CS 2073 Computer Programming wEng Applications Ch 6
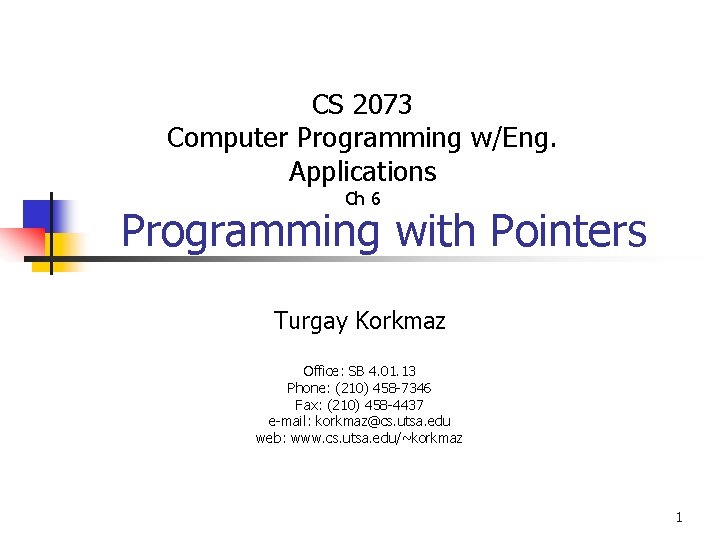
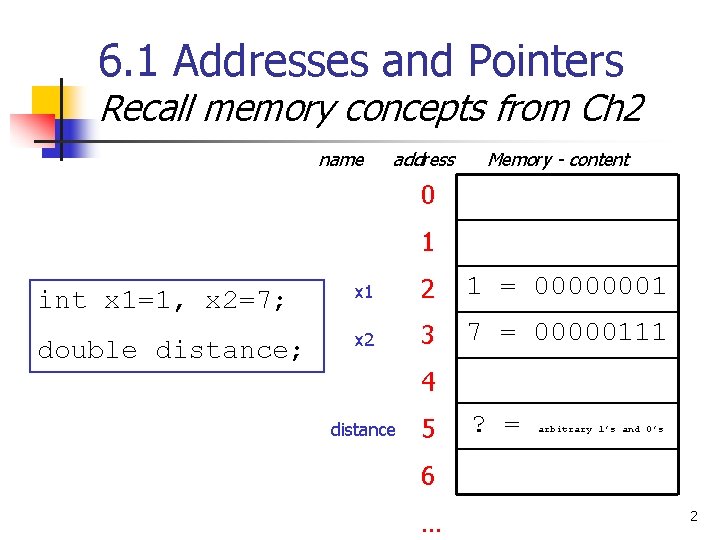
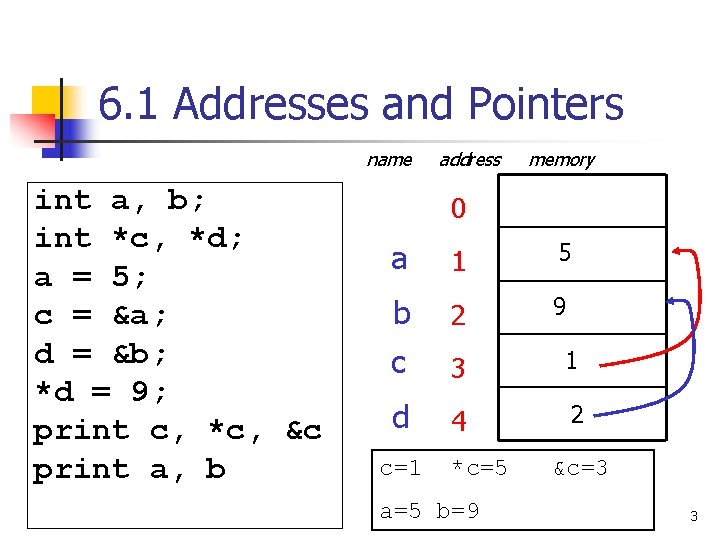
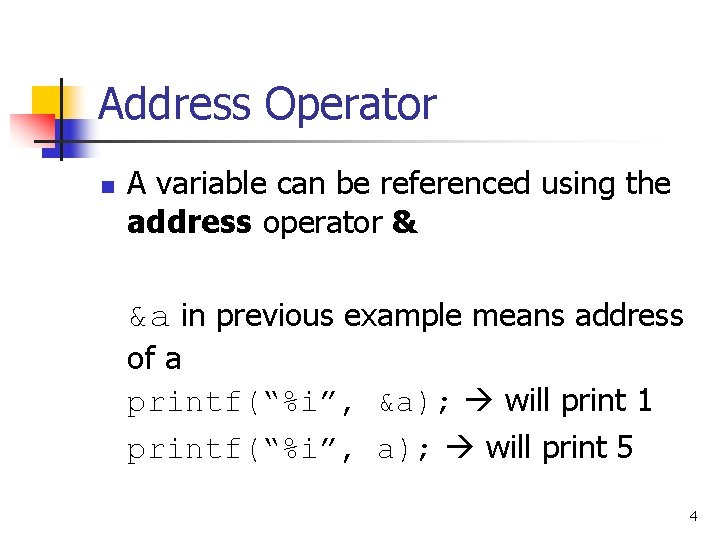
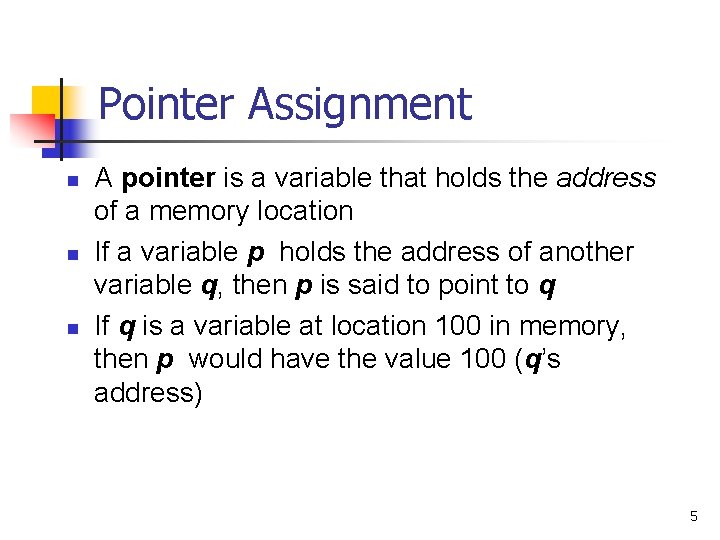
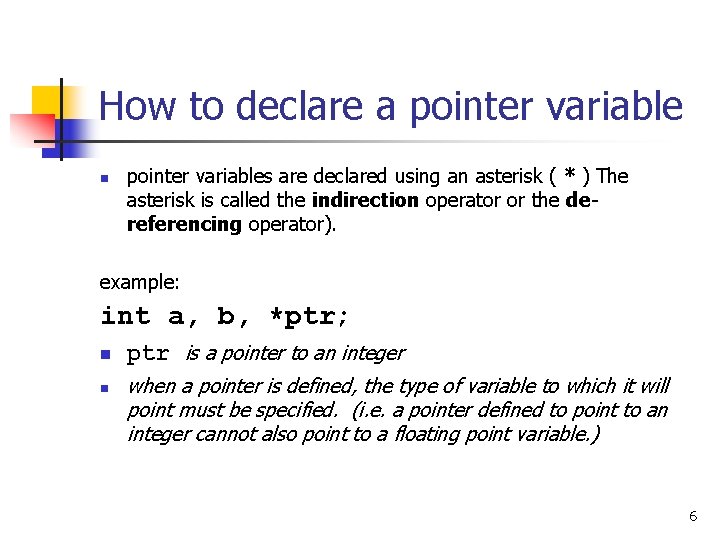
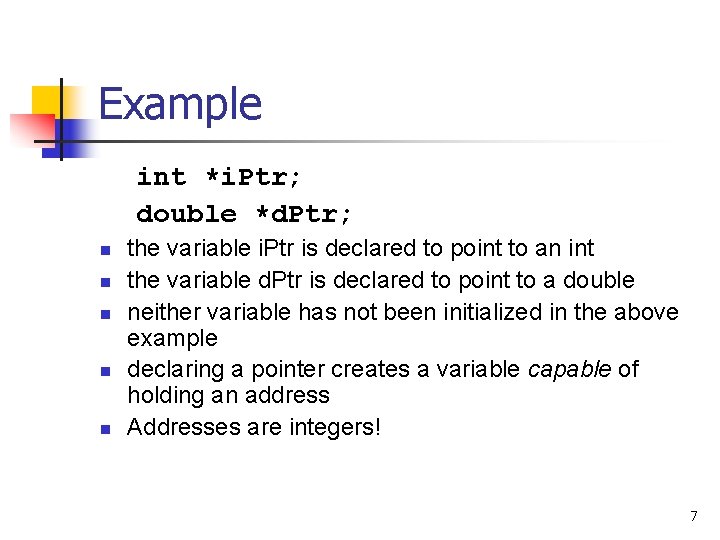
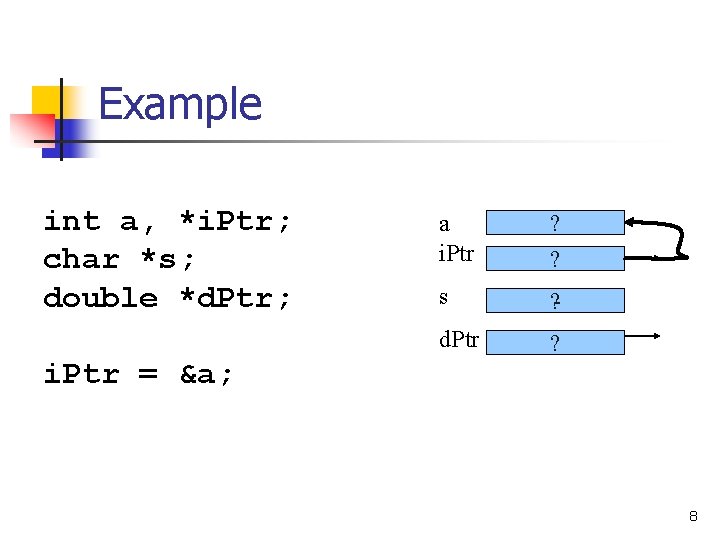
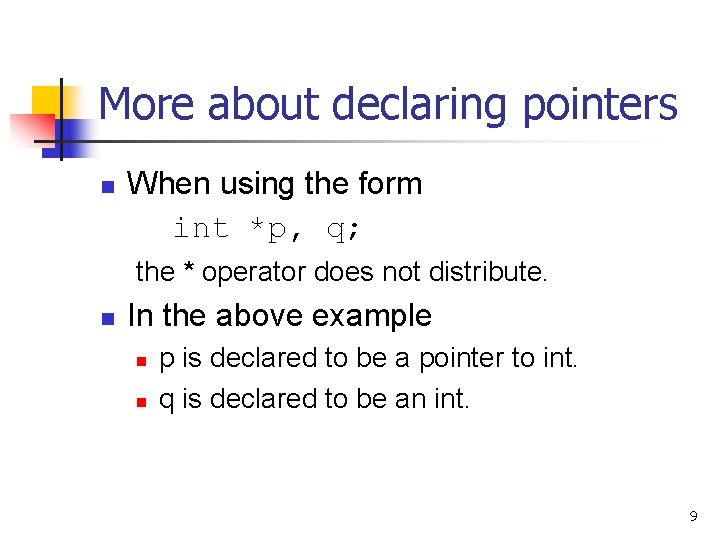
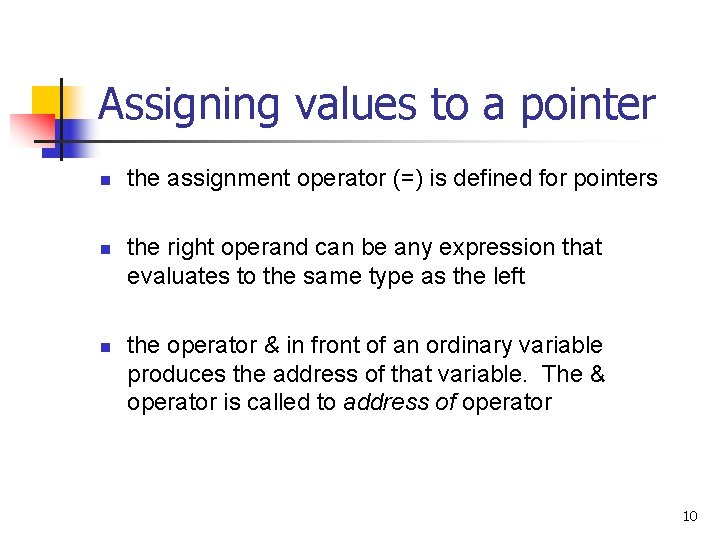
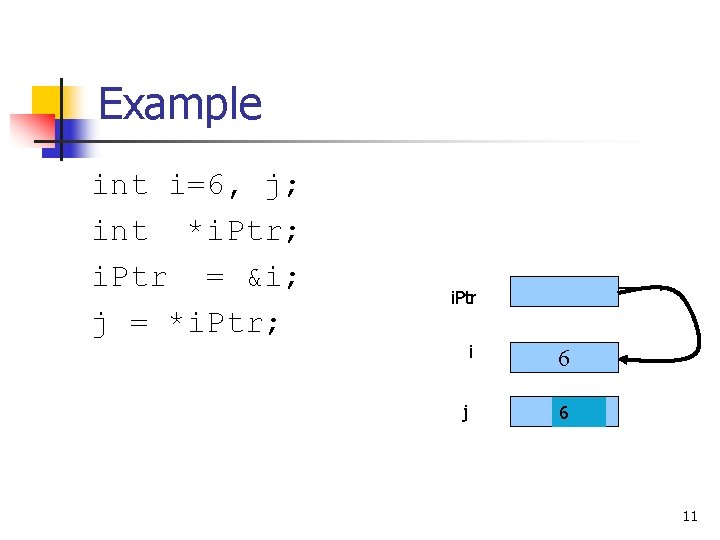
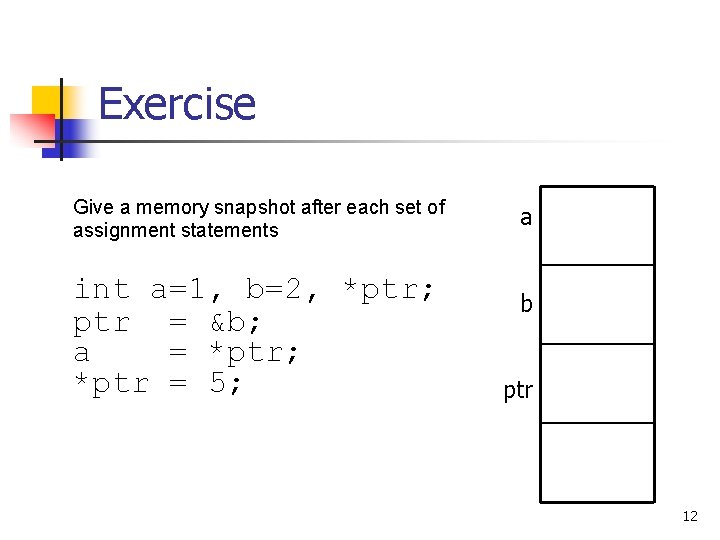
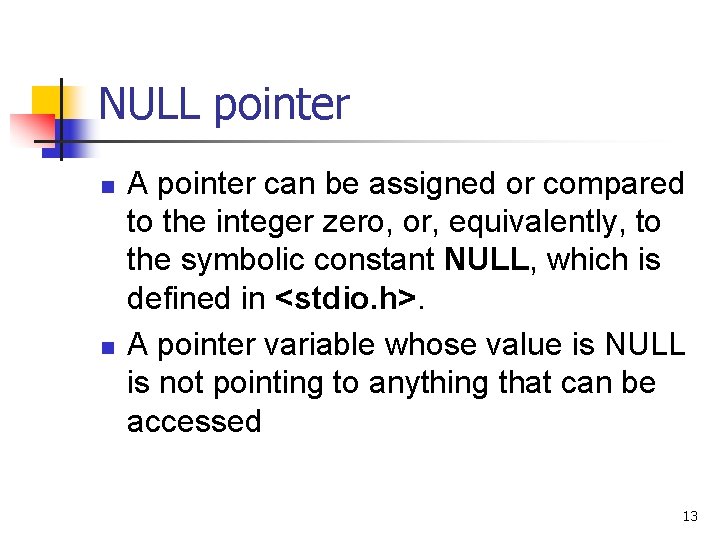
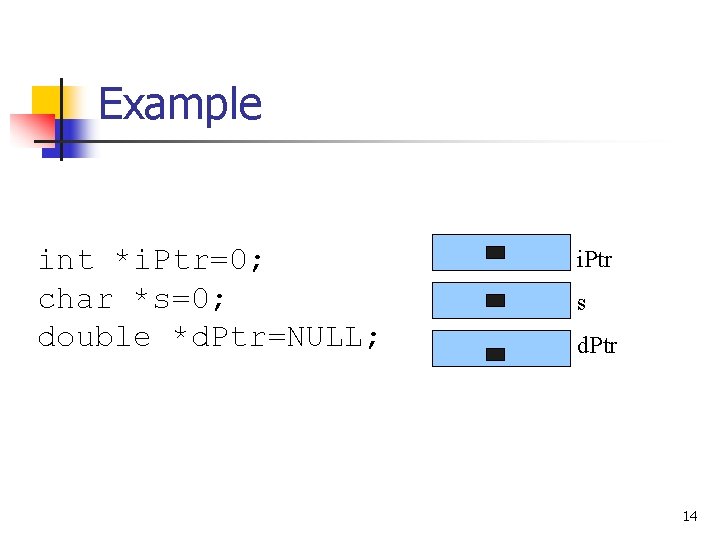
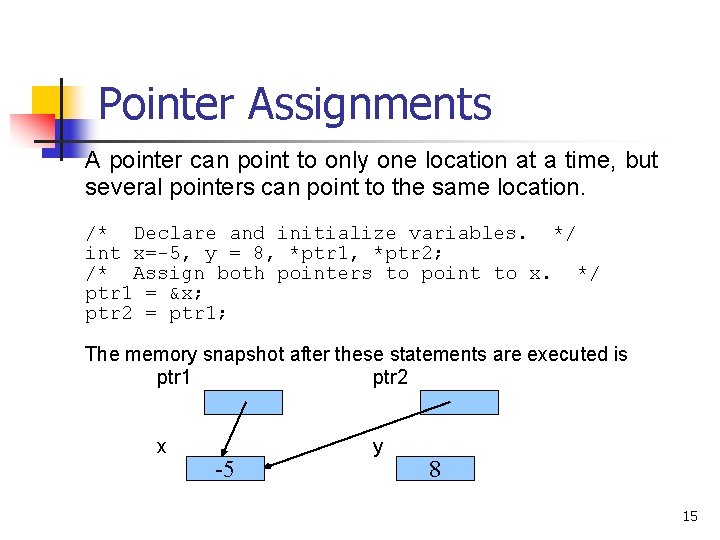
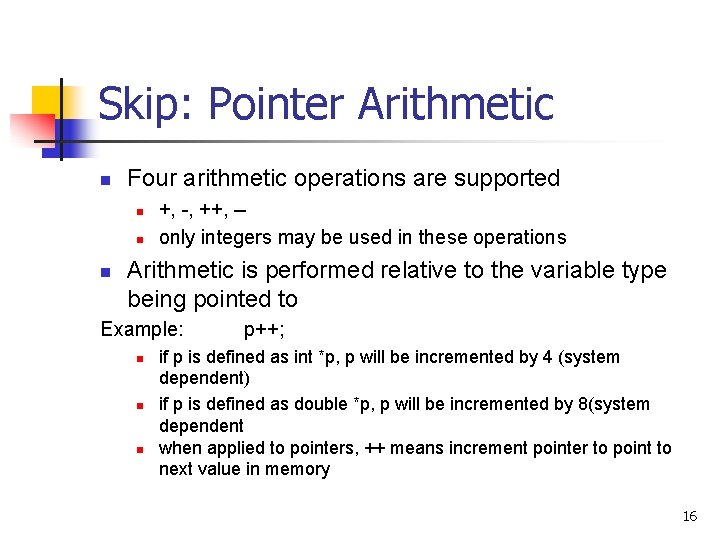
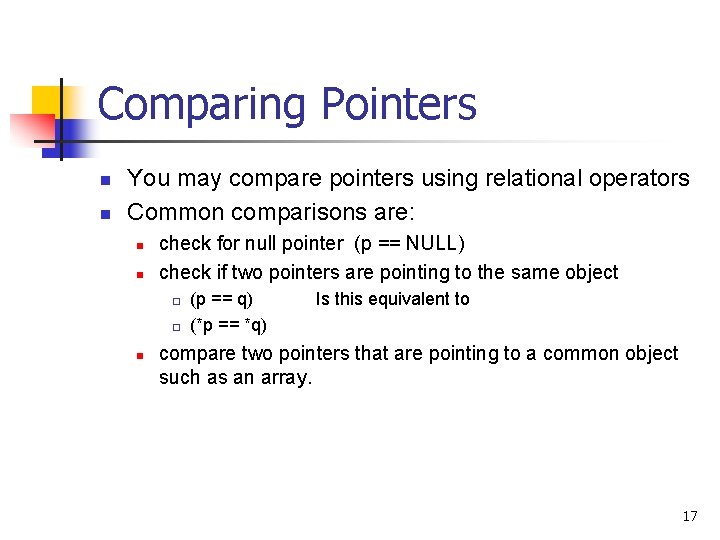
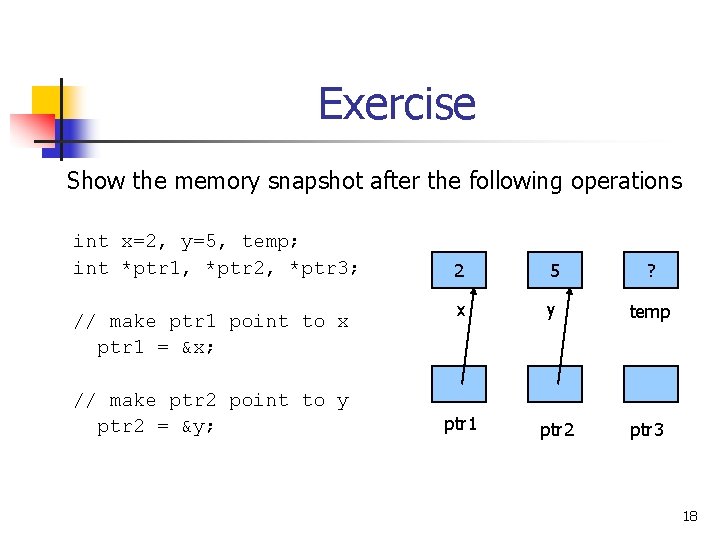
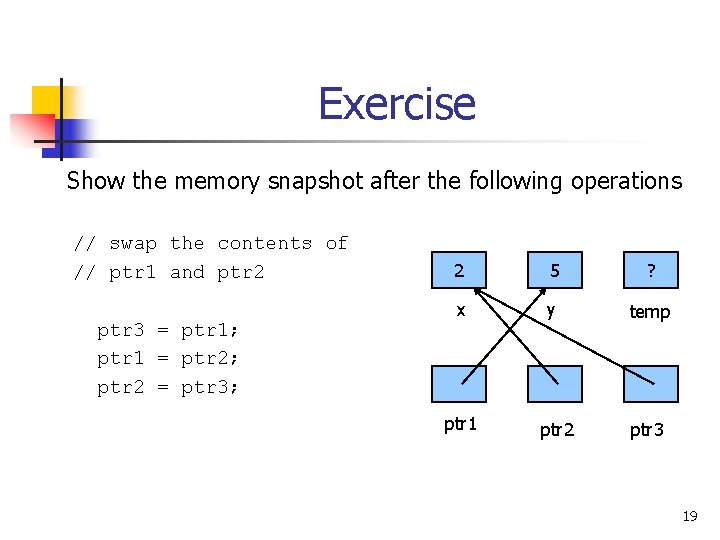
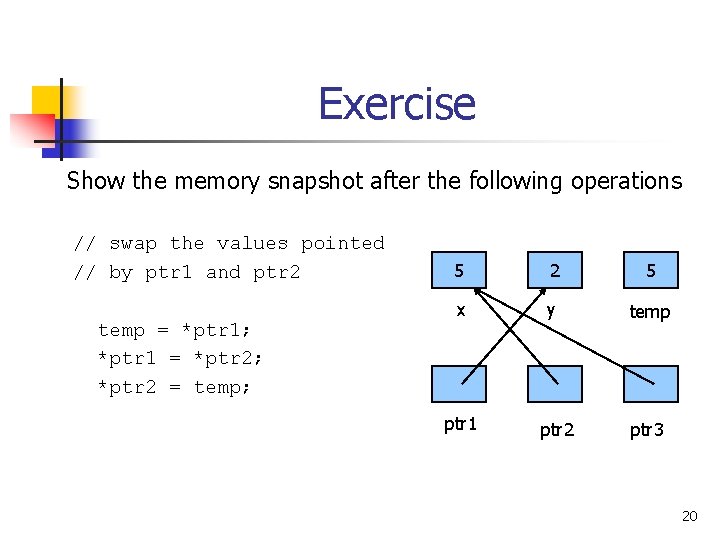
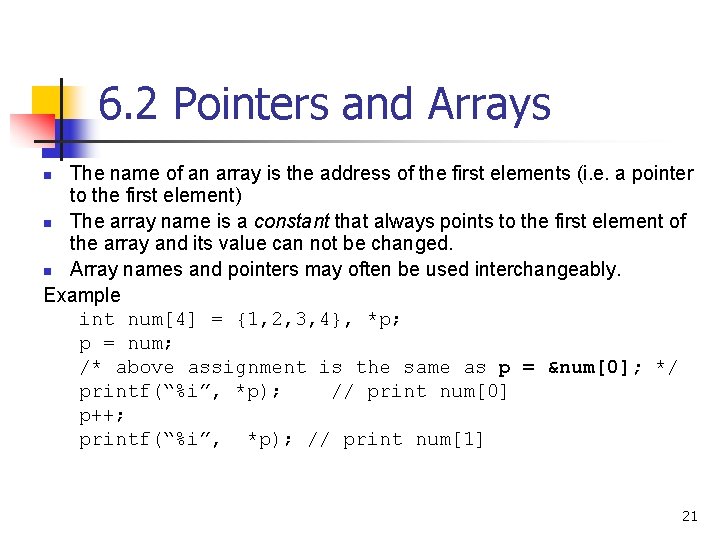
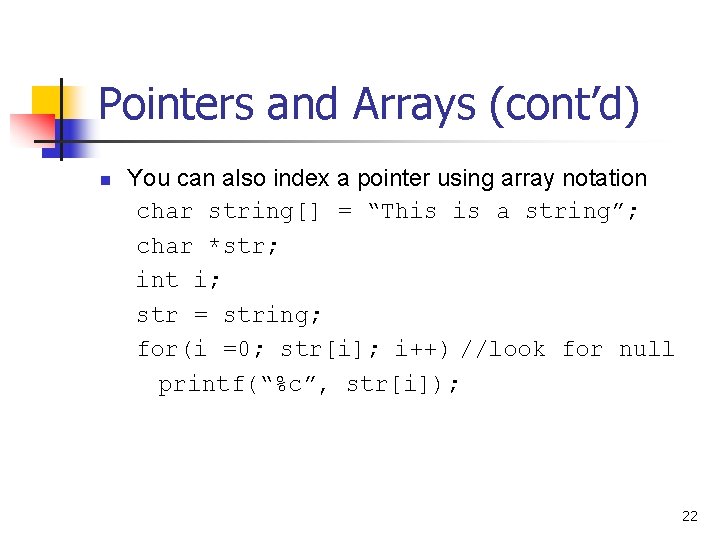
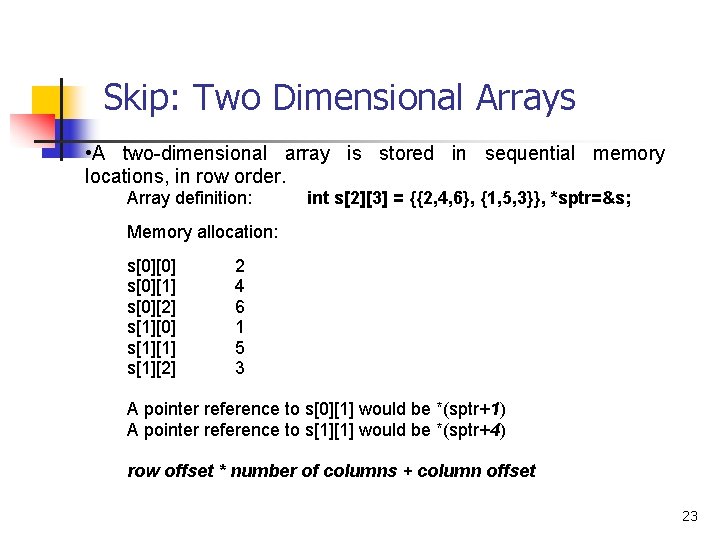
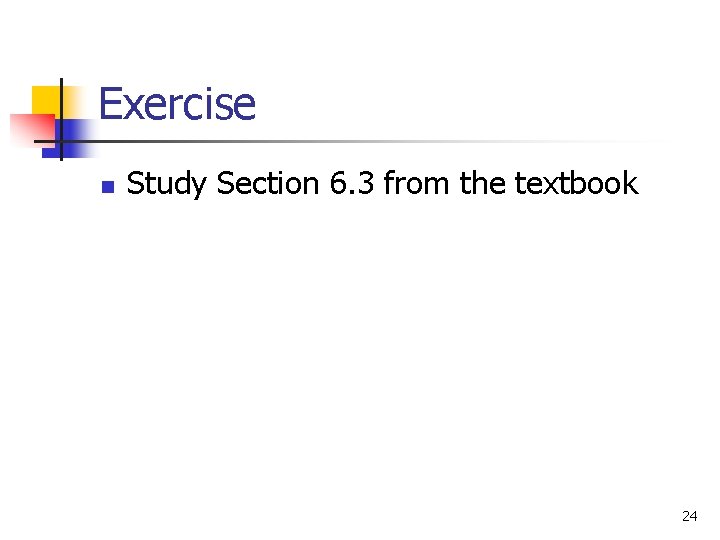
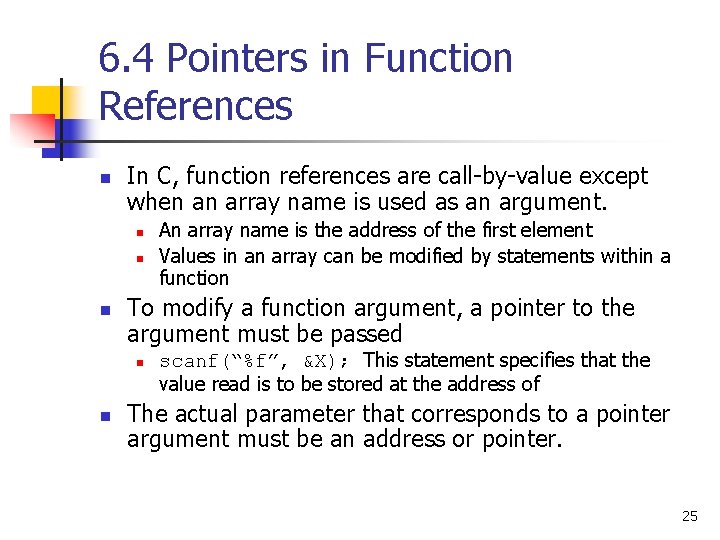
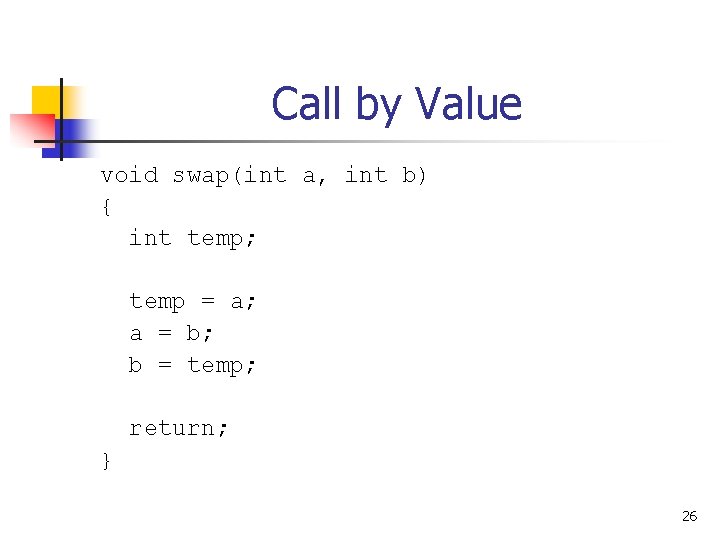
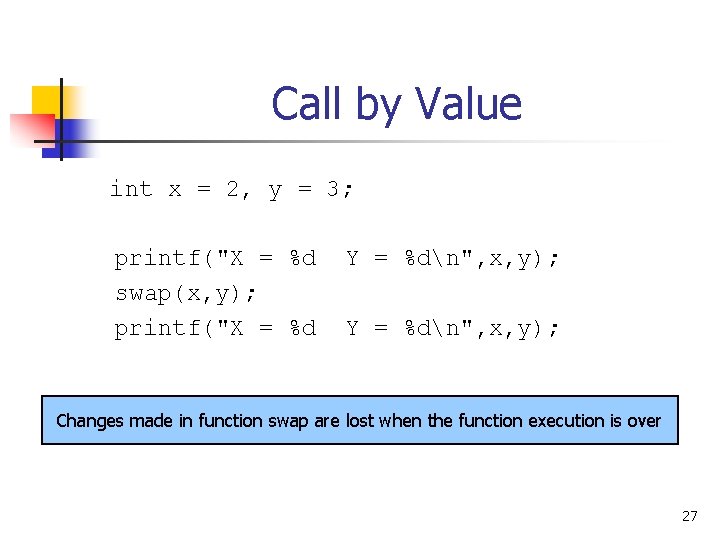
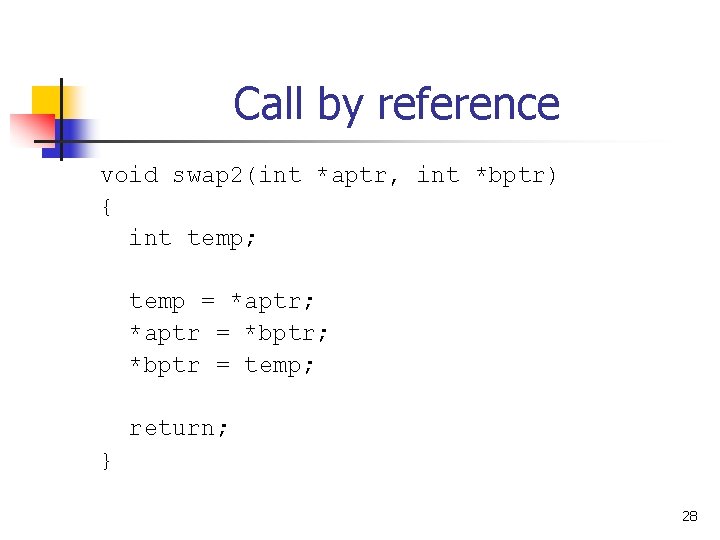
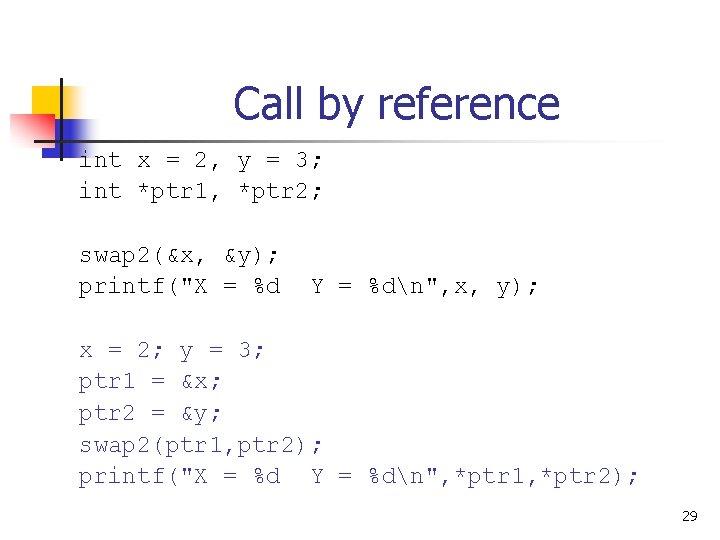
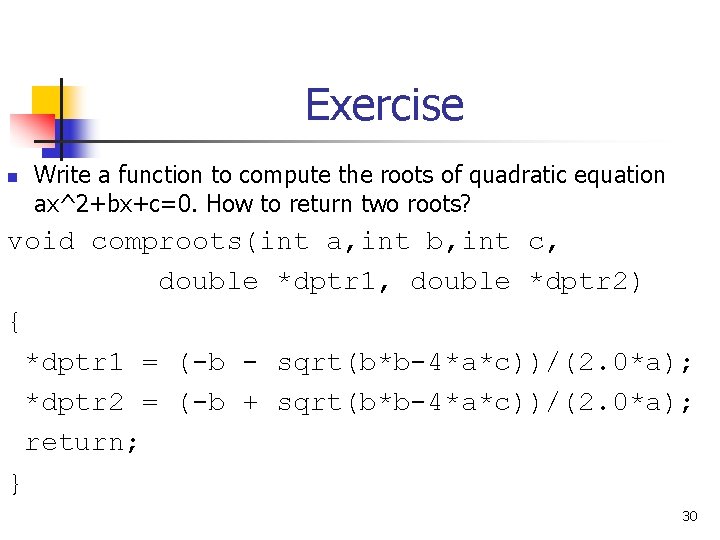
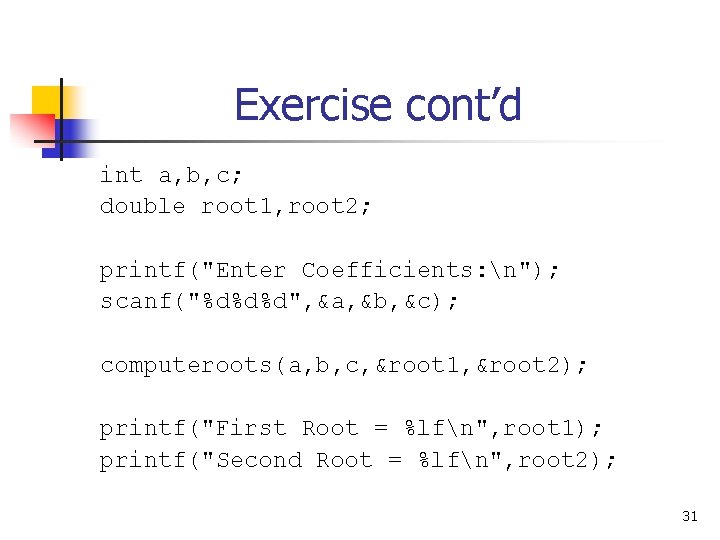
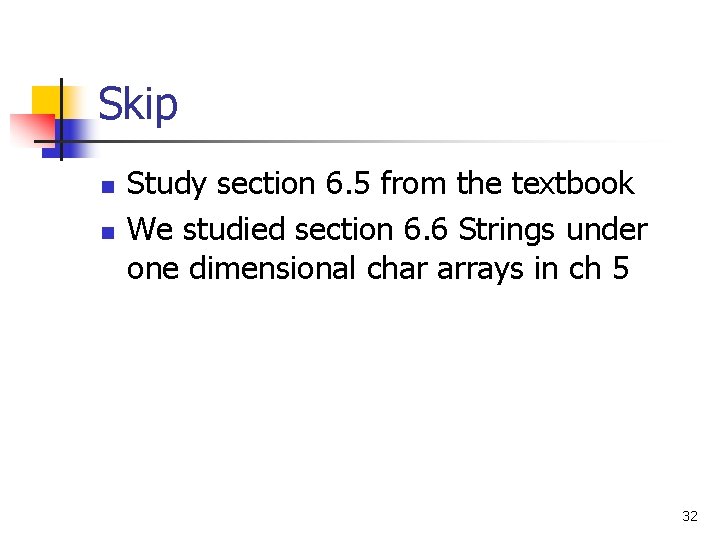
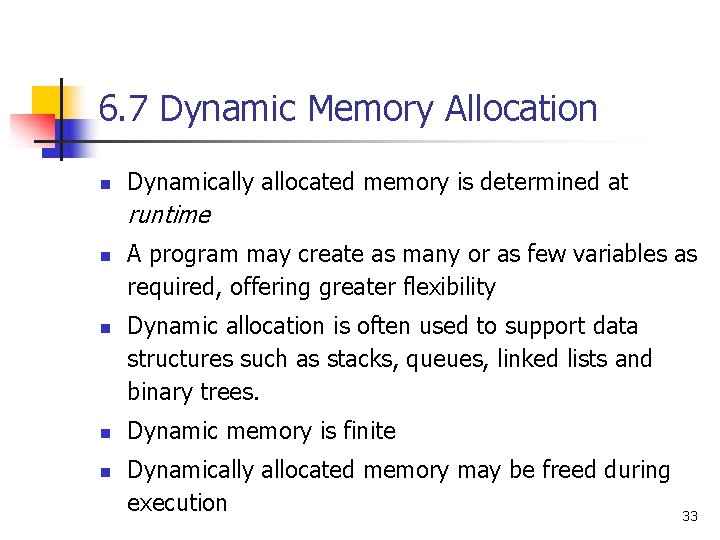
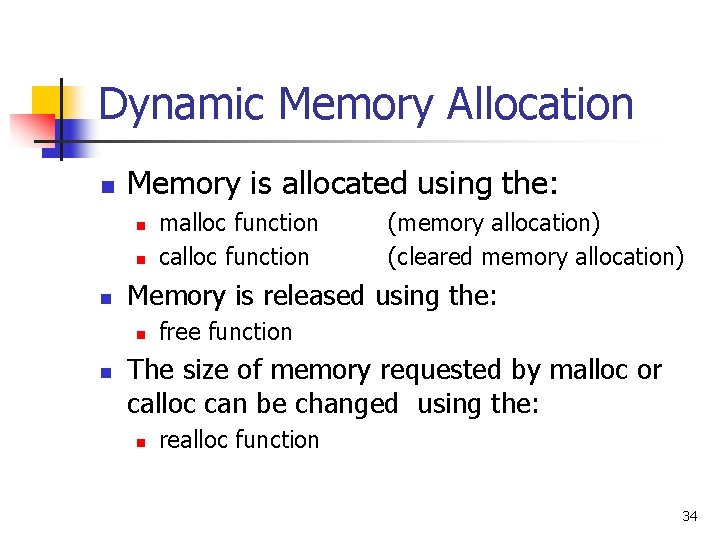
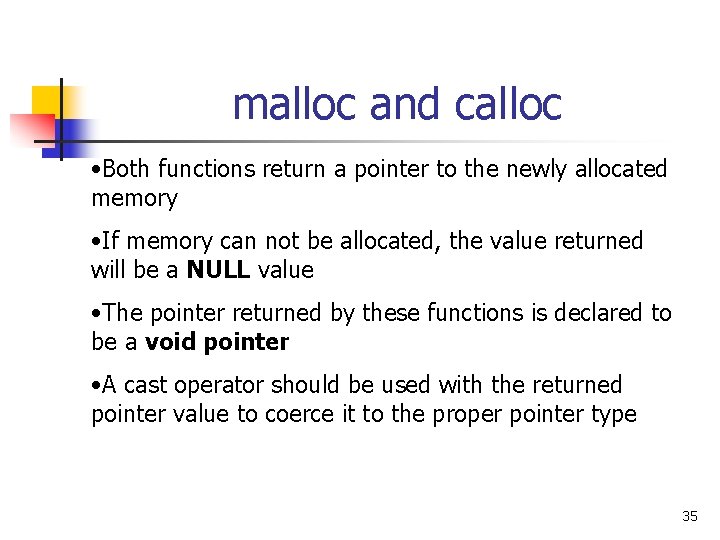
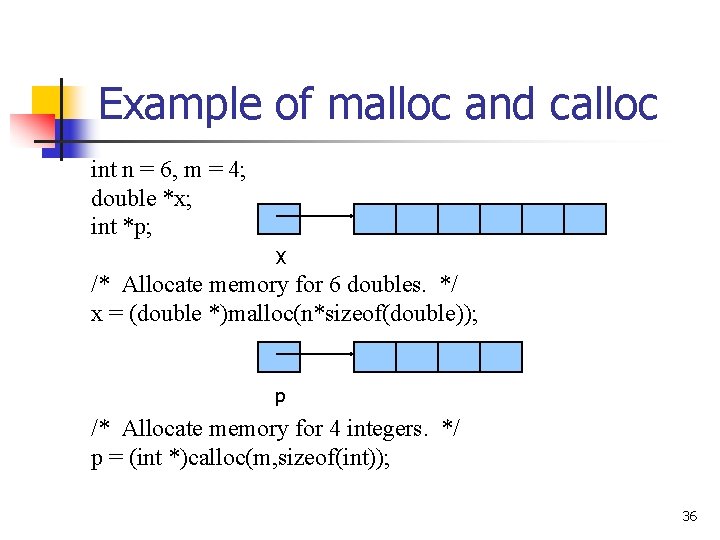
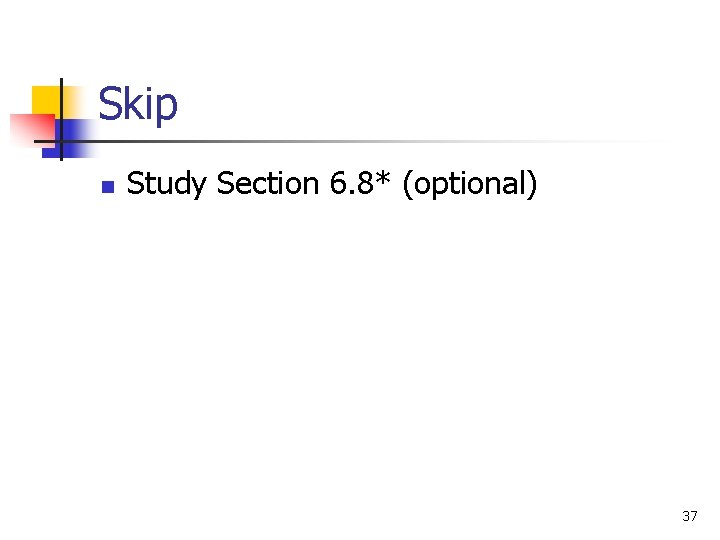
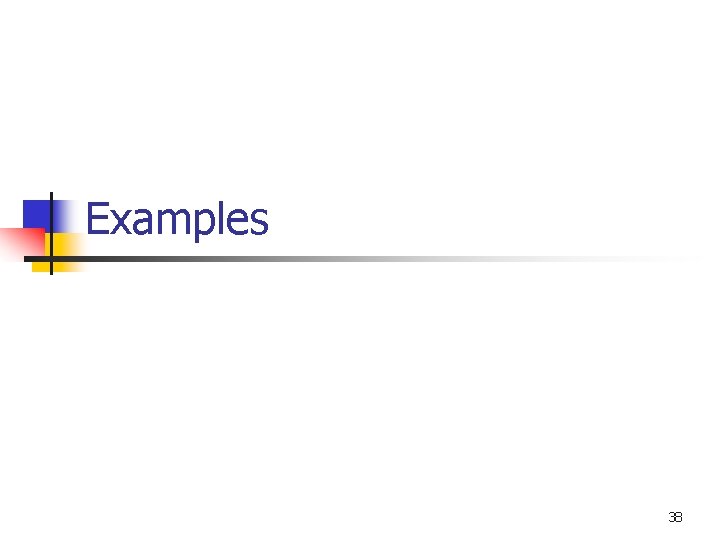
![Trace a program main() { int x[3] = {5, 2, 3}; int i; void Trace a program main() { int x[3] = {5, 2, 3}; int i; void](https://slidetodoc.com/presentation_image/2b5350ea66296ca00d8c6d3beea6c976/image-39.jpg)
