Array 1 352021 2 352021 3 352021 4
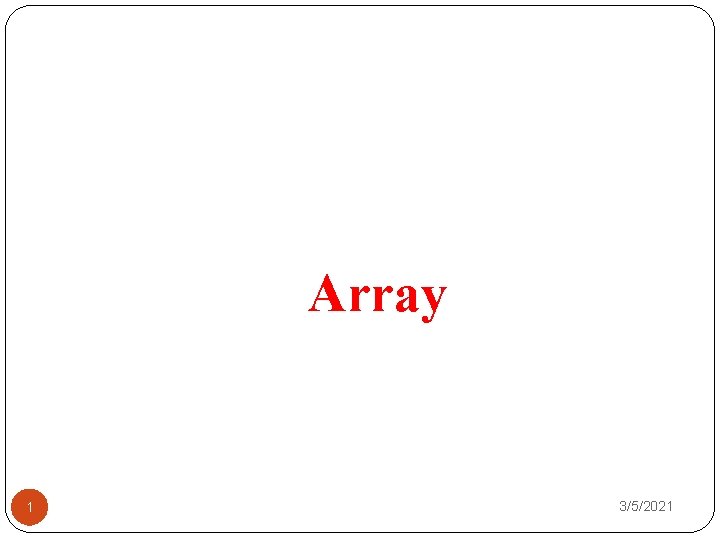
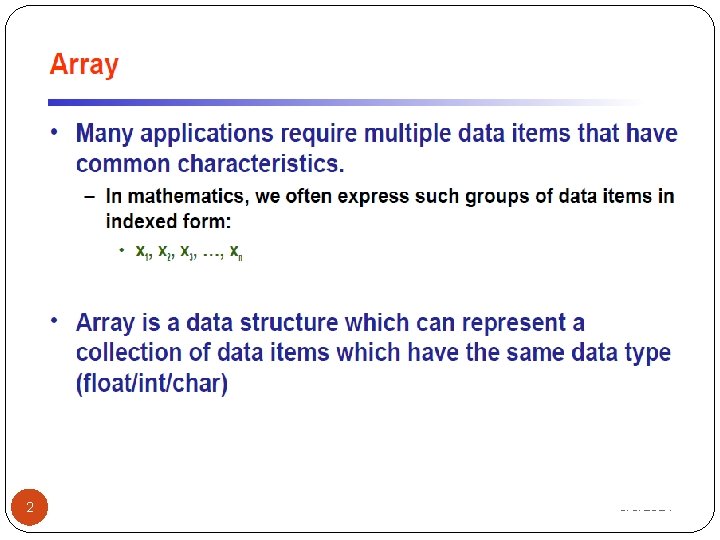
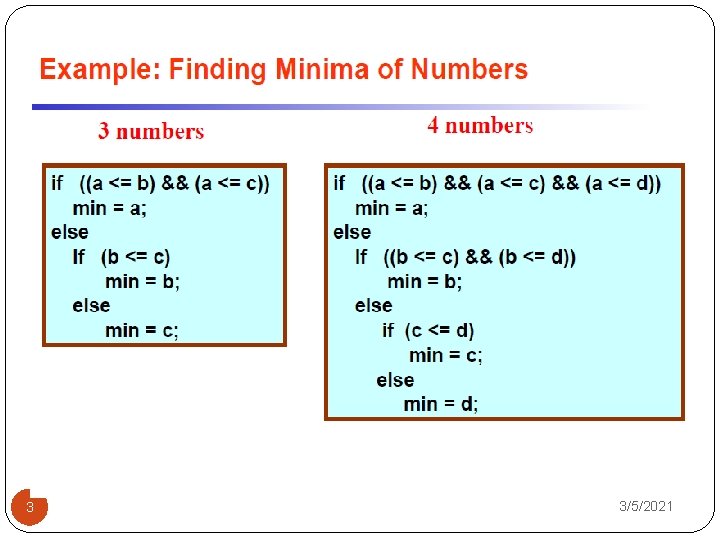
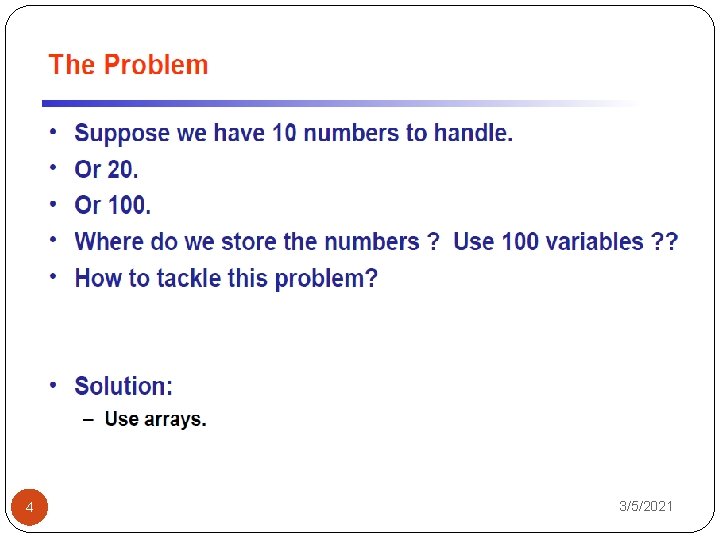
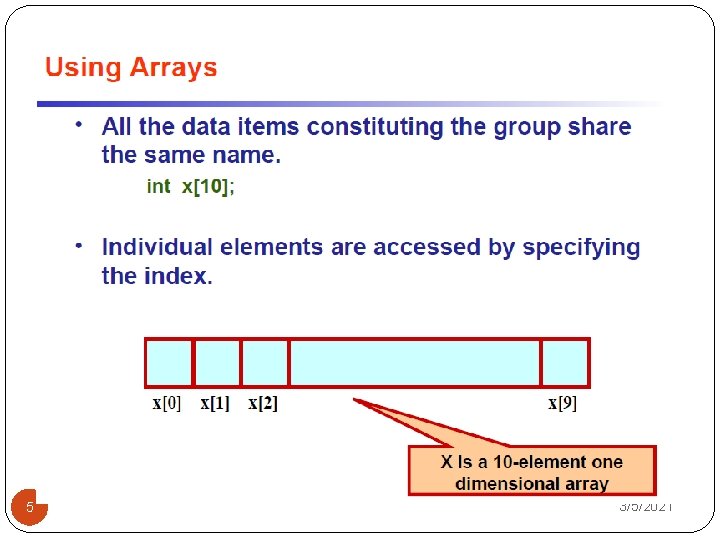
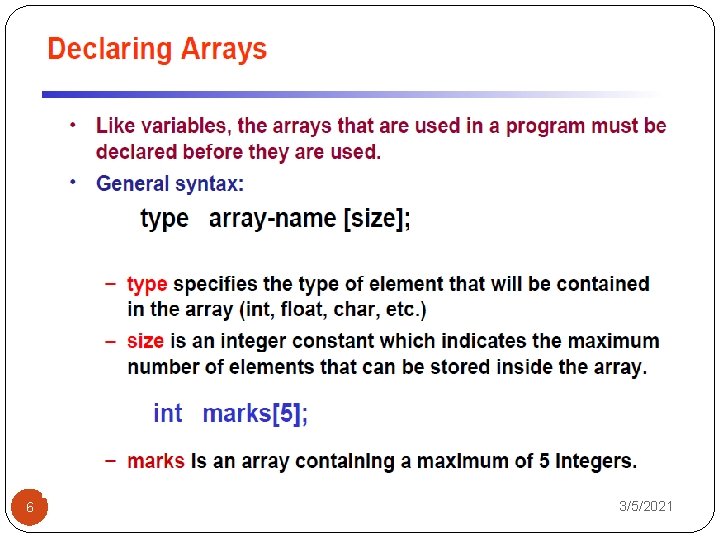
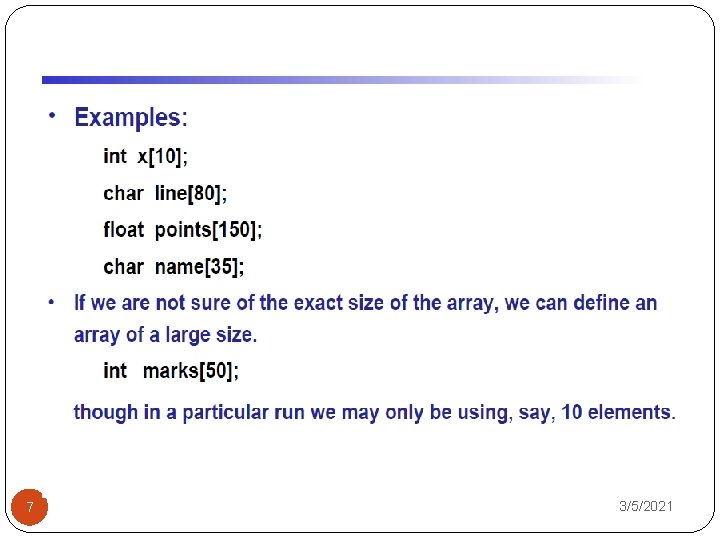
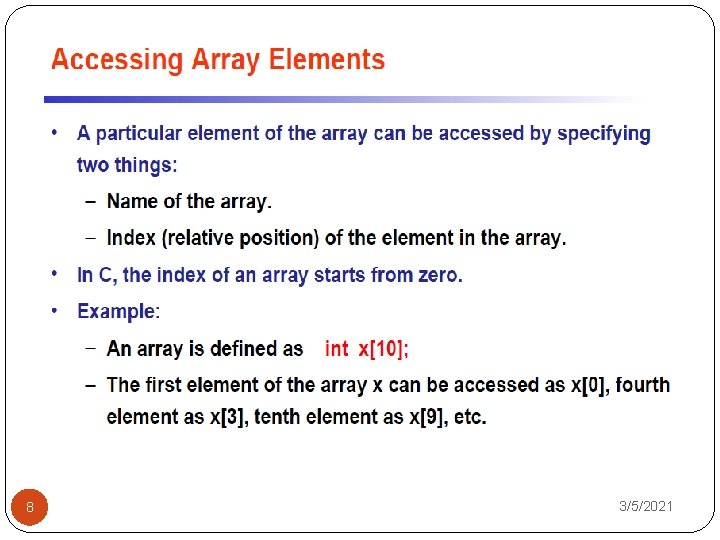
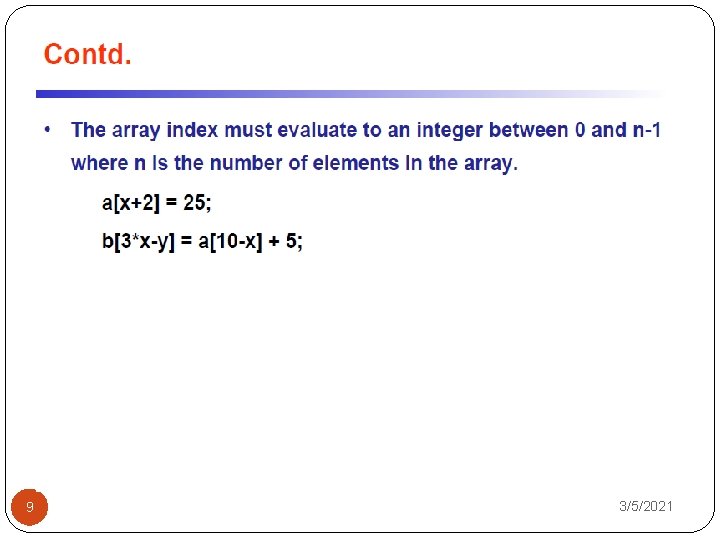
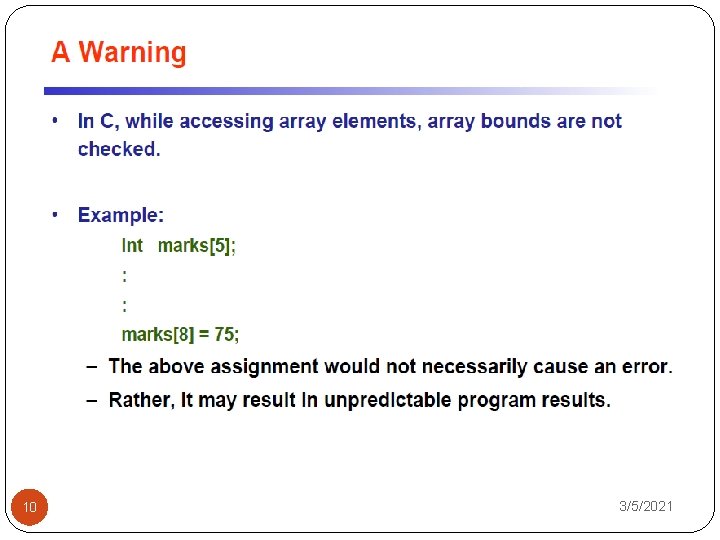
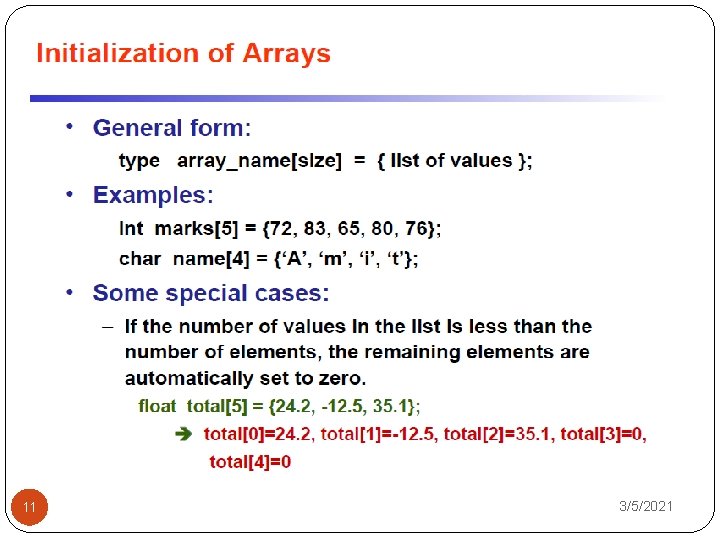
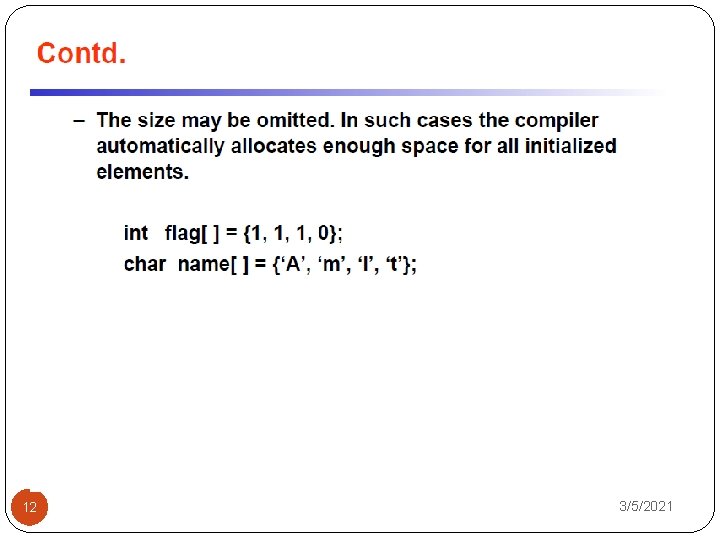
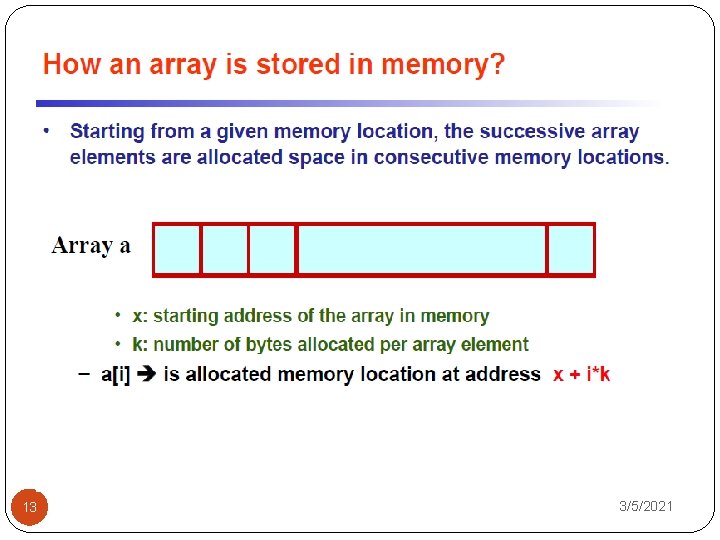
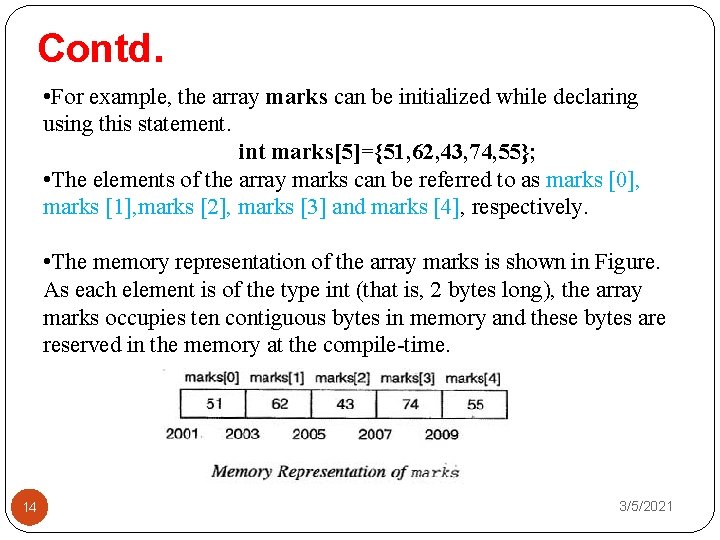
![Problem double percentage[50]; If this array percentage is stored in memory starting from address Problem double percentage[50]; If this array percentage is stored in memory starting from address](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-15.jpg)
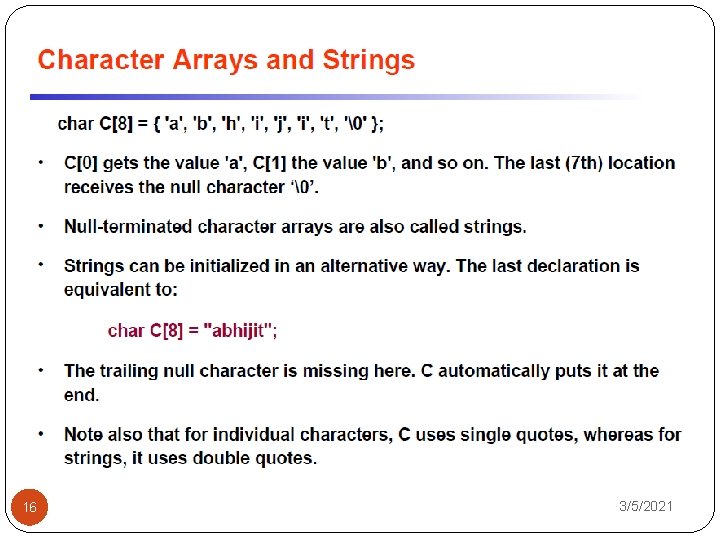
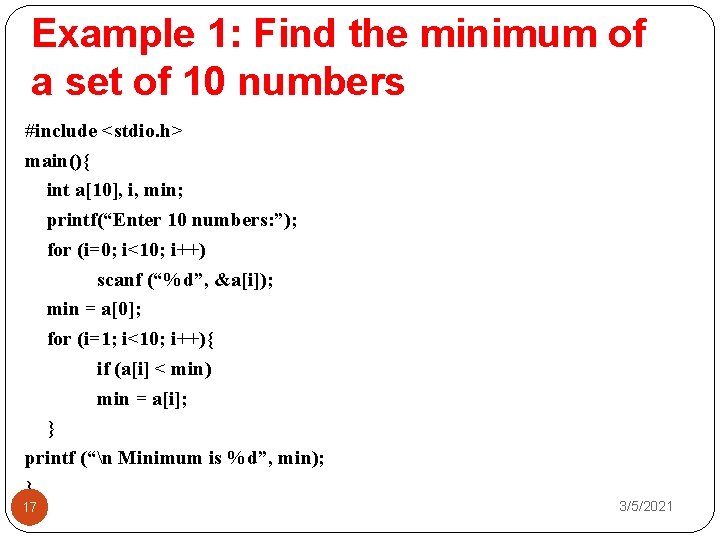
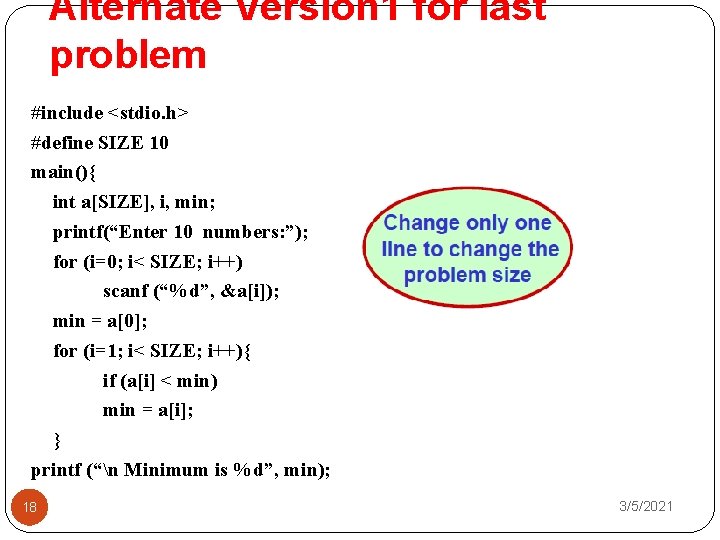
![Alternate Version 2 for last problem #include <stdio. h> main(){ int a[500], i, min; Alternate Version 2 for last problem #include <stdio. h> main(){ int a[500], i, min;](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-19.jpg)
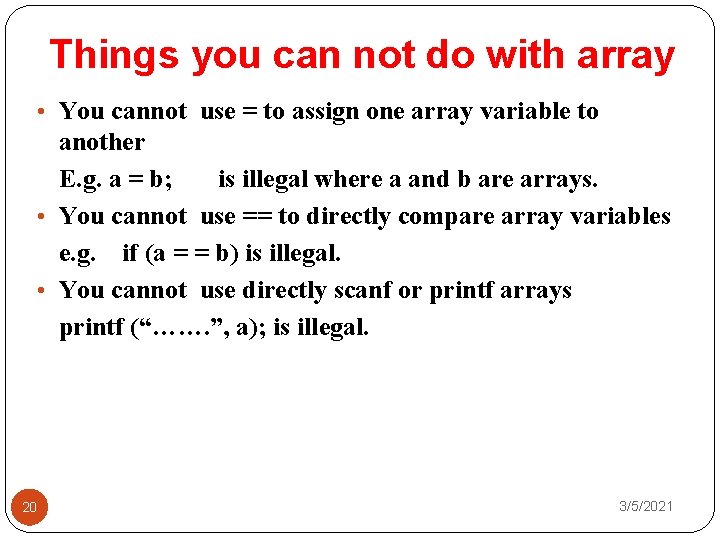
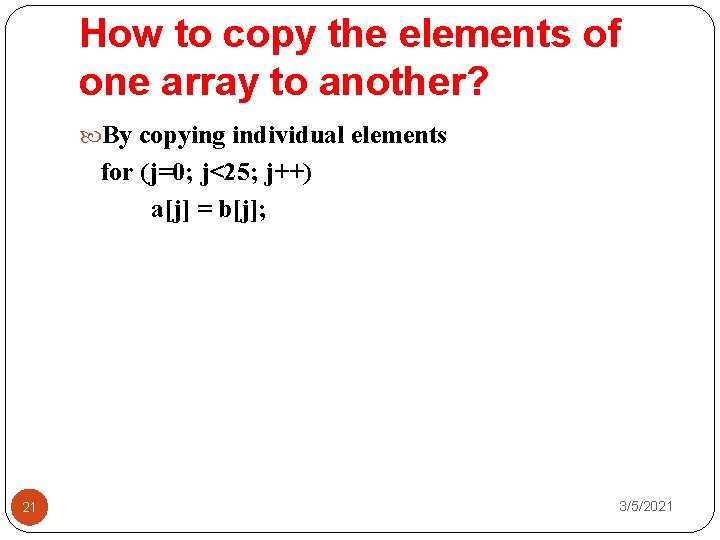
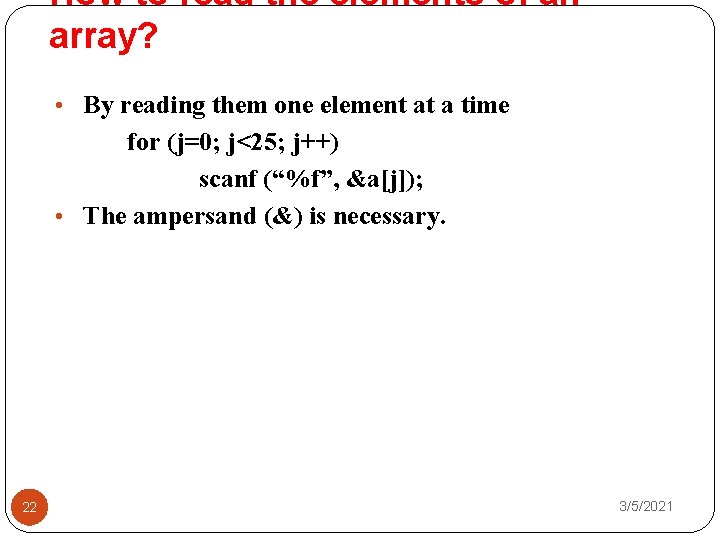
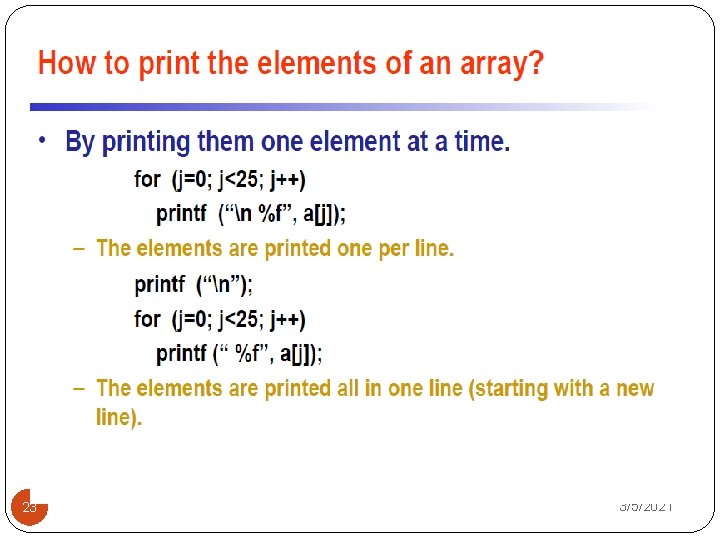
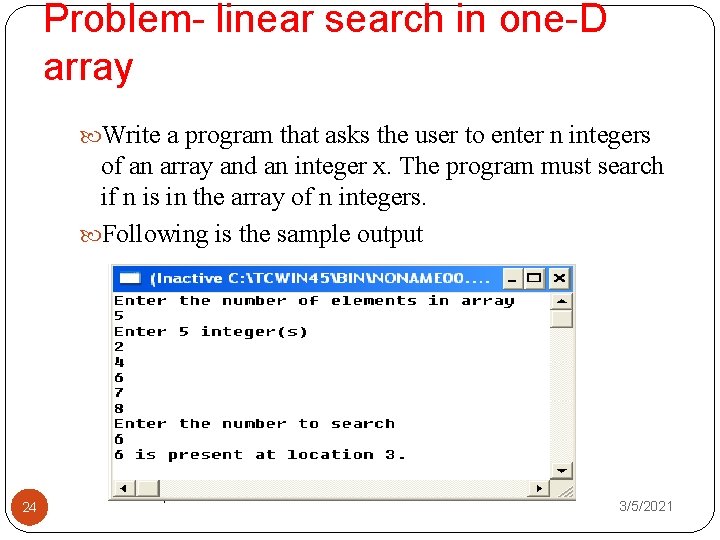
![void main() { 25 int array[100], search, c, n; printf("Enter the number of void main() { 25 int array[100], search, c, n; printf("Enter the number of](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-25.jpg)
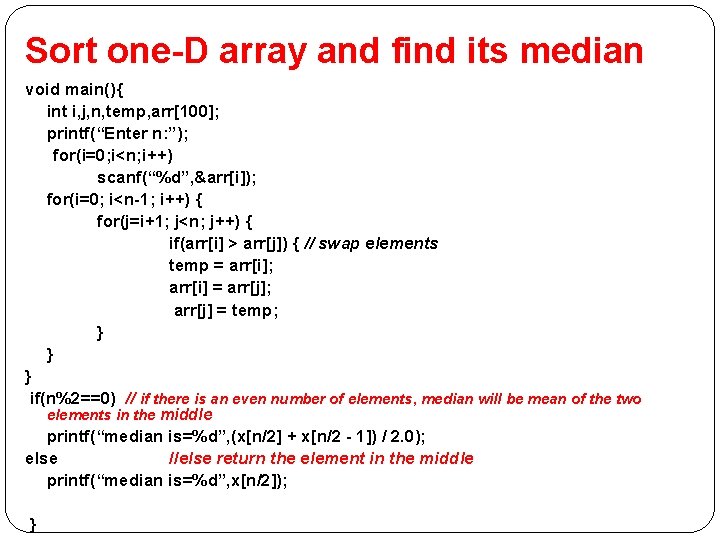
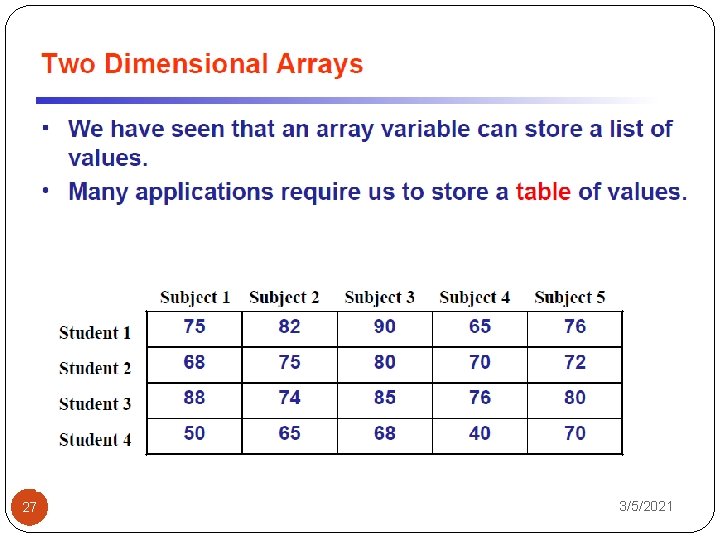
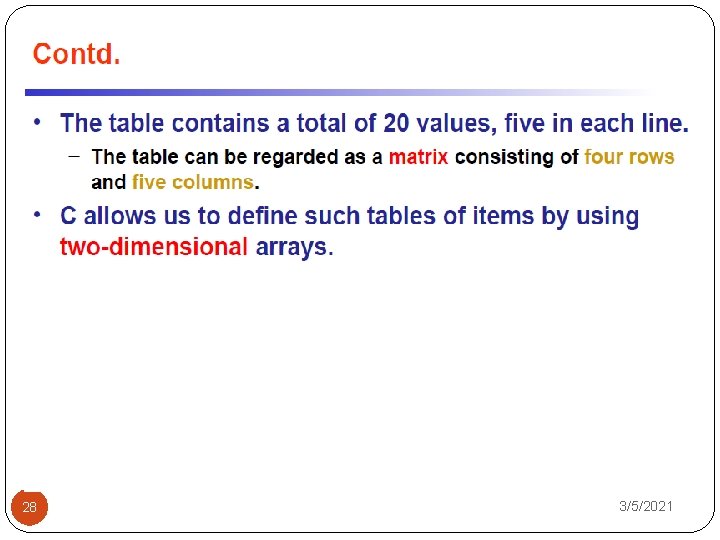
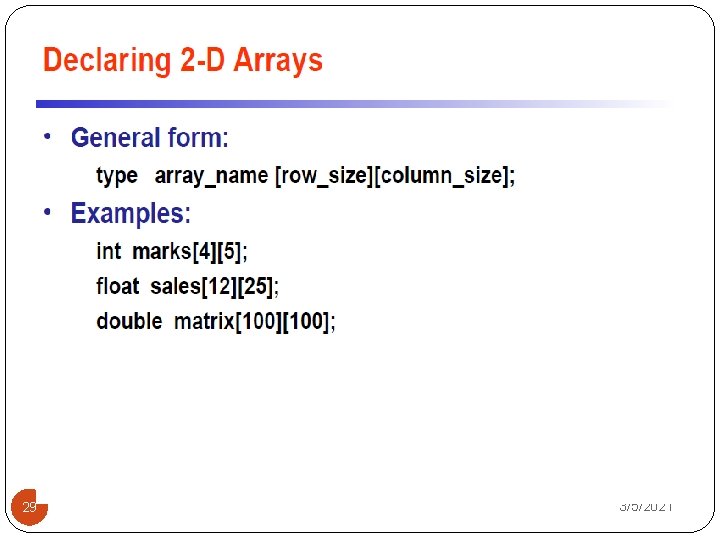
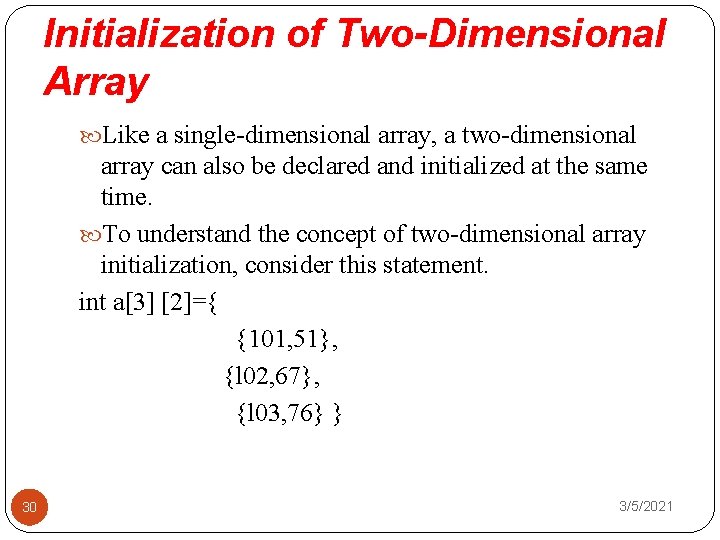
