CPS 506 Comparative Programming Languages Imperative Programming Language
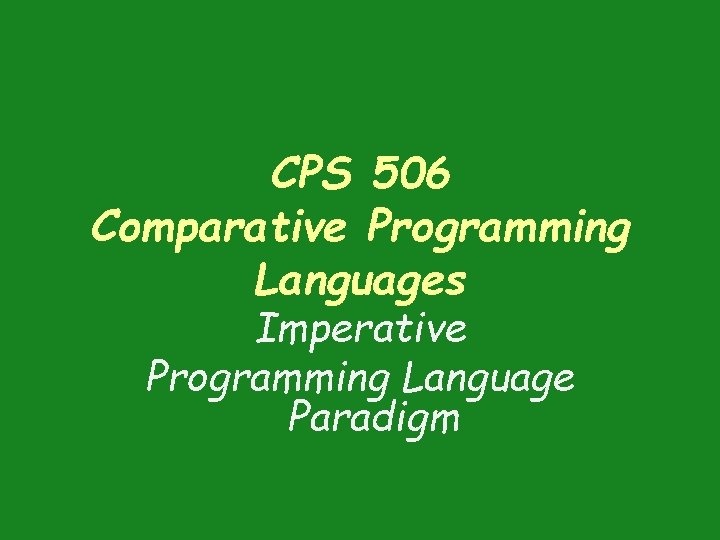
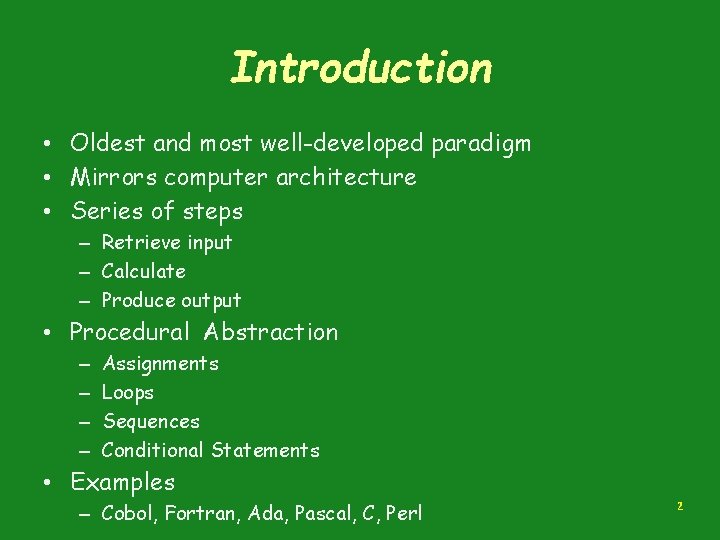
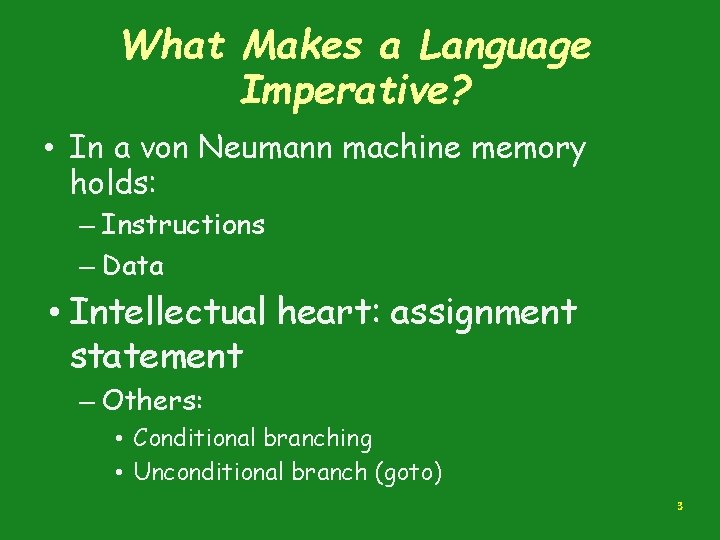
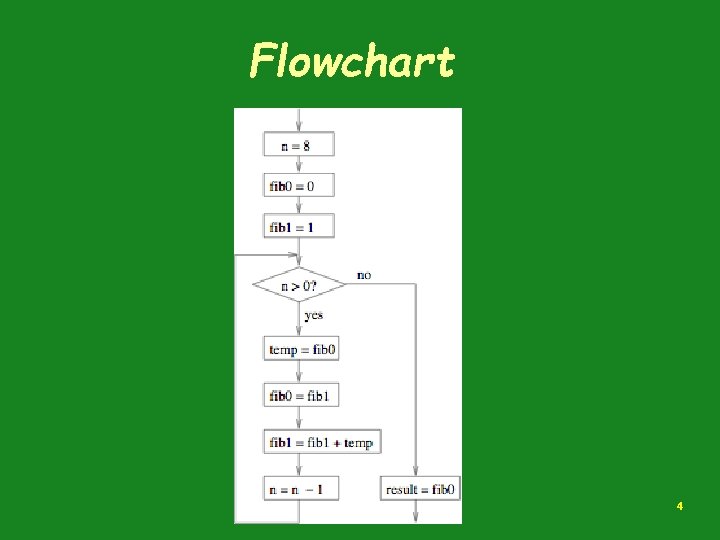
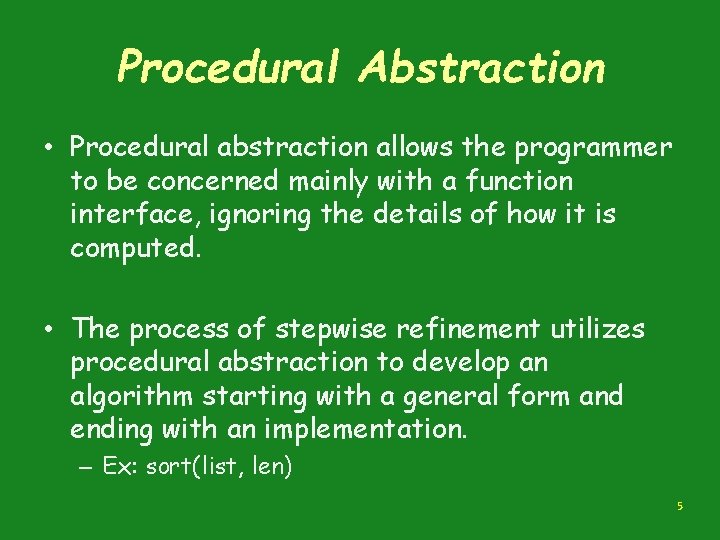
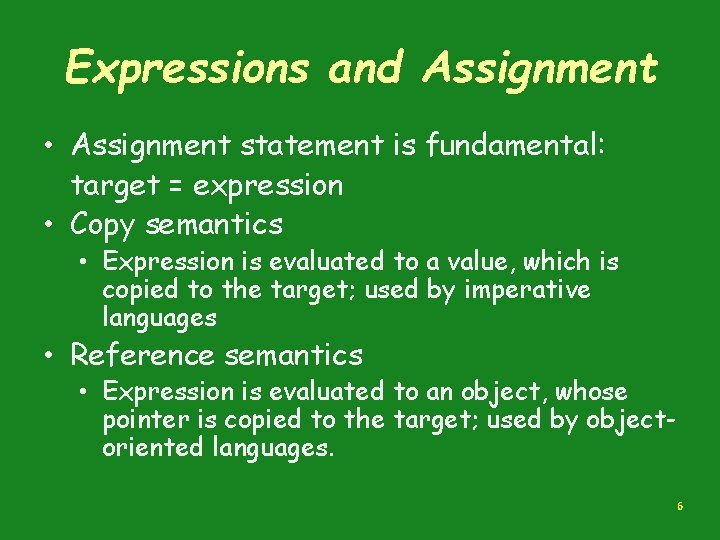
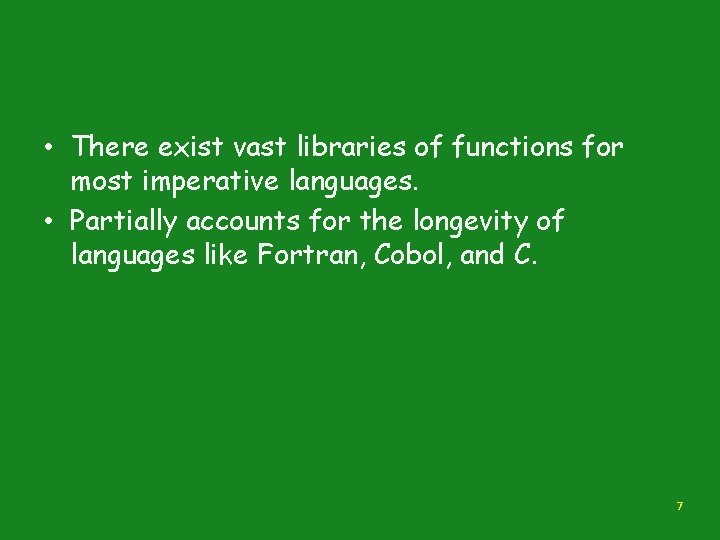
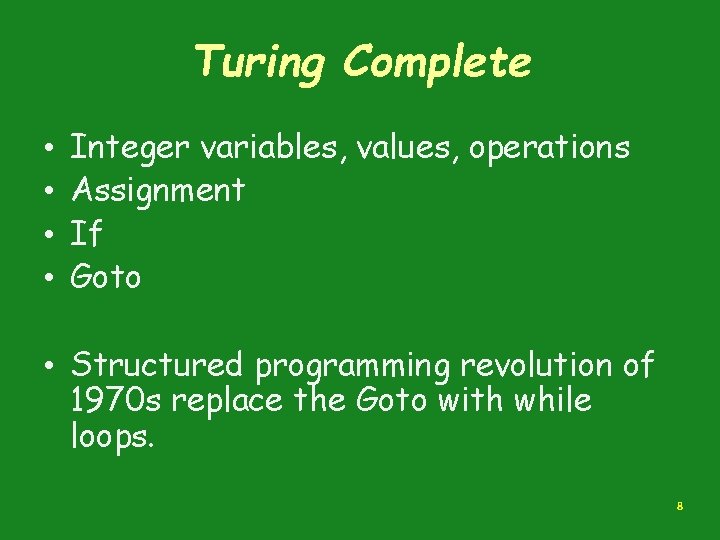
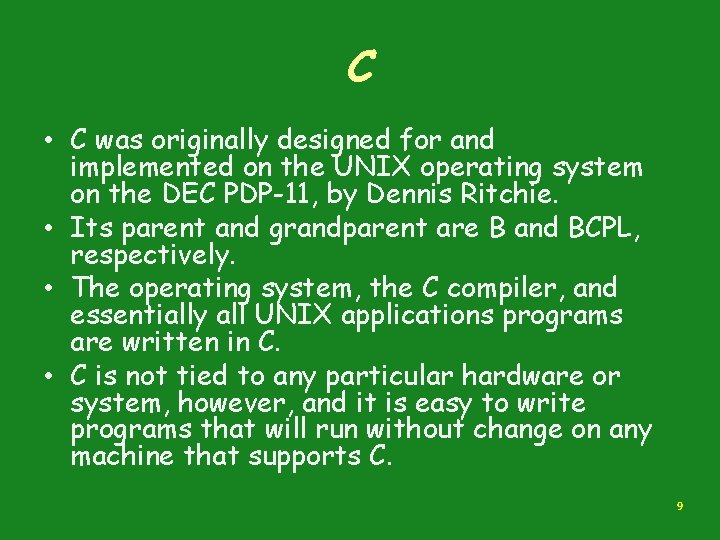
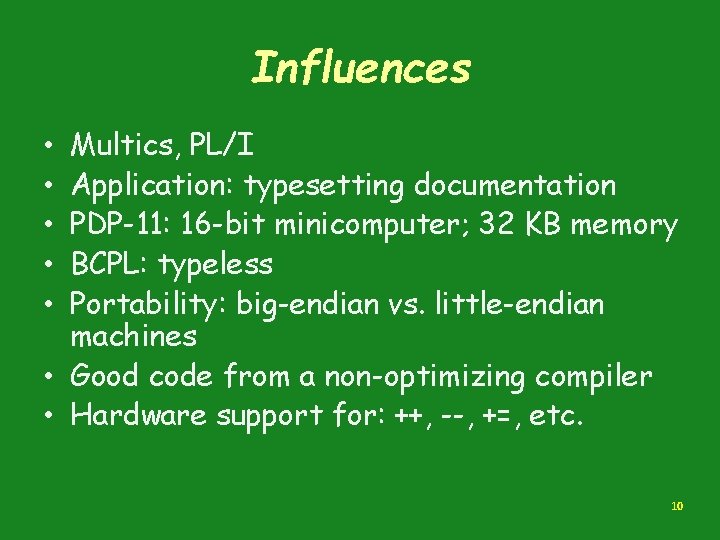
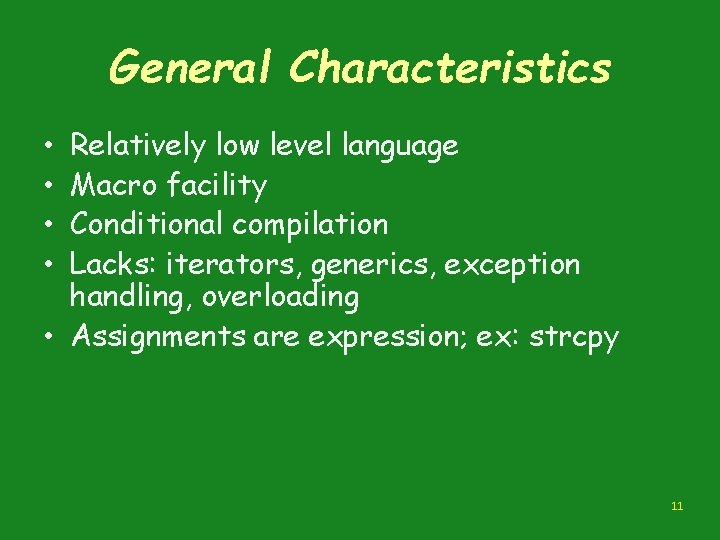
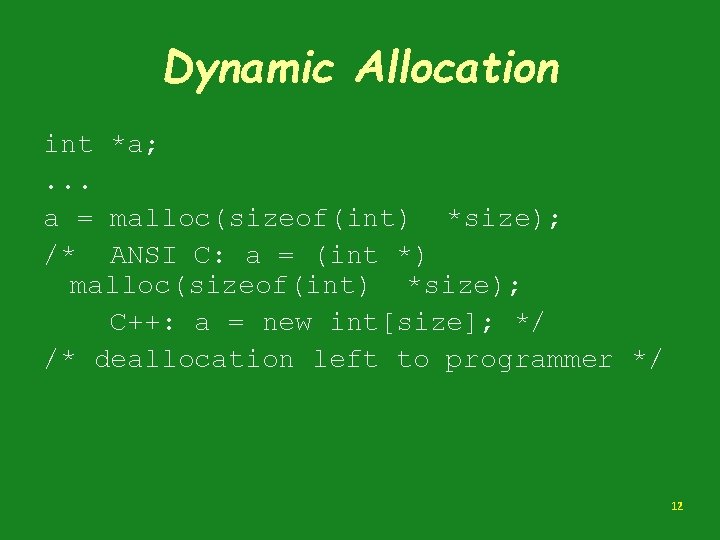
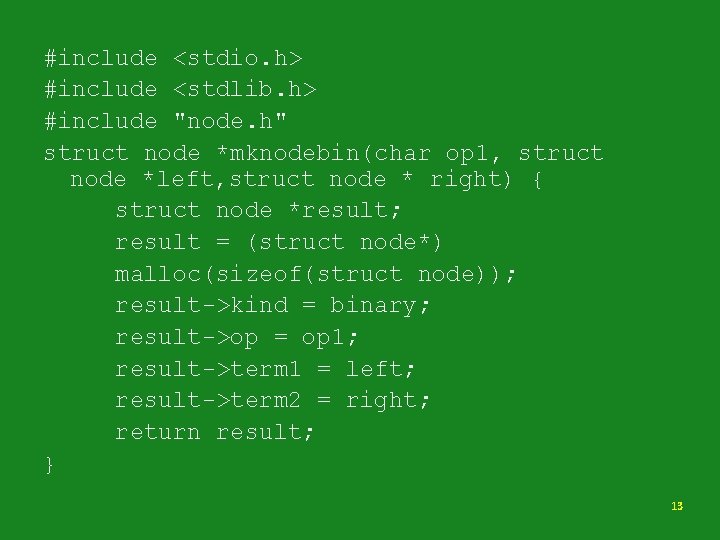
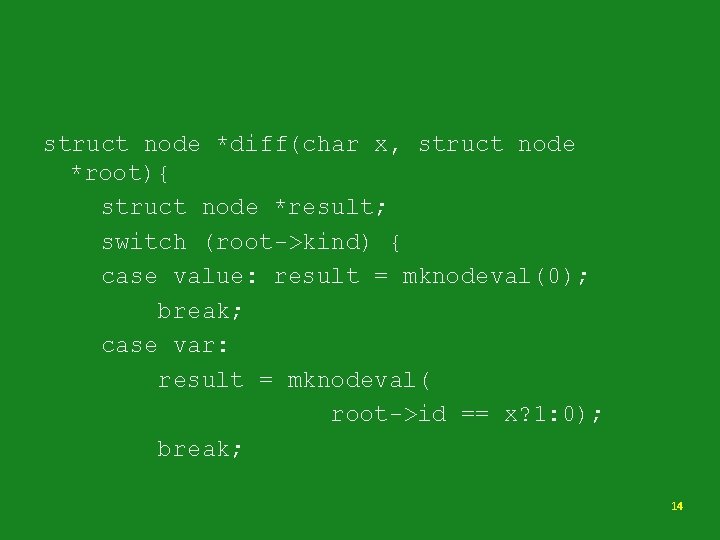
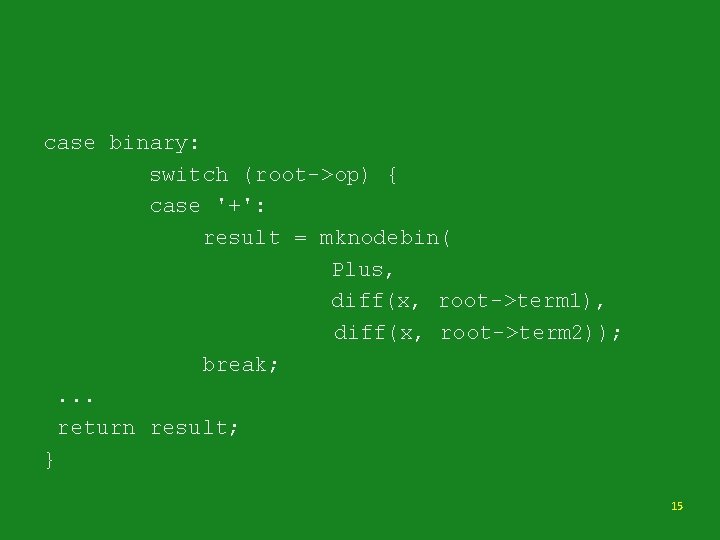
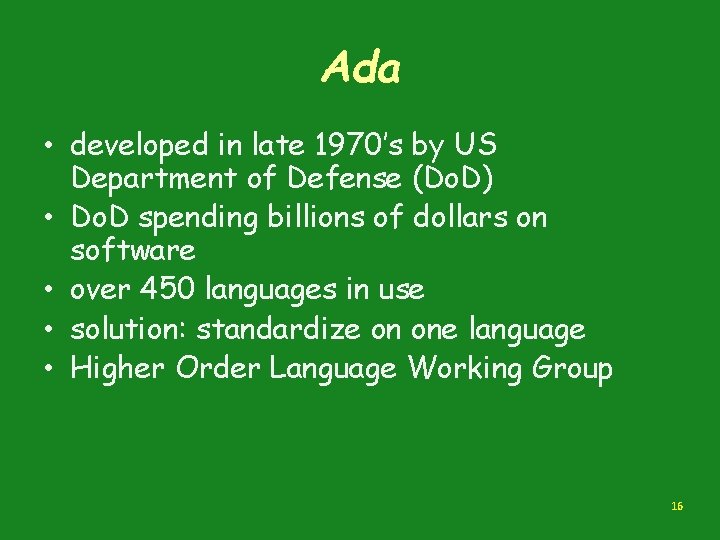
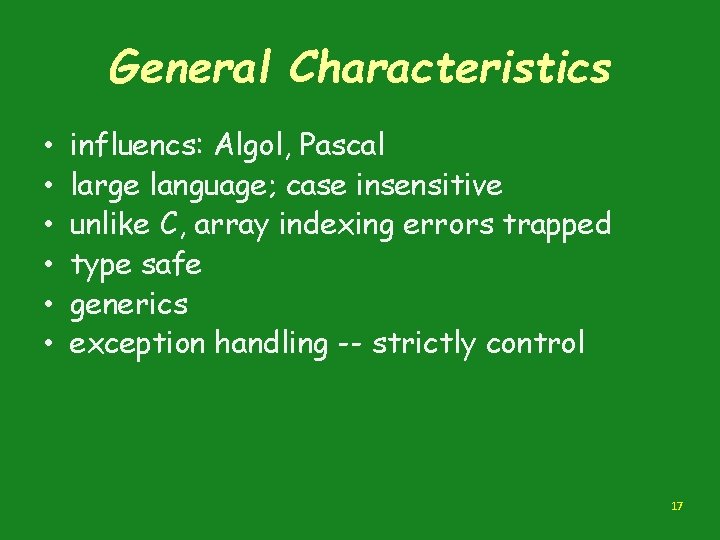
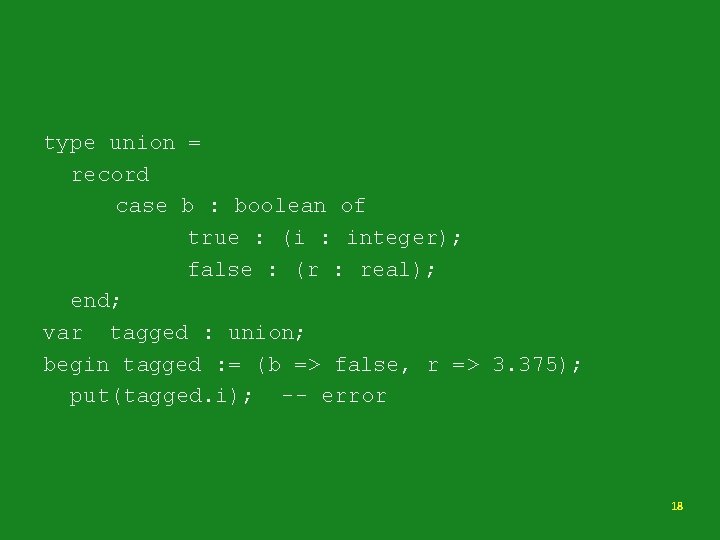
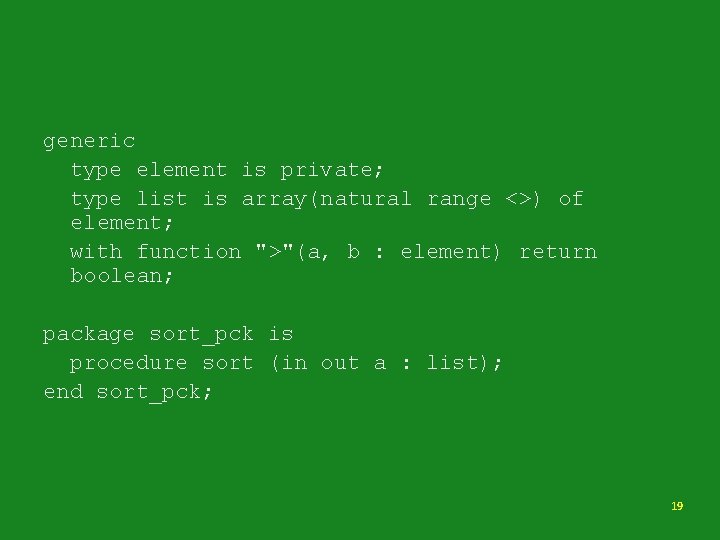
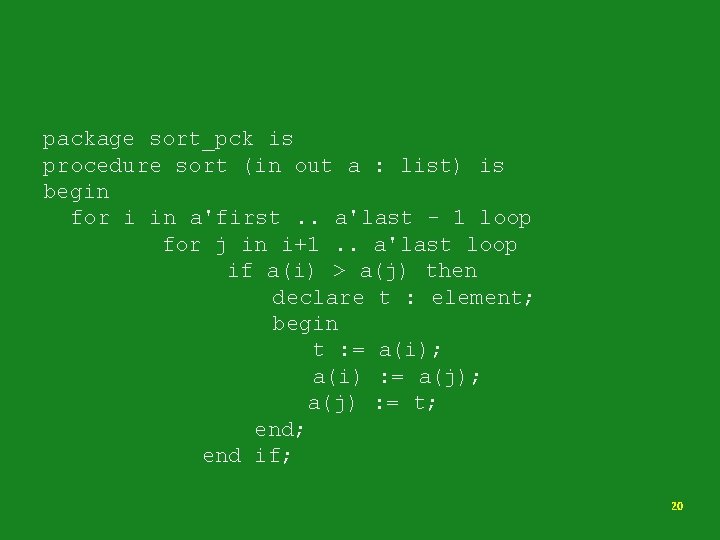
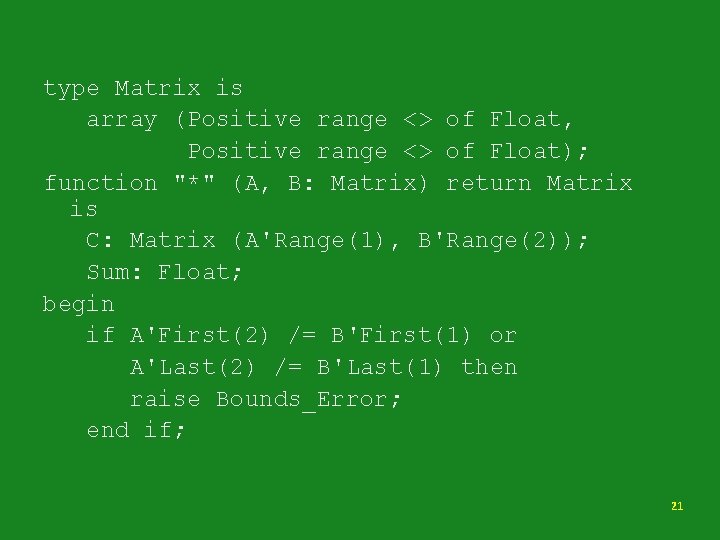
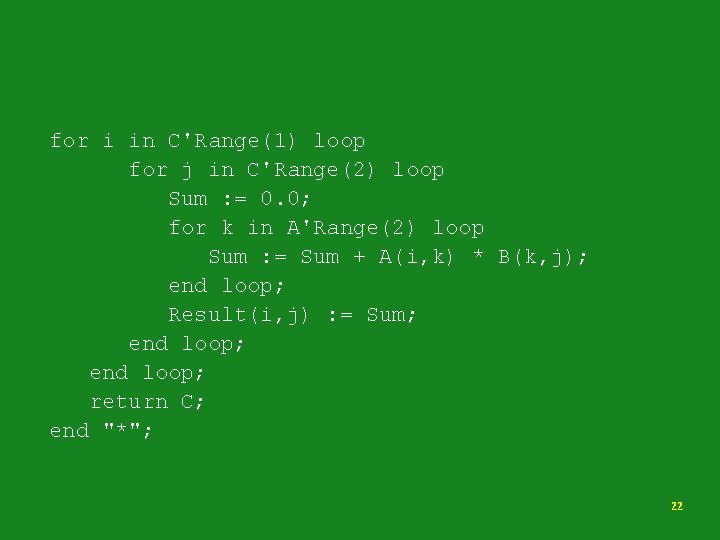
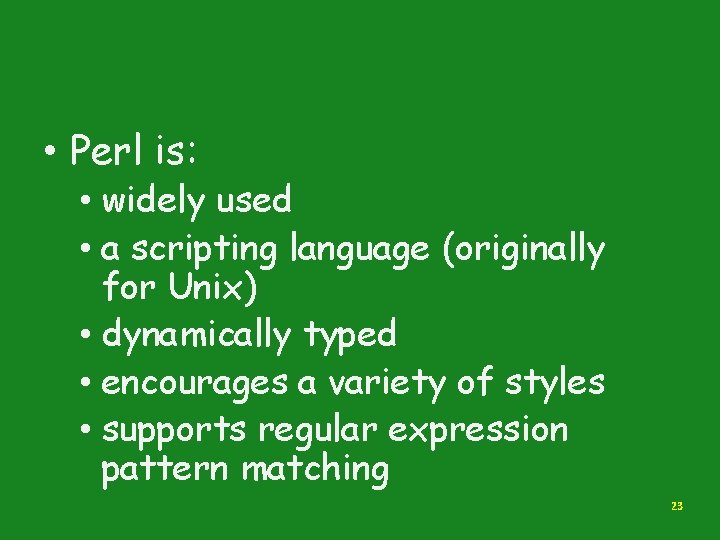
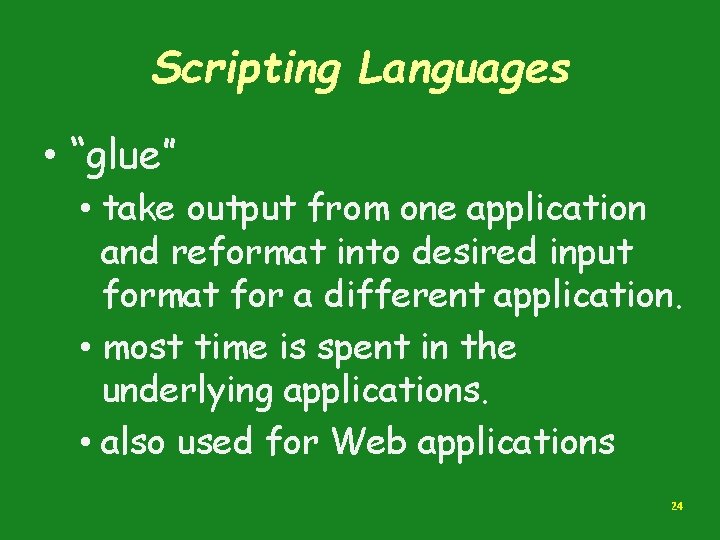
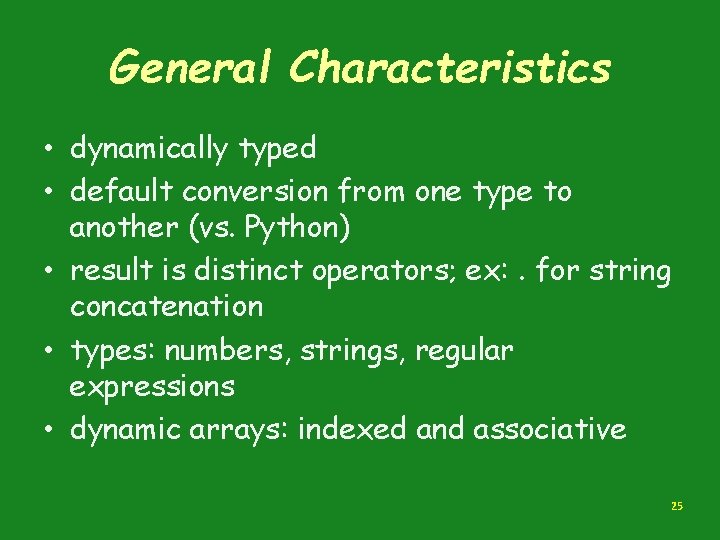
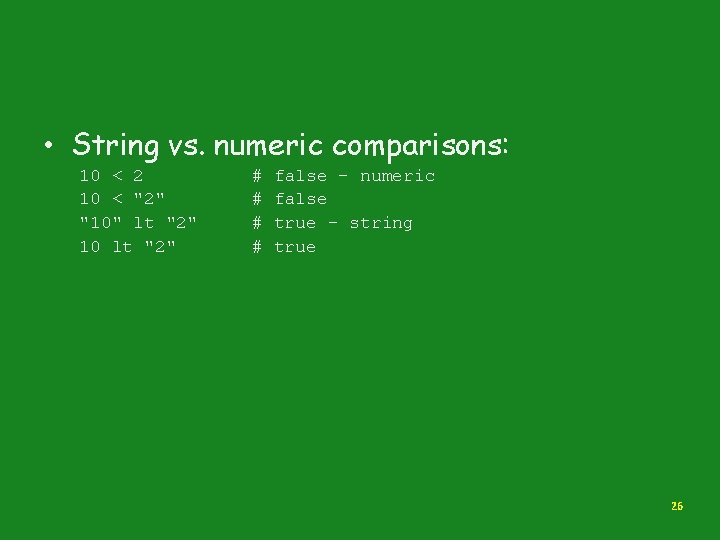
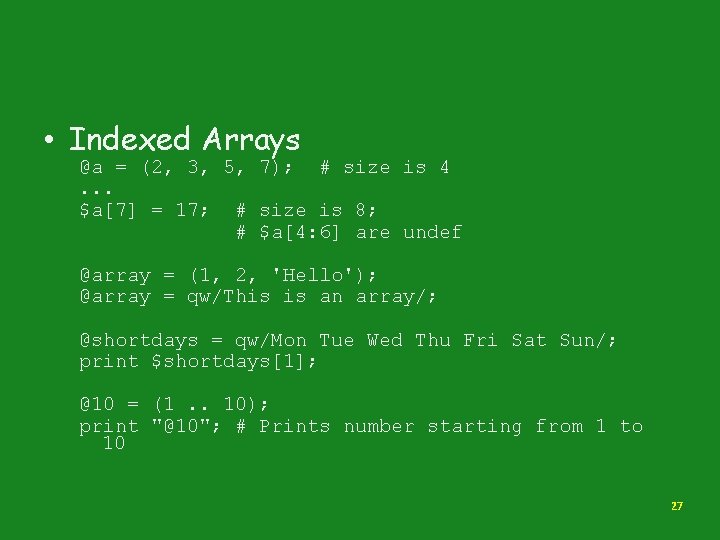
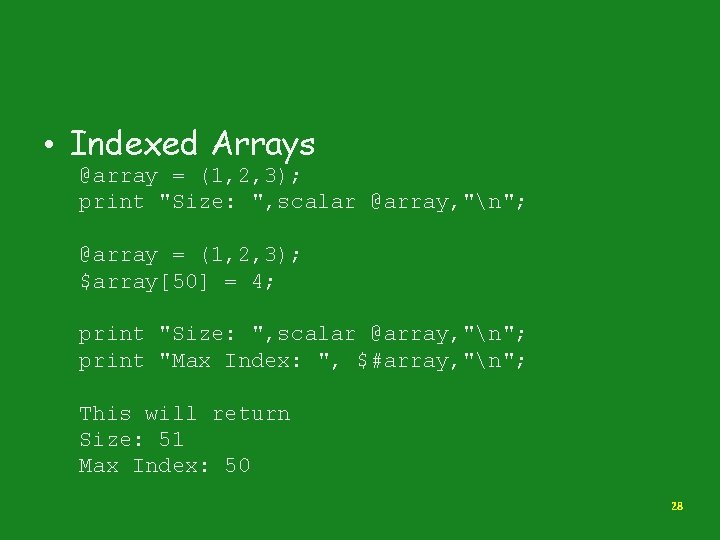
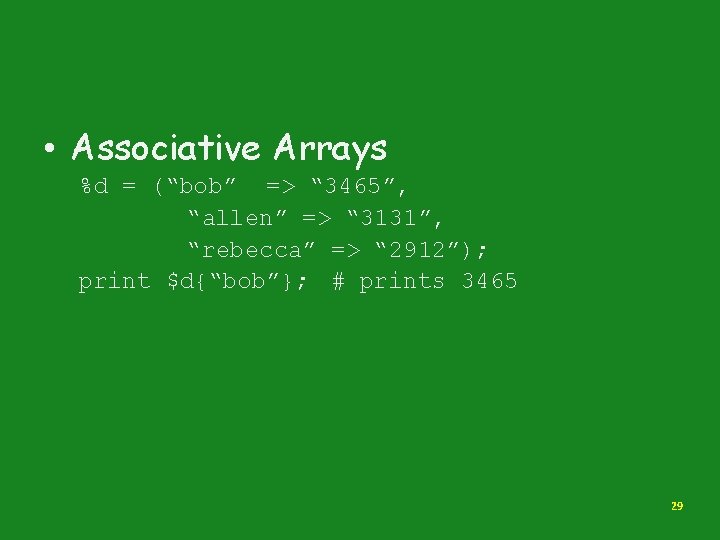
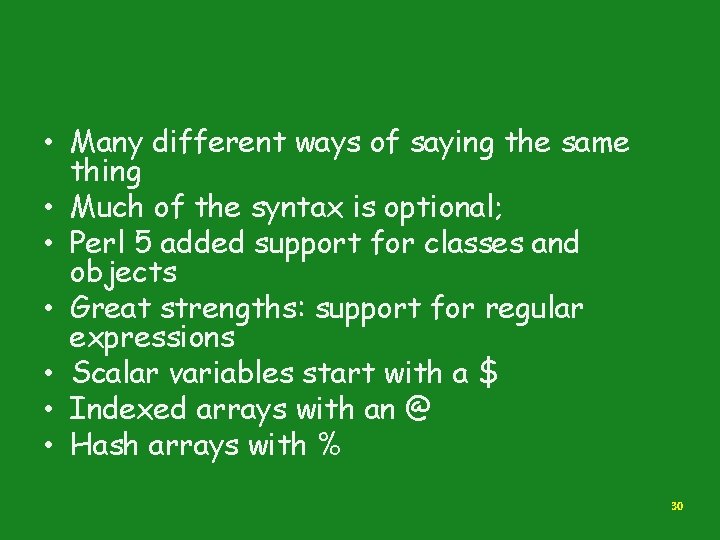
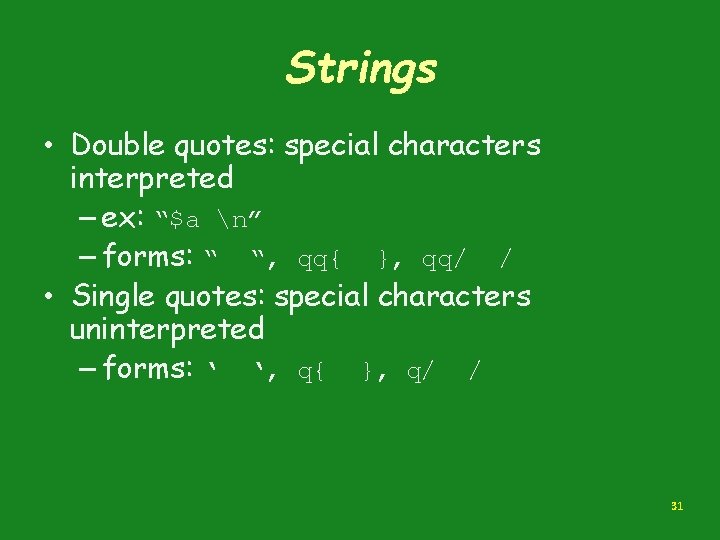
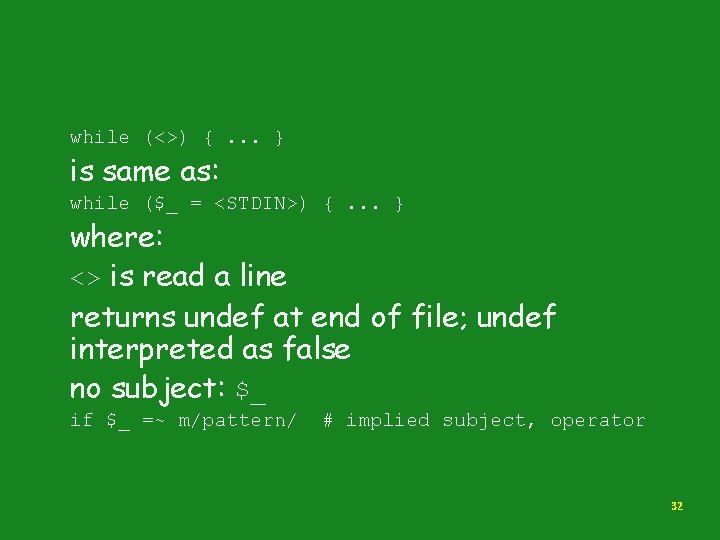
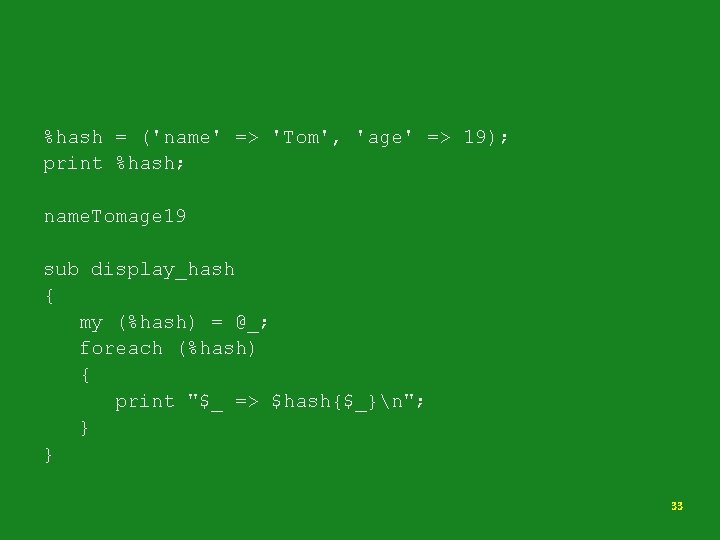
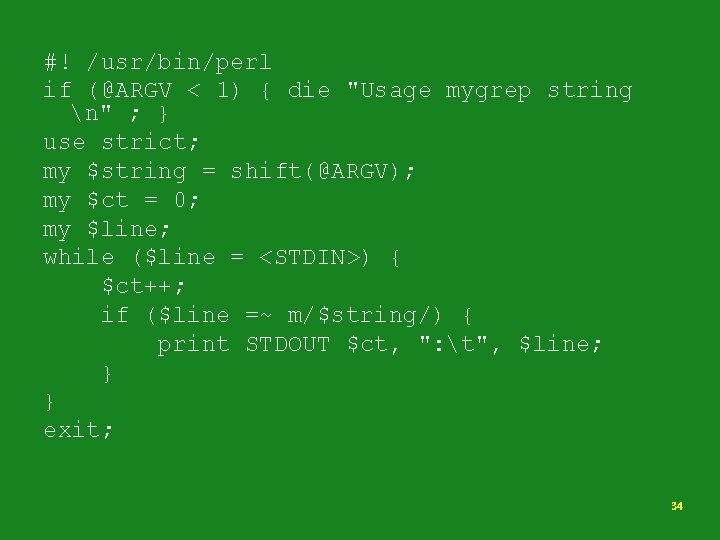
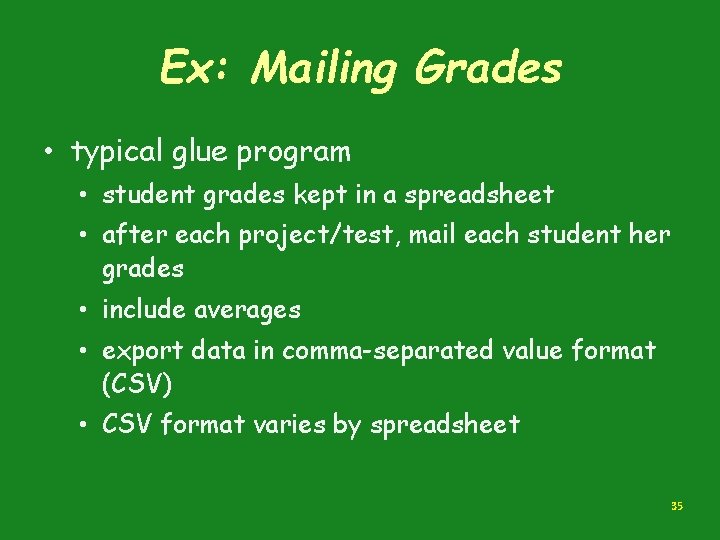
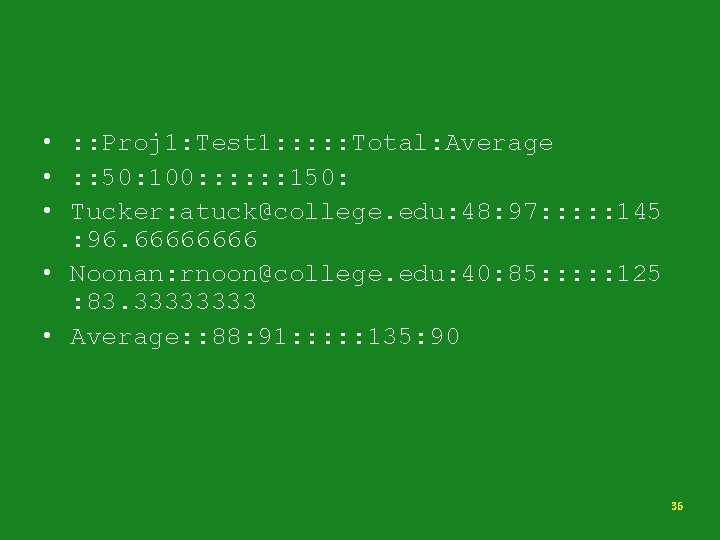
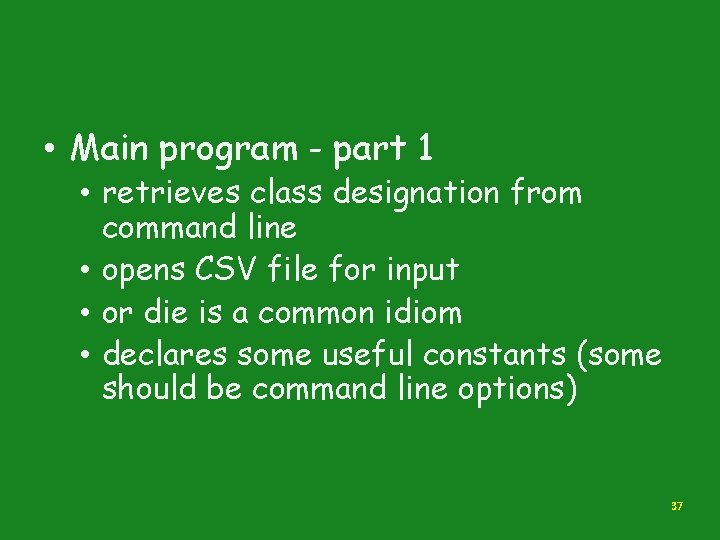
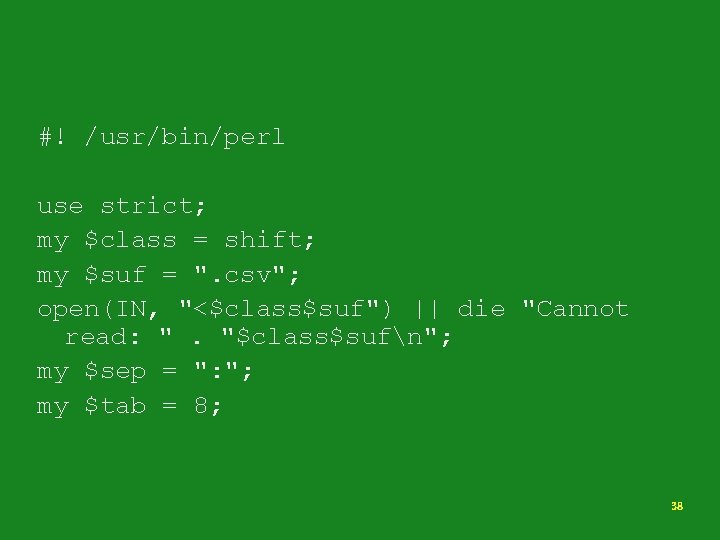
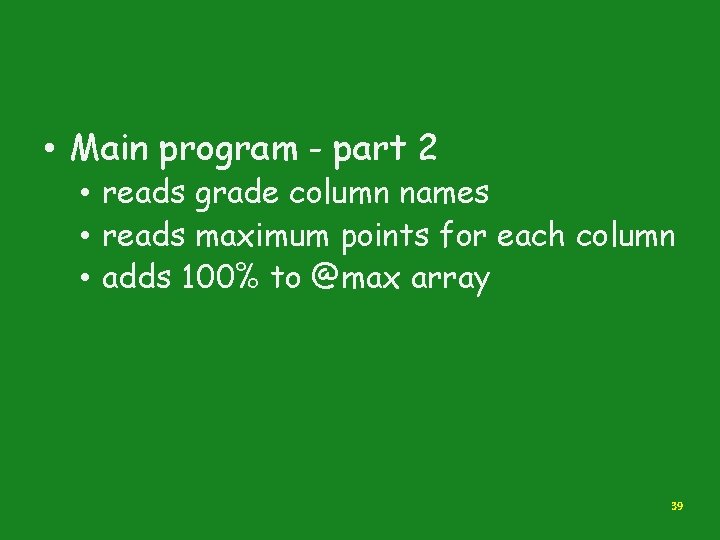
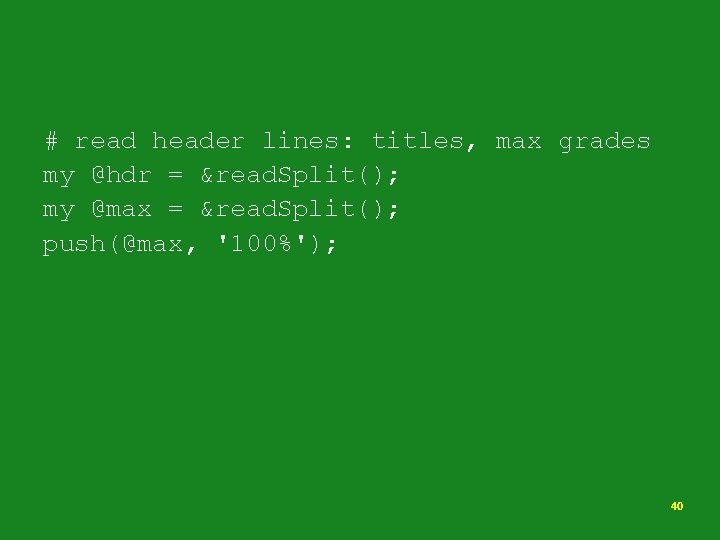
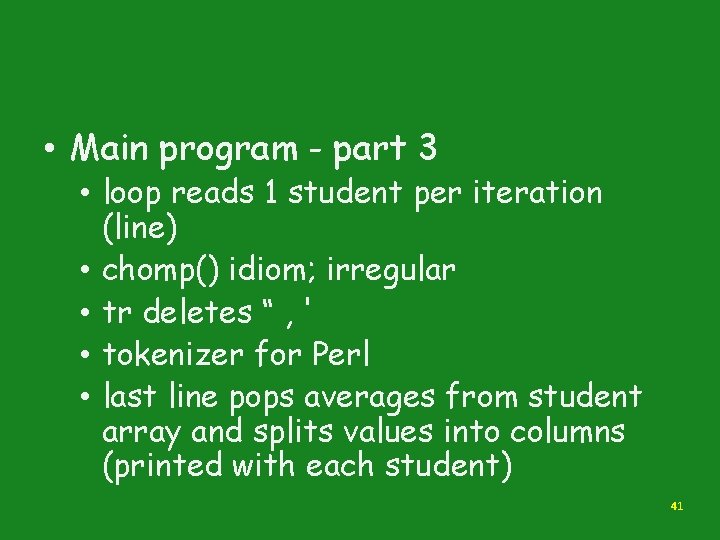
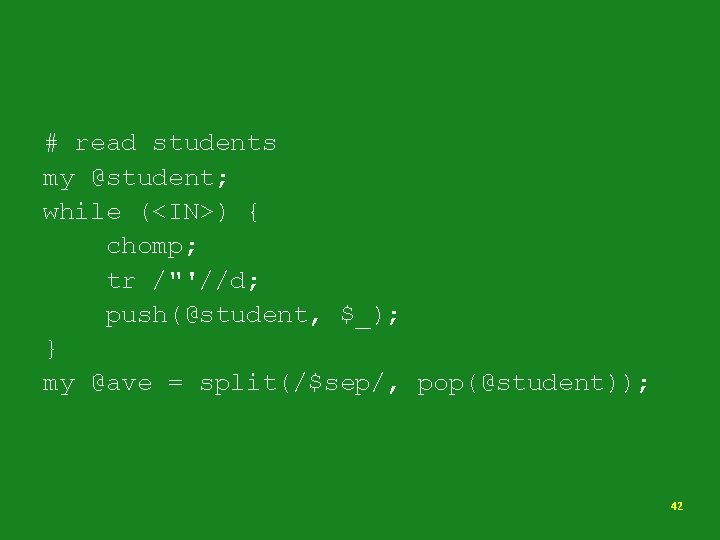
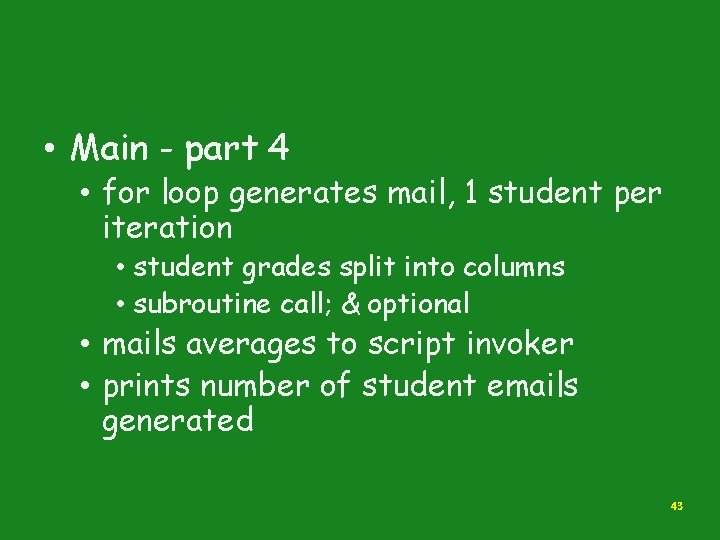
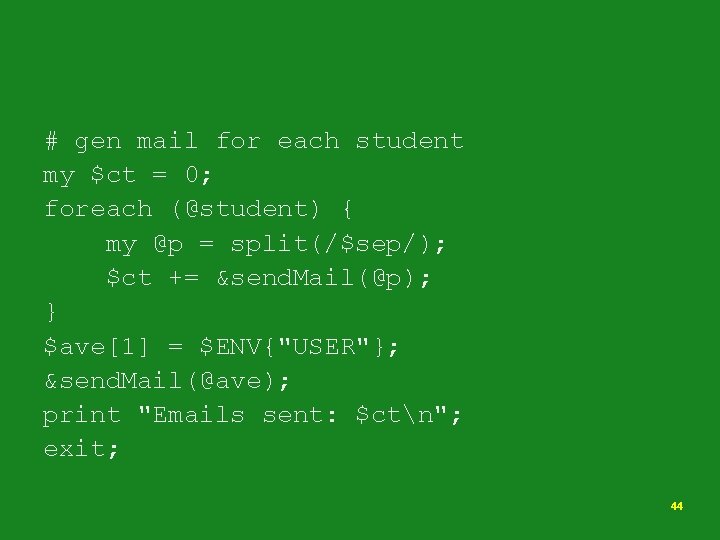
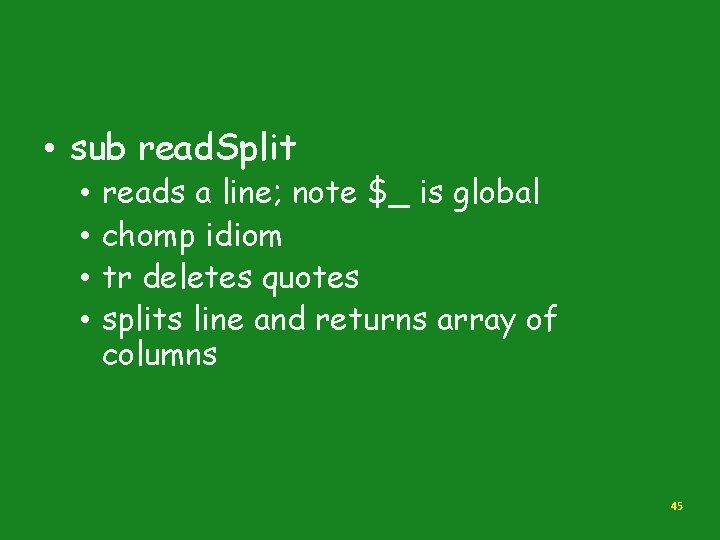
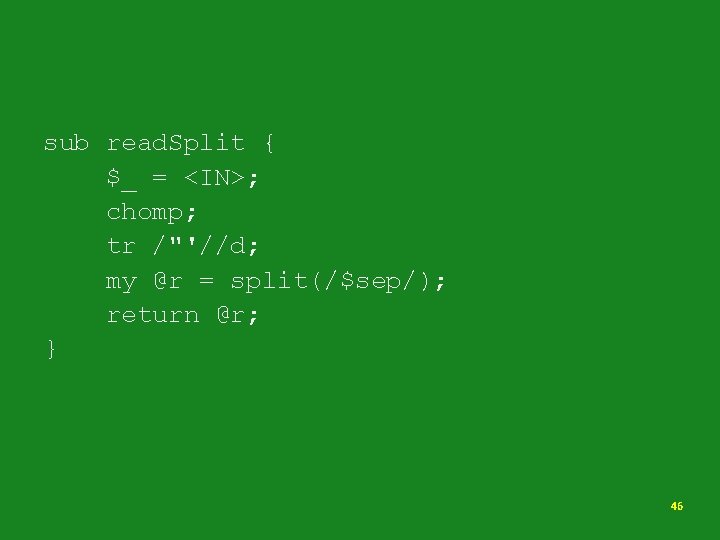
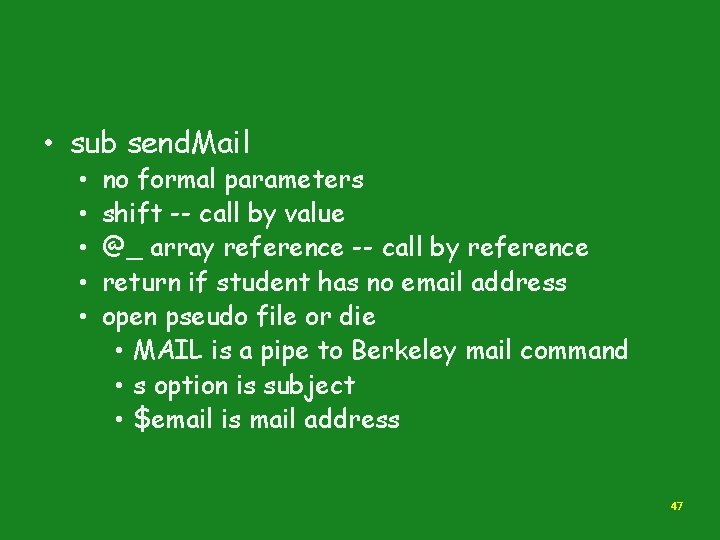
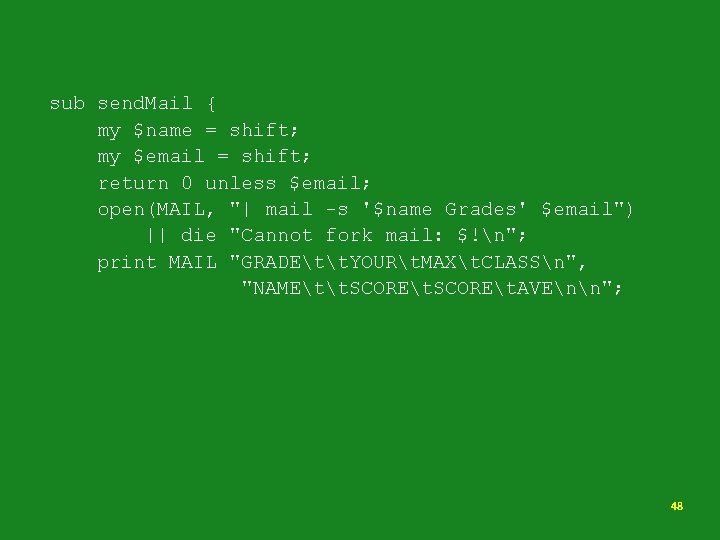
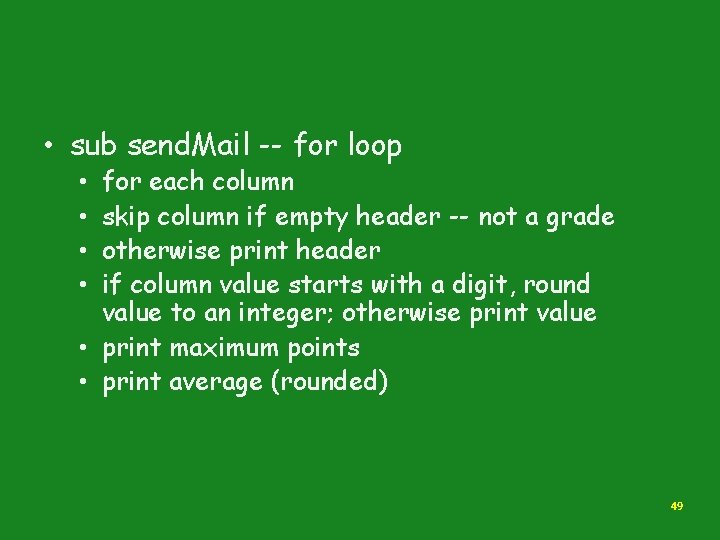
![my $ct = 1; foreach (@_) { $ct++; next unless $hdr[$ct]; print MAIL "$hdr[$ct]t"; my $ct = 1; foreach (@_) { $ct++; next unless $hdr[$ct]; print MAIL "$hdr[$ct]t";](https://slidetodoc.com/presentation_image_h2/572a39988cfe878832445211d08983be/image-50.jpg)
