1 JAVA REVIEW II CISC 6795 Xiaolan Zhang
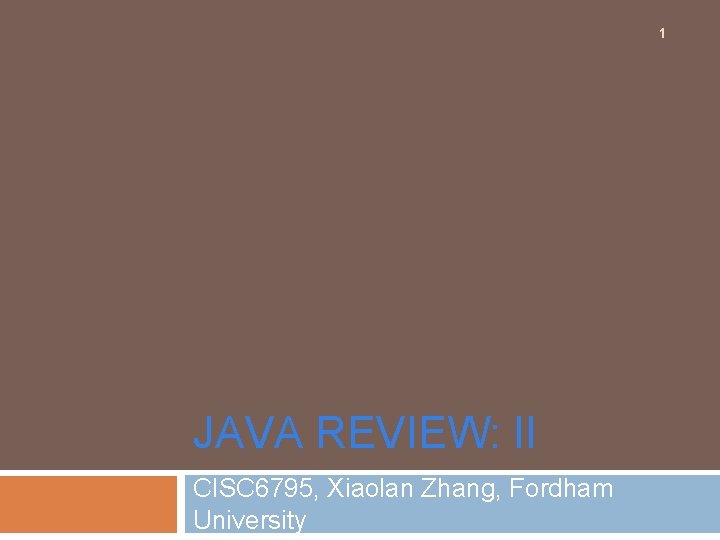
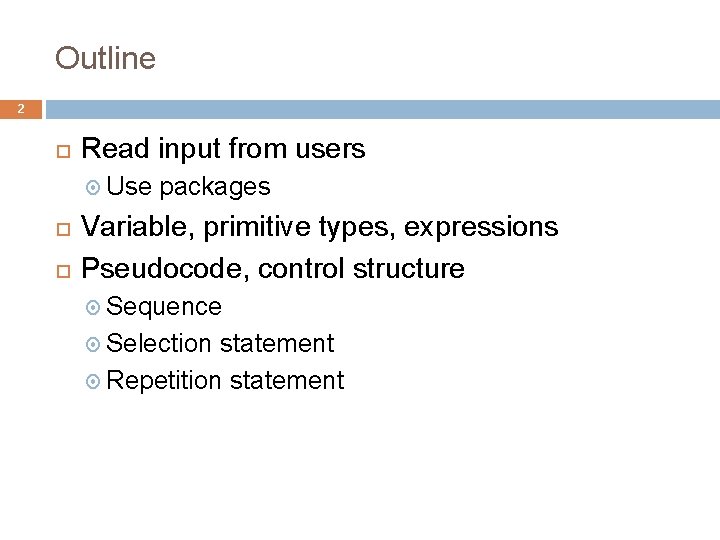
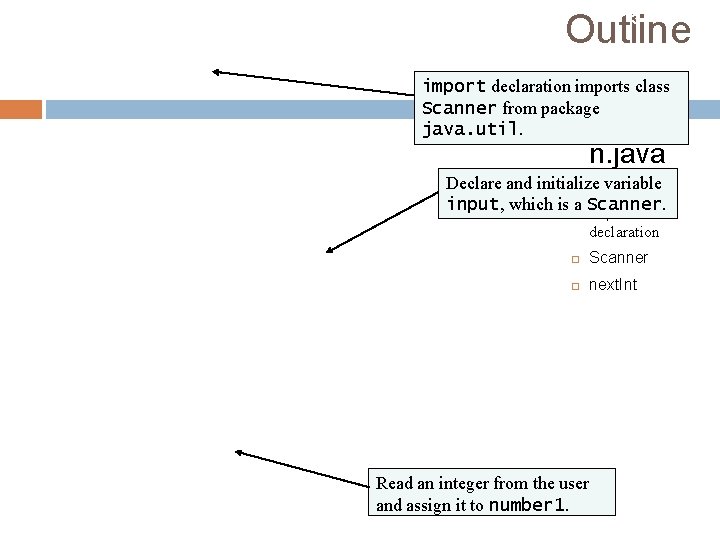
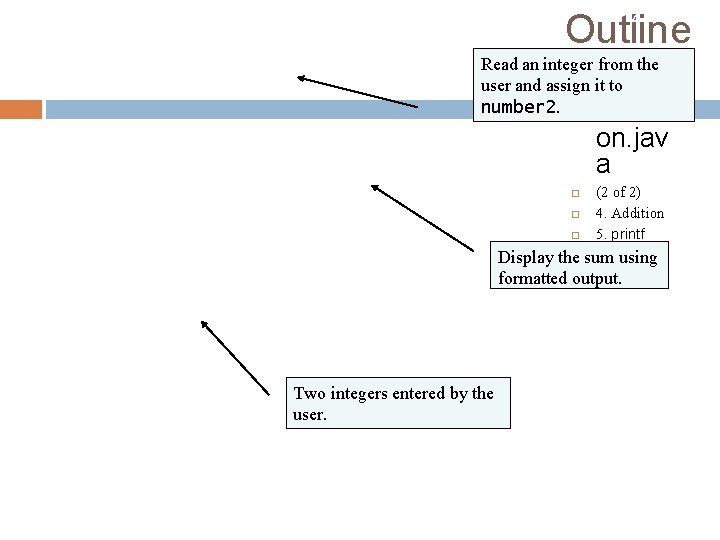
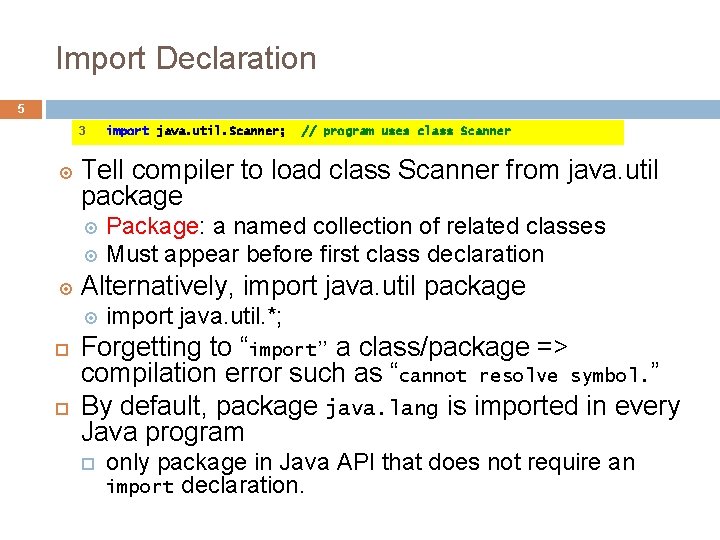
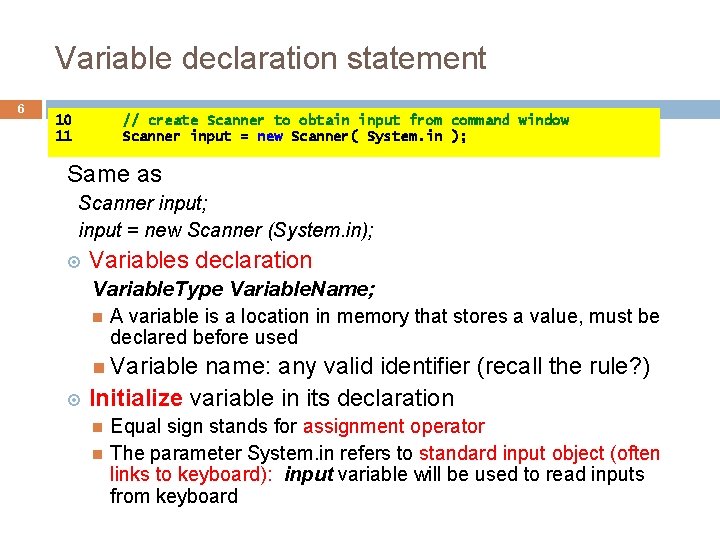
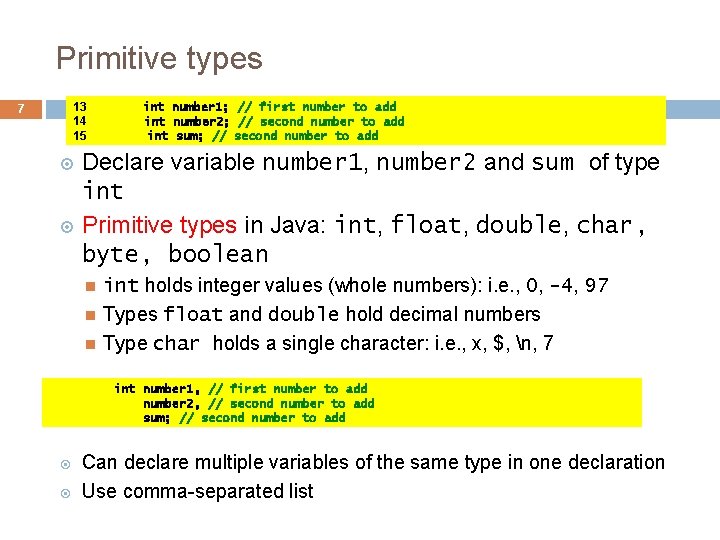
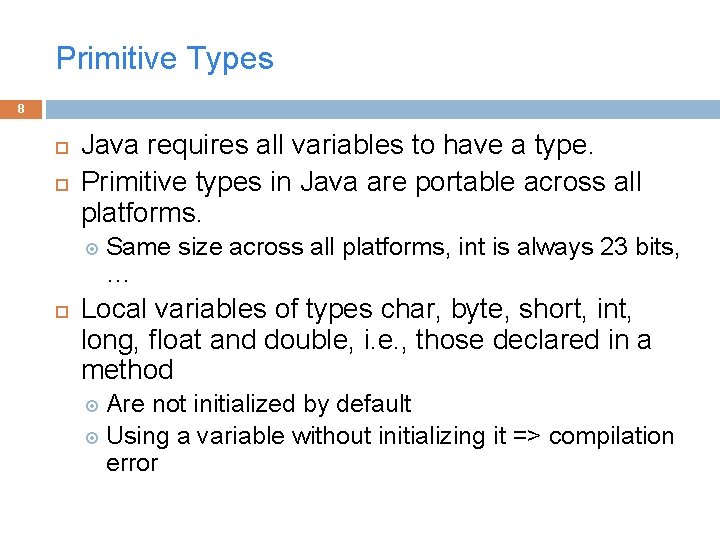
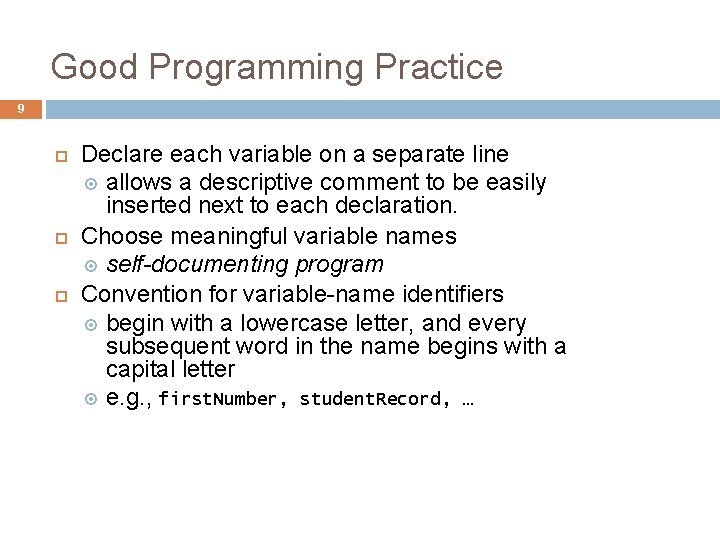
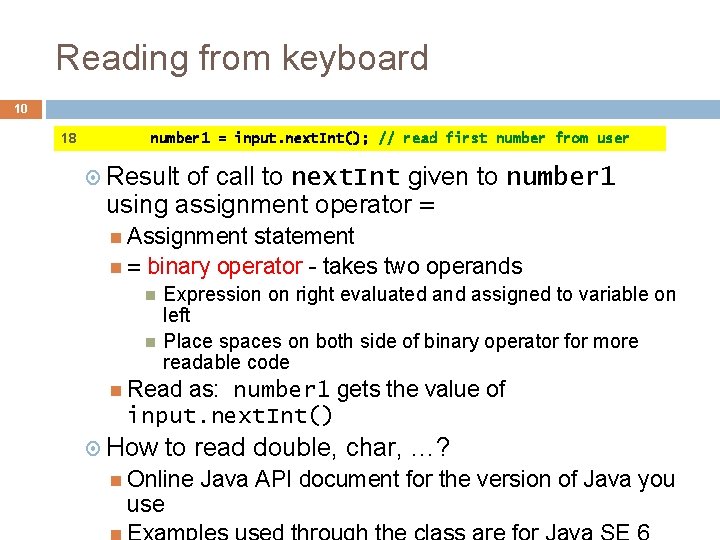
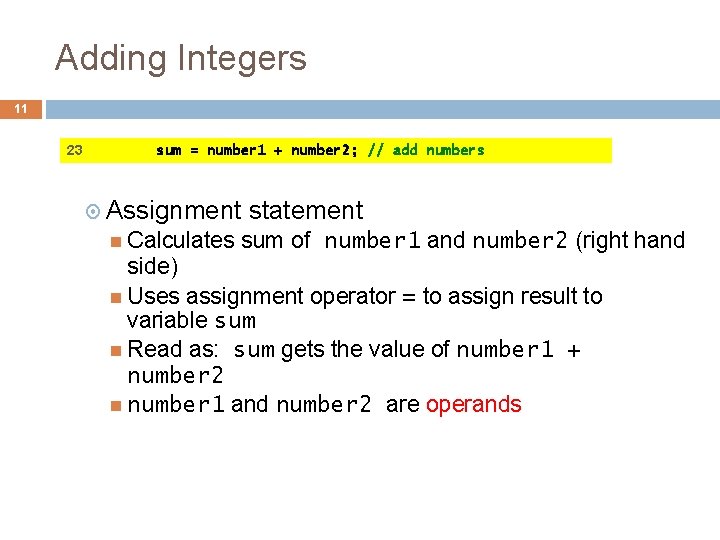
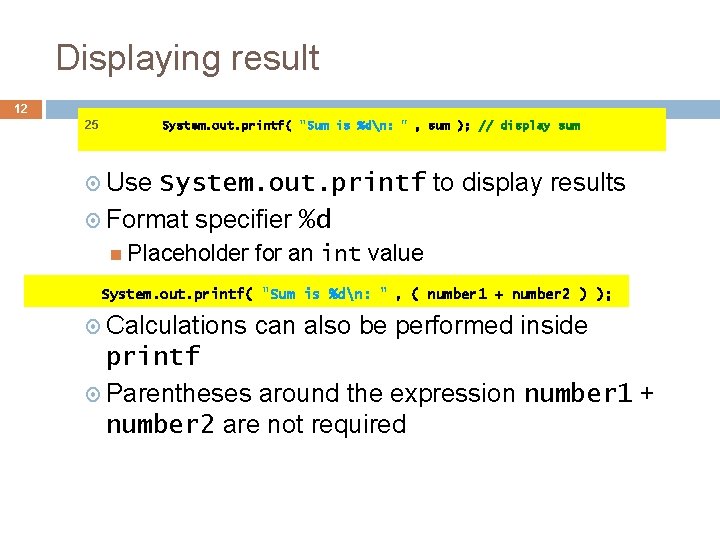
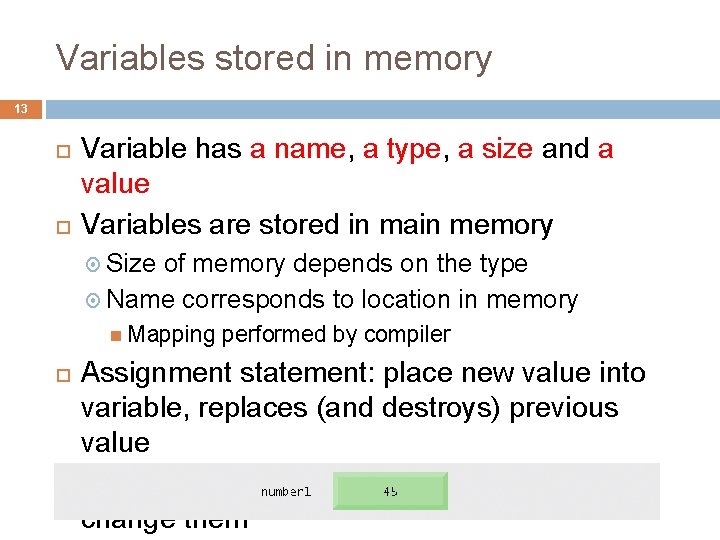
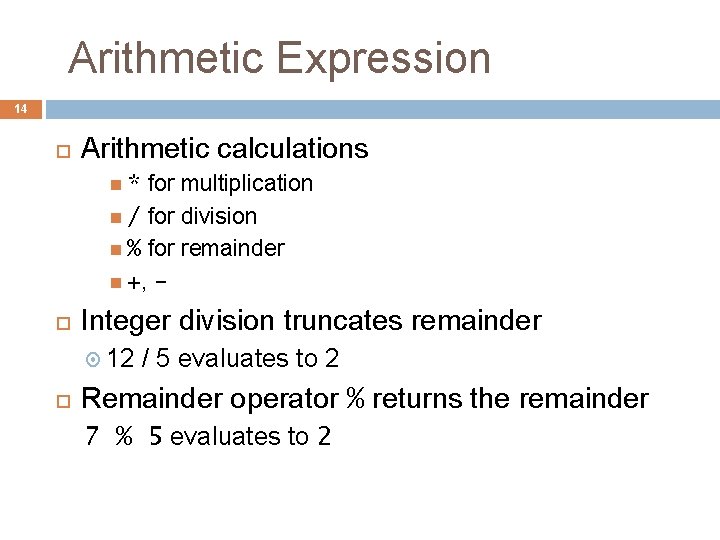
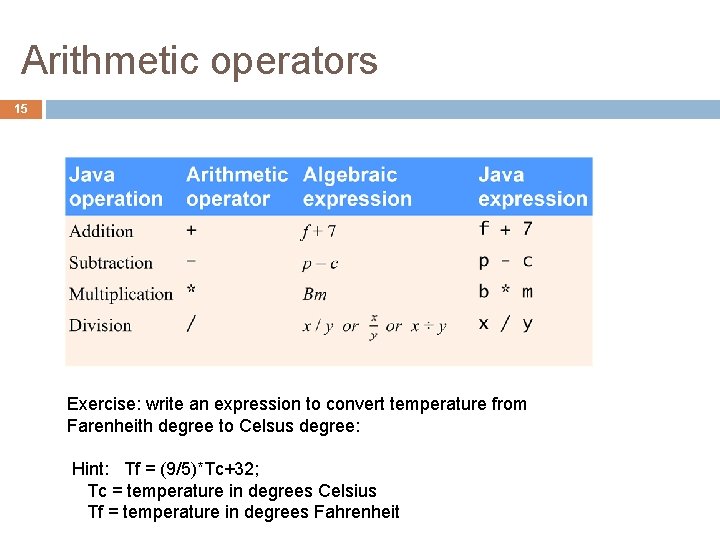
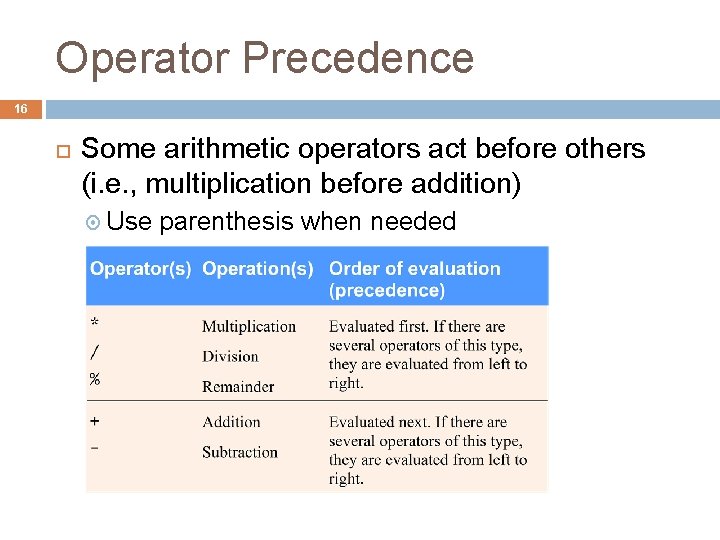
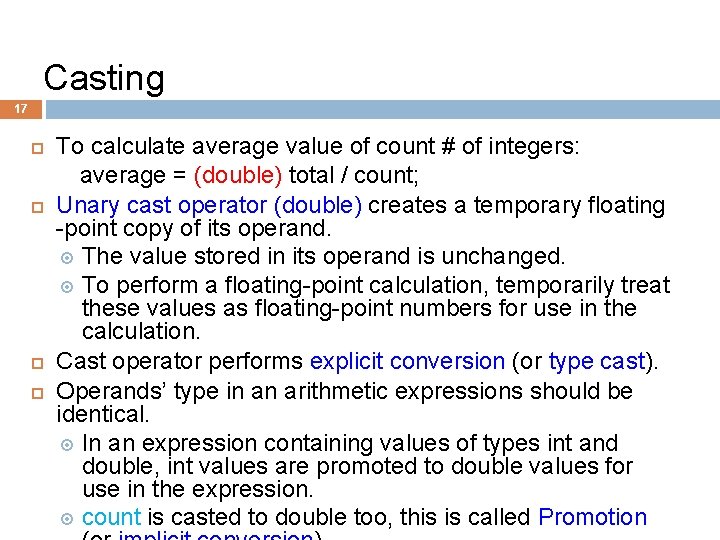
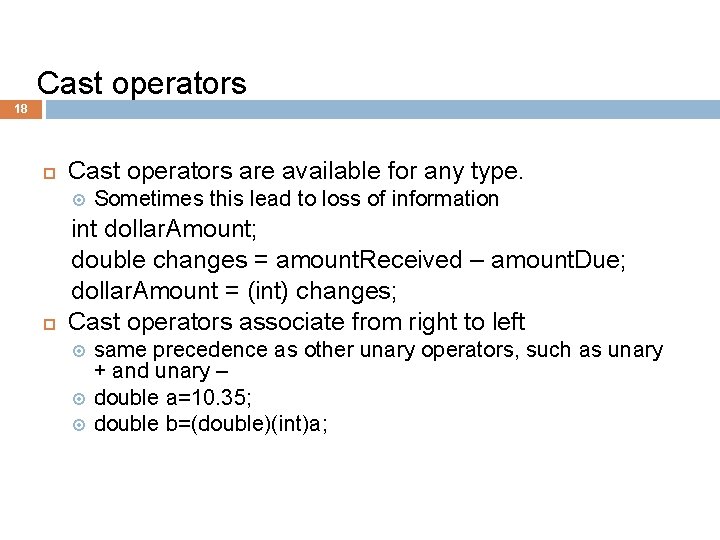
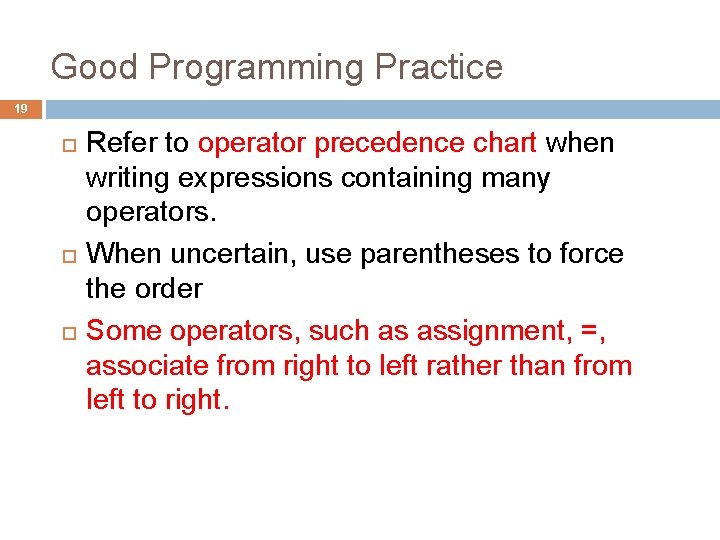
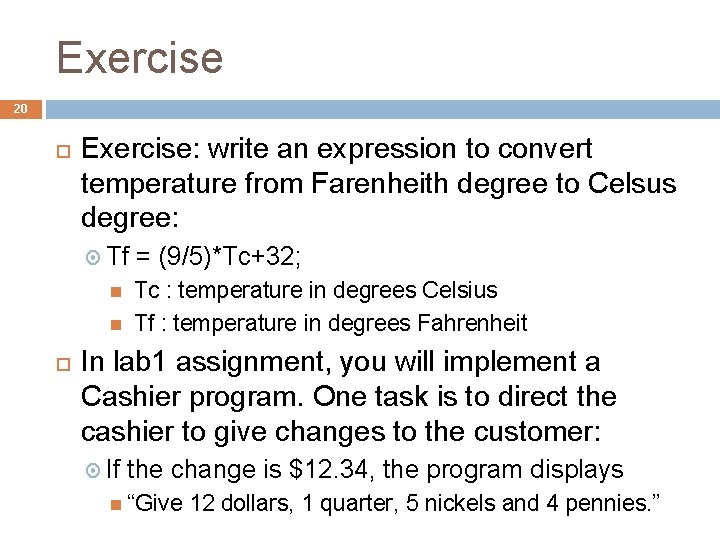
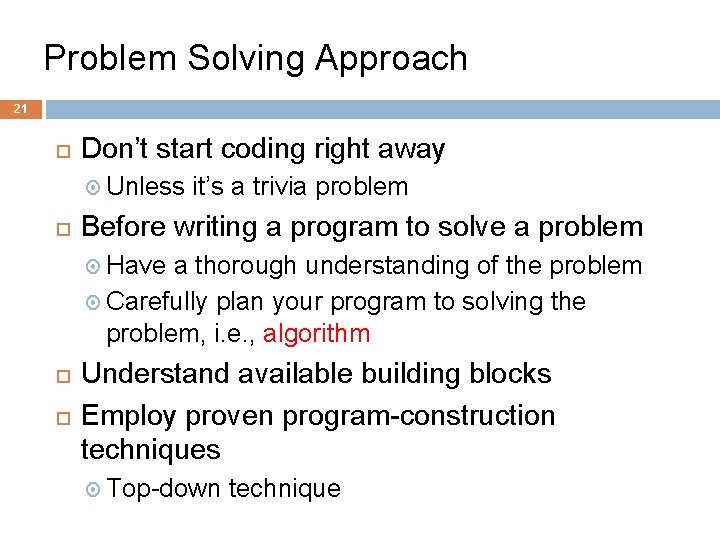
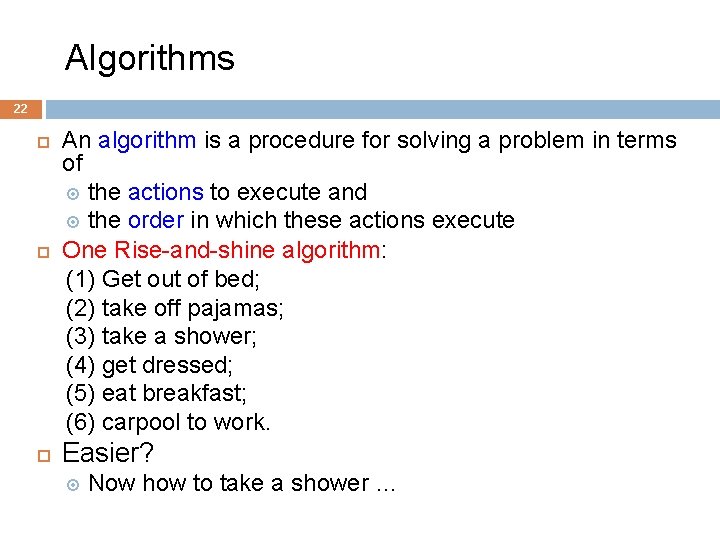
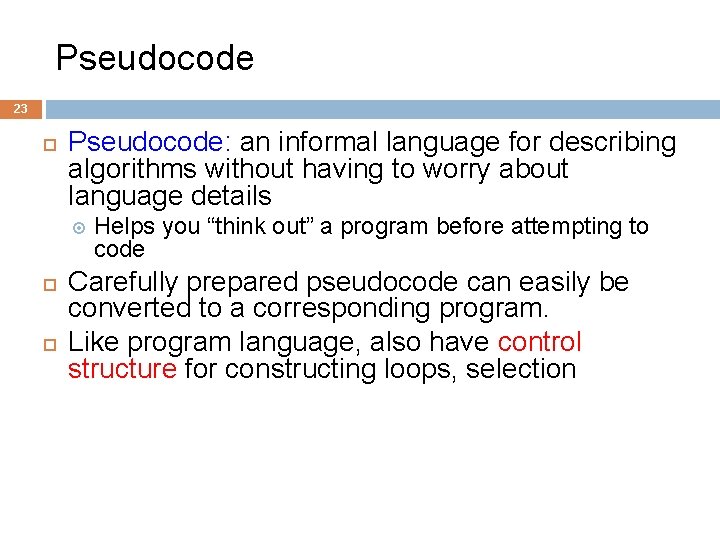
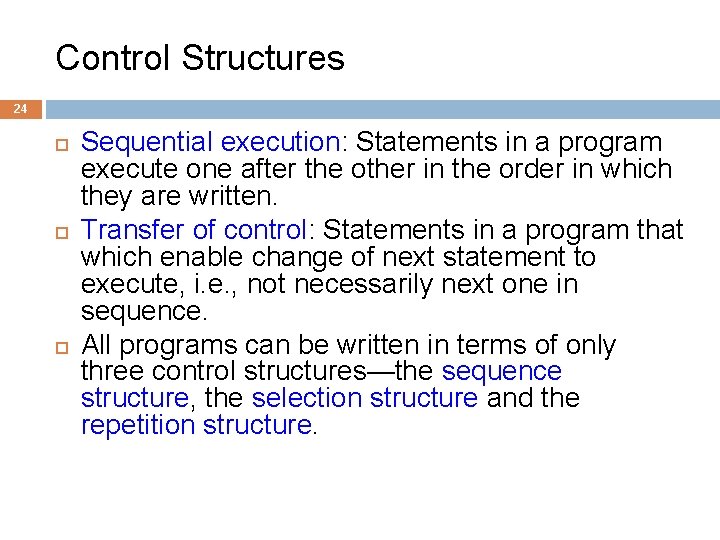
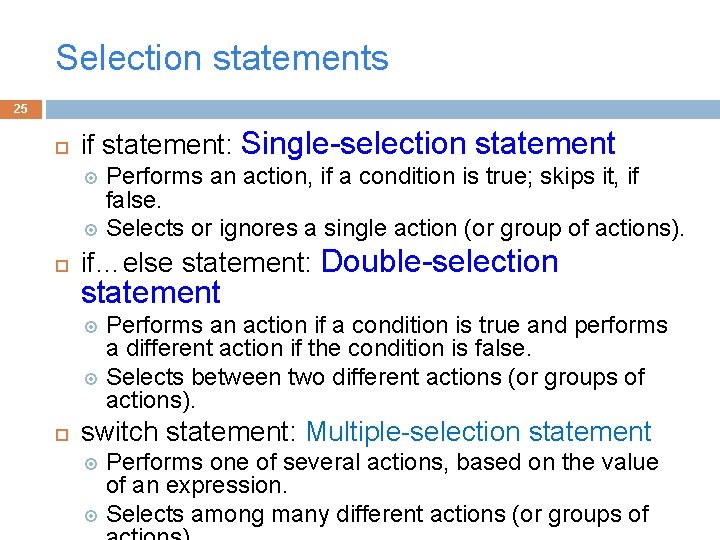
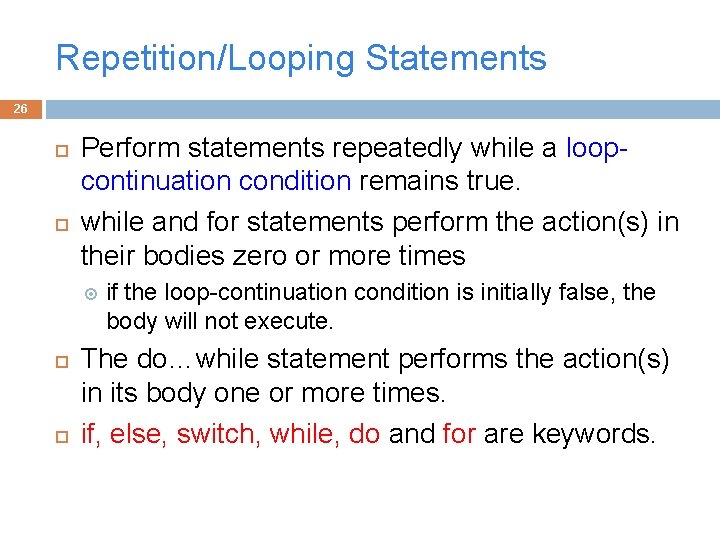
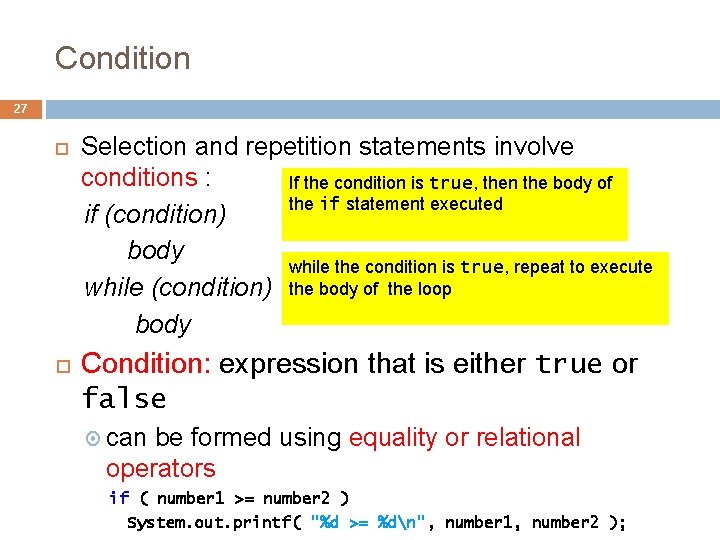
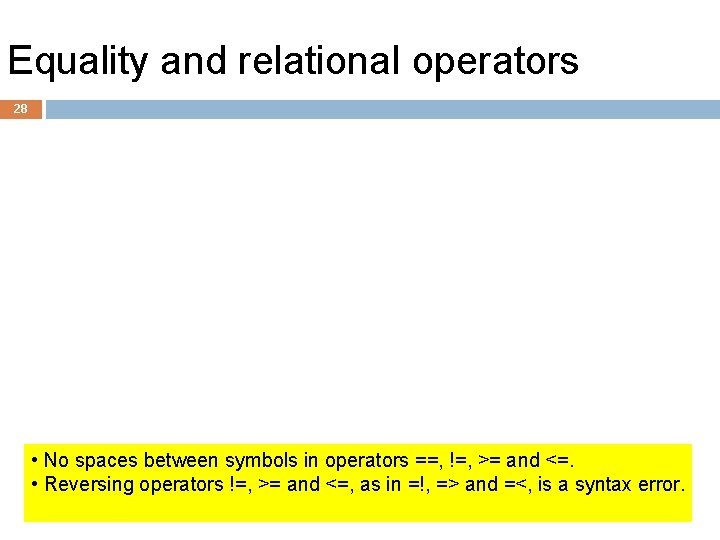
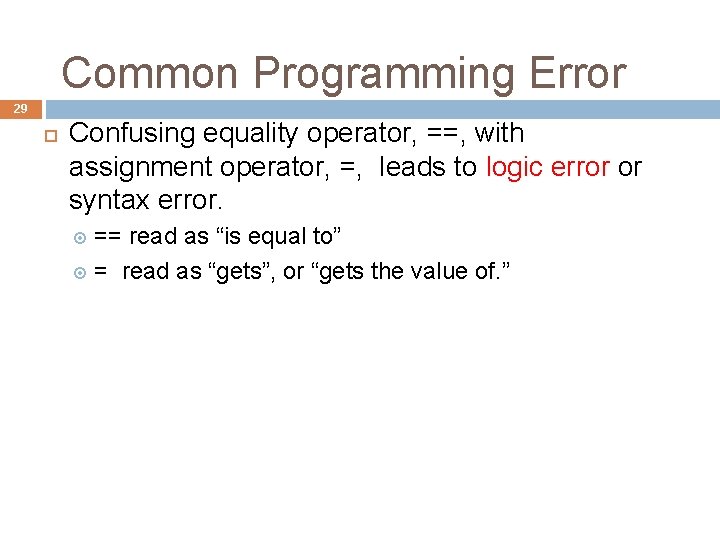
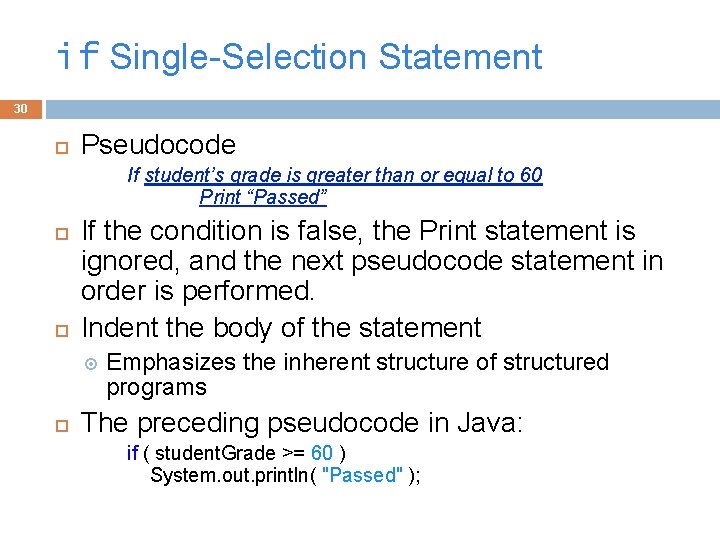
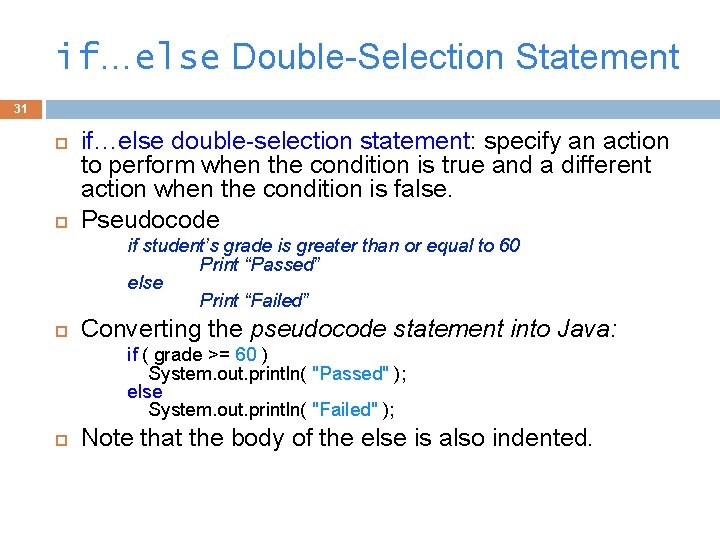
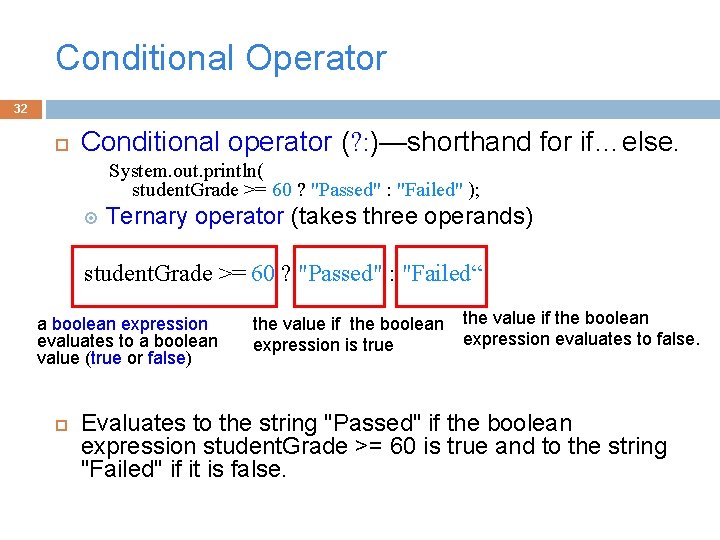
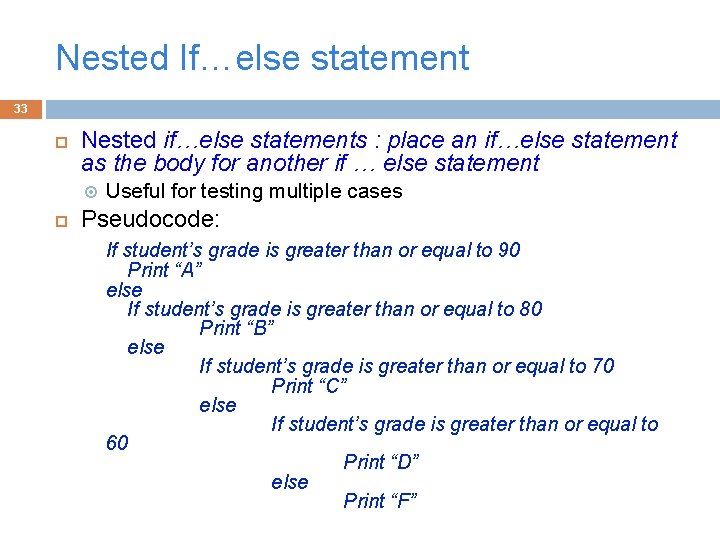
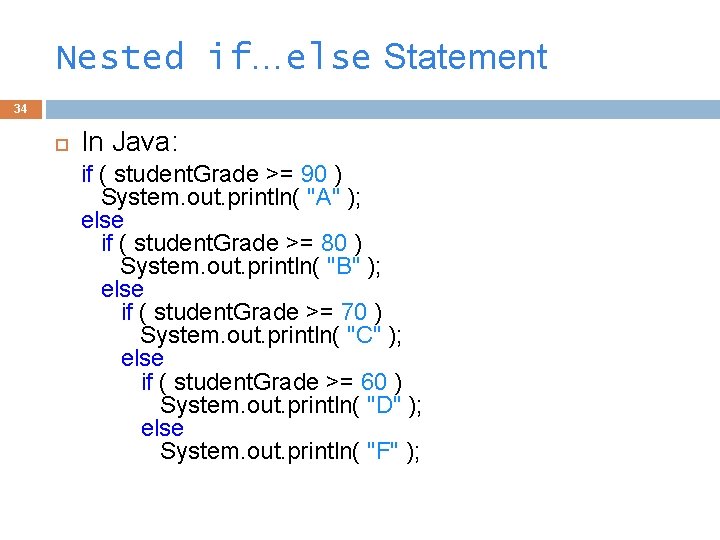
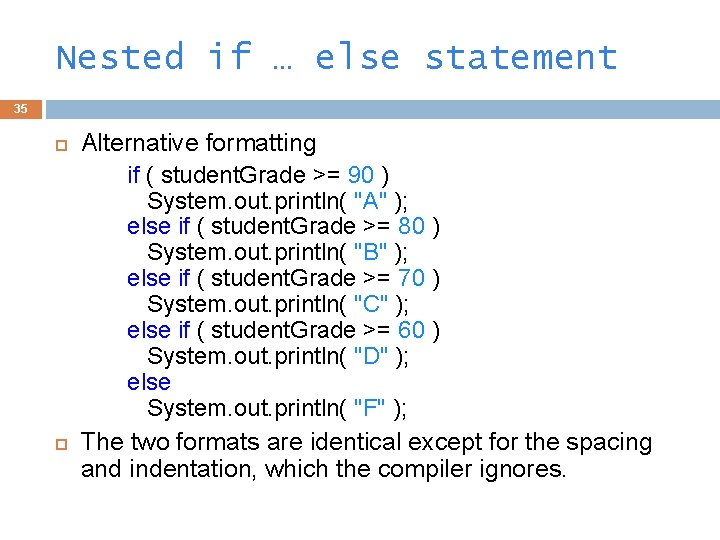
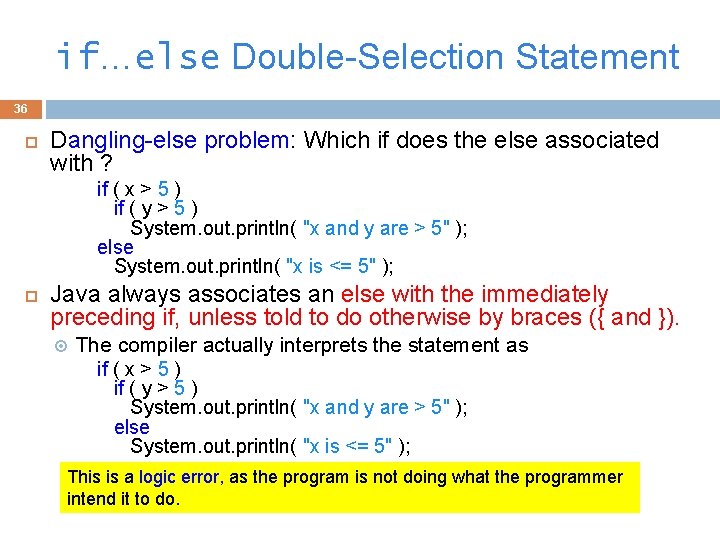
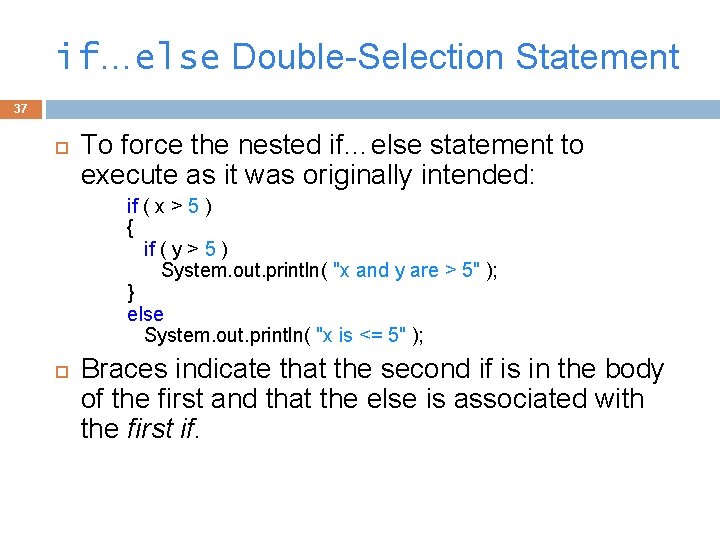
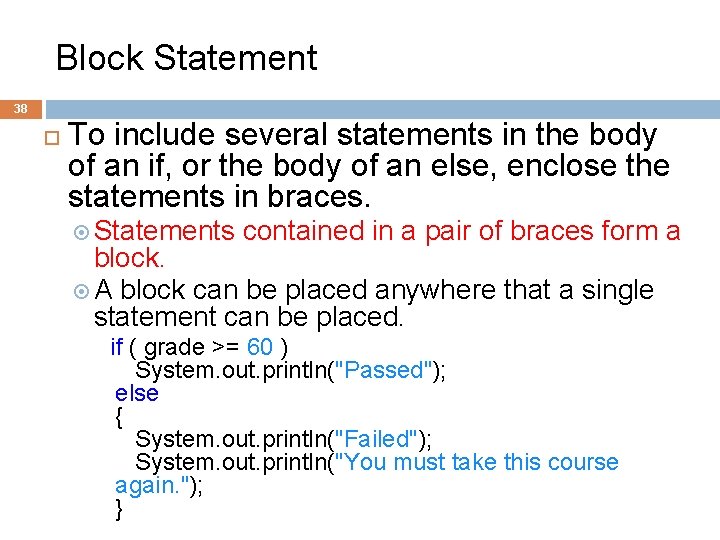
