Function Function Declaration Function Definition Calling a Function
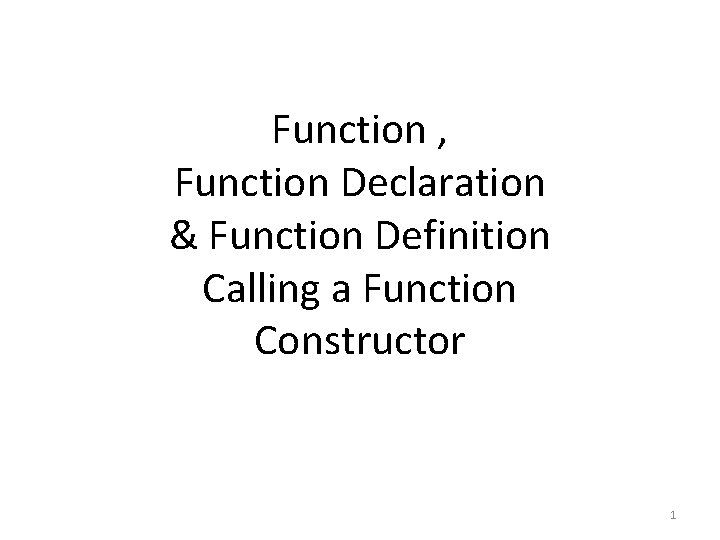
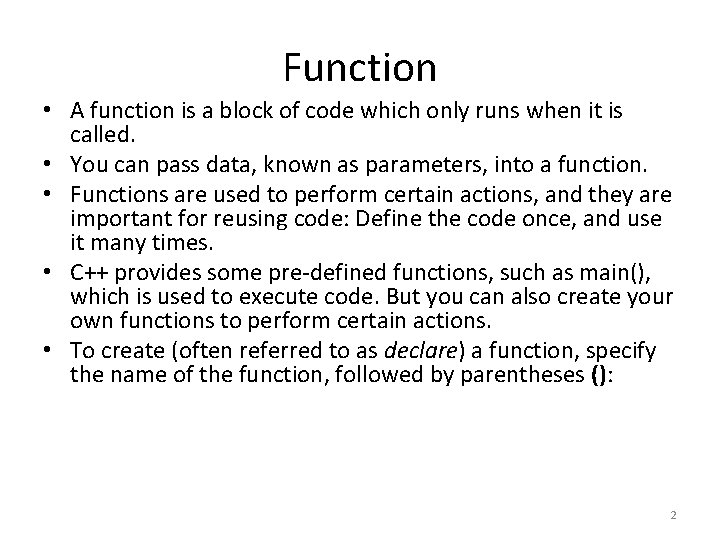
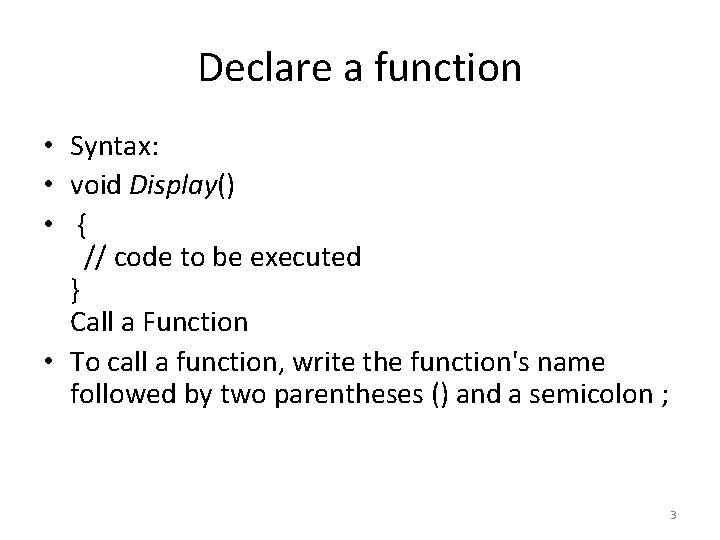
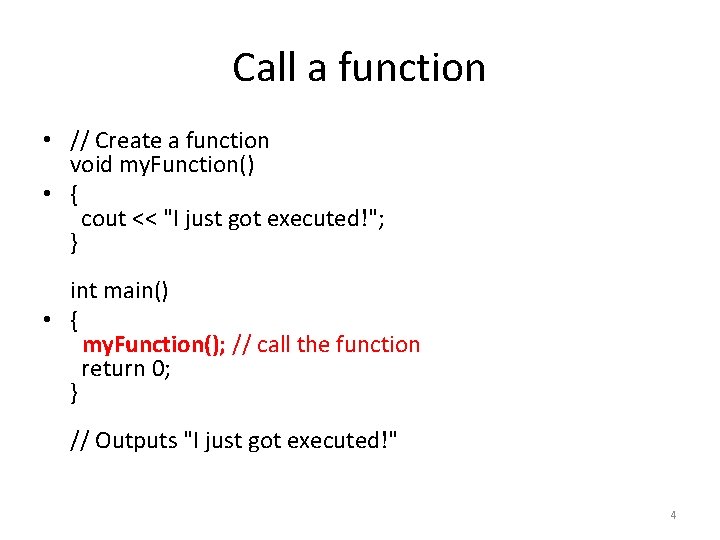
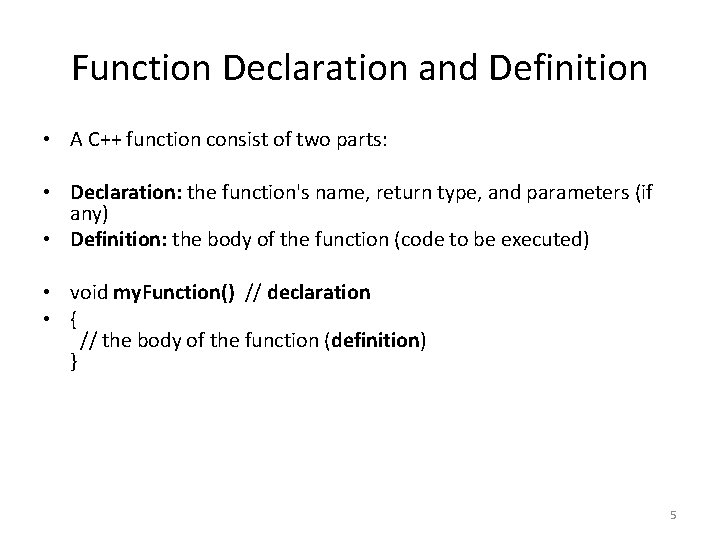
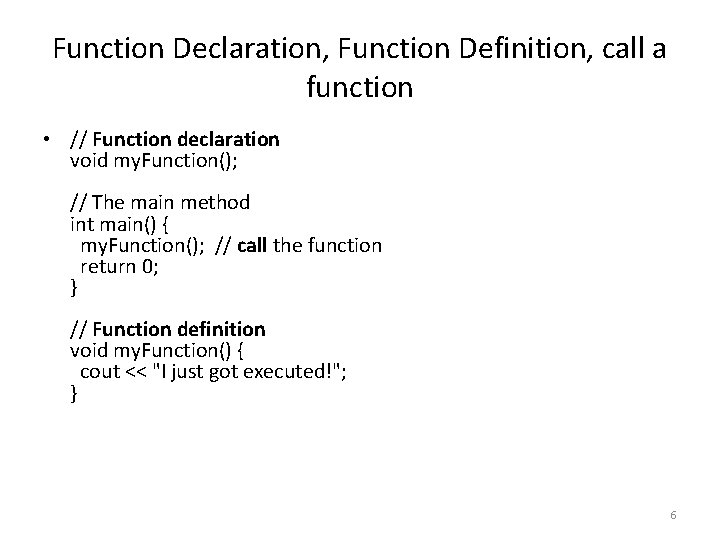
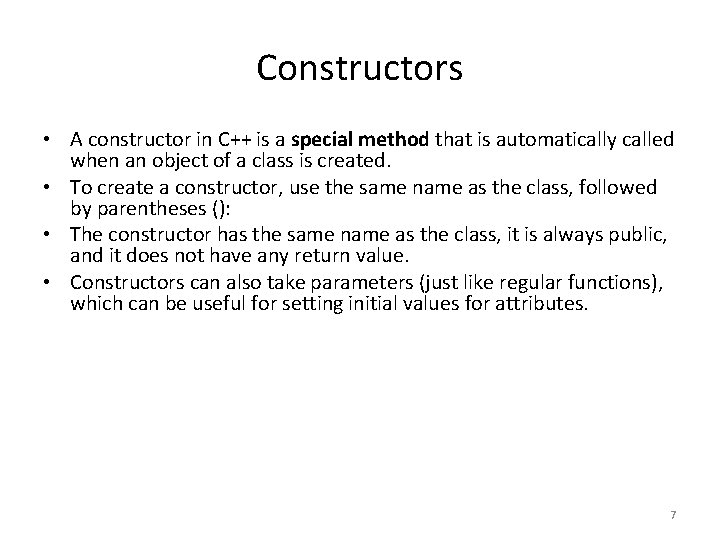
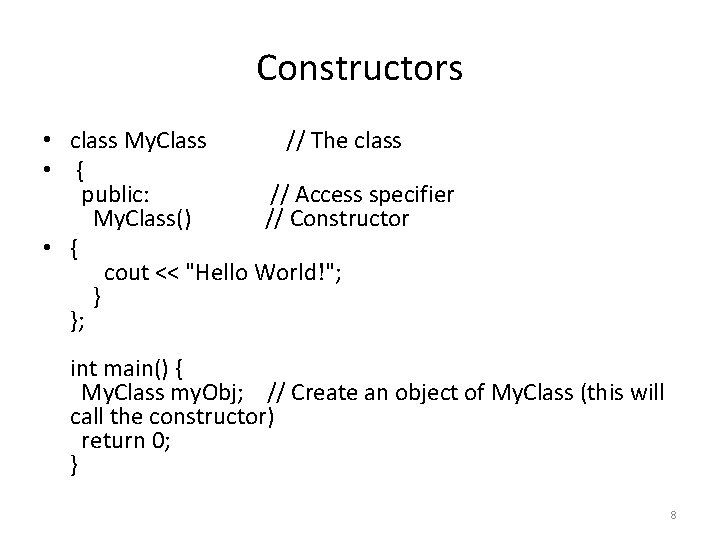
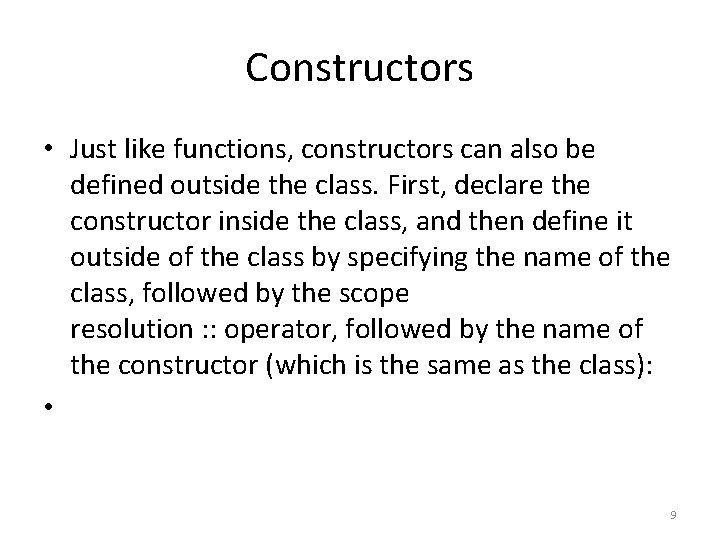
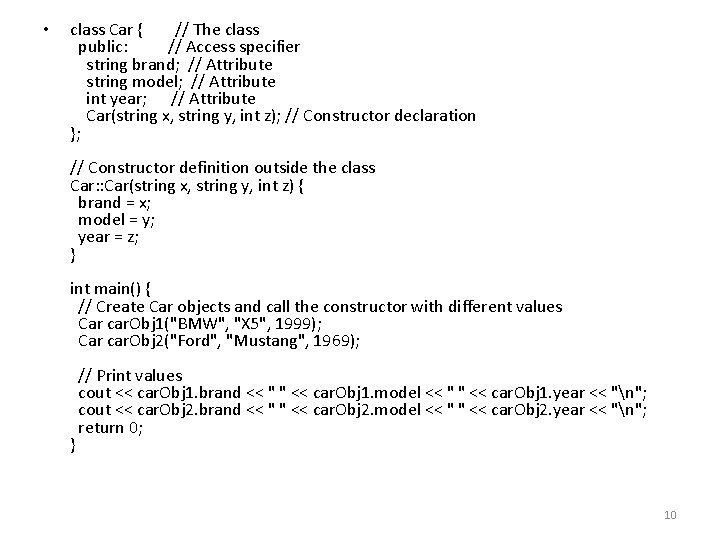
- Slides: 10
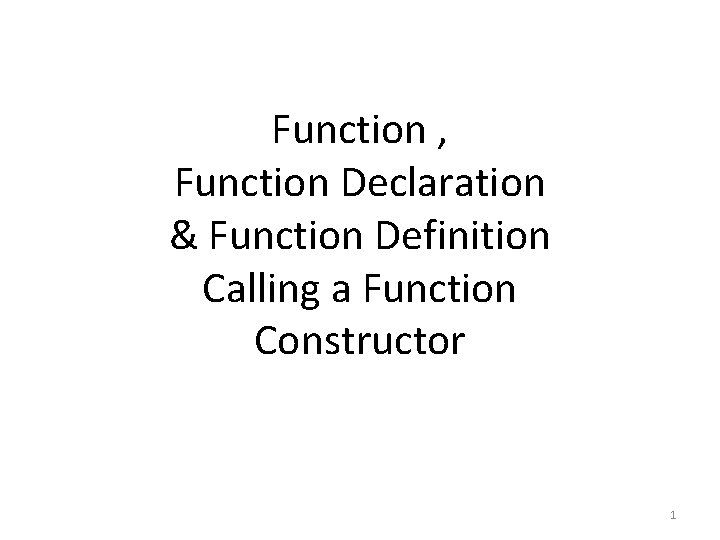
Function , Function Declaration & Function Definition Calling a Function Constructor 1
