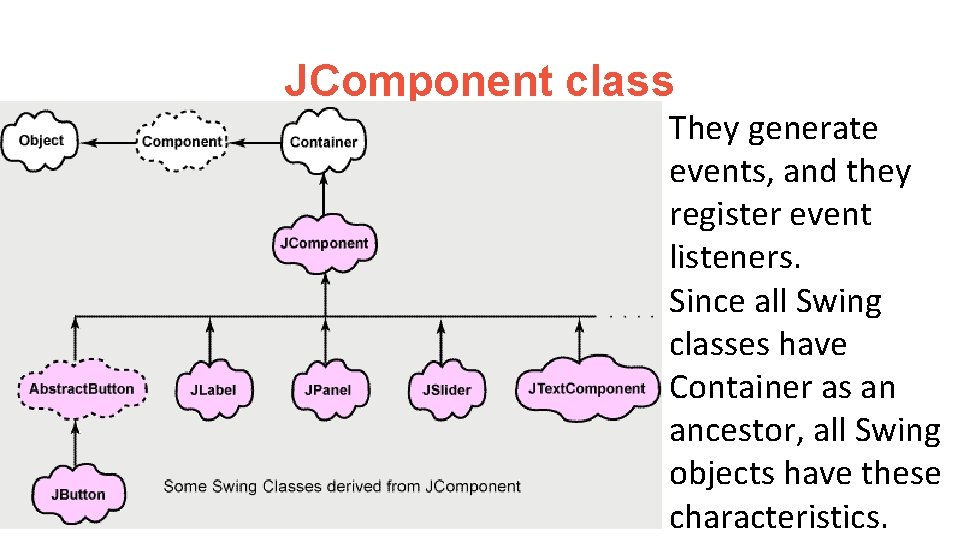
JComponent class They generate events, and they register event listeners. Since all Swing classes have Container as an ancestor, all Swing objects have these characteristics.
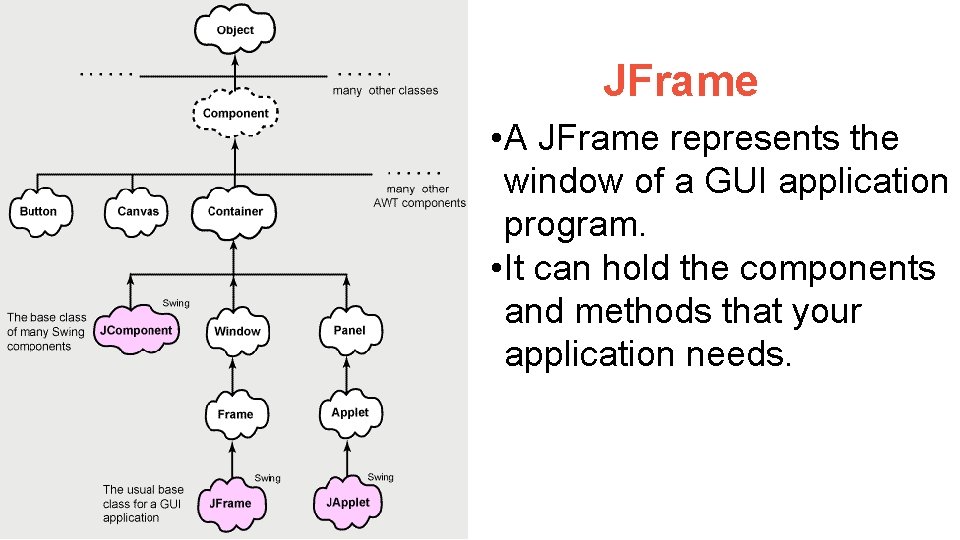
JFrame • A JFrame represents the window of a GUI application program. • It can hold the components and methods that your application needs.
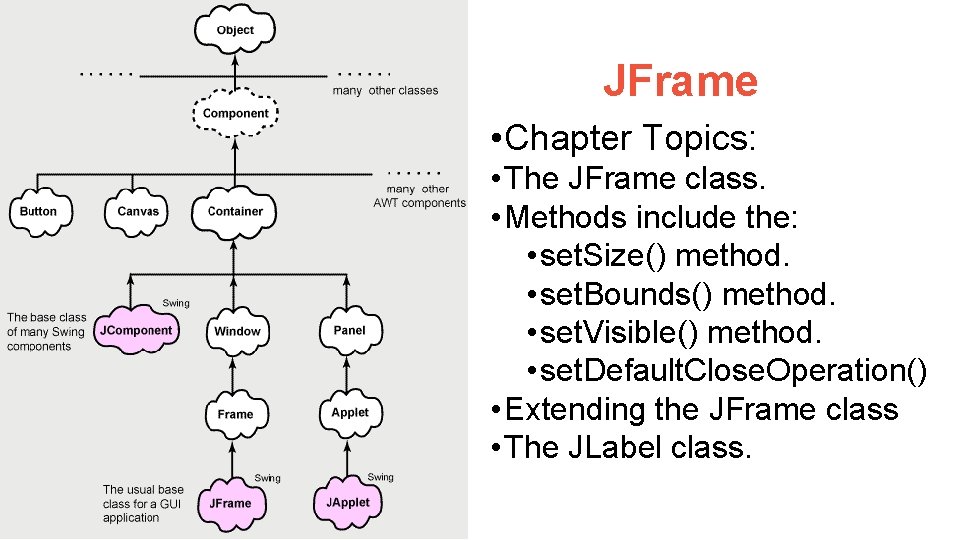
JFrame • Chapter Topics: • The JFrame class. • Methods include the: • set. Size() method. • set. Bounds() method. • set. Visible() method. • set. Default. Close. Operation() • Extending the JFrame class • The JLabel class.
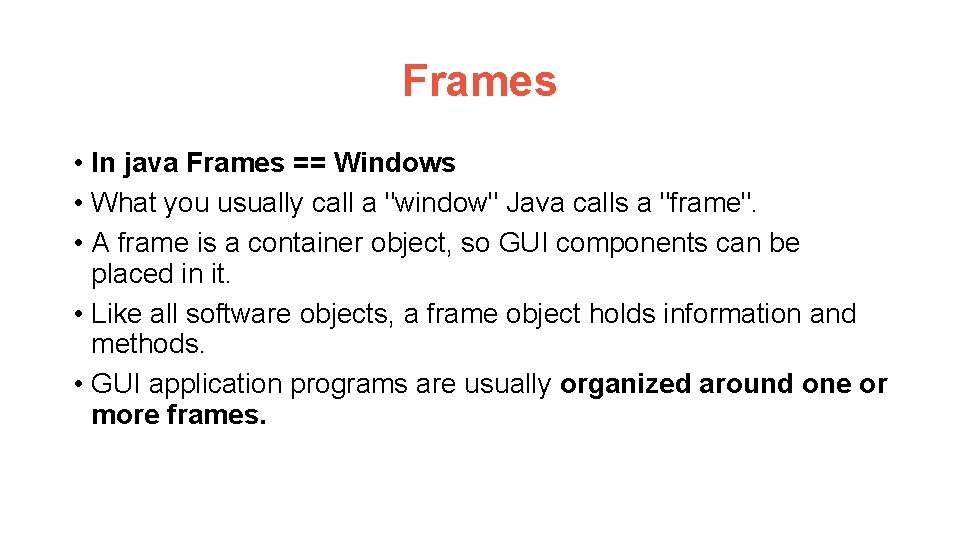
Frames • In java Frames == Windows • What you usually call a "window" Java calls a "frame". • A frame is a container object, so GUI components can be placed in it. • Like all software objects, a frame object holds information and methods. • GUI application programs are usually organized around one or more frames.
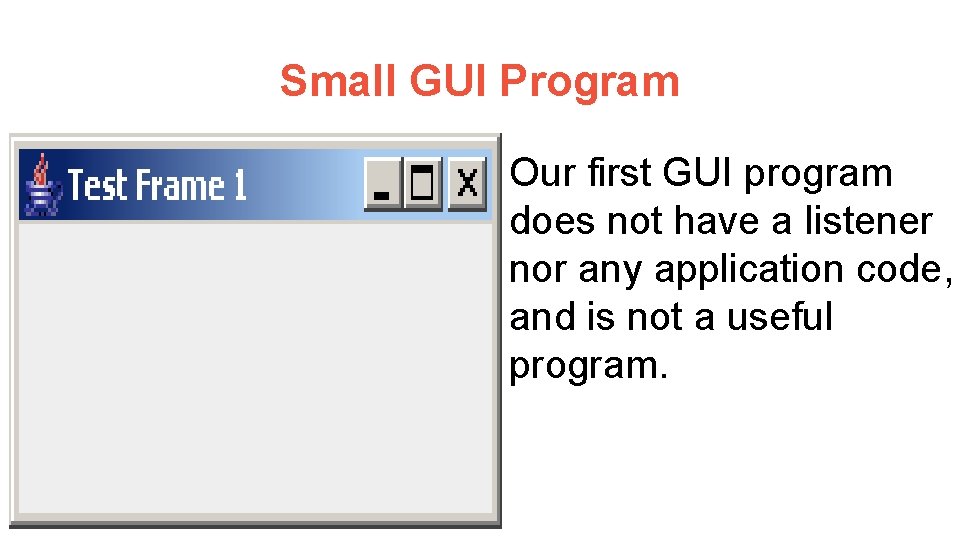
Small GUI Program Our first GUI program does not have a listener nor any application code, and is not a useful program.
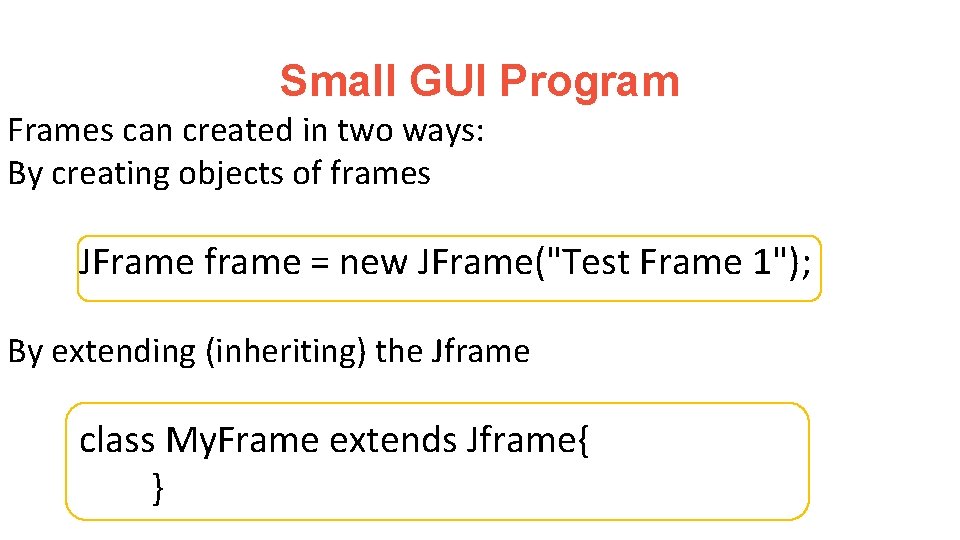
Small GUI Program Frames can created in two ways: By creating objects of frames JFrame frame = new JFrame("Test Frame 1"); By extending (inheriting) the Jframe class My. Frame extends Jframe{ }
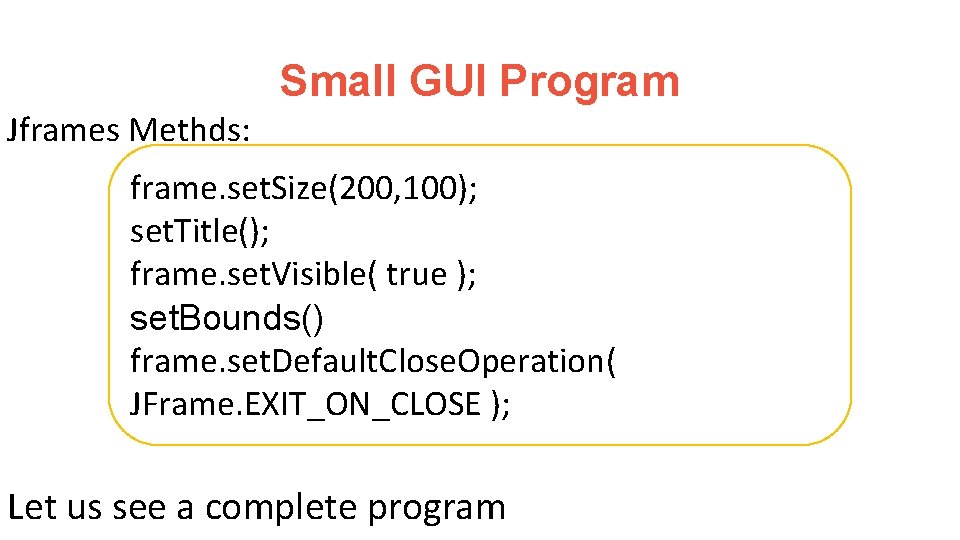
Small GUI Program Jframes Methds: frame. set. Size(200, 100); set. Title(); frame. set. Visible( true ); set. Bounds() frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); Let us see a complete program
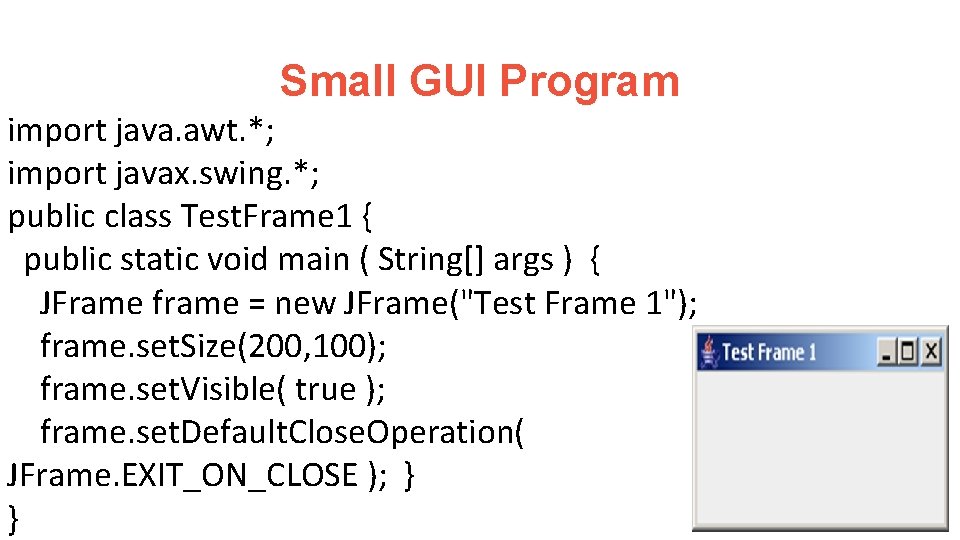
Small GUI Program import java. awt. *; import javax. swing. *; public class Test. Frame 1 { public static void main ( String[] args ) { JFrame frame = new JFrame("Test Frame 1"); frame. set. Size(200, 100); frame. set. Visible( true ); frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } }
