FUNCTIONS PART II 1 Function without return statement
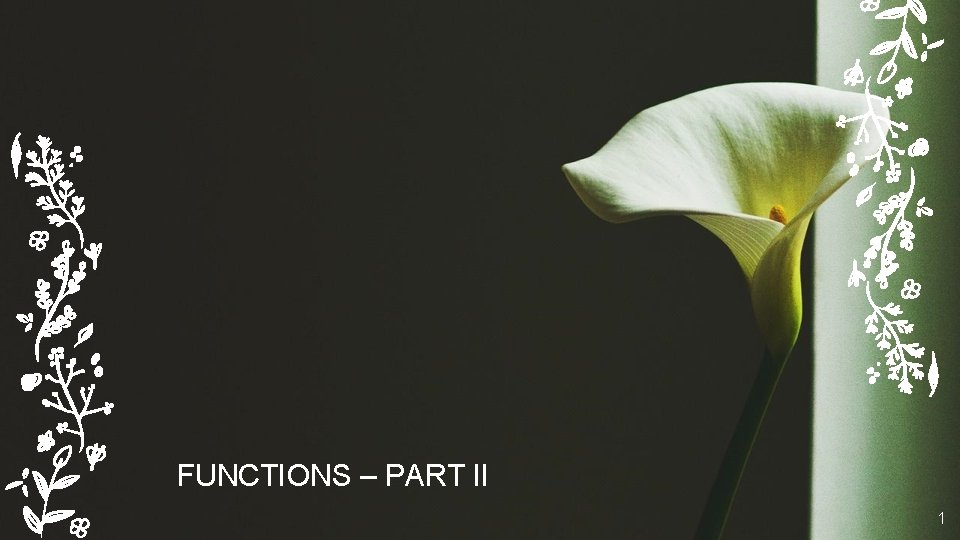
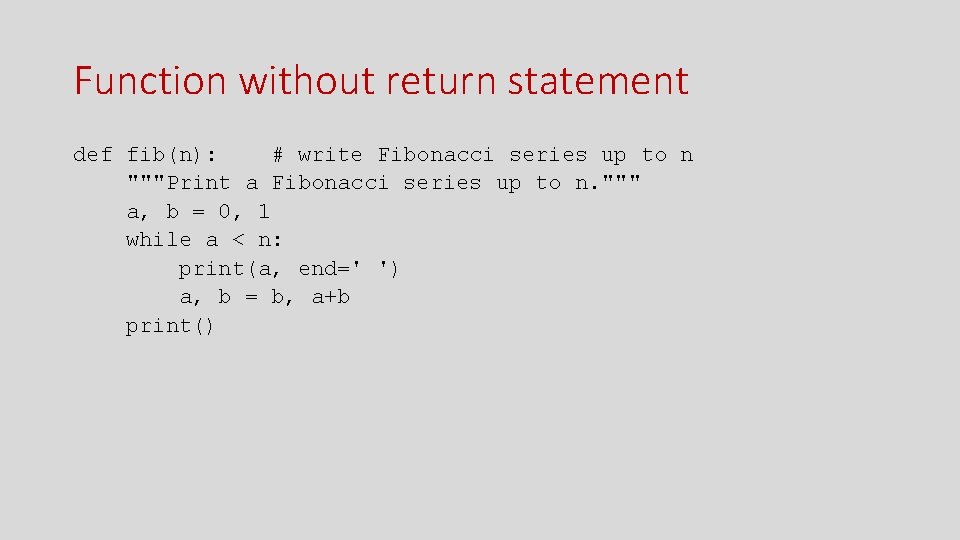
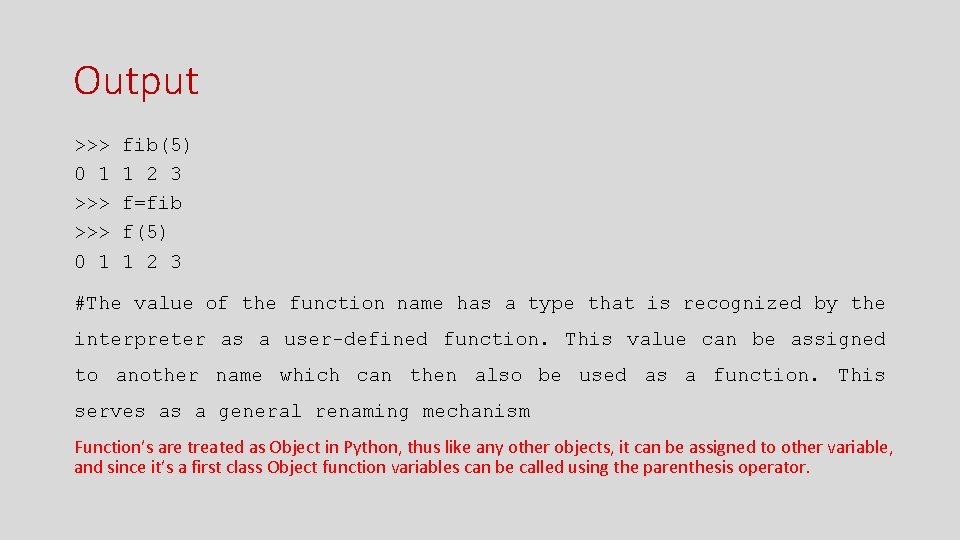
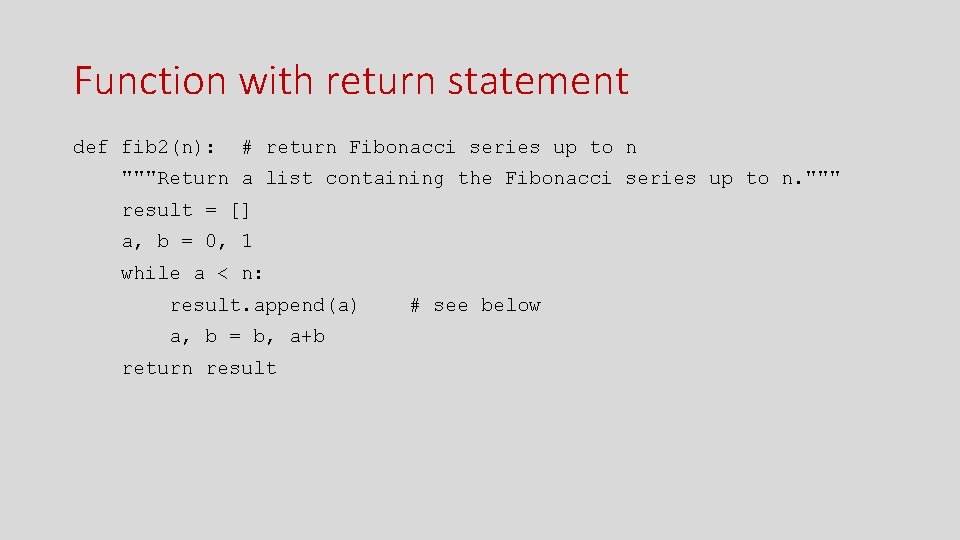
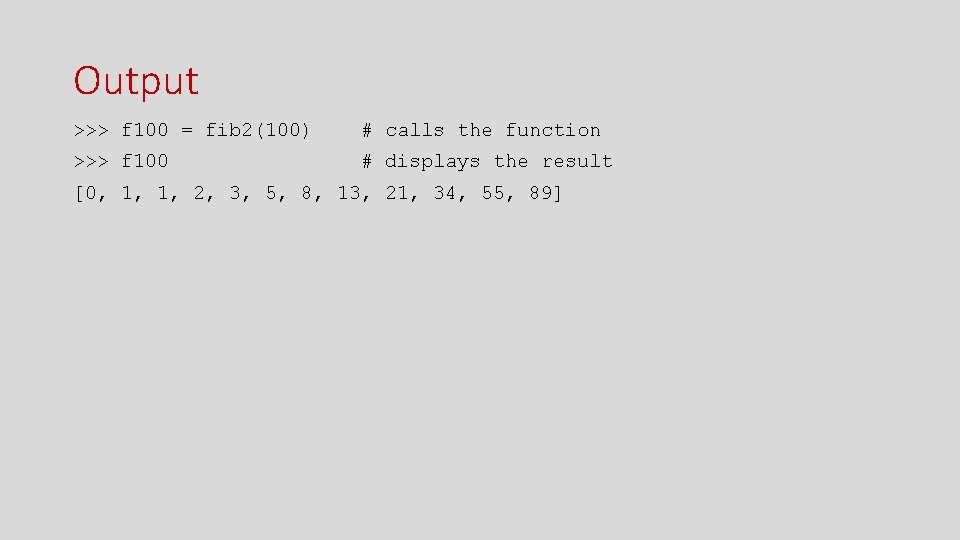
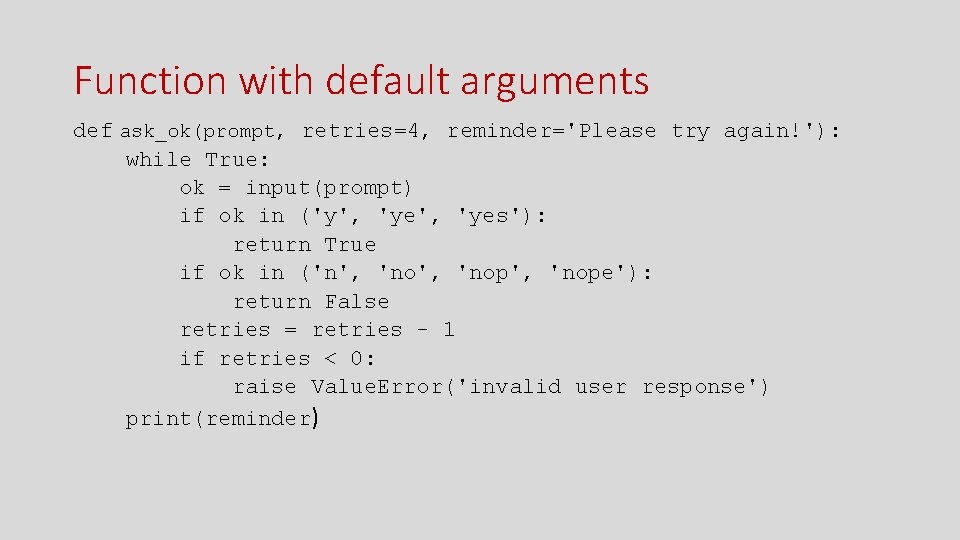
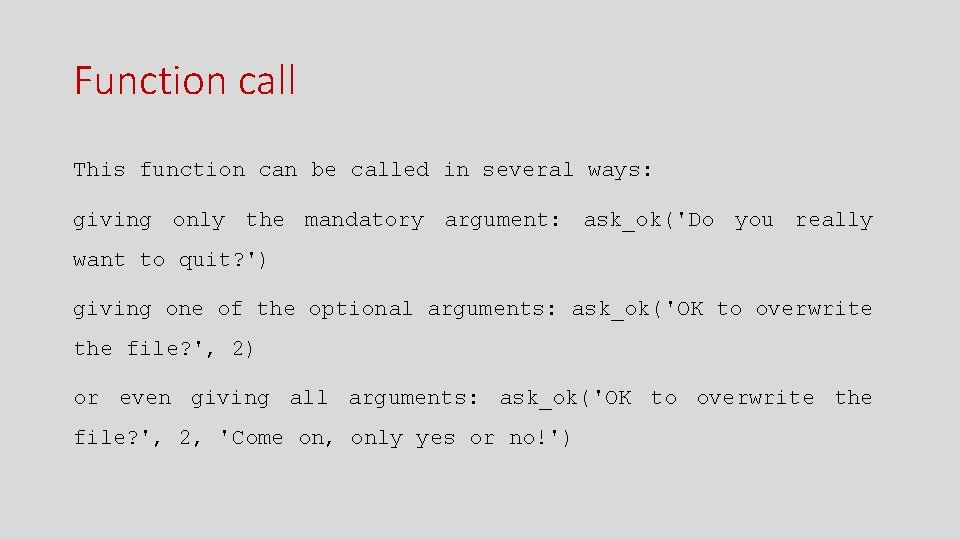
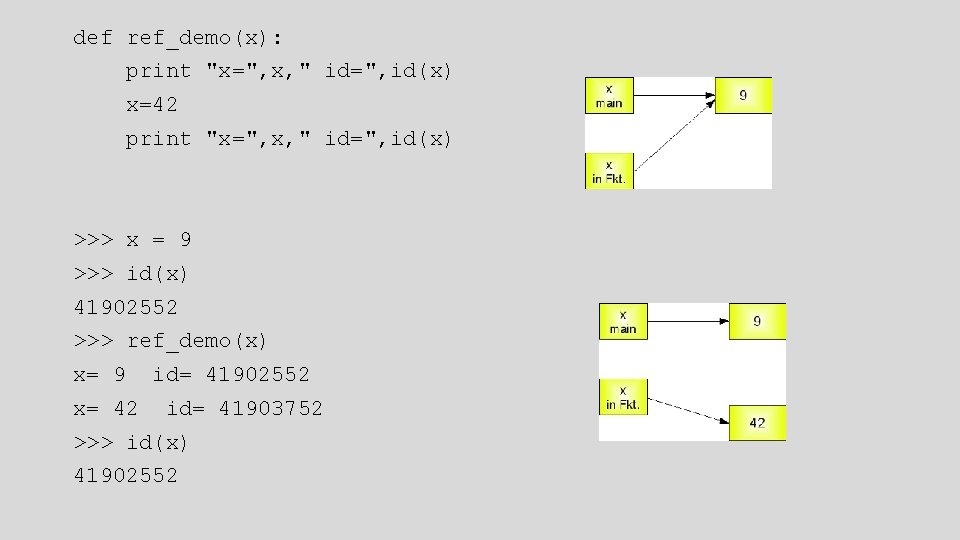
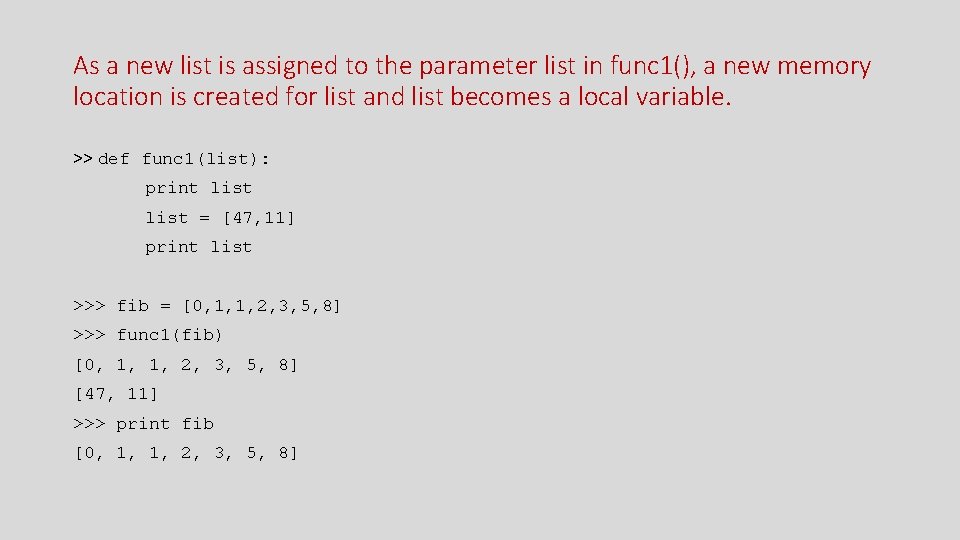
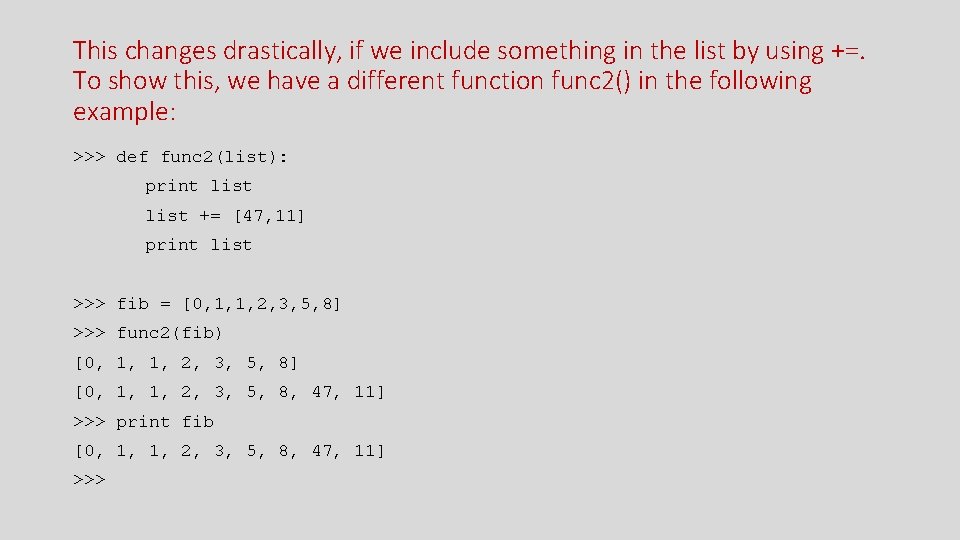
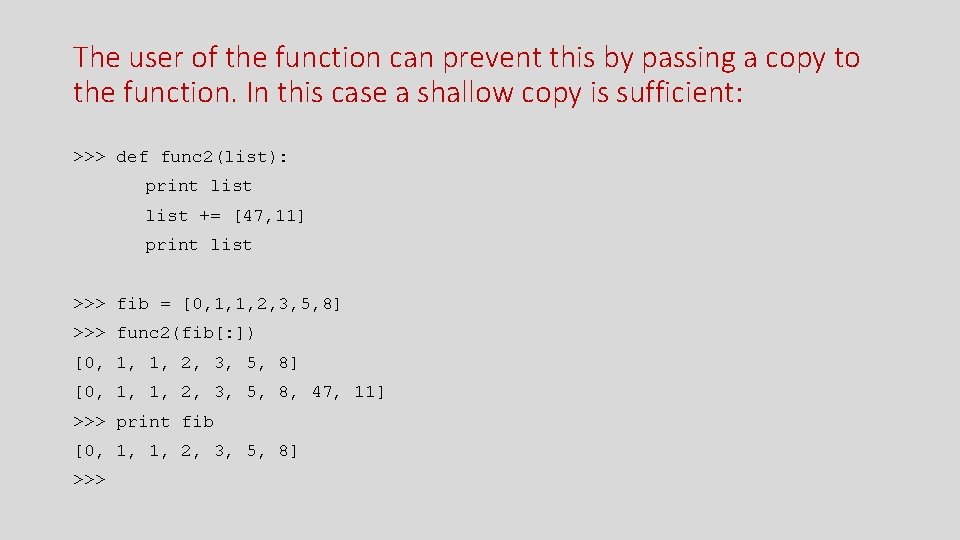
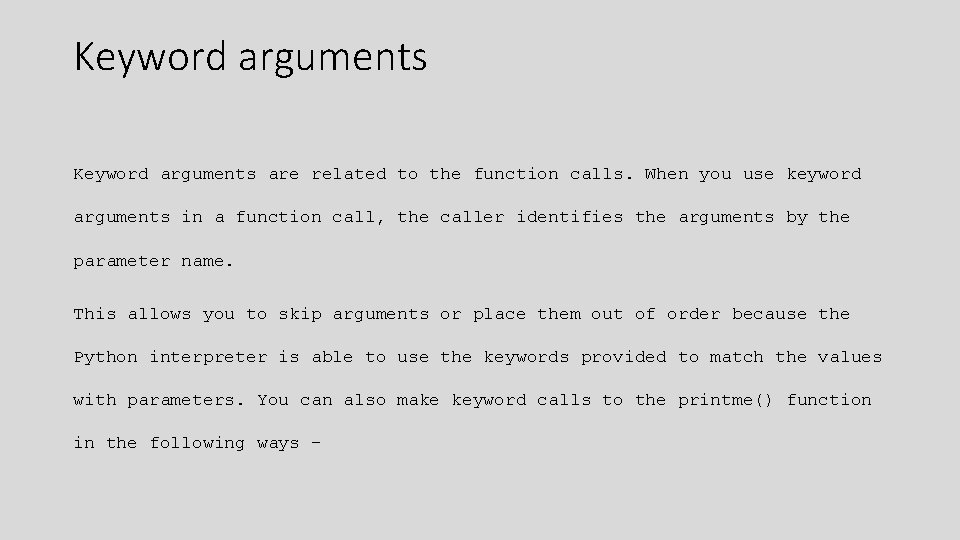
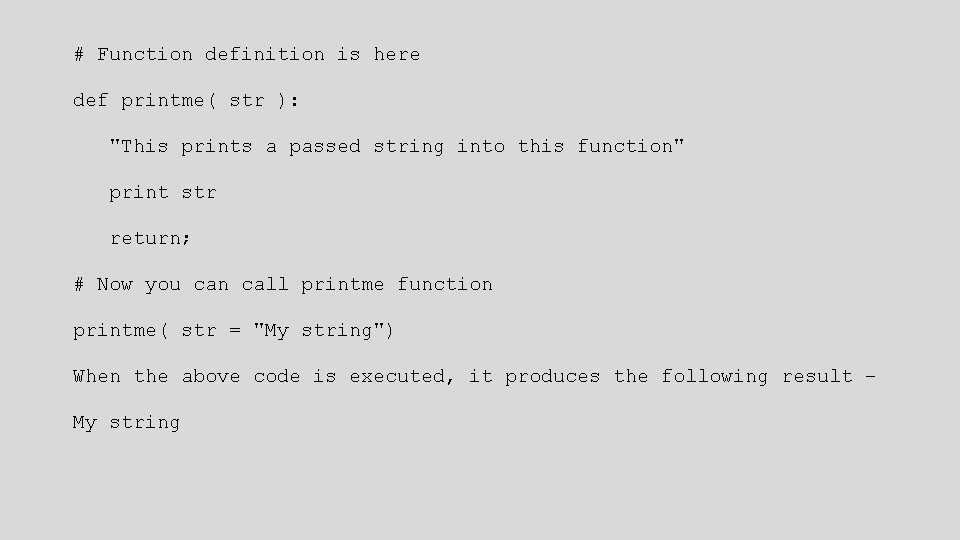