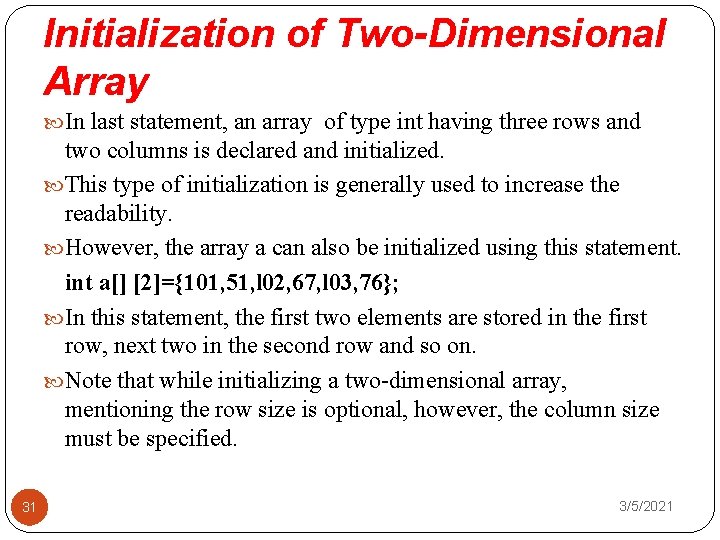
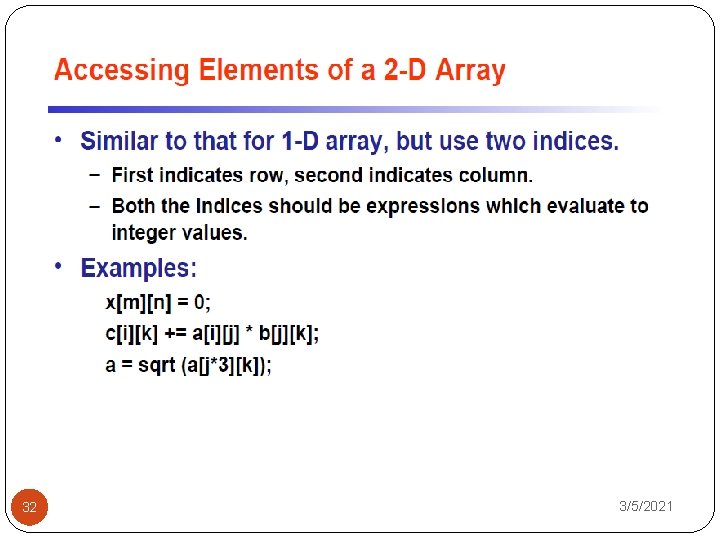
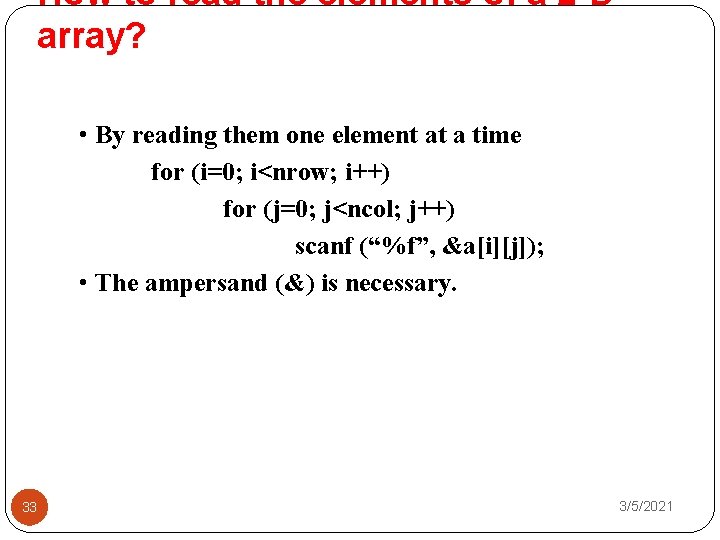
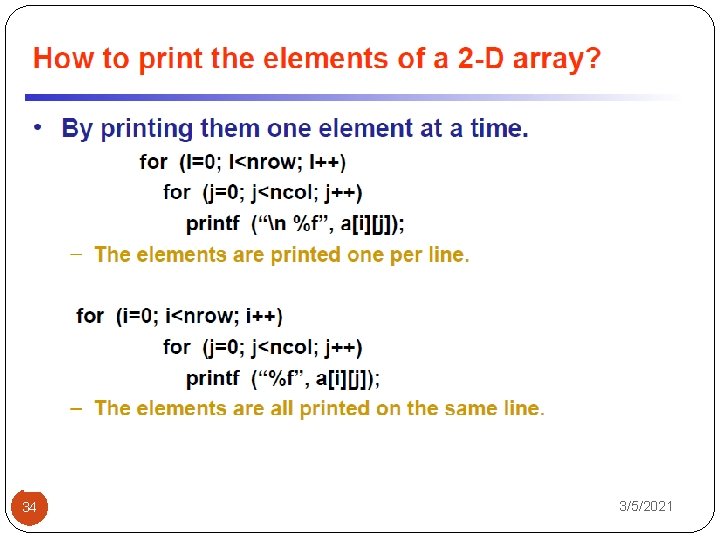
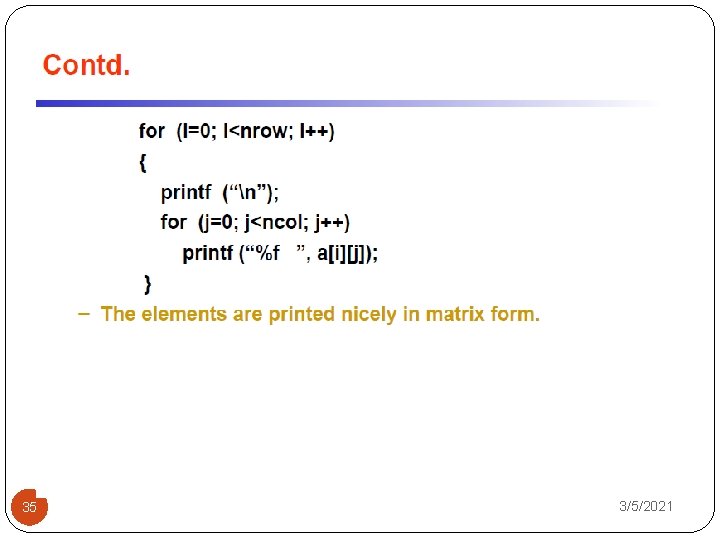
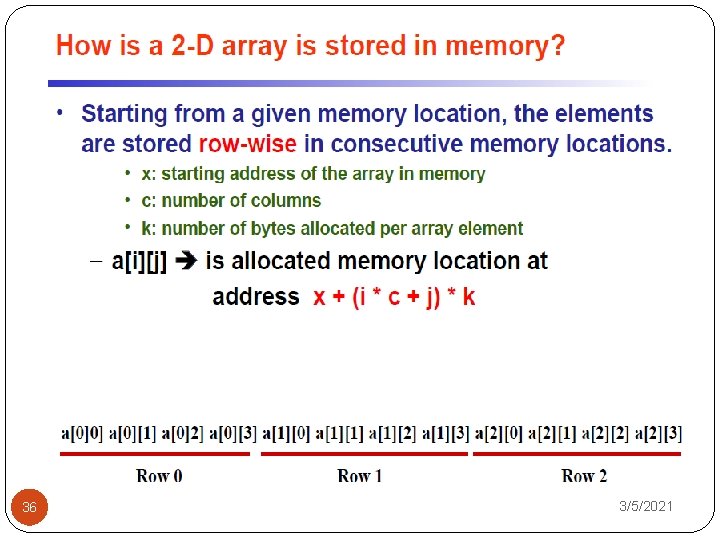
![• int a[] [2]={101, 51, l 02, 67, l 03, 76}; • For • int a[] [2]={101, 51, l 02, 67, l 03, 76}; • For](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-37.jpg)
![Problem long double array[10][15]; If base address is 2000 then what will be the Problem long double array[10][15]; If base address is 2000 then what will be the](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-38.jpg)
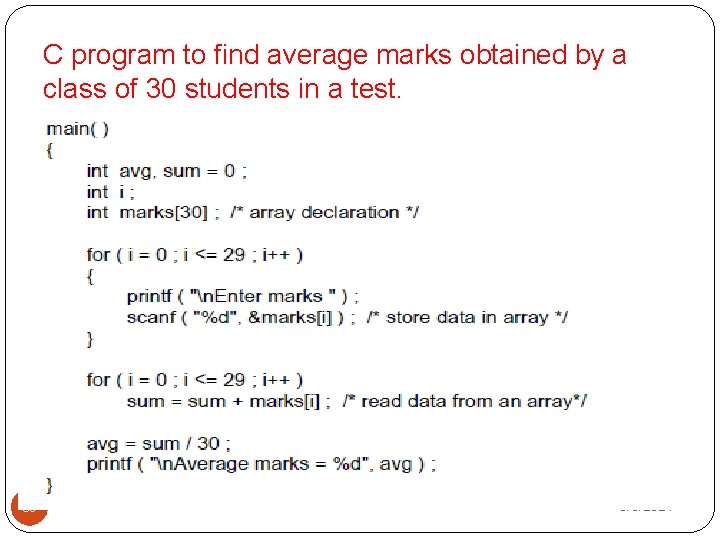
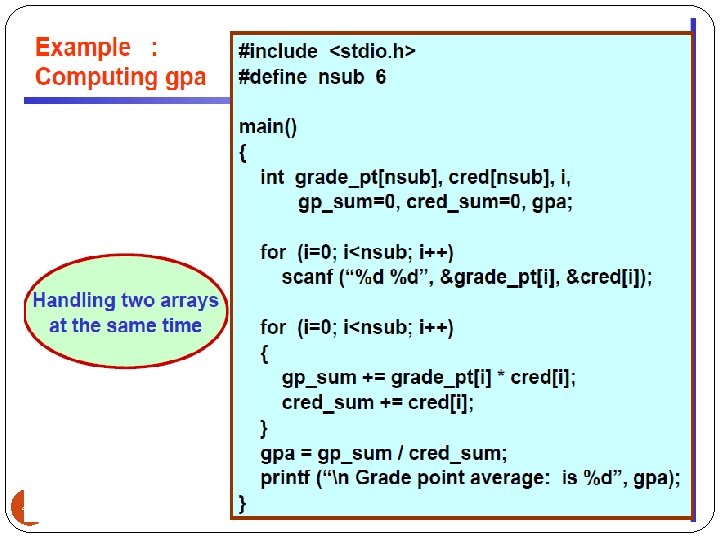
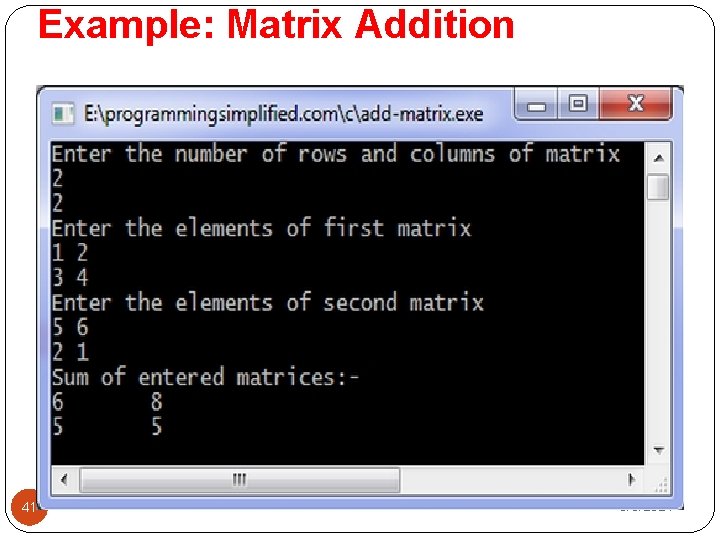
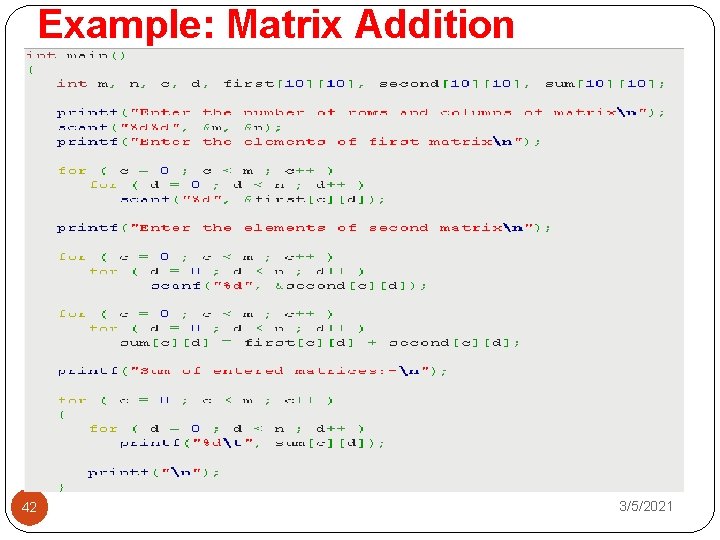
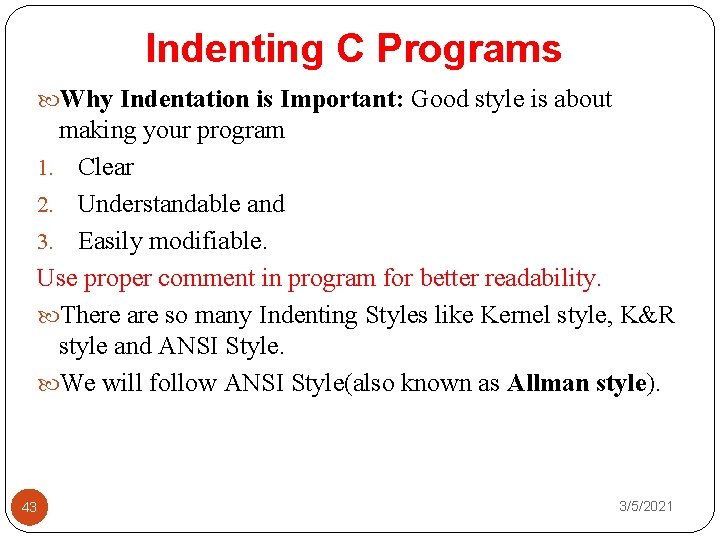
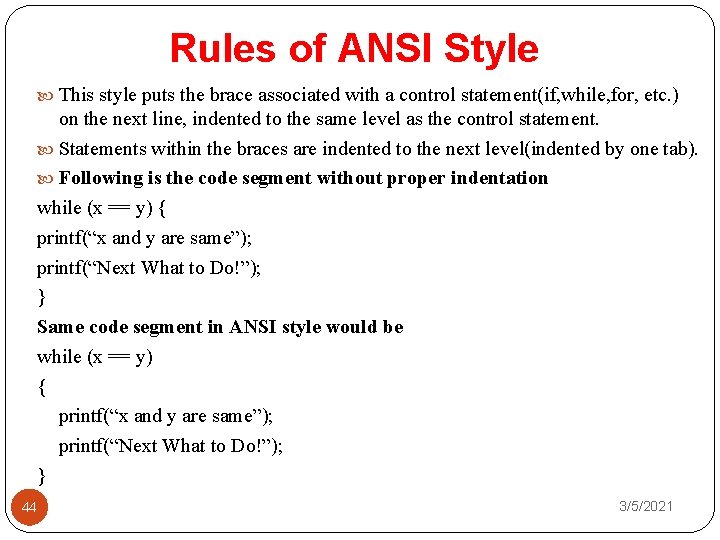
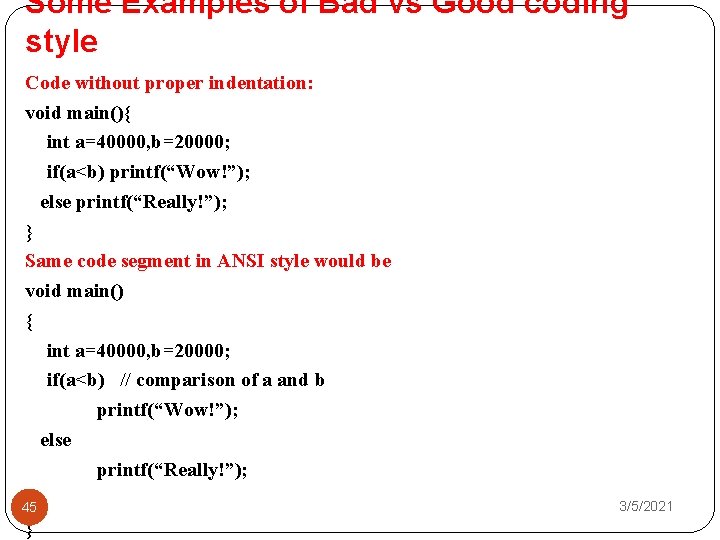
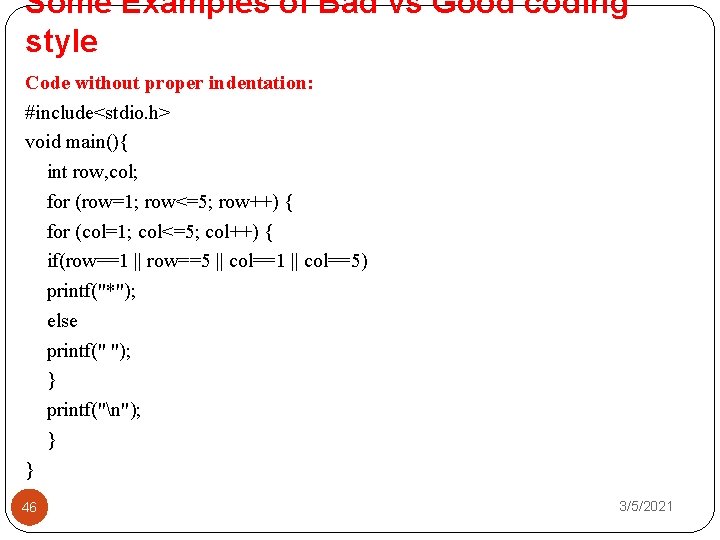
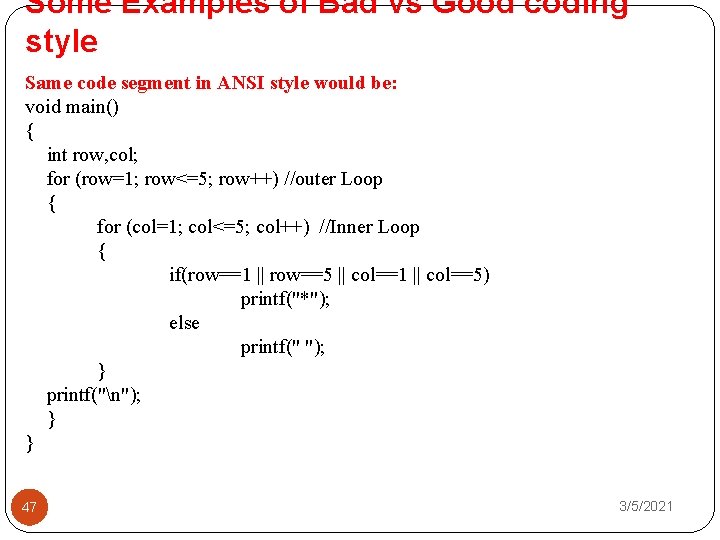
![O/P? main() { int arr[]=(12, 13, 14, 15, 16}; printf(“n%d , %d”, sizeof(arr), sizeof(arr[0])); O/P? main() { int arr[]=(12, 13, 14, 15, 16}; printf(“n%d , %d”, sizeof(arr), sizeof(arr[0]));](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-48.jpg)
![O/P? main() { float a[]= { 12. 4, 2. 3, 4. 5, 6. 7}; O/P? main() { float a[]= { 12. 4, 2. 3, 4. 5, 6. 7};](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-49.jpg)
![O/p? main( ) { int three[3][ ] = { 2, 4, 3, 6, 8, O/p? main( ) { int three[3][ ] = { 2, 4, 3, 6, 8,](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-50.jpg)
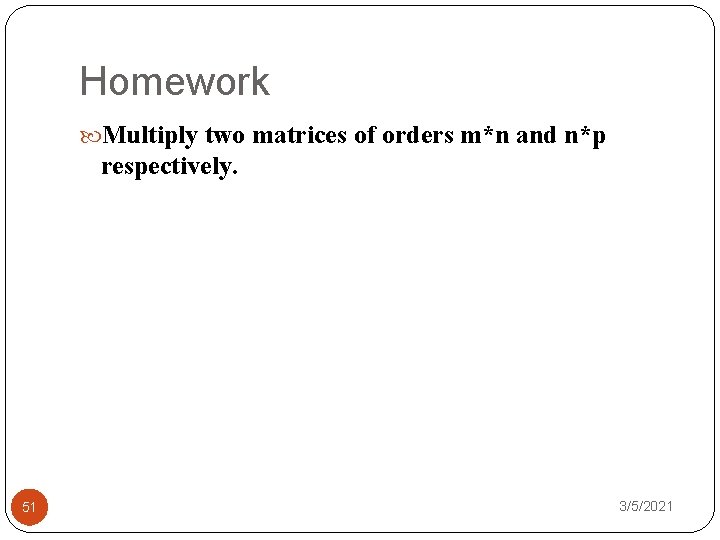
- Slides: 51
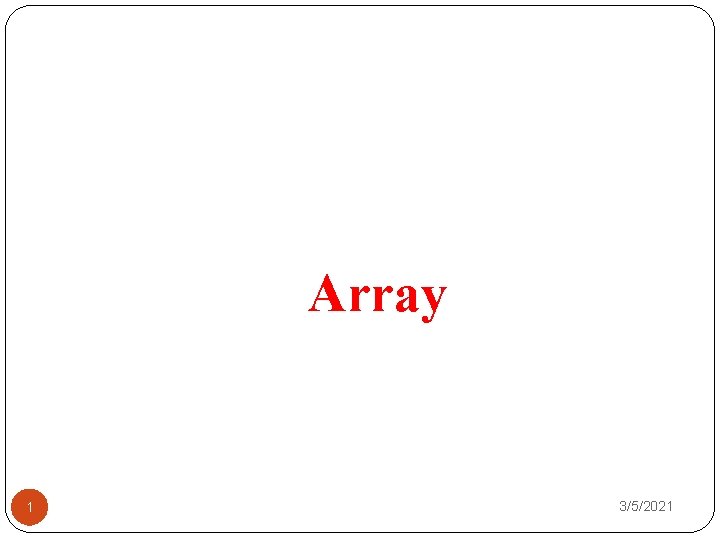
Array 1 3/5/2021
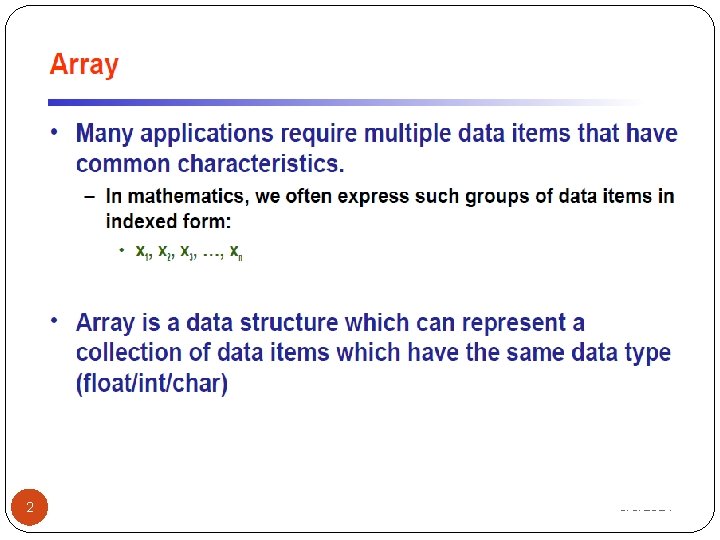
2 3/5/2021
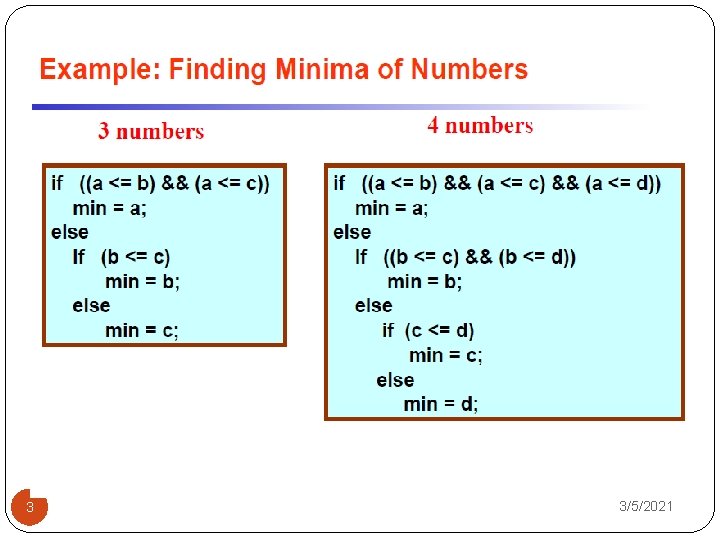
3 3/5/2021