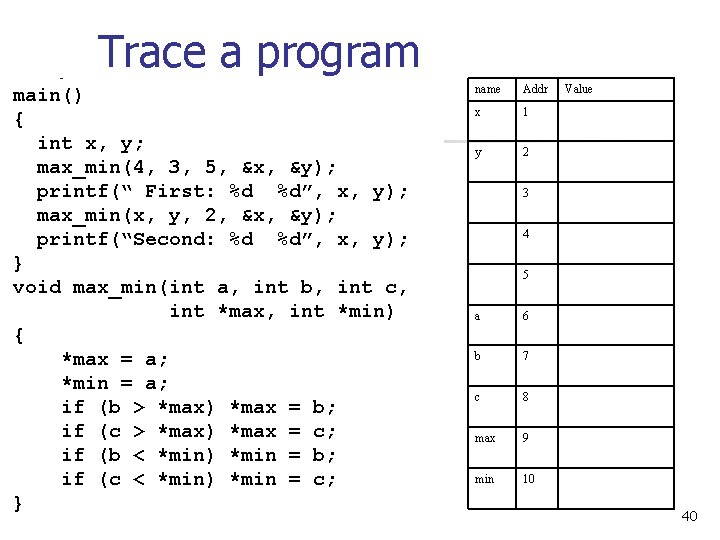
- Slides: 40
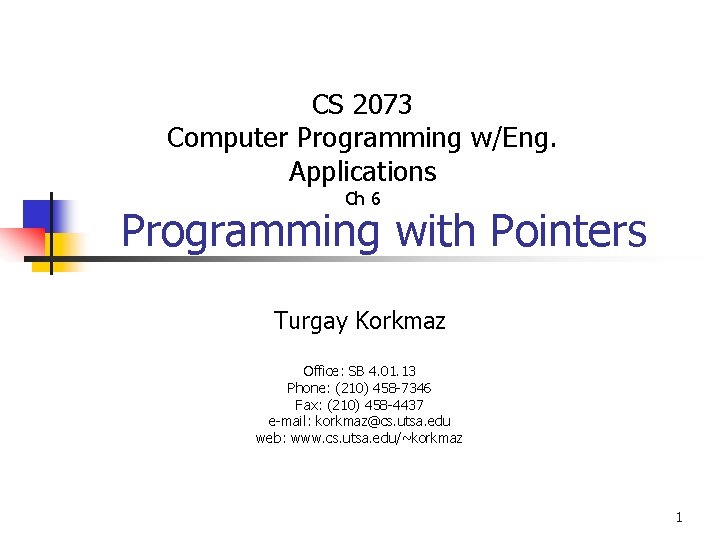
CS 2073 Computer Programming w/Eng. Applications Ch 6 Programming with Pointers Turgay Korkmaz Office: SB 4. 01. 13 Phone: (210) 458 -7346 Fax: (210) 458 -4437 e-mail: korkmaz@cs. utsa. edu web: www. cs. utsa. edu/~korkmaz 1
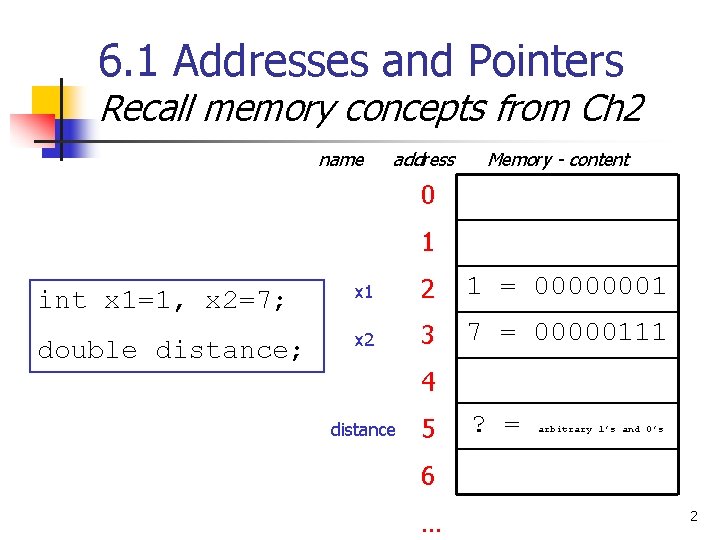
6. 1 Addresses and Pointers Recall memory concepts from Ch 2 name address Memory - content 0 1 int x 1=1, x 2=7; x 1 2 1 = 00000001 double distance; x 2 3 7 = 00000111 4 distance 5 ? = arbitrary 1’s and 0’s 6 … 2
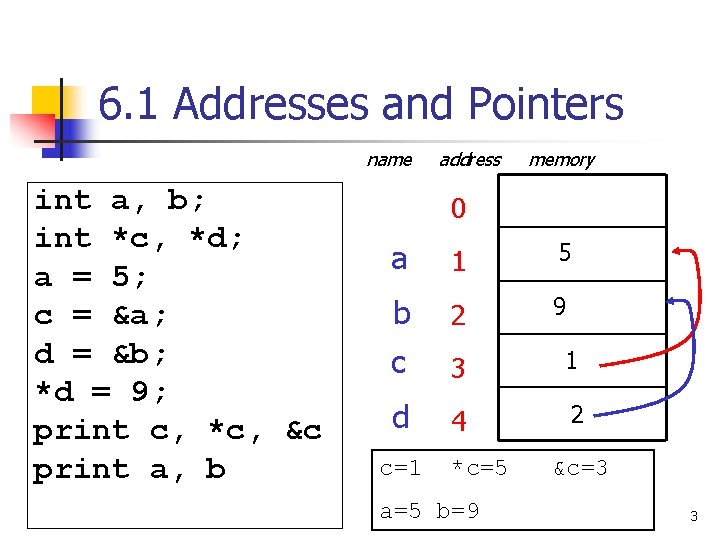
6. 1 Addresses and Pointers name int a, b; int *c, *d; a = 5; c = &a; d = &b; *d = 9; print c, *c, &c print a, b address memory 0 a 1 5? b 2 9? c 3 1? d 4 2? c=1 *c=5 a=5 b=9 &c=3 3
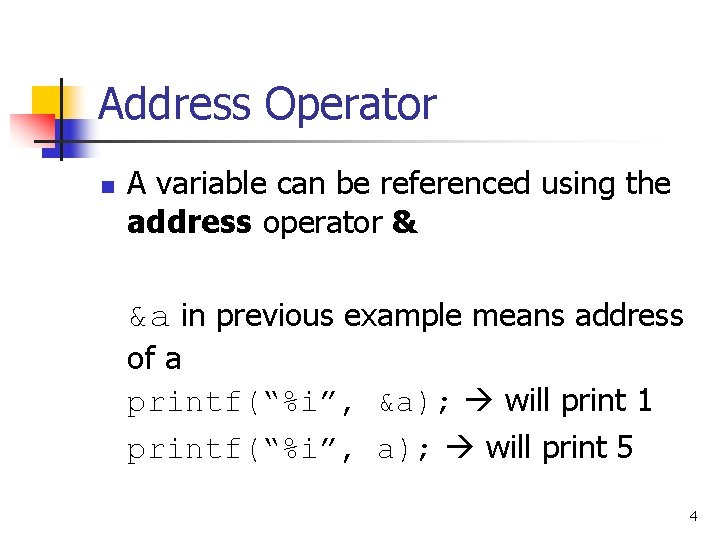
Address Operator n A variable can be referenced using the address operator & &a in previous example means address of a printf(“%i”, &a); will print 1 printf(“%i”, a); will print 5 4
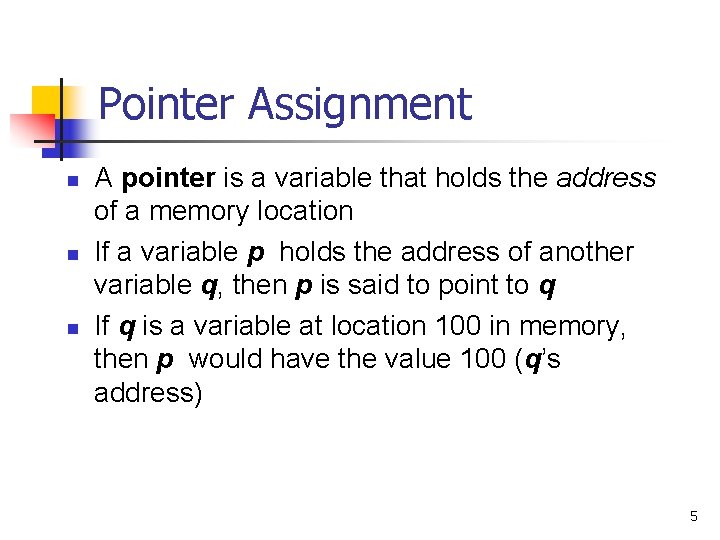
Pointer Assignment n n n A pointer is a variable that holds the address of a memory location If a variable p holds the address of another variable q, then p is said to point to q If q is a variable at location 100 in memory, then p would have the value 100 (q’s address) 5
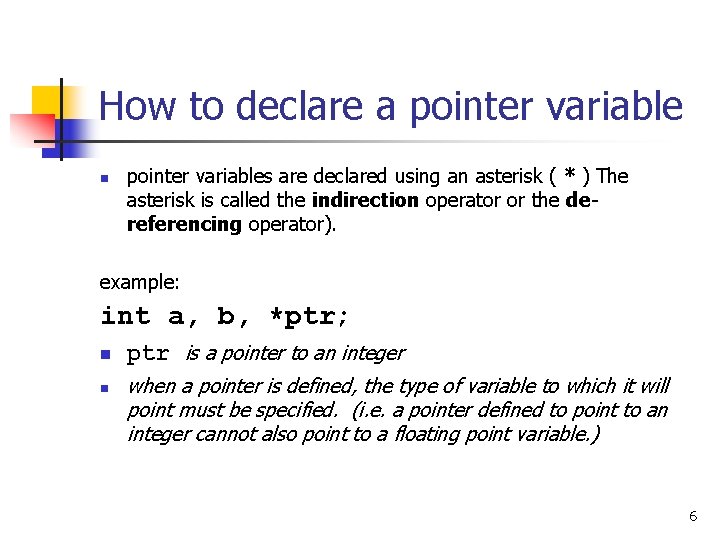
How to declare a pointer variable n pointer variables are declared using an asterisk ( * ) The asterisk is called the indirection operator or the dereferencing operator). example: int a, b, *ptr; n n ptr is a pointer to an integer when a pointer is defined, the type of variable to which it will point must be specified. (i. e. a pointer defined to point to an integer cannot also point to a floating point variable. ) 6
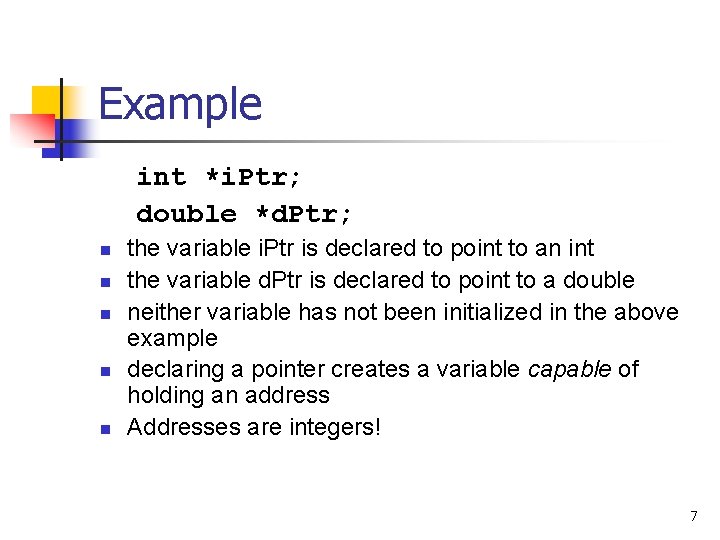