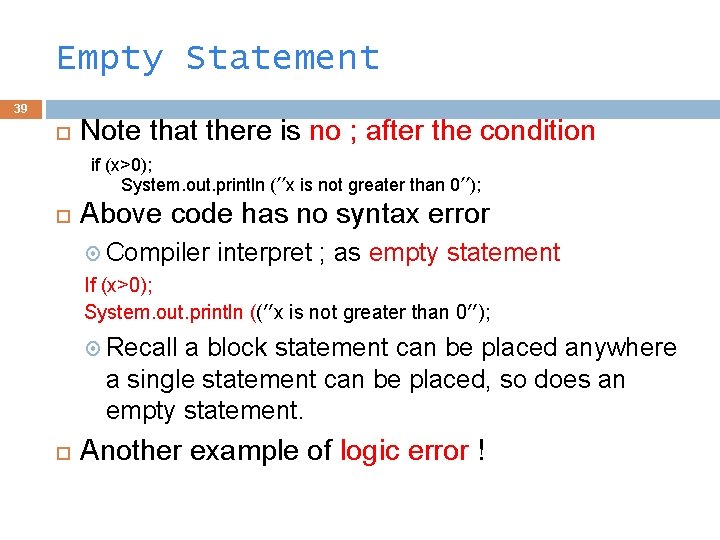
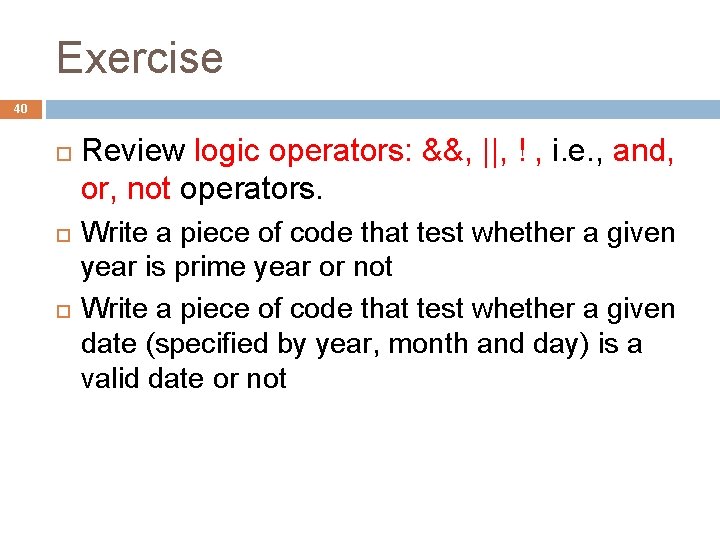
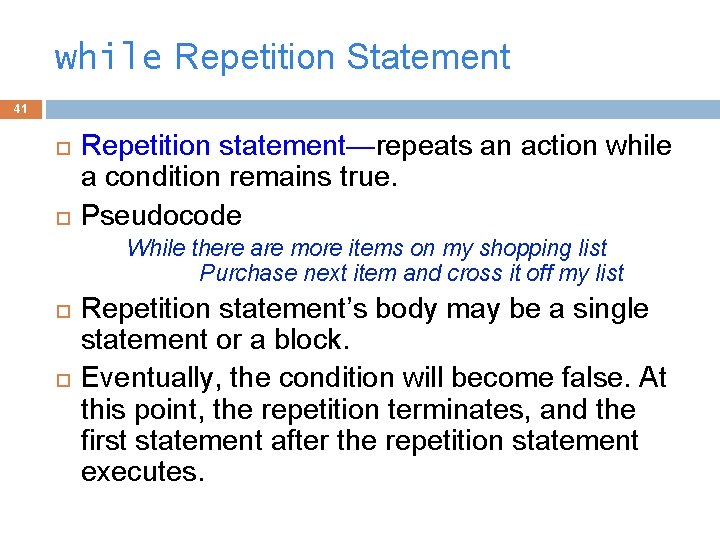
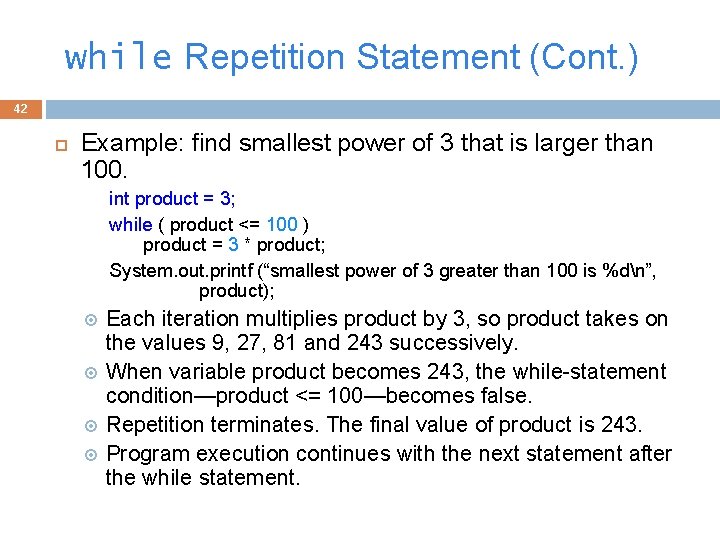
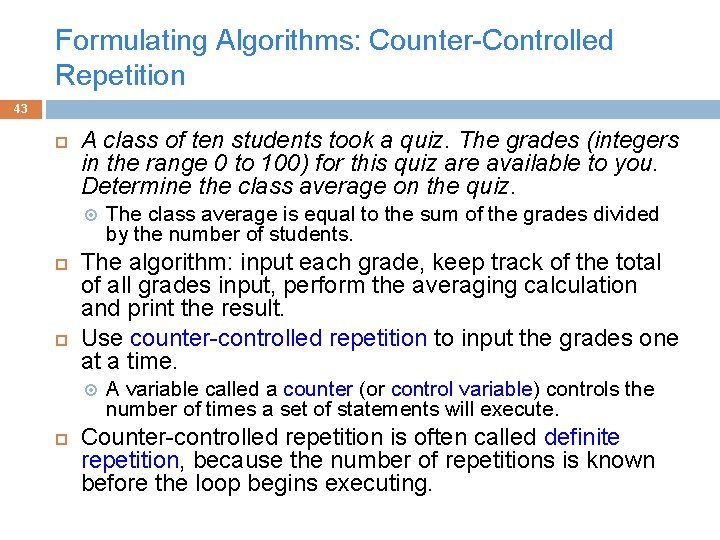
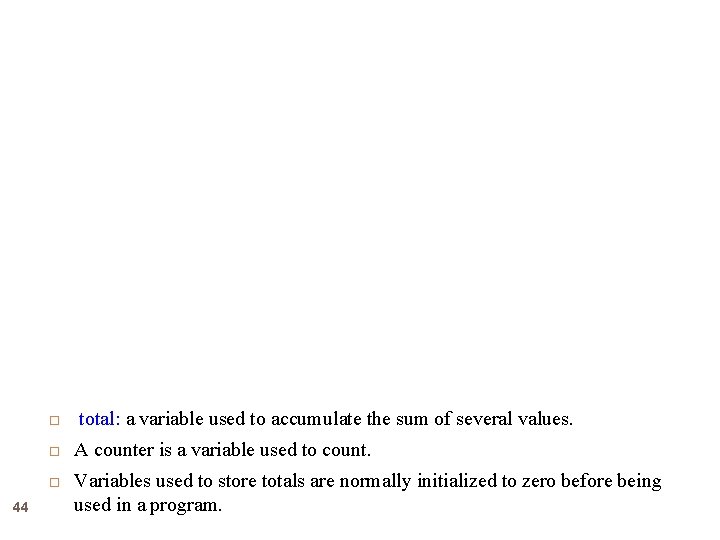
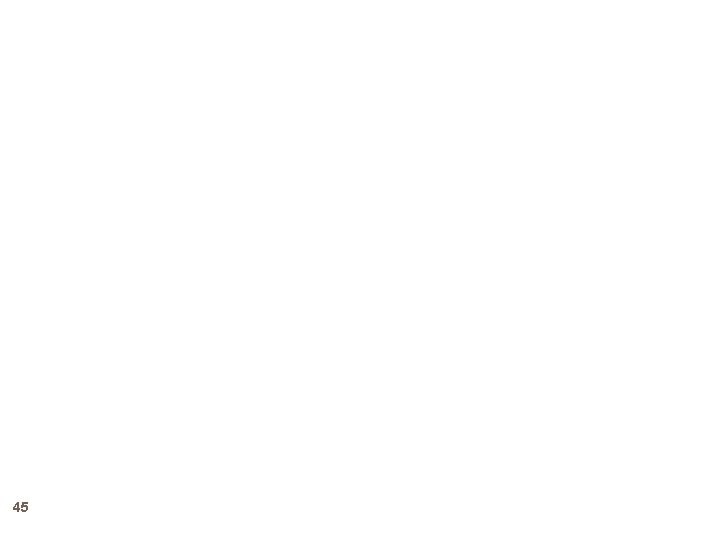
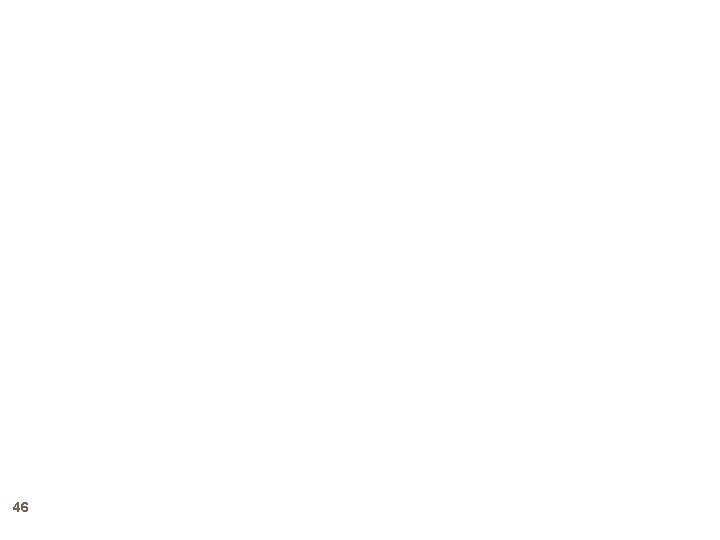
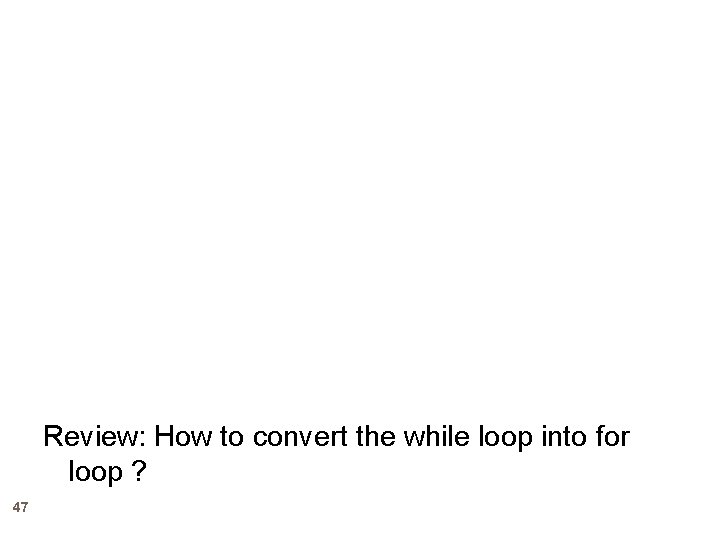
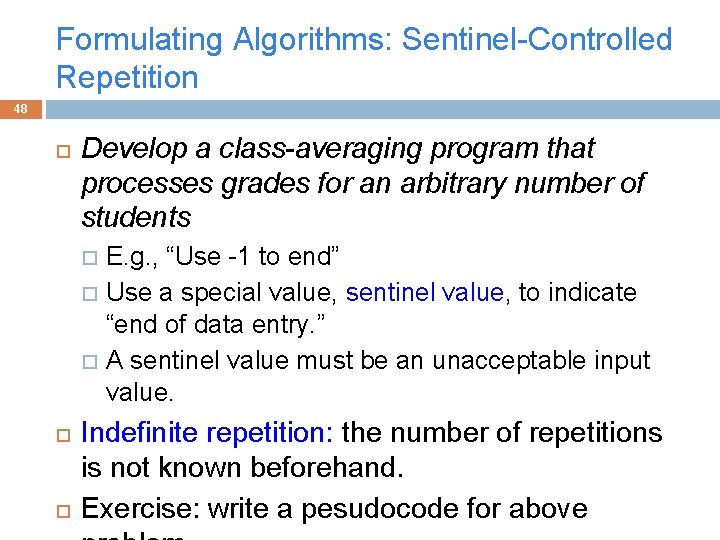
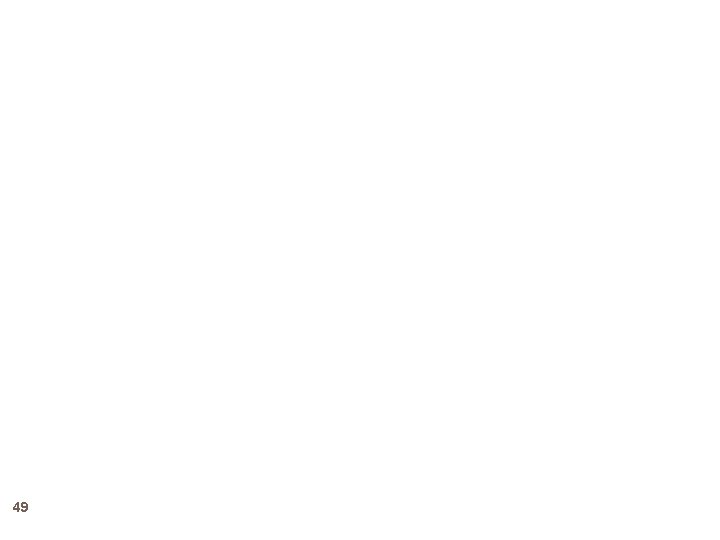
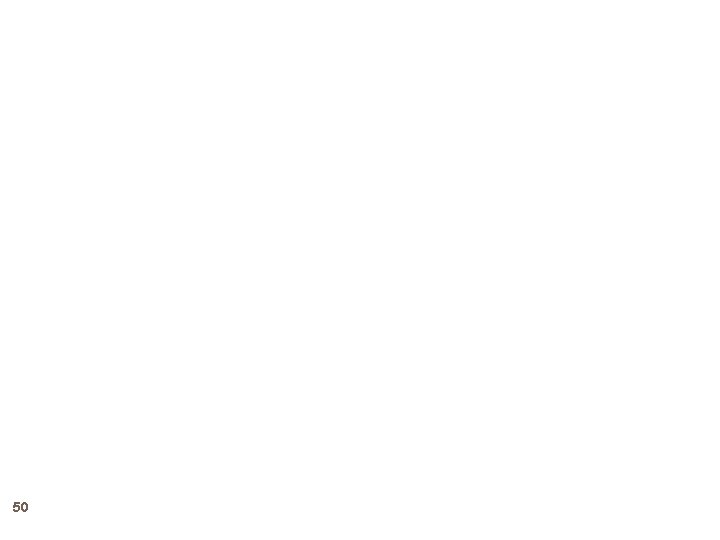
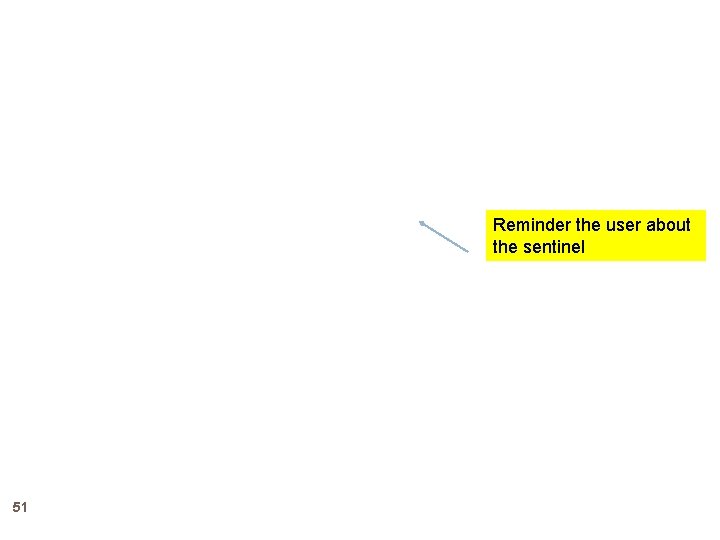
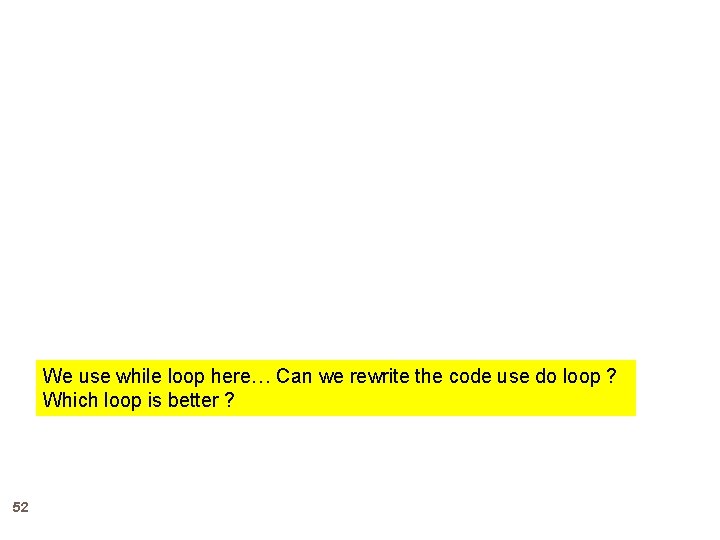
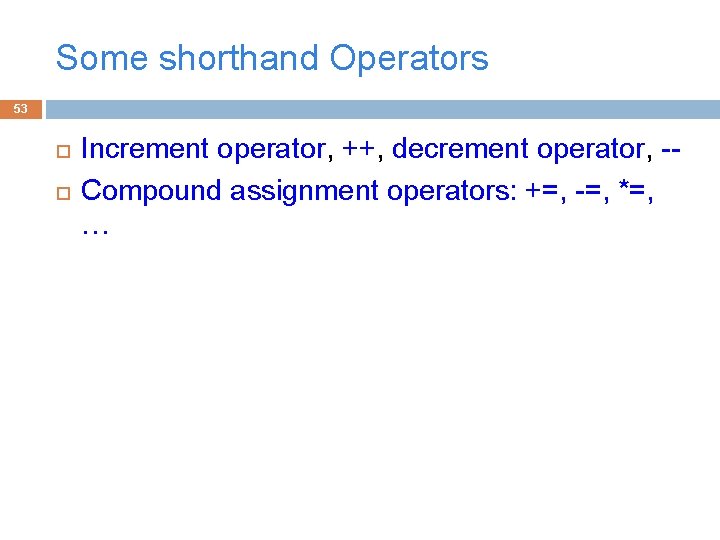
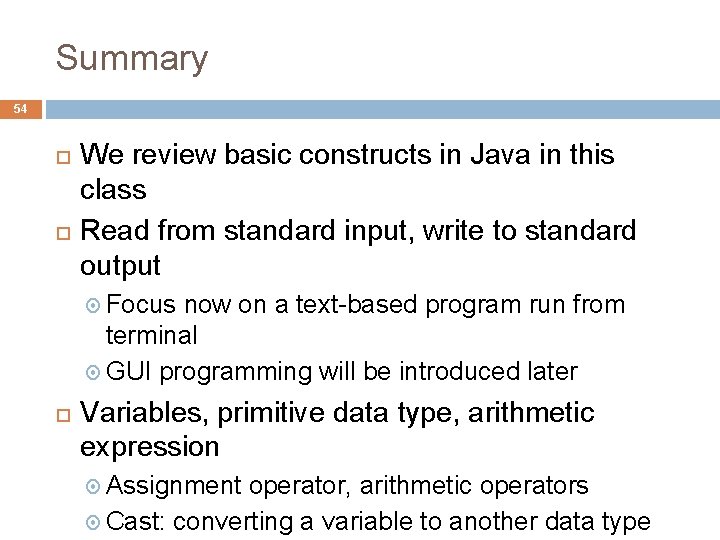
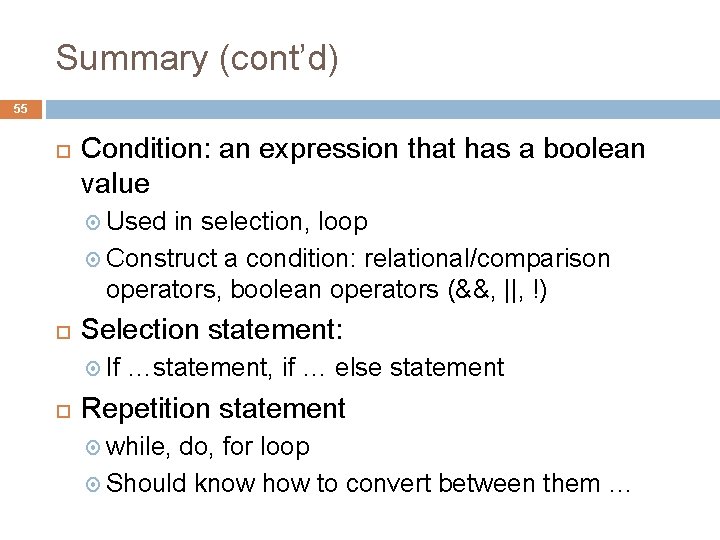
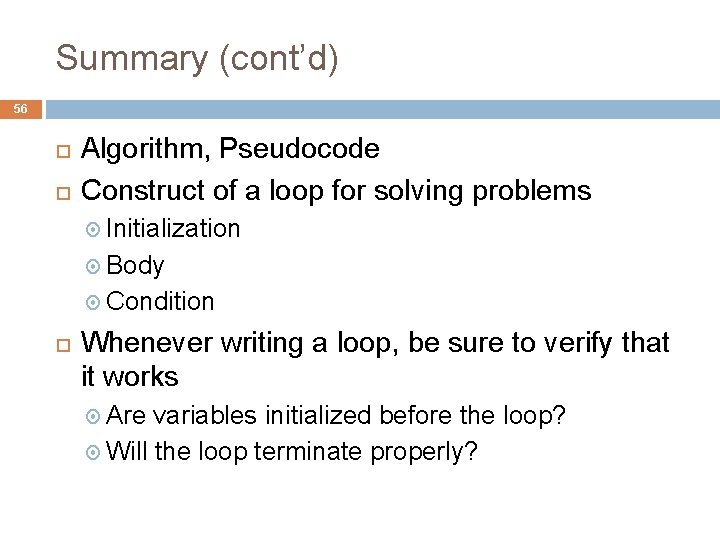
- Slides: 56
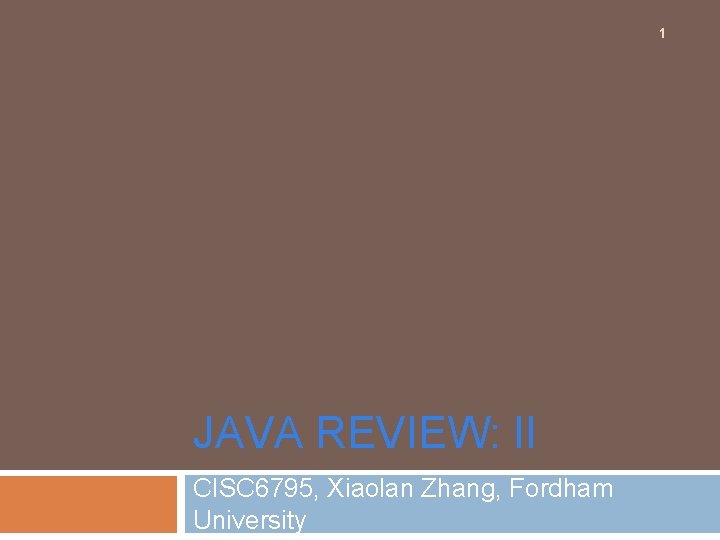
1 JAVA REVIEW: II CISC 6795, Xiaolan Zhang, Fordham University
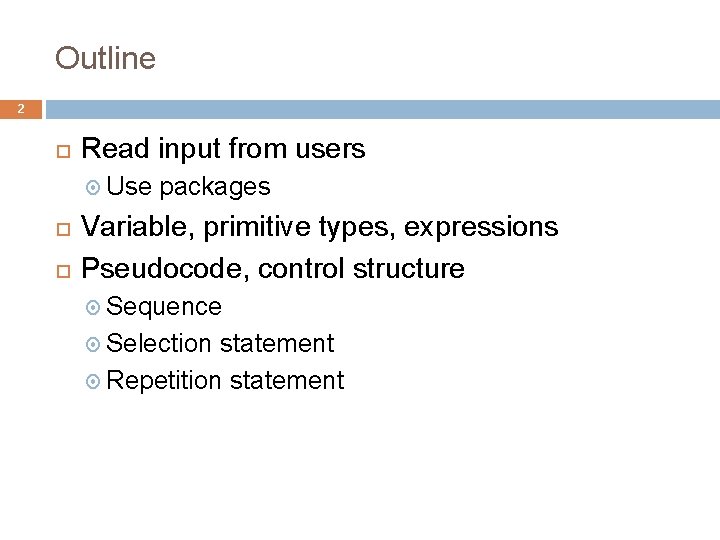
Outline 2 Read input from users Use packages Variable, primitive types, expressions Pseudocode, control structure Sequence Selection statement Repetition statement
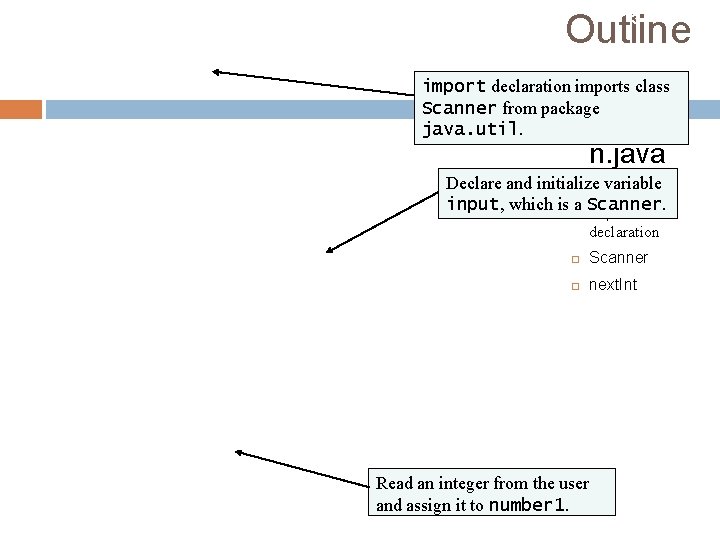
Outline 3 import declaration imports class Scanner from package java. util. Additio n. java Declare and initialize (1 variable of 2) input, which is a Scanner. import declaration Scanner next. Int Read an integer from the user and assign it to number 1.
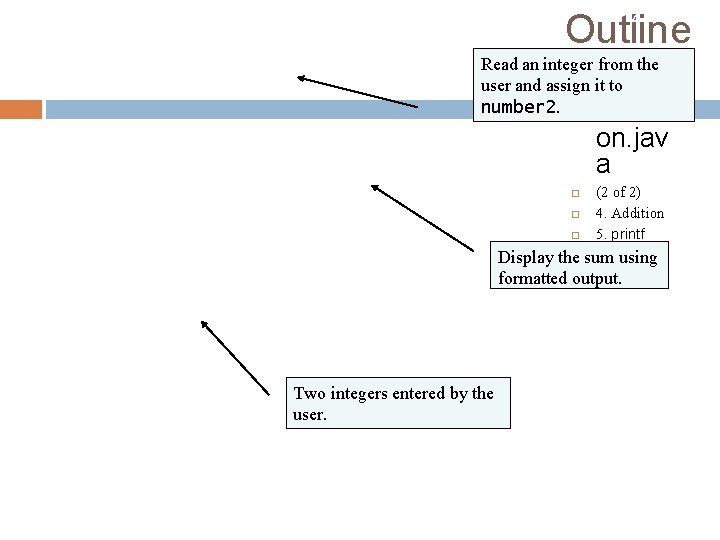
Outline 4 Read an integer from the user and assign it to number 2. Additi on. jav a (2 of 2) 4. Addition 5. printf Display the sum using formatted output. Two integers entered by the user.
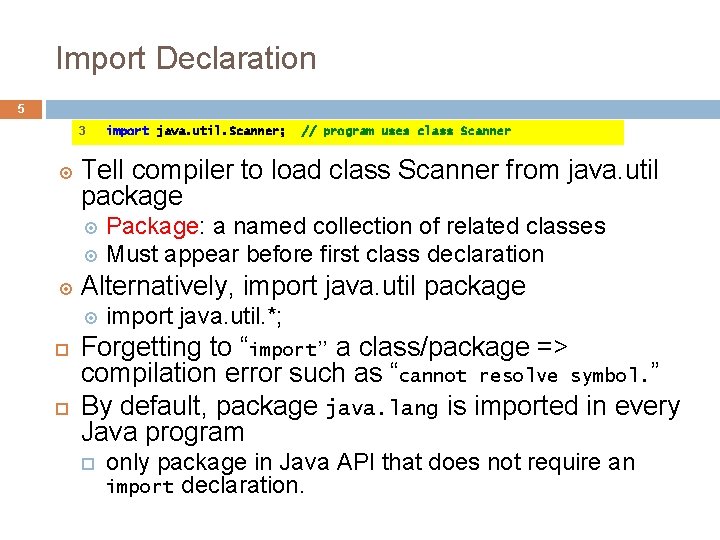
