CSE 1321 Module 1 A Programming Primer 6102021
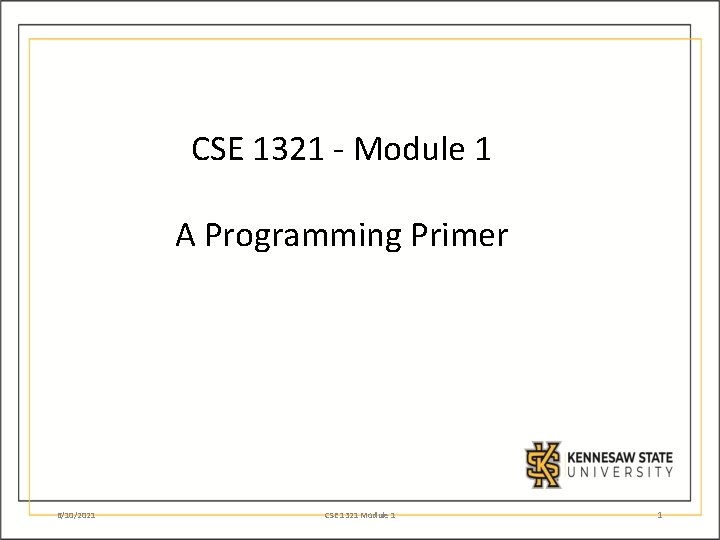
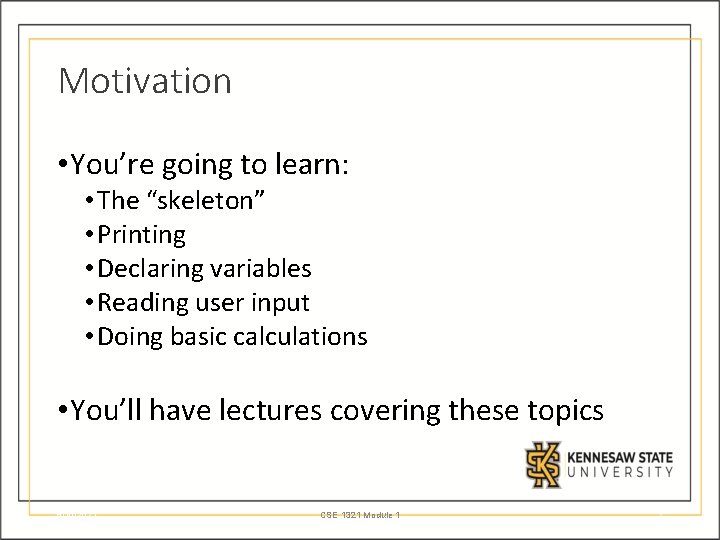
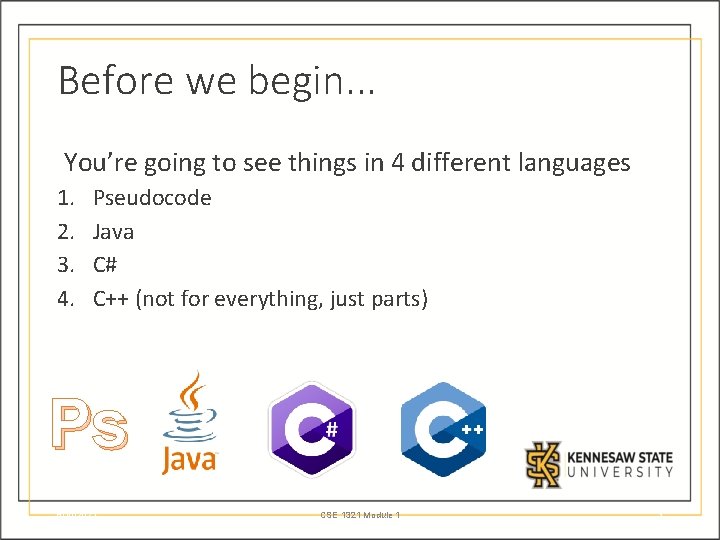
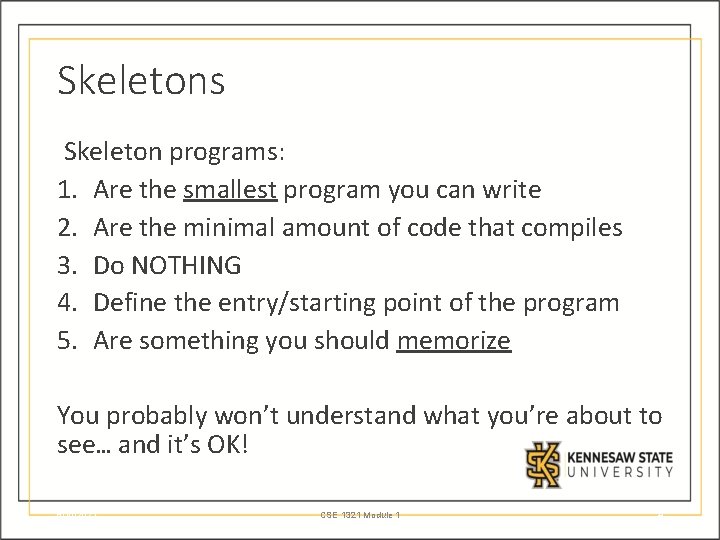
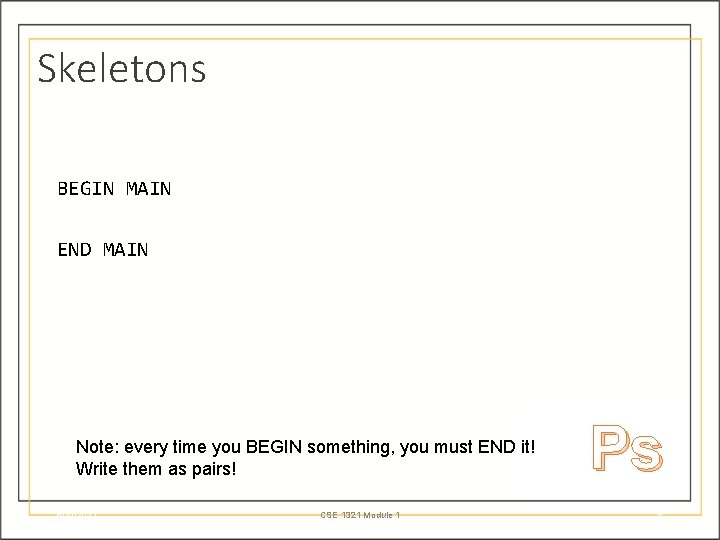
![Skeletons using System; class Main. Class { public static void Main (string[] args) { Skeletons using System; class Main. Class { public static void Main (string[] args) {](https://slidetodoc.com/presentation_image_h2/6113329493bdb08d5c653ed60230da4a/image-6.jpg)
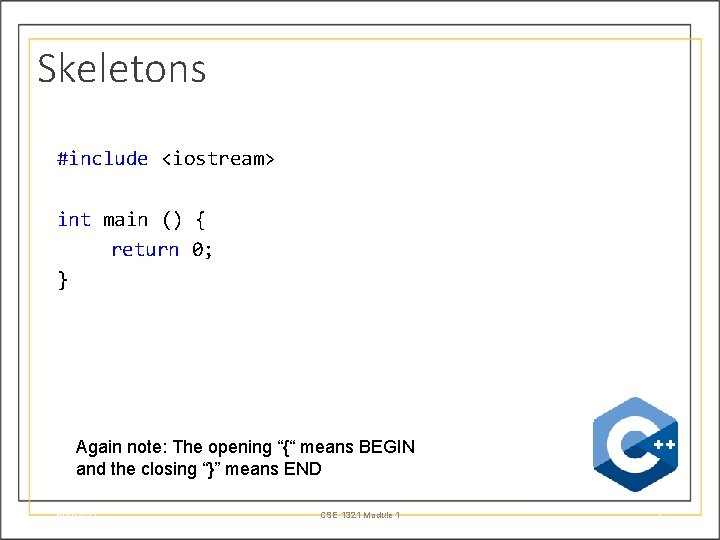
![Skeletons class Main. Class { public static void main (String[] args) { } } Skeletons class Main. Class { public static void main (String[] args) { } }](https://slidetodoc.com/presentation_image_h2/6113329493bdb08d5c653ed60230da4a/image-8.jpg)
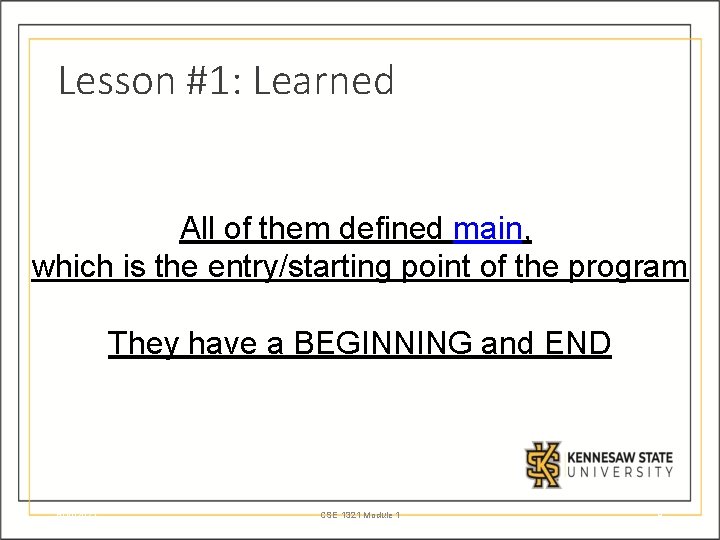
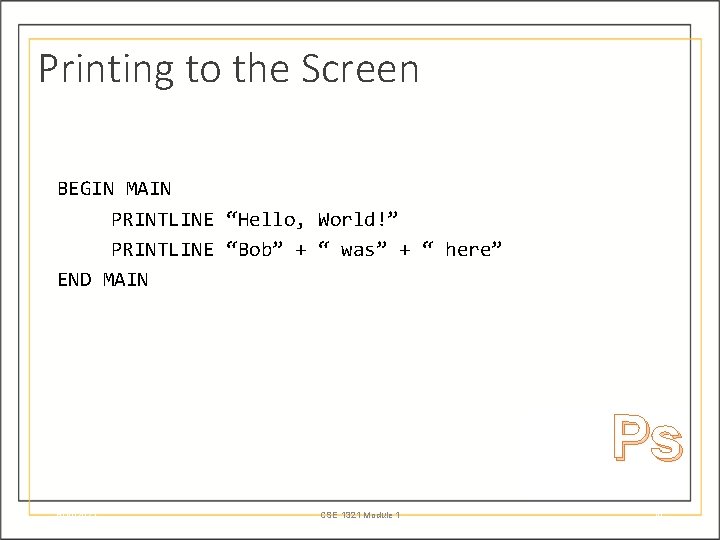
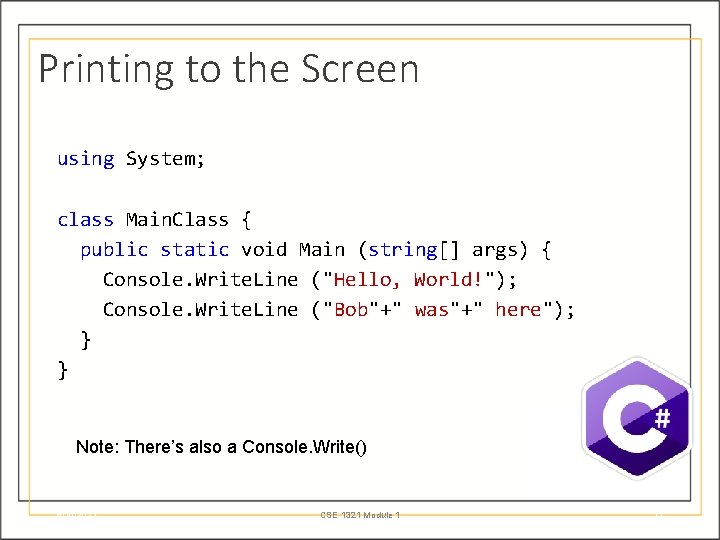
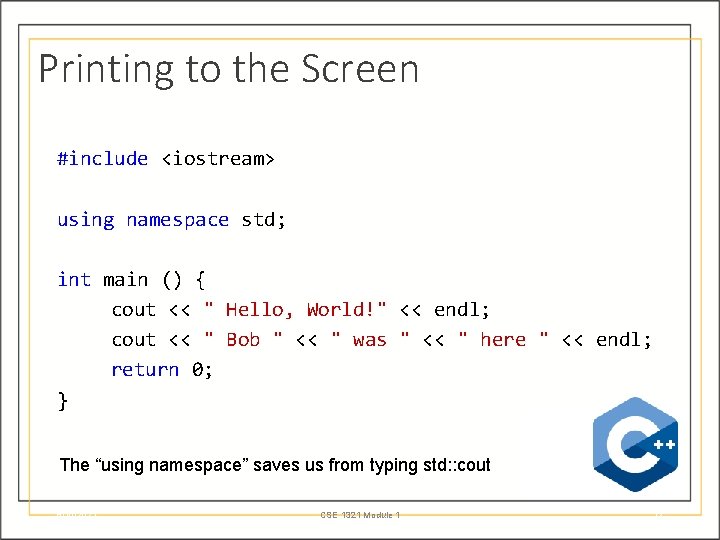
![Printing to the Screen class Main. Class { public static void main (String[] args) Printing to the Screen class Main. Class { public static void main (String[] args)](https://slidetodoc.com/presentation_image_h2/6113329493bdb08d5c653ed60230da4a/image-13.jpg)
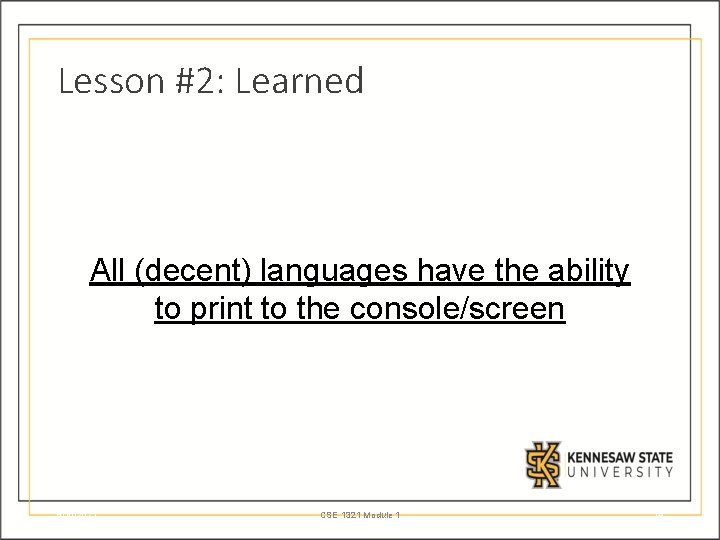
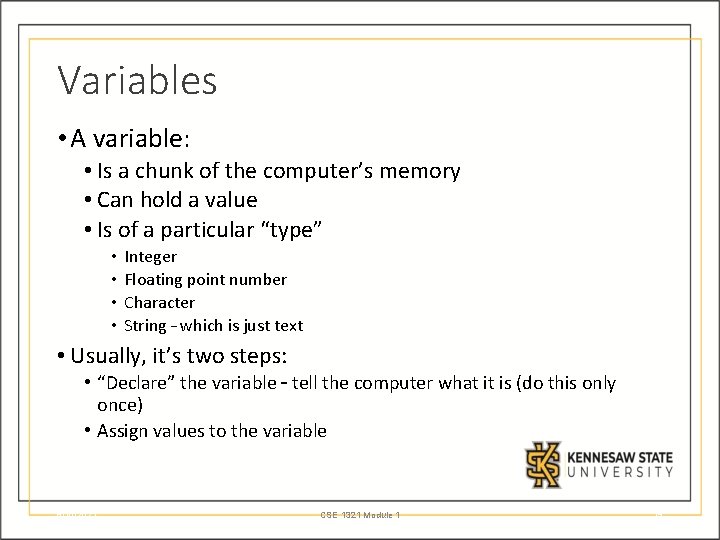
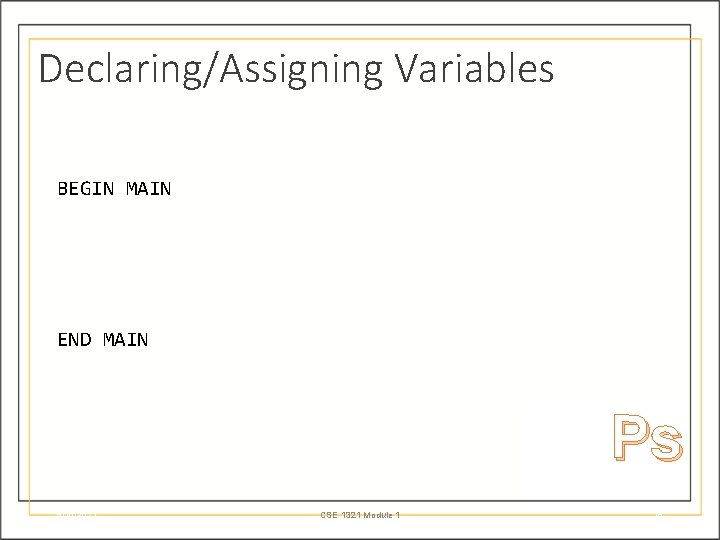
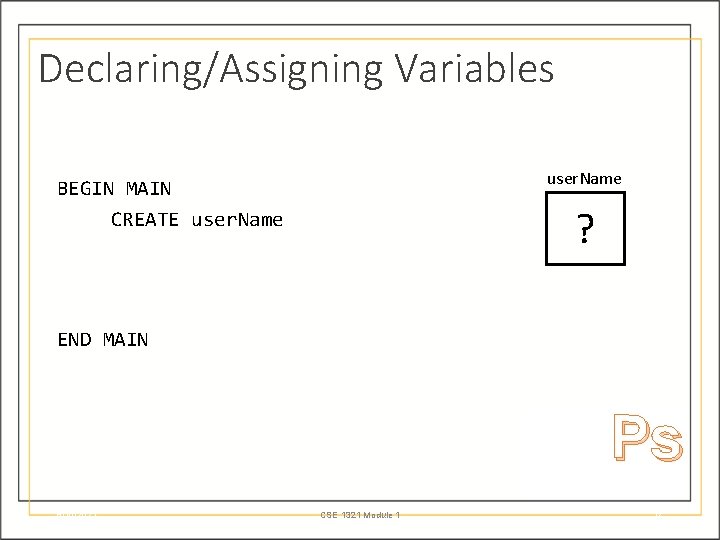
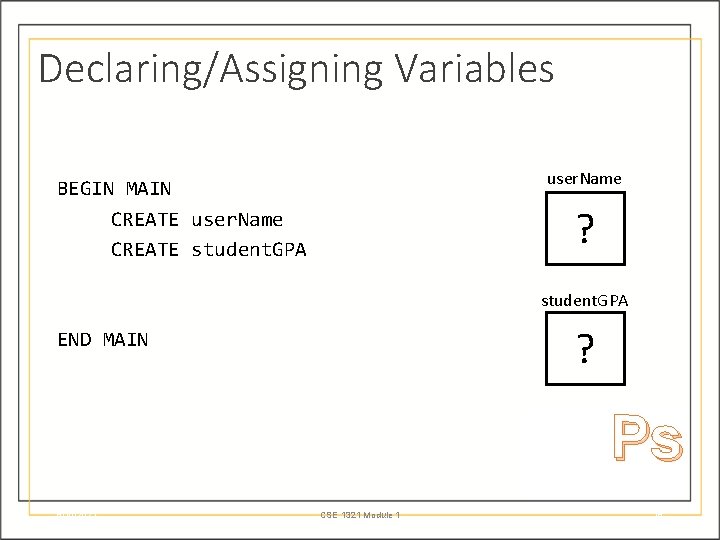
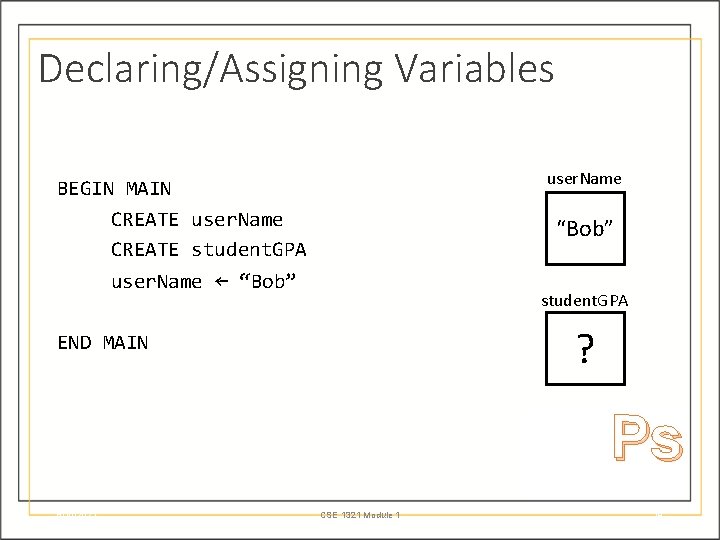
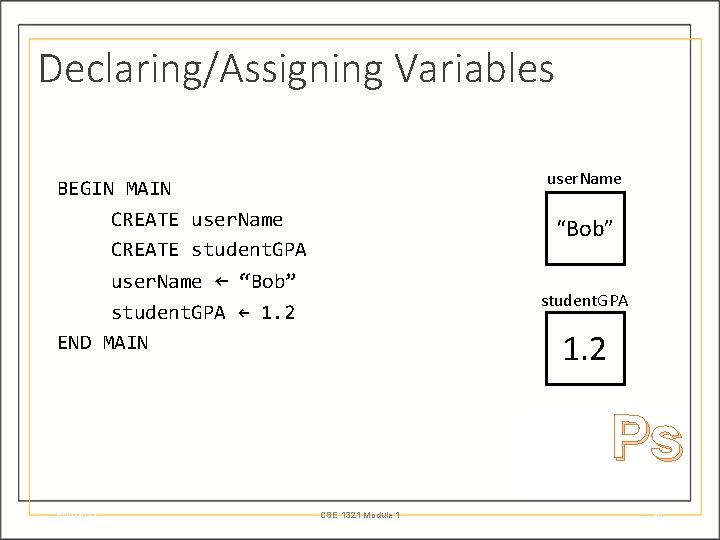
![Declaring/Assigning Variables using System; class Main. Class { public static void Main (string[] args) Declaring/Assigning Variables using System; class Main. Class { public static void Main (string[] args)](https://slidetodoc.com/presentation_image_h2/6113329493bdb08d5c653ed60230da4a/image-21.jpg)
