Creating User Interfaces EventDriven Programming Frequently used GUI
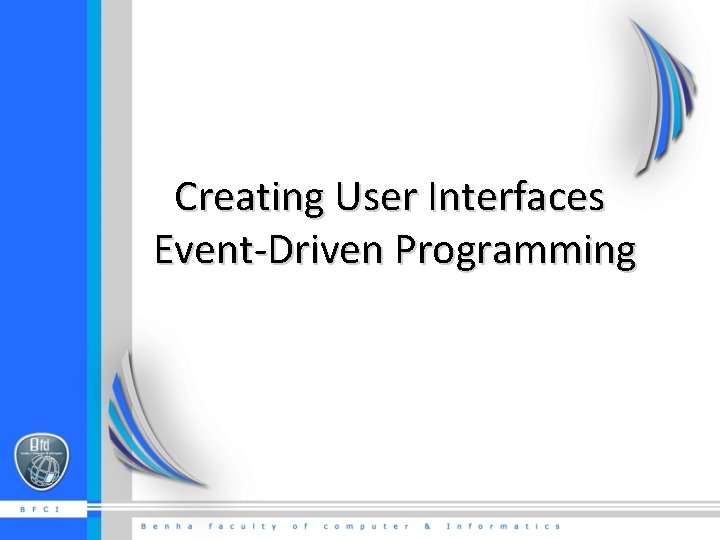
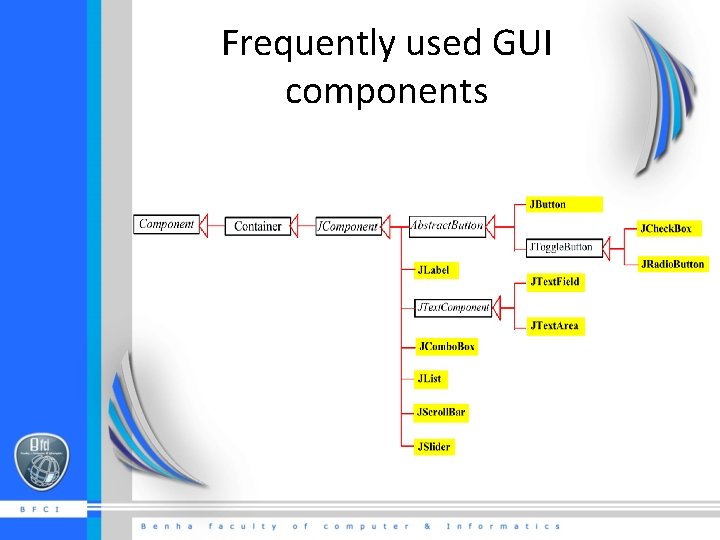
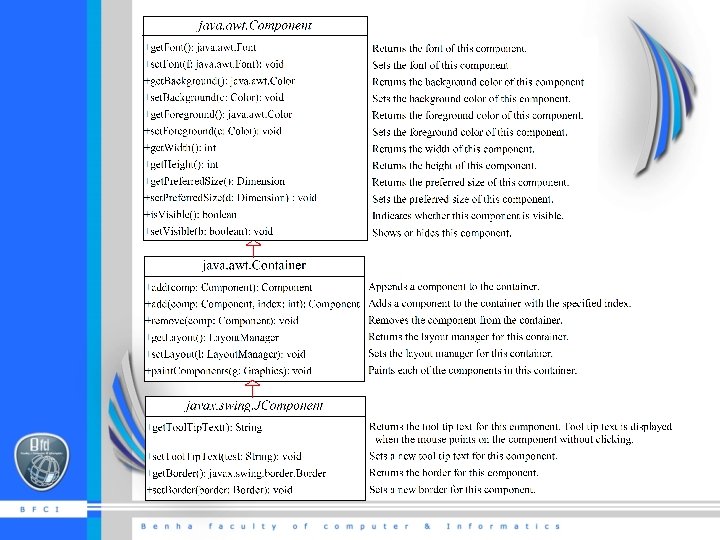
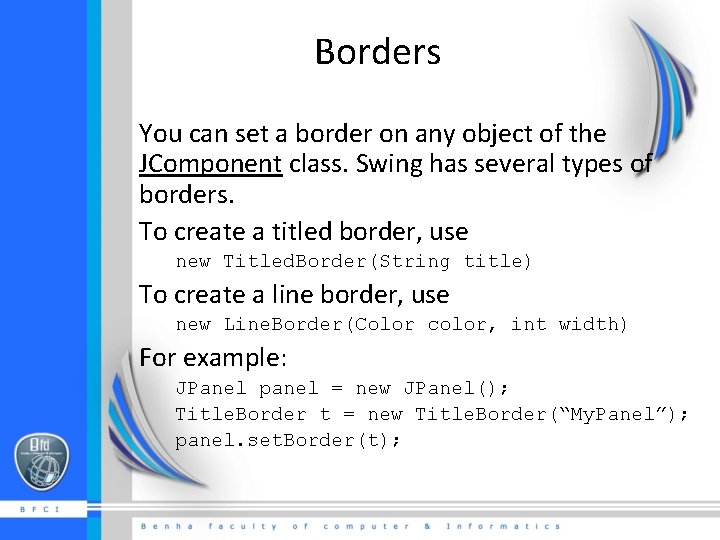
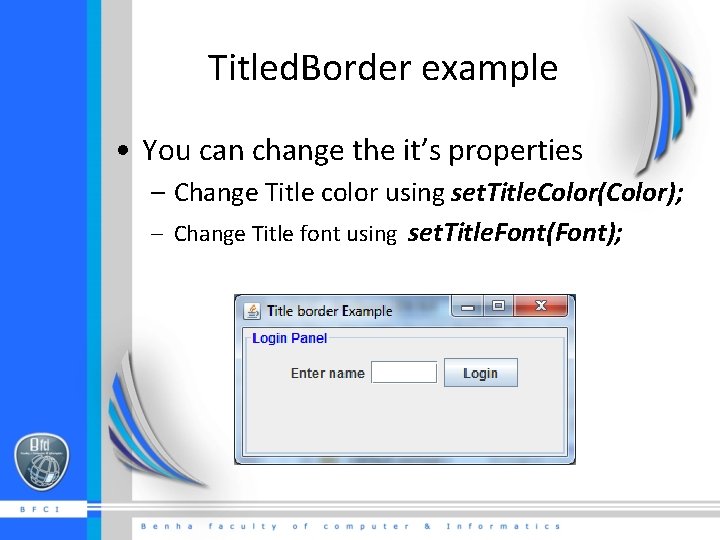
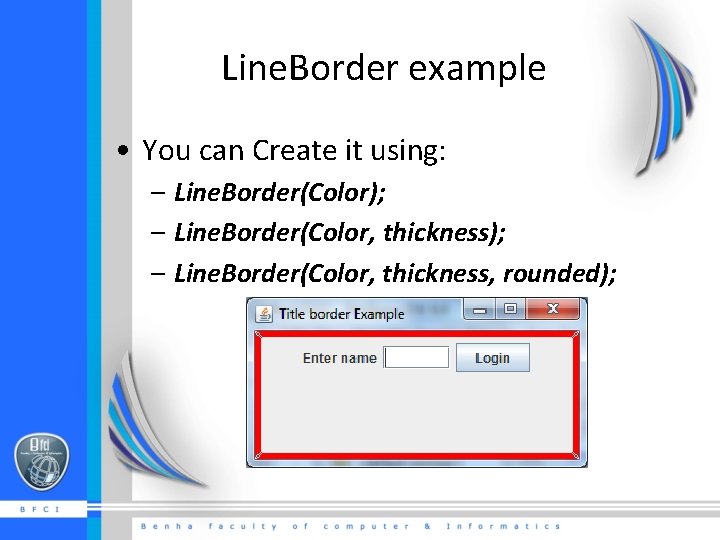
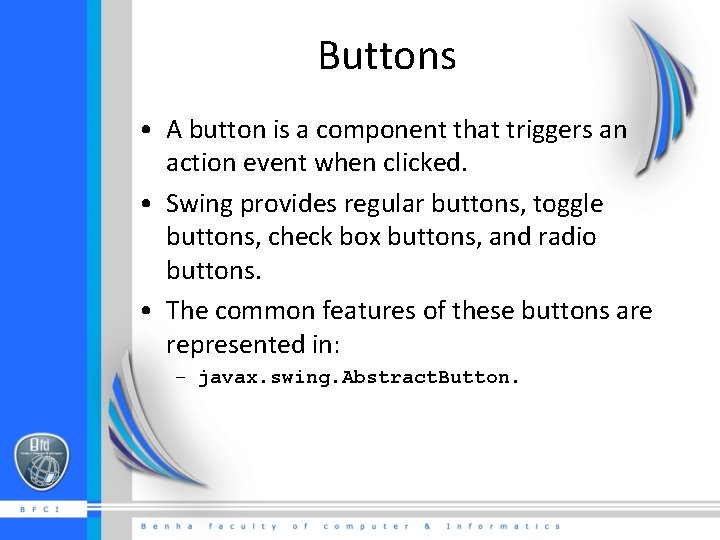
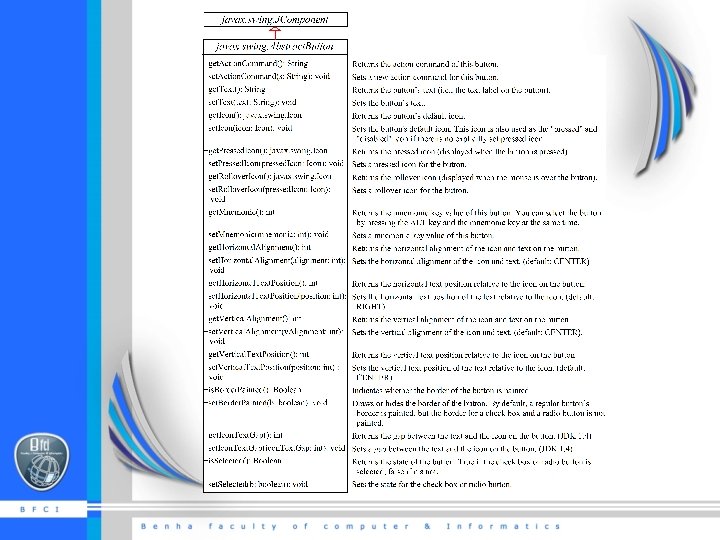
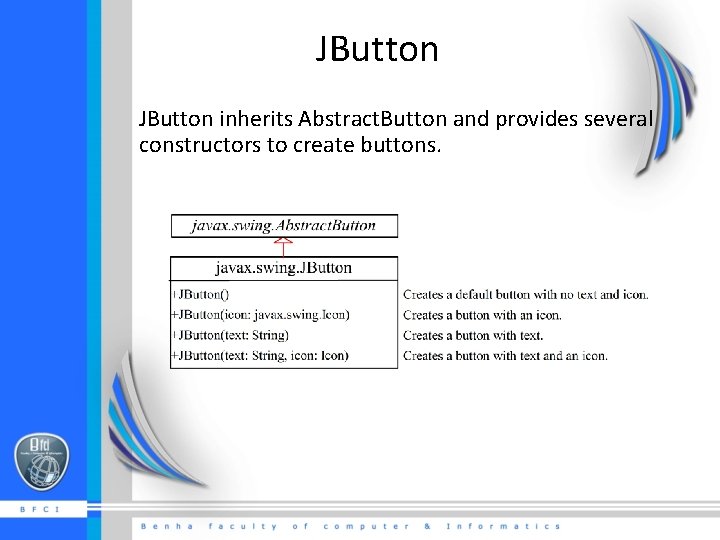
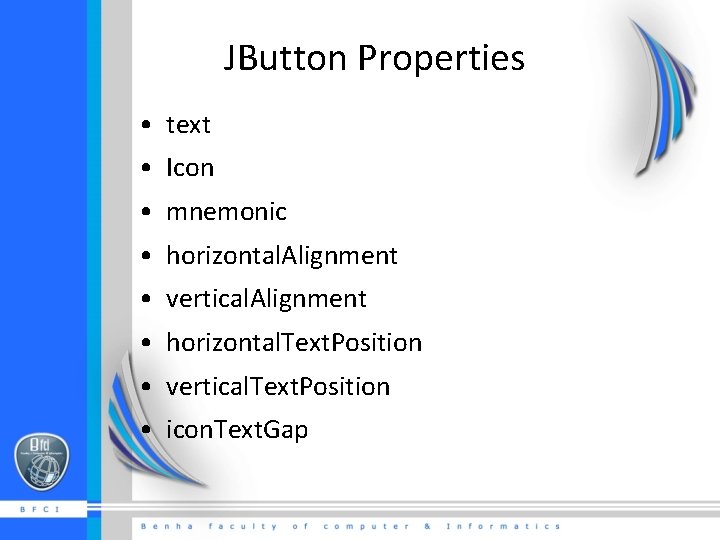
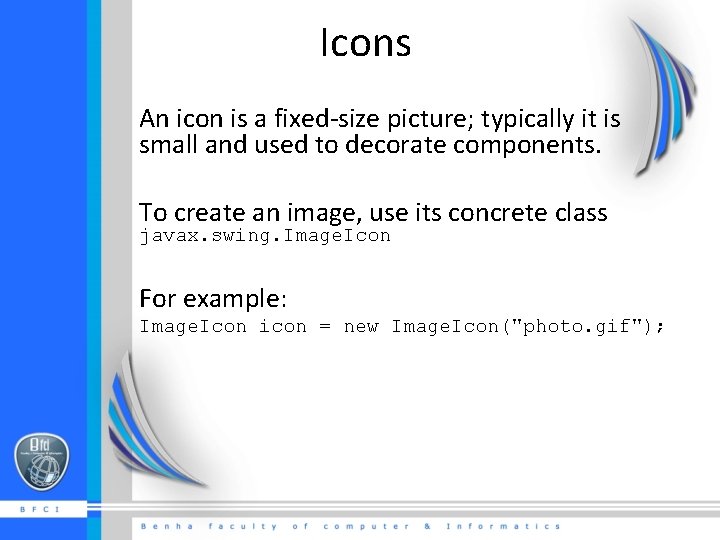
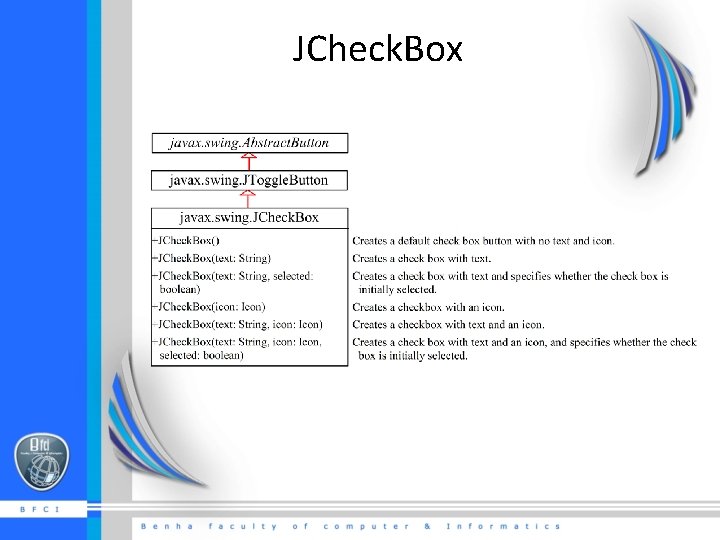
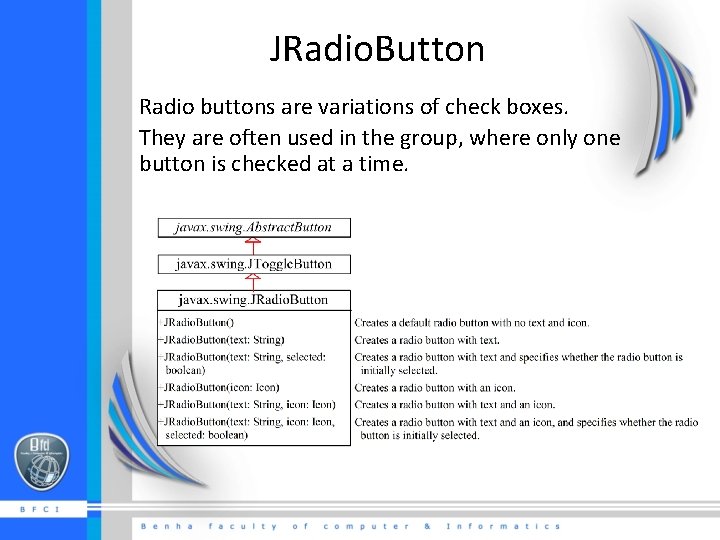
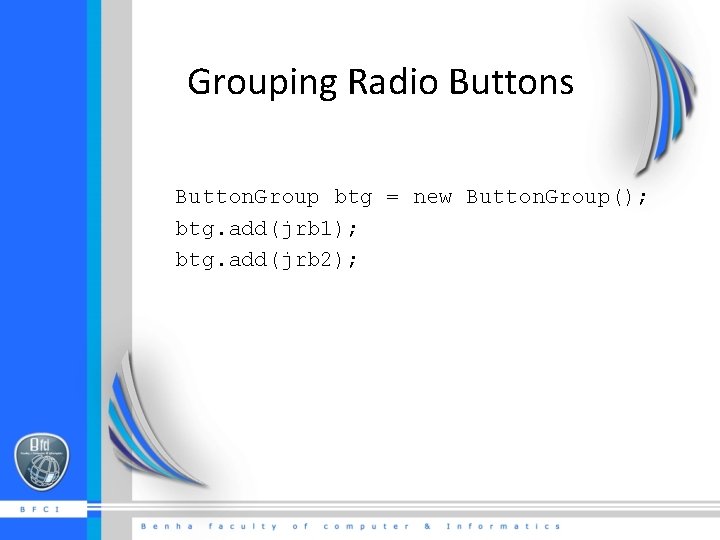
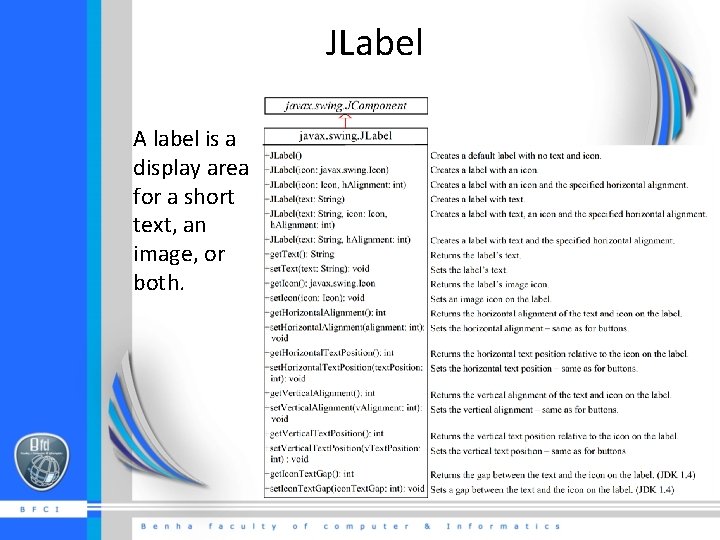
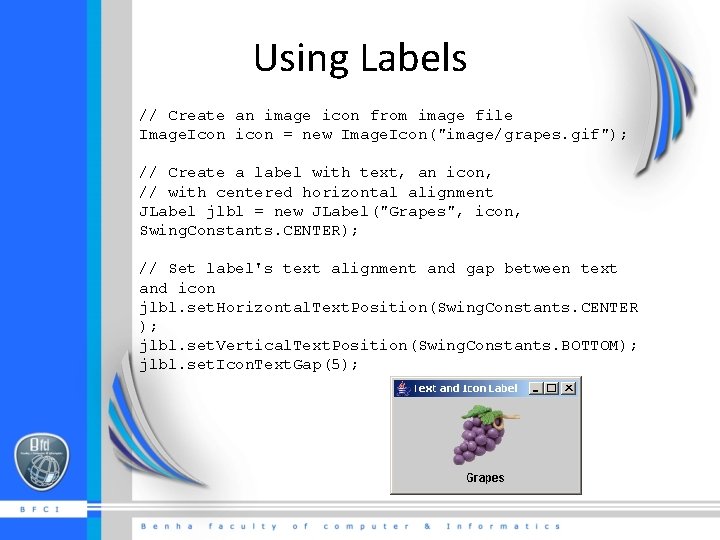
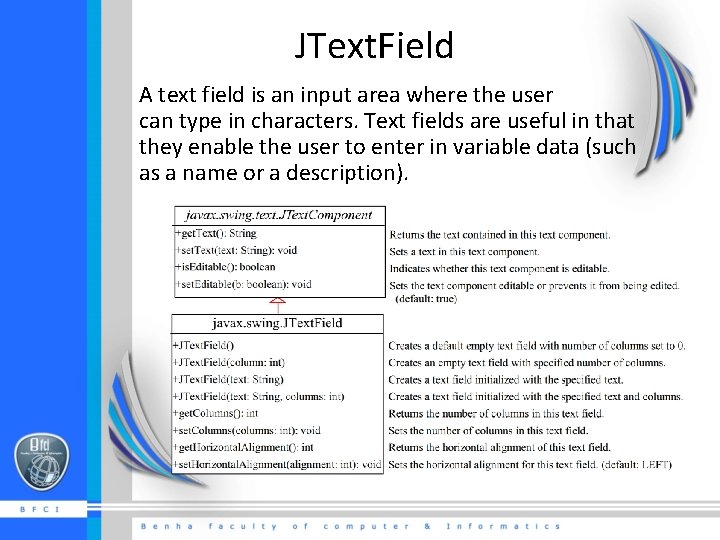
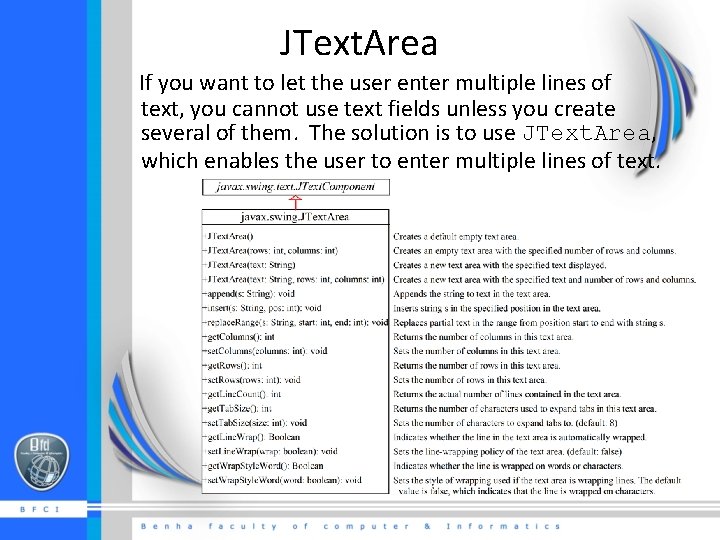
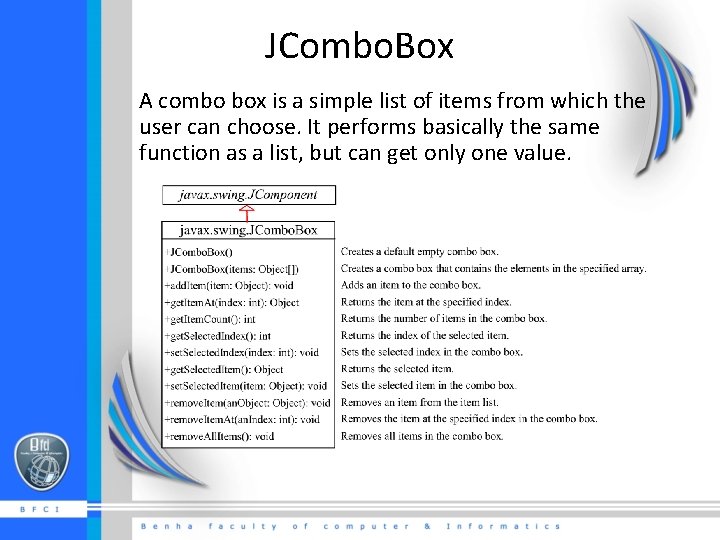
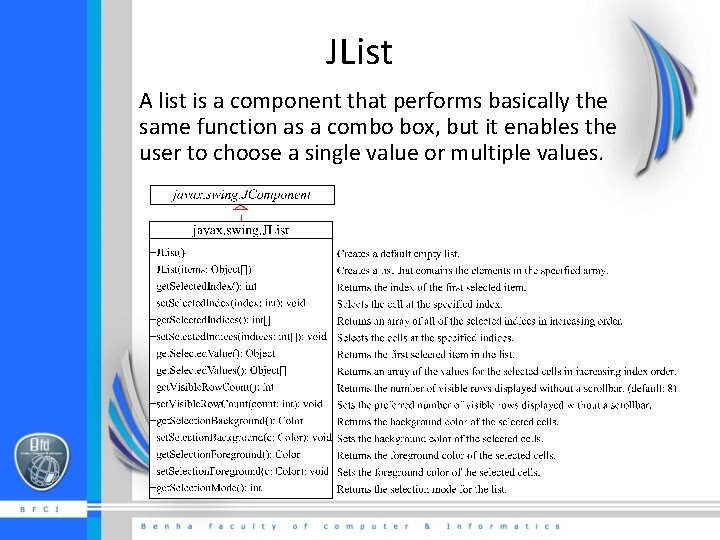
