Data Types and Data Structures Data Types Keywords
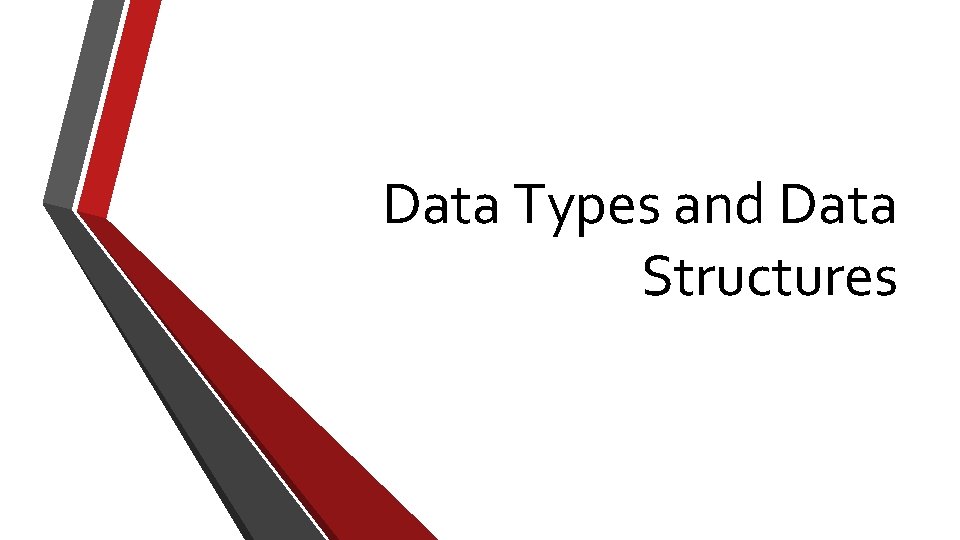
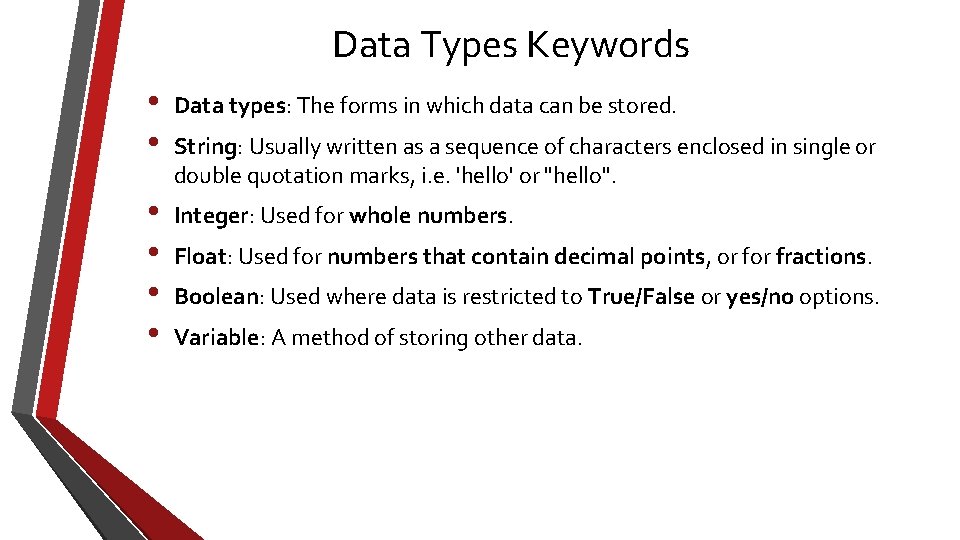
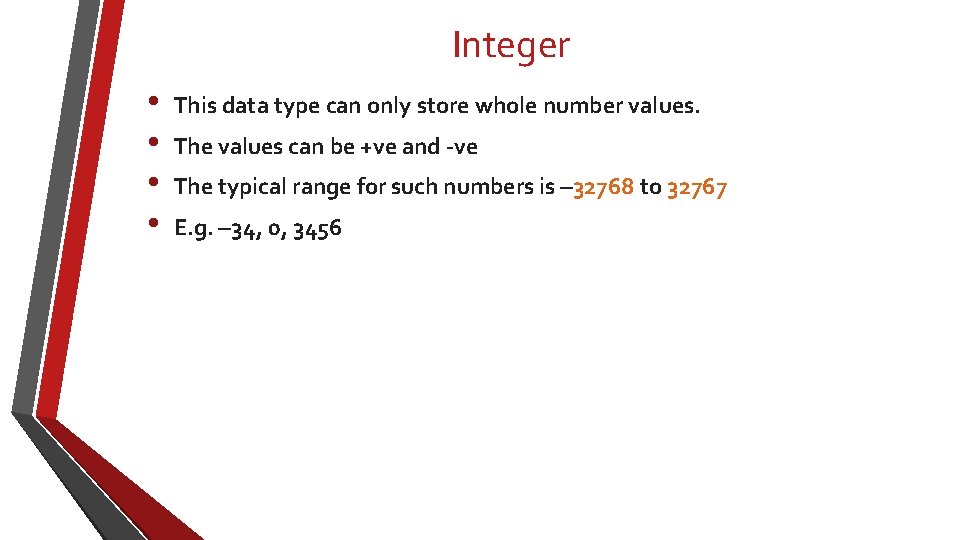
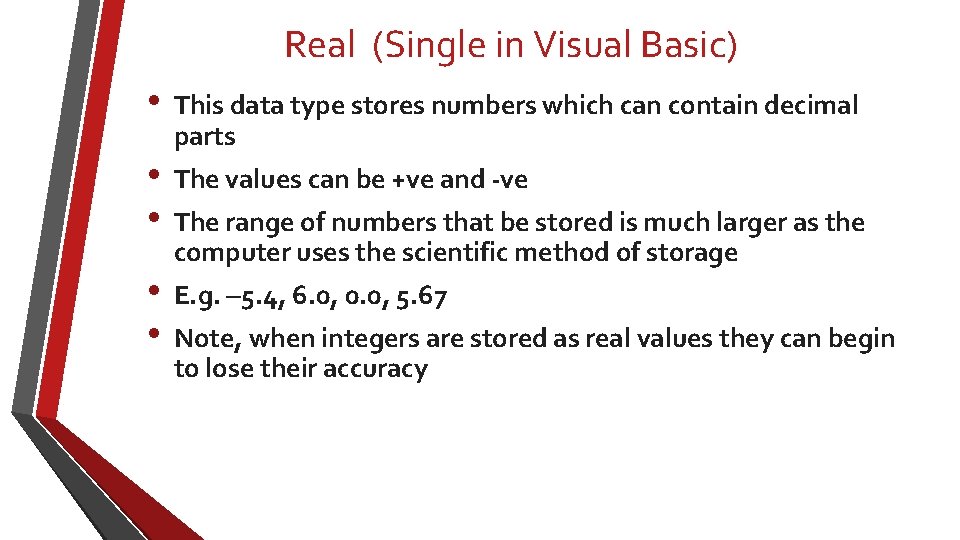
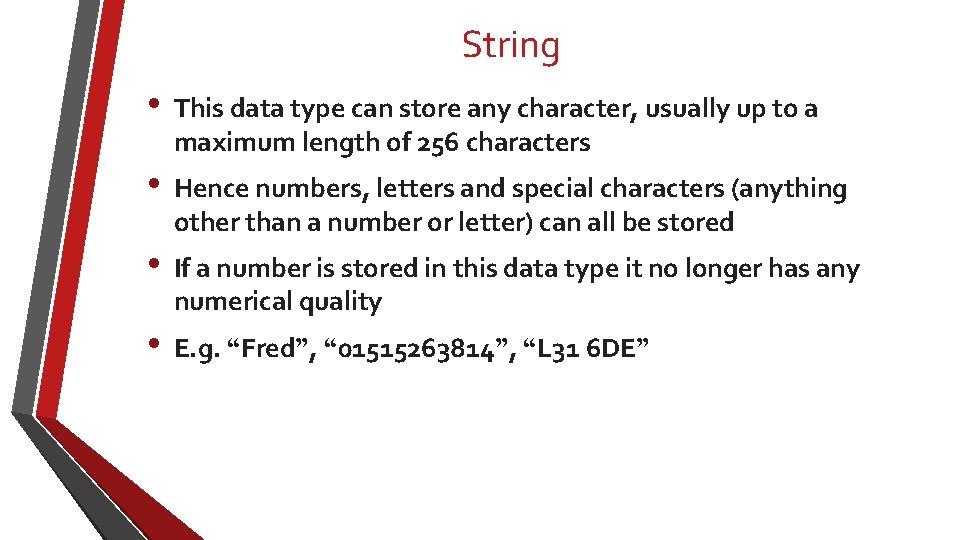
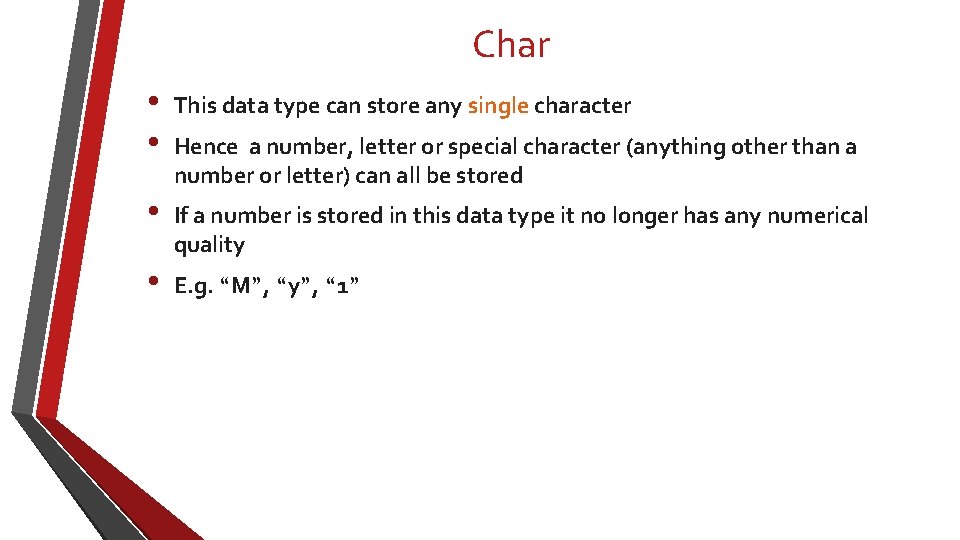
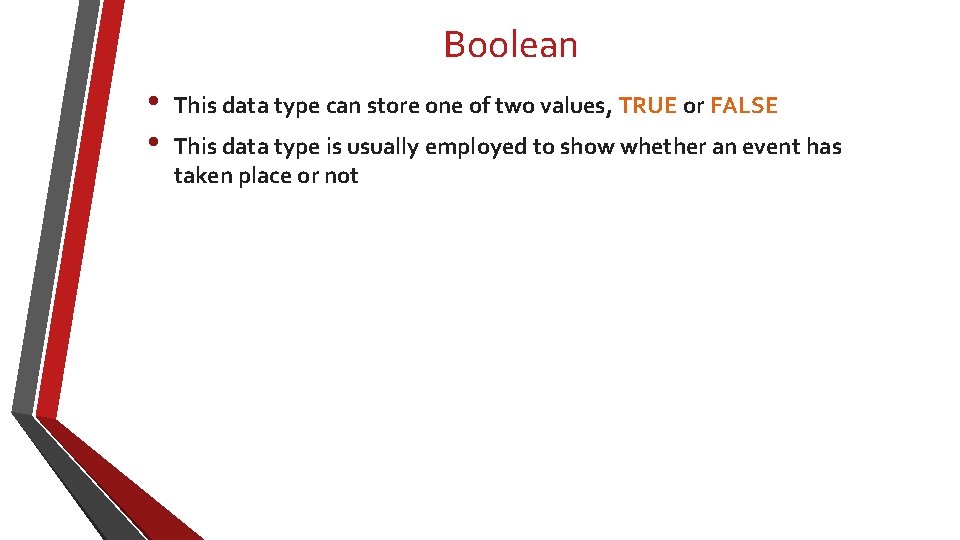
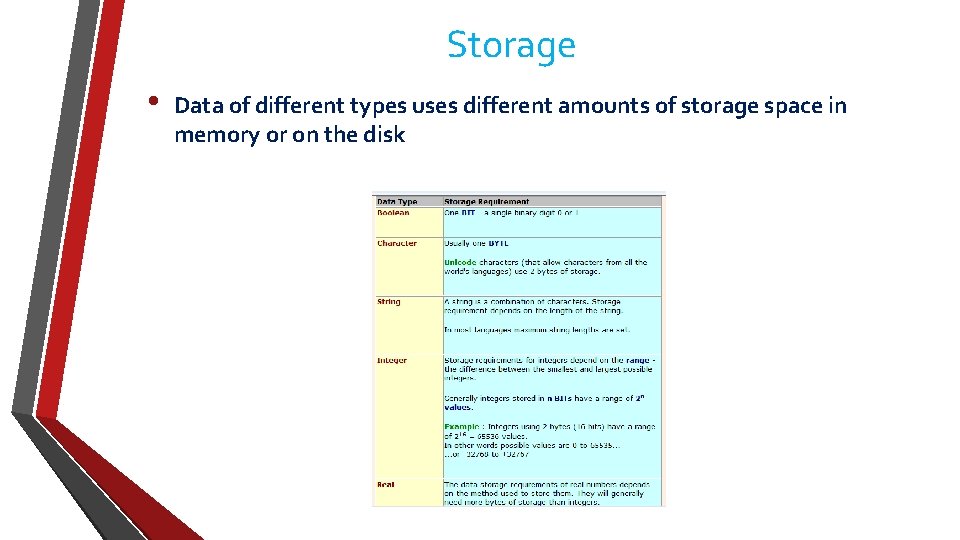
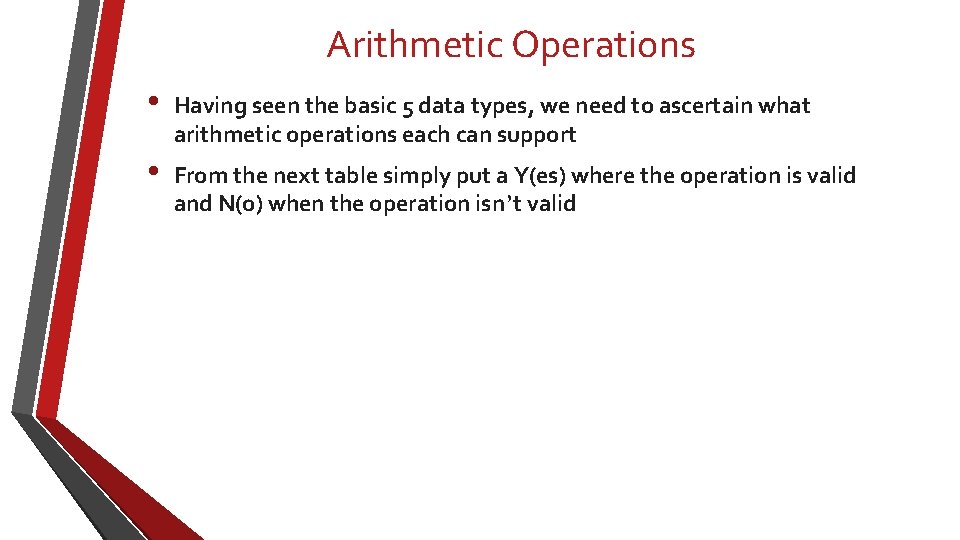
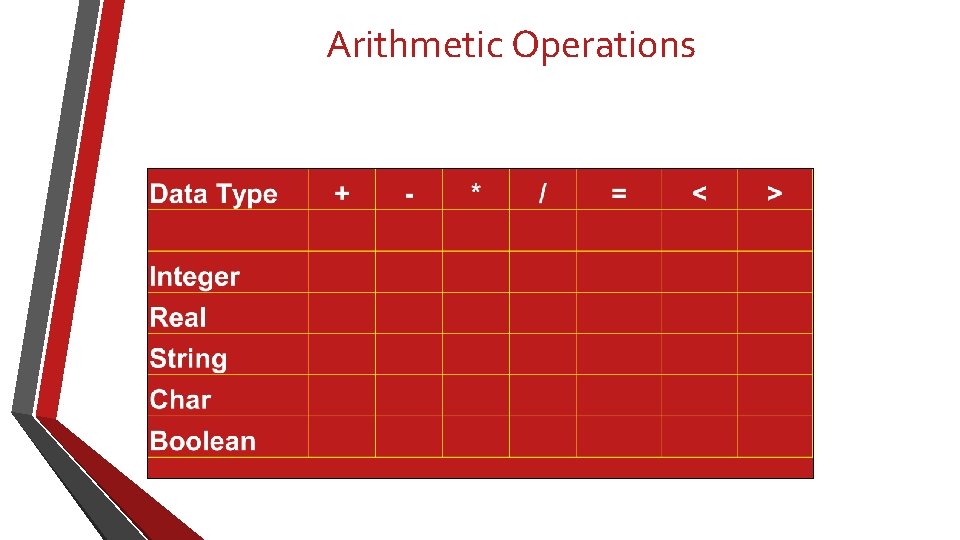
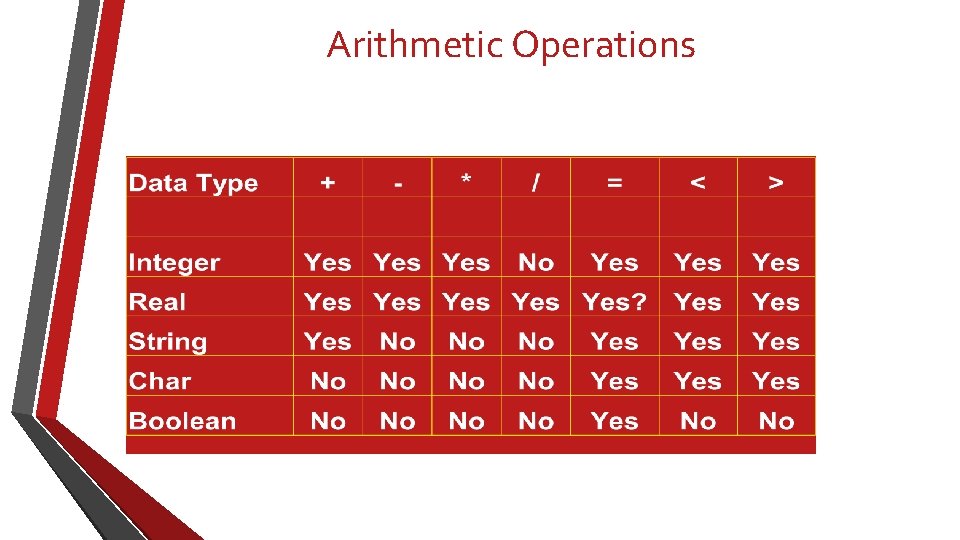
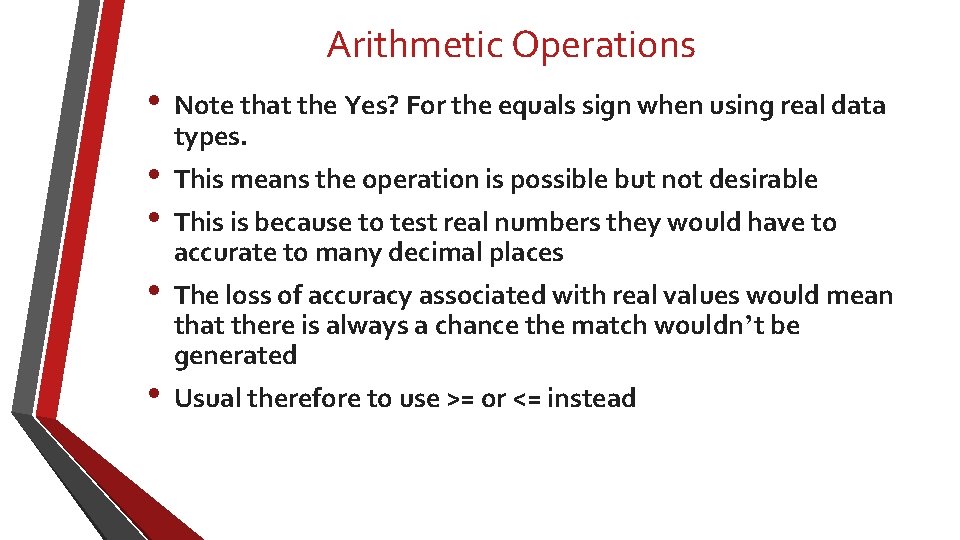
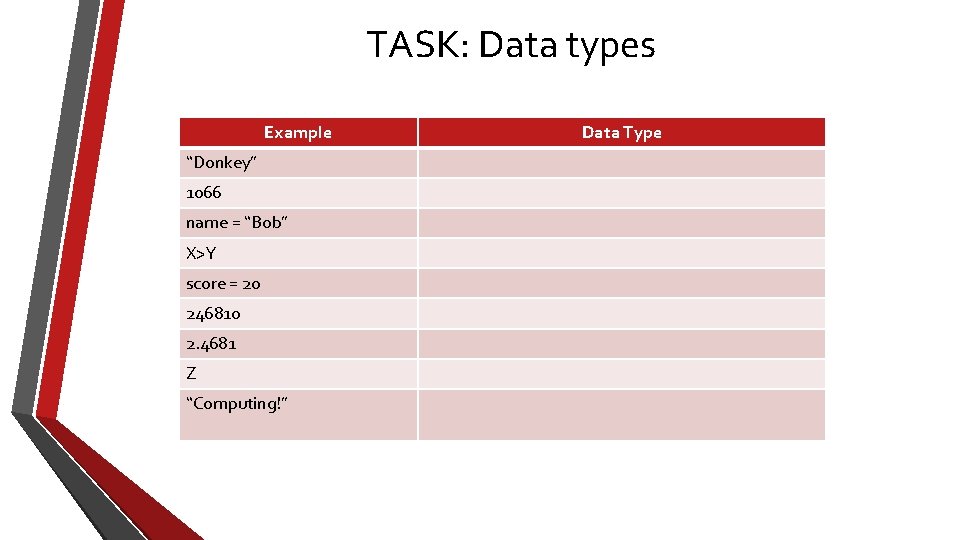
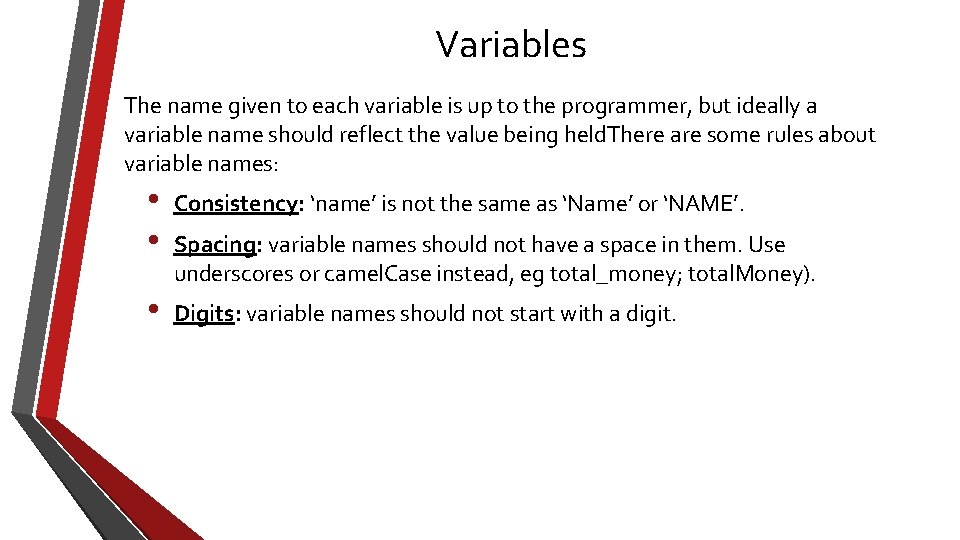
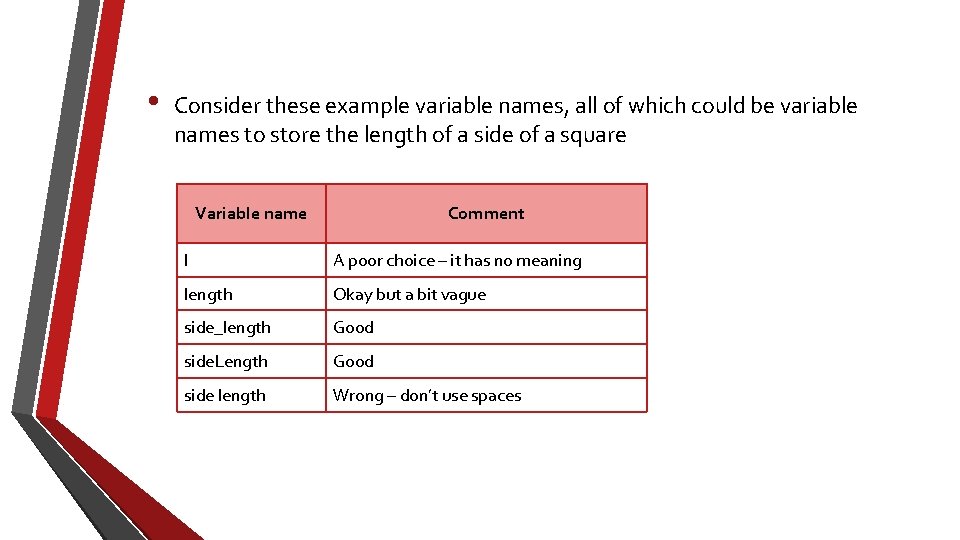