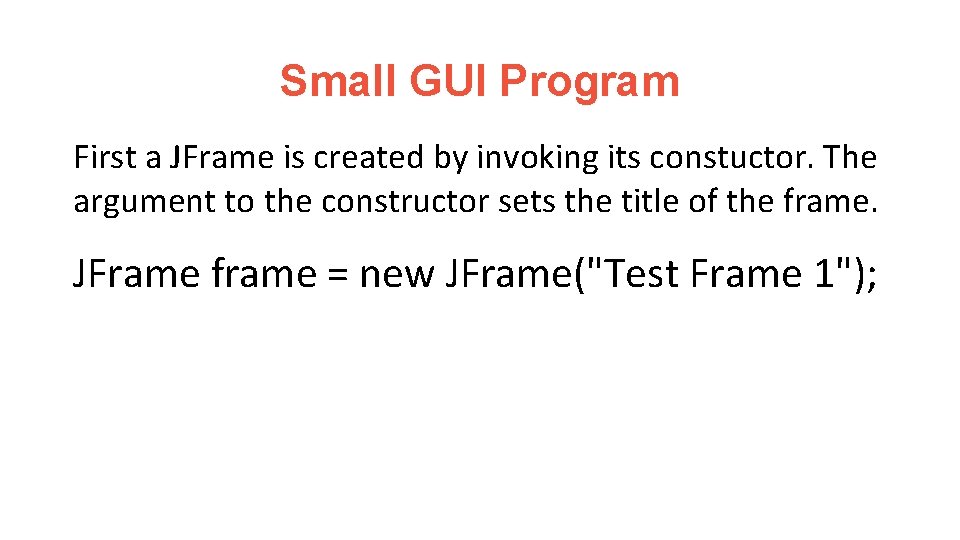
Small GUI Program First a JFrame is created by invoking its constuctor. The argument to the constructor sets the title of the frame. JFrame frame = new JFrame("Test Frame 1");
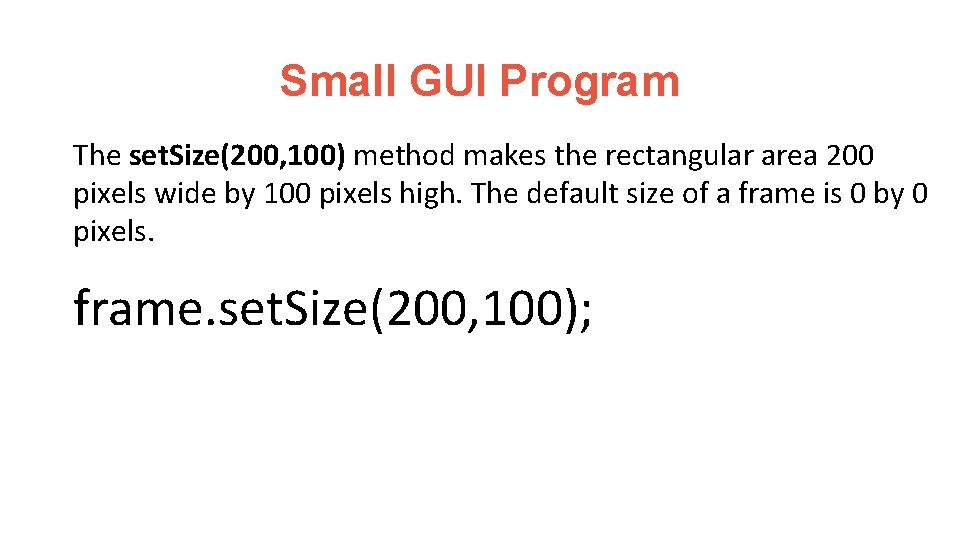
Small GUI Program The set. Size(200, 100) method makes the rectangular area 200 pixels wide by 100 pixels high. The default size of a frame is 0 by 0 pixels. frame. set. Size(200, 100);
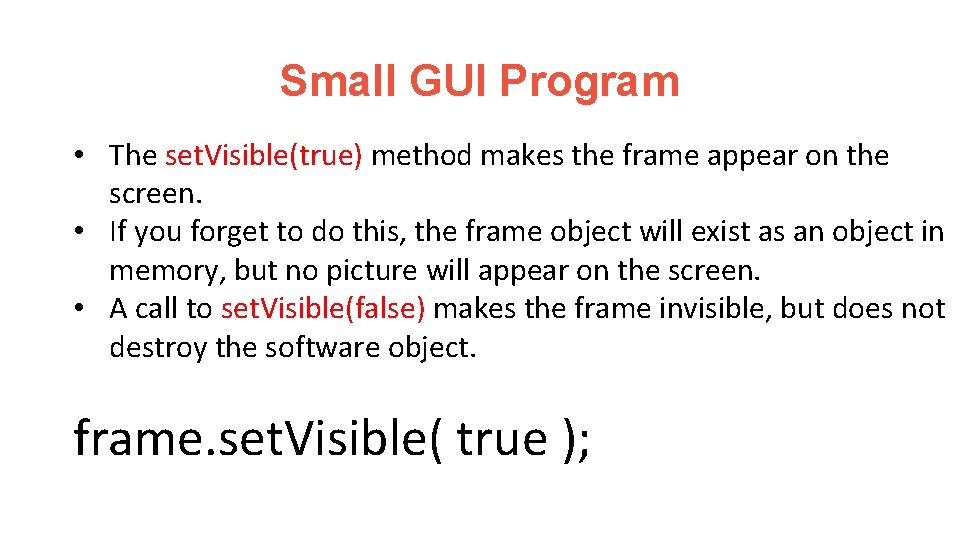
Small GUI Program • The set. Visible(true) method makes the frame appear on the screen. • If you forget to do this, the frame object will exist as an object in memory, but no picture will appear on the screen. • A call to set. Visible(false) makes the frame invisible, but does not destroy the software object. frame. set. Visible( true );
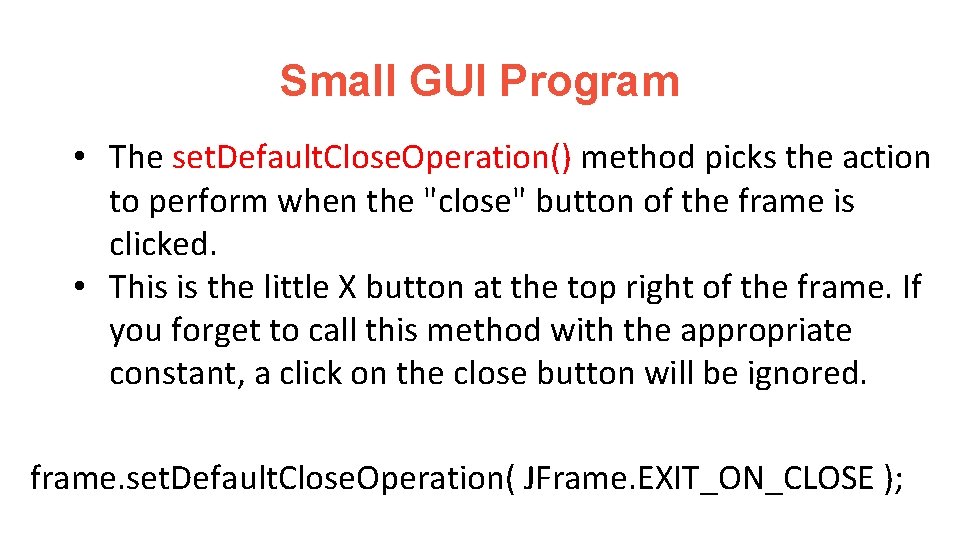
Small GUI Program • The set. Default. Close. Operation() method picks the action to perform when the "close" button of the frame is clicked. • This is the little X button at the top right of the frame. If you forget to call this method with the appropriate constant, a click on the close button will be ignored. frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE );
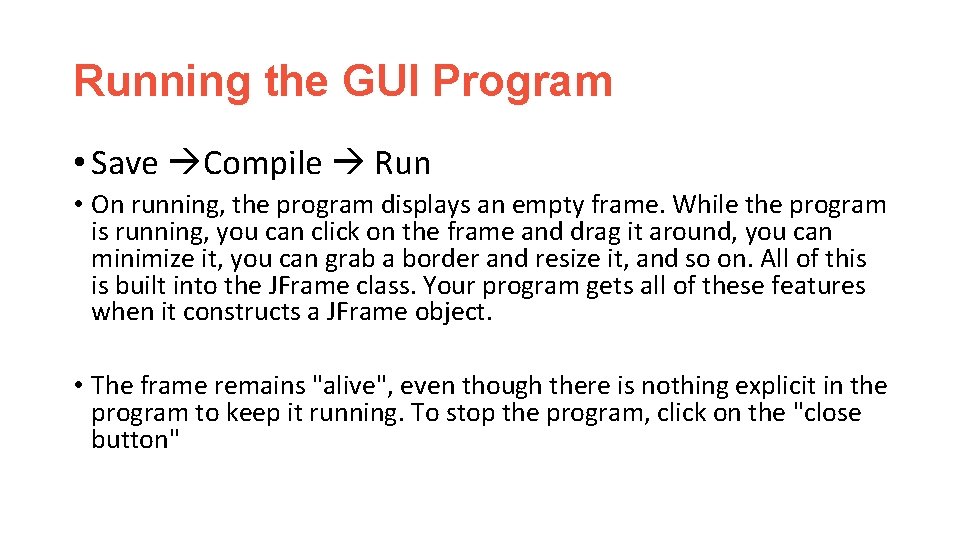
Running the GUI Program • Save Compile Run • On running, the program displays an empty frame. While the program is running, you can click on the frame and drag it around, you can minimize it, you can grab a border and resize it, and so on. All of this is built into the JFrame class. Your program gets all of these features when it constructs a JFrame object. • The frame remains "alive", even though there is nothing explicit in the program to keep it running. To stop the program, click on the "close button"
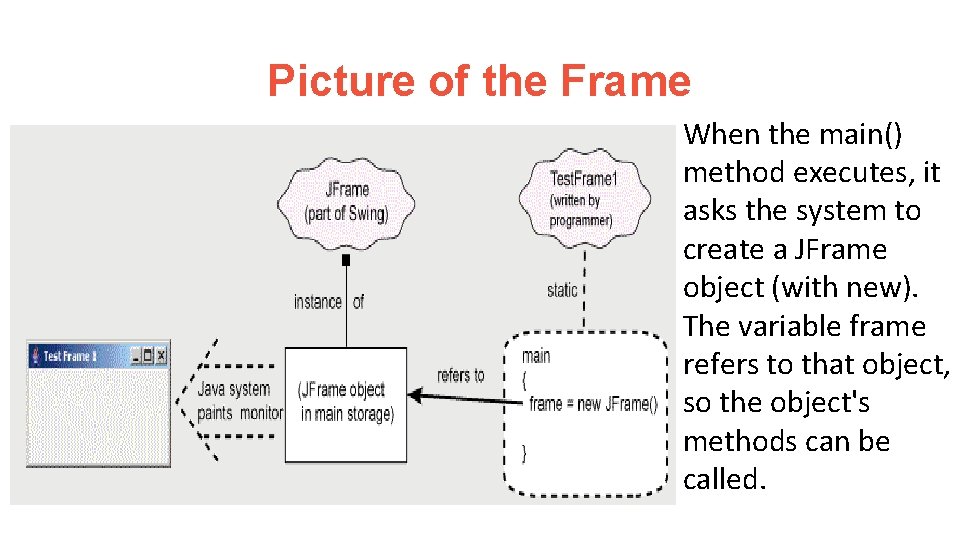
Picture of the Frame When the main() method executes, it asks the system to create a JFrame object (with new). The variable frame refers to that object, so the object's methods can be called.