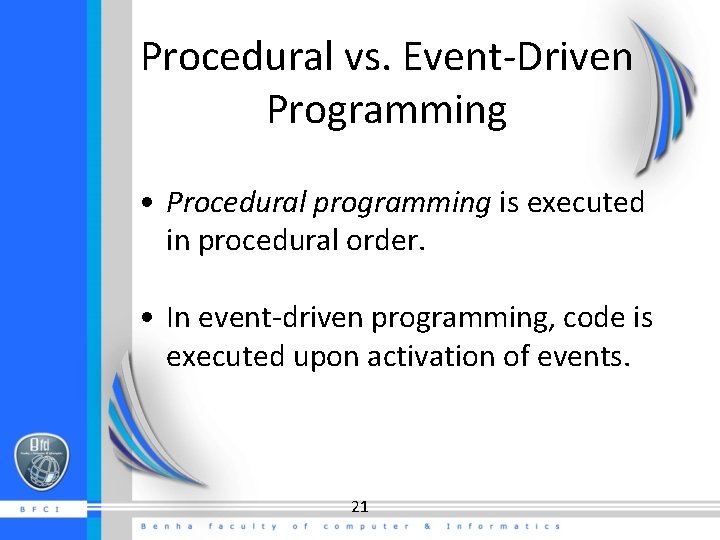
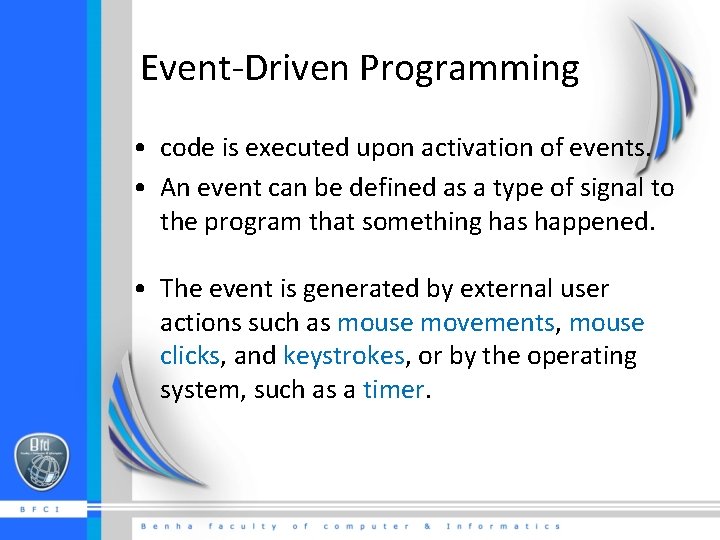
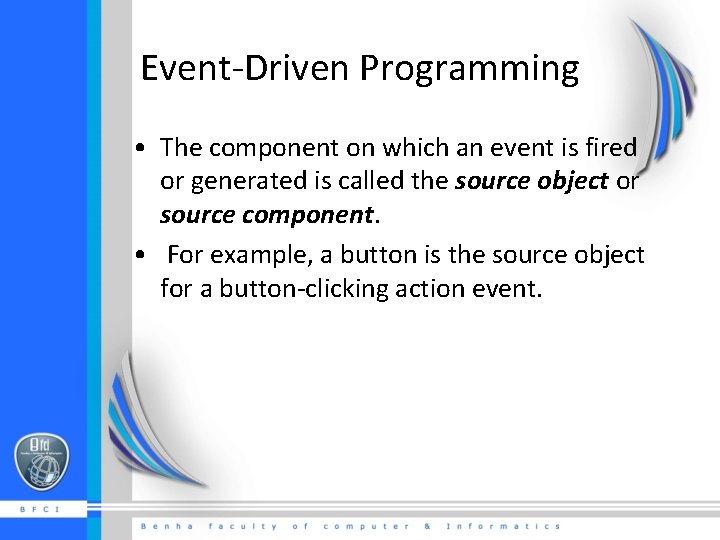
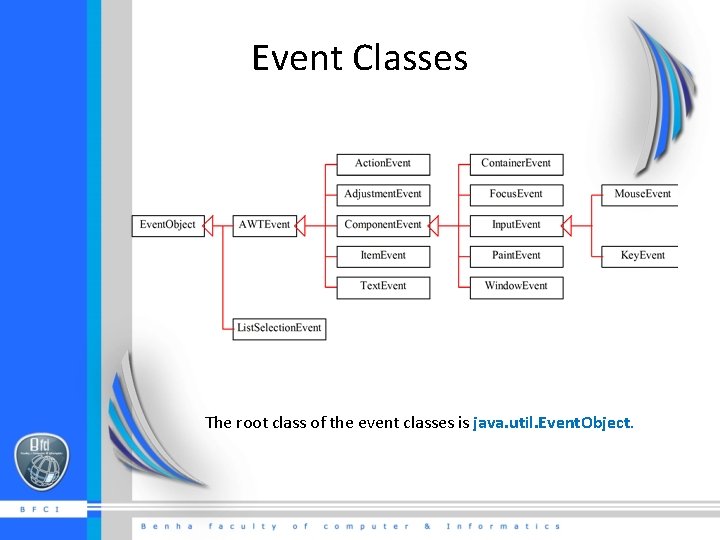
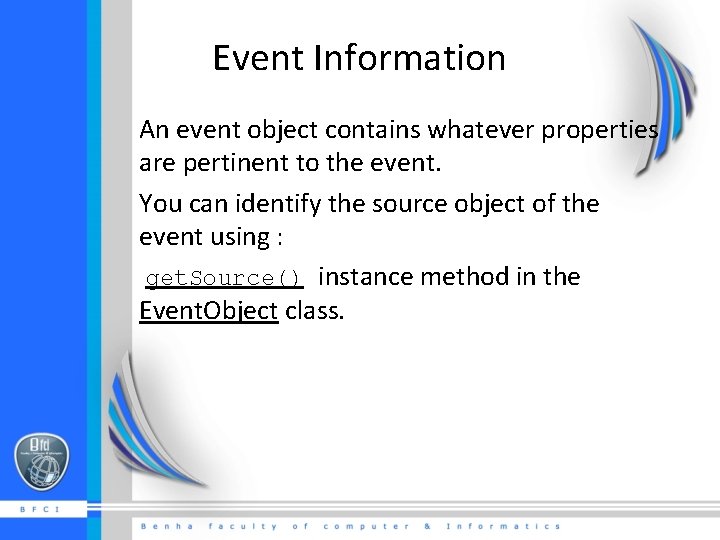
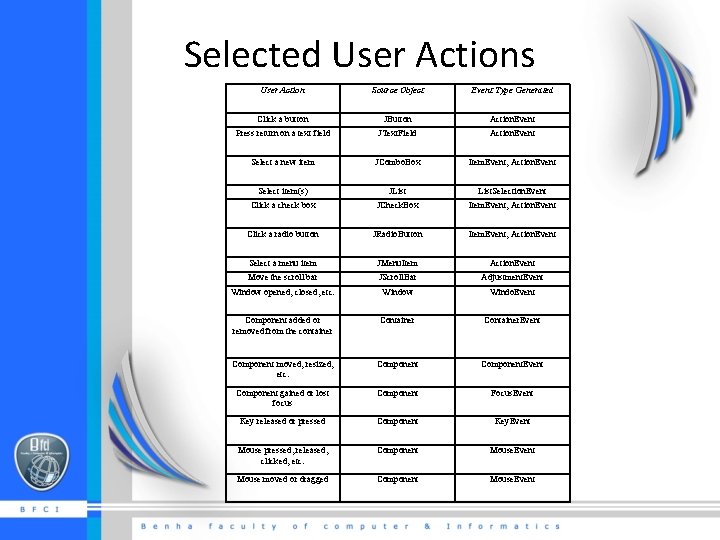
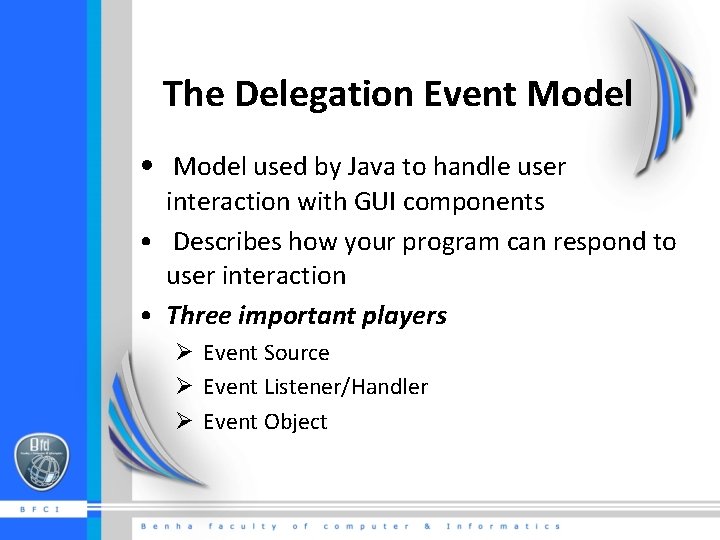
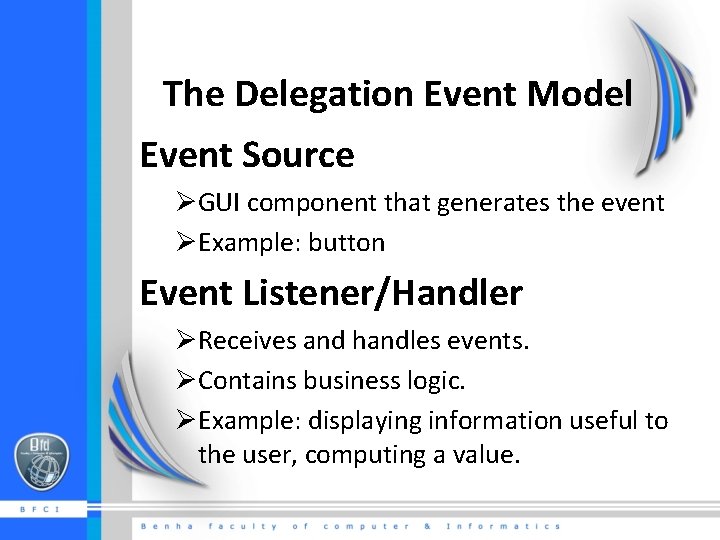
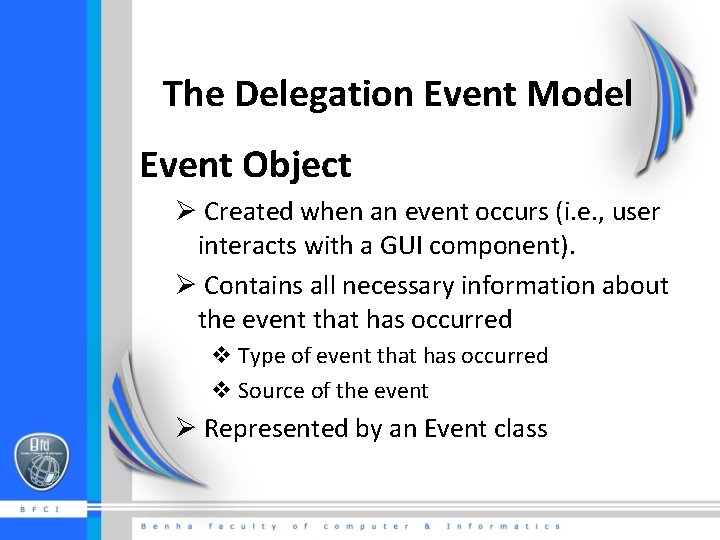
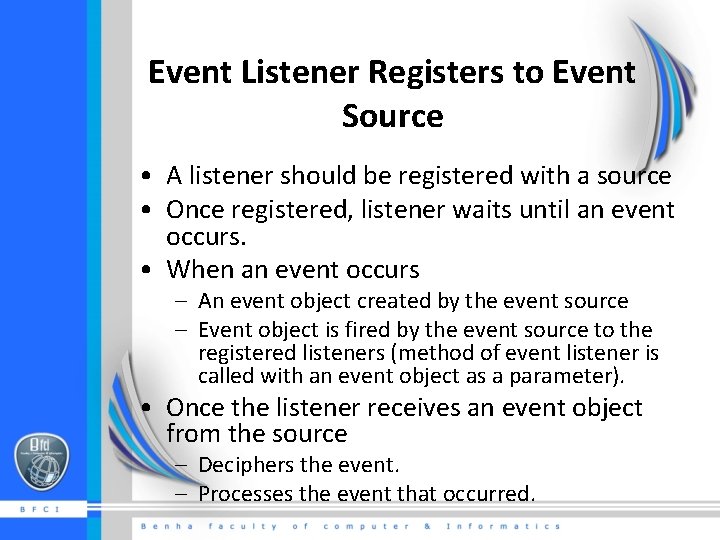
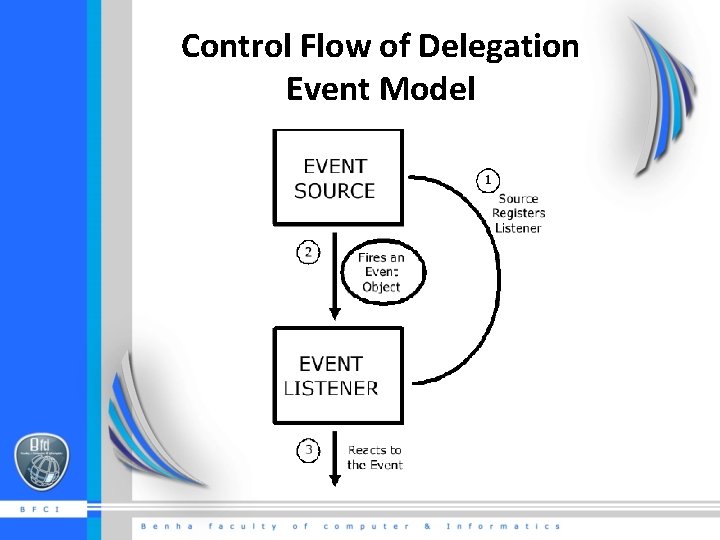
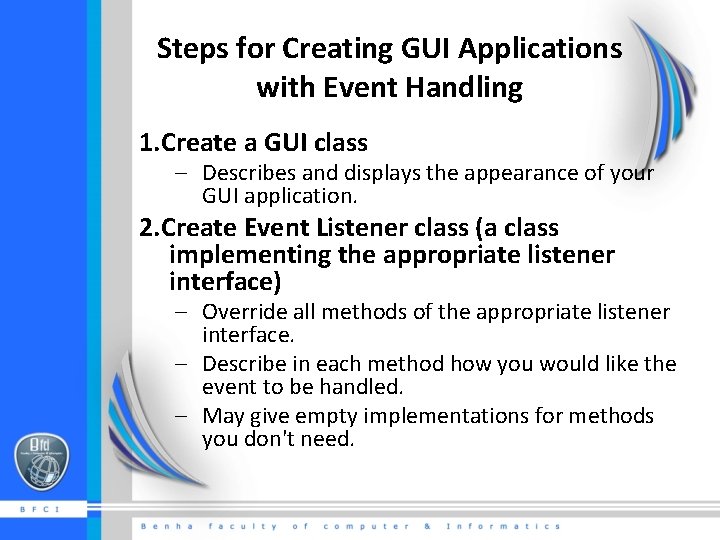
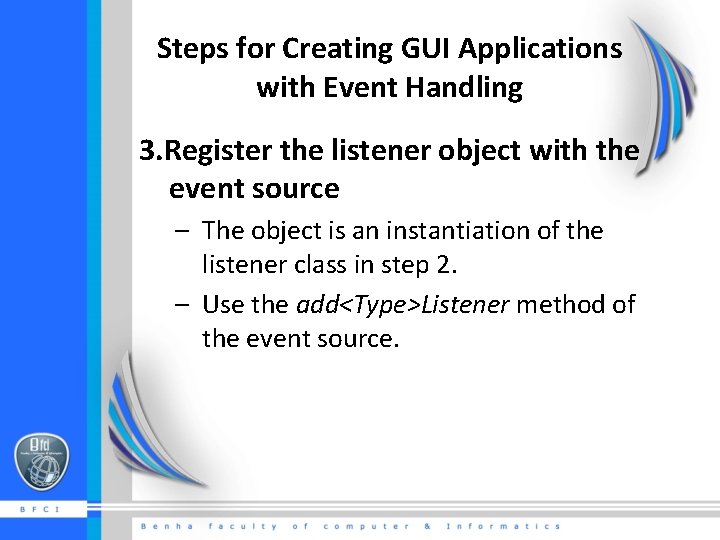
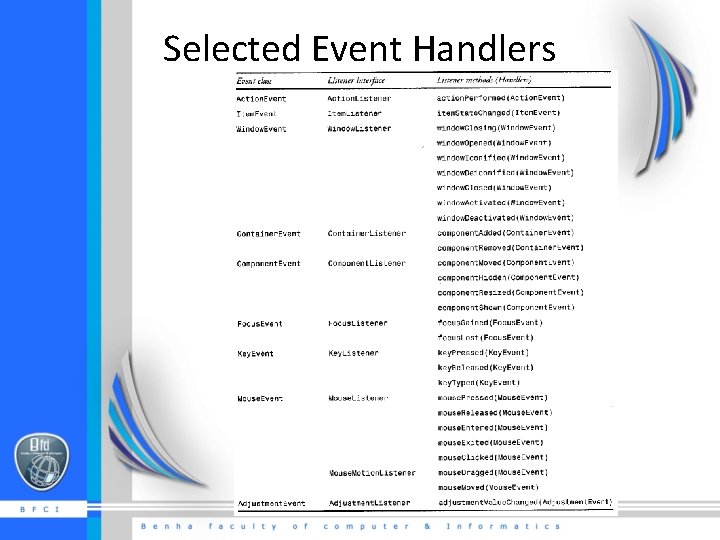
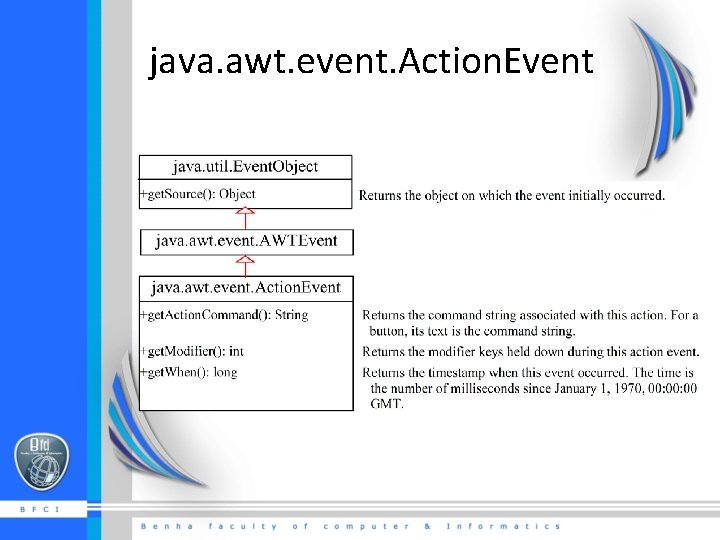
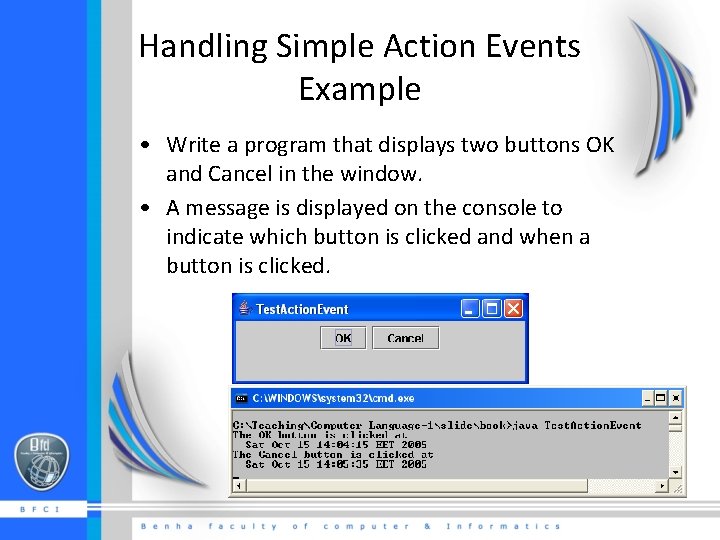
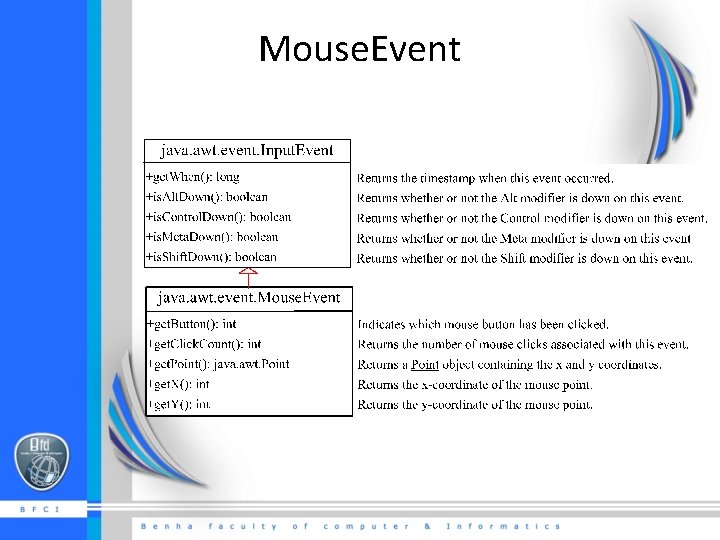
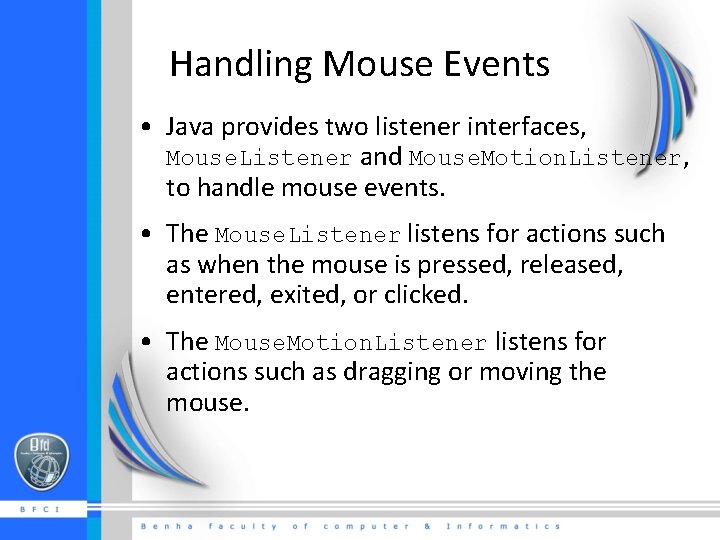
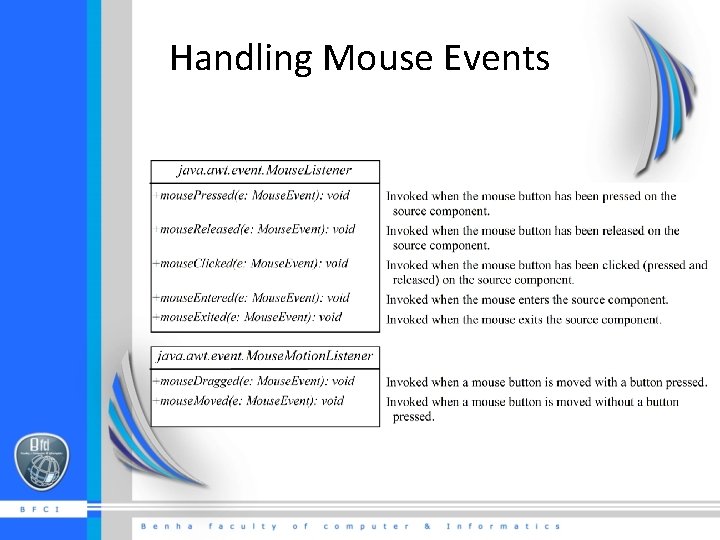
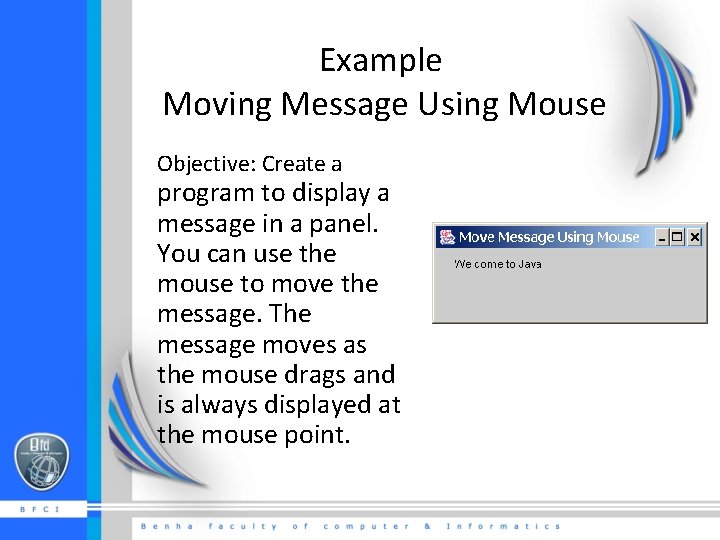
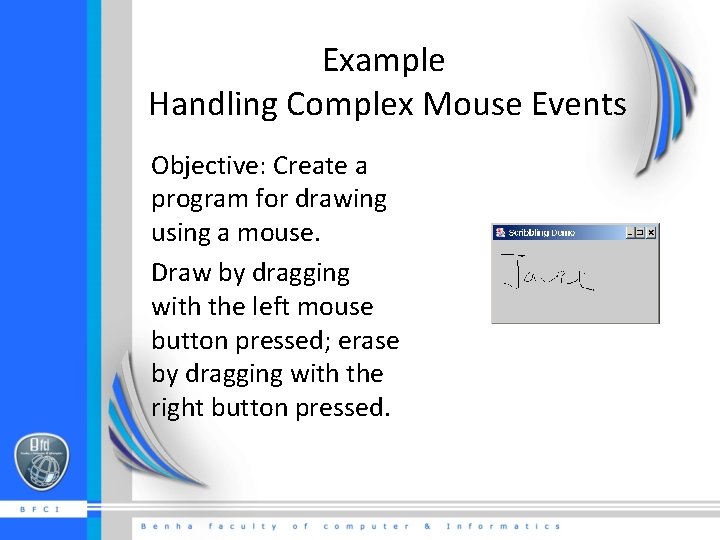
