CS 2073 Computer Programming w Eng Applications Ch
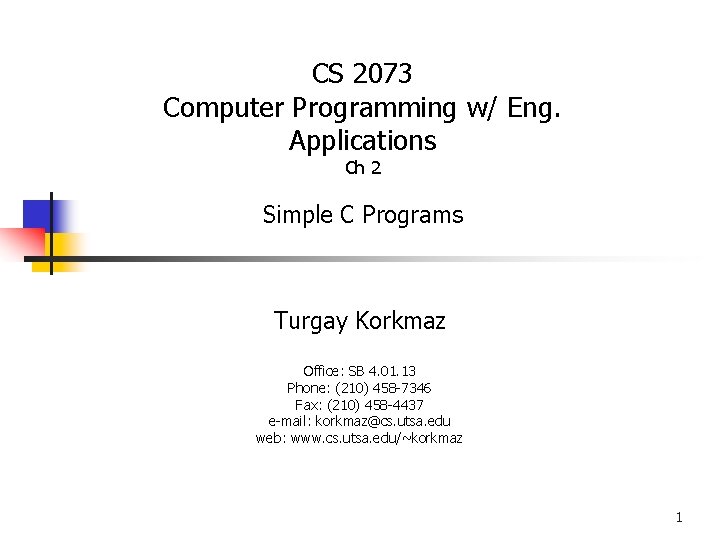
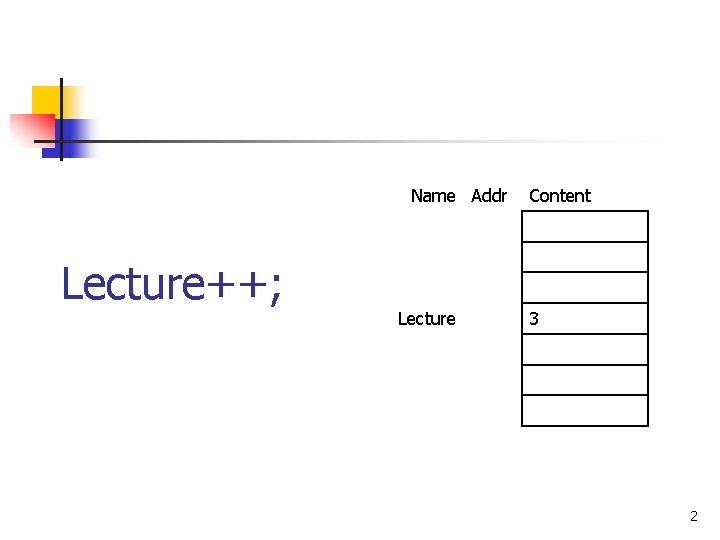
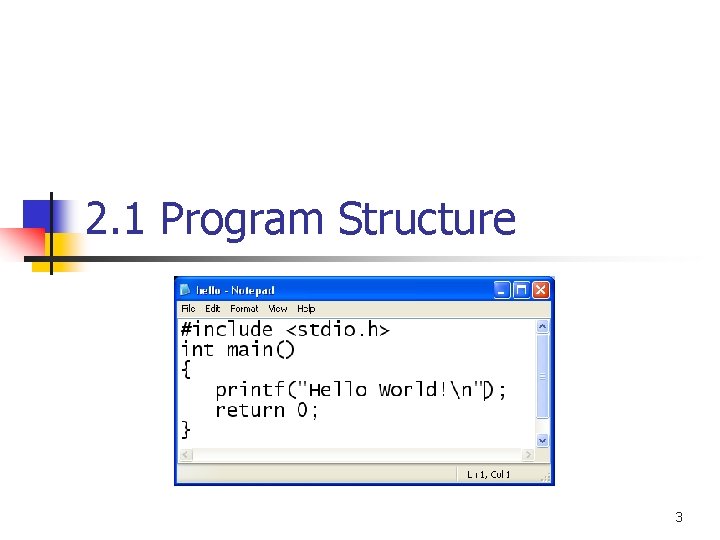
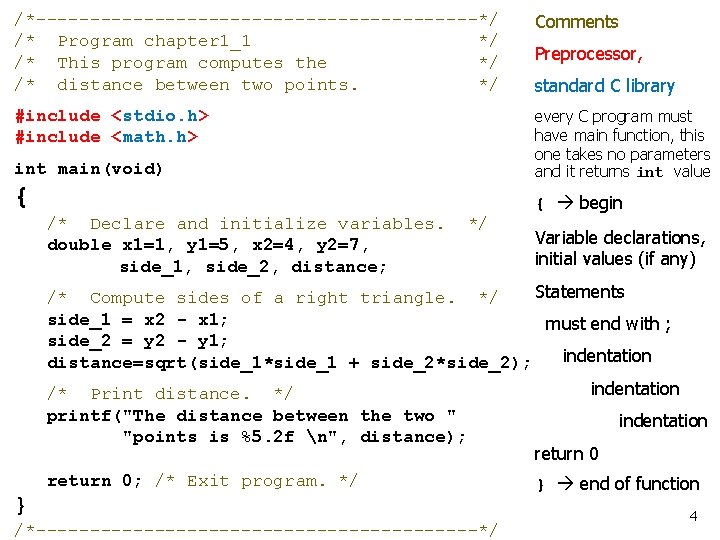
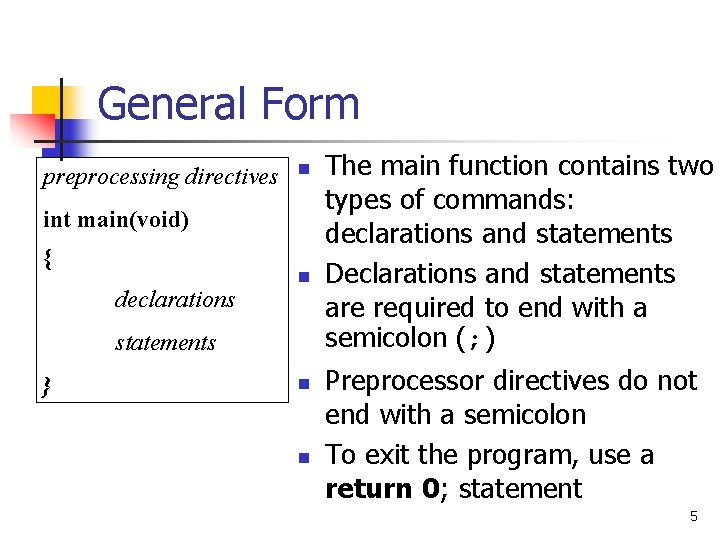
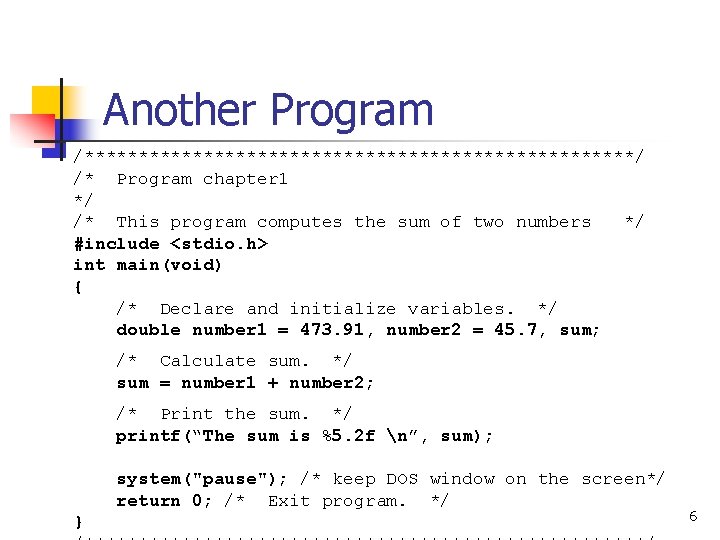
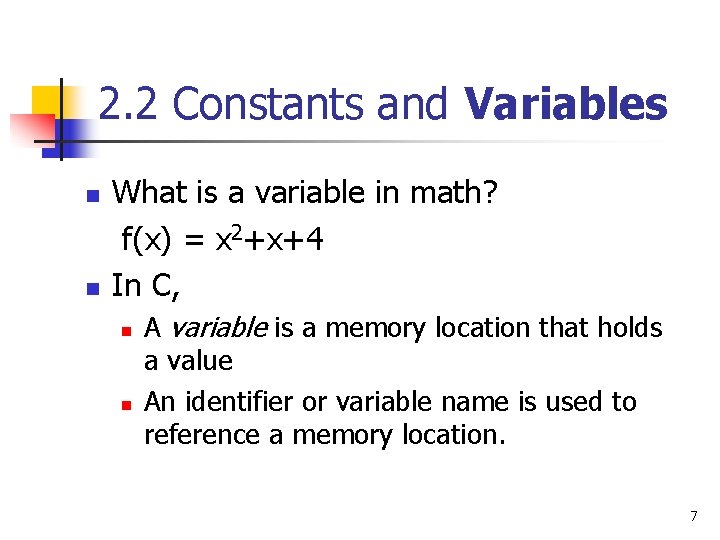
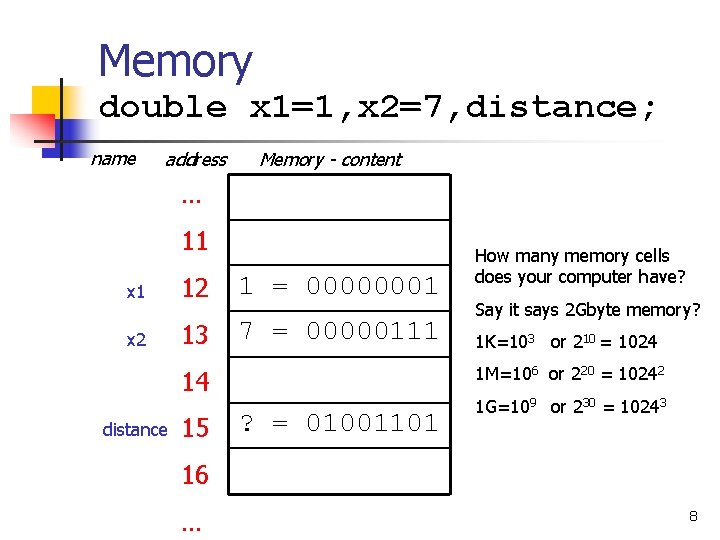
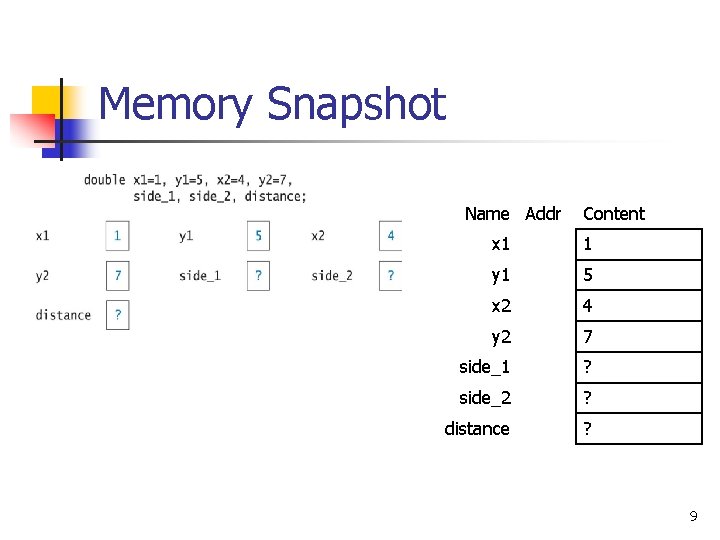
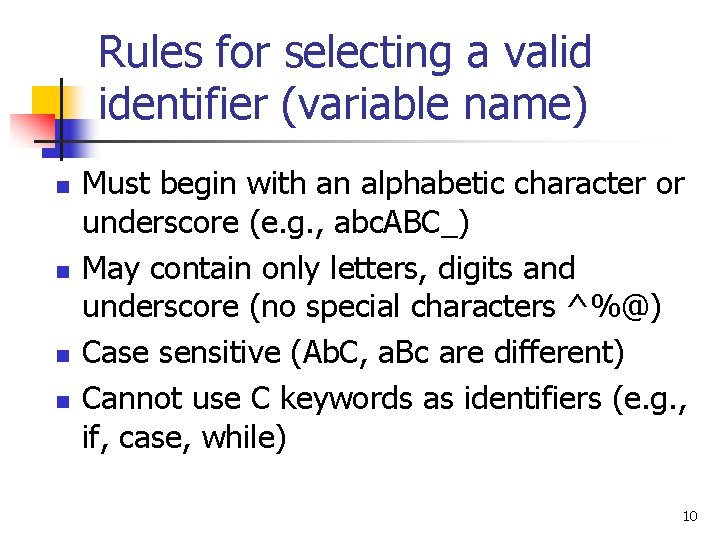
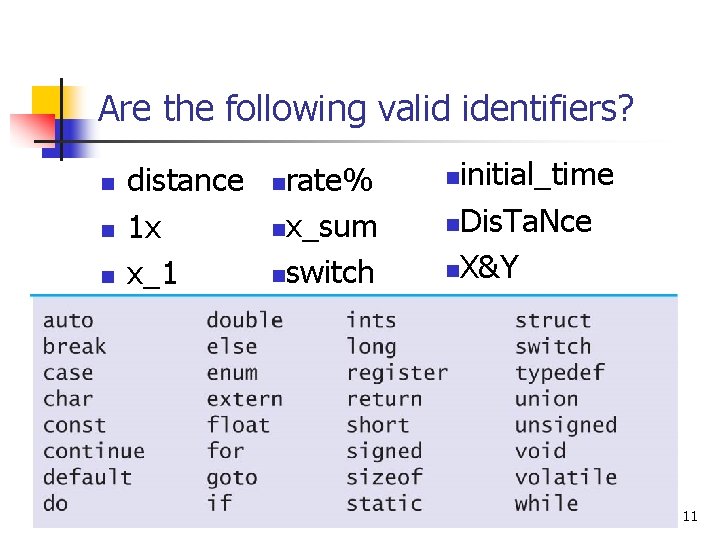
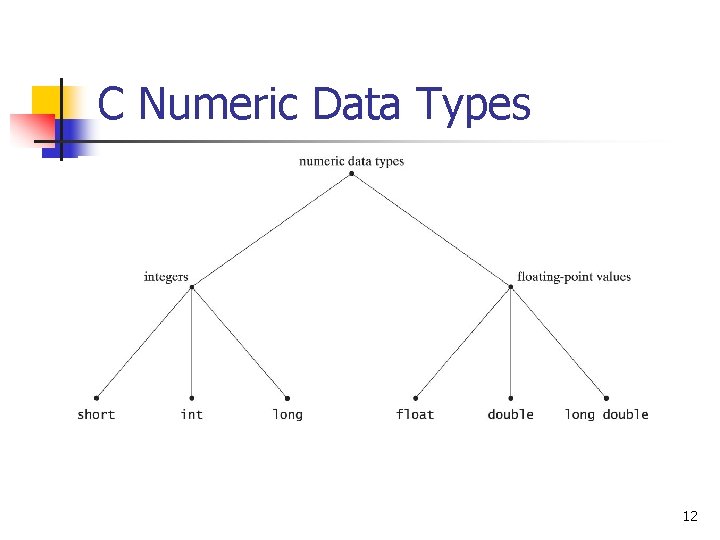
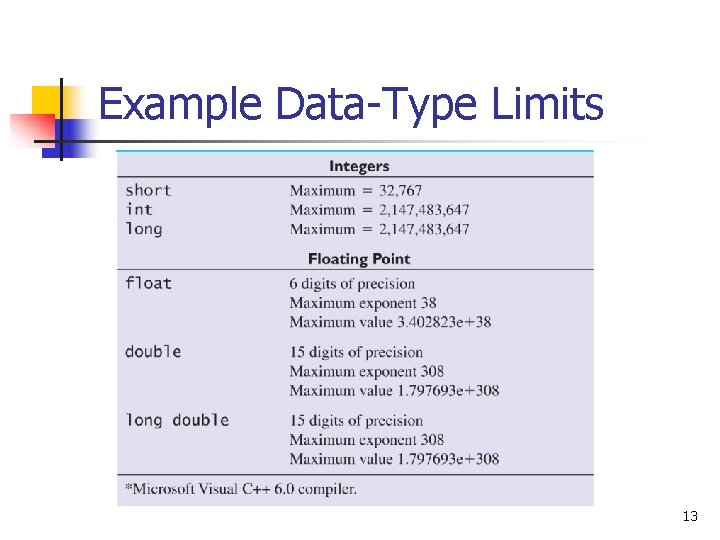
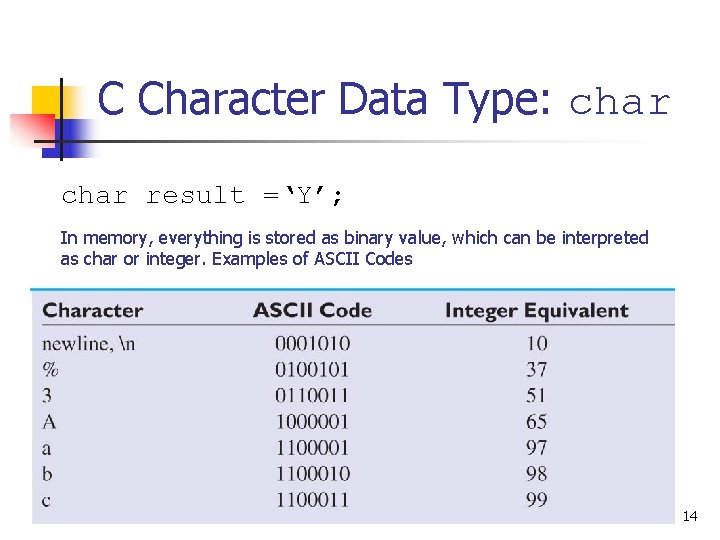
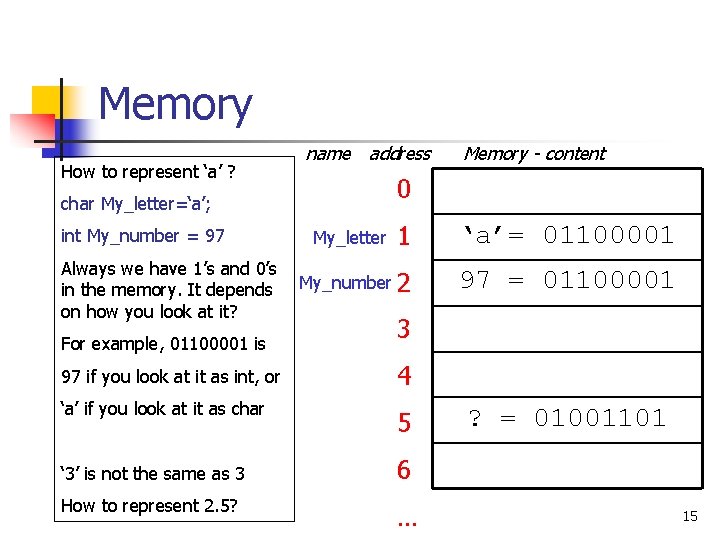
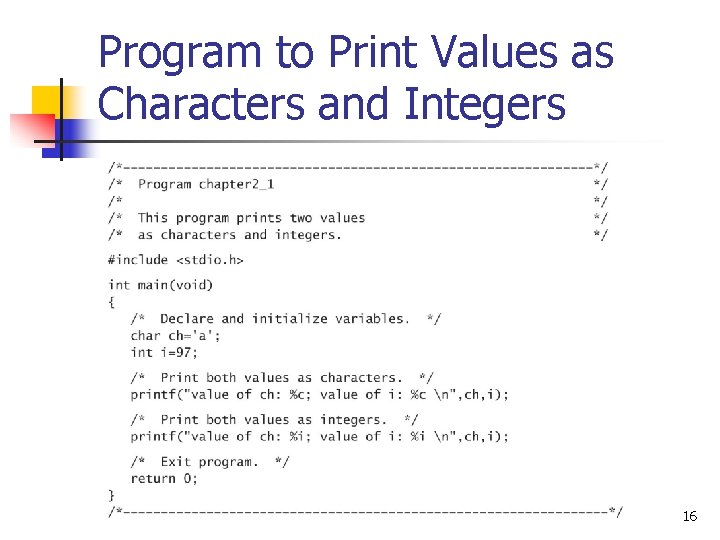
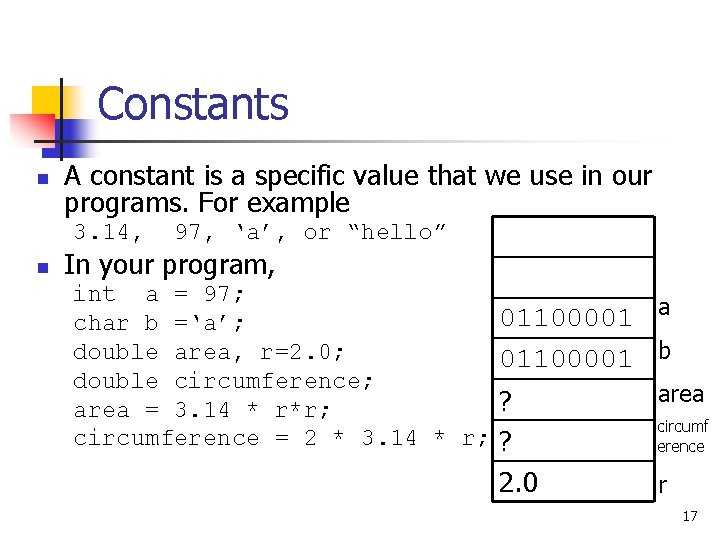
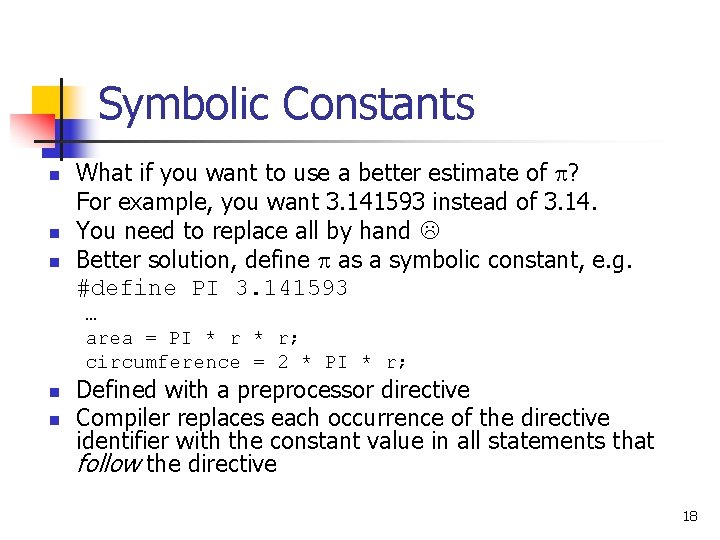
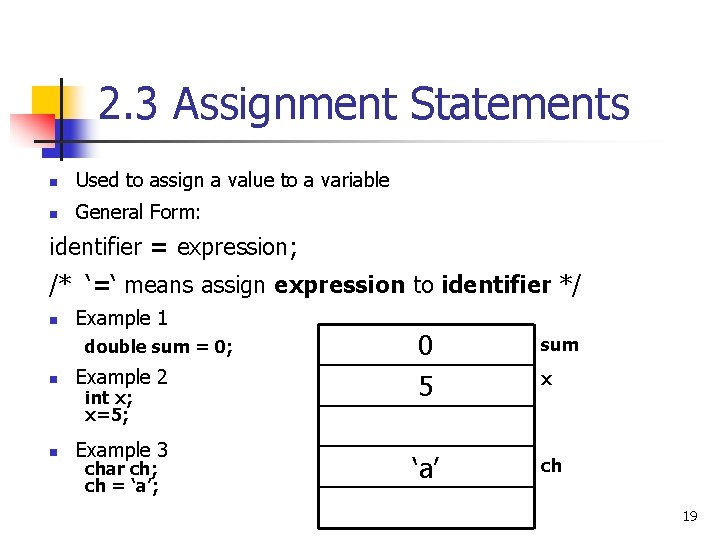
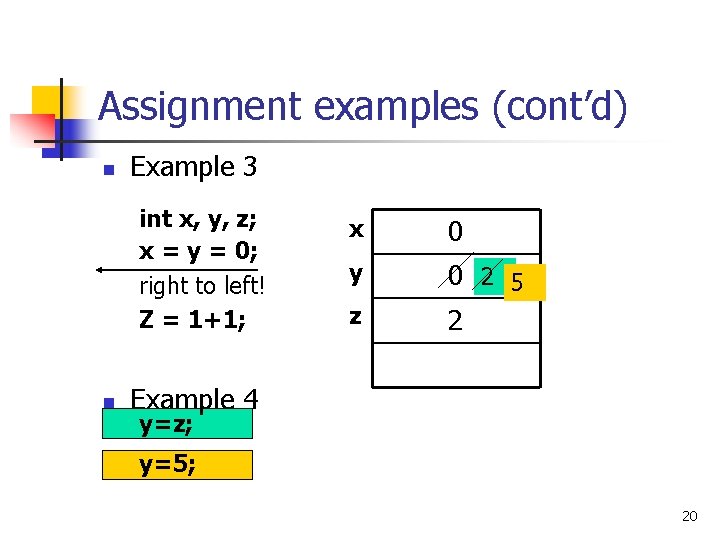
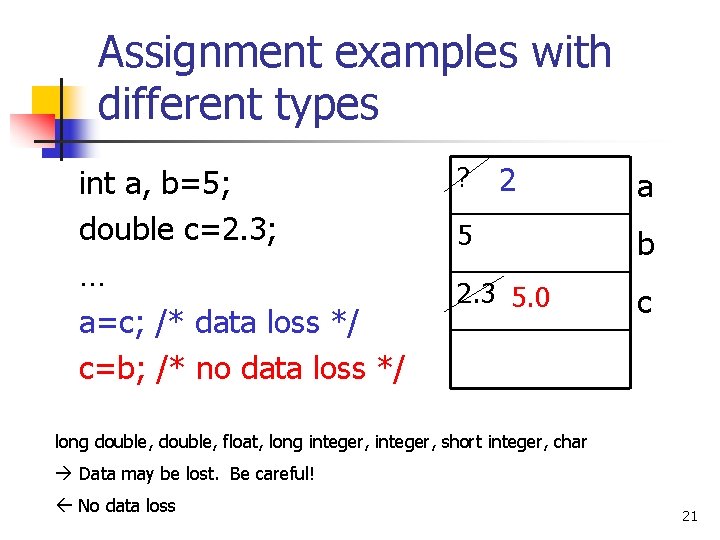
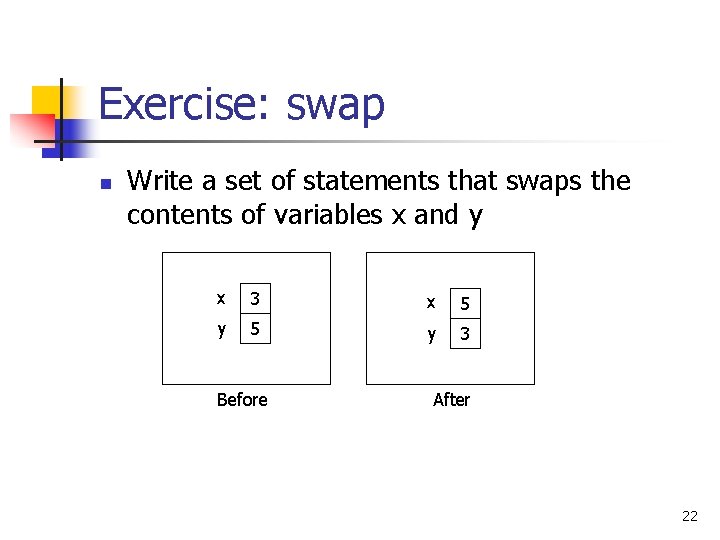
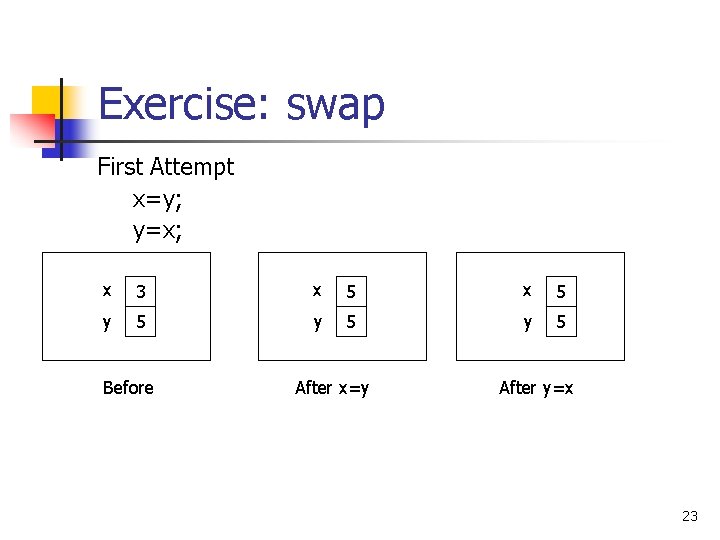
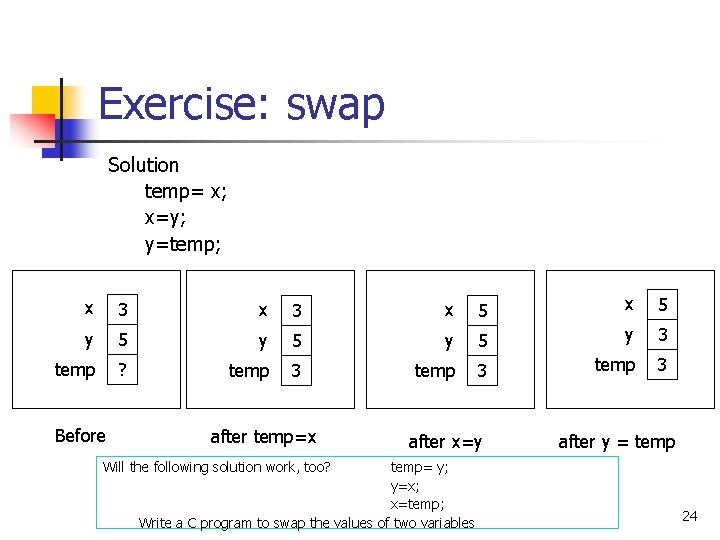
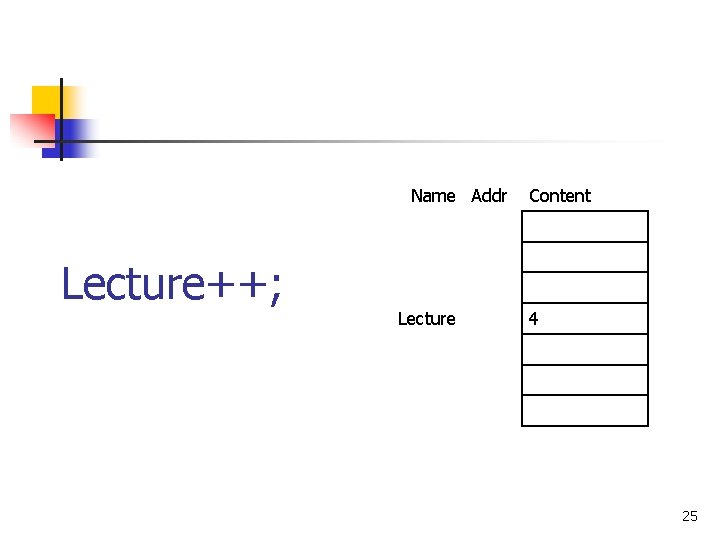
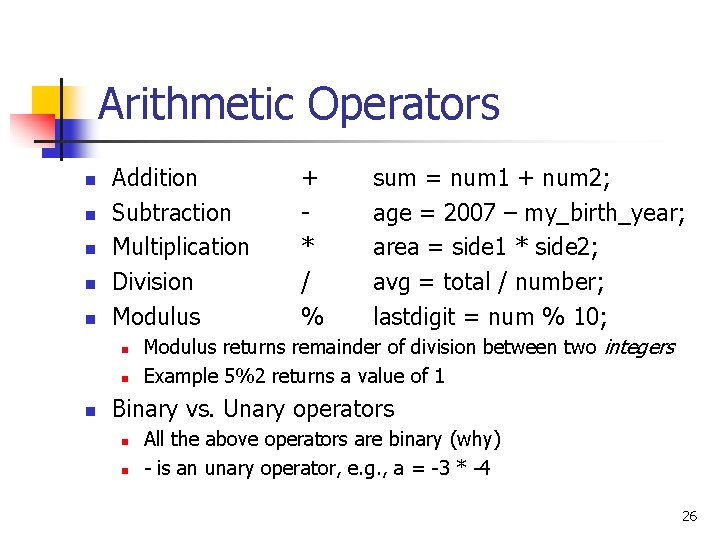
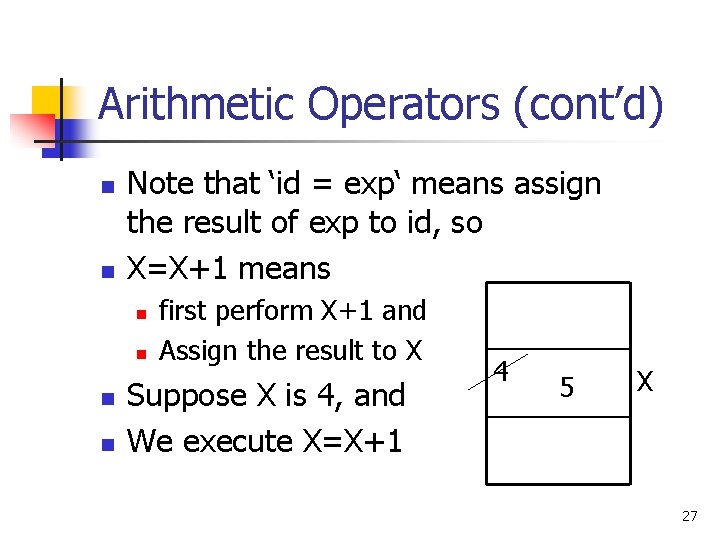
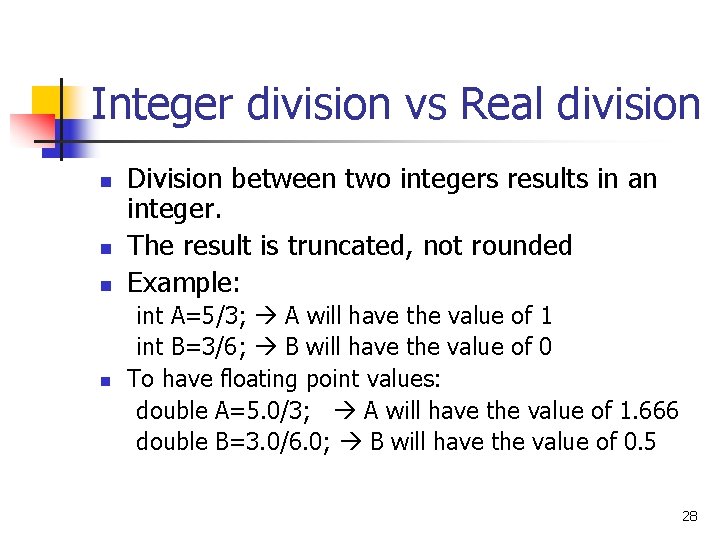
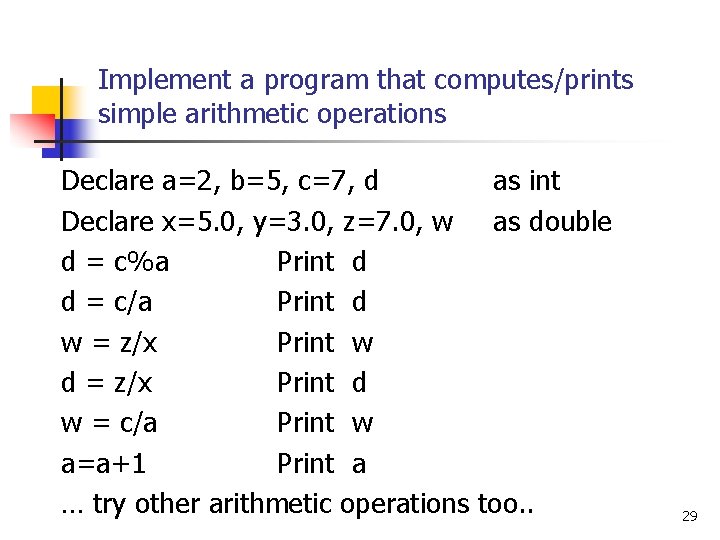
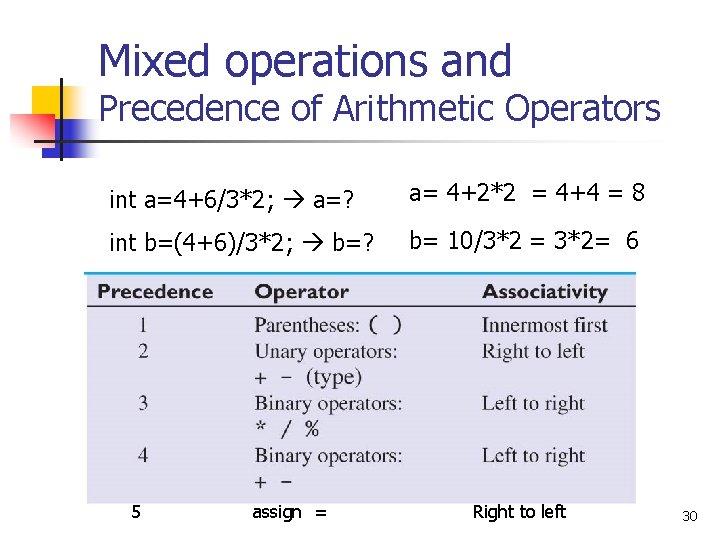
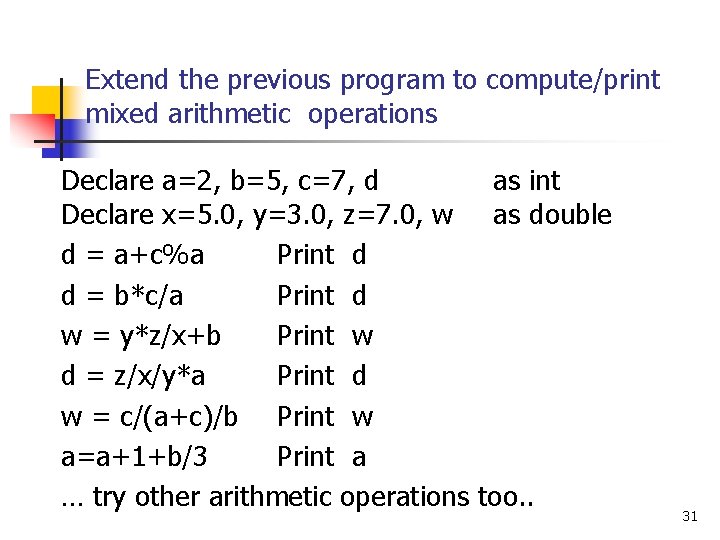
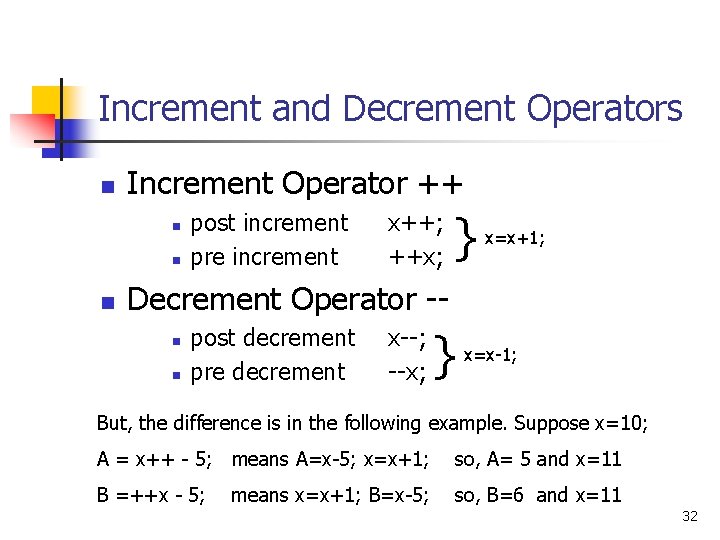
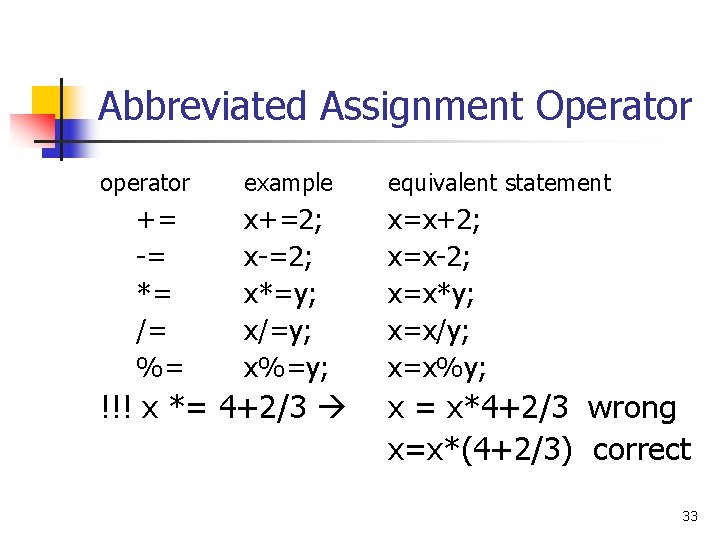
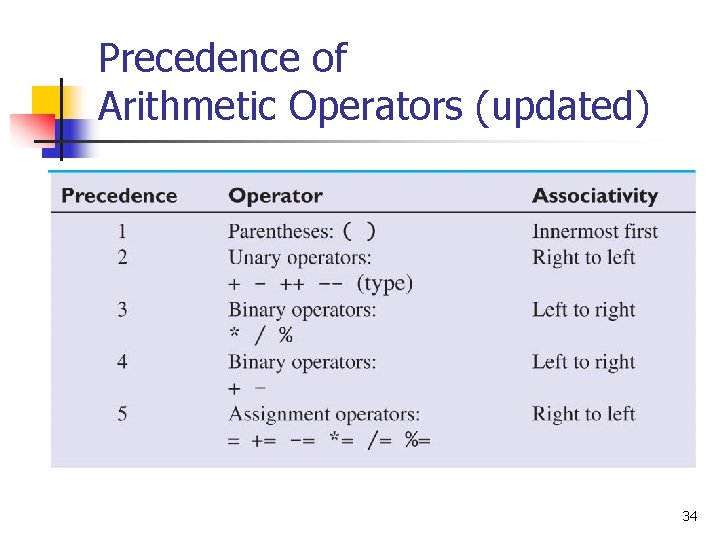
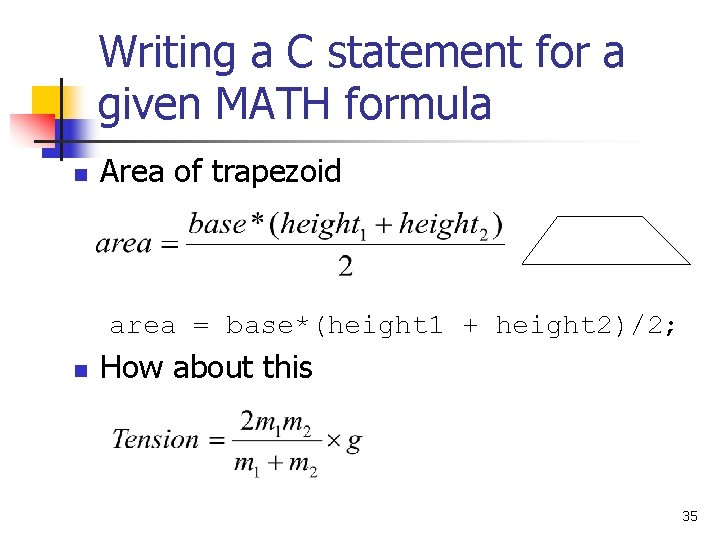
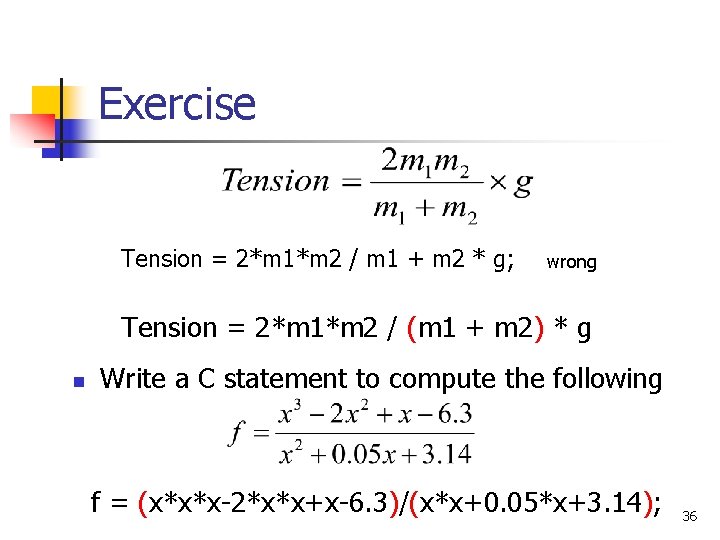
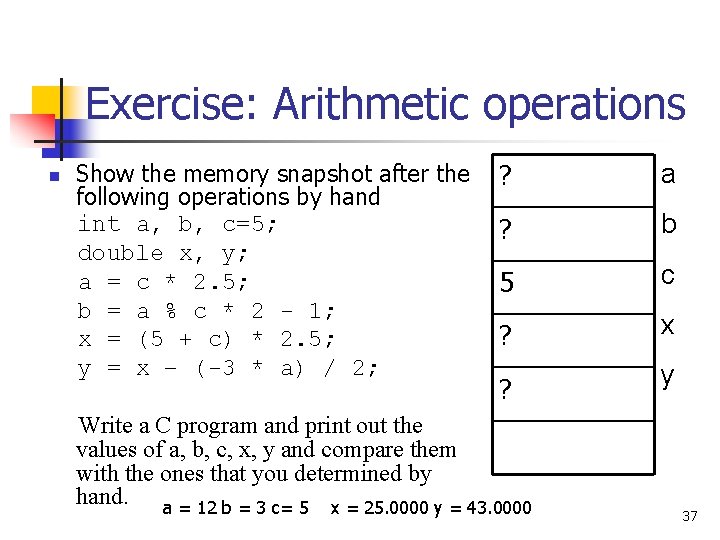
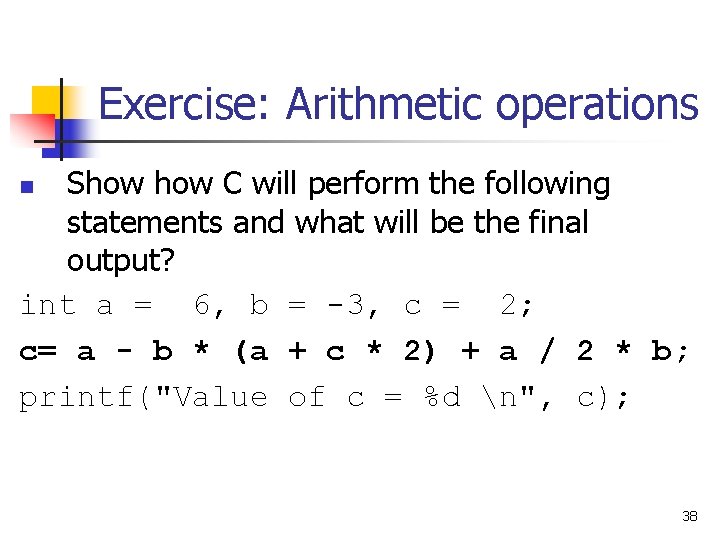
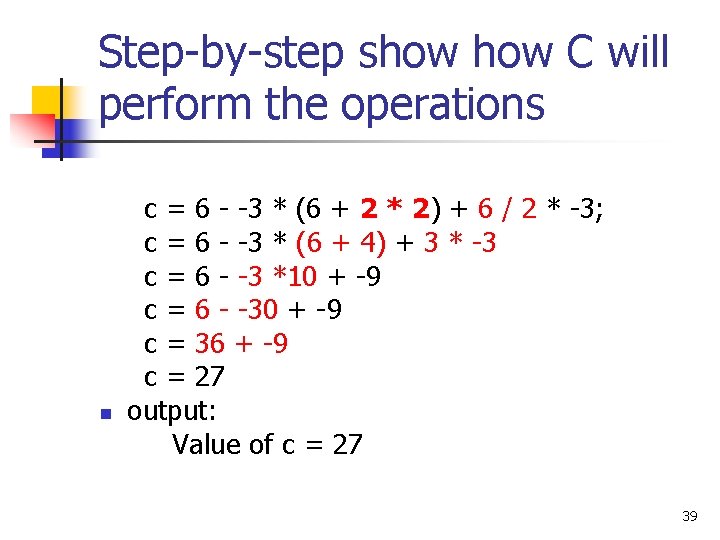
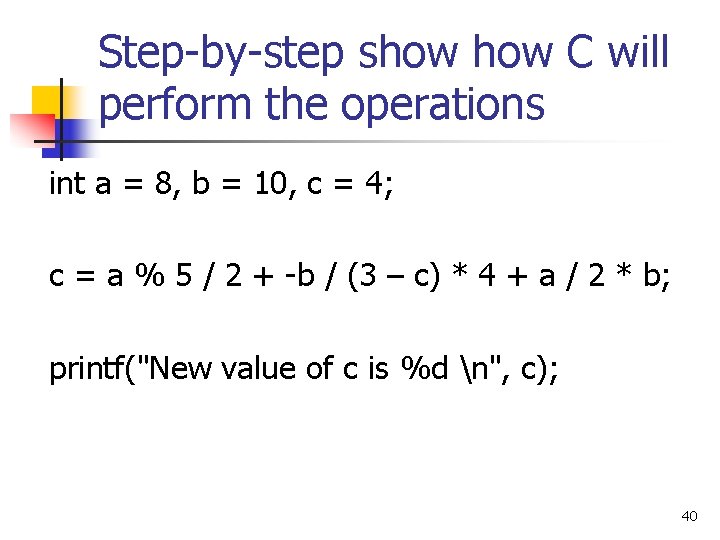
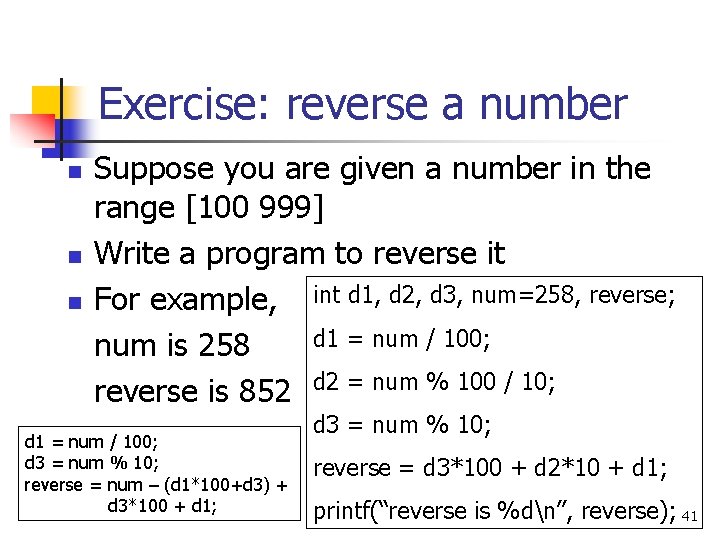
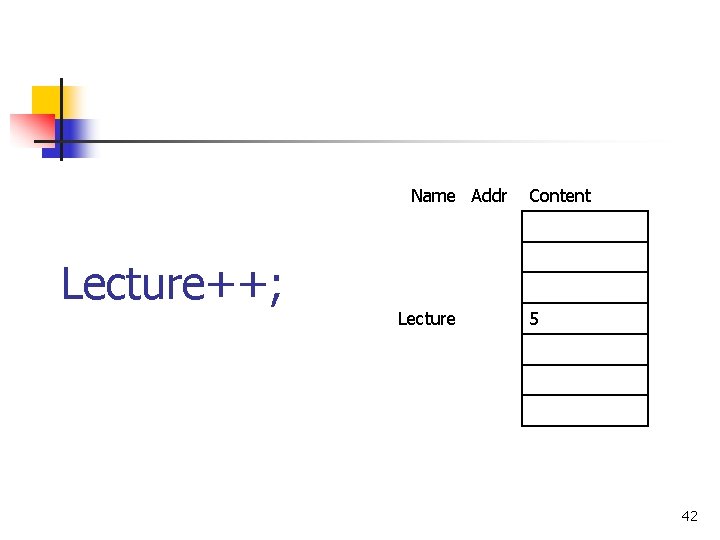
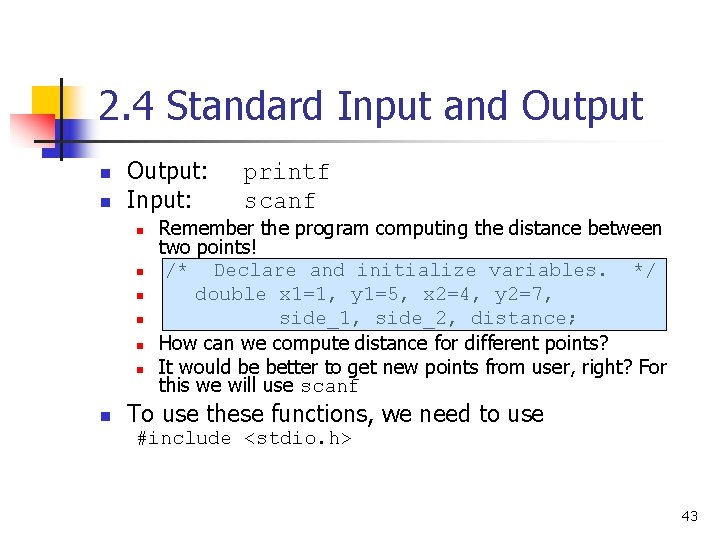
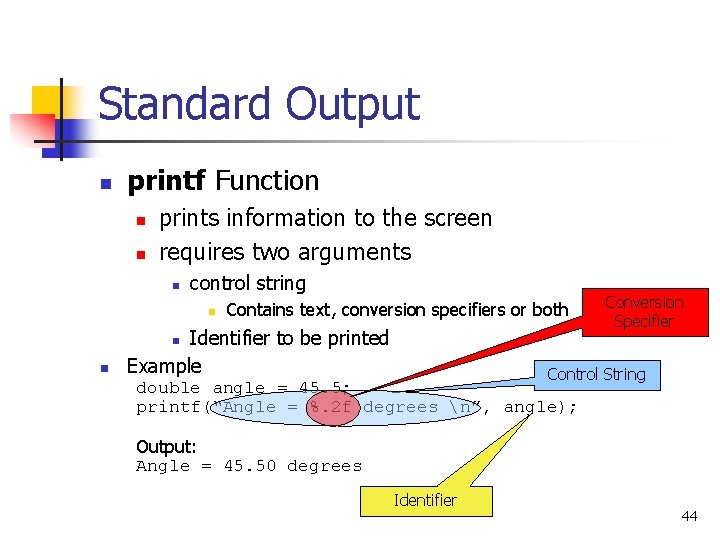
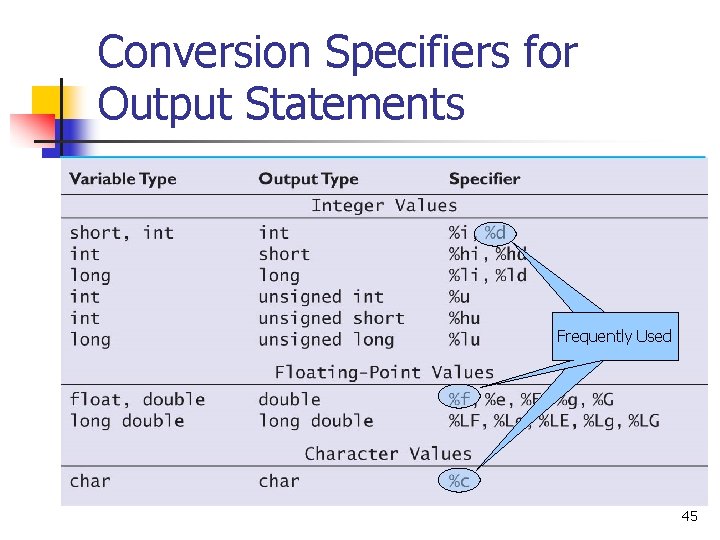
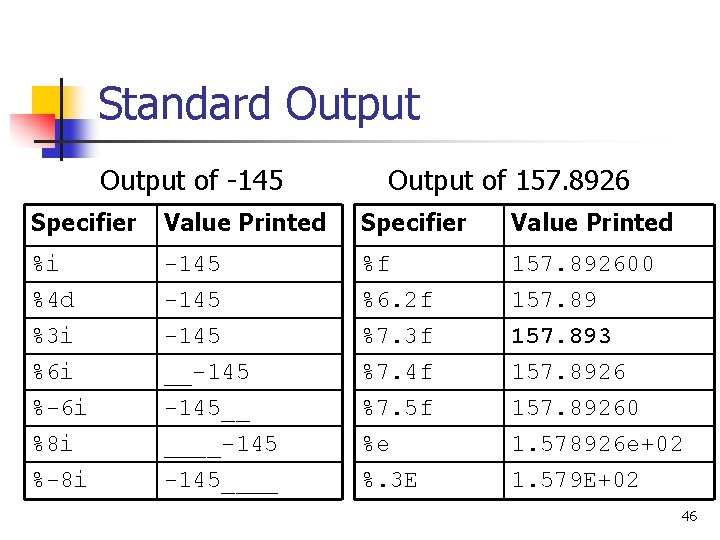
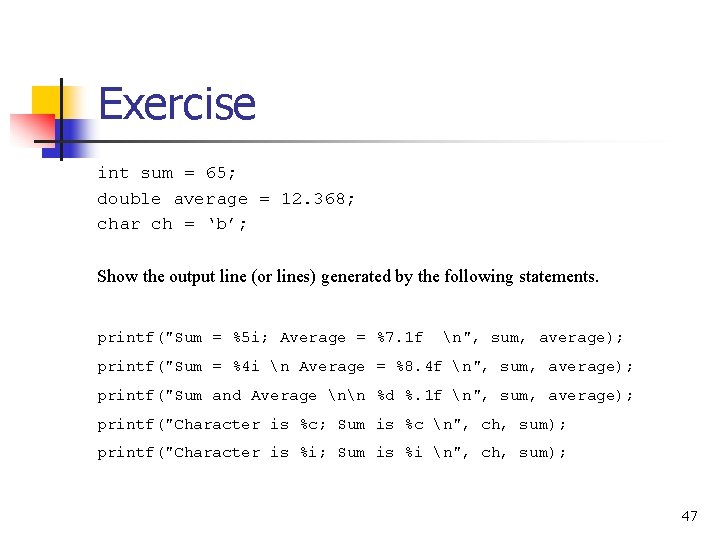
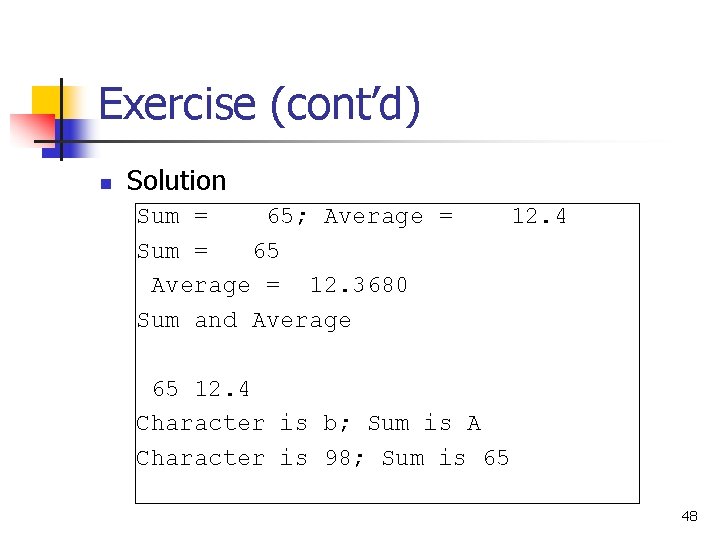
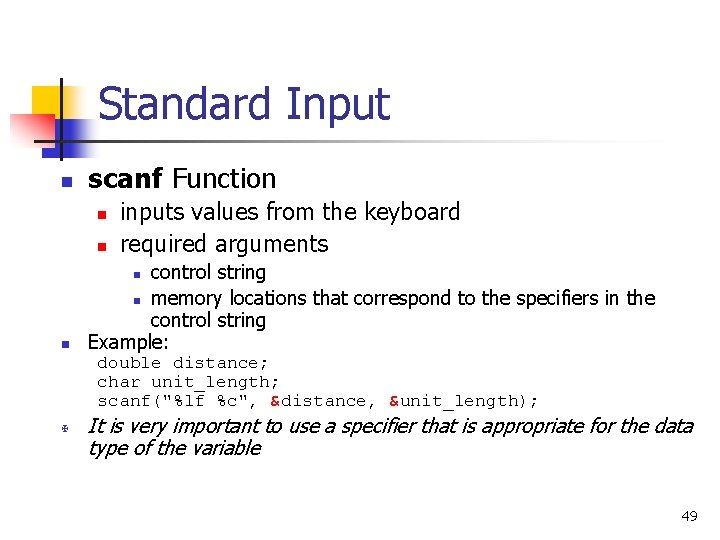
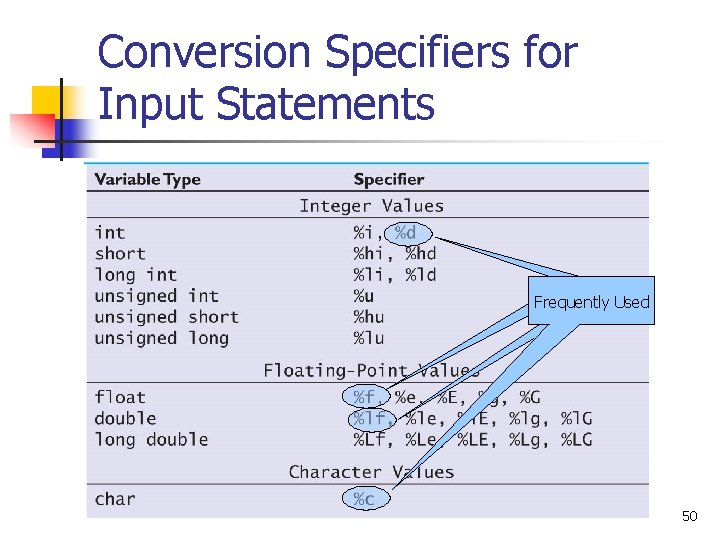
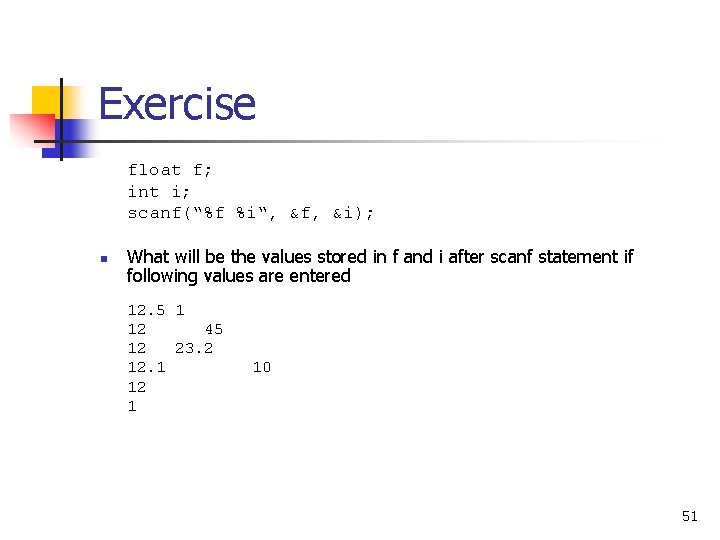
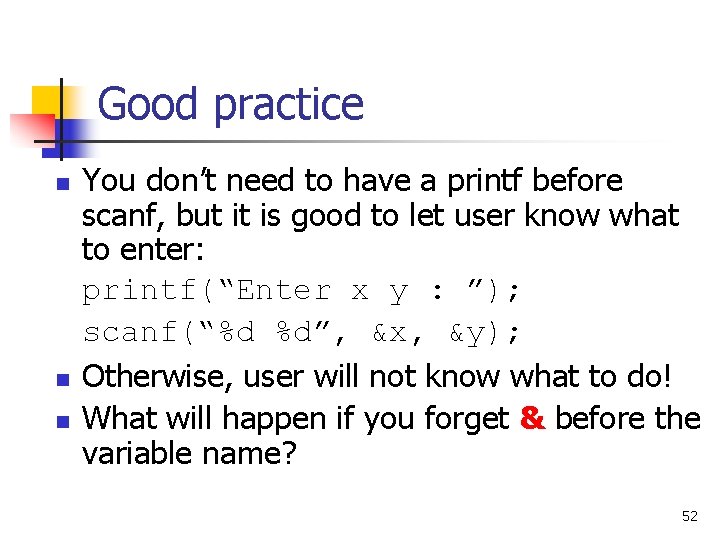
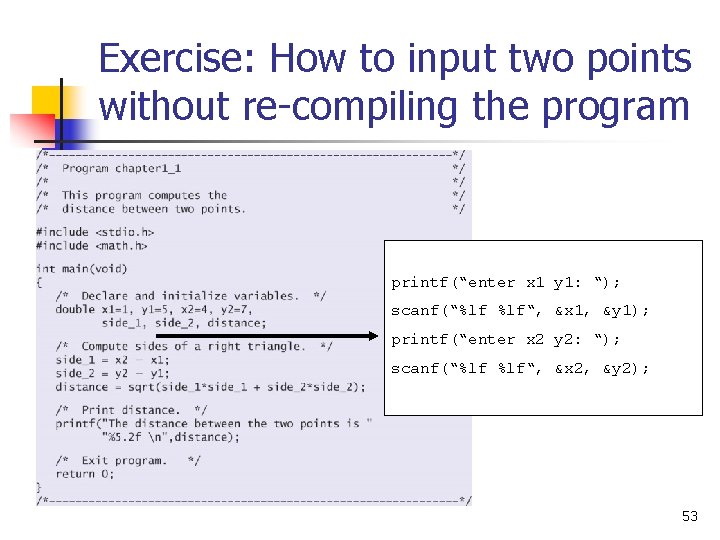
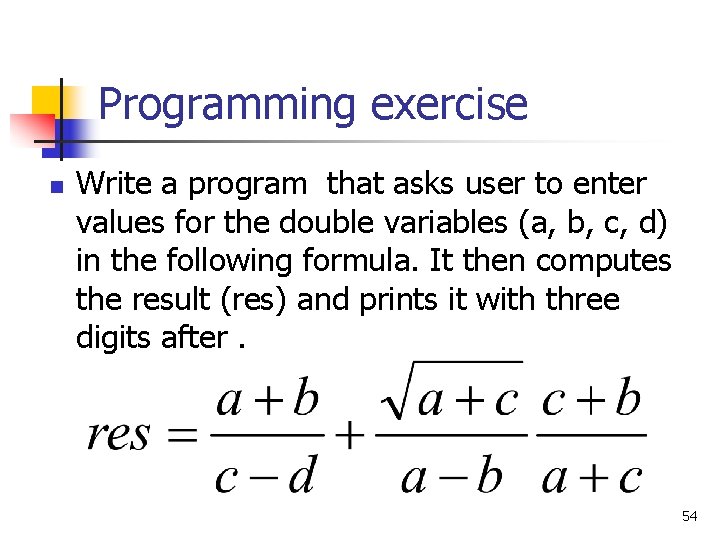
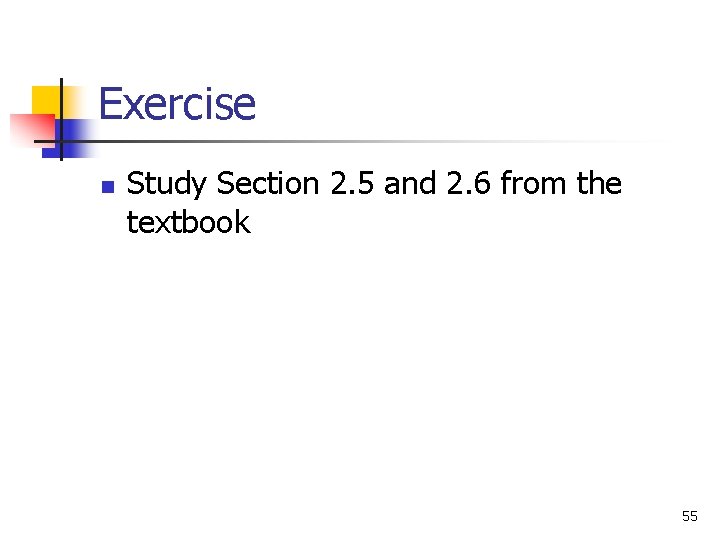
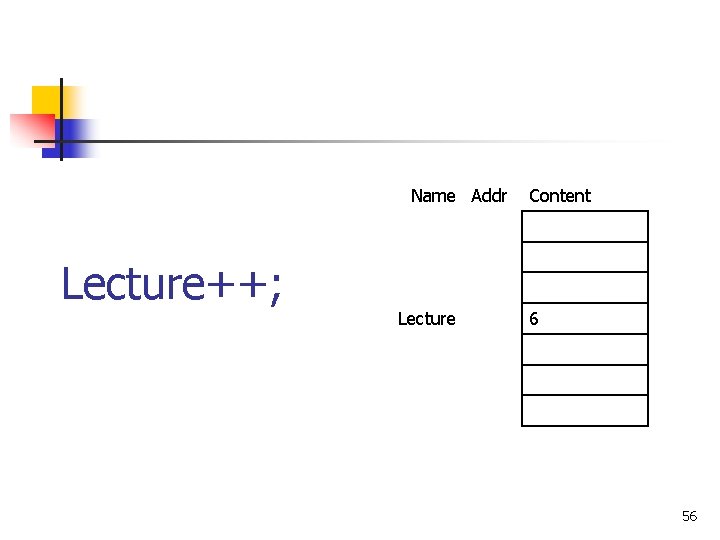
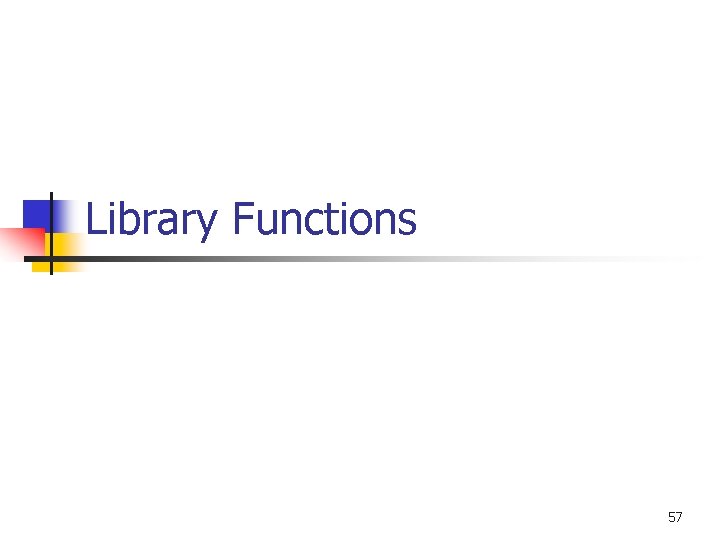
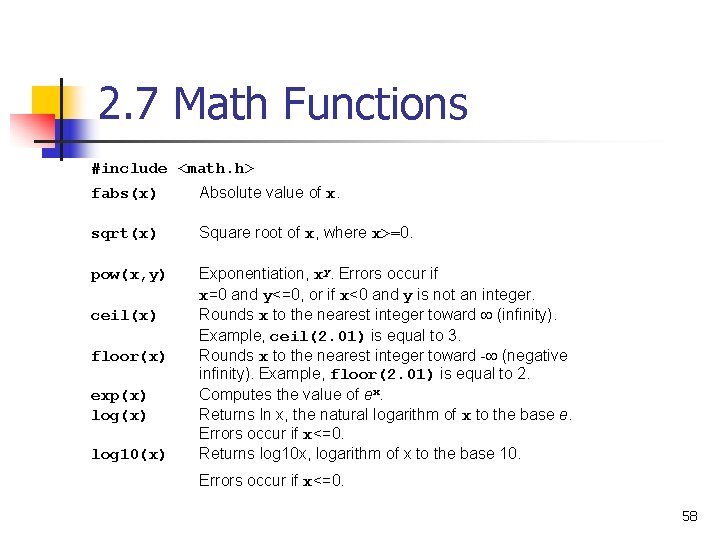
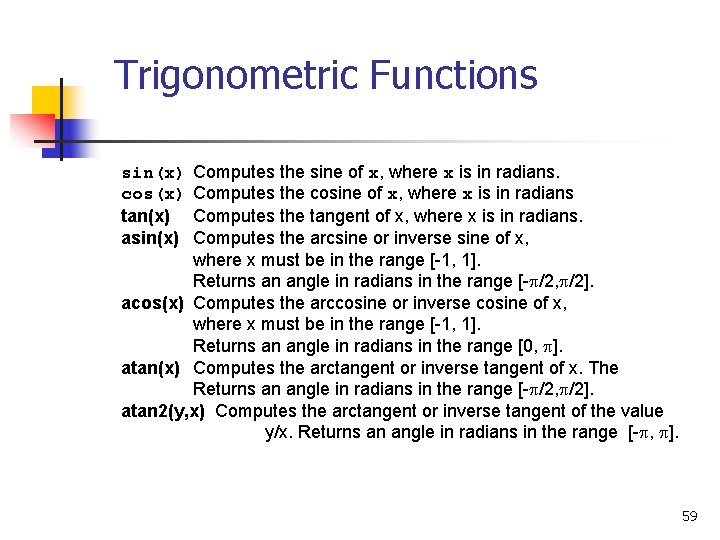
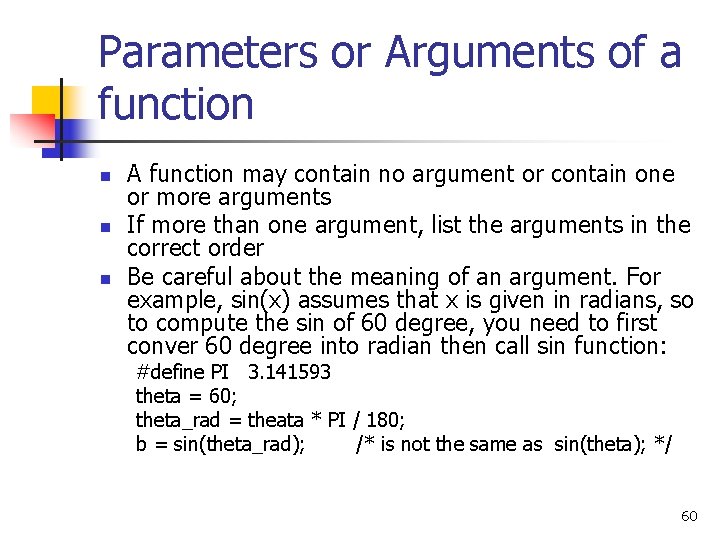
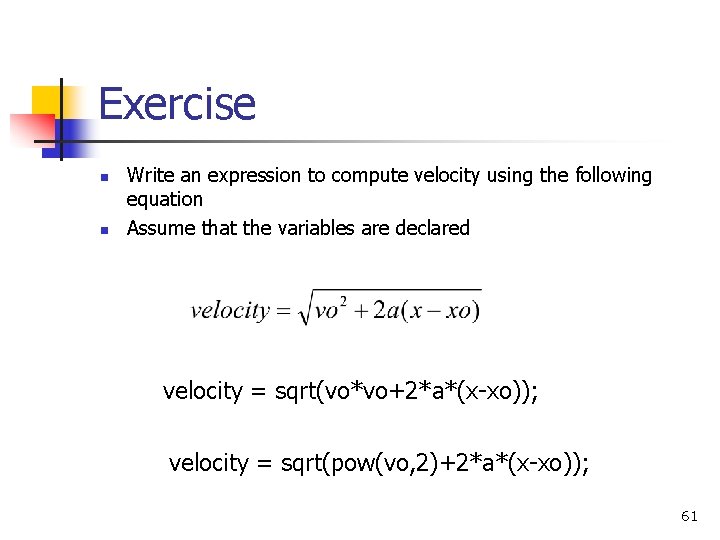
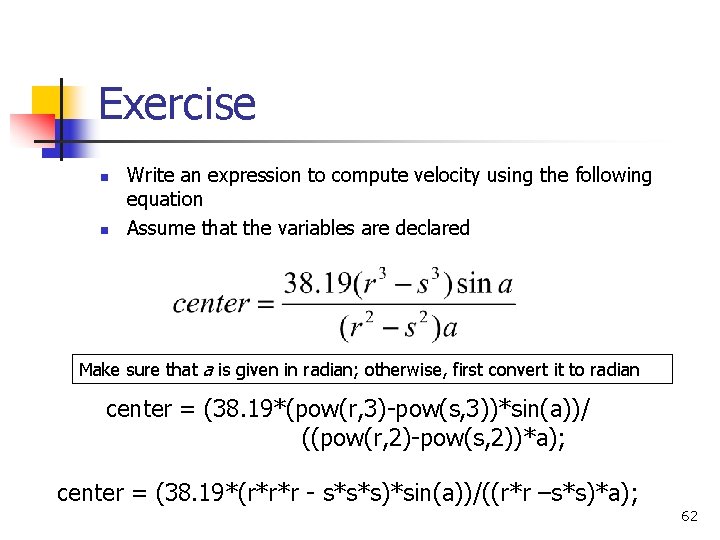
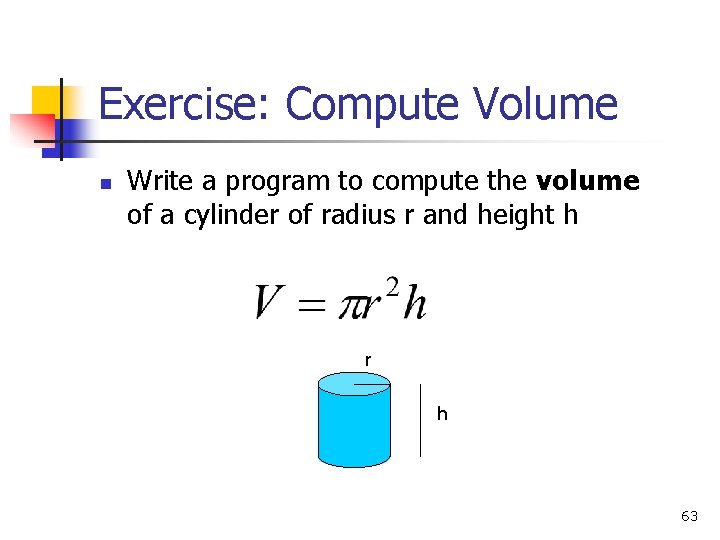
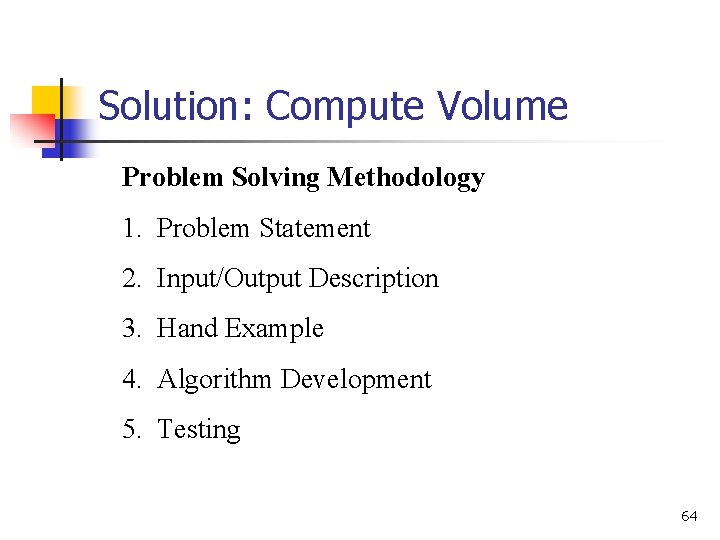
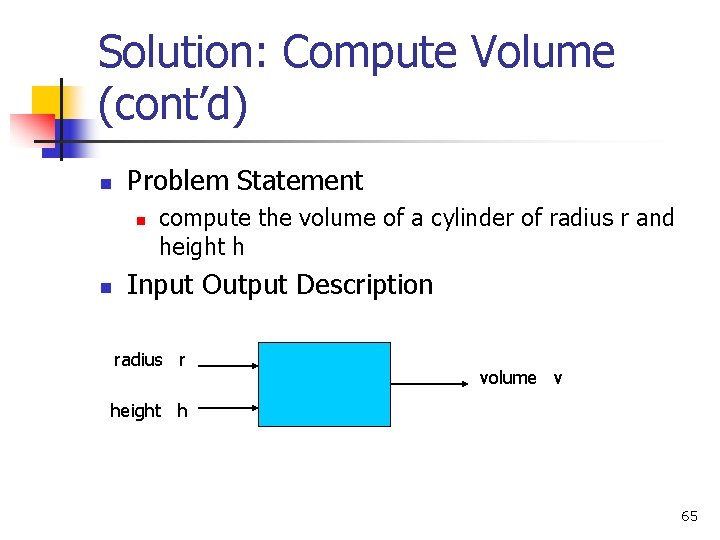
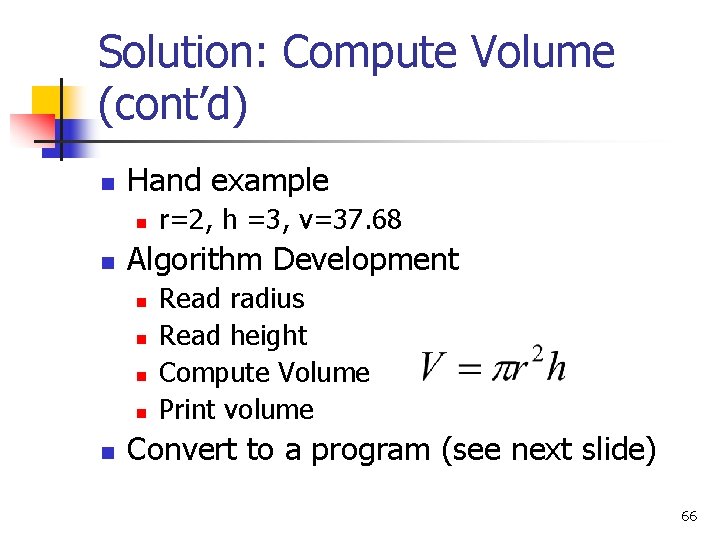
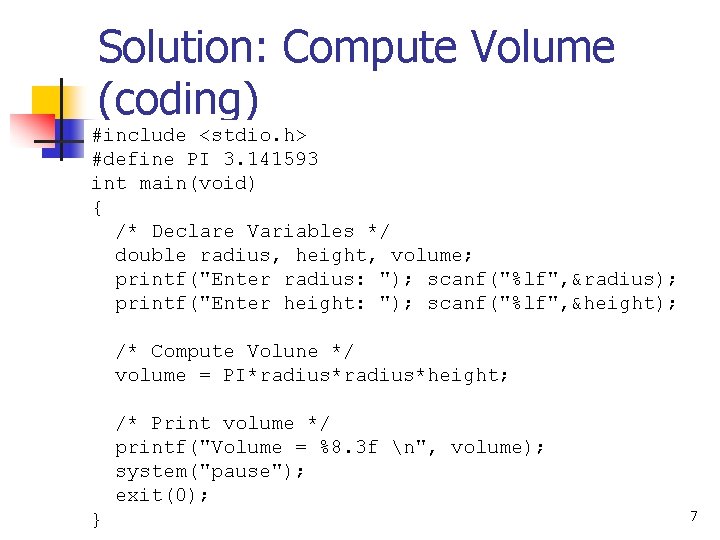
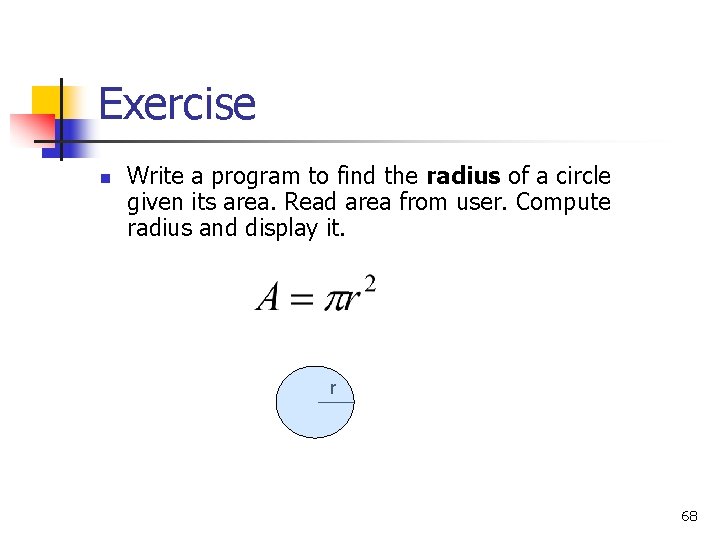
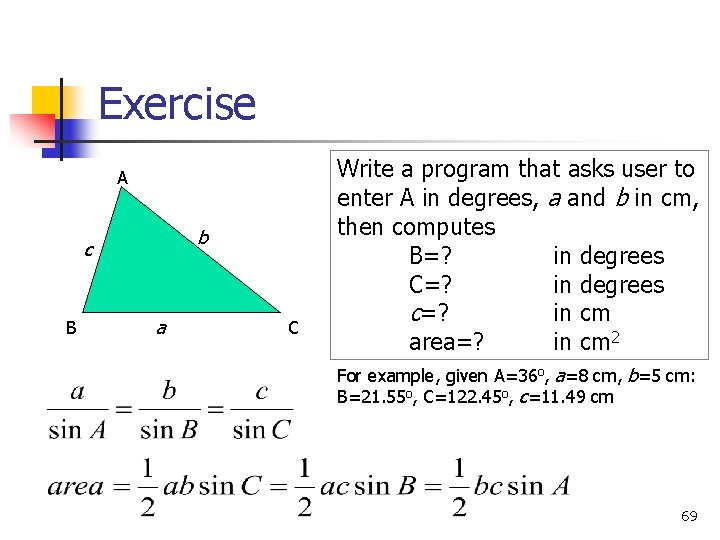
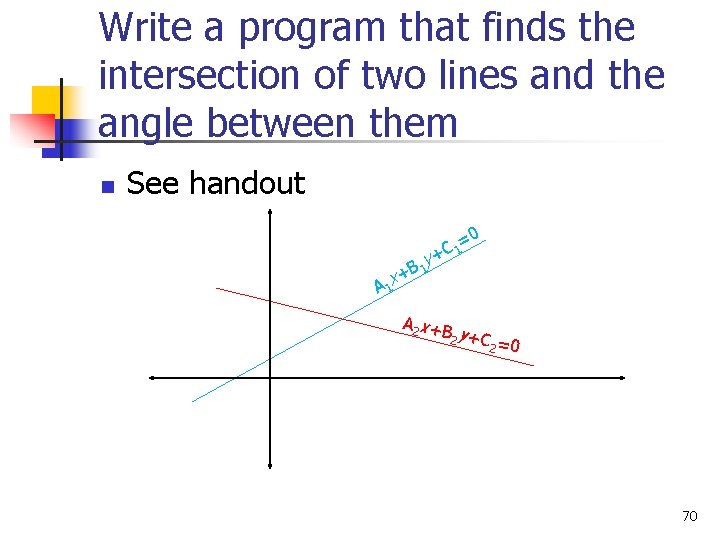
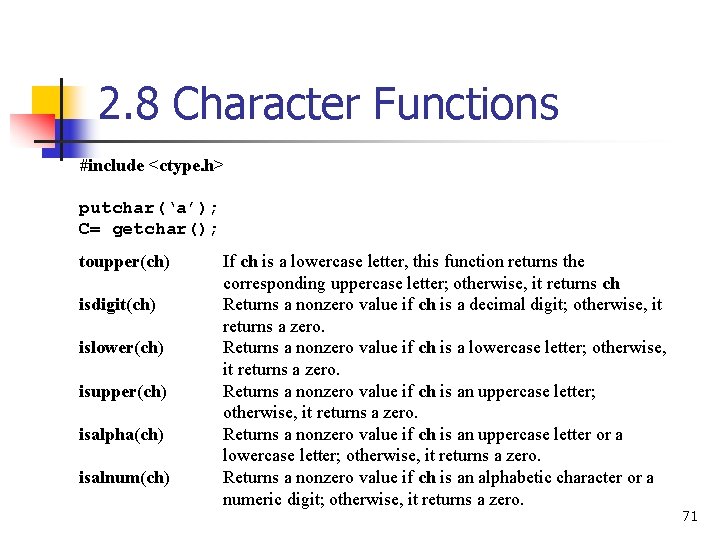
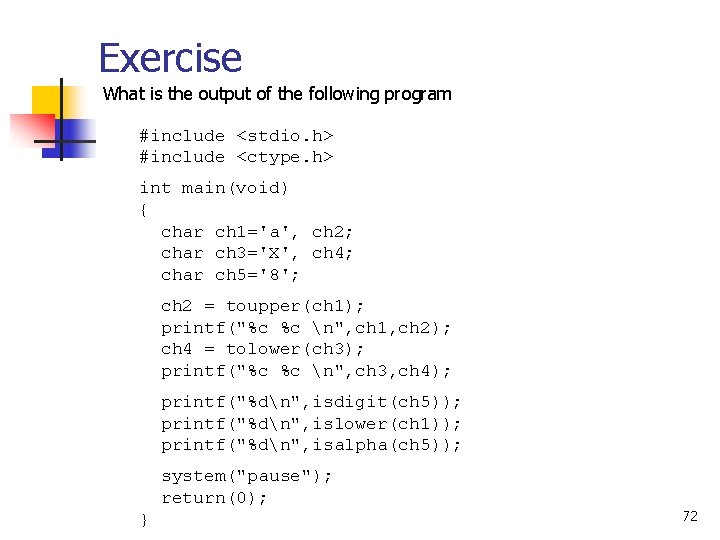
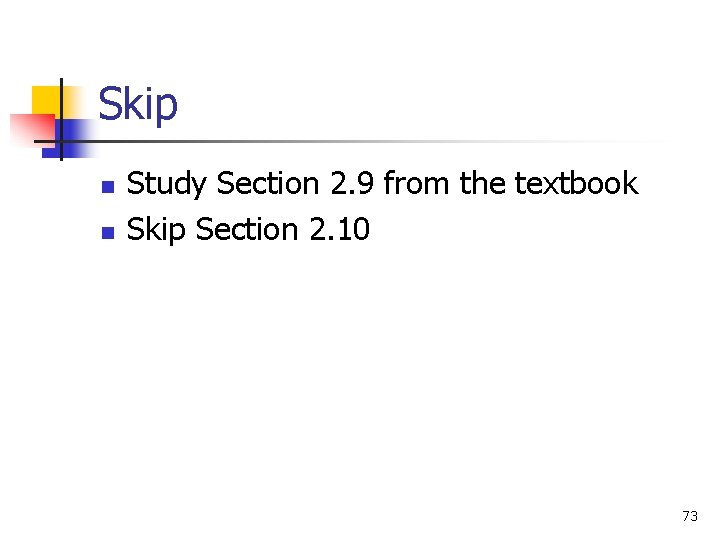
- Slides: 73
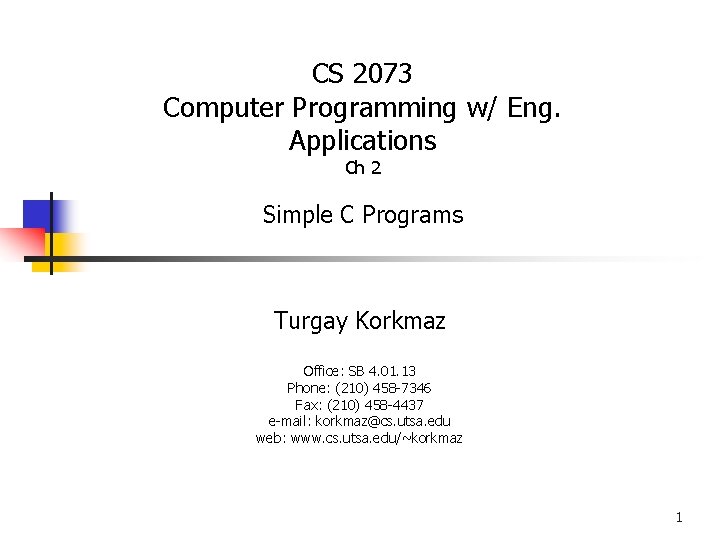
CS 2073 Computer Programming w/ Eng. Applications Ch 2 Simple C Programs Turgay Korkmaz Office: SB 4. 01. 13 Phone: (210) 458 -7346 Fax: (210) 458 -4437 e-mail: korkmaz@cs. utsa. edu web: www. cs. utsa. edu/~korkmaz 1