![print MAIL "t$max[$ct]t"; if ($ave[$ct] =~ /^d/) { print MAIL int($ave[$ct] + 0. 5); print MAIL "t$max[$ct]t"; if ($ave[$ct] =~ /^d/) { print MAIL int($ave[$ct] + 0. 5);](https://slidetodoc.com/presentation_image_h2/572a39988cfe878832445211d08983be/image-51.jpg)
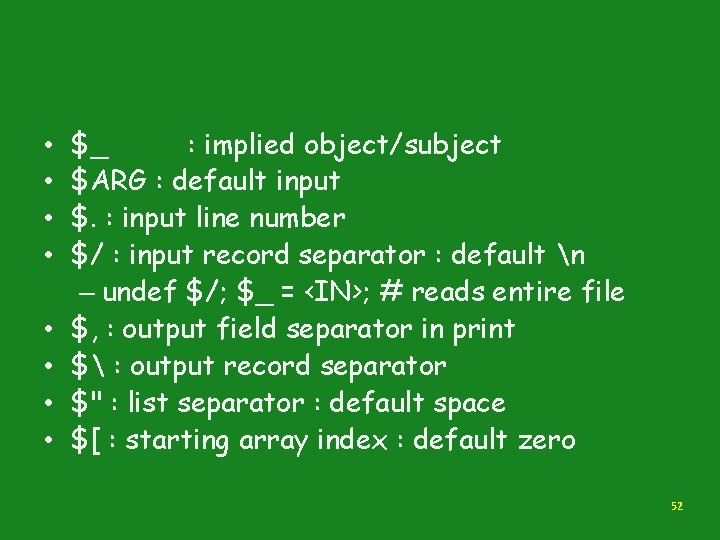
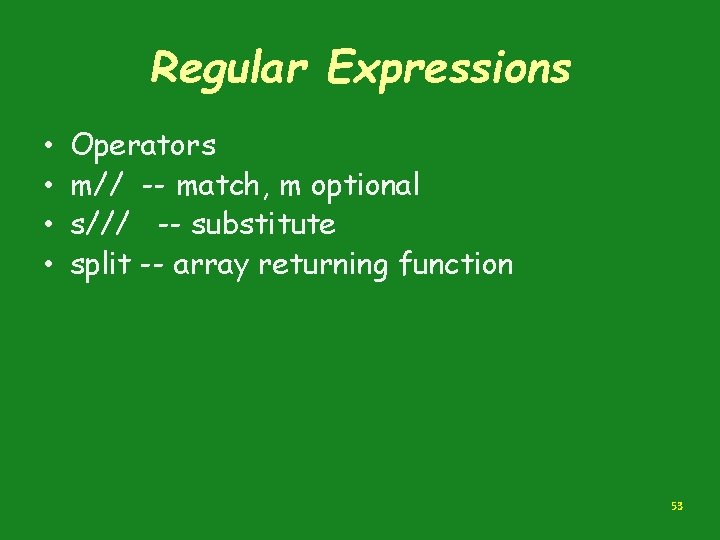
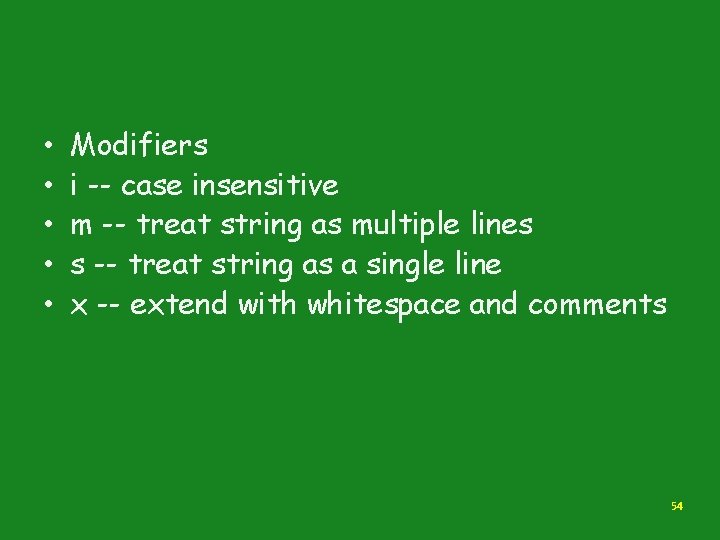
- Slides: 54
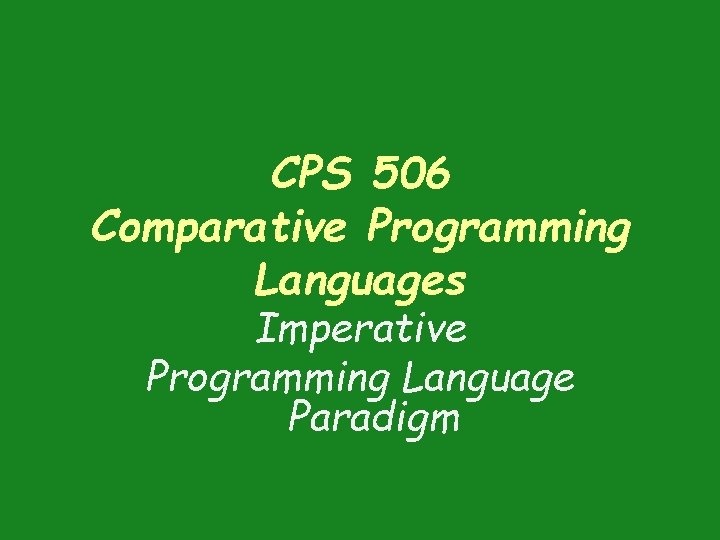
CPS 506 Comparative Programming Languages Imperative Programming Language Paradigm
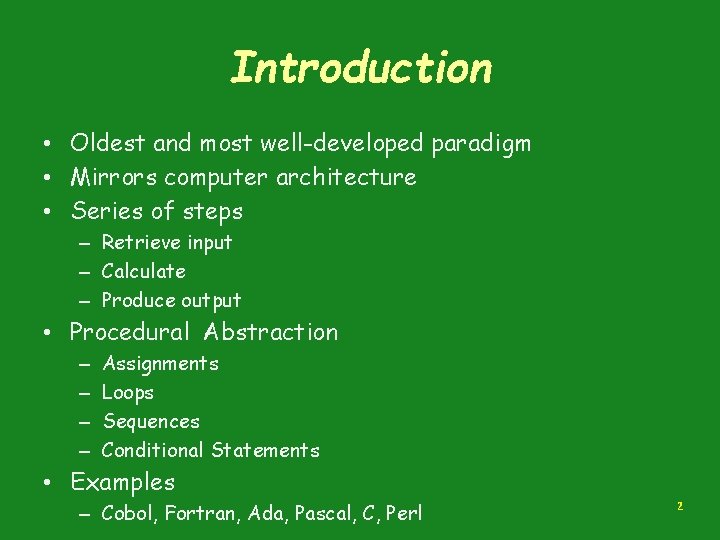
Introduction • Oldest and most well-developed paradigm • Mirrors computer architecture • Series of steps – Retrieve input – Calculate – Produce output • Procedural Abstraction – – Assignments Loops Sequences Conditional Statements • Examples – Cobol, Fortran, Ada, Pascal, C, Perl 2
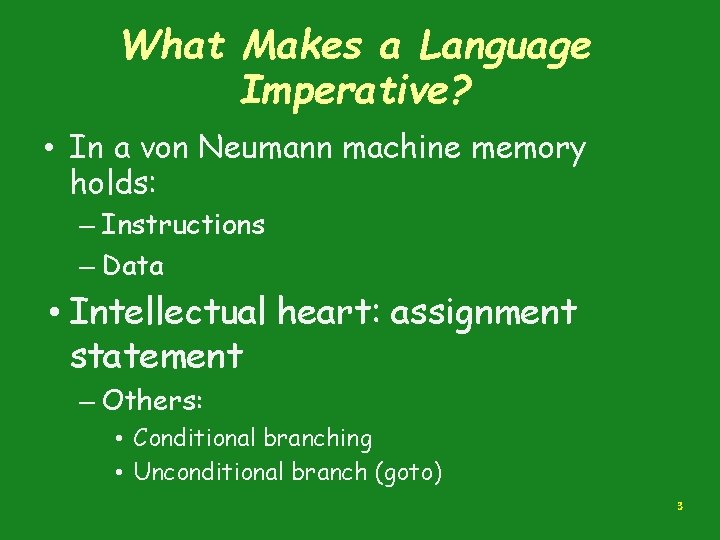
What Makes a Language Imperative? • In a von Neumann machine memory holds: – Instructions – Data • Intellectual heart: assignment statement – Others: • Conditional branching • Unconditional branch (goto) 3
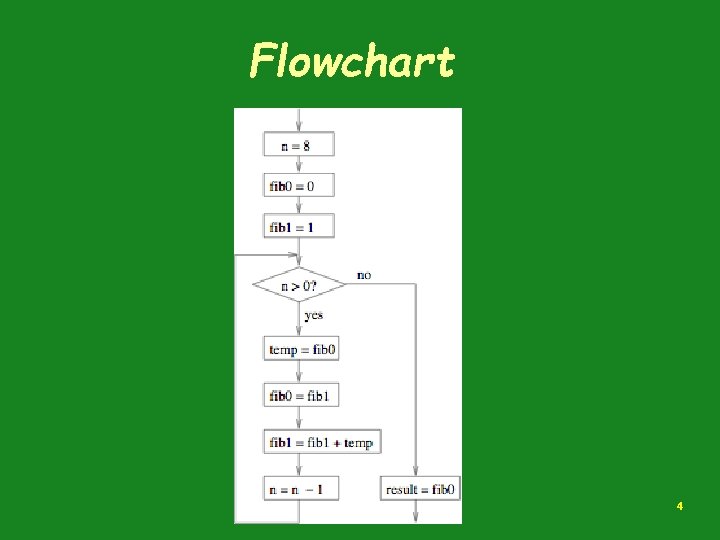
Flowchart 4
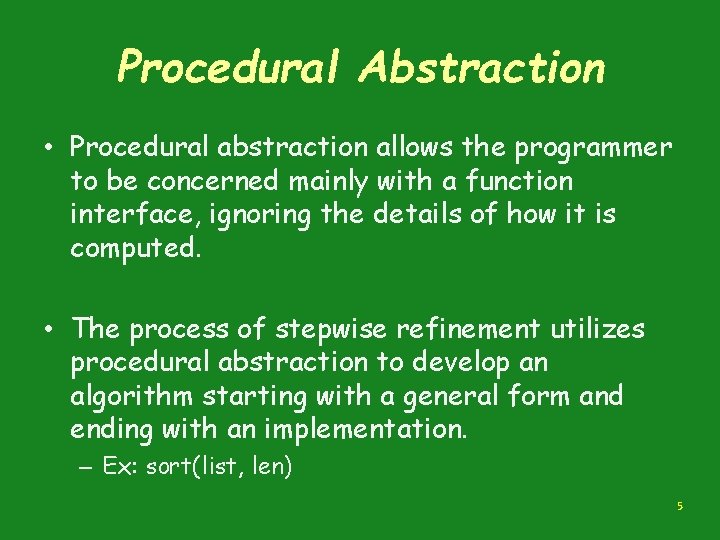
Procedural Abstraction • Procedural abstraction allows the programmer to be concerned mainly with a function interface, ignoring the details of how it is computed. • The process of stepwise refinement utilizes procedural abstraction to develop an algorithm starting with a general form and ending with an implementation. – Ex: sort(list, len) 5
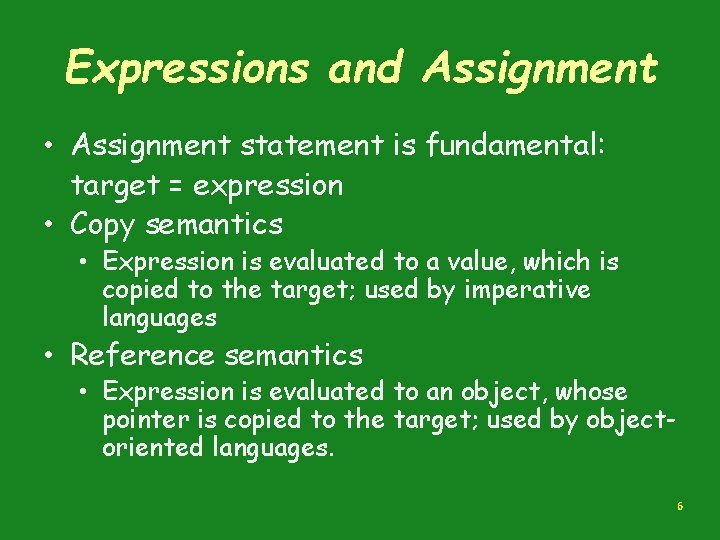
Expressions and Assignment • Assignment statement is fundamental: target = expression • Copy semantics • Expression is evaluated to a value, which is copied to the target; used by imperative languages • Reference semantics • Expression is evaluated to an object, whose pointer is copied to the target; used by objectoriented languages. 6
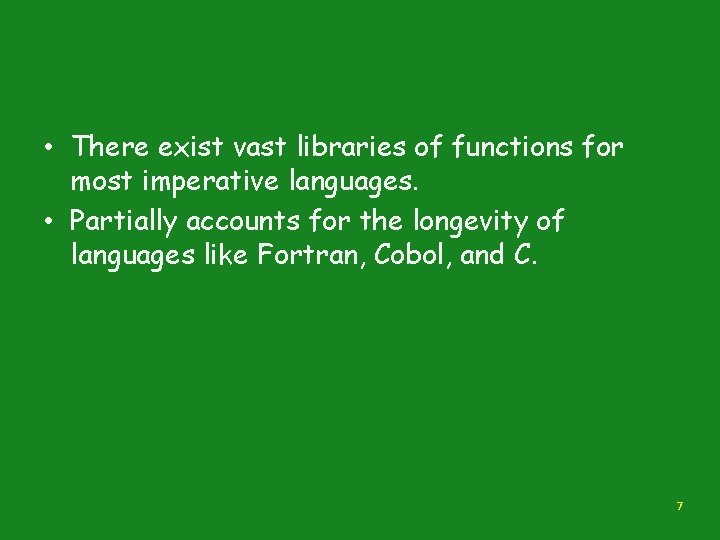
• There exist vast libraries of functions for most imperative languages. • Partially accounts for the longevity of languages like Fortran, Cobol, and C. 7