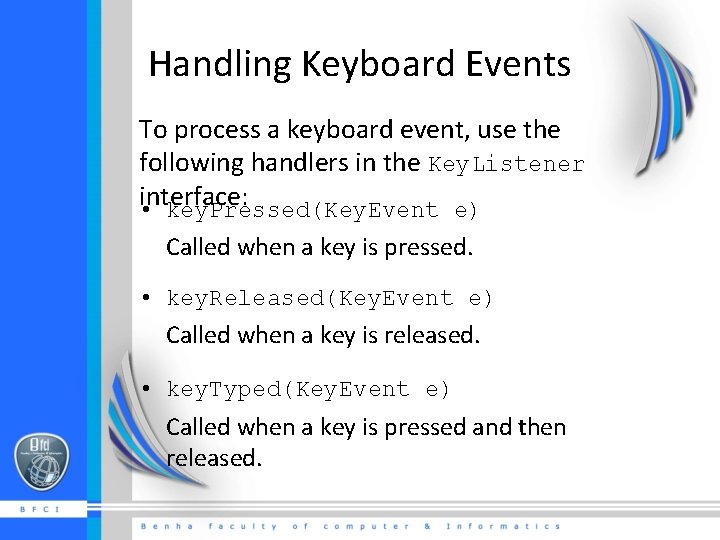
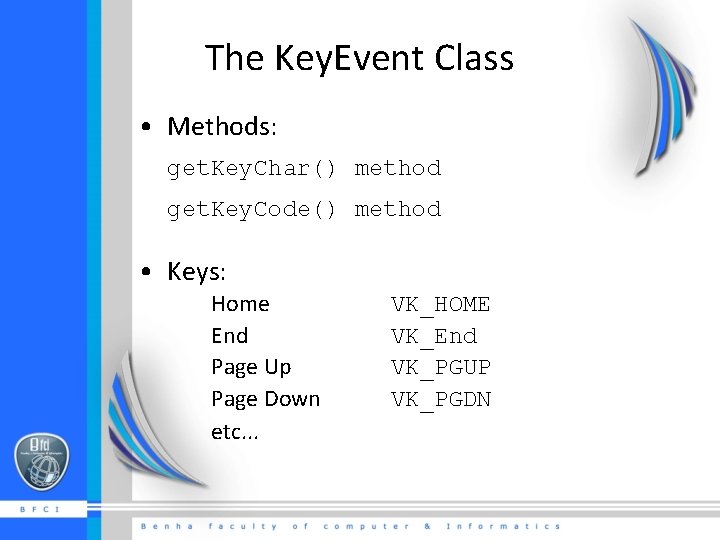
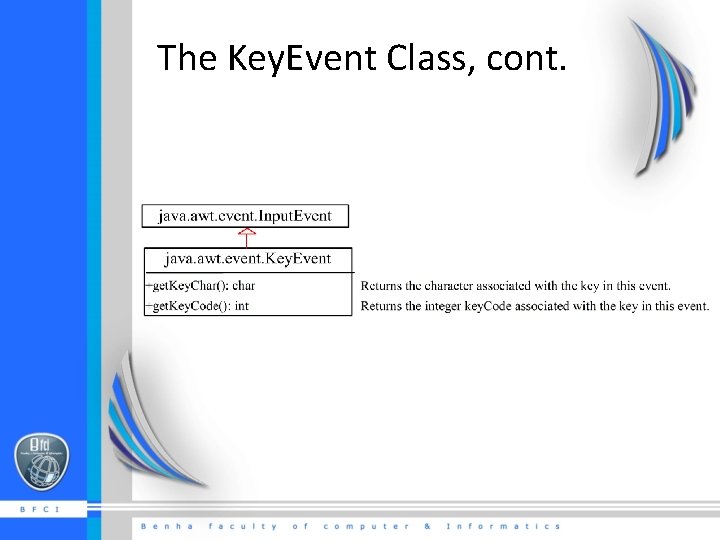
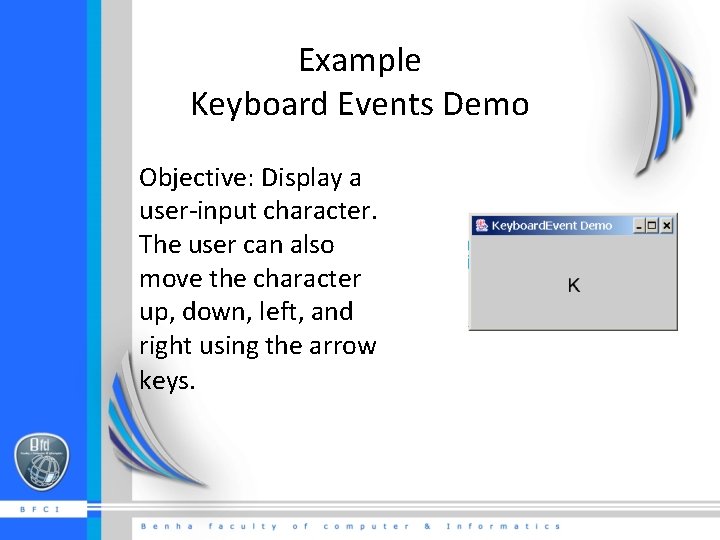
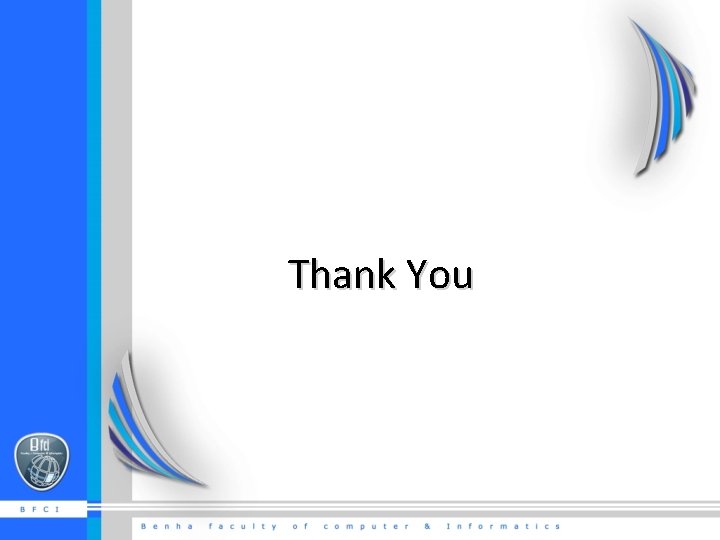
- Slides: 46
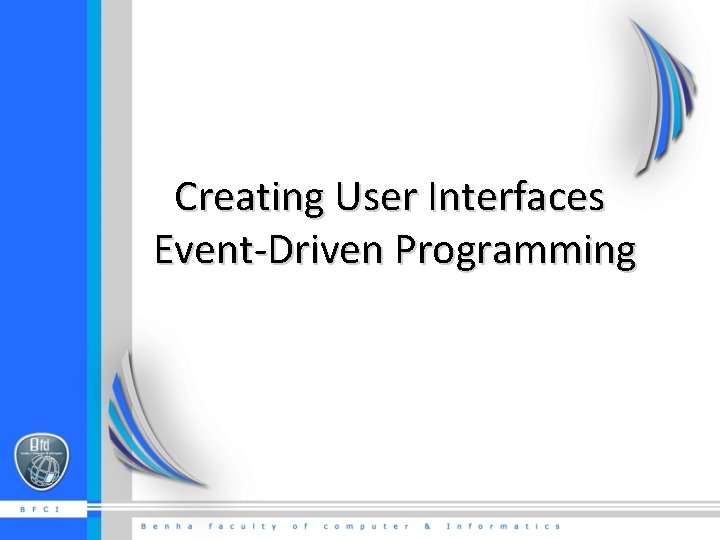
Creating User Interfaces Event-Driven Programming
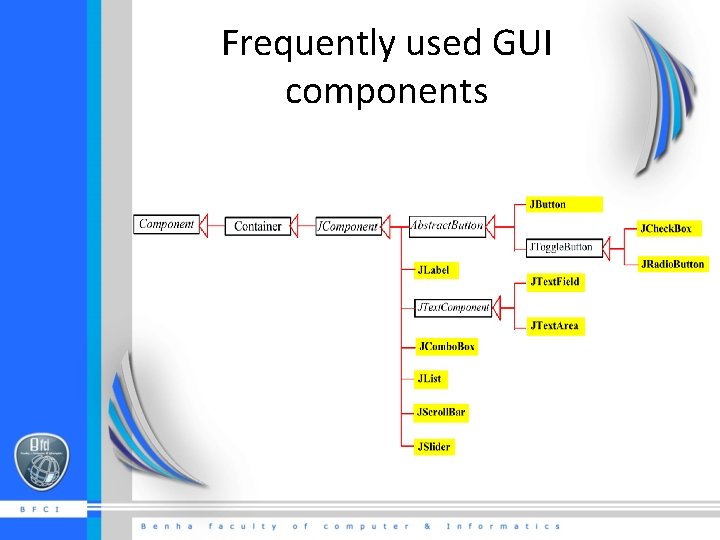
Frequently used GUI components
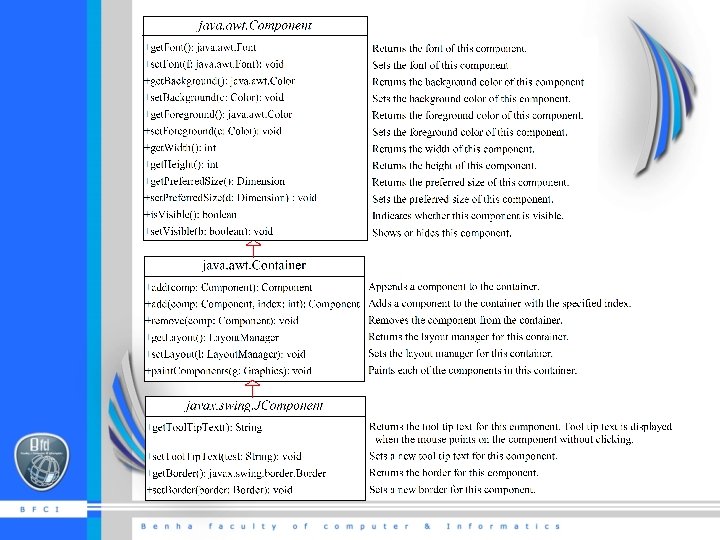
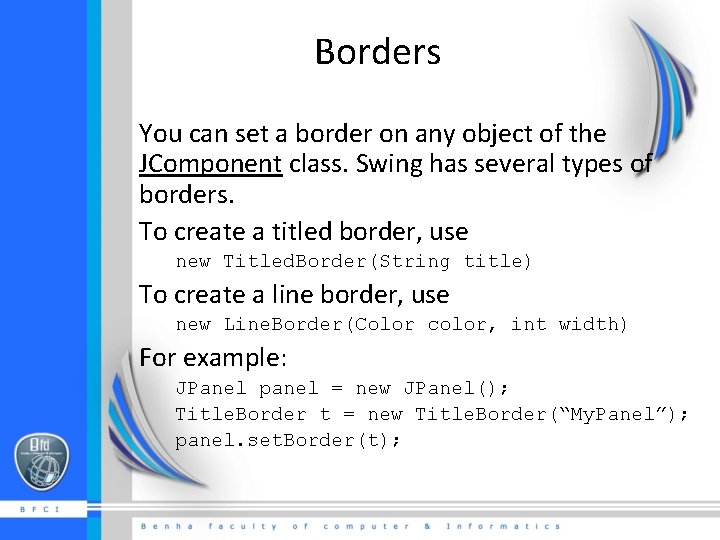
Borders You can set a border on any object of the JComponent class. Swing has several types of borders. To create a titled border, use new Titled. Border(String title) To create a line border, use new Line. Border(Color color, int width) For example: JPanel panel = new JPanel(); Title. Border t = new Title. Border(“My. Panel”); panel. set. Border(t);
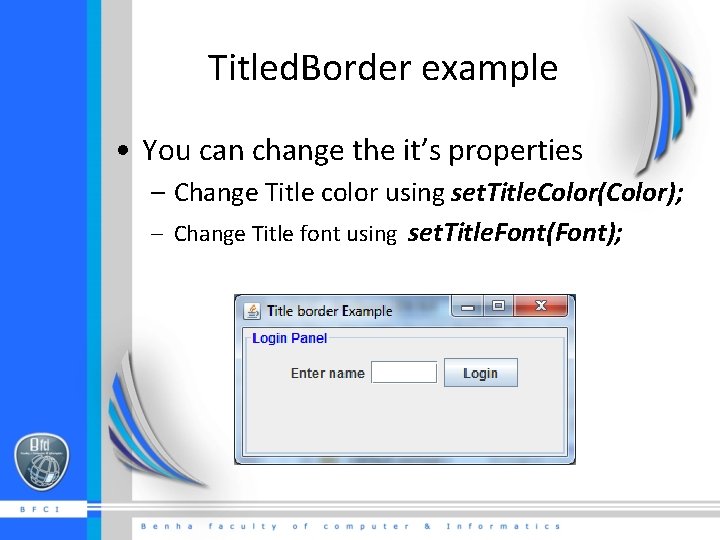