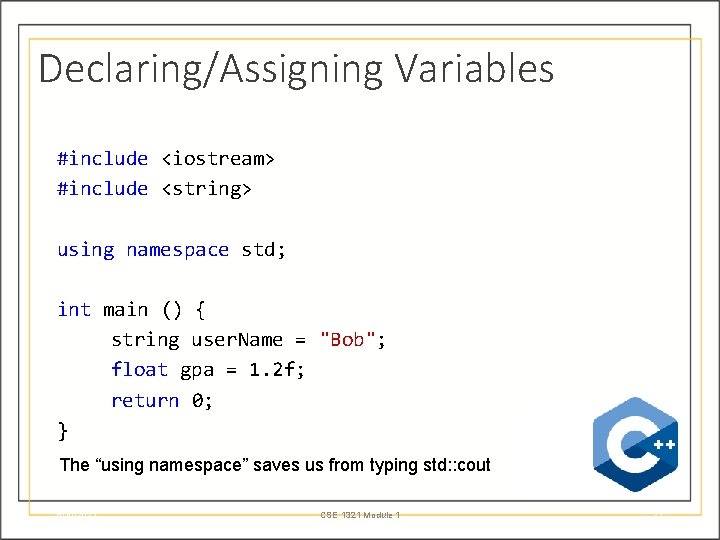
![Declaring/Assigning Variables class Main. Class { public static void main (String[] args) { String Declaring/Assigning Variables class Main. Class { public static void main (String[] args) { String](https://slidetodoc.com/presentation_image_h2/6113329493bdb08d5c653ed60230da4a/image-23.jpg)
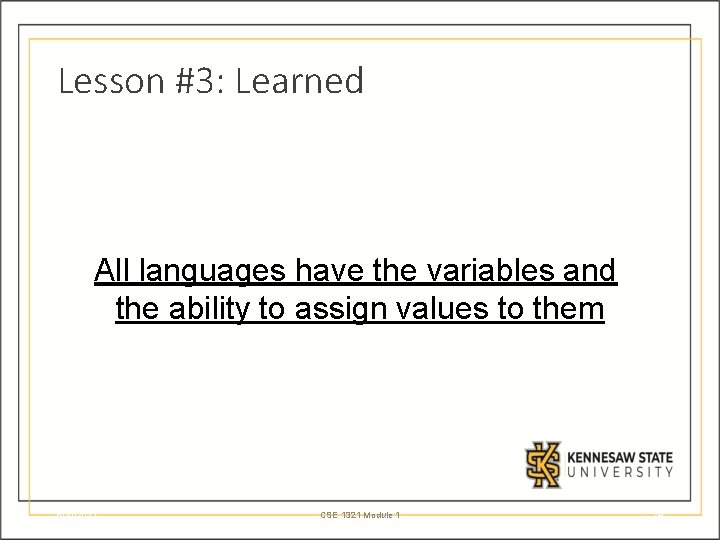
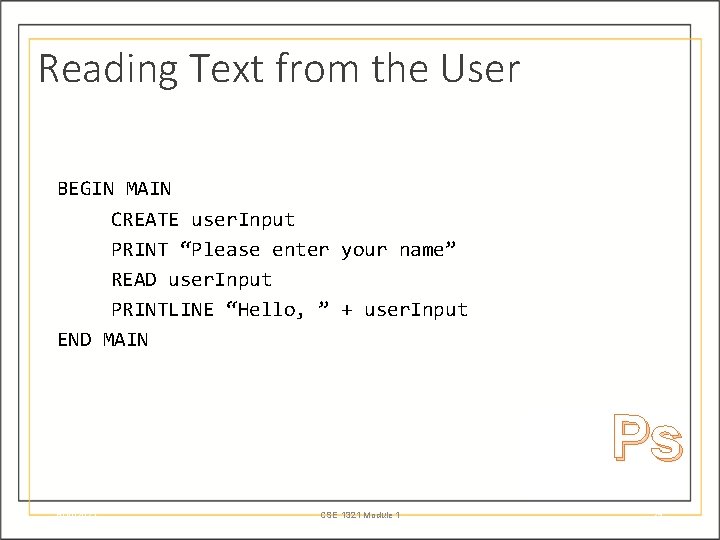
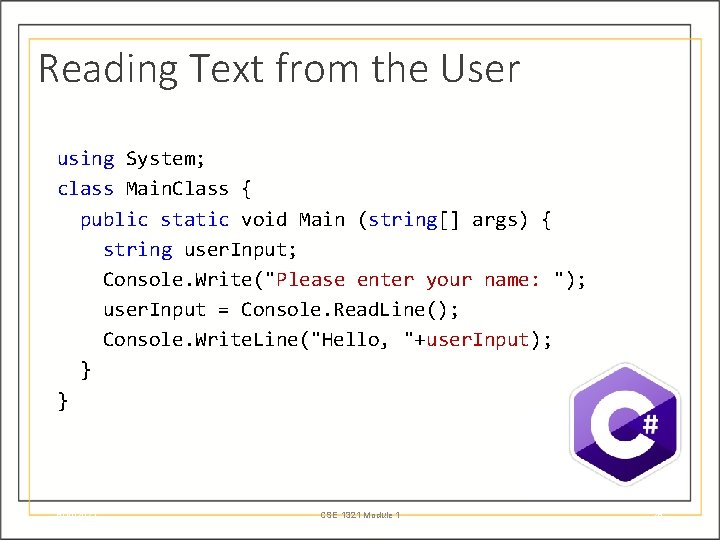
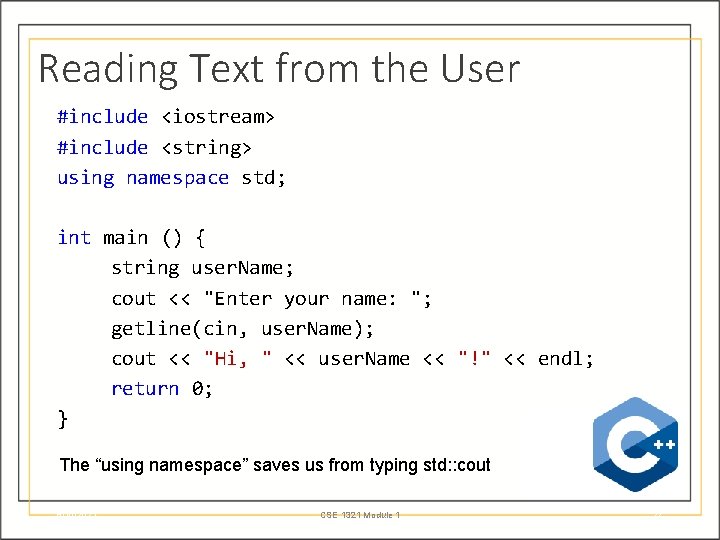
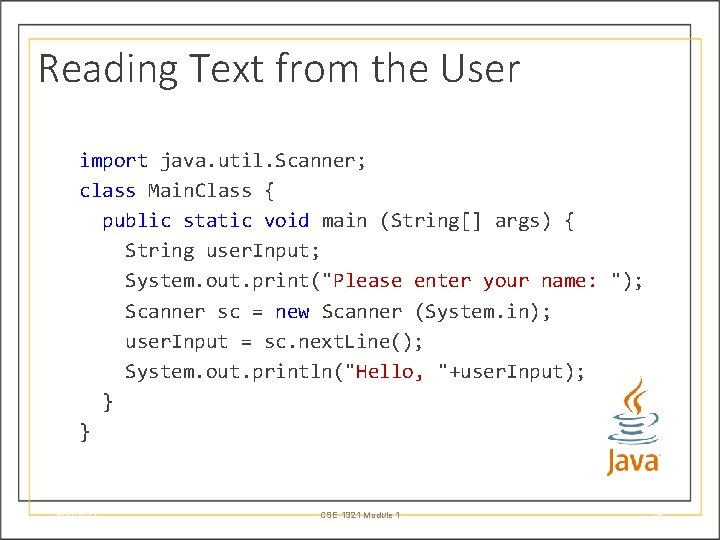
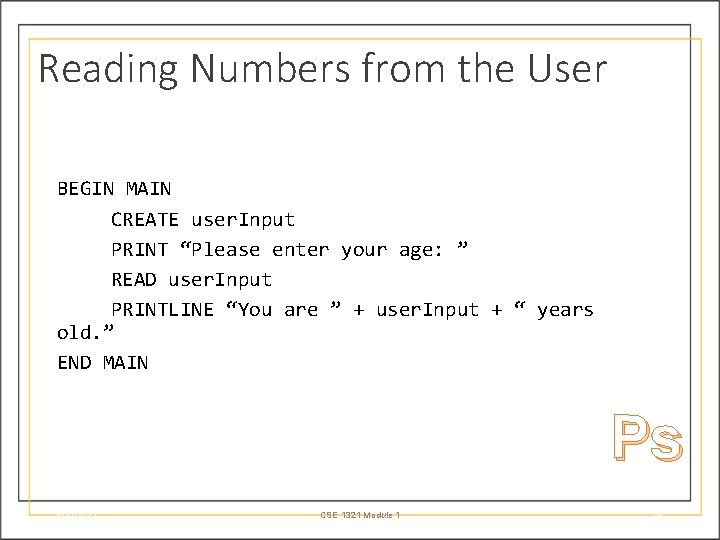
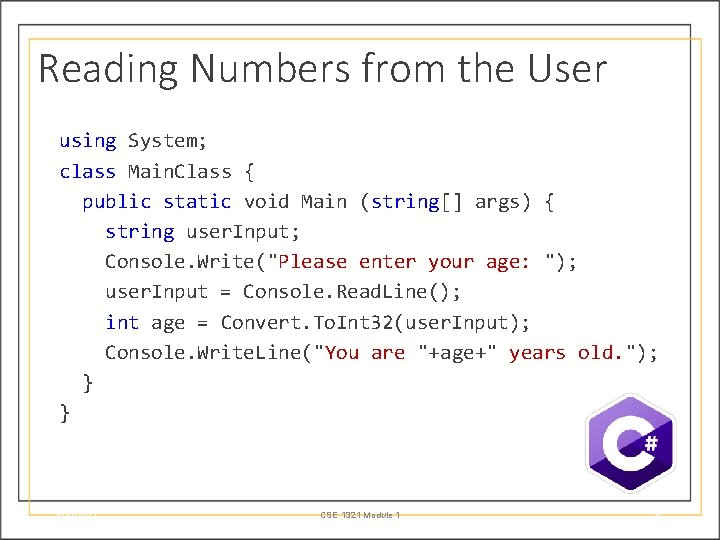
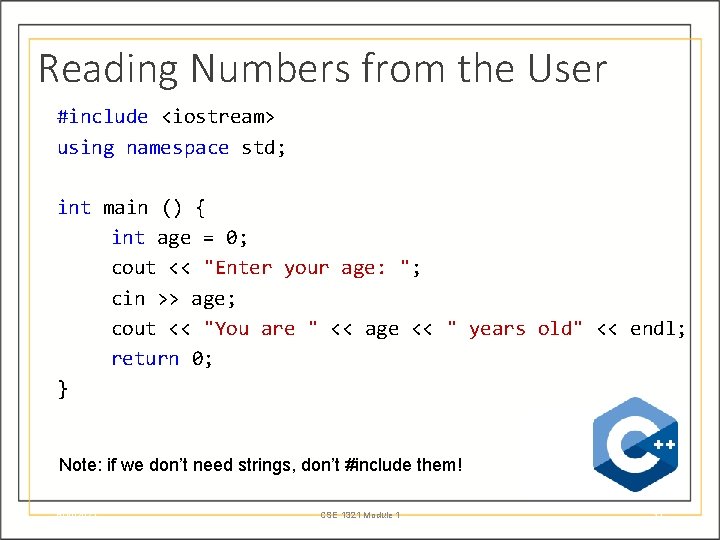
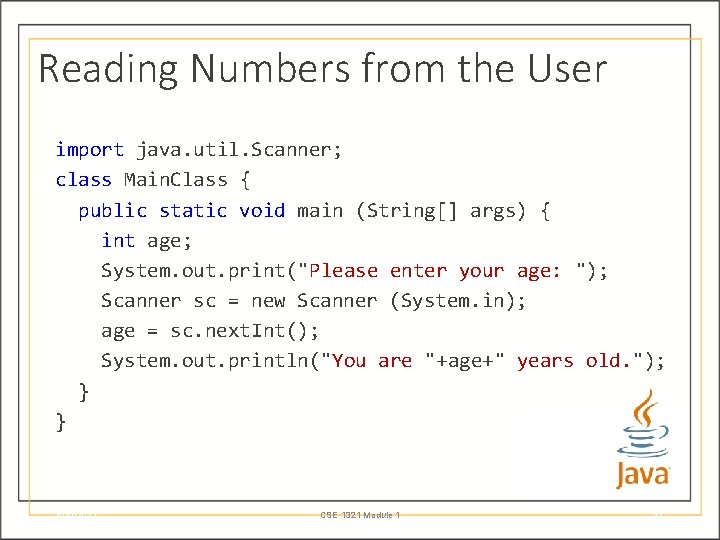
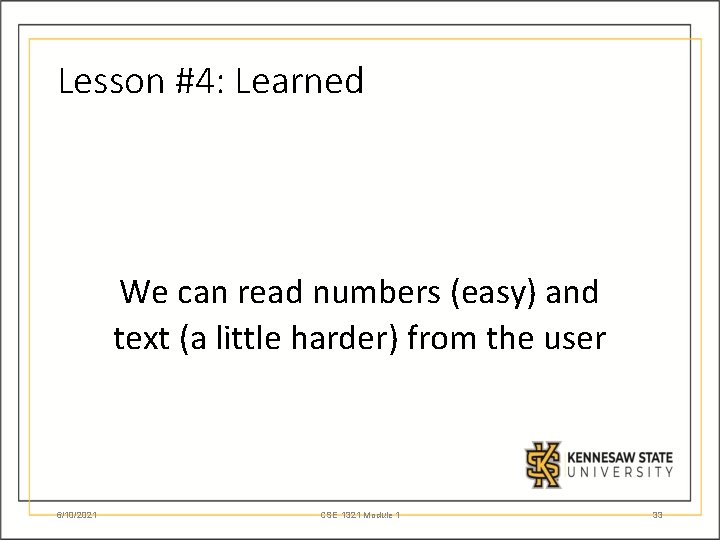
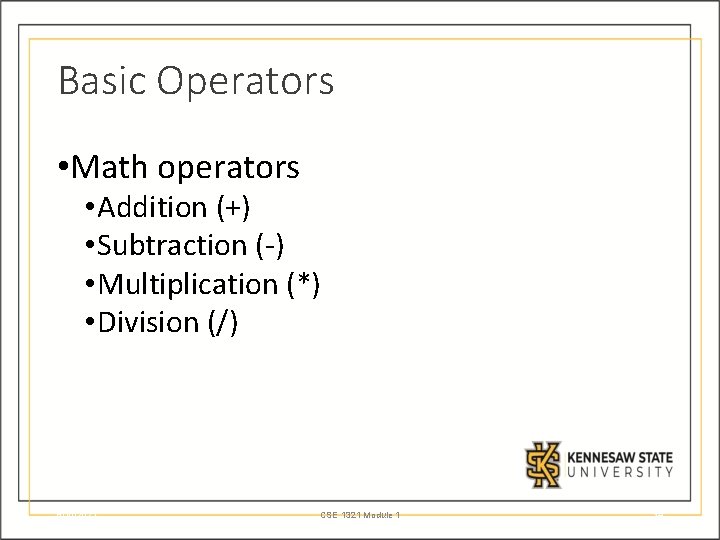
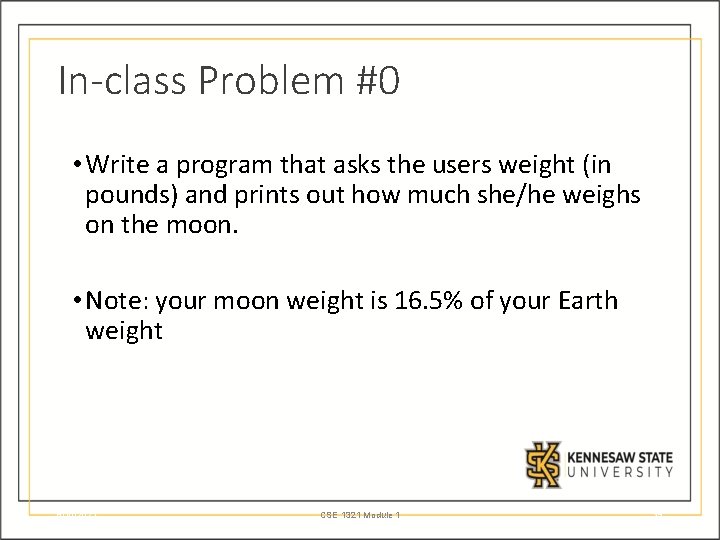
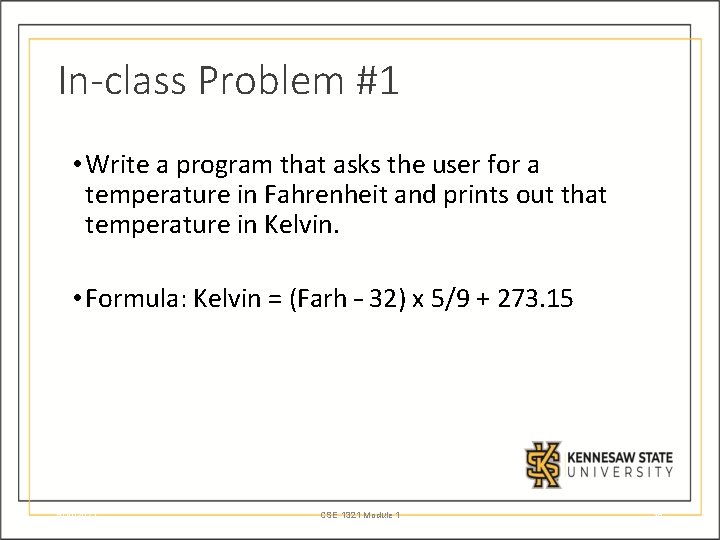
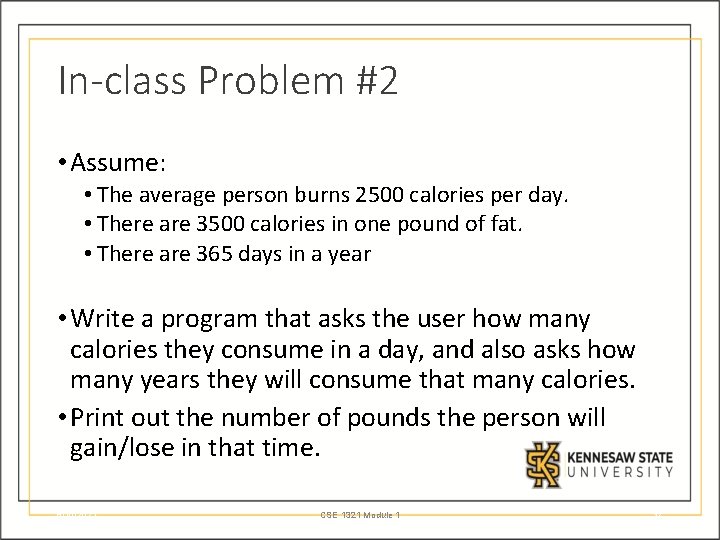
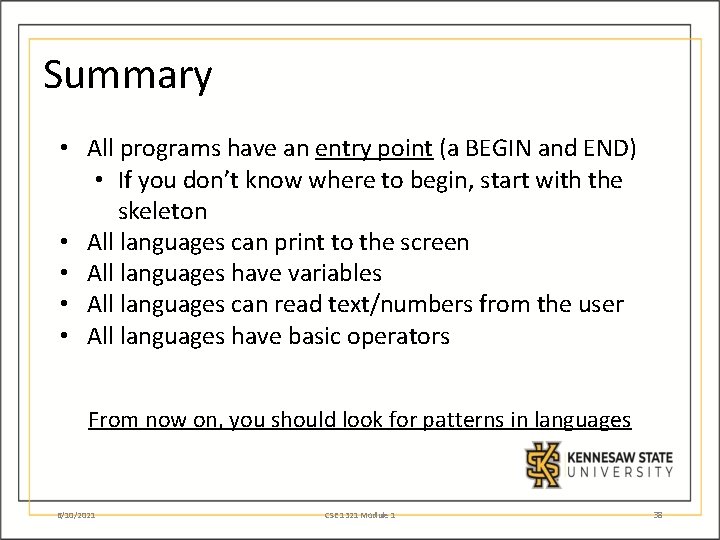
- Slides: 38
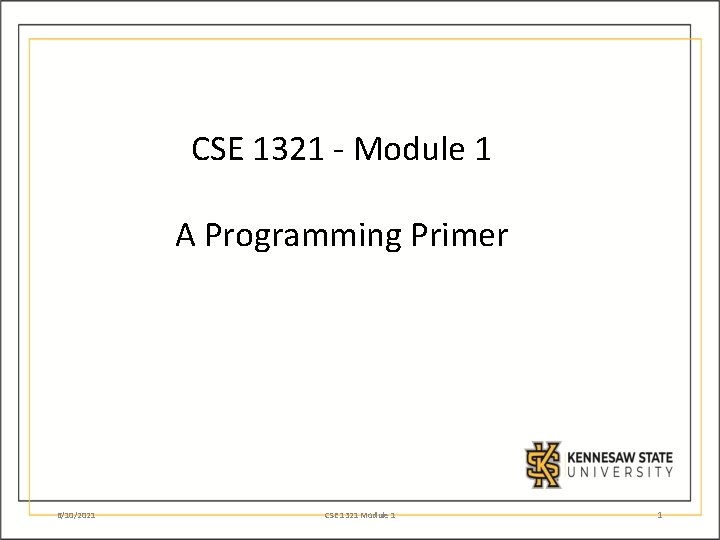
CSE 1321 - Module 1 A Programming Primer 6/10/2021 CSE 1321 Module 1 1
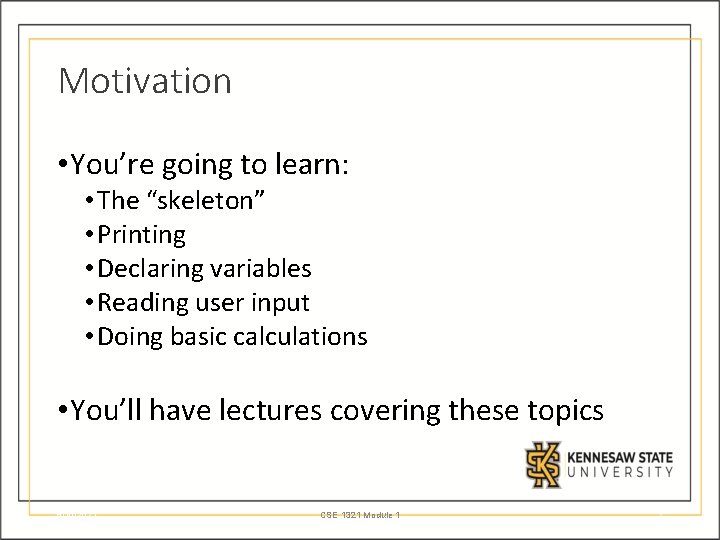
Motivation • You’re going to learn: • The “skeleton” • Printing • Declaring variables • Reading user input • Doing basic calculations • You’ll have lectures covering these topics 6/10/2021 CSE 1321 Module 1 2
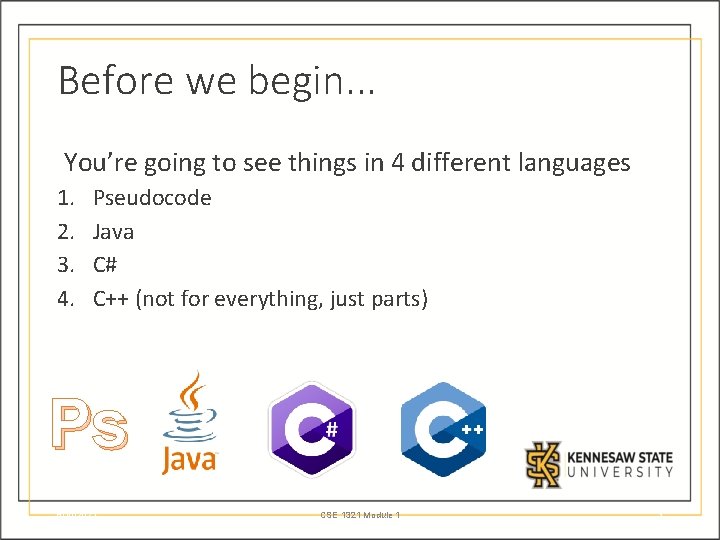
Before we begin. . . You’re going to see things in 4 different languages 1. 2. 3. 4. Pseudocode Java C# C++ (not for everything, just parts) Ps 6/10/2021 CSE 1321 Module 1 3
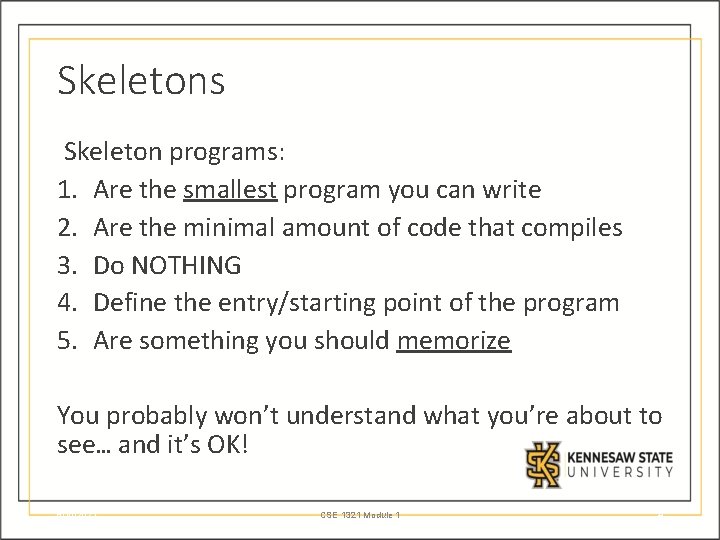