Example int *i. Ptr; double *d. Ptr; n n n the variable i. Ptr is declared to point to an int the variable d. Ptr is declared to point to a double neither variable has not been initialized in the above example declaring a pointer creates a variable capable of holding an address Addresses are integers! 7
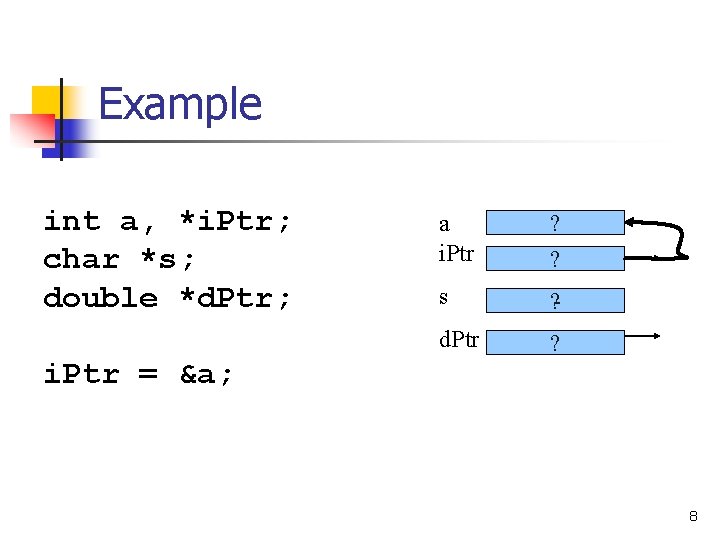
Example int a, *i. Ptr; char *s; double *d. Ptr; a i. Ptr ? ? s ? - d. Ptr ? i. Ptr = &a; 8
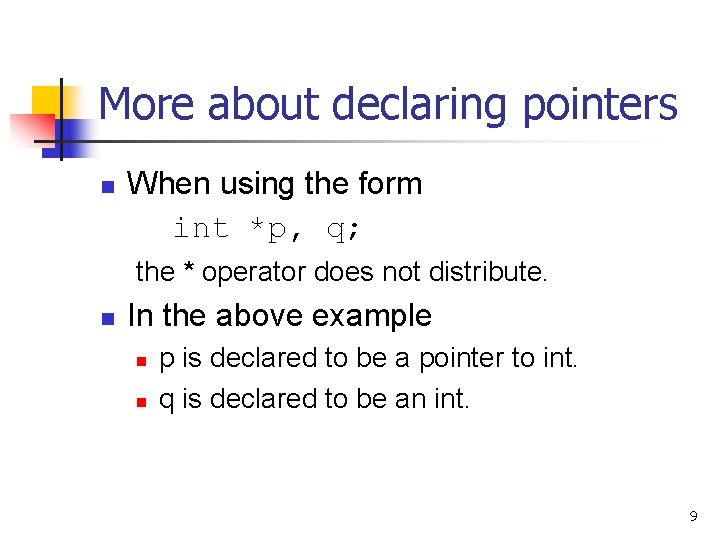
More about declaring pointers n When using the form int *p, q; the * operator does not distribute. n In the above example n n p is declared to be a pointer to int. q is declared to be an int. 9
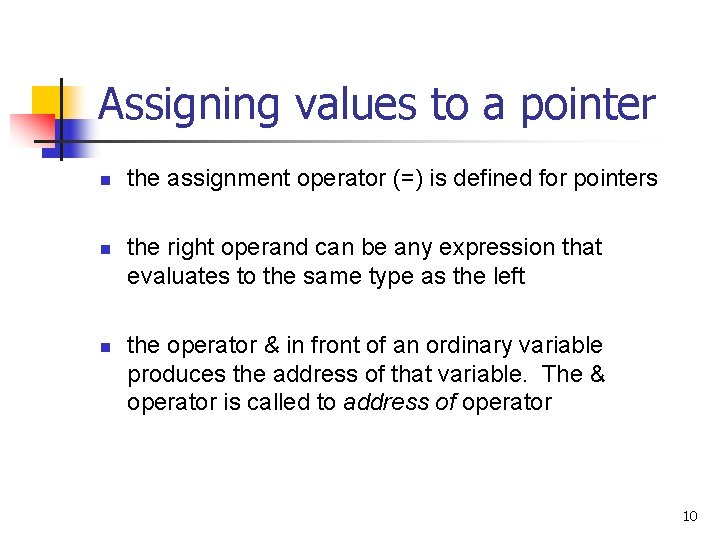
Assigning values to a pointer n n n the assignment operator (=) is defined for pointers the right operand can be any expression that evaluates to the same type as the left the operator & in front of an ordinary variable produces the address of that variable. The & operator is called to address of operator 10
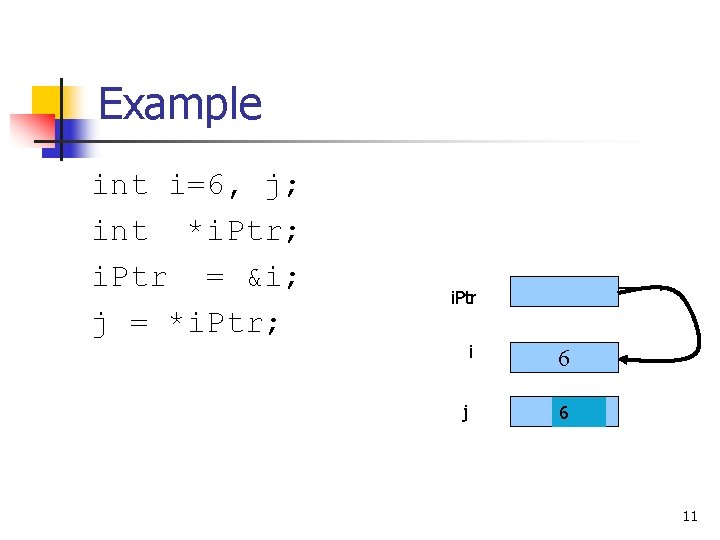
Example int i=6, j; int *i. Ptr; i. Ptr = &i; j = *i. Ptr; i. Ptr i j 6 ? 6 11
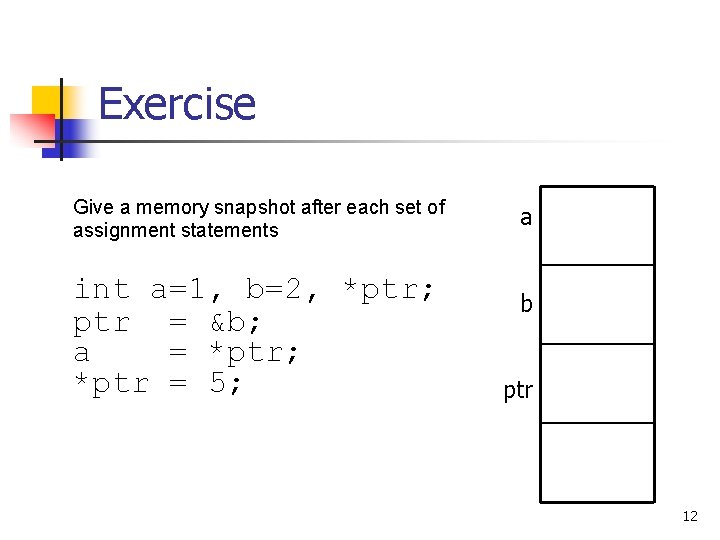
Exercise Give a memory snapshot after each set of assignment statements int a=1, b=2, *ptr; ptr = &b; a = *ptr; *ptr = 5; a b ptr 12
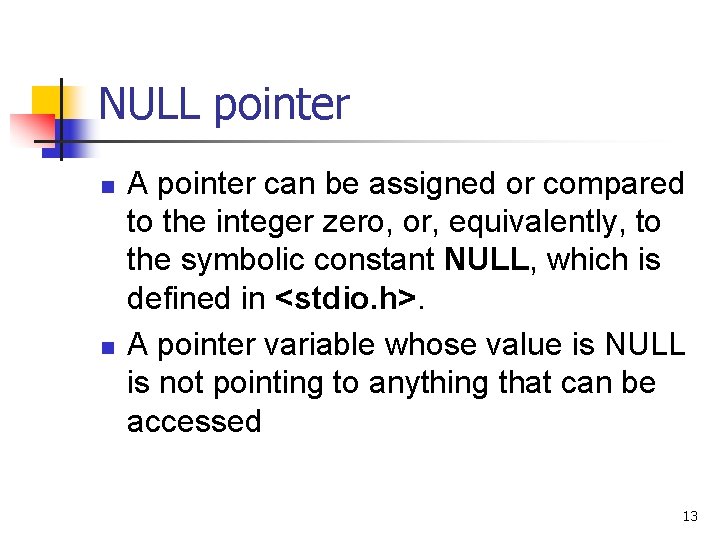
NULL pointer n n A pointer can be assigned or compared to the integer zero, or, equivalently, to the symbolic constant NULL, which is defined in <stdio. h>. A pointer variable whose value is NULL is not pointing to anything that can be accessed 13
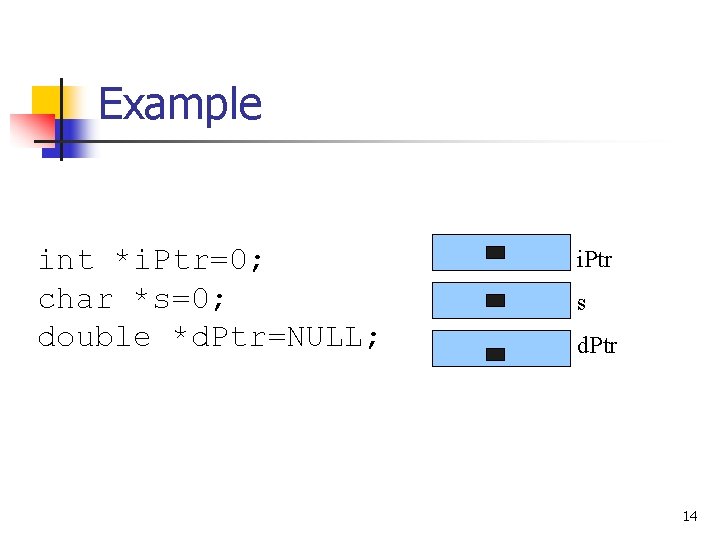
Example int *i. Ptr=0; char *s=0; double *d. Ptr=NULL; i. Ptr s d. Ptr 14
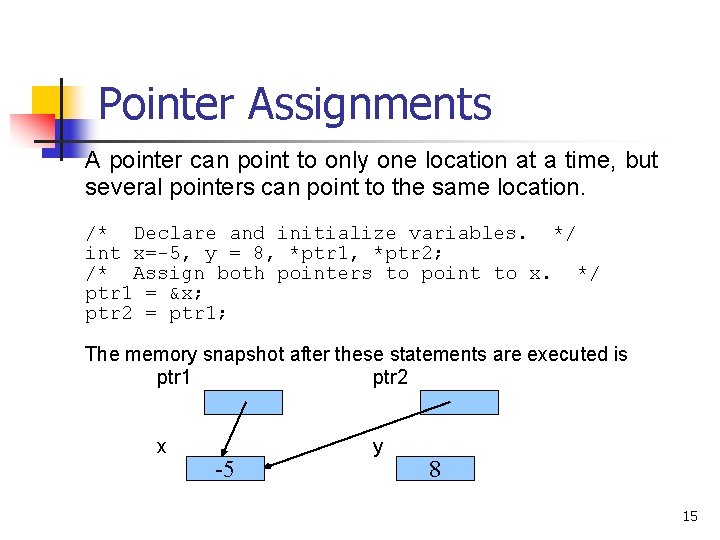
