Module 3 Selection Structures 352021 CSE 1321 Module
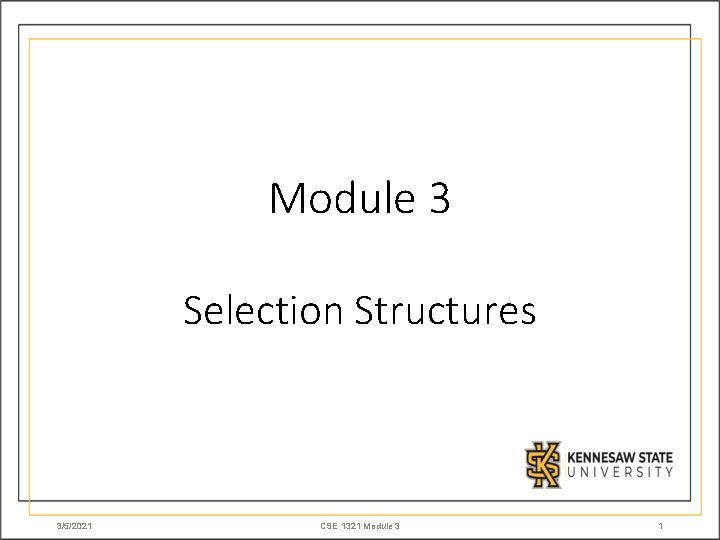
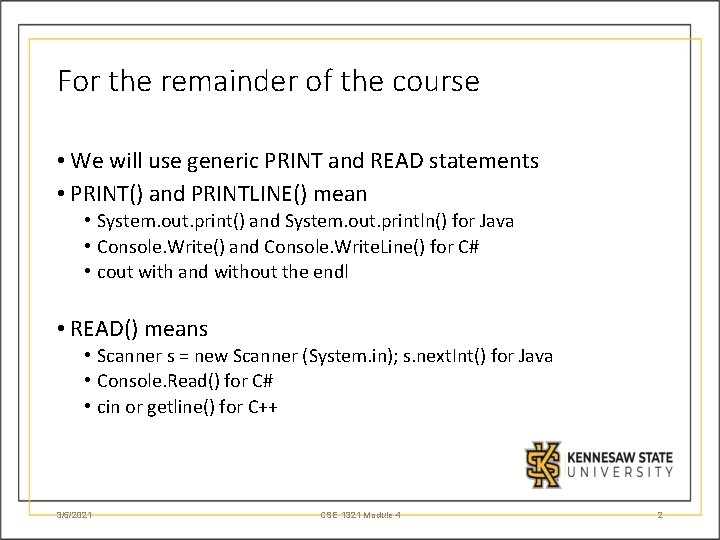
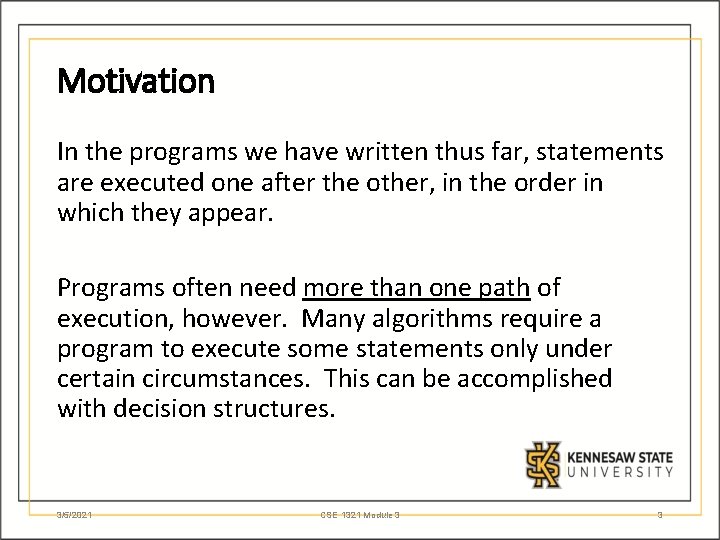
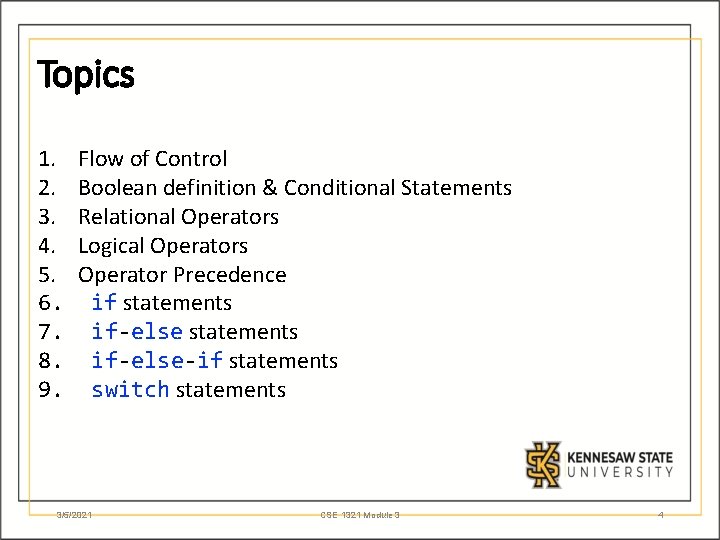
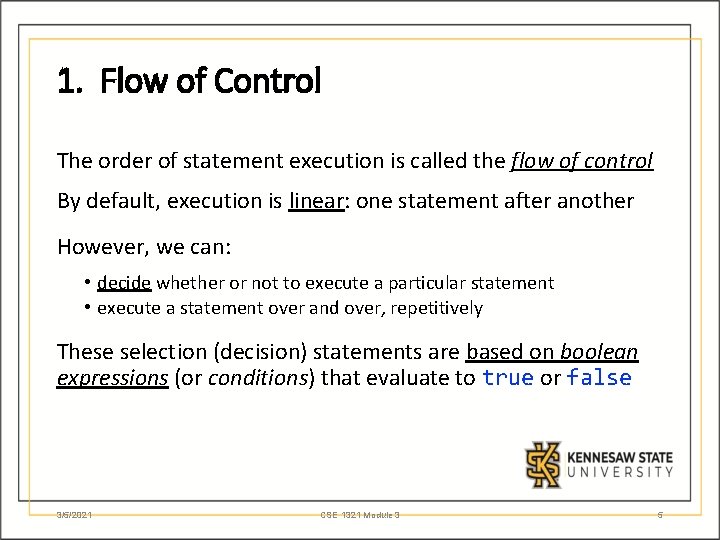
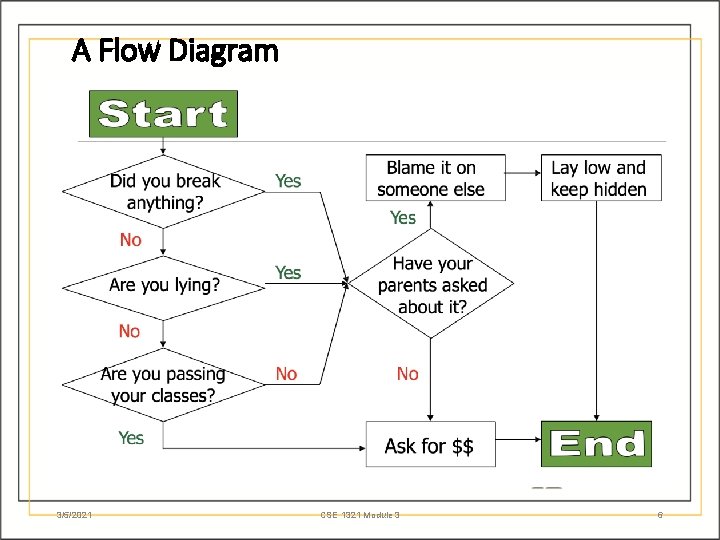
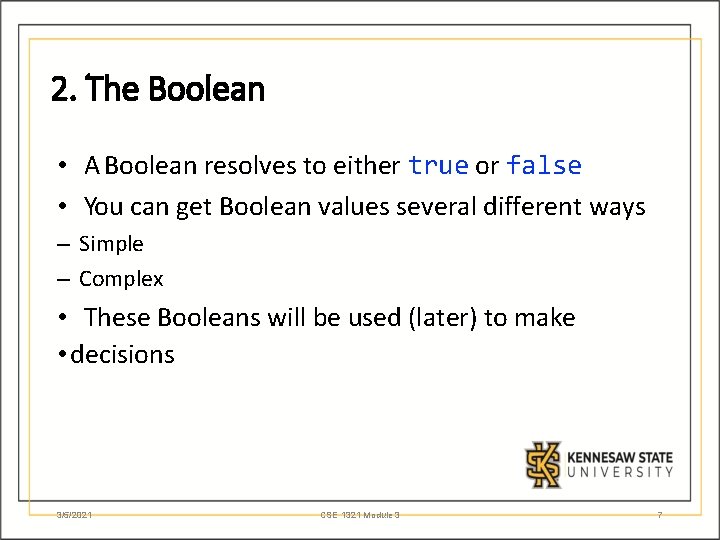
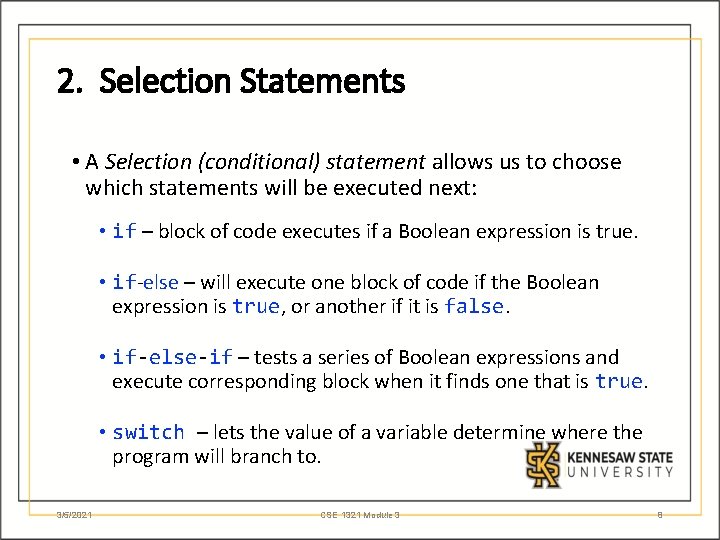
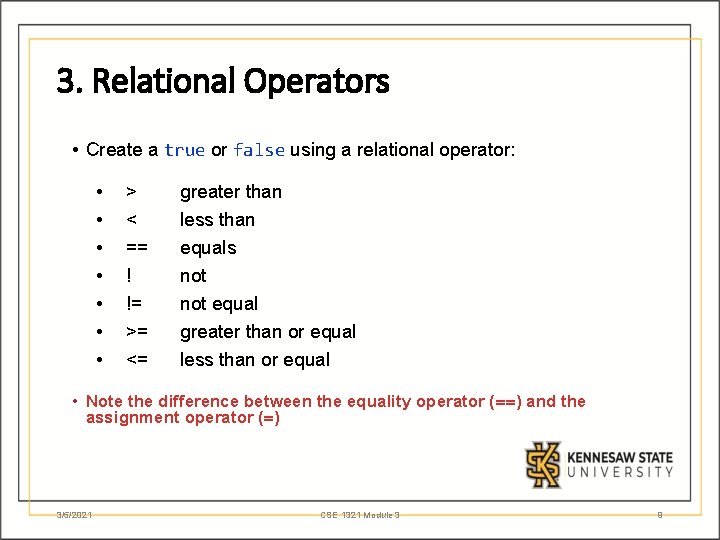
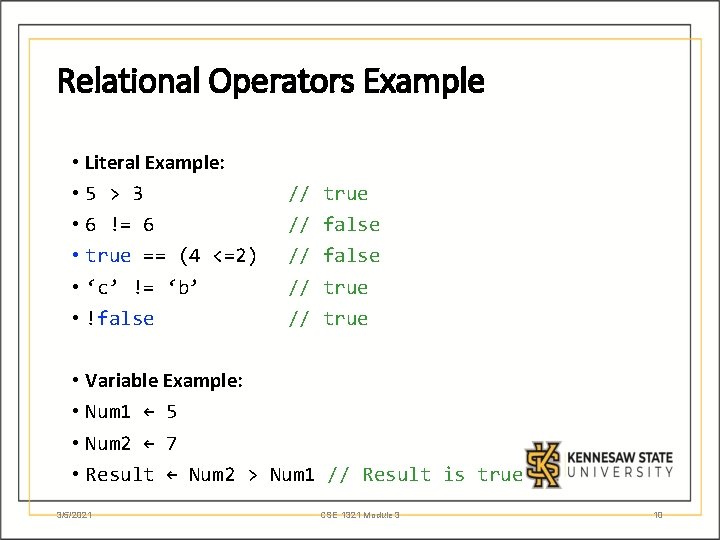
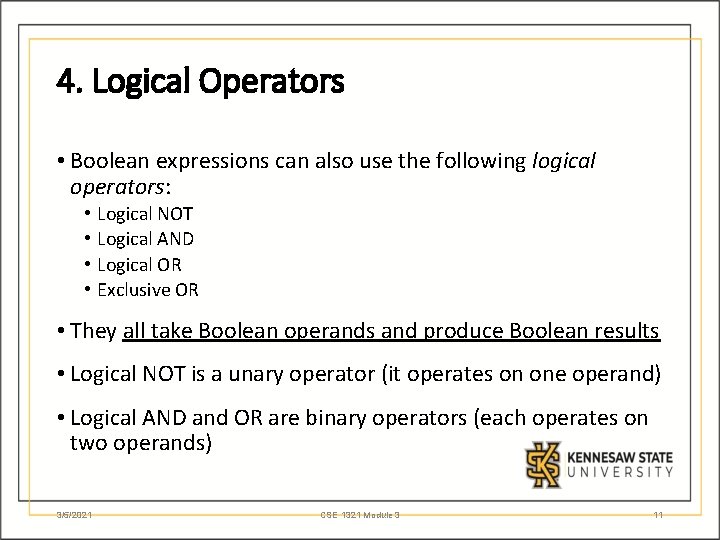
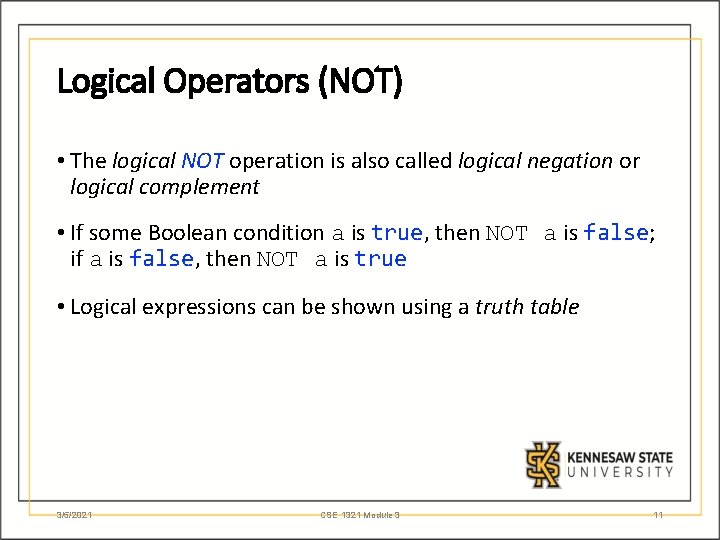
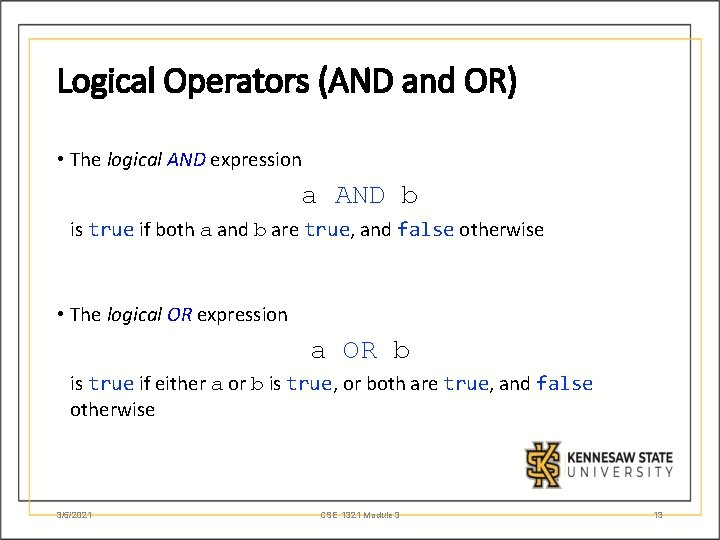
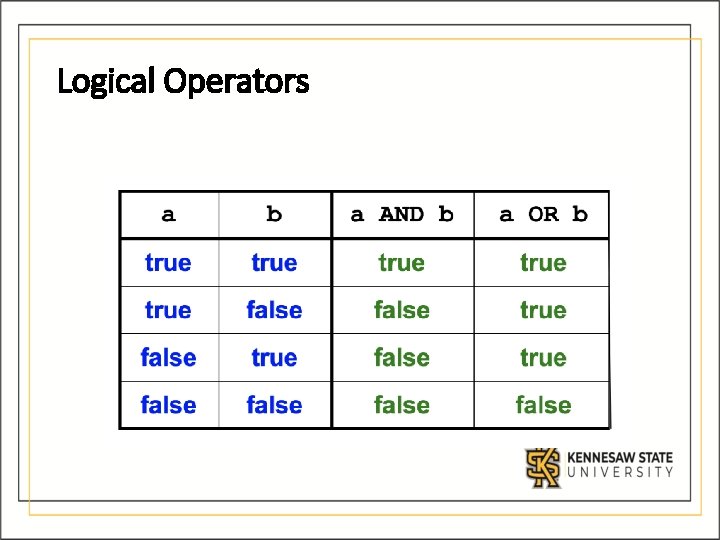
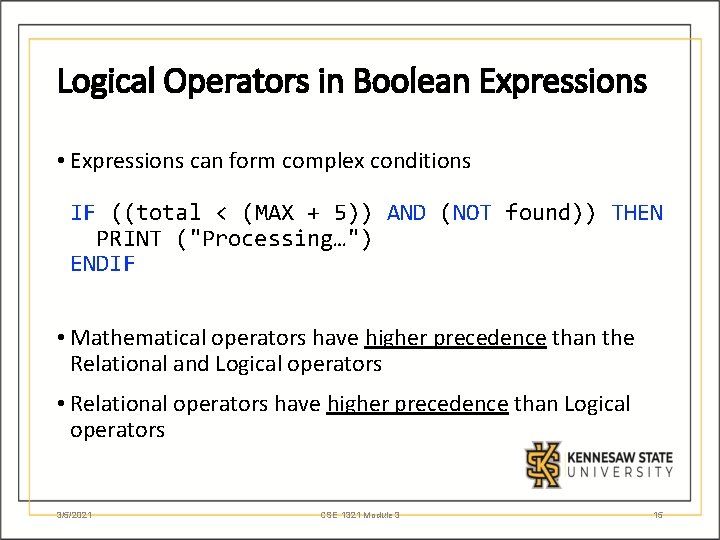
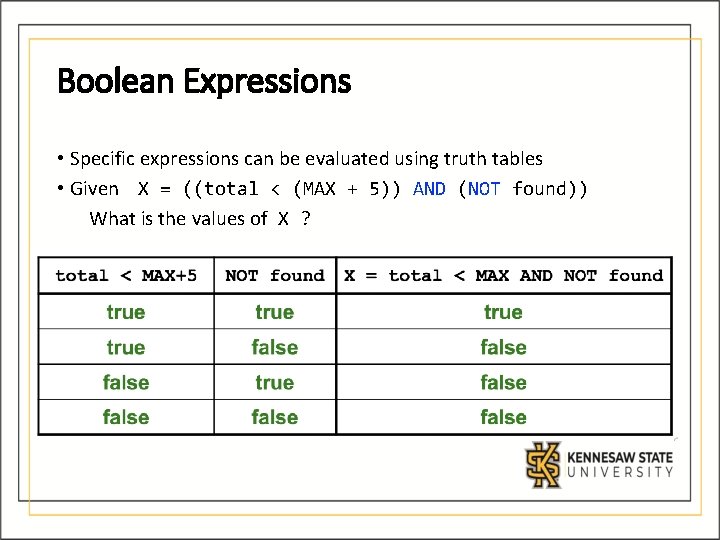
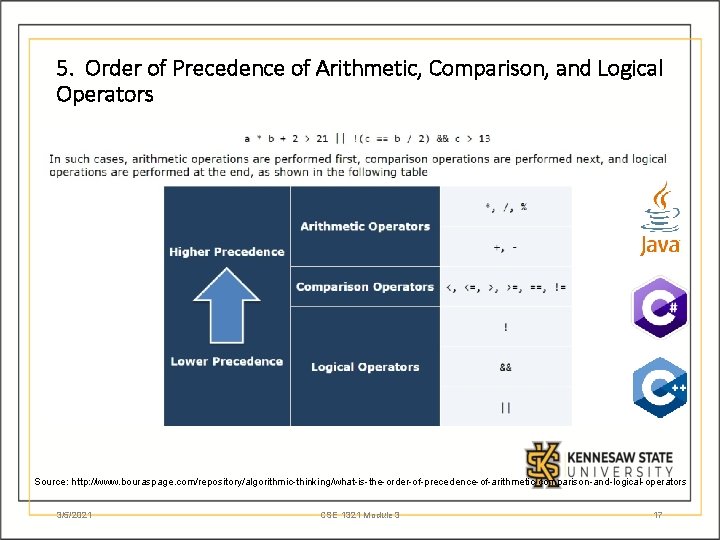
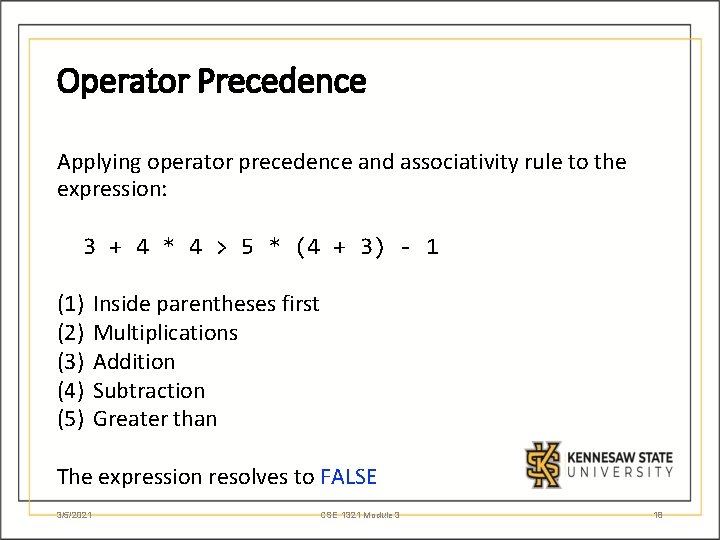
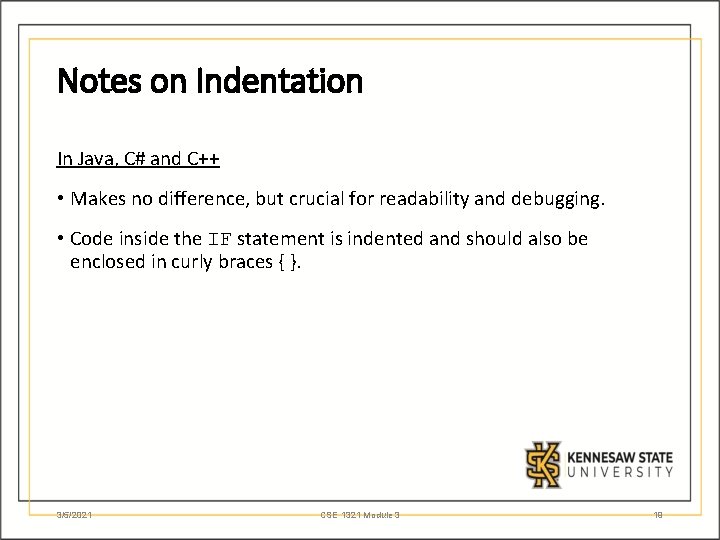
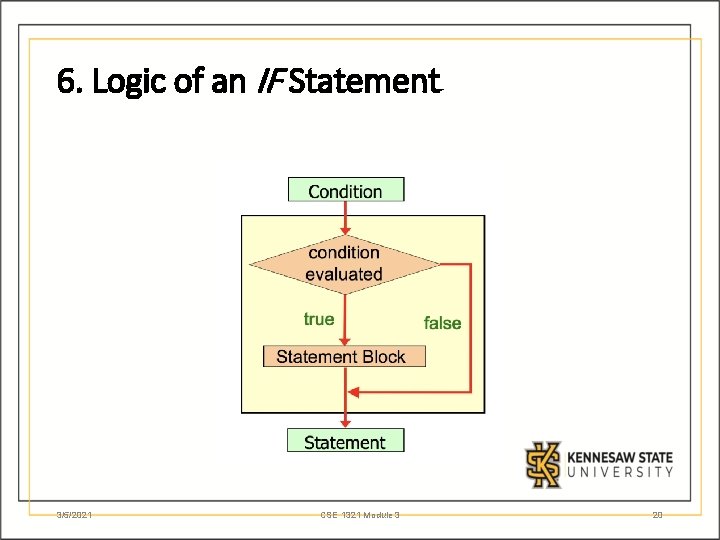
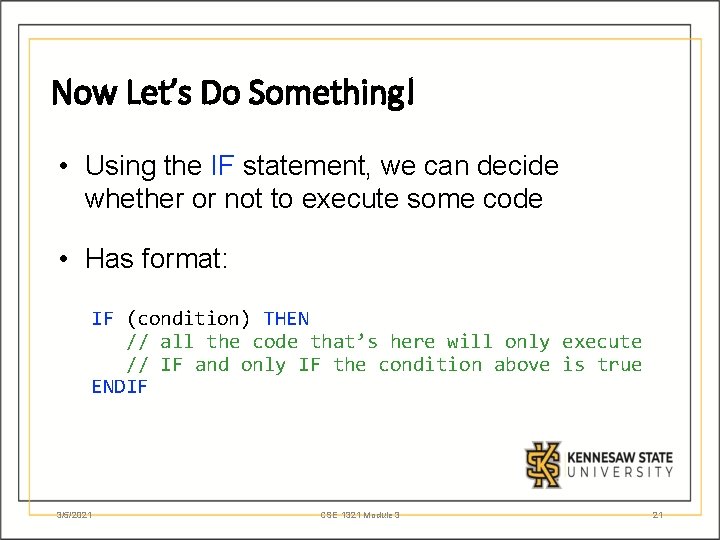
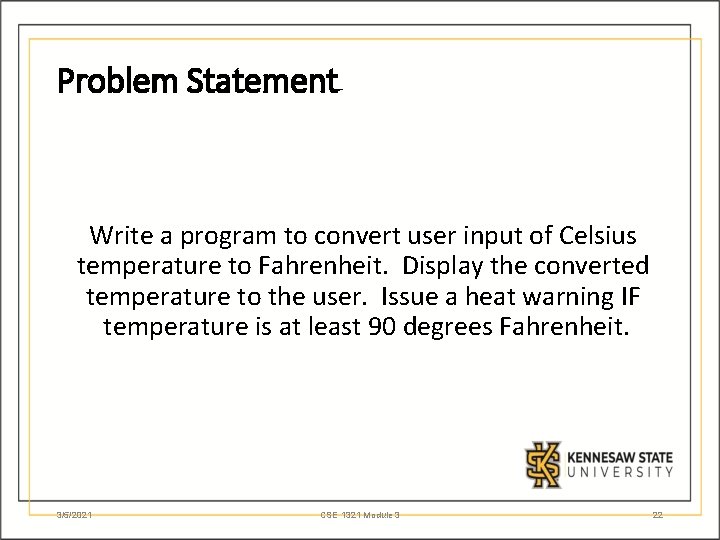
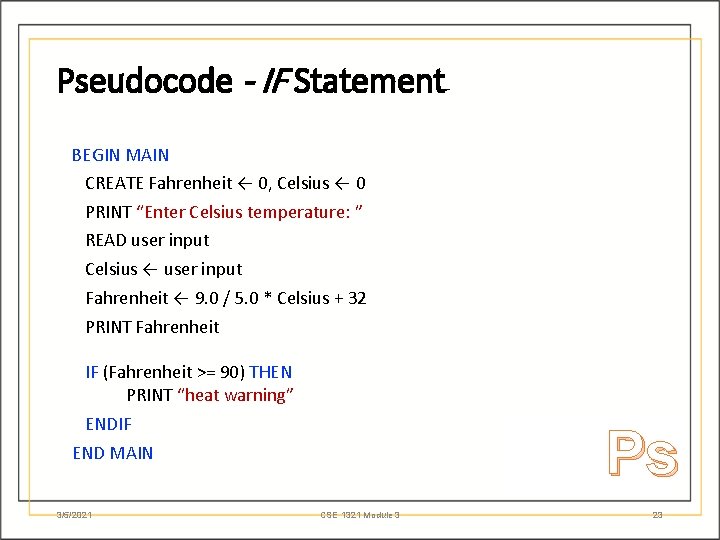
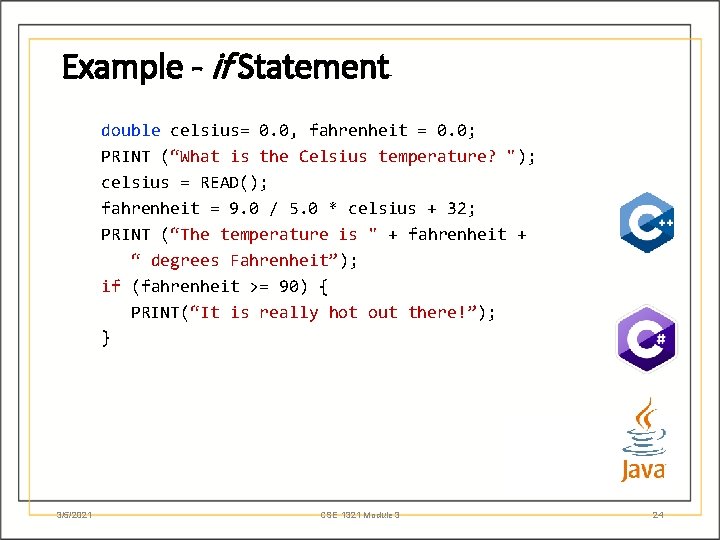
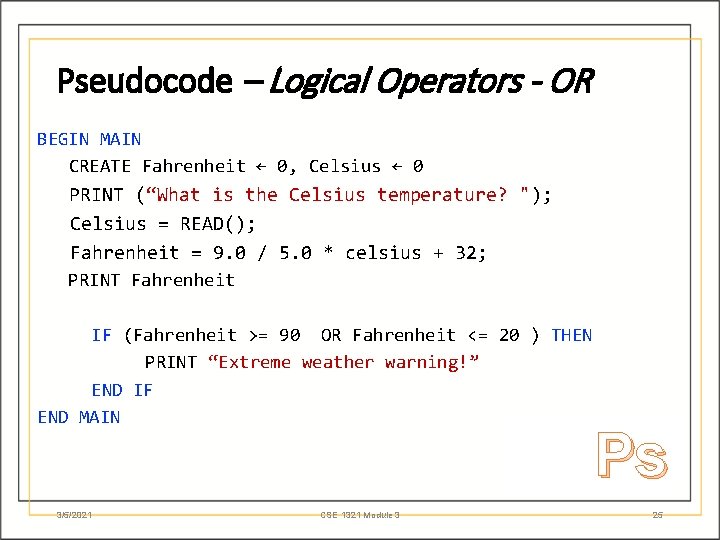
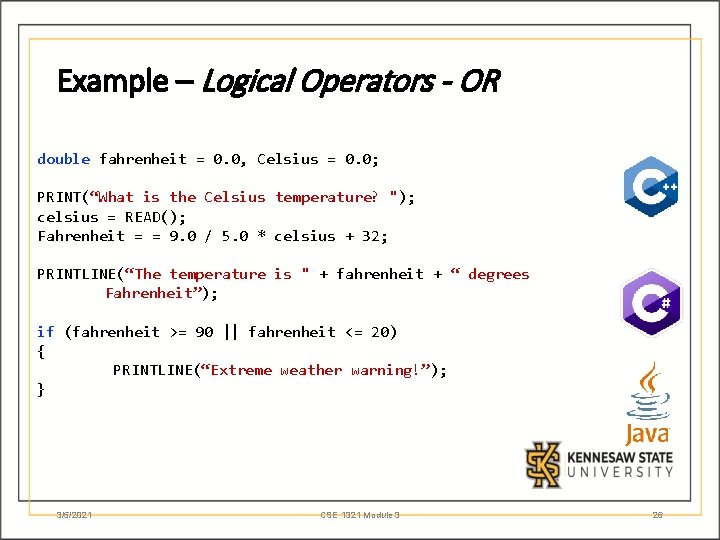
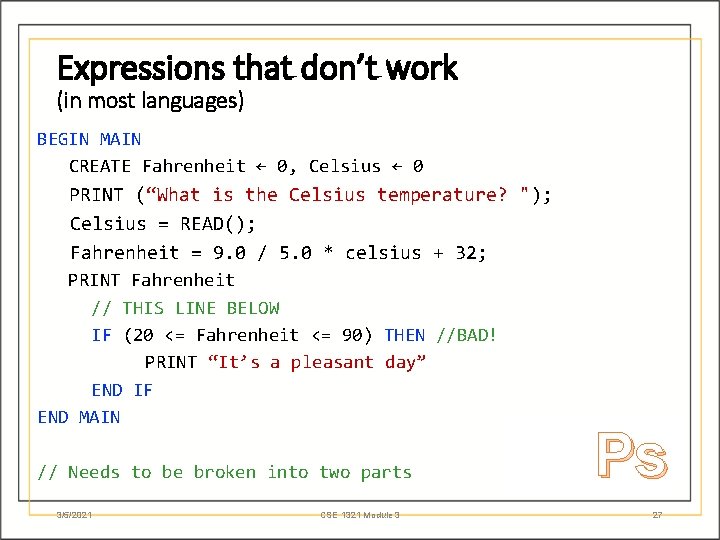
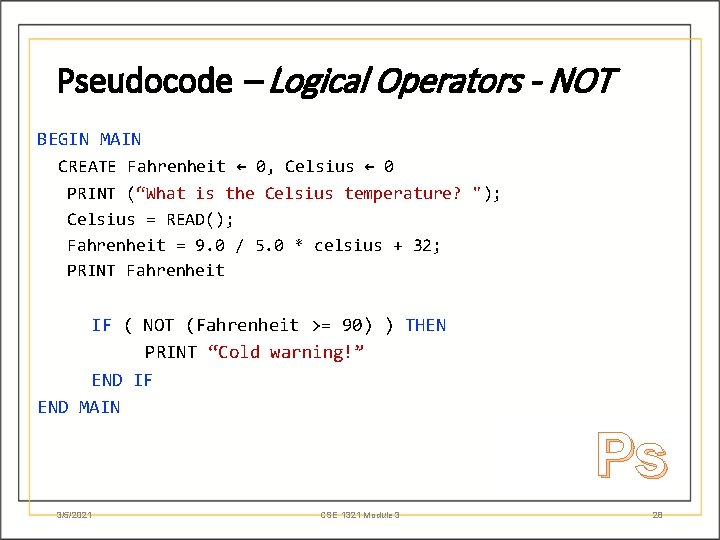
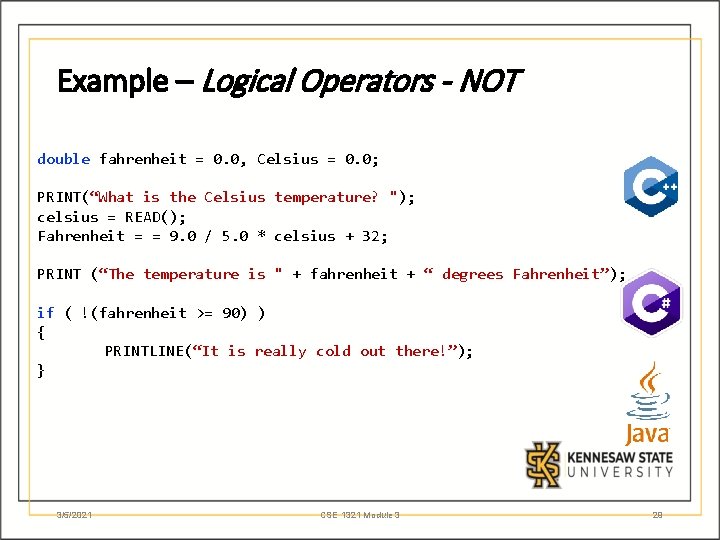
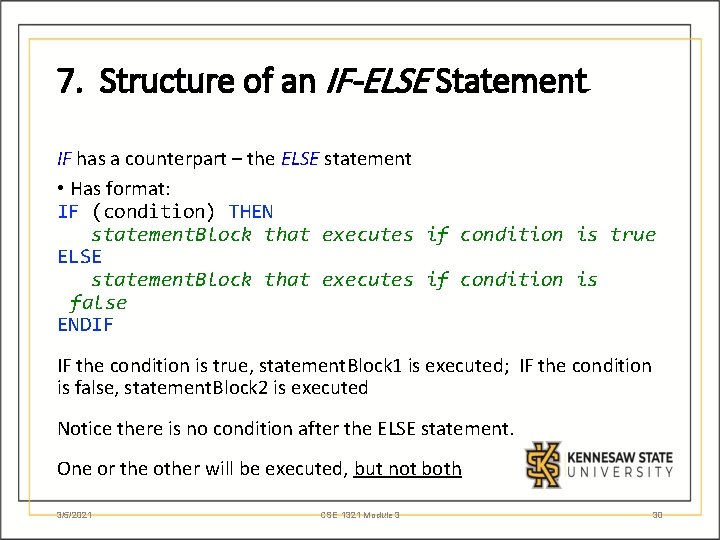
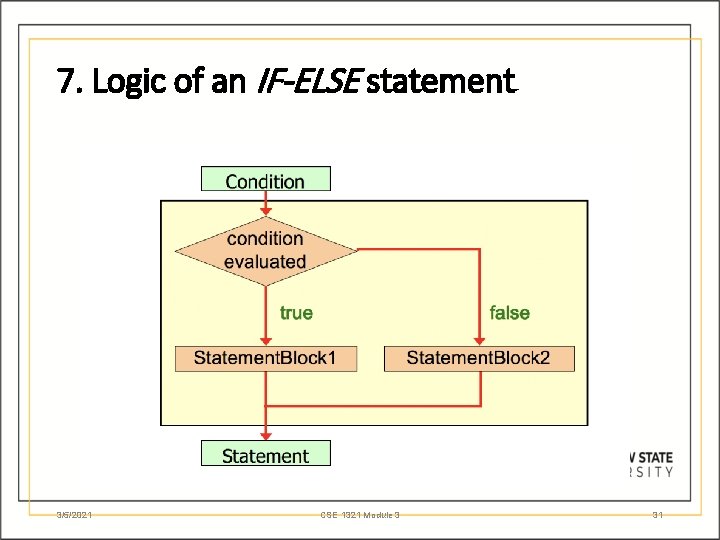
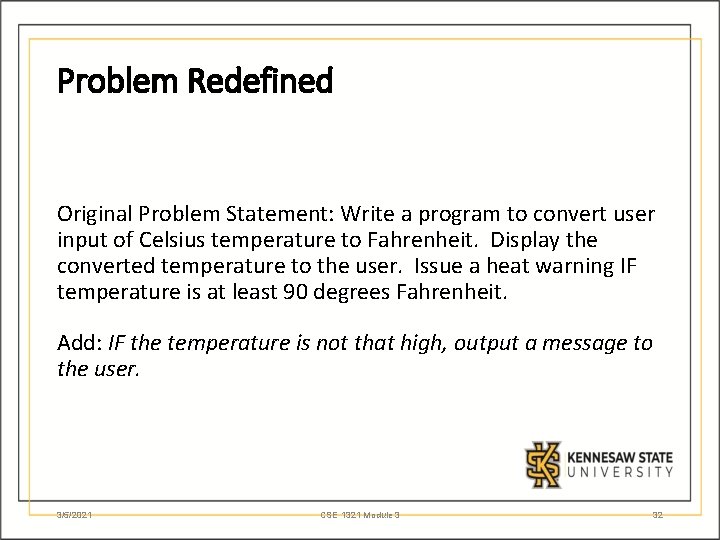
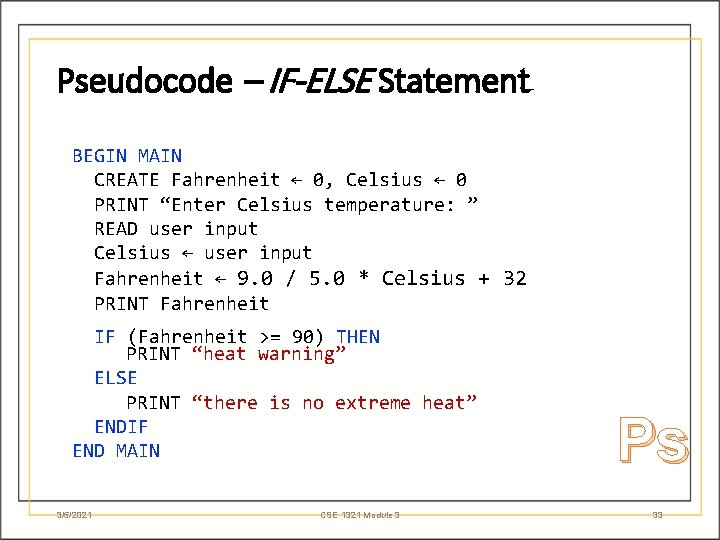
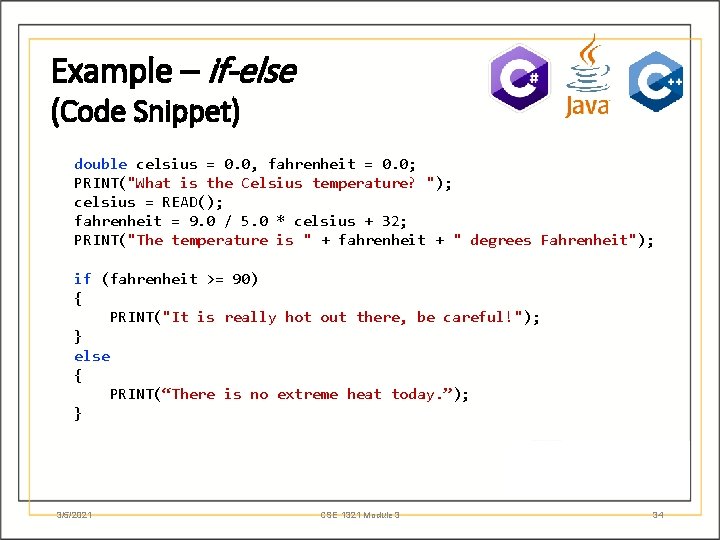
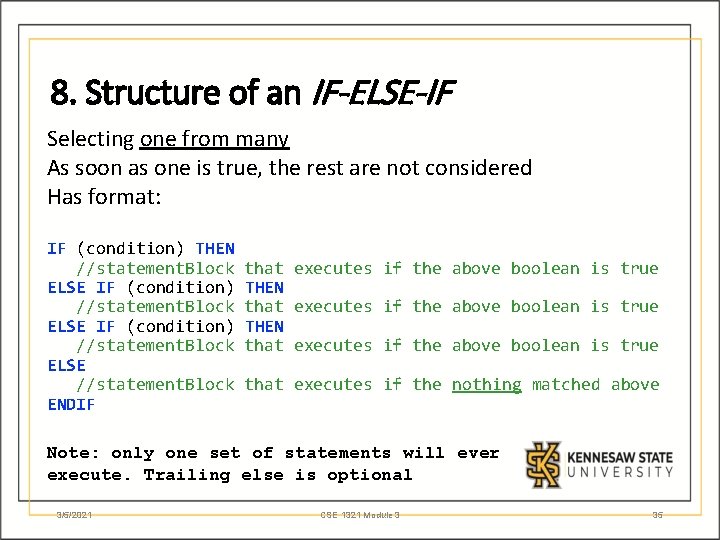
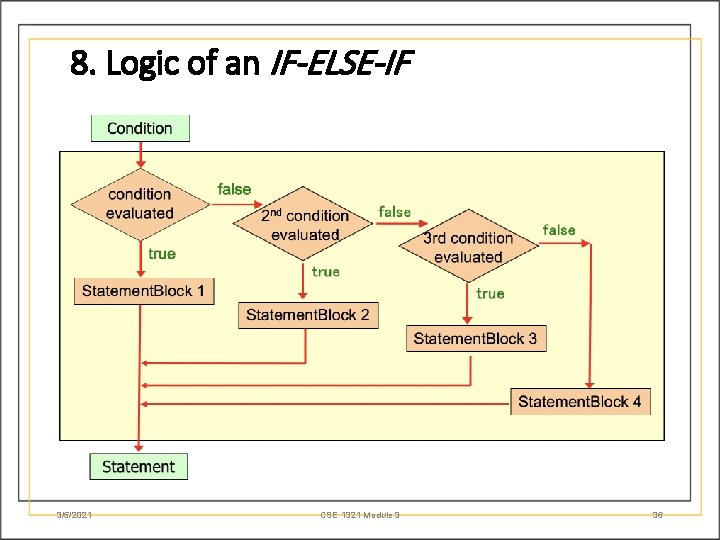
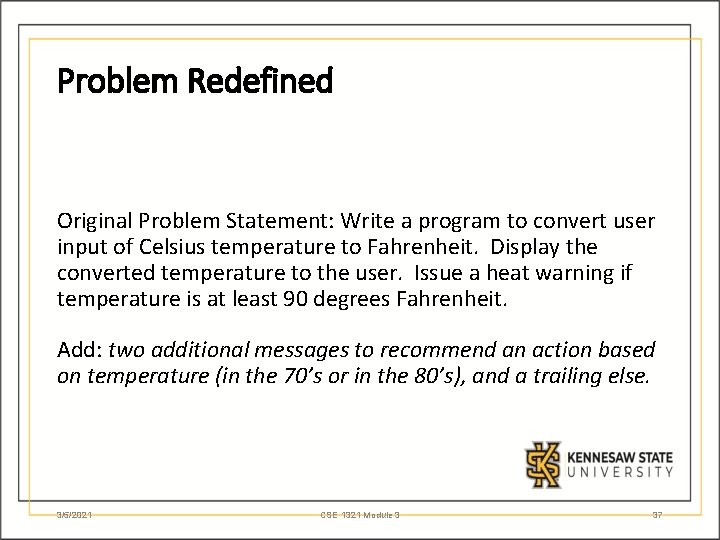
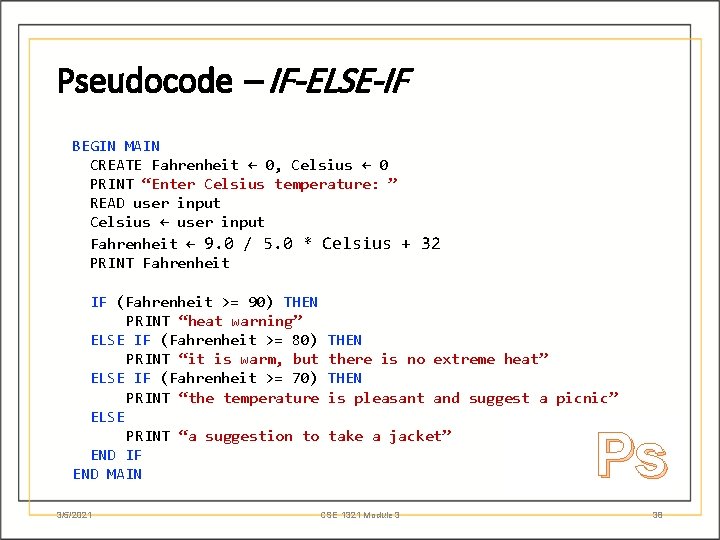
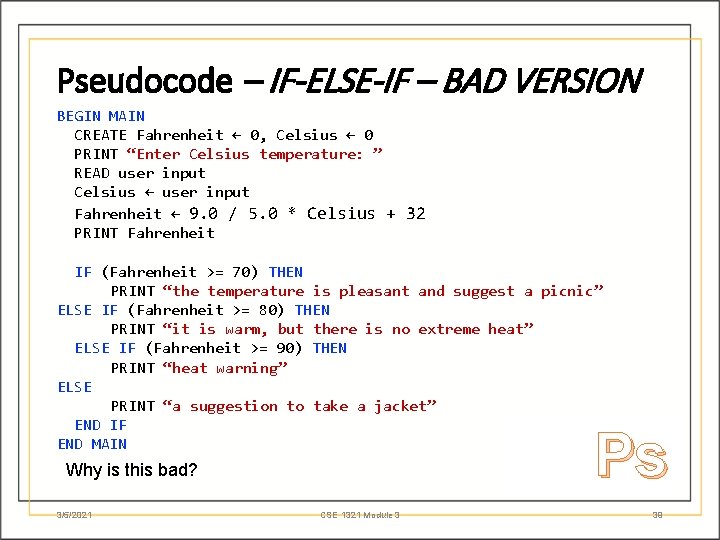
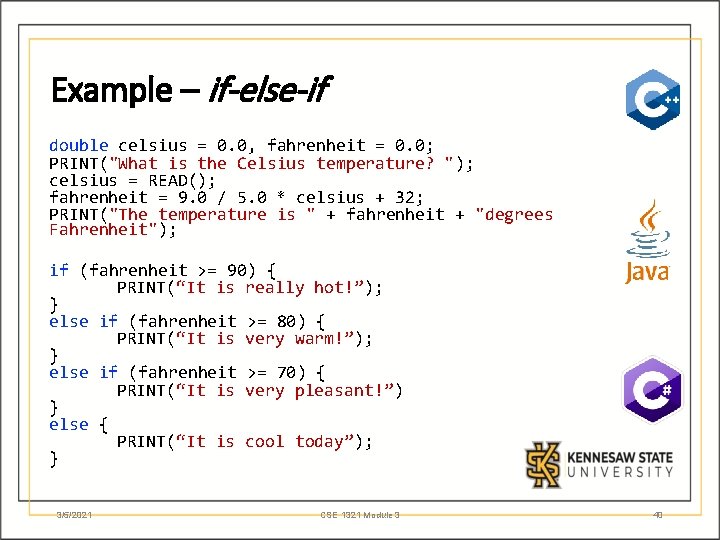
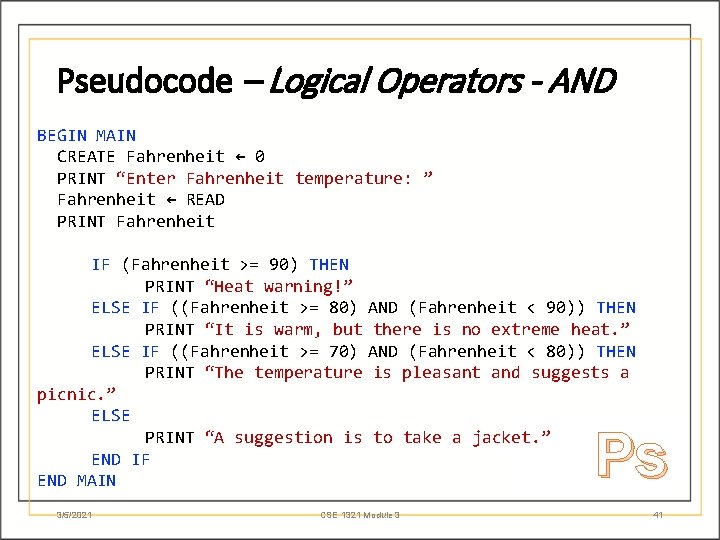
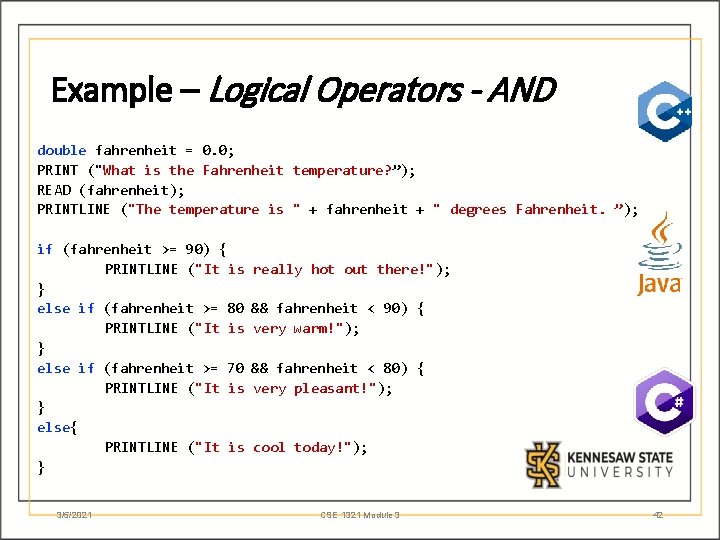
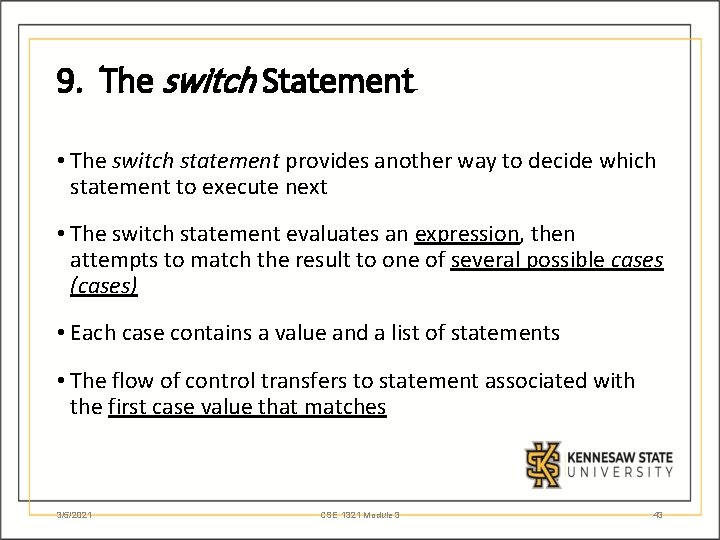
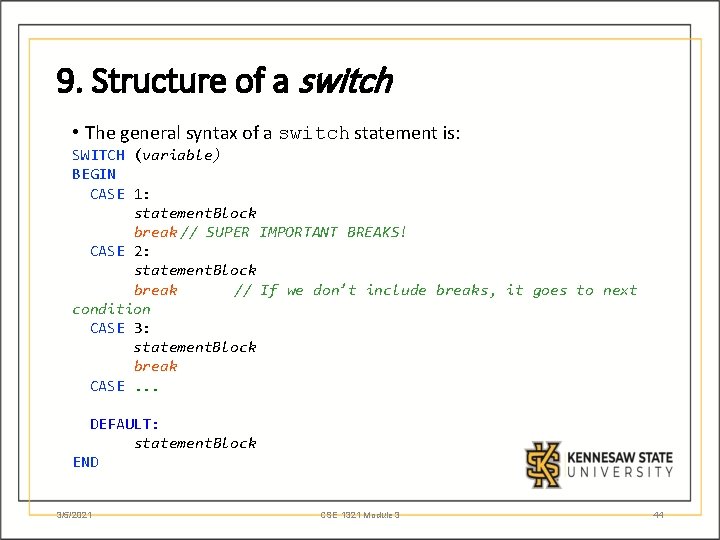
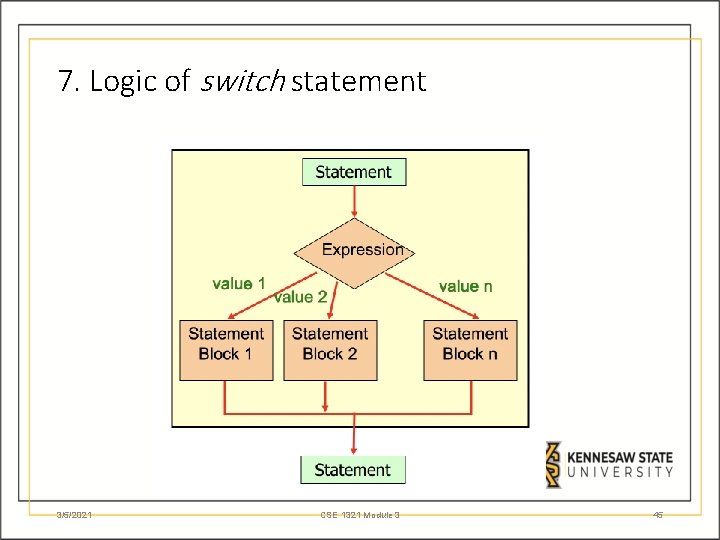
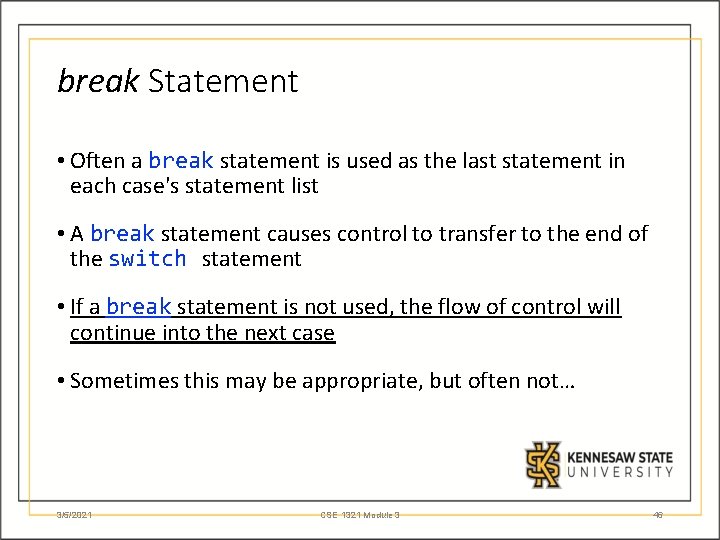
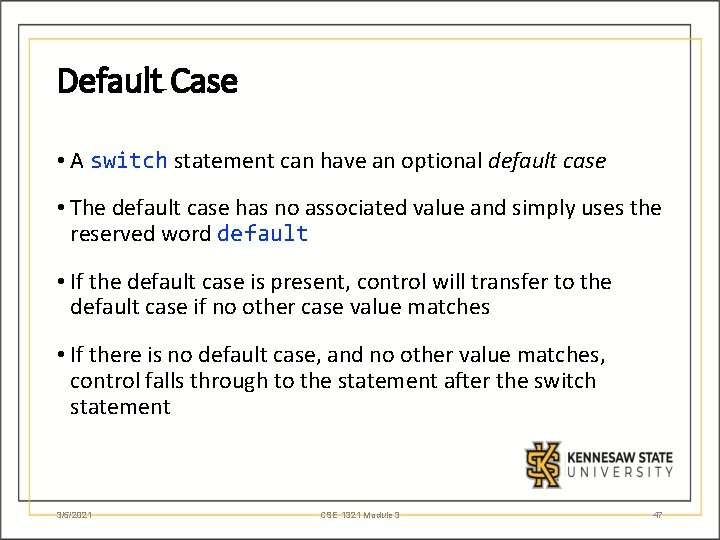
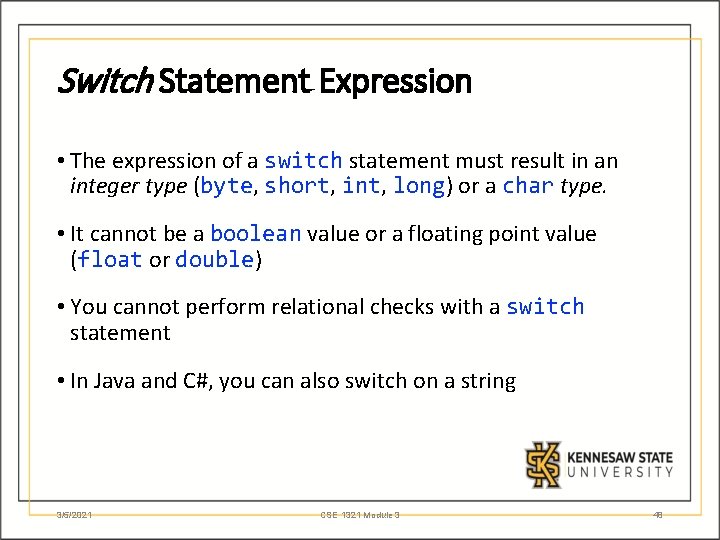
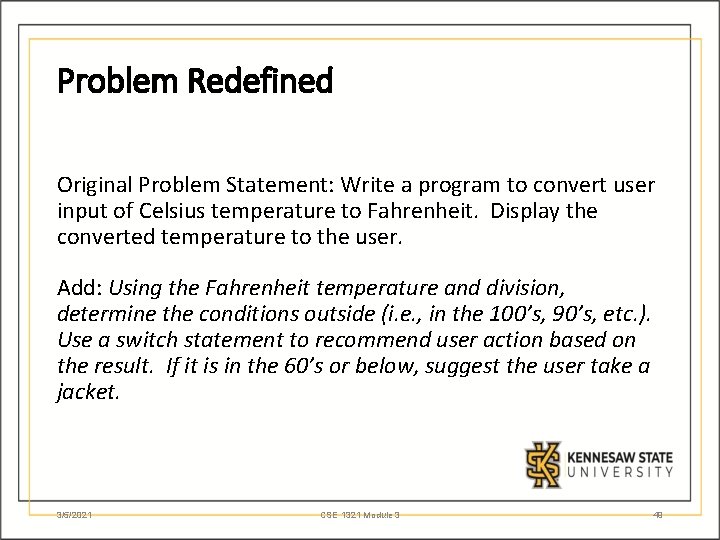
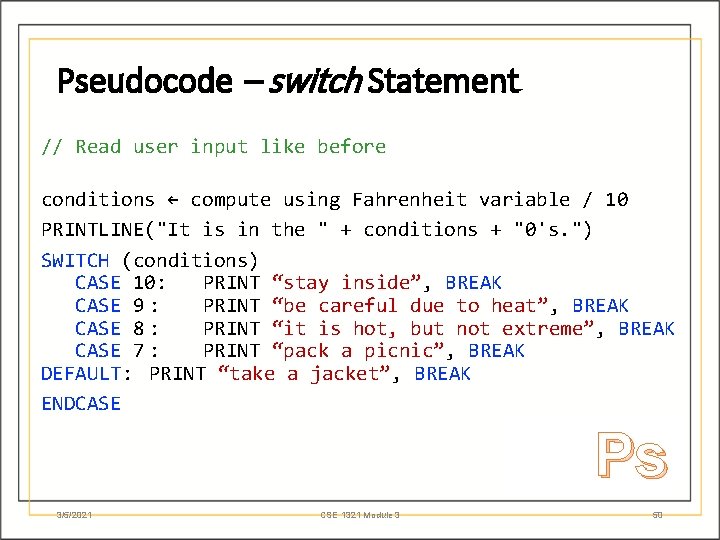
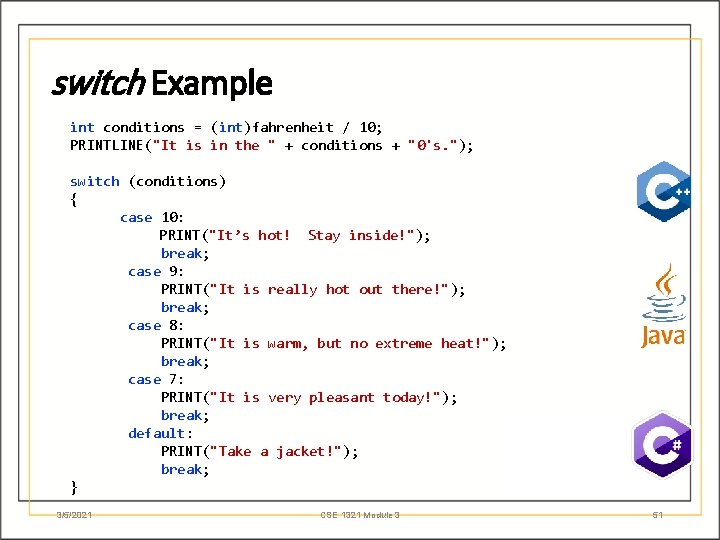
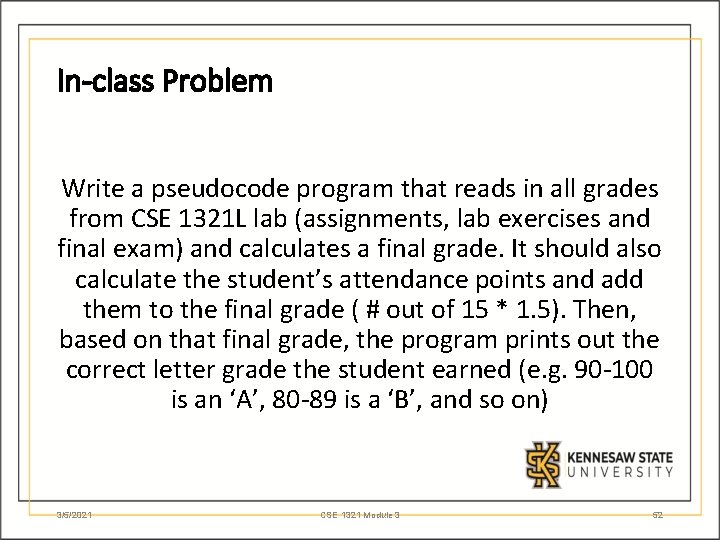
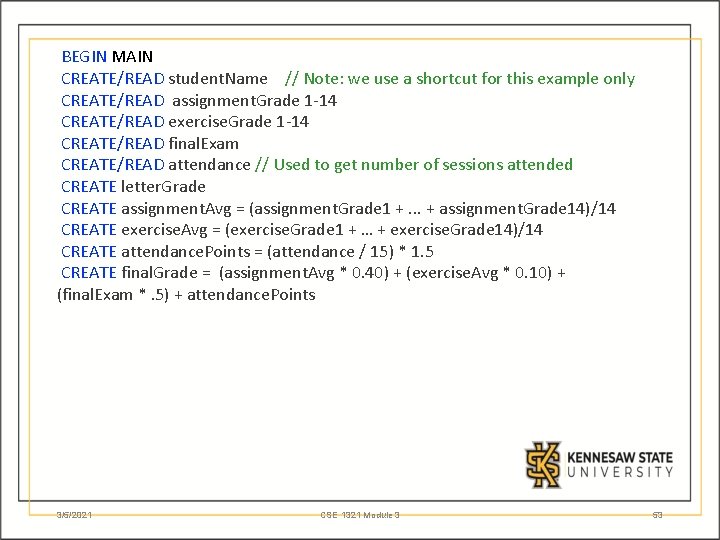
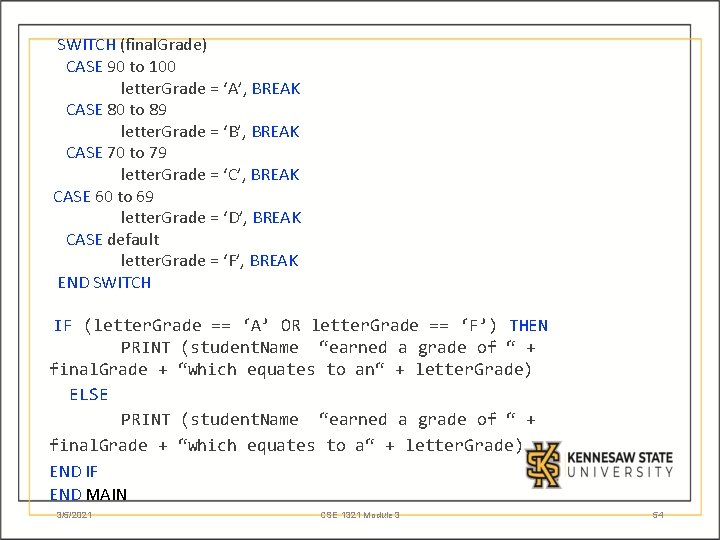
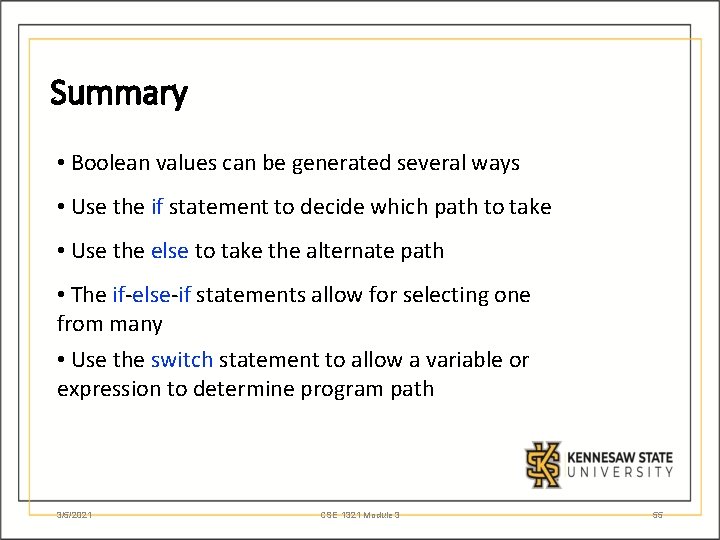
- Slides: 55
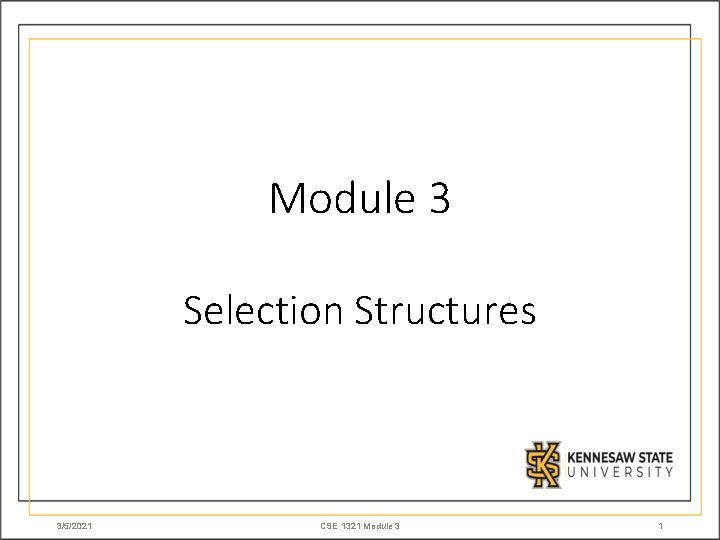
Module 3 Selection Structures 3/5/2021 CSE 1321 Module 3 1
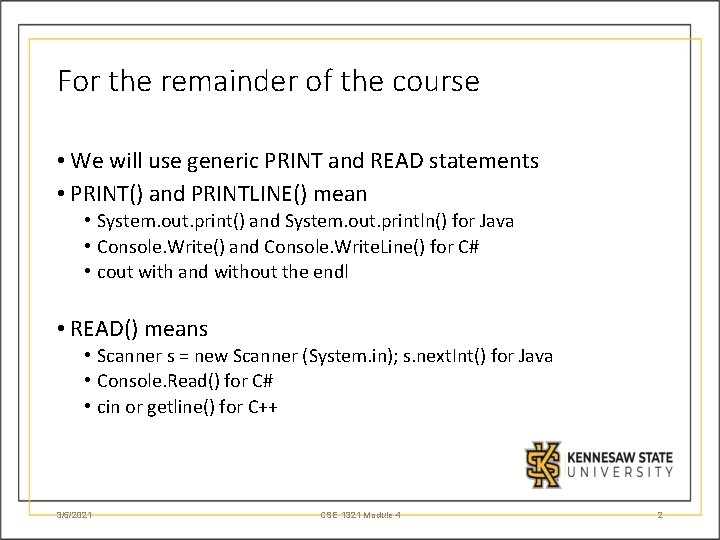
For the remainder of the course • We will use generic PRINT and READ statements • PRINT() and PRINTLINE() mean • System. out. print() and System. out. println() for Java • Console. Write() and Console. Write. Line() for C# • cout with and without the endl • READ() means • Scanner s = new Scanner (System. in); s. next. Int() for Java • Console. Read() for C# • cin or getline() for C++ 3/5/2021 CSE 1321 Module 4 2
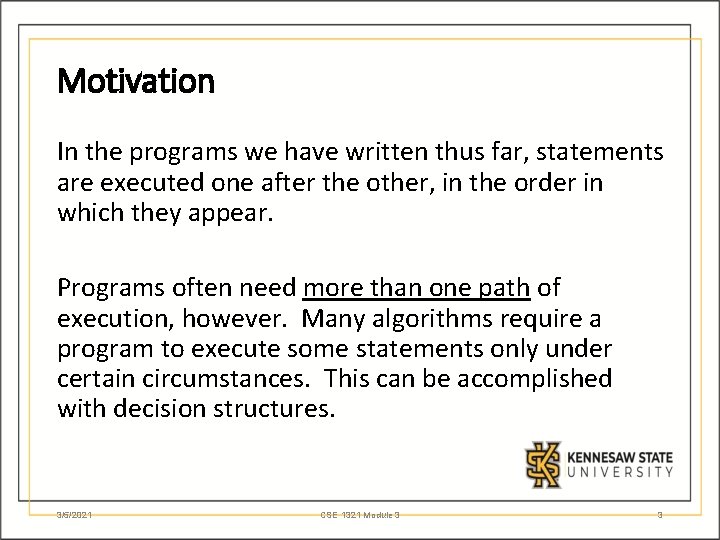