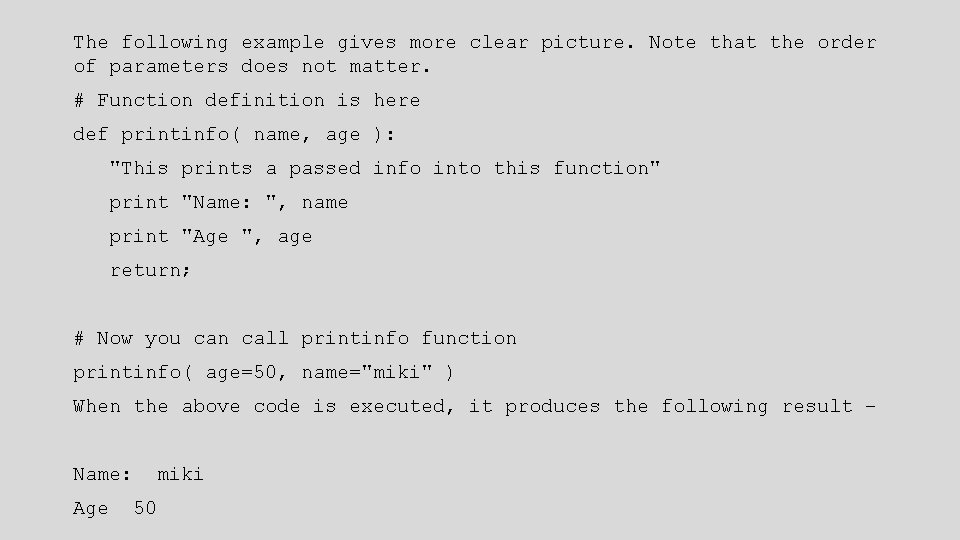
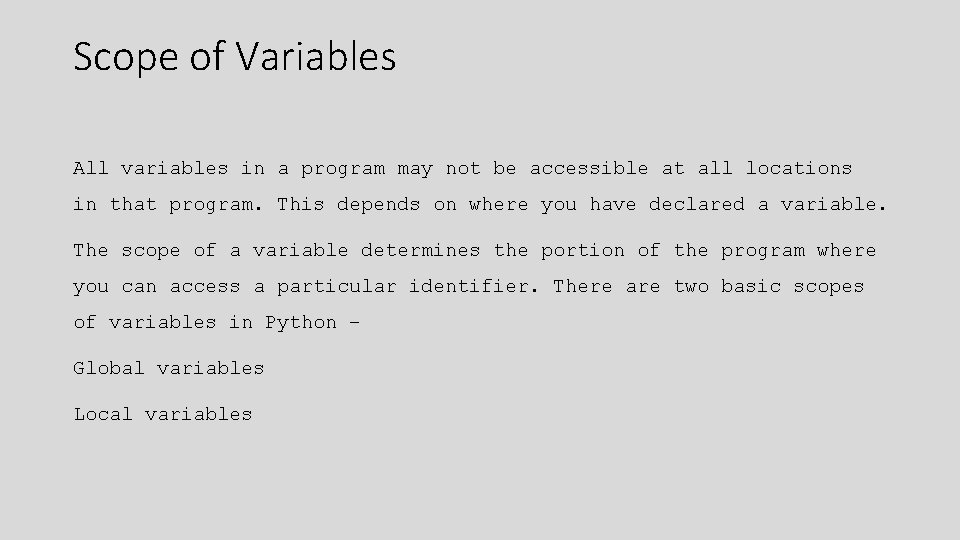
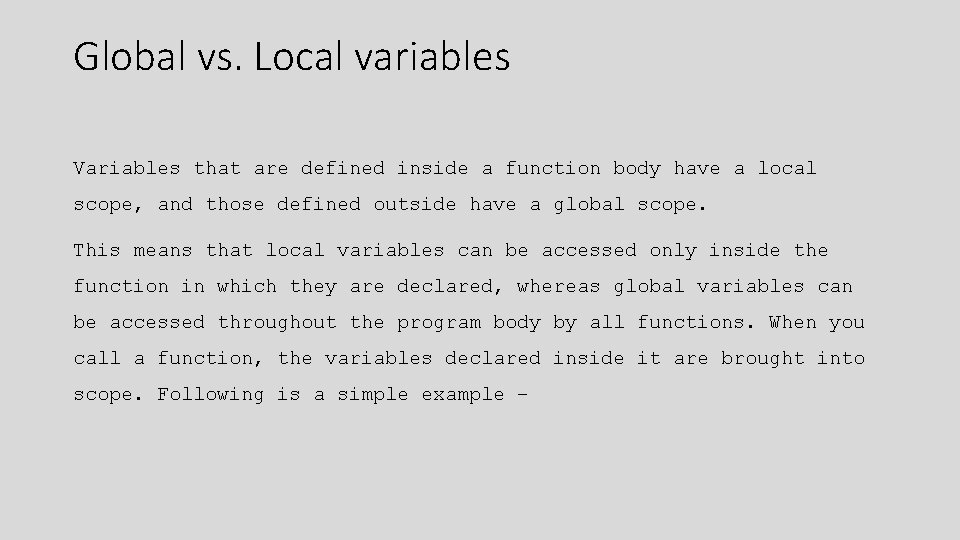
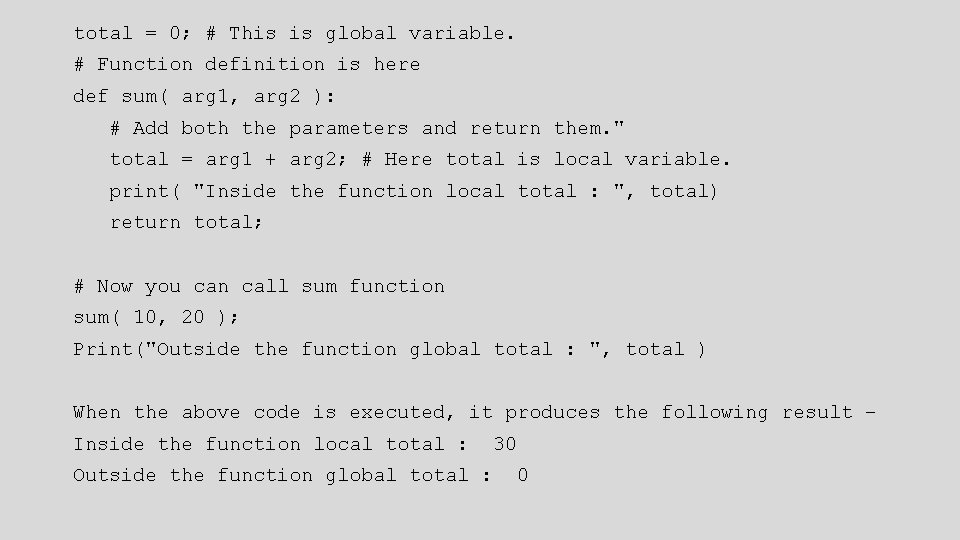
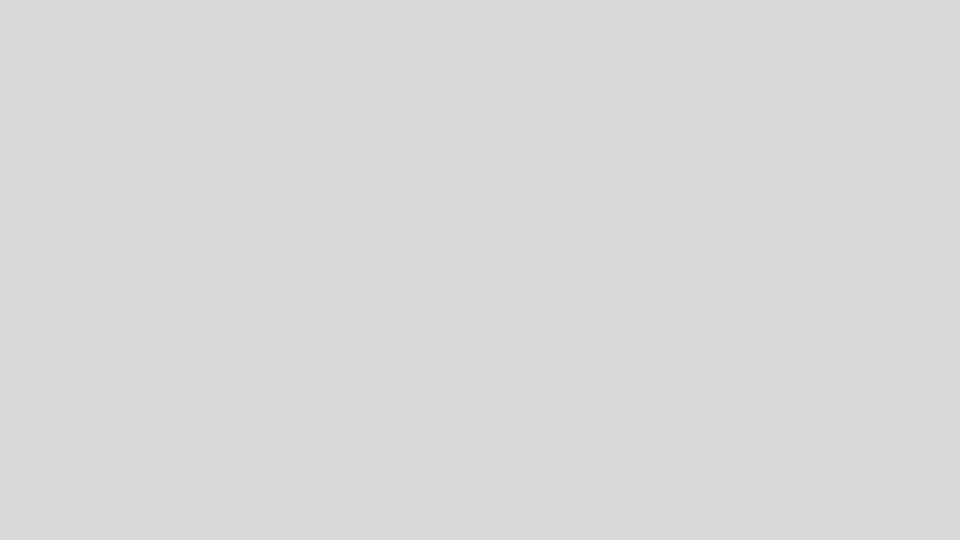
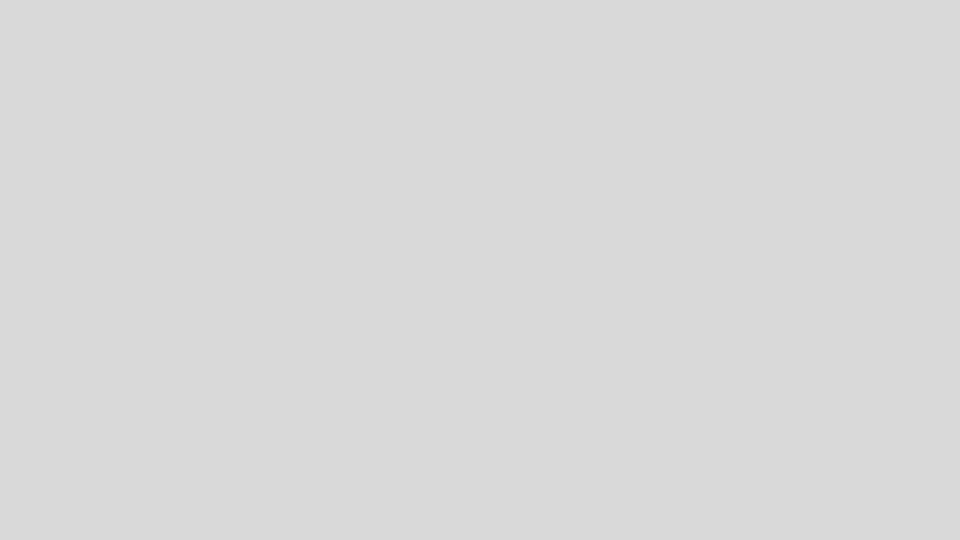
- Slides: 19
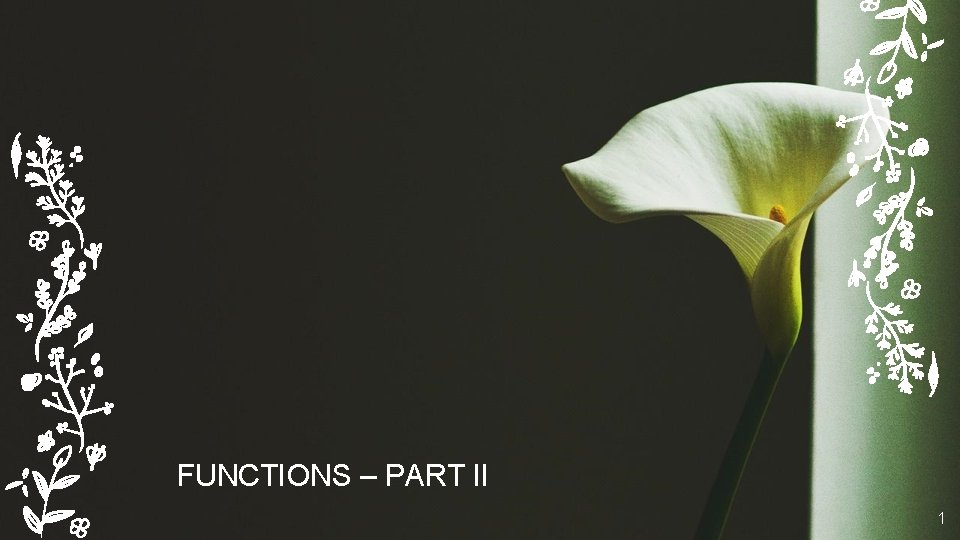
FUNCTIONS – PART II 1
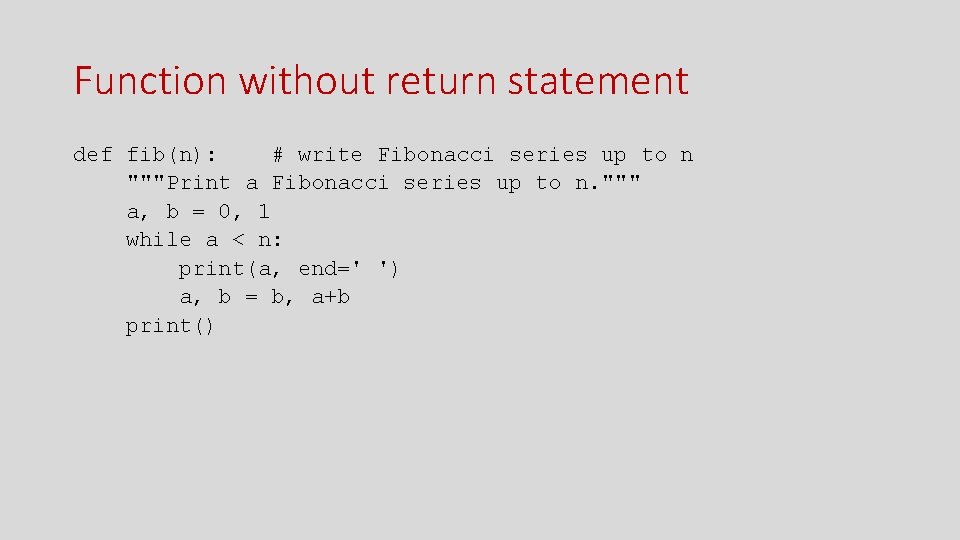
Function without return statement def fib(n): # write Fibonacci series up to n """Print a Fibonacci series up to n. """ a, b = 0, 1 while a < n: print(a, end=' ') a, b = b, a+b print()