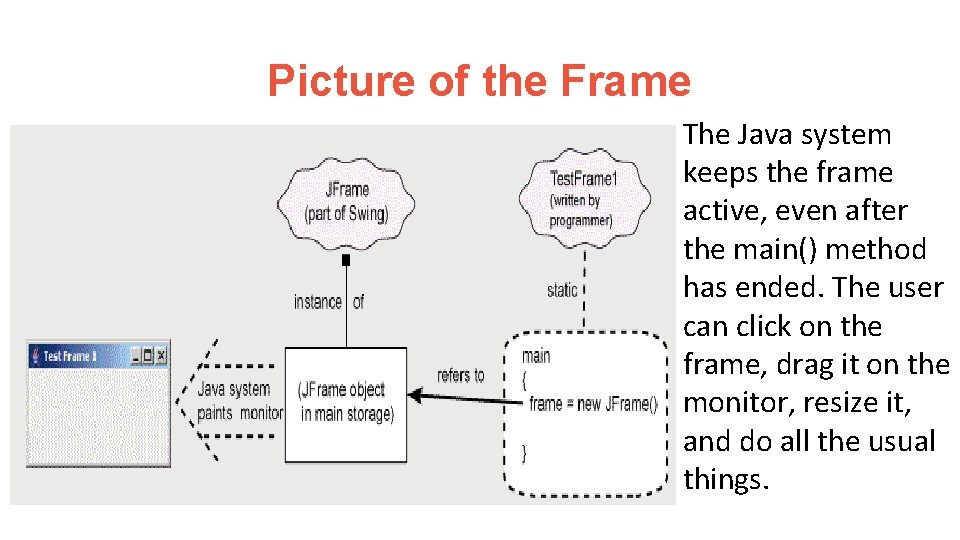
Picture of the Frame The Java system keeps the frame active, even after the main() method has ended. The user can click on the frame, drag it on the monitor, resize it, and do all the usual things.
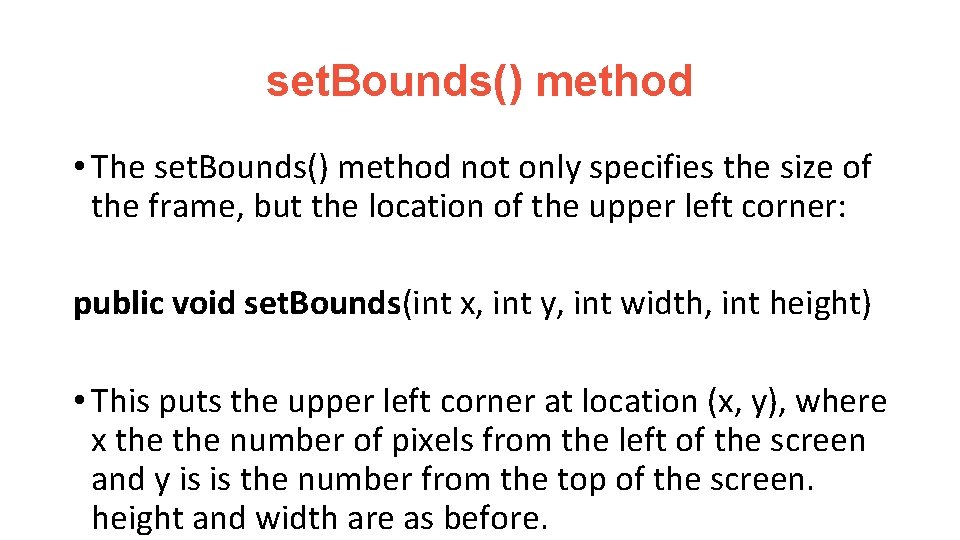
set. Bounds() method • The set. Bounds() method not only specifies the size of the frame, but the location of the upper left corner: public void set. Bounds(int x, int y, int width, int height) • This puts the upper left corner at location (x, y), where x the number of pixels from the left of the screen and y is is the number from the top of the screen. height and width are as before.
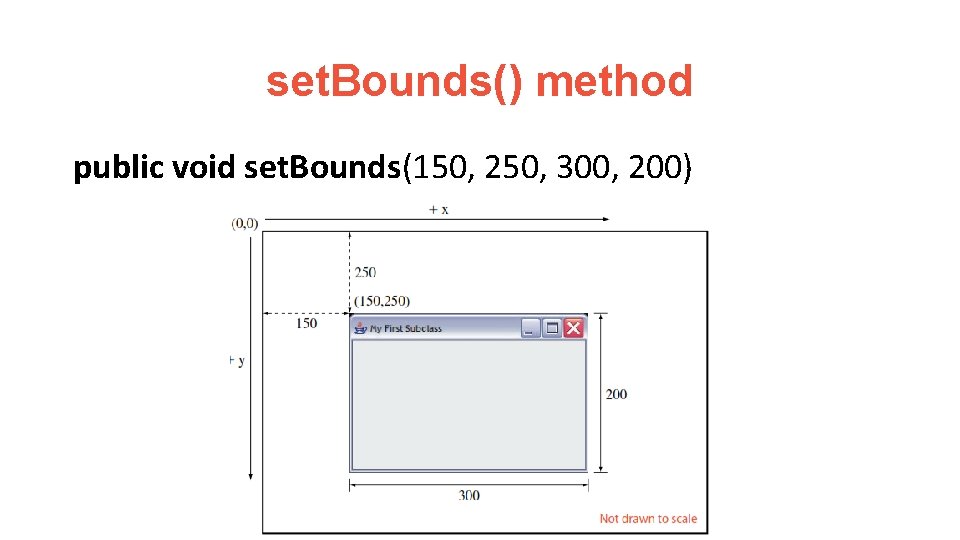
set. Bounds() method public void set. Bounds(150, 250, 300, 200)
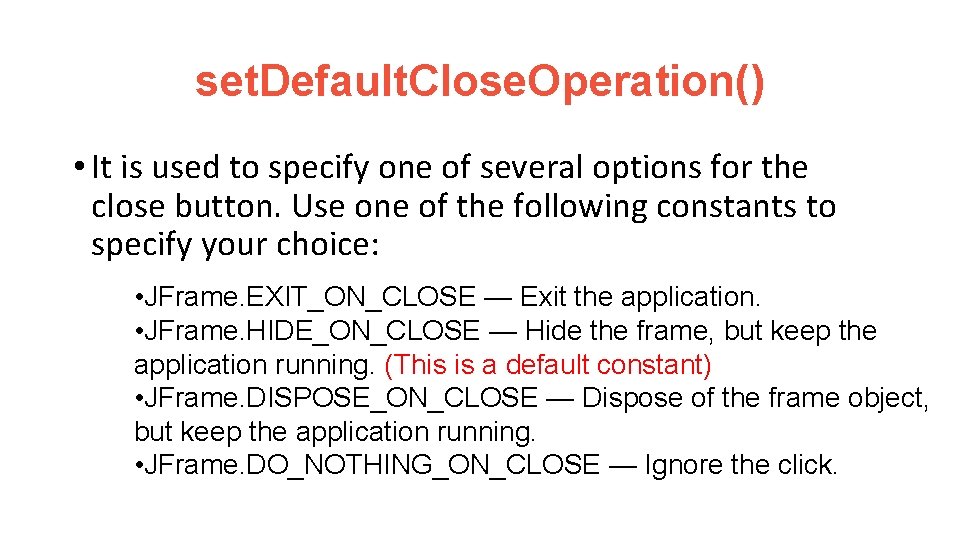
set. Default. Close. Operation() • It is used to specify one of several options for the close button. Use one of the following constants to specify your choice: • JFrame. EXIT_ON_CLOSE — Exit the application. • JFrame. HIDE_ON_CLOSE — Hide the frame, but keep the application running. (This is a default constant) • JFrame. DISPOSE_ON_CLOSE — Dispose of the frame object, but keep the application running. • JFrame. DO_NOTHING_ON_CLOSE — Ignore the click.
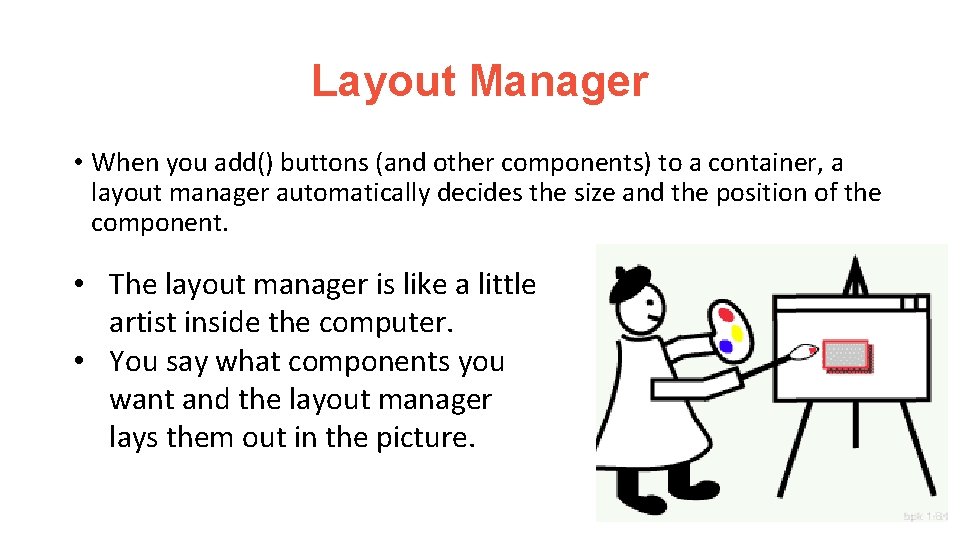
Layout Manager • When you add() buttons (and other components) to a container, a layout manager automatically decides the size and the position of the component. • The layout manager is like a little artist inside the computer. • You say what components you want and the layout manager lays them out in the picture.
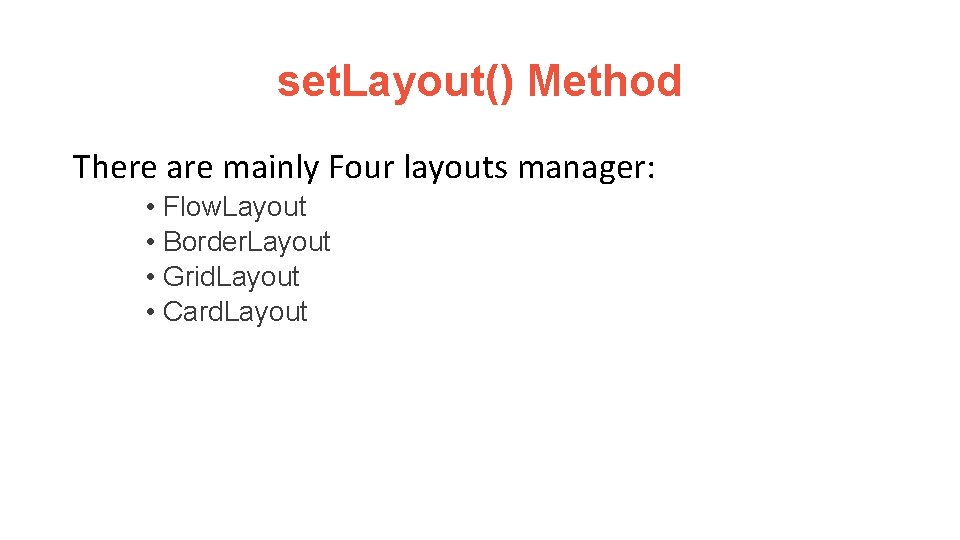
set. Layout() Method There are mainly Four layouts manager: • Flow. Layout • Border. Layout • Grid. Layout • Card. Layout
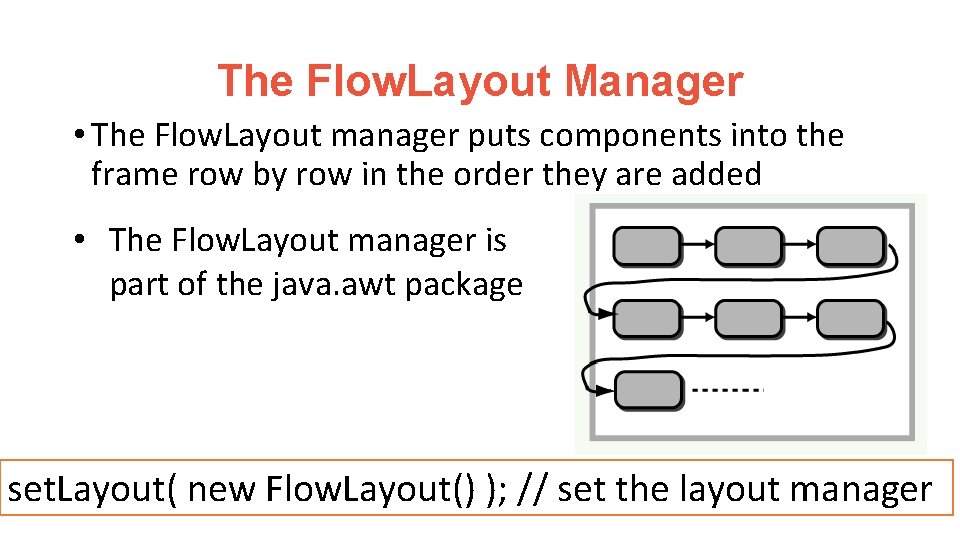
The Flow. Layout Manager • The Flow. Layout manager puts components into the frame row by row in the order they are added • The Flow. Layout manager is part of the java. awt package set. Layout( new Flow. Layout() ); // set the layout manager
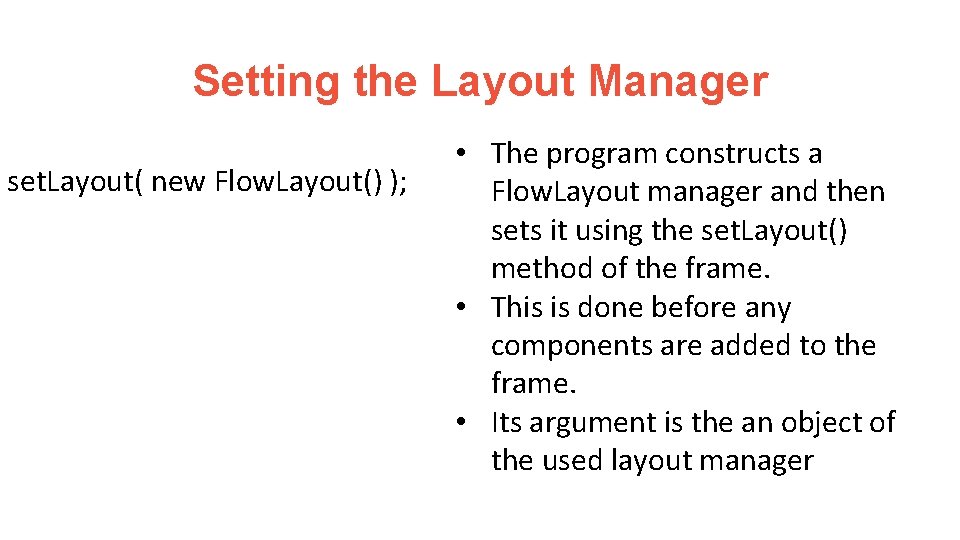
Setting the Layout Manager set. Layout( new Flow. Layout() ); • The program constructs a Flow. Layout manager and then sets it using the set. Layout() method of the frame. • This is done before any components are added to the frame. • Its argument is the an object of the used layout manager