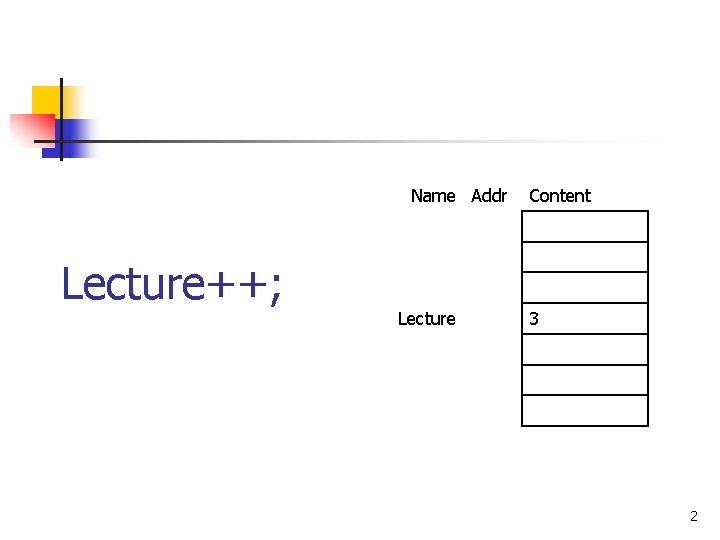
Name Addr Content Lecture++; Lecture 3 2
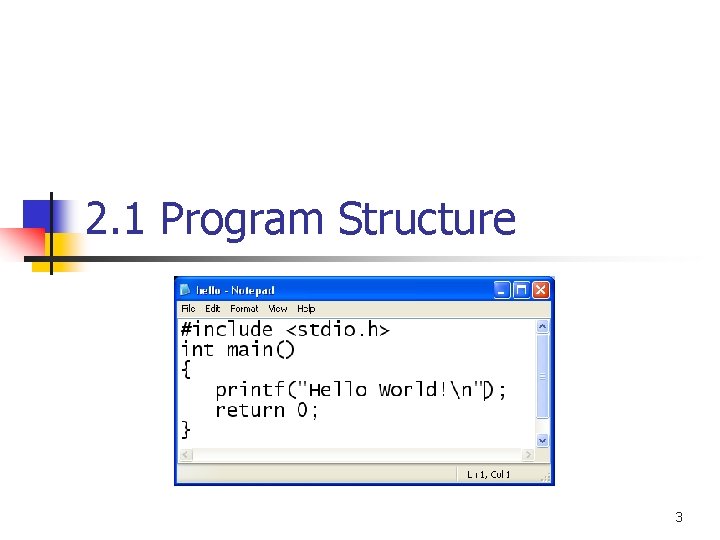
2. 1 Program Structure 3
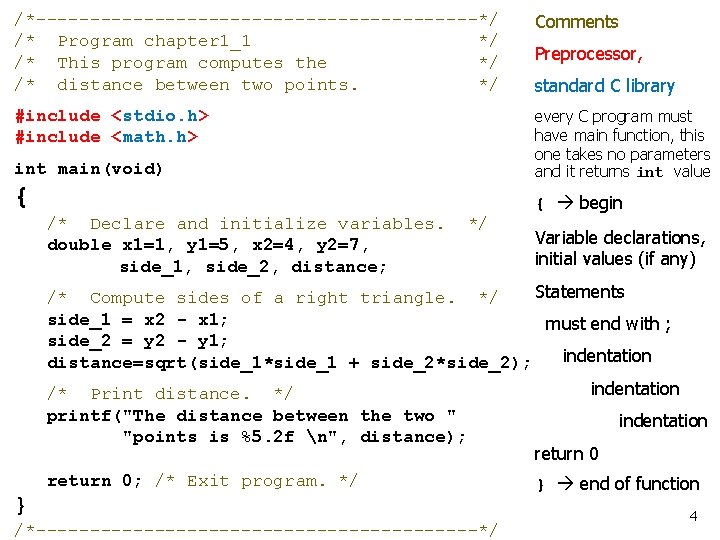
/*---------------------*/ /* Program chapter 1_1 */ /* This program computes the */ /* distance between two points. */ Comments #include <stdio. h> #include <math. h> int main(void) every C program must have main function, this one takes no parameters and it returns int value { { begin /* Declare and initialize variables. double x 1=1, y 1=5, x 2=4, y 2=7, side_1, side_2, distance; } */ Preprocessor, standard C library Variable declarations, initial values (if any) Statements /* Compute sides of a right triangle. */ side_1 = x 2 - x 1; must end with ; side_2 = y 2 - y 1; distance=sqrt(side_1*side_1 + side_2*side_2); indentation /* Print distance. */ printf("The distance between the two " indentation "points is %5. 2 f n", distance); return 0; /* Exit program. */ } end of function /*---------------------*/ 4
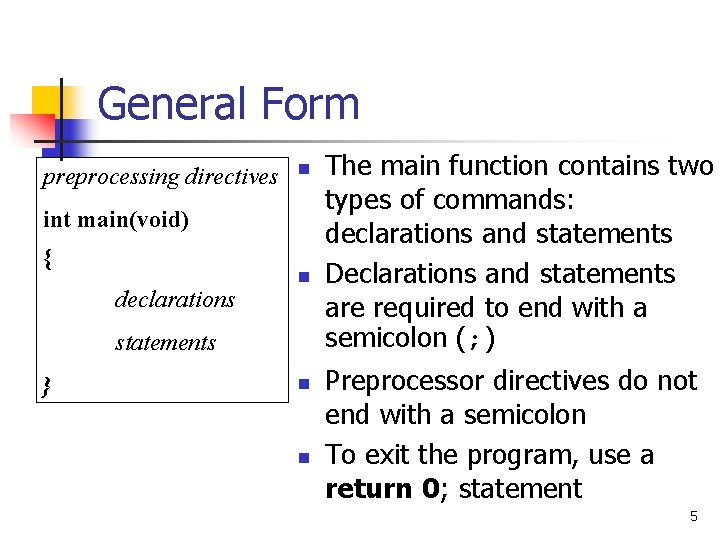
General Form preprocessing directives n int main(void) { declarations n statements } n n The main function contains two types of commands: declarations and statements Declarations and statements are required to end with a semicolon (; ) Preprocessor directives do not end with a semicolon To exit the program, use a return 0; statement 5
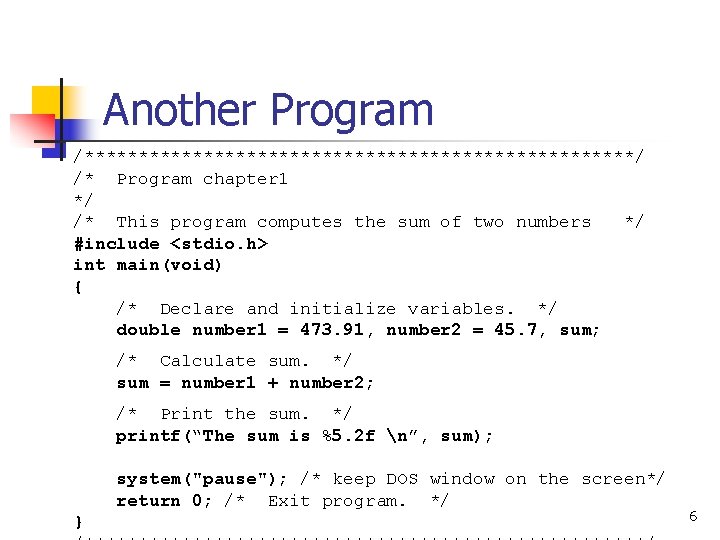
Another Program /**************************/ /* Program chapter 1 */ /* This program computes the sum of two numbers */ #include <stdio. h> int main(void) { /* Declare and initialize variables. */ double number 1 = 473. 91, number 2 = 45. 7, sum; /* Calculate sum. */ sum = number 1 + number 2; /* Print the sum. */ printf(“The sum is %5. 2 f n”, sum); system("pause"); /* keep DOS window on the screen*/ return 0; /* Exit program. */ } 6
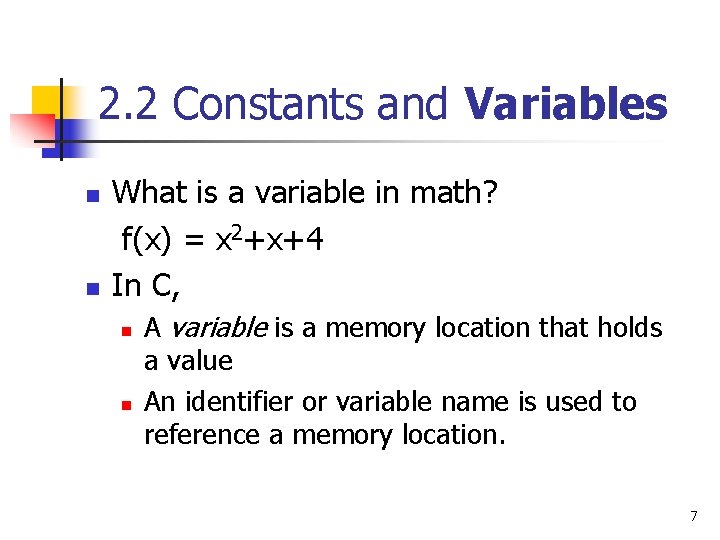
2. 2 Constants and Variables n n What is a variable in math? f(x) = x 2+x+4 In C, n n A variable is a memory location that holds a value An identifier or variable name is used to reference a memory location. 7
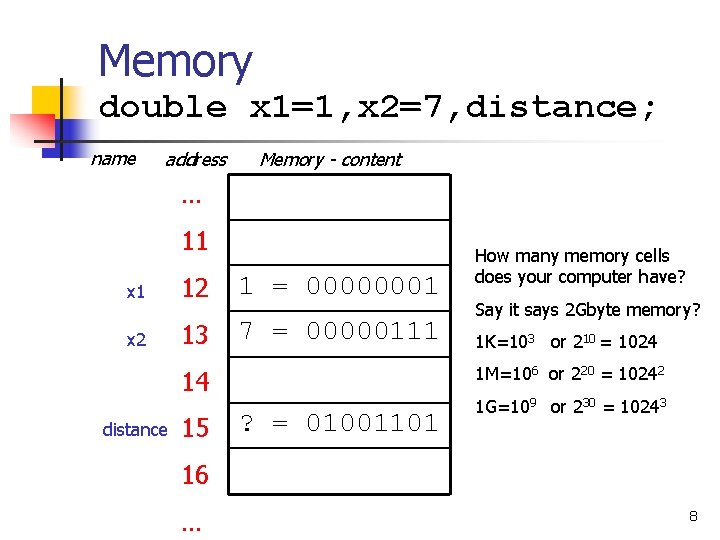
Memory double x 1=1, x 2=7, distance; name address Memory - content … 11 x 1 12 1 = 00000001 x 2 13 7 = 00000111 15 Say it says 2 Gbyte memory? 1 K=103 or 210 = 1024 1 M=106 or 220 = 10242 14 distance How many memory cells does your computer have? ? = 01001101 1 G=109 or 230 = 10243 16 … 8
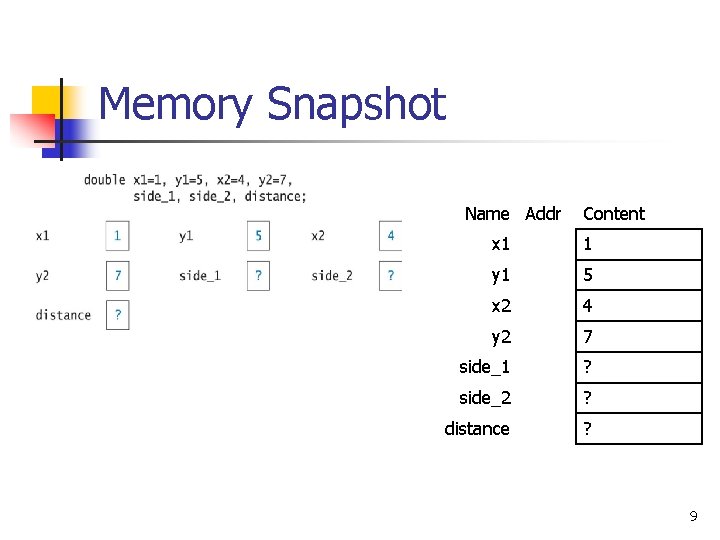
Memory Snapshot Name Addr Content x 1 1 y 1 5 x 2 4 y 2 7 side_1 ? side_2 ? distance ? 9
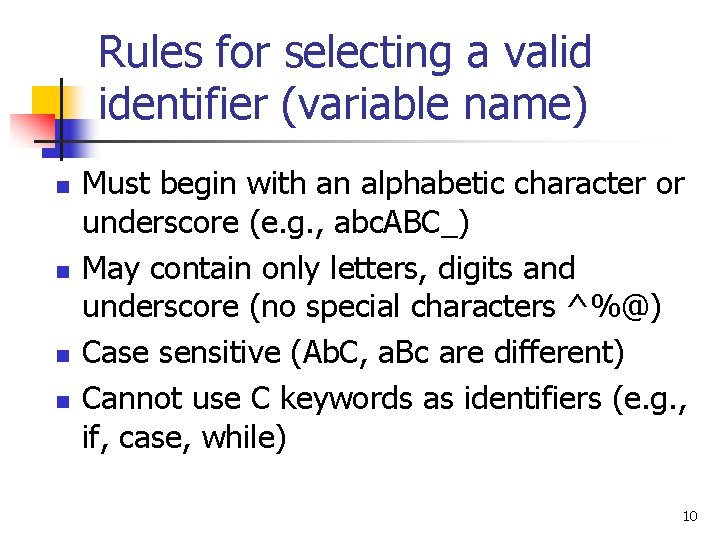
Rules for selecting a valid identifier (variable name) n n Must begin with an alphabetic character or underscore (e. g. , abc. ABC_) May contain only letters, digits and underscore (no special characters ^%@) Case sensitive (Ab. C, a. Bc are different) Cannot use C keywords as identifiers (e. g. , if, case, while) 10
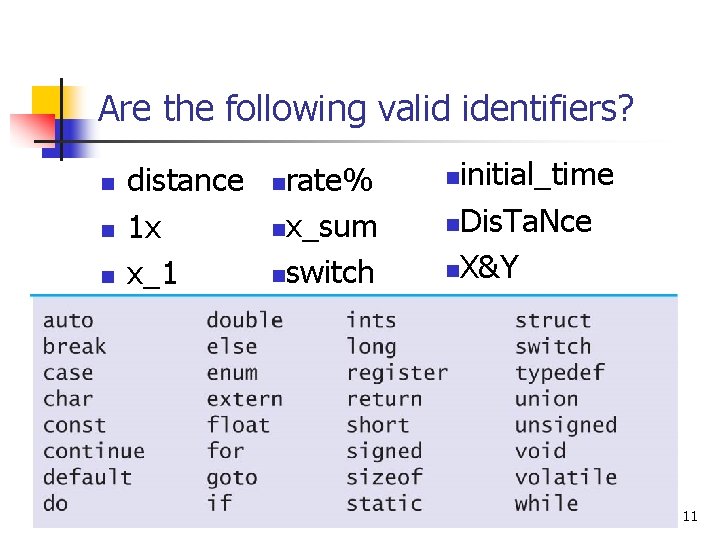
Are the following valid identifiers? n n n distance 1 x x_1 rate% nx_sum nswitch n initial_time n. Dis. Ta. Nce n. X&Y n 11
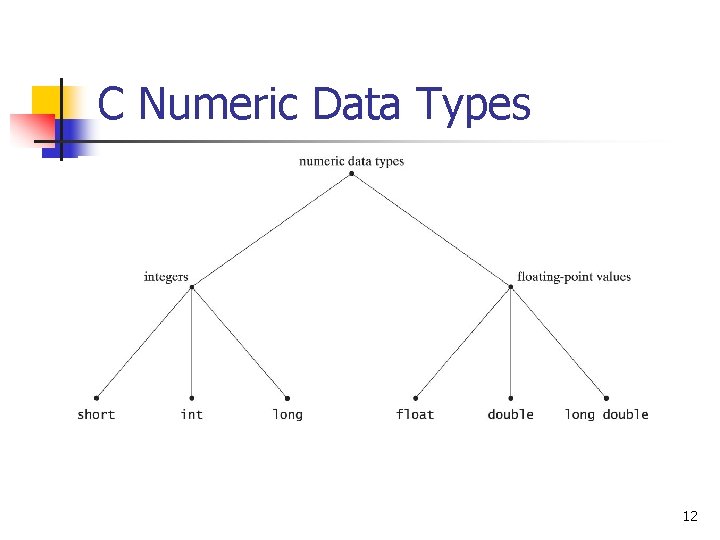
C Numeric Data Types 12
