CONTROL STATEMENTS LOOPS TYPES OF CONTROL STRUCTURES WHY
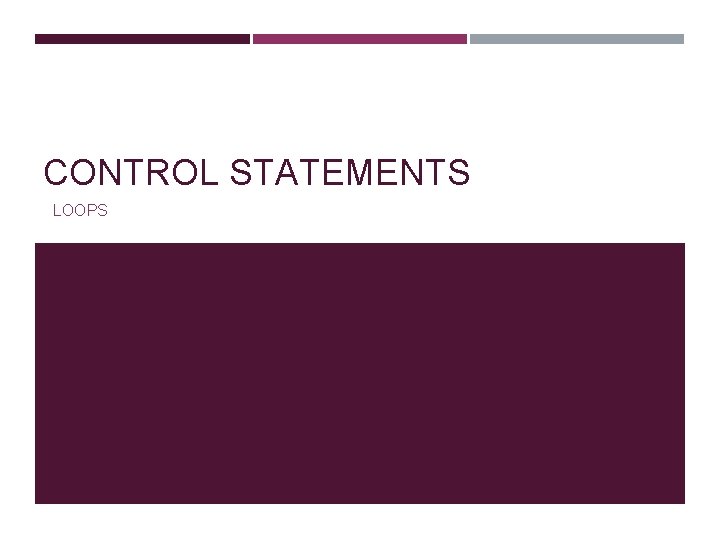
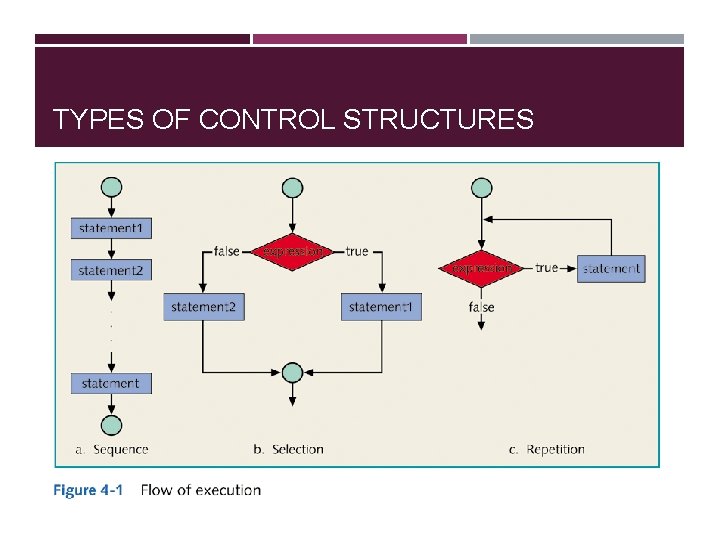
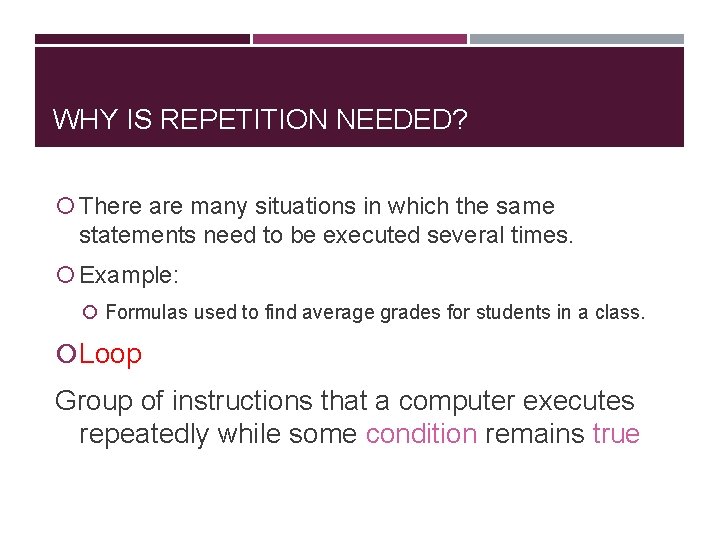
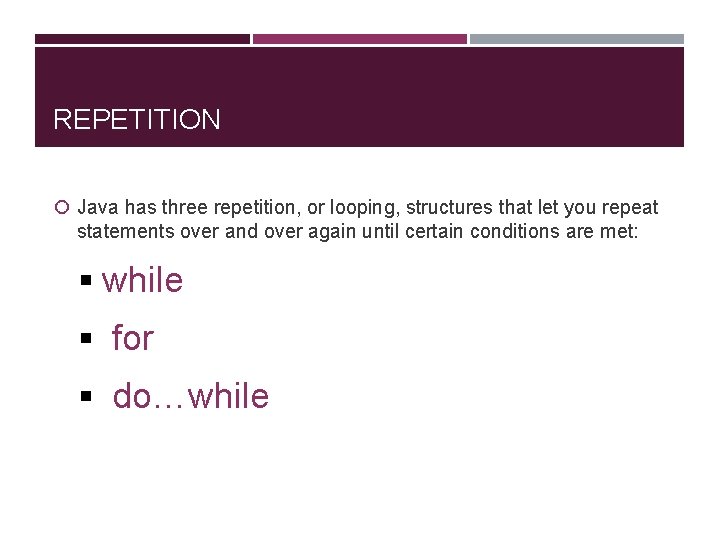
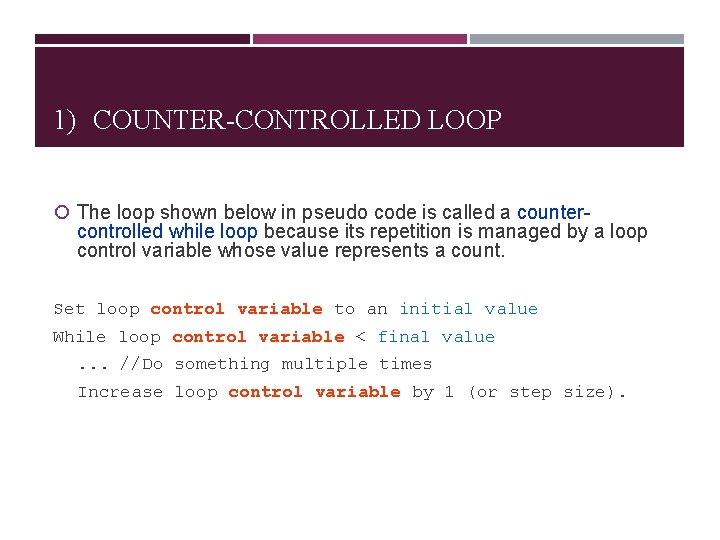
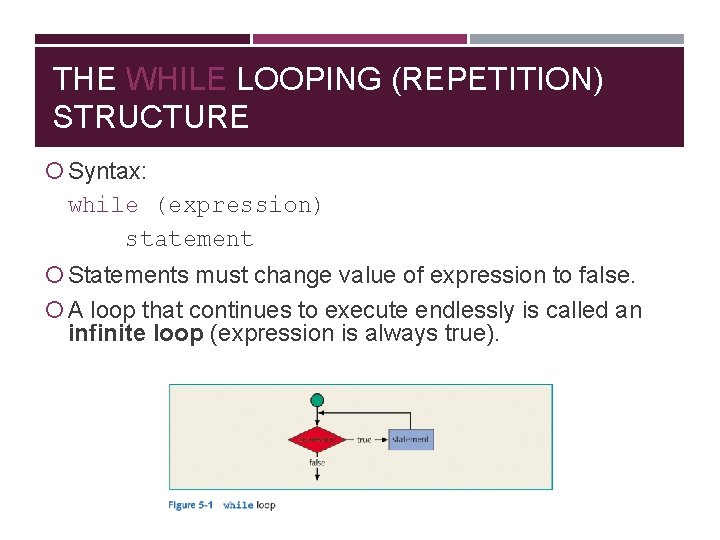
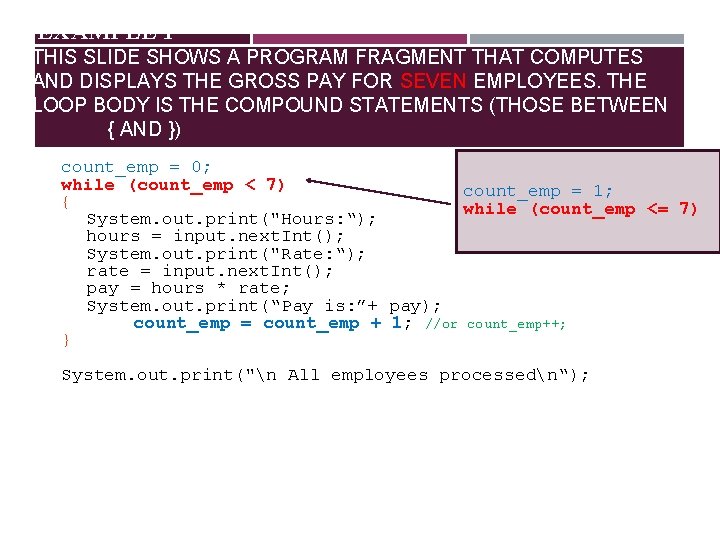
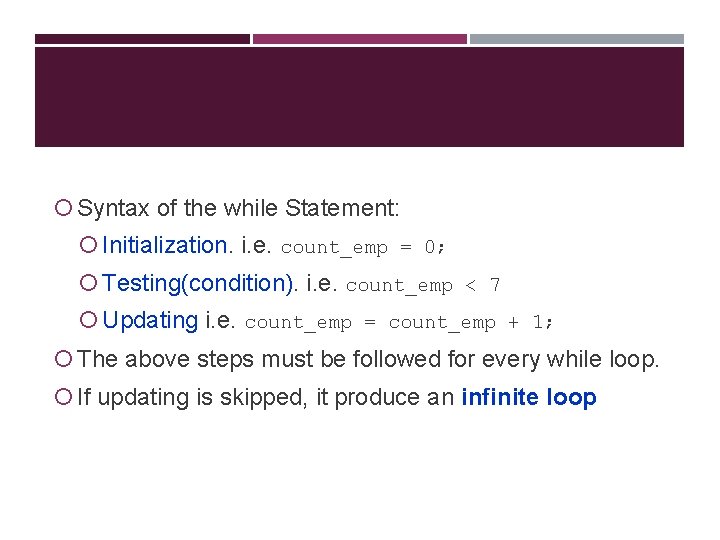
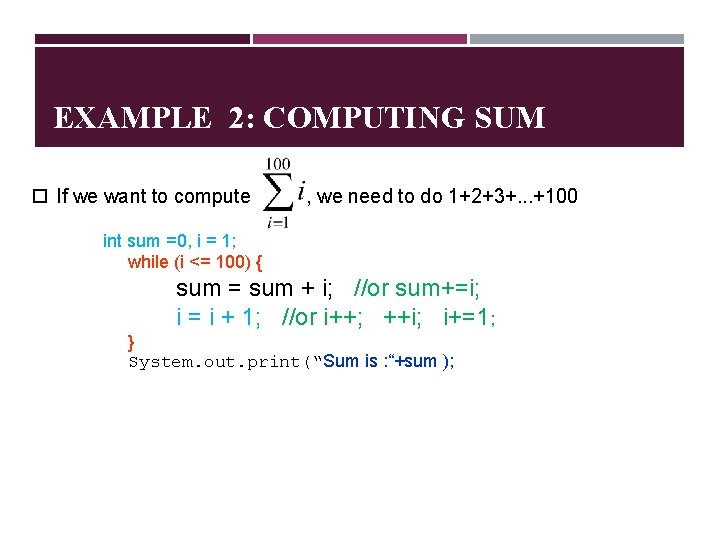
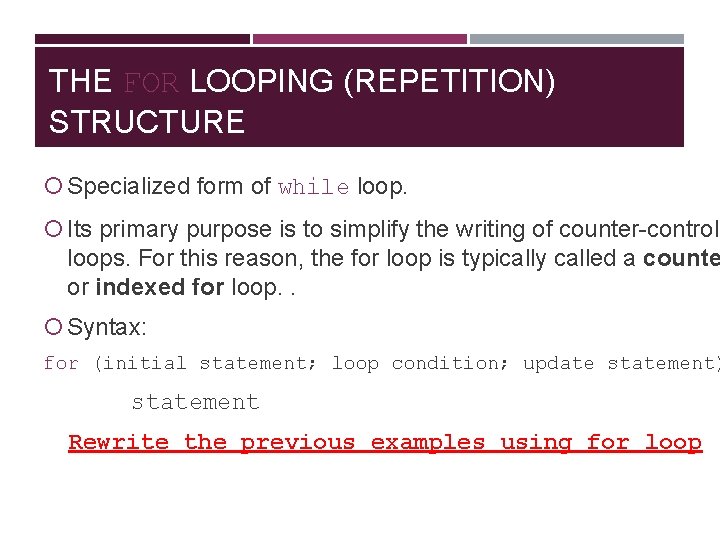
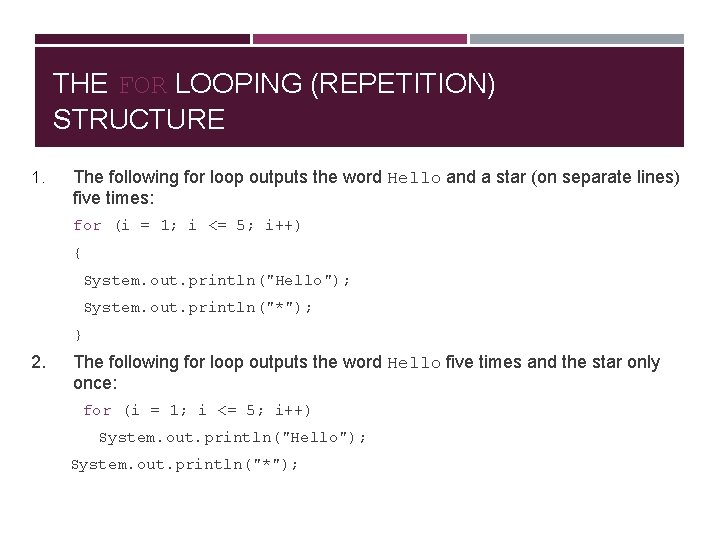
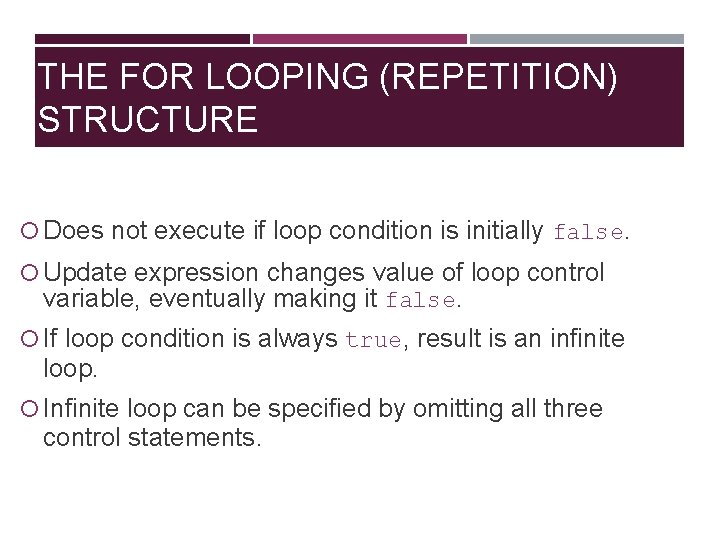
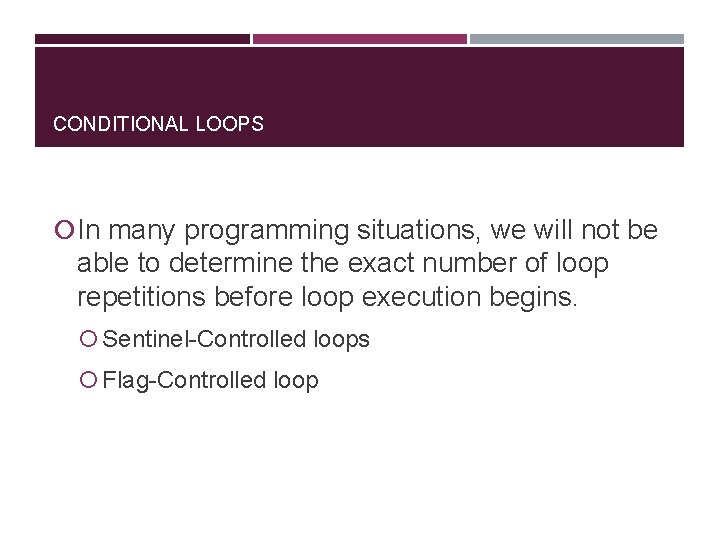
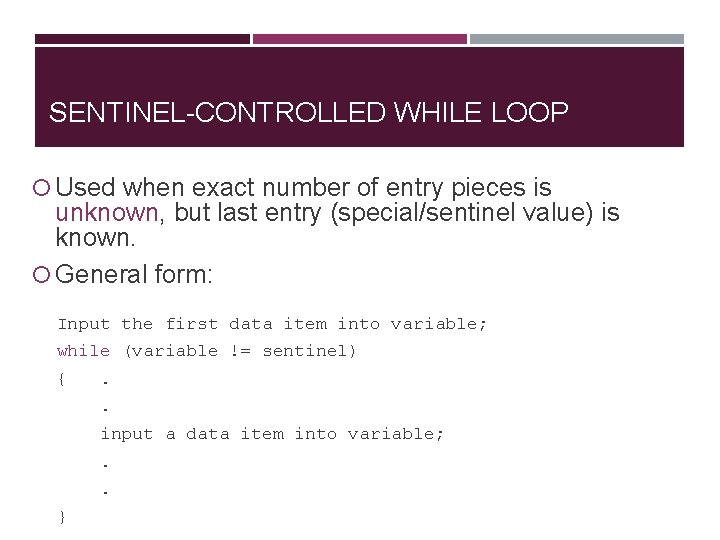
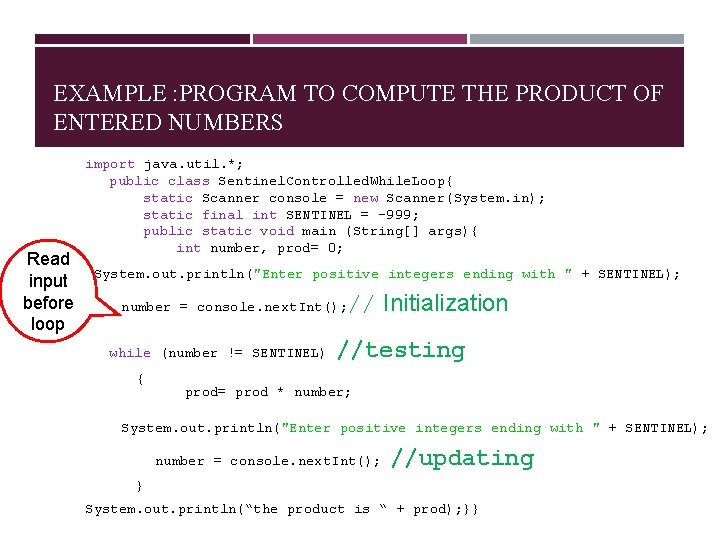
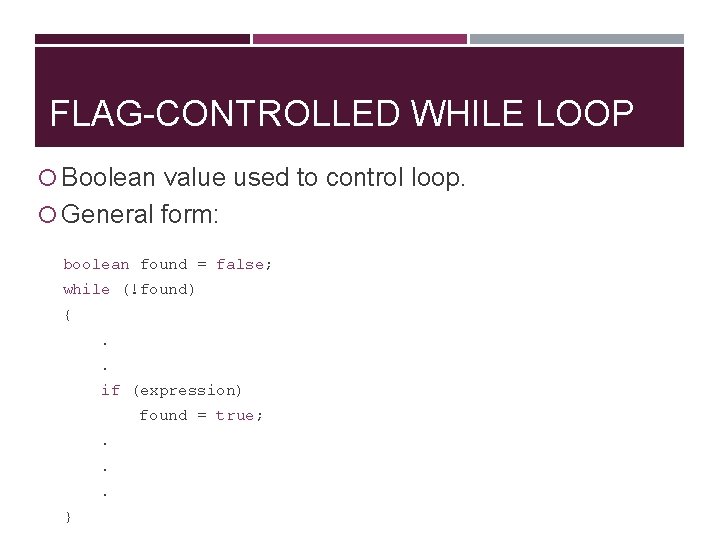
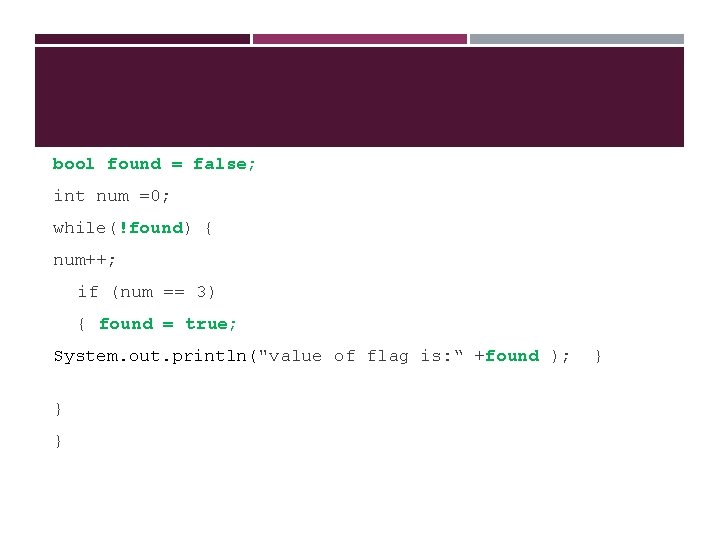
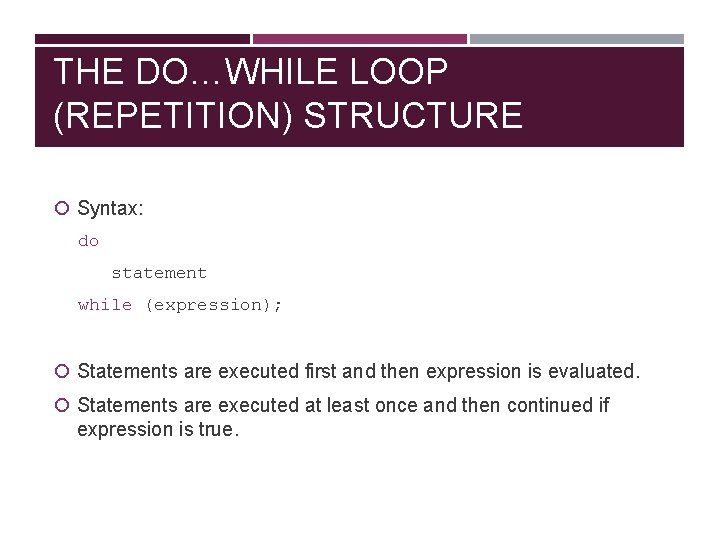
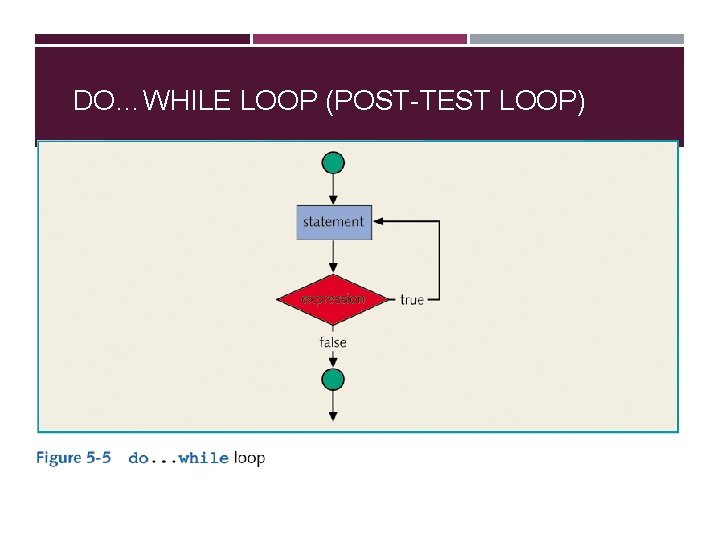
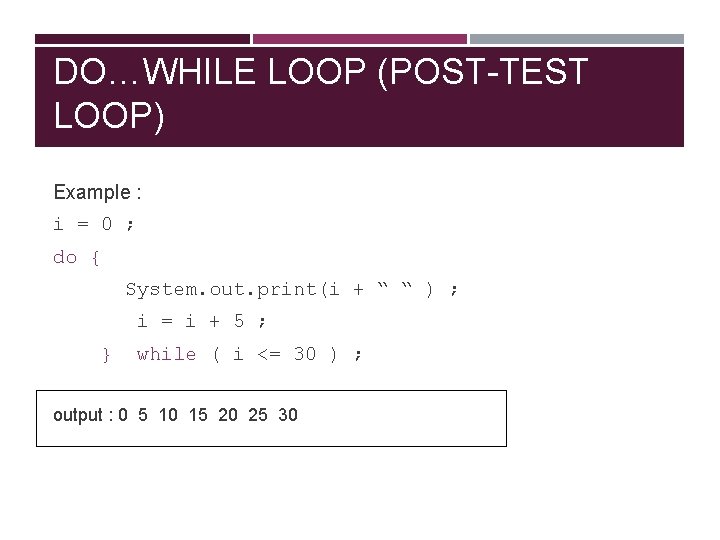
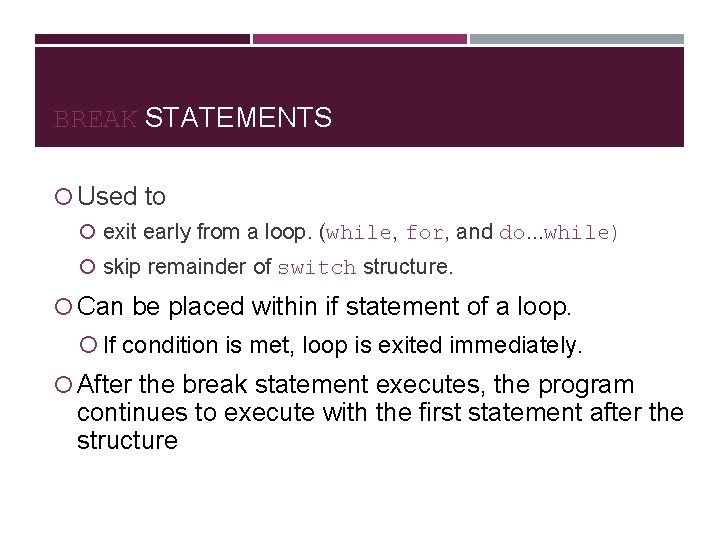
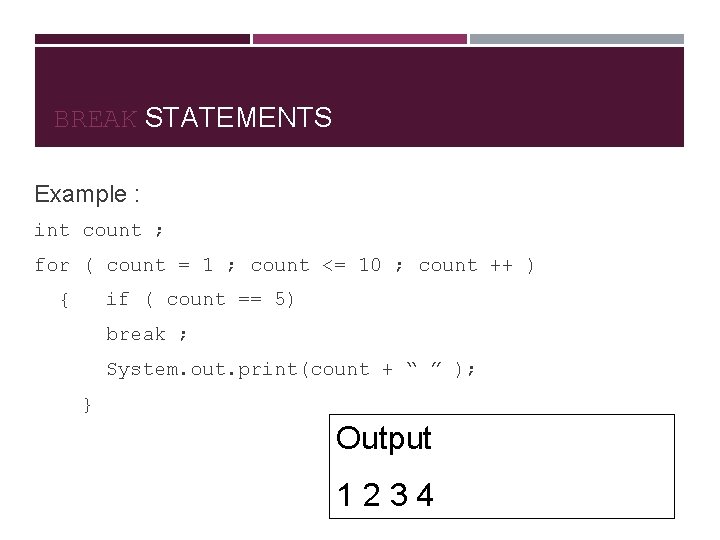
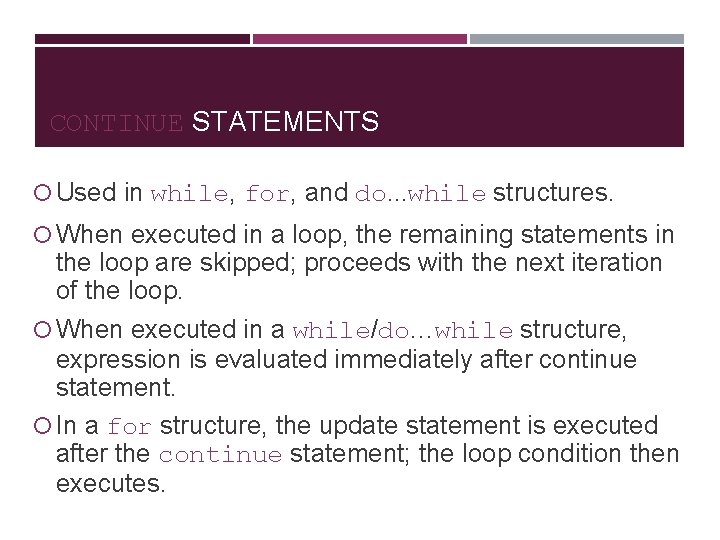
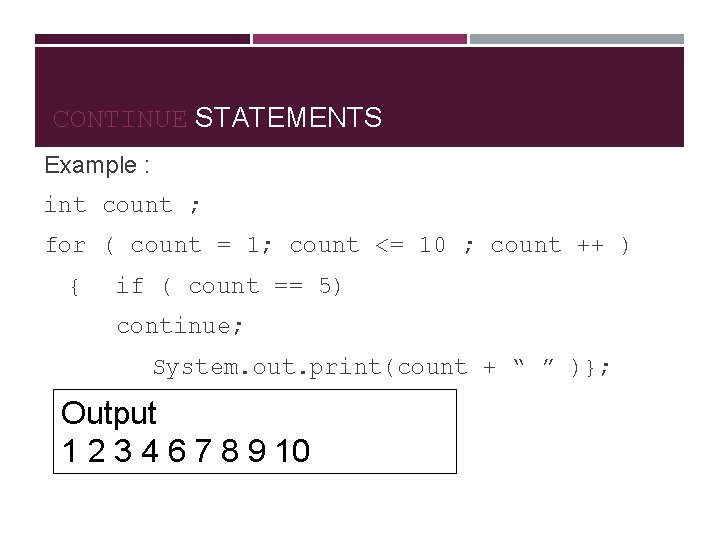
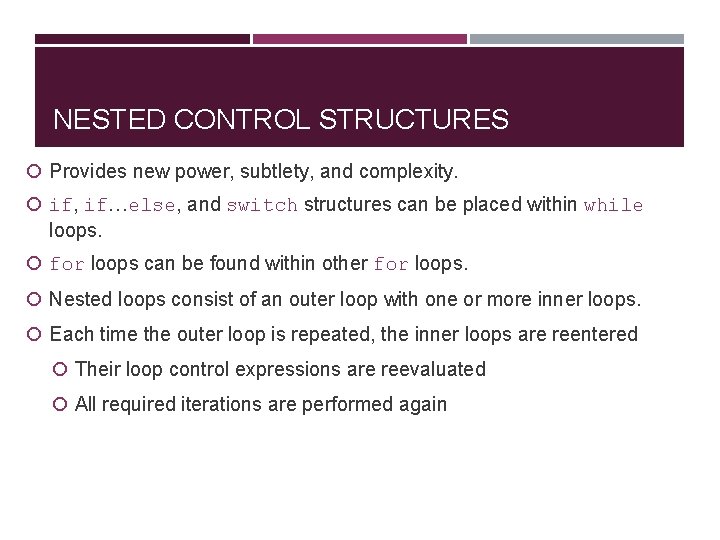
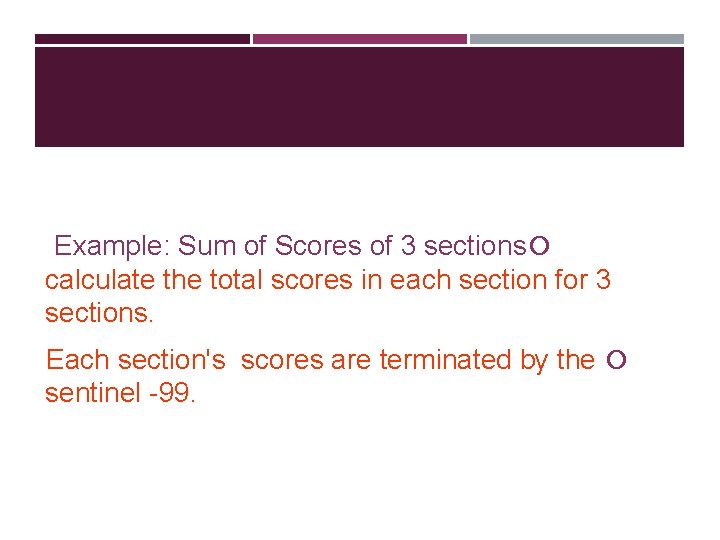
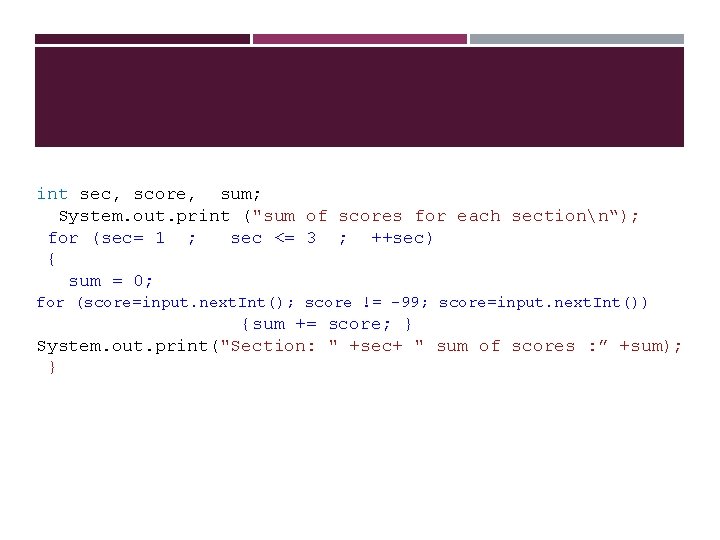
