6 7 The return Statement The return statement
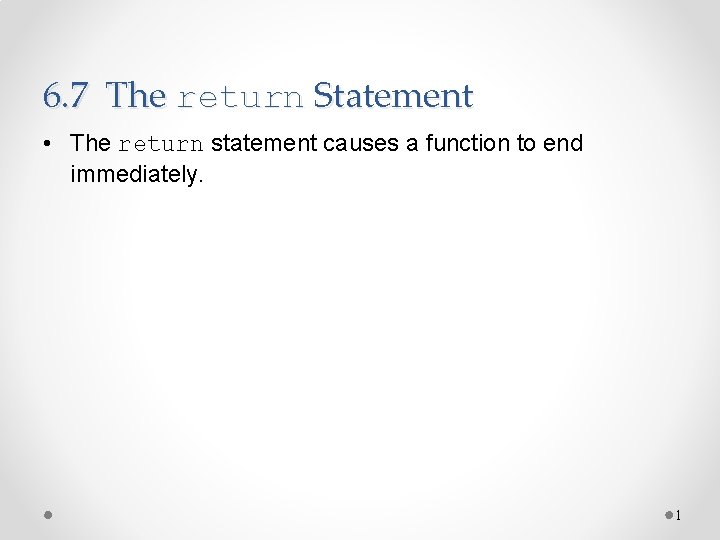
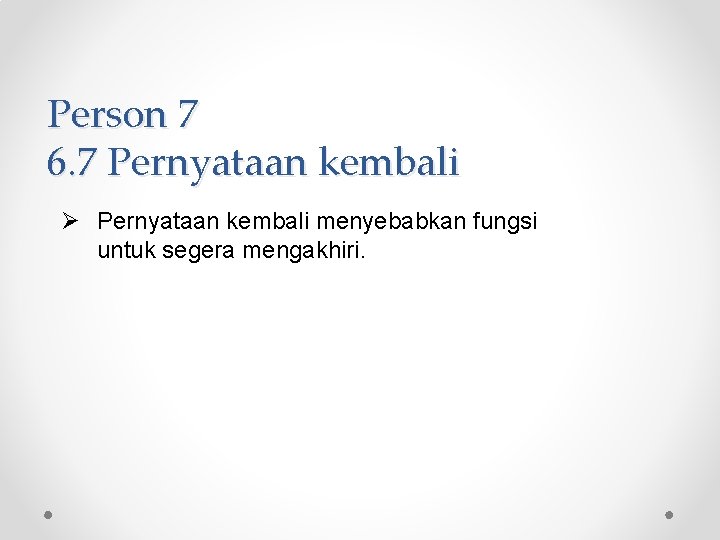
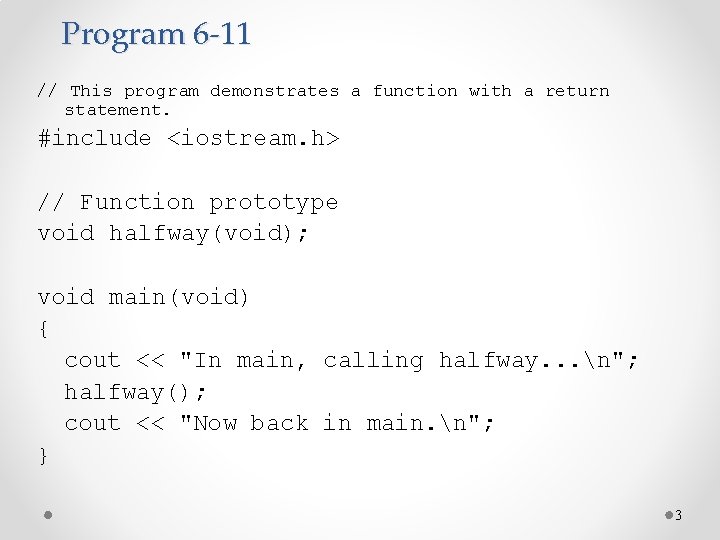
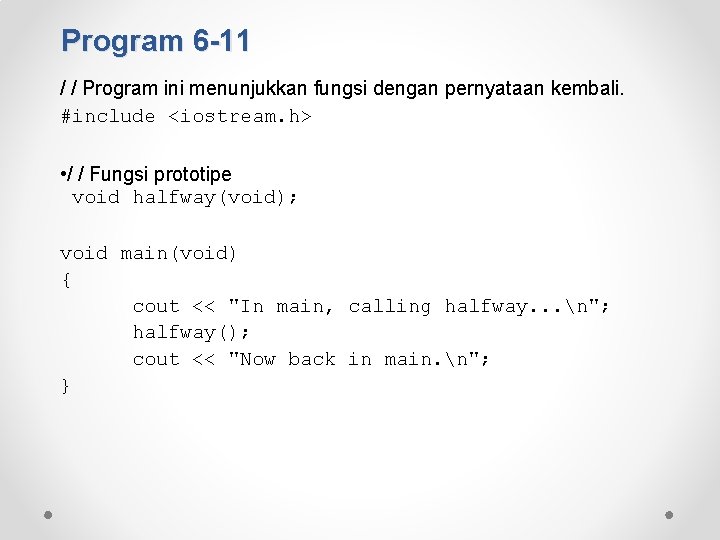