Import Declaration 5 3 import java. util. Scanner; // program uses class Scanner Tell compiler to load class Scanner from java. util package Package: a named collection of related classes Must appear before first class declaration Alternatively, import java. util package import java. util. *; Forgetting to “import” a class/package => compilation error such as “cannot resolve symbol. ” By default, package java. lang is imported in every Java program only package in Java API that does not require an import declaration.
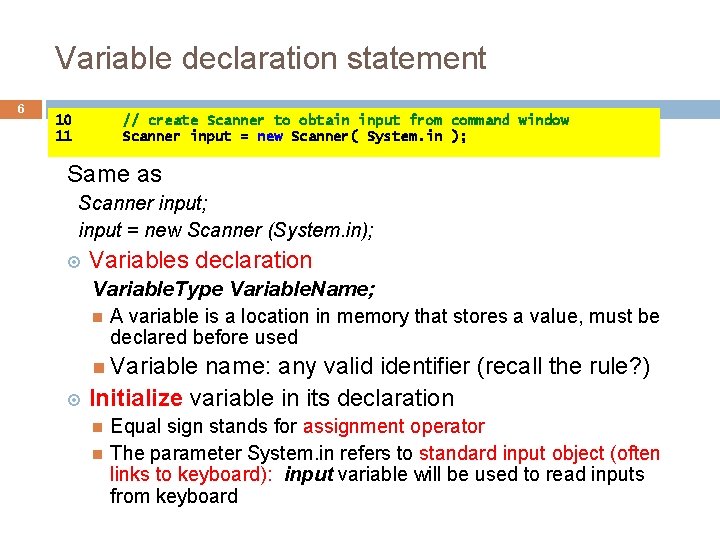
Variable declaration statement 6 10 11 // create Scanner to obtain input from command window Scanner input = new Scanner( System. in ); Same as Scanner input; input = new Scanner (System. in); Variables declaration Variable. Type Variable. Name; A variable is a location in memory that stores a value, must be declared before used Variable name: any valid identifier (recall the rule? ) Initialize variable in its declaration Equal sign stands for assignment operator The parameter System. in refers to standard input object (often links to keyboard): input variable will be used to read inputs from keyboard
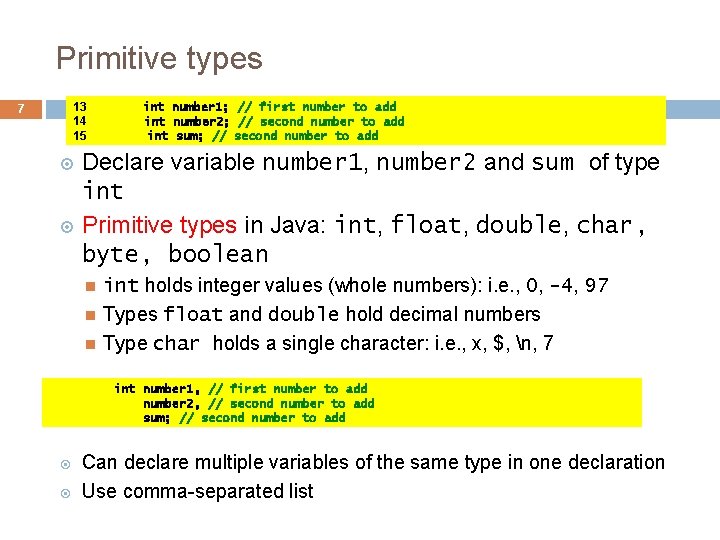
Primitive types 13 14 15 7 int number 1; // first number to add int number 2; // second number to add int sum; // second number to add Declare variable number 1, number 2 and sum of type int Primitive types in Java: int, float, double, char, byte, boolean int holds integer values (whole numbers): i. e. , 0, -4, 97 Types float and double hold decimal numbers Type char holds a single character: i. e. , x, $, n, 7 int number 1, // first number to add number 2, // second number to add sum; // second number to add Can declare multiple variables of the same type in one declaration Use comma-separated list