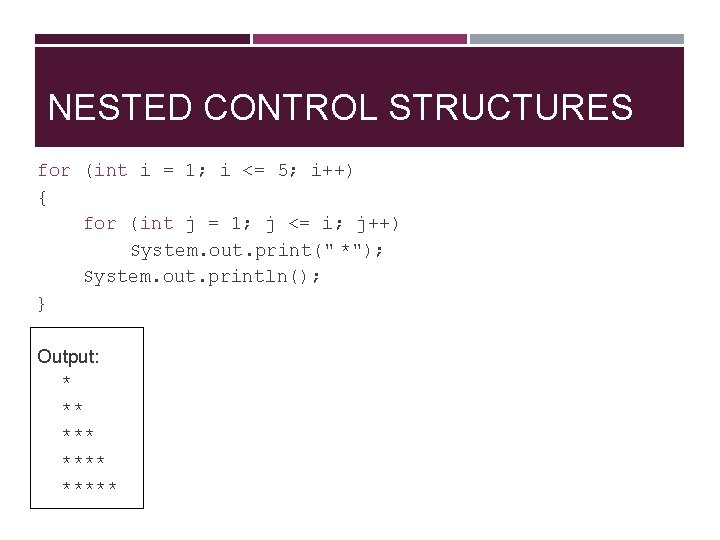
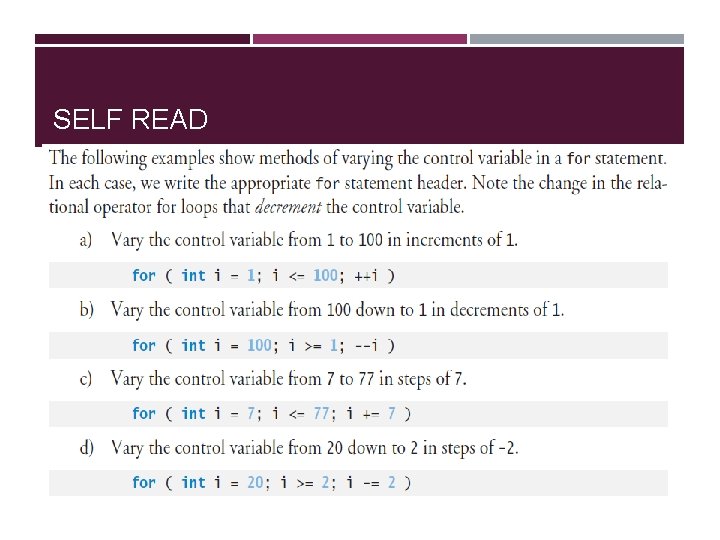
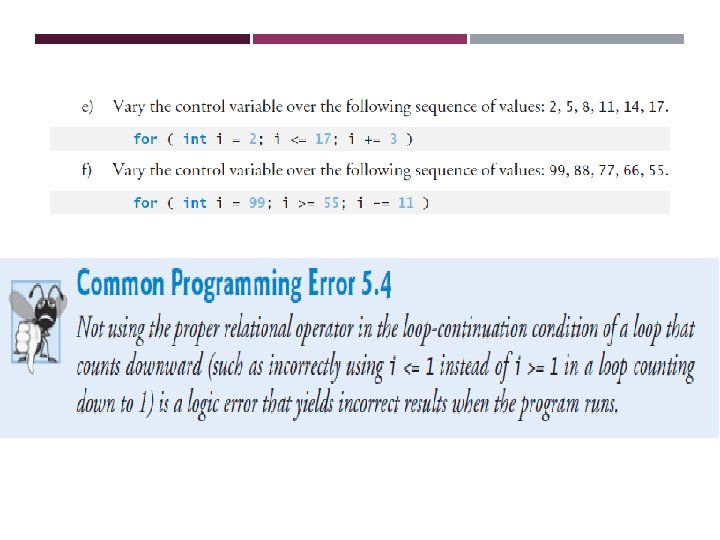
- Slides: 30
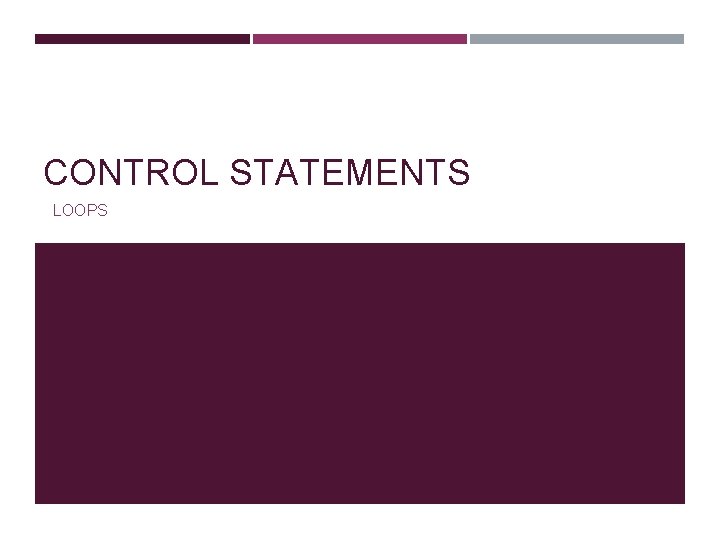
CONTROL STATEMENTS LOOPS
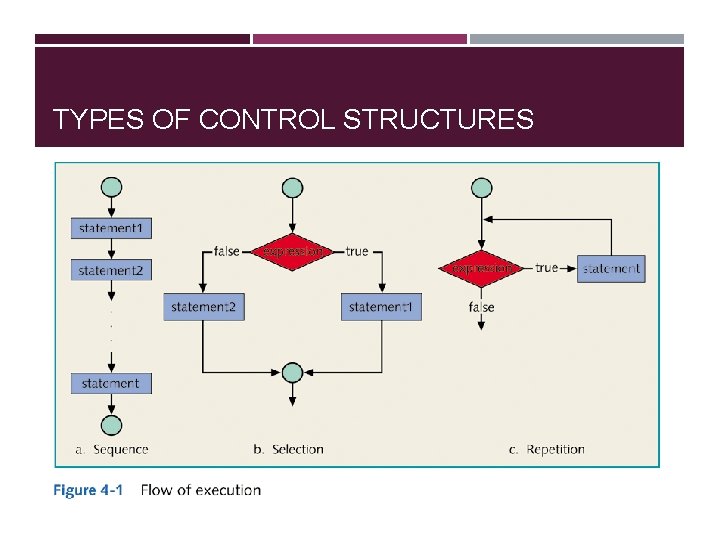
TYPES OF CONTROL STRUCTURES
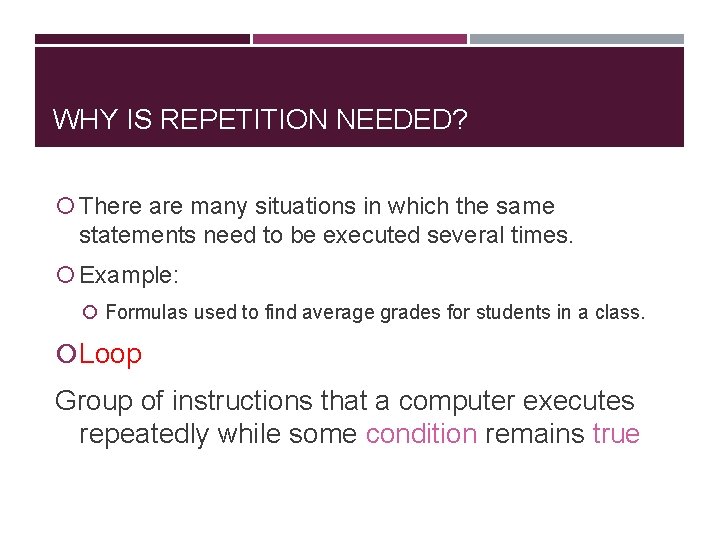
WHY IS REPETITION NEEDED? There are many situations in which the same statements need to be executed several times. Example: Formulas used to find average grades for students in a class. Loop Group of instructions that a computer executes repeatedly while some condition remains true
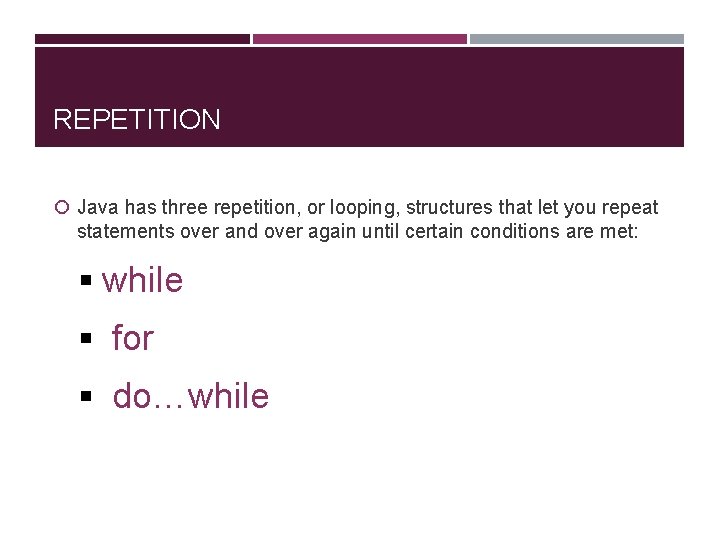
REPETITION Java has three repetition, or looping, structures that let you repeat statements over and over again until certain conditions are met: § while § for § do…while
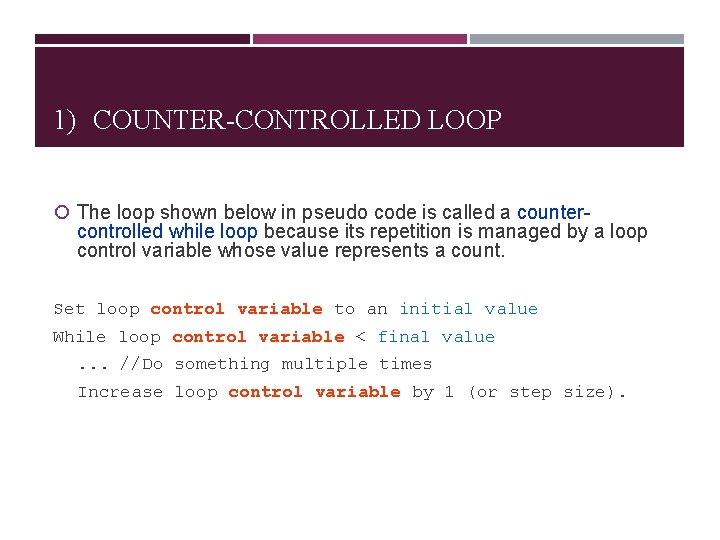
1) COUNTER-CONTROLLED LOOP The loop shown below in pseudo code is called a counter- controlled while loop because its repetition is managed by a loop control variable whose value represents a count. Set loop control variable to an initial value While loop control variable < final value. . . //Do something multiple times Increase loop control variable by 1 (or step size).