Pointer Assignments A pointer can point to only one location at a time, but several pointers can point to the same location. /* Declare and initialize variables. */ int x=-5, y = 8, *ptr 1, *ptr 2; /* Assign both pointers to point to x. */ ptr 1 = &x; ptr 2 = ptr 1; The memory snapshot after these statements are executed is ptr 1 ptr 2 x -5 y 8 15
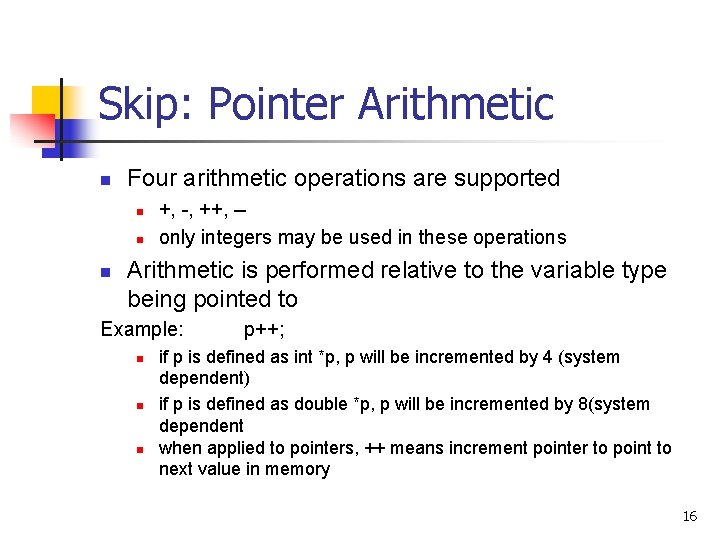
Skip: Pointer Arithmetic n Four arithmetic operations are supported n n n +, -, ++, -only integers may be used in these operations Arithmetic is performed relative to the variable type being pointed to Example: n n n p++; if p is defined as int *p, p will be incremented by 4 (system dependent) if p is defined as double *p, p will be incremented by 8(system dependent when applied to pointers, ++ means increment pointer to point to next value in memory 16
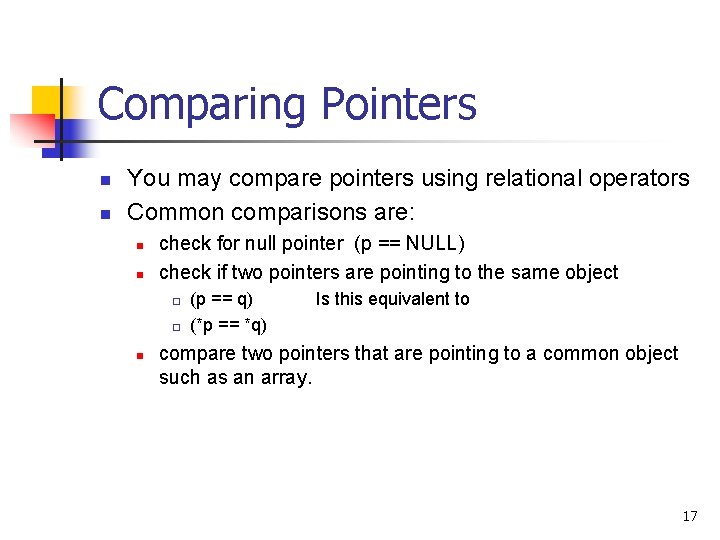
Comparing Pointers n n You may compare pointers using relational operators Common comparisons are: n n check for null pointer (p == NULL) check if two pointers are pointing to the same object � � n (p == q) (*p == *q) Is this equivalent to compare two pointers that are pointing to a common object such as an array. 17
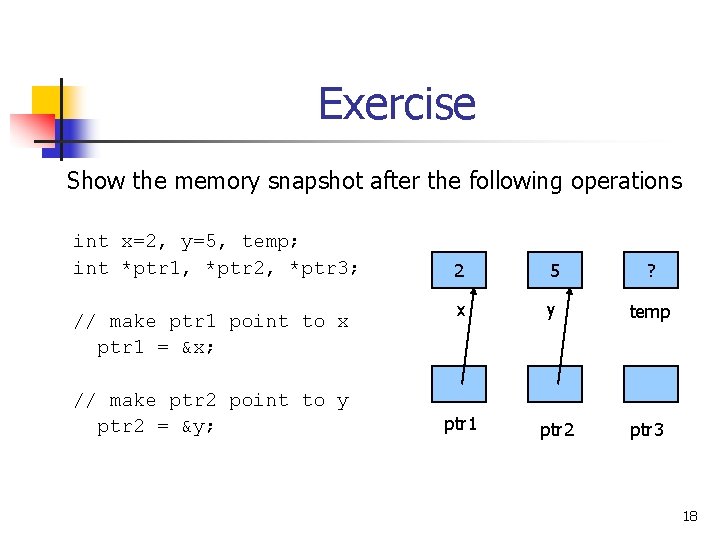
Exercise Show the memory snapshot after the following operations int x=2, y=5, temp; int *ptr 1, *ptr 2, *ptr 3; // make ptr 1 point to x ptr 1 = &x; // make ptr 2 point to y ptr 2 = &y; 2 5 ? x y temp ptr 1 ptr 2 ptr 3 18
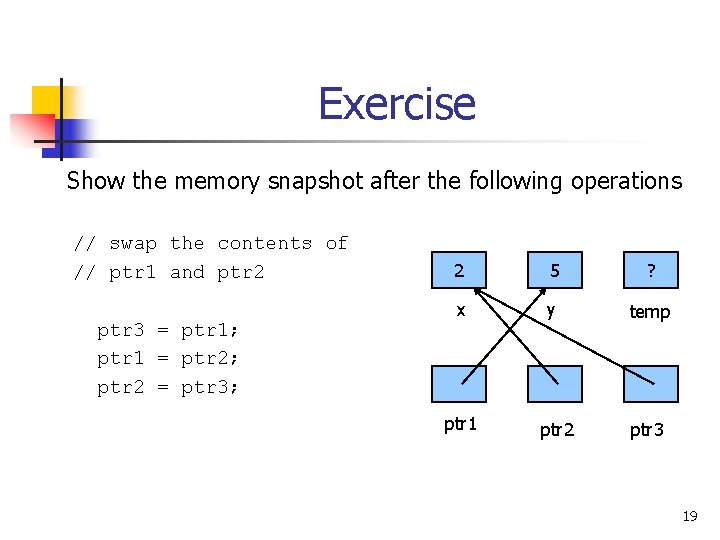
Exercise Show the memory snapshot after the following operations // swap the contents of // ptr 1 and ptr 2 ptr 3 = ptr 1; ptr 1 = ptr 2; ptr 2 = ptr 3; 2 5 ? x y temp ptr 1 ptr 2 ptr 3 19
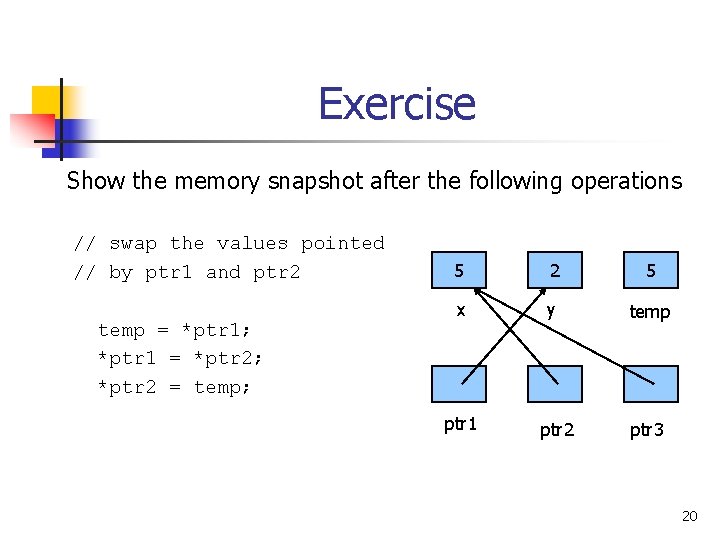
Exercise Show the memory snapshot after the following operations // swap the values pointed // by ptr 1 and ptr 2 temp = *ptr 1; *ptr 1 = *ptr 2; *ptr 2 = temp; 5 2 5 x y temp ptr 1 ptr 2 ptr 3 20