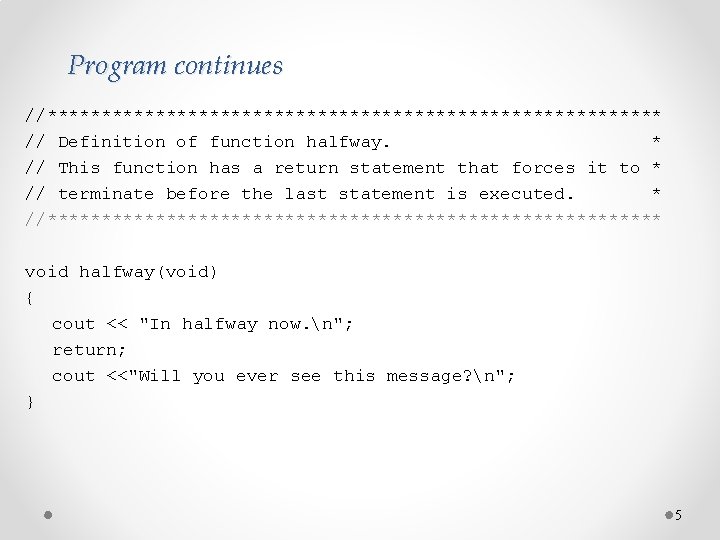
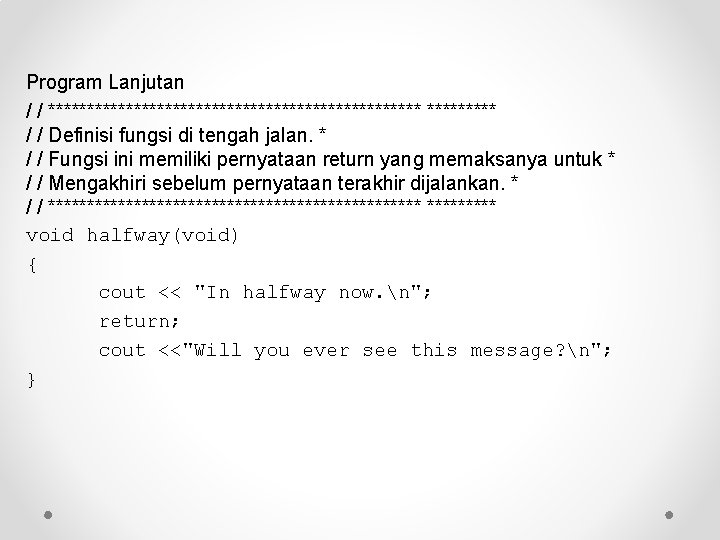
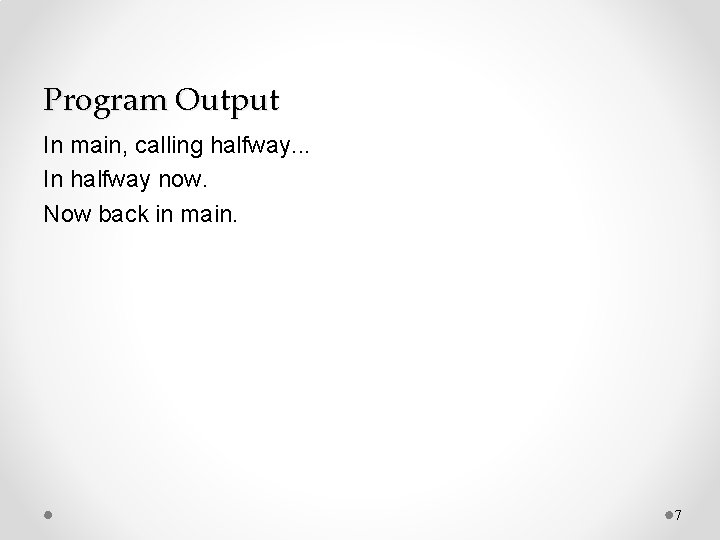
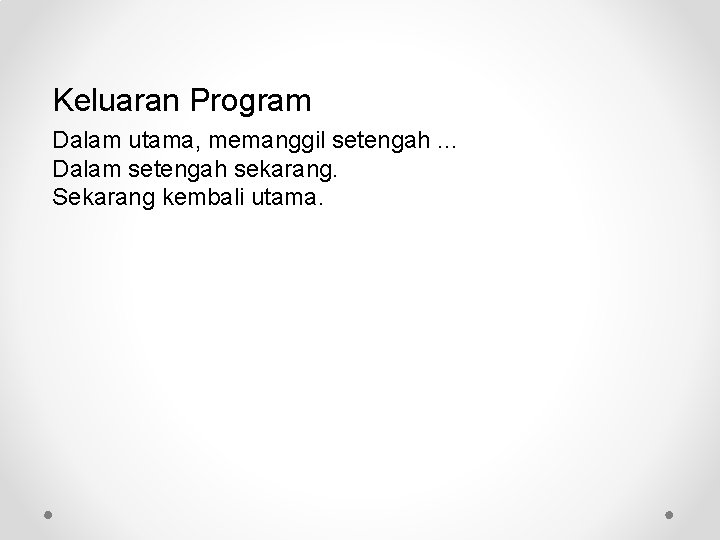
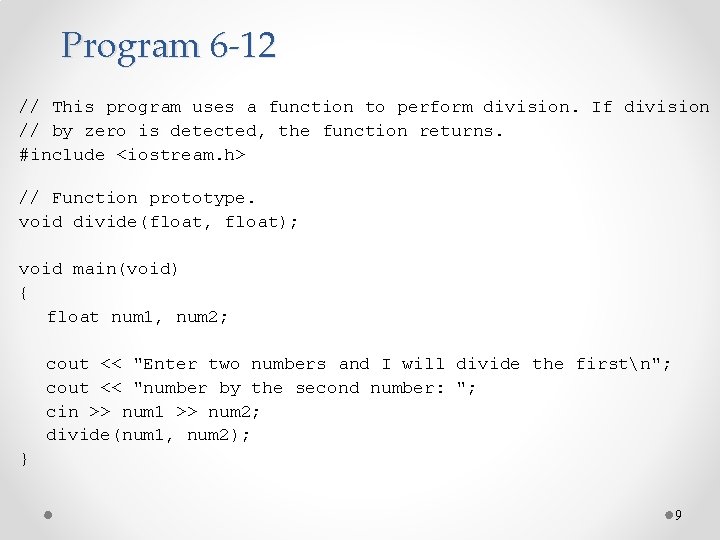
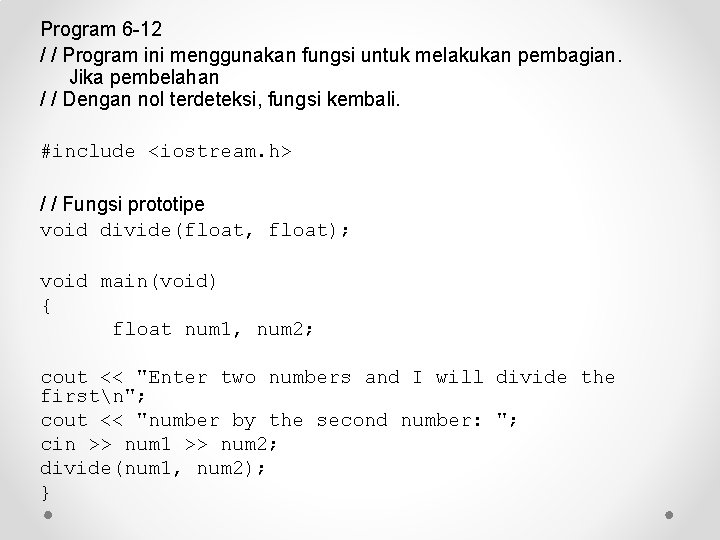