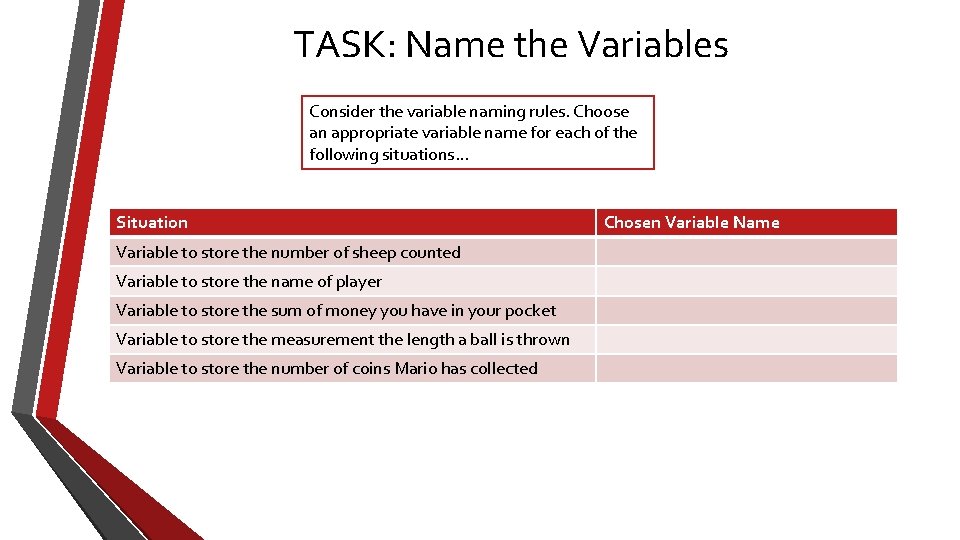
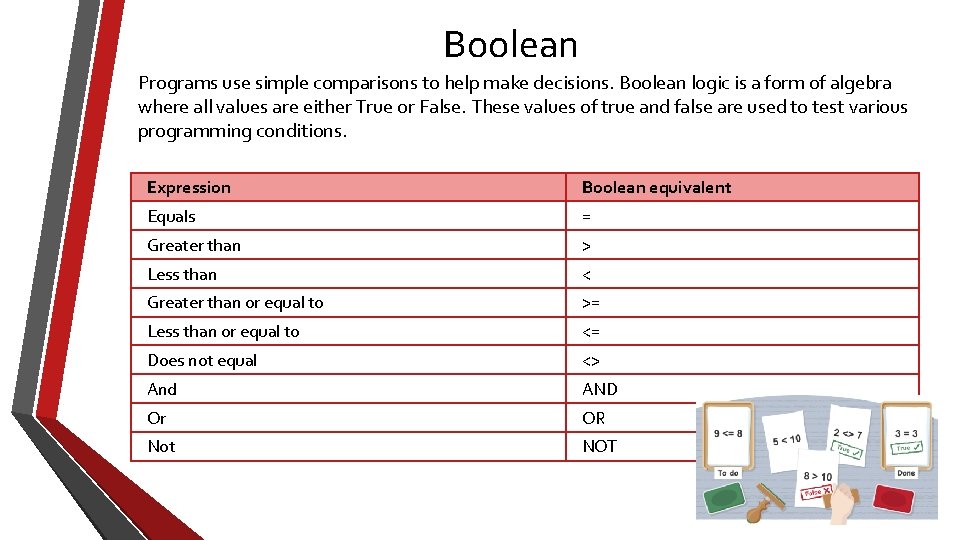
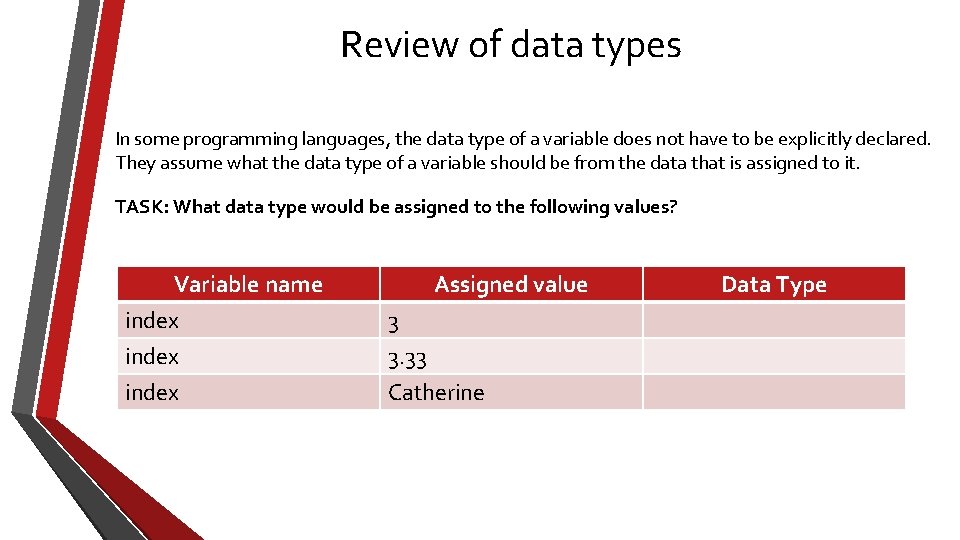
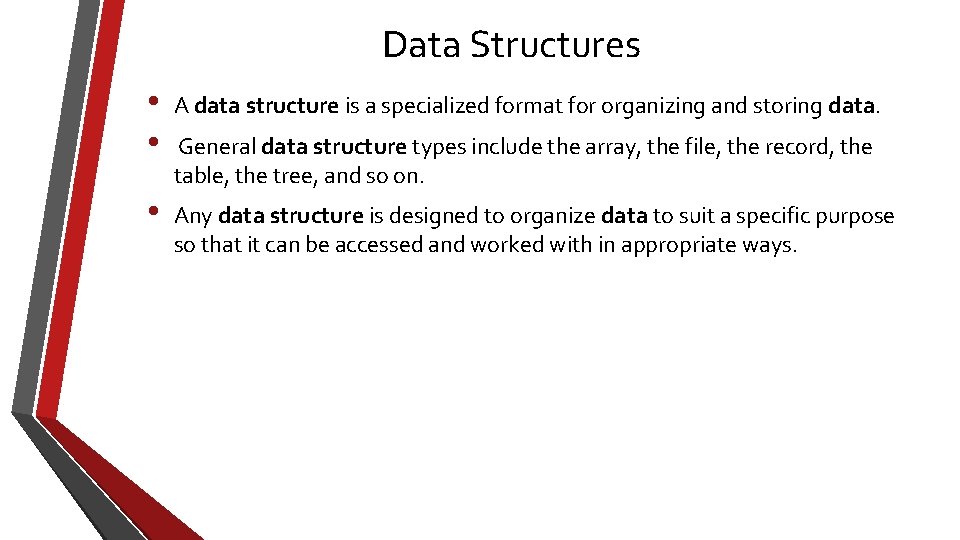
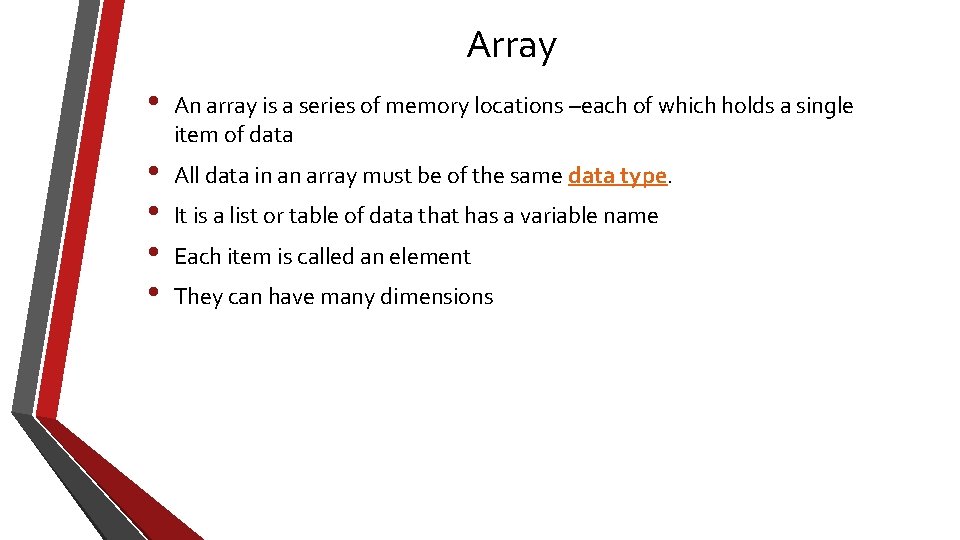
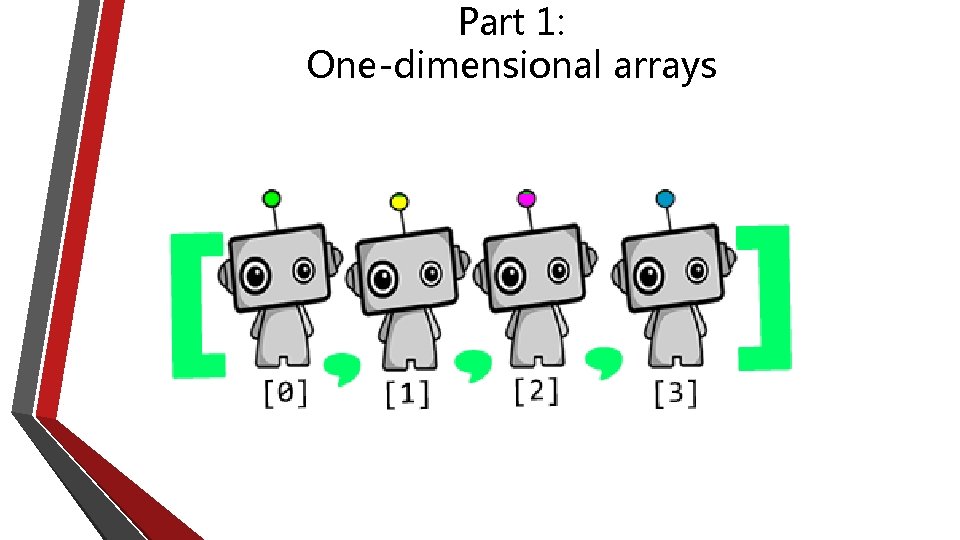
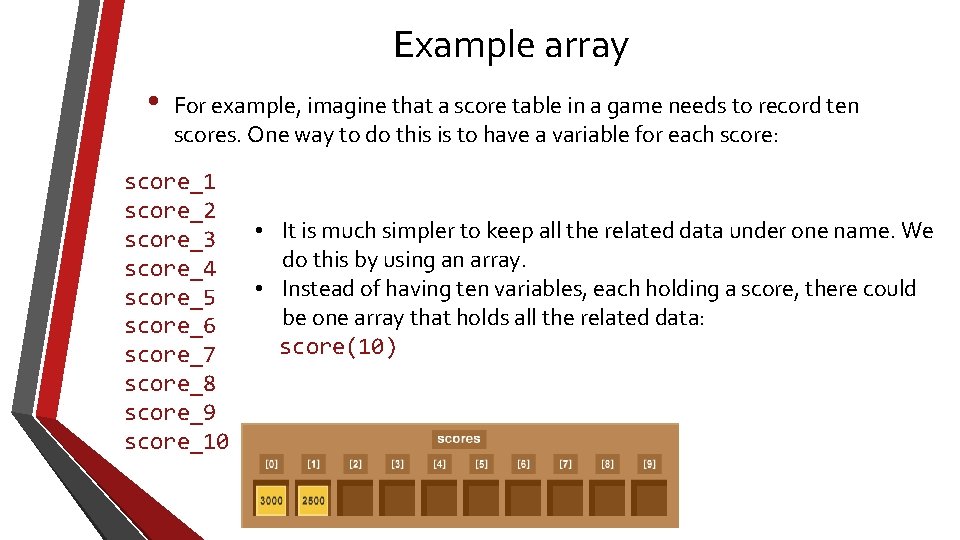
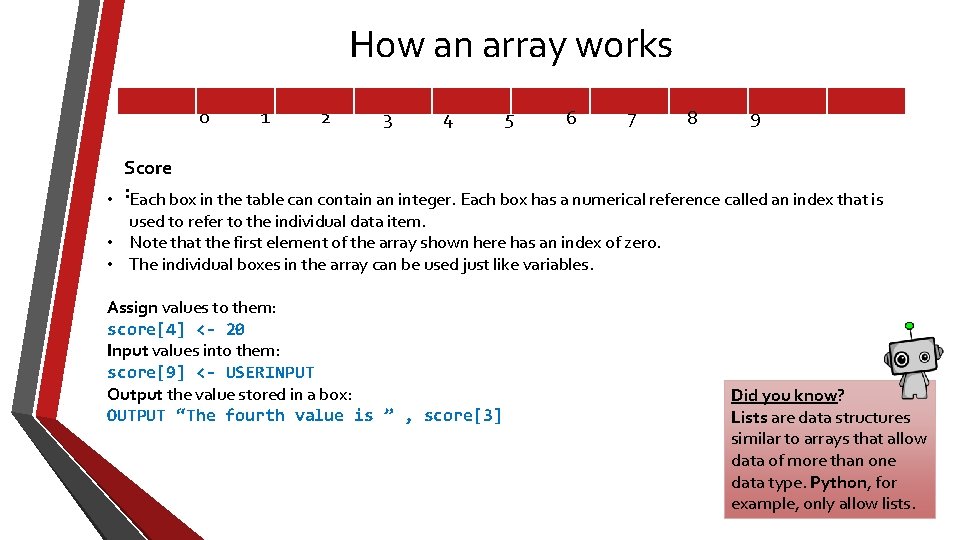
![Understanding an array total <- 0 FOR game <- 0 TO 11 score [game] Understanding an array total <- 0 FOR game <- 0 TO 11 score [game]](https://slidetodoc.com/presentation_image_h/c283c49836795c4a4def5aad8c9b842b/image-24.jpg)
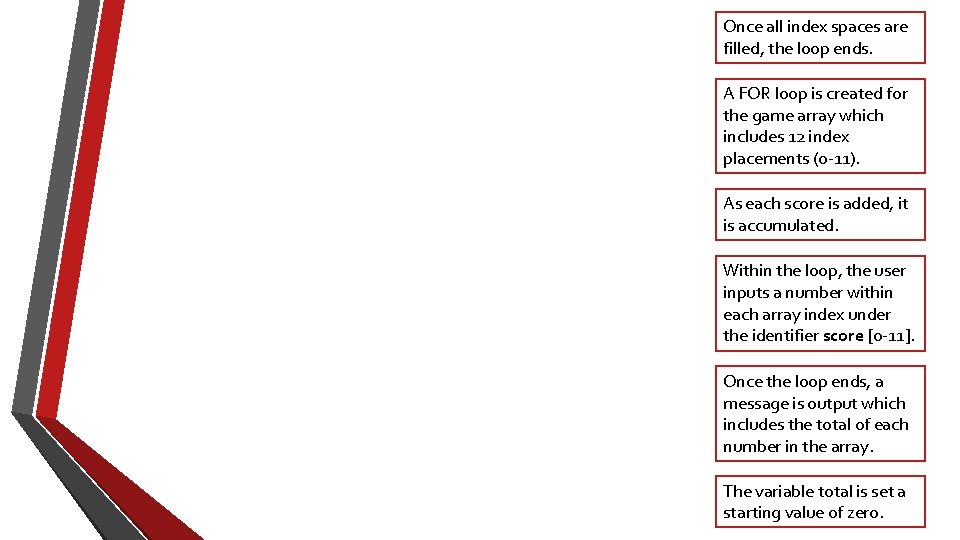
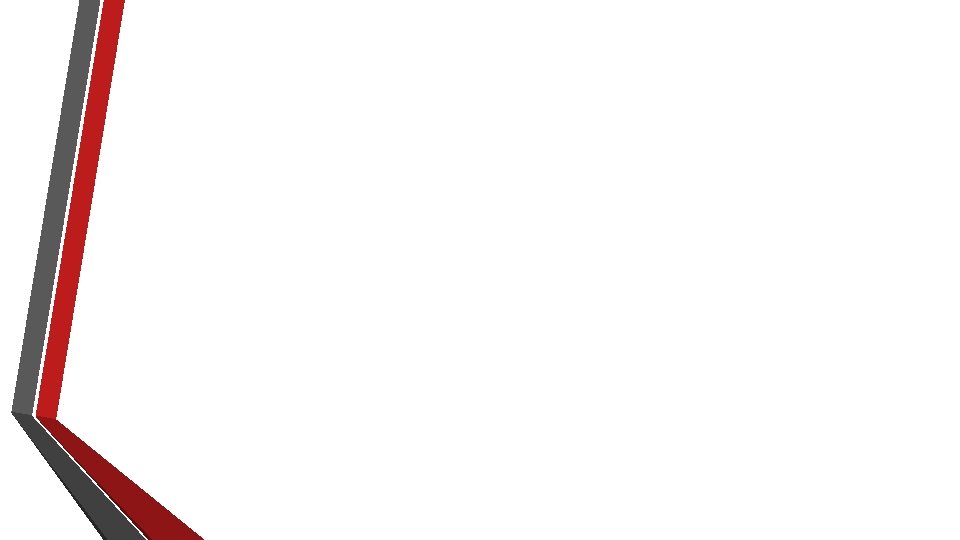