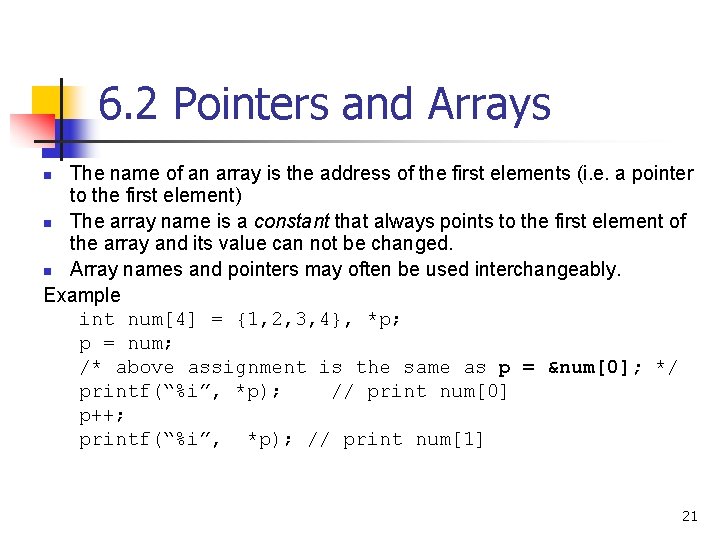
6. 2 Pointers and Arrays The name of an array is the address of the first elements (i. e. a pointer to the first element) n The array name is a constant that always points to the first element of the array and its value can not be changed. n Array names and pointers may often be used interchangeably. Example int num[4] = {1, 2, 3, 4}, *p; p = num; /* above assignment is the same as p = &num[0]; */ printf(“%i”, *p); // print num[0] p++; printf(“%i”, *p); // print num[1] n 21
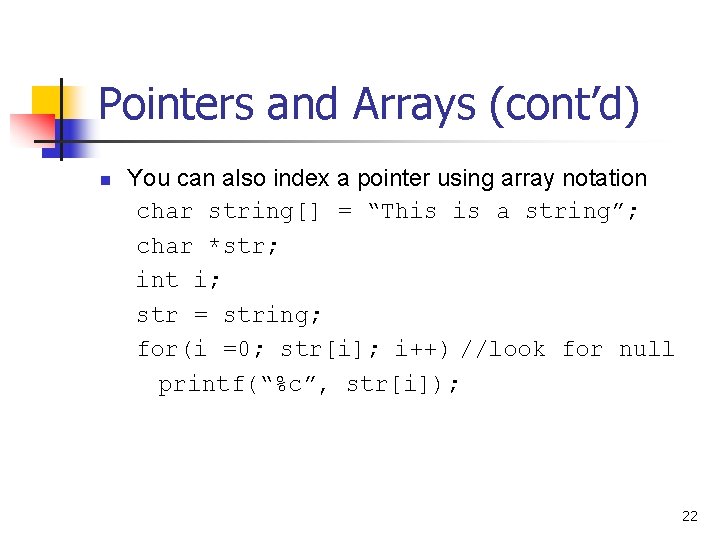
Pointers and Arrays (cont’d) n You can also index a pointer using array notation char string[] = “This is a string”; char *str; int i; str = string; for(i =0; str[i]; i++) //look for null printf(“%c”, str[i]); 22
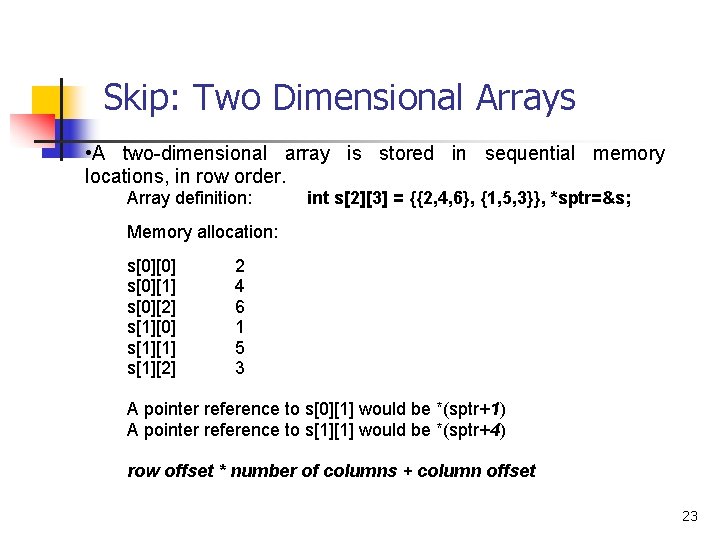
Skip: Two Dimensional Arrays • A two-dimensional array is stored in sequential memory locations, in row order. Array definition: int s[2][3] = {{2, 4, 6}, {1, 5, 3}}, *sptr=&s; Memory allocation: s[0][0] s[0][1] s[0][2] s[1][0] s[1][1] s[1][2] 2 4 6 1 5 3 A pointer reference to s[0][1] would be *(sptr+1) A pointer reference to s[1][1] would be *(sptr+4) row offset * number of columns + column offset 23
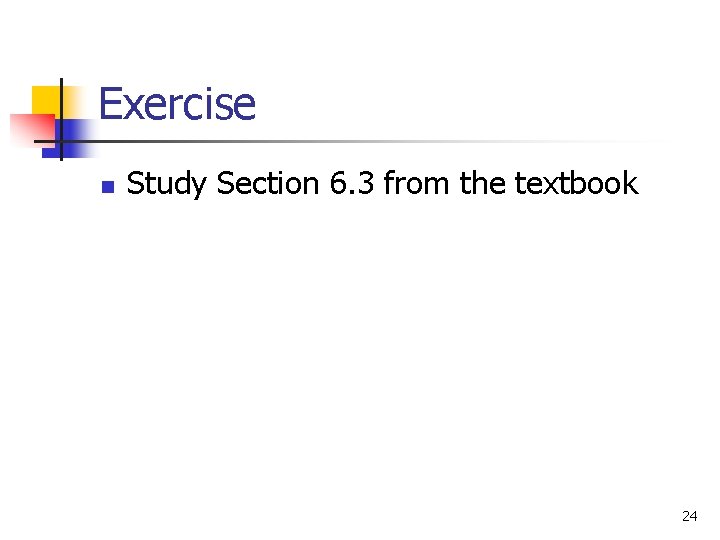
Exercise n Study Section 6. 3 from the textbook 24
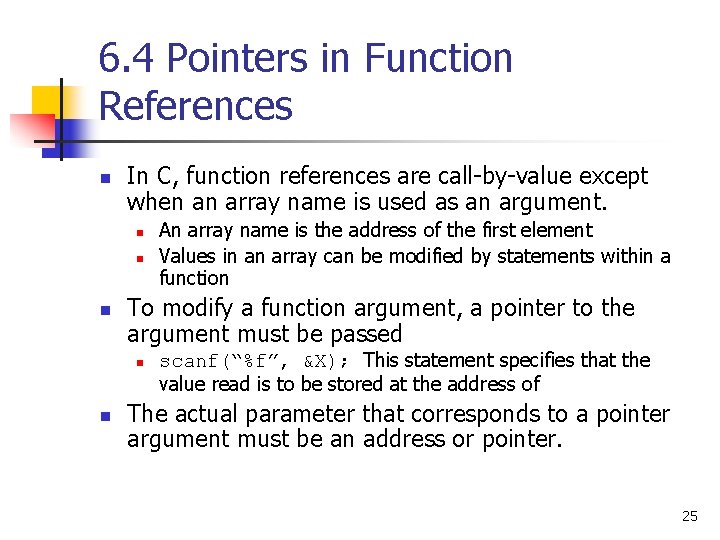
6. 4 Pointers in Function References n In C, function references are call-by-value except when an array name is used as an argument. n n n To modify a function argument, a pointer to the argument must be passed n n An array name is the address of the first element Values in an array can be modified by statements within a function scanf(“%f”, &X); This statement specifies that the value read is to be stored at the address of The actual parameter that corresponds to a pointer argument must be an address or pointer. 25