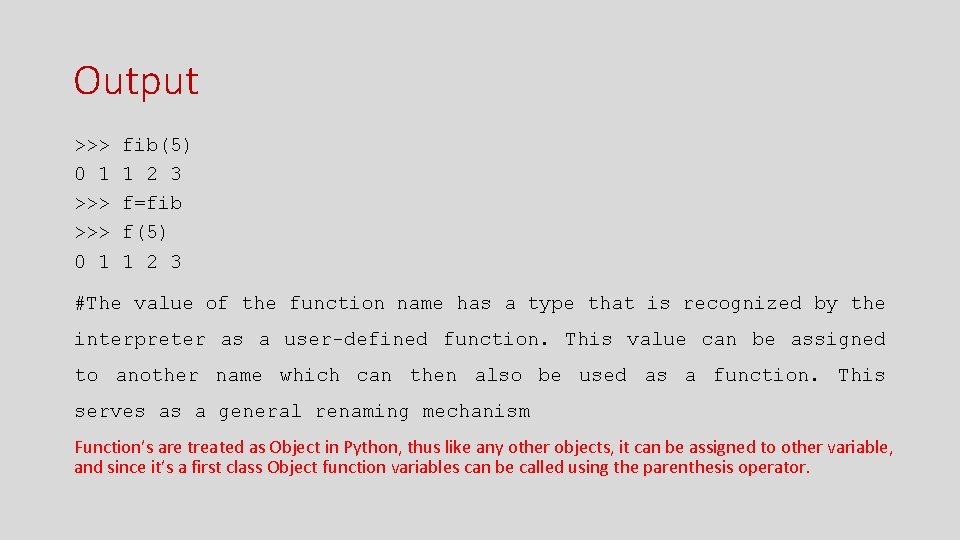
Output >>> fib(5) 0 1 1 2 3 >>> f=fib >>> f(5) 0 1 1 2 3 #The value of the function name has a type that is recognized by the interpreter as a user-defined function. This value can be assigned to another name which can then also be used as a function. This serves as a general renaming mechanism Function’s are treated as Object in Python, thus like any other objects, it can be assigned to other variable, and since it’s a first class Object function variables can be called using the parenthesis operator.