Titled. Border example • You can change the it’s properties – Change Title color using set. Title. Color(Color); – Change Title font using set. Title. Font(Font);
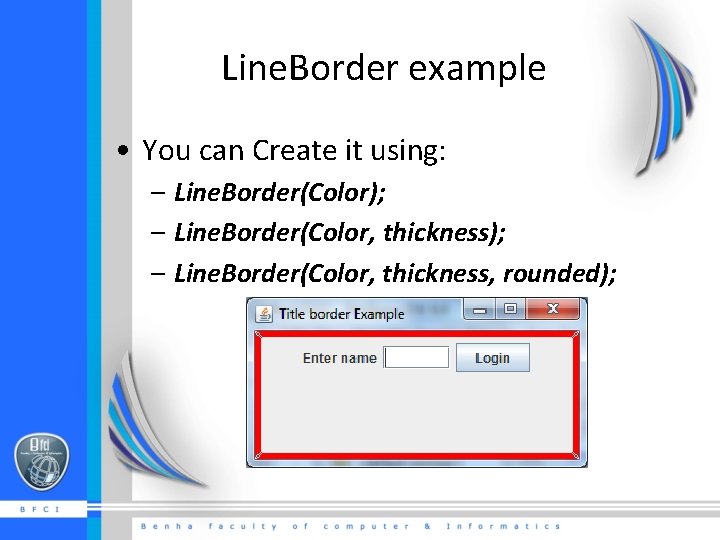
Line. Border example • You can Create it using: – Line. Border(Color); – Line. Border(Color, thickness, rounded);
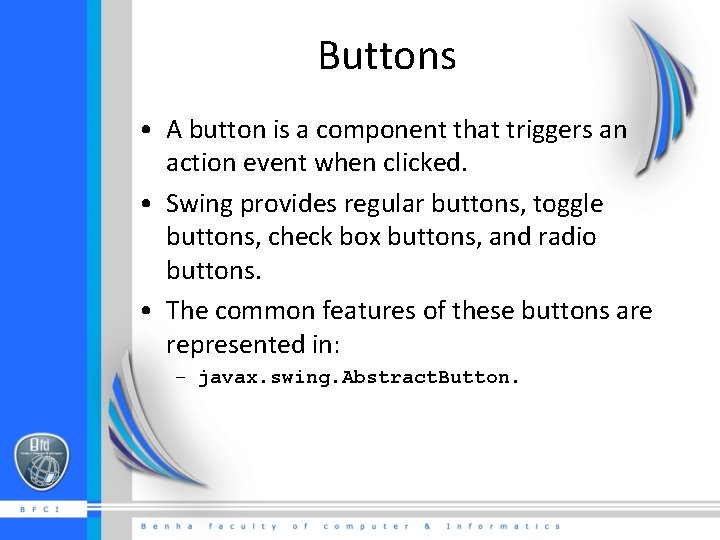
Buttons • A button is a component that triggers an action event when clicked. • Swing provides regular buttons, toggle buttons, check box buttons, and radio buttons. • The common features of these buttons are represented in: – javax. swing. Abstract. Button.
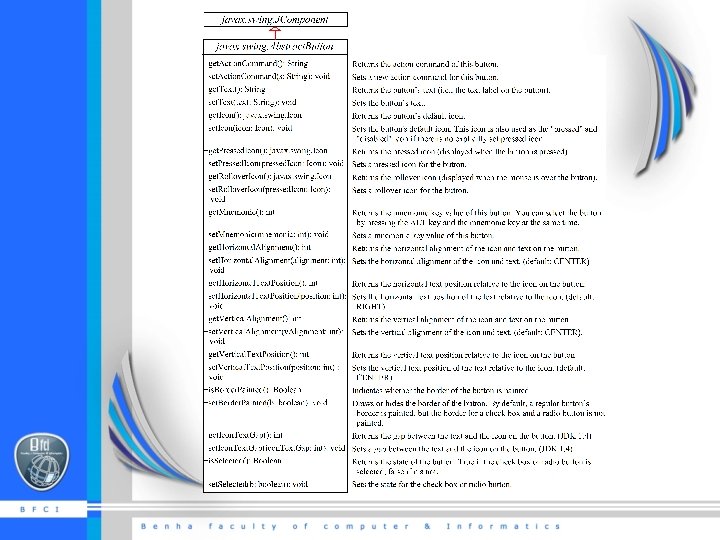
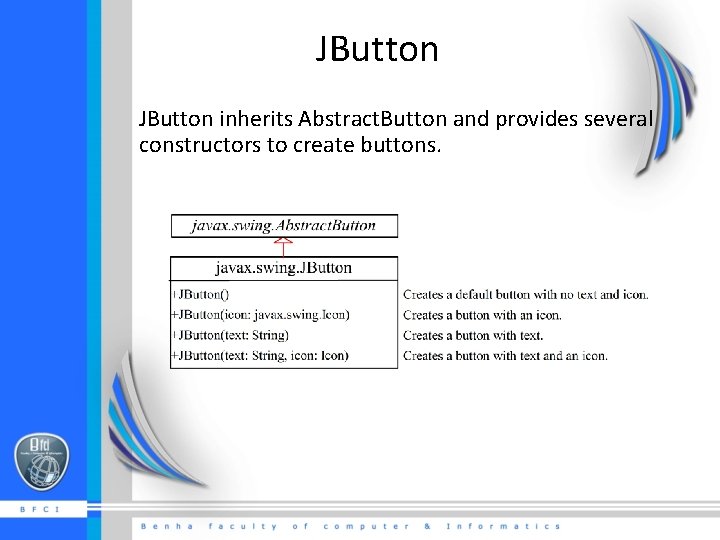
JButton inherits Abstract. Button and provides several constructors to create buttons.
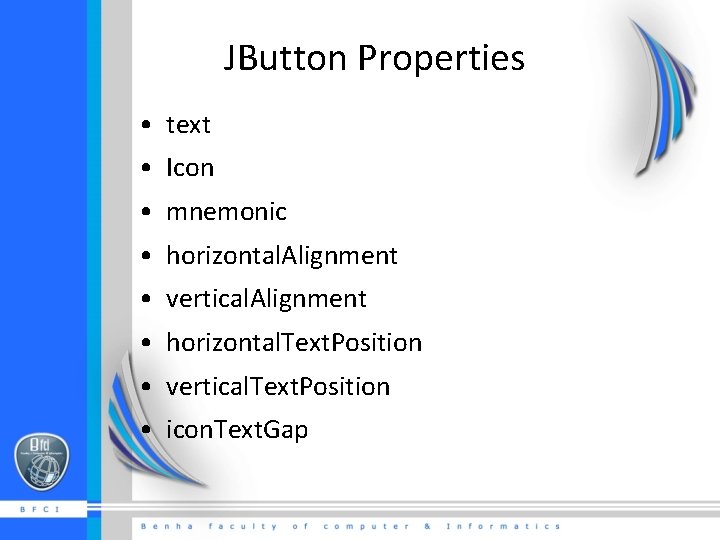