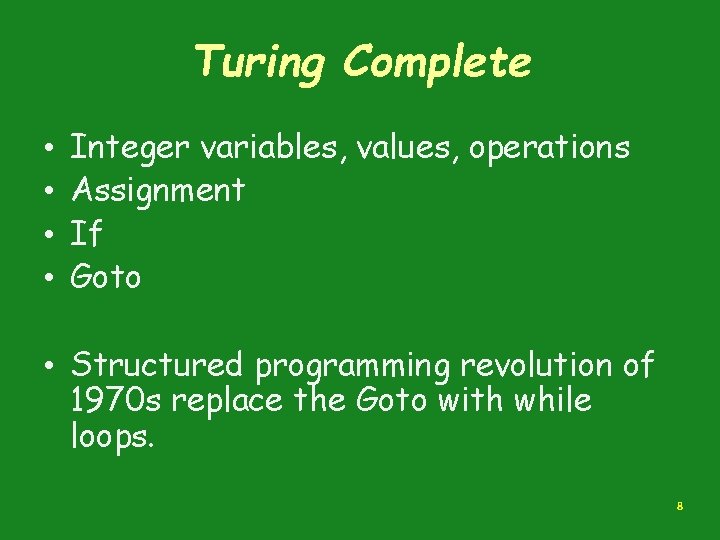
Turing Complete • • Integer variables, values, operations Assignment If Goto • Structured programming revolution of 1970 s replace the Goto with while loops. 8
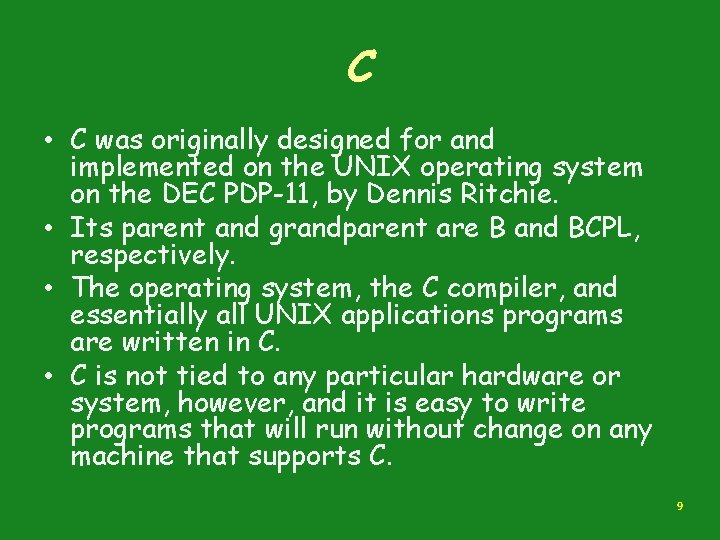
C • C was originally designed for and implemented on the UNIX operating system on the DEC PDP-11, by Dennis Ritchie. • Its parent and grandparent are B and BCPL, respectively. • The operating system, the C compiler, and essentially all UNIX applications programs are written in C. • C is not tied to any particular hardware or system, however, and it is easy to write programs that will run without change on any machine that supports C. 9
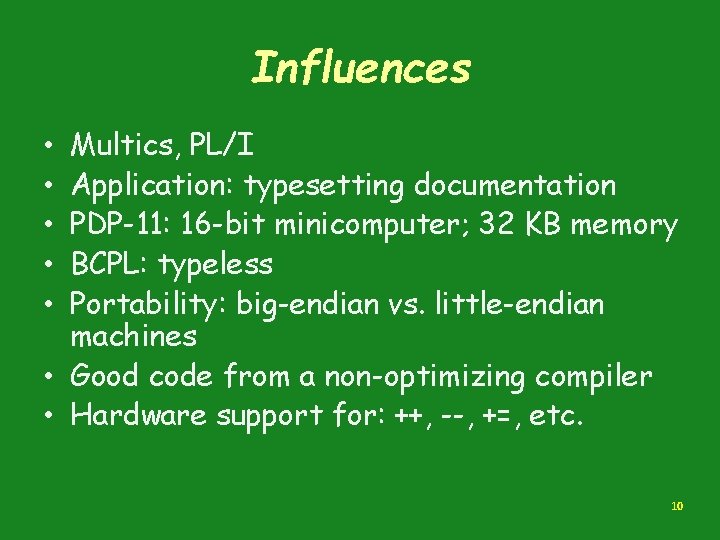
Influences Multics, PL/I Application: typesetting documentation PDP-11: 16 -bit minicomputer; 32 KB memory BCPL: typeless Portability: big-endian vs. little-endian machines • Good code from a non-optimizing compiler • Hardware support for: ++, --, +=, etc. • • • 10
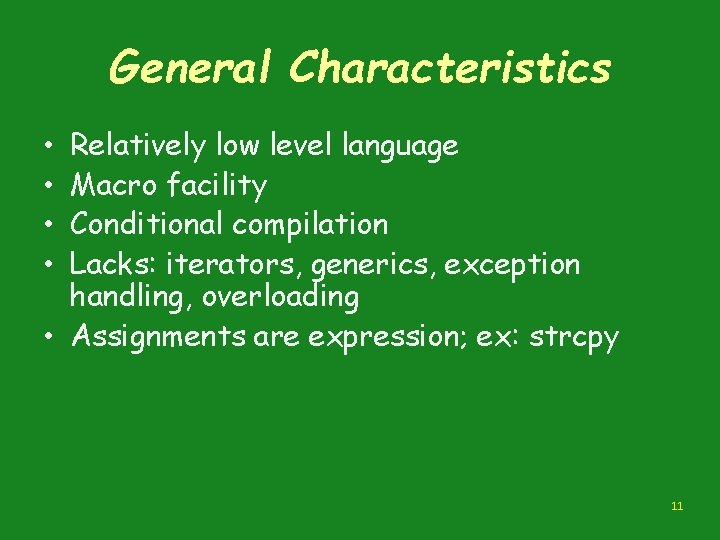
General Characteristics Relatively low level language Macro facility Conditional compilation Lacks: iterators, generics, exception handling, overloading • Assignments are expression; ex: strcpy • • 11
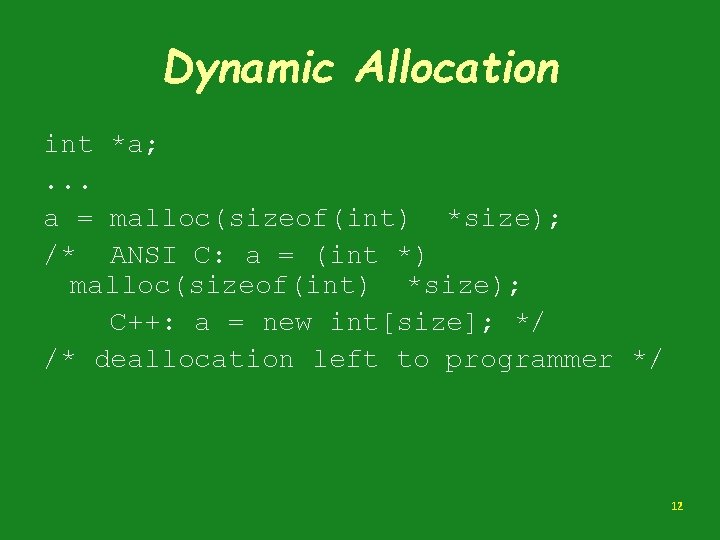
Dynamic Allocation int *a; . . . a = malloc(sizeof(int) *size); /* ANSI C: a = (int *) malloc(sizeof(int) *size); C++: a = new int[size]; */ /* deallocation left to programmer */ 12
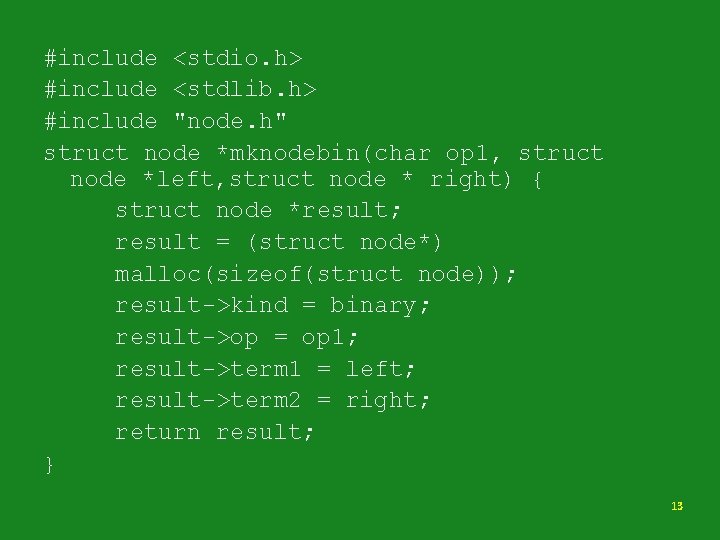
#include <stdio. h> #include <stdlib. h> #include "node. h" struct node *mknodebin(char op 1, struct node *left, struct node * right) { struct node *result; result = (struct node*) malloc(sizeof(struct node)); result->kind = binary; result->op = op 1; result->term 1 = left; result->term 2 = right; return result; } 13
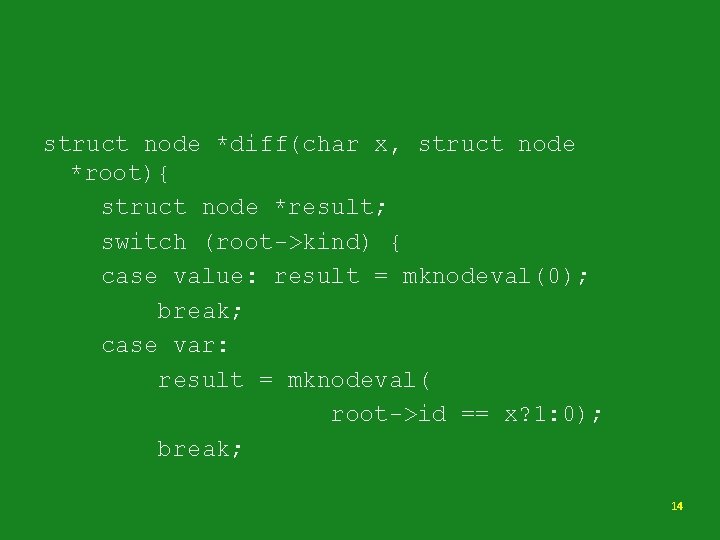
struct node *diff(char x, struct node *root){ struct node *result; switch (root->kind) { case value: result = mknodeval(0); break; case var: result = mknodeval( root->id == x? 1: 0); break; 14
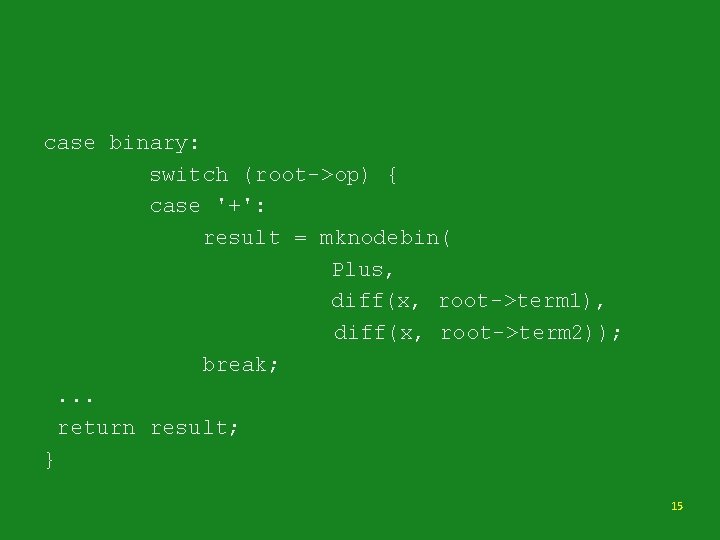
case binary: switch (root->op) { case '+': result = mknodebin( Plus, diff(x, root->term 1), diff(x, root->term 2)); break; . . . return result; } 15