Skeletons Skeleton programs: 1. Are the smallest program you can write 2. Are the minimal amount of code that compiles 3. Do NOTHING 4. Define the entry/starting point of the program 5. Are something you should memorize You probably won’t understand what you’re about to see… and it’s OK! 6/10/2021 CSE 1321 Module 1 4
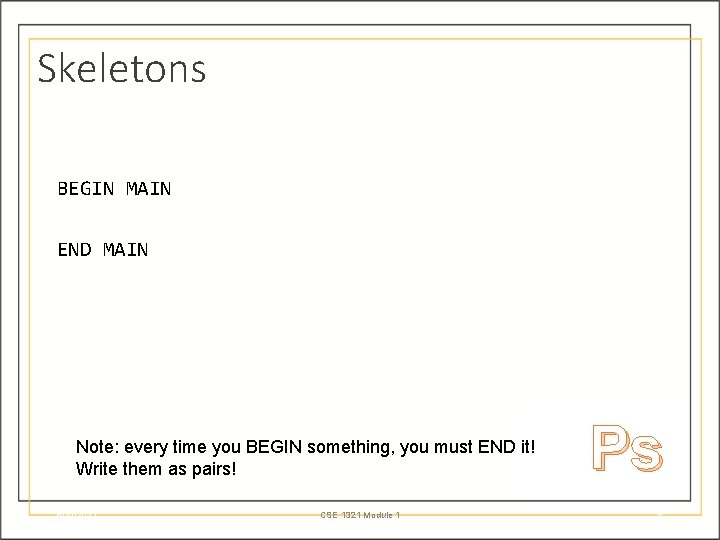
Skeletons BEGIN MAIN END MAIN Note: every time you BEGIN something, you must END it! Write them as pairs! 6/10/2021 CSE 1321 Module 1 Ps 5
![Skeletons using System class Main Class public static void Main string args Skeletons using System; class Main. Class { public static void Main (string[] args) {](https://slidetodoc.com/presentation_image_h2/6113329493bdb08d5c653ed60230da4a/image-6.jpg)
Skeletons using System; class Main. Class { public static void Main (string[] args) { } } Note: The opening “{“ means BEGIN and the closing “}” means END 6/10/2021 CSE 1321 Module 1 6
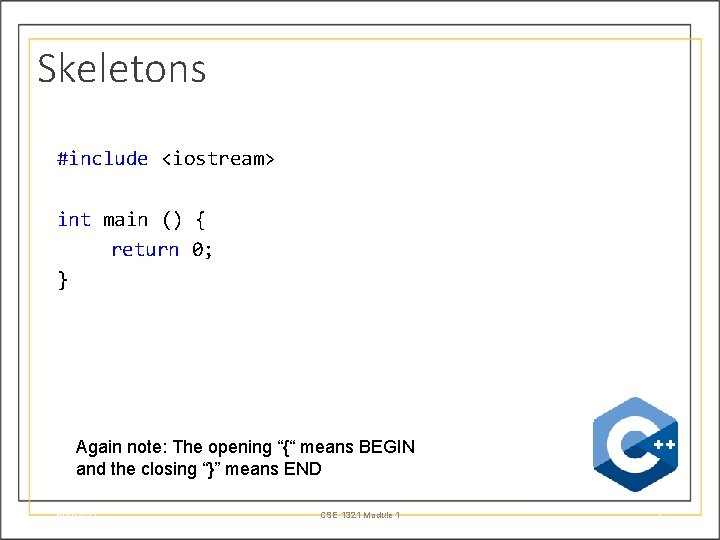
Skeletons #include <iostream> int main () { return 0; } Again note: The opening “{“ means BEGIN and the closing “}” means END 6/10/2021 CSE 1321 Module 1 7
![Skeletons class Main Class public static void main String args Skeletons class Main. Class { public static void main (String[] args) { } }](https://slidetodoc.com/presentation_image_h2/6113329493bdb08d5c653ed60230da4a/image-8.jpg)
Skeletons class Main. Class { public static void main (String[] args) { } } Note: Capitalization matters! 6/10/2021 CSE 1321 Module 1 8
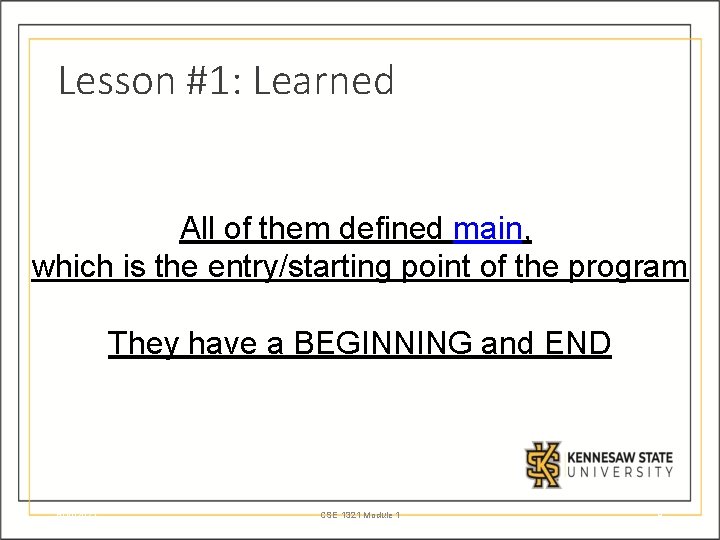
Lesson #1: Learned All of them defined main, which is the entry/starting point of the program They have a BEGINNING and END 6/10/2021 CSE 1321 Module 1 9
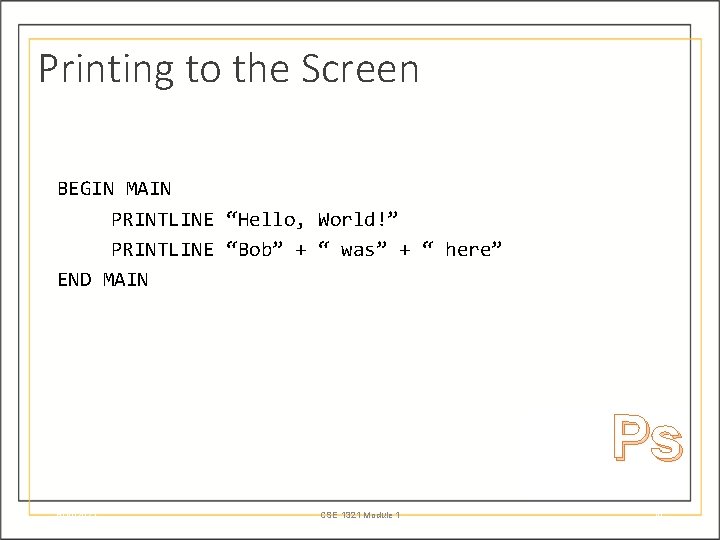
Printing to the Screen BEGIN MAIN PRINTLINE “Hello, World!” PRINTLINE “Bob” + “ was” + “ here” END MAIN Ps 6/10/2021 CSE 1321 Module 1 10
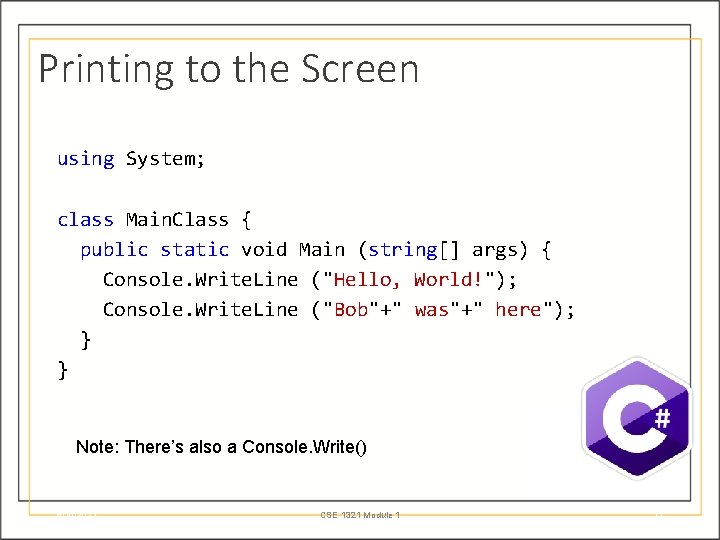