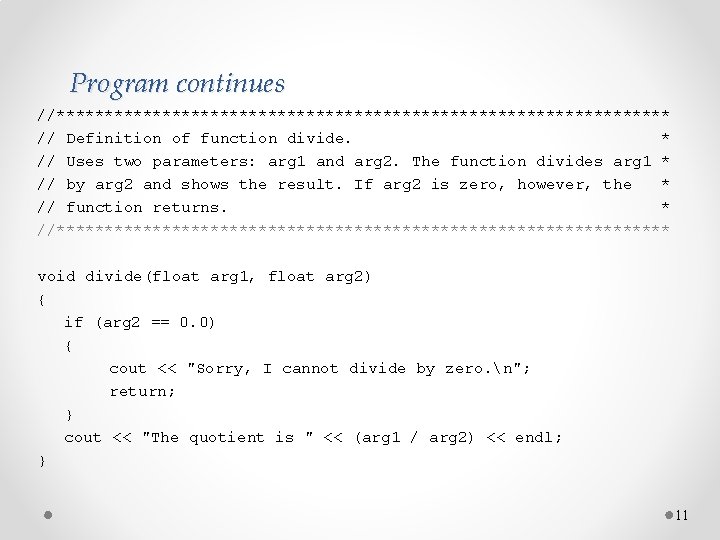
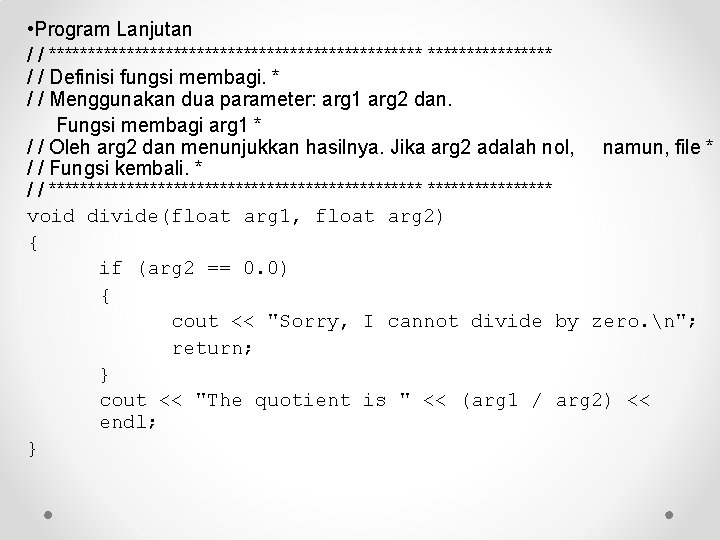
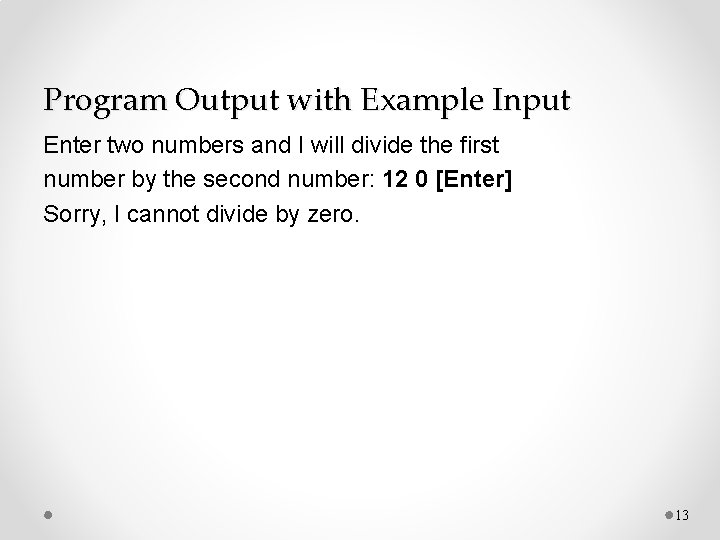
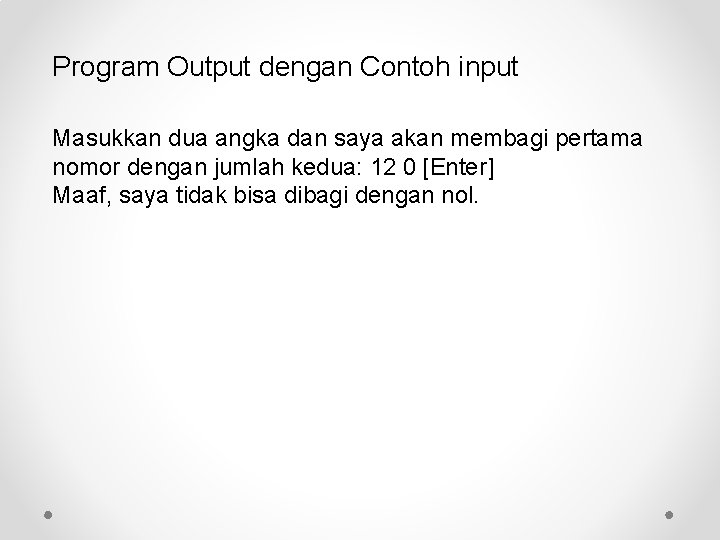
- Slides: 14
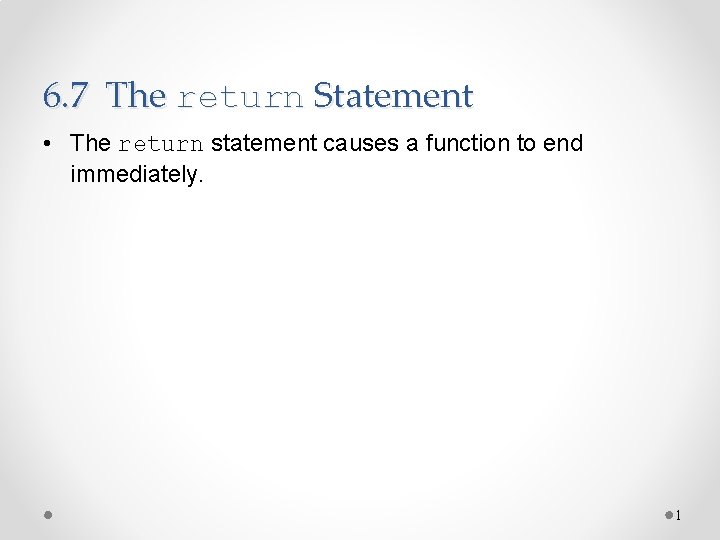