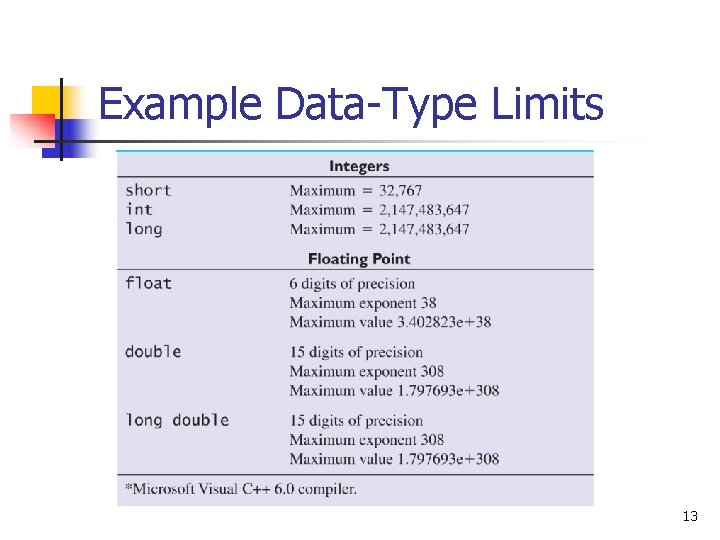
Example Data-Type Limits 13
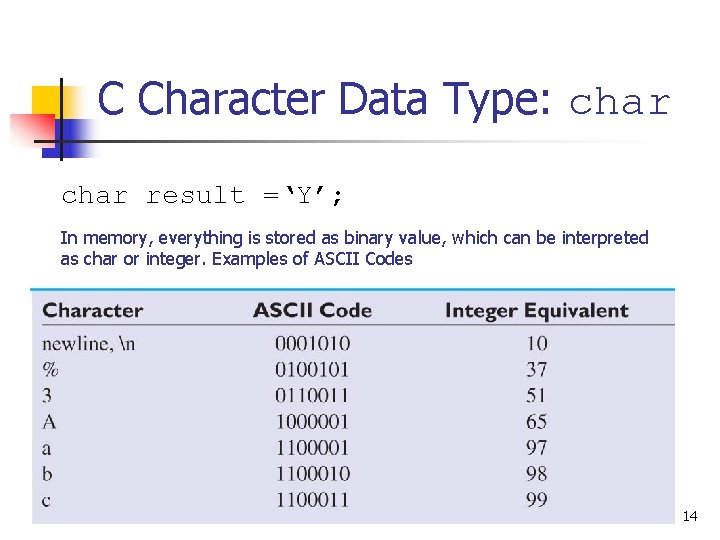
C Character Data Type: char result =‘Y’; In memory, everything is stored as binary value, which can be interpreted as char or integer. Examples of ASCII Codes 14
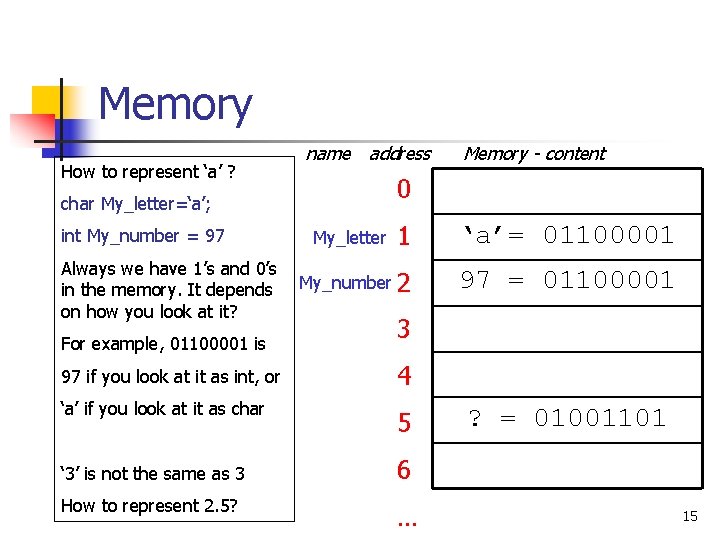
Memory How to represent ‘a’ ? name address Memory - content 0 char My_letter=‘a’; 1 ‘a’= 01100001 Always we have 1’s and 0’s in the memory. It depends My_number 2 on how you look at it? 97 = 01100001 int My_number = 97 For example, 01100001 is My_letter 3 97 if you look at it as int, or 4 ‘a’ if you look at it as char 5 ‘ 3’ is not the same as 3 How to represent 2. 5? ? = 01001101 6 … 15
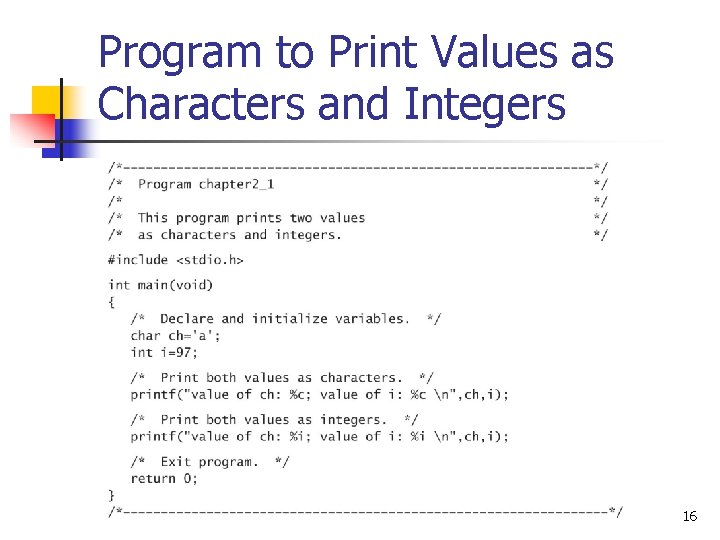
Program to Print Values as Characters and Integers 16
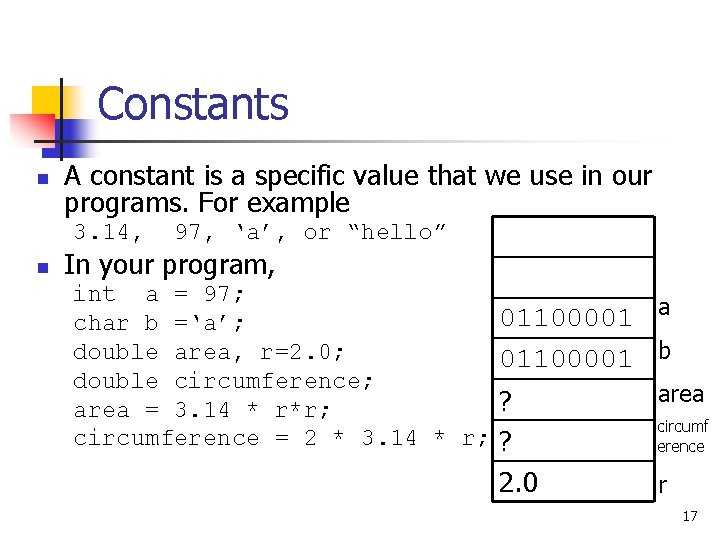
Constants n A constant is a specific value that we use in our programs. For example 3. 14, n 97, ‘a’, or “hello” In your program, int a = 97; 01100001 char b =‘a’; double area, r=2. 0; 01100001 double circumference; ? area = 3. 14 * r*r; circumference = 2 * 3. 14 * r; ? 2. 0 a b area circumf erence r 17
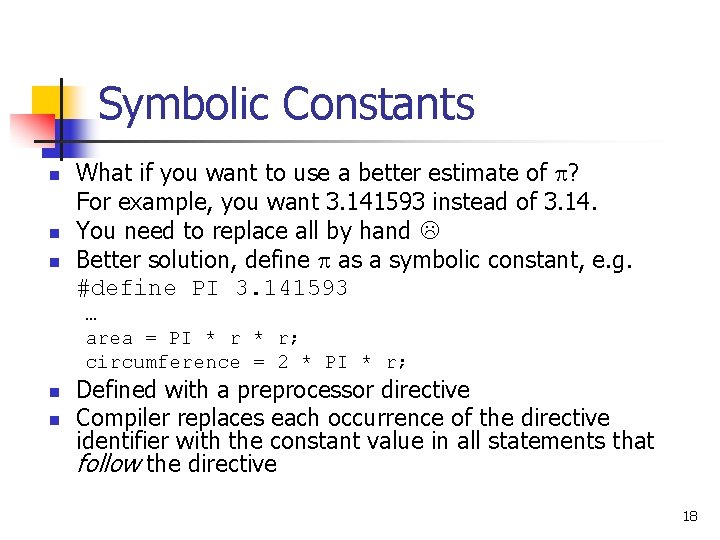
Symbolic Constants n n n What if you want to use a better estimate of ? For example, you want 3. 141593 instead of 3. 14. You need to replace all by hand Better solution, define as a symbolic constant, e. g. #define PI 3. 141593 … area = PI * r; circumference = 2 * PI * r; n n Defined with a preprocessor directive Compiler replaces each occurrence of the directive identifier with the constant value in all statements that follow the directive 18
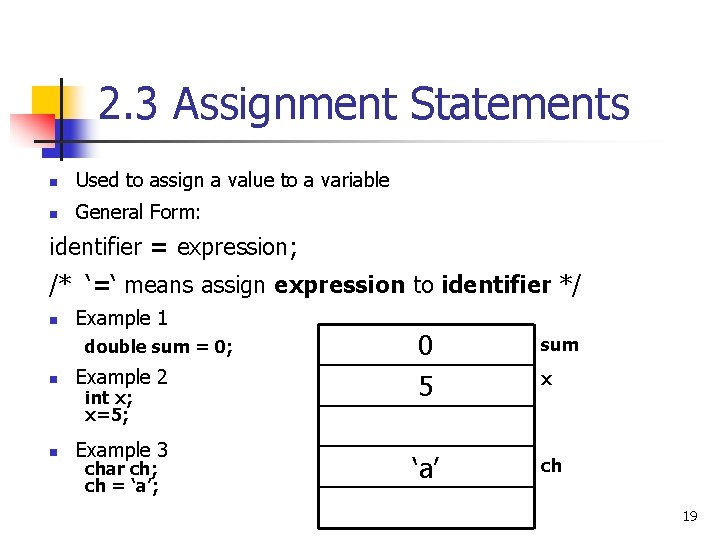
2. 3 Assignment Statements n Used to assign a value to a variable n General Form: identifier = expression; /* ‘=‘ means assign expression to identifier */ n Example 1 double sum = 0; n Example 2 n Example 3 int x; x=5; char ch; ch = ‘a’; 0 5 ‘a’ sum x ch 19
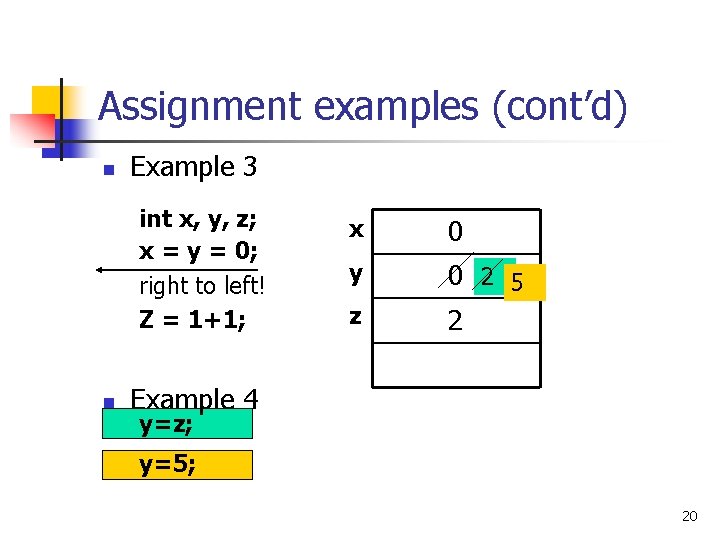
Assignment examples (cont’d) n Example 3 int x, y, z; x = y = 0; right to left! Z = 1+1; n x 0 y 0 2 5 2 z Example 4 y=z; y=5; 20
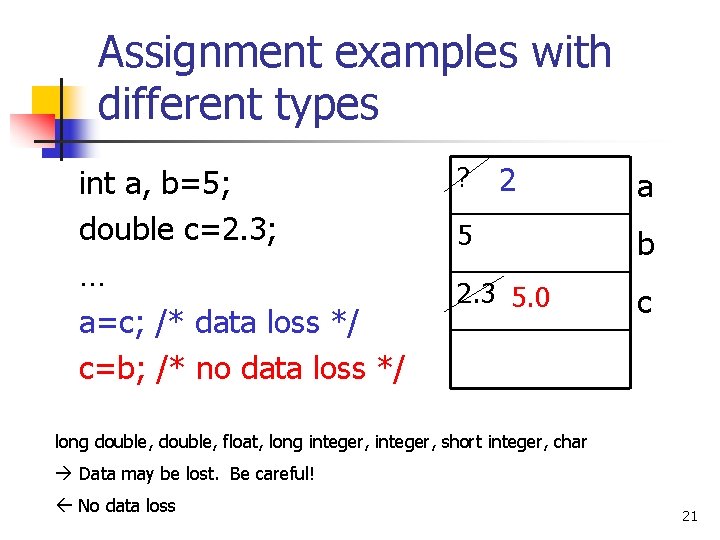
Assignment examples with different types int a, b=5; double c=2. 3; … a=c; /* data loss */ c=b; /* no data loss */ ? 2 a 5 b 2. 3 5. 0 c long double, float, long integer, short integer, char Data may be lost. Be careful! No data loss 21
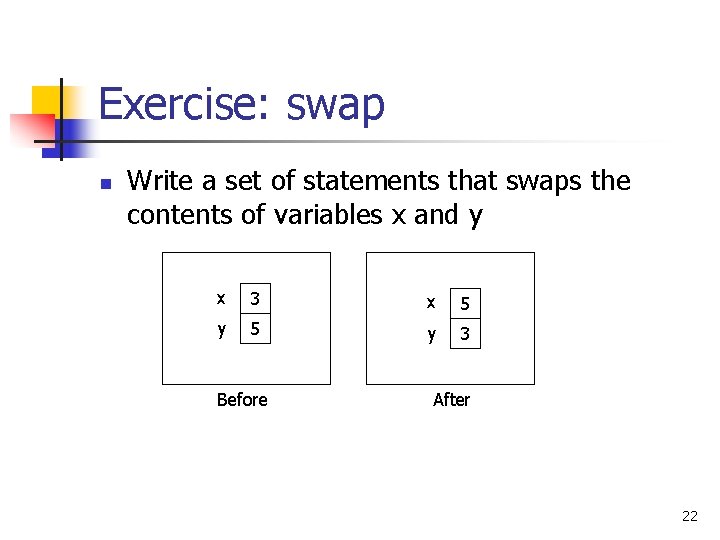
Exercise: swap n Write a set of statements that swaps the contents of variables x and y x 3 x 5 y 3 Before After 22
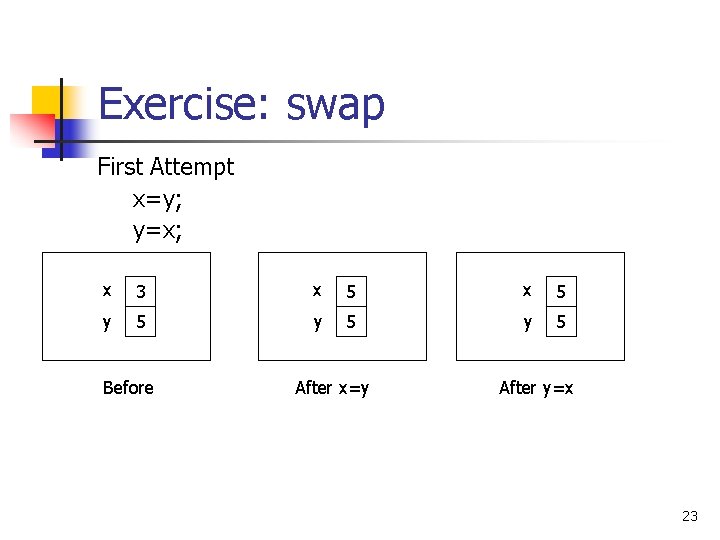
Exercise: swap First Attempt x=y; y=x; x 3 x 5 y 5 y 5 Before After x=y After y=x 23
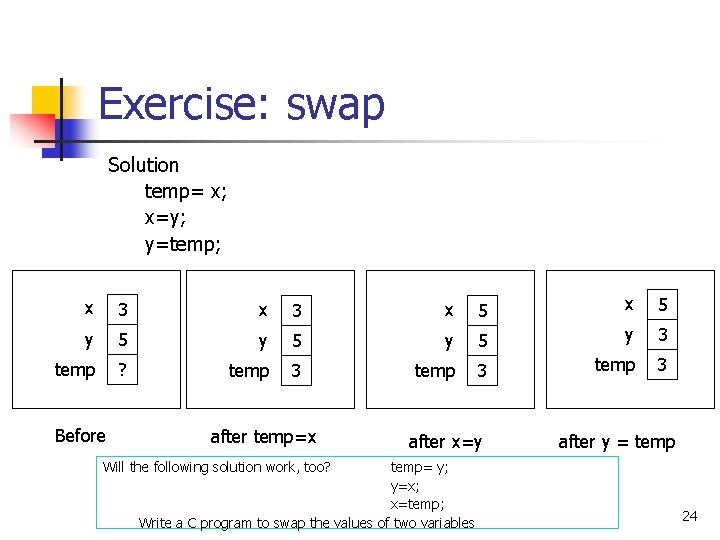
Exercise: swap Solution temp= x; x=y; y=temp; x 3 x 5 y 5 y 5 y 3 temp ? temp 3 Before after temp=x after x=y temp= y; y=x; x=temp; Write a C program to swap the values of two variables after y = temp Will the following solution work, too? 24
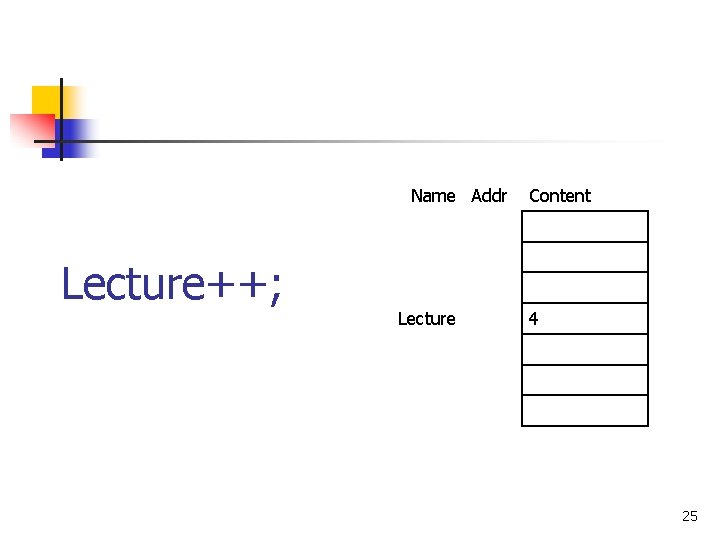
Name Addr Content Lecture++; Lecture 4 25
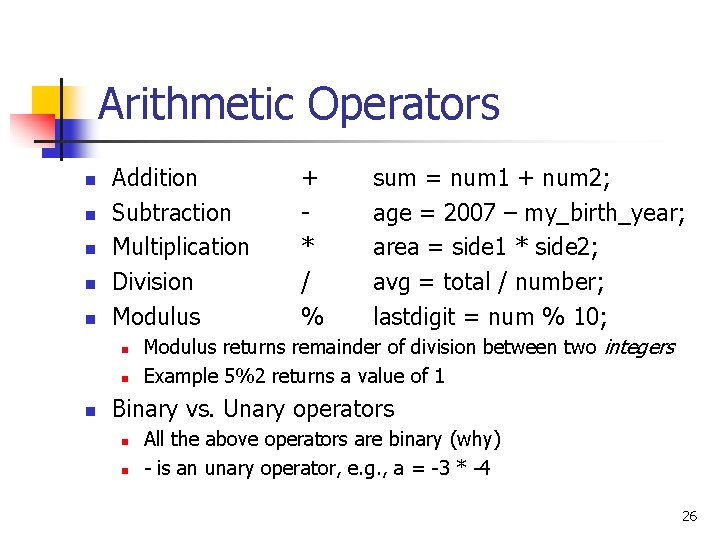