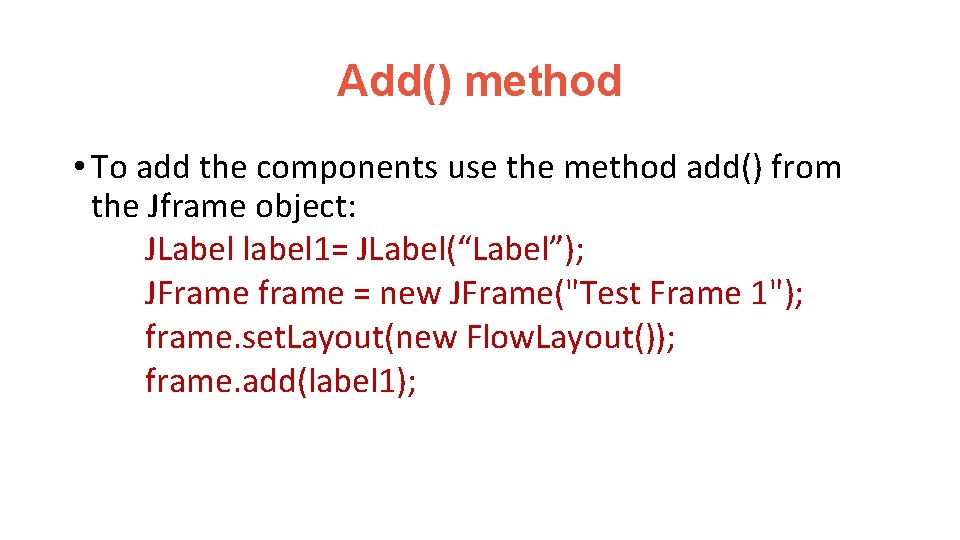
Add() method • To add the components use the method add() from the Jframe object: JLabel label 1= JLabel(“Label”); JFrame frame = new JFrame("Test Frame 1"); frame. set. Layout(new Flow. Layout()); frame. add(label 1);
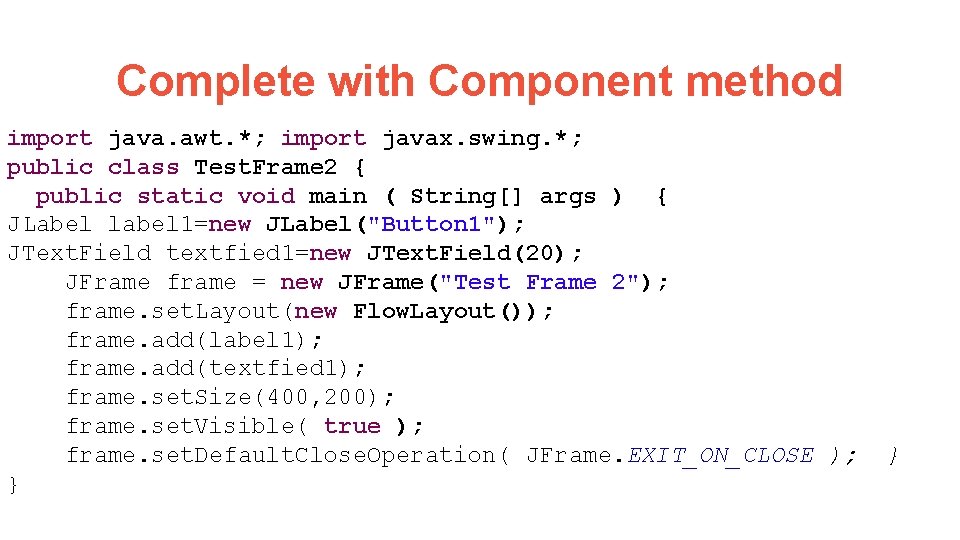
Complete with Component method import java. awt. *; import javax. swing. *; public class Test. Frame 2 { public static void main ( String[] args ) { JLabel label 1=new JLabel("Button 1"); JText. Field textfied 1=new JText. Field(20); JFrame frame = new JFrame("Test Frame 2"); frame. set. Layout(new Flow. Layout()); frame. add(label 1); frame. add(textfied 1); frame. set. Size(400, 200); frame. set. Visible( true ); frame. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } }
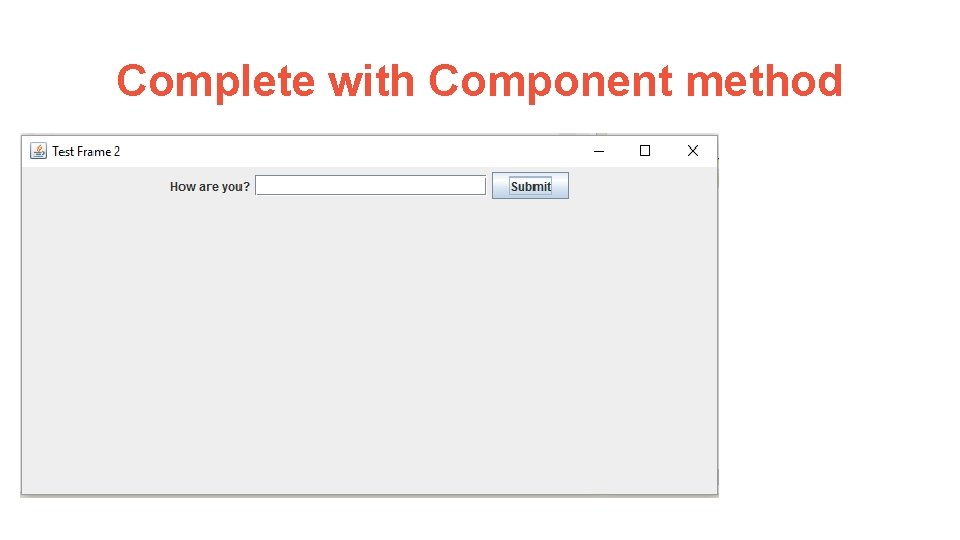
Complete with Component method
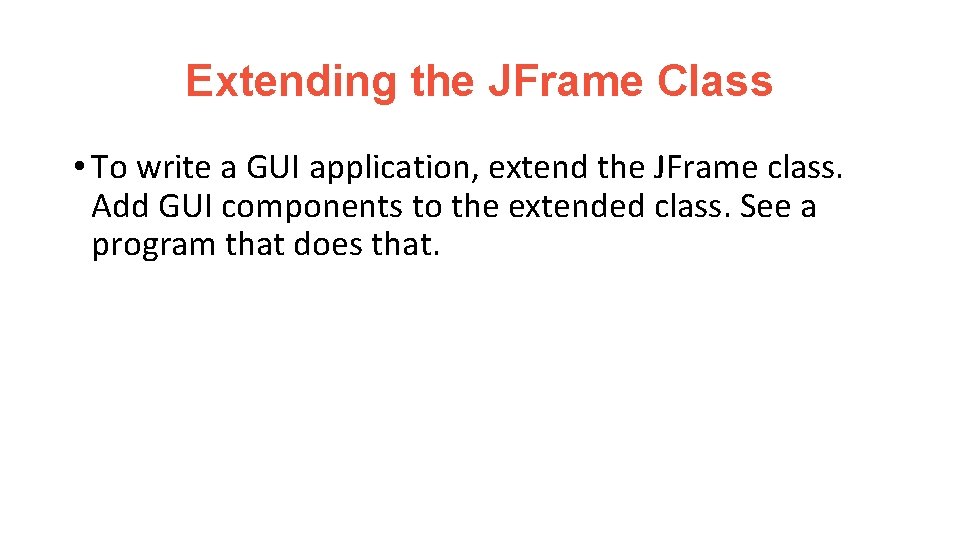
Extending the JFrame Class • To write a GUI application, extend the JFrame class. Add GUI components to the extended class. See a program that does that.
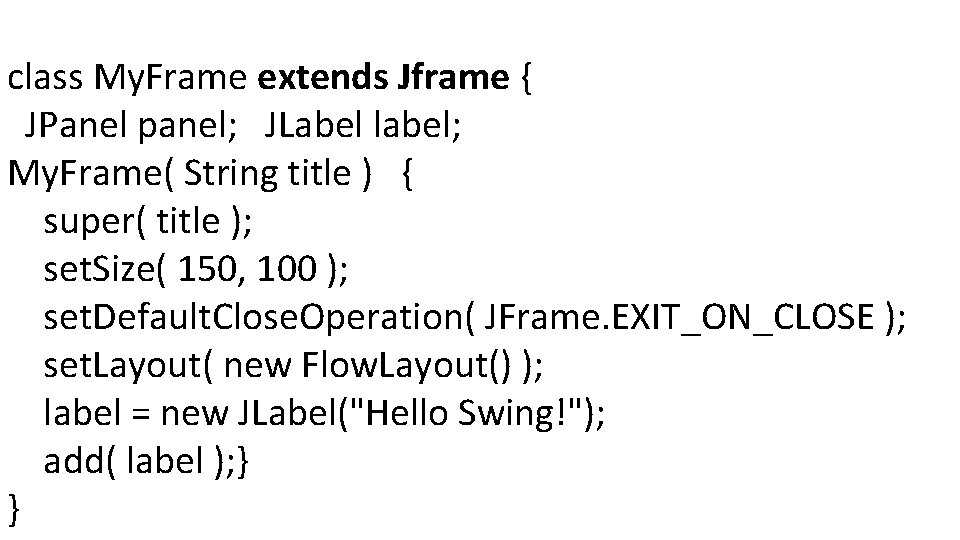
class My. Frame extends Jframe { JPanel panel; JLabel label; My. Frame( String title ) { super( title ); set. Size( 150, 100 ); set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); set. Layout( new Flow. Layout() ); label = new JLabel("Hello Swing!"); add( label ); } }
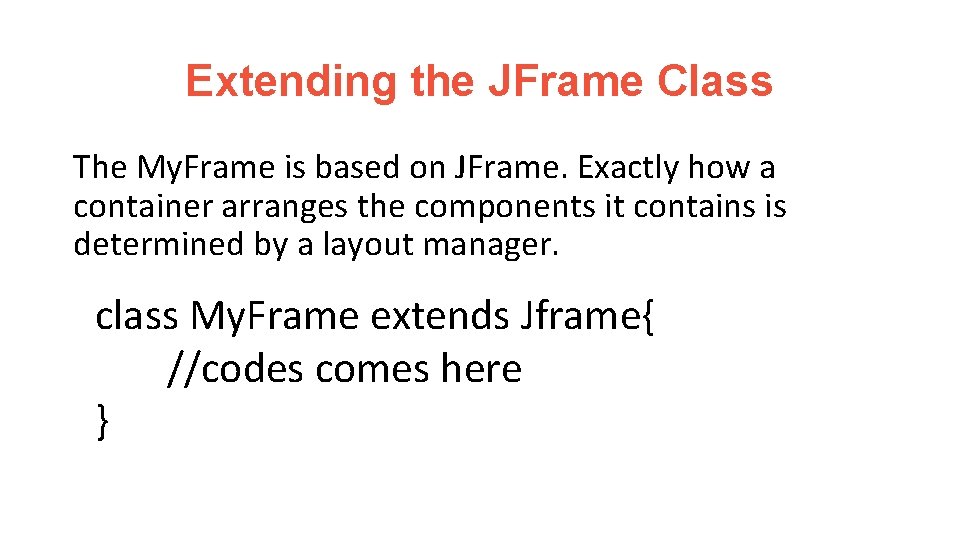
Extending the JFrame Class The My. Frame is based on JFrame. Exactly how a container arranges the components it contains is determined by a layout manager. class My. Frame extends Jframe{ //codes comes here }
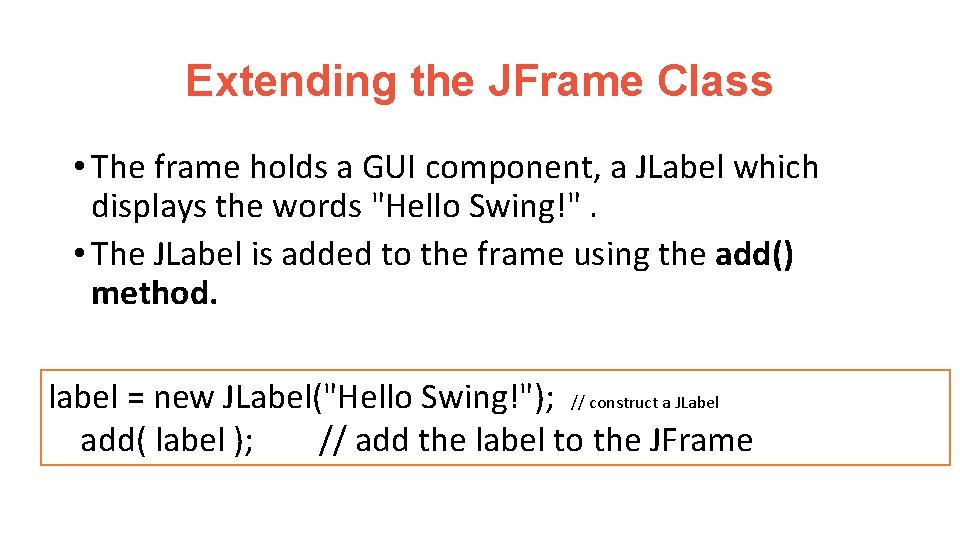
Extending the JFrame Class • The frame holds a GUI component, a JLabel which displays the words "Hello Swing!". • The JLabel is added to the frame using the add() method. label = new JLabel("Hello Swing!"); // construct a JLabel add( label ); // add the label to the JFrame
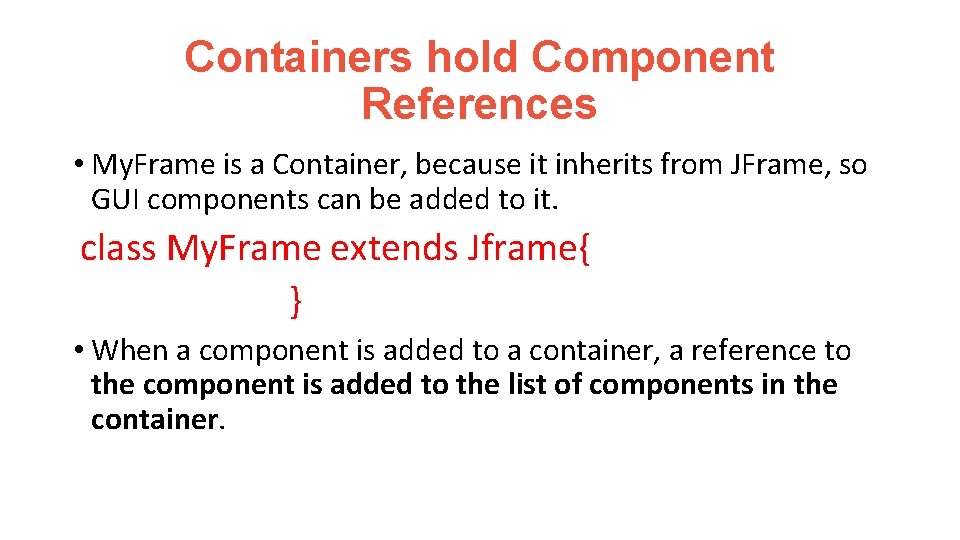
Containers hold Component References • My. Frame is a Container, because it inherits from JFrame, so GUI components can be added to it. class My. Frame extends Jframe{ } • When a component is added to a container, a reference to the component is added to the list of components in the container.