6. 7 The return Statement • The return statement causes a function to end immediately. 1
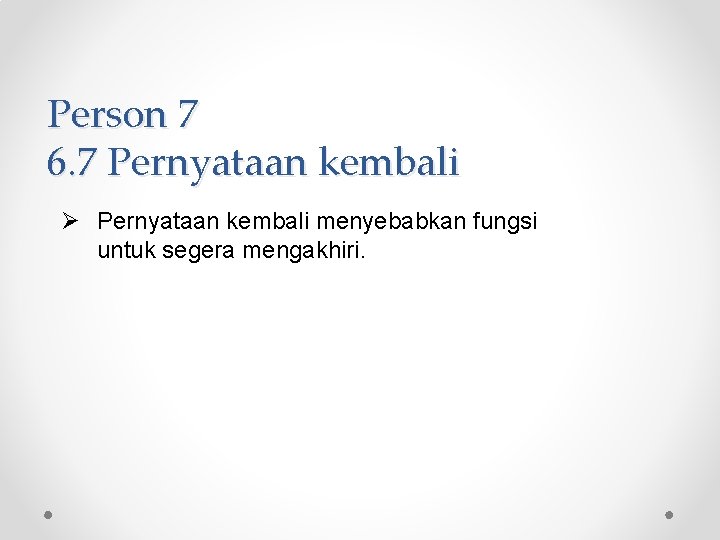
Person 7 6. 7 Pernyataan kembali Ø Pernyataan kembali menyebabkan fungsi untuk segera mengakhiri.