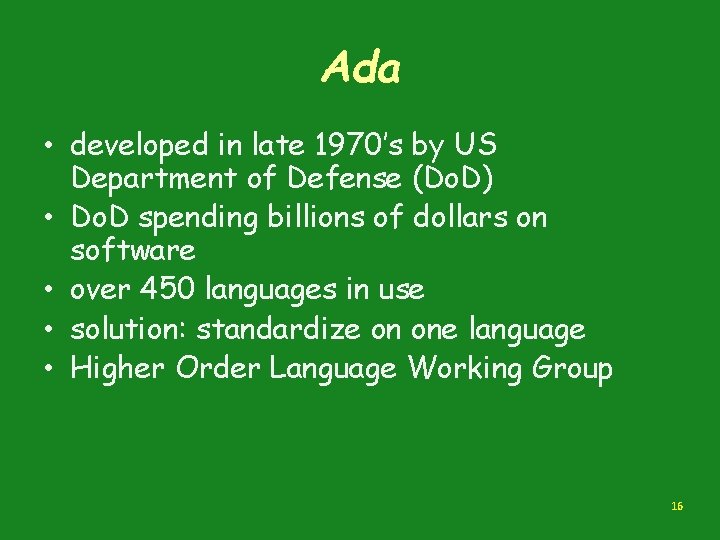
Ada • developed in late 1970’s by US Department of Defense (Do. D) • Do. D spending billions of dollars on software • over 450 languages in use • solution: standardize on one language • Higher Order Language Working Group 16
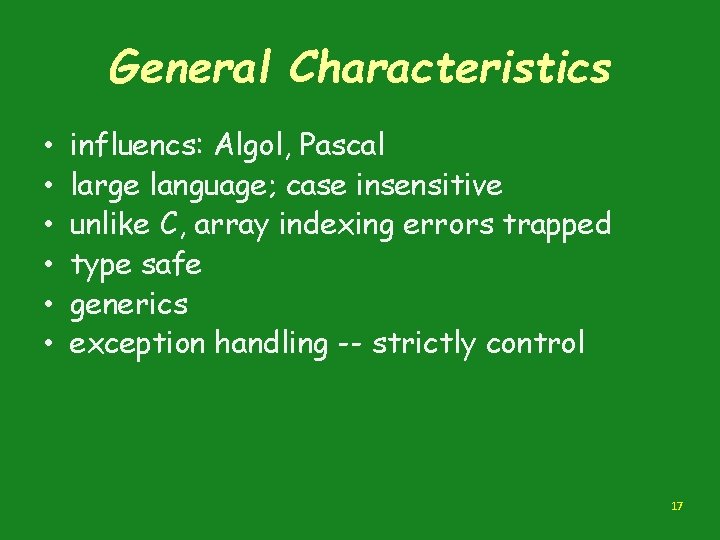
General Characteristics • • • influencs: Algol, Pascal large language; case insensitive unlike C, array indexing errors trapped type safe generics exception handling -- strictly control 17
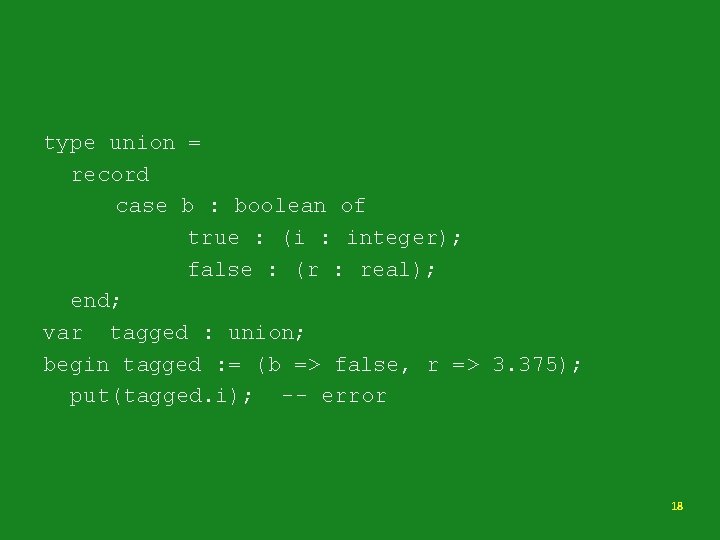
type union = record case b : boolean of true : (i : integer); false : (r : real); end; var tagged : union; begin tagged : = (b => false, r => 3. 375); put(tagged. i); -- error 18
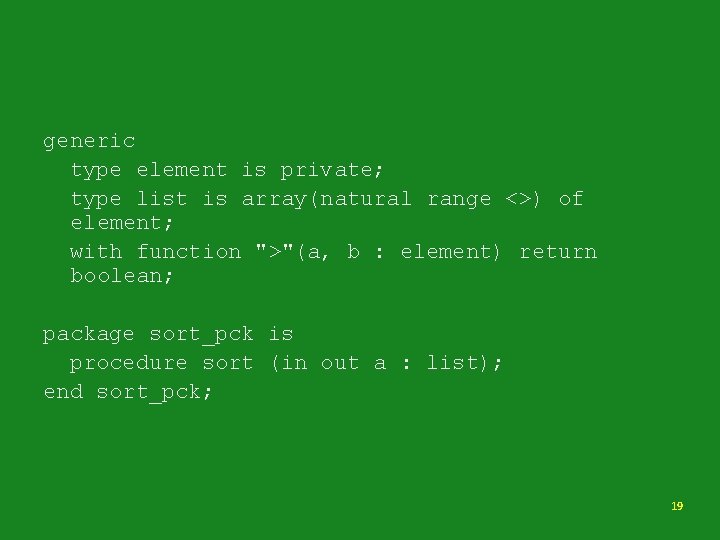
generic type element is private; type list is array(natural range <>) of element; with function ">"(a, b : element) return boolean; package sort_pck is procedure sort (in out a : list); end sort_pck; 19
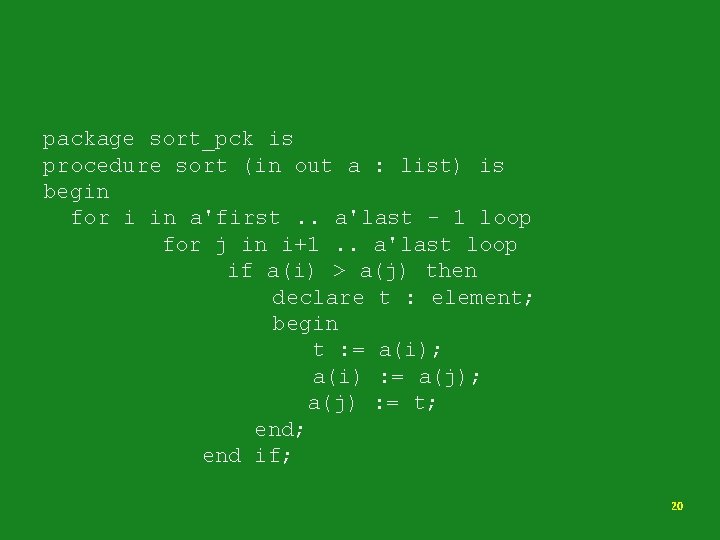
package sort_pck is procedure sort (in out a : list) is begin for i in a'first. . a'last - 1 loop for j in i+1. . a'last loop if a(i) > a(j) then declare t : element; begin t : = a(i); a(i) : = a(j); a(j) : = t; end if; 20
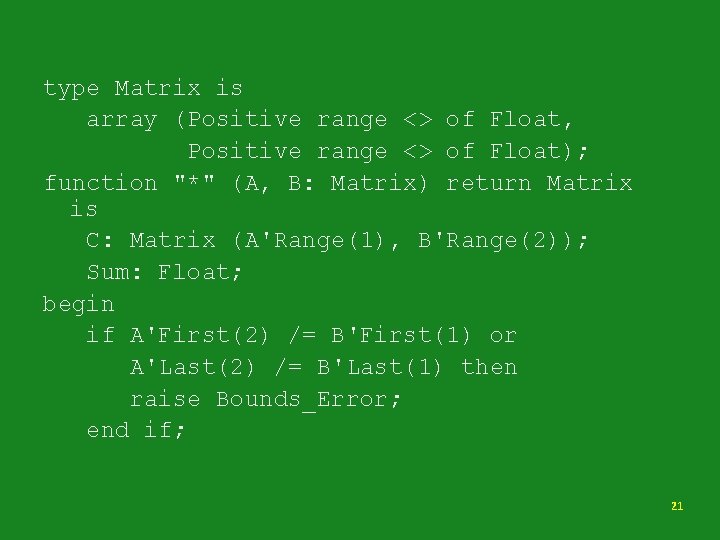
type Matrix is array (Positive range <> of Float, Positive range <> of Float); function "*" (A, B: Matrix) return Matrix is C: Matrix (A'Range(1), B'Range(2)); Sum: Float; begin if A'First(2) /= B'First(1) or A'Last(2) /= B'Last(1) then raise Bounds_Error; end if; 21
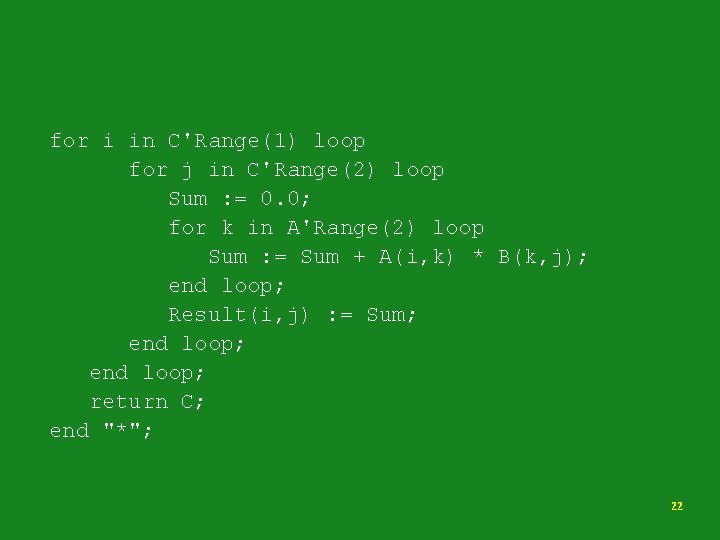
for i in C'Range(1) loop for j in C'Range(2) loop Sum : = 0. 0; for k in A'Range(2) loop Sum : = Sum + A(i, k) * B(k, j); end loop; Result(i, j) : = Sum; end loop; return C; end "*"; 22
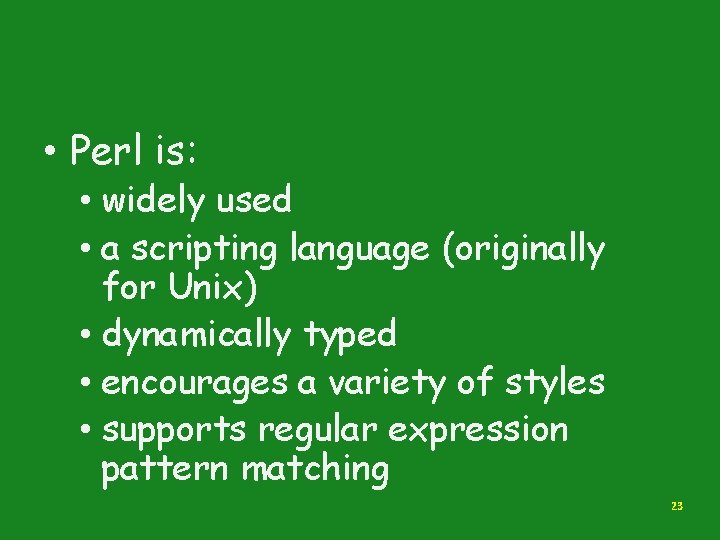
• Perl is: • widely used • a scripting language (originally for Unix) • dynamically typed • encourages a variety of styles • supports regular expression pattern matching 23
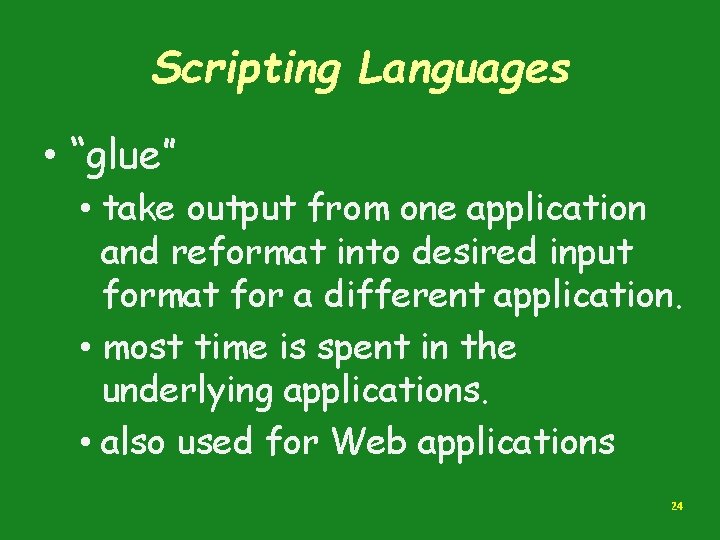
Scripting Languages • “glue” • take output from one application and reformat into desired input format for a different application. • most time is spent in the underlying applications. • also used for Web applications 24