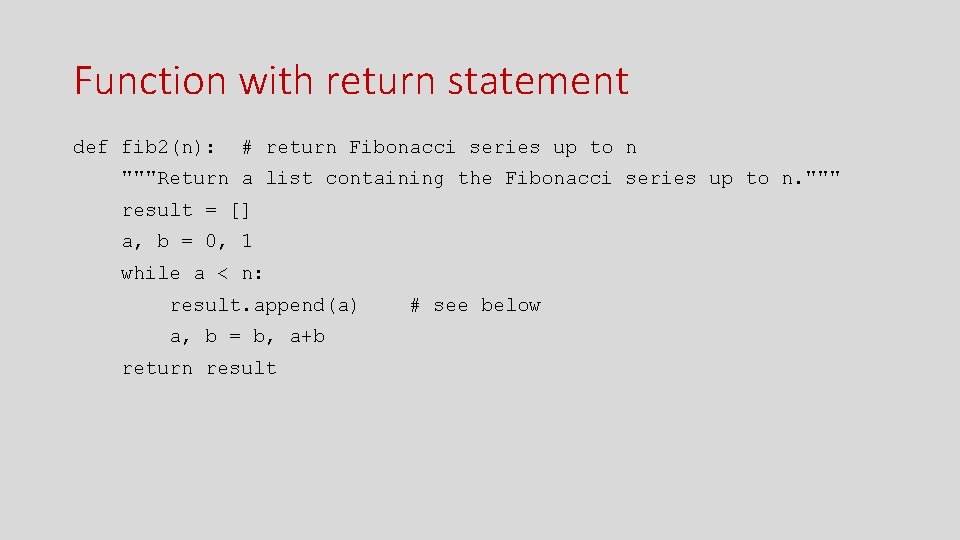
Function with return statement def fib 2(n): # return Fibonacci series up to n """Return a list containing the Fibonacci series up to n. """ result = [] a, b = 0, 1 while a < n: result. append(a) # see below a, b = b, a+b return result
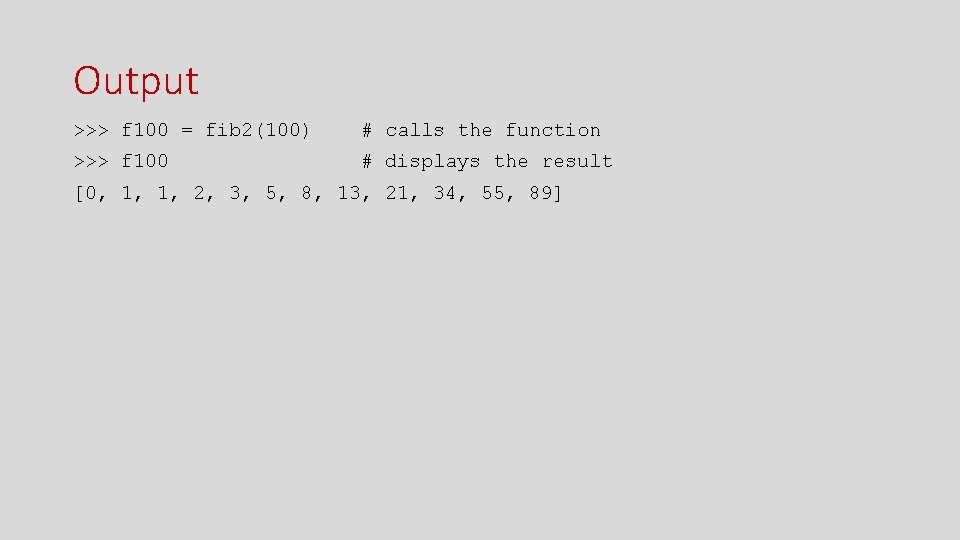
Output >>> f 100 = fib 2(100) # calls the function >>> f 100 # displays the result [0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
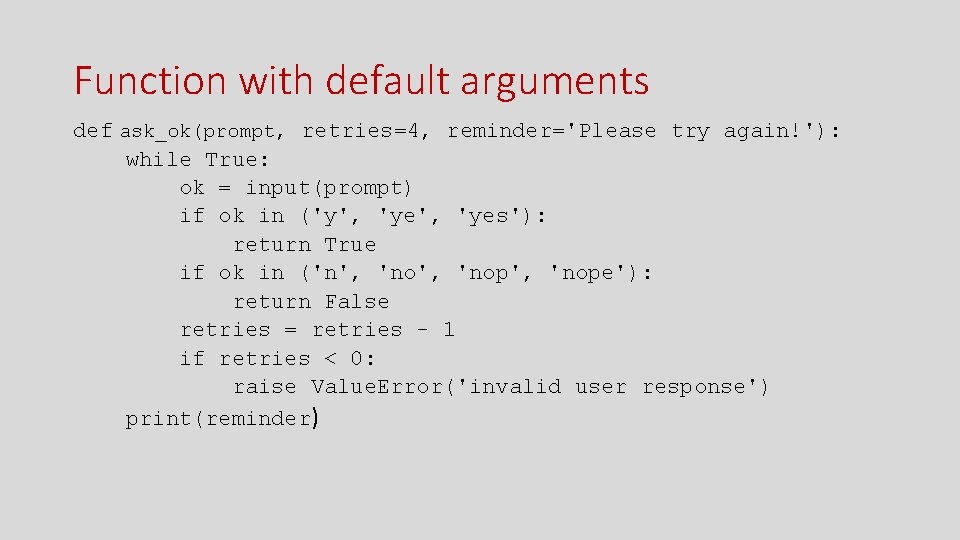
Function with default arguments def ask_ok(prompt, retries=4, reminder='Please try