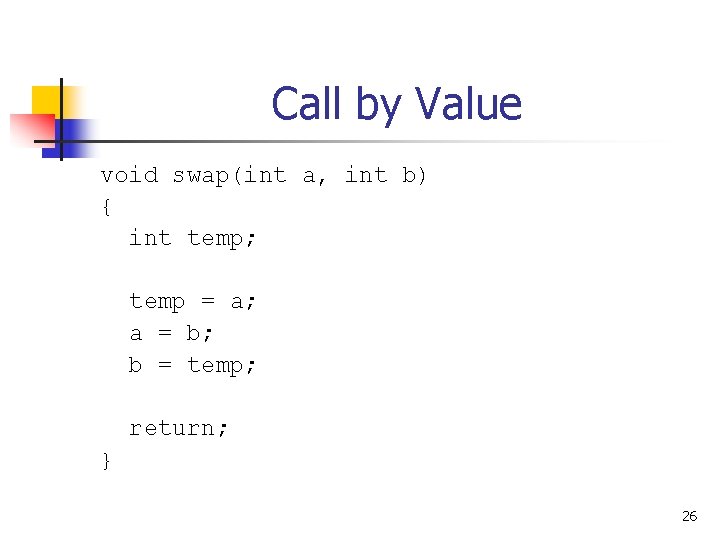
Call by Value void swap(int a, int b) { int temp; temp = a; a = b; b = temp; return; } 26
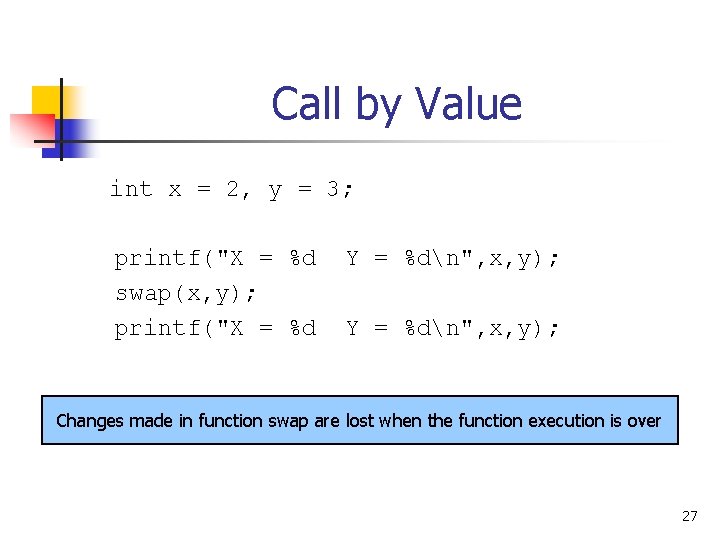
Call by Value int x = 2, y = 3; printf("X = %d swap(x, y); printf("X = %d Y = %dn", x, y); Changes made in function swap are lost when the function execution is over 27
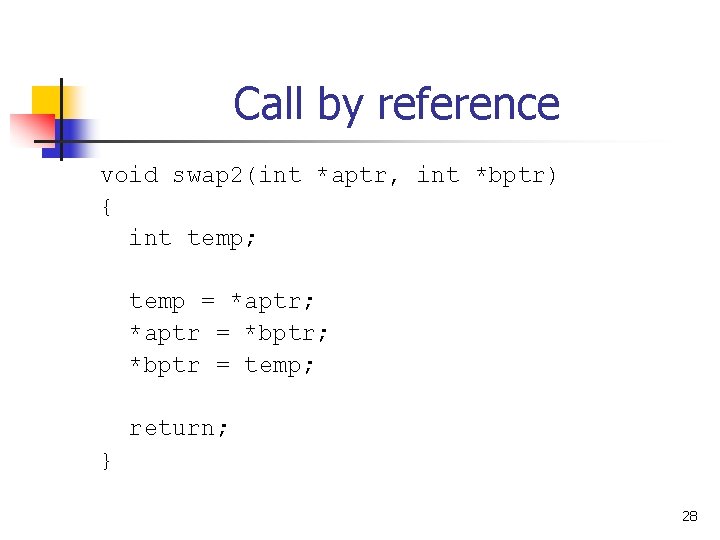
Call by reference void swap 2(int *aptr, int *bptr) { int temp; temp = *aptr; *aptr = *bptr; *bptr = temp; return; } 28
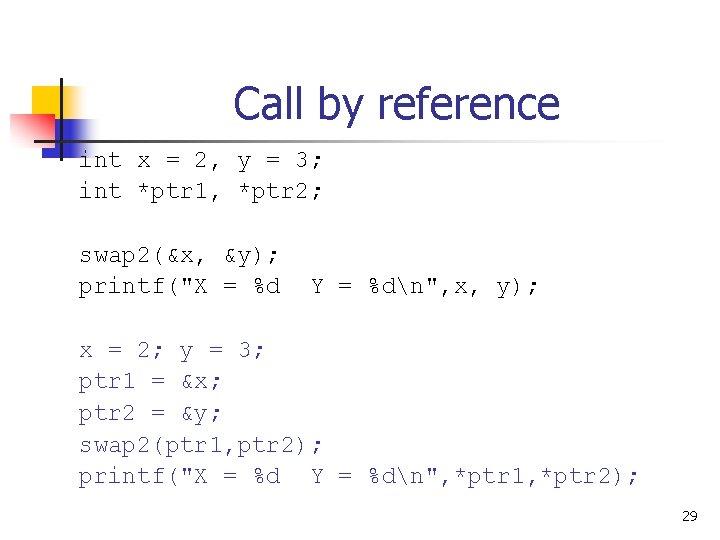
Call by reference int x = 2, y = 3; int *ptr 1, *ptr 2; swap 2(&x, &y); printf("X = %d Y = %dn", x, y); x = 2; y = 3; ptr 1 = &x; ptr 2 = &y; swap 2(ptr 1, ptr 2); printf("X = %d Y = %dn", *ptr 1, *ptr 2); 29
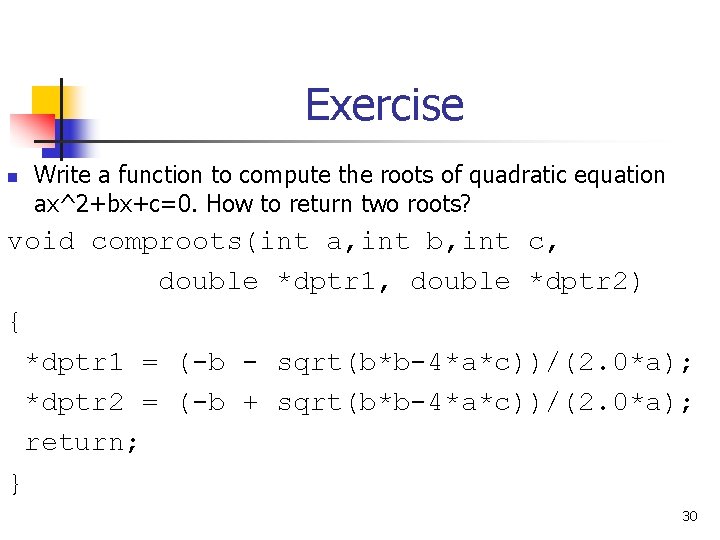
Exercise n Write a function to compute the roots of quadratic equation ax^2+bx+c=0. How to return two roots? void comproots(int a, int b, int c, double *dptr 1, double *dptr 2) { *dptr 1 = (-b - sqrt(b*b-4*a*c))/(2. 0*a); *dptr 2 = (-b + sqrt(b*b-4*a*c))/(2. 0*a); return; } 30
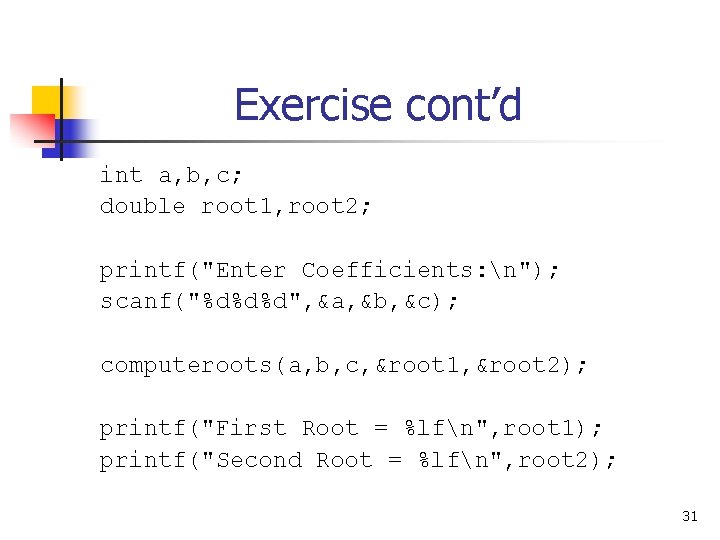
Exercise cont’d int a, b, c; double root 1, root 2; printf("Enter Coefficients: n"); scanf("%d%d%d", &a, &b, &c); computeroots(a, b, c, &root 1, &root 2); printf("First Root = %lfn", root 1); printf("Second Root = %lfn", root 2); 31
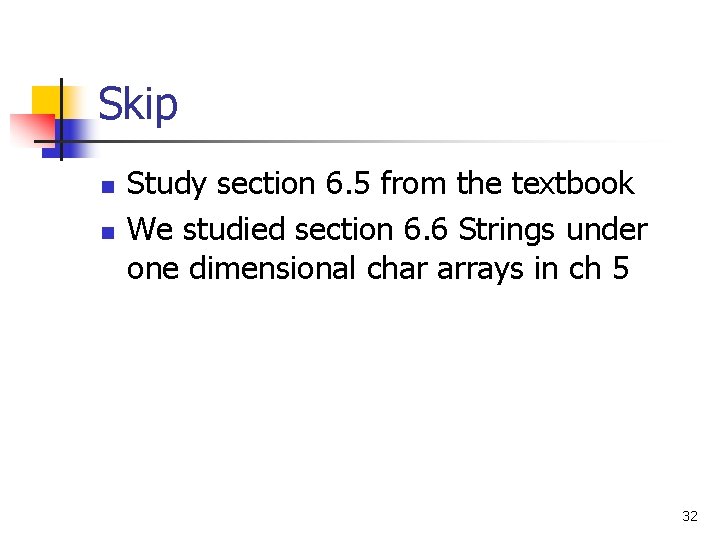
Skip n n Study section 6. 5 from the textbook We studied section 6. 6 Strings under one dimensional char arrays in ch 5 32
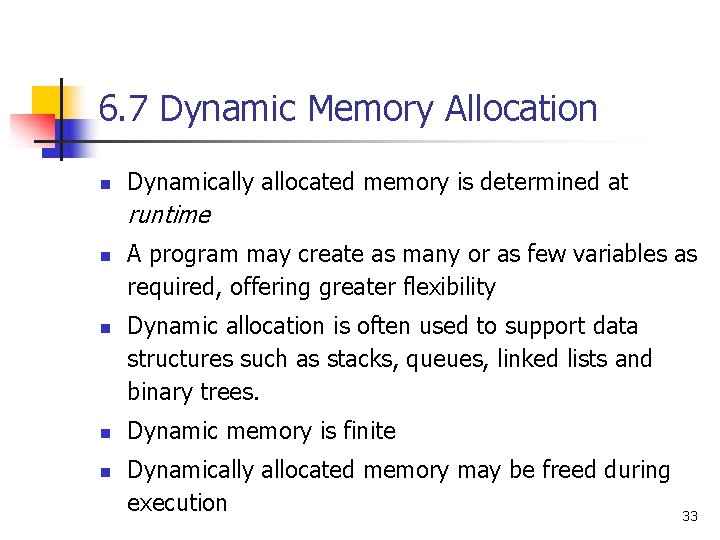