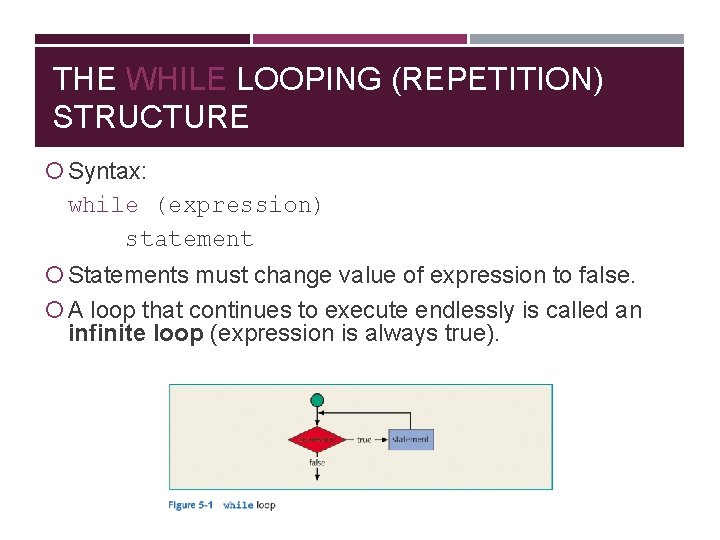
THE WHILE LOOPING (REPETITION) STRUCTURE Syntax: while (expression) statement Statements must change value of expression to false. A loop that continues to execute endlessly is called an infinite loop (expression is always true).
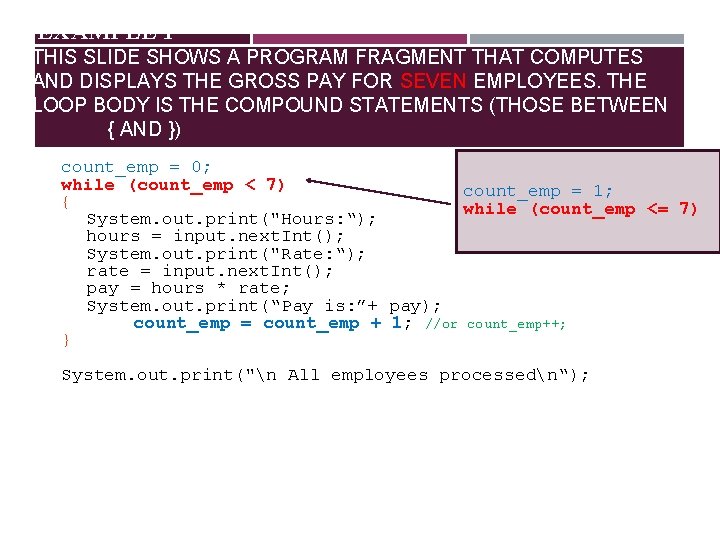
EXAMPLE 1 THIS SLIDE SHOWS A PROGRAM FRAGMENT THAT COMPUTES AND DISPLAYS THE GROSS PAY FOR SEVEN EMPLOYEES. THE LOOP BODY IS THE COMPOUND STATEMENTS (THOSE BETWEEN { AND }) count_emp = 0; while (count_emp < 7) count_emp = 1; { while (count_emp <= 7) System. out. print("Hours: “); hours = input. next. Int(); System. out. print("Rate: “); rate = input. next. Int(); pay = hours * rate; System. out. print(“Pay is: ”+ pay); count_emp = count_emp + 1; //or count_emp++; } System. out. print("n All employees processedn“);
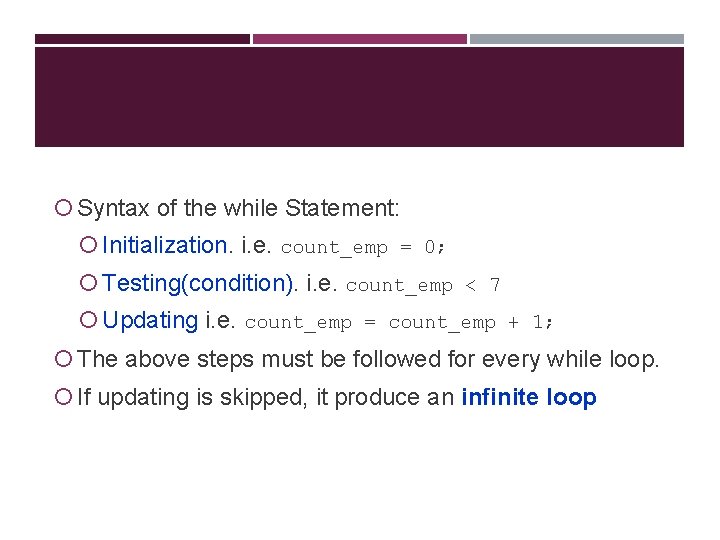
Syntax of the while Statement: Initialization. i. e. count_emp = 0; Testing(condition). i. e. count_emp < 7 Updating i. e. count_emp = count_emp + 1; The above steps must be followed for every while loop. If updating is skipped, it produce an infinite loop