JButton Properties • text • Icon • mnemonic • horizontal. Alignment • vertical. Alignment • horizontal. Text. Position • vertical. Text. Position • icon. Text. Gap
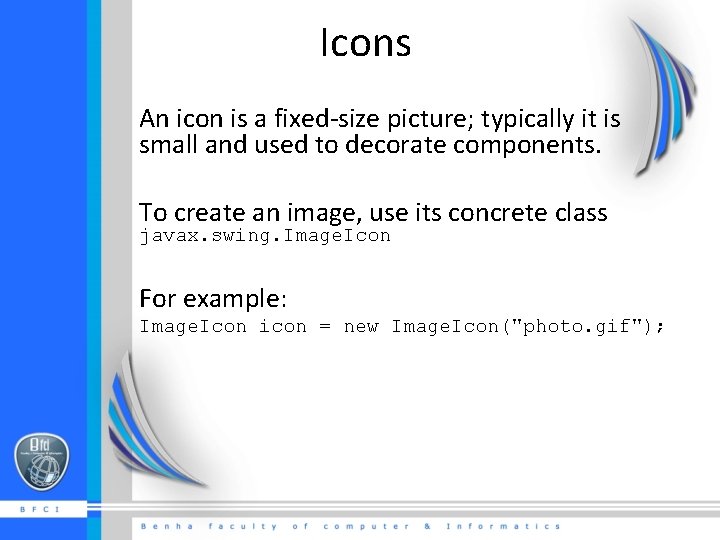
Icons An icon is a fixed-size picture; typically it is small and used to decorate components. To create an image, use its concrete class javax. swing. Image. Icon For example: Image. Icon icon = new Image. Icon("photo. gif");
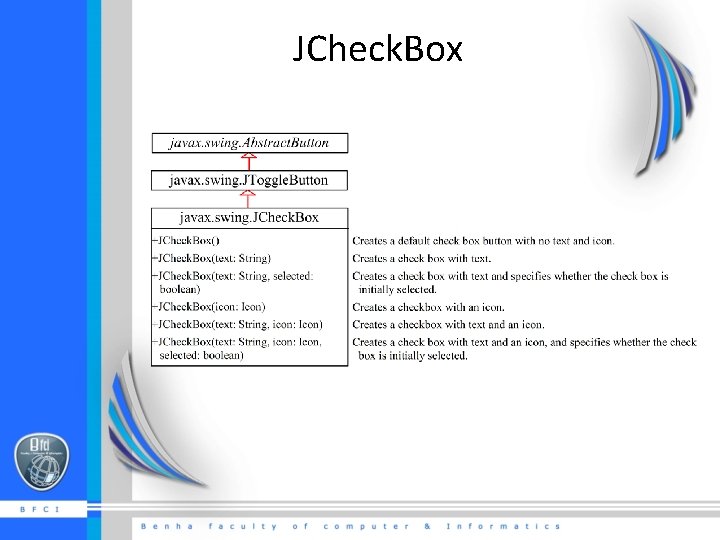
JCheck. Box
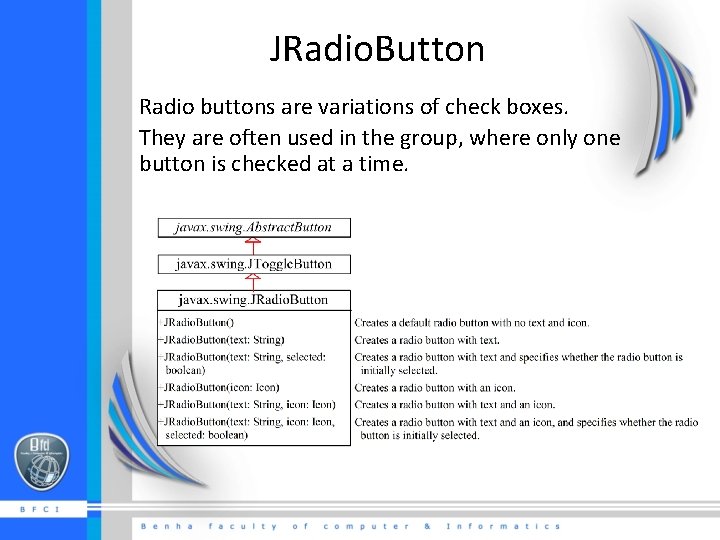
JRadio. Button Radio buttons are variations of check boxes. They are often used in the group, where only one button is checked at a time.
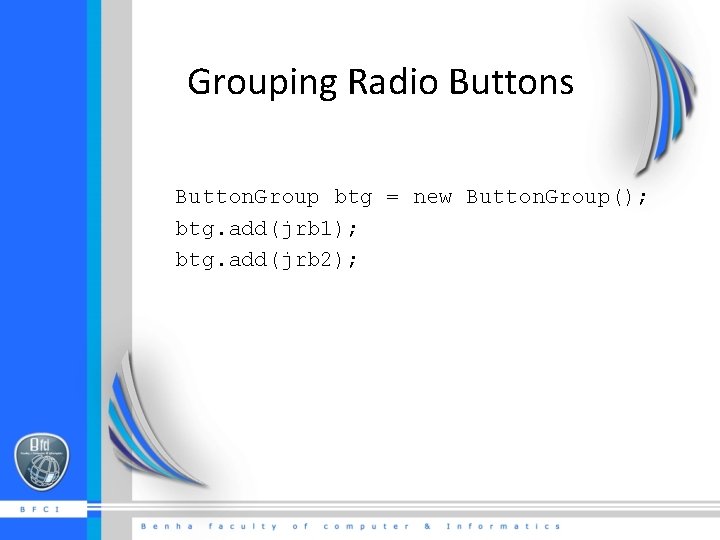
Grouping Radio Buttons Button. Group btg = new Button. Group(); btg. add(jrb 1); btg. add(jrb 2);
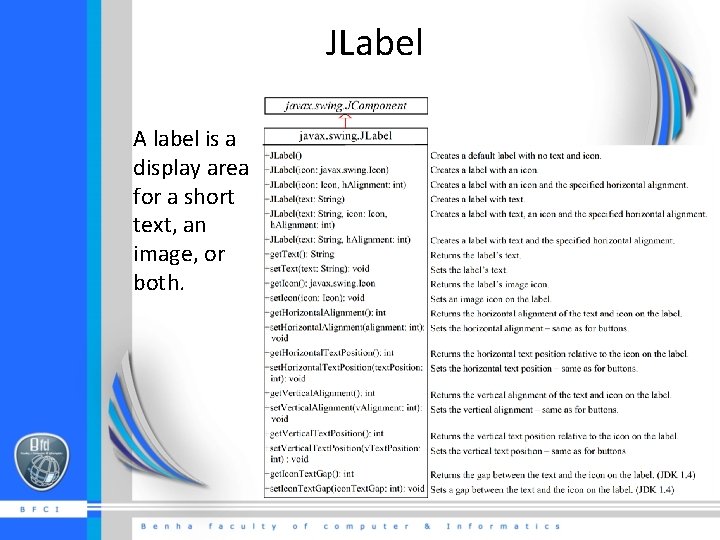
JLabel A label is a display area for a short text, an image, or both.
