Functions in Python Function A function is a
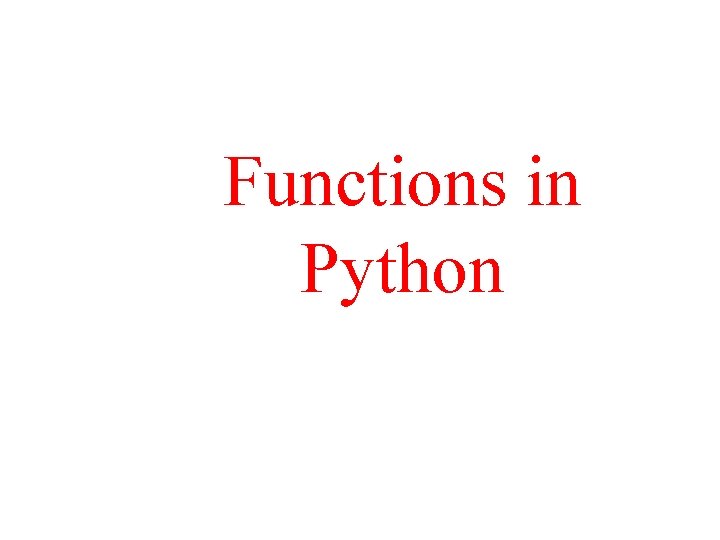
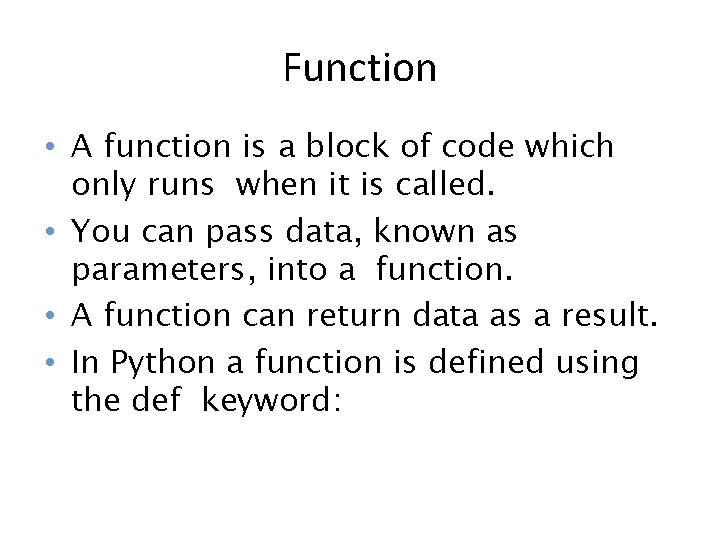
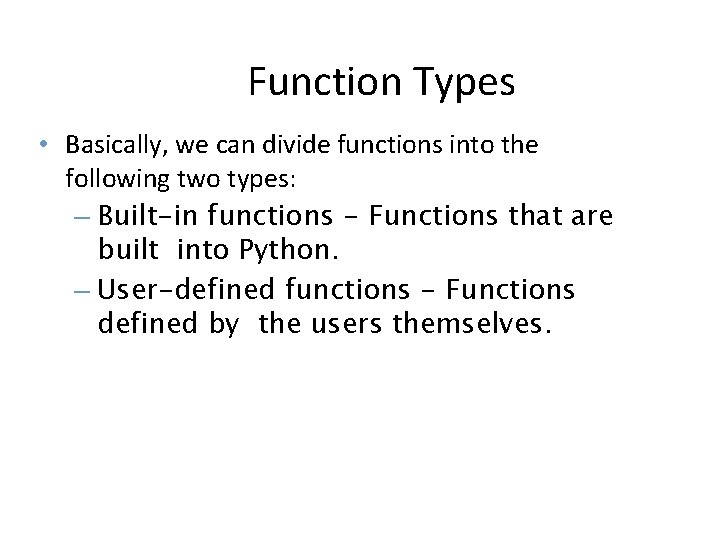
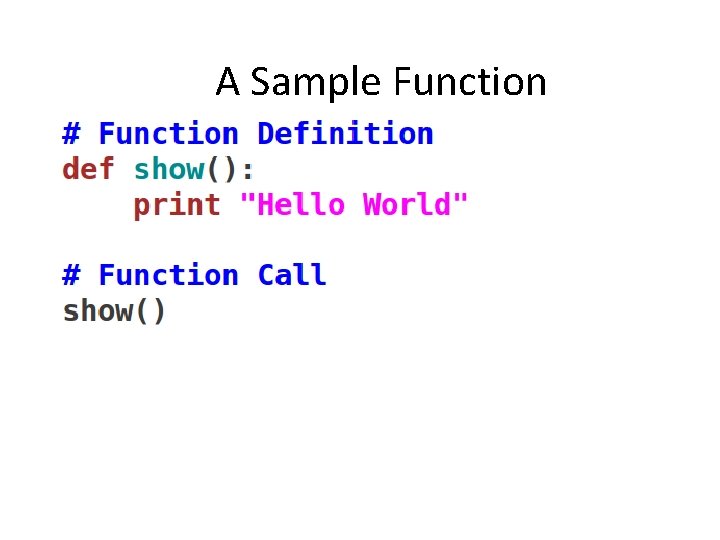
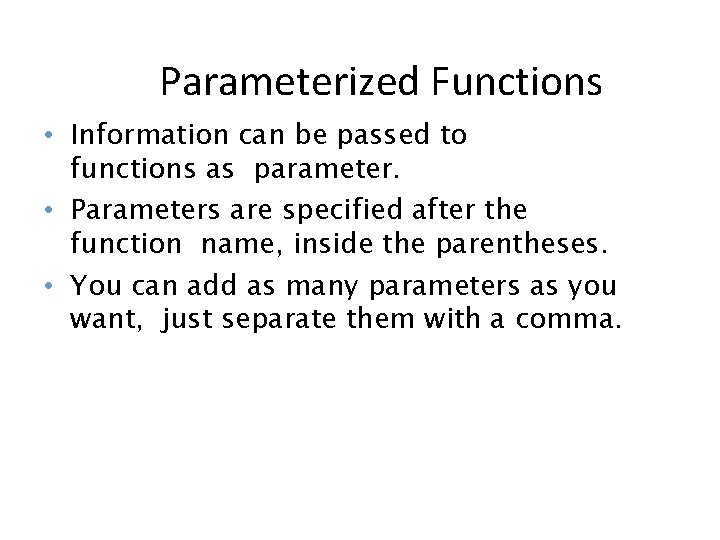
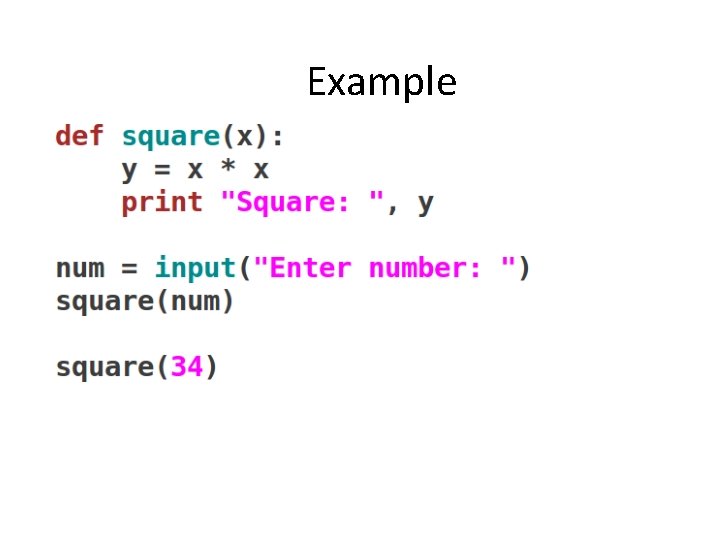
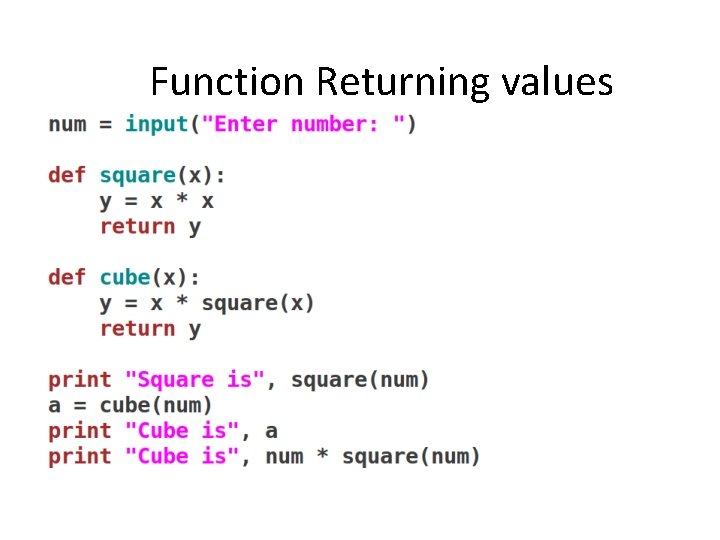
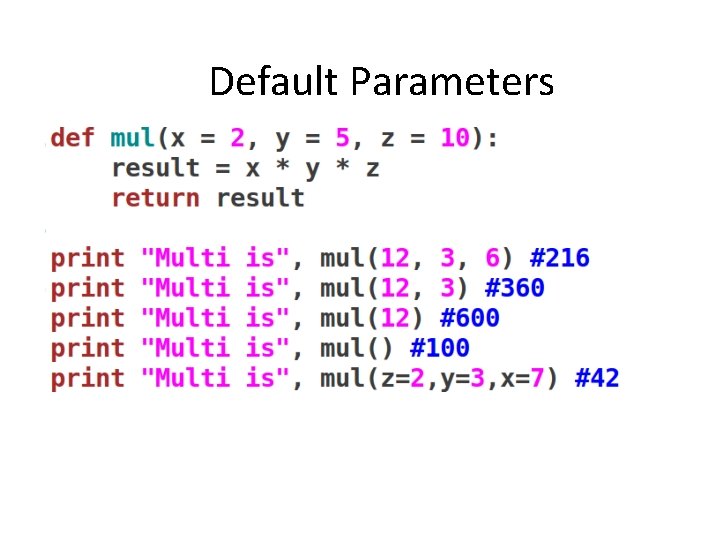
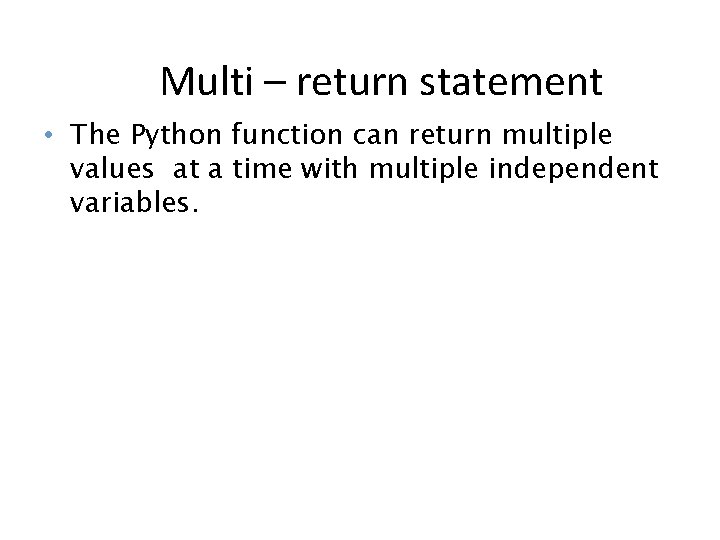
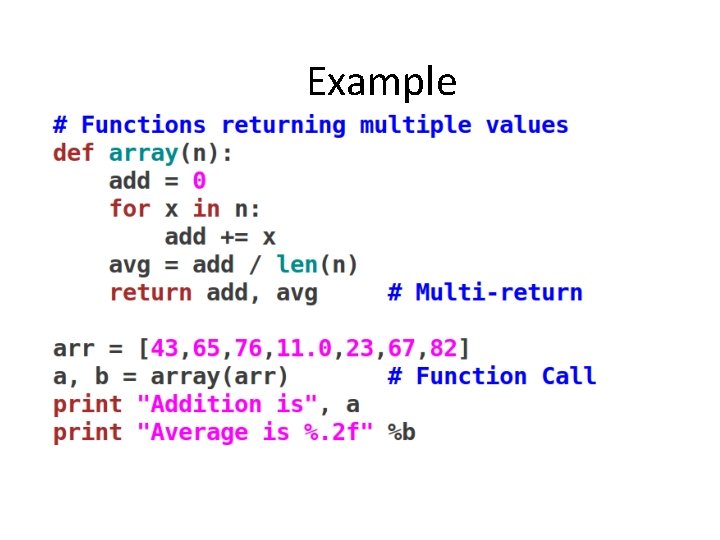
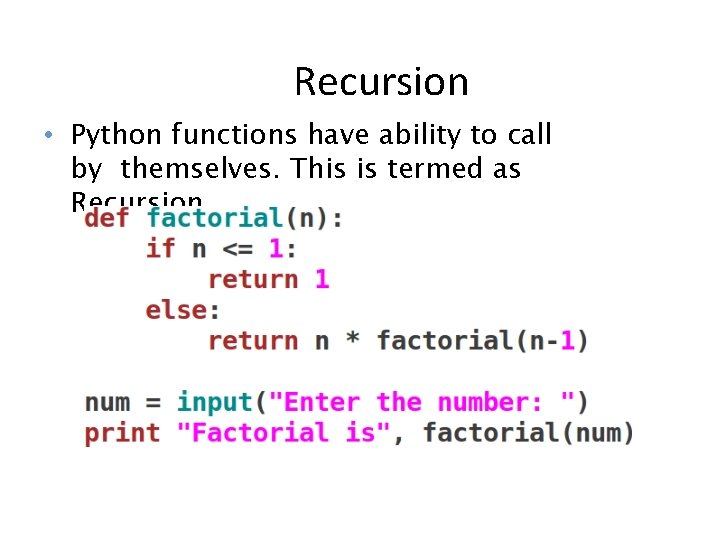
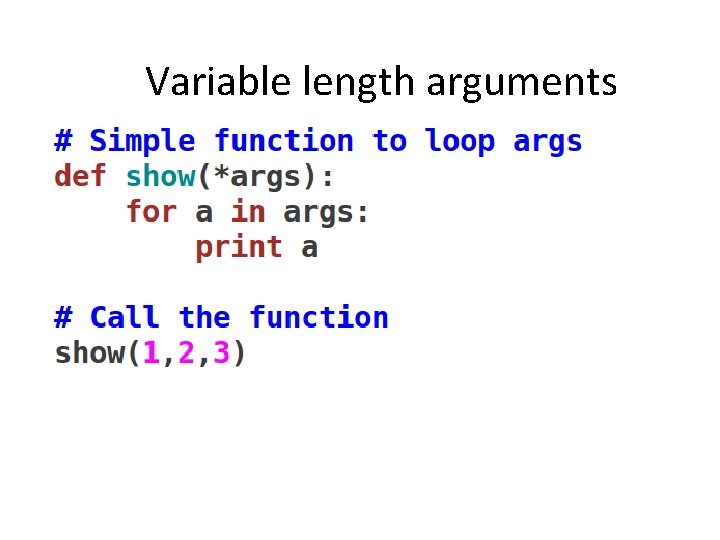
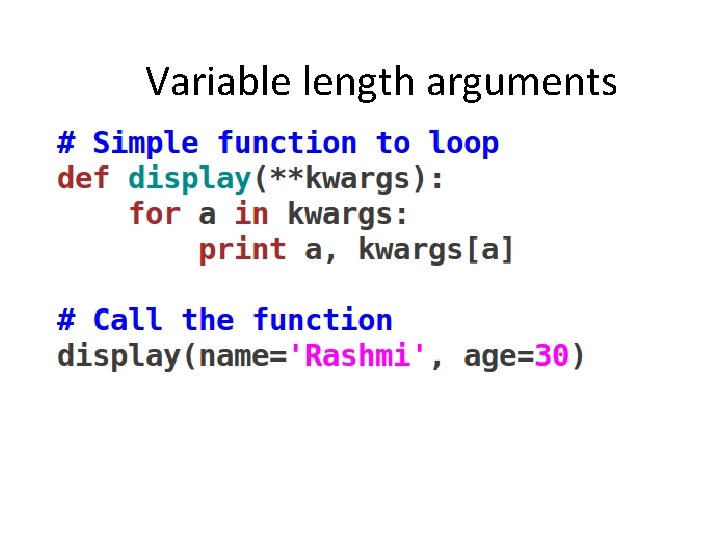
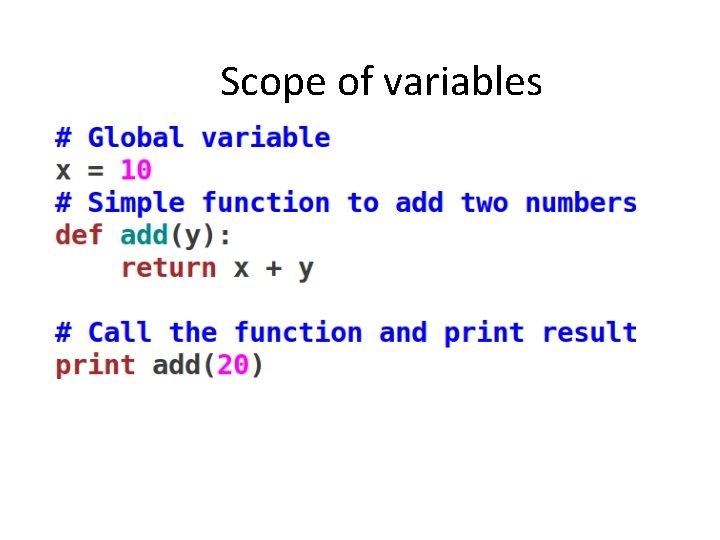
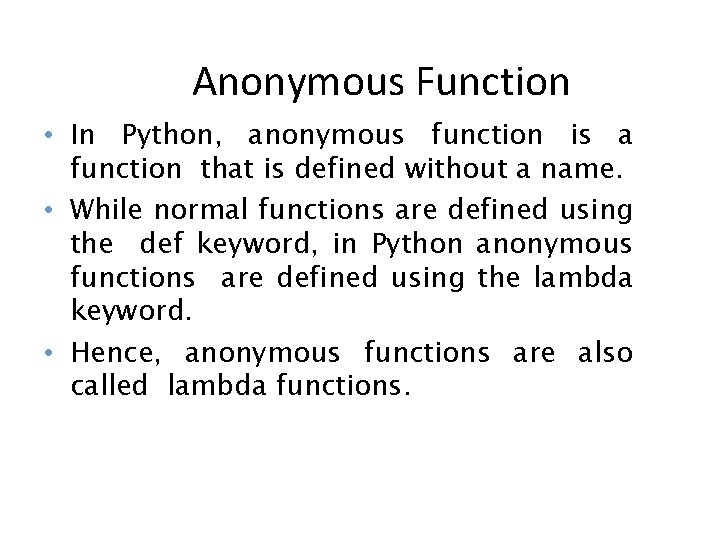
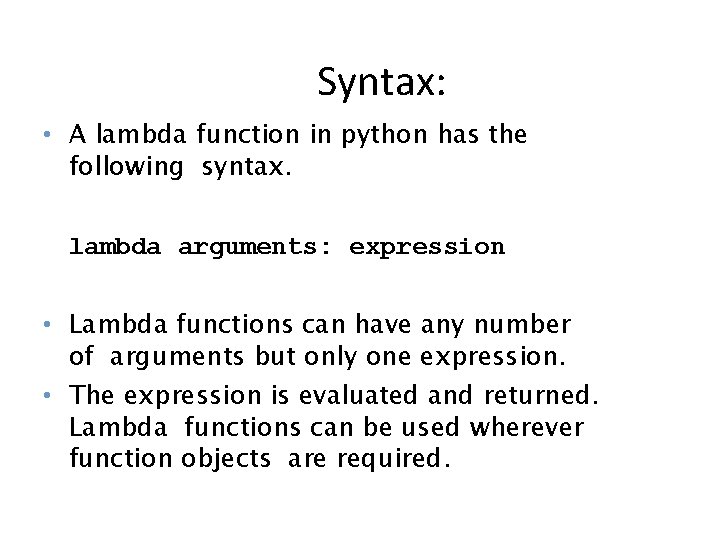
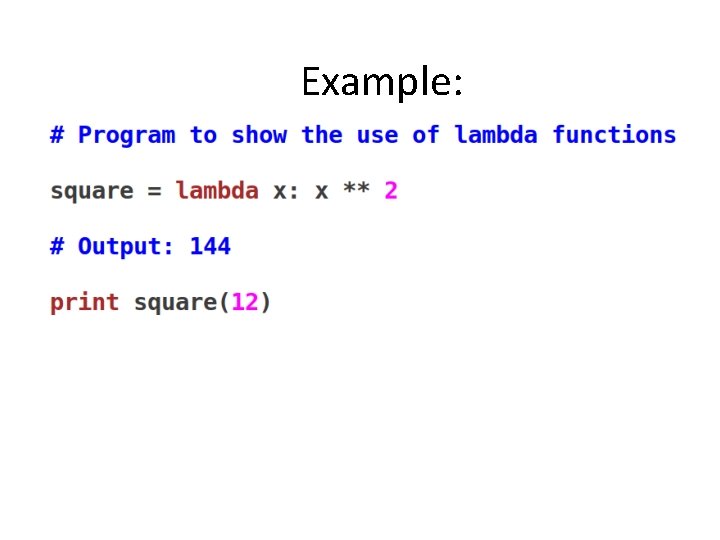
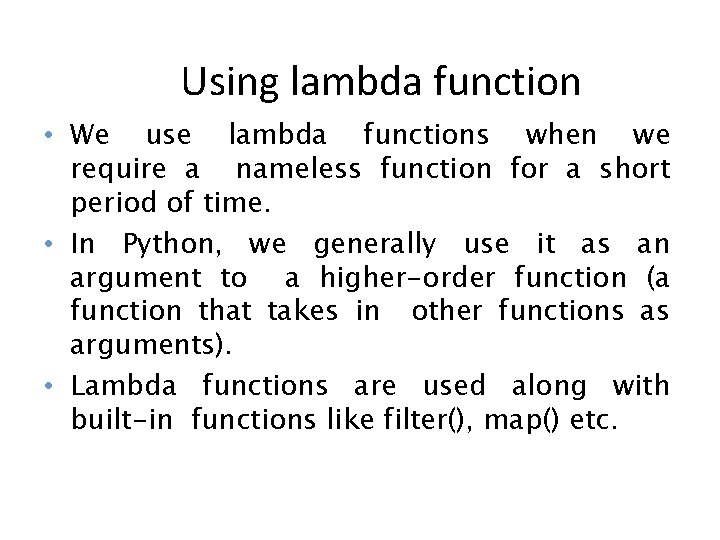