again!'): while True: ok = input(prompt) if ok in ('y', 'yes'): return True if ok in ('n', 'nop', 'nope'): return False retries = retries - 1 if retries < 0: raise Value. Error('invalid user response') print(reminder)
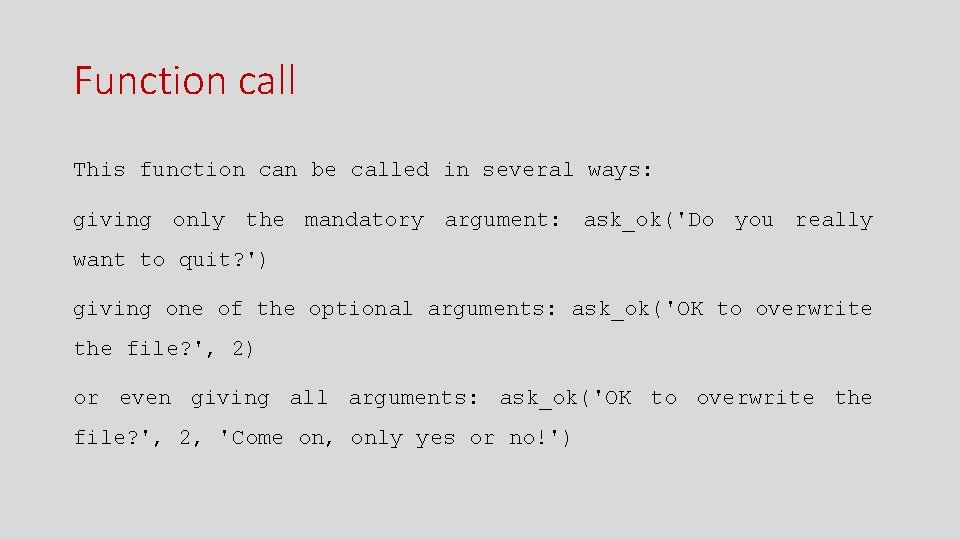
Function call This function can be called in several ways: giving only the mandatory argument: ask_ok('Do you really want to quit? ') giving one of the optional arguments: ask_ok('OK to overwrite the file? ', 2) or even giving all arguments: ask_ok('OK to overwrite the file? ', 2, 'Come on, only yes or no!')