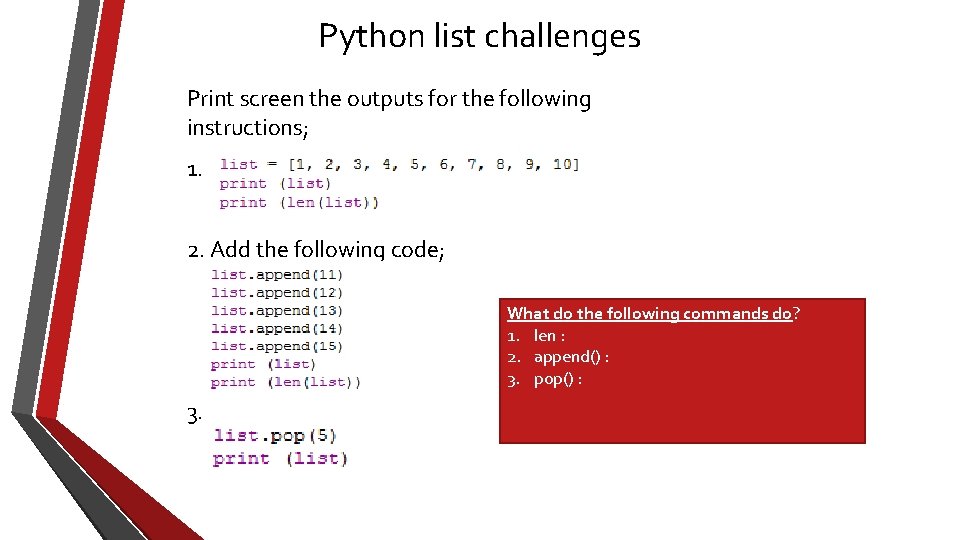
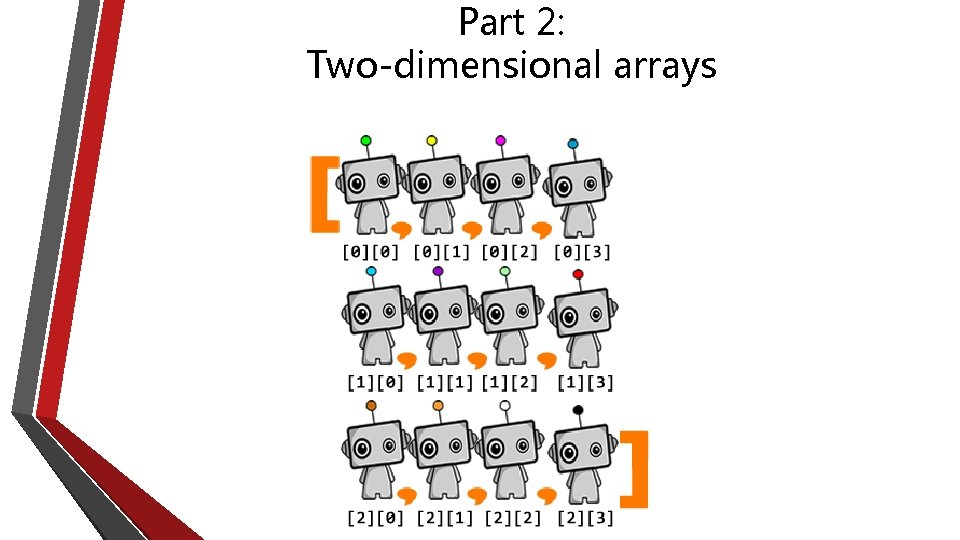
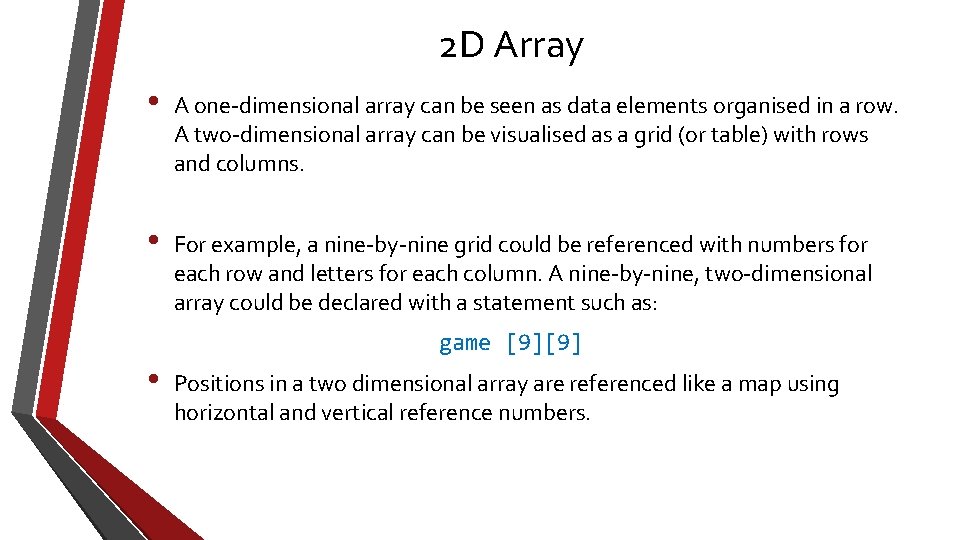
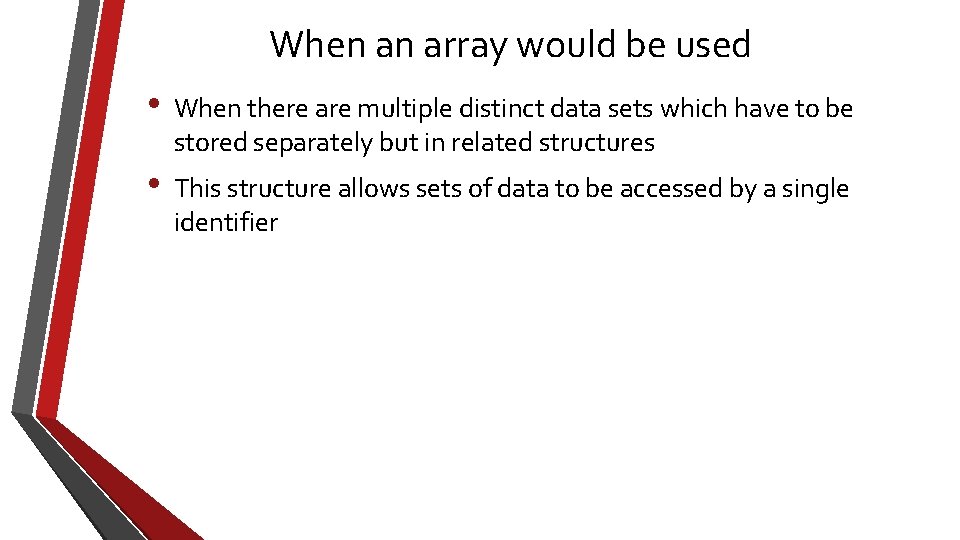
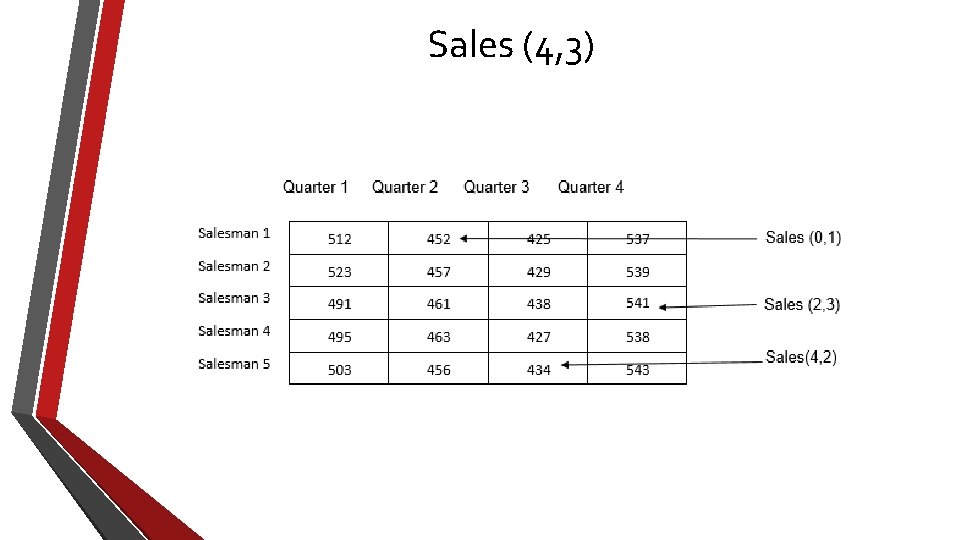
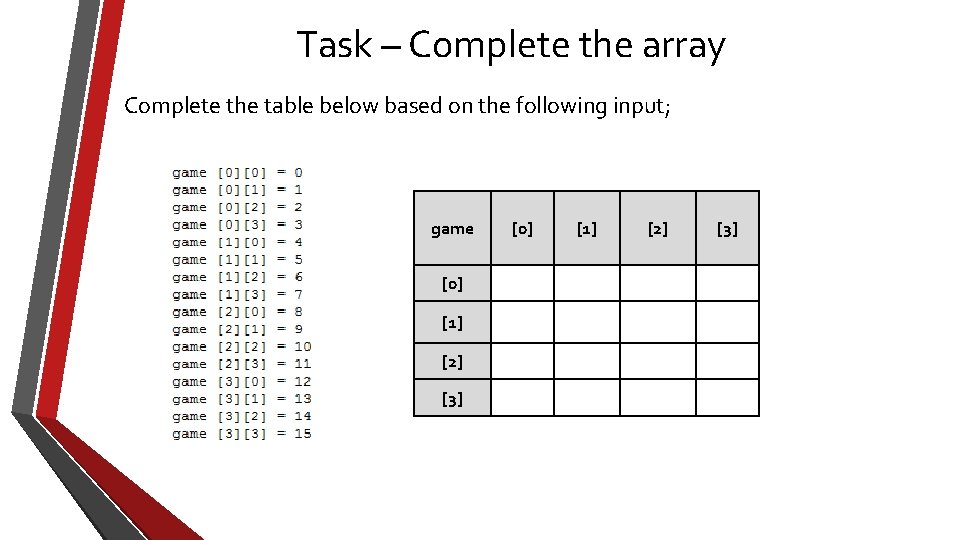
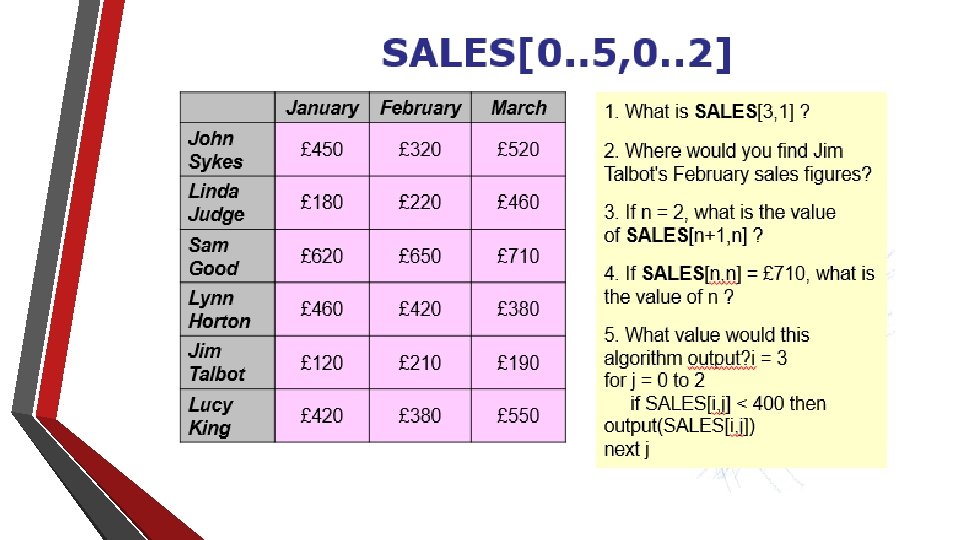
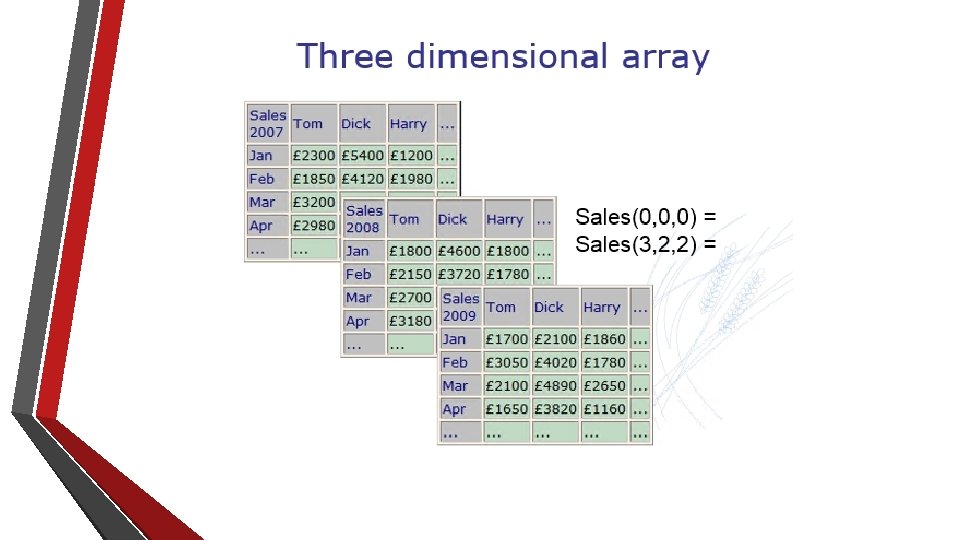
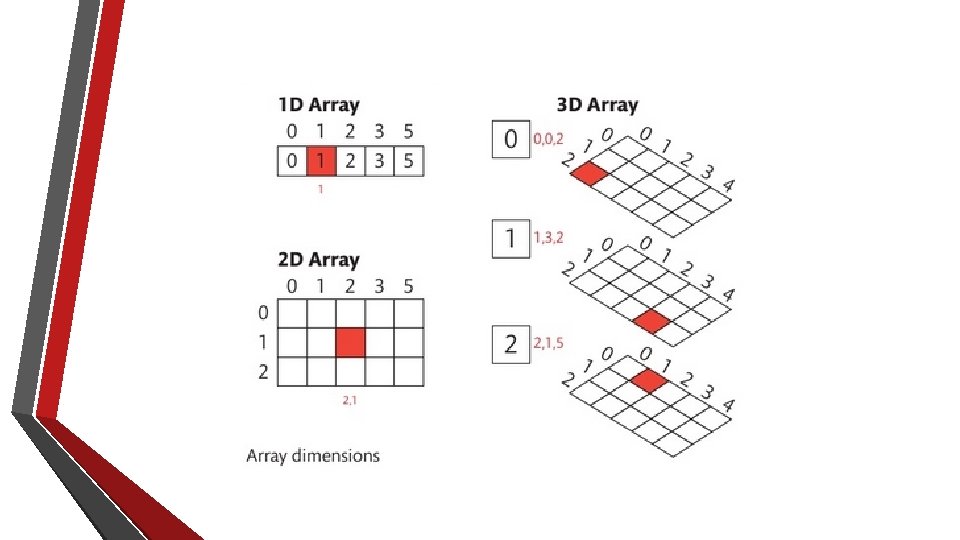
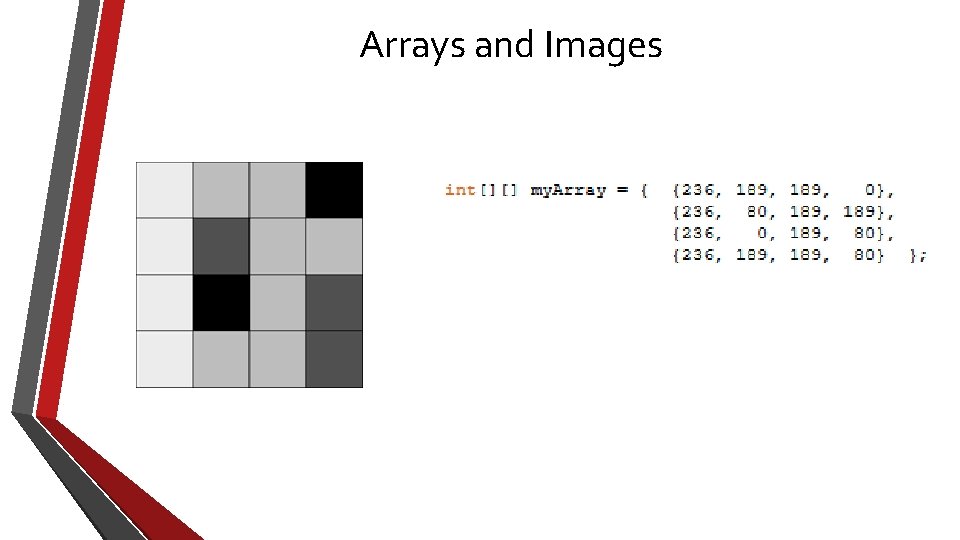
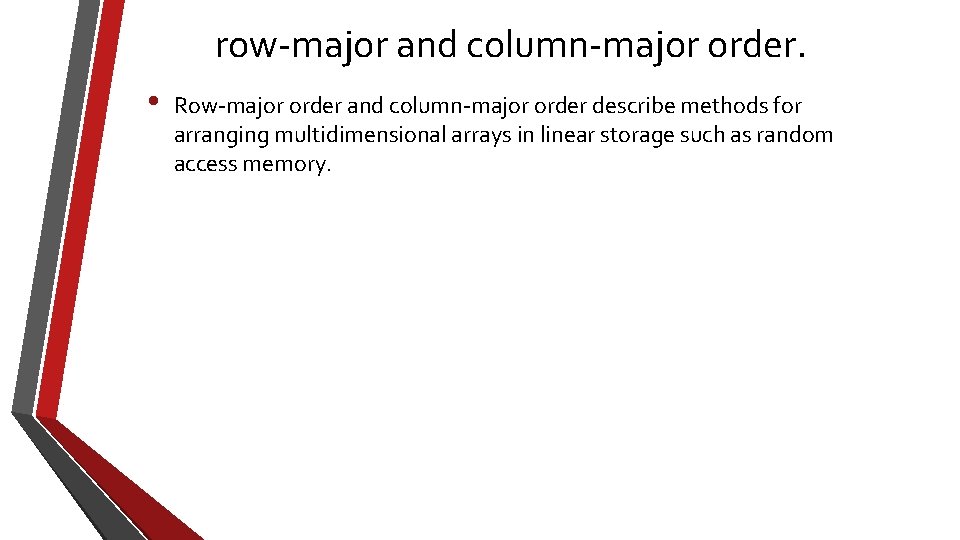
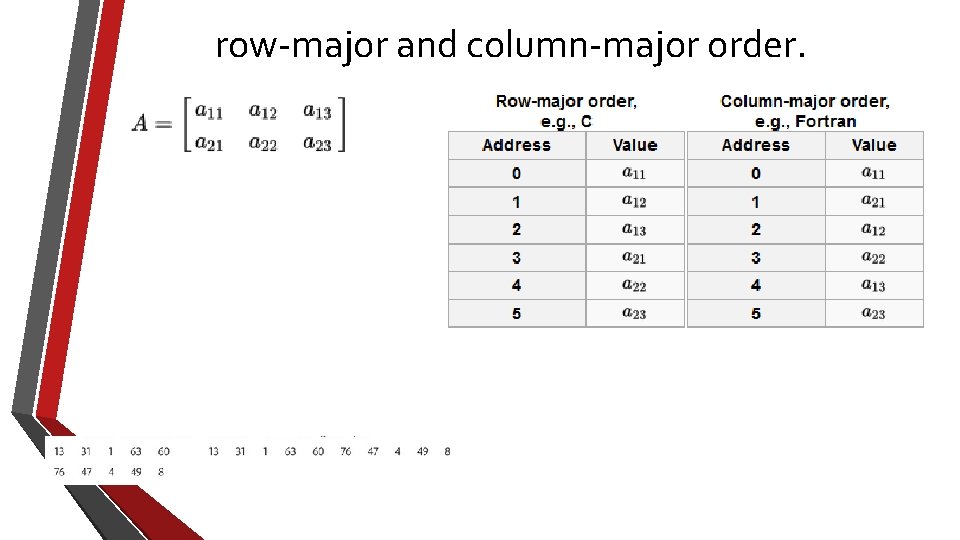
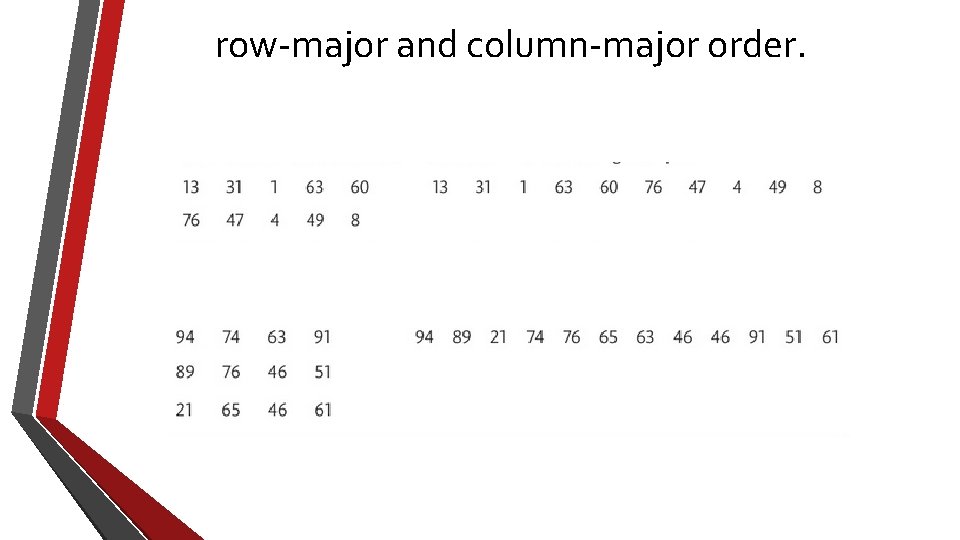
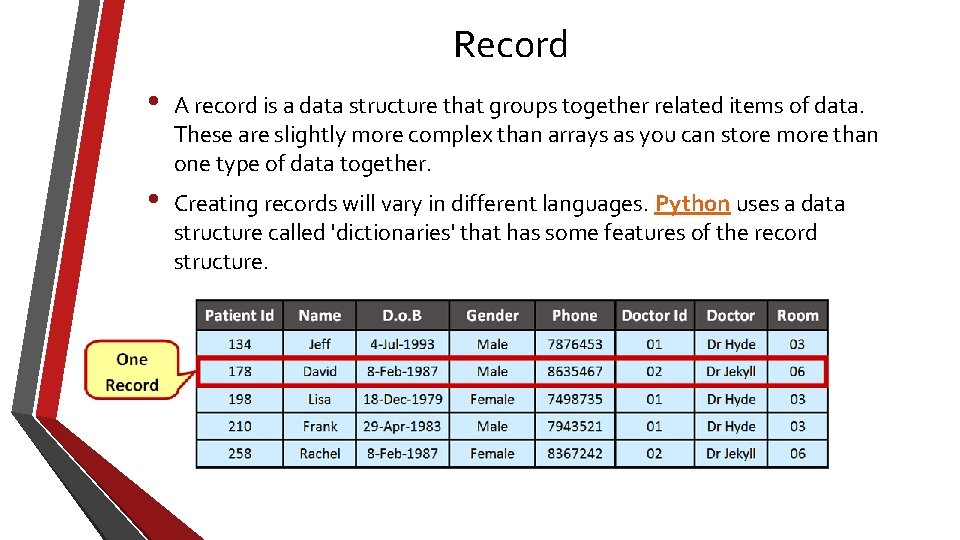
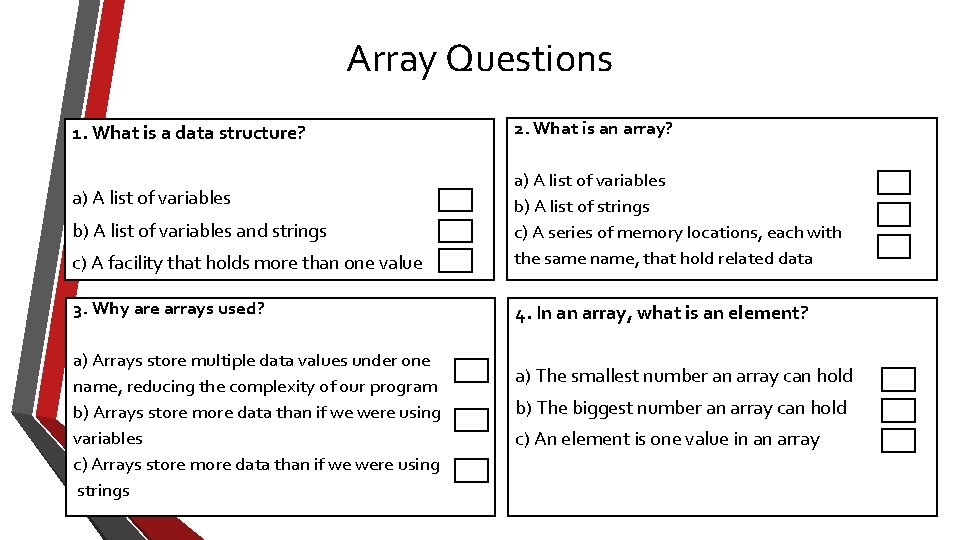