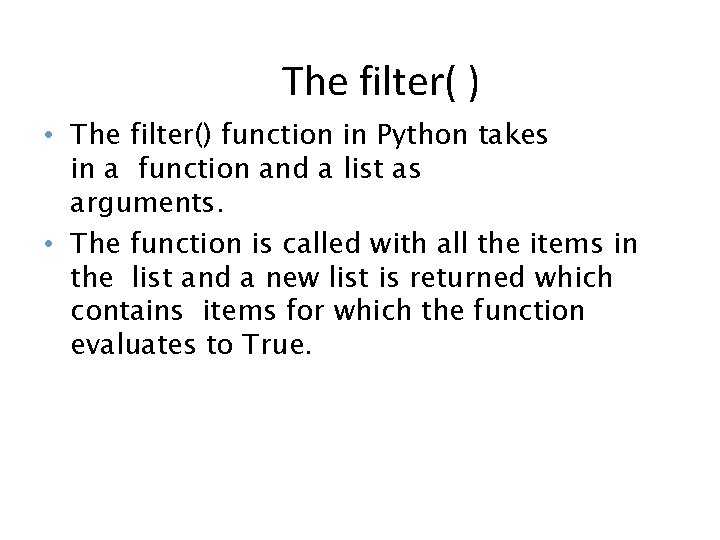
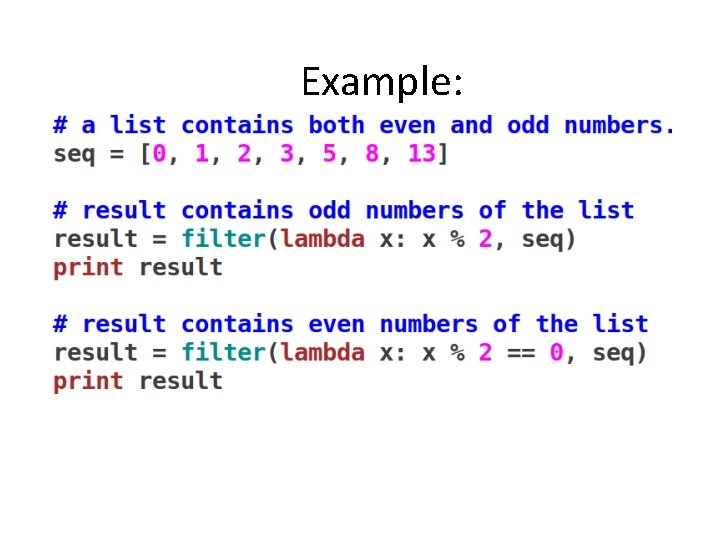
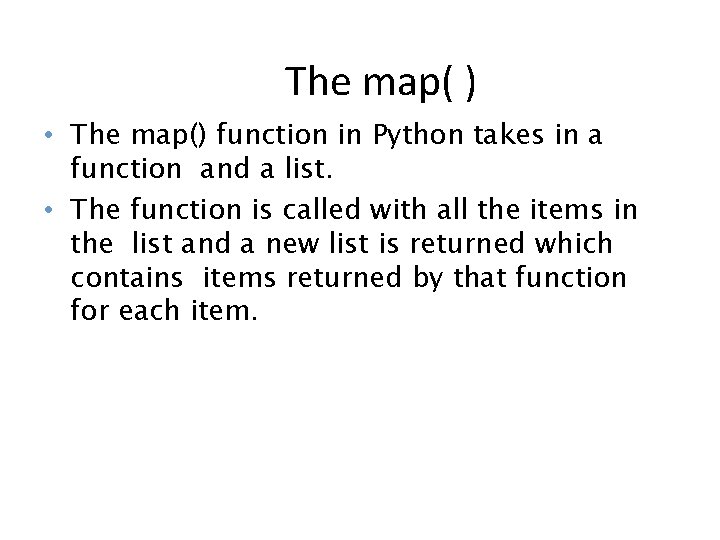
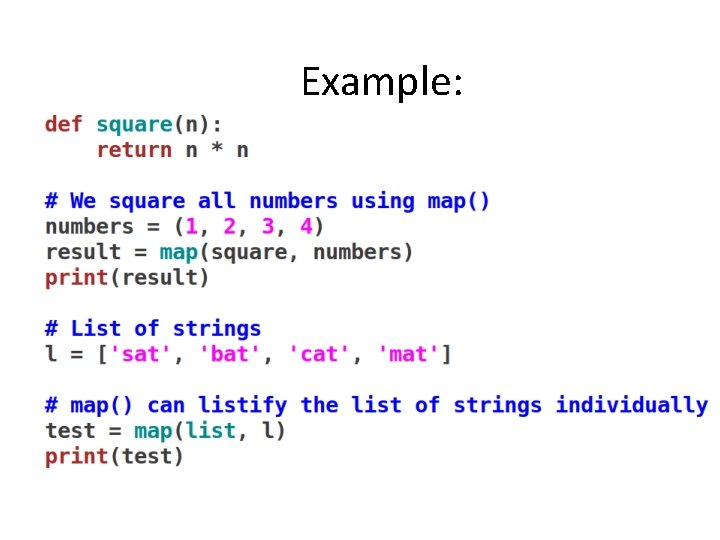
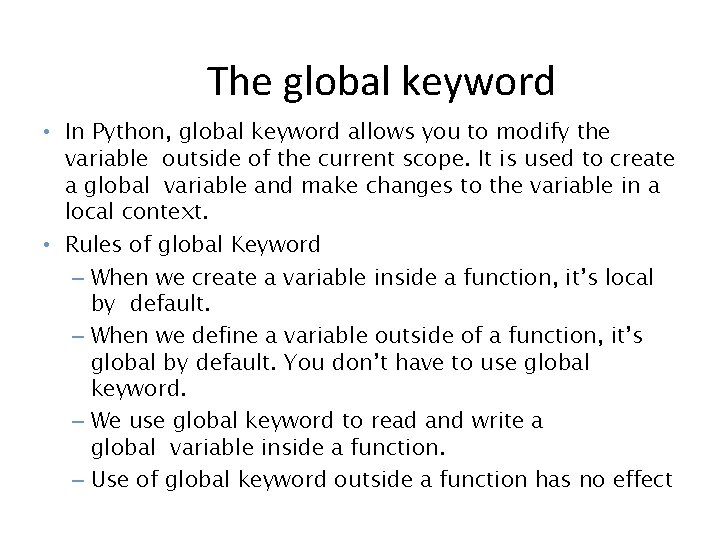
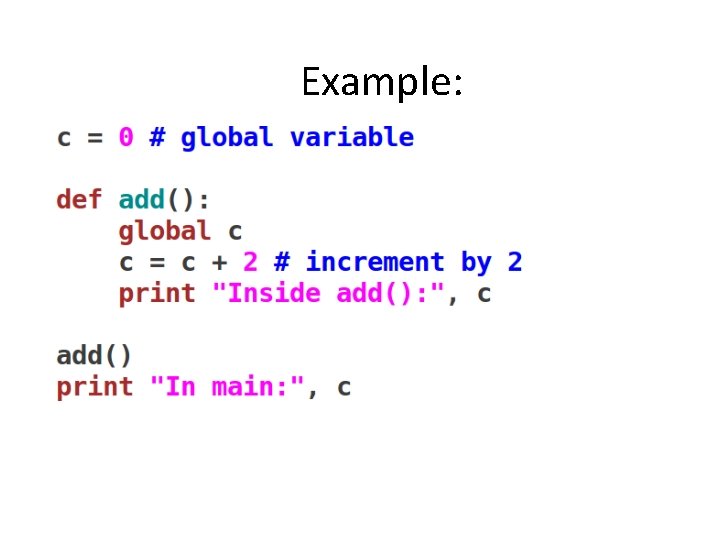
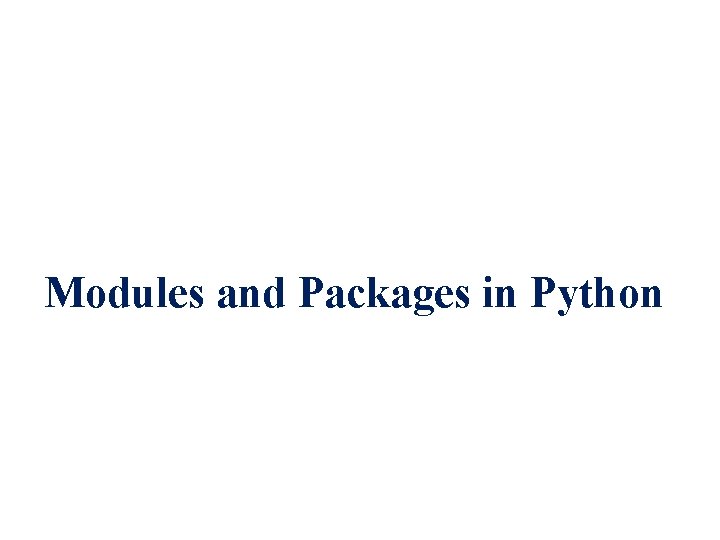
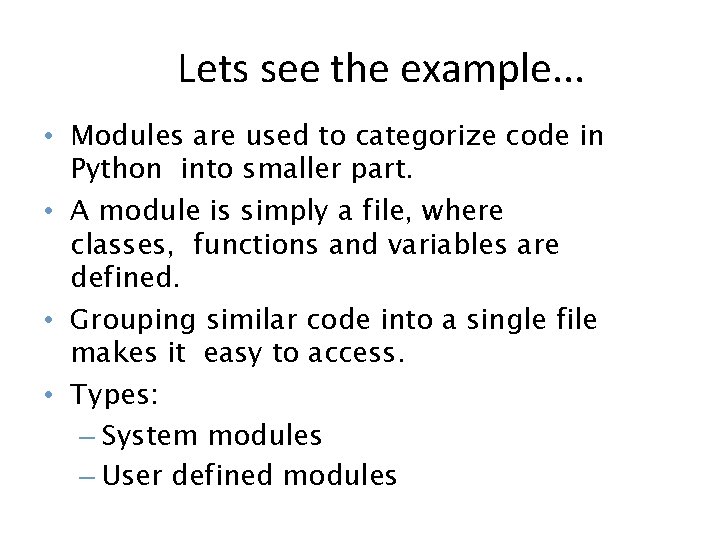
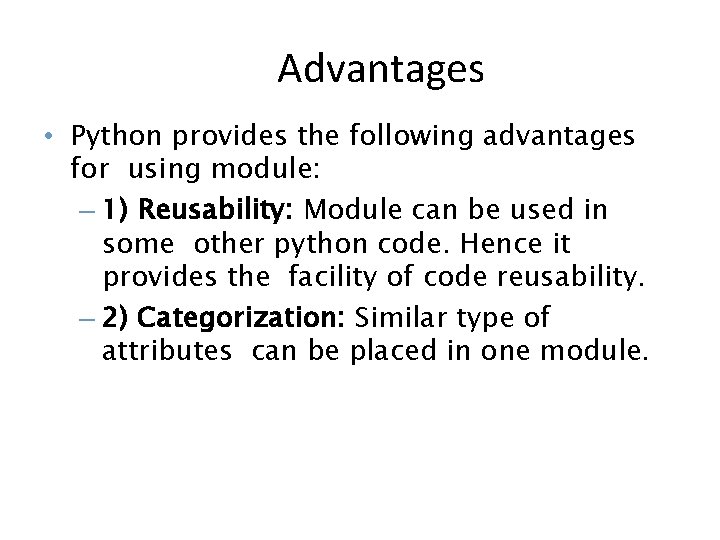
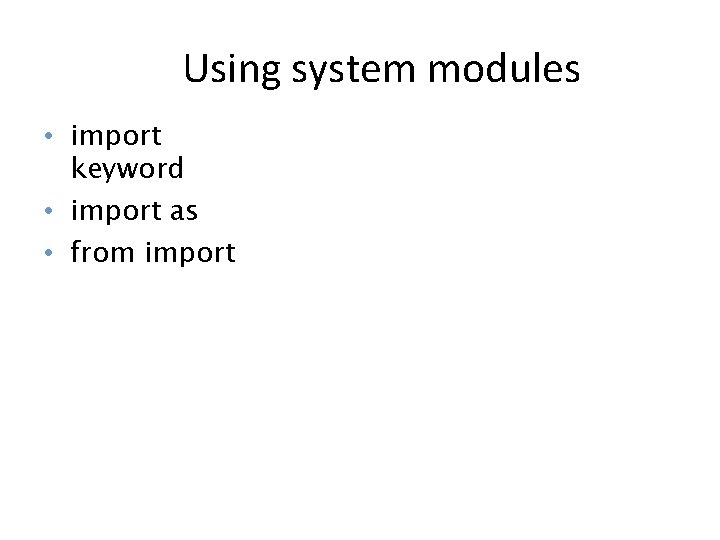
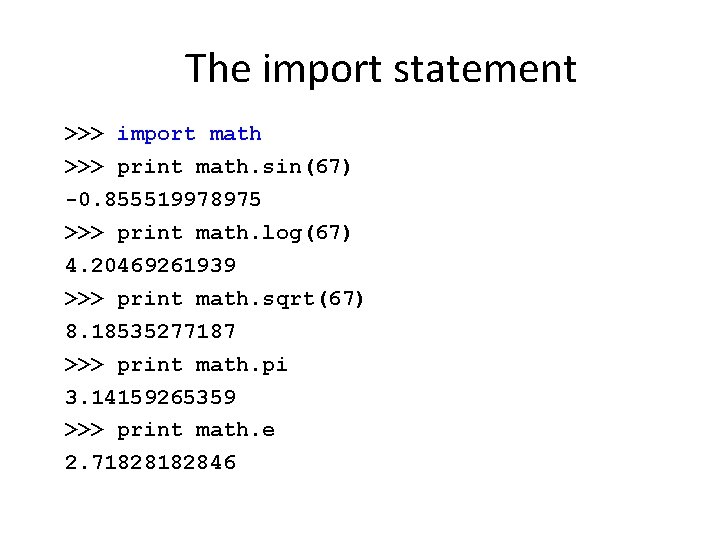
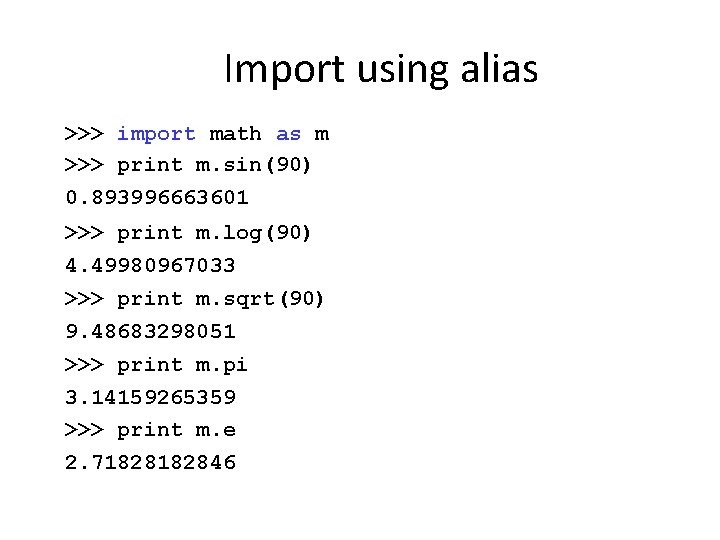
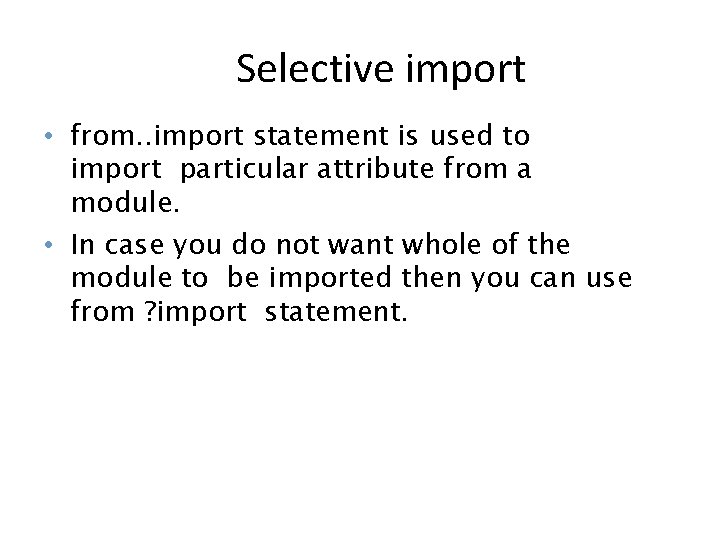
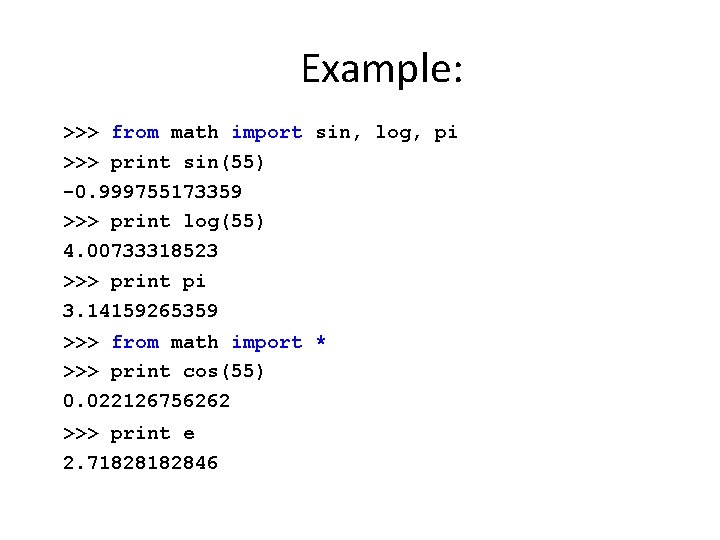
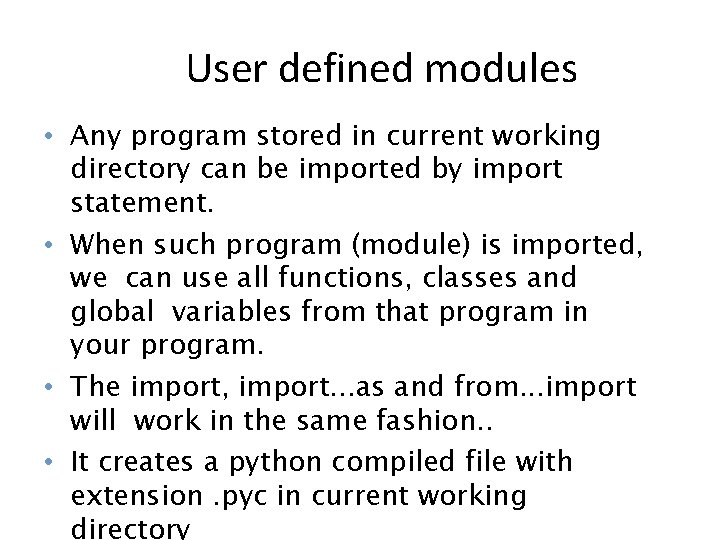
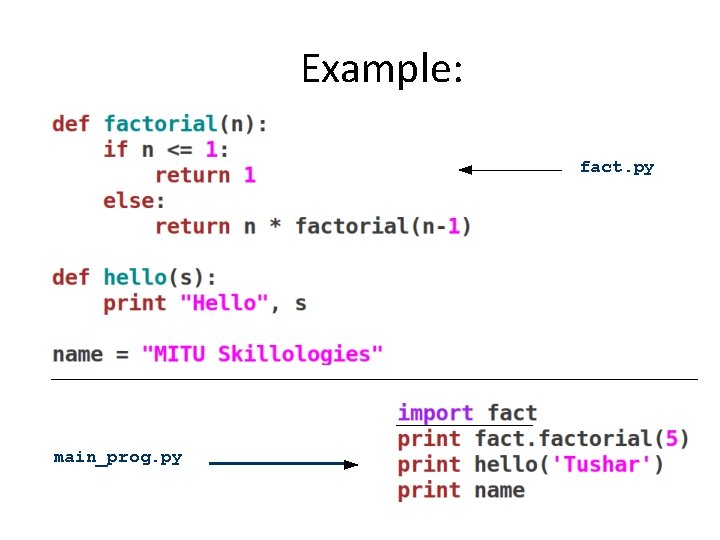
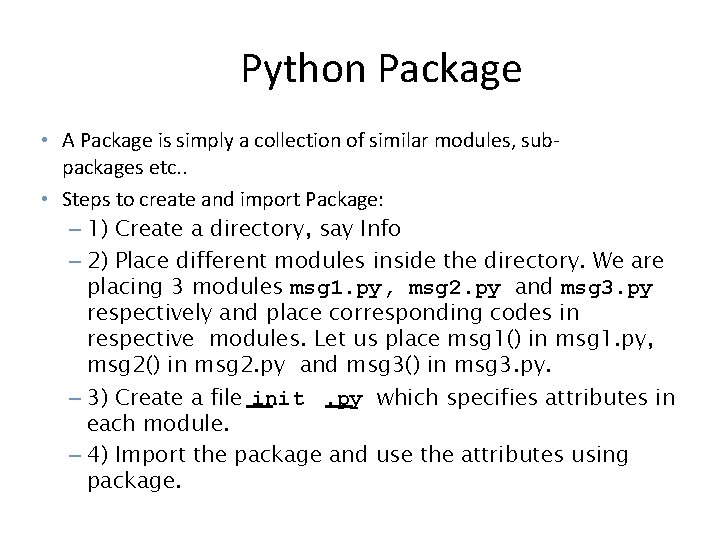
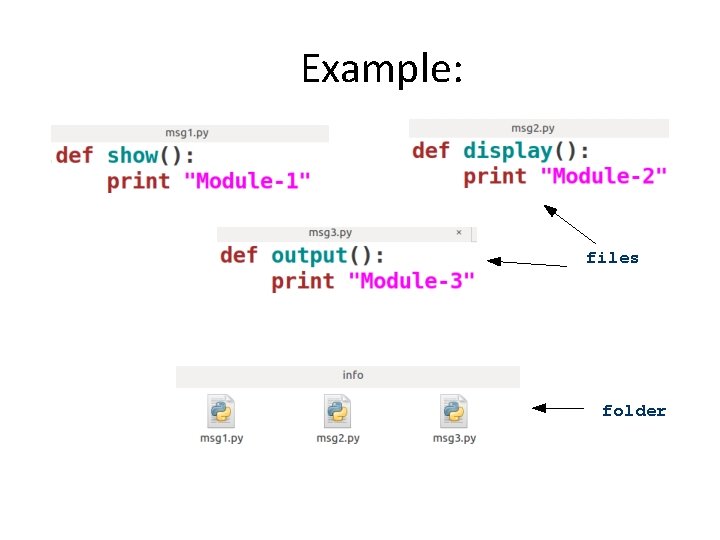
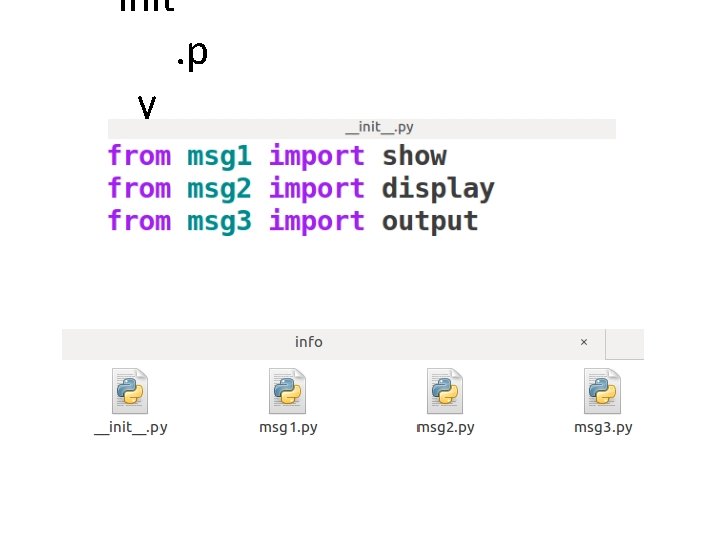
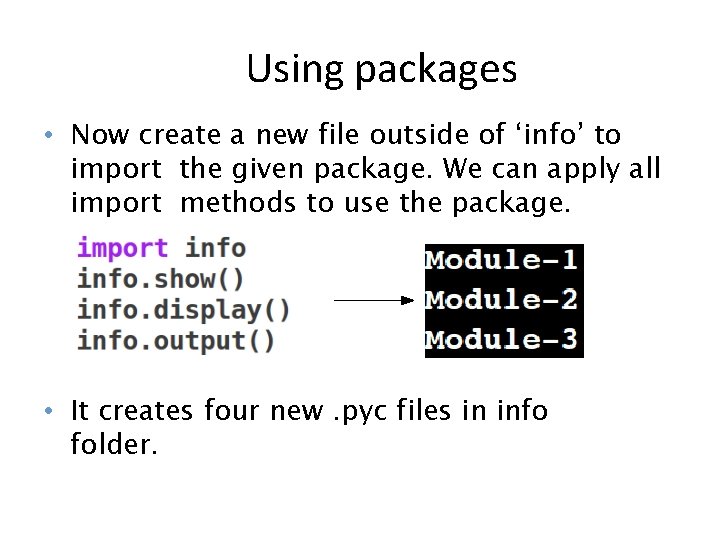