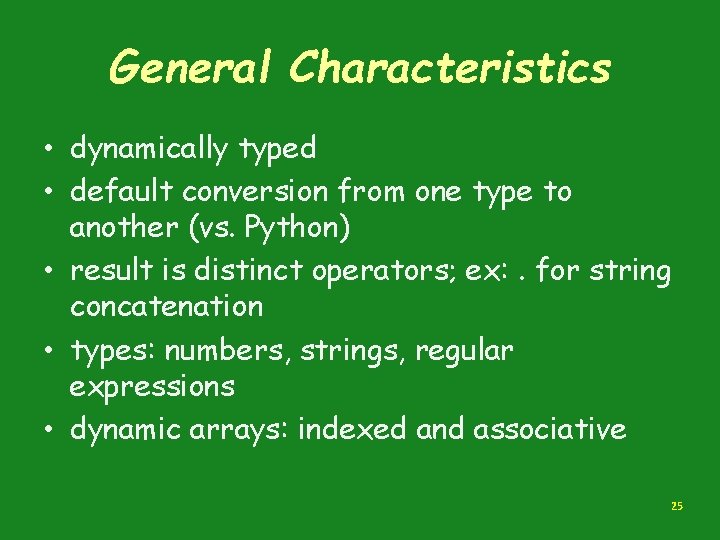
General Characteristics • dynamically typed • default conversion from one type to another (vs. Python) • result is distinct operators; ex: . for string concatenation • types: numbers, strings, regular expressions • dynamic arrays: indexed and associative 25
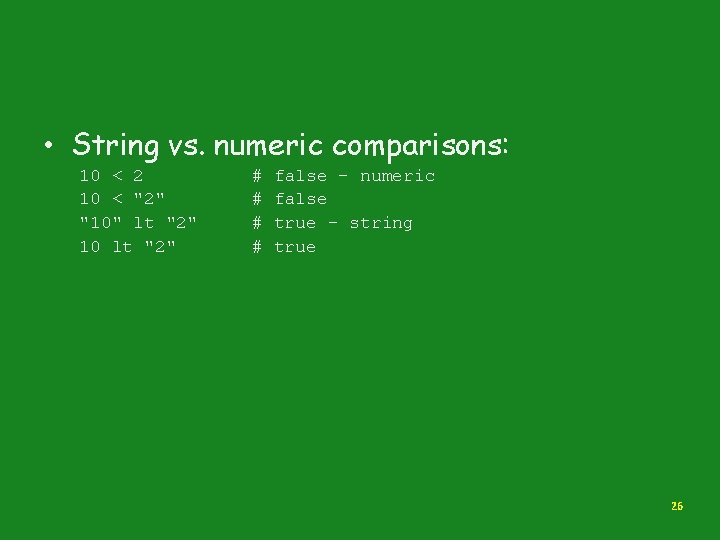
• String vs. numeric comparisons: 10 < 2 10 < "2" "10" lt "2" 10 lt "2" # # false - numeric false true - string true 26
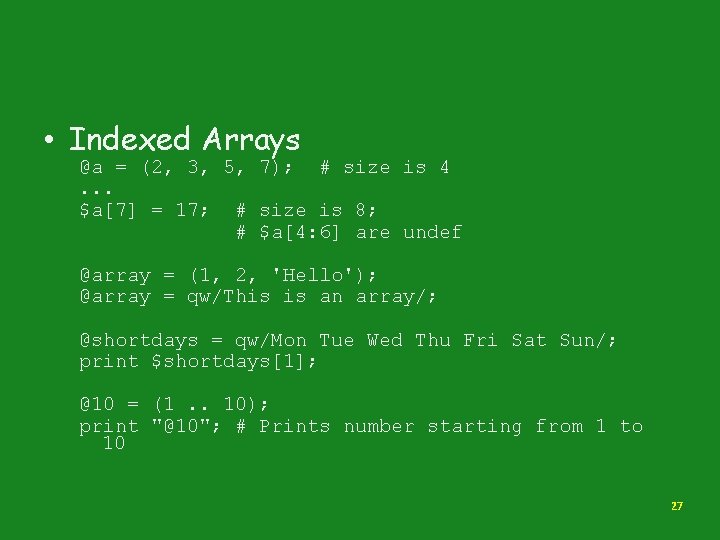
• Indexed Arrays @a = (2, 3, 5, 7); # size is 4. . . $a[7] = 17; # size is 8; # $a[4: 6] are undef @array = (1, 2, 'Hello'); @array = qw/This is an array/; @shortdays = qw/Mon Tue Wed Thu Fri Sat Sun/; print $shortdays[1]; @10 = (1. . 10); print "@10"; # Prints number starting from 1 to 10 27
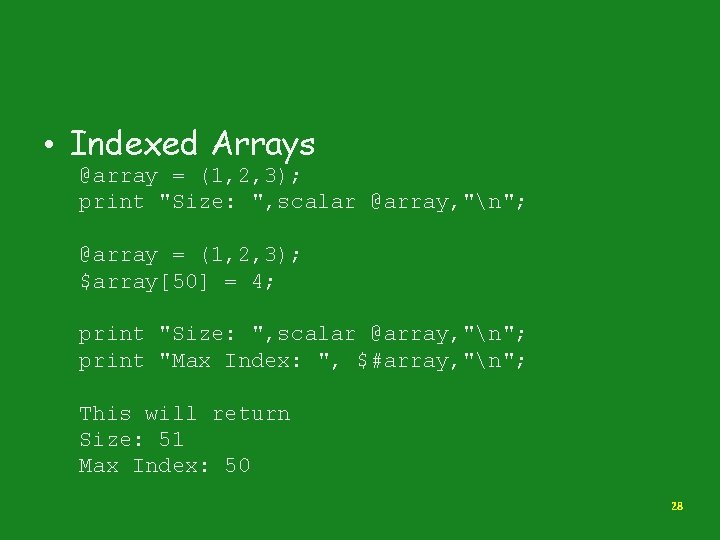
• Indexed Arrays @array = (1, 2, 3); print "Size: ", scalar @array, "n"; @array = (1, 2, 3); $array[50] = 4; print "Size: ", scalar @array, "n"; print "Max Index: ", $#array, "n"; This will return Size: 51 Max Index: 50 28
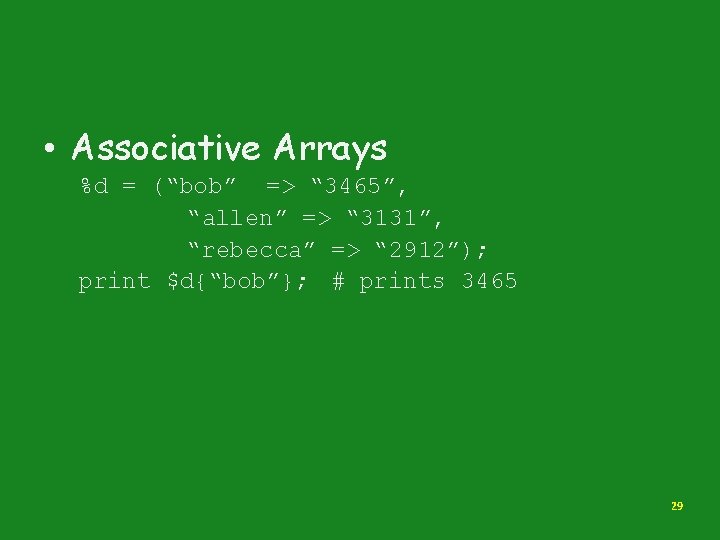
• Associative Arrays %d = (“bob” => “ 3465”, “allen” => “ 3131”, “rebecca” => “ 2912”); print $d{“bob”}; # prints 3465 29
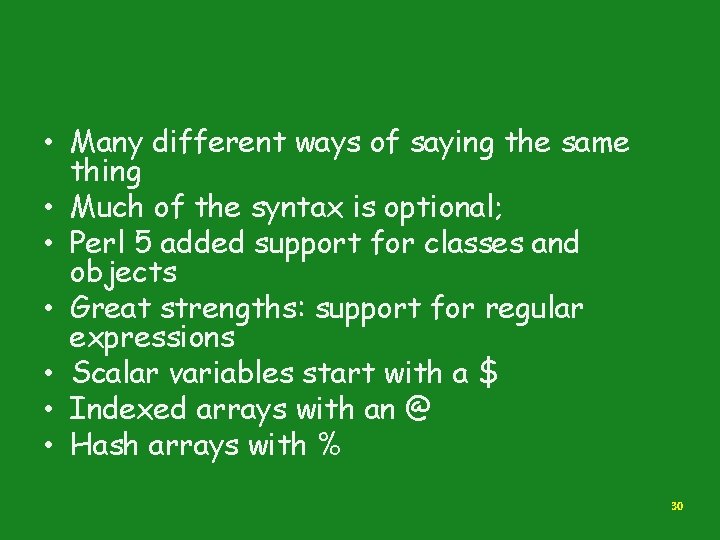
• Many different ways of saying the same thing • Much of the syntax is optional; • Perl 5 added support for classes and objects • Great strengths: support for regular expressions • Scalar variables start with a $ • Indexed arrays with an @ • Hash arrays with % 30
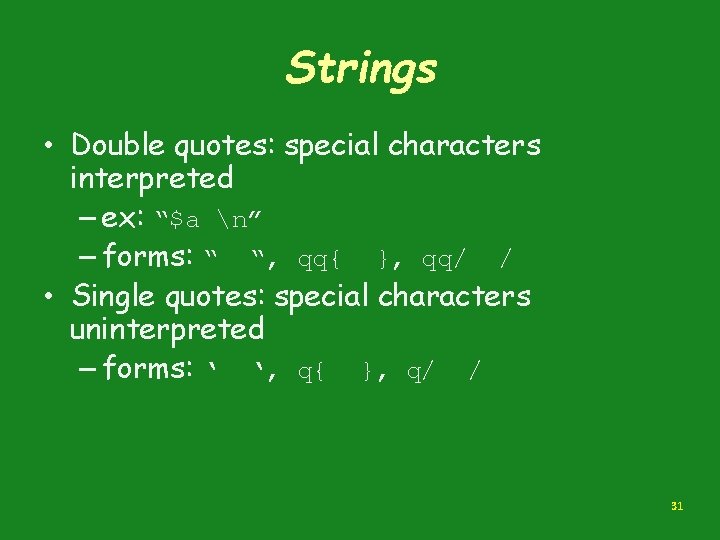
Strings • Double quotes: special characters interpreted – ex: “$a n” – forms: “ “, qq{ }, qq/ / • Single quotes: special characters uninterpreted – forms: ‘ ‘, q{ }, q/ / 31
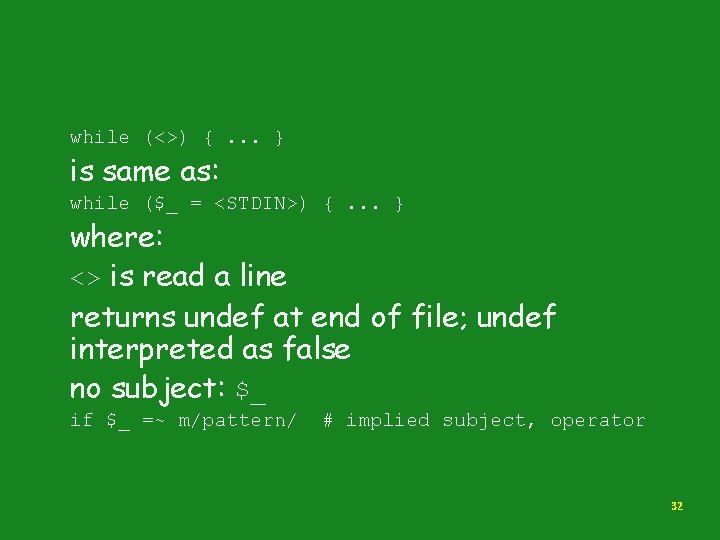
while (<>) {. . . } is same as: while ($_ = <STDIN>) {. . . } where: <> is read a line returns undef at end of file; undef interpreted as false no subject: $_ if $_ =~ m/pattern/ # implied subject, operator 32
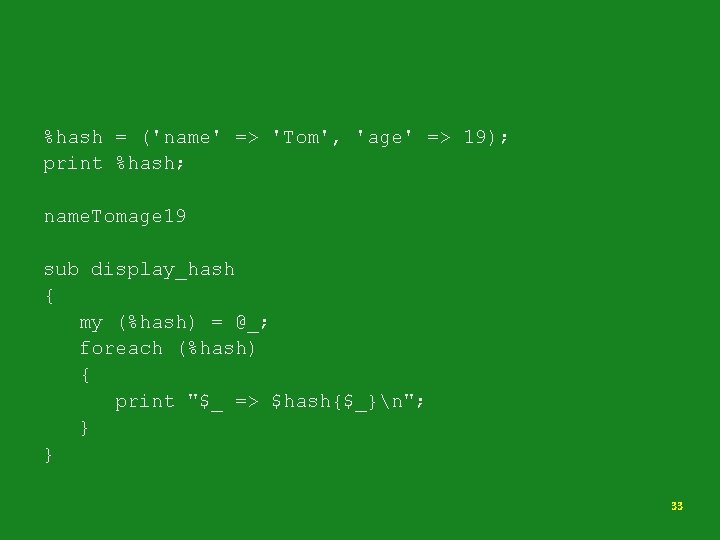
%hash = ('name' => 'Tom', 'age' => 19); print %hash; name. Tomage 19 sub display_hash { my (%hash) = @_; foreach (%hash) { print "$_ => $hash{$_}n"; } } 33
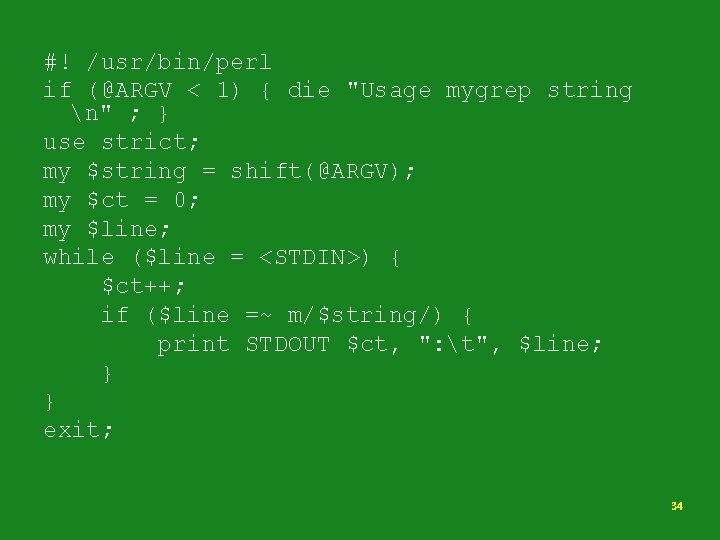