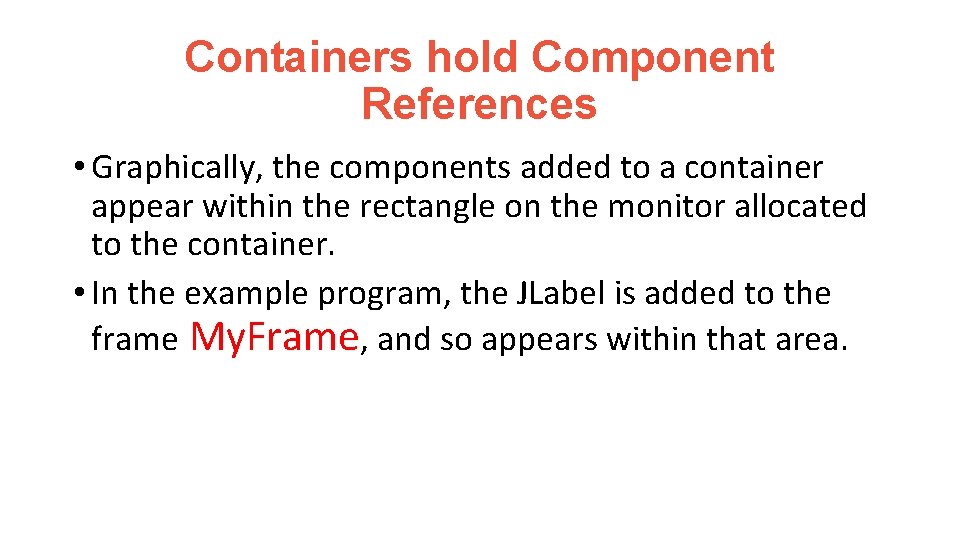
Containers hold Component References • Graphically, the components added to a container appear within the rectangle on the monitor allocated to the container. • In the example program, the JLabel is added to the frame My. Frame, and so appears within that area.
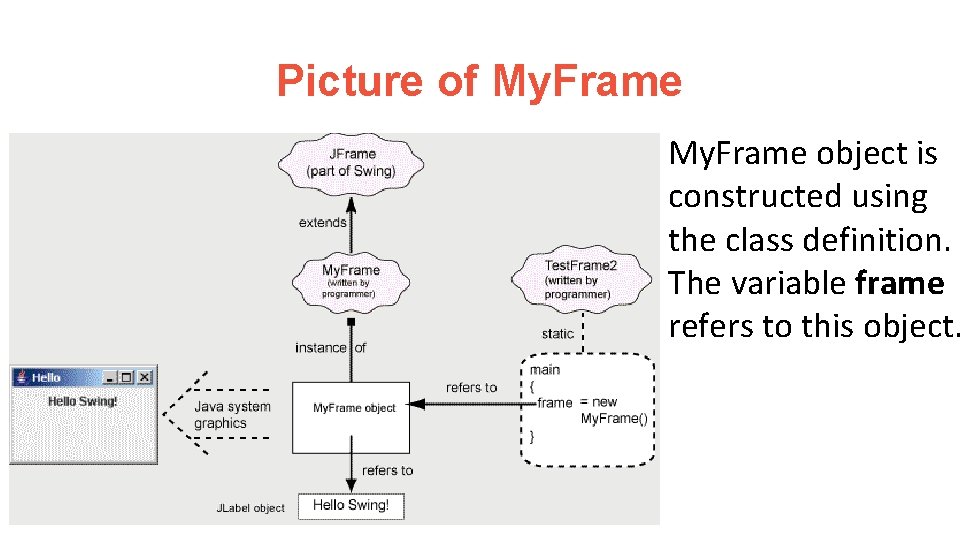
Picture of My. Frame object is constructed using the class definition. The variable frame refers to this object.
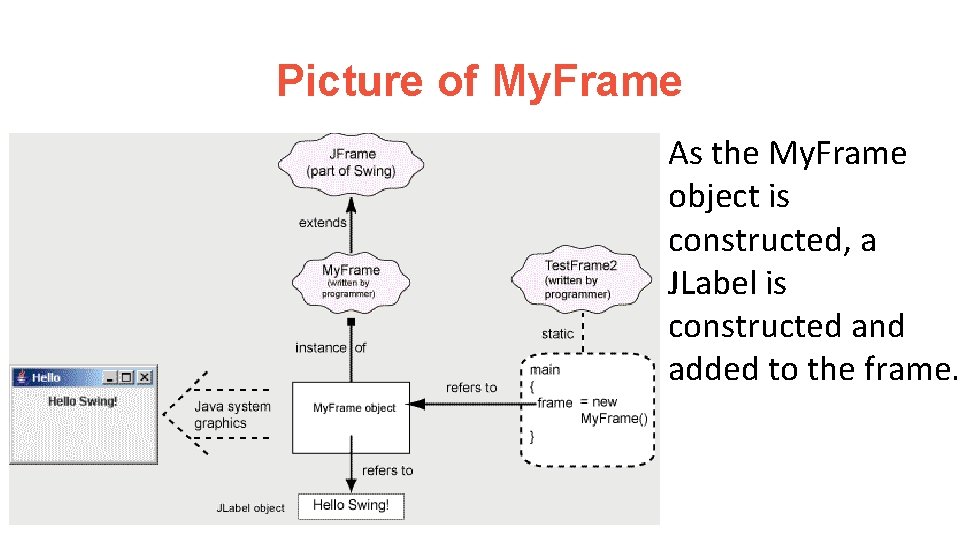
Picture of My. Frame As the My. Frame object is constructed, a JLabel is constructed and added to the frame.
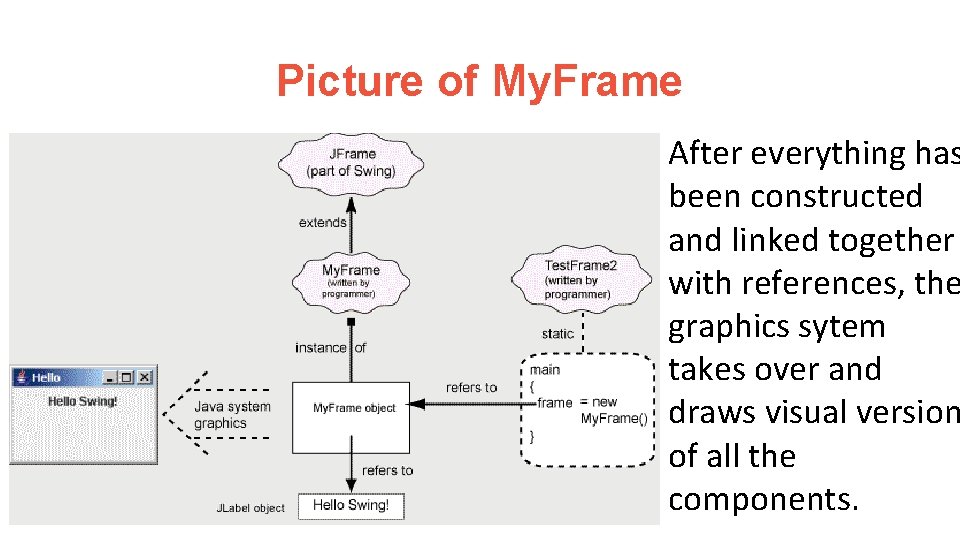
Picture of My. Frame After everything has been constructed and linked together with references, the graphics sytem takes over and draws visual version of all the components.