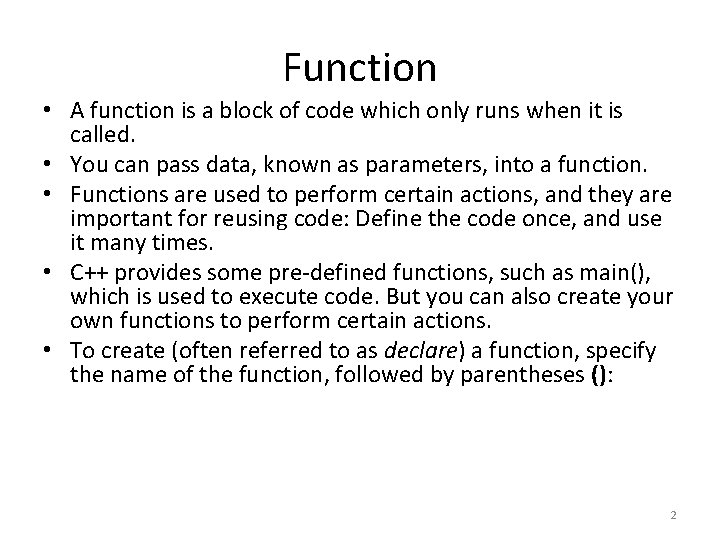
Function • A function is a block of code which only runs when it is called. • You can pass data, known as parameters, into a function. • Functions are used to perform certain actions, and they are important for reusing code: Define the code once, and use it many times. • C++ provides some pre-defined functions, such as main(), which is used to execute code. But you can also create your own functions to perform certain actions. • To create (often referred to as declare) a function, specify the name of the function, followed by parentheses (): 2