#! /usr/bin/perl if (@ARGV < 1) { die "Usage mygrep string n" ; } use strict; my $string = shift(@ARGV); my $ct = 0; my $line; while ($line = <STDIN>) { $ct++; if ($line =~ m/$string/) { print STDOUT $ct, ": t", $line; } } exit; 34
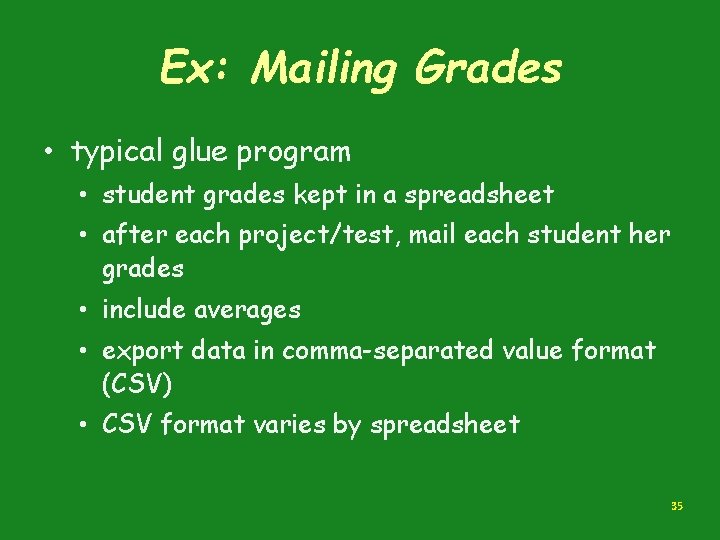
Ex: Mailing Grades • typical glue program • student grades kept in a spreadsheet • after each project/test, mail each student her grades • include averages • export data in comma-separated value format (CSV) • CSV format varies by spreadsheet 35
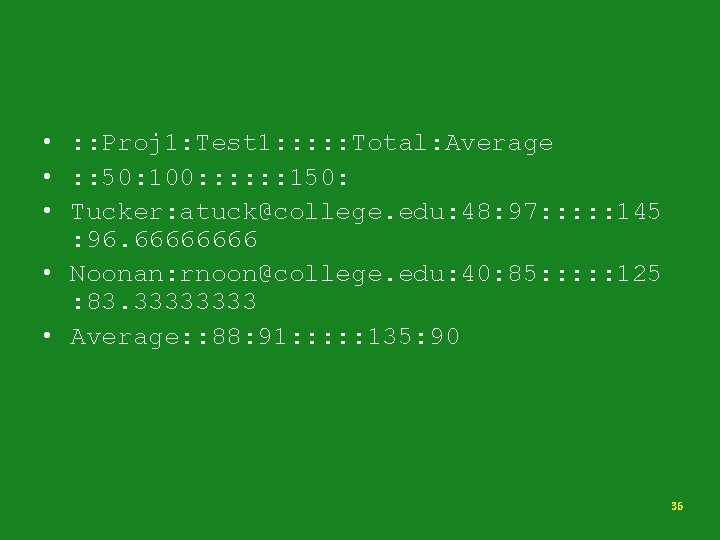
• : : Proj 1: Test 1: : : Total: Average • : : 50: 100: : : 150: • Tucker: atuck@college. edu: 48: 97: : : 145 : 96. 6666 • Noonan: rnoon@college. edu: 40: 85: : : 125 : 83. 3333 • Average: : 88: 91: : : 135: 90 36
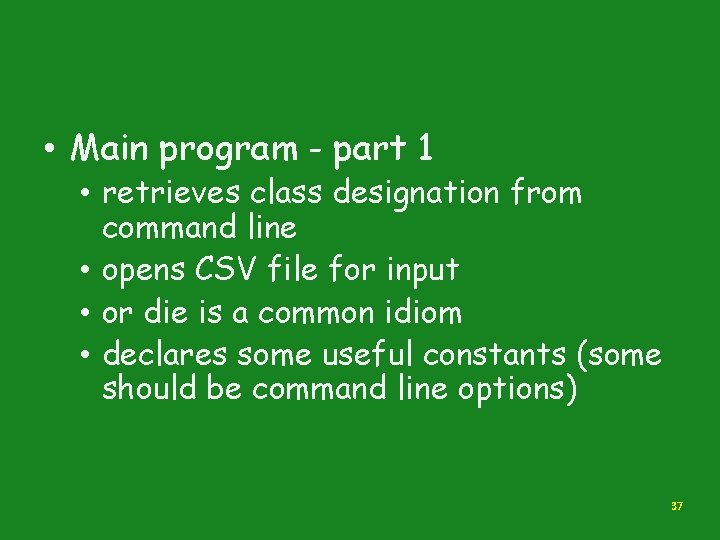
• Main program - part 1 • retrieves class designation from command line • opens CSV file for input • or die is a common idiom • declares some useful constants (some should be command line options) 37
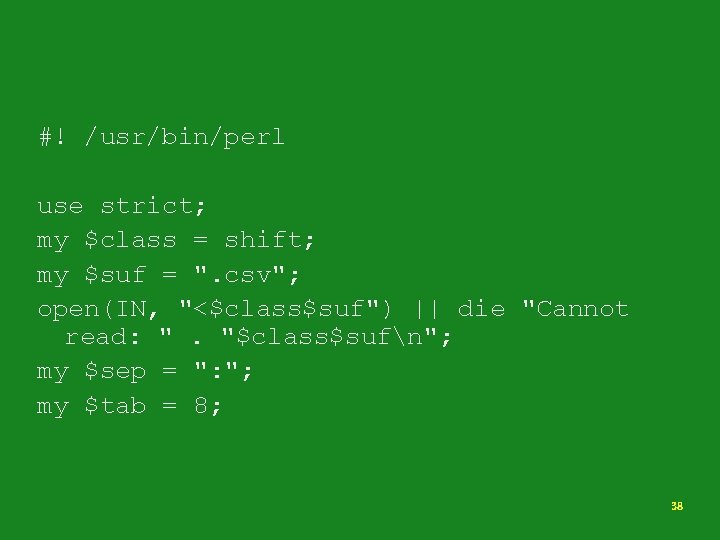
#! /usr/bin/perl use strict; my $class = shift; my $suf = ". csv"; open(IN, "<$class$suf") || die "Cannot read: ". "$class$sufn"; my $sep = ": "; my $tab = 8; 38
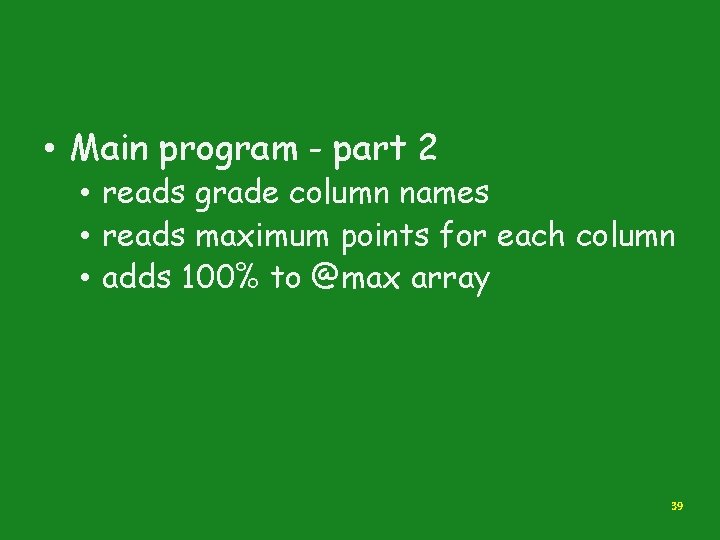
• Main program - part 2 • reads grade column names • reads maximum points for each column • adds 100% to @max array 39
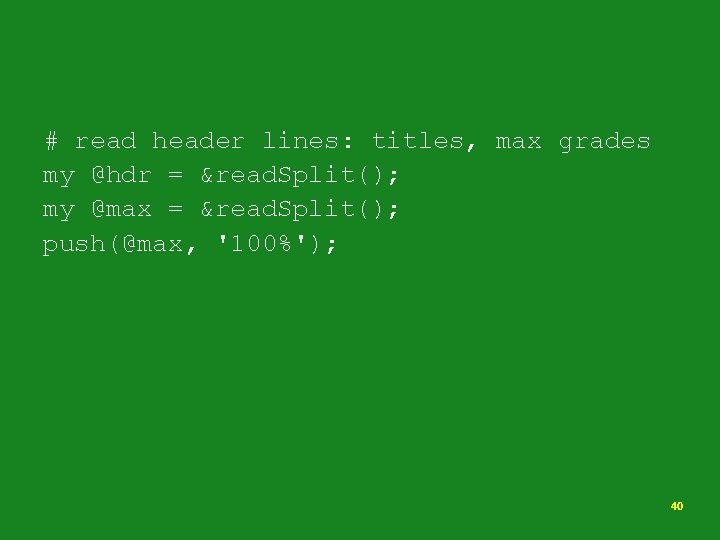
# read header lines: titles, max grades my @hdr = &read. Split(); my @max = &read. Split(); push(@max, '100%'); 40
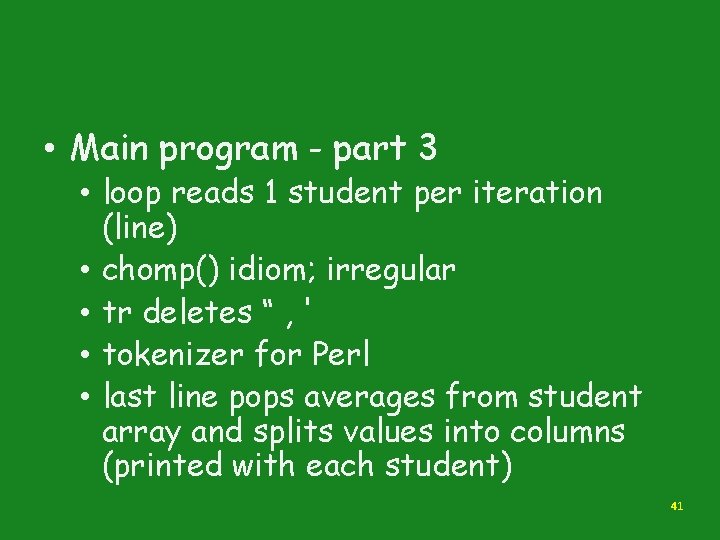
• Main program - part 3 • loop reads 1 student per iteration (line) • chomp() idiom; irregular • tr deletes “ , ' • tokenizer for Perl • last line pops averages from student array and splits values into columns (printed with each student) 41
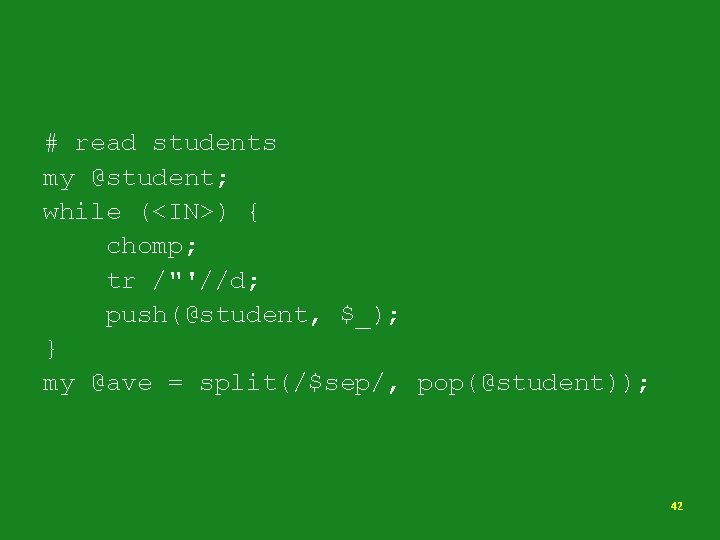
# read students my @student; while (<IN>) { chomp; tr /"'//d; push(@student, $_); } my @ave = split(/$sep/, pop(@student)); 42
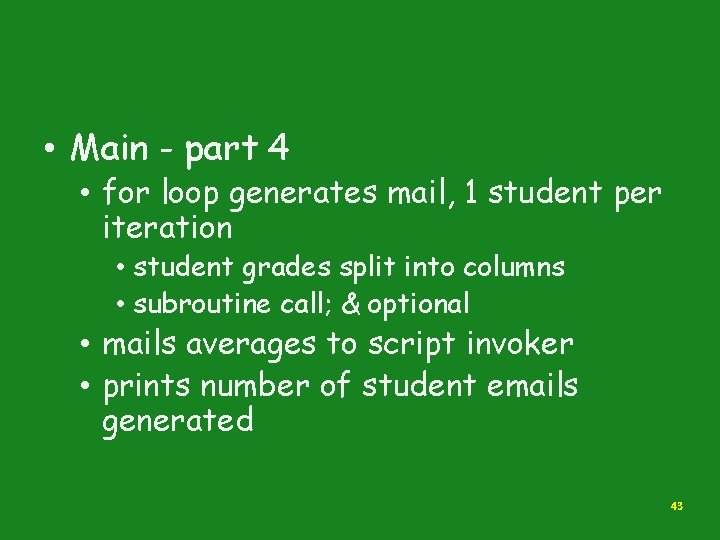
• Main - part 4 • for loop generates mail, 1 student per iteration • student grades split into columns • subroutine call; & optional • mails averages to script invoker • prints number of student emails generated 43