Motivation In the programs we have written thus far, statements are executed one after the other, in the order in which they appear. Programs often need more than one path of execution, however. Many algorithms require a program to execute some statements only under certain circumstances. This can be accomplished with decision structures. 3/5/2021 CSE 1321 Module 3 3
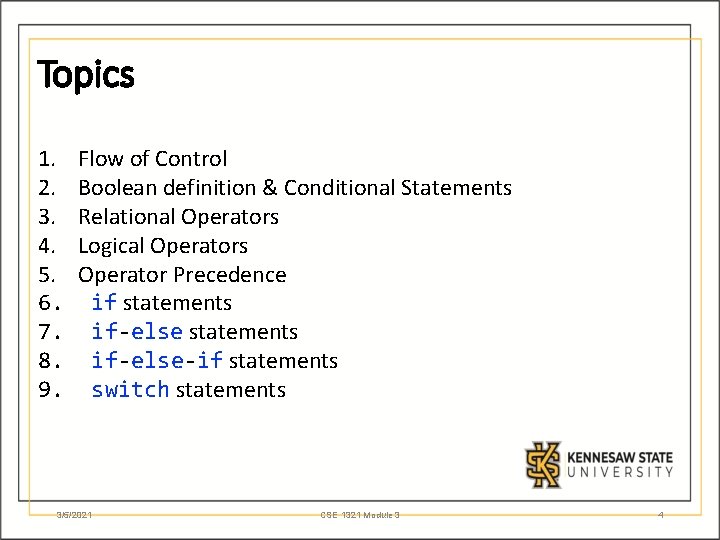
Topics 1. 2. 3. 4. 5. 6. 7. 8. 9. Flow of Control Boolean definition & Conditional Statements Relational Operators Logical Operators Operator Precedence if statements if-else-if statements switch statements 3/5/2021 CSE 1321 Module 3 4
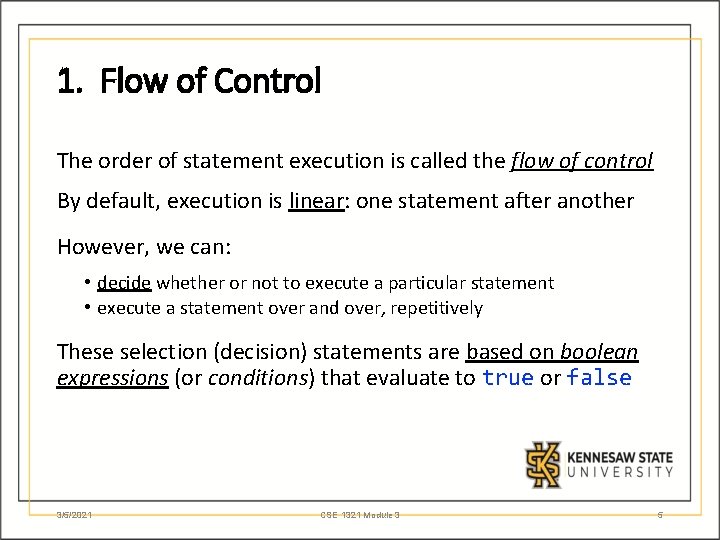
1. Flow of Control The order of statement execution is called the flow of control By default, execution is linear: one statement after another However, we can: • decide whether or not to execute a particular statement • execute a statement over and over, repetitively These selection (decision) statements are based on boolean expressions (or conditions) that evaluate to true or false 3/5/2021 CSE 1321 Module 3 5
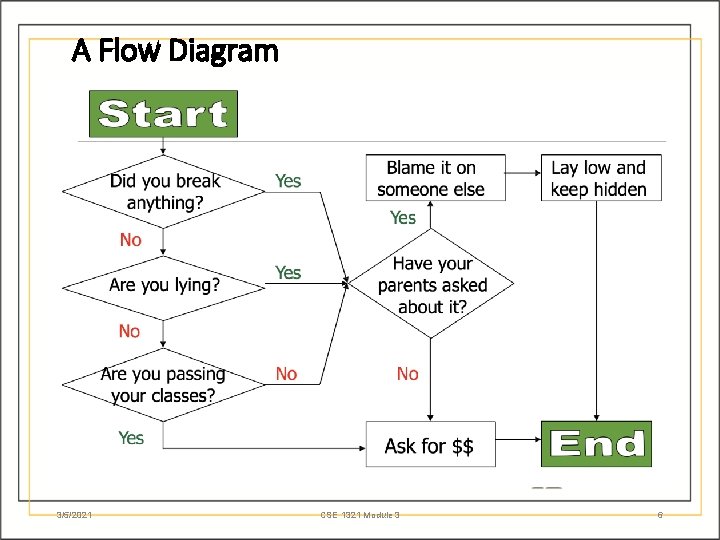
A Flow Diagram 3/5/2021 CSE 1321 Module 3 6
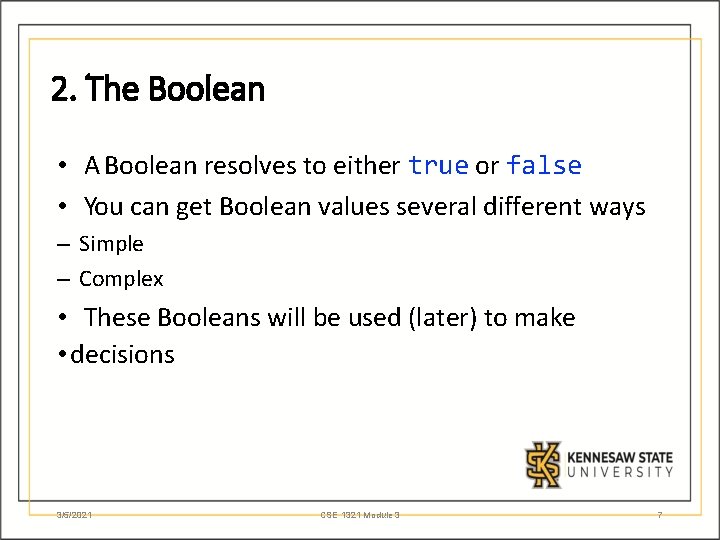
2. The Boolean • A Boolean resolves to either true or false • You can get Boolean values several different ways – Simple – Complex • These Booleans will be used (later) to make • decisions 3/5/2021 CSE 1321 Module 3 7
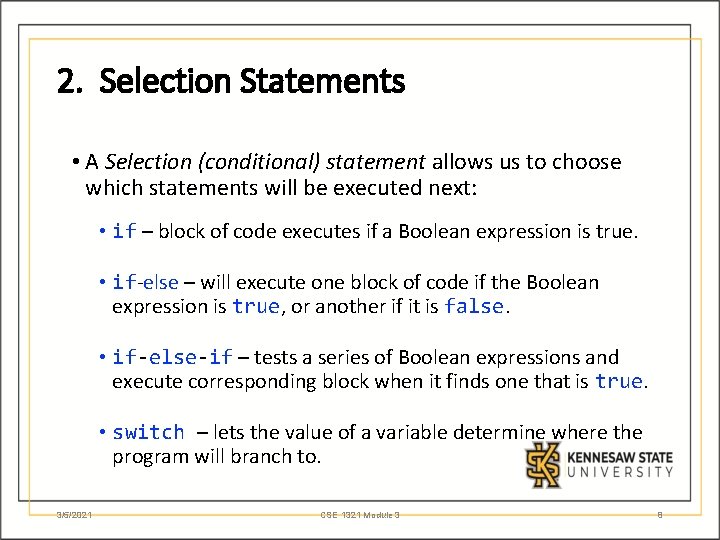
2. Selection Statements • A Selection (conditional) statement allows us to choose which statements will be executed next: • if – block of code executes if a Boolean expression is true. • if-else – will execute one block of code if the Boolean expression is true, or another if it is false. • if-else-if – tests a series of Boolean expressions and execute corresponding block when it finds one that is true. • switch – lets the value of a variable determine where the program will branch to. 3/5/2021 CSE 1321 Module 3 8
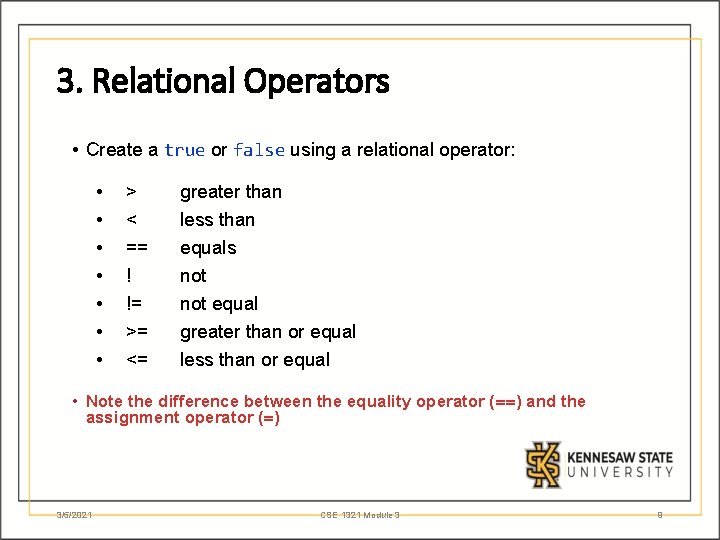
3. Relational Operators • Create a true or false using a relational operator: • • > < == ! != >= <= greater than less than equals not equal greater than or equal less than or equal • Note the difference between the equality operator (==) and the assignment operator (=) 3/5/2021 CSE 1321 Module 3 9
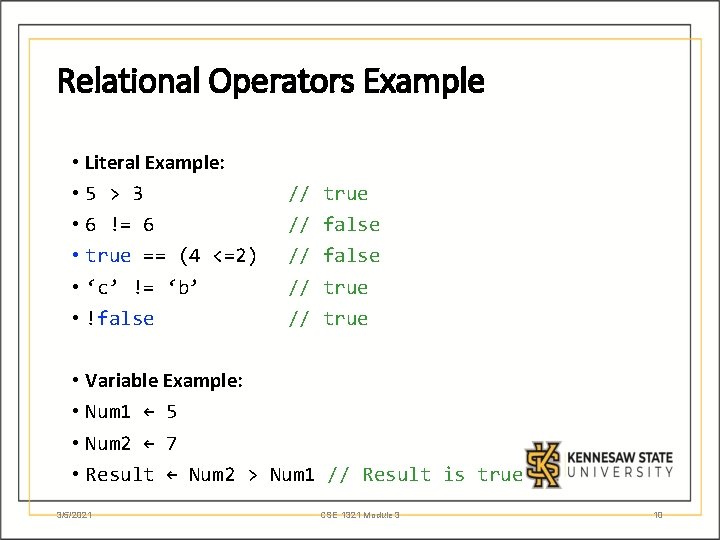
Relational Operators Example • Literal Example: • 5 > 3 • 6 != 6 • true == (4 <=2) • ‘c’ != ‘b’ • !false // // // true false true • Variable Example: • Num 1 ← 5 • Num 2 ← 7 • Result ← Num 2 > Num 1 // Result is true 3/5/2021 CSE 1321 Module 3 10
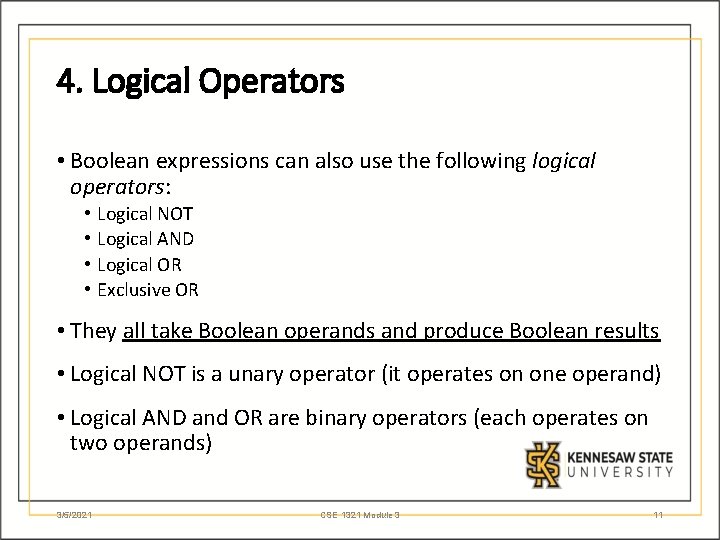
4. Logical Operators • Boolean expressions can also use the following logical operators: • • Logical NOT Logical AND Logical OR Exclusive OR • They all take Boolean operands and produce Boolean results • Logical NOT is a unary operator (it operates on one operand) • Logical AND and OR are binary operators (each operates on two operands) 3/5/2021 CSE 1321 Module 3 11