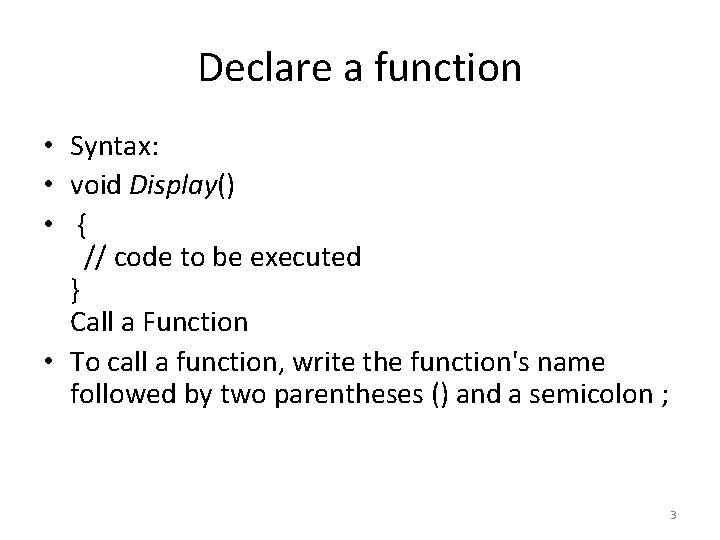
Declare a function • Syntax: • void Display() • { // code to be executed } Call a Function • To call a function, write the function's name followed by two parentheses () and a semicolon ; 3
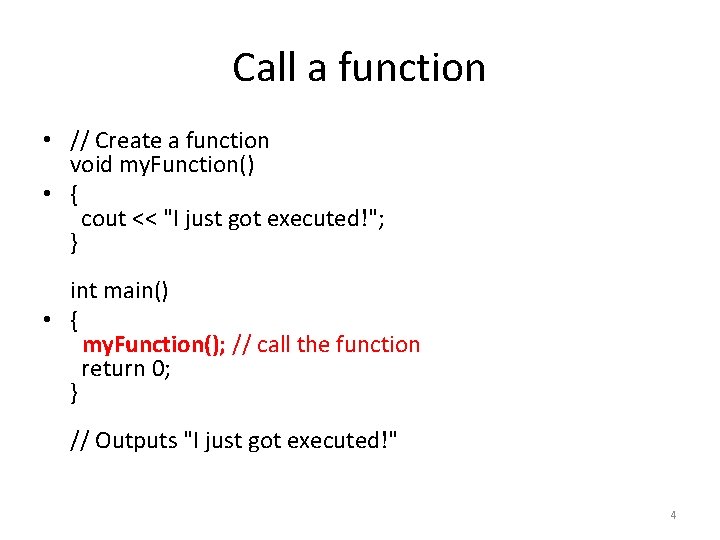
Call a function • // Create a function void my. Function() • { cout << "I just got executed!"; } int main() • { my. Function(); // call the function return 0; } // Outputs "I just got executed!" 4
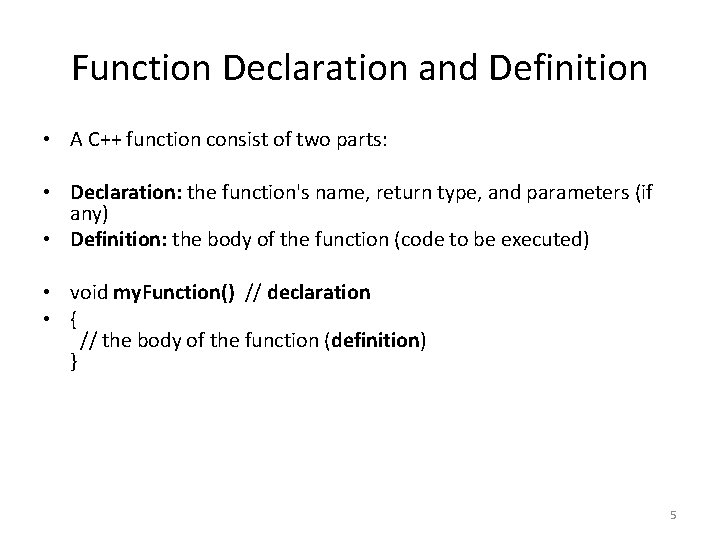