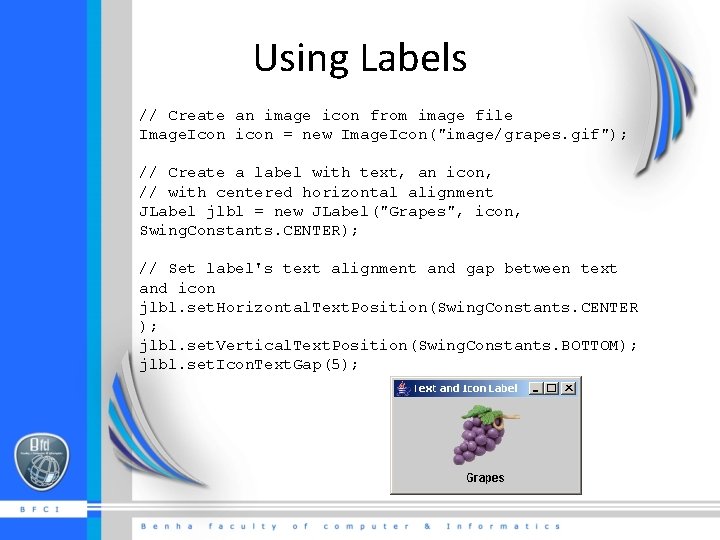
Using Labels // Create an image icon from image file Image. Icon icon = new Image. Icon("image/grapes. gif"); // Create a label with text, an icon, // with centered horizontal alignment JLabel jlbl = new JLabel("Grapes", icon, Swing. Constants. CENTER); // Set label's text alignment and gap between text and icon jlbl. set. Horizontal. Text. Position(Swing. Constants. CENTER ); jlbl. set. Vertical. Text. Position(Swing. Constants. BOTTOM); jlbl. set. Icon. Text. Gap(5);
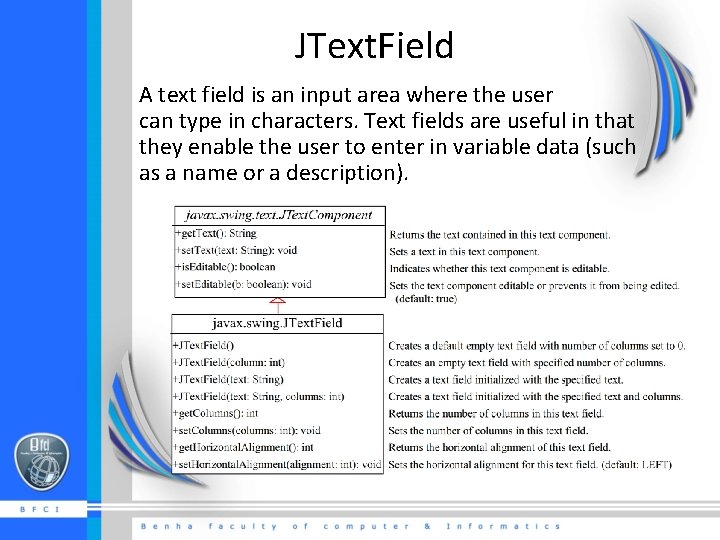
JText. Field A text field is an input area where the user can type in characters. Text fields are useful in that they enable the user to enter in variable data (such as a name or a description).
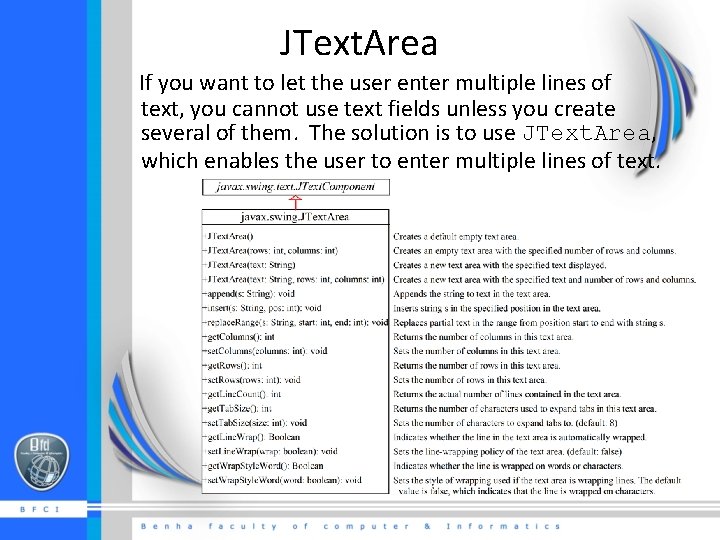
JText. Area If you want to let the user enter multiple lines of text, you cannot use text fields unless you create several of them. The solution is to use JText. Area, which enables the user to enter multiple lines of text.
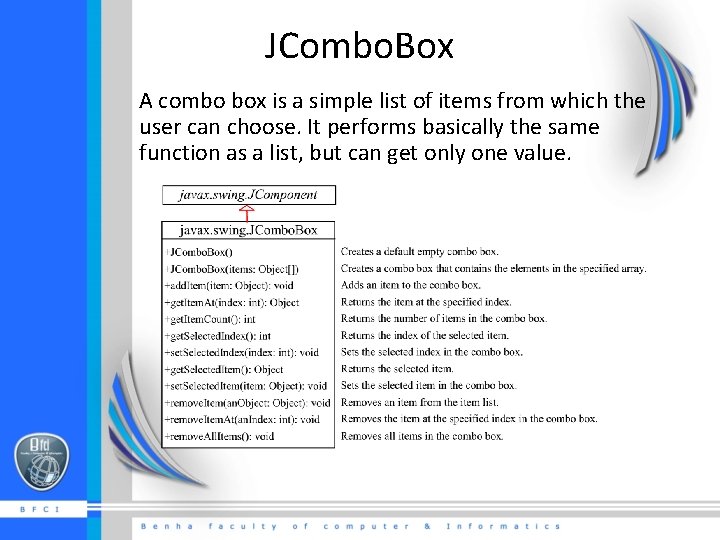
JCombo. Box A combo box is a simple list of items from which the user can choose. It performs basically the same function as a list, but can get only one value.
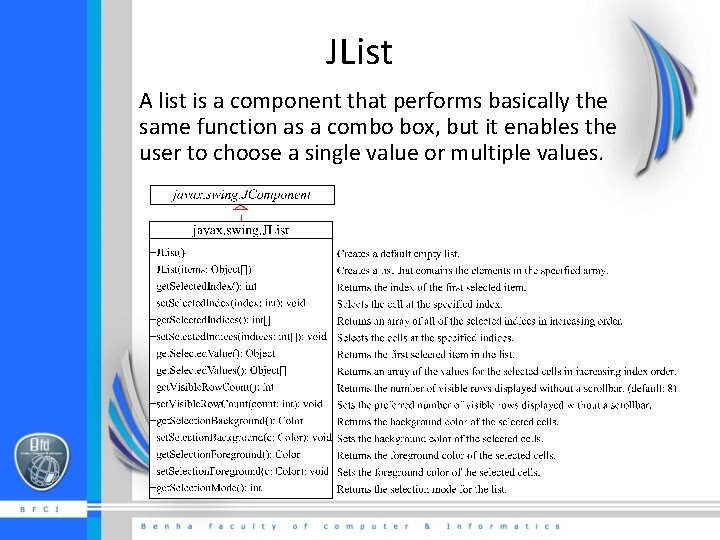
JList A list is a component that performs basically the same function as a combo box, but it enables the user to choose a single value or multiple values.
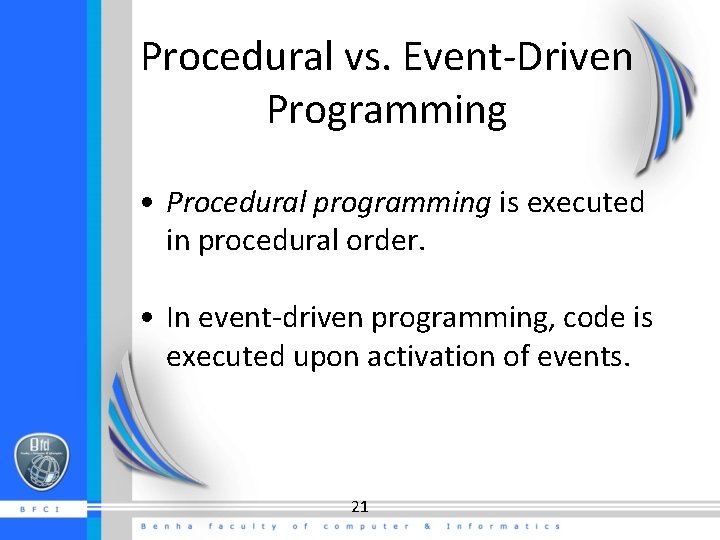
Procedural vs. Event-Driven Programming • Procedural programming is executed in procedural order. • In event-driven programming, code is executed upon activation of events. 21
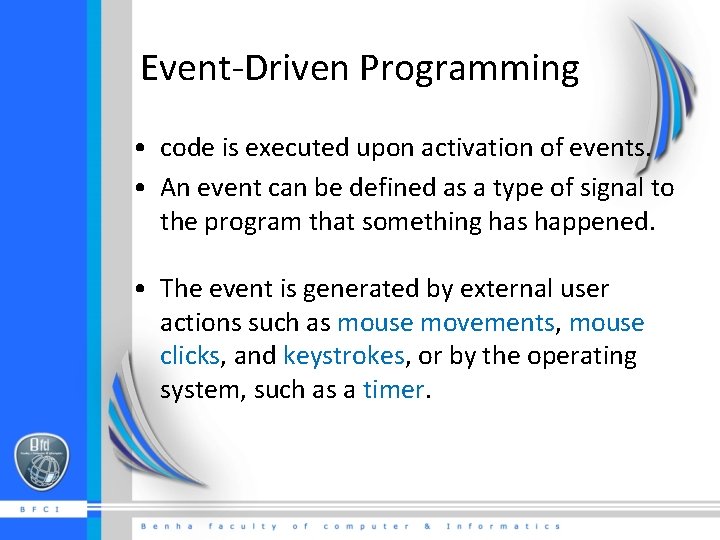
Event-Driven Programming • code is executed upon activation of events. • An event can be defined as a type of signal to the program that something has happened. • The event is generated by external user actions such as mouse movements, mouse clicks, and keystrokes, or by the operating system, such as a timer.
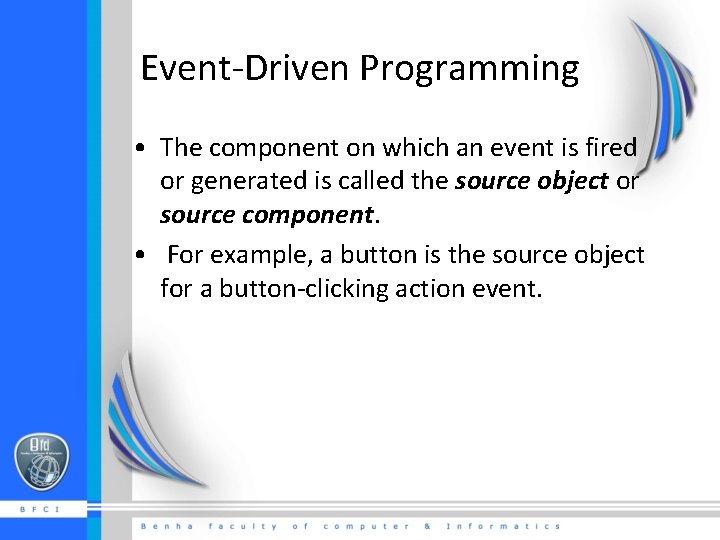
Event-Driven Programming • The component on which an event is fired or generated is called the source object or source component. • For example, a button is the source object for a button-clicking action event.
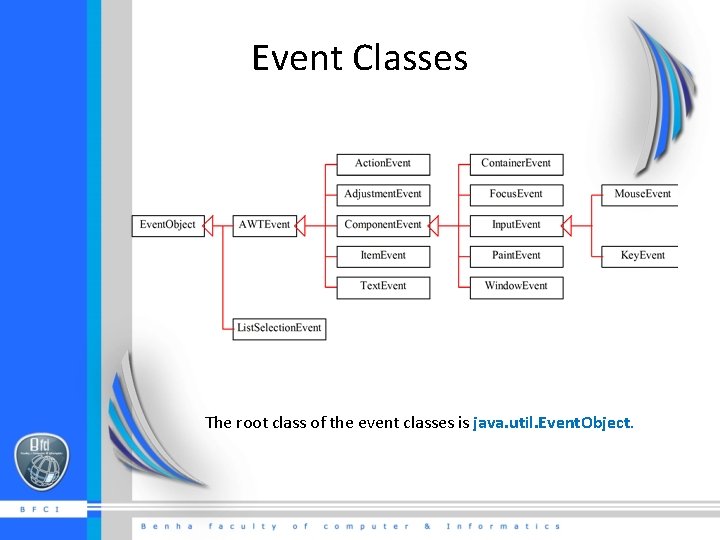
Event Classes The root class of the event classes is java. util. Event. Object.
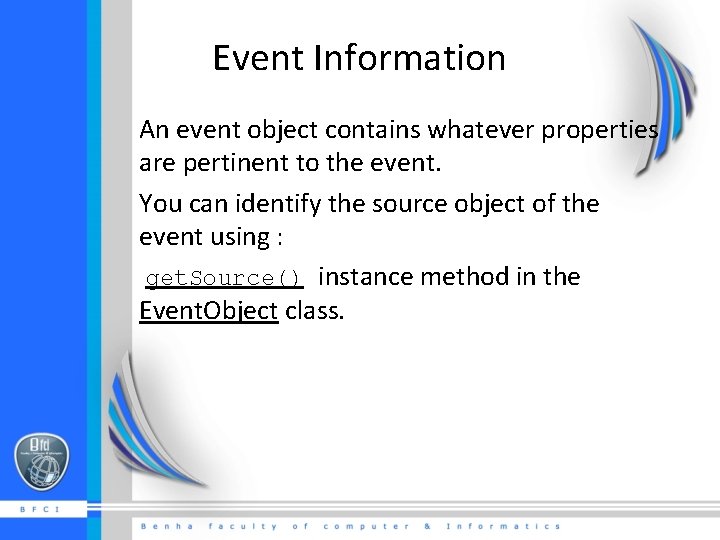
Event Information An event object contains whatever properties are pertinent to the event. You can identify the source object of the event using : get. Source() instance method in the Event. Object class.