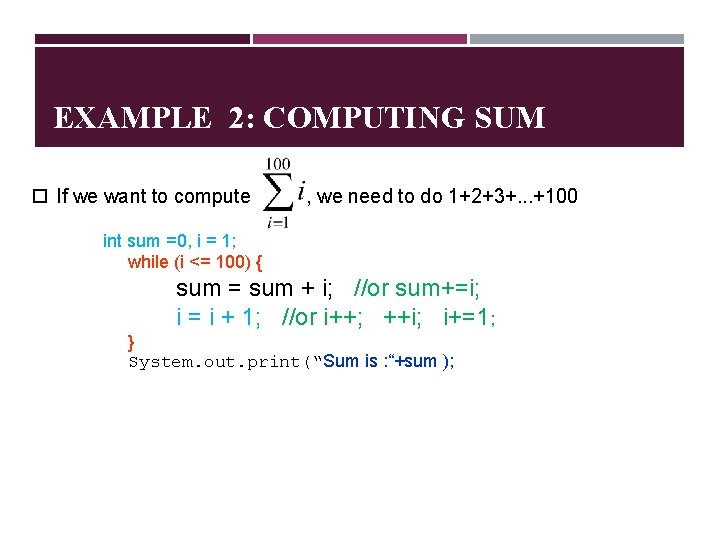
EXAMPLE 2: COMPUTING SUM If we want to compute , we need to do 1+2+3+. . . +100 int sum =0, i = 1; while (i <= 100) { sum = sum + i; //or sum+=i; i = i + 1; //or i++; ++i; i+=1; } System. out. print(“Sum is : “+sum );
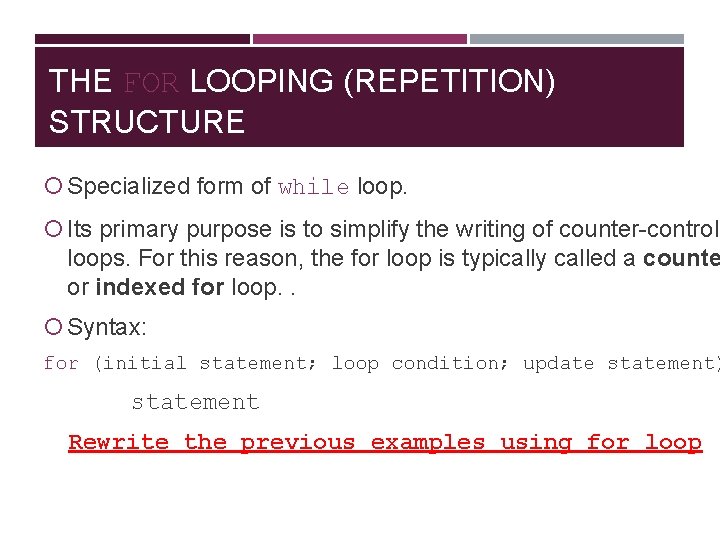
THE FOR LOOPING (REPETITION) STRUCTURE Specialized form of while loop. Its primary purpose is to simplify the writing of counter-controll loops. For this reason, the for loop is typically called a counte or indexed for loop. . Syntax: for (initial statement; loop condition; update statement) statement Rewrite the previous examples using for loop
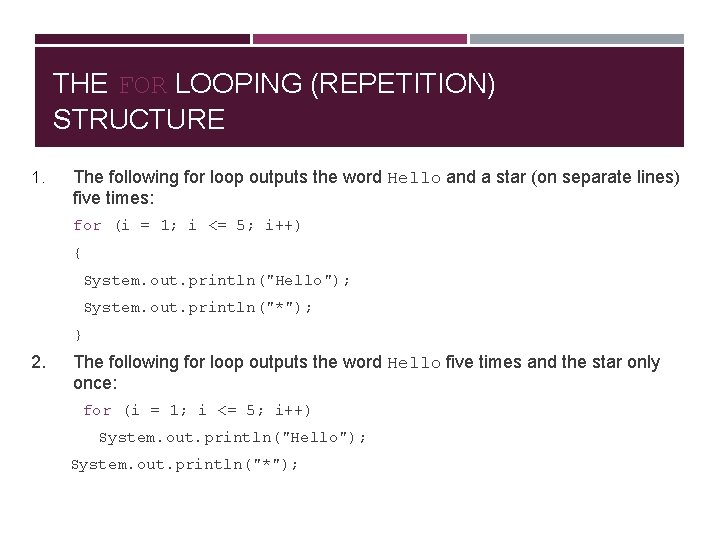
THE FOR LOOPING (REPETITION) STRUCTURE 1. The following for loop outputs the word Hello and a star (on separate lines) five times: for (i = 1; i <= 5; i++) { System. out. println("Hello"); System. out. println("*"); } 2. The following for loop outputs the word Hello five times and the star only once: for (i = 1; i <= 5; i++) System. out. println("Hello"); System. out. println("*");
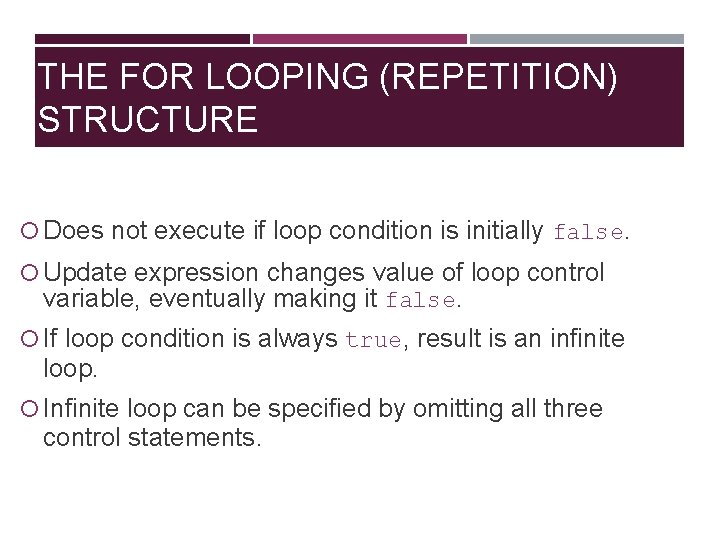