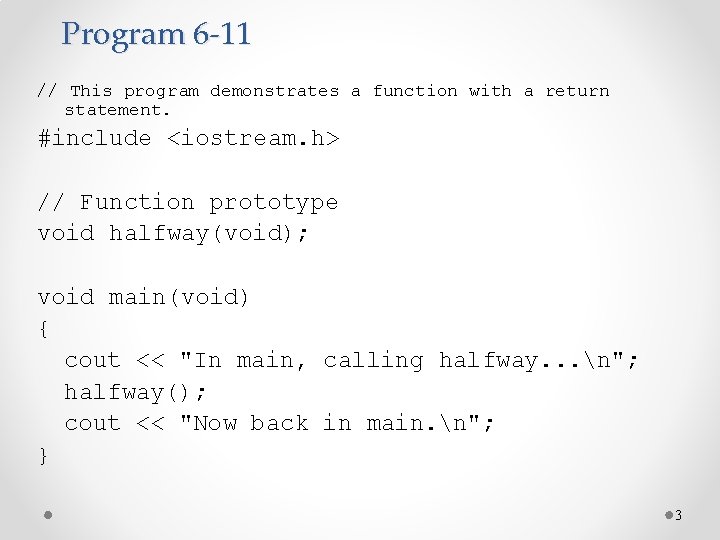
Program 6 -11 // This program demonstrates a function with a return statement. #include <iostream. h> // Function prototype void halfway(void); void main(void) { cout << "In main, calling halfway. . . n"; halfway(); cout << "Now back in main. n"; } 3
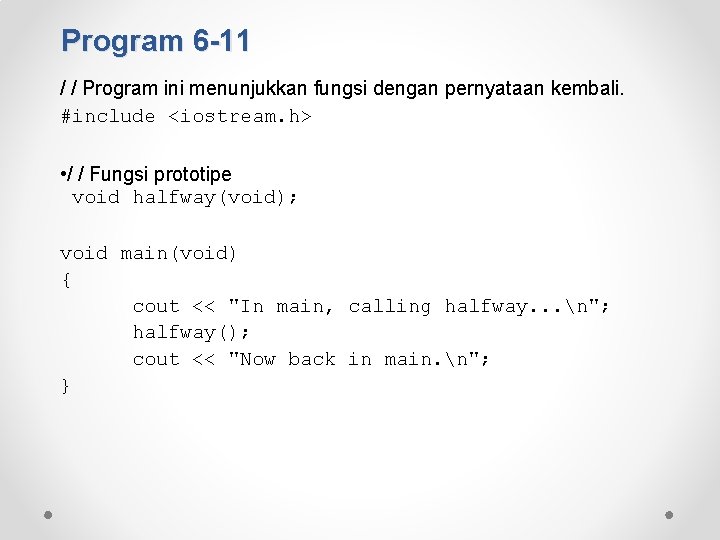
Program 6 -11 / / Program ini menunjukkan fungsi dengan pernyataan kembali. #include <iostream. h> • / / Fungsi prototipe void halfway(void); void main(void) { cout << "In main, calling halfway. . . n"; halfway(); cout << "Now back in main. n"; }
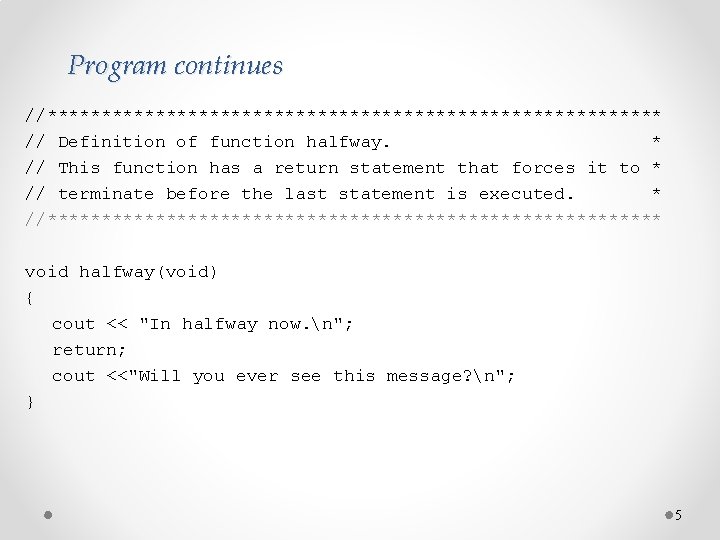
Program continues //***************************** // Definition of function halfway. * // This function has a return statement that forces it to * // terminate before the last statement