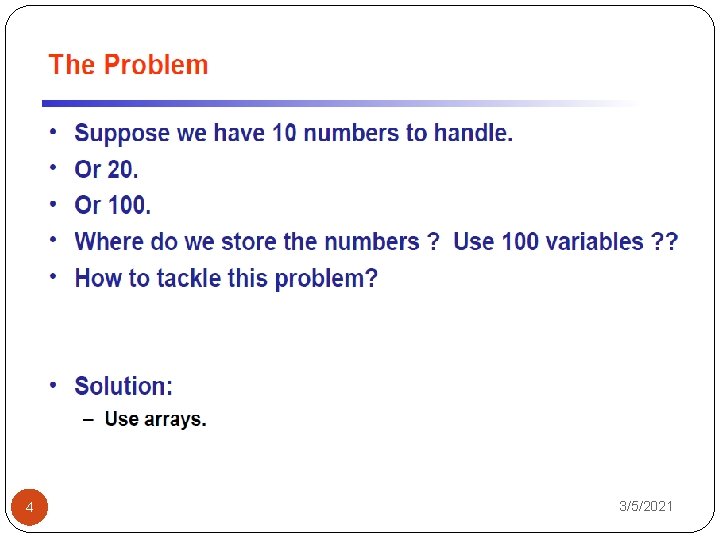
4 3/5/2021
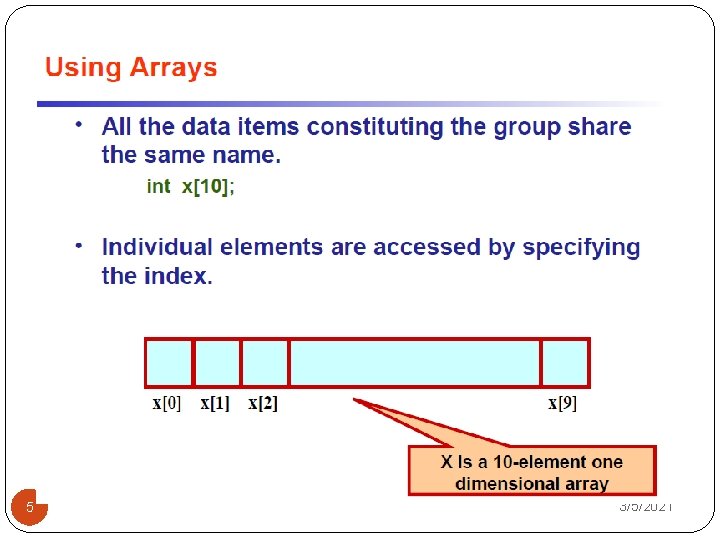
5 3/5/2021
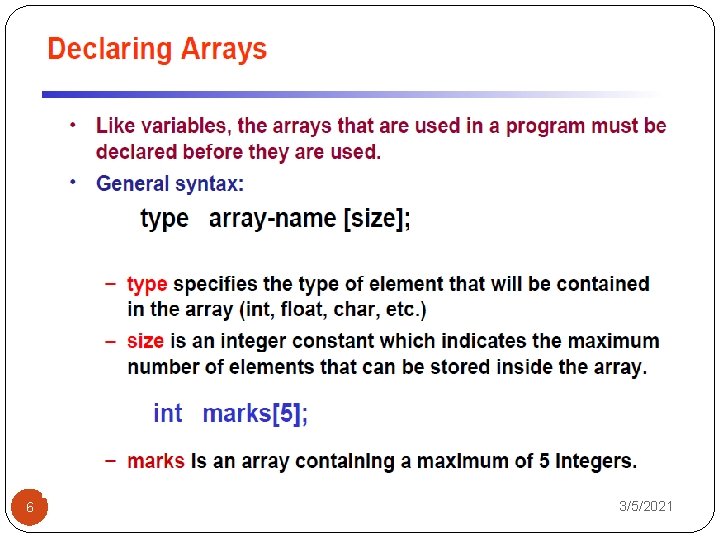
6 3/5/2021
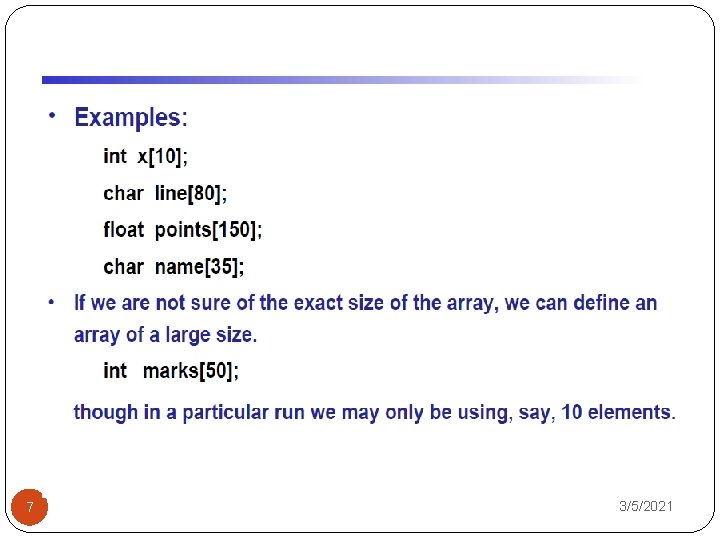
7 3/5/2021
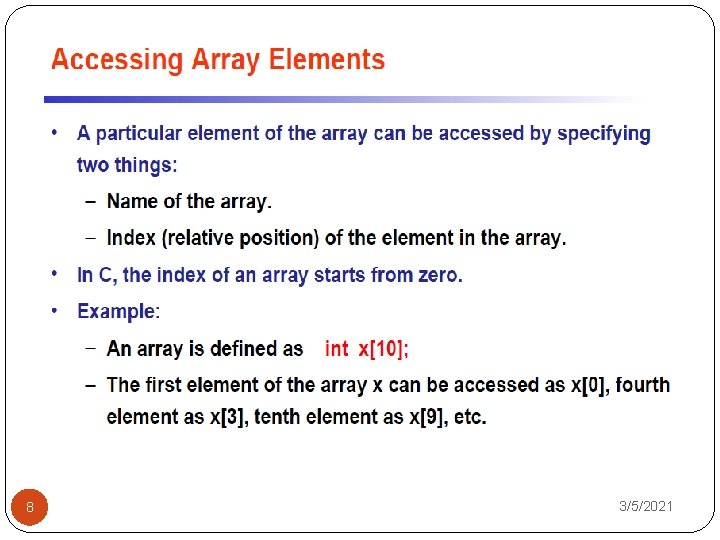
8 3/5/2021
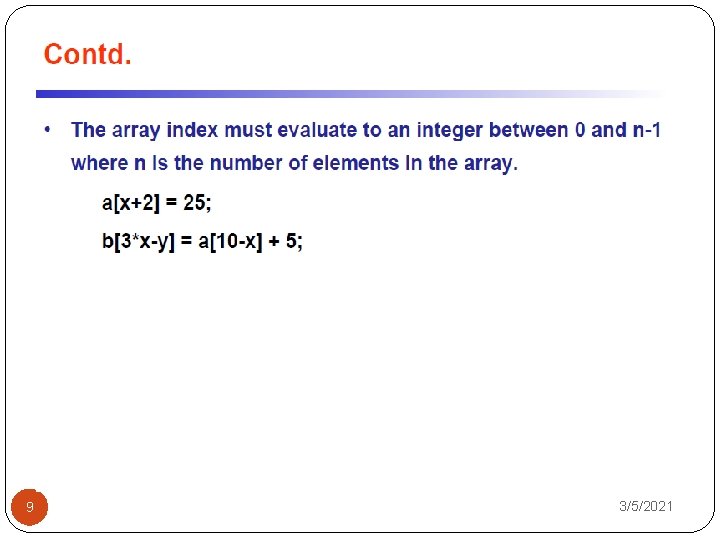
9 3/5/2021
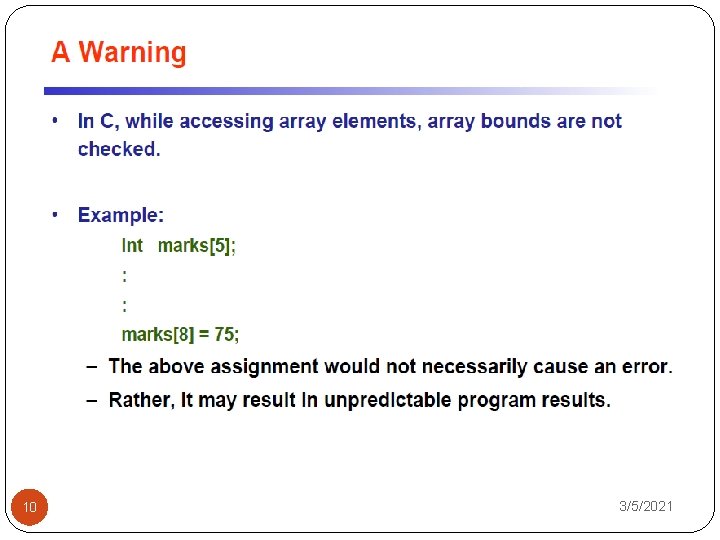
10 3/5/2021
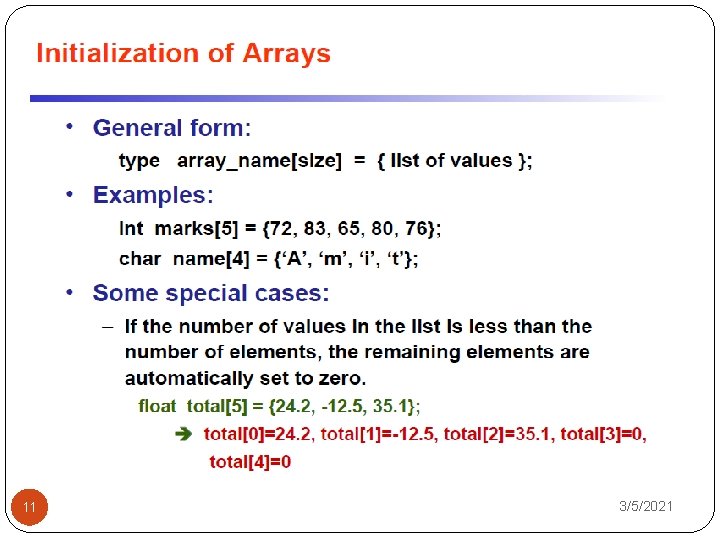
11 3/5/2021
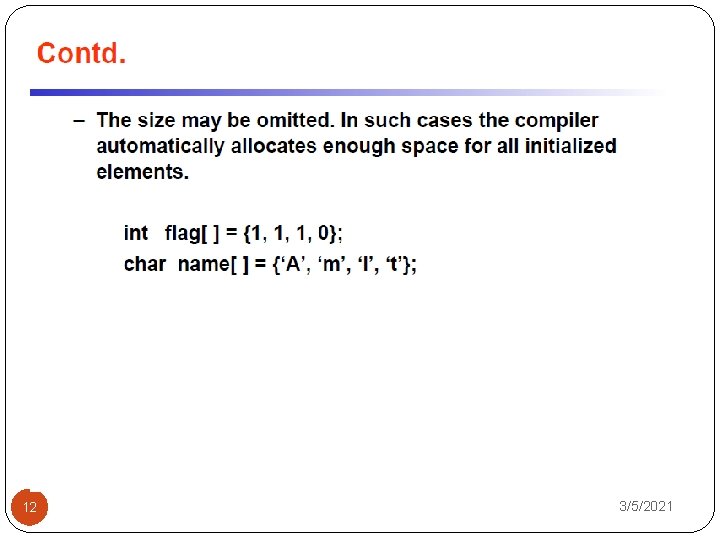
12 3/5/2021
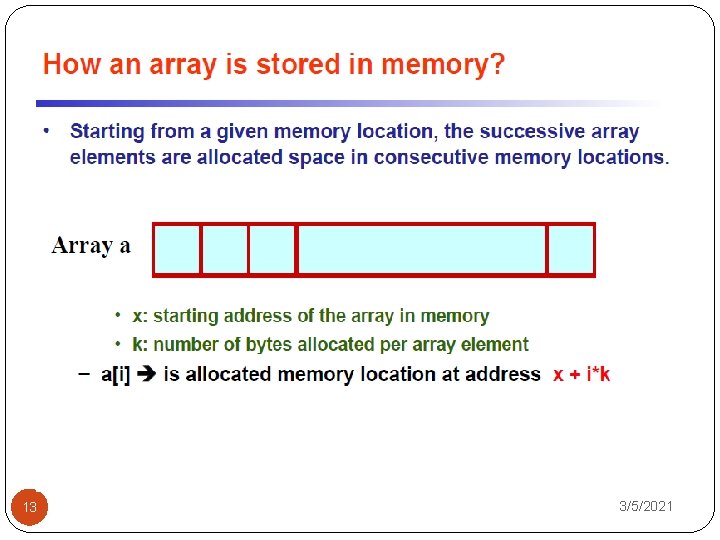
13 3/5/2021
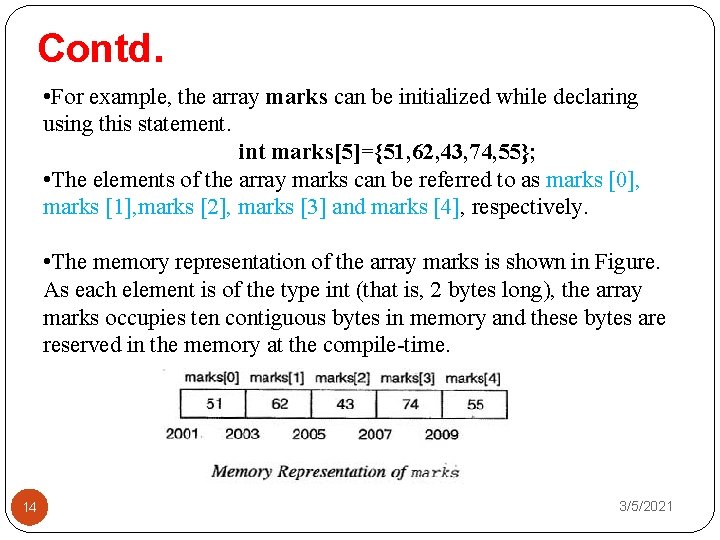
Contd. • For example, the array marks can be initialized while declaring using this statement. int marks[5]={51, 62, 43, 74, 55}; • The elements of the array marks can be referred to as marks [0], marks [1], marks [2], marks [3] and marks [4], respectively. • The memory representation of the array marks is shown in Figure. As each element is of the type int (that is, 2 bytes long), the array marks occupies ten contiguous bytes in memory and these bytes are reserved in the memory at the compile-time. 14 3/5/2021
![Problem double percentage50 If this array percentage is stored in memory starting from address Problem double percentage[50]; If this array percentage is stored in memory starting from address](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-15.jpg)
Problem double percentage[50]; If this array percentage is stored in memory starting from address 3000. then what will be the address of percentage[20] element. Ans: 3000+20*8= 3160 What will be size of array? Ans: 400 15 3/5/2021
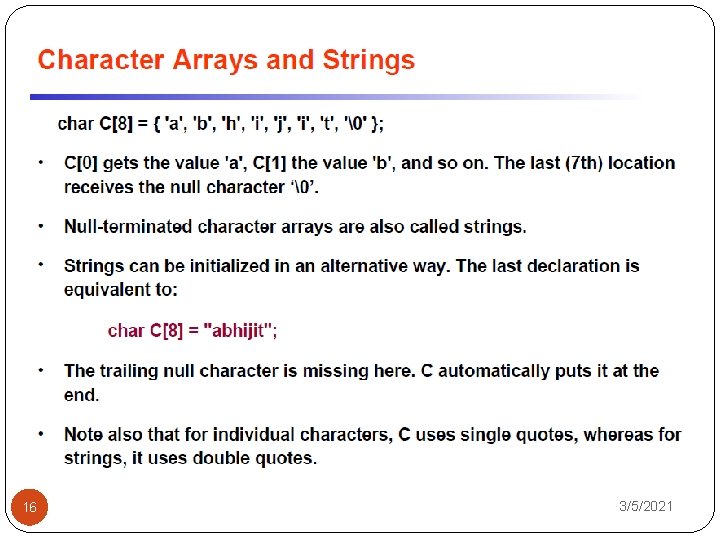
16 3/5/2021
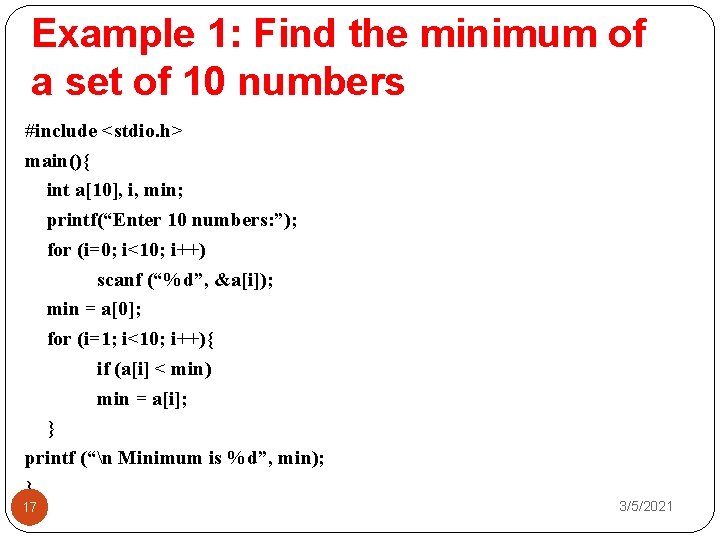
Example 1: Find the minimum of a set of 10 numbers #include <stdio. h> main(){ int a[10], i, min; printf(“Enter 10 numbers: ”); for (i=0; i<10; i++) scanf (“%d”, &a[i]); min = a[0]; for (i=1; i<10; i++){ if (a[i] < min) min = a[i]; } printf (“n Minimum is %d”, min); } 17 3/5/2021