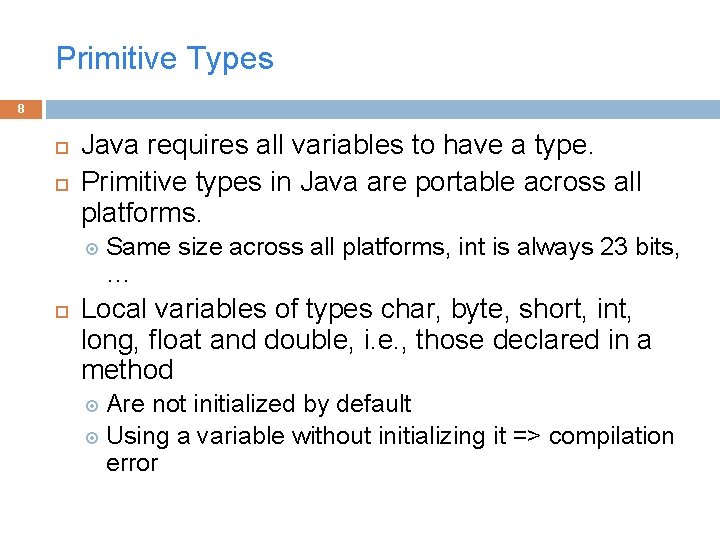
Primitive Types 8 Java requires all variables to have a type. Primitive types in Java are portable across all platforms. Same size across all platforms, int is always 23 bits, … Local variables of types char, byte, short, int, long, float and double, i. e. , those declared in a method Are not initialized by default Using a variable without initializing it => compilation error
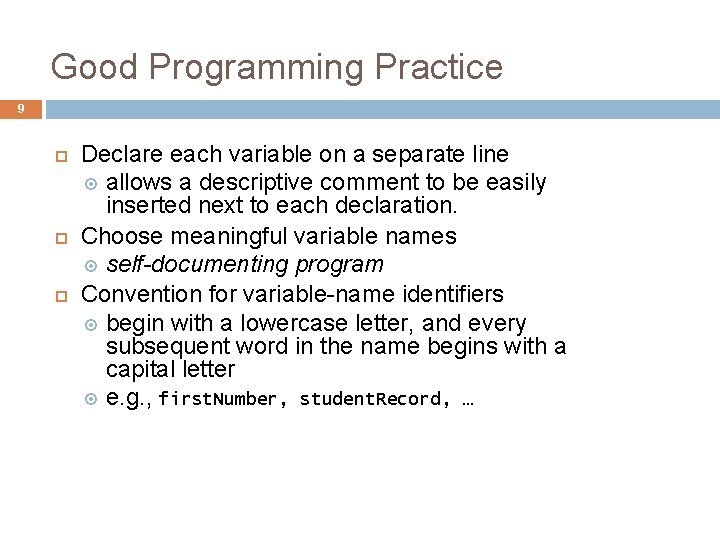
Good Programming Practice 9 Declare each variable on a separate line allows a descriptive comment to be easily inserted next to each declaration. Choose meaningful variable names self-documenting program Convention for variable-name identifiers begin with a lowercase letter, and every subsequent word in the name begins with a capital letter e. g. , first. Number, student. Record, …
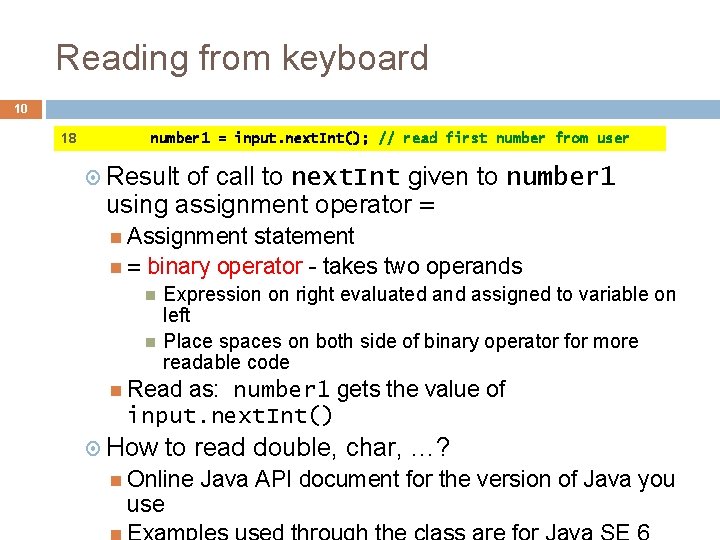
Reading from keyboard 10 18 number 1 = input. next. Int(); // read first number from user Result of call to next. Int given to number 1 using assignment operator = Assignment statement = binary operator - takes two operands Expression on right evaluated and assigned to variable on left Place spaces on both side of binary operator for more readable code Read as: number 1 gets the value of input. next. Int() How to read double, char, …? Online Java API document for the version of Java you use
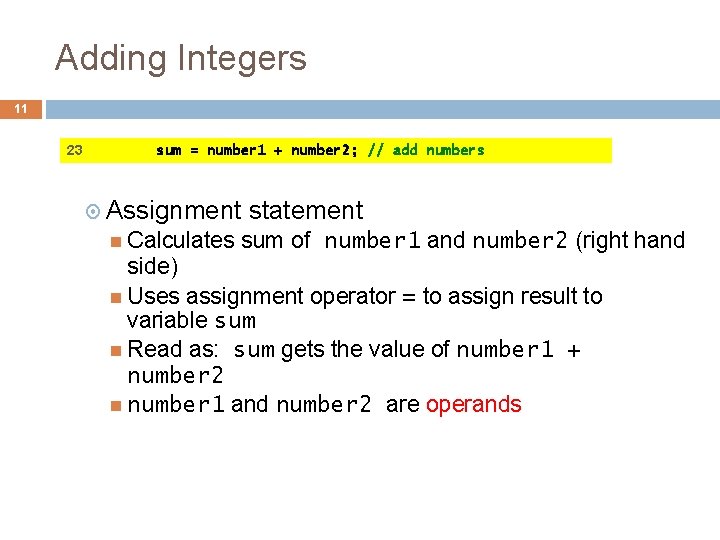