Printing to the Screen using System; class Main. Class { public static void Main (string[] args) { Console. Write. Line ("Hello, World!"); Console. Write. Line ("Bob"+" was"+" here"); } } Note: There’s also a Console. Write() 6/10/2021 CSE 1321 Module 1 11
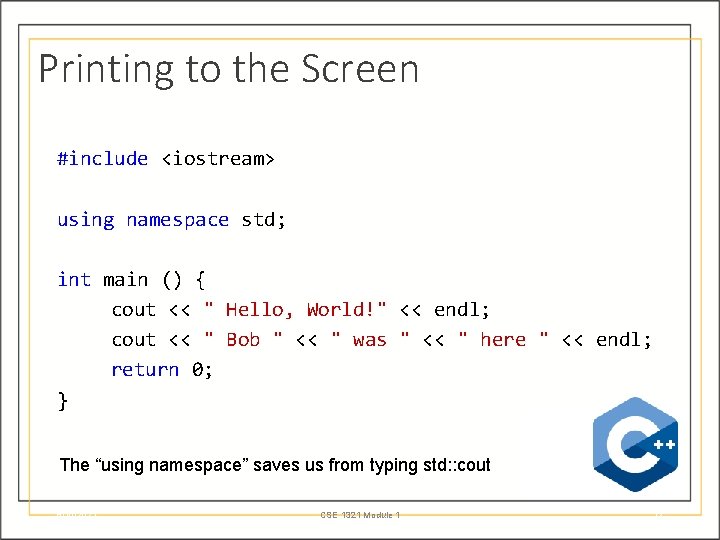
Printing to the Screen #include <iostream> using namespace std; int main () { cout << " Hello, World!" << endl; cout << " Bob " << " was " << " here " << endl; return 0; } The “using namespace” saves us from typing std: : cout 6/10/2021 CSE 1321 Module 1 12
![Printing to the Screen class Main Class public static void main String args Printing to the Screen class Main. Class { public static void main (String[] args)](https://slidetodoc.com/presentation_image_h2/6113329493bdb08d5c653ed60230da4a/image-13.jpg)
Printing to the Screen class Main. Class { public static void main (String[] args) { System. out. println("Hello, World!"); System. out. println("Bob"+" was"+" here"); } } Note: There’s also a System. out. print() 6/10/2021 CSE 1321 Module 1 13
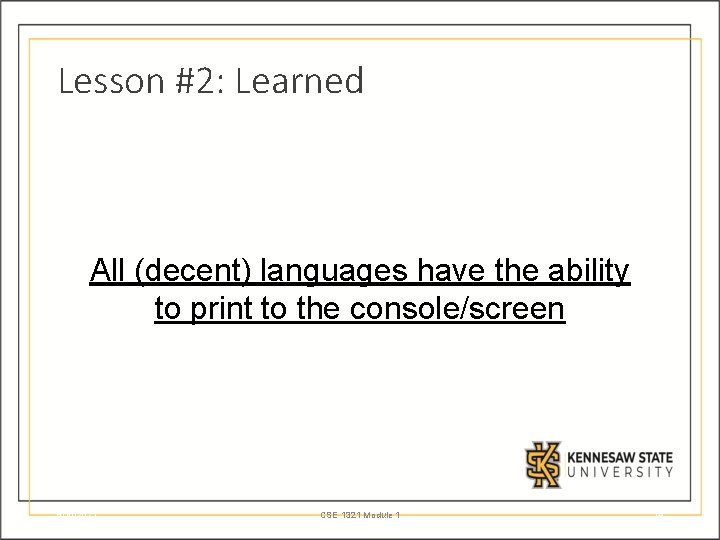
Lesson #2: Learned All (decent) languages have the ability to print to the console/screen 6/10/2021 CSE 1321 Module 1 14
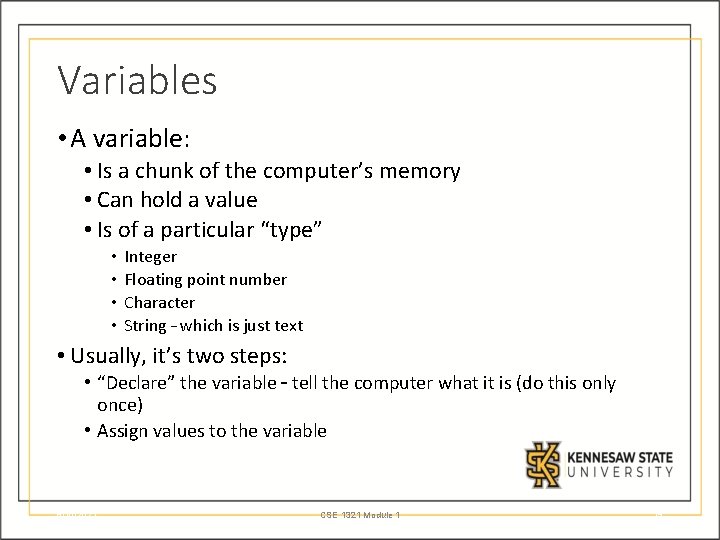
Variables • A variable: • Is a chunk of the computer’s memory • Can hold a value • Is of a particular “type” • • Integer Floating point number Character String – which is just text • Usually, it’s two steps: • “Declare” the variable – tell the computer what it is (do this only once) • Assign values to the variable 6/10/2021 CSE 1321 Module 1 15
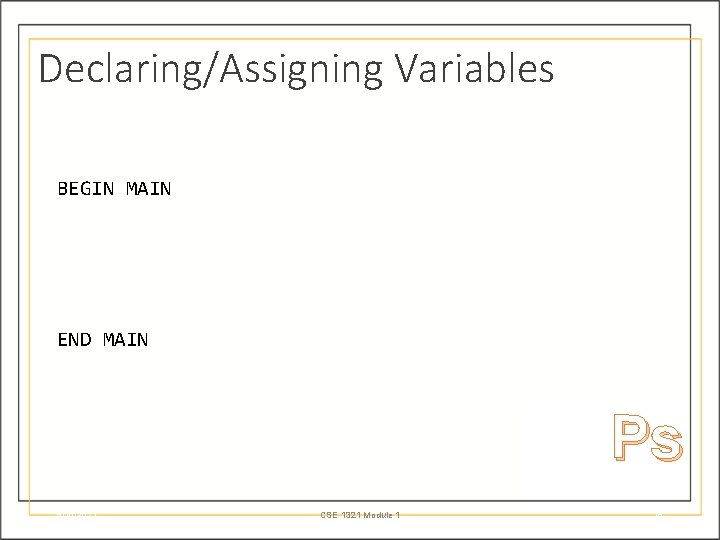
Declaring/Assigning Variables BEGIN MAIN END MAIN Ps 6/10/2021 CSE 1321 Module 1 16