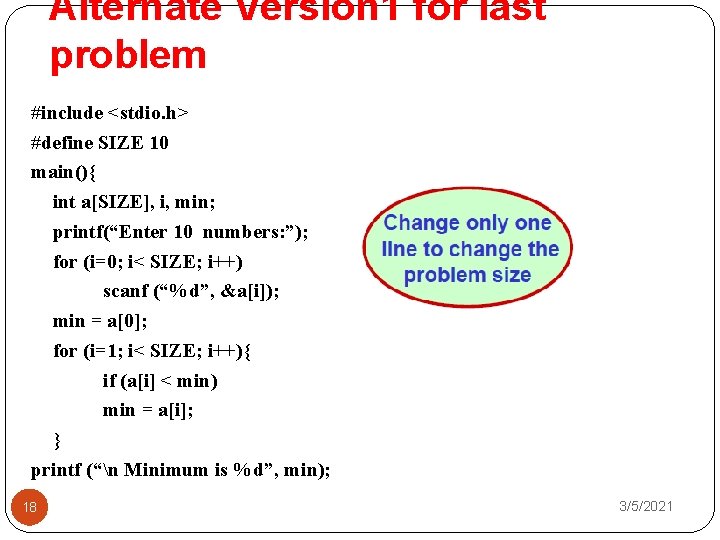
Alternate Version 1 for last problem #include <stdio. h> #define SIZE 10 main(){ int a[SIZE], i, min; printf(“Enter 10 numbers: ”); for (i=0; i< SIZE; i++) scanf (“%d”, &a[i]); min = a[0]; for (i=1; i< SIZE; i++){ if (a[i] < min) min = a[i]; } printf (“n Minimum is %d”, min); 18} 3/5/2021
![Alternate Version 2 for last problem include stdio h main int a500 i min Alternate Version 2 for last problem #include <stdio. h> main(){ int a[500], i, min;](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-19.jpg)
Alternate Version 2 for last problem #include <stdio. h> main(){ int a[500], i, min; printf(“Enter number of elements: ”); scanf(“%d”, &n); printf(“Enter n numbers: ”); for (i=0; i< n; i++) scanf (“%d”, &a[i]); min = a[0]; for (i=1; i< n; i++){ if (a[i] < min) min = a[i]; } 19 printf (“n Minimum is %d”, min); } 3/5/2021
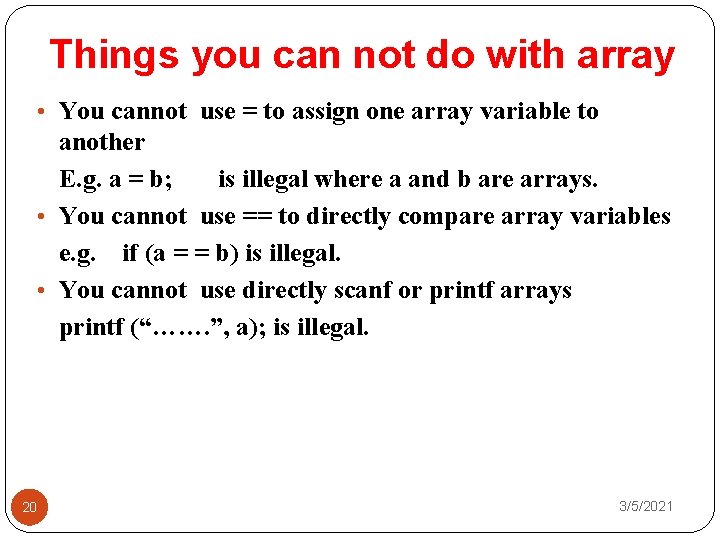
Things you can not do with array • You cannot use = to assign one array variable to another E. g. a = b; is illegal where a and b are arrays. • You cannot use == to directly compare array variables e. g. if (a = = b) is illegal. • You cannot use directly scanf or printf arrays printf (“……. ”, a); is illegal. 20 3/5/2021
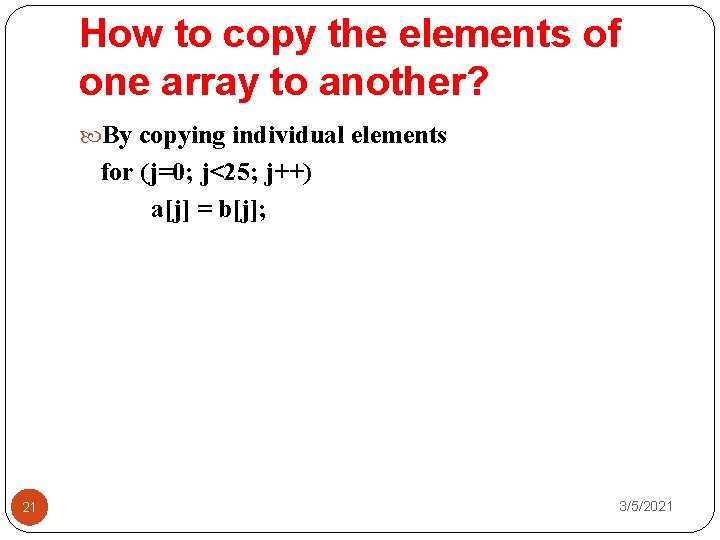
How to copy the elements of one array to another? By copying individual elements for (j=0; j<25; j++) a[j] = b[j]; 21 3/5/2021
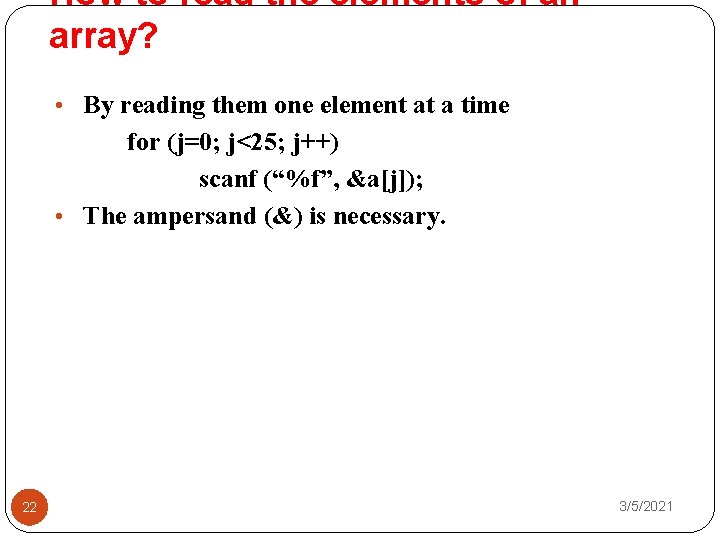
How to read the elements of an array? • By reading them one element at a time for (j=0; j<25; j++) scanf (“%f”, &a[j]); • The ampersand (&) is necessary. 22 3/5/2021
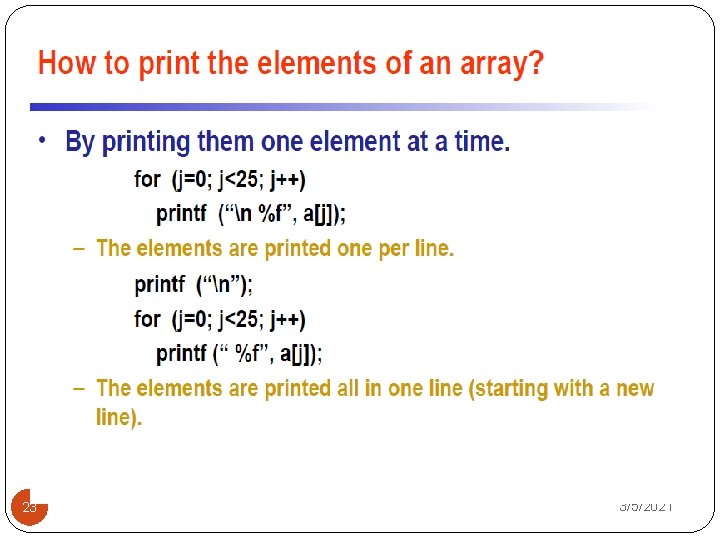
23 3/5/2021
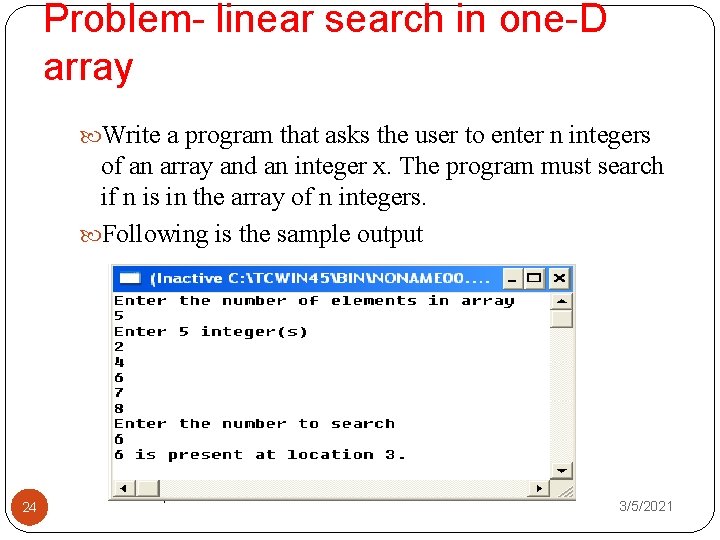