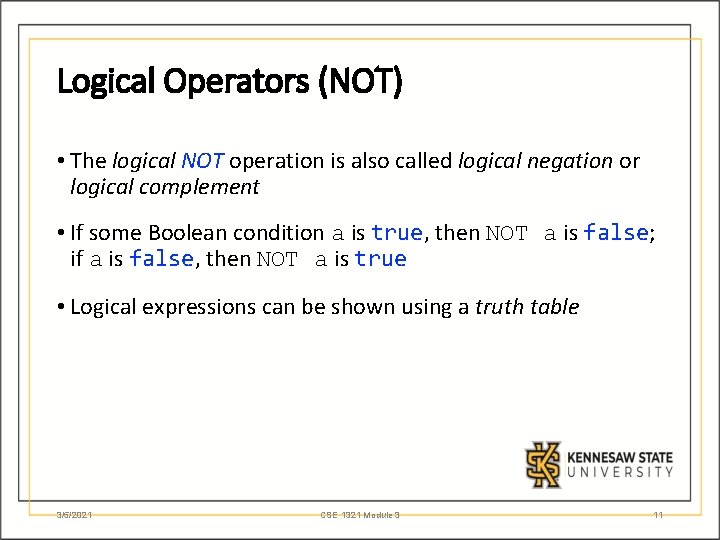
Logical Operators (NOT) • The logical NOT operation is also called logical negation or logical complement • If some Boolean condition a is true, then NOT a is false; if a is false, then NOT a is true • Logical expressions can be shown using a truth table 3/5/2021 CSE 1321 Module 3 11
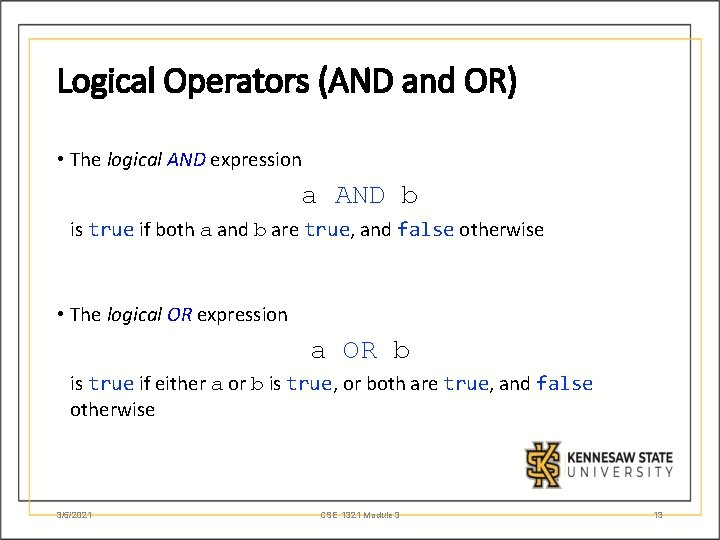
Logical Operators (AND and OR) • The logical AND expression a AND b is true if both a and b are true, and false otherwise • The logical OR expression a OR b is true if either a or b is true, or both are true, and false otherwise 3/5/2021 CSE 1321 Module 3 13
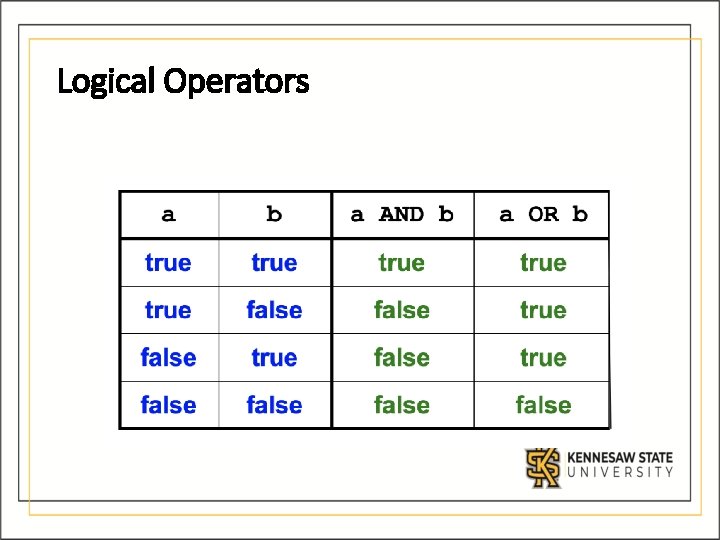
Logical Operators
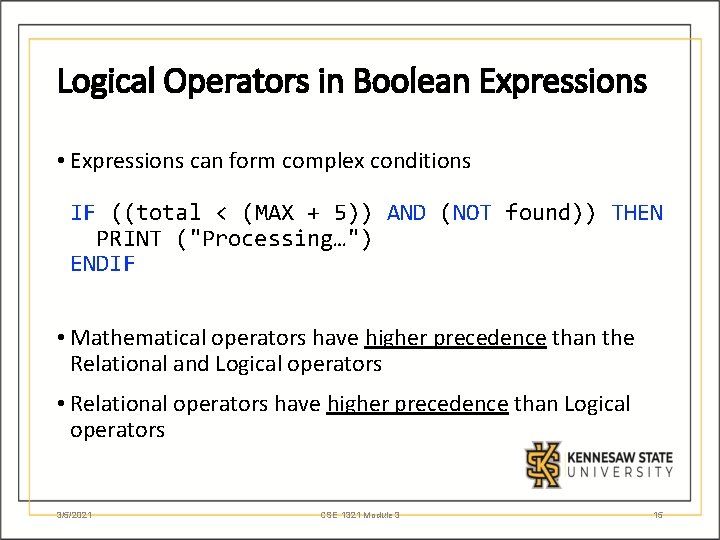
Logical Operators in Boolean Expressions • Expressions can form complex conditions IF ((total < (MAX + 5)) AND (NOT found)) THEN PRINT ("Processing…") ENDIF • Mathematical operators have higher precedence than the Relational and Logical operators • Relational operators have higher precedence than Logical operators 3/5/2021 CSE 1321 Module 3 15
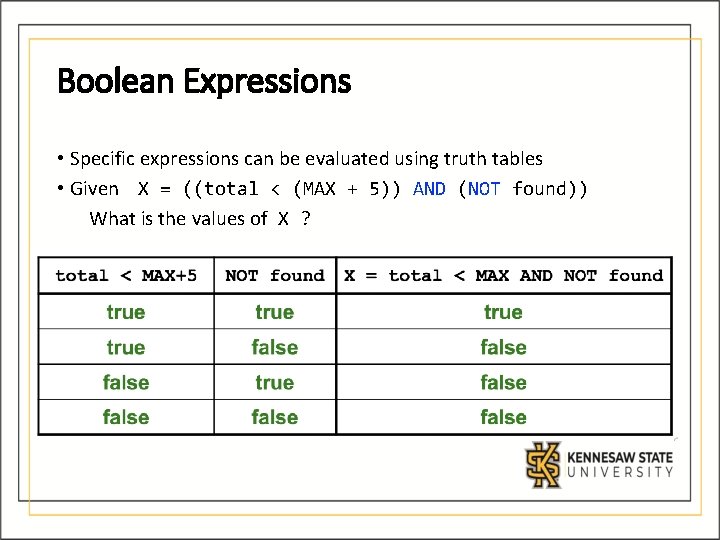
Boolean Expressions • Specific expressions can be evaluated using truth tables • Given X = ((total < (MAX + 5)) AND (NOT found)) What is the values of X ?
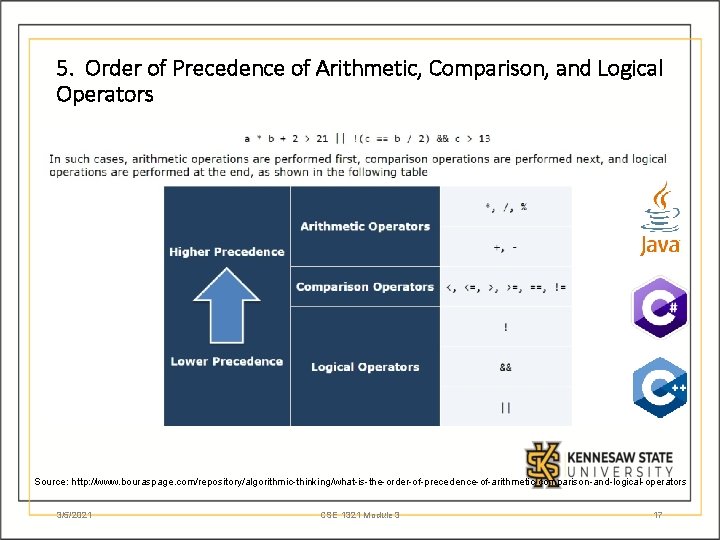
5. Order of Precedence of Arithmetic, Comparison, and Logical Operators Source: http: //www. bouraspage. com/repository/algorithmic-thinking/what-is-the-order-of-precedence-of-arithmetic-comparison-and-logical-operators 3/5/2021 CSE 1321 Module 3 17
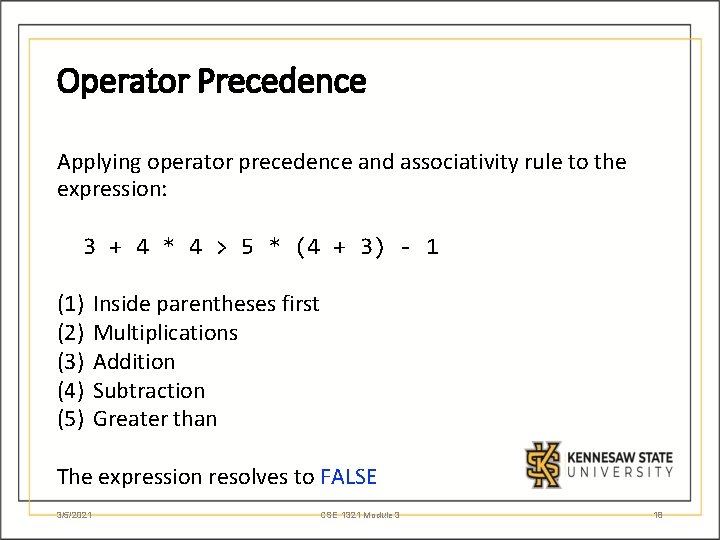
Operator Precedence Applying operator precedence and associativity rule to the expression: 3 + 4 * 4 > 5 * (4 + 3) - 1 (1) (2) (3) (4) (5) Inside parentheses first Multiplications Addition Subtraction Greater than The expression resolves to FALSE 3/5/2021 CSE 1321 Module 3 18
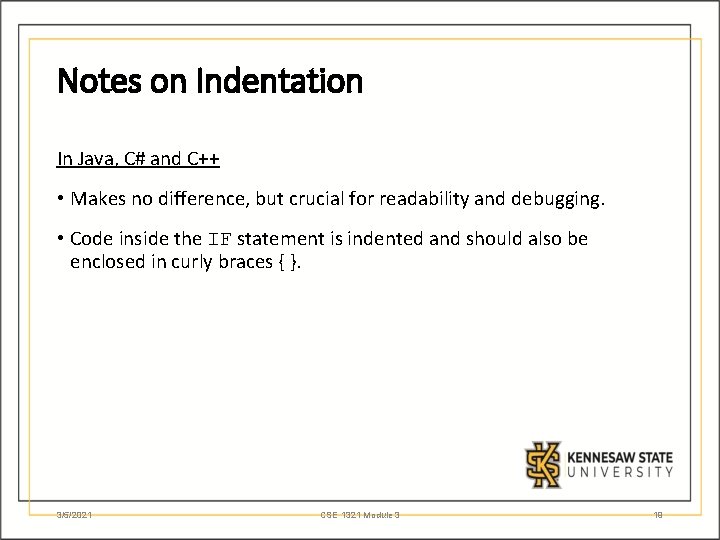
Notes on Indentation In Java, C# and C++ • Makes no difference, but crucial for readability and debugging. • Code inside the IF statement is indented and should also be enclosed in curly braces { }. 3/5/2021 CSE 1321 Module 3 19
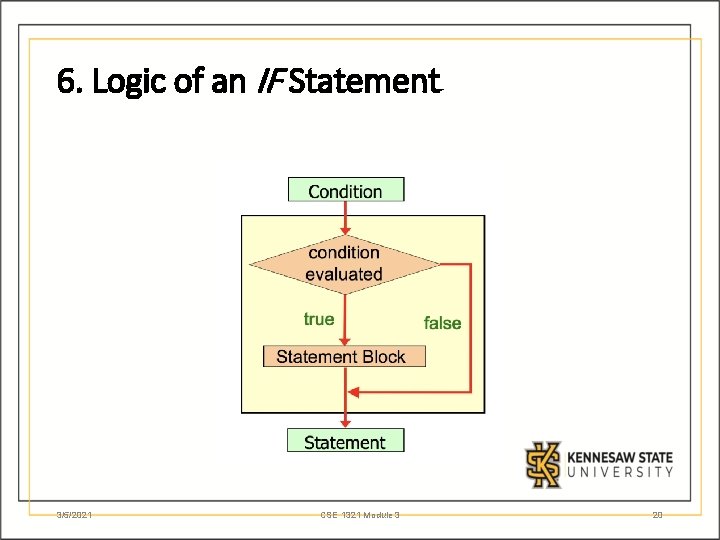
6. Logic of an IF Statement 3/5/2021 CSE 1321 Module 3 20
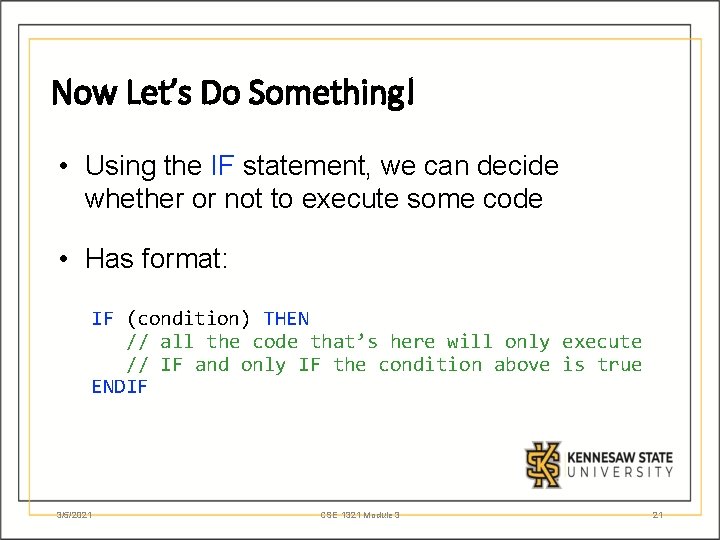
Now Let’s Do Something! • Using the IF statement, we can decide whether or not to execute some code • Has format: IF (condition) THEN // all the code that’s here will only execute // IF and only IF the condition above is true ENDIF 3/5/2021 CSE 1321 Module 3 21
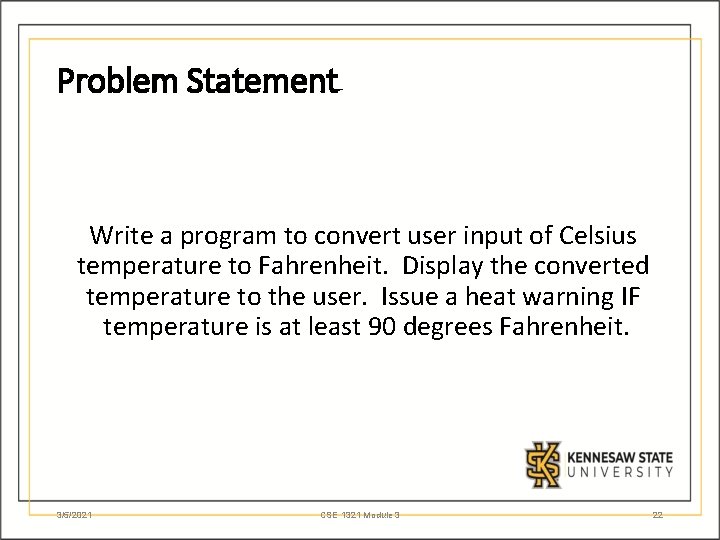
Problem Statement Write a program to convert user input of Celsius temperature to Fahrenheit. Display the converted temperature to the user. Issue a heat warning IF temperature is at least 90 degrees Fahrenheit. 3/5/2021 CSE 1321 Module 3 22
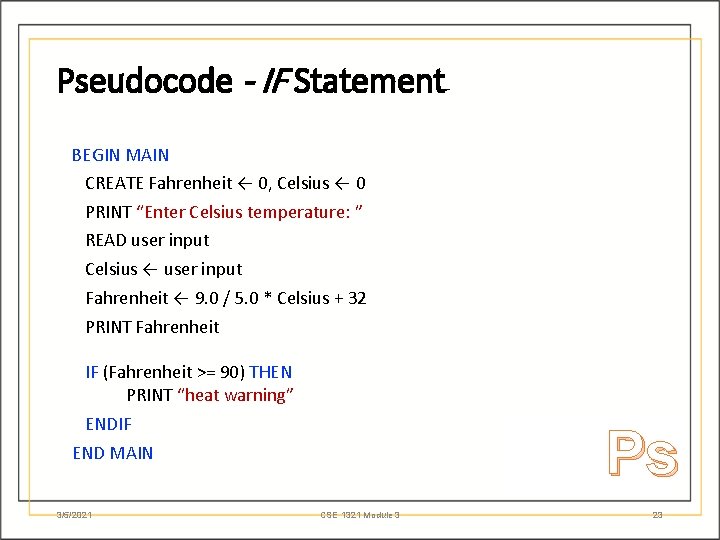