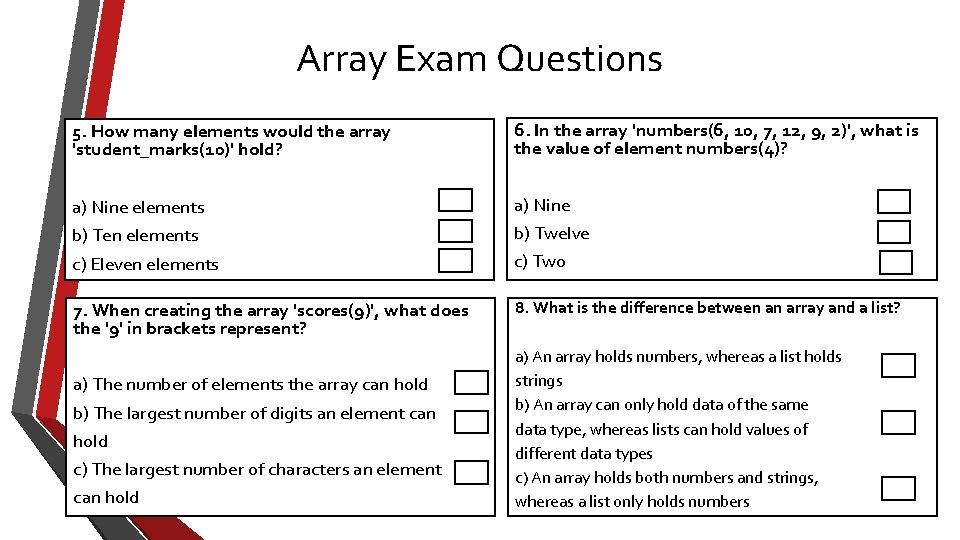
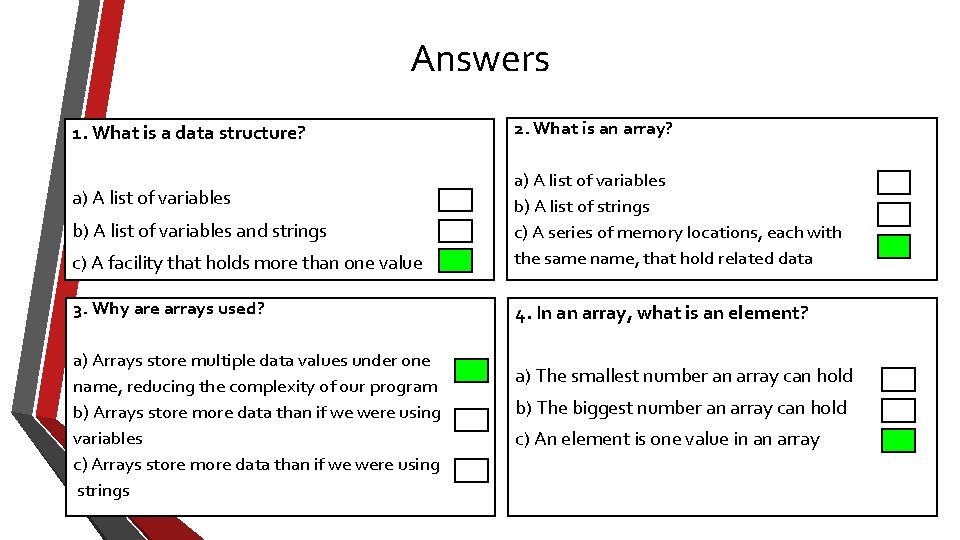
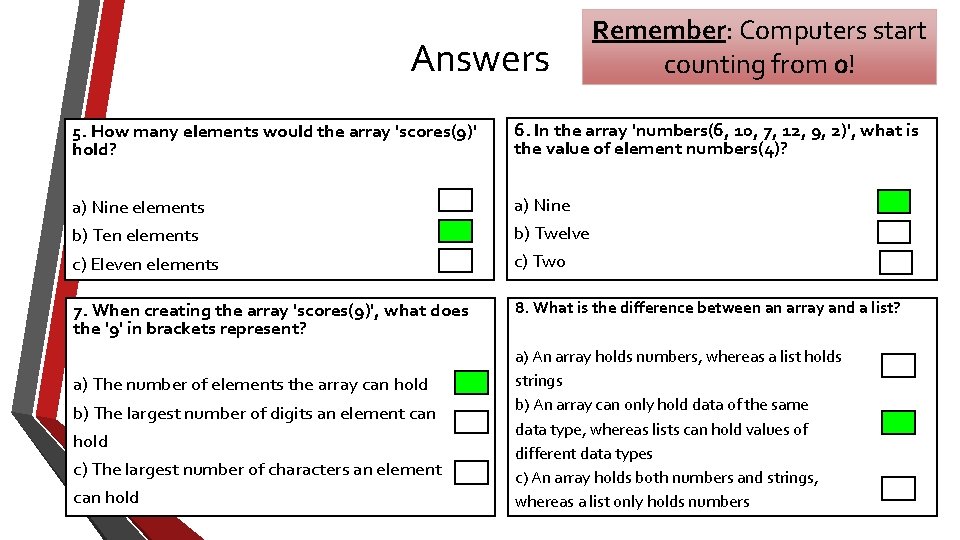
- Slides: 44
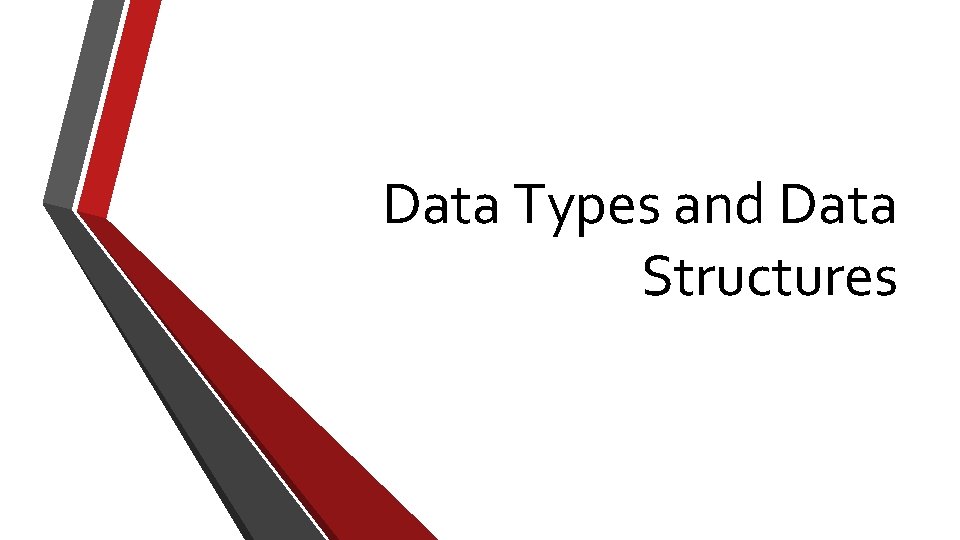
Data Types and Data Structures
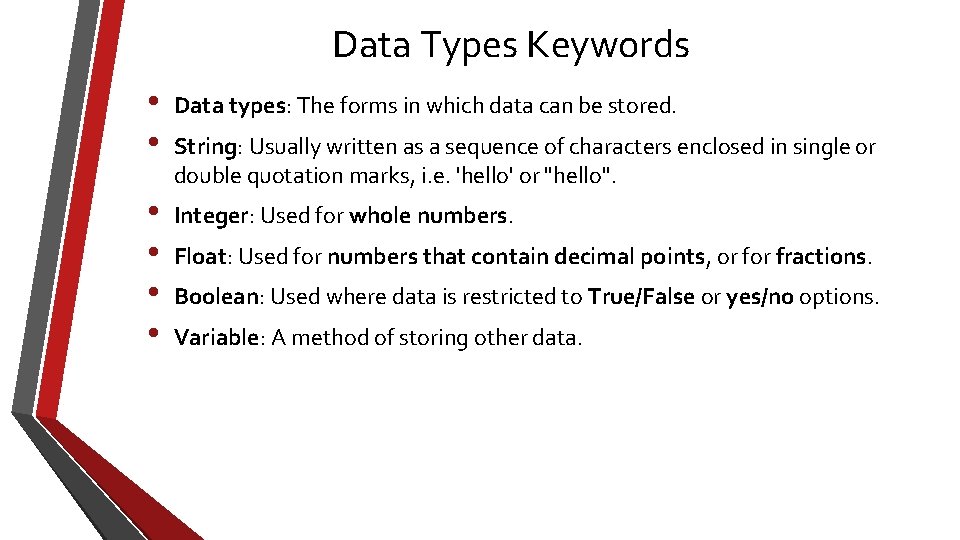
Data Types Keywords • • Data types: The forms in which data can be stored. • • Integer: Used for whole numbers. String: Usually written as a sequence of characters enclosed in single or double quotation marks, i. e. 'hello' or "hello". Float: Used for numbers that contain decimal points, or fractions. Boolean: Used where data is restricted to True/False or yes/no options. Variable: A method of storing other data.