Problem- linear search in one-D array Write a program that asks the user to enter n integers of an array and an integer x. The program must search if n is in the array of n integers. Following is the sample output 24 3/5/2021
![void main 25 int array100 search c n printfEnter the number of void main() { 25 int array[100], search, c, n; printf("Enter the number of](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-25.jpg)
void main() { 25 int array[100], search, c, n; printf("Enter the number of elements in arrayn"); scanf("%d", &n); printf("Enter %d integer(s)n", n); for (c = 0; c < n; c++) scanf("%d", &array[c]); printf("Enter the number to searchn"); scanf("%d", &search); for (c = 0; c < n; c++) { if (array[c] == search){ /* if required element found */ printf("%d is present at location %d. n", search, c+1); break; } } if (c == n) printf("%d is not present in array. n", search); 3/5/2021 }
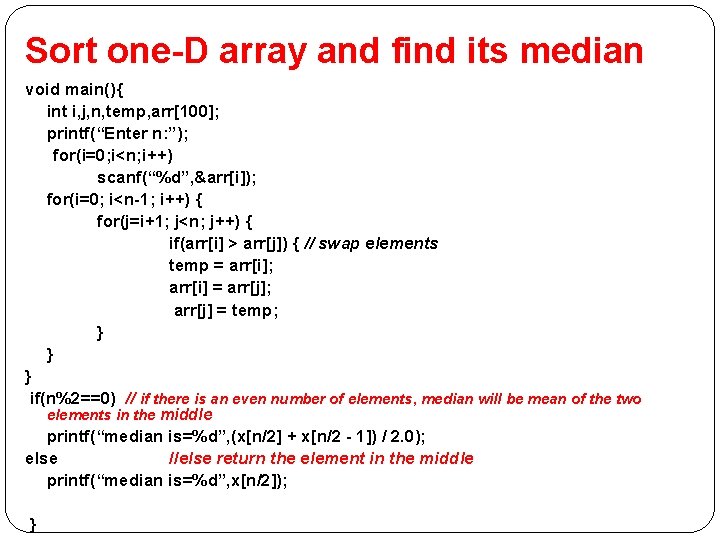
Sort one-D array and find its median void main(){ int i, j, n, temp, arr[100]; printf(“Enter n: ”); for(i=0; i<n; i++) scanf(“%d”, &arr[i]); for(i=0; i<n-1; i++) { for(j=i+1; j<n; j++) { if(arr[i] > arr[j]) { // swap elements temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } } } if(n%2==0) // if there is an even number of elements, median will be mean of the two elements in the middle printf(“median is=%d”, (x[n/2] + x[n/2 - 1]) / 2. 0); else //else return the element in the middle printf(“median is=%d”, x[n/2]); }
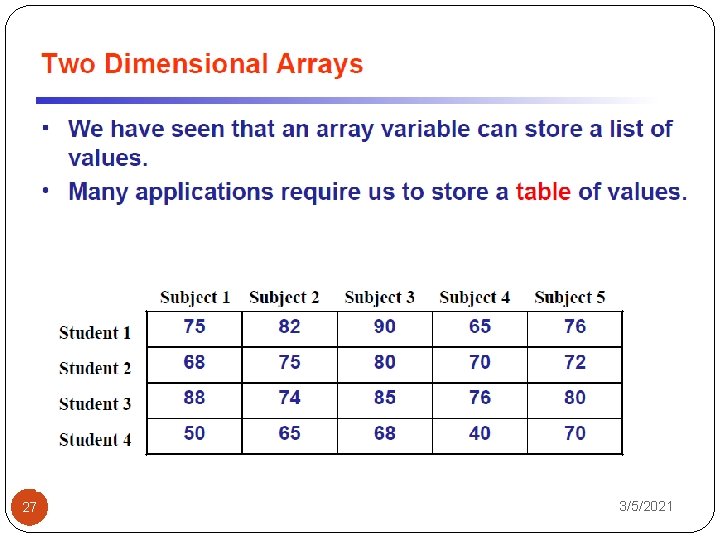
27 3/5/2021
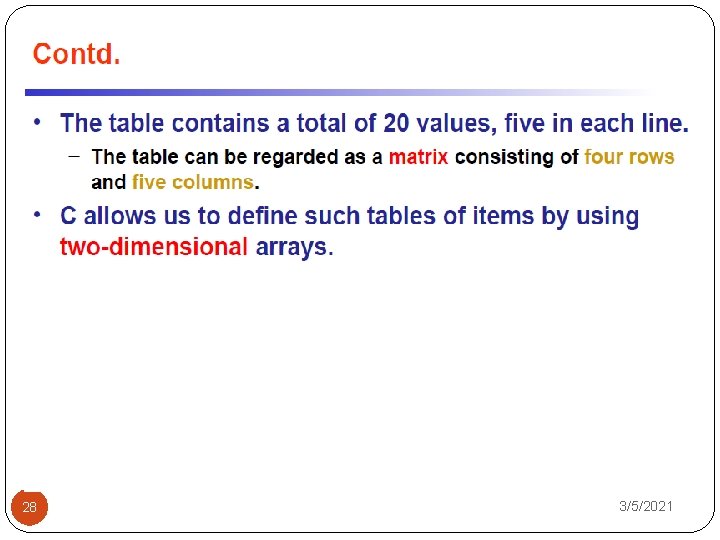
28 3/5/2021
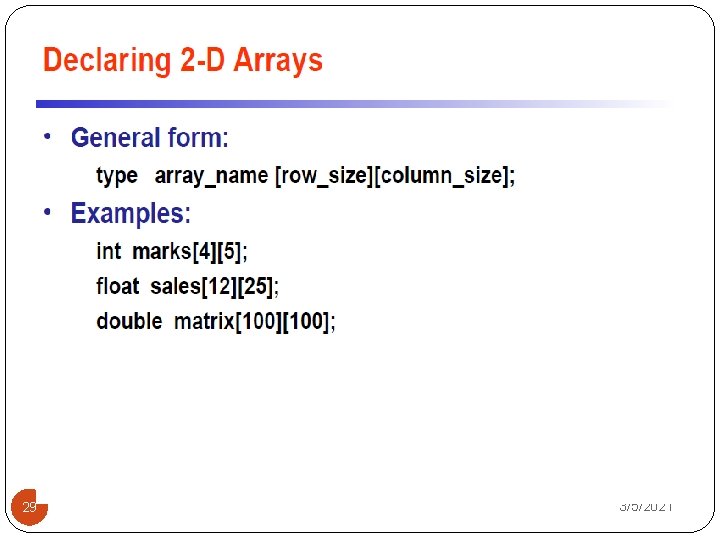
29 3/5/2021
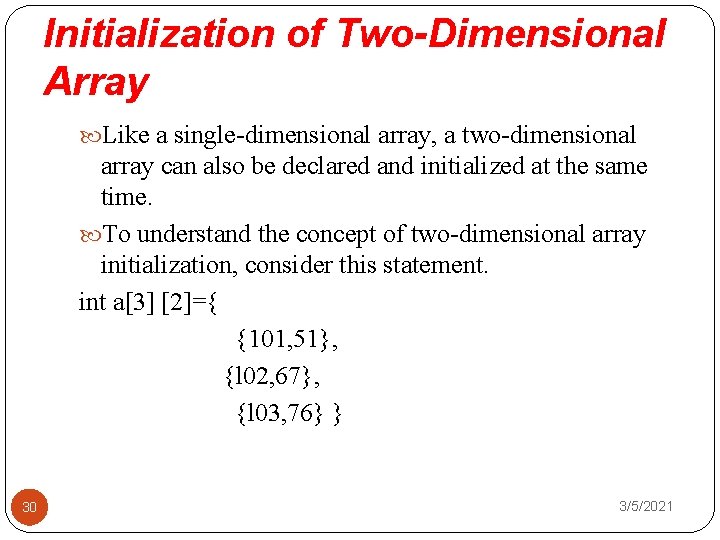
Initialization of Two-Dimensional Array Like a single-dimensional array, a two-dimensional array can also be declared and initialized at the same time. To understand the concept of two-dimensional array initialization, consider this statement. int a[3] [2]={ {101, 51}, {l 02, 67}, {l 03, 76} } 30 3/5/2021