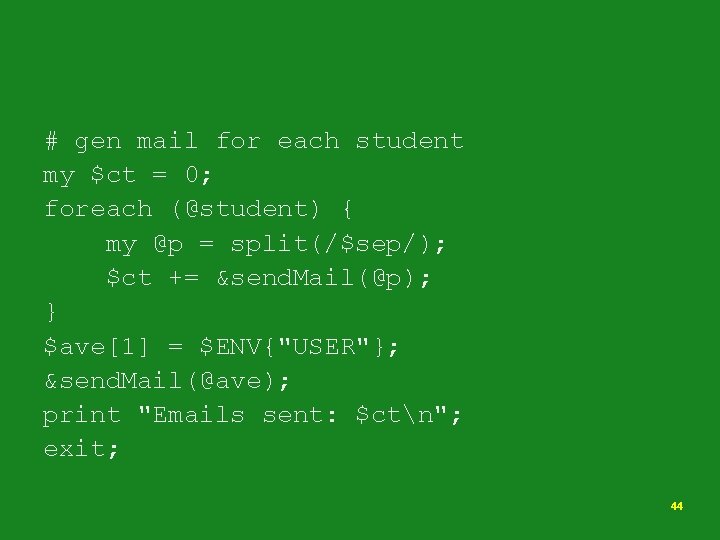
# gen mail for each student my $ct = 0; foreach (@student) { my @p = split(/$sep/); $ct += &send. Mail(@p); } $ave[1] = $ENV{"USER"}; &send. Mail(@ave); print "Emails sent: $ctn"; exit; 44
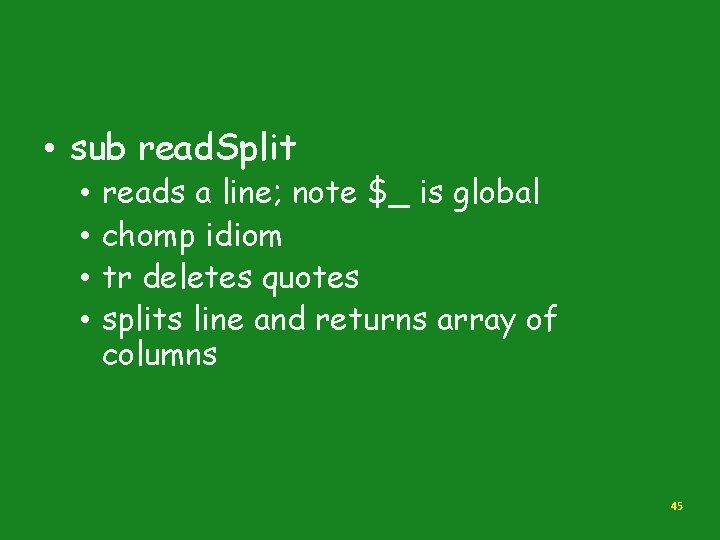
• sub read. Split • • reads a line; note $_ is global chomp idiom tr deletes quotes splits line and returns array of columns 45
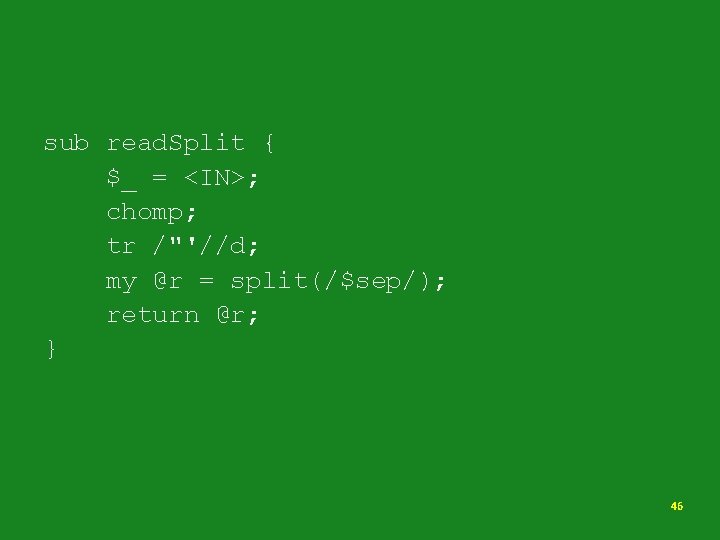
sub read. Split { $_ = <IN>; chomp; tr /"'//d; my @r = split(/$sep/); return @r; } 46
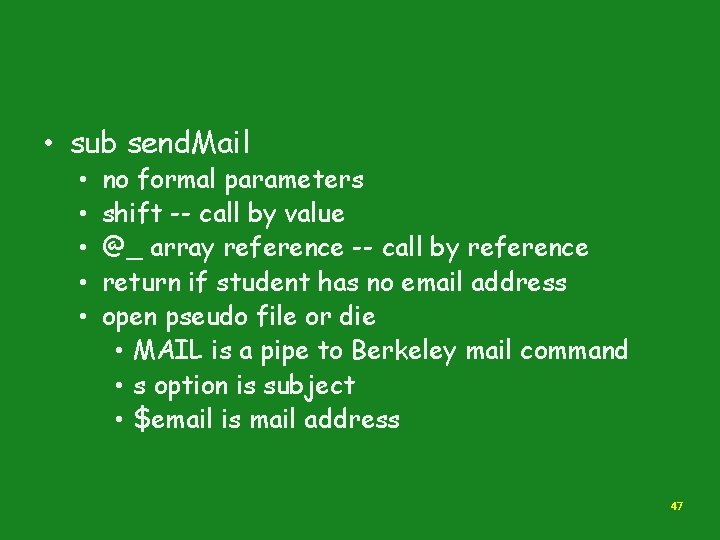
• sub send. Mail • • • no formal parameters shift -- call by value @_ array reference -- call by reference return if student has no email address open pseudo file or die • MAIL is a pipe to Berkeley mail command • s option is subject • $email is mail address 47
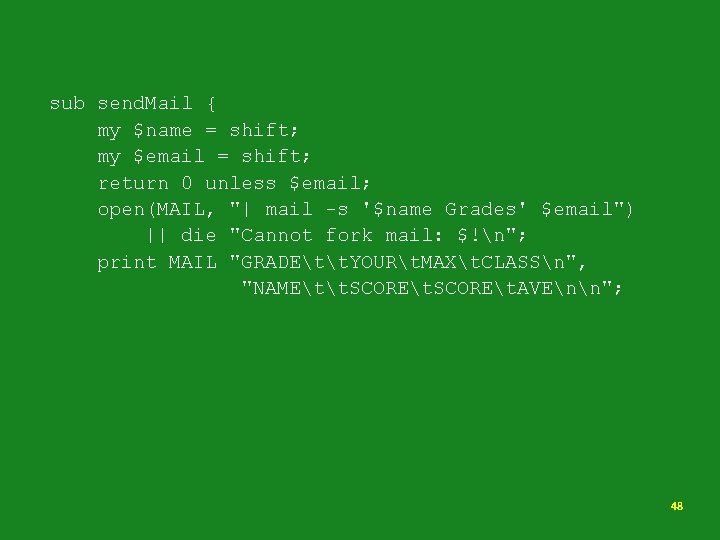
sub send. Mail { my $name = shift; my $email = shift; return 0 unless $email; open(MAIL, "| mail -s '$name Grades' $email") || die "Cannot fork mail: $!n"; print MAIL "GRADEtt. YOURt. MAXt. CLASSn", "NAMEtt. SCOREt. AVEnn"; 48
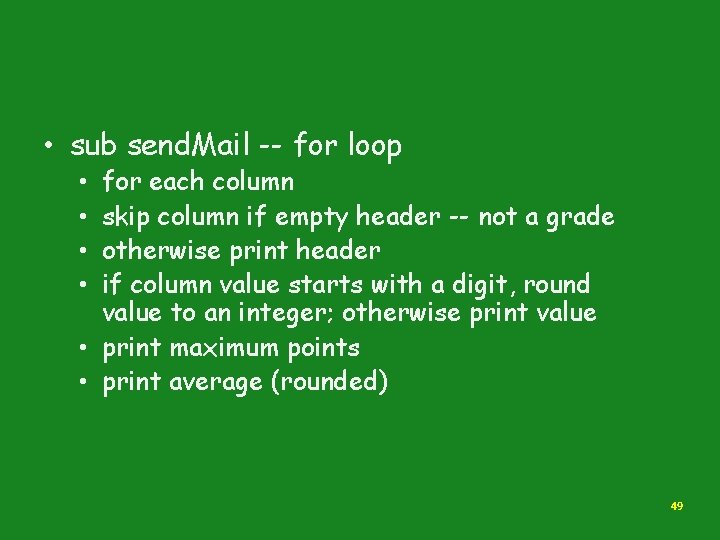
• sub send. Mail -- for loop for each column skip column if empty header -- not a grade otherwise print header if column value starts with a digit, round value to an integer; otherwise print value • print maximum points • print average (rounded) • • 49
![my ct 1 foreach ct next unless hdrct print MAIL hdrctt my $ct = 1; foreach (@_) { $ct++; next unless $hdr[$ct]; print MAIL "$hdr[$ct]t";](https://slidetodoc.com/presentation_image_h2/572a39988cfe878832445211d08983be/image-50.jpg)
my $ct = 1; foreach (@_) { $ct++; next unless $hdr[$ct]; print MAIL "$hdr[$ct]t"; print MAIL "t" if length($hdr[$ct]) < $tab; if (/^d/) { print MAIL int($_ + 0. 5); } else { print MAIL $_; } 50
![print MAIL tmaxctt if avect d print MAIL intavect 0 5 print MAIL "t$max[$ct]t"; if ($ave[$ct] =~ /^d/) { print MAIL int($ave[$ct] + 0. 5);](https://slidetodoc.com/presentation_image_h2/572a39988cfe878832445211d08983be/image-51.jpg)
print MAIL "t$max[$ct]t"; if ($ave[$ct] =~ /^d/) { print MAIL int($ave[$ct] + 0. 5); } else { print MAIL $ave[$ct]; } print MAIL "n"; } # foreach return 1; } # sub send. Mail 51