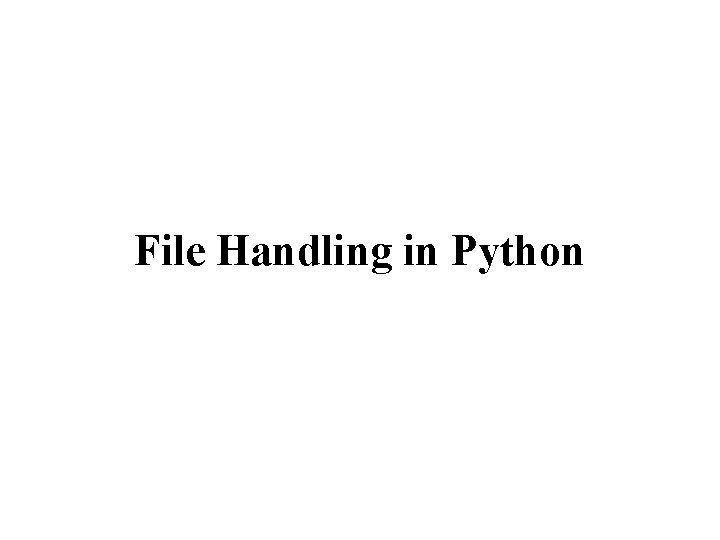
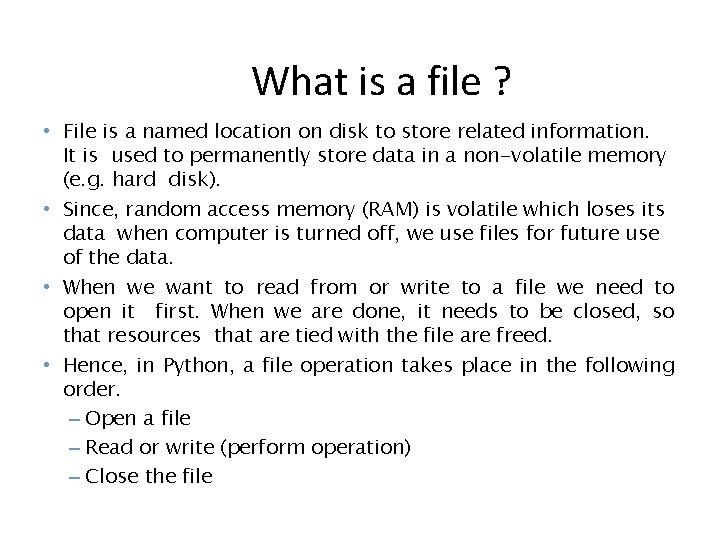
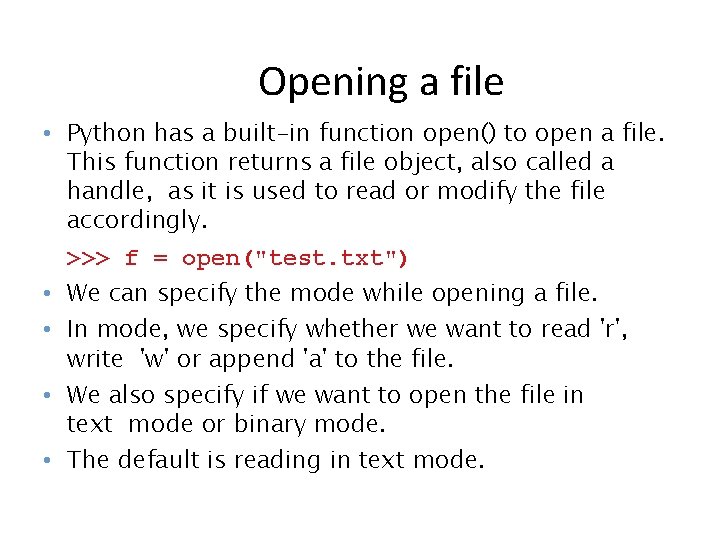
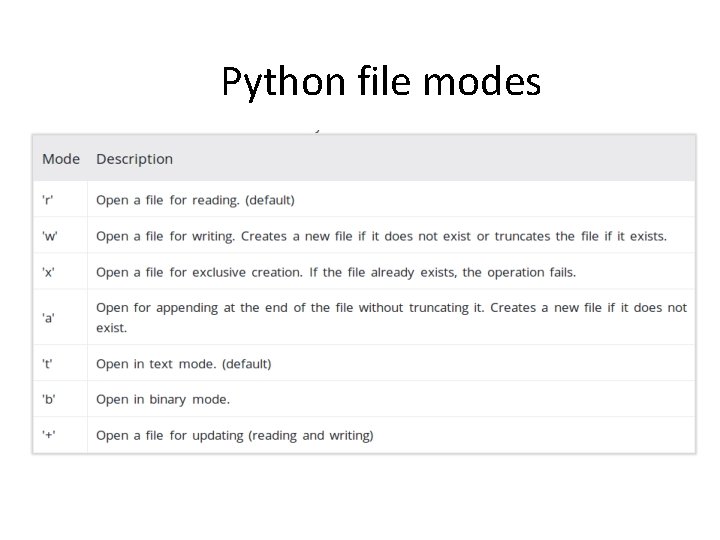
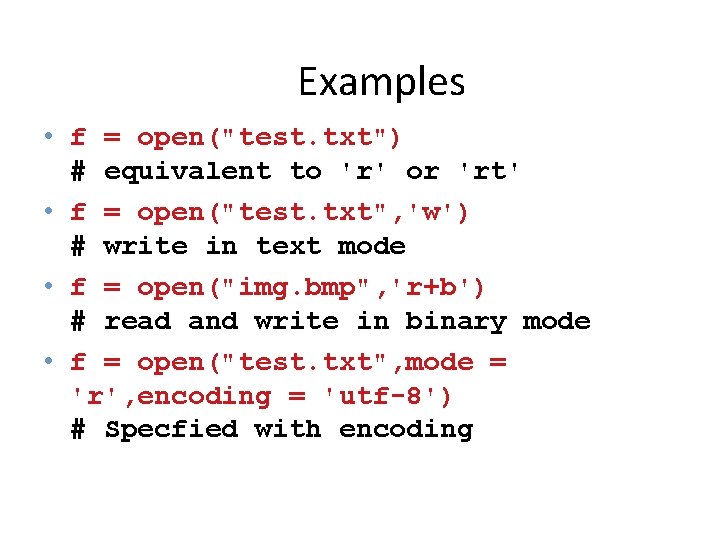
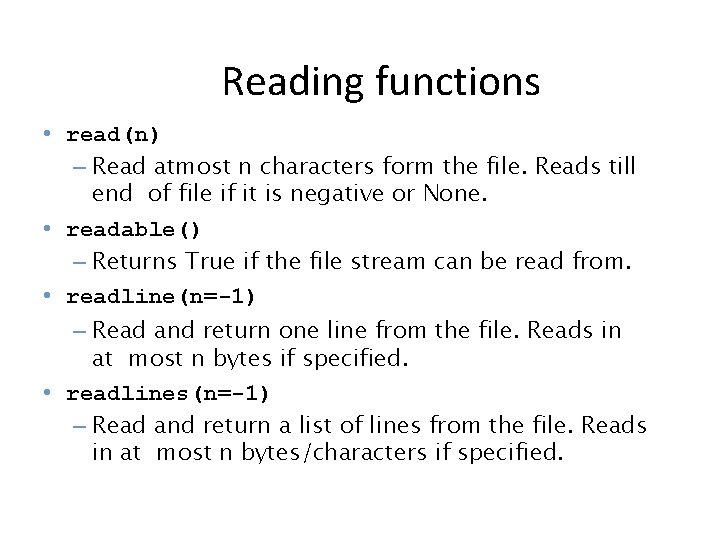
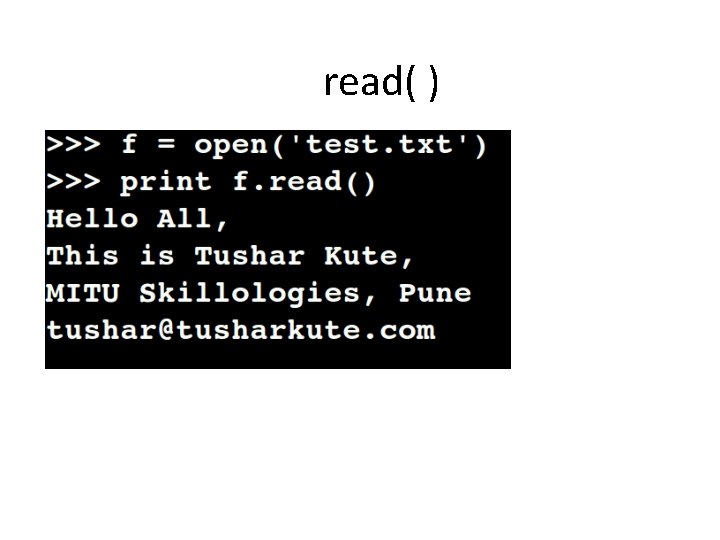
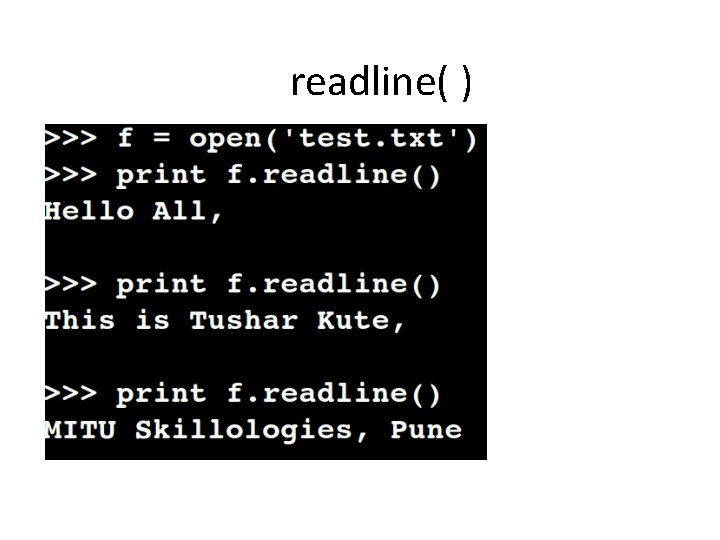
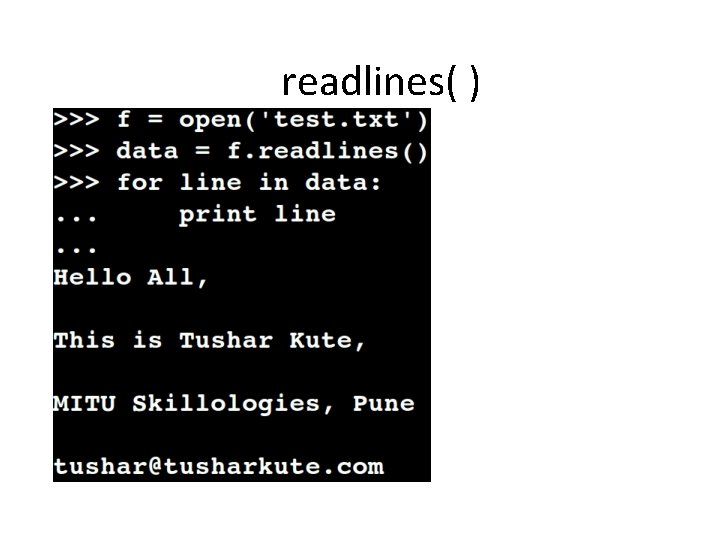
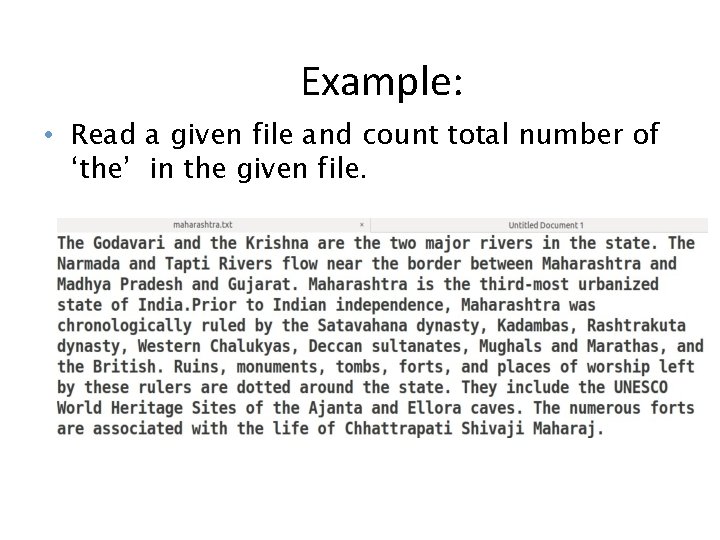
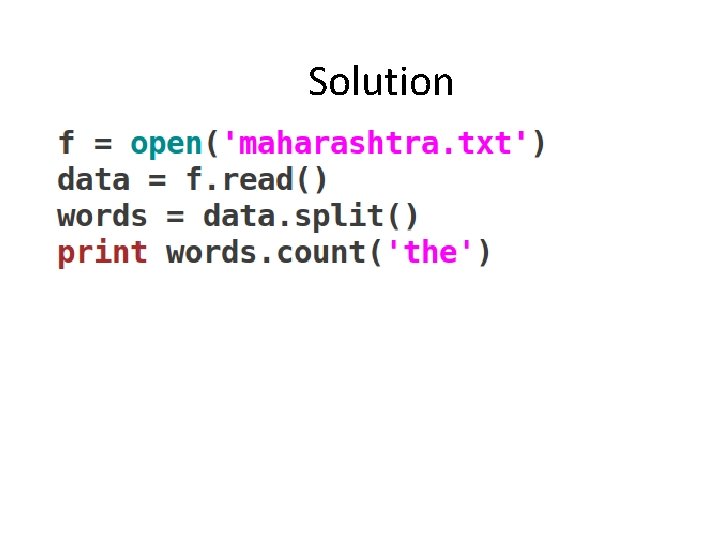
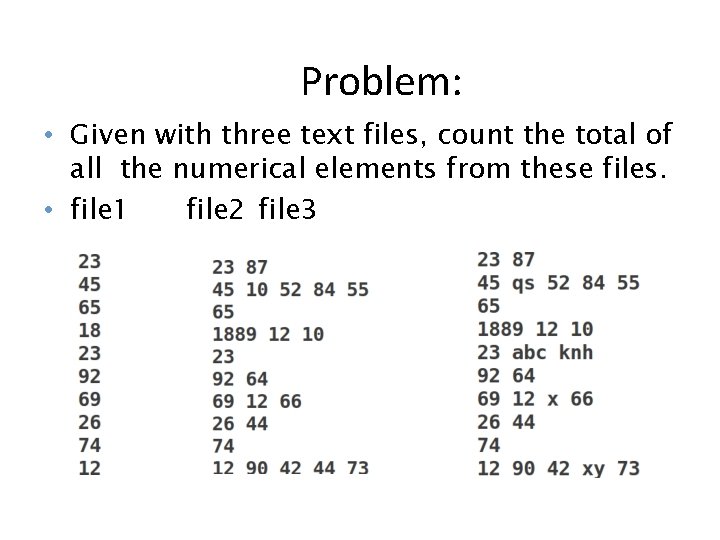
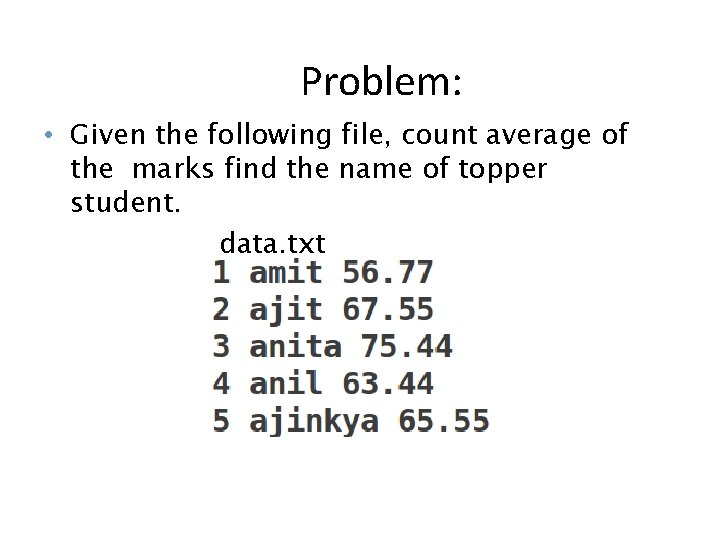
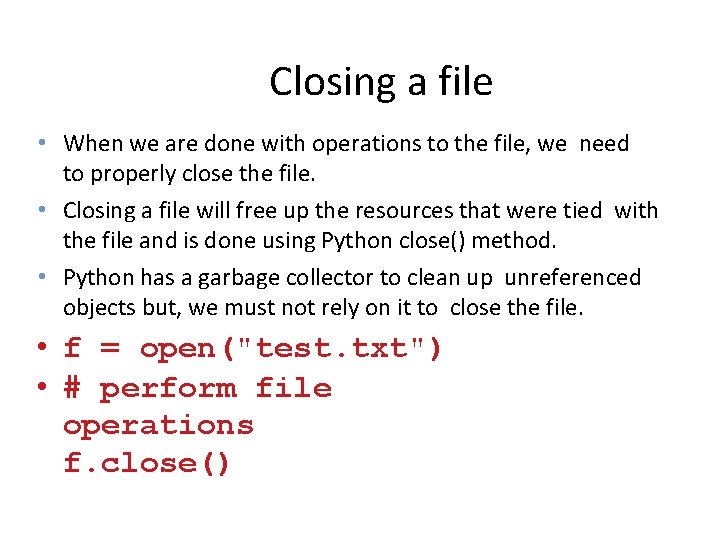
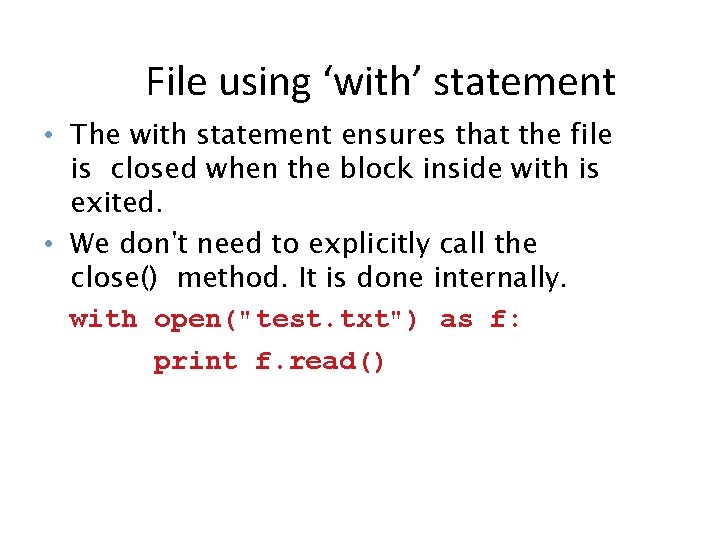
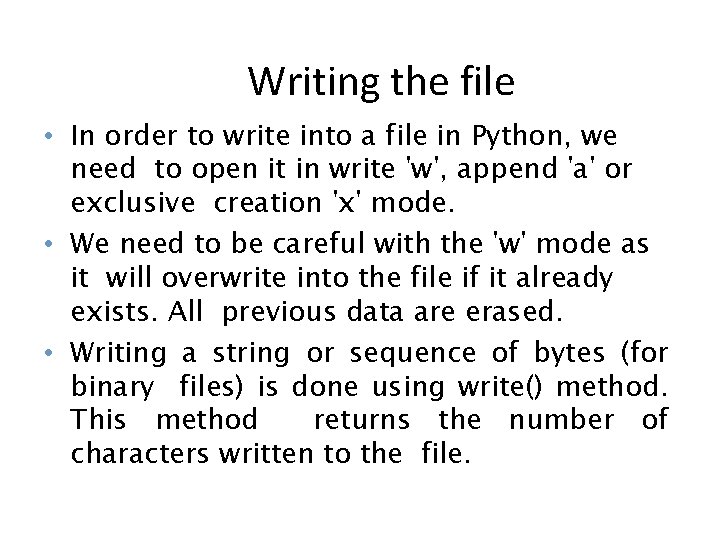
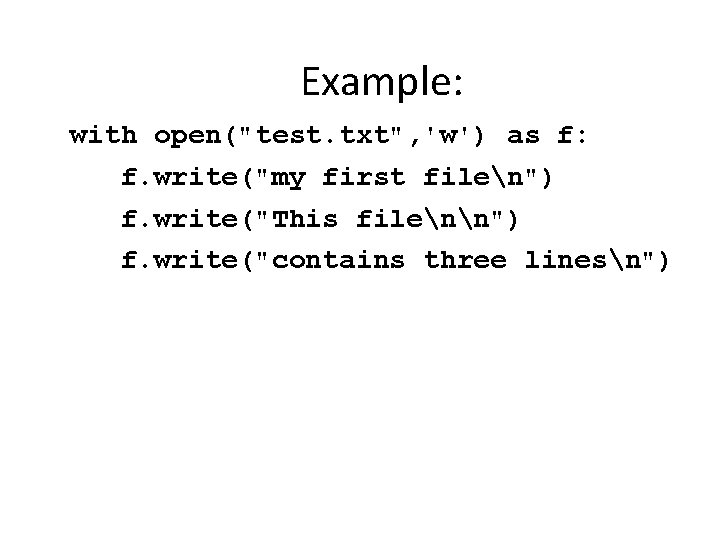
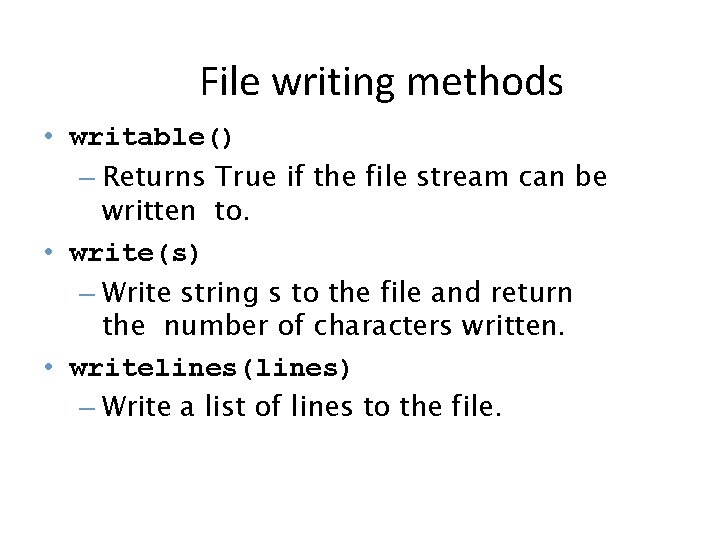
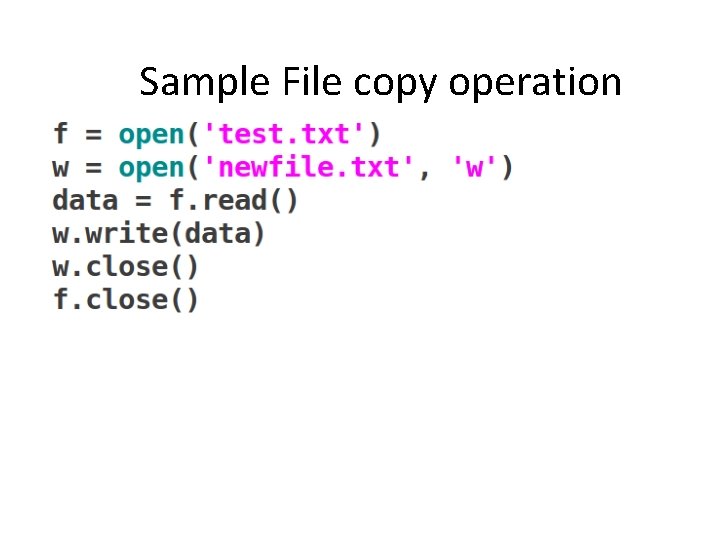