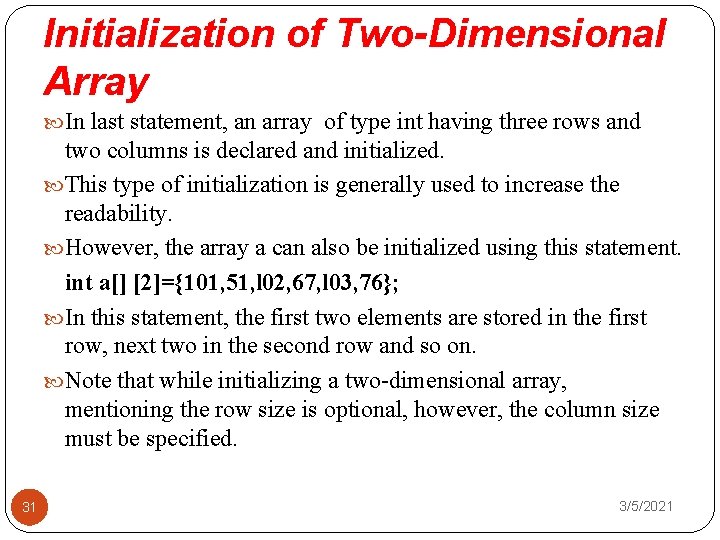
Initialization of Two-Dimensional Array In last statement, an array of type int having three rows and two columns is declared and initialized. This type of initialization is generally used to increase the readability. However, the array a can also be initialized using this statement. int a[] [2]={101, 51, l 02, 67, l 03, 76}; In this statement, the first two elements are stored in the first row, next two in the second row and so on. Note that while initializing a two-dimensional array, mentioning the row size is optional, however, the column size must be specified. 31 3/5/2021
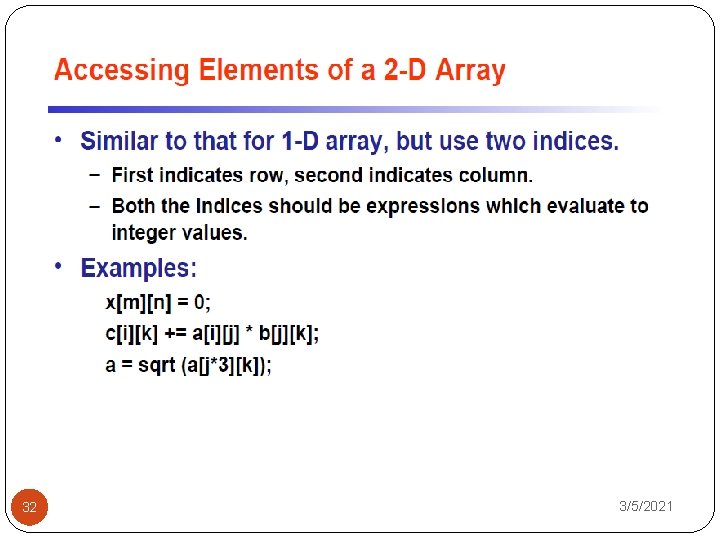
32 3/5/2021
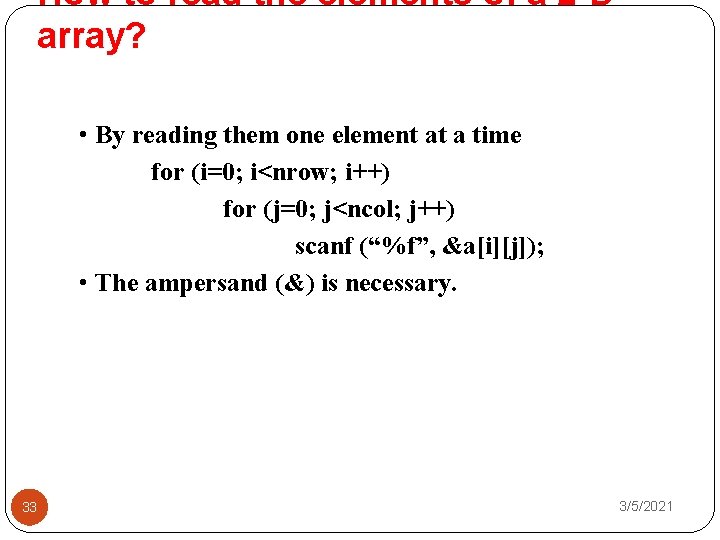
How to read the elements of a 2 -D array? • By reading them one element at a time for (i=0; i<nrow; i++) for (j=0; j<ncol; j++) scanf (“%f”, &a[i][j]); • The ampersand (&) is necessary. 33 3/5/2021
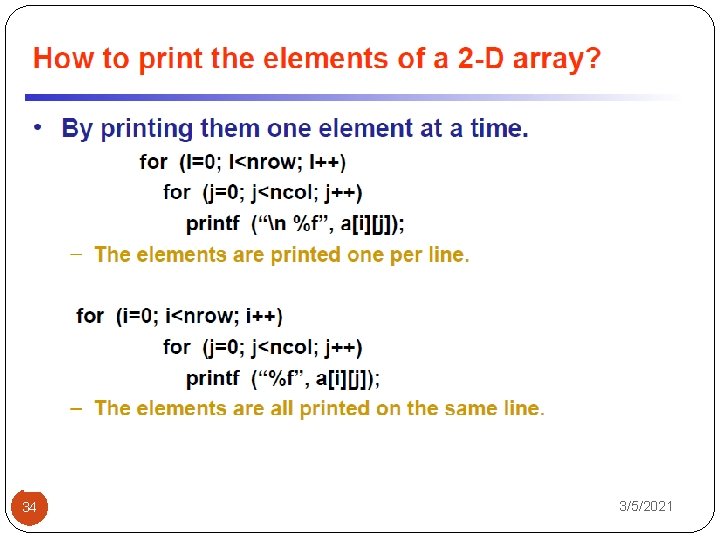
34 3/5/2021
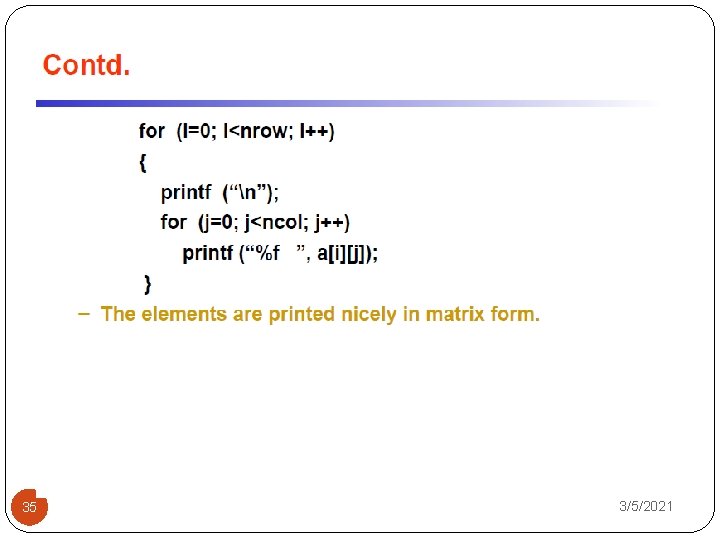
35 3/5/2021
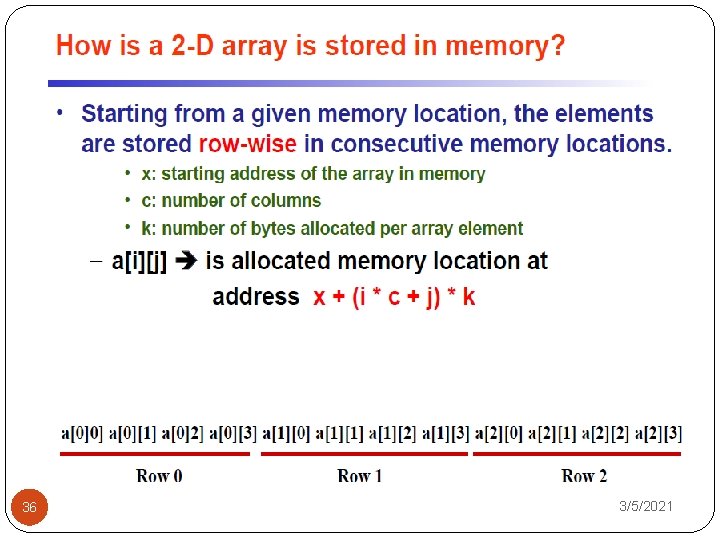
36 3/5/2021
![int a 2101 51 l 02 67 l 03 76 For • int a[] [2]={101, 51, l 02, 67, l 03, 76}; • For](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-37.jpg)
• int a[] [2]={101, 51, l 02, 67, l 03, 76}; • For example, the elements of array are referred to as a [0], a [0] [1], a [1] [0], a [1], a [2] [0] and a [2] [1] respectively. • Generally, two-dimensional arrays are represented with the help of a matrix. • However, in actual implementation, two-dimensional arrays are always allocated contiguous blocks of memory. • Figure shows the matrix and memory representation of two-dimensional array a. 37 3/5/2021