THE FOR LOOPING (REPETITION) STRUCTURE Does not execute if loop condition is initially false. Update expression changes value of loop control variable, eventually making it false. If loop condition is always true, result is an infinite loop. Infinite loop can be specified by omitting all three control statements.
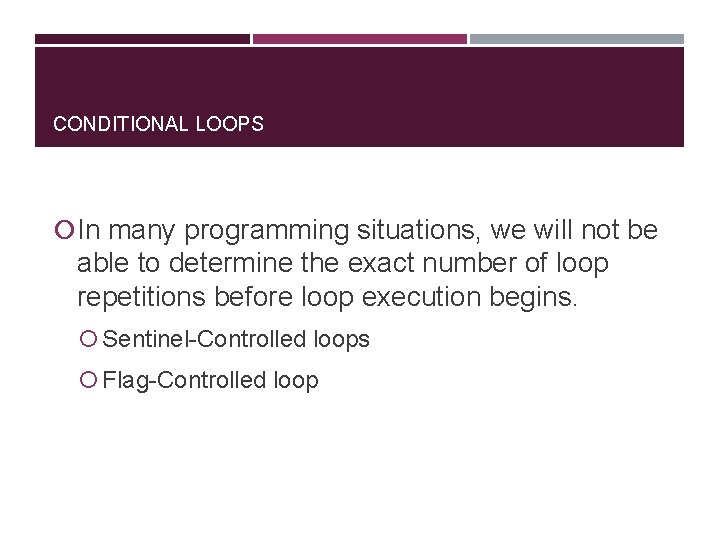
CONDITIONAL LOOPS In many programming situations, we will not be able to determine the exact number of loop repetitions before loop execution begins. Sentinel-Controlled loops Flag-Controlled loop
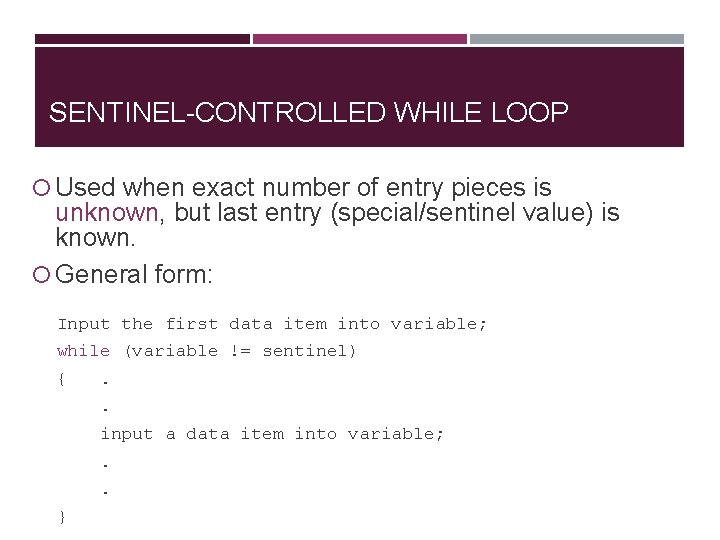
SENTINEL-CONTROLLED WHILE LOOP Used when exact number of entry pieces is unknown, but last entry (special/sentinel value) is known. General form: Input the first data item into variable; while (variable != sentinel) { . . input a data item into variable; . . }
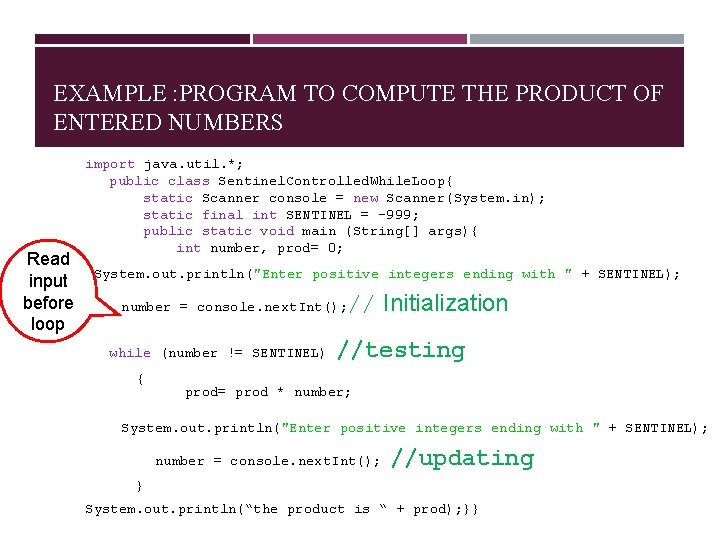
EXAMPLE : PROGRAM TO COMPUTE THE PRODUCT OF ENTERED NUMBERS Read input before loop import java. util. *; public class Sentinel. Controlled. While. Loop{ static Scanner console = new Scanner(System. in); static final int SENTINEL = -999; public static void main (String[] args){ int number, prod= 0; SSystem. out. println("Enter positive integers ending with " + SENTINEL); number = console. next. Int(); while (number != SENTINEL) // Initialization //testing { prod= prod * number; System. out. println("Enter positive integers ending with " + SENTINEL); number = console. next. Int(); //updating } System. out. println(“the product is “ + prod); }}
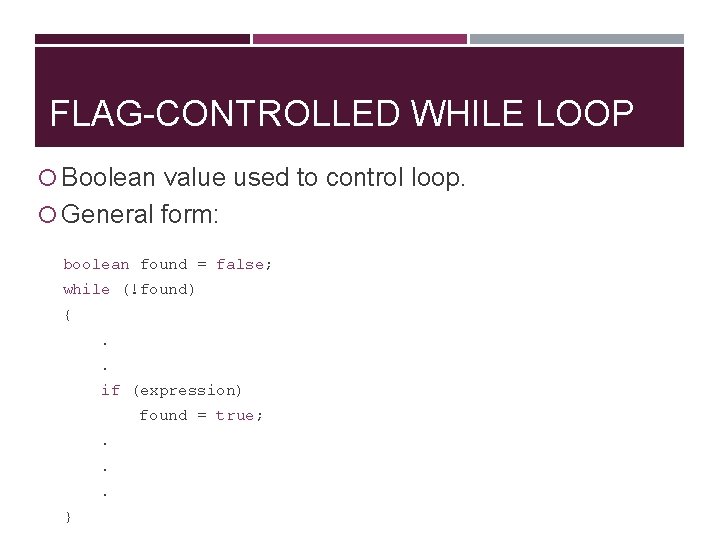
FLAG-CONTROLLED WHILE LOOP Boolean value used to control loop. General form: boolean found = false; while (!found) { . . if (expression) found = true; . . . }
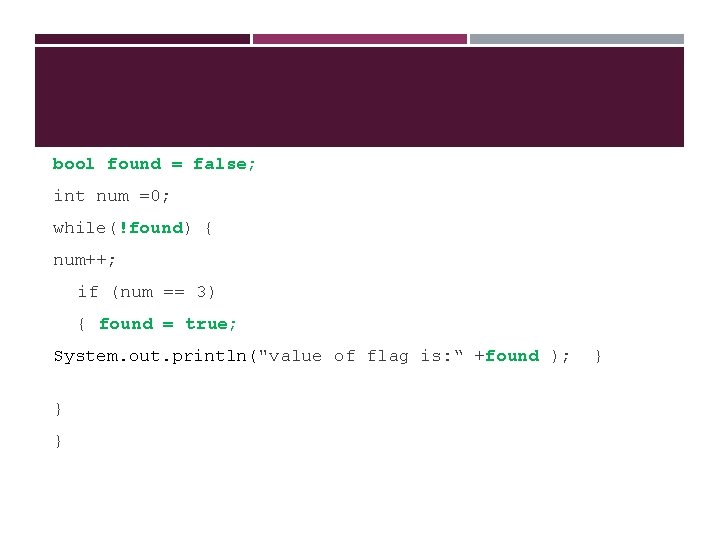
bool found = false; int num =0; while(!found) { num++; if (num == 3) { found = true; System. out. println("value of flag is: “ +found ); } } }
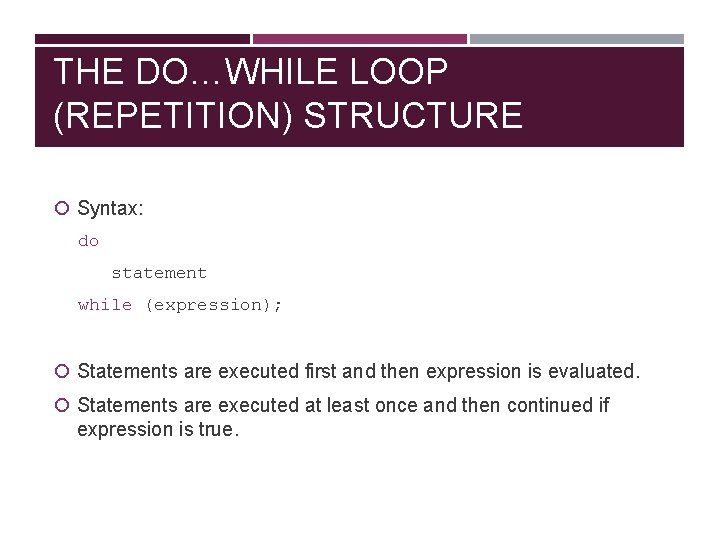
THE DO…WHILE LOOP (REPETITION) STRUCTURE Syntax: do statement while (expression); Statements are executed first and then expression is evaluated. Statements are executed at least once and then continued if expression is true.
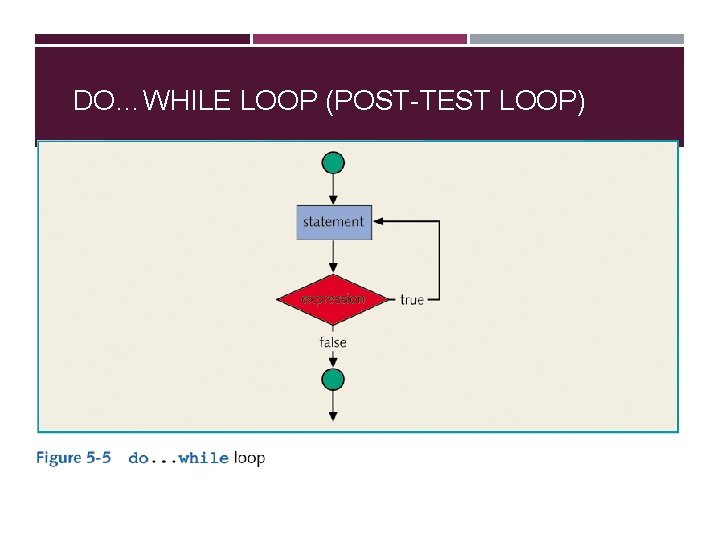
DO…WHILE LOOP (POST-TEST LOOP)
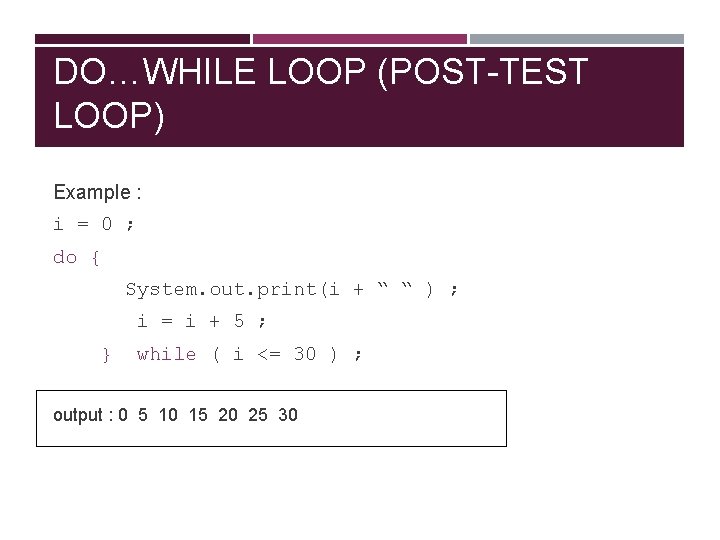