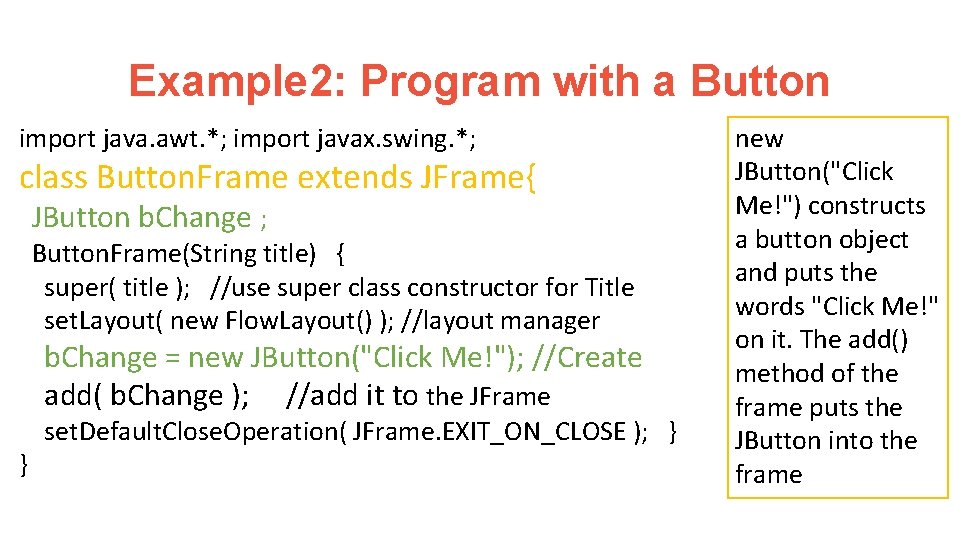
Example 2: Program with a Button import java. awt. *; import javax. swing. *; class Button. Frame extends JFrame{ JButton b. Change ; Button. Frame(String title) { super( title ); //use super class constructor for Title set. Layout( new Flow. Layout() ); //layout manager b. Change = new JButton("Click Me!"); //Create add( b. Change ); //add it to the JFrame set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } } new JButton("Click Me!") constructs a button object and puts the words "Click Me!" on it. The add() method of the frame puts the JButton into the frame
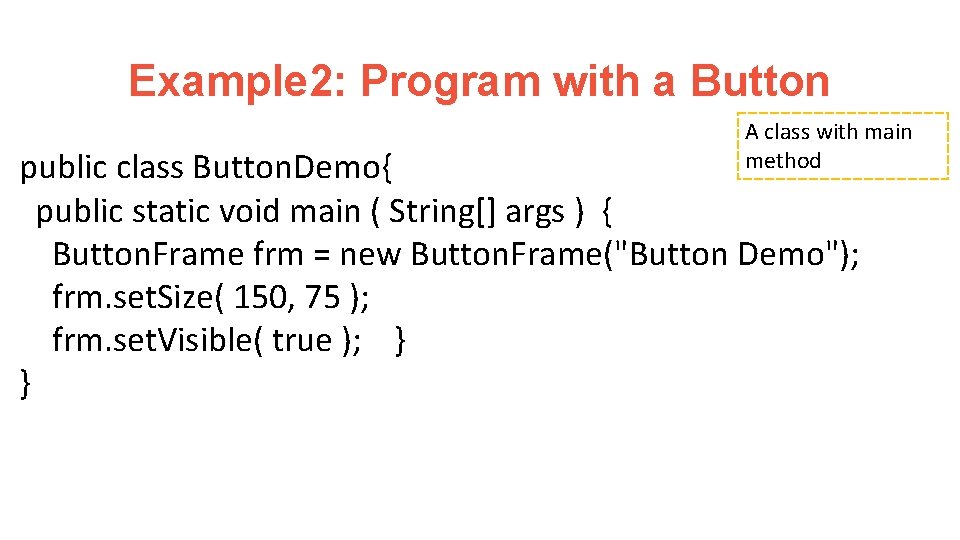
Example 2: Program with a Button A class with main method public class Button. Demo{ public static void main ( String[] args ) { Button. Frame frm = new Button. Frame("Button Demo"); frm. set. Size( 150, 75 ); frm. set. Visible( true ); } }
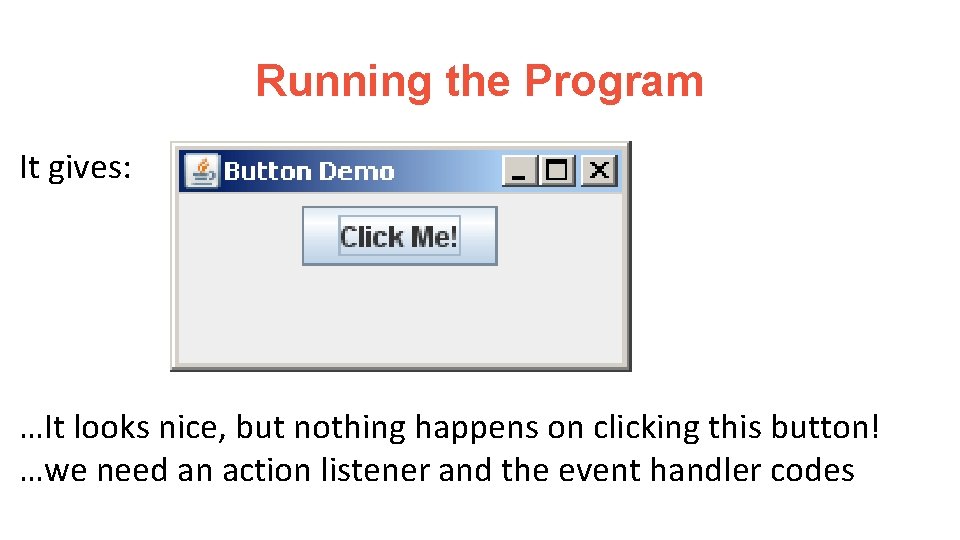
Running the Program It gives: …It looks nice, but nothing happens on clicking this button! …we need an action listener and the event handler codes
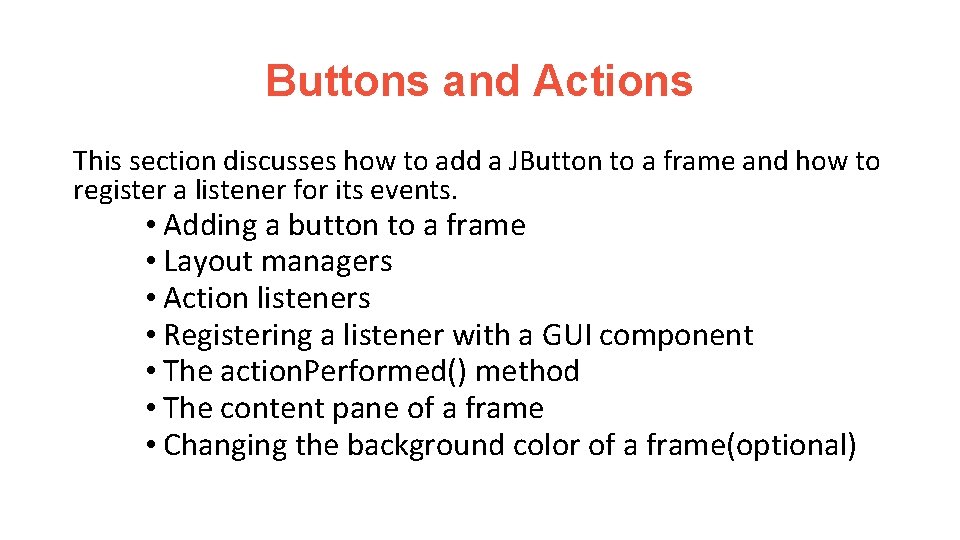
Buttons and Actions This section discusses how to add a JButton to a frame and how to register a listener for its events. • Adding a button to a frame • Layout managers • Action listeners • Registering a listener with a GUI component • The action. Performed() method • The content pane of a frame • Changing the background color of a frame(optional)
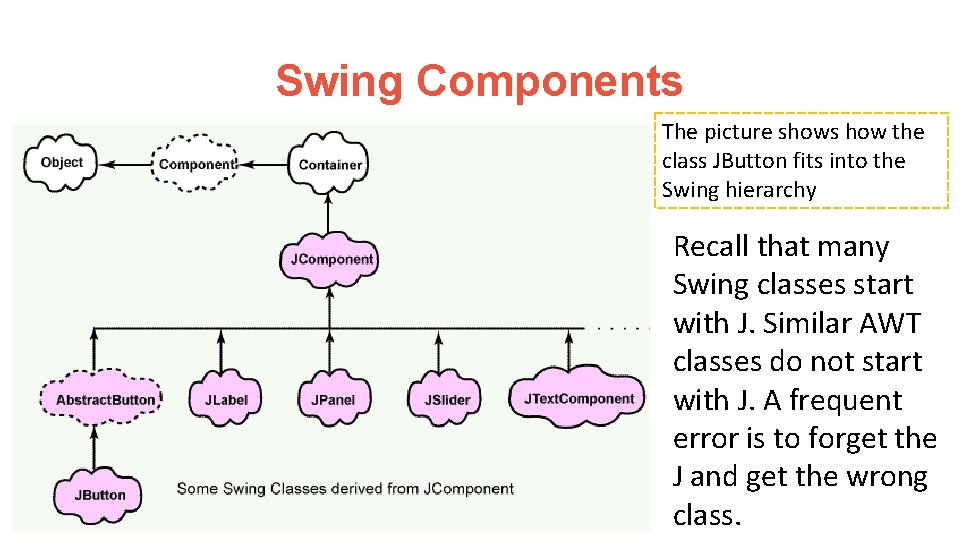
Swing Components The picture shows how the class JButton fits into the Swing hierarchy Recall that many Swing classes start with J. Similar AWT classes do not start with J. A frequent error is to forget the J and get the wrong class.
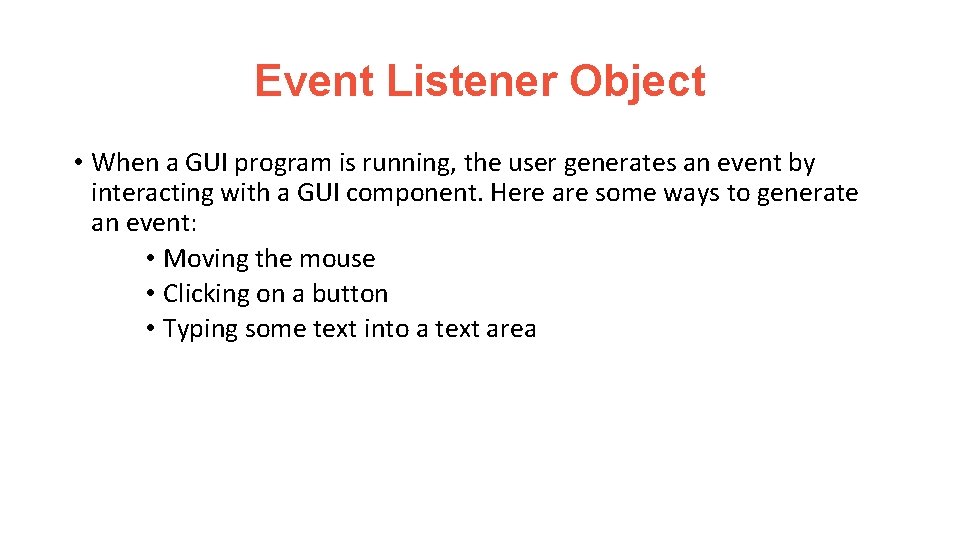
Event Listener Object • When a GUI program is running, the user generates an event by interacting with a GUI component. Here are some ways to generate an event: • Moving the mouse • Clicking on a button • Typing some text into a text area
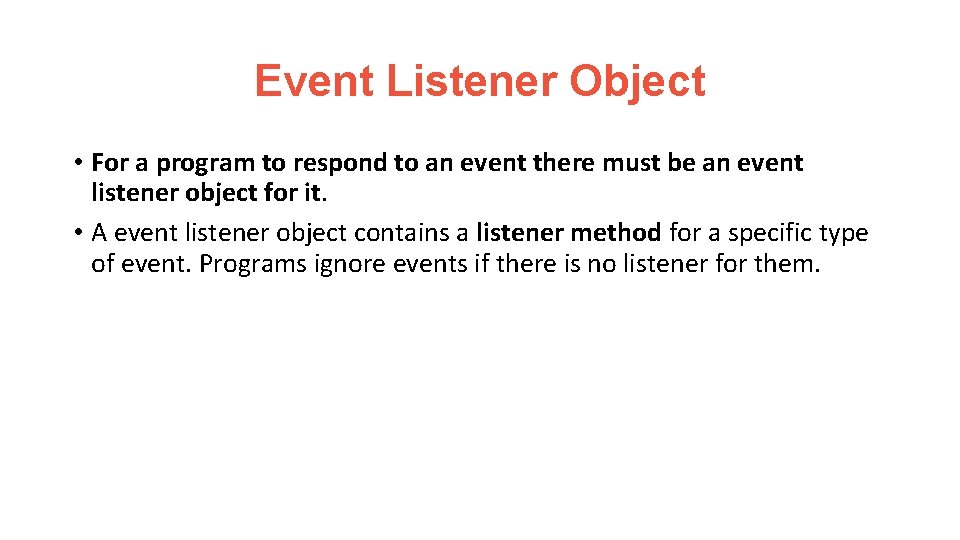
Event Listener Object • For a program to respond to an event there must be an event listener object for it. • A event listener object contains a listener method for a specific type of event. Programs ignore events if there is no listener for them.
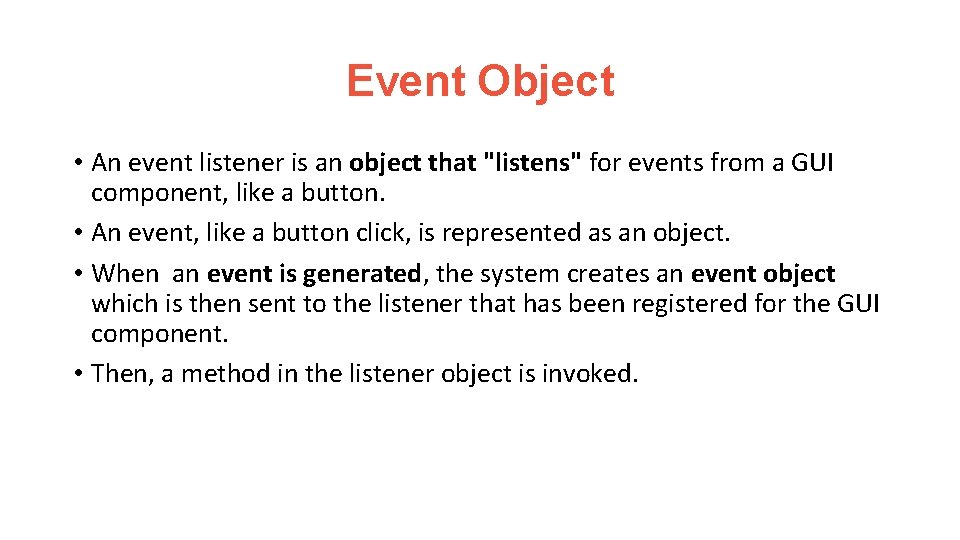
Event Object • An event listener is an object that "listens" for events from a GUI component, like a button. • An event, like a button click, is represented as an object. • When an event is generated, the system creates an event object which is then sent to the listener that has been registered for the GUI component. • Then, a method in the listener object is invoked.
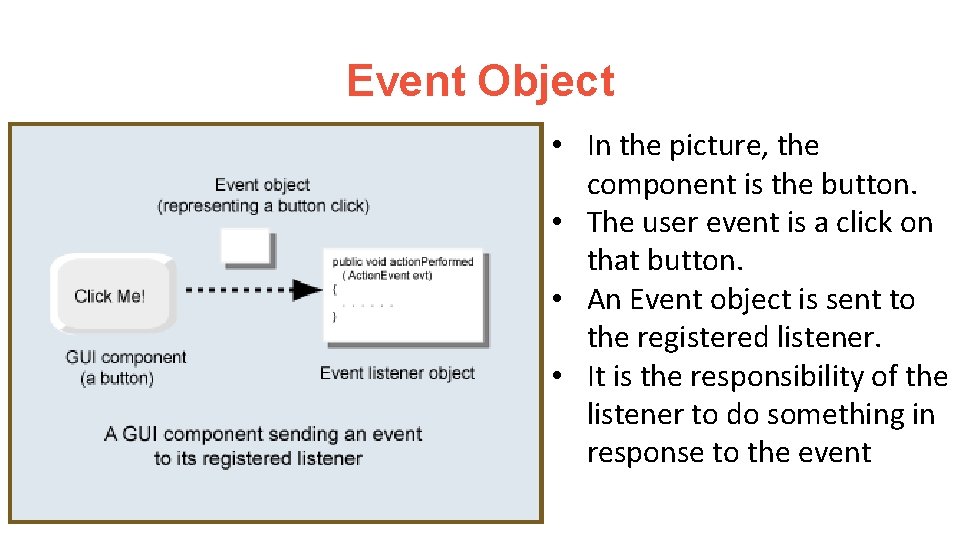
Event Object • In the picture, the component is the button. • The user event is a click on that button. • An Event object is sent to the registered listener. • It is the responsibility of the listener to do something in response to the event
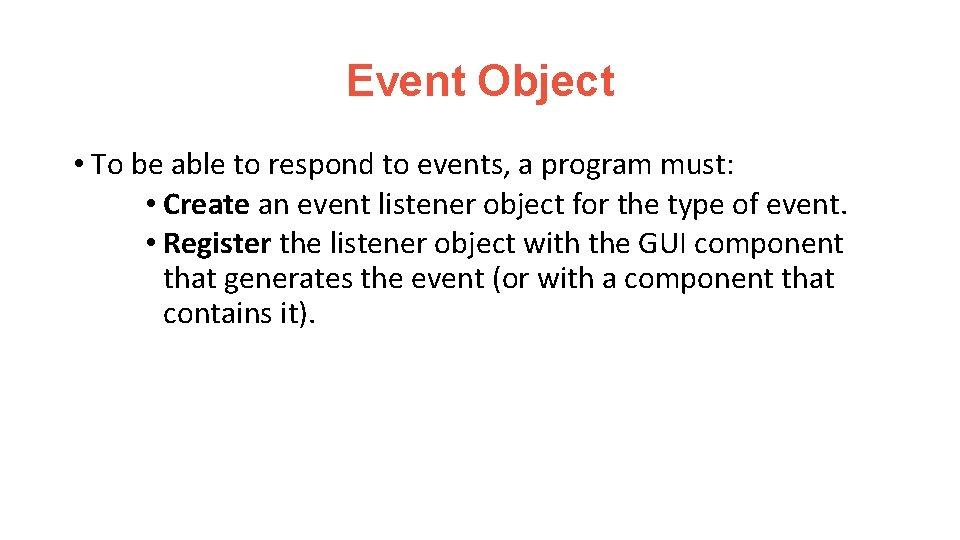
Event Object • To be able to respond to events, a program must: • Create an event listener object for the type of event. • Register the listener object with the GUI component that generates the event (or with a component that contains it).