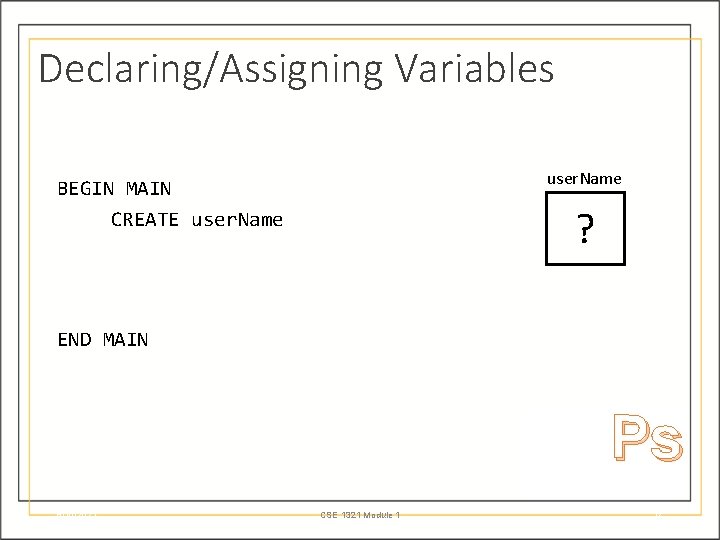
Declaring/Assigning Variables user. Name BEGIN MAIN CREATE user. Name ? END MAIN Ps 6/10/2021 CSE 1321 Module 1 17
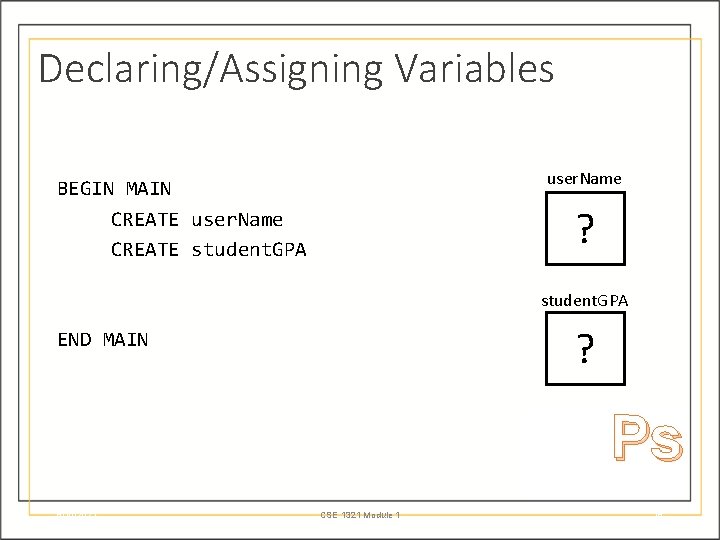
Declaring/Assigning Variables user. Name BEGIN MAIN CREATE user. Name CREATE student. GPA ? END MAIN Ps 6/10/2021 CSE 1321 Module 1 18
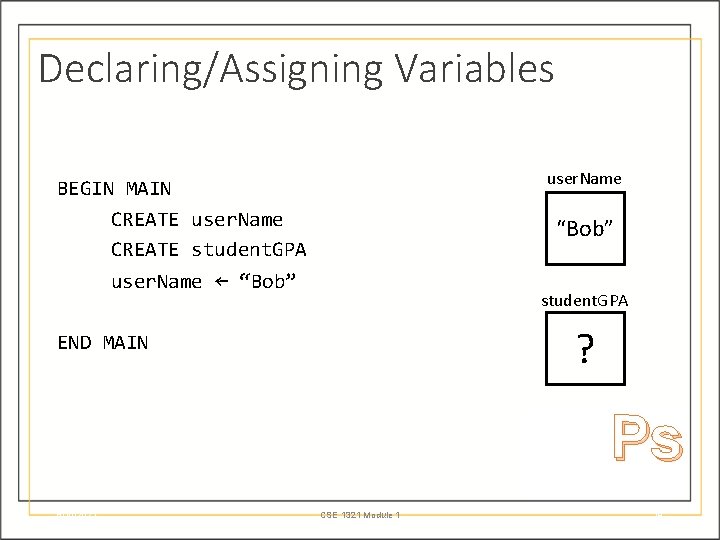
Declaring/Assigning Variables user. Name BEGIN MAIN CREATE user. Name CREATE student. GPA “Bob” user. Name ← “Bob” student. GPA ? END MAIN Ps 6/10/2021 CSE 1321 Module 1 19
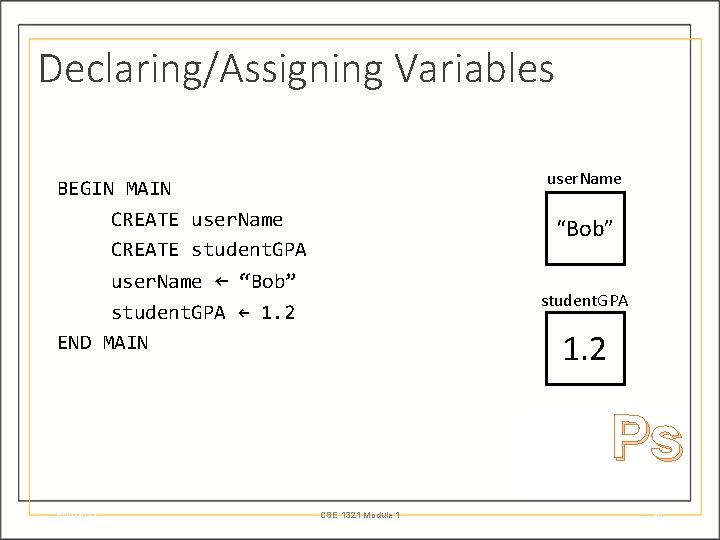
Declaring/Assigning Variables user. Name BEGIN MAIN CREATE user. Name CREATE student. GPA “Bob” user. Name ← “Bob” student. GPA ← 1. 2 END MAIN student. GPA 1. 2 Ps 6/10/2021 CSE 1321 Module 1 20
![DeclaringAssigning Variables using System class Main Class public static void Main string args Declaring/Assigning Variables using System; class Main. Class { public static void Main (string[] args)](https://slidetodoc.com/presentation_image_h2/6113329493bdb08d5c653ed60230da4a/image-21.jpg)
Declaring/Assigning Variables using System; class Main. Class { public static void Main (string[] args) { string user. Name; float gpa; user. Name = "Bob"; gpa = 1. 2 f; } } 6/10/2021 CSE 1321 Module 1 21
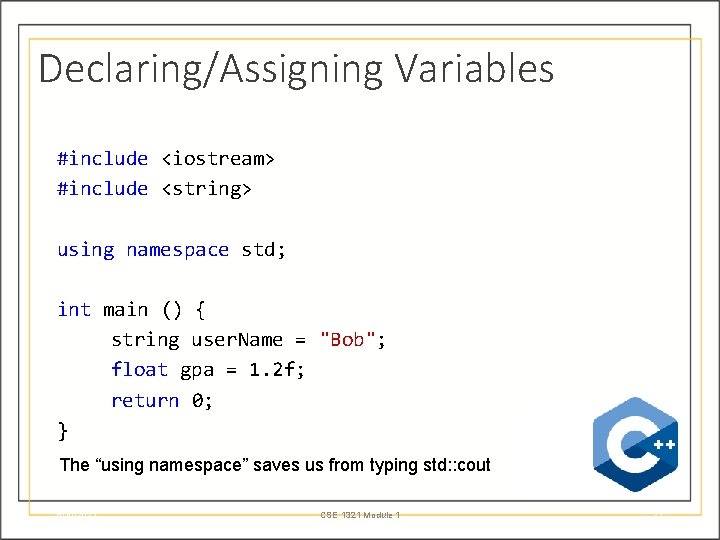
Declaring/Assigning Variables #include <iostream> #include <string> using namespace std; int main () { string user. Name = "Bob"; float gpa = 1. 2 f; return 0; } The “using namespace” saves us from typing std: : cout 6/10/2021 CSE 1321 Module 1 22
![DeclaringAssigning Variables class Main Class public static void main String args String Declaring/Assigning Variables class Main. Class { public static void main (String[] args) { String](https://slidetodoc.com/presentation_image_h2/6113329493bdb08d5c653ed60230da4a/image-23.jpg)