DO…WHILE LOOP (POST-TEST LOOP) Example : i = 0 ; do { System. out. print(i + “ “ ) ; i = i + 5 ; } while ( i <= 30 ) ; output : 0 5 10 15 20 25 30
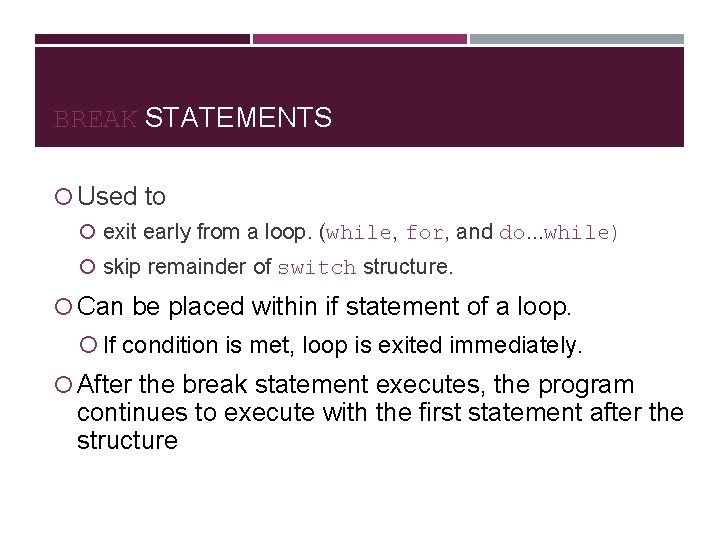
BREAK STATEMENTS Used to exit early from a loop. (while, for, and do. . . while) skip remainder of switch structure. Can be placed within if statement of a loop. If condition is met, loop is exited immediately. After the break statement executes, the program continues to execute with the first statement after the structure
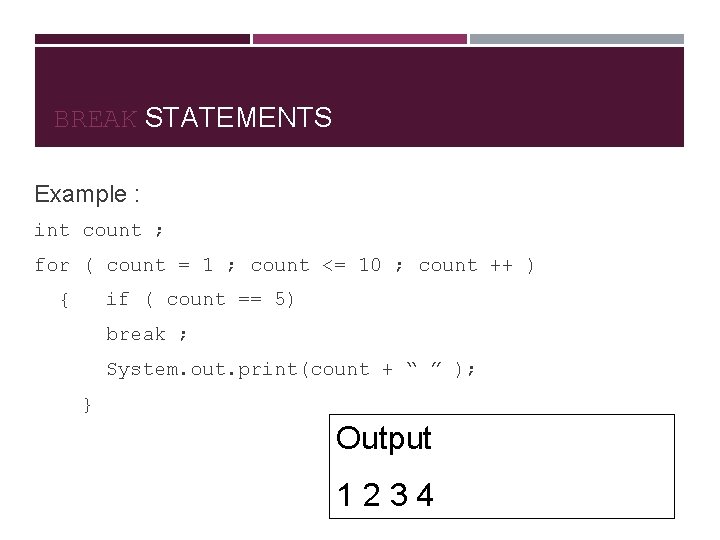
BREAK STATEMENTS Example : int count ; for ( count = 1 ; count <= 10 ; count ++ ) { if ( count == 5) break ; System. out. print(count + “ ” ); } Output 1234
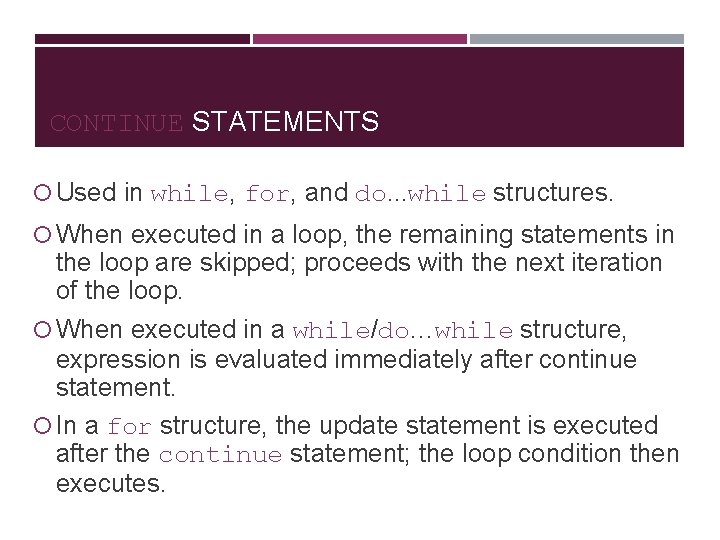
CONTINUE STATEMENTS Used in while, for, and do. . . while structures. When executed in a loop, the remaining statements in the loop are skipped; proceeds with the next iteration of the loop. When executed in a while/do…while structure, expression is evaluated immediately after continue statement. In a for structure, the update statement is executed after the continue statement; the loop condition then executes.