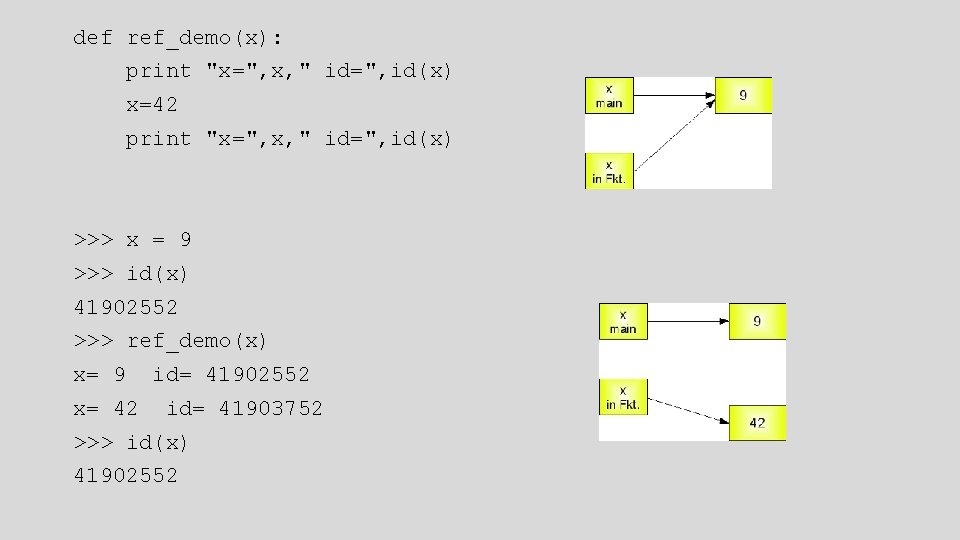
def ref_demo(x): print "x=", x, " id=", id(x) x=42 print "x=", x, " id=", id(x) >>> x = 9 >>> id(x) 41902552 >>> ref_demo(x) x= 9 id= 41902552 x= 42 id= 41903752 >>> id(x) 41902552
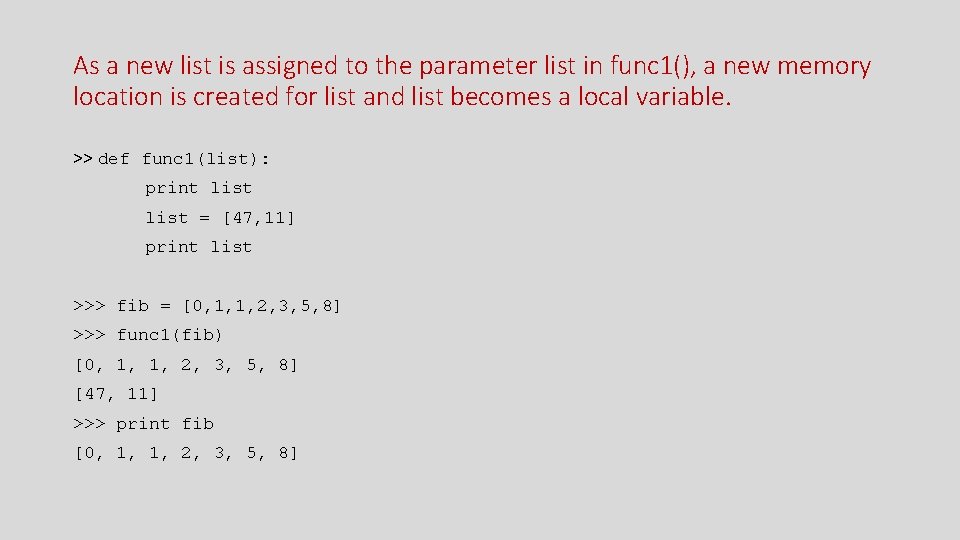
As a new list is assigned to the parameter list in func 1(), a new memory location is created for list and list becomes a local variable. >> def func 1(list): print list = [47, 11] print list >>> fib = [0, 1, 1, 2, 3, 5, 8] >>> func 1(fib) [0, 1, 1, 2, 3, 5, 8] [47, 11] >>> print fib [0, 1, 1, 2, 3, 5, 8]
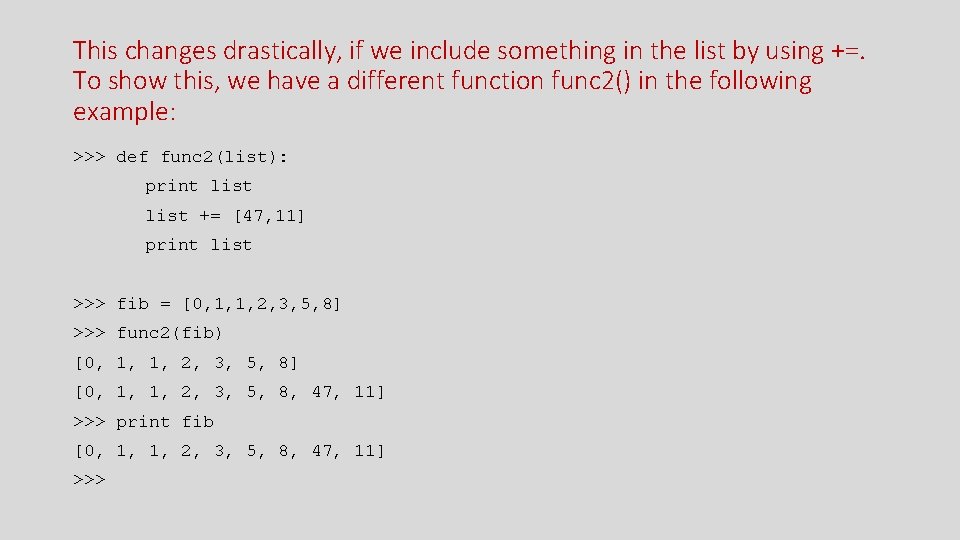
This changes drastically, if we include something in the list by using +=. To show this, we have a different function func 2() in the following example: >>> def func 2(list): print list += [47, 11] print list >>> fib = [0, 1, 1, 2, 3, 5, 8] >>> func 2(fib) [0, 1, 1, 2, 3, 5, 8] [0, 1, 1, 2, 3, 5, 8, 47, 11] >>> print fib [0, 1, 1, 2, 3, 5, 8, 47, 11] >>>
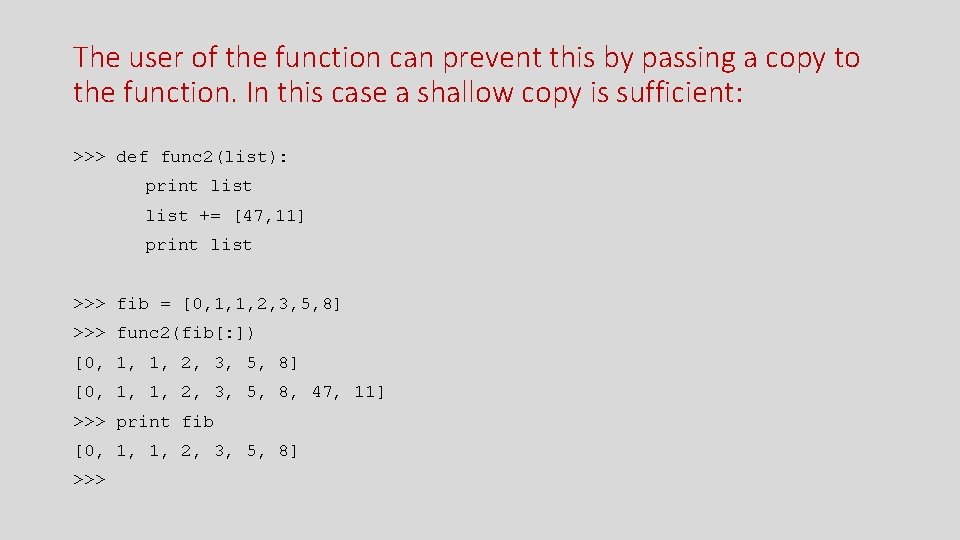
The user of the function can prevent this by passing a copy to the function. In this case a shallow copy is sufficient: >>> def func 2(list): print list += [47, 11] print list >>> fib = [0, 1, 1, 2, 3, 5, 8] >>> func 2(fib[: ]) [0, 1, 1, 2, 3, 5, 8] [0, 1, 1, 2, 3, 5, 8, 47, 11] >>> print fib [0, 1, 1, 2, 3, 5, 8] >>>