Declaring/Assigning Variables class Main. Class { public static void main (String[] args) { String user. Name; float gpa; user. Name = "Bob"; gpa = 1. 2 f; } } 6/10/2021 CSE 1321 Module 1 23
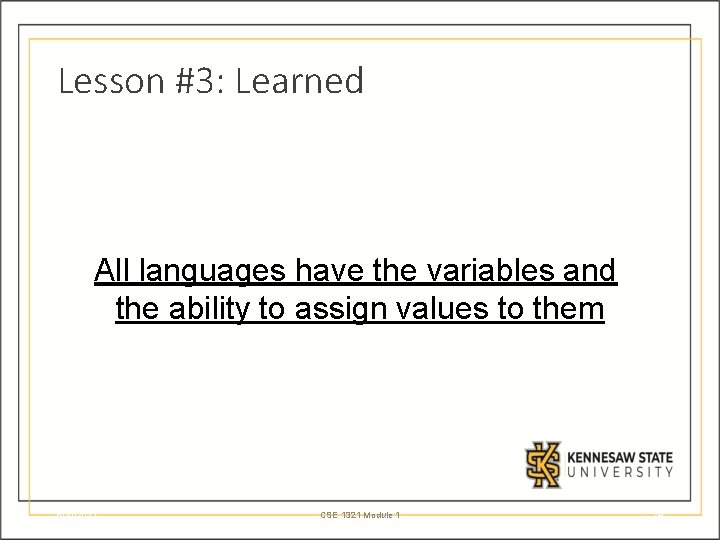
Lesson #3: Learned All languages have the variables and the ability to assign values to them 6/10/2021 CSE 1321 Module 1 24
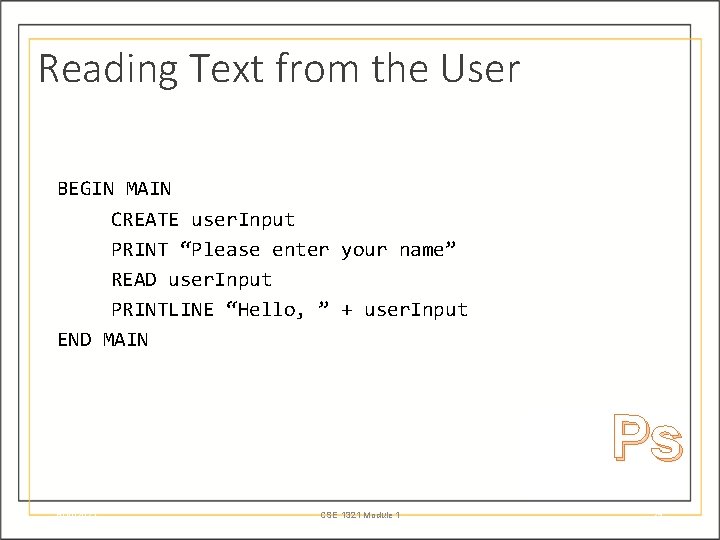
Reading Text from the User BEGIN MAIN CREATE user. Input PRINT “Please enter your name” READ user. Input PRINTLINE “Hello, ” + user. Input END MAIN Ps 6/10/2021 CSE 1321 Module 1 25
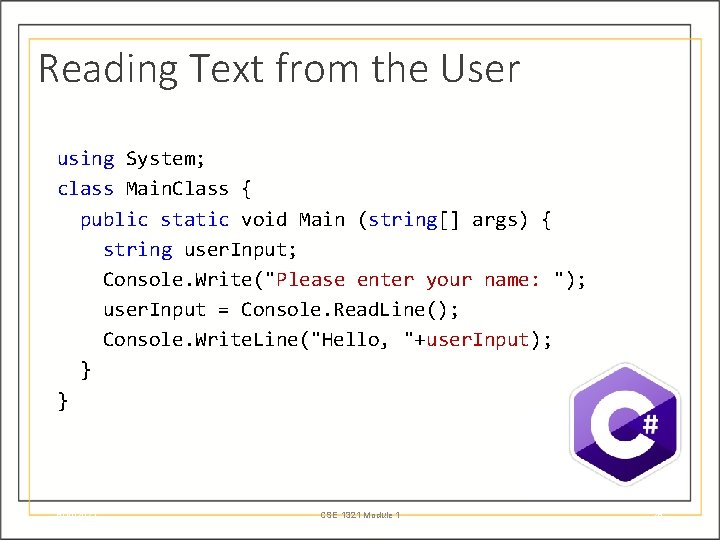
Reading Text from the User using System; class Main. Class { public static void Main (string[] args) { string user. Input; Console. Write("Please enter your name: "); user. Input = Console. Read. Line(); Console. Write. Line("Hello, "+user. Input); } } 6/10/2021 CSE 1321 Module 1 26
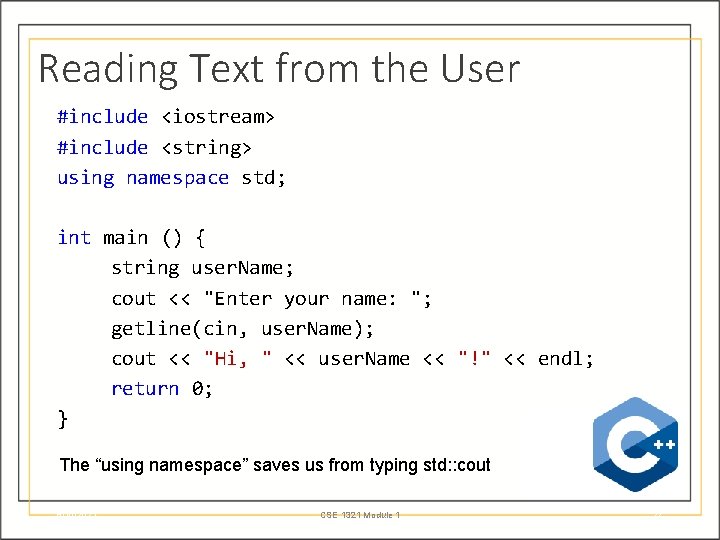
Reading Text from the User #include <iostream> #include <string> using namespace std; int main () { string user. Name; cout << "Enter your name: "; getline(cin, user. Name); cout << "Hi, " << user. Name << "!" << endl; return 0; } The “using namespace” saves us from typing std: : cout 6/10/2021 CSE 1321 Module 1 27
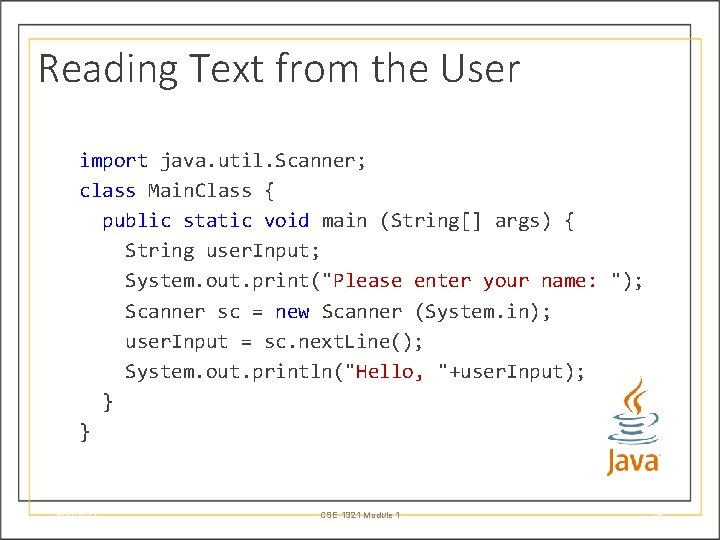
Reading Text from the User import java. util. Scanner; class Main. Class { public static void main (String[] args) { String user. Input; System. out. print("Please enter your name: "); Scanner sc = new Scanner (System. in); user. Input = sc. next. Line(); System. out. println("Hello, "+user. Input); } } 6/10/2021 CSE 1321 Module 1 28
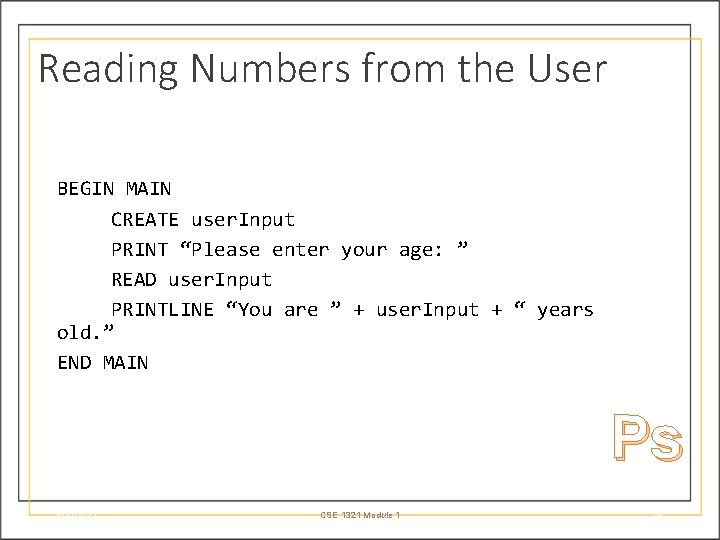