Pseudocode - IF Statement BEGIN MAIN CREATE Fahrenheit ← 0, Celsius ← 0 PRINT “Enter Celsius temperature: ” READ user input Celsius ← user input Fahrenheit ← 9. 0 / 5. 0 * Celsius + 32 PRINT Fahrenheit IF (Fahrenheit >= 90) THEN PRINT “heat warning” ENDIF Ps END MAIN 3/5/2021 CSE 1321 Module 3 23
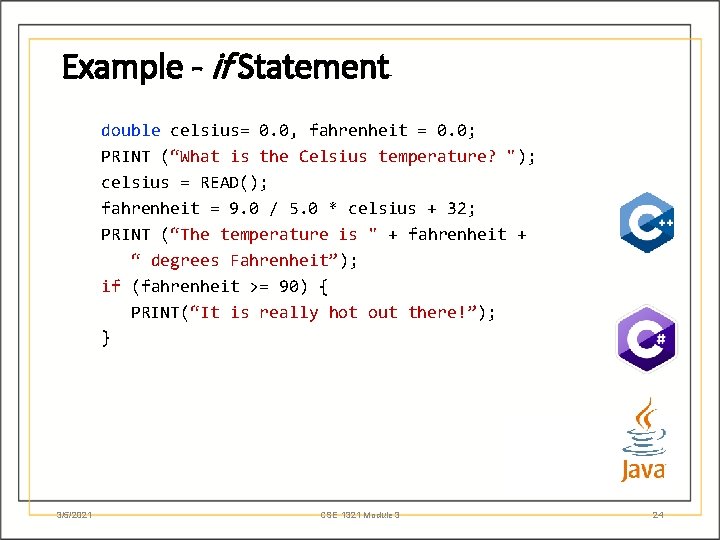
Example - if Statement double celsius= 0. 0, fahrenheit = 0. 0; PRINT (“What is the Celsius temperature? "); celsius = READ(); fahrenheit = 9. 0 / 5. 0 * celsius + 32; PRINT (“The temperature is " + fahrenheit + “ degrees Fahrenheit”); if (fahrenheit >= 90) { PRINT(“It is really hot out there!”); } 3/5/2021 CSE 1321 Module 3 24
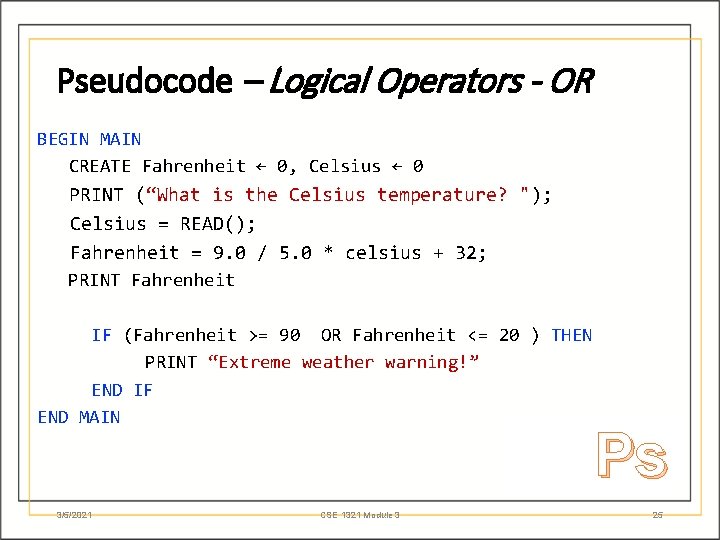
Pseudocode – Logical Operators - OR BEGIN MAIN CREATE Fahrenheit ← 0, Celsius ← 0 PRINT (“What is the Celsius temperature? "); Celsius = READ(); Fahrenheit = 9. 0 / 5. 0 * celsius + 32; PRINT Fahrenheit IF (Fahrenheit >= 90 OR Fahrenheit <= 20 ) THEN PRINT “Extreme weather warning!” END IF END MAIN 3/5/2021 CSE 1321 Module 3 Ps 25
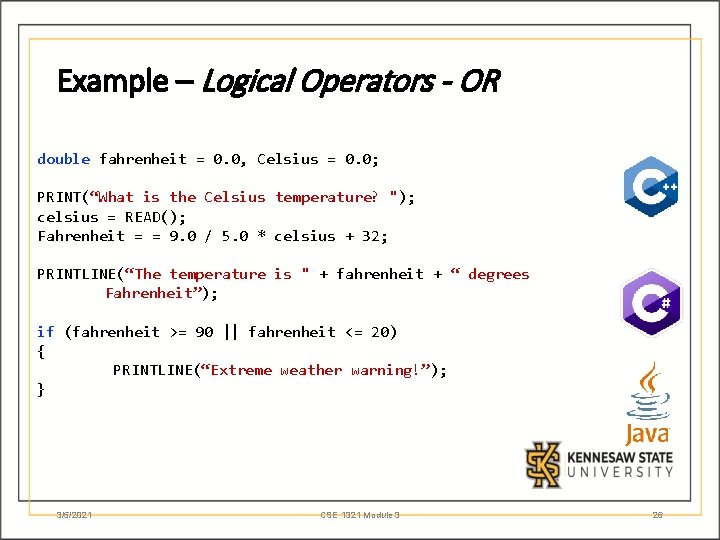
Example – Logical Operators - OR double fahrenheit = 0. 0, Celsius = 0. 0; PRINT(“What is the Celsius temperature? "); celsius = READ(); Fahrenheit = = 9. 0 / 5. 0 * celsius + 32; PRINTLINE(“The temperature is " + fahrenheit + “ degrees Fahrenheit”); if (fahrenheit >= 90 || fahrenheit <= 20) { PRINTLINE(“Extreme weather warning!”); } 3/5/2021 CSE 1321 Module 3 26
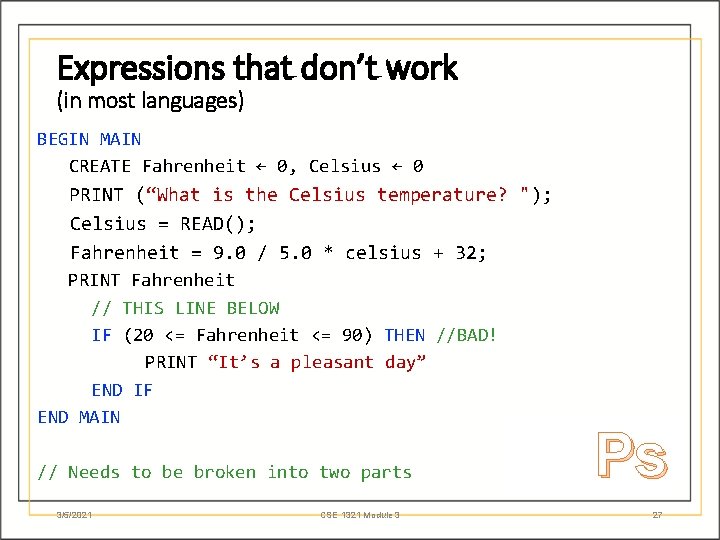
Expressions that don’t work (in most languages) BEGIN MAIN CREATE Fahrenheit ← 0, Celsius ← 0 PRINT (“What is the Celsius temperature? "); Celsius = READ(); Fahrenheit = 9. 0 / 5. 0 * celsius + 32; PRINT Fahrenheit // THIS LINE BELOW IF (20 <= Fahrenheit <= 90) THEN //BAD! PRINT “It’s a pleasant day” END IF END MAIN // Needs to be broken into two parts 3/5/2021 CSE 1321 Module 3 Ps 27
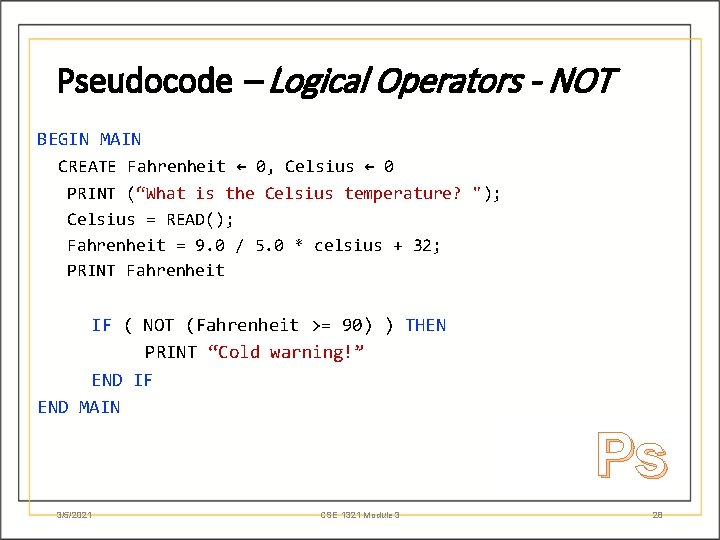
Pseudocode – Logical Operators - NOT BEGIN MAIN CREATE Fahrenheit ← 0, Celsius ← 0 PRINT (“What is the Celsius temperature? "); Celsius = READ(); Fahrenheit = 9. 0 / 5. 0 * celsius + 32; PRINT Fahrenheit IF ( NOT (Fahrenheit >= 90) ) THEN PRINT “Cold warning!” END IF END MAIN Ps 3/5/2021 CSE 1321 Module 3 28
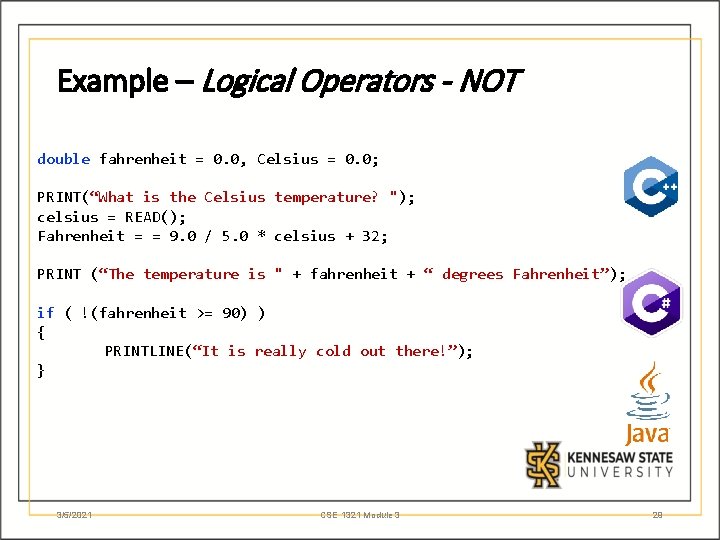
Example – Logical Operators - NOT double fahrenheit = 0. 0, Celsius = 0. 0; PRINT(“What is the Celsius temperature? "); celsius = READ(); Fahrenheit = = 9. 0 / 5. 0 * celsius + 32; PRINT (“The temperature is " + fahrenheit + “ degrees Fahrenheit”); if ( !(fahrenheit >= 90) ) { PRINTLINE(“It is really cold out there!”); } 3/5/2021 CSE 1321 Module 3 29
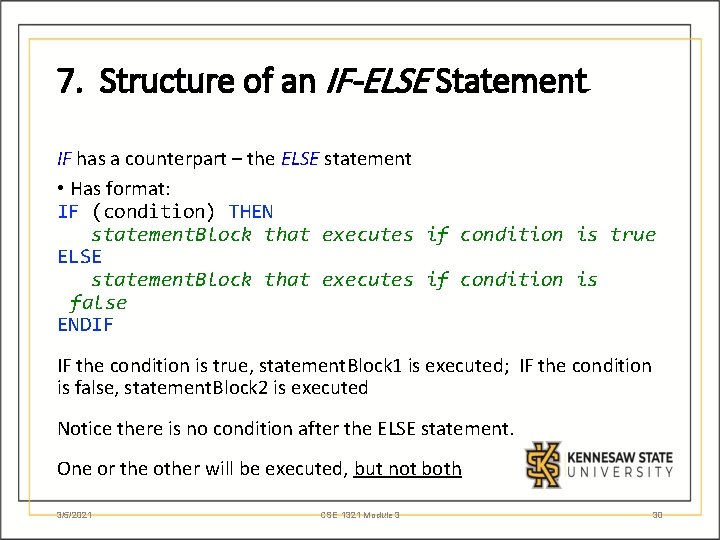
7. Structure of an IF-ELSE Statement IF has a counterpart – the ELSE statement • Has format: IF (condition) THEN statement. Block that executes if condition is true ELSE statement. Block that executes if condition is false ENDIF IF the condition is true, statement. Block 1 is executed; IF the condition is false, statement. Block 2 is executed Notice there is no condition after the ELSE statement. One or the other will be executed, but not both 3/5/2021 CSE 1321 Module 3 30