6. 7 Dynamic Memory Allocation n Dynamically allocated memory is determined at runtime n n A program may create as many or as few variables as required, offering greater flexibility Dynamic allocation is often used to support data structures such as stacks, queues, linked lists and binary trees. Dynamic memory is finite Dynamically allocated memory may be freed during execution 33
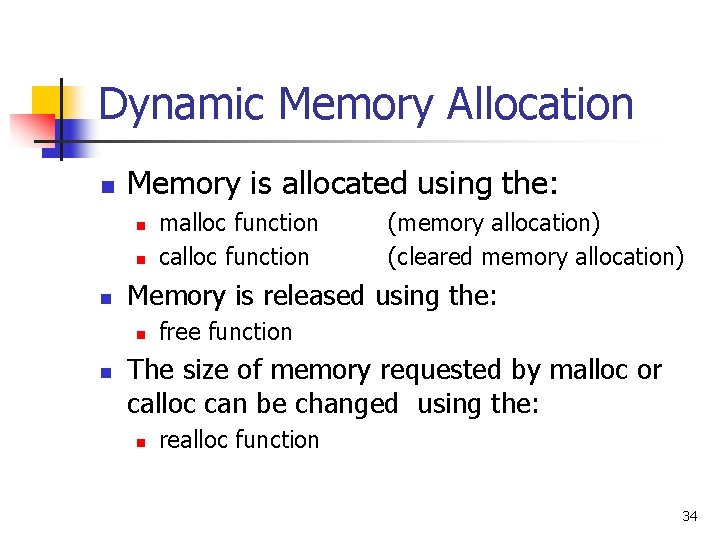
Dynamic Memory Allocation n Memory is allocated using the: n n n (memory allocation) (cleared memory allocation) Memory is released using the: n n malloc function calloc function free function The size of memory requested by malloc or calloc can be changed using the: n realloc function 34
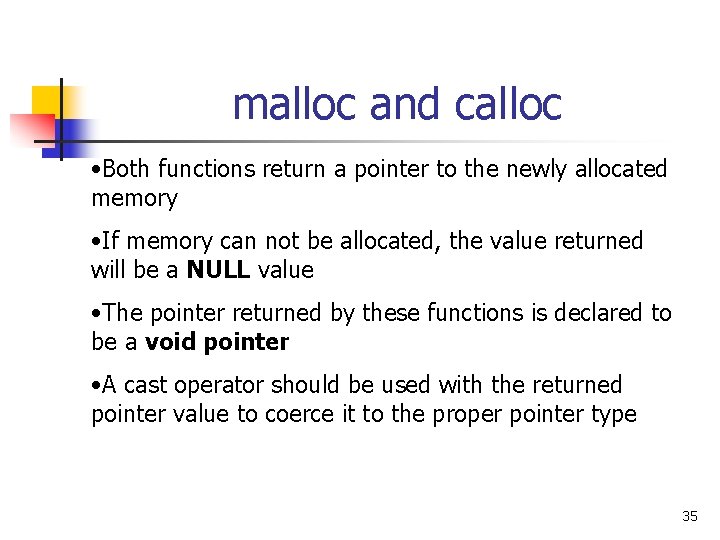
malloc and calloc • Both functions return a pointer to the newly allocated memory • If memory can not be allocated, the value returned will be a NULL value • The pointer returned by these functions is declared to be a void pointer • A cast operator should be used with the returned pointer value to coerce it to the proper pointer type 35
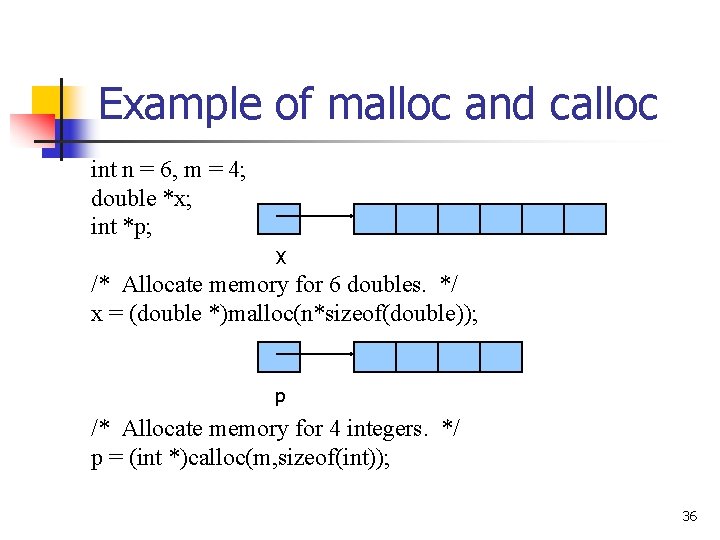
Example of malloc and calloc int n = 6, m = 4; double *x; int *p; X /* Allocate memory for 6 doubles. */ x = (double *)malloc(n*sizeof(double)); p /* Allocate memory for 4 integers. */ p = (int *)calloc(m, sizeof(int)); 36
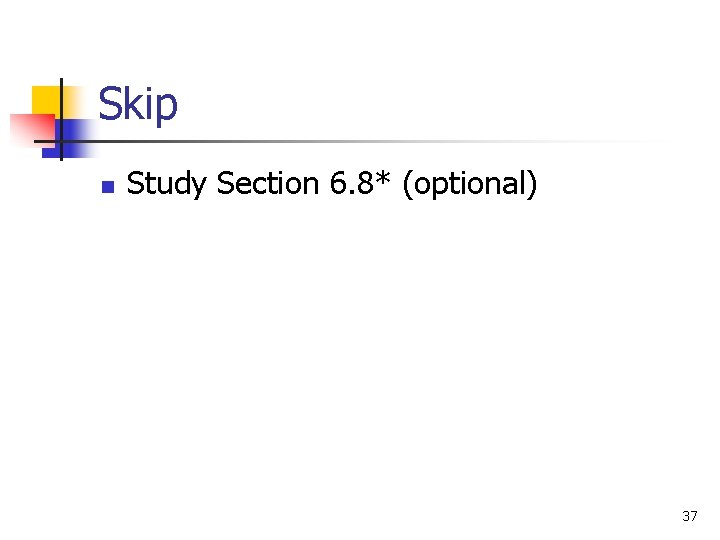
Skip n Study Section 6. 8* (optional) 37
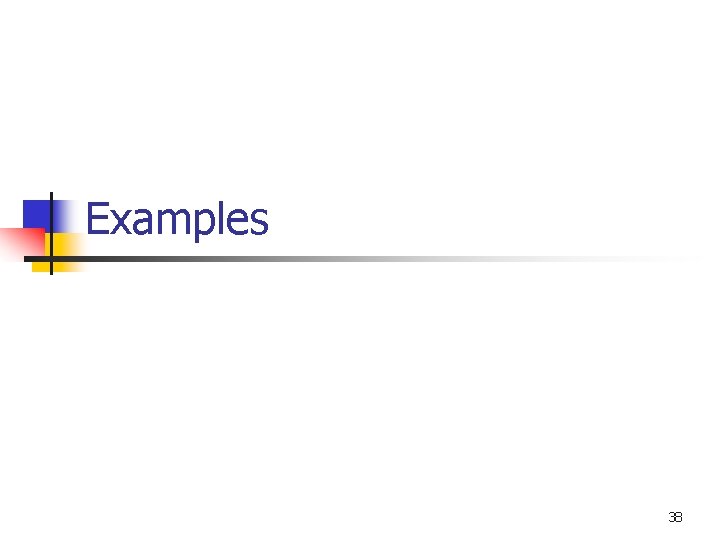
Examples 38
![Trace a program main int x3 5 2 3 int i void Trace a program main() { int x[3] = {5, 2, 3}; int i; void](https://slidetodoc.com/presentation_image/2b5350ea66296ca00d8c6d3beea6c976/image-39.jpg)
Trace a program main() { int x[3] = {5, 2, 3}; int i; void swap(int *a, int *b); for(i=0; i < 2; i++){ swap(&x[i], &x[i+1]); } for(i=0; i < 3; i++){ printf(“%d n”, x[i]); } } void swap(int *a, int *b) { int tmp; tmp = *a; *a = *b; *b = tmp; return; } name Addr x[0] 1 x[1] 2 x[2] 3 i 4 Value 5 6 a 7 b 8 tmp 9 39