Adding Integers 11 23 sum = number 1 + number 2; // add numbers Assignment statement Calculates sum of number 1 and number 2 (right hand side) Uses assignment operator = to assign result to variable sum Read as: sum gets the value of number 1 + number 2 number 1 and number 2 are operands
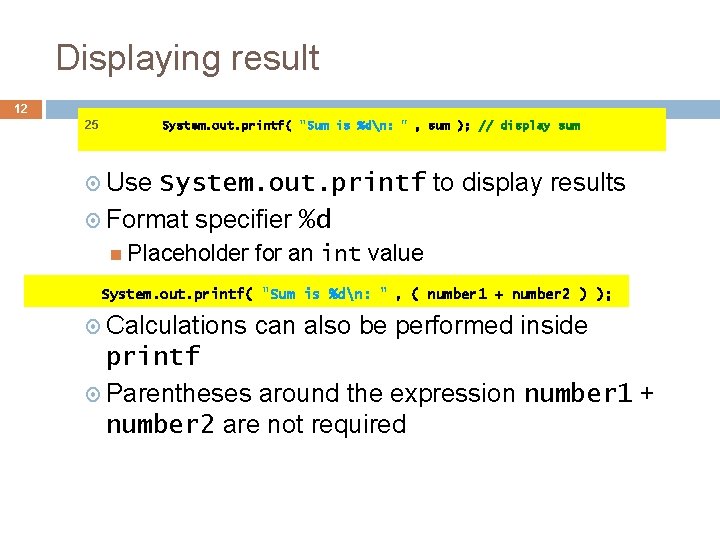
Displaying result 12 25 System. out. printf( "Sum is %dn: " , sum ); // display sum Use System. out. printf to display results Format specifier %d Placeholder for an int value System. out. printf( "Sum is %dn: " , ( number 1 + number 2 ) ); Calculations can also be performed inside printf Parentheses around the expression number 1 + number 2 are not required
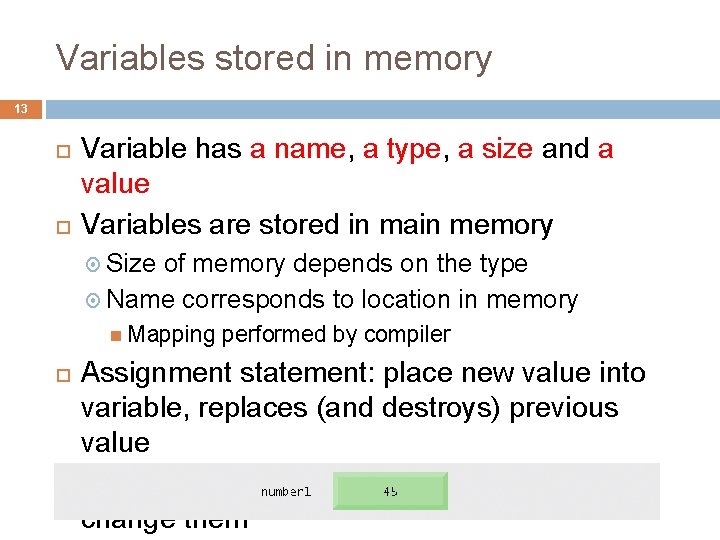
Variables stored in memory 13 Variable has a name, a type, a size and a value Variables are stored in main memory Size of memory depends on the type Name corresponds to location in memory Mapping performed by compiler Assignment statement: place new value into variable, replaces (and destroys) previous value Reading variables from memory does not change them
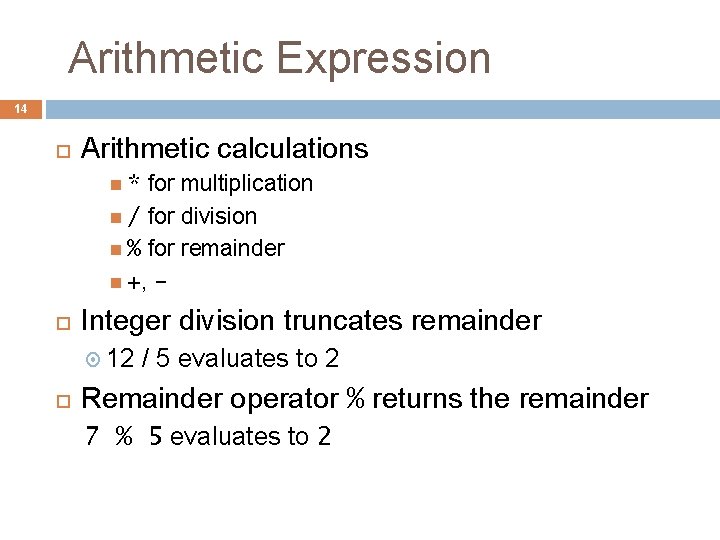
Arithmetic Expression 14 Arithmetic calculations * for multiplication / for division % for remainder +, - Integer division truncates remainder 12 / 5 evaluates to 2 Remainder operator % returns the remainder 7 % 5 evaluates to 2
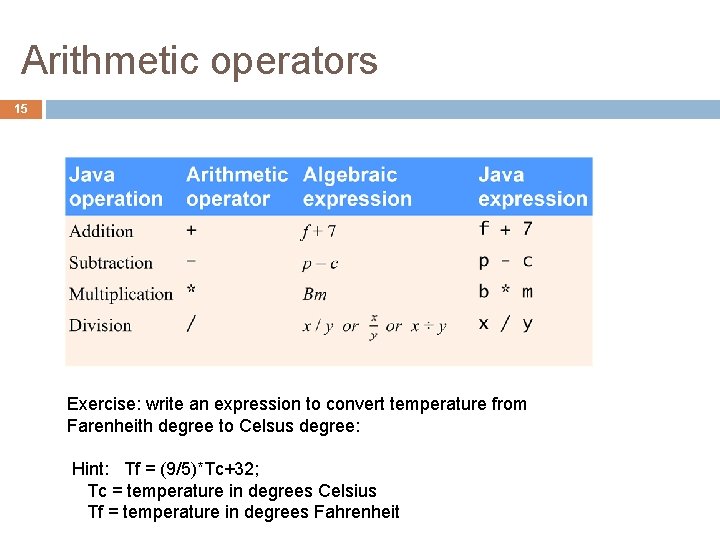
Arithmetic operators 15 Exercise: write an expression to convert temperature from Farenheith degree to Celsus degree: Hint: Tf = (9/5)*Tc+32; Tc = temperature in degrees Celsius Tf = temperature in degrees Fahrenheit