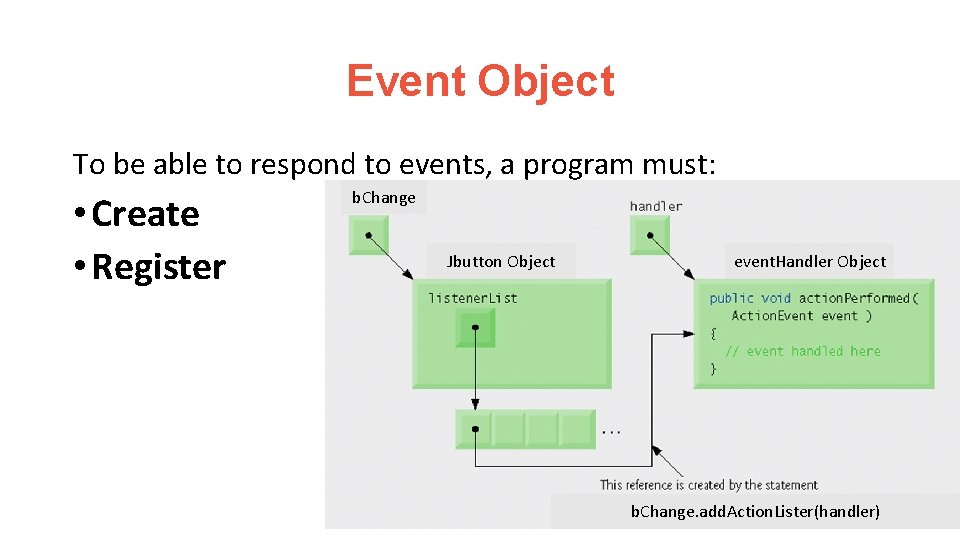
Event Object To be able to respond to events, a program must: • Create • Register b. Change Jbutton Object event. Handler Object b. Change. add. Action. Lister(handler)
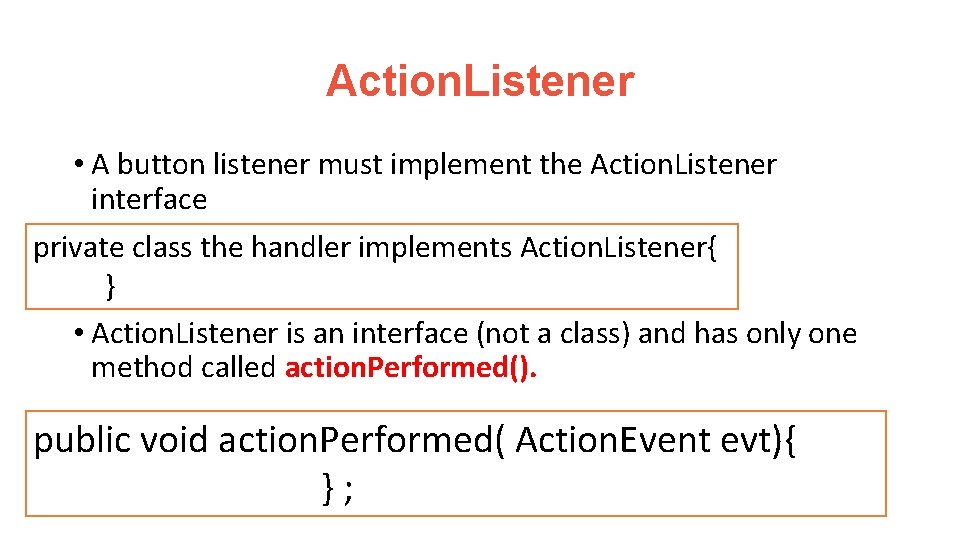
Action. Listener • A button listener must implement the Action. Listener interface private class the handler implements Action. Listener{ } • Action. Listener is an interface (not a class) and has only one method called action. Performed(). public void action. Performed( Action. Event evt){ } ;
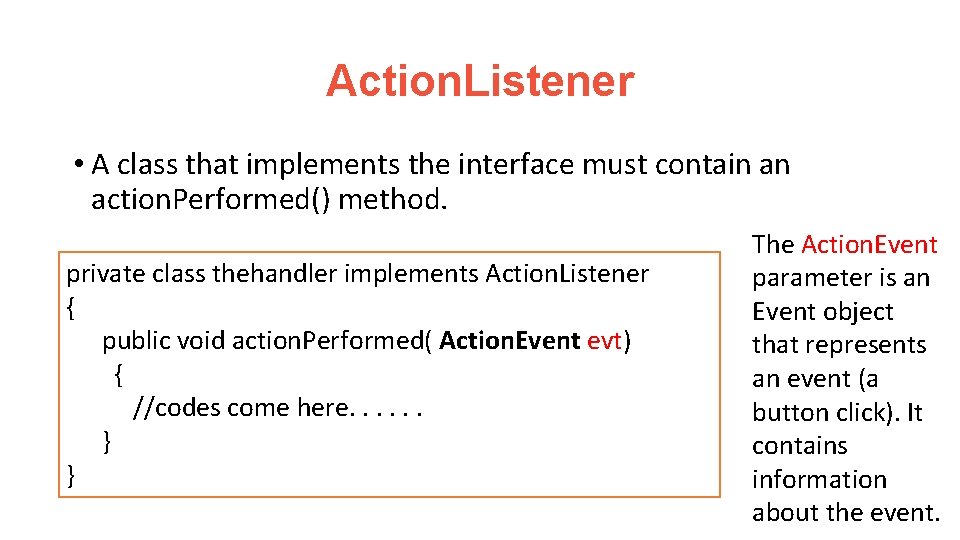
Action. Listener • A class that implements the interface must contain an action. Performed() method. private class thehandler implements Action. Listener { public void action. Performed( Action. Event evt) { //codes come here. . . } } The Action. Event parameter is an Event object that represents an event (a button click). It contains information about the event.
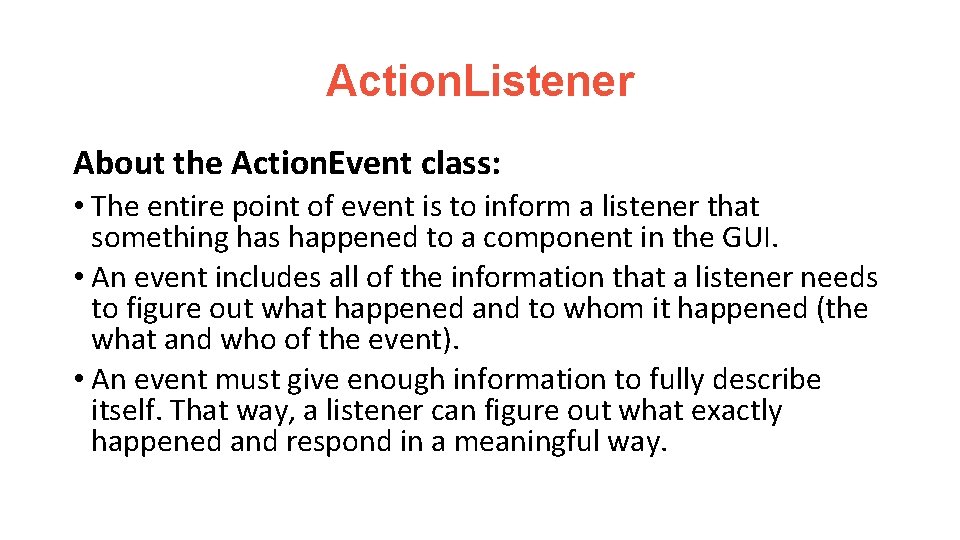
Action. Listener About the Action. Event class: • The entire point of event is to inform a listener that something has happened to a component in the GUI. • An event includes all of the information that a listener needs to figure out what happened and to whom it happened (the what and who of the event). • An event must give enough information to fully describe itself. That way, a listener can figure out what exactly happened and respond in a meaningful way.
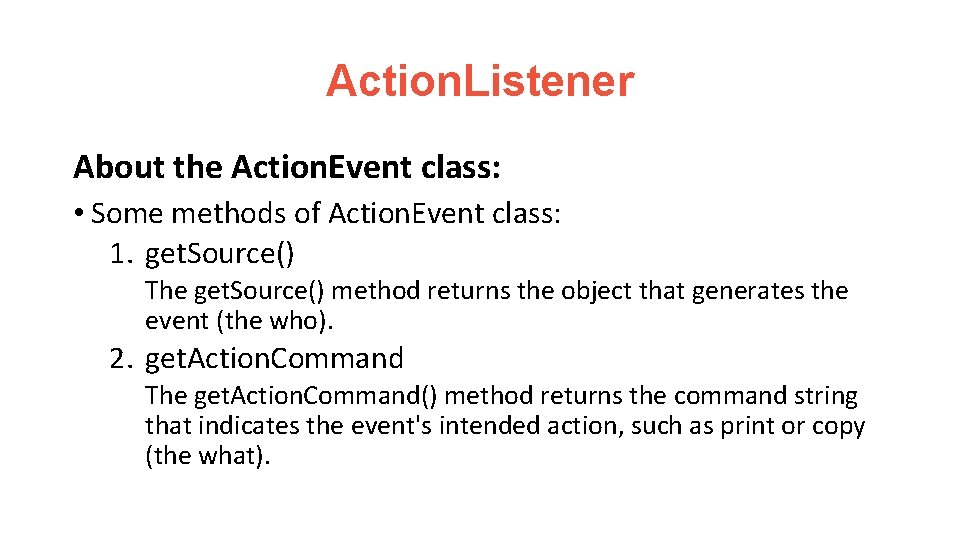
Action. Listener About the Action. Event class: • Some methods of Action. Event class: 1. get. Source() The get. Source() method returns the object that generates the event (the who). 2. get. Action. Command The get. Action. Command() method returns the command string that indicates the event's intended action, such as print or copy (the what).