Reading Numbers from the User BEGIN MAIN CREATE user. Input PRINT “Please enter your age: ” READ user. Input PRINTLINE “You are ” + user. Input + “ years old. ” END MAIN Ps 6/10/2021 CSE 1321 Module 1 29
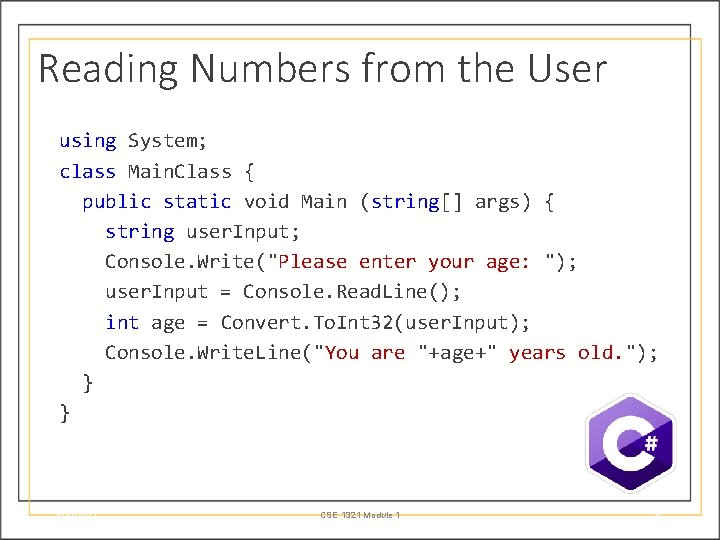
Reading Numbers from the User using System; class Main. Class { public static void Main (string[] args) { string user. Input; Console. Write("Please enter your age: "); user. Input = Console. Read. Line(); int age = Convert. To. Int 32(user. Input); Console. Write. Line("You are "+age+" years old. "); } } 6/10/2021 CSE 1321 Module 1 30
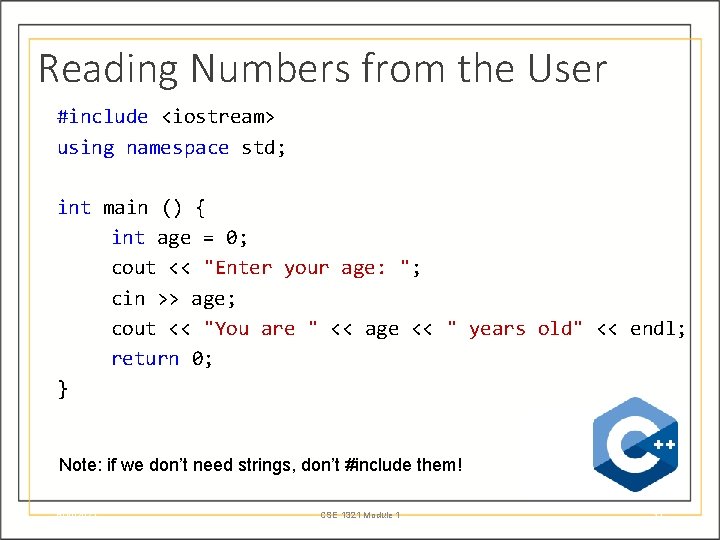
Reading Numbers from the User #include <iostream> using namespace std; int main () { int age = 0; cout << "Enter your age: "; cin >> age; cout << "You are " << age << " years old" << endl; return 0; } Note: if we don’t need strings, don’t #include them! 6/10/2021 CSE 1321 Module 1 31
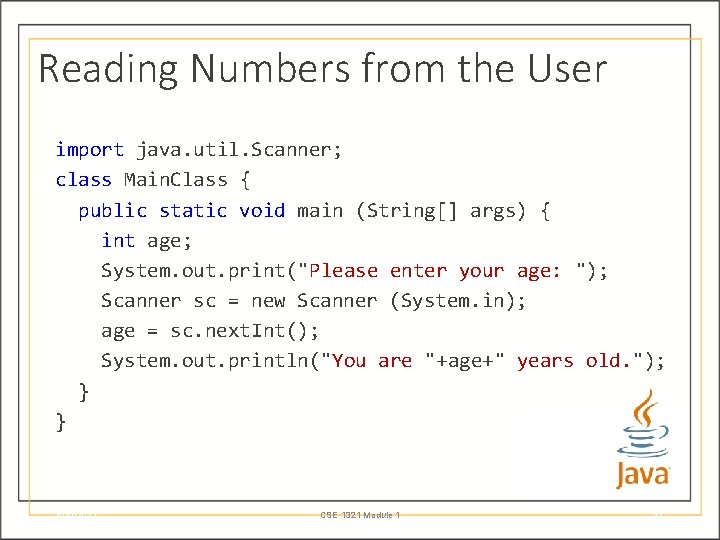
Reading Numbers from the User import java. util. Scanner; class Main. Class { public static void main (String[] args) { int age; System. out. print("Please enter your age: "); Scanner sc = new Scanner (System. in); age = sc. next. Int(); System. out. println("You are "+age+" years old. "); } } 6/10/2021 CSE 1321 Module 1 32
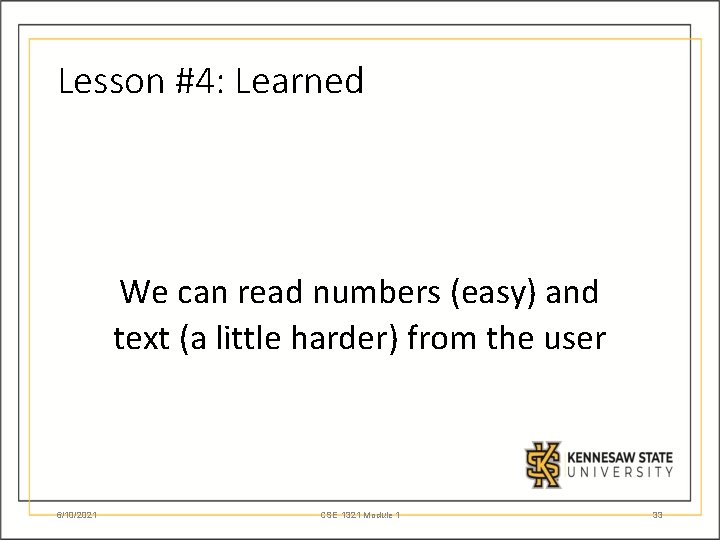
Lesson #4: Learned We can read numbers (easy) and text (a little harder) from the user 6/10/2021 CSE 1321 Module 1 33
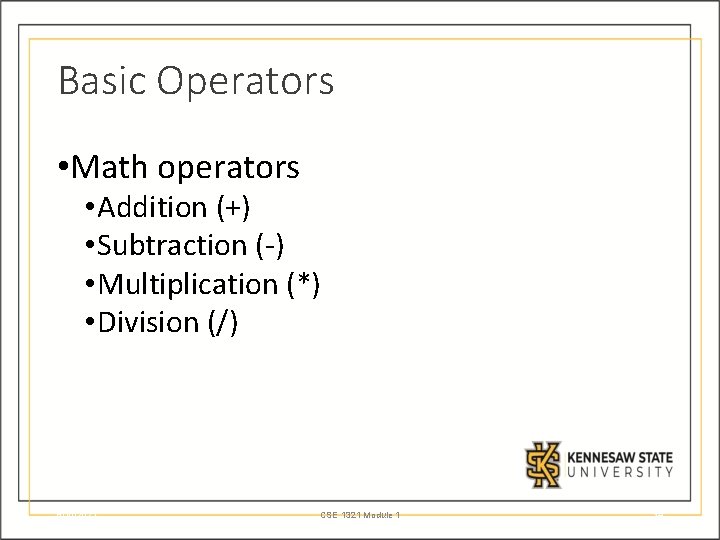
Basic Operators • Math operators • Addition (+) • Subtraction (-) • Multiplication (*) • Division (/) 6/10/2021 CSE 1321 Module 1 34
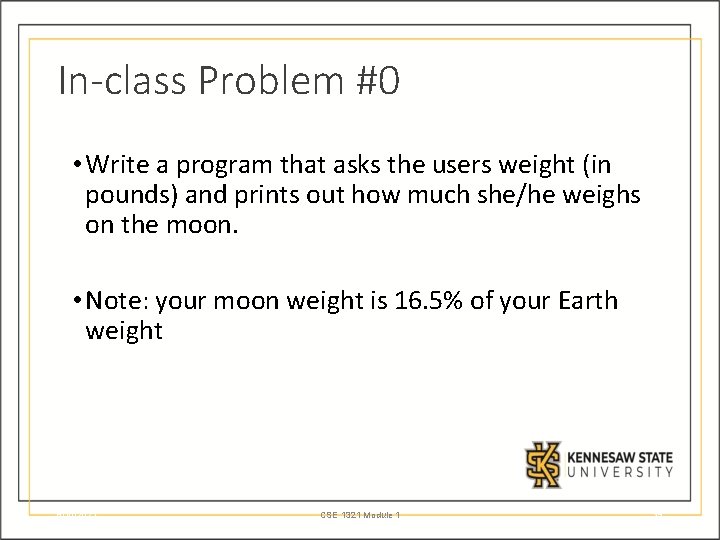
In-class Problem #0 • Write a program that asks the users weight (in pounds) and prints out how much she/he weighs on the moon. • Note: your moon weight is 16. 5% of your Earth weight 6/10/2021 CSE 1321 Module 1 35