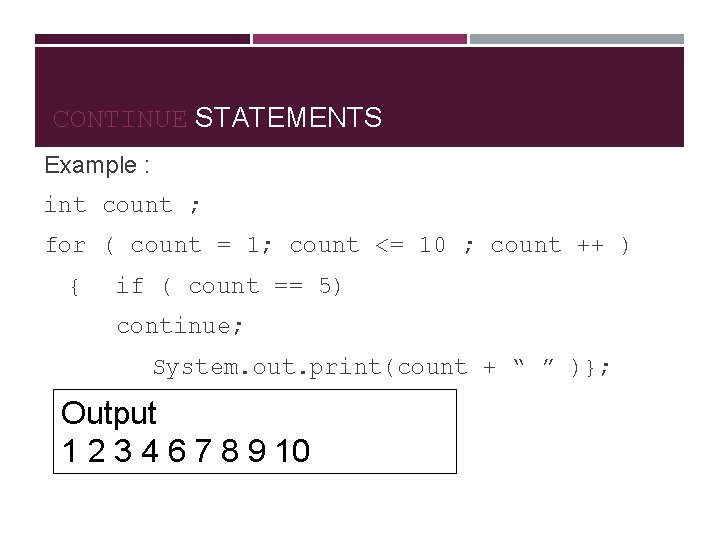
CONTINUE STATEMENTS Example : int count ; for ( count = 1; count <= 10 ; count ++ ) { if ( count == 5) continue; System. out. print(count + “ ” )}; } Output 1 2 3 4 6 7 8 9 10
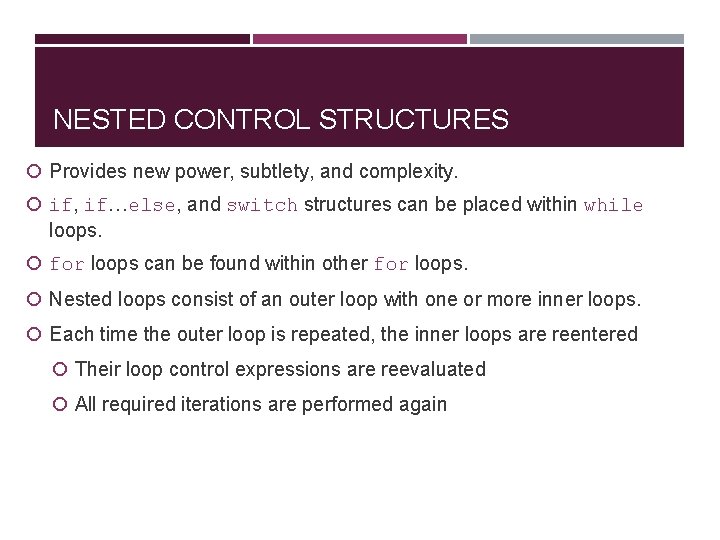
NESTED CONTROL STRUCTURES Provides new power, subtlety, and complexity. if, if…else, and switch structures can be placed within while loops. for loops can be found within other for loops. Nested loops consist of an outer loop with one or more inner loops. Each time the outer loop is repeated, the inner loops are reentered Their loop control expressions are reevaluated All required iterations are performed again
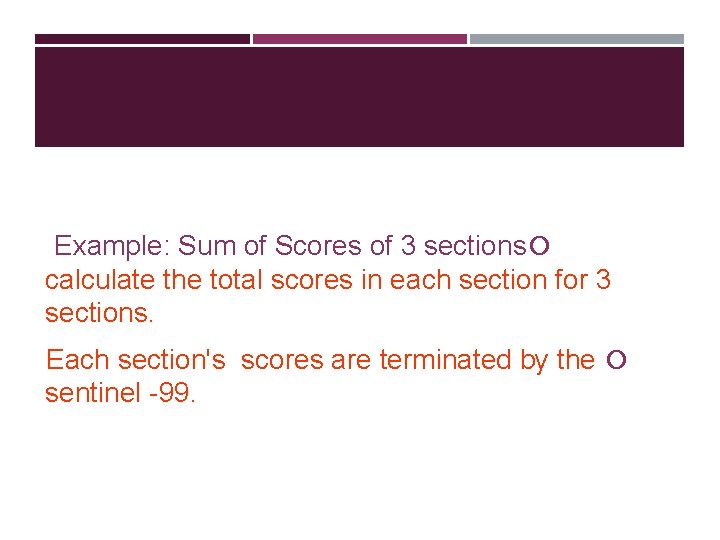
Example: Sum of Scores of 3 sections calculate the total scores in each section for 3 sections. Each section's scores are terminated by the sentinel -99.
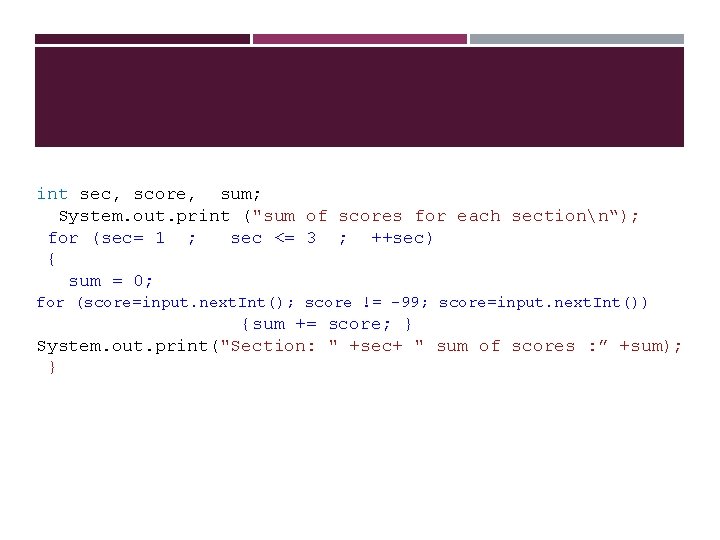
int sec, score, sum; System. out. print ("sum of scores for each sectionn“); for (sec= 1 ; sec <= 3 ; ++sec) { sum = 0; for (score=input. next. Int(); score != -99; score=input. next. Int()) {sum += score; } System. out. print("Section: " +sec+ " sum of scores : ” +sum); }
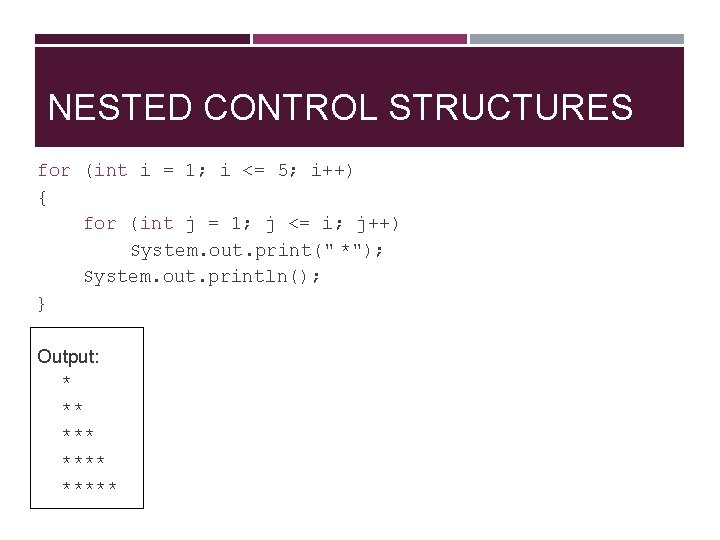
NESTED CONTROL STRUCTURES for (int i = 1; i <= 5; i++) { for (int j = 1; j <= i; j++) System. out. print(" *"); System. out. println(); } Output: * ** *****
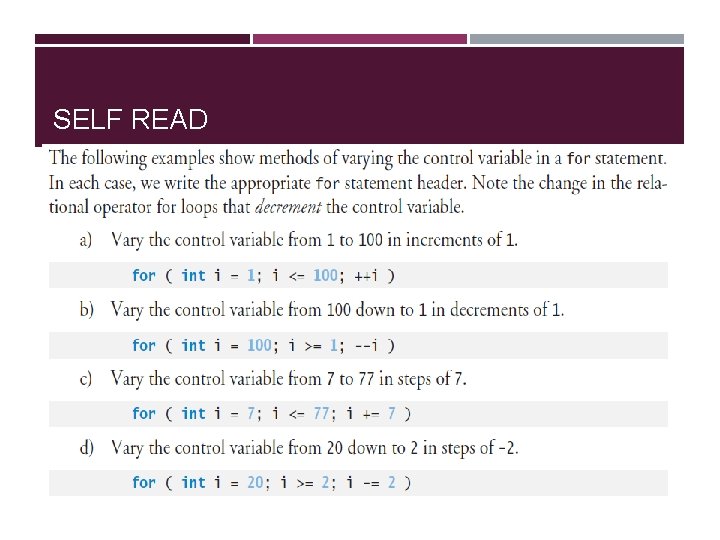
SELF READ
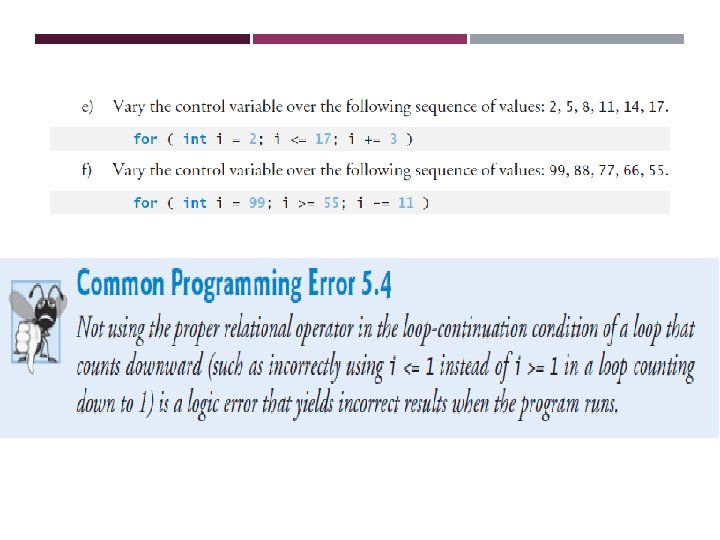