Function Declaration and Definition • A C++ function consist of two parts: • Declaration: the function's name, return type, and parameters (if any) • Definition: the body of the function (code to be executed) • void my. Function() // declaration • { // the body of the function (definition) } 5
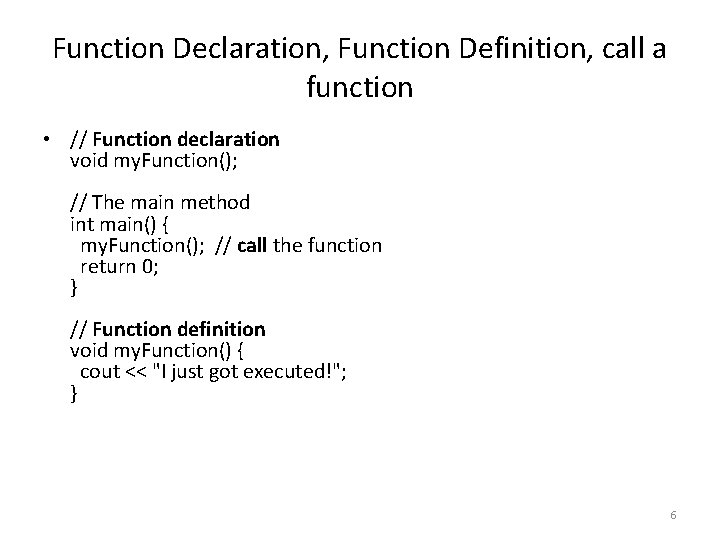
Function Declaration, Function Definition, call a function • // Function declaration void my. Function(); // The main method int main() { my. Function(); // call the function return 0; } // Function definition void my. Function() { cout << "I just got executed!"; } 6
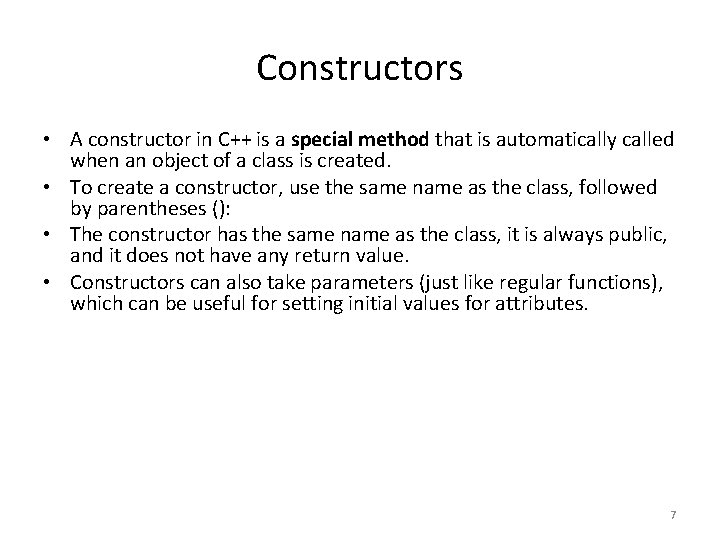