Arithmetic Operators n n n Addition Subtraction Multiplication Division Modulus n n n + * / % sum = num 1 + num 2; age = 2007 – my_birth_year; area = side 1 * side 2; avg = total / number; lastdigit = num % 10; Modulus returns remainder of division between two integers Example 5%2 returns a value of 1 Binary vs. Unary operators n n All the above operators are binary (why) - is an unary operator, e. g. , a = -3 * -4 26
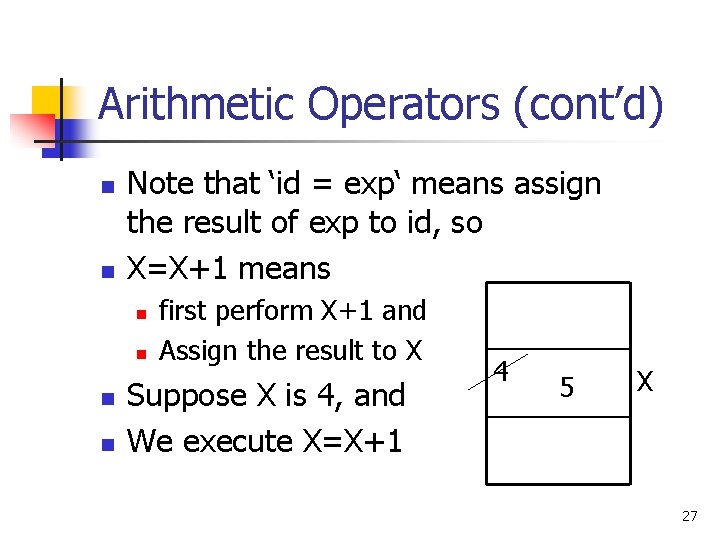
Arithmetic Operators (cont’d) n n Note that ‘id = exp‘ means assign the result of exp to id, so X=X+1 means n n first perform X+1 and Assign the result to X Suppose X is 4, and We execute X=X+1 4 5 X 27
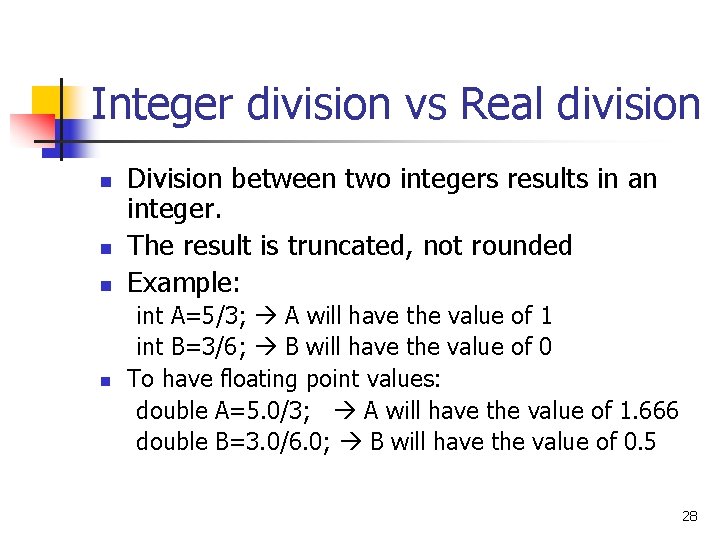
Integer division vs Real division n n Division between two integers results in an integer. The result is truncated, not rounded Example: int A=5/3; A will have the value of 1 int B=3/6; B will have the value of 0 To have floating point values: double A=5. 0/3; A will have the value of 1. 666 double B=3. 0/6. 0; B will have the value of 0. 5 28
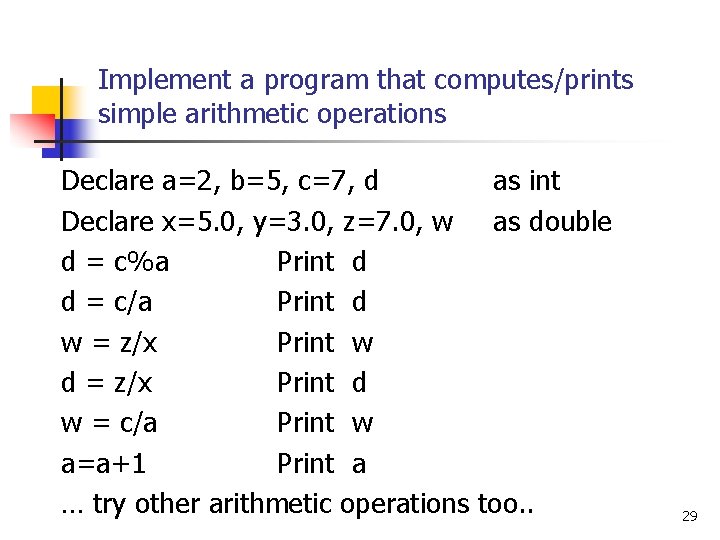
Implement a program that computes/prints simple arithmetic operations Declare a=2, b=5, c=7, d as int Declare x=5. 0, y=3. 0, z=7. 0, w as double d = c%a Print d d = c/a Print d w = z/x Print w d = z/x Print d w = c/a Print w a=a+1 Print a … try other arithmetic operations too. . 29
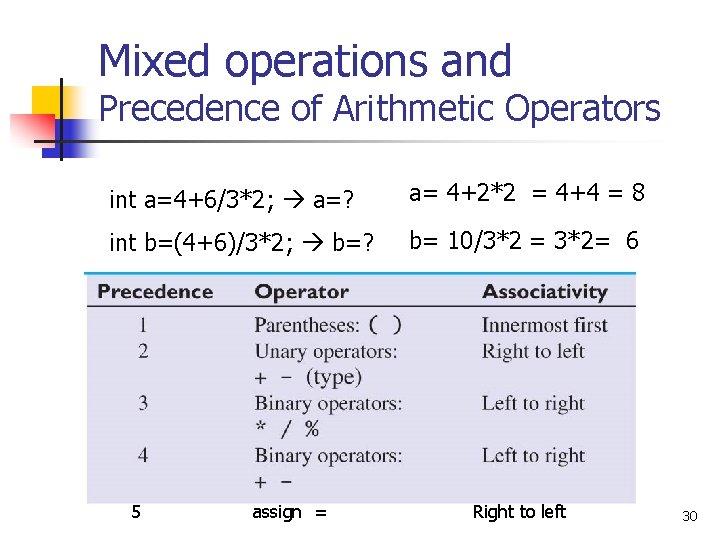
Mixed operations and Precedence of Arithmetic Operators int a=4+6/3*2; a=? a= 4+2*2 = 4+4 = 8 int b=(4+6)/3*2; b=? b= 10/3*2 = 3*2= 6 5 assign = Right to left 30
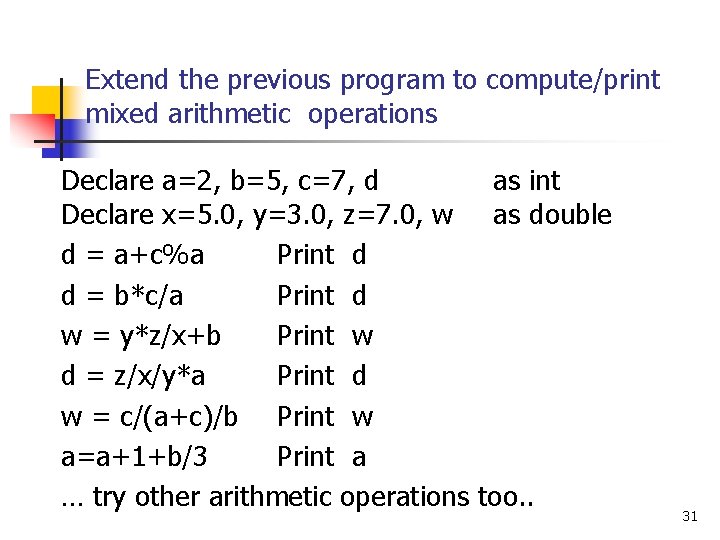
Extend the previous program to compute/print mixed arithmetic operations Declare a=2, b=5, c=7, d as int Declare x=5. 0, y=3. 0, z=7. 0, w as double d = a+c%a Print d d = b*c/a Print d w = y*z/x+b Print w d = z/x/y*a Print d w = c/(a+c)/b Print w a=a+1+b/3 Print a … try other arithmetic operations too. . 31
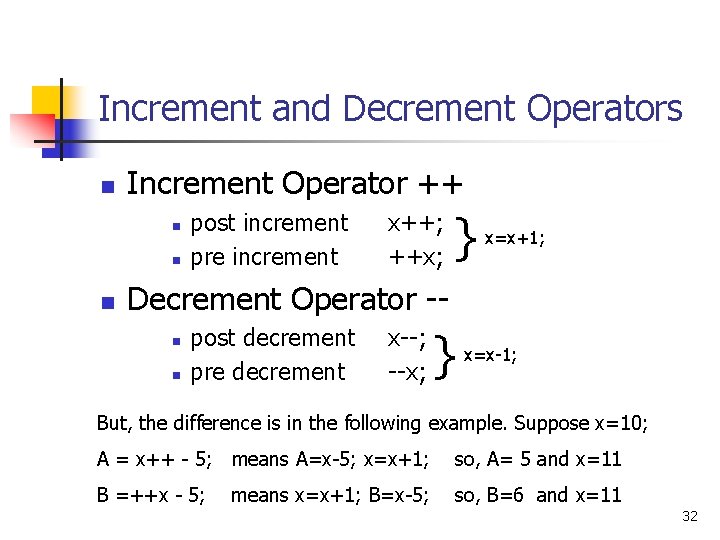
Increment and Decrement Operators n Increment Operator ++ n n n post increment pre increment x++; ++x; } x=x+1; Decrement Operator -n n post decrement pre decrement x--; --x; } x=x-1; But, the difference is in the following example. Suppose x=10; A = x++ - 5; means A=x-5; x=x+1; so, A= 5 and x=11 B =++x - 5; means x=x+1; B=x-5; so, B=6 and x=11 32
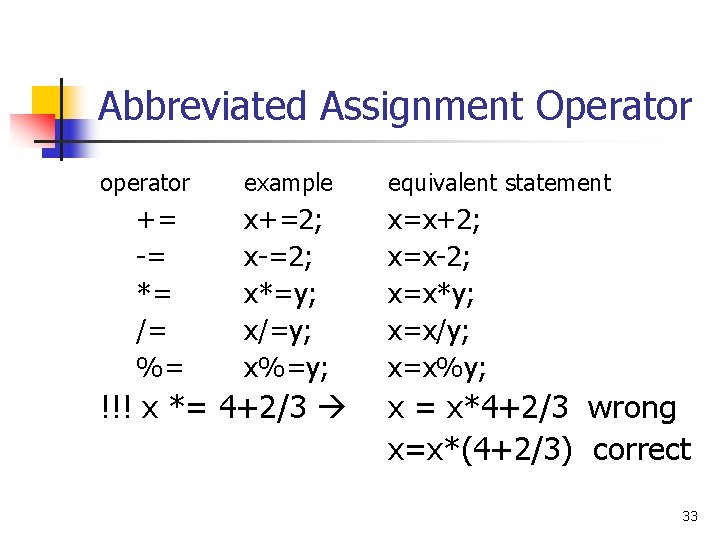
Abbreviated Assignment Operator operator example equivalent statement += -= *= /= %= x+=2; x-=2; x*=y; x/=y; x%=y; x=x+2; x=x-2; x=x*y; x=x/y; x=x%y; !!! x *= 4+2/3 x = x*4+2/3 wrong x=x*(4+2/3) correct 33
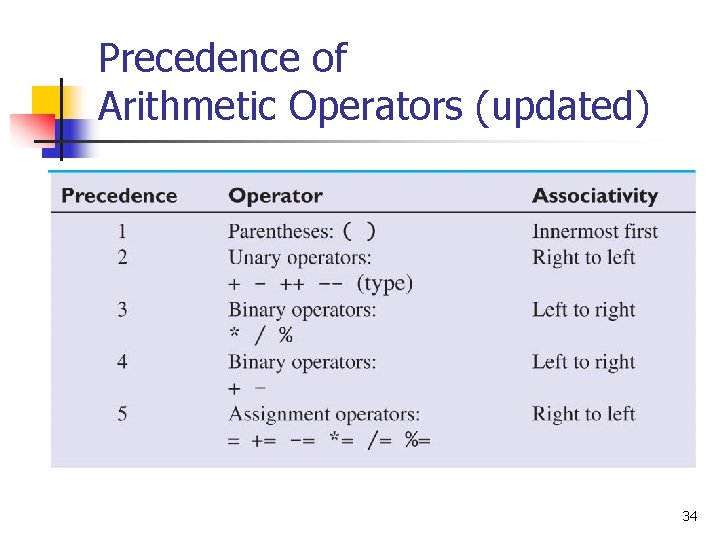
Precedence of Arithmetic Operators (updated) 34
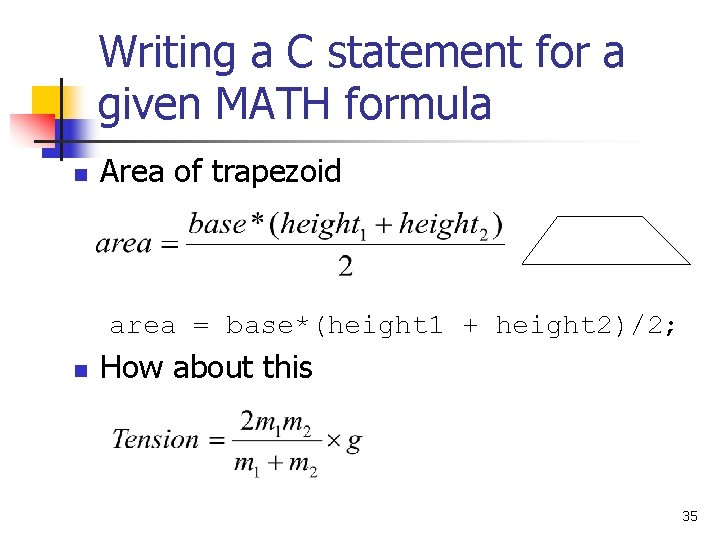
Writing a C statement for a given MATH formula n Area of trapezoid area = base*(height 1 + height 2)/2; n How about this 35
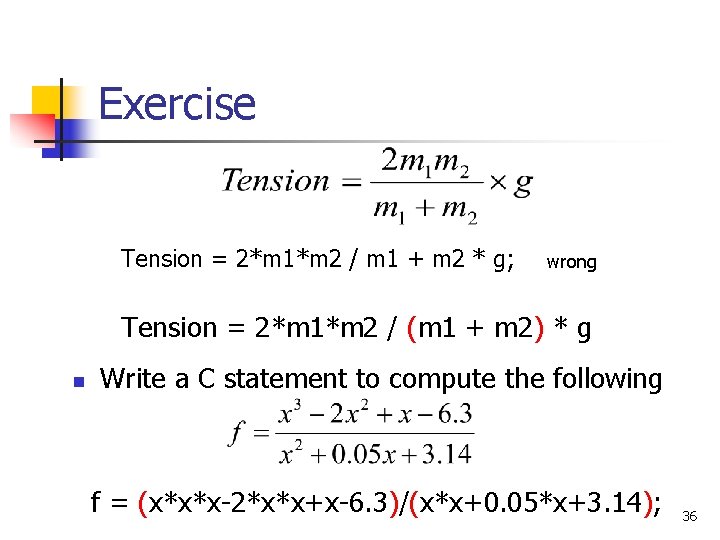
Exercise Tension = 2*m 1*m 2 / m 1 + m 2 * g; wrong Tension = 2*m 1*m 2 / (m 1 + m 2) * g n Write a C statement to compute the following f = (x*x*x-2*x*x+x-6. 3)/(x*x+0. 05*x+3. 14); 36
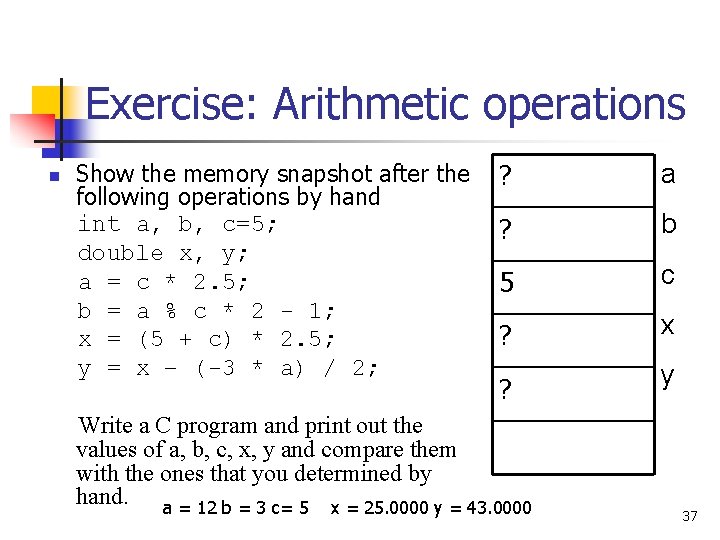
Exercise: Arithmetic operations n Show the memory snapshot after the following operations by hand int a, b, c=5; double x, y; a = c * 2. 5; b = a % c * 2 - 1; x = (5 + c) * 2. 5; y = x – (-3 * a) / 2; ? a ? b 5 c ? x ? y Write a C program and print out the values of a, b, c, x, y and compare them with the ones that you determined by hand. a = 12 b = 3 c= 5 x = 25. 0000 y = 43. 0000 37