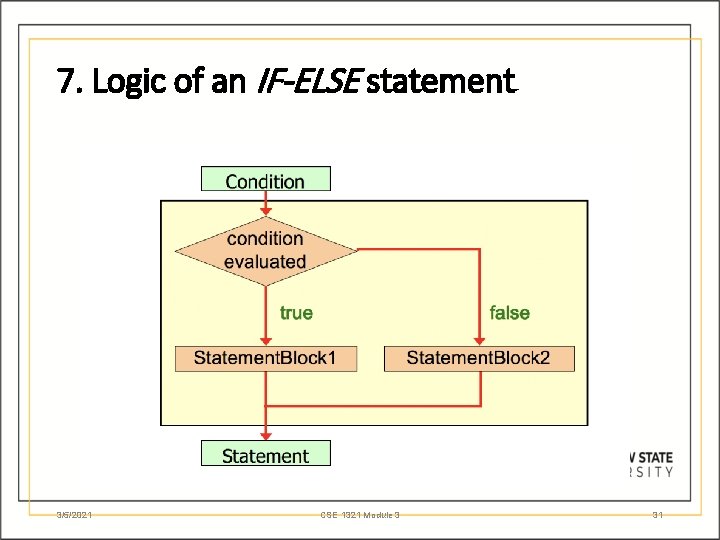
7. Logic of an IF-ELSE statement 3/5/2021 CSE 1321 Module 3 31
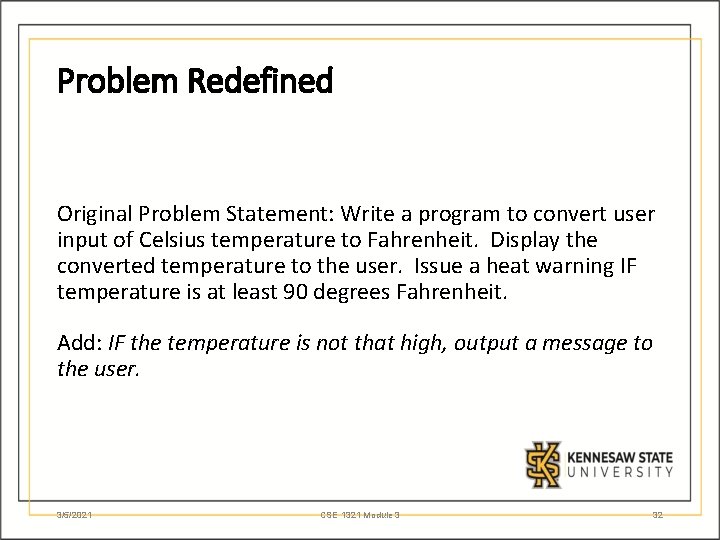
Problem Redefined Original Problem Statement: Write a program to convert user input of Celsius temperature to Fahrenheit. Display the converted temperature to the user. Issue a heat warning IF temperature is at least 90 degrees Fahrenheit. Add: IF the temperature is not that high, output a message to the user. 3/5/2021 CSE 1321 Module 3 32
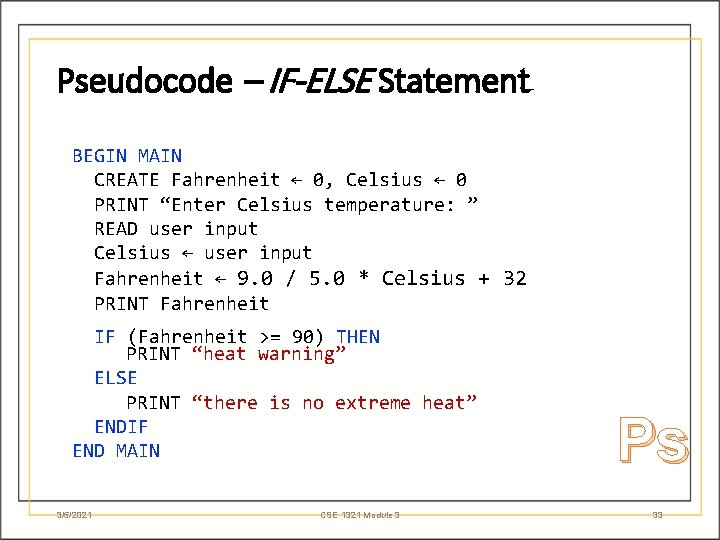
Pseudocode – IF-ELSE Statement BEGIN MAIN CREATE Fahrenheit ← 0, Celsius ← 0 PRINT “Enter Celsius temperature: ” READ user input Celsius ← user input Fahrenheit ← 9. 0 / 5. 0 * Celsius + 32 PRINT Fahrenheit IF (Fahrenheit >= 90) THEN PRINT “heat warning” ELSE PRINT “there is no extreme heat” ENDIF END MAIN 3/5/2021 CSE 1321 Module 3 Ps 33
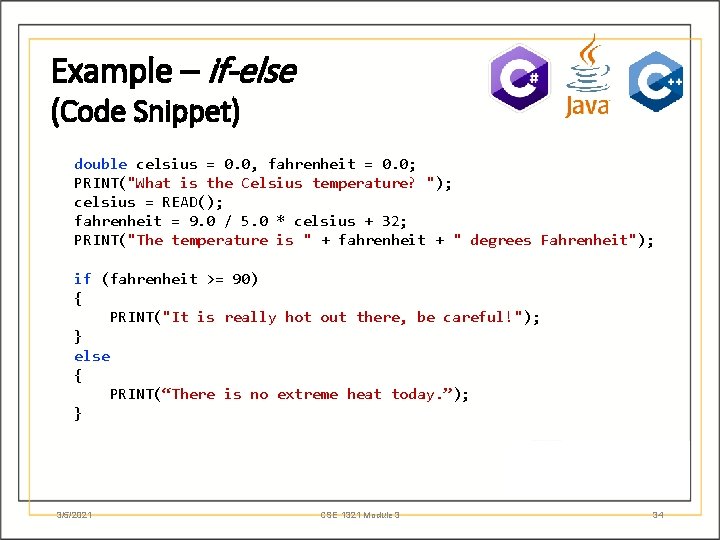
Example – if-else (Code Snippet) double celsius = 0. 0, fahrenheit = 0. 0; PRINT("What is the Celsius temperature? "); celsius = READ(); fahrenheit = 9. 0 / 5. 0 * celsius + 32; PRINT("The temperature is " + fahrenheit + " degrees Fahrenheit"); if (fahrenheit >= 90) { PRINT("It is really hot out there, be careful!"); } else { PRINT(“There is no extreme heat today. ”); } 3/5/2021 CSE 1321 Module 3 34
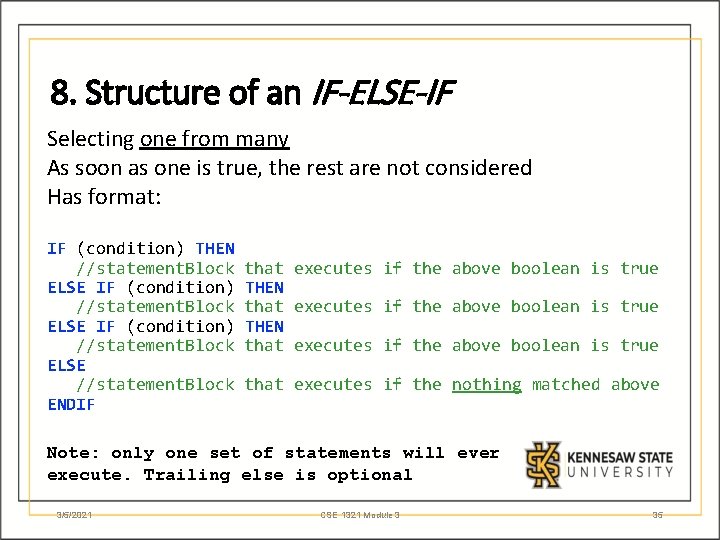
8. Structure of an IF-ELSE-IF Selecting one from many As soon as one is true, the rest are not considered Has format: IF (condition) THEN //statement. Block ELSE IF (condition) //statement. Block ELSE //statement. Block ENDIF that executes if the above boolean is true THEN that executes if the above boolean is true that executes if the nothing matched above Note: only one set of statements will ever execute. Trailing else is optional 3/5/2021 CSE 1321 Module 3 35
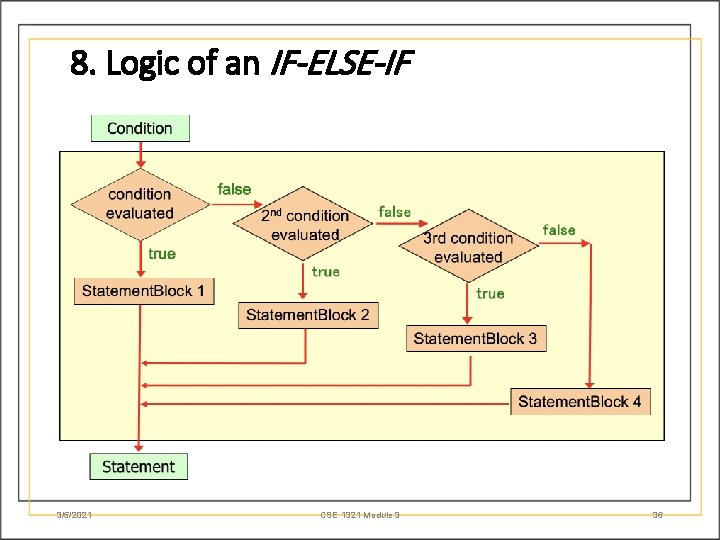
8. Logic of an IF-ELSE-IF 3/5/2021 CSE 1321 Module 3 36
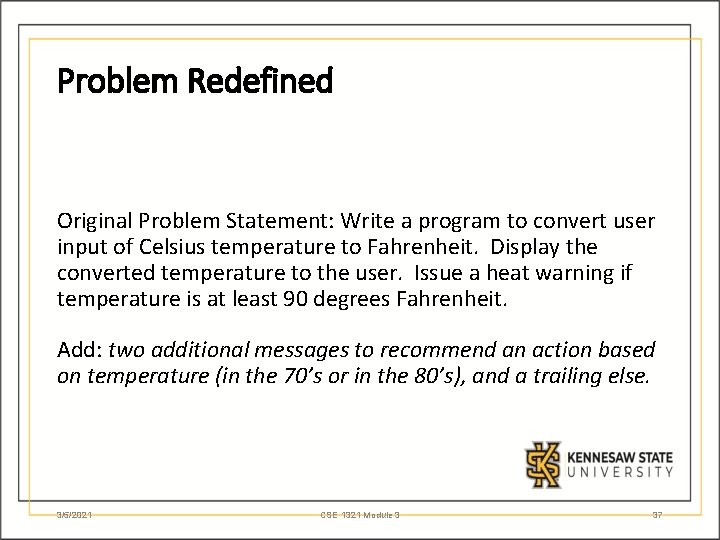
Problem Redefined Original Problem Statement: Write a program to convert user input of Celsius temperature to Fahrenheit. Display the converted temperature to the user. Issue a heat warning if temperature is at least 90 degrees Fahrenheit. Add: two additional messages to recommend an action based on temperature (in the 70’s or in the 80’s), and a trailing else. 3/5/2021 CSE 1321 Module 3 37
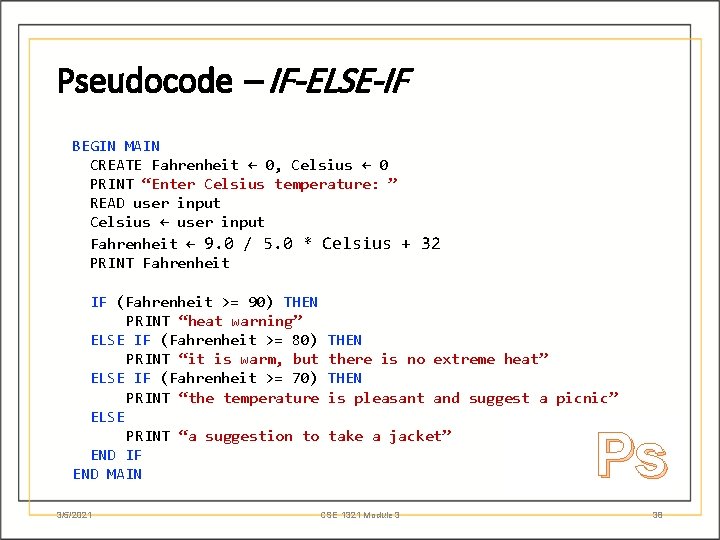
Pseudocode – IF-ELSE-IF BEGIN MAIN CREATE Fahrenheit ← 0, Celsius ← 0 PRINT “Enter Celsius temperature: ” READ user input Celsius ← user input Fahrenheit ← 9. 0 / 5. 0 * Celsius + 32 PRINT Fahrenheit IF (Fahrenheit >= 90) THEN PRINT “heat warning” ELSE IF (Fahrenheit >= 80) PRINT “it is warm, but ELSE IF (Fahrenheit >= 70) PRINT “the temperature ELSE PRINT “a suggestion to END IF END MAIN 3/5/2021 THEN there is no extreme heat” THEN is pleasant and suggest a picnic” take a jacket” CSE 1321 Module 3 Ps 38