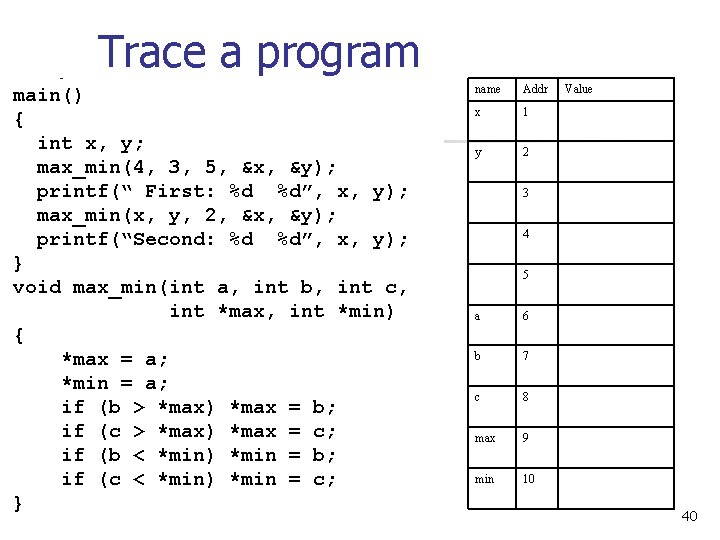
Trace a program main() { int x, y; max_min(4, 3, 5, &x, &y); printf(“ First: %d %d”, x, y); max_min(x, y, 2, &x, &y); printf(“Second: %d %d”, x, y); } void max_min(int a, int b, int c, int *max, int *min) { *max = a; *min = a; if (b > *max) *max = b; if (c > *max) *max = c; if (b < *min) *min = b; if (c < *min) *min = c; } name Addr x 1 y 2 Value 3 4 5 a 6 b 7 c 8 max 9 min 10 40
Zhiping weng
Weng-keen wong
Weng-keen wong
Lil weng
Chapter 8 linear programming applications solutions
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
Runtime programming
Linear vs integer programming
Definisi integer
Business application in computer network
A computer company receives 350 applications
Classification of supercomputer
Principles of network applications
Objectives of computer applications
Computer integrated manufacturing applications
Elements of computer
Computer networks and internets with internet applications
Business application in computer network
Business application in computer network
Cstnet
Nocti computer programming practice test
Nano programming in computer architecture
Concepts techniques and models of computer programming
Language
Uil computer science programming problems
Types of variables in computer programming
Programming raster display system in computer graphics
Computerite
Computer programming chapter 1
Computer aided part programming
A computer programming team has 13 members
Computer programming chapter 1
History of python
Computer programming with matlab
Linear programming models: graphical and computer methods
Decision making in computer programming
Computer organization
Fundamentals of computer programming syllabus
Python programming an introduction to computer science
3 components of computer system