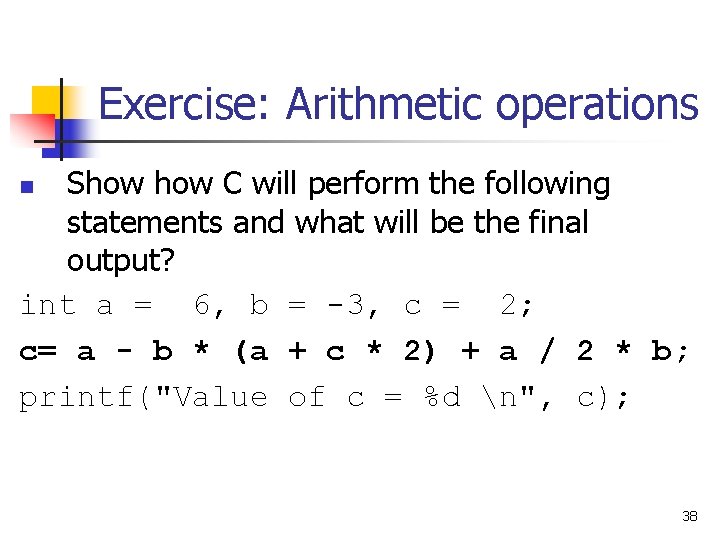
Exercise: Arithmetic operations Show C will perform the following statements and what will be the final output? int a = 6, b = -3, c = 2; c= a - b * (a + c * 2) + a / 2 * b; printf("Value of c = %d n", c); n 38
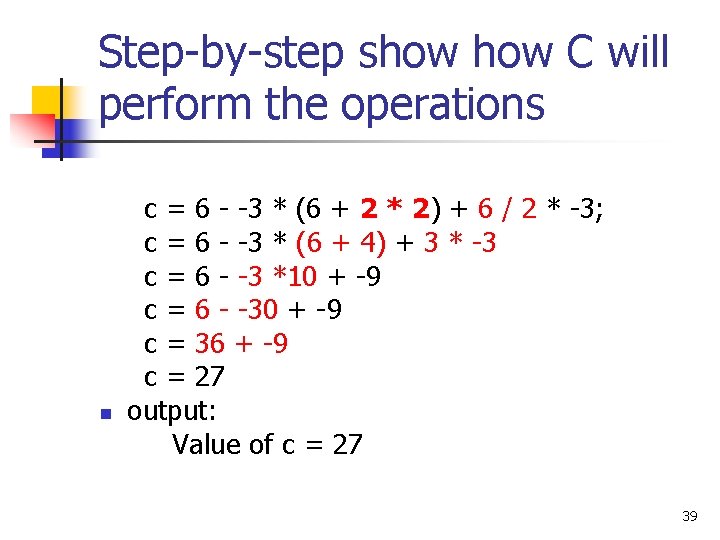
Step-by-step show C will perform the operations c = 6 - -3 * (6 + 2 * 2) + 6 / 2 * -3; c = 6 - -3 * (6 + 4) + 3 * -3 c = 6 - -3 *10 + -9 c = 6 - -30 + -9 c = 36 + -9 c = 27 n output: Value of c = 27 39
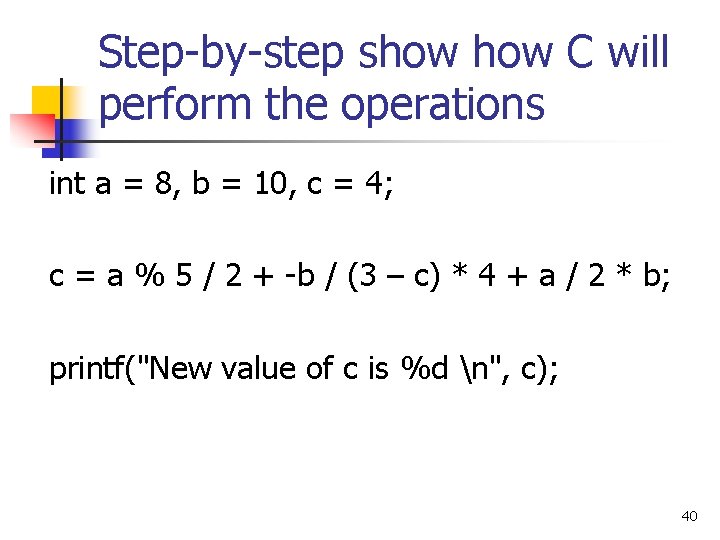
Step-by-step show C will perform the operations int a = 8, b = 10, c = 4; c = a % 5 / 2 + -b / (3 – c) * 4 + a / 2 * b; printf("New value of c is %d n", c); 40
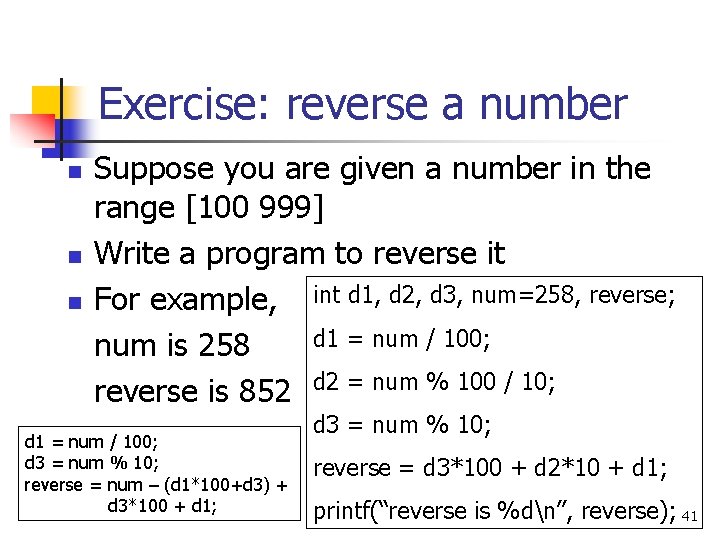
Exercise: reverse a number n n n Suppose you are given a number in the range [100 999] Write a program to reverse it For example, int d 1, d 2, d 3, num=258, reverse; d 1 = num / 100; num is 258 reverse is 852 d 2 = num % 100 / 10; d 1 = num / 100; d 3 = num % 10; reverse = num – (d 1*100+d 3) + d 3*100 + d 1; d 3 = num % 10; reverse = d 3*100 + d 2*10 + d 1; printf(“reverse is %dn”, reverse); 41
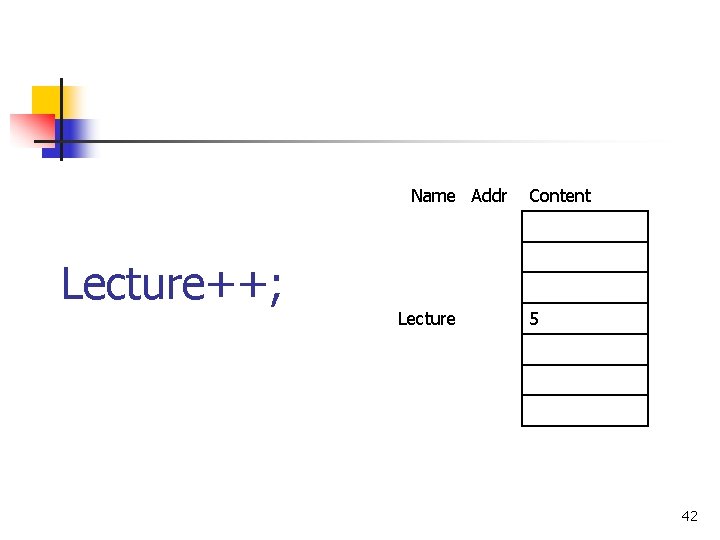
Name Addr Content Lecture++; Lecture 5 42
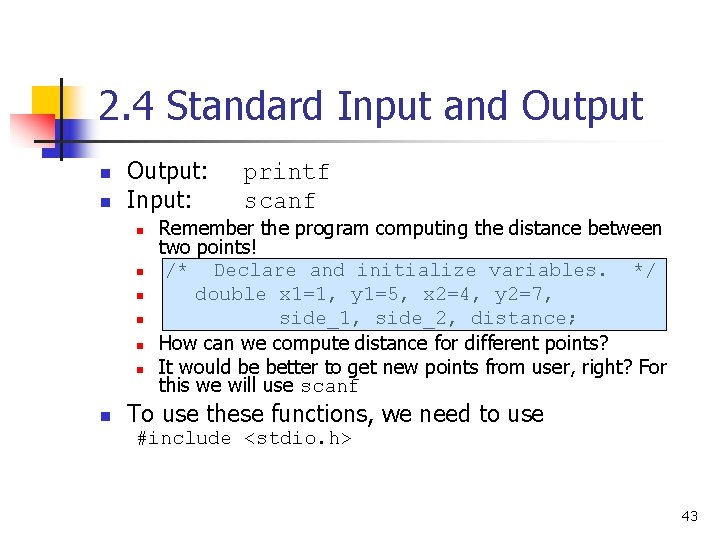
2. 4 Standard Input and Output n n Output: Input: n n n n printf scanf Remember the program computing the distance between two points! /* Declare and initialize variables. */ double x 1=1, y 1=5, x 2=4, y 2=7, side_1, side_2, distance; How can we compute distance for different points? It would be better to get new points from user, right? For this we will use scanf To use these functions, we need to use #include <stdio. h> 43
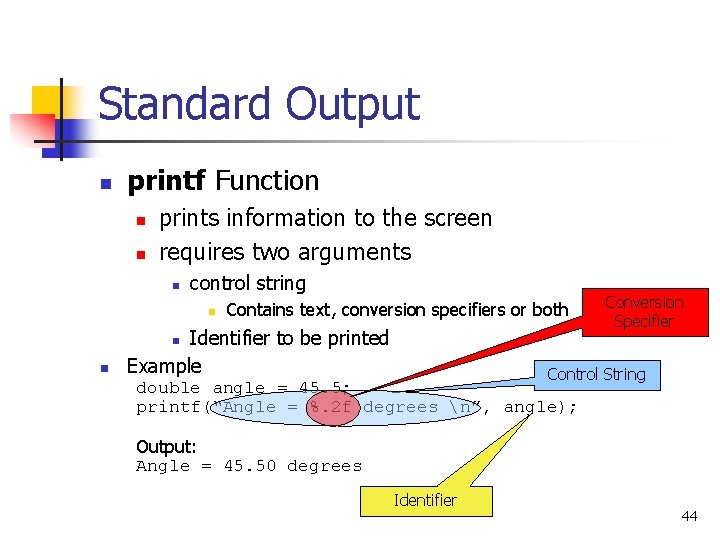
Standard Output n printf Function n n prints information to the screen requires two arguments n control string n Contains text, conversion specifiers or both Identifier to be printed Example Conversion Specifier n n Control String double angle = 45. 5; printf(“Angle = %. 2 f degrees n”, angle); Output: Angle = 45. 50 degrees Identifier 44
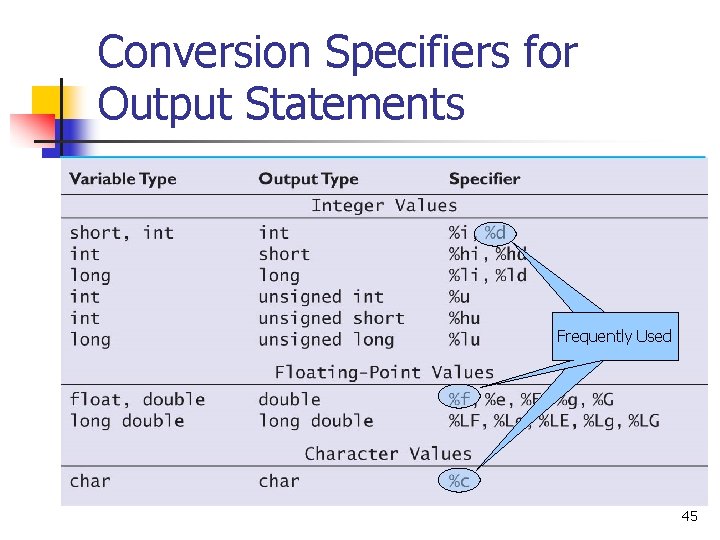
Conversion Specifiers for Output Statements Frequently Used 45
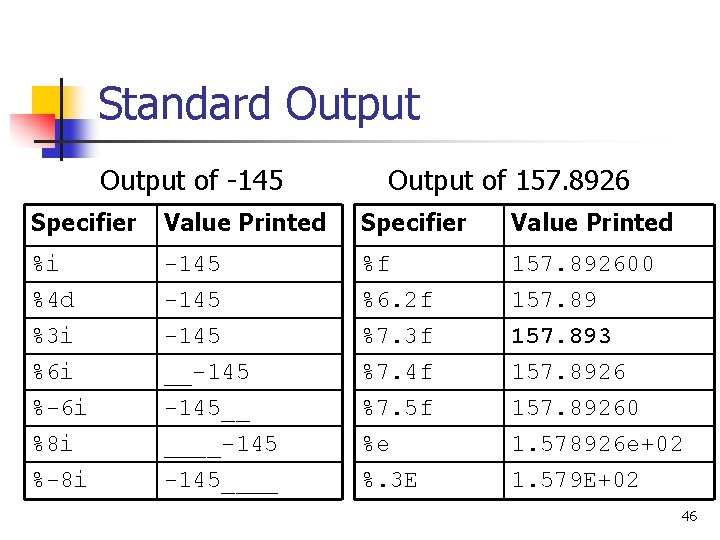
Standard Output of -145 Output of 157. 8926 Specifier Value Printed %i %4 d %3 i -145 %f %6. 2 f %7. 3 f 157. 892600 157. 893 %6 i %-6 i %8 i %-8 i __-145__ ____-145____ %7. 4 f %7. 5 f %e %. 3 E 157. 89260 1. 578926 e+02 1. 579 E+02 46
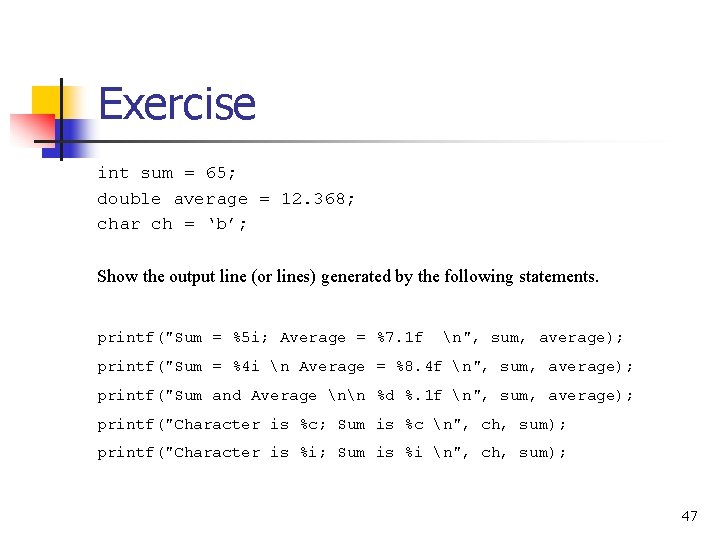
Exercise int sum = 65; double average = 12. 368; char ch = ‘b’; Show the output line (or lines) generated by the following statements. printf("Sum = %5 i; Average = %7. 1 f n", sum, average); printf("Sum = %4 i n Average = %8. 4 f n", sum, average); printf("Sum and Average nn %d %. 1 f n", sum, average); printf("Character is %c; Sum is %c n", ch, sum); printf("Character is %i; Sum is %i n", ch, sum); 47
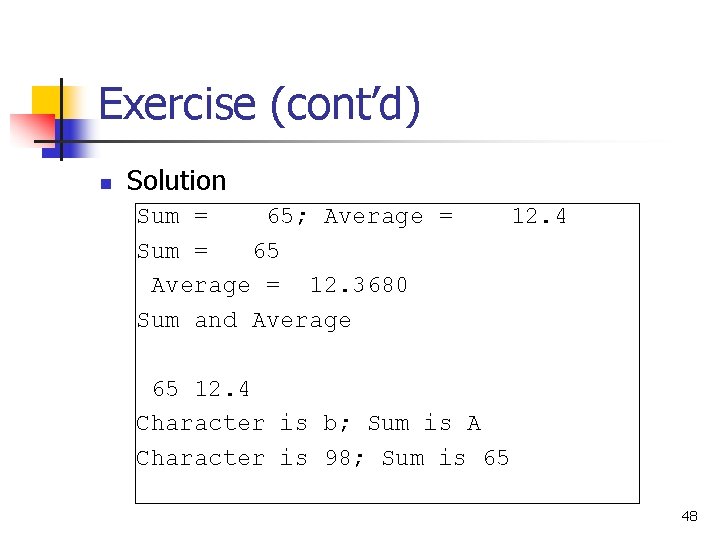
Exercise (cont’d) n Solution Sum = 65; Average = Sum = 65 Average = 12. 3680 Sum and Average 12. 4 65 12. 4 Character is b; Sum is A Character is 98; Sum is 65 48
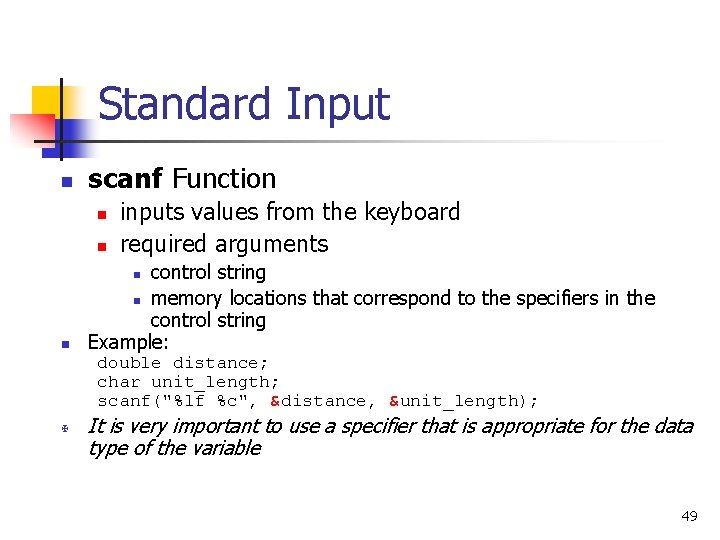