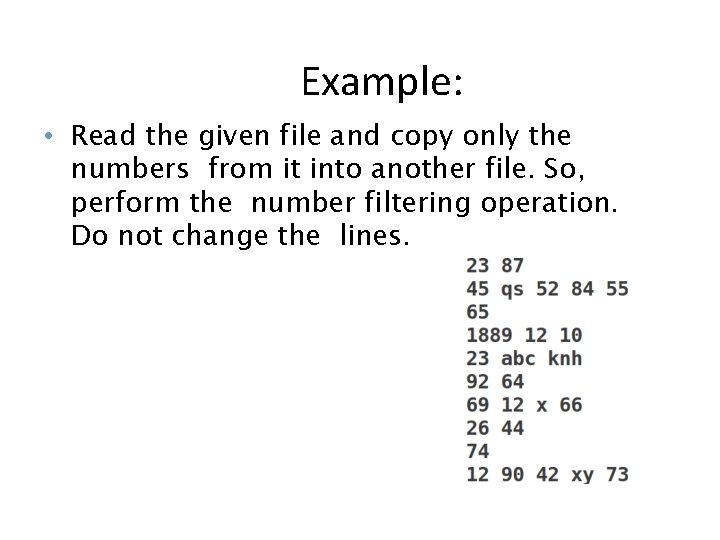
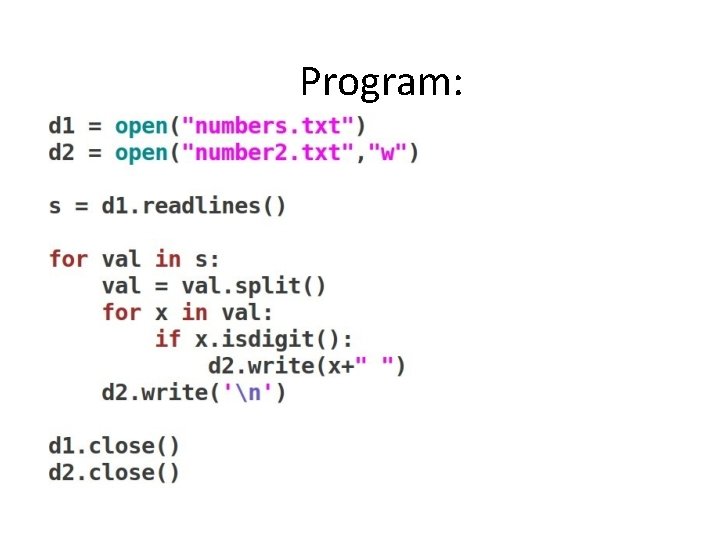
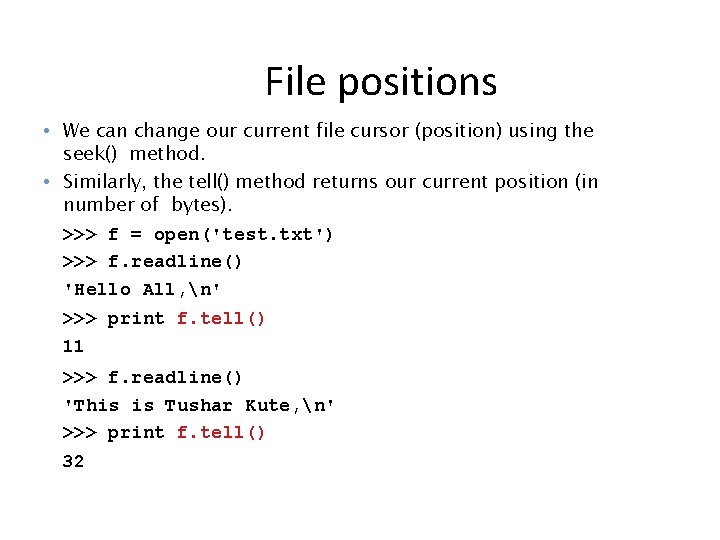
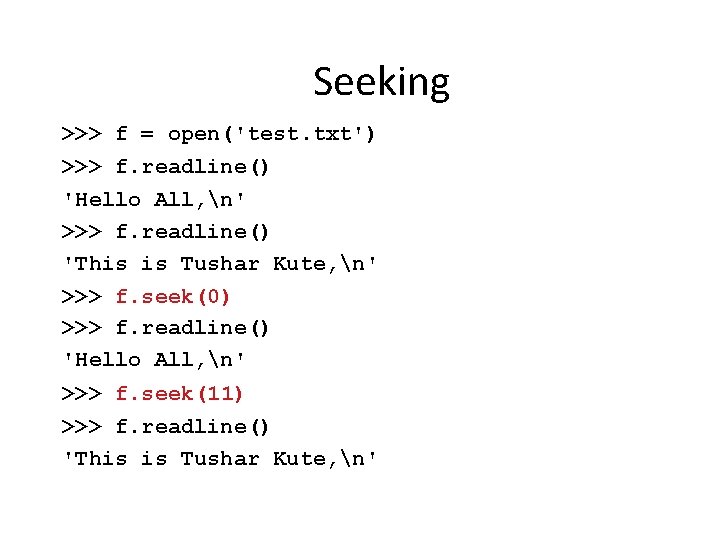
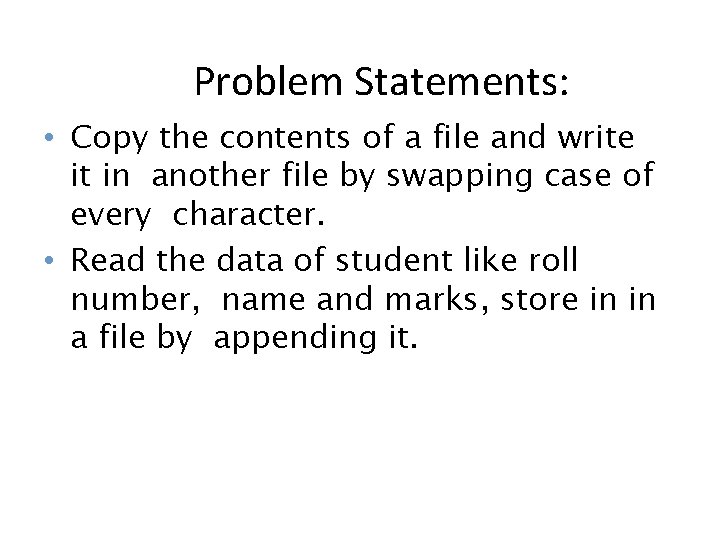
- Slides: 62
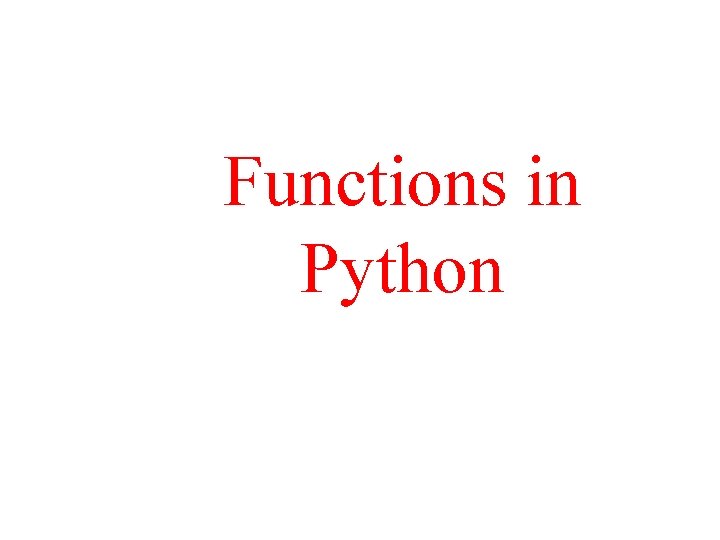
Functions in Python
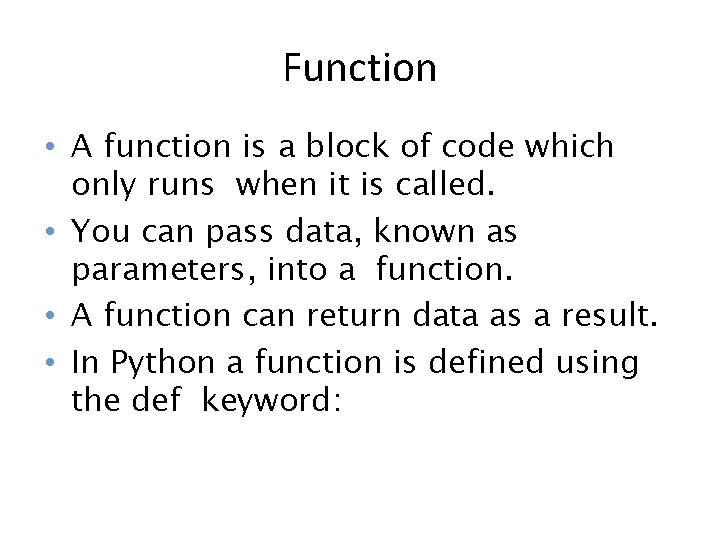
Function • A function is a block of code which only runs when it is called. • You can pass data, known as parameters, into a function. • A function can return data as a result. • In Python a function is defined using the def keyword:
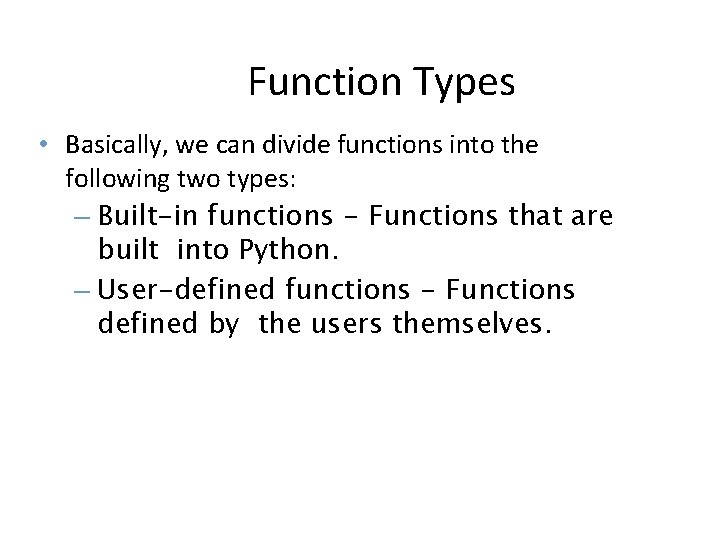
Function Types • Basically, we can divide functions into the following two types: – Built-in functions - Functions that are built into Python. – User-defined functions - Functions defined by the users themselves.