![Problem long double array1015 If base address is 2000 then what will be the Problem long double array[10][15]; If base address is 2000 then what will be the](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-38.jpg)
Problem long double array[10][15]; If base address is 2000 then what will be the address of Array[5][6] element; Ans: 38 2000+(5*15+6)*10=2810 3/5/2021
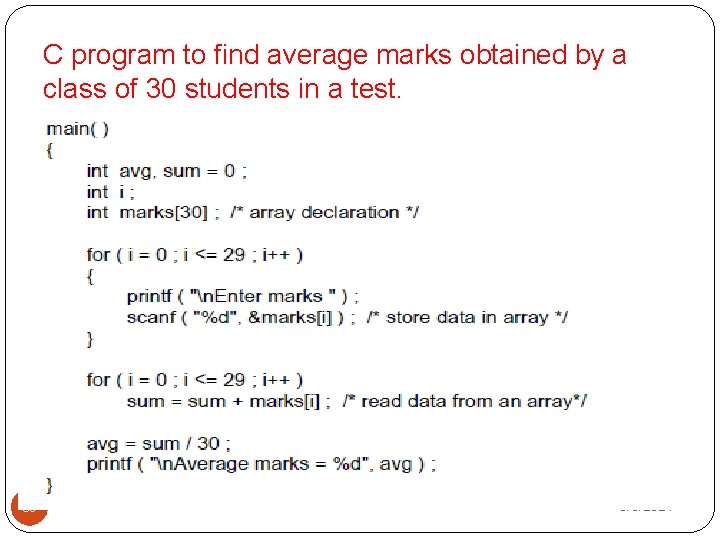
C program to find average marks obtained by a class of 30 students in a test. 39 3/5/2021
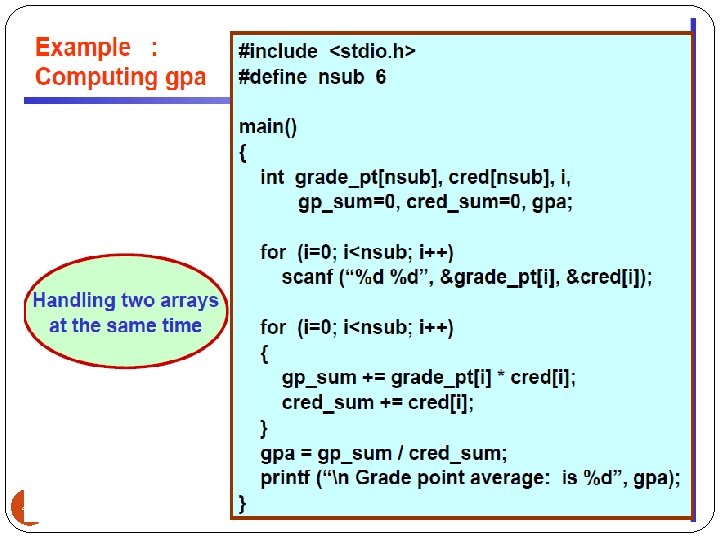
40 3/5/2021
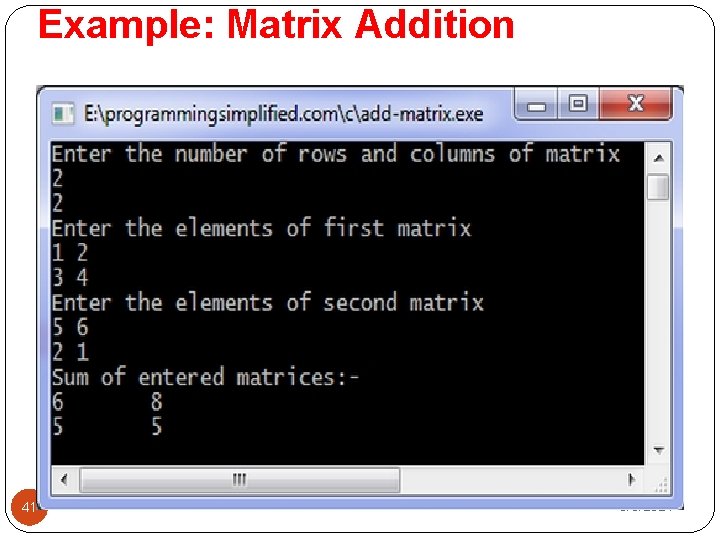
Example: Matrix Addition 41 3/5/2021
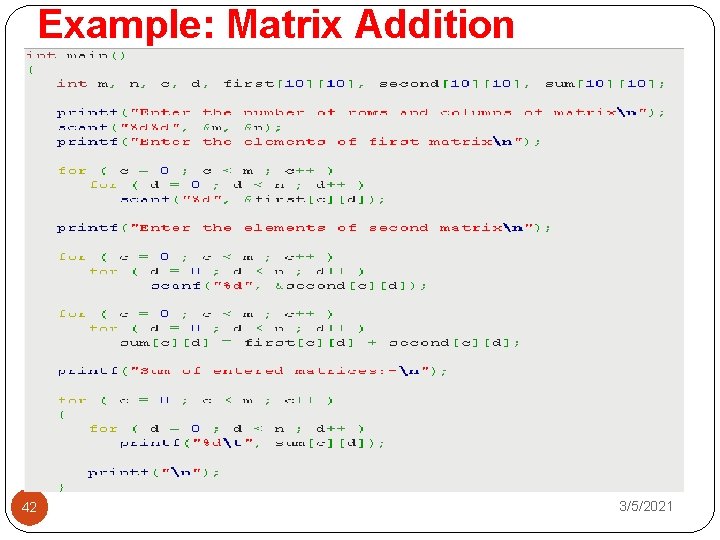
Example: Matrix Addition 42 3/5/2021
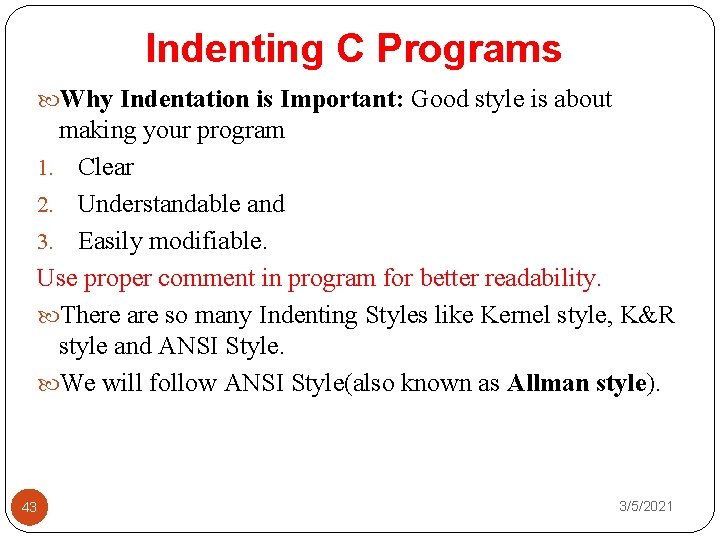
Indenting C Programs Why Indentation is Important: Good style is about making your program 1. Clear 2. Understandable and 3. Easily modifiable. Use proper comment in program for better readability. There are so many Indenting Styles like Kernel style, K&R style and ANSI Style. We will follow ANSI Style(also known as Allman style). 43 3/5/2021
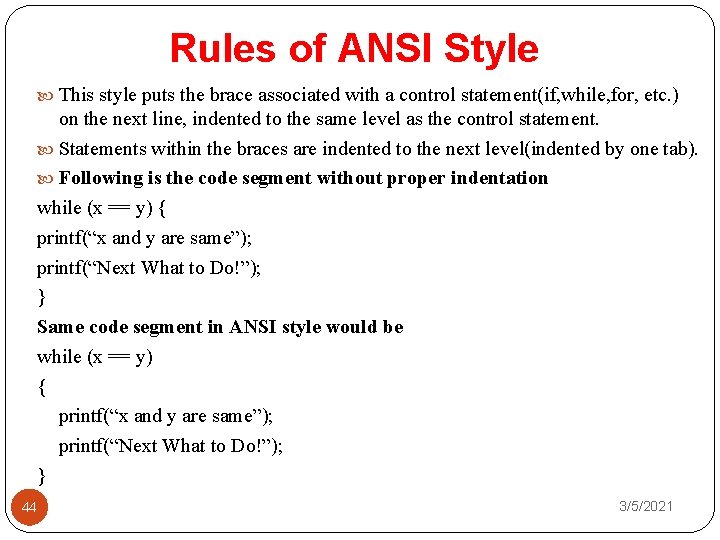
Rules of ANSI Style This style puts the brace associated with a control statement(if, while, for, etc. ) on the next line, indented to the same level as the control statement. Statements within the braces are indented to the next level(indented by one tab). Following is the code segment without proper indentation while (x == y) { printf(“x and y are same”); printf(“Next What to Do!”); } Same code segment in ANSI style would be while (x == y) { printf(“x and y are same”); printf(“Next What to Do!”); } 44 3/5/2021
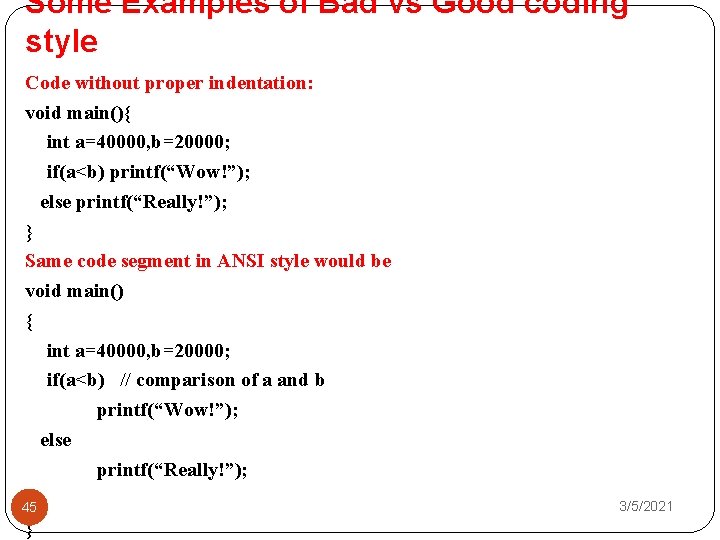
Some Examples of Bad vs Good coding style Code without proper indentation: void main(){ int a=40000, b=20000; if(a<b) printf(“Wow!”); else printf(“Really!”); } Same code segment in ANSI style would be void main() { int a=40000, b=20000; if(a<b) // comparison of a and b printf(“Wow!”); else printf(“Really!”); } 45 } 3/5/2021