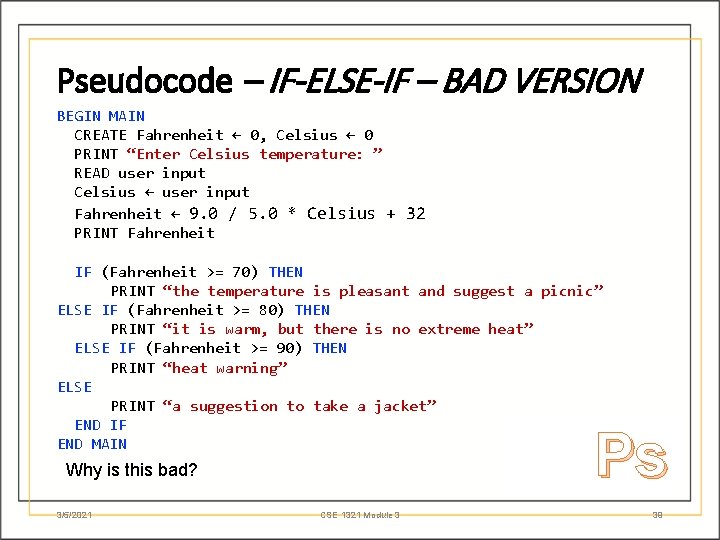
Pseudocode – IF-ELSE-IF – BAD VERSION BEGIN MAIN CREATE Fahrenheit ← 0, Celsius ← 0 PRINT “Enter Celsius temperature: ” READ user input Celsius ← user input Fahrenheit ← 9. 0 / 5. 0 * Celsius + 32 PRINT Fahrenheit IF (Fahrenheit >= 70) THEN PRINT “the temperature is pleasant and suggest a picnic” ELSE IF (Fahrenheit >= 80) THEN PRINT “it is warm, but there is no extreme heat” ELSE IF (Fahrenheit >= 90) THEN PRINT “heat warning” ELSE PRINT “a suggestion to take a jacket” END IF END MAIN Ps Why is this bad? 3/5/2021 CSE 1321 Module 3 39
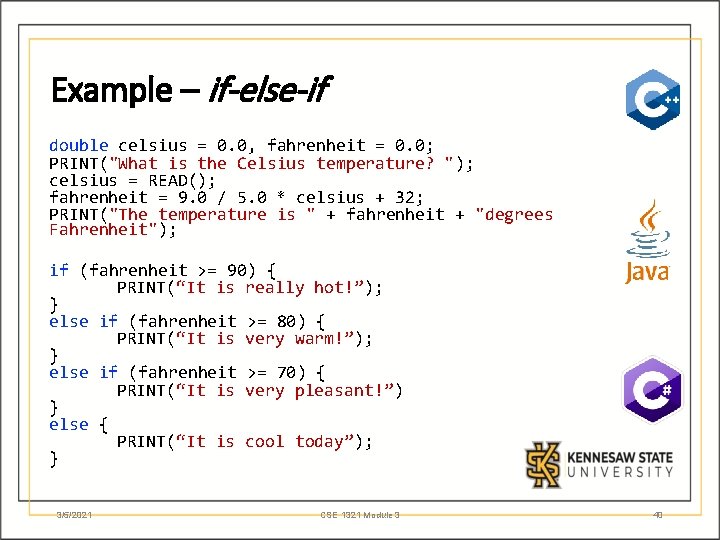
Example – if-else-if double celsius = 0. 0, fahrenheit = 0. 0; PRINT("What is the Celsius temperature? "); celsius = READ(); fahrenheit = 9. 0 / 5. 0 * celsius + 32; PRINT("The temperature is " + fahrenheit + "degrees Fahrenheit"); if (fahrenheit >= 90) { PRINT(“It is really hot!”); } else if (fahrenheit >= 80) { PRINT(“It is very warm!”); } else if (fahrenheit >= 70) { PRINT(“It is very pleasant!”) } else { PRINT(“It is cool today”); } 3/5/2021 CSE 1321 Module 3 40
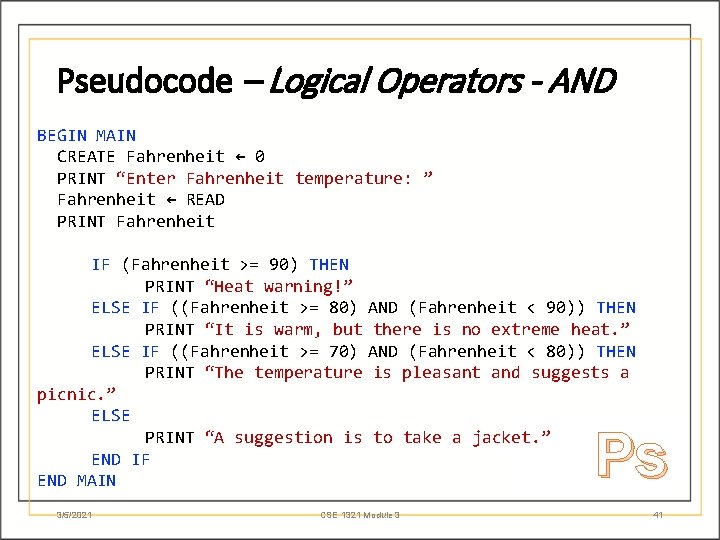
Pseudocode – Logical Operators - AND BEGIN MAIN CREATE Fahrenheit ← 0 PRINT “Enter Fahrenheit temperature: ” Fahrenheit ← READ PRINT Fahrenheit IF (Fahrenheit >= 90) THEN PRINT “Heat warning!” ELSE IF ((Fahrenheit >= 80) AND (Fahrenheit < 90)) THEN PRINT “It is warm, but there is no extreme heat. ” ELSE IF ((Fahrenheit >= 70) AND (Fahrenheit < 80)) THEN PRINT “The temperature is pleasant and suggests a picnic. ” ELSE PRINT “A suggestion is to take a jacket. ” END IF END MAIN Ps 3/5/2021 CSE 1321 Module 3 41
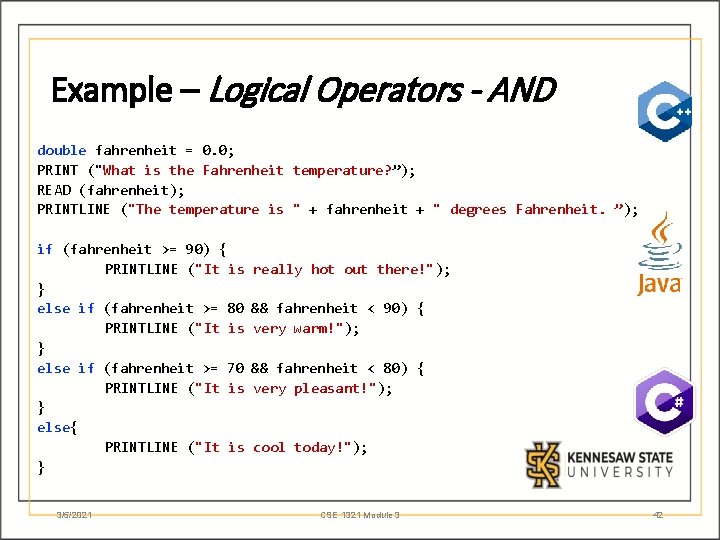
Example – Logical Operators - AND double fahrenheit = 0. 0; PRINT ("What is the Fahrenheit temperature? ”); READ (fahrenheit); PRINTLINE ("The temperature is " + fahrenheit + " degrees Fahrenheit. ”); if (fahrenheit >= 90) { PRINTLINE ("It is } else if (fahrenheit >= 80 PRINTLINE ("It is } else if (fahrenheit >= 70 PRINTLINE ("It is } else{ PRINTLINE ("It is } 3/5/2021 really hot out there!"); && fahrenheit < 90) { very warm!"); && fahrenheit < 80) { very pleasant!"); cool today!"); CSE 1321 Module 3 42
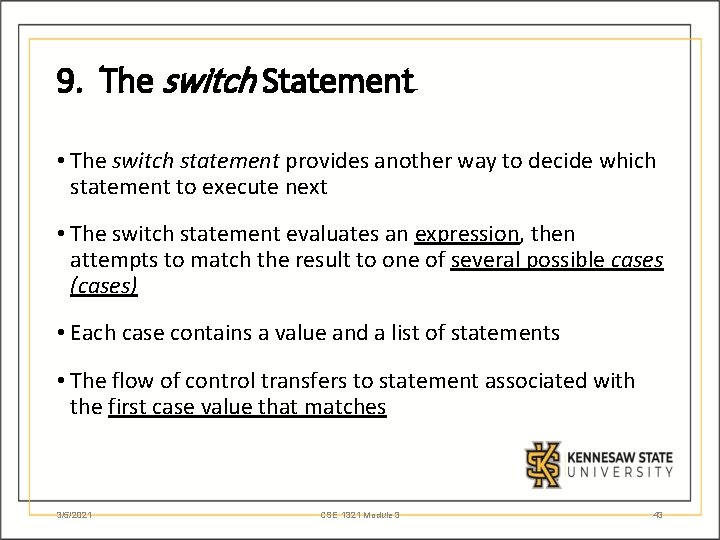
9. The switch Statement • The switch statement provides another way to decide which statement to execute next • The switch statement evaluates an expression, then attempts to match the result to one of several possible cases (cases) • Each case contains a value and a list of statements • The flow of control transfers to statement associated with the first case value that matches 3/5/2021 CSE 1321 Module 3 43
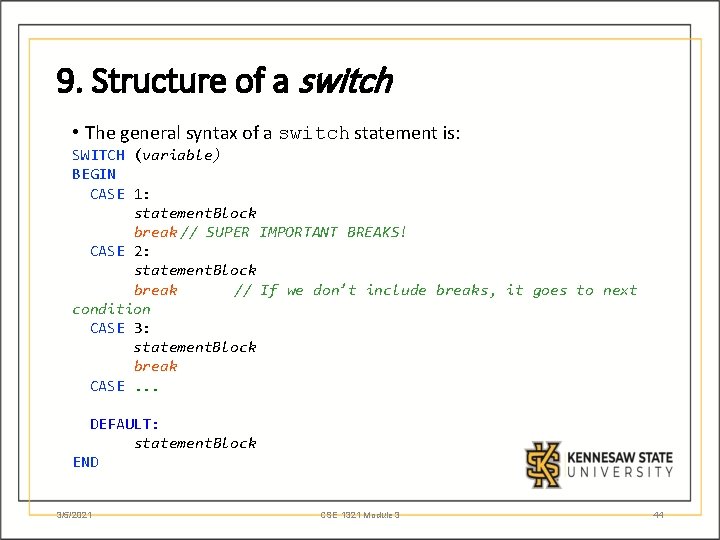
9. Structure of a switch • The general syntax of a switch statement is: SWITCH (variable) BEGIN CASE 1: statement. Block break // SUPER IMPORTANT BREAKS! CASE 2: statement. Block break // If we don’t include breaks, it goes to next condition CASE 3: statement. Block break CASE. . . DEFAULT: statement. Block END 3/5/2021 CSE 1321 Module 3 44