Standard Input n scanf Function n n inputs values from the keyboard required arguments control string n memory locations that correspond to the specifiers in the control string Example: n n double distance; char unit_length; scanf("%lf %c", &distance, &unit_length); X It is very important to use a specifier that is appropriate for the data type of the variable 49
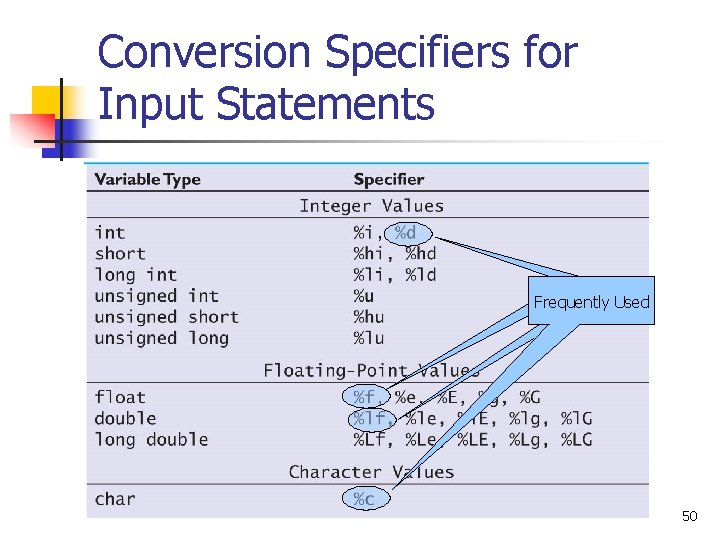
Conversion Specifiers for Input Statements Frequently Used 50
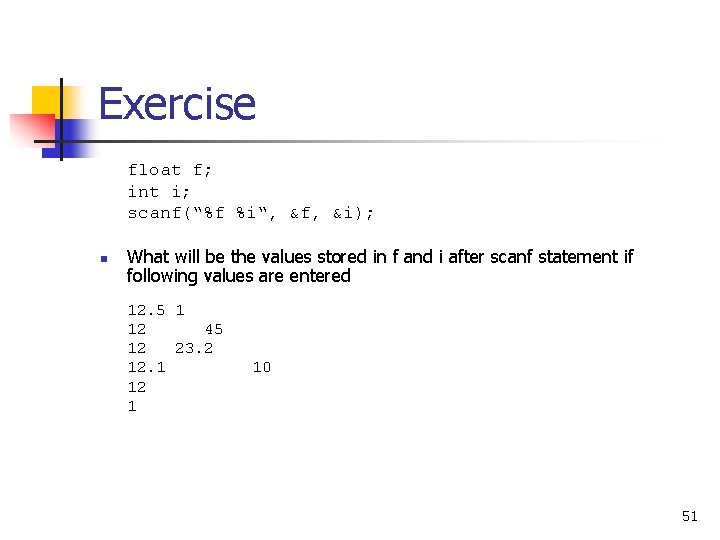
Exercise float f; int i; scanf(“%f %i“, &f, &i); n What will be the values stored in f and i after scanf statement if following values are entered 12. 5 1 12 45 12 23. 2 12. 1 12 1 10 51
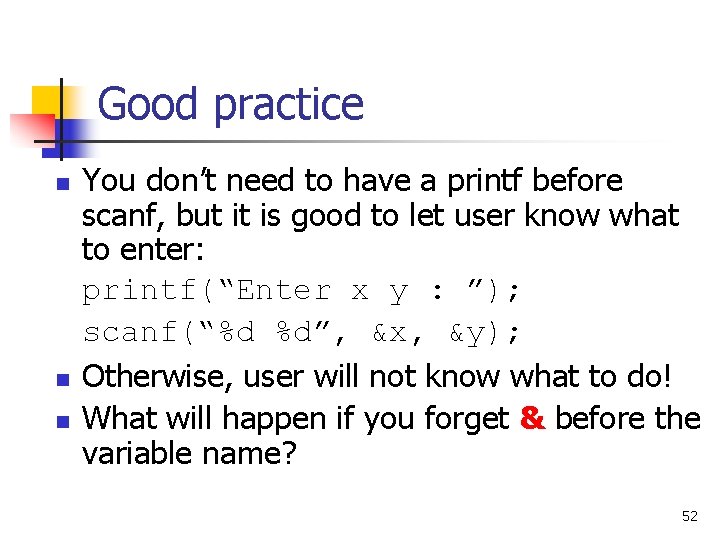
Good practice n n n You don’t need to have a printf before scanf, but it is good to let user know what to enter: printf(“Enter x y : ”); scanf(“%d %d”, &x, &y); Otherwise, user will not know what to do! What will happen if you forget & before the variable name? 52
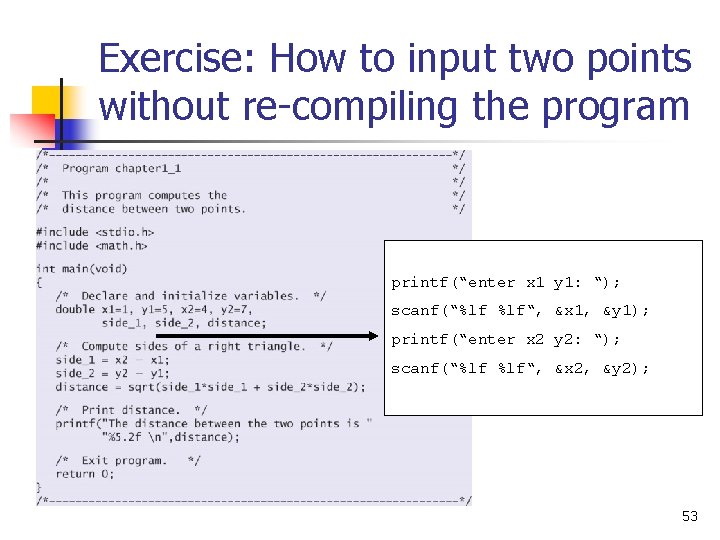
Exercise: How to input two points without re-compiling the program printf(“enter x 1 y 1: “); scanf(“%lf %lf“, &x 1, &y 1); printf(“enter x 2 y 2: “); scanf(“%lf %lf“, &x 2, &y 2); 53
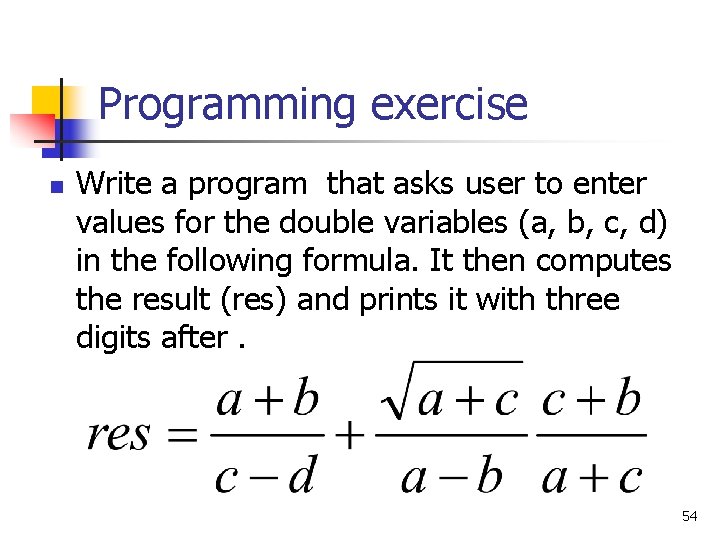
Programming exercise n Write a program that asks user to enter values for the double variables (a, b, c, d) in the following formula. It then computes the result (res) and prints it with three digits after. 54
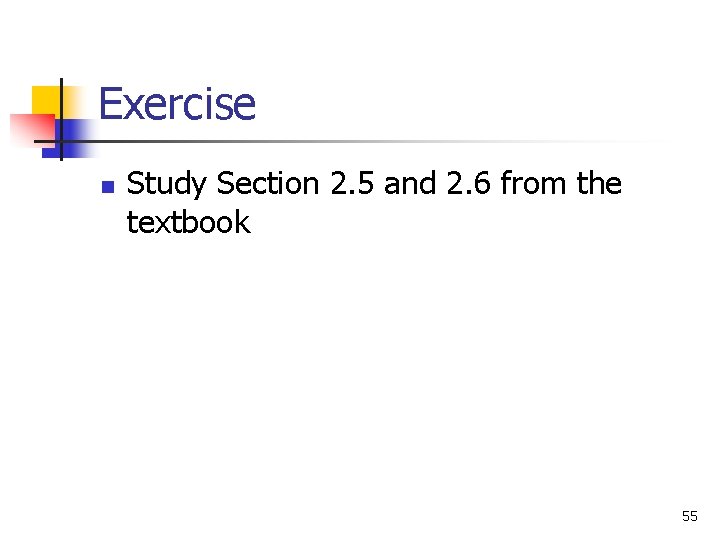
Exercise n Study Section 2. 5 and 2. 6 from the textbook 55
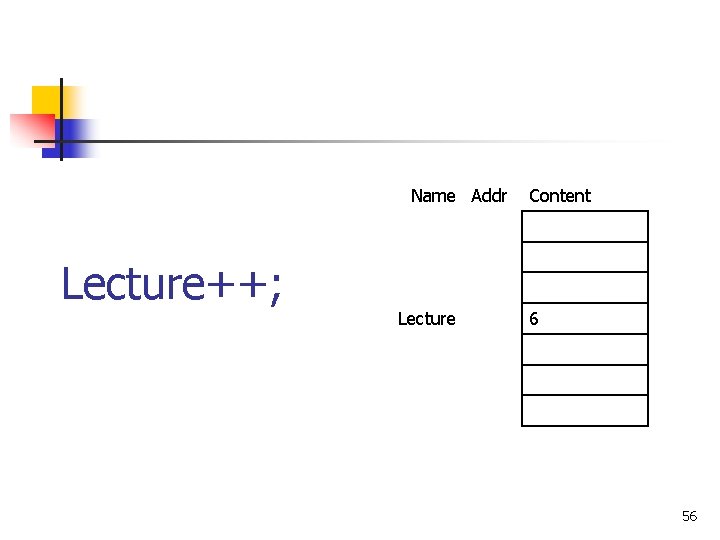
Name Addr Content Lecture++; Lecture 6 56
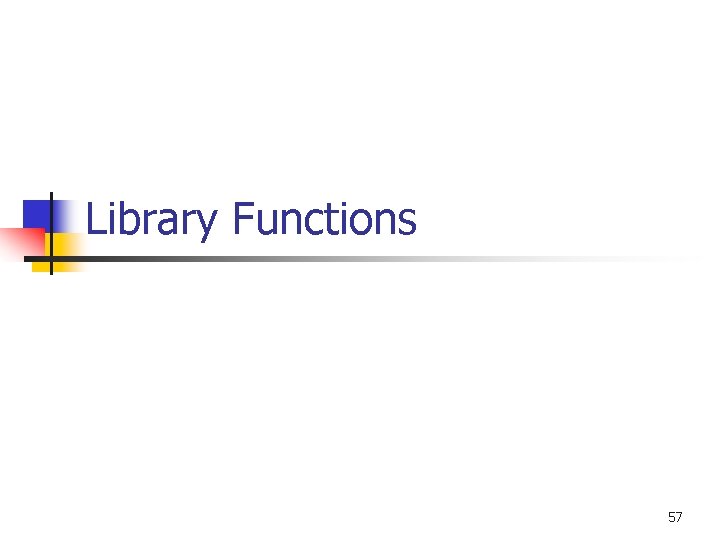
Library Functions 57
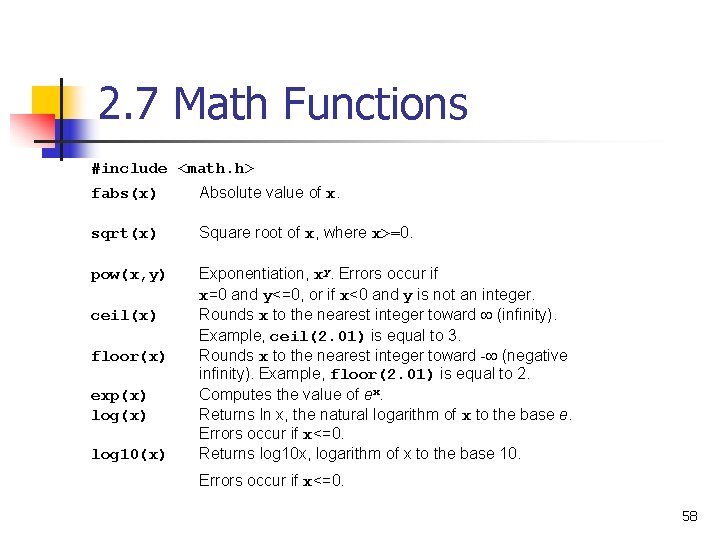
2. 7 Math Functions #include <math. h> fabs(x) Absolute value of x. sqrt(x) Square root of x, where x>=0. pow(x, y) Exponentiation, xy. Errors occur if x=0 and y<=0, or if x<0 and y is not an integer. Rounds x to the nearest integer toward (infinity). Example, ceil(2. 01) is equal to 3. Rounds x to the nearest integer toward - (negative infinity). Example, floor(2. 01) is equal to 2. Computes the value of ex. Returns ln x, the natural logarithm of x to the base e. Errors occur if x<=0. Returns log 10 x, logarithm of x to the base 10. ceil(x) floor(x) exp(x) log 10(x) Errors occur if x<=0. 58
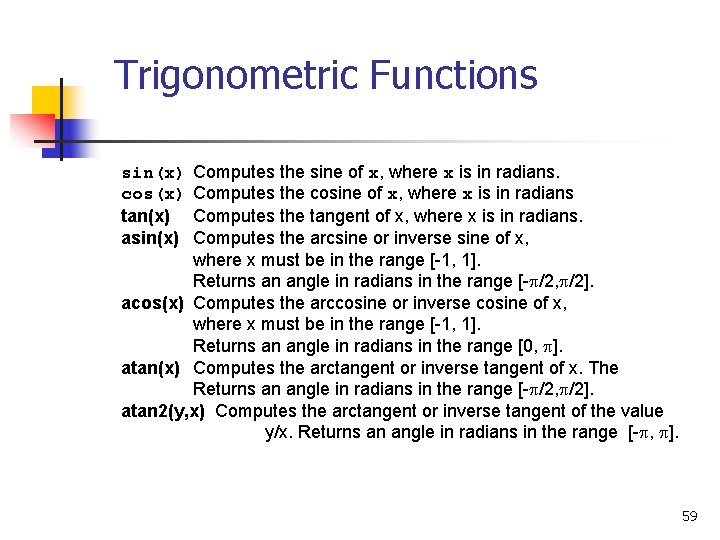
Trigonometric Functions Computes the sine of x, where x is in radians. Computes the cosine of x, where x is in radians Computes the tangent of x, where x is in radians. Computes the arcsine or inverse sine of x, where x must be in the range [-1, 1]. Returns an angle in radians in the range [- /2, /2]. acos(x) Computes the arccosine or inverse cosine of x, where x must be in the range [-1, 1]. Returns an angle in radians in the range [0, ]. atan(x) Computes the arctangent or inverse tangent of x. The Returns an angle in radians in the range [- /2, /2]. atan 2(y, x) Computes the arctangent or inverse tangent of the value y/x. Returns an angle in radians in the range [- , ]. sin(x) cos(x) tan(x) asin(x) 59
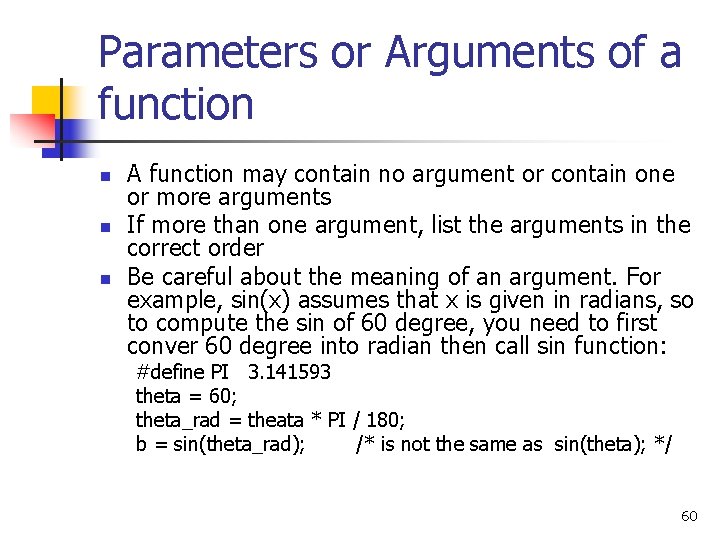