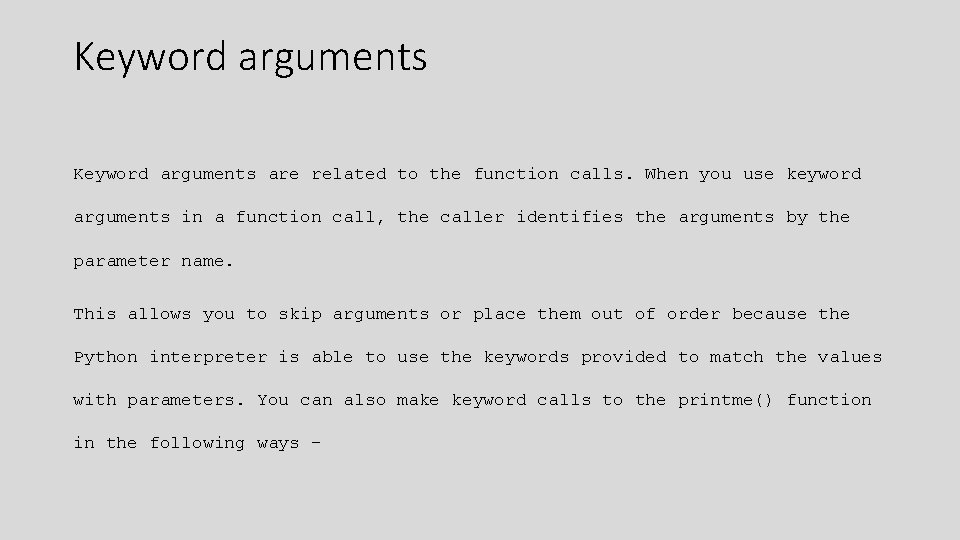
Keyword arguments are related to the function calls. When you use keyword arguments in a function call, the caller identifies the arguments by the parameter name. This allows you to skip arguments or place them out of order because the Python interpreter is able to use the keywords provided to match the values with parameters. You can also make keyword calls to the printme() function in the following ways −
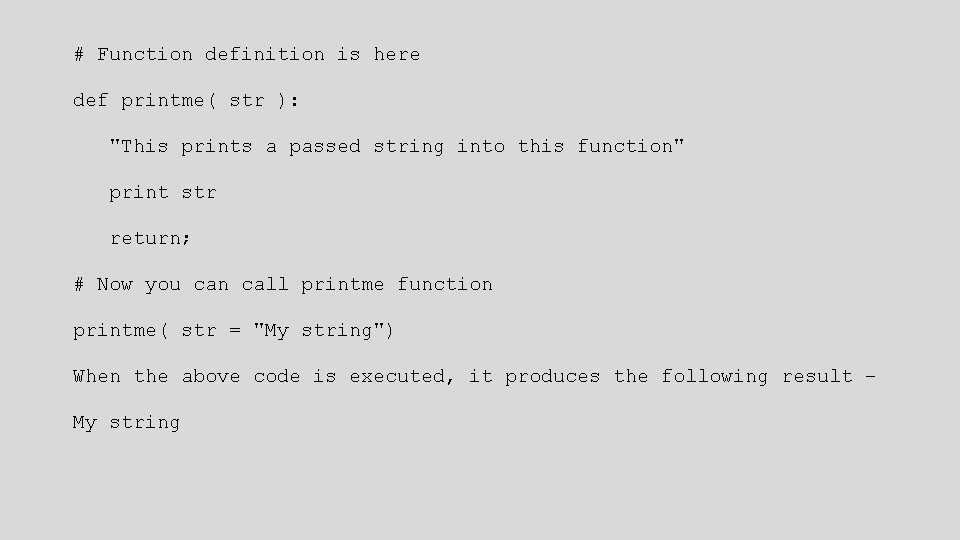
# Function definition is here def printme( str ): "This prints a passed string into this function" print str return; # Now you can call printme function printme( str = "My string") When the above code is executed, it produces the following result − My string
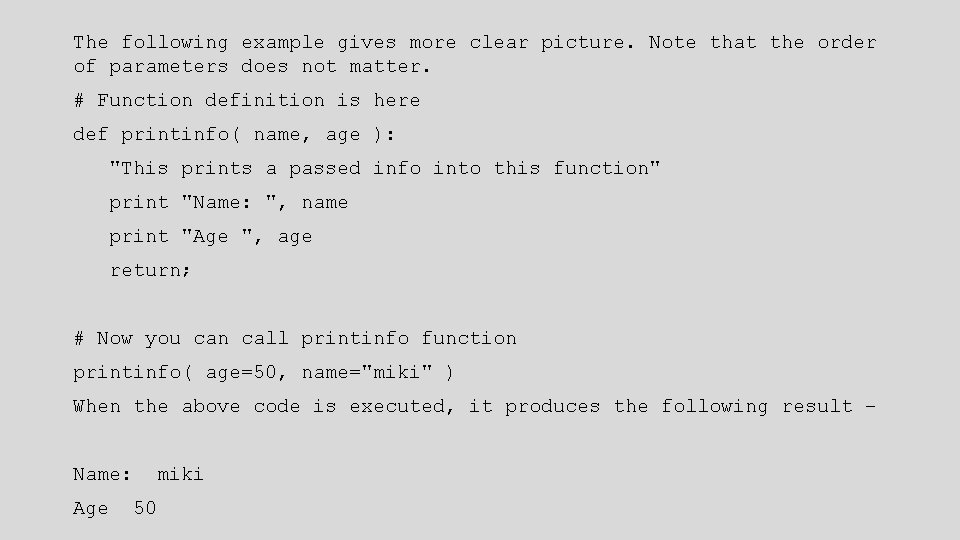
The following example gives more clear picture. Note that the order of parameters does not matter. # Function definition is here def printinfo( name, age ): "This prints a passed info into this function" print "Name: ", name print "Age ", age return; # Now you can call printinfo function printinfo( age=50, name="miki" ) When the above code is executed, it produces the following result − Name: miki Age 50
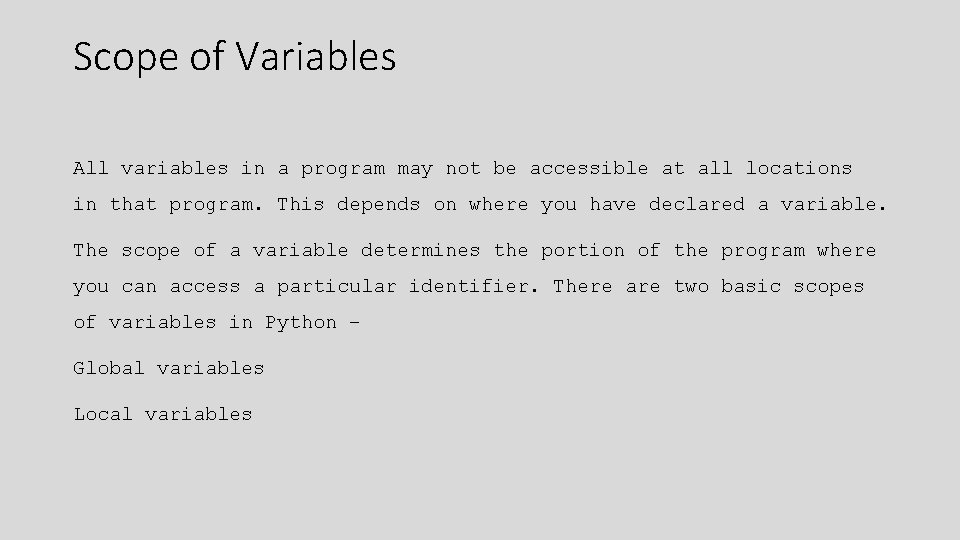