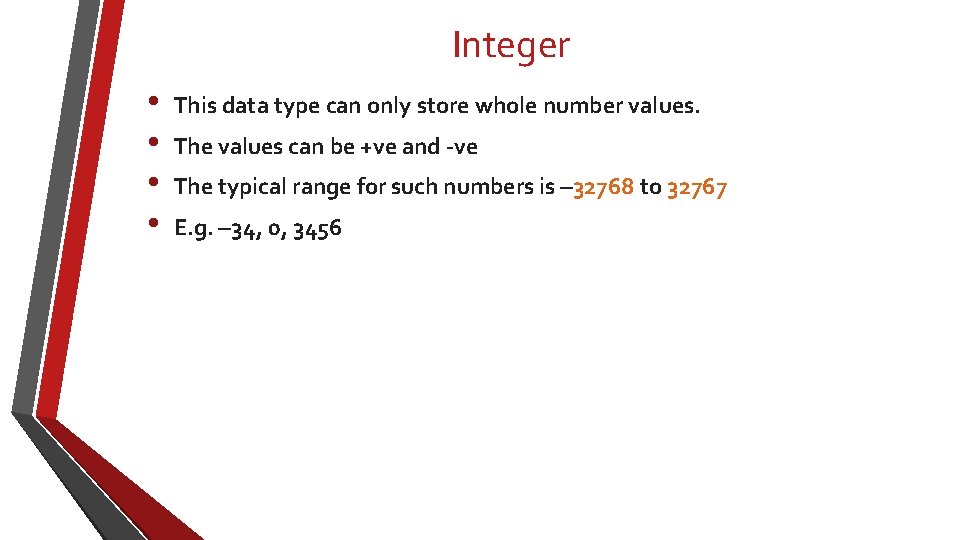
Integer • • This data type can only store whole number values. The values can be +ve and -ve The typical range for such numbers is – 32768 to 32767 E. g. – 34, 0, 3456
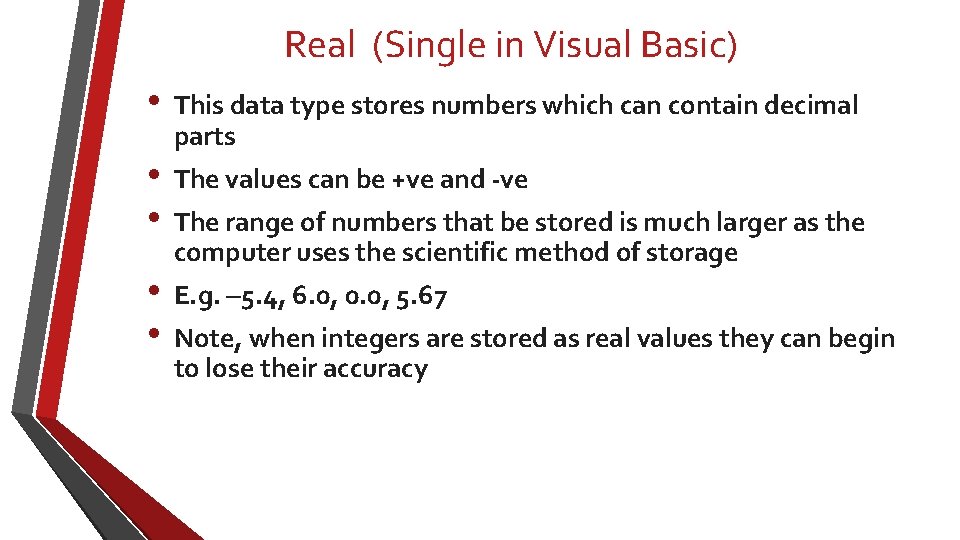
Real (Single in Visual Basic) • • • This data type stores numbers which can contain decimal parts The values can be +ve and -ve The range of numbers that be stored is much larger as the computer uses the scientific method of storage E. g. – 5. 4, 6. 0, 0. 0, 5. 67 Note, when integers are stored as real values they can begin to lose their accuracy
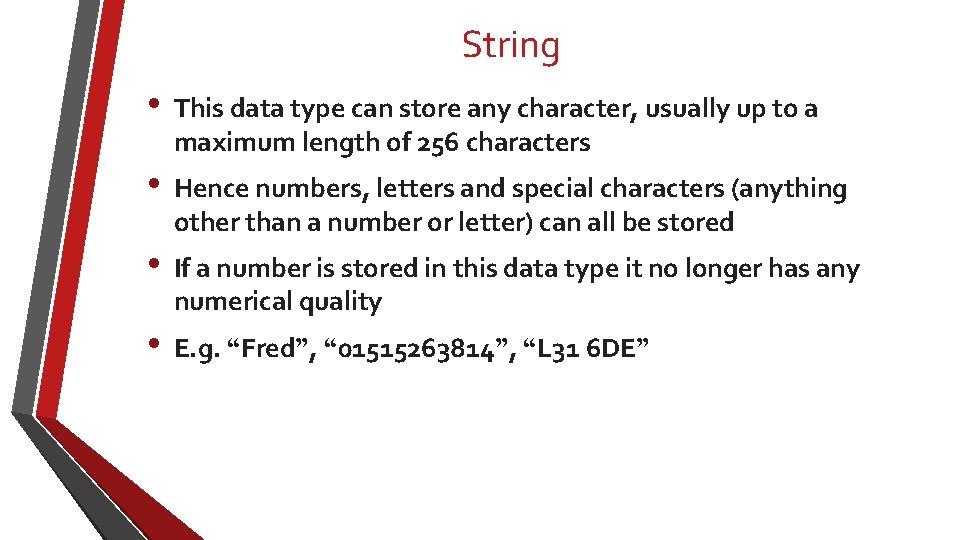
String • This data type can store any character, usually up to a maximum length of 256 characters • Hence numbers, letters and special characters (anything other than a number or letter) can all be stored • If a number is stored in this data type it no longer has any numerical quality • E. g. “Fred”, “ 01515263814”, “L 31 6 DE”
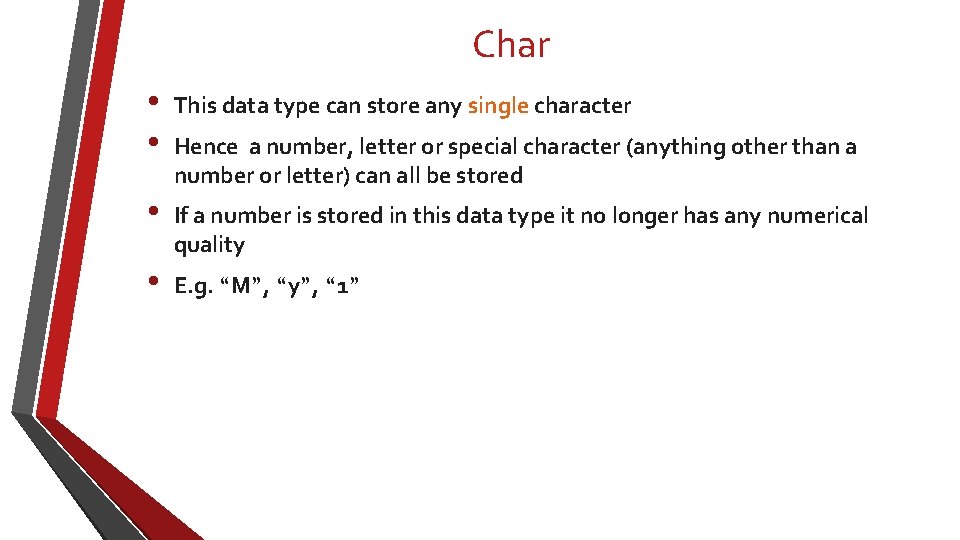
Char • • This data type can store any single character • If a number is stored in this data type it no longer has any numerical quality • E. g. “M”, “y”, “ 1” Hence a number, letter or special character (anything other than a number or letter) can all be stored
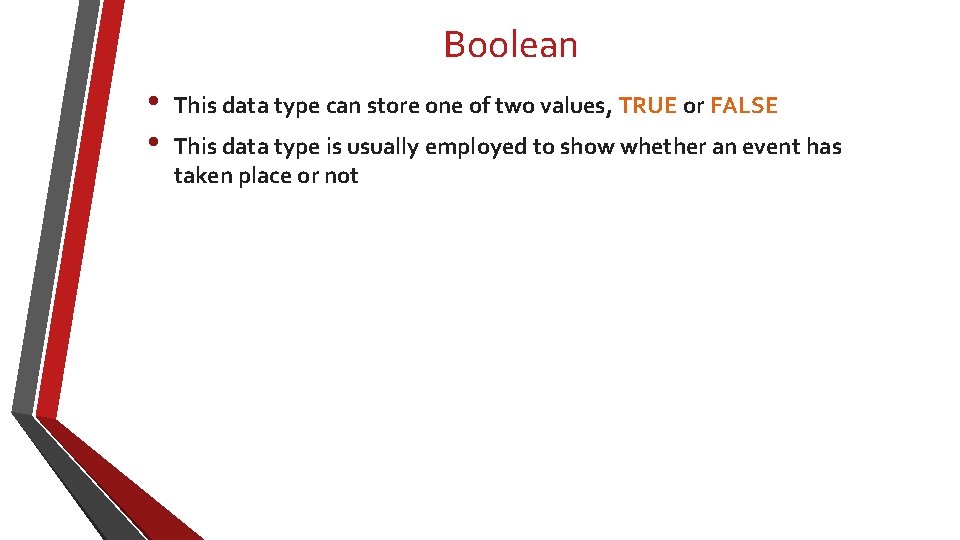
Boolean • • This data type can store one of two values, TRUE or FALSE This data type is usually employed to show whether an event has taken place or not
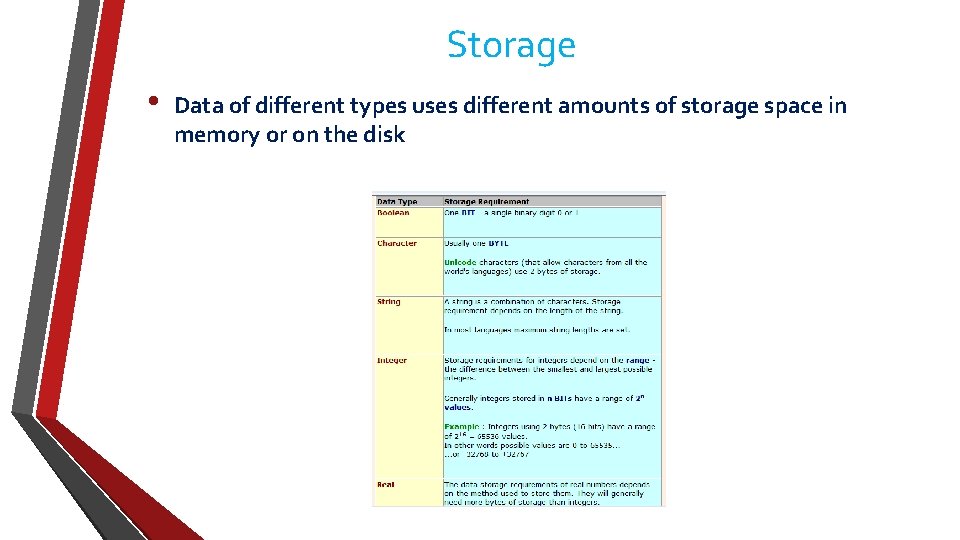
Storage • Data of different types uses different amounts of storage space in memory or on the disk
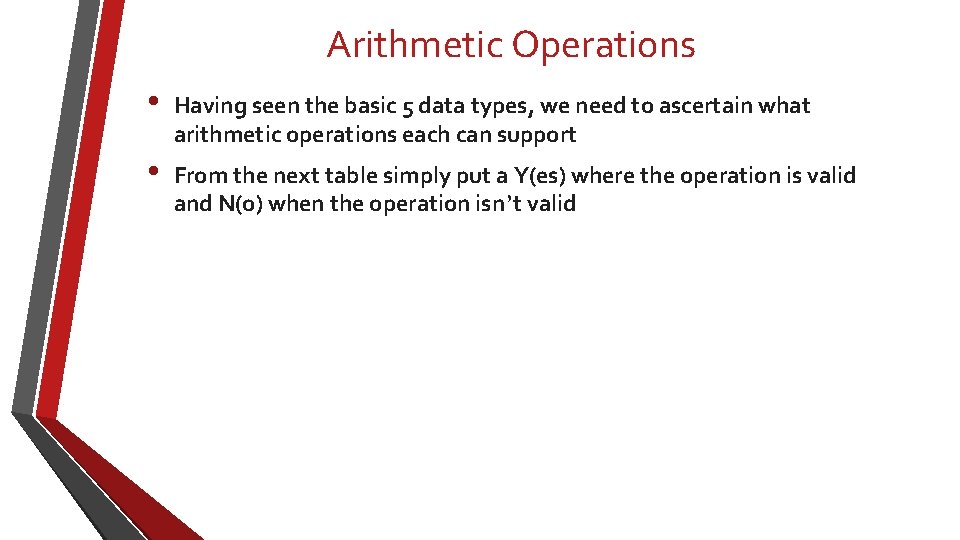
Arithmetic Operations • Having seen the basic 5 data types, we need to ascertain what arithmetic operations each can support • From the next table simply put a Y(es) where the operation is valid and N(o) when the operation isn’t valid
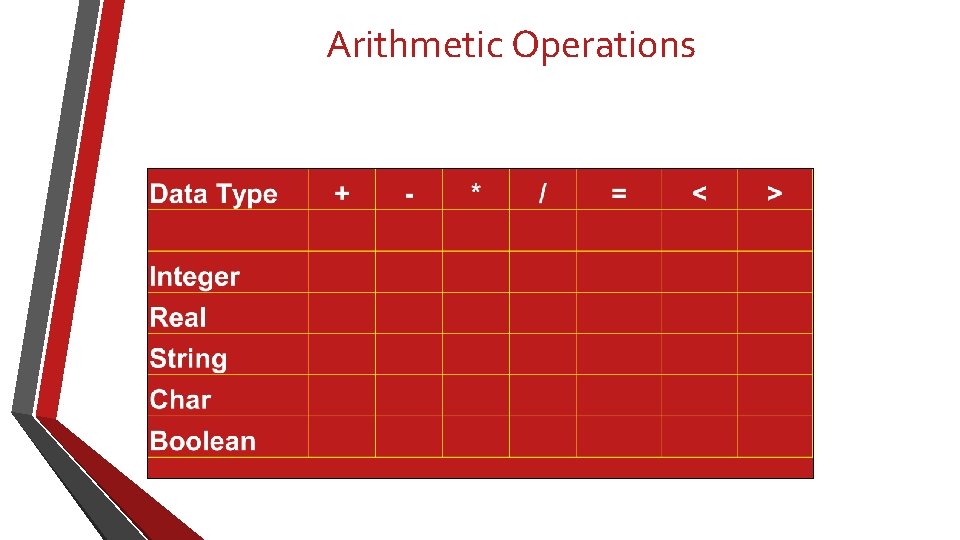
Arithmetic Operations
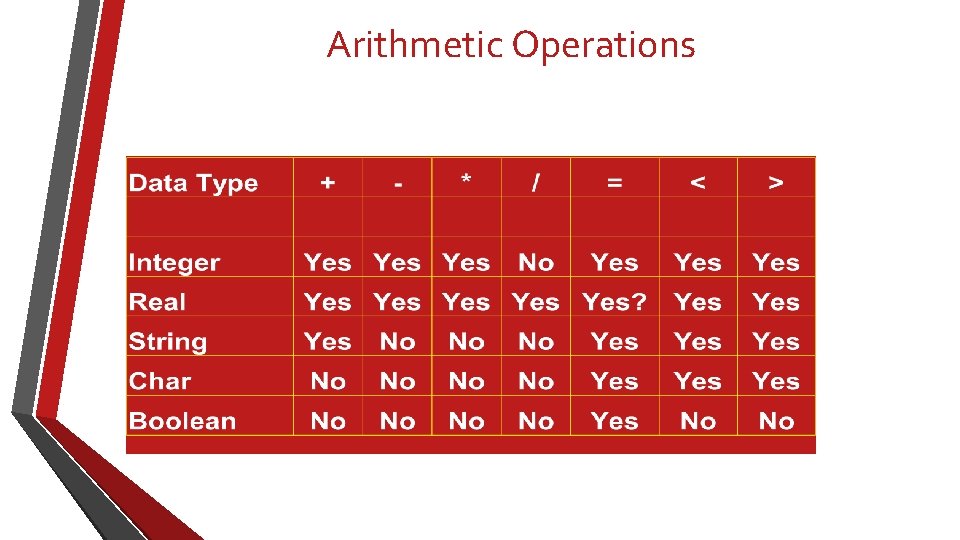
Arithmetic Operations
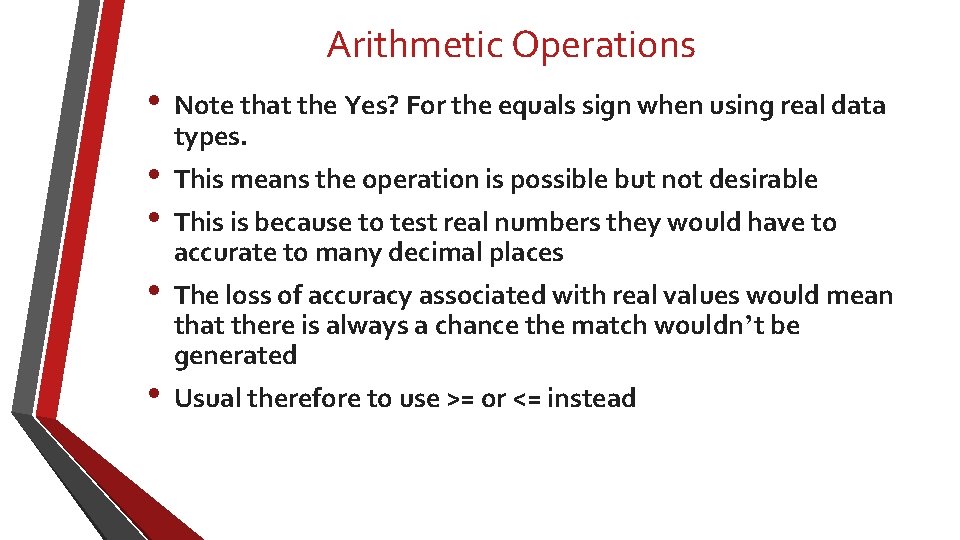
Arithmetic Operations • • • Note that the Yes? For the equals sign when using real data types. This means the operation is possible but not desirable This is because to test real numbers they would have to accurate to many decimal places The loss of accuracy associated with real values would mean that there is always a chance the match wouldn’t be generated Usual therefore to use >= or <= instead
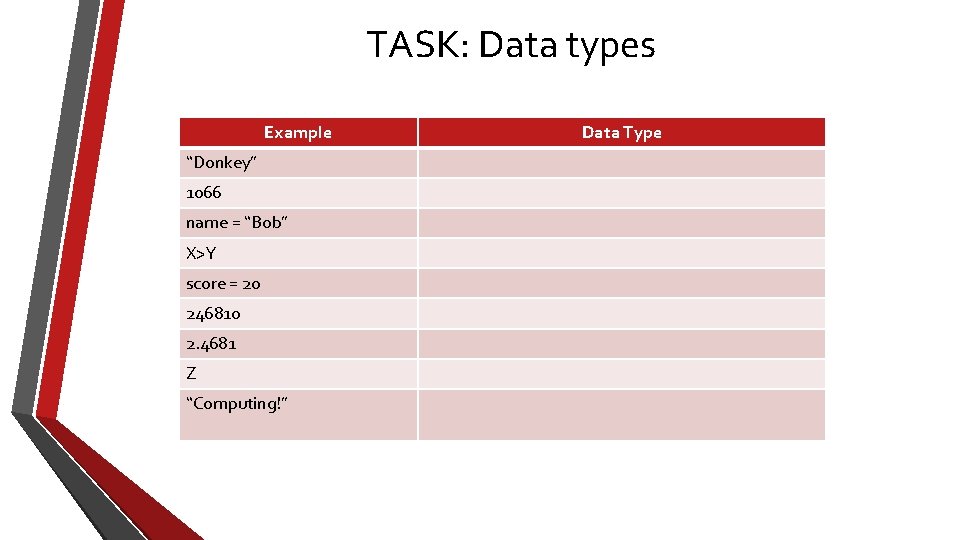
TASK: Data types Example “Donkey” 1066 name = “Bob” X>Y score = 20 246810 2. 4681 Z “Computing!” Data Type
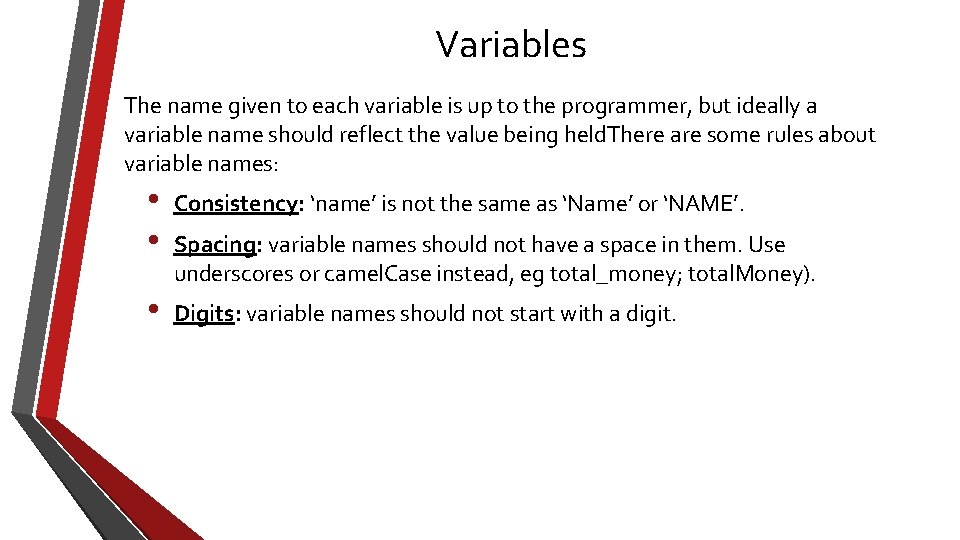
Variables The name given to each variable is up to the programmer, but ideally a variable name should reflect the value being held. There are some rules about variable names: • • Consistency: ‘name’ is not the same as ‘Name’ or ‘NAME’. • Digits: variable names should not start with a digit. Spacing: variable names should not have a space in them. Use underscores or camel. Case instead, eg total_money; total. Money).