is executed. * //***************************** void halfway(void) { cout << "In halfway now. n"; return; cout <<"Will you ever see this message? n"; } 5
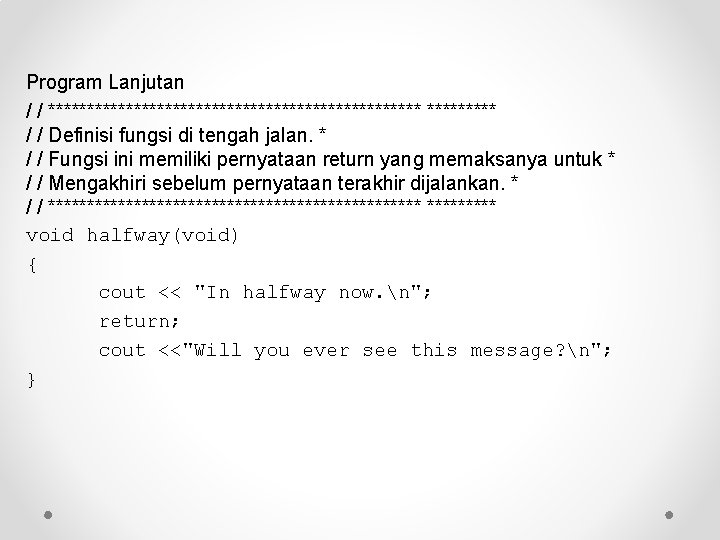
Program Lanjutan / / ************************ / / Definisi fungsi di tengah jalan. * / / Fungsi ini memiliki pernyataan return yang memaksanya untuk * / / Mengakhiri sebelum pernyataan terakhir dijalankan. * / / ************************ void halfway(void) { cout << "In halfway now. n"; return; cout <<"Will you ever see this message? n"; }
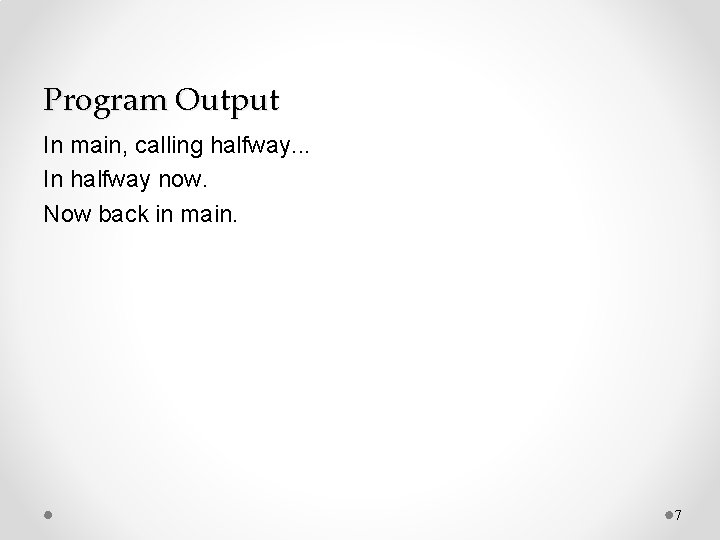
Program Output In main, calling halfway. . . In halfway now. Now back in main. 7
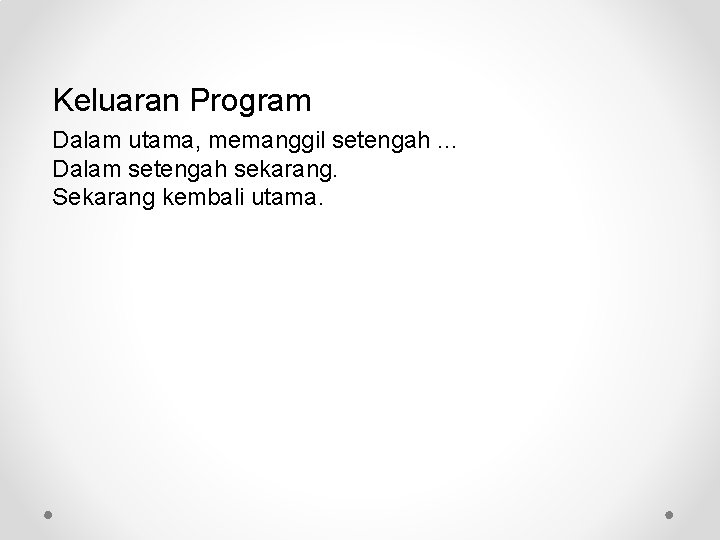
Keluaran Program Dalam utama, memanggil setengah. . . Dalam setengah sekarang. Sekarang kembali utama.