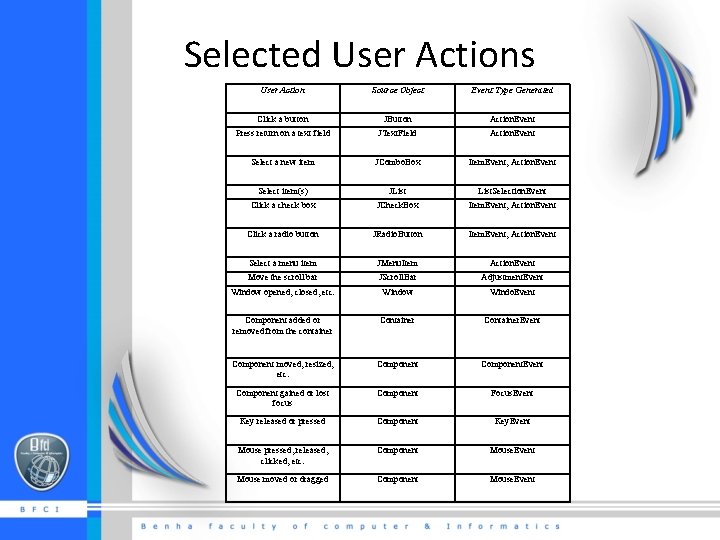
Selected User Actions User Action Source Object Event Type Generated Click a button JButton Action. Event Press return on a text field JText. Field Action. Event Select a new item JCombo. Box Item. Event, Action. Event Select item(s) JList. Selection. Event Click a check box JCheck. Box Item. Event, Action. Event Click a radio button JRadio. Button Item. Event, Action. Event Select a menu item JMenu. Item Action. Event Move the scroll bar JScroll. Bar Adjustment. Event Window opened, closed, etc. Window Windo. Event Component added or removed from the container Container. Event Component moved, resized, etc. Component. Event Component gained or lost focus Component Focus. Event Key released or pressed Component Key. Event Mouse pressed, released, clicked, etc. Component Mouse. Event Mouse moved or dragged Component Mouse. Event
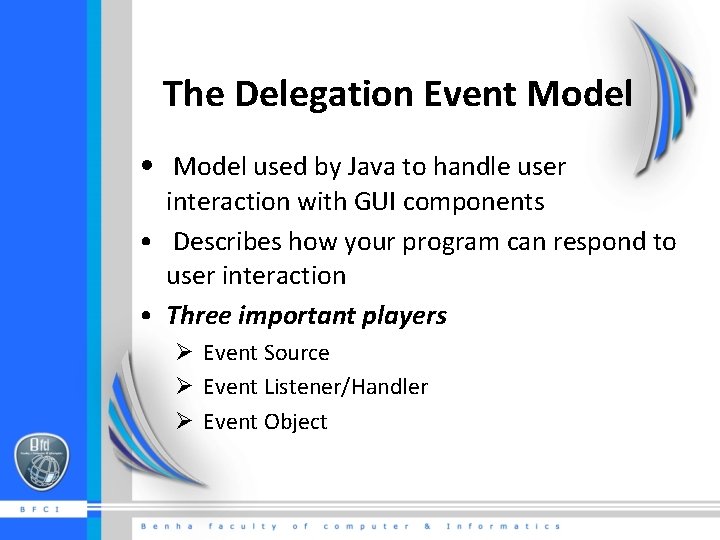
The Delegation Event Model • Model used by Java to handle user interaction with GUI components • Describes how your program can respond to user interaction • Three important players Ø Event Source Ø Event Listener/Handler Ø Event Object
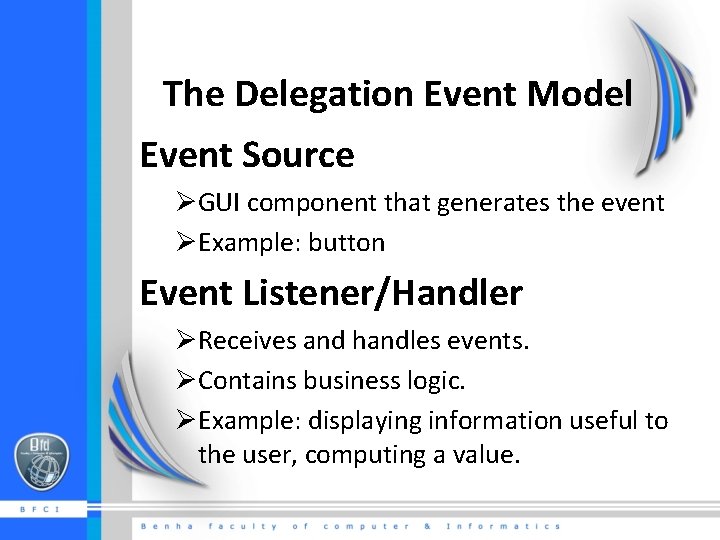
The Delegation Event Model Event Source ØGUI component that generates the event ØExample: button Event Listener/Handler ØReceives and handles events. ØContains business logic. ØExample: displaying information useful to the user, computing a value.
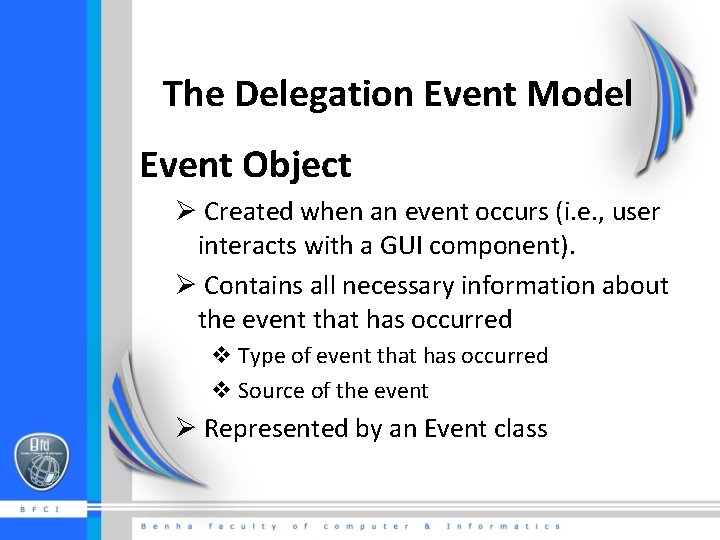
The Delegation Event Model Event Object Ø Created when an event occurs (i. e. , user interacts with a GUI component). Ø Contains all necessary information about the event that has occurred v Type of event that has occurred v Source of the event Ø Represented by an Event class
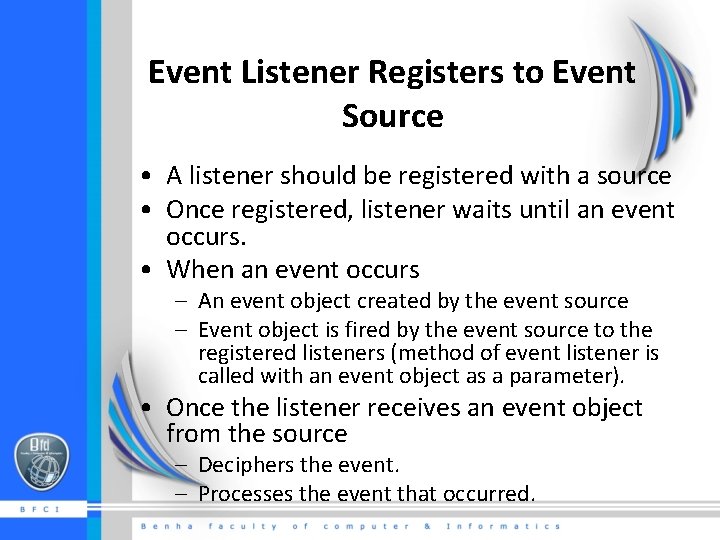
Event Listener Registers to Event Source • A listener should be registered with a source • Once registered, listener waits until an event occurs. • When an event occurs – An event object created by the event source – Event object is fired by the event source to the registered listeners (method of event listener is called with an event object as a parameter). • Once the listener receives an event object from the source – Deciphers the event. – Processes the event that occurred.
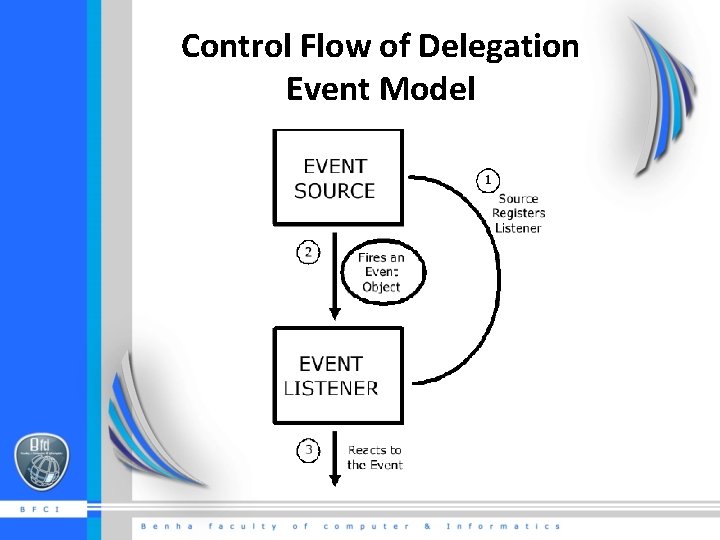
Control Flow of Delegation Event Model
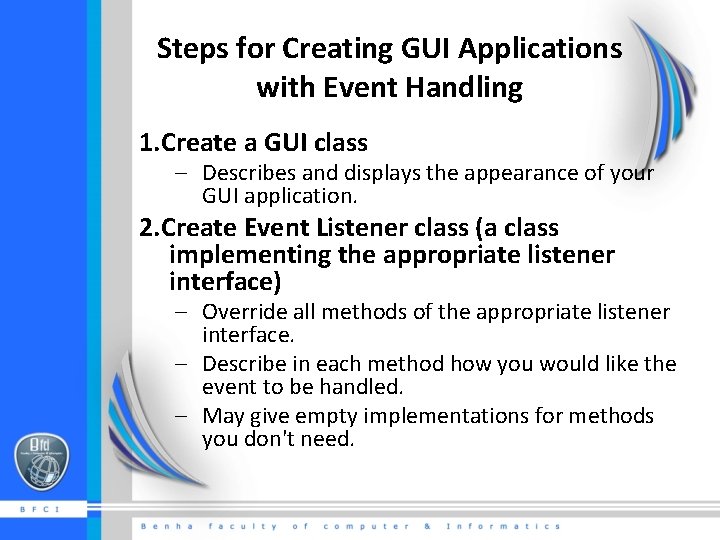
Steps for Creating GUI Applications with Event Handling 1. Create a GUI class – Describes and displays the appearance of your GUI application. 2. Create Event Listener class (a class implementing the appropriate listener interface) – Override all methods of the appropriate listener interface. – Describe in each method how you would like the event to be handled. – May give empty implementations for methods you don't need.
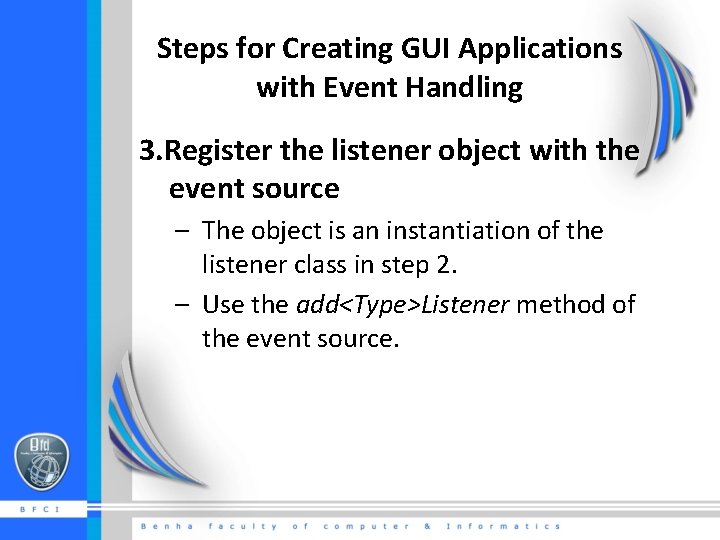
Steps for Creating GUI Applications with Event Handling 3. Register the listener object with the event source – The object is an instantiation of the listener class in step 2. – Use the add<Type>Listener method of the event source.
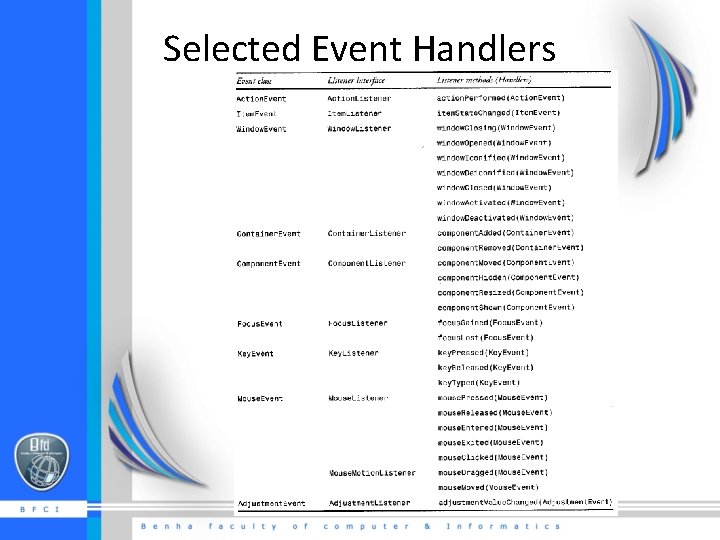
Selected Event Handlers
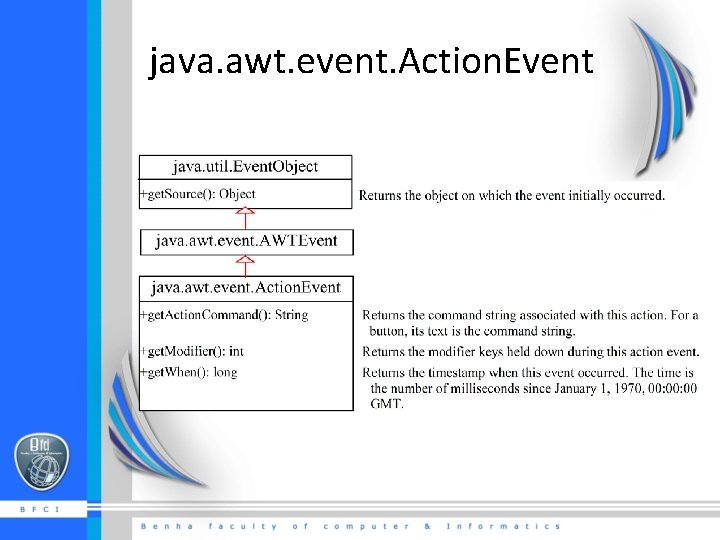
java. awt. event. Action. Event
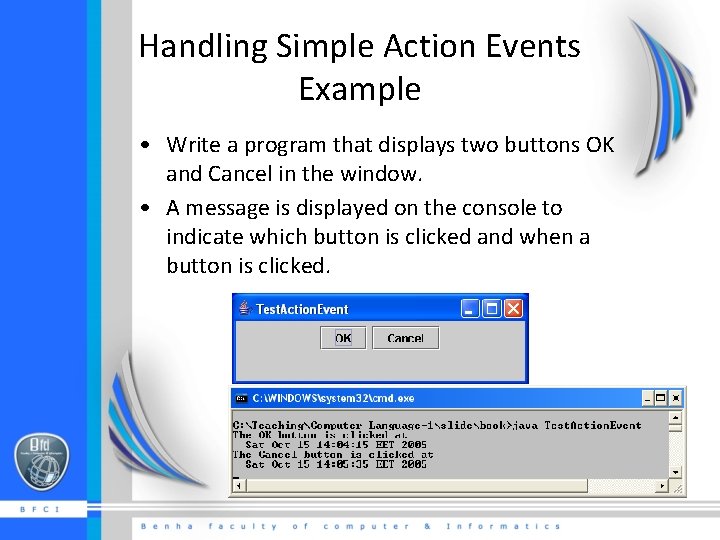
Handling Simple Action Events Example • Write a program that displays two buttons OK and Cancel in the window. • A message is displayed on the console to indicate which button is clicked and when a button is clicked.