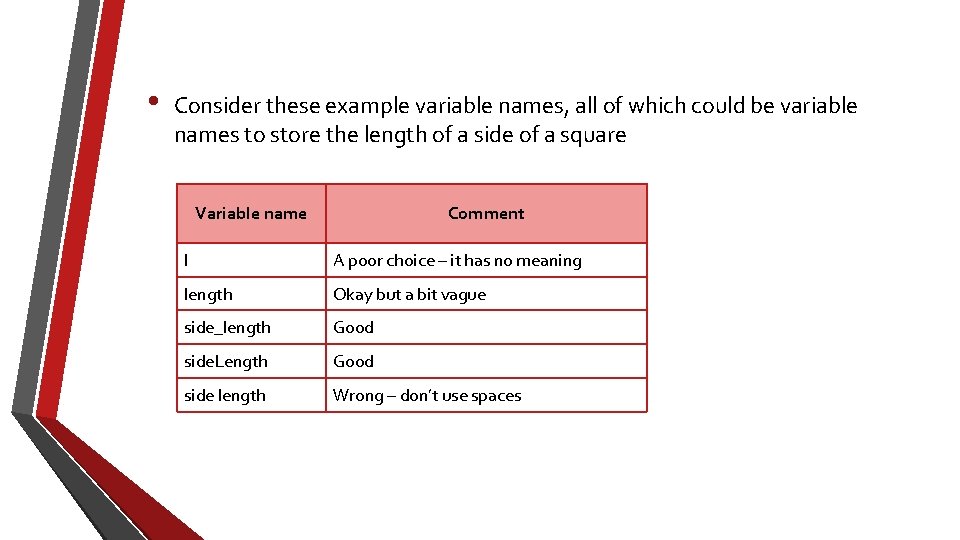
• Consider these example variable names, all of which could be variable names to store the length of a side of a square Variable name Comment l A poor choice – it has no meaning length Okay but a bit vague side_length Good side. Length Good side length Wrong – don’t use spaces
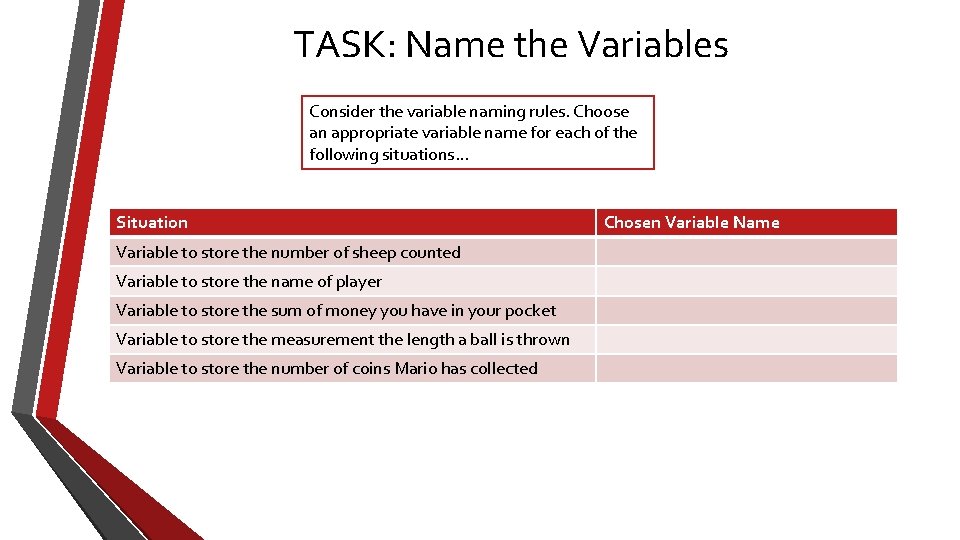
TASK: Name the Variables Consider the variable naming rules. Choose an appropriate variable name for each of the following situations… Situation Variable to store the number of sheep counted Variable to store the name of player Variable to store the sum of money you have in your pocket Variable to store the measurement the length a ball is thrown Variable to store the number of coins Mario has collected Chosen Variable Name
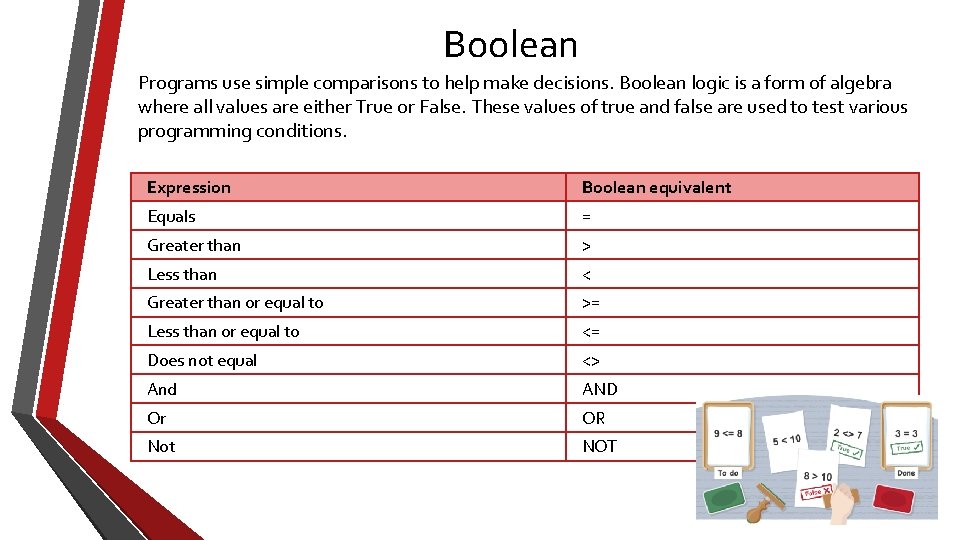
Boolean Programs use simple comparisons to help make decisions. Boolean logic is a form of algebra where all values are either True or False. These values of true and false are used to test various programming conditions. Expression Boolean equivalent Equals = Greater than > Less than < Greater than or equal to >= Less than or equal to <= Does not equal <> And AND Or OR Not NOT
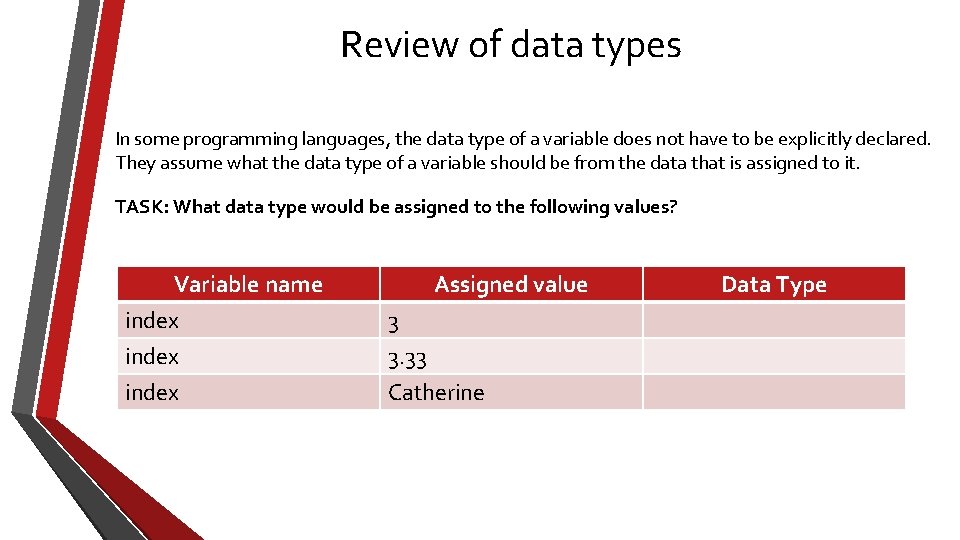
Review of data types In some programming languages, the data type of a variable does not have to be explicitly declared. They assume what the data type of a variable should be from the data that is assigned to it. TASK: What data type would be assigned to the following values? Variable name index Assigned value 3 3. 33 Catherine Data Type
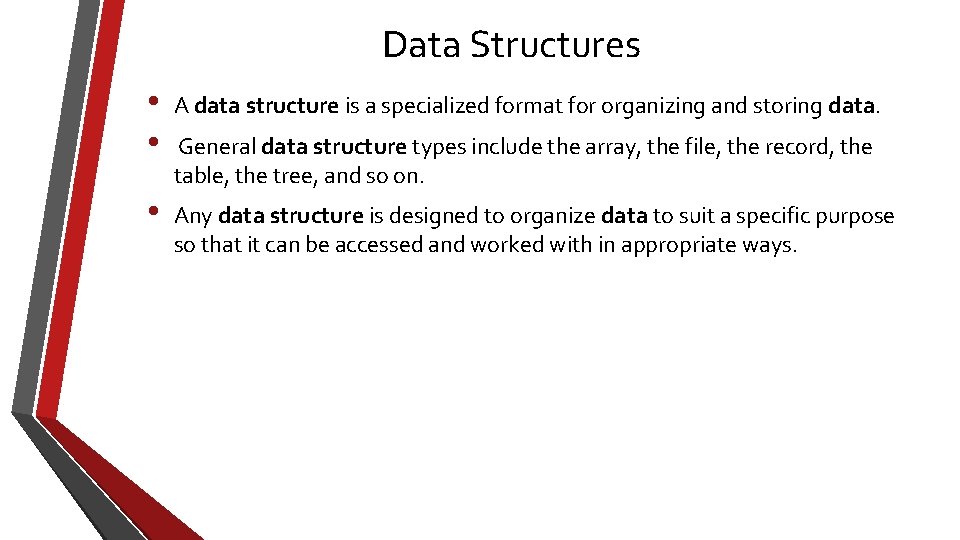
Data Structures • • A data structure is a specialized format for organizing and storing data. • Any data structure is designed to organize data to suit a specific purpose so that it can be accessed and worked with in appropriate ways. General data structure types include the array, the file, the record, the table, the tree, and so on.
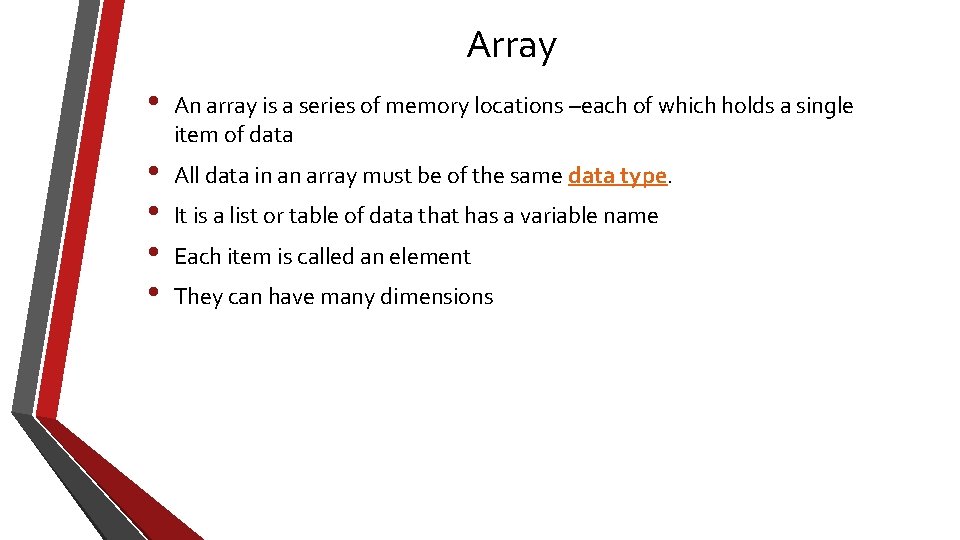
Array • An array is a series of memory locations –each of which holds a single item of data • • All data in an array must be of the same data type. It is a list or table of data that has a variable name Each item is called an element They can have many dimensions
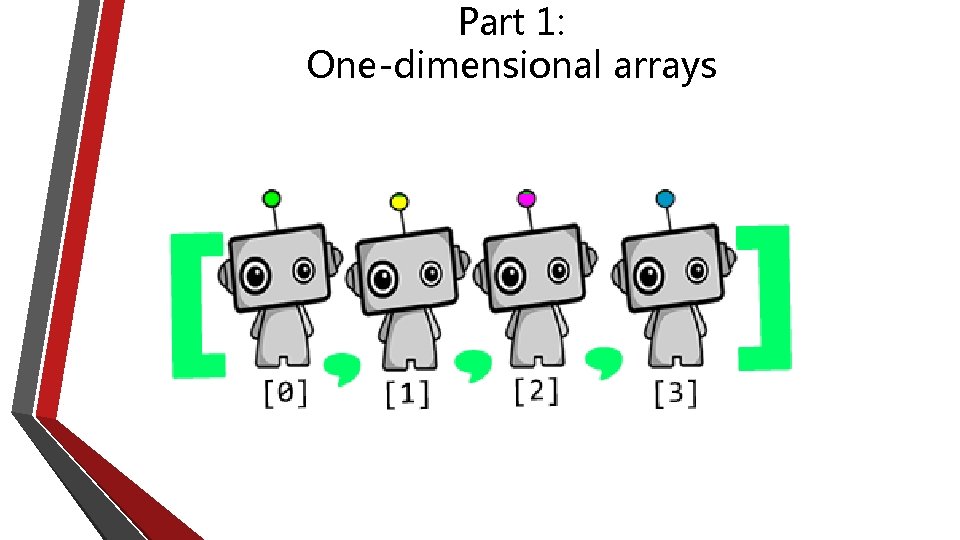
Part 1: One-dimensional arrays
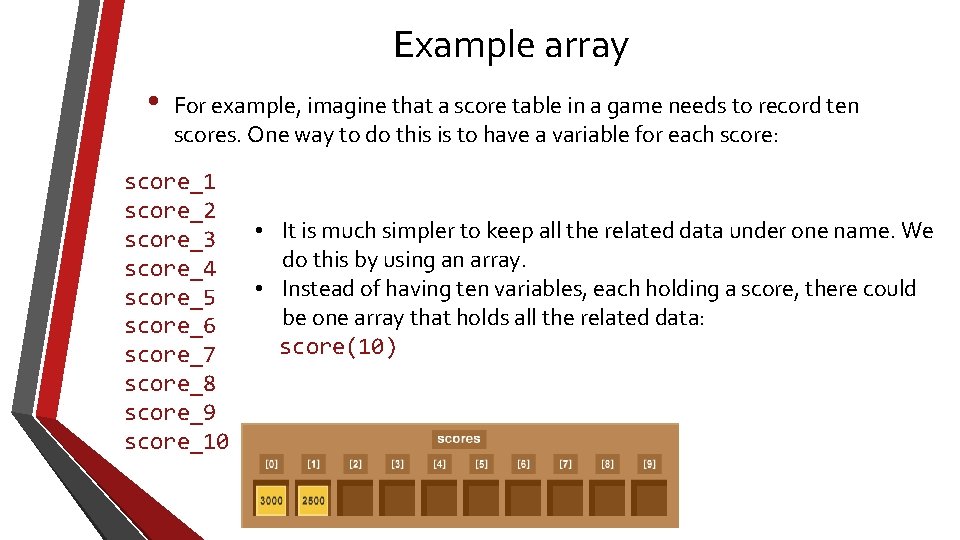
Example array • For example, imagine that a score table in a game needs to record ten scores. One way to do this is to have a variable for each score: score_1 score_2 score_3 score_4 score_5 score_6 score_7 score_8 score_9 score_10 • It is much simpler to keep all the related data under one name. We do this by using an array. • Instead of having ten variables, each holding a score, there could be one array that holds all the related data: score(10)
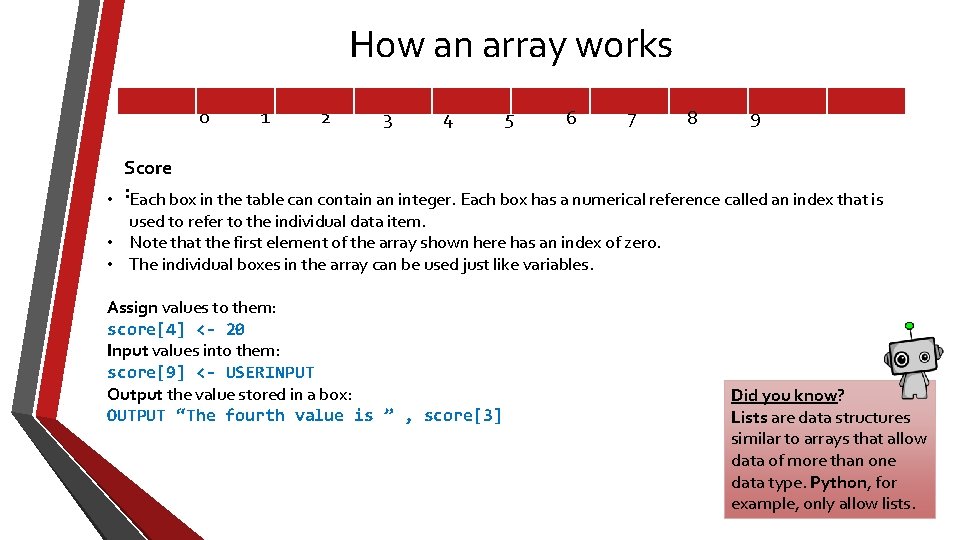
How an array works 0 1 2 3 4 5 6 7 8 9 Score • : Each box in the table can contain an integer. Each box has a numerical reference called an index that is used to refer to the individual data item. • Note that the first element of the array shown here has an index of zero. • The individual boxes in the array can be used just like variables. Assign values to them: score[4] <- 20 Input values into them: score[9] <- USERINPUT Output the value stored in a box: OUTPUT “The fourth value is ” , score[3] Did you know? Lists are data structures similar to arrays that allow data of more than one data type. Python, for example, only allow lists.