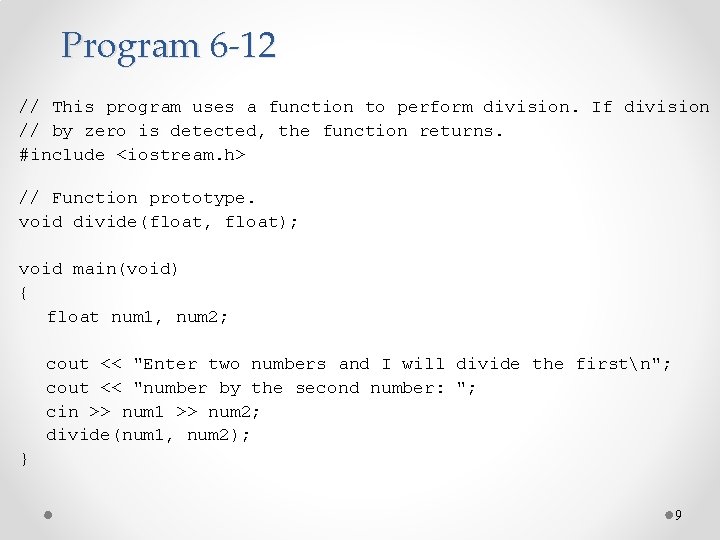
Program 6 -12 // This program uses a function to perform division. If division // by zero is detected, the function returns. #include <iostream. h> // Function prototype. void divide(float, float); void main(void) { float num 1, num 2; cout << "Enter two numbers and I will divide the firstn"; cout << "number by the second number: "; cin >> num 1 >> num 2; divide(num 1, num 2); } 9
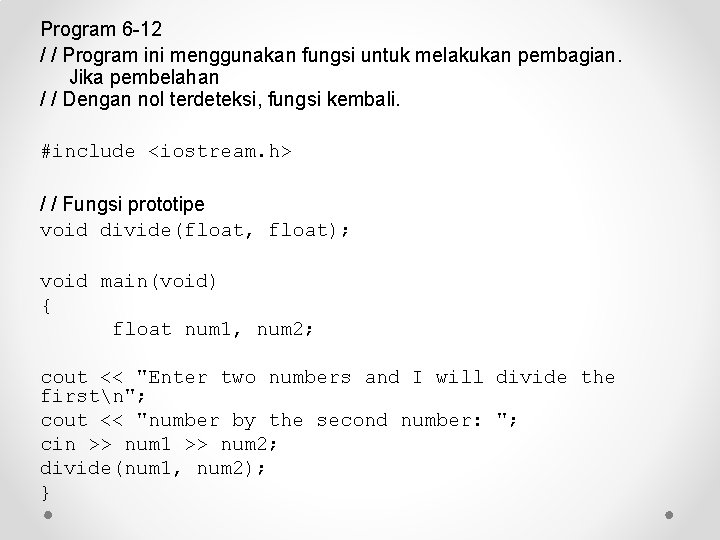
Program 6 -12 / / Program ini menggunakan fungsi untuk melakukan pembagian. Jika pembelahan / / Dengan nol terdeteksi, fungsi kembali. #include <iostream. h> / / Fungsi prototipe void divide(float, float); void main(void) { float num 1, num 2; cout << "Enter two numbers and I will divide the firstn"; cout << "number by the second number: "; cin >> num 1 >> num 2; divide(num 1, num 2); }
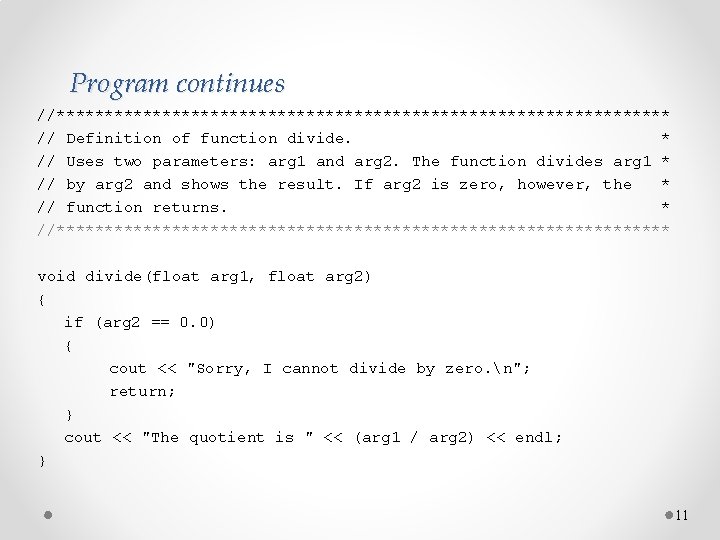
Program continues //******************************** // Definition of function divide. * // Uses two parameters: arg 1 and arg 2. The function divides arg 1 * // by arg 2 and shows the result. If arg 2 is zero, however, the * // function returns. * //******************************** void divide(float arg 1, float arg 2) { if (arg 2 == 0. 0) { cout << "Sorry, I cannot divide by zero. n"; return; } cout << "The quotient is " << (arg 1 / arg 2) << endl; } 11