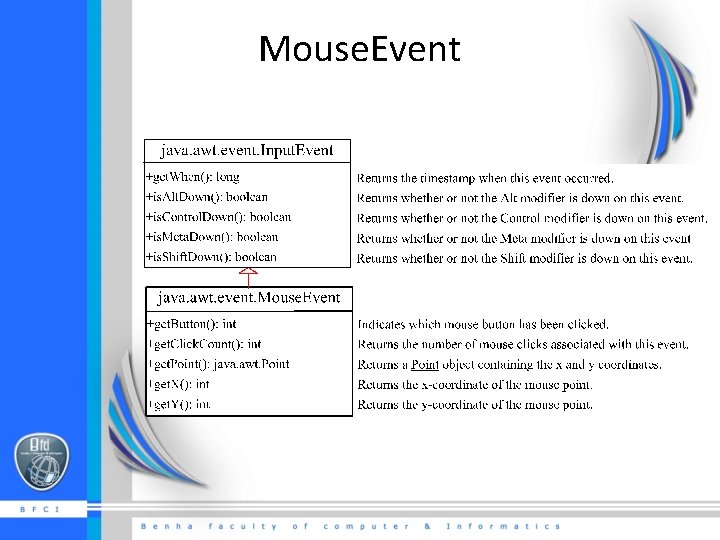
Mouse. Event
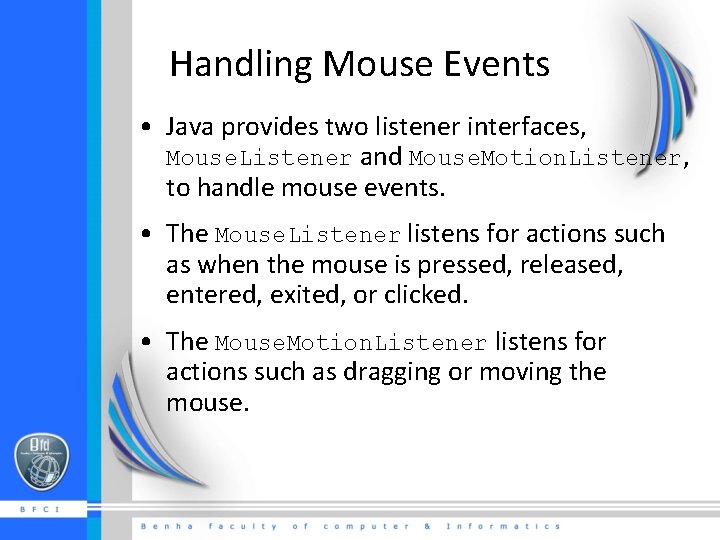
Handling Mouse Events • Java provides two listener interfaces, Mouse. Listener and Mouse. Motion. Listener, to handle mouse events. • The Mouse. Listener listens for actions such as when the mouse is pressed, released, entered, exited, or clicked. • The Mouse. Motion. Listener listens for actions such as dragging or moving the mouse.
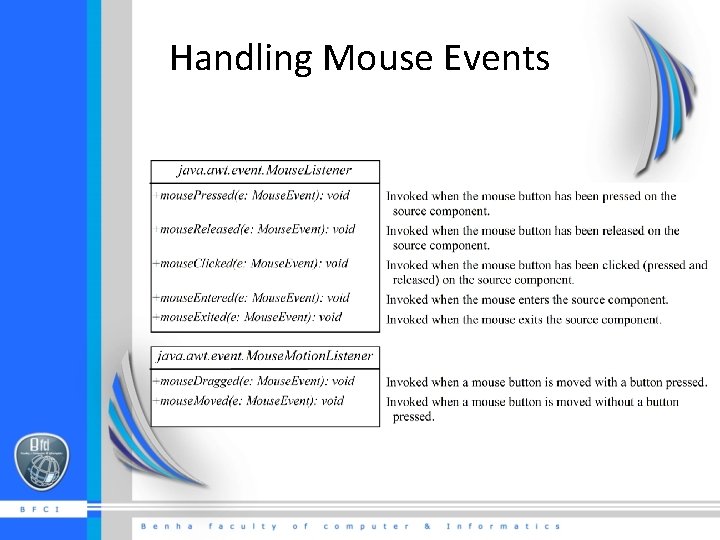
Handling Mouse Events
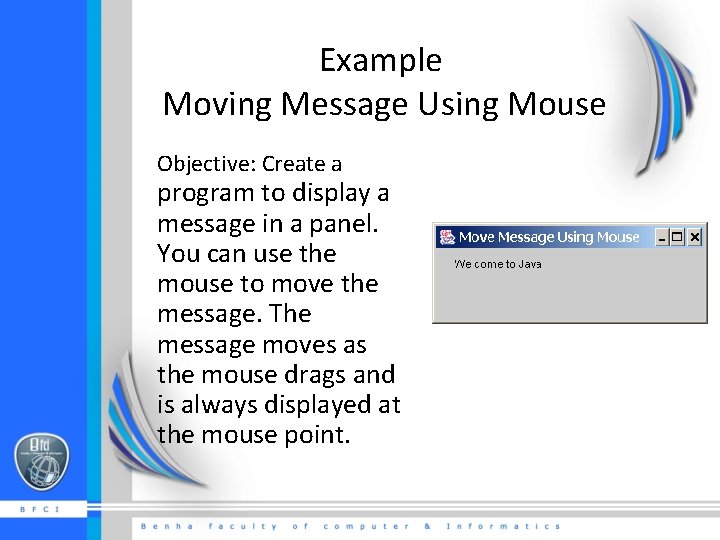
Example Moving Message Using Mouse Objective: Create a program to display a message in a panel. You can use the mouse to move the message. The message moves as the mouse drags and is always displayed at the mouse point.
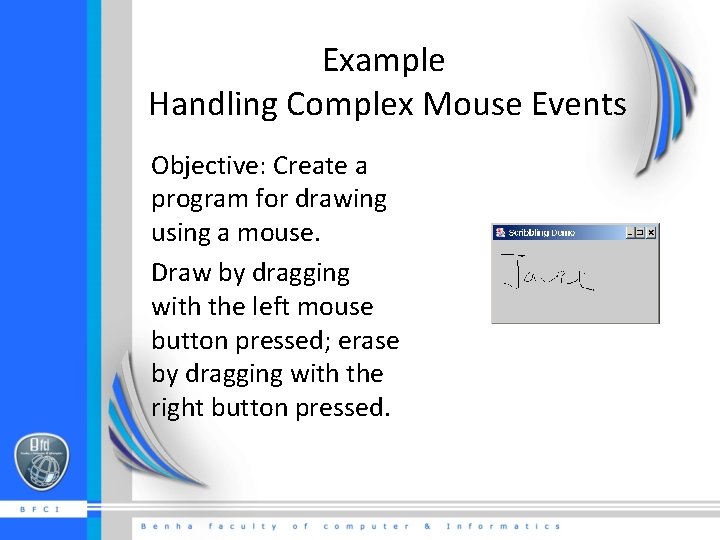
Example Handling Complex Mouse Events Objective: Create a program for drawing using a mouse. Draw by dragging with the left mouse button pressed; erase by dragging with the right button pressed.
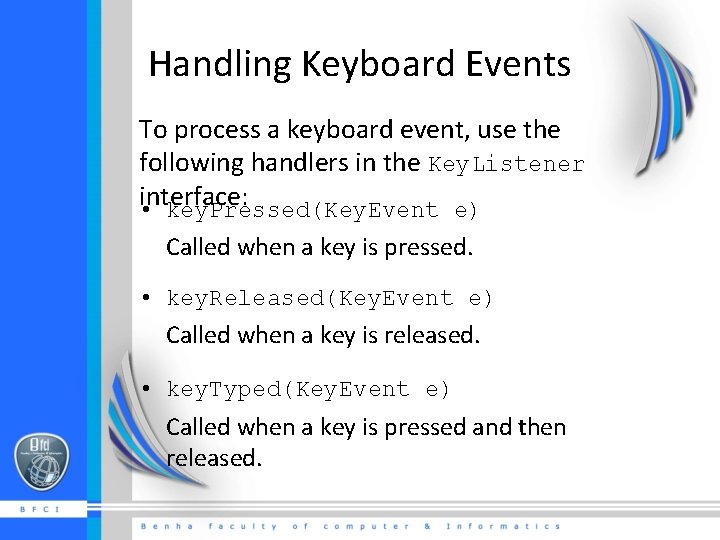
Handling Keyboard Events To process a keyboard event, use the following handlers in the Key. Listener interface: • key. Pressed(Key. Event e) Called when a key is pressed. • key. Released(Key. Event e) Called when a key is released. • key. Typed(Key. Event e) Called when a key is pressed and then released.
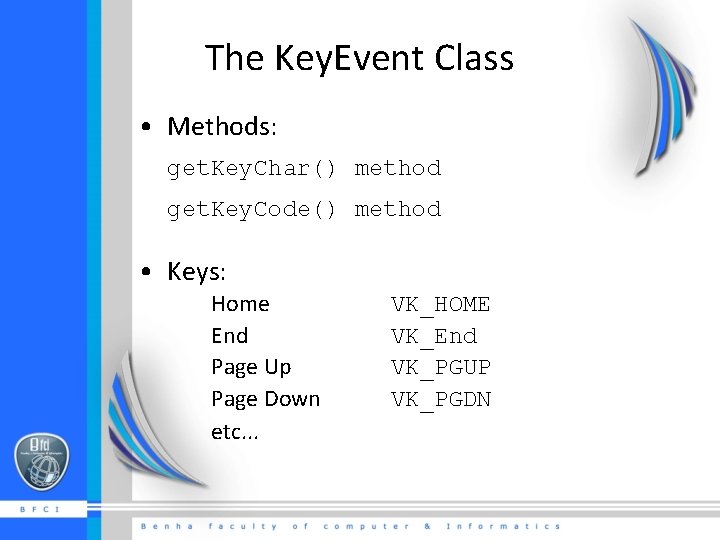
The Key. Event Class • Methods: get. Key. Char() method get. Key. Code() method • Keys: Home End Page Up Page Down etc. . . VK_HOME VK_End VK_PGUP VK_PGDN
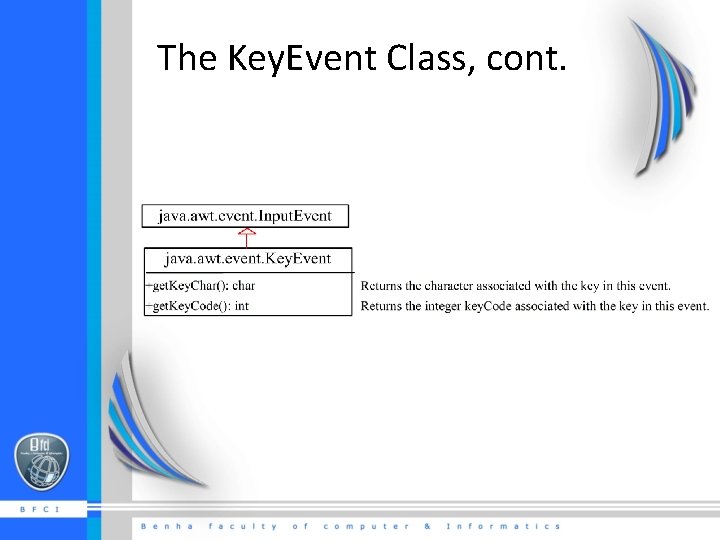
The Key. Event Class, cont.
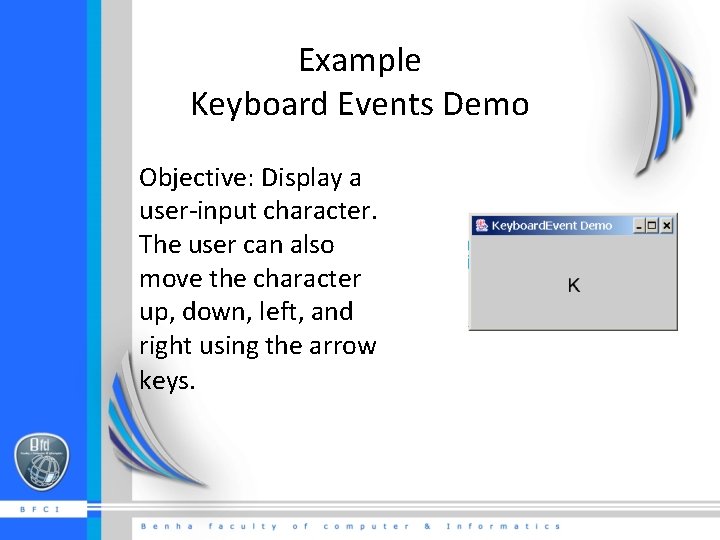
Example Keyboard Events Demo Objective: Display a user-input character. The user can also move the character up, down, left, and right using the arrow keys.
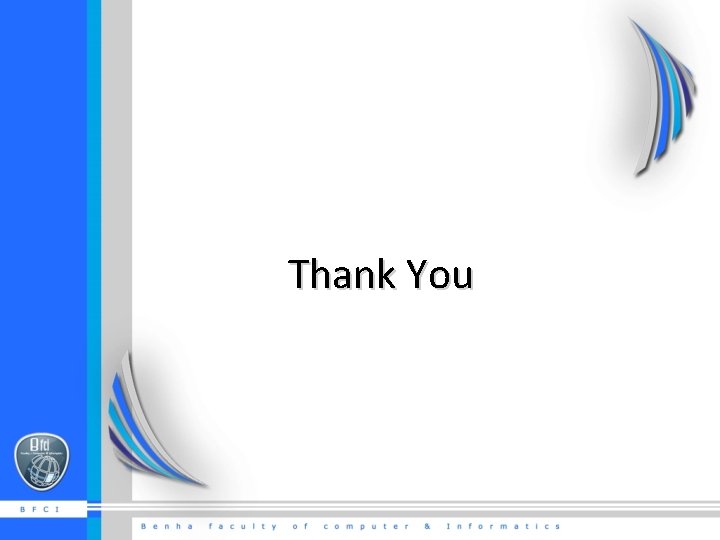
Thank You
Java gui for r
Common gui event types and listener interfaces in java
Web user interface
Why are user interfaces hard to implement
Design heuristic
User interfaces design dc
Ge gi gue gui güe güi
Uml interfaces are used to:
Fifo page replacement calculator
Florida technology integration matrix
Gui vs web interface
Java gui toolkit
Linux gui programming
Rapid gui programming with python and qt
Single user and multiple user operating system
Single user and multiple user operating system
User requirements are expressed as in extreme programming
Expressive interface
Office interface vs industrial interface
What is difference between abstract class and interface
Difference between abstract class and interface
Expressive interface
Interface and dialogue design
Blueprint interfaces
Which is not an objective of designing interfaces?
Property management system interfaces
Examples of colloids
Similar to
Joe hogan openet
Designing web interfaces in hci
Gsm um
Expressive interfaces
Srs prototype outline
Team interfaces
Micros e interfaces
Sdi vs mdi
Interfaces inteligentes
Abstract classes in java
What is interface
F-14
Communication interface in embedded systems
How to answer how often
An action that was frequently repeated in the past
Always often sometimes rarely never
Why was john proctor frequently absent from church?
Paraphrase example
Hipaa frequently asked questions