![Understanding an array total 0 FOR game 0 TO 11 score game Understanding an array total <- 0 FOR game <- 0 TO 11 score [game]](https://slidetodoc.com/presentation_image_h/c283c49836795c4a4def5aad8c9b842b/image-24.jpg)
Understanding an array total <- 0 FOR game <- 0 TO 11 score [game] <- USERINPUT total <- total + score [game] ENDFOR OUTPUT “The total is “, total Annotate the following pseudocode to show explain what is happening;
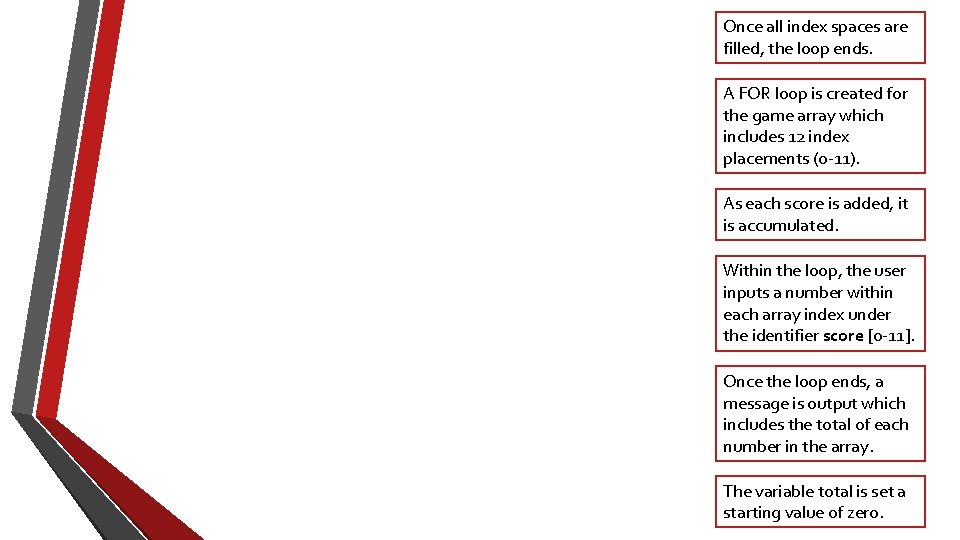
Once all index spaces are filled, the loop ends. A FOR loop is created for the game array which includes 12 index placements (0 -11). As each score is added, it is accumulated. Within the loop, the user inputs a number within each array index under the identifier score [0 -11]. Once the loop ends, a message is output which includes the total of each number in the array. The variable total is set a starting value of zero.
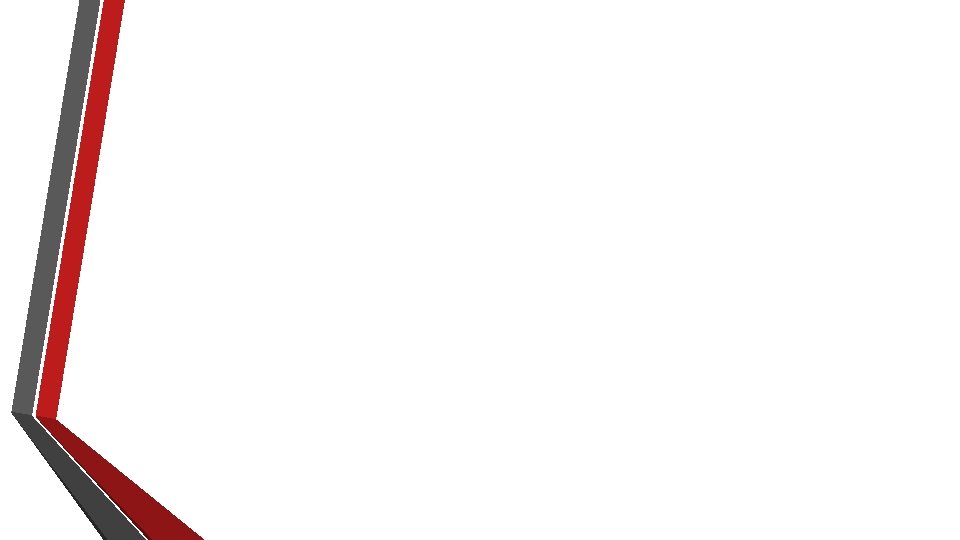
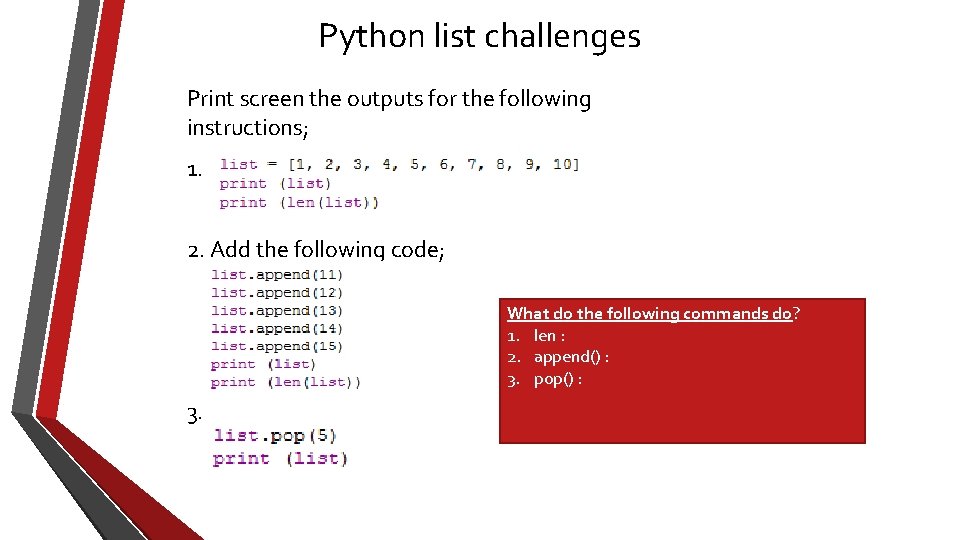
Python list challenges Print screen the outputs for the following instructions; 1. 2. Add the following code; What do the following commands do? 1. len : 2. append() : 3. pop() : 3.
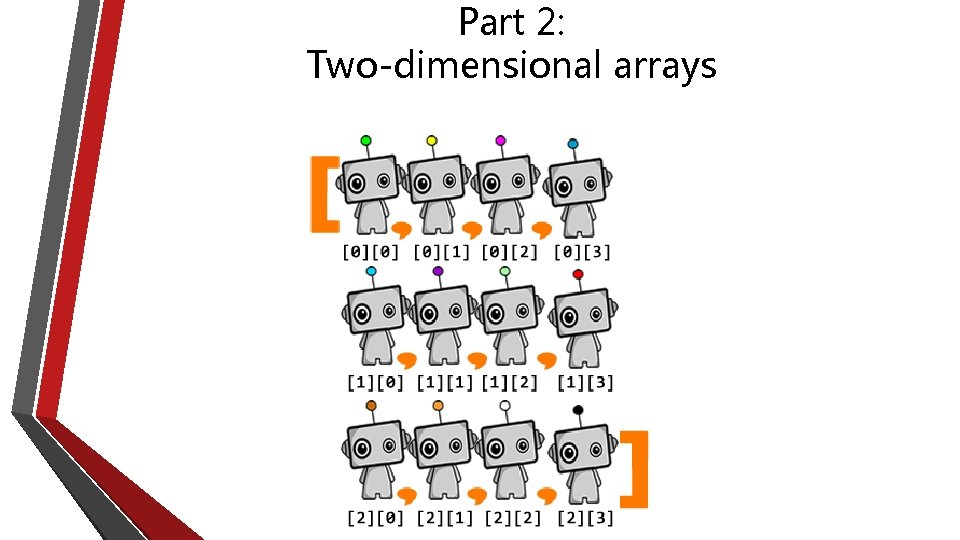
Part 2: Two-dimensional arrays
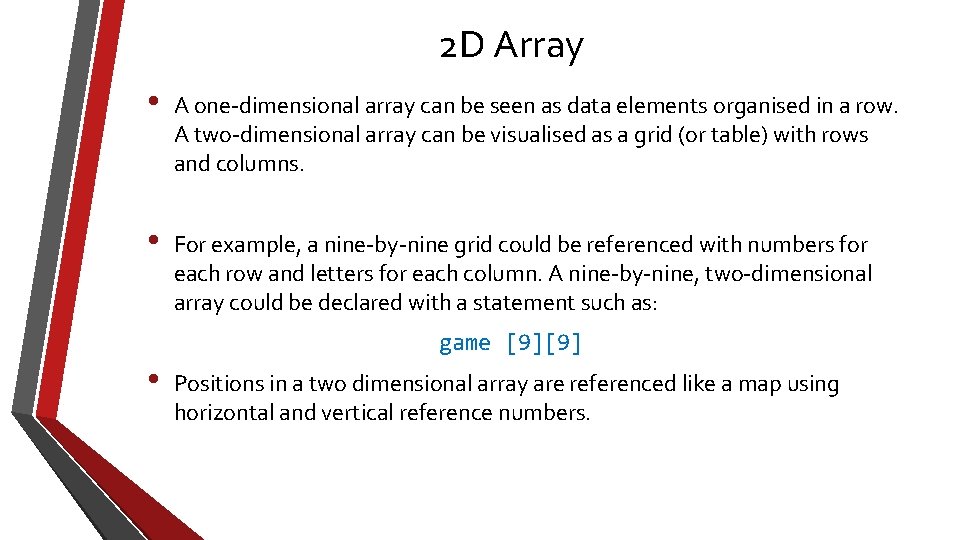
2 D Array • A one-dimensional array can be seen as data elements organised in a row. A two-dimensional array can be visualised as a grid (or table) with rows and columns. • For example, a nine-by-nine grid could be referenced with numbers for each row and letters for each column. A nine-by-nine, two-dimensional array could be declared with a statement such as: game [9][9] • Positions in a two dimensional array are referenced like a map using horizontal and vertical reference numbers.
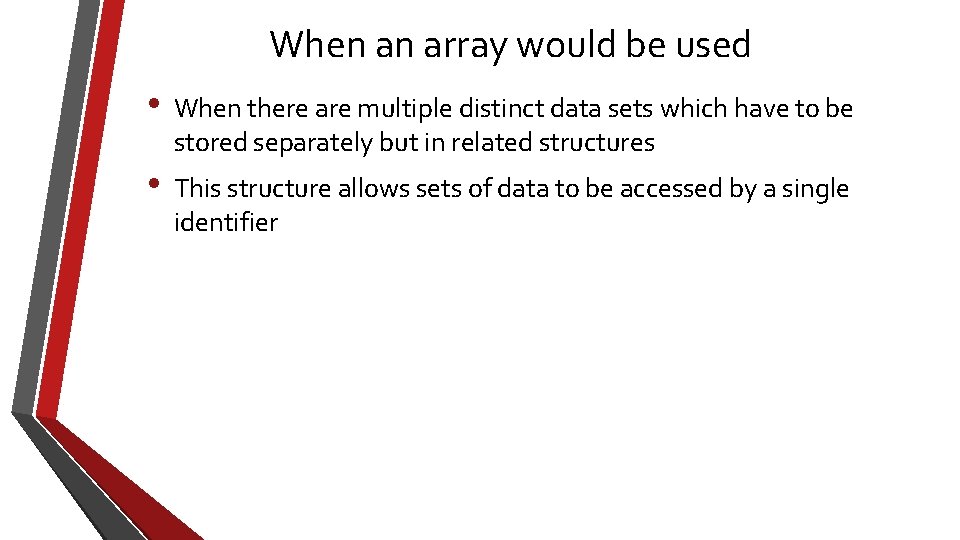
When an array would be used • When there are multiple distinct data sets which have to be stored separately but in related structures • This structure allows sets of data to be accessed by a single identifier
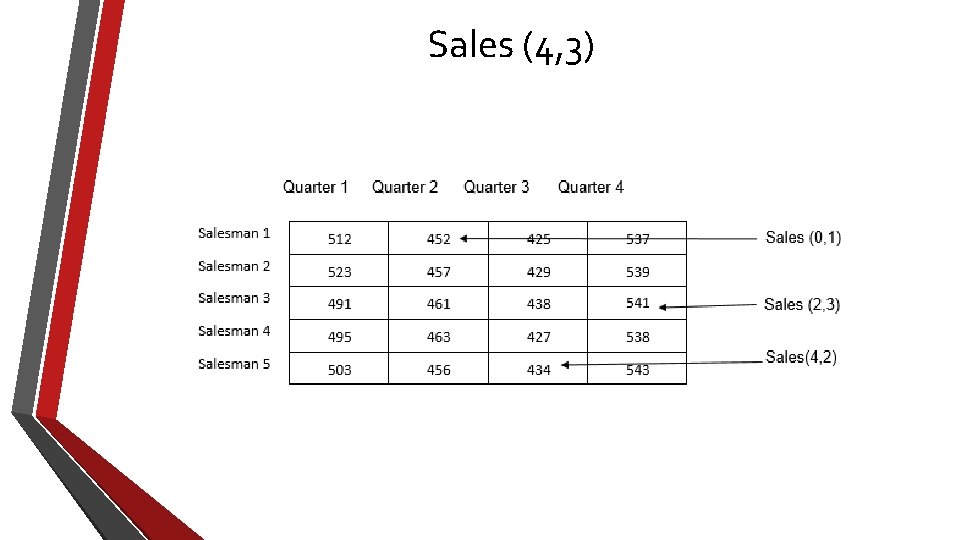
Sales (4, 3)
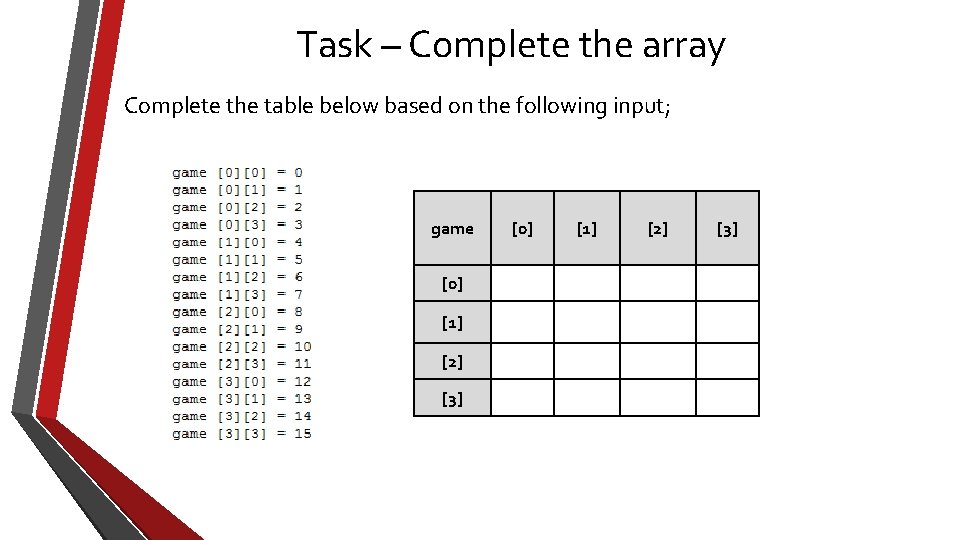
Task – Complete the array Complete the table below based on the following input; game [0] [1] [2] [3]
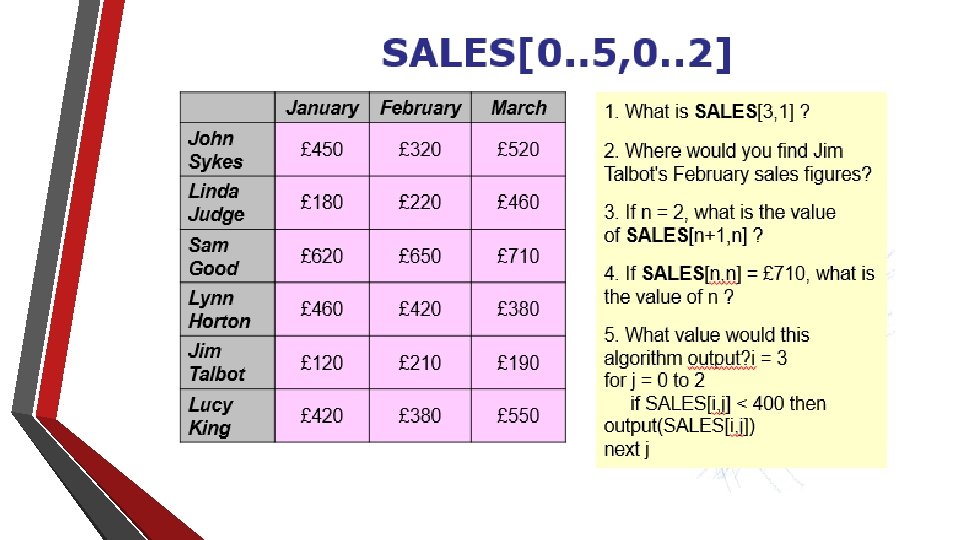
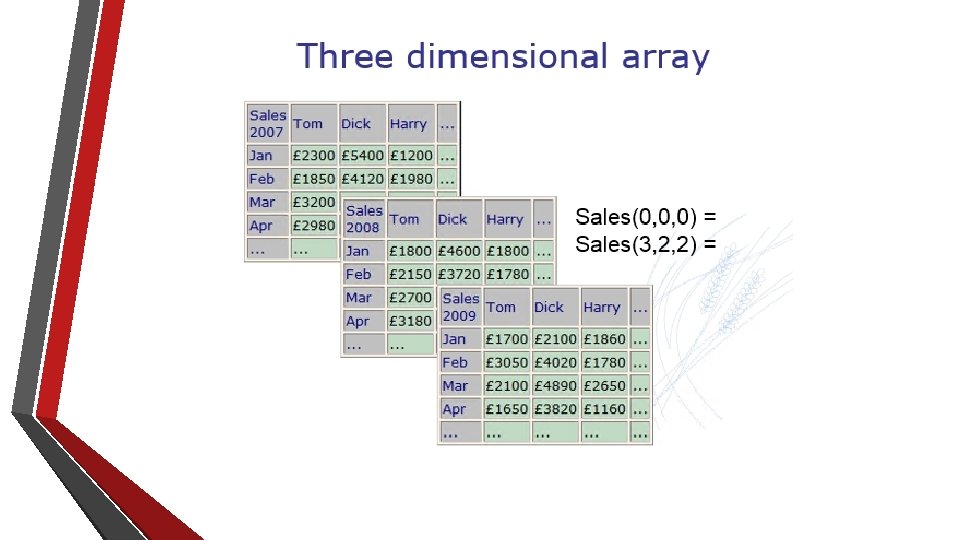
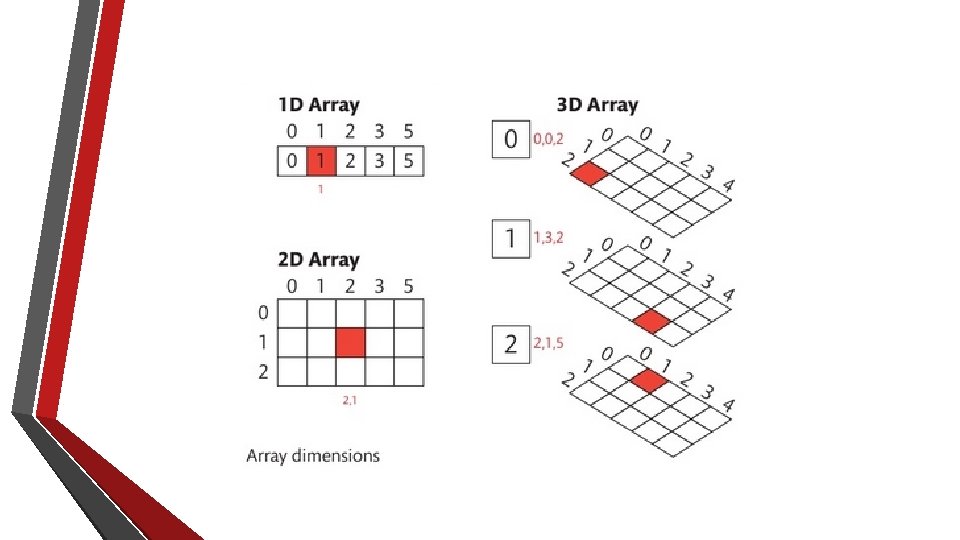
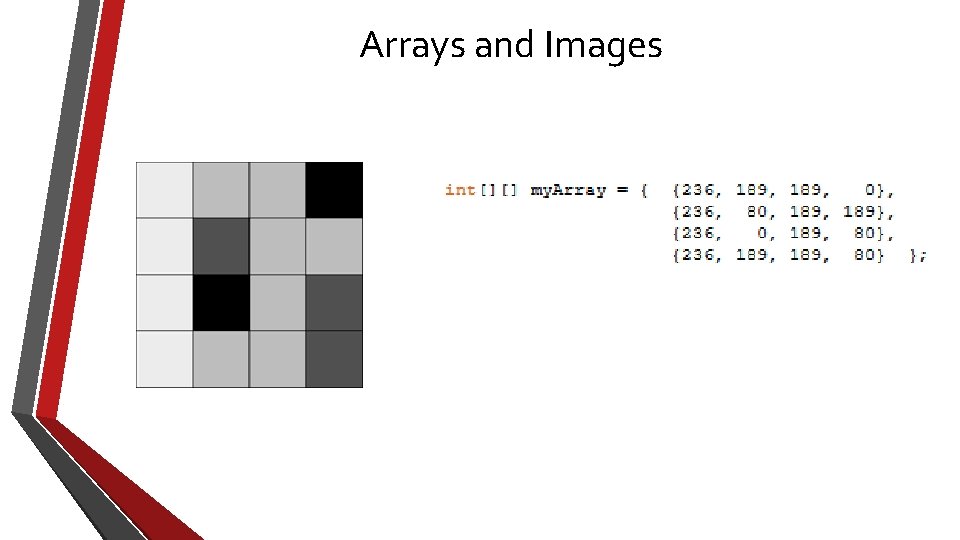
Arrays and Images
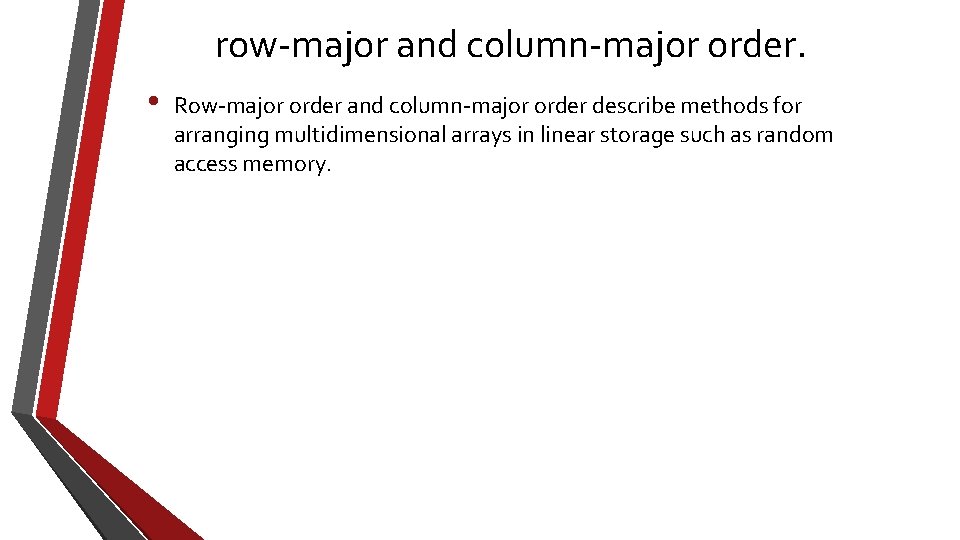
row-major and column-major order. • Row-major order and column-major order describe methods for arranging multidimensional arrays in linear storage such as random access memory.