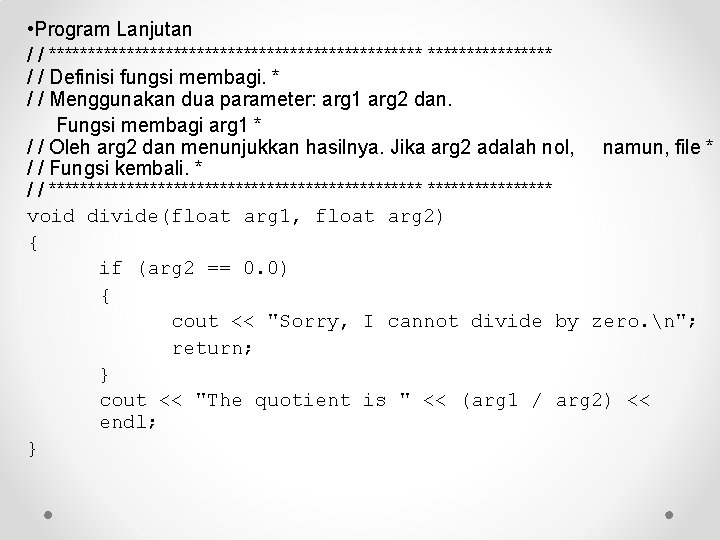
• Program Lanjutan / / ************************ / / Definisi fungsi membagi. * / / Menggunakan dua parameter: arg 1 arg 2 dan. Fungsi membagi arg 1 * / / Oleh arg 2 dan menunjukkan hasilnya. Jika arg 2 adalah nol, namun, file * / / Fungsi kembali. * / / ************************ void divide(float arg 1, float arg 2) { if (arg 2 == 0. 0) { cout << "Sorry, I cannot divide by zero. n"; return; } cout << "The quotient is " << (arg 1 / arg 2) << endl; }
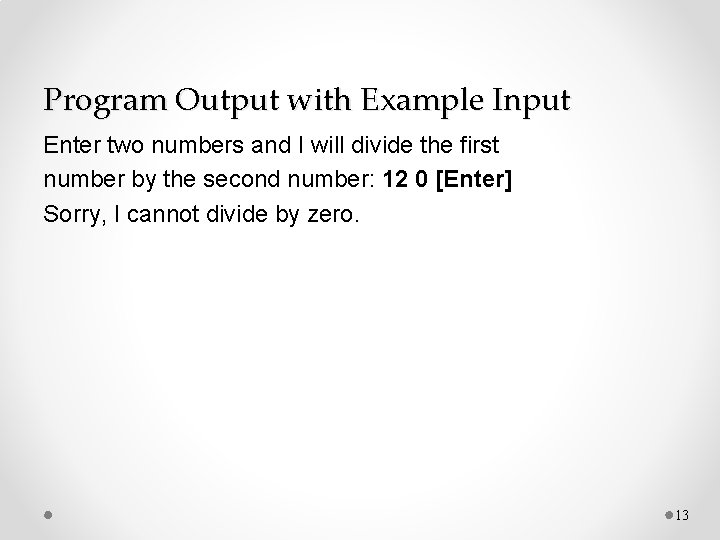
Program Output with Example Input Enter two numbers and I will divide the first number by the second number: 12 0 [Enter] Sorry, I cannot divide by zero. 13
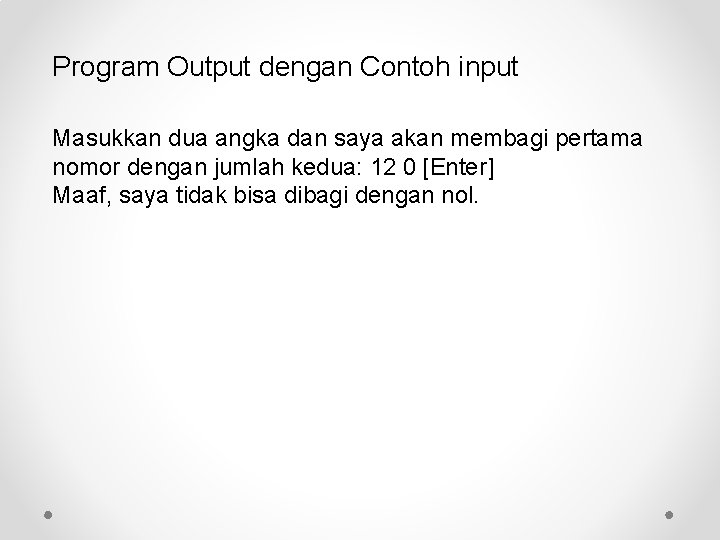
Program Output dengan Contoh input Masukkan dua angka dan saya akan membagi pertama nomor dengan jumlah kedua: 12 0 [Enter] Maaf, saya tidak bisa dibagi dengan nol.
Principle of statement-departure-return
Return outwards in income statement
Return statement in c
Python function without return statement
C++
Môn thể thao bắt đầu bằng từ đua
Tư thế ngồi viết
Bàn tay mà dây bẩn
Hát kết hợp bộ gõ cơ thể
Cách giải mật thư tọa độ
Tư thế ngồi viết
Chó sói
Thẻ vin
Ví dụ giọng cùng tên
Thơ thất ngôn tứ tuyệt đường luật