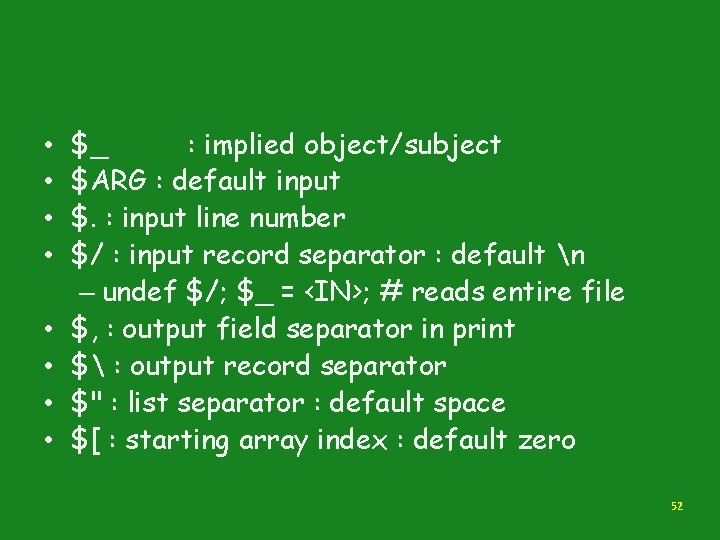
• • $_ : implied object/subject $ARG : default input $. : input line number $/ : input record separator : default n – undef $/; $_ = <IN>; # reads entire file $, : output field separator in print $ : output record separator $" : list separator : default space $[ : starting array index : default zero 52
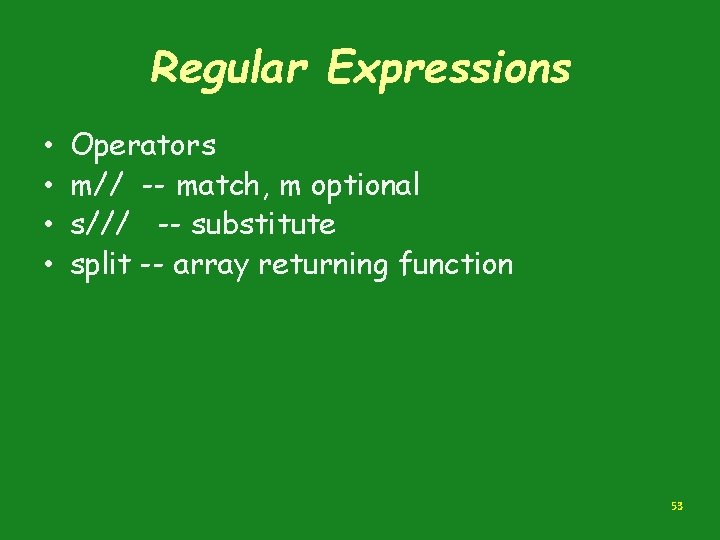
Regular Expressions • • Operators m// -- match, m optional s/// -- substitute split -- array returning function 53
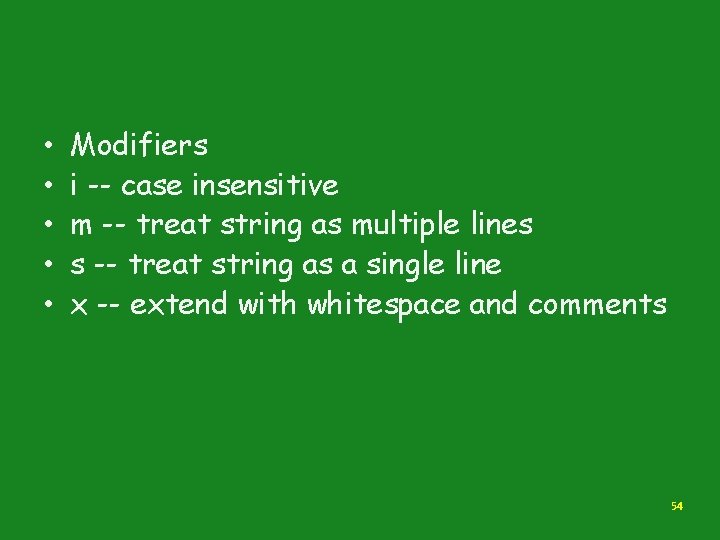
• • • Modifiers i -- case insensitive m -- treat string as multiple lines s -- treat string as a single line x -- extend with whitespace and comments 54
Cps 506
Cps506
Imperative programming languages
506 area
Ustal ile różnych monochloropochodnych
Akta 506
Cs 506
Ece 506 ncsu
Dígito
Wruld examples
Real-time systems and programming languages
Cs 421
Multithreading program in java
Programming languages levels
Introduction to programming languages
Plc coding language
Procedural programming languages
Alternative programming languages
Strongly typed vs weakly typed
Transmission programming languages
Cse 340 principles of programming languages
Types of programming languages
Xenia programming languages
Advantages and disadvantages of programming languages
Mainstream programming languages
Cse 340 principles of programming languages
Programming languages
Programming languages
Programming languages
Programming languages
Tiny programming language
Brief history of programming languages
Lisp_q
Real-time systems and programming languages
Xkcd programming languages comparison
If programming languages were cars
Reasons for studying concepts of programming languages
Cornell programming languages
Low level programming languages
Middle level programming languages
Programming languages flowchart
Cs 421 programming languages and compilers
Multimedia programming languages
Storage management in programming languages
What is imperative statement in assembly language
An imperative statement
Imperative statement in system programming
Hardware description language
Assembly language example
Imperative language
Imperative language
Imperative language
Cps algebra exit exam
Cps in project management
Ipums cps