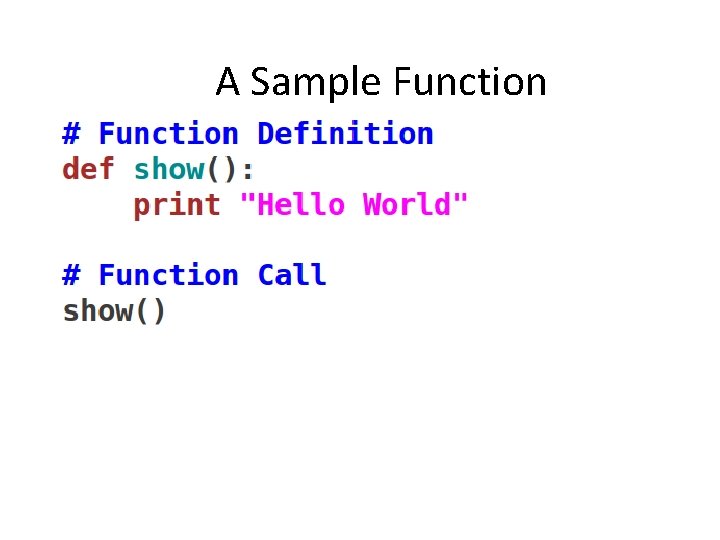
A Sample Function
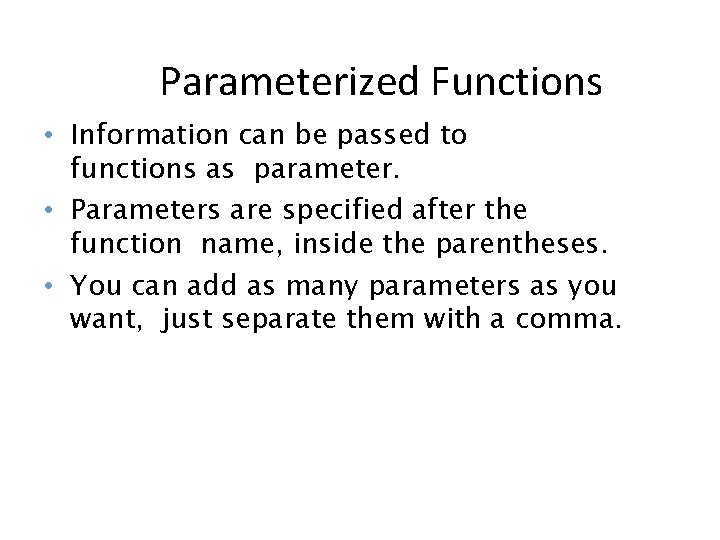
Parameterized Functions • Information can be passed to functions as parameter. • Parameters are specified after the function name, inside the parentheses. • You can add as many parameters as you want, just separate them with a comma.
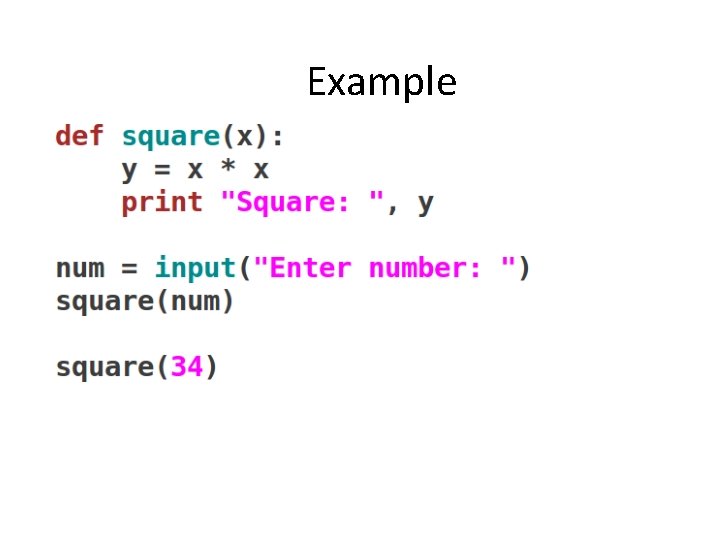
Example
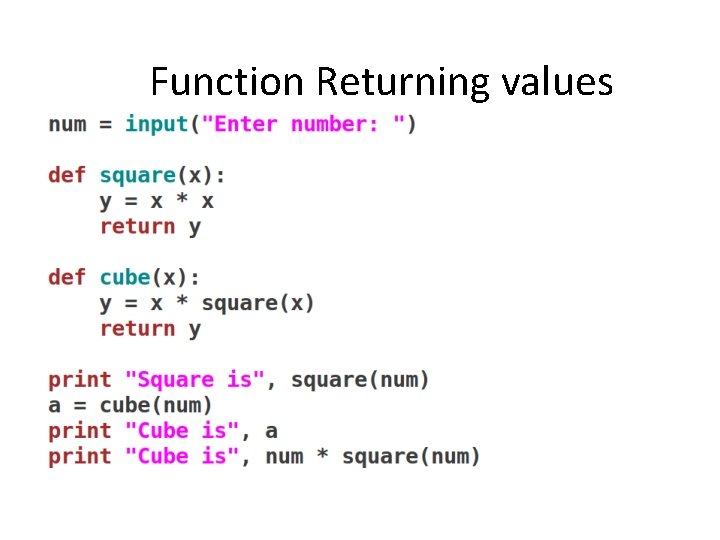
Function Returning values
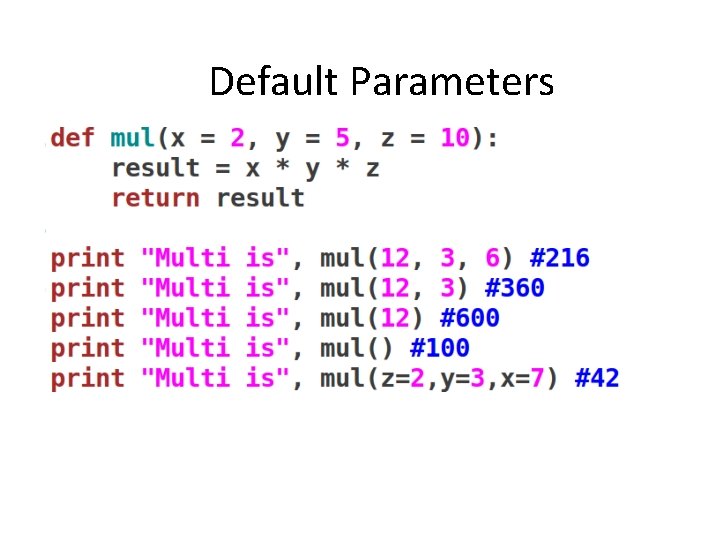
Default Parameters
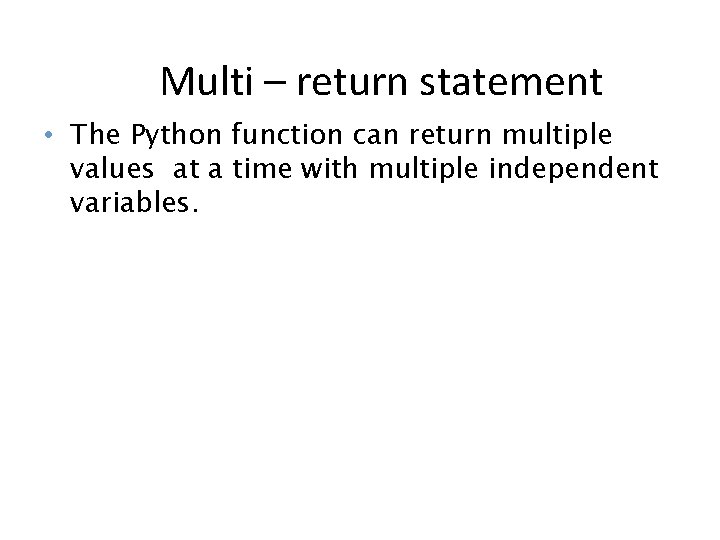
Multi – return statement • The Python function can return multiple values at a time with multiple independent variables.
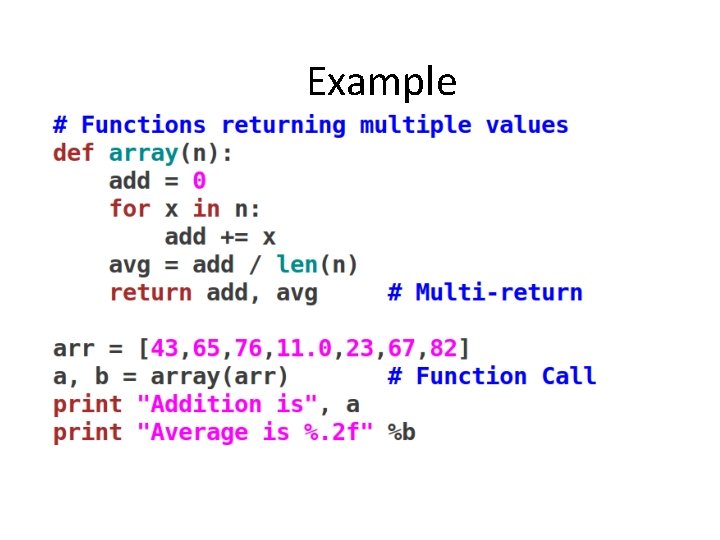
Example
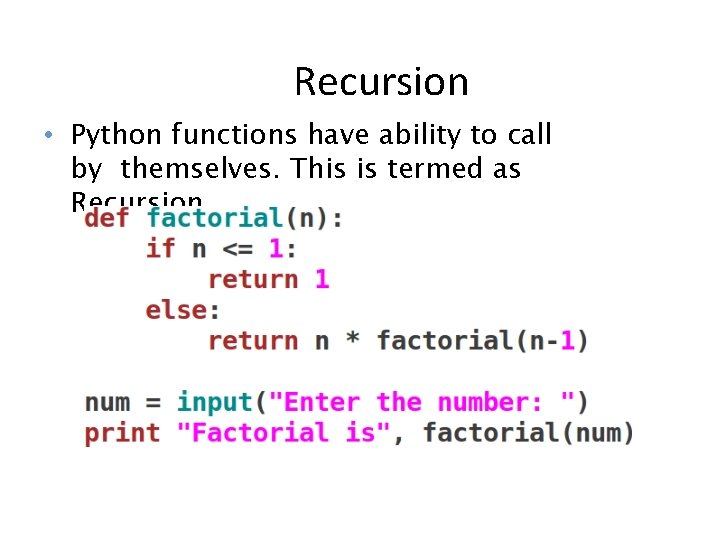
Recursion • Python functions have ability to call by themselves. This is termed as Recursion.
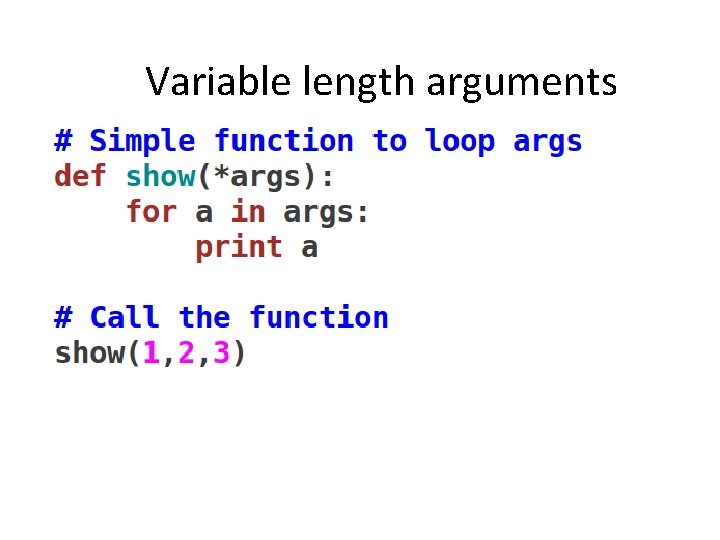
Variable length arguments
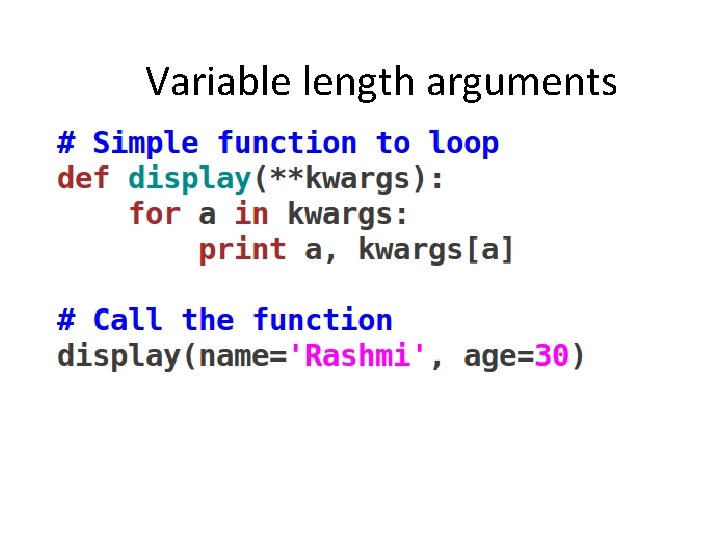
Variable length arguments
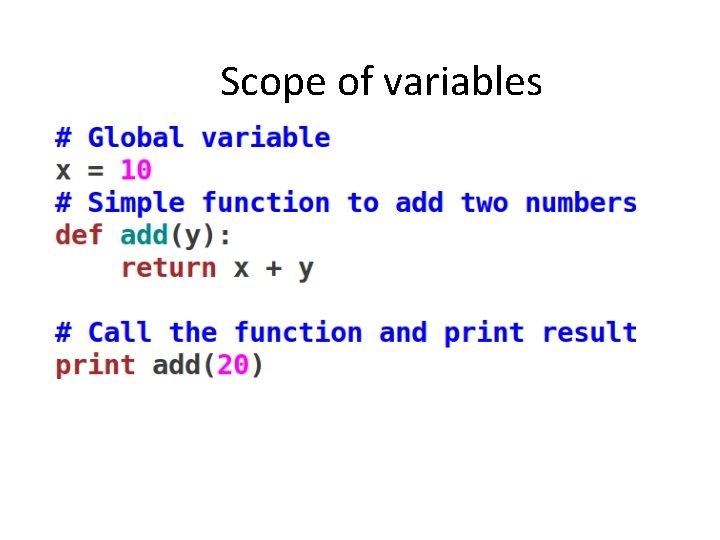
Scope of variables
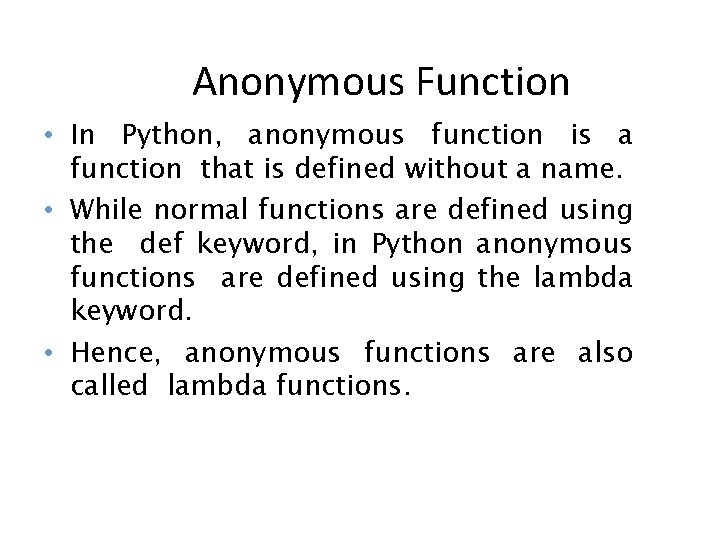
Anonymous Function • In Python, anonymous function is a function that is defined without a name. • While normal functions are defined using the def keyword, in Python anonymous functions are defined using the lambda keyword. • Hence, anonymous functions are also called lambda functions.
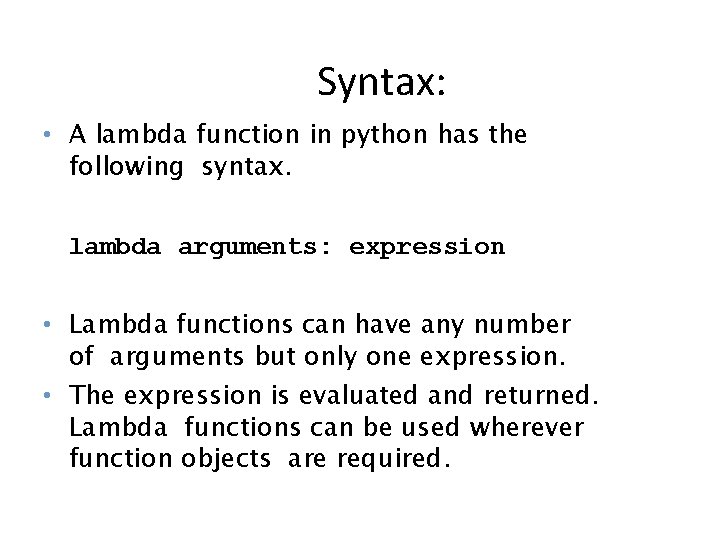
Syntax: • A lambda function in python has the following syntax. lambda arguments: expression • Lambda functions can have any number of arguments but only one expression. • The expression is evaluated and returned. Lambda functions can be used wherever function objects are required.
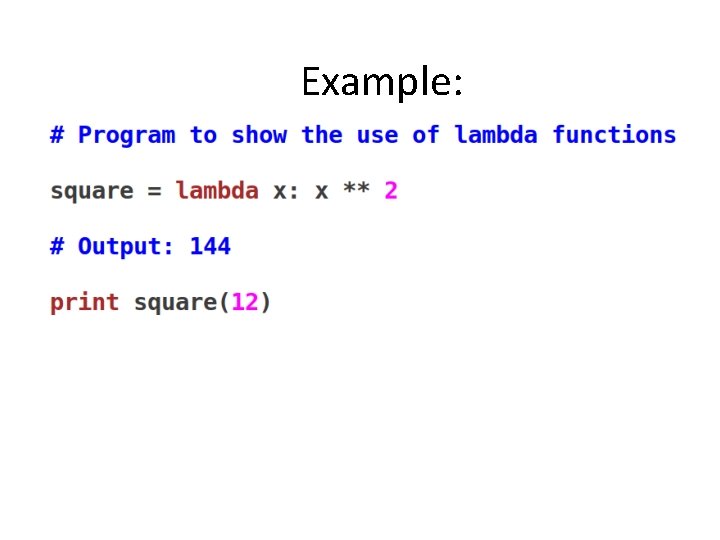
Example:
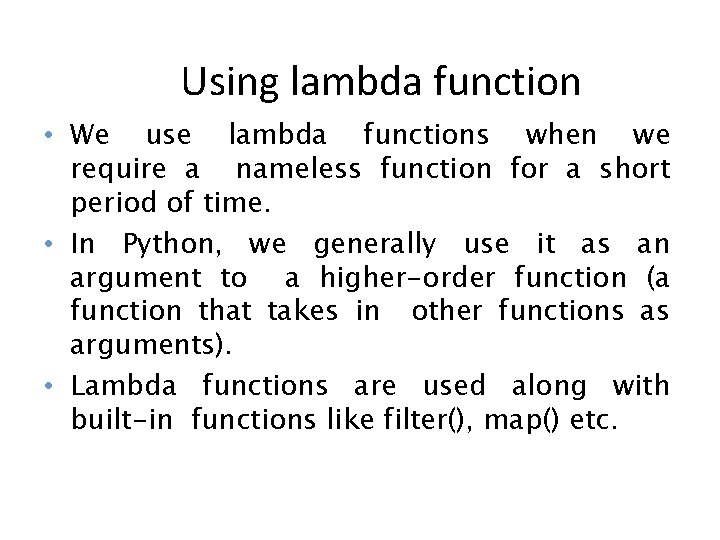
Using lambda function • We use lambda functions when we require a nameless function for a short period of time. • In Python, we generally use it as an argument to a higher-order function (a function that takes in other functions as arguments). • Lambda functions are used along with built-in functions like filter(), map() etc.
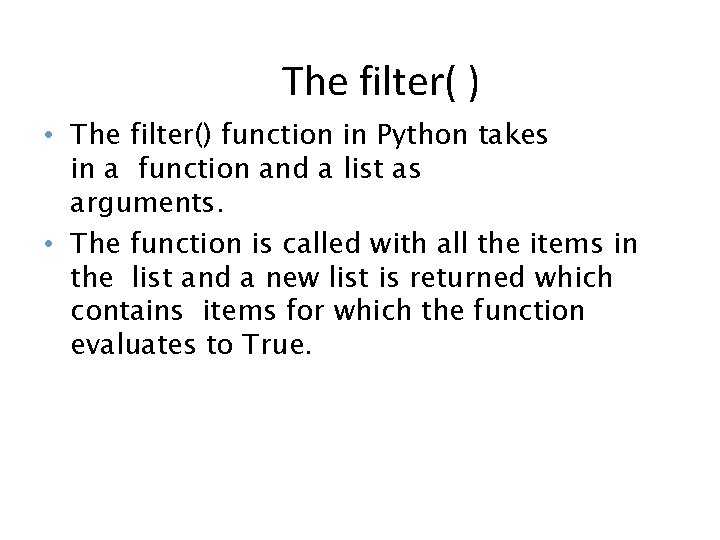
The filter( ) • The filter() function in Python takes in a function and a list as arguments. • The function is called with all the items in the list and a new list is returned which contains items for which the function evaluates to True.