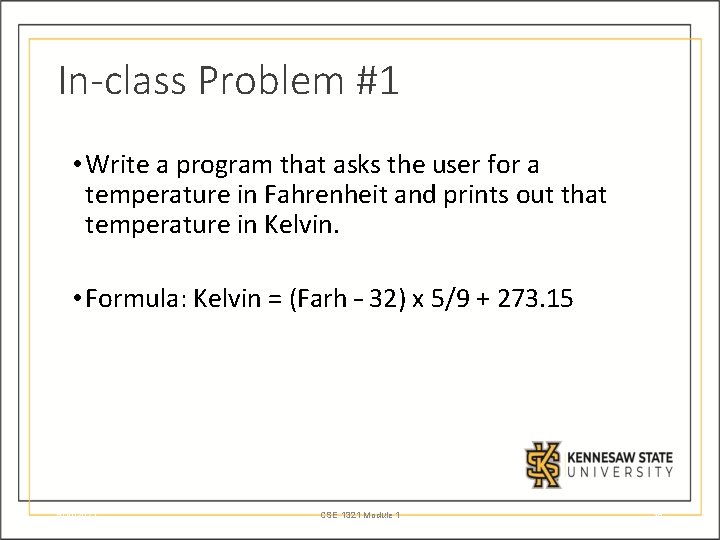
In-class Problem #1 • Write a program that asks the user for a temperature in Fahrenheit and prints out that temperature in Kelvin. • Formula: Kelvin = (Farh – 32) x 5/9 + 273. 15 6/10/2021 CSE 1321 Module 1 36
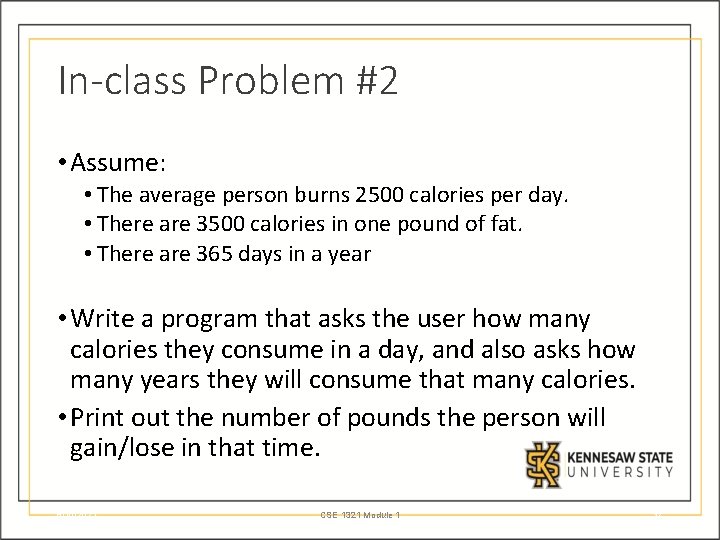
In-class Problem #2 • Assume: • The average person burns 2500 calories per day. • There are 3500 calories in one pound of fat. • There are 365 days in a year • Write a program that asks the user how many calories they consume in a day, and also asks how many years they will consume that many calories. • Print out the number of pounds the person will gain/lose in that time. 6/10/2021 CSE 1321 Module 1 37
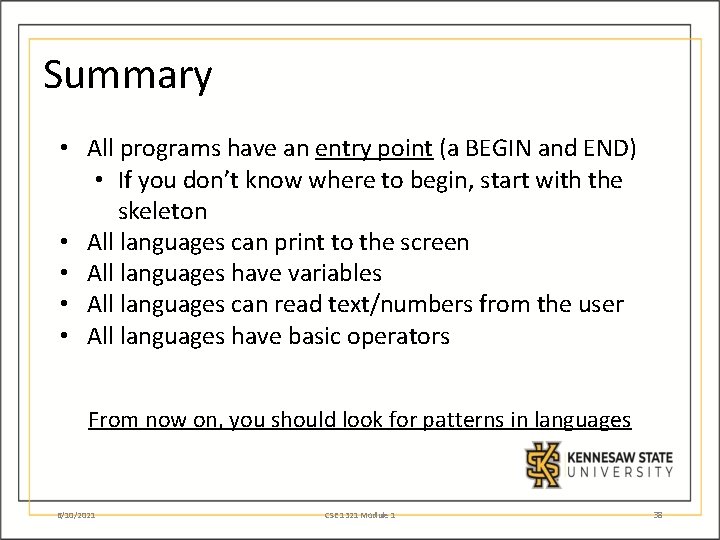
Summary • All programs have an entry point (a BEGIN and END) • If you don’t know where to begin, start with the skeleton • All languages can print to the screen • All languages have variables • All languages can read text/numbers from the user • All languages have basic operators From now on, you should look for patterns in languages 6/10/2021 CSE 1321 Module 1 38
Cse 1321
Ksu ccse tutoring
Cse 1321 slides
Cse 1321
6102021
6102021
6102021
6102021
1321cc
Risoluzione
Parabola del trigo y la cizaña enseñanza
1321-1265
Adam doupe cse 340
Vineeth kashyap
C device module module 1
Perbedaan linear programming dan integer programming
Greedy vs dynamic
System programming definition
Integer programming vs linear programming
Programing adalah
Cse 5236
Cse 6331 uta
Cse 423
Cse 423
Cse 576
Cse 344
Cse 30
Cse 2431
String matching cses
Cse 312
Cse 402
Cse 3318
Cse323
Roger crawfis
Cse 2431
Lll programming language
Cse 6740
Cse 351 lab5
Cse 5236