Constructors • A constructor in C++ is a special method that is automatically called when an object of a class is created. • To create a constructor, use the same name as the class, followed by parentheses (): • The constructor has the same name as the class, it is always public, and it does not have any return value. • Constructors can also take parameters (just like regular functions), which can be useful for setting initial values for attributes. 7
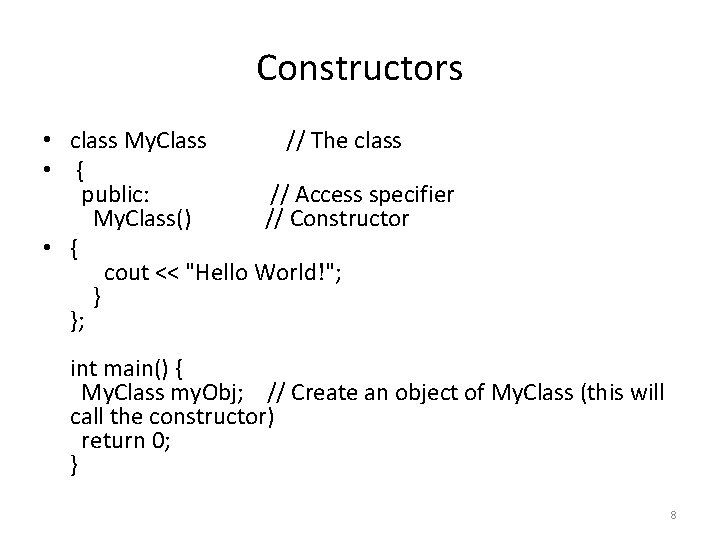
Constructors • class My. Class // The class • { public: // Access specifier My. Class() // Constructor • { cout << "Hello World!"; } }; int