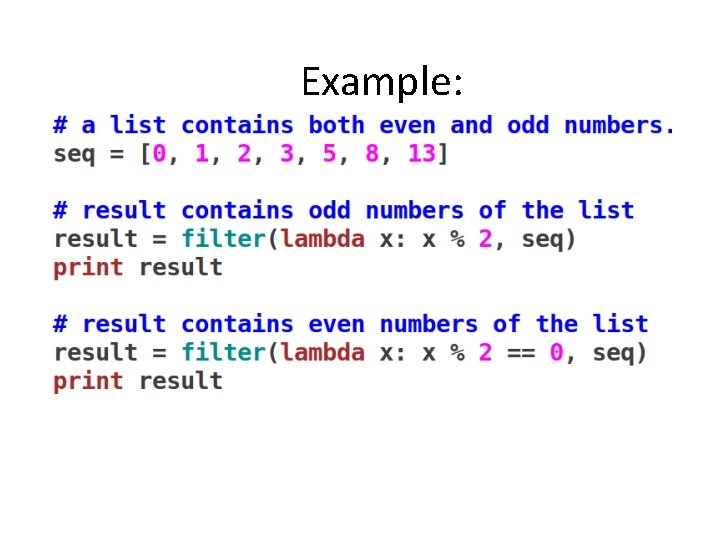
Example:
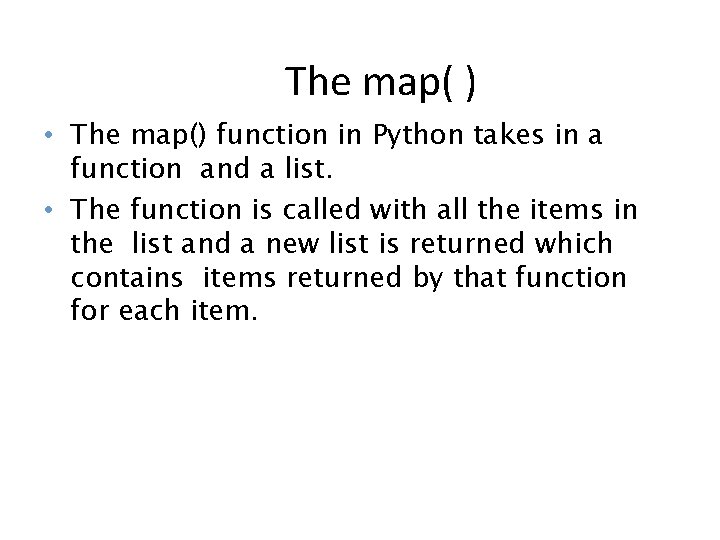
The map( ) • The map() function in Python takes in a function and a list. • The function is called with all the items in the list and a new list is returned which contains items returned by that function for each item.
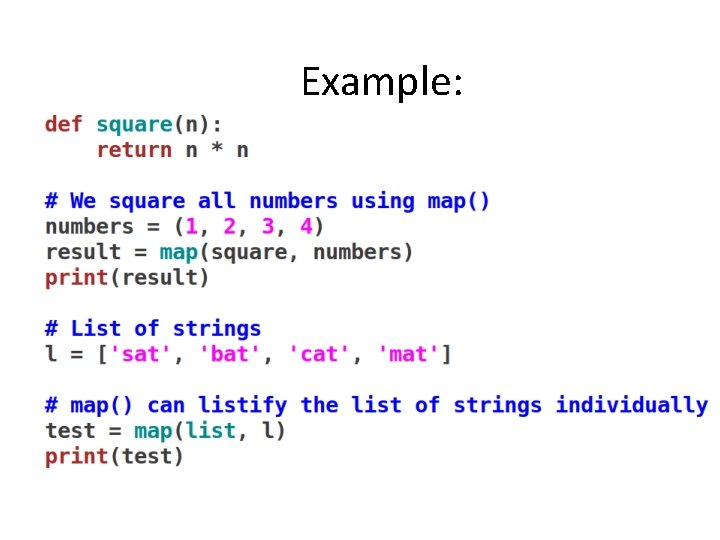
Example:
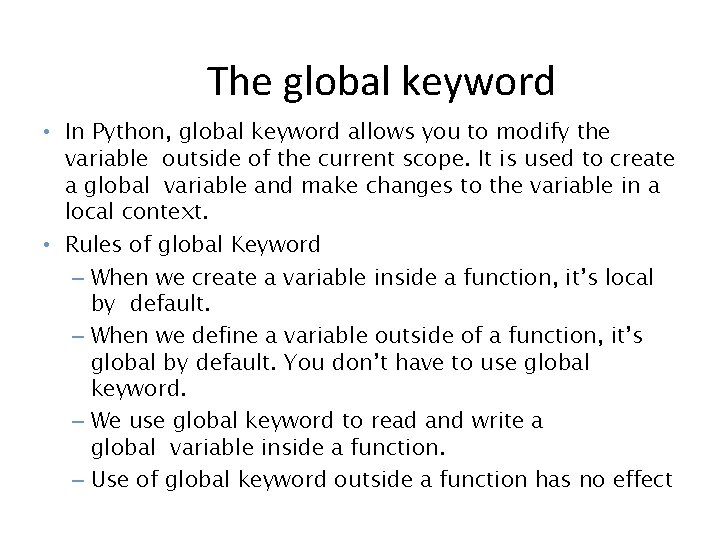
The global keyword • In Python, global keyword allows you to modify the variable outside of the current scope. It is used to create a global variable and make changes to the variable in a local context. • Rules of global Keyword – When we create a variable inside a function, it’s local by default. – When we define a variable outside of a function, it’s global by default. You don’t have to use global keyword. – We use global keyword to read and write a global variable inside a function. – Use of global keyword outside a function has no effect
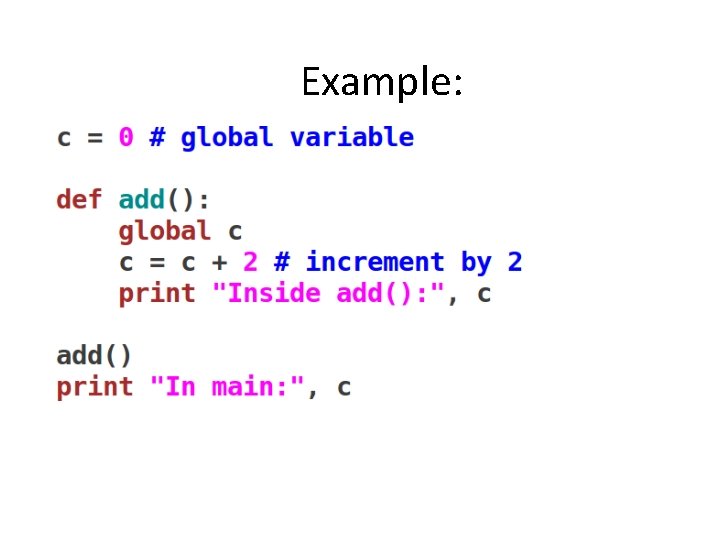
Example:
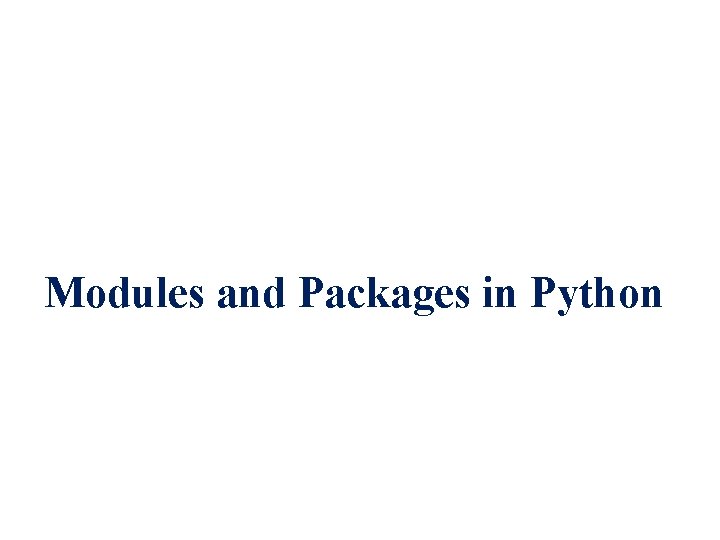
Modules and Packages in Python
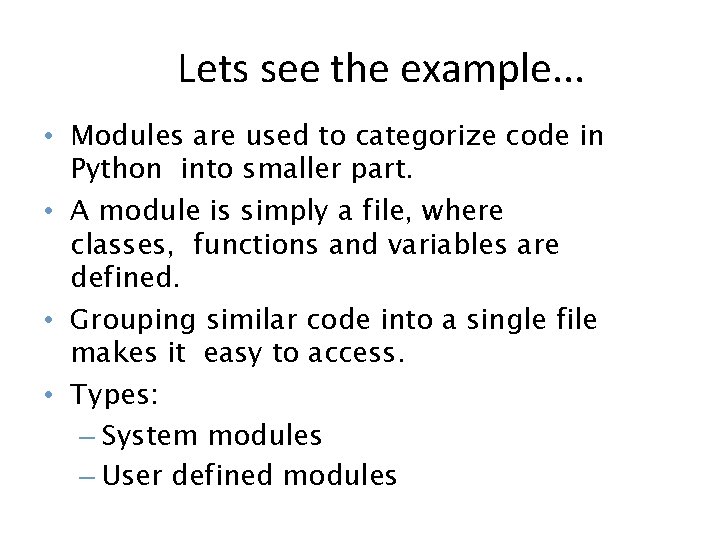
Lets see the example. . . • Modules are used to categorize code in Python into smaller part. • A module is simply a file, where classes, functions and variables are defined. • Grouping similar code into a single file makes it easy to access. • Types: – System modules – User defined modules
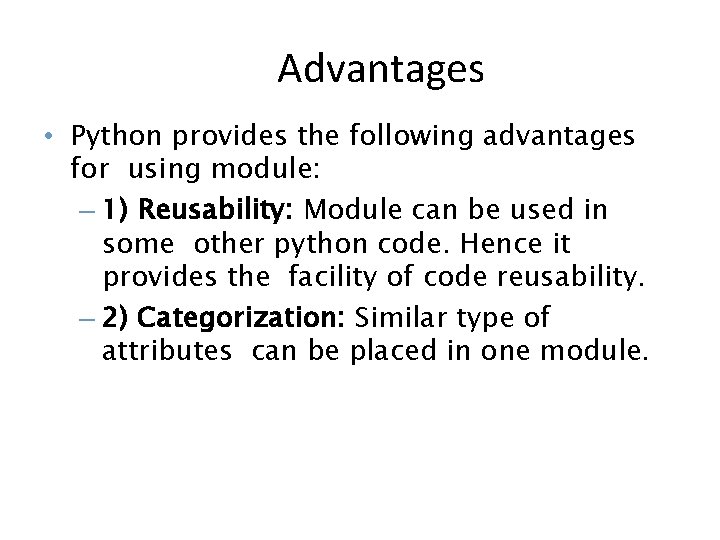
Advantages • Python provides the following advantages for using module: – 1) Reusability: Module can be used in some other python code. Hence it provides the facility of code reusability. – 2) Categorization: Similar type of attributes can be placed in one module.
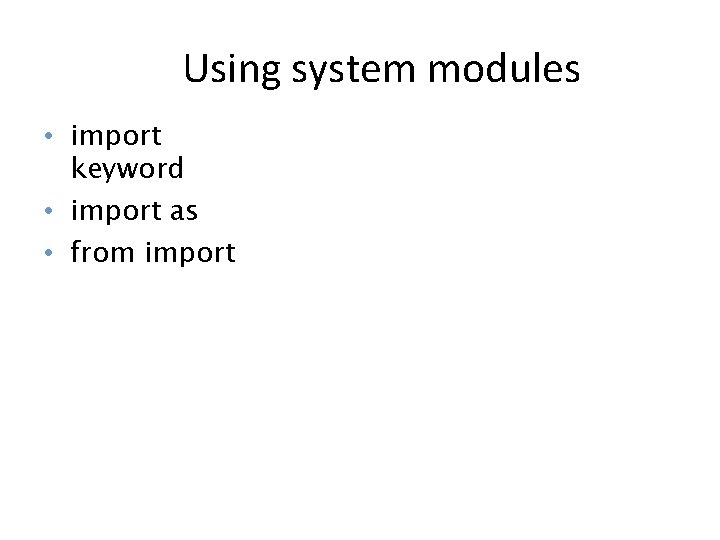
Using system modules • import keyword • import as • from import
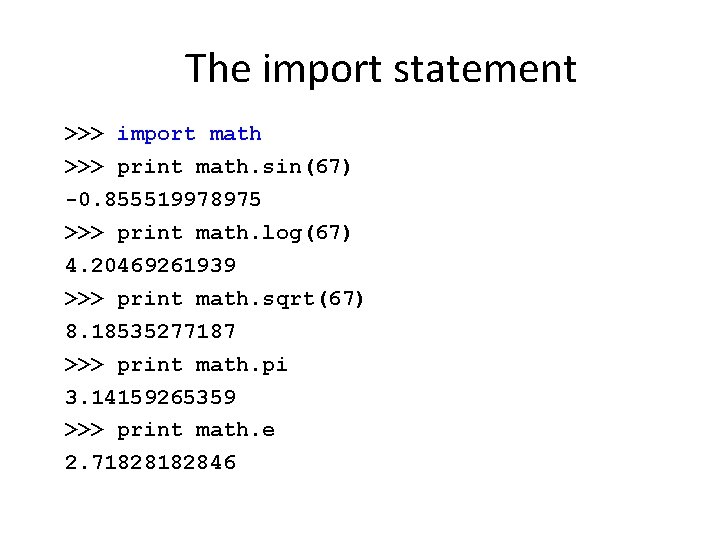
The import statement >>> import math >>> print math. sin(67) 0. 855519978975 >>> print math. log(67) 4. 20469261939 >>> print math. sqrt(67) 8. 18535277187 >>> print math. pi 3. 14159265359 >>> print math. e 2. 7182846
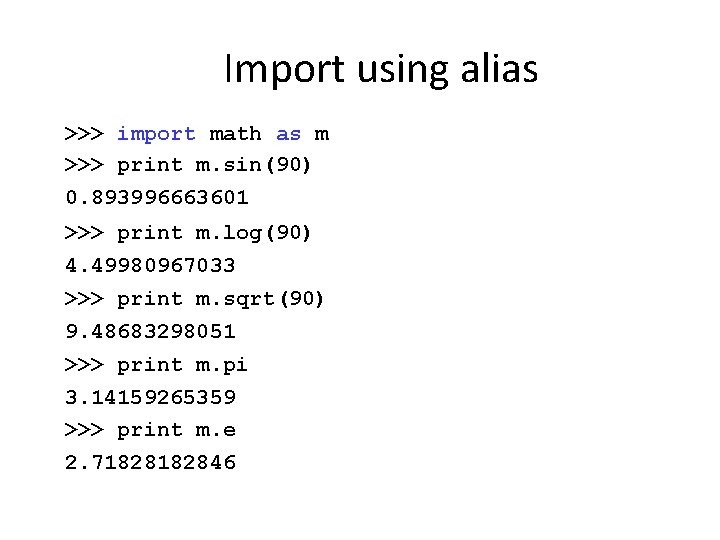
Import using alias >>> import math as m >>> print m. sin(90) 0. 893996663601 >>> print m. log(90) 4. 49980967033 >>> print m. sqrt(90) 9. 48683298051 >>> print m. pi 3. 14159265359 >>> print m. e 2. 7182846
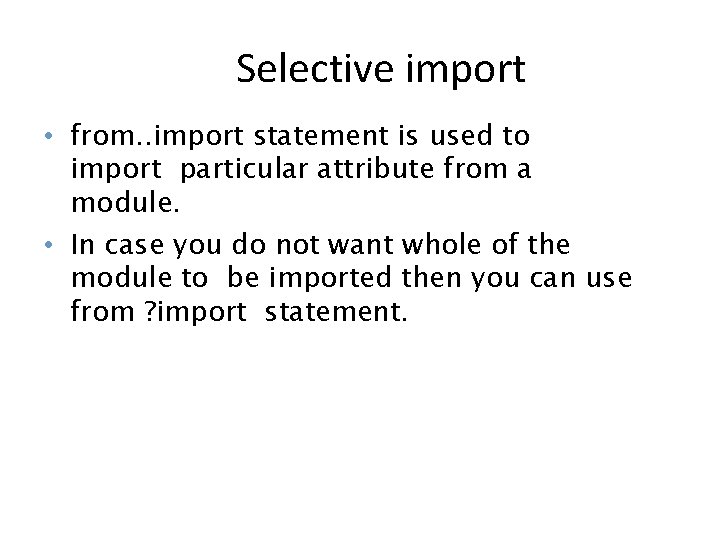
Selective import • from. . import statement is used to import particular attribute from a module. • In case you do not want whole of the module to be imported then you can use from ? import statement.
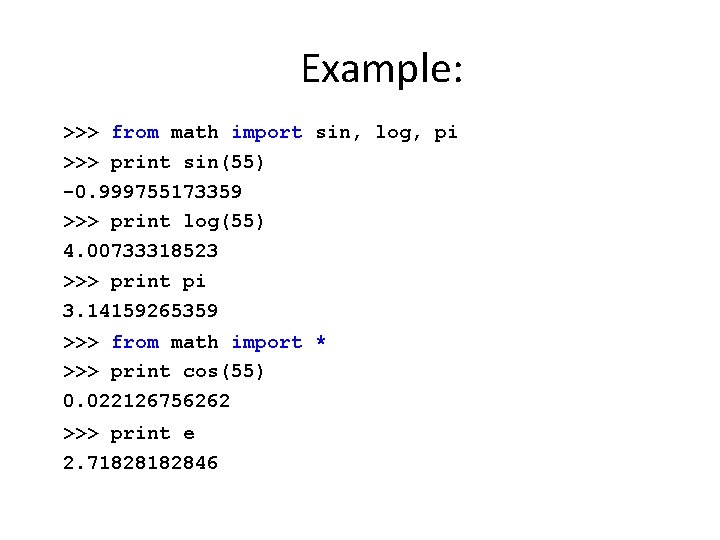
Example: >>> from math import sin, log, pi >>> print sin(55) 0. 999755173359 >>> print log(55) 4. 00733318523 >>> print pi 3. 14159265359 >>> from math import * >>> print cos(55) 0. 022126756262 >>> print e 2. 7182846
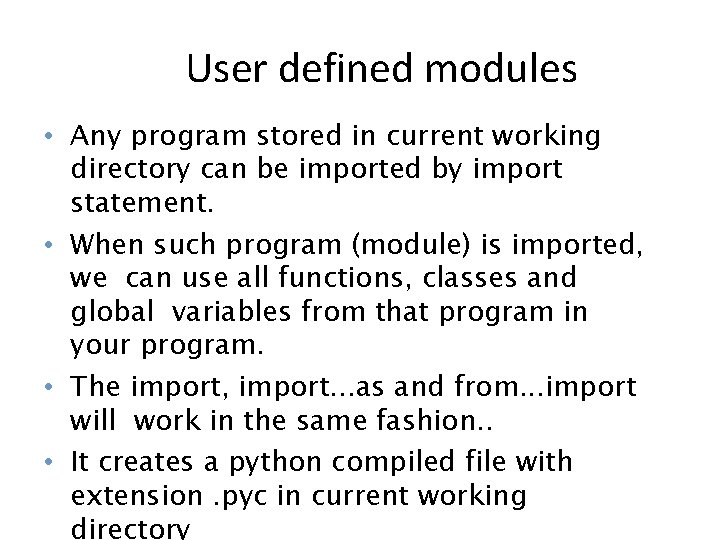
User defined modules • Any program stored in current working directory can be imported by import statement. • When such program (module) is imported, we can use all functions, classes and global variables from that program in your program. • The import, import. . . as and from. . . import will work in the same fashion. . • It creates a python compiled file with extension. pyc in current working directory
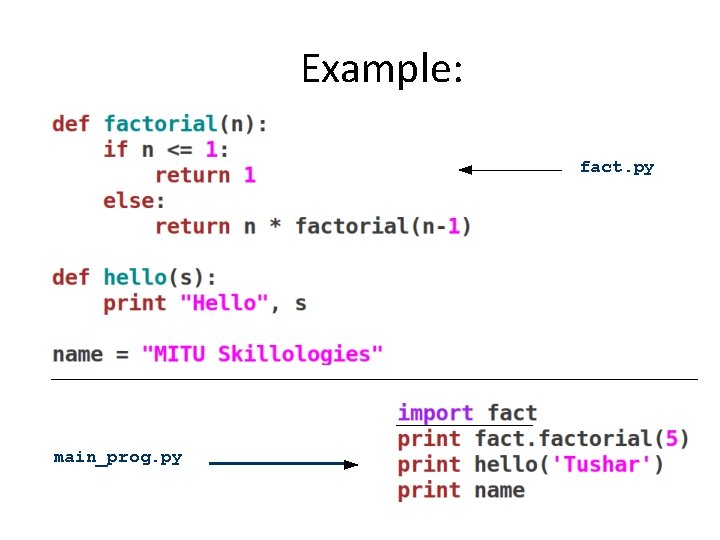
Example: fact. py main_prog. py
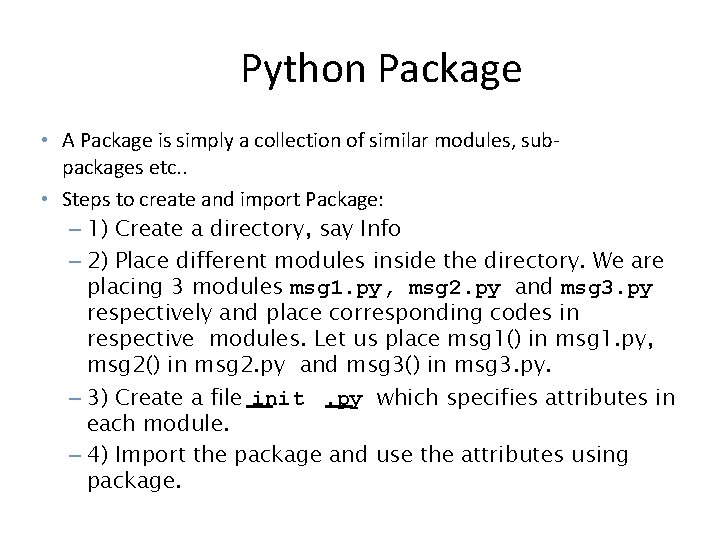
Python Package • A Package is simply a collection of similar modules, subpackages etc. . • Steps to create and import Package: – 1) Create a directory, say Info – 2) Place different modules inside the directory. We are placing 3 modules msg 1. py, msg 2. py and msg 3. py respectively and place corresponding codes in respective modules. Let us place msg 1() in msg 1. py, msg 2() in msg 2. py and msg 3() in msg 3. py. – 3) Create a file init. py which specifies attributes in each module. – 4) Import the package and use the attributes using package.