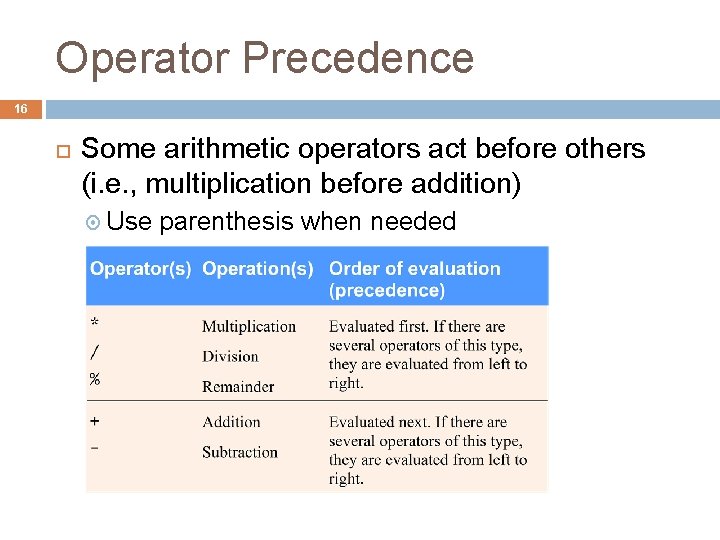
Operator Precedence 16 Some arithmetic operators act before others (i. e. , multiplication before addition) Use parenthesis when needed
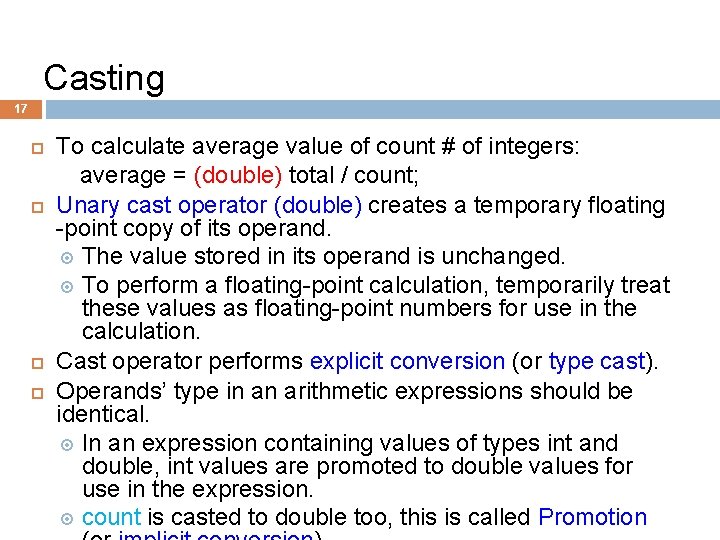
Casting 17 To calculate average value of count # of integers: average = (double) total / count; Unary cast operator (double) creates a temporary floating -point copy of its operand. The value stored in its operand is unchanged. To perform a floating-point calculation, temporarily treat these values as floating-point numbers for use in the calculation. Cast operator performs explicit conversion (or type cast). Operands’ type in an arithmetic expressions should be identical. In an expression containing values of types int and double, int values are promoted to double values for use in the expression. count is casted to double too, this is called Promotion
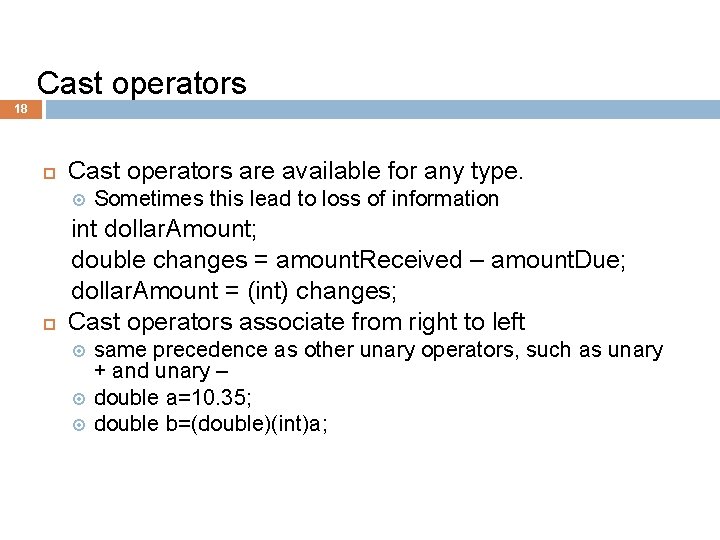
Cast operators 18 Cast operators are available for any type. Sometimes this lead to loss of information int dollar. Amount; double changes = amount. Received – amount. Due; dollar. Amount = (int) changes; Cast operators associate from right to left same precedence as other unary operators, such as unary + and unary – double a=10. 35; double b=(double)(int)a;
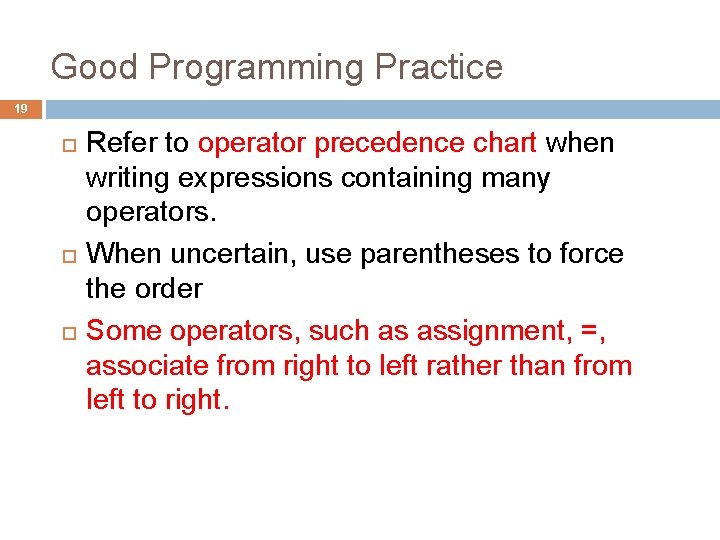
Good Programming Practice 19 Refer to operator precedence chart when writing expressions containing many operators. When uncertain, use parentheses to force the order Some operators, such as assignment, =, associate from right to left rather than from left to right.