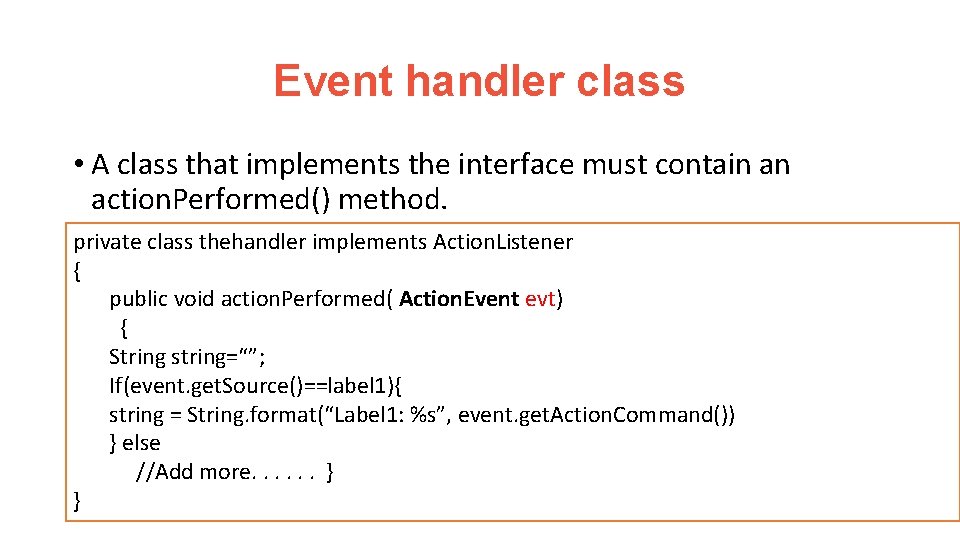
Event handler class • A class that implements the interface must contain an action. Performed() method. private class thehandler implements Action. Listener { public void action. Performed( Action. Event evt) { String string=“”; If(event. get. Source()==label 1){ string = String. format(“Label 1: %s”, event. get. Action. Command()) } else //Add more. . . } }
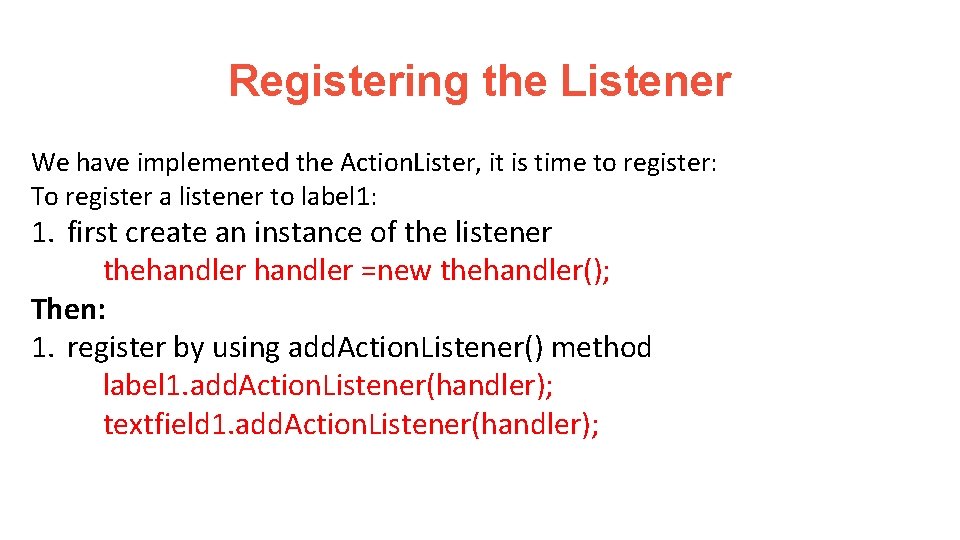
Registering the Listener We have implemented the Action. Lister, it is time to register: To register a listener to label 1: 1. first create an instance of the listener thehandler =new thehandler(); Then: 1. register by using add. Action. Listener() method label 1. add. Action. Listener(handler); textfield 1. add. Action. Listener(handler);
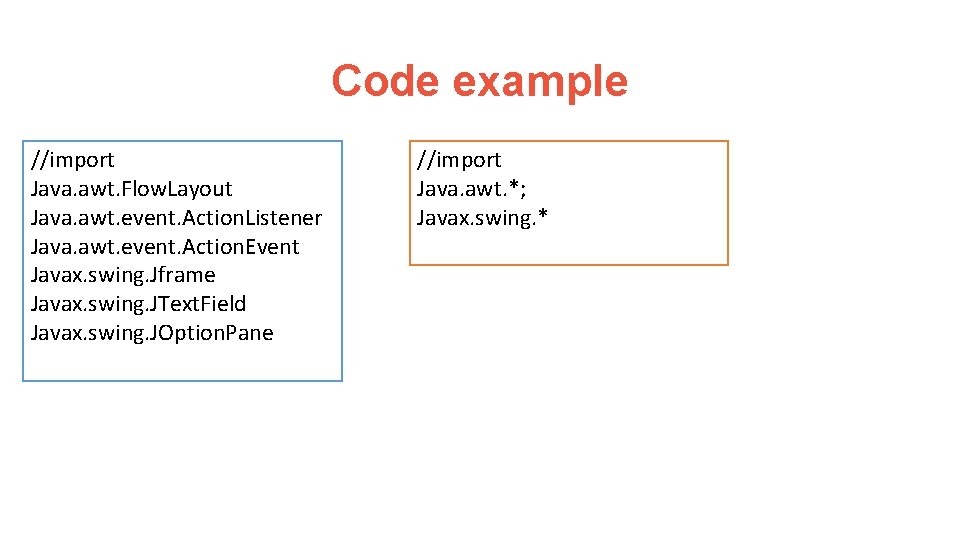
Code example //import Java. awt. Flow. Layout Java. awt. event. Action. Listener Java. awt. event. Action. Event Javax. swing. Jframe Javax. swing. JText. Field Javax. swing. JOption. Pane //import Java. awt. *; Javax. swing. *
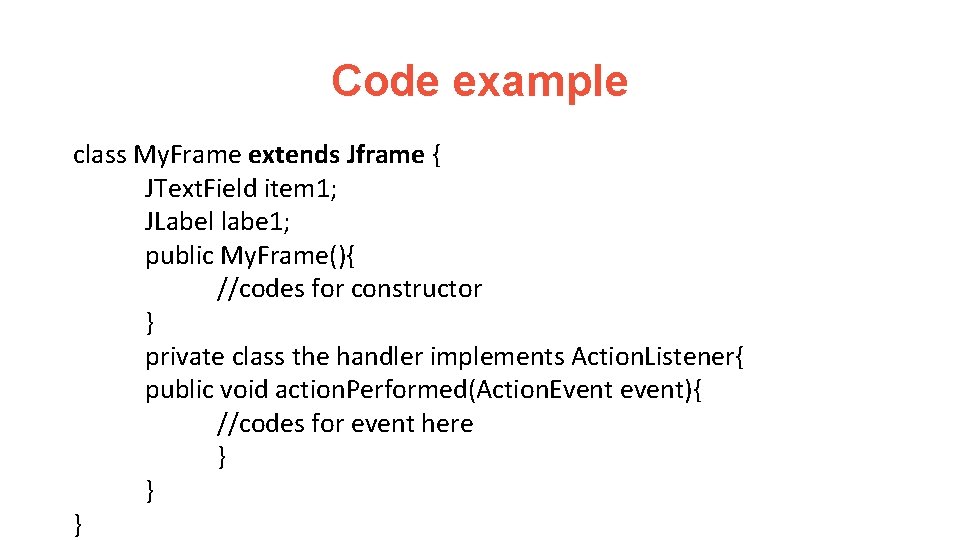
Code example class My. Frame extends Jframe { JText. Field item 1; JLabel labe 1; public My. Frame(){ //codes for constructor } private class the handler implements Action. Listener{ public void action. Performed(Action. Event event){ //codes for event here } } }
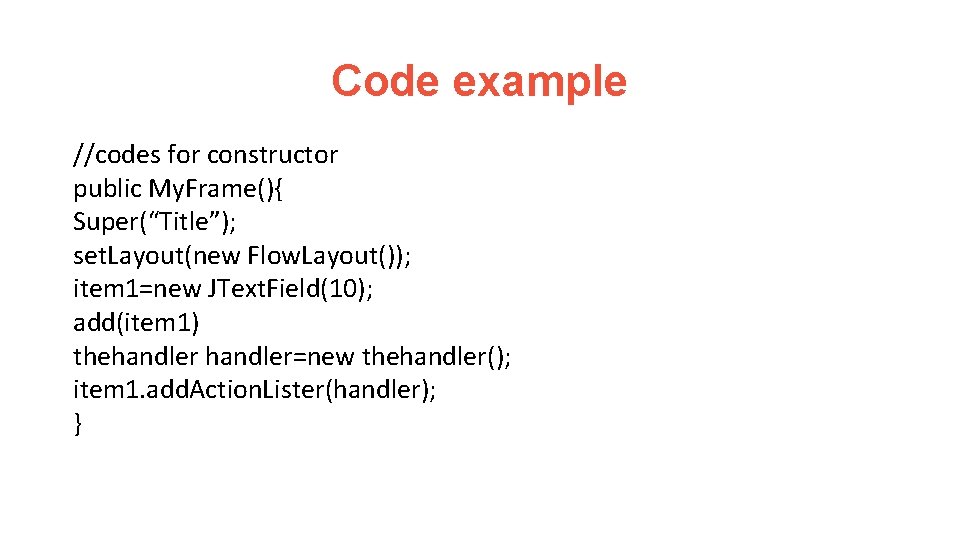
Code example //codes for constructor public My. Frame(){ Super(“Title”); set. Layout(new Flow. Layout()); item 1=new JText. Field(10); add(item 1) thehandler=new thehandler(); item 1. add. Action. Lister(handler); }
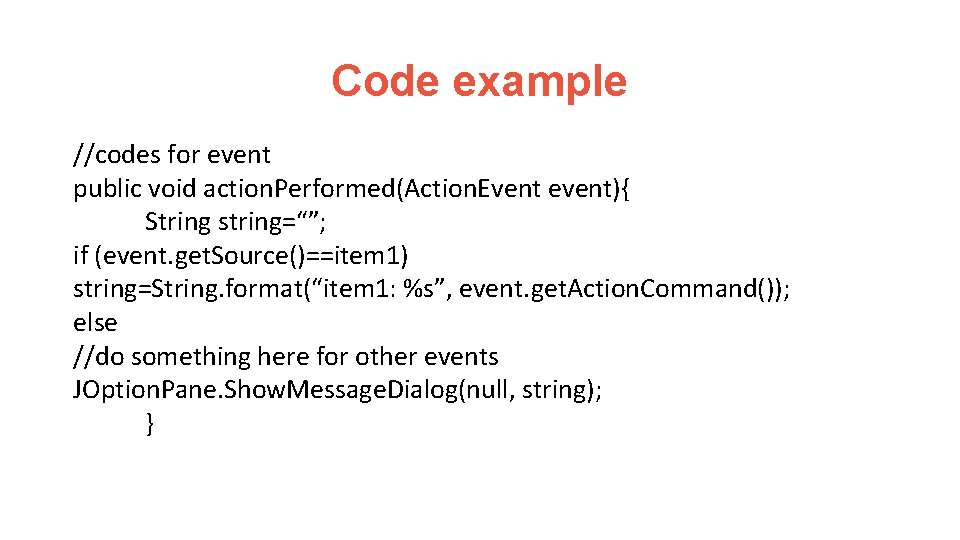
Code example //codes for event public void action. Performed(Action. Event event){ String string=“”; if (event. get. Source()==item 1) string=String. format(“item 1: %s”, event. get. Action. Command()); else //do something here for other events JOption. Pane. Show. Message. Dialog(null, string); }
![Code example main method public class My Frame Demo Public static void mainString args Code example //main method public class My. Frame. Demo{ Public static void main(String[] args){](https://slidetodoc.com/presentation_image_h/0c3d932d7ad923f768bb9733747892ff/image-89.jpg)
Code example //main method public class My. Frame. Demo{ Public static void main(String[] args){ My. Frame frame 1= new My. Frame(); frame 1. set. Size( 400, 200 ); frame 1. set. Visible(true); Frame 1. set. Default. Close. Operation( JFrame. EXIT_ON_CLOSE ); } }
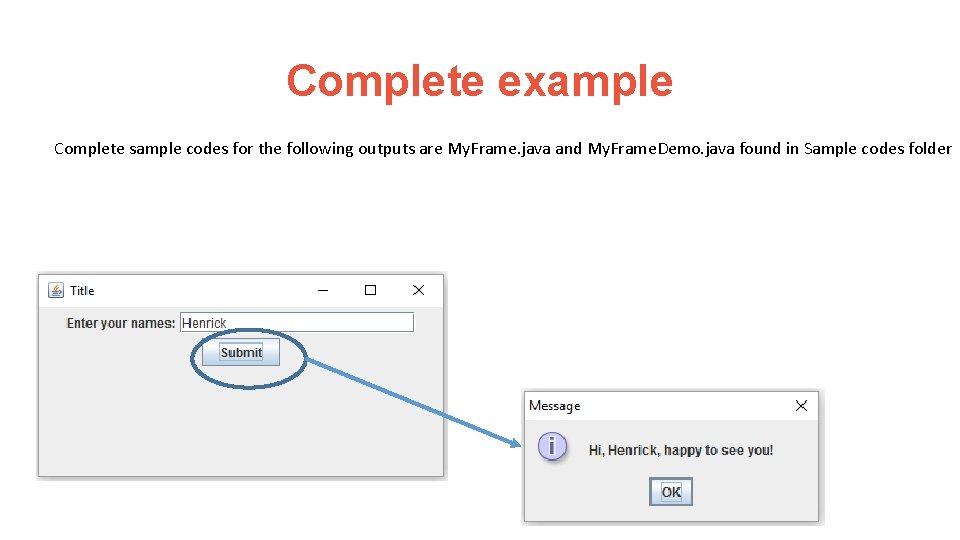
Complete example Complete sample codes for the following outputs are My. Frame. java and My. Frame. Demo. java found in Sample codes folder
Ge gi gue gui güe güi
Rapid gui programming with python and qt
Gui toolkits
Linux gui development
Chapter 9 topics in analytic geometry
Perbedaan linear programming dan integer programming
Greedy vs dynamic
What is in system programming
Integer programming vs linear programming
Programing adalah
Common gui event types and listener interfaces in java
Surprising reversal essay example
Formulating and clarifying the research topic
Division and classification examples
Uiuc cs 527
Cause and effect essays topics
How to write advantages and disadvantages
Importance of research problem
Computer and society topics
International trade and finance topics
Ib sports science topic 4
Ib sports exercise and health science
Research question about factor isolating question
Global citizenship education topics and learning objectives
Behavioral diagrams
Gui in software engineering
Brokertec gui
Pengertian gui
Jui gui
The gui for metasploit is given by
Cli vs gui
Aplikasi desktop linux yang banyak digunakan pada mode gui
Containers in java swing
Swing 4
Java swing libraries
Java gui awt
Java gui basics
Immediate mode ui
Gui history
Gui programmierung java
Pengertian gui
Gui vs hmi
Gui bloopers
Basics of gui
Sopa de letras ga gue gui go gu
Mots en gue gui
Contou
Cui gui
Gui bloopers
User interface characteristics
Windows builder eclipse
Dns server with gui
Indowordnet
Wpf d3dimage
Rveillon
Instalasi sistem operasi berbasis gui dapat dilakukan dari
Matlab gui design
Instalasi sistem operasi berbasis gui dapat dilakukan dari
Kelebihan dan kekurangan gui
Gui meaning
Gui programmierung java
Java gui
Java graphics set color
Java gui thread
Gui architectures
Graphical user interface testing tools
台大 matlab
Gui meaning
Autor
Gui event handling
Istituto universitario sophia
Virginia gui
Lua mobile app development
Gui design
Colegio fenmapu
Qing wen nin gui xing
Min gui
Tegragui
Swing scala
Lammps gui
Which operating system is a multiuser os developed in 1969
Gui for r
Java gui design
Modern java gui
Java animation example
Introduction of gui
Guided, stochastic model-based gui testing of android apps
Gui xt
Programs that operate in a gui environment must be
Java gui fx
What is gui