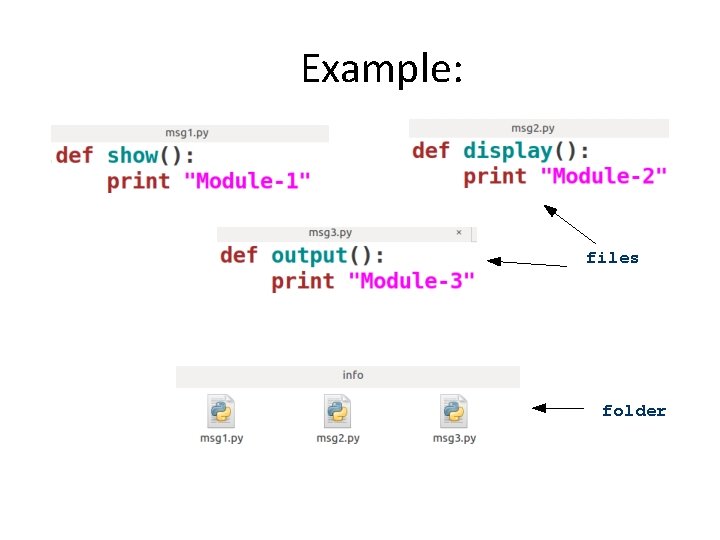
Example: files folder
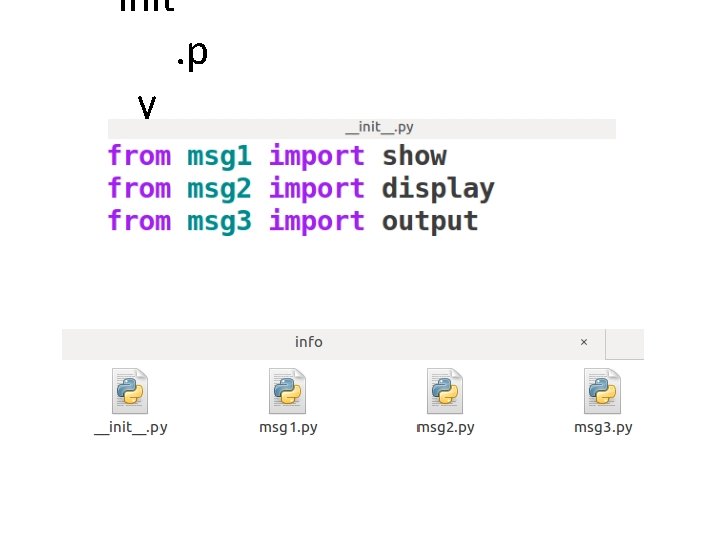
init. p y
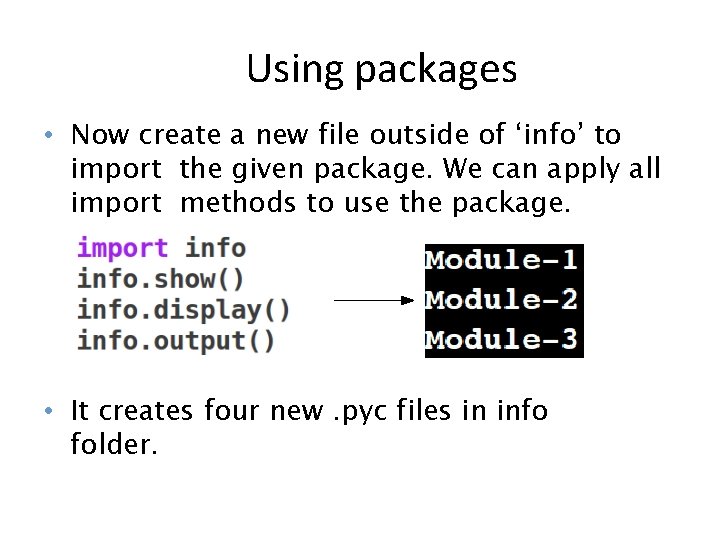
Using packages • Now create a new file outside of ‘info’ to import the given package. We can apply all import methods to use the package. • It creates four new. pyc files in info folder.
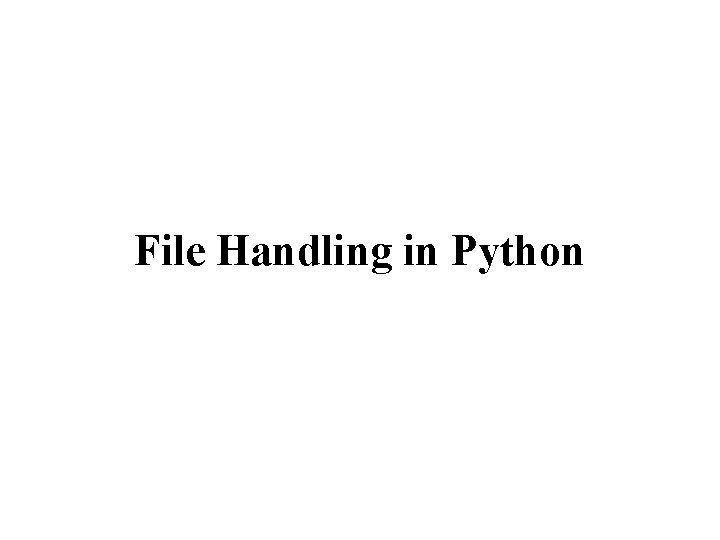
File Handling in Python
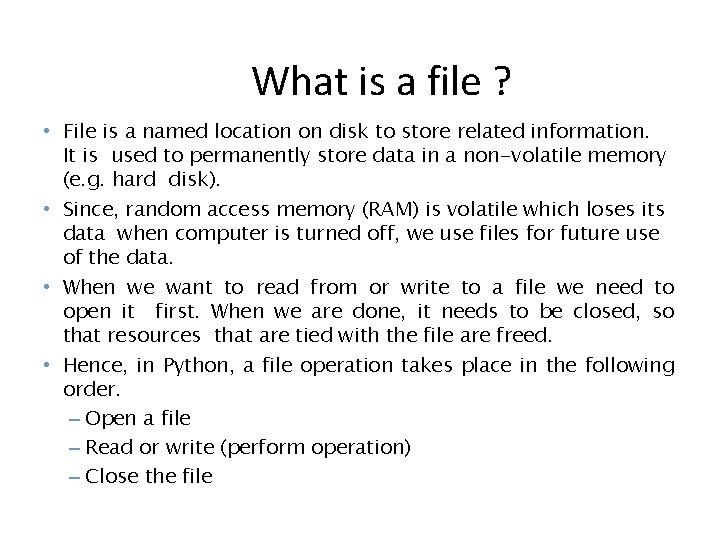
What is a file ? • File is a named location on disk to store related information. It is used to permanently store data in a non-volatile memory (e. g. hard disk). • Since, random access memory (RAM) is volatile which loses its data when computer is turned off, we use files for future use of the data. • When we want to read from or write to a file we need to open it first. When we are done, it needs to be closed, so that resources that are tied with the file are freed. • Hence, in Python, a file operation takes place in the following order. – Open a file – Read or write (perform operation) – Close the file
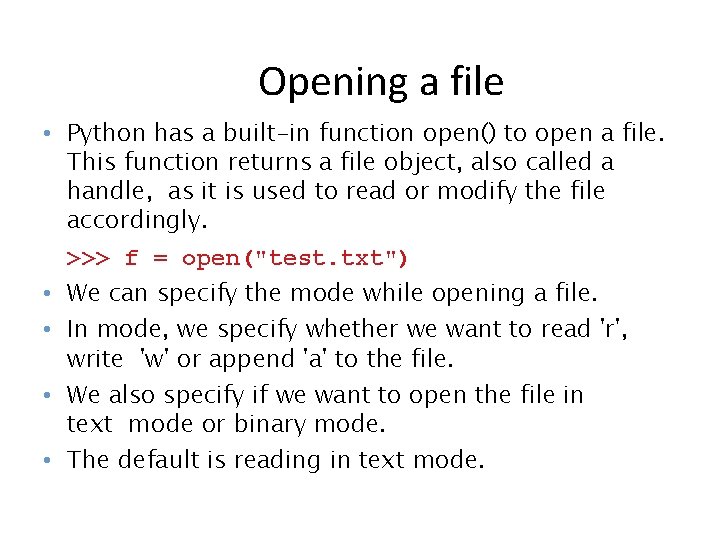
Opening a file • Python has a built-in function open() to open a file. This function returns a file object, also called a handle, as it is used to read or modify the file accordingly. >>> f = open("test. txt") • We can specify the mode while opening a file. • In mode, we specify whether we want to read 'r', write 'w' or append 'a' to the file. • We also specify if we want to open the file in text mode or binary mode. • The default is reading in text mode.
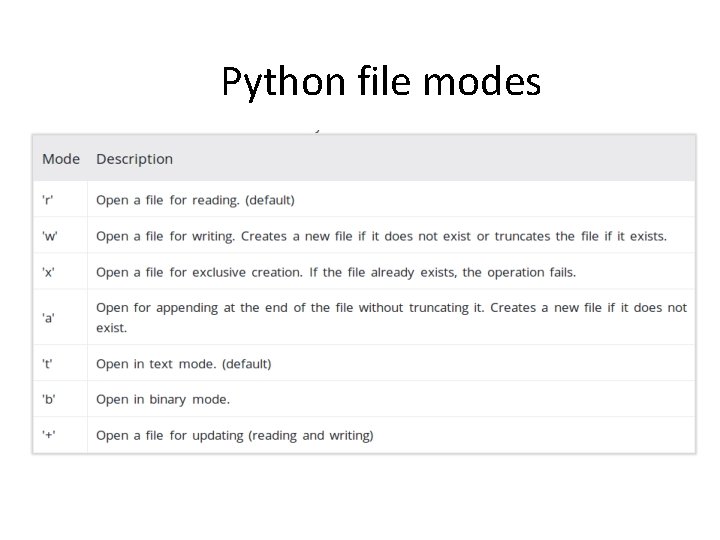
Python file modes
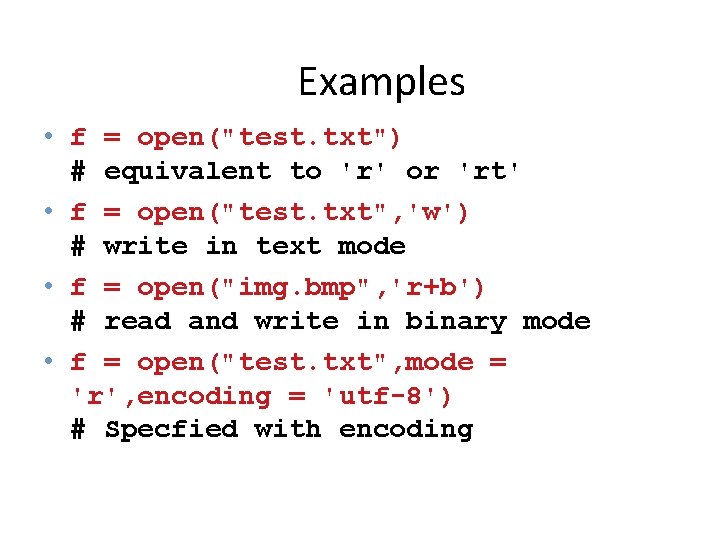
Examples • f = open("test. txt") # equivalent to 'r' or 'rt' • f = open("test. txt", 'w') # write in text mode • f = open("img. bmp", 'r+b') # read and write in binary mode • f = open("test. txt", mode = 'r', encoding = 'utf 8') # Specfied with encoding
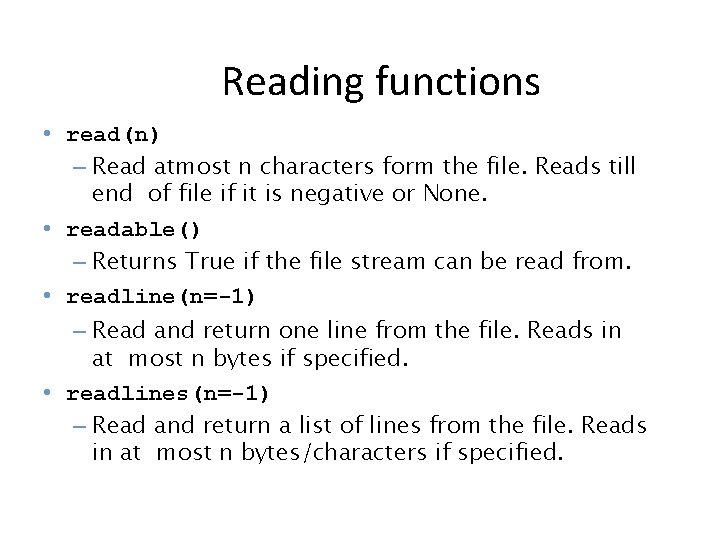
Reading functions • read(n) – Read atmost n characters form the file. Reads till end of file if it is negative or None. • readable() – Returns True if the file stream can be read from. • readline(n= 1) – Read and return one line from the file. Reads in at most n bytes if specified. • readlines(n= 1) – Read and return a list of lines from the file. Reads in at most n bytes/characters if specified.
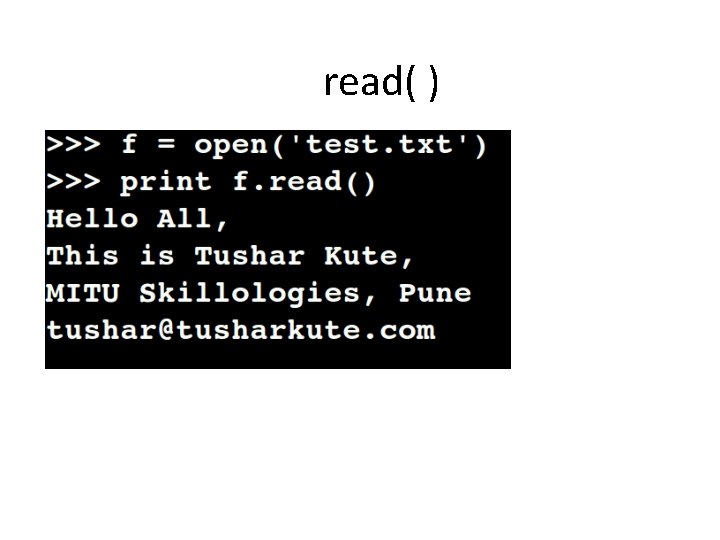
read( )
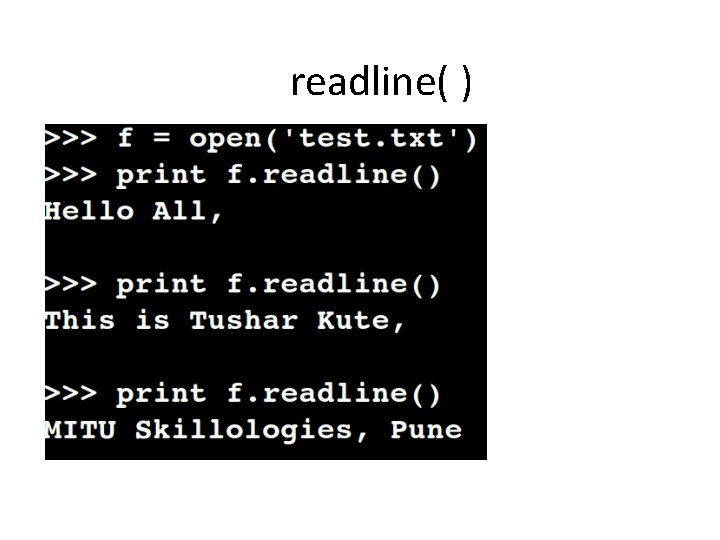
readline( )
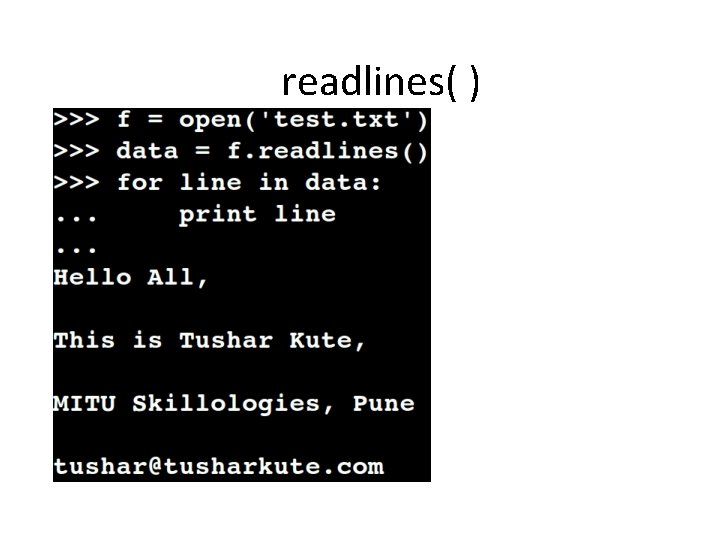
readlines( )
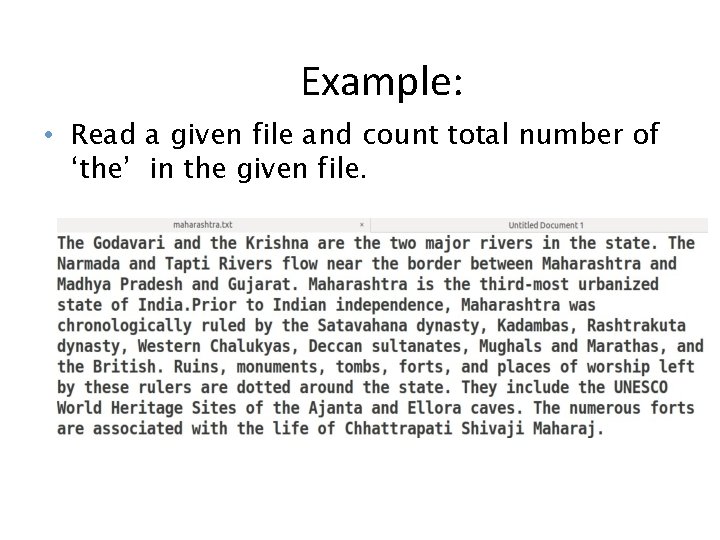
Example: • Read a given file and count total number of ‘the’ in the given file.