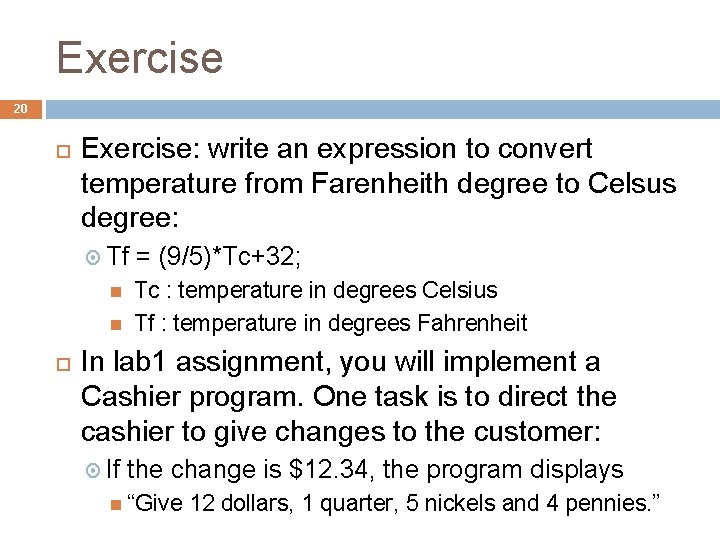
Exercise 20 Exercise: write an expression to convert temperature from Farenheith degree to Celsus degree: Tf = (9/5)*Tc+32; Tc : temperature in degrees Celsius Tf : temperature in degrees Fahrenheit In lab 1 assignment, you will implement a Cashier program. One task is to direct the cashier to give changes to the customer: If the change is $12. 34, the program displays “Give 12 dollars, 1 quarter, 5 nickels and 4 pennies. ”
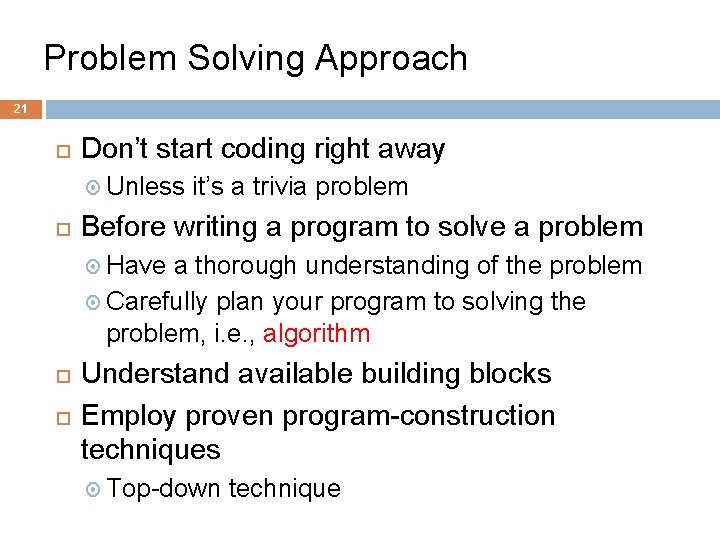
Problem Solving Approach 21 Don’t start coding right away Unless it’s a trivia problem Before writing a program to solve a problem Have a thorough understanding of the problem Carefully plan your program to solving the problem, i. e. , algorithm Understand available building blocks Employ proven program-construction techniques Top-down technique
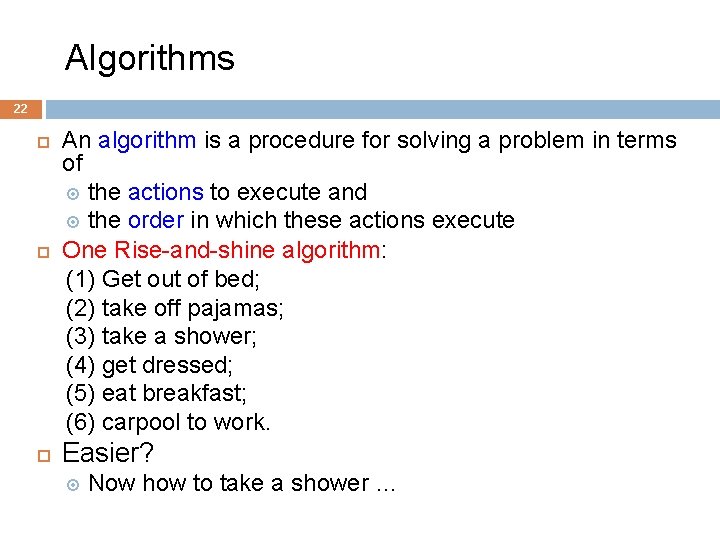
Algorithms 22 An algorithm is a procedure for solving a problem in terms of the actions to execute and the order in which these actions execute One Rise-and-shine algorithm: (1) Get out of bed; (2) take off pajamas; (3) take a shower; (4) get dressed; (5) eat breakfast; (6) carpool to work. Easier? Now how to take a shower …
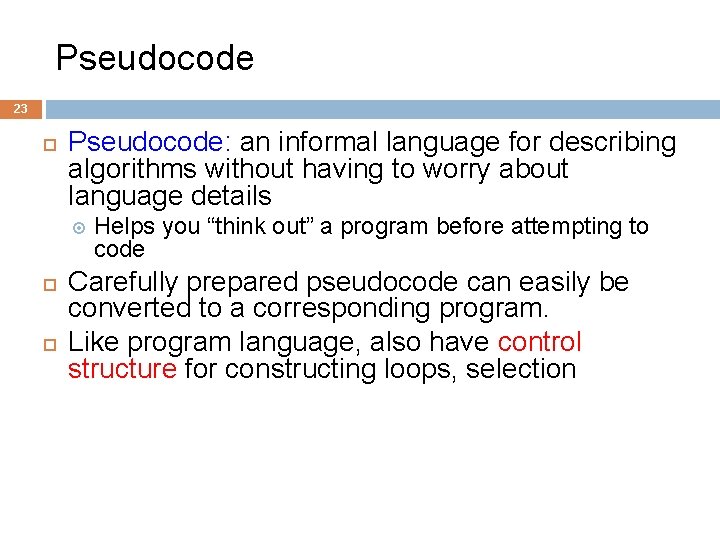
Pseudocode 23 Pseudocode: an informal language for describing algorithms without having to worry about language details Helps you “think out” a program before attempting to code Carefully prepared pseudocode can easily be converted to a corresponding program. Like program language, also have control structure for constructing loops, selection
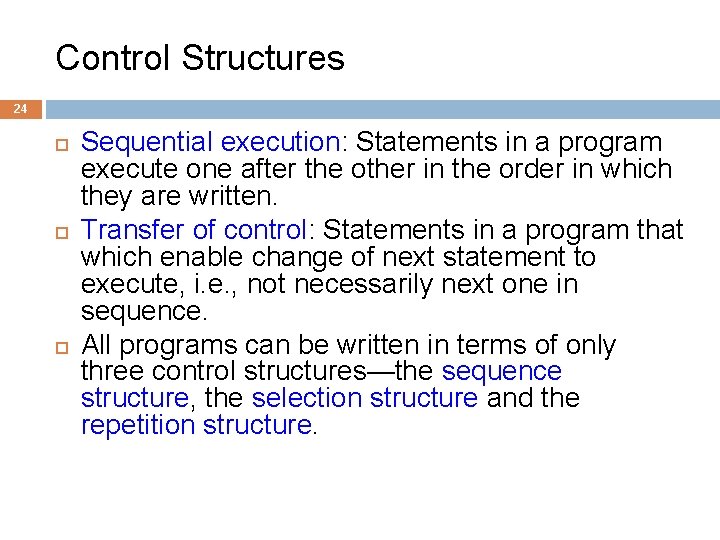
Control Structures 24 Sequential execution: Statements in a program execute one after the other in the order in which they are written. Transfer of control: Statements in a program that which enable change of next statement to execute, i. e. , not necessarily next one in sequence. All programs can be written in terms of only three control structures—the sequence structure, the selection structure and the repetition structure.
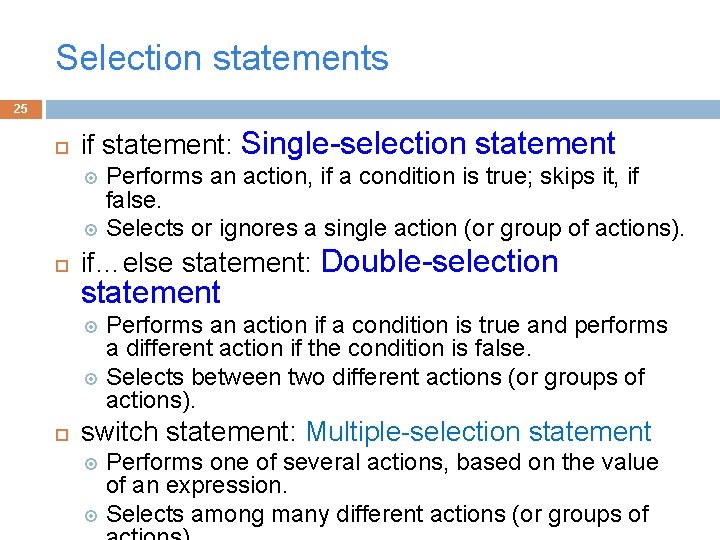
Selection statements 25 if statement: Single-selection statement Performs an action, if a condition is true; skips it, if false. Selects or ignores a single action (or group of actions). if…else statement: Double-selection statement Performs an action if a condition is true and performs a different action if the condition is false. Selects between two different actions (or groups of actions). switch statement: Multiple-selection statement Performs one of several actions, based on the value of an expression. Selects among many different actions (or groups of
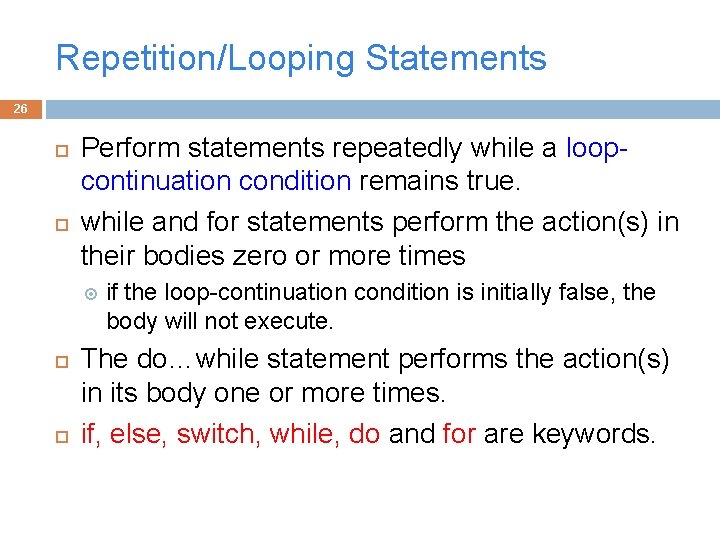
Repetition/Looping Statements 26 Perform statements repeatedly while a loopcontinuation condition remains true. while and for statements perform the action(s) in their bodies zero or more times if the loop-continuation condition is initially false, the body will not execute. The do…while statement performs the action(s) in its body one or more times. if, else, switch, while, do and for are keywords.
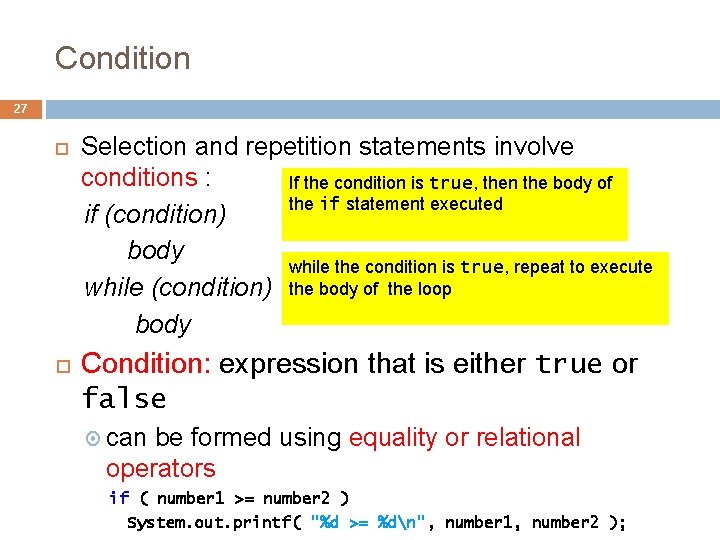
Condition 27 Selection and repetition statements involve conditions : If the condition is true, then the body of the if statement executed if (condition) body while the condition is true, repeat to execute while (condition) the body of the loop body Condition: expression that is either true or false can be formed using equality or relational operators if ( number 1 >= number 2 ) System. out. printf( "%d >= %dn", number 1, number 2 );
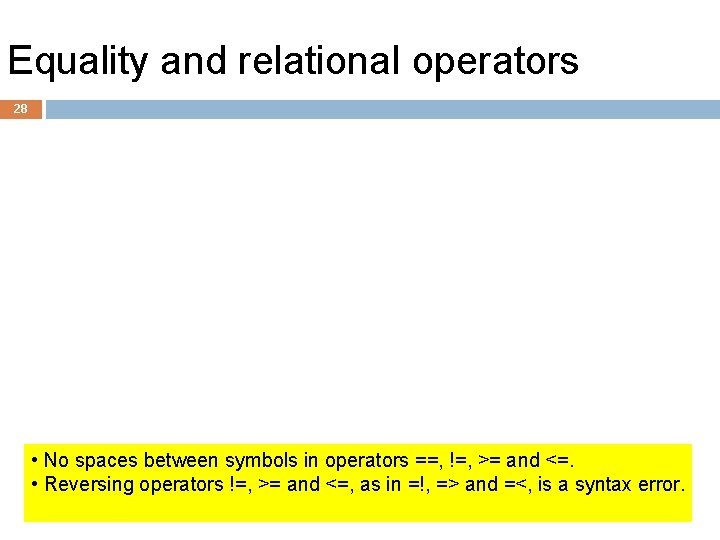
Equality and relational operators 28 • No spaces between symbols in operators ==, !=, >= and <=. • Reversing operators !=, >= and <=, as in =!, => and =<, is a syntax error.
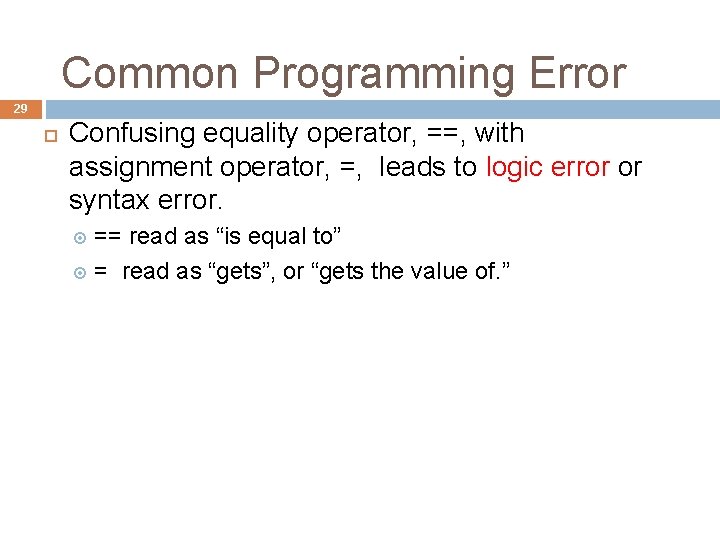
Common Programming Error 29 Confusing equality operator, ==, with assignment operator, =, leads to logic error or syntax error. == read as “is equal to” = read as “gets”, or “gets the value of. ”
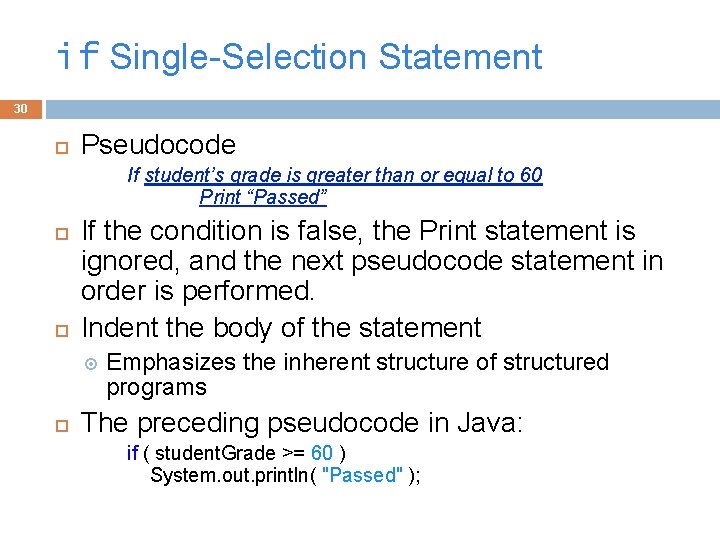
if Single-Selection Statement 30 Pseudocode If student’s grade is greater than or equal to 60 Print “Passed” If the condition is false, the Print statement is ignored, and the next pseudocode statement in order is performed. Indent the body of the statement Emphasizes the inherent structure of structured programs The preceding pseudocode in Java: if ( student. Grade >= 60 ) System. out. println( "Passed" );
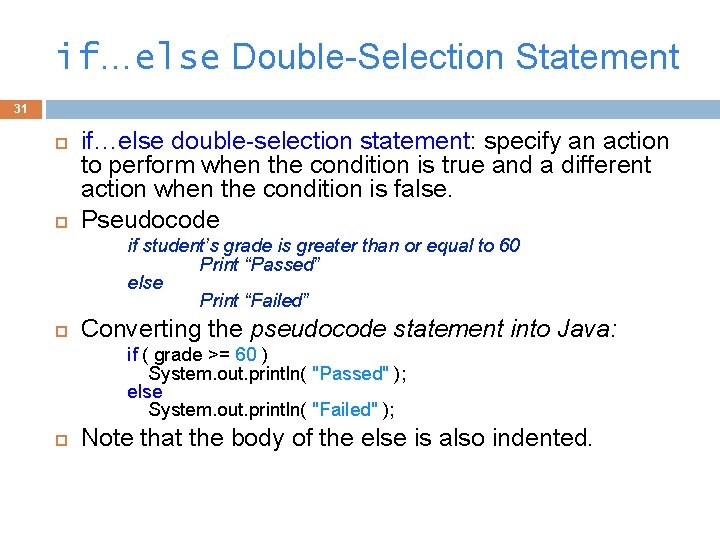
if…else Double-Selection Statement 31 if…else double-selection statement: specify an action to perform when the condition is true and a different action when the condition is false. Pseudocode if student’s grade is greater than or equal to 60 Print “Passed” else Print “Failed” Converting the pseudocode statement into Java: if ( grade >= 60 ) System. out. println( "Passed" ); else System. out. println( "Failed" ); Note that the body of the else is also indented.