Parameters or Arguments of a function n A function may contain no argument or contain one or more arguments If more than one argument, list the arguments in the correct order Be careful about the meaning of an argument. For example, sin(x) assumes that x is given in radians, so to compute the sin of 60 degree, you need to first conver 60 degree into radian then call sin function: #define PI 3. 141593 theta = 60; theta_rad = theata * PI / 180; b = sin(theta_rad); /* is not the same as sin(theta); */ 60
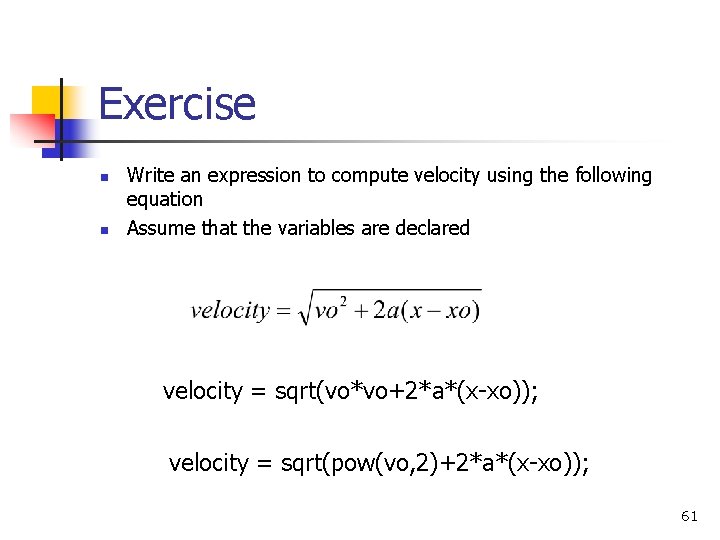
Exercise n n Write an expression to compute velocity using the following equation Assume that the variables are declared velocity = sqrt(vo*vo+2*a*(x-xo)); velocity = sqrt(pow(vo, 2)+2*a*(x-xo)); 61
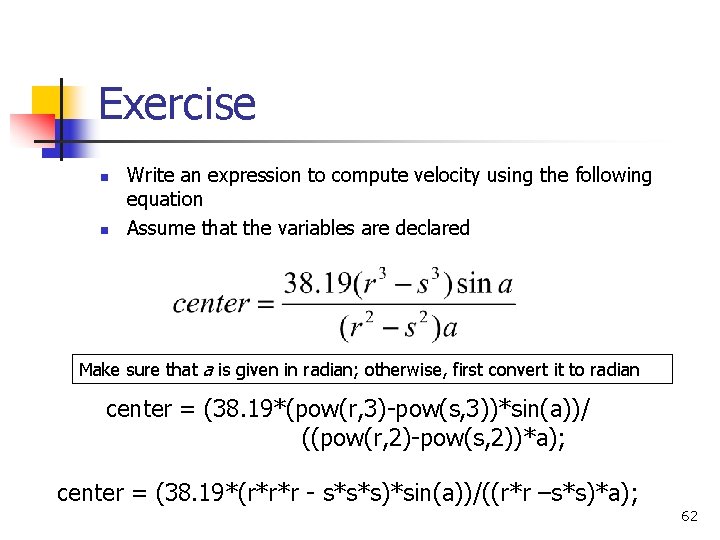
Exercise n n Write an expression to compute velocity using the following equation Assume that the variables are declared Make sure that a is given in radian; otherwise, first convert it to radian center = (38. 19*(pow(r, 3)-pow(s, 3))*sin(a))/ ((pow(r, 2)-pow(s, 2))*a); center = (38. 19*(r*r*r - s*s*s)*sin(a))/((r*r –s*s)*a); 62
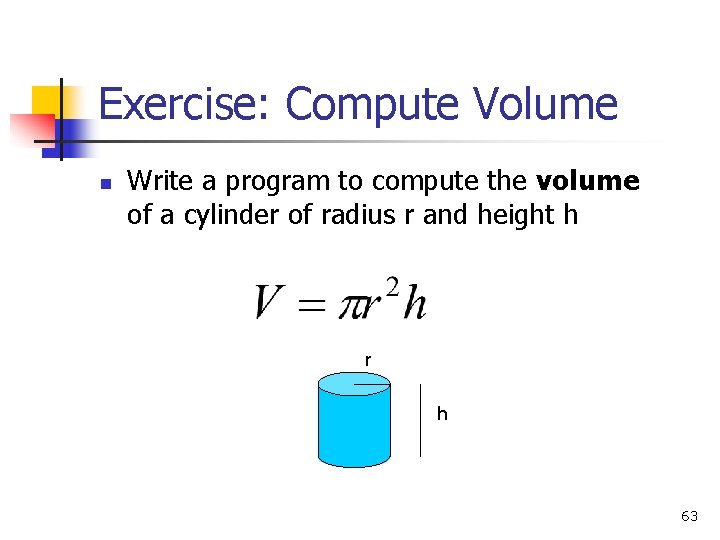
Exercise: Compute Volume n Write a program to compute the volume of a cylinder of radius r and height h r h 63
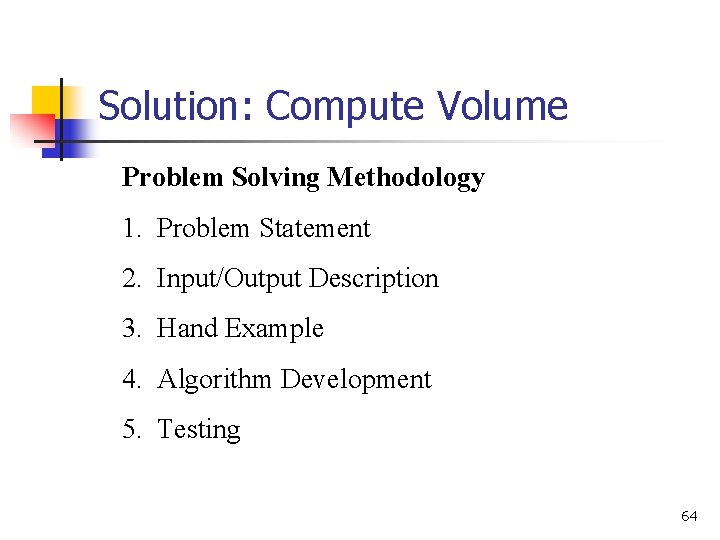
Solution: Compute Volume Problem Solving Methodology 1. Problem Statement 2. Input/Output Description 3. Hand Example 4. Algorithm Development 5. Testing 64
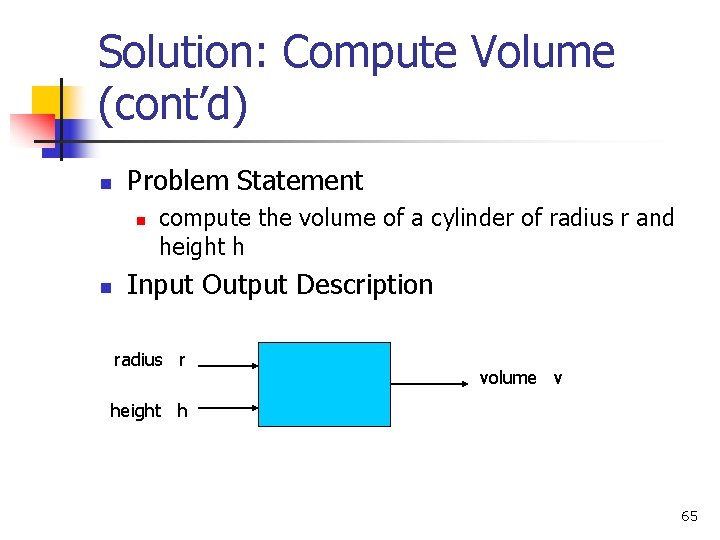
Solution: Compute Volume (cont’d) n Problem Statement n n compute the volume of a cylinder of radius r and height h Input Output Description radius r volume v height h 65
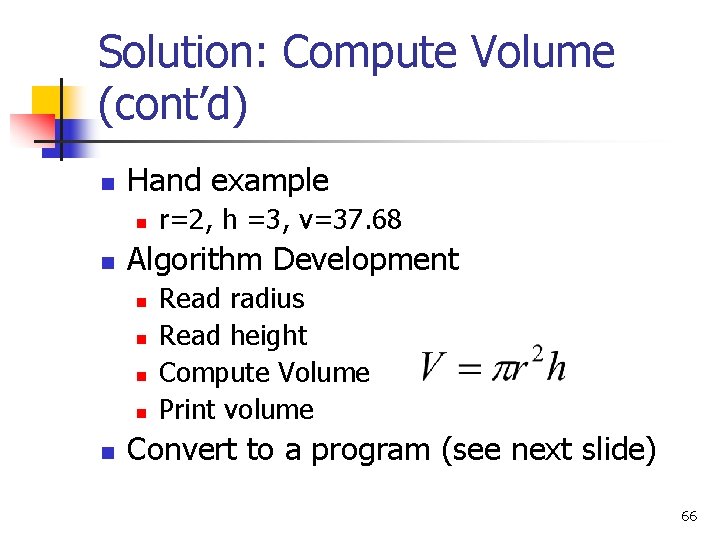
Solution: Compute Volume (cont’d) n Hand example n n Algorithm Development n n n r=2, h =3, v=37. 68 Read radius Read height Compute Volume Print volume Convert to a program (see next slide) 66
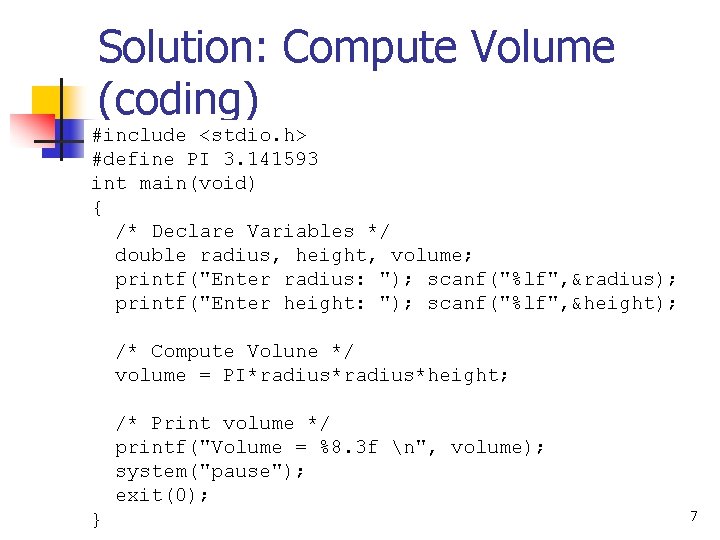
Solution: Compute Volume (coding) #include <stdio. h> #define PI 3. 141593 int main(void) { /* Declare Variables */ double radius, height, volume; printf("Enter radius: "); scanf("%lf", &radius); printf("Enter height: "); scanf("%lf", &height); /* Compute Volune */ volume = PI*radius*height; /* Print volume */ printf("Volume = %8. 3 f n", volume); system("pause"); exit(0); } 67
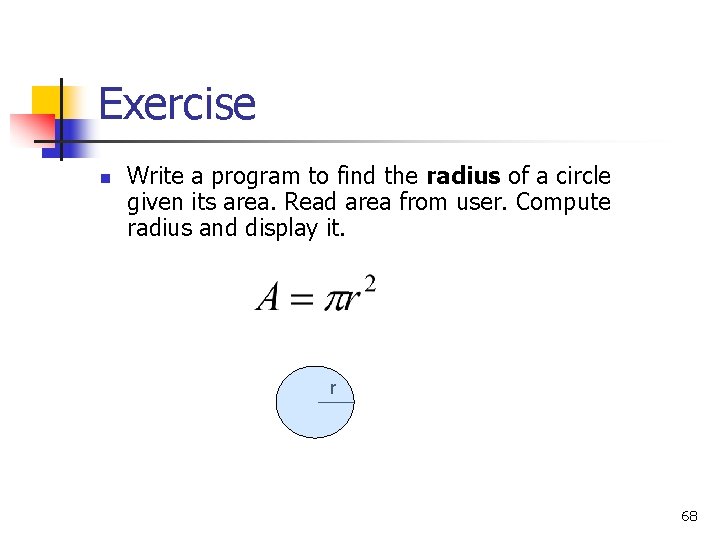
Exercise n Write a program to find the radius of a circle given its area. Read area from user. Compute radius and display it. r 68
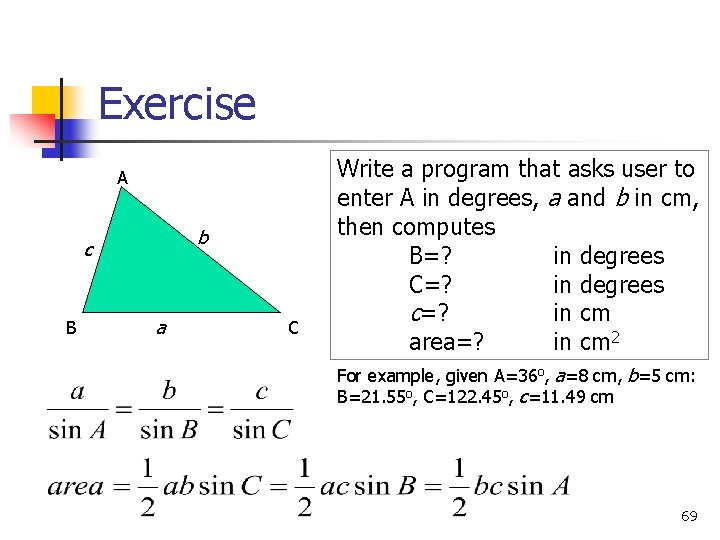
Exercise A b c B a C Write a program that asks user to enter A in degrees, a and b in cm, then computes B=? in degrees C=? in degrees c=? in cm area=? in cm 2 For example, given A=36 o, a=8 cm, b=5 cm: B=21. 55 o, C=122. 45 o, c=11. 49 cm 69
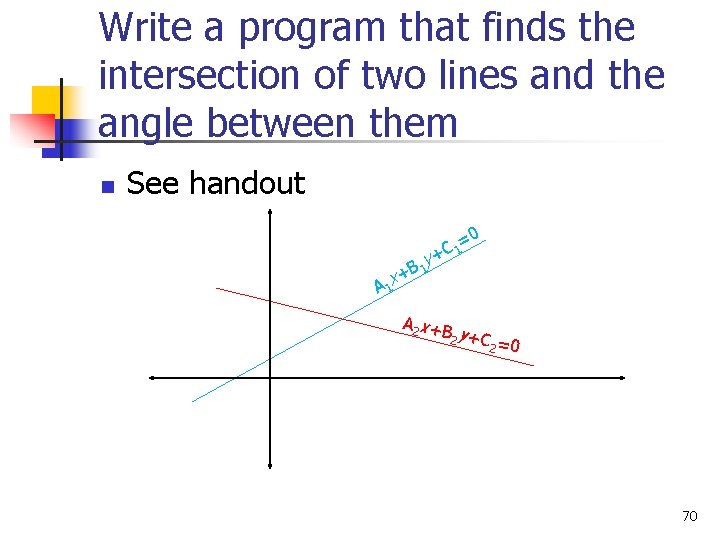
Write a program that finds the intersection of two lines and the angle between them n See handout A 1 x y 1 B + =0 C 1 + A 2 x+B 2 y+C = 2 0 70
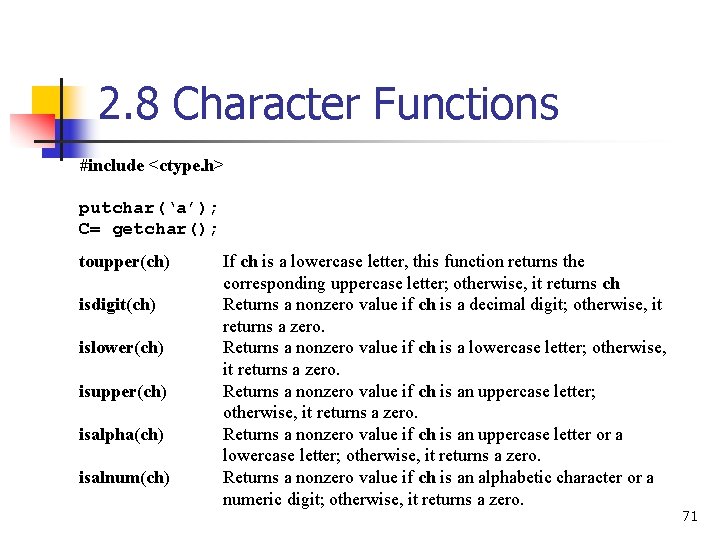
2. 8 Character Functions #include <ctype. h> putchar(‘a’); C= getchar(); toupper(ch) isdigit(ch) islower(ch) isupper(ch) isalpha(ch) isalnum(ch) If ch is a lowercase letter, this function returns the corresponding uppercase letter; otherwise, it returns ch Returns a nonzero value if ch is a decimal digit; otherwise, it returns a zero. Returns a nonzero value if ch is a lowercase letter; otherwise, it returns a zero. Returns a nonzero value if ch is an uppercase letter or a lowercase letter; otherwise, it returns a zero. Returns a nonzero value if ch is an alphabetic character or a numeric digit; otherwise, it returns a zero. 71