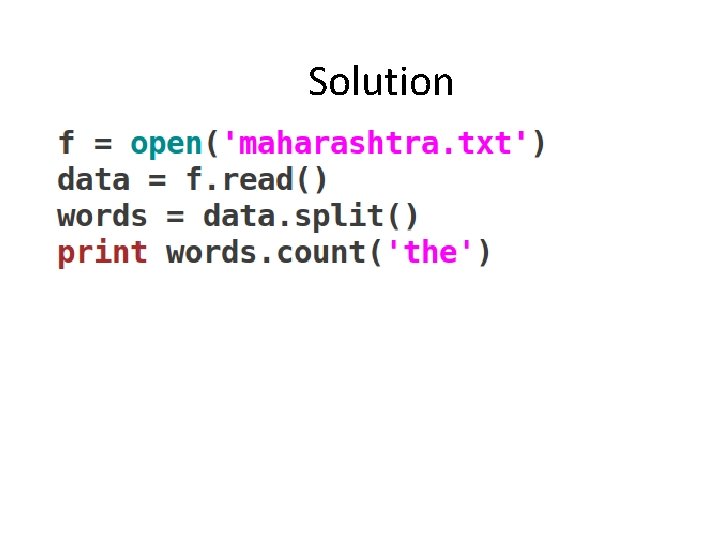
Solution
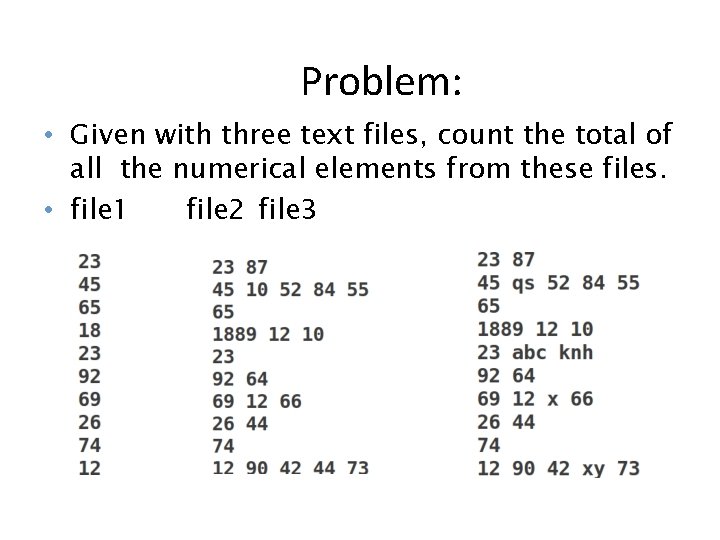
Problem: • Given with three text files, count the total of all the numerical elements from these files. • file 1 file 2 file 3
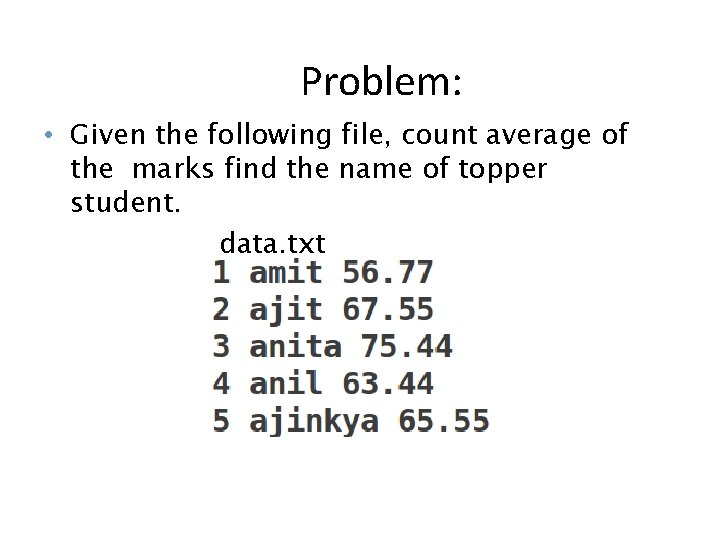
Problem: • Given the following file, count average of the marks find the name of topper student. data. txt
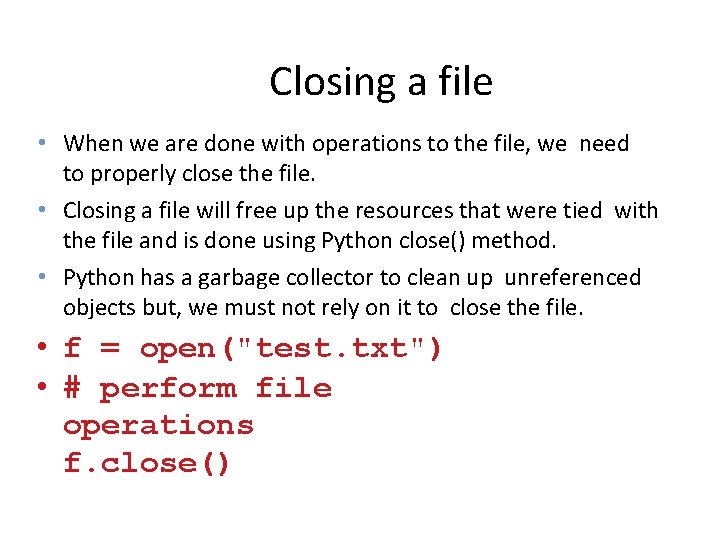
Closing a file • When we are done with operations to the file, we need to properly close the file. • Closing a file will free up the resources that were tied with the file and is done using Python close() method. • Python has a garbage collector to clean up unreferenced objects but, we must not rely on it to close the file. • f = open("test. txt") • # perform file operations f. close()
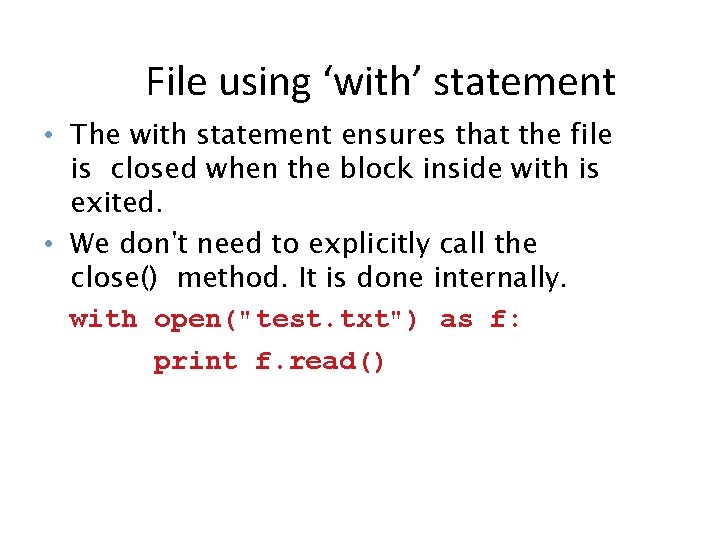
File using ‘with’ statement • The with statement ensures that the file is closed when the block inside with is exited. • We don't need to explicitly call the close() method. It is done internally. with open("test. txt") as f: print f. read()
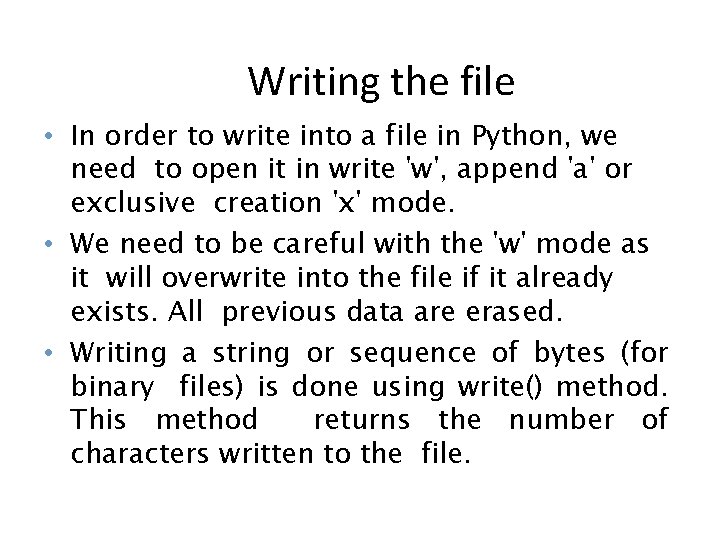
Writing the file • In order to write into a file in Python, we need to open it in write 'w', append 'a' or exclusive creation 'x' mode. • We need to be careful with the 'w' mode as it will overwrite into the file if it already exists. All previous data are erased. • Writing a string or sequence of bytes (for binary files) is done using write() method. This method returns the number of characters written to the file.
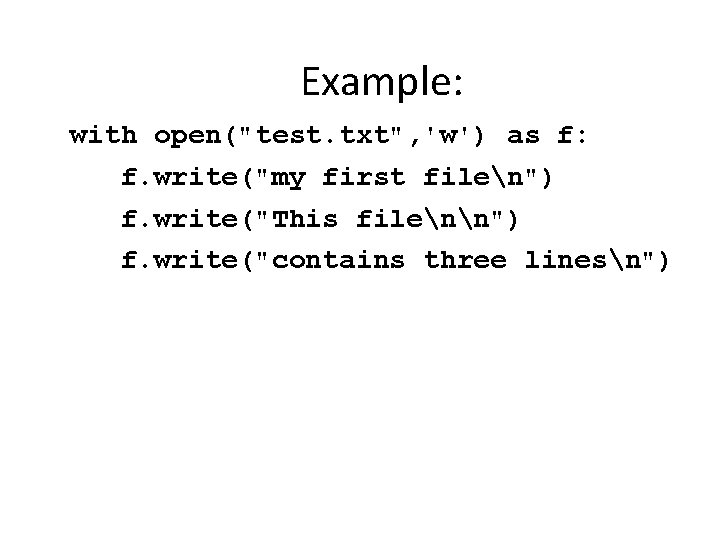
Example: with open("test. txt", 'w') as f: f. write("my first filen") f. write("This filenn") f. write("contains three linesn")
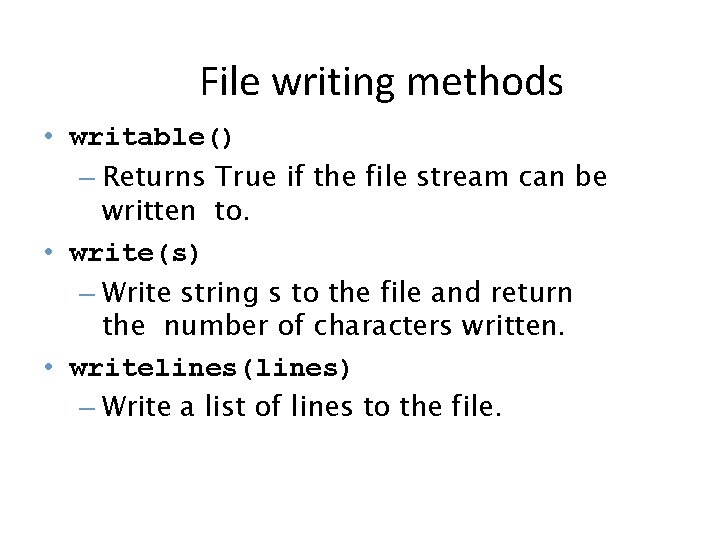
File writing methods • writable() – Returns True if the file stream can be written to. • write(s) – Write string s to the file and return the number of characters written. • writelines(lines) – Write a list of lines to the file.
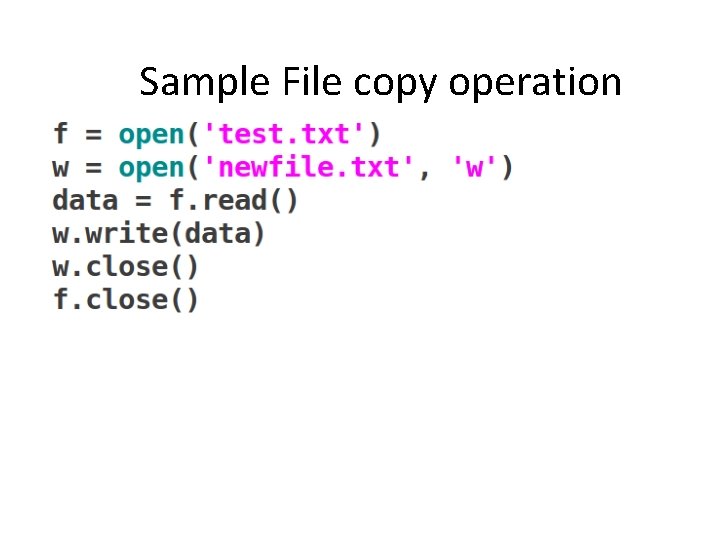
Sample File copy operation
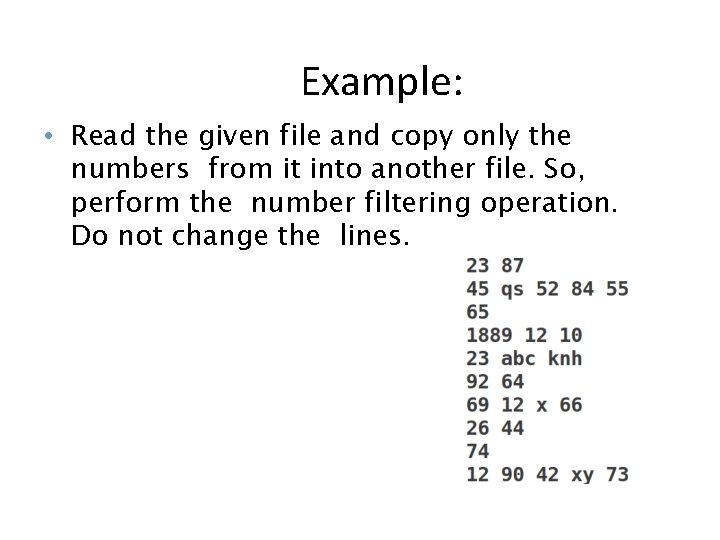
Example: • Read the given file and copy only the numbers from it into another file. So, perform the number filtering operation. Do not change the lines.
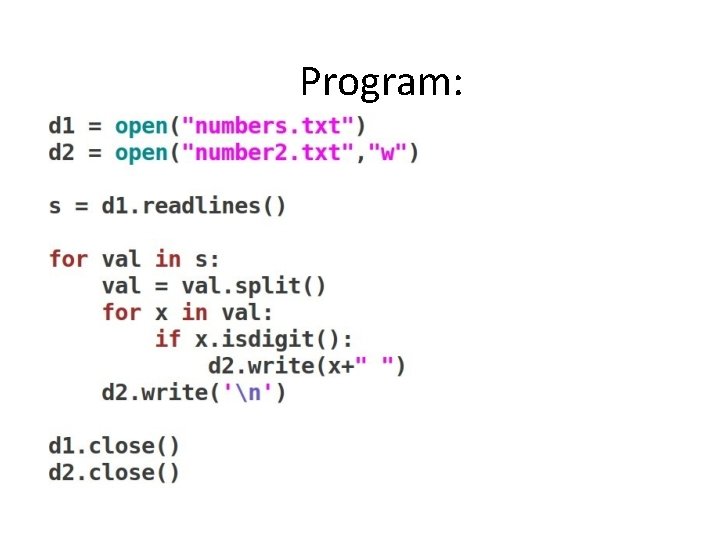
Program:
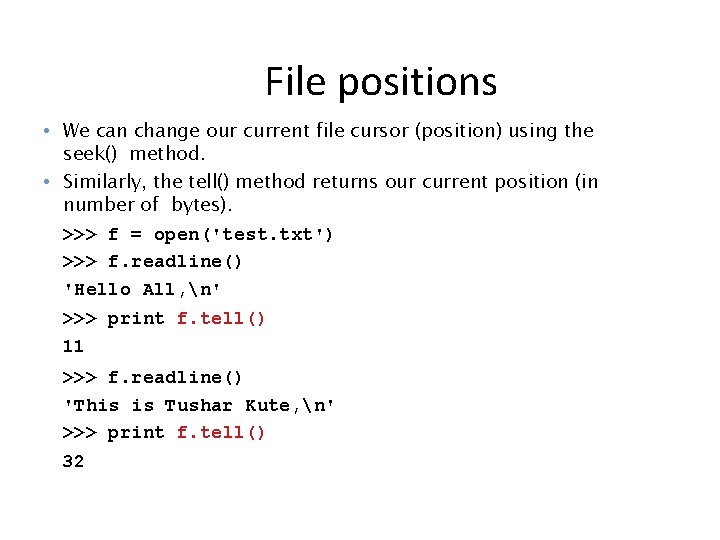
File positions • We can change our current file cursor (position) using the seek() method. • Similarly, the tell() method returns our current position (in number of bytes). >>> f = open('test. txt') >>> f. readline() 'Hello All, n' >>> print f. tell() 11 >>> f. readline() 'This is Tushar Kute, n' >>> print f. tell() 32
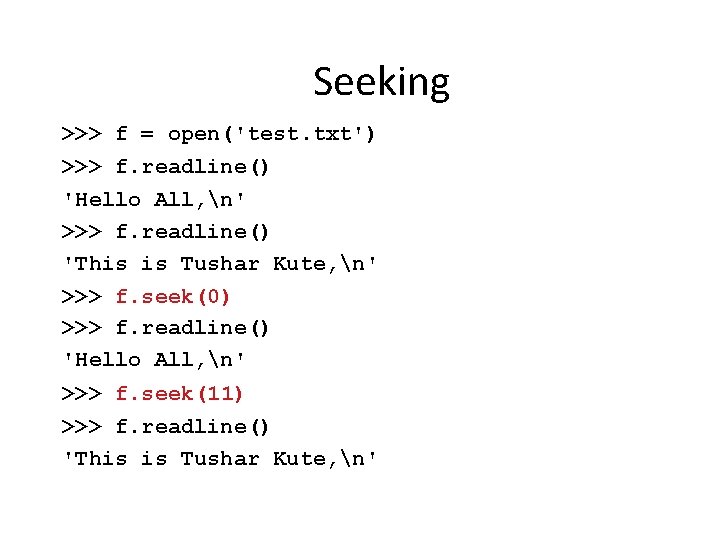
Seeking >>> f = open('test. txt') >>> f. readline() 'Hello All, n' >>> f. readline() 'This is Tushar Kute, n' >>> f. seek(0) >>> f. readline() 'Hello All, n' >>> f. seek(11) >>> f. readline() 'This is Tushar Kute, n'
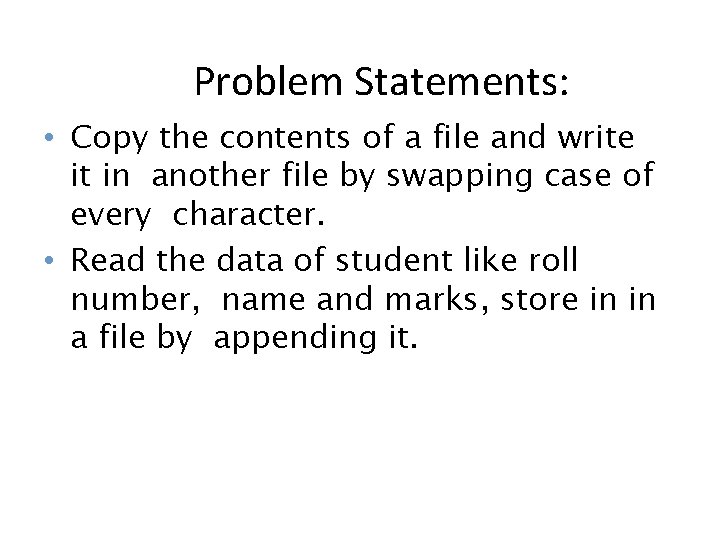
Problem Statements: • Copy the contents of a file and write it in another file by swapping case of every character. • Read the data of student like roll number, name and marks, store in in a file by appending it.
Method vs function python
Methods python list
Pure functions and modifiers in python
Pure function and modifiers in python
Python intrinsic functions
Fruitful function example in python
Fruitful functions in python
What is split function in python
What is a function header in python
Python boolean
Block function python
Def hello()
Eight puzzle problem in python
What is non fruitful function in python
Tower of hanoi python
A python function definition begins with
Bmi function python
Python function without return statement
Absolute value piecewise function
How to evaluate function
Evaluating functions and operations on functions
Rational functions parent function
Transformations of rational functions
Exponential function parent function
Function notation vocabulary
Python nww
Ergm python
Python full form
Xor in python
Arabic wordnet python
Hitran python
Tres en raya python
String list tuple dictionary in python
Scipy audio processing
Potprogrami u pythonu
Golang duck typing
Python flag code
Python flag
Comentar no python
Things fall apart chapter 19
Nelson-siegel model python
The accumulator pattern
Python factoriel
Gethostbyaddr python
Contoh program stack
Terminator algoritma
Python string buffer
Stepwise refinement python
Python documentation generator sphinx
Complex float python
Vrest medical
Right sided binding in python
Python supply chain optimization
Python scheme
Re.finditer in python
Re.findall in python
Survival trees python
Kinetic monte carlo python
Python 64 bit vista
Python cgi
Python turtle圖案
Python sort list of tuples
Python stack and queue