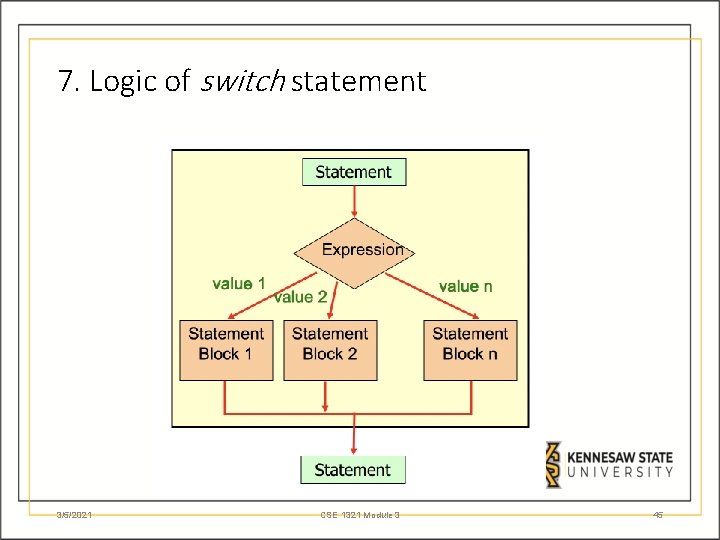
7. Logic of switch statement 3/5/2021 CSE 1321 Module 3 45
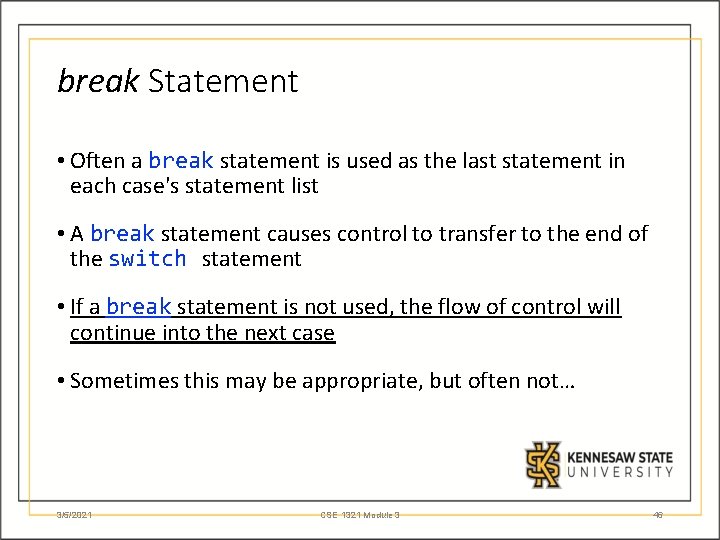
break Statement • Often a break statement is used as the last statement in each case's statement list • A break statement causes control to transfer to the end of the switch statement • If a break statement is not used, the flow of control will continue into the next case • Sometimes this may be appropriate, but often not… 3/5/2021 CSE 1321 Module 3 46
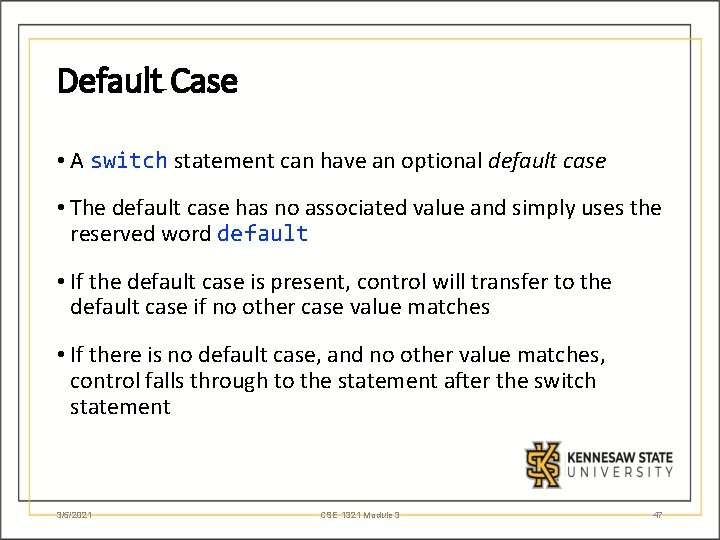
Default Case • A switch statement can have an optional default case • The default case has no associated value and simply uses the reserved word default • If the default case is present, control will transfer to the default case if no other case value matches • If there is no default case, and no other value matches, control falls through to the statement after the switch statement 3/5/2021 CSE 1321 Module 3 47
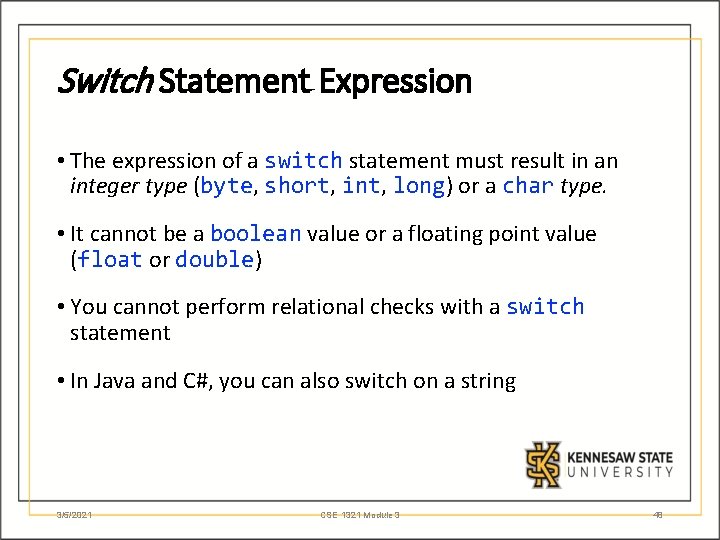
Switch Statement Expression • The expression of a switch statement must result in an integer type (byte, short, int, long) or a char type. • It cannot be a boolean value or a floating point value (float or double) • You cannot perform relational checks with a switch statement • In Java and C#, you can also switch on a string 3/5/2021 CSE 1321 Module 3 48
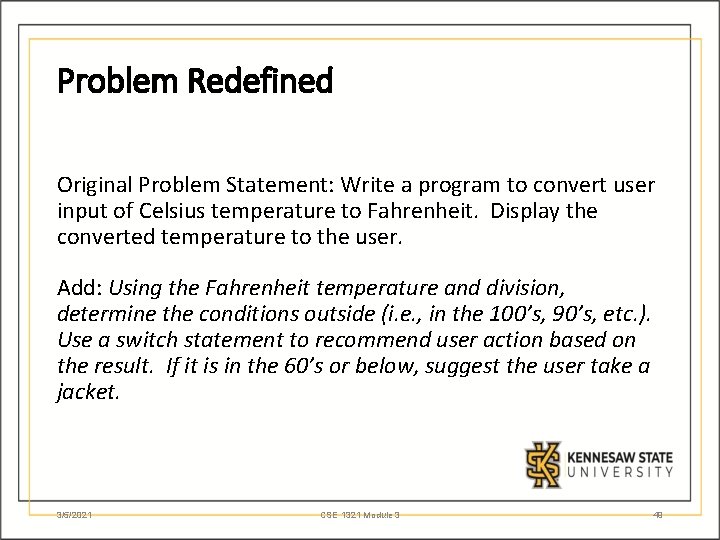
Problem Redefined Original Problem Statement: Write a program to convert user input of Celsius temperature to Fahrenheit. Display the converted temperature to the user. Add: Using the Fahrenheit temperature and division, determine the conditions outside (i. e. , in the 100’s, 90’s, etc. ). Use a switch statement to recommend user action based on the result. If it is in the 60’s or below, suggest the user take a jacket. 3/5/2021 CSE 1321 Module 3 49
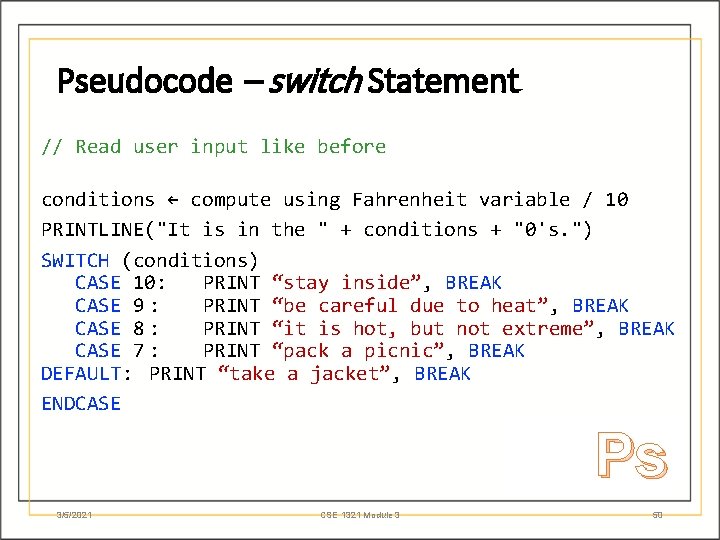
Pseudocode – switch Statement // Read user input like before conditions ← compute using Fahrenheit variable / 10 PRINTLINE("It is in the " + conditions + "0's. ") SWITCH (conditions) CASE 10: PRINT “stay inside”, BREAK CASE 9 : PRINT “be careful due to heat”, BREAK CASE 8 : PRINT “it is hot, but not extreme”, BREAK CASE 7 : PRINT “pack a picnic”, BREAK DEFAULT: PRINT “take a jacket”, BREAK ENDCASE Ps 3/5/2021 CSE 1321 Module 3 50
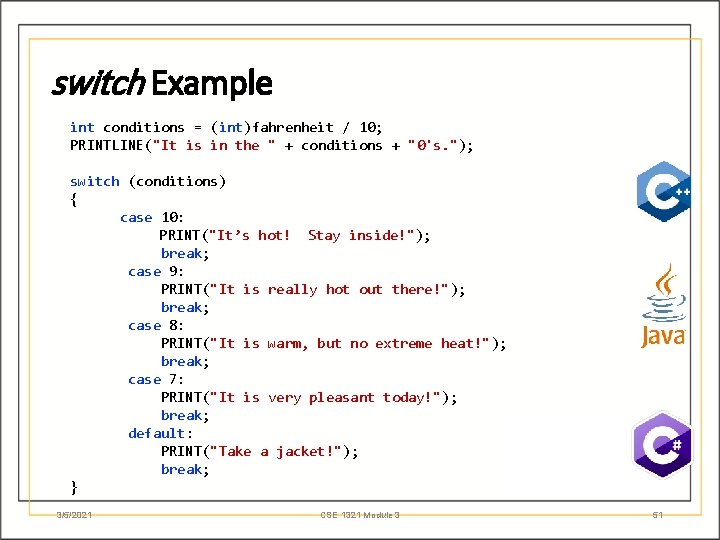
switch Example int conditions = (int)fahrenheit / 10; PRINTLINE("It is in the " + conditions + "0's. "); switch (conditions) { case 10: PRINT("It’s hot! Stay inside!"); break; case 9: PRINT("It is really hot out there!"); break; case 8: PRINT("It is warm, but no extreme heat!"); break; case 7: PRINT("It is very pleasant today!"); break; default: PRINT("Take a jacket!"); break; } 3/5/2021 CSE 1321 Module 3 51
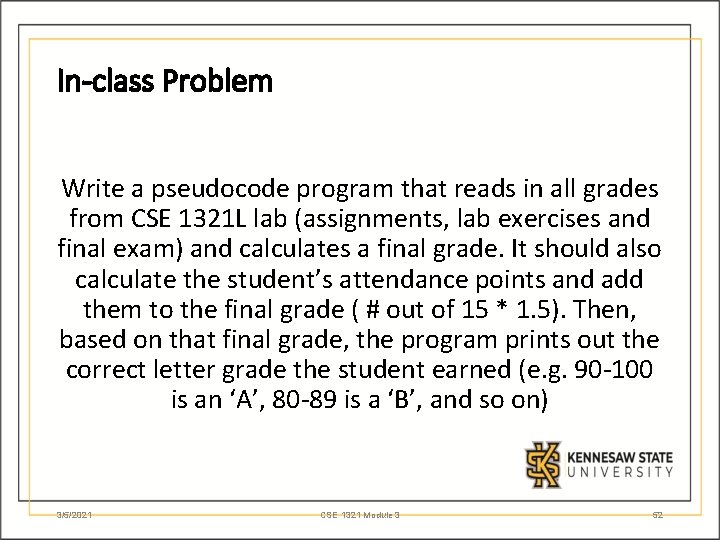