Scope of Variables All variables in a program may not be accessible at all locations in that program. This depends on where you have declared a variable. The scope of a variable determines the portion of the program where you can access a particular identifier. There are two basic scopes of variables in Python − Global variables Local variables
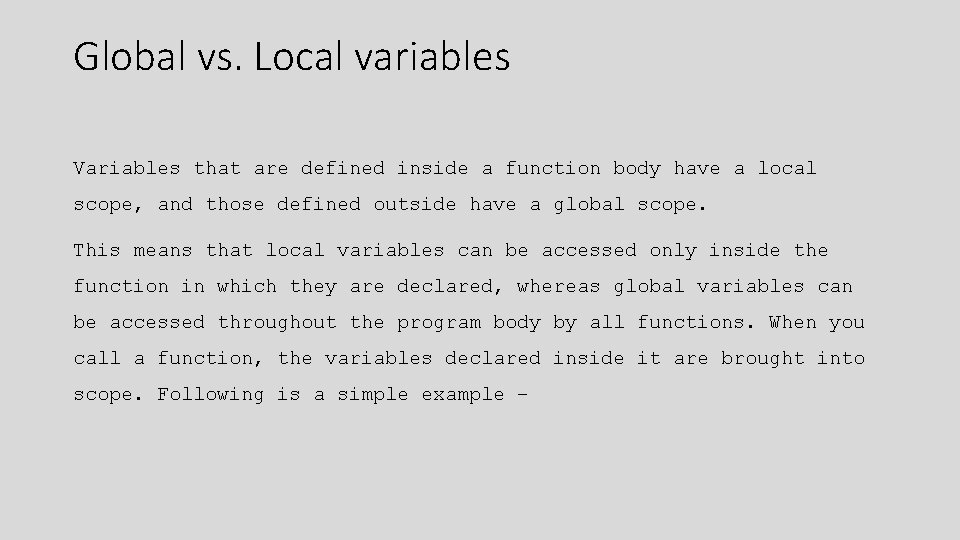
Global vs. Local variables Variables that are defined inside a function body have a local scope, and those defined outside have a global scope. This means that local variables can be accessed only inside the function in which they are declared, whereas global variables can be accessed throughout the program body by all functions. When you call a function, the variables declared inside it are brought into scope. Following is a simple example −
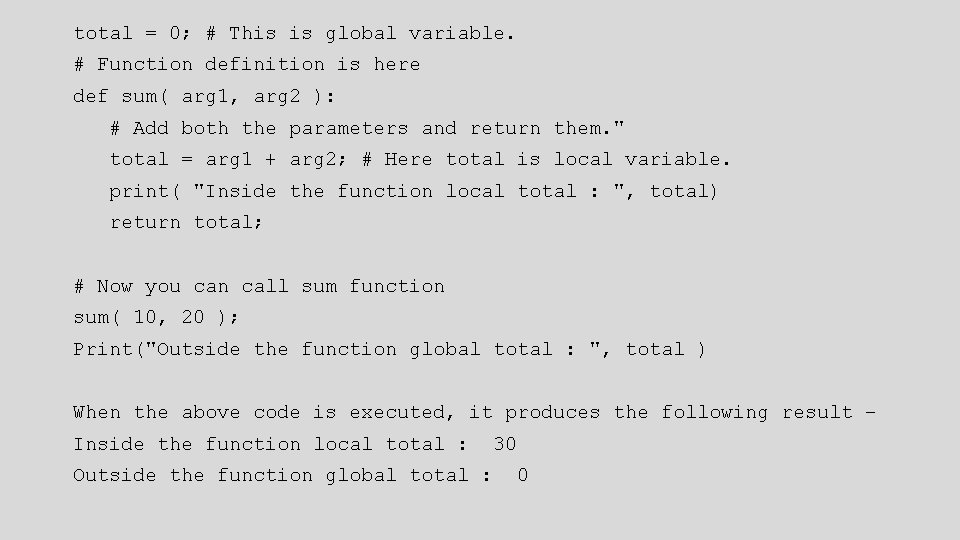
total = 0; # This is global variable. # Function definition is here def sum( arg 1, arg 2 ): # Add both the parameters and return them. " total = arg 1 + arg 2; # Here total is local variable. print( "Inside the function local total : ", total) return total; # Now you can call sum function sum( 10, 20 ); Print("Outside the function global total : ", total ) When the above code is executed, it produces the following result − Inside the function local total : 30 Outside the function global total : 0
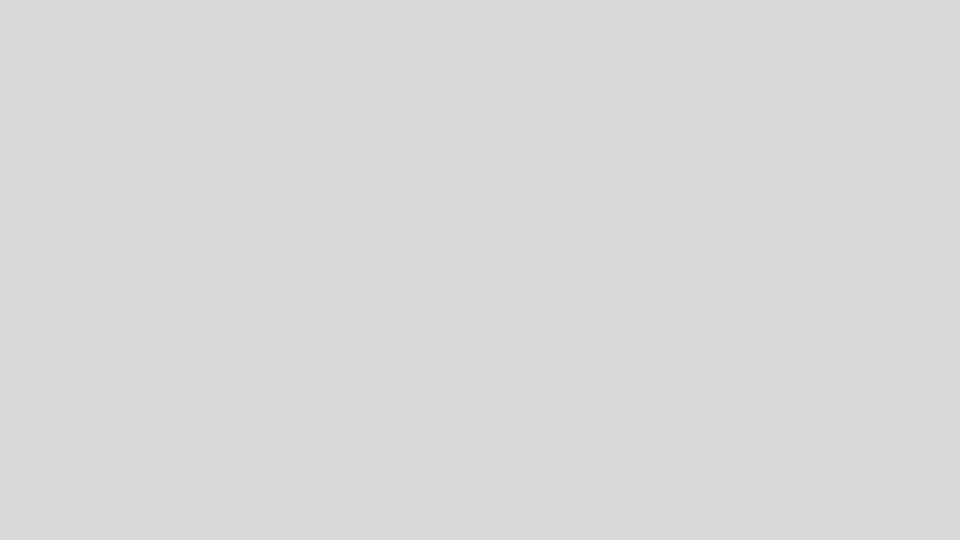
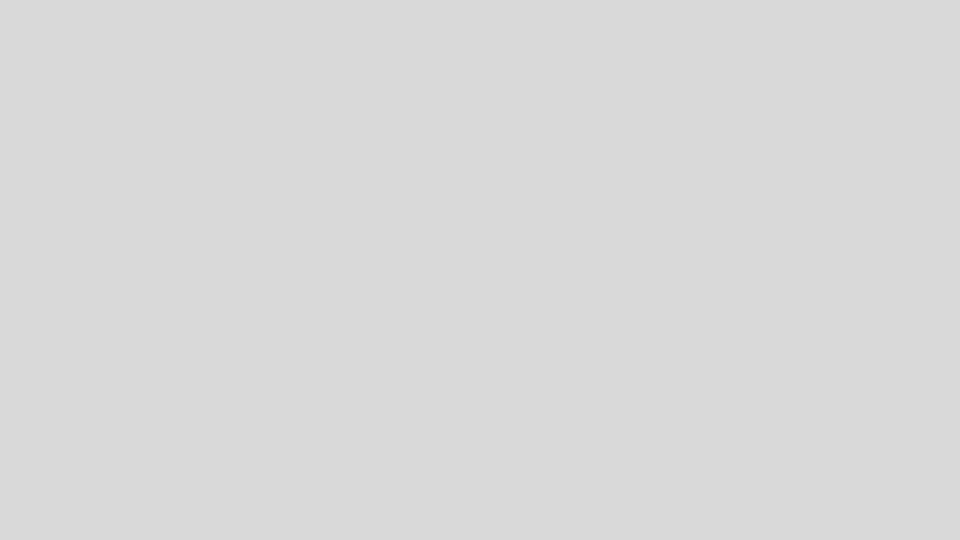