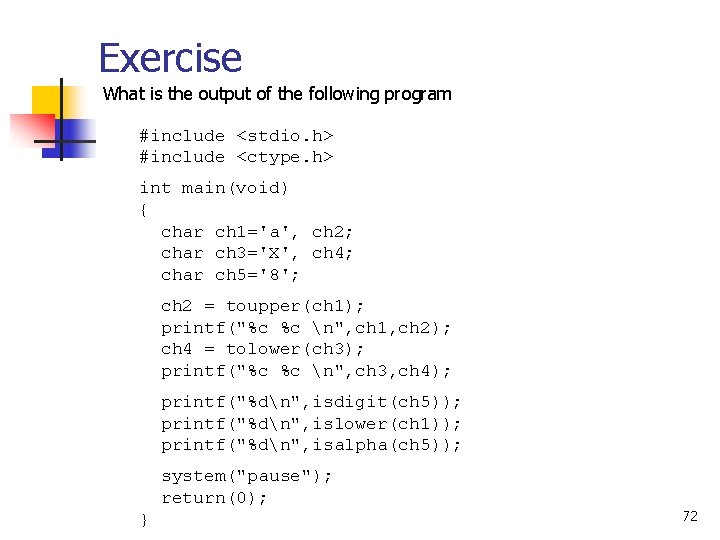
Exercise What is the output of the following program #include <stdio. h> #include <ctype. h> int main(void) { char ch 1='a', ch 2; char ch 3='X', ch 4; char ch 5='8'; ch 2 = toupper(ch 1); printf("%c %c n", ch 1, ch 2); ch 4 = tolower(ch 3); printf("%c %c n", ch 3, ch 4); printf("%dn", isdigit(ch 5)); printf("%dn", islower(ch 1)); printf("%dn", isalpha(ch 5)); system("pause"); return(0); } 72
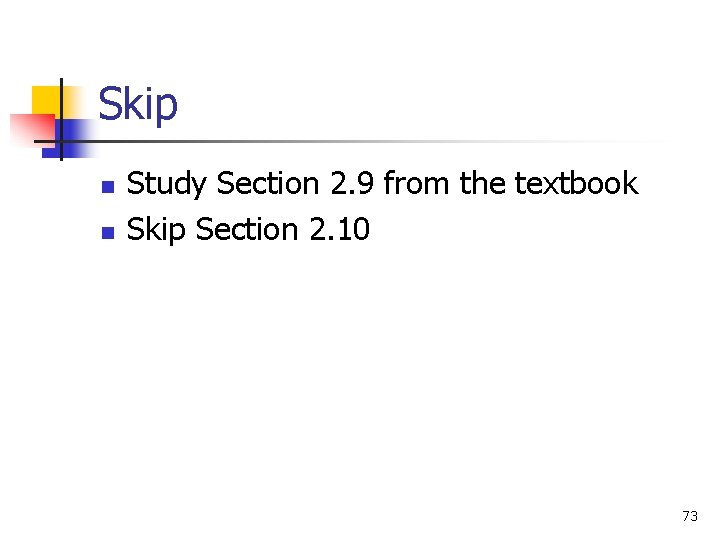
Skip n n Study Section 2. 9 from the textbook Skip Section 2. 10 73
Funksiyaning eng katta qiymati
Chapter 8 linear programming applications solutions
Perbedaan linear programming dan integer programming
Greedy vs dynamic
System programming vs application programming
Integer programming vs linear programming
Programing adalah
Business application in computer network
Subtraction rule example
Characteristics of mainframe computers
Principles of network applications
Objectives of computer applications
Computer integrated manufacturing applications
The elements of a computer
Computer networks and internets with internet applications
Business application in computer network
Business application in computer network
What is computer network and its applications
Nocti computer programming
What is nano programming
Concepts, techniques, and models of computer programming
Visual computer programming
American computer science league practice problems
Types of variables in computer programming
Programming raster display system in computer graphics
Components of computer programming
Computer programming chapter 1
Computer aided part programming
Discrete mathematics with applications susanna s. epp
Computer programming chapter 1
Computer programming chapter 1
Computer programming with matlab
Four special cases in linear programming
Decision making in computer programming
Computer organization
Programming fundamentals 1
Python programming an introduction to computer science
Trigonometrik tenglamalar yechish usullari
Numericalmethods.eng.usf.edu
Eng sodda ratsional kasrlarni integrallash
Eng efp camera
เฉลย my english lab unit 1
Pardasi
Pedagogika fanining asosiy manbalari
Dse english paper 3 weighting
English language paper 2 transactional writing
Eng vs efp
Eng m 401
Eng m 501
Eng electronic news gathering
Dse 2015 eng
Ta'lim turlari va shakllari
Numericalmethods.eng.usf.edu
Avestoni o'rgangan olimlar
Anorganik birikmalar sinflari
O'zaro tub sonlar
Komenskiyning pedagogik qarashlari
Sakrash yengil atletika
Sonlar yozilishi
Decimal to engineering notation
Fonetika bo'limi
Muskullar va ularning funksiyasi
Eng shdn
Monologik nutqni shakllantirishning nazariy asoslari
Zamonaviy axloqiy tarbiyaning yutuqlari
Heerlijk klonk het lied der eng'len
Eng katta natural son
Ilgarilama harakatda tezlik va tezlanish
Ip-eng-001
Axborot nima
Axborot qanday sifatlarga ega bo‘lishi kerak
سیگنال eng
Coefficient of traction for crawler tractor
Eng 1112