In-class Problem Write a pseudocode program that reads in all grades from CSE 1321 L lab (assignments, lab exercises and final exam) and calculates a final grade. It should also calculate the student’s attendance points and add them to the final grade ( # out of 15 * 1. 5). Then, based on that final grade, the program prints out the correct letter grade the student earned (e. g. 90 -100 is an ‘A’, 80 -89 is a ‘B’, and so on) 3/5/2021 CSE 1321 Module 3 52
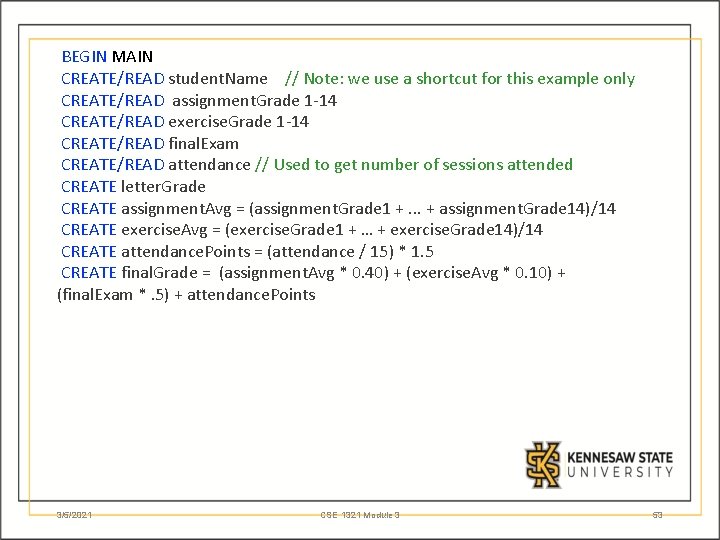
BEGIN MAIN CREATE/READ student. Name // Note: we use a shortcut for this example only CREATE/READ assignment. Grade 1 -14 CREATE/READ exercise. Grade 1 -14 CREATE/READ final. Exam CREATE/READ attendance // Used to get number of sessions attended CREATE letter. Grade CREATE assignment. Avg = (assignment. Grade 1 +. . . + assignment. Grade 14)/14 CREATE exercise. Avg = (exercise. Grade 1 + … + exercise. Grade 14)/14 CREATE attendance. Points = (attendance / 15) * 1. 5 CREATE final. Grade = (assignment. Avg * 0. 40) + (exercise. Avg * 0. 10) + (final. Exam *. 5) + attendance. Points 3/5/2021 CSE 1321 Module 3 53
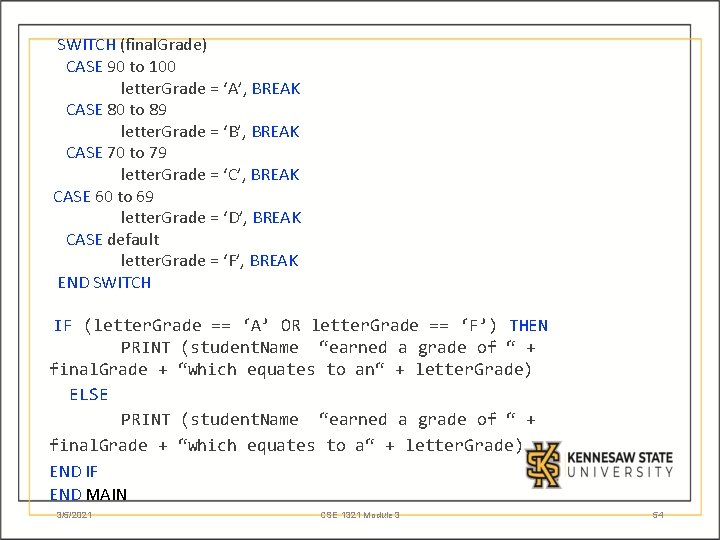
SWITCH (final. Grade) CASE 90 to 100 letter. Grade = ‘A’, BREAK CASE 80 to 89 letter. Grade = ‘B’, BREAK CASE 70 to 79 letter. Grade = ‘C’, BREAK CASE 60 to 69 letter. Grade = ‘D’, BREAK CASE default letter. Grade = ‘F’, BREAK END SWITCH IF (letter. Grade == ‘A’ OR letter. Grade == ‘F’) THEN PRINT (student. Name “earned a grade of “ + final. Grade + “which equates to an“ + letter. Grade) ELSE PRINT (student. Name “earned a grade of “ + final. Grade + “which equates to a“ + letter. Grade) END IF END MAIN 3/5/2021 CSE 1321 Module 3 54
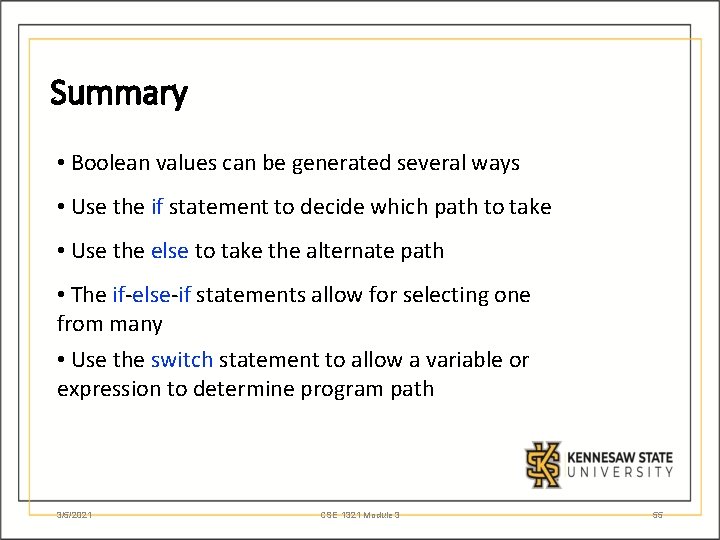
Summary • Boolean values can be generated several ways • Use the if statement to decide which path to take • Use the else to take the alternate path • The if-else-if statements allow for selecting one from many • Use the switch statement to allow a variable or expression to determine program path 3/5/2021 CSE 1321 Module 3 55
Cse 1321
Gradescope ksu
Cse 1321 slides
Cse 1321
Dot 1321
Risoluzione
Enseñanza de la parábola del sembrador
1321-1265
Homologous structures
Matlab selection structure
Selection structures
Balancing selection vs stabilizing selection
Similarities
K selected
Natural selection vs artificial selection
Artificial selection vs natural selection
Stabilizing selection human birth weight
Logistic model of population growth
Natural selection vs artificial selection
Two way selection and multiway selection in c
Multiway selection
Mass selection and pure line selection
Module 11 studying the brain
C device module module 1
Application mobile cse
Cse 6331 uta
Cse 423
Cse 423
Steve seitz uw
Uw cse 311
Cse 30
Cse 2431 lab 3
String matching cses
Cse 312
Cse 402
Cse 3318
Cse323
Cts in c#
Cse 2431 lab 3
Cse452
Cse 6740
Cse 351 lab5
Cse 5236
Asu cse 365
Cse401
Cse 312
Cse 3902 github
Fyp hkust
Cse 5236
Equivalence relation
Cse 422
Cse 197
Cse 2231 wolf
Hcmut edu
Cse 572
Daffodil university cse course