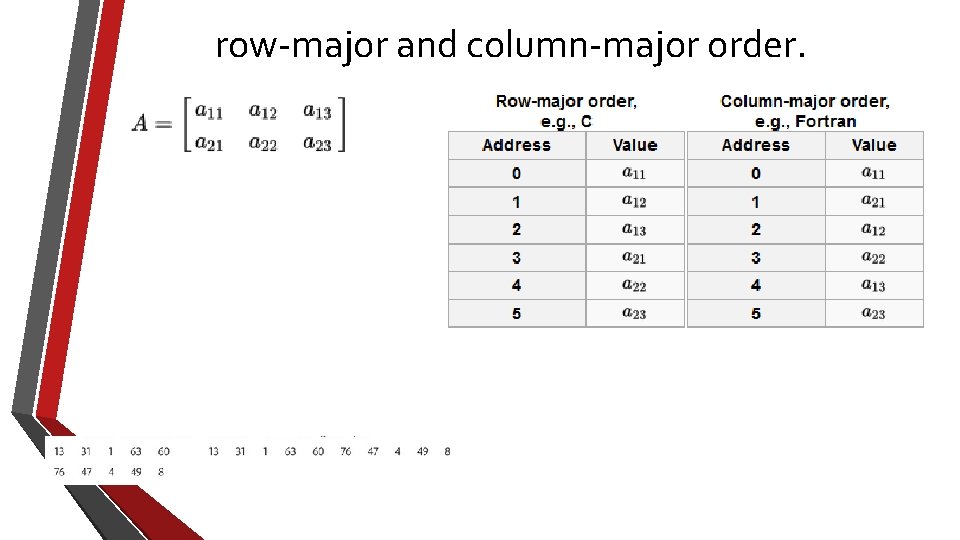
row-major and column-major order.
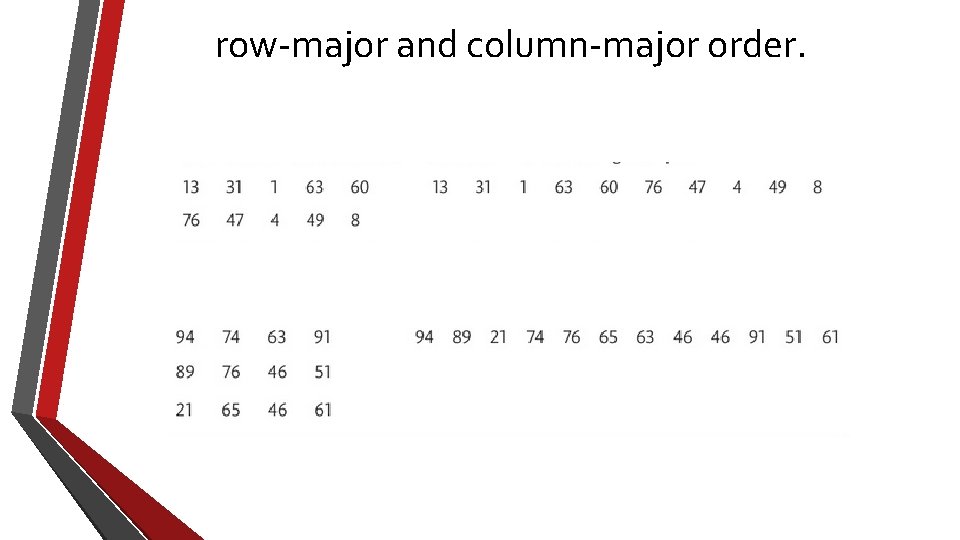
row-major and column-major order.
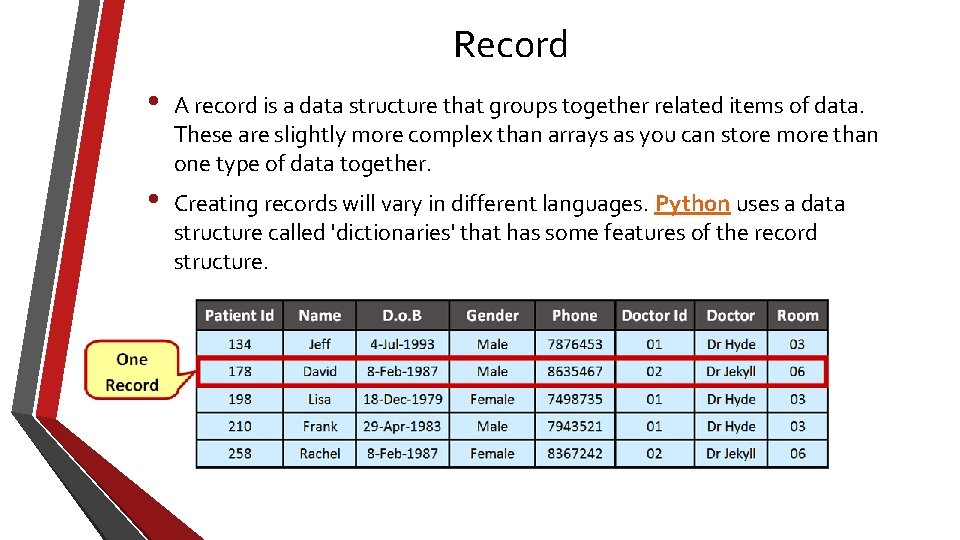
Record • A record is a data structure that groups together related items of data. These are slightly more complex than arrays as you can store more than one type of data together. • Creating records will vary in different languages. Python uses a data structure called 'dictionaries' that has some features of the record structure.
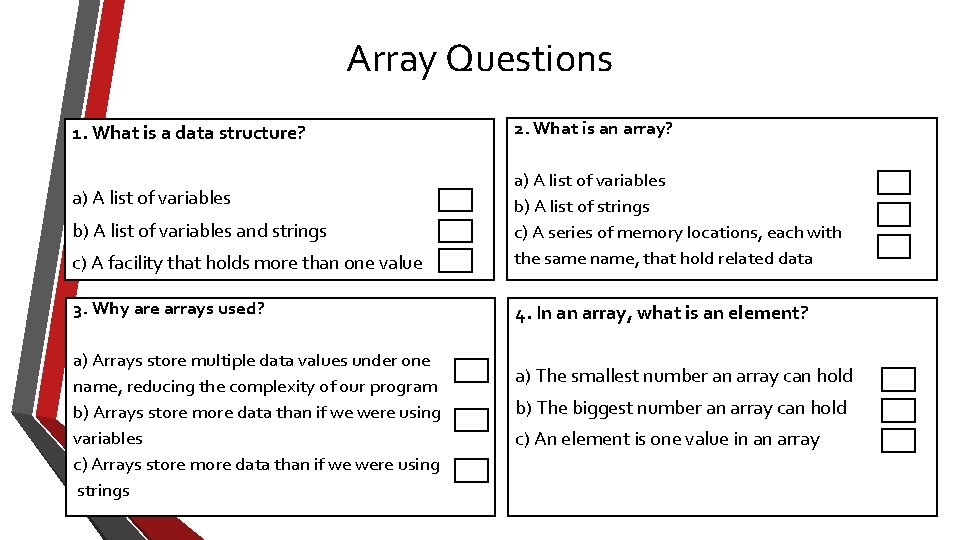
Array Questions 1. What is a data structure? a) A list of variables b) A list of variables and strings c) A facility that holds more than one value 3. Why are arrays used? a) Arrays store multiple data values under one name, reducing the complexity of our program b) Arrays store more data than if we were using variables c) Arrays store more data than if we were using strings 2. What is an array? a) A list of variables b) A list of strings c) A series of memory locations, each with the same name, that hold related data 4. In an array, what is an element? a) The smallest number an array can hold b) The biggest number an array can hold c) An element is one value in an array
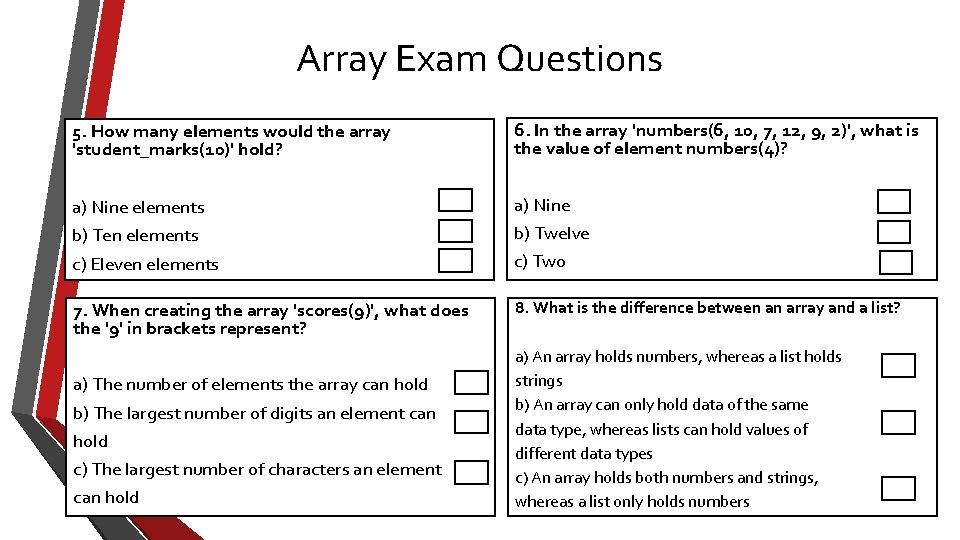
Array Exam Questions 5. How many elements would the array 'student_marks(10)' hold? 6. In the array 'numbers(6, 10, 7, 12, 9, 2)', what is the value of element numbers(4)? a) Nine elements a) Nine b) Twelve c) Two b) Ten elements c) Eleven elements 7. When creating the array 'scores(9)', what does the '9' in brackets represent? a) The number of elements the array can hold b) The largest number of digits an element can hold c) The largest number of characters an element can hold 8. What is the difference between an array and a list? a) An array holds numbers, whereas a list holds strings b) An array can only hold data of the same data type, whereas lists can hold values of different data types c) An array holds both numbers and strings, whereas a list only holds numbers
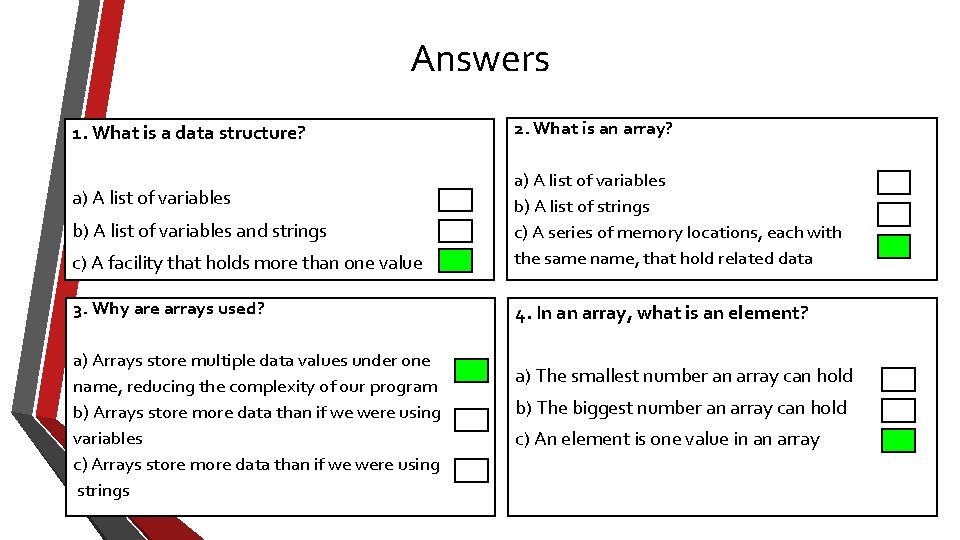
Answers 1. What is a data structure? a) A list of variables b) A list of variables and strings c) A facility that holds more than one value 3. Why are arrays used? a) Arrays store multiple data values under one name, reducing the complexity of our program b) Arrays store more data than if we were using variables c) Arrays store more data than if we were using strings 2. What is an array? a) A list of variables b) A list of strings c) A series of memory locations, each with the same name, that hold related data 4. In an array, what is an element? a) The smallest number an array can hold b) The biggest number an array can hold c) An element is one value in an array
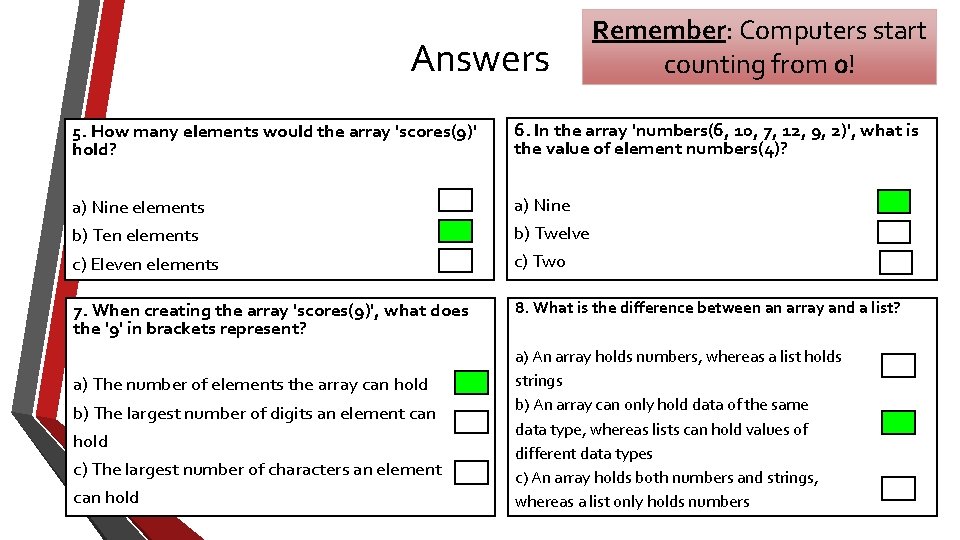
Answers Remember: Computers start counting from 0! 5. How many elements would the array 'scores(9)' hold? 6. In the array 'numbers(6, 10, 7, 12, 9, 2)', what is the value of element numbers(4)? a) Nine elements a) Nine b) Twelve c) Two b) Ten elements c) Eleven elements 7. When creating the array 'scores(9)', what does the '9' in brackets represent? a) The number of elements the array can hold b) The largest number of digits an element can hold c) The largest number of characters an element can hold 8. What is the difference between an array and a list? a) An array holds numbers, whereas a list holds strings b) An array can only hold data of the same data type, whereas lists can hold values of different data types c) An array holds both numbers and strings, whereas a list only holds numbers