main() { My. Class my. Obj; // Create an object of My. Class (this will call the constructor) return 0; } 8
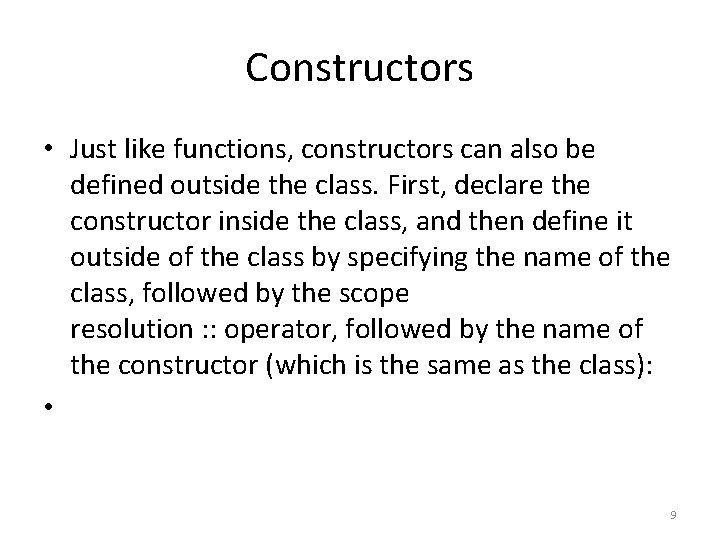
Constructors • Just like functions, constructors can also be defined outside the class. First, declare the constructor inside the class, and then define it outside of the class by specifying the name of the class, followed by the scope resolution : : operator, followed by the name of the constructor (which is the same as the class): • 9
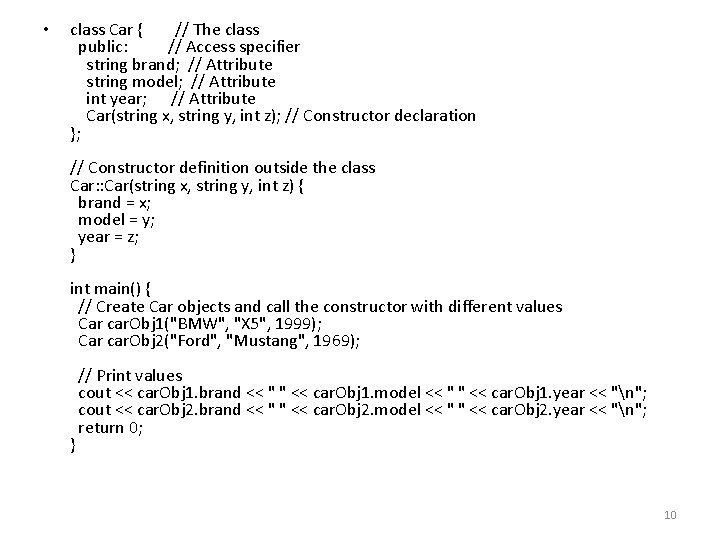
• class Car { // The class public: // Access specifier string brand; // Attribute string model; // Attribute int year; // Attribute Car(string x, string y, int z); // Constructor declaration }; // Constructor definition outside the class Car: : Car(string x, string y, int z) { brand = x; model = y; year = z; } int main() { // Create Car objects and call the constructor with different values Car car. Obj 1("BMW", "X 5", 1999); Car car. Obj 2("Ford", "Mustang", 1969); } // Print values cout << car. Obj 1. brand << " " << car. Obj 1. model << " " << car. Obj 1. year << "n"; cout << car. Obj 2. brand << " " << car. Obj 2. model << " " << car. Obj 2. year << "n"; return 0; 10