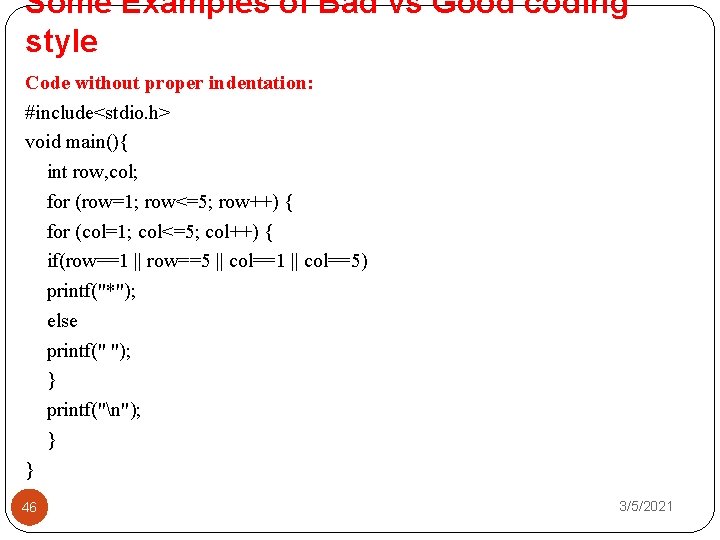
Some Examples of Bad vs Good coding style Code without proper indentation: #include<stdio. h> void main(){ int row, col; for (row=1; row<=5; row++) { for (col=1; col<=5; col++) { if(row==1 || row==5 || col==1 || col==5) printf("*"); else printf(" "); } printf("n"); } } 46 3/5/2021
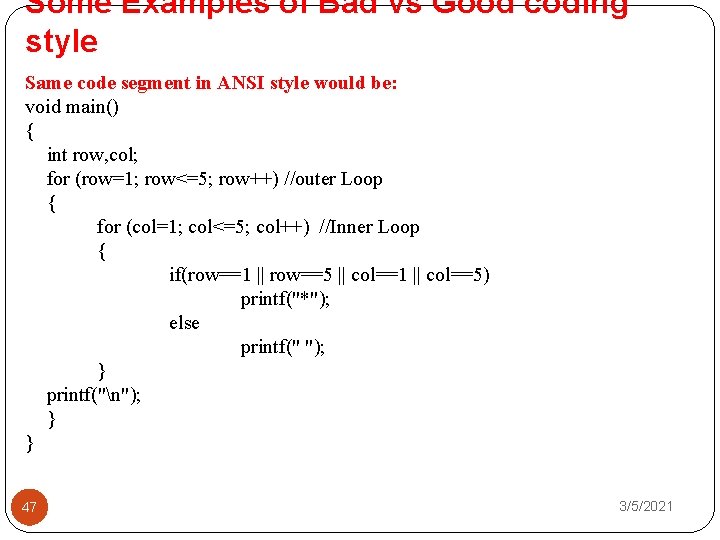
Some Examples of Bad vs Good coding style Same code segment in ANSI style would be: void main() { int row, col; for (row=1; row<=5; row++) //outer Loop { for (col=1; col<=5; col++) //Inner Loop { if(row==1 || row==5 || col==1 || col==5) printf("*"); else printf(" "); } printf("n"); } } 47 3/5/2021
![OP main int arr12 13 14 15 16 printfnd d sizeofarr sizeofarr0 O/P? main() { int arr[]=(12, 13, 14, 15, 16}; printf(“n%d , %d”, sizeof(arr), sizeof(arr[0]));](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-48.jpg)
O/P? main() { int arr[]=(12, 13, 14, 15, 16}; printf(“n%d , %d”, sizeof(arr), sizeof(arr[0])); } O/p 10, 2 48 3/5/2021
![OP main float a 12 4 2 3 4 5 6 7 O/P? main() { float a[]= { 12. 4, 2. 3, 4. 5, 6. 7};](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-49.jpg)
O/P? main() { float a[]= { 12. 4, 2. 3, 4. 5, 6. 7}; printf(“n %d”, sizeof(a)/sizeof(a[0])); } O/p 16/4=4 49 3/5/2021
![Op main int three3 2 4 3 6 8 O/p? main( ) { int three[3][ ] = { 2, 4, 3, 6, 8,](https://slidetodoc.com/presentation_image_h/24c522f9fde2d4681f27c008face25a8/image-50.jpg)
O/p? main( ) { int three[3][ ] = { 2, 4, 3, 6, 8, 2, 2, 3 , 1 } ; printf ( "n%d", three[1][1] ) ; } Syntax error 50 3/5/2021
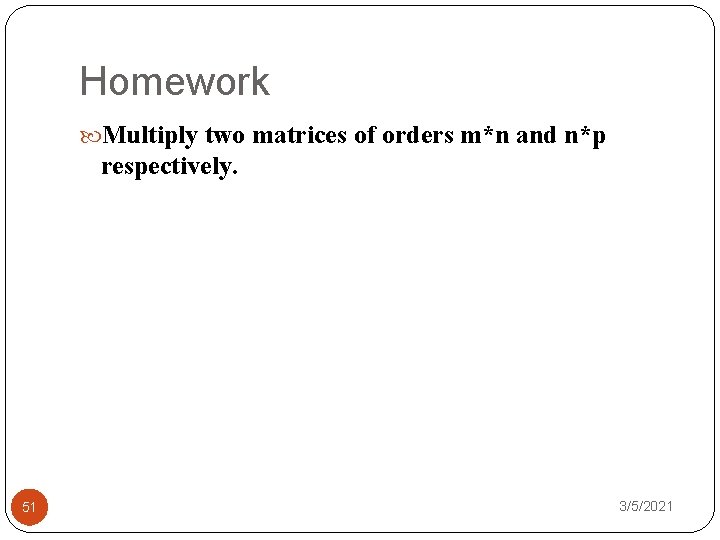
Homework Multiply two matrices of orders m*n and n*p respectively. 51 3/5/2021