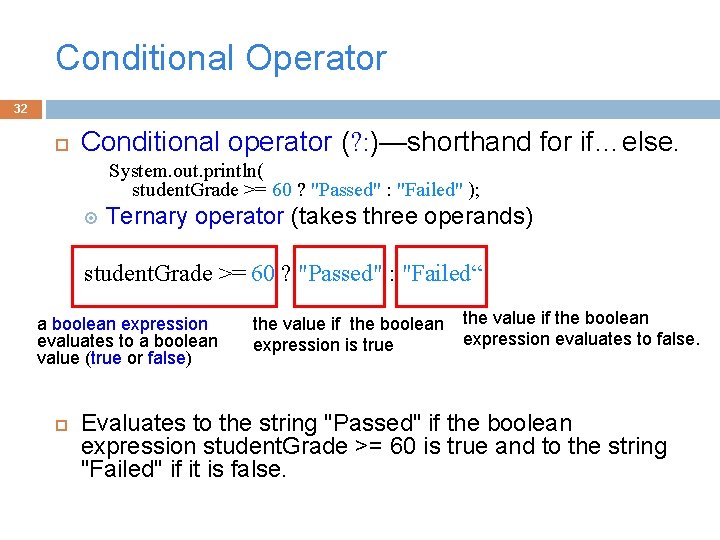
Conditional Operator 32 Conditional operator (? : )—shorthand for if…else. System. out. println( student. Grade >= 60 ? "Passed" : "Failed" ); Ternary operator (takes three operands) student. Grade >= 60 ? "Passed" : "Failed“ a boolean expression evaluates to a boolean value (true or false) the value if the boolean expression evaluates to false. expression is true Evaluates to the string "Passed" if the boolean expression student. Grade >= 60 is true and to the string "Failed" if it is false.
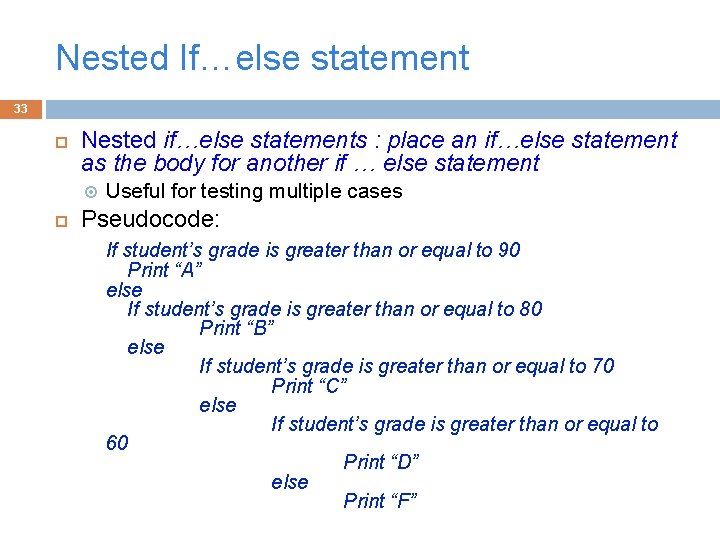
Nested If…else statement 33 Nested if…else statements : place an if…else statement as the body for another if … else statement Useful for testing multiple cases Pseudocode: If student’s grade is greater than or equal to 90 Print “A” else If student’s grade is greater than or equal to 80 Print “B” else If student’s grade is greater than or equal to 70 Print “C” else If student’s grade is greater than or equal to 60 Print “D” else Print “F”
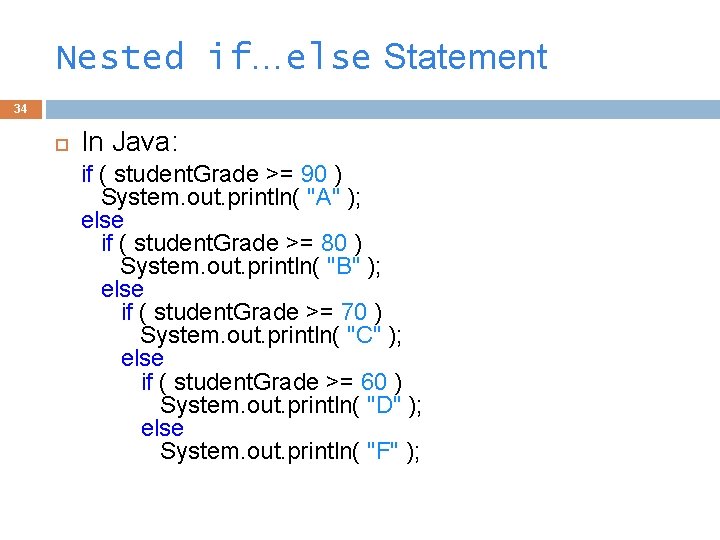
Nested if…else Statement 34 In Java: if ( student. Grade >= 90 ) System. out. println( "A" ); else if ( student. Grade >= 80 ) System. out. println( "B" ); else if ( student. Grade >= 70 ) System. out. println( "C" ); else if ( student. Grade >= 60 ) System. out. println( "D" ); else System. out. println( "F" );
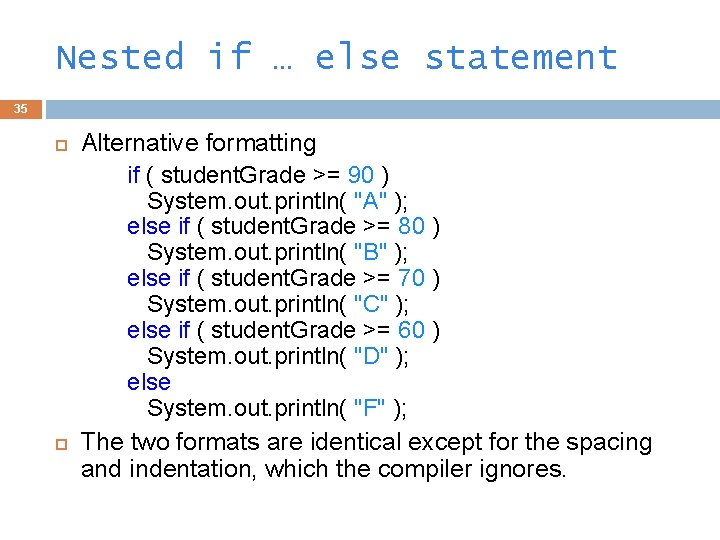
Nested if … else statement 35 Alternative formatting if ( student. Grade >= 90 ) System. out. println( "A" ); else if ( student. Grade >= 80 ) System. out. println( "B" ); else if ( student. Grade >= 70 ) System. out. println( "C" ); else if ( student. Grade >= 60 ) System. out. println( "D" ); else System. out. println( "F" ); The two formats are identical except for the spacing and indentation, which the compiler ignores.
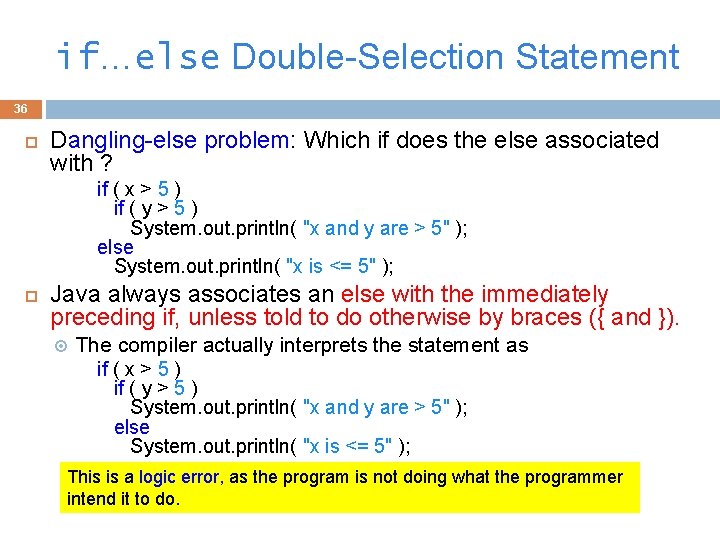
if…else Double-Selection Statement 36 Dangling-else problem: Which if does the else associated with ? if ( x > 5 ) if ( y > 5 ) System. out. println( "x and y are > 5" ); else System. out. println( "x is <= 5" ); Java always associates an else with the immediately preceding if, unless told to do otherwise by braces ({ and }). The compiler actually interprets the statement as if ( x > 5 ) if ( y > 5 ) System. out. println( "x and y are > 5" ); else System. out. println( "x is <= 5" ); This is a logic error, as the program is not doing what the programmer intend it to do.
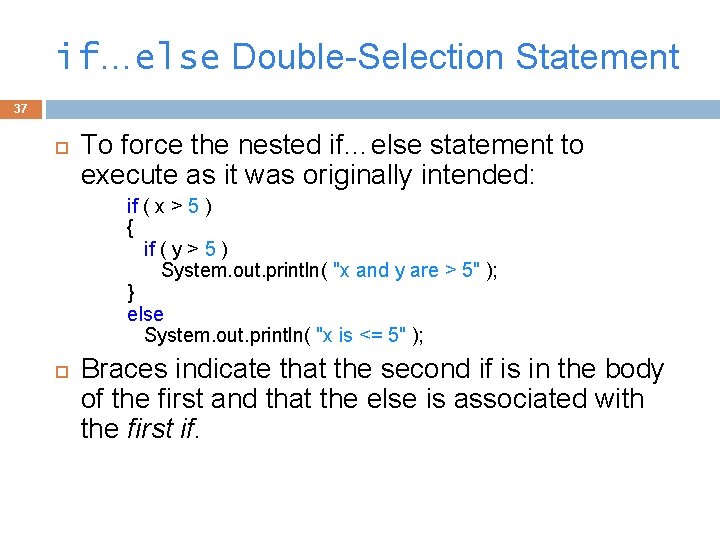
if…else Double-Selection Statement 37 To force the nested if…else statement to execute as it was originally intended: if ( x > 5 ) { if ( y > 5 ) System. out. println( "x and y are > 5" ); } else System. out. println( "x is <= 5" ); Braces indicate that the second if is in the body of the first and that the else is associated with the first if.
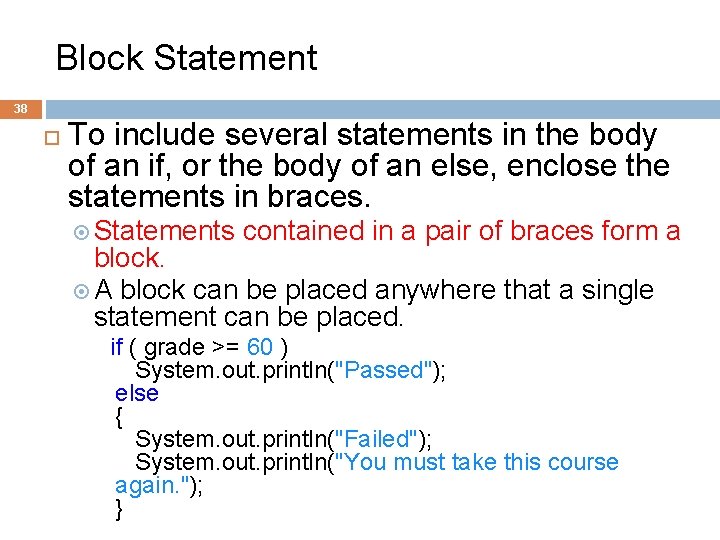
Block Statement 38 To include several statements in the body of an if, or the body of an else, enclose the statements in braces. Statements contained in a pair of braces form a block. A block can be placed anywhere that a single statement can be placed. if ( grade >= 60 ) System. out. println("Passed"); else { System. out. println("Failed"); System. out. println("You must take this course again. "); }
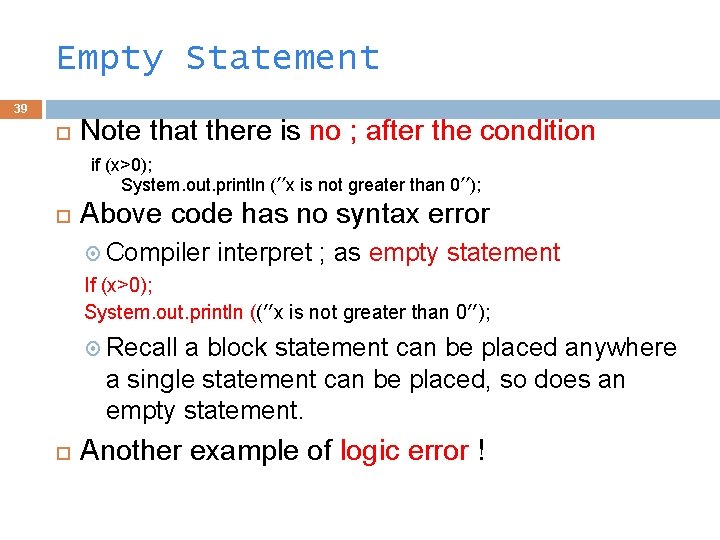
Empty Statement 39 Note that there is no ; after the condition if (x>0); System. out. println (″x is not greater than 0″); Above code has no syntax error Compiler interpret ; as empty statement If (x>0); System. out. println ((″x is not greater than 0″); Recall a block statement can be placed anywhere a single statement can be placed, so does an empty statement. Another example of logic error !
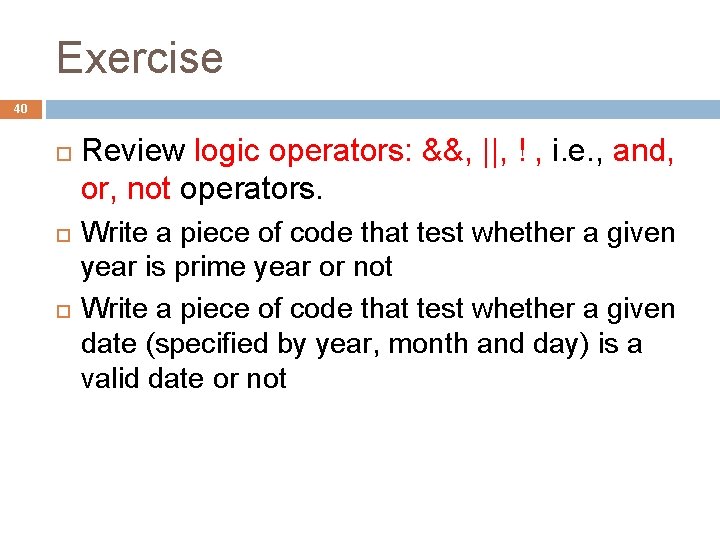
Exercise 40 Review logic operators: &&, ||, ! , i. e. , and, or, not operators. Write a piece of code that test whether a given year is prime year or not Write a piece of code that test whether a given date (specified by year, month and day) is a valid date or not
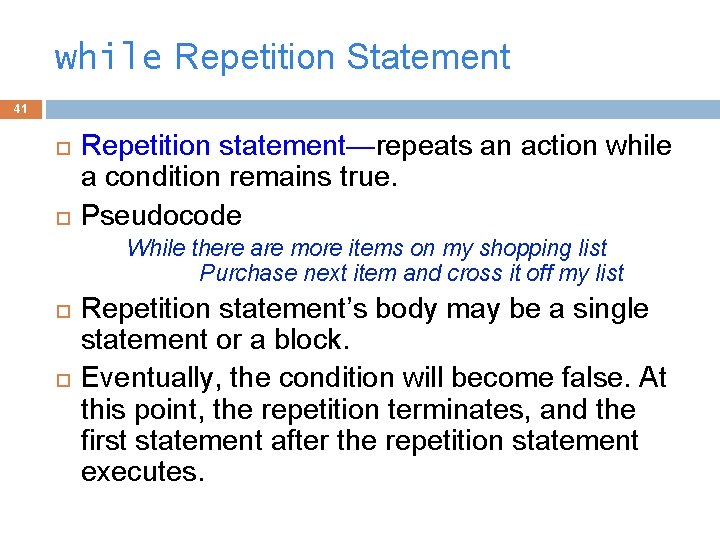
while Repetition Statement 41 Repetition statement—repeats an action while a condition remains true. Pseudocode While there are more items on my shopping list Purchase next item and cross it off my list Repetition statement’s body may be a single statement or a block. Eventually, the condition will become false. At this point, the repetition terminates, and the first statement after the repetition statement executes.
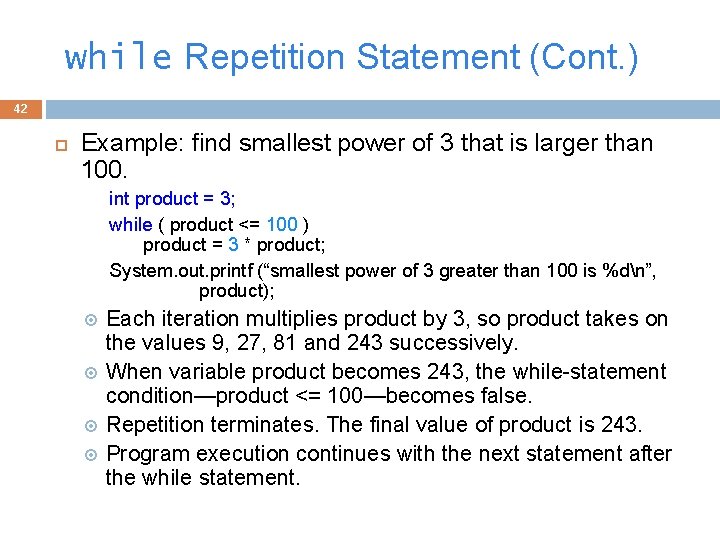
while Repetition Statement (Cont. ) 42 Example: find smallest power of 3 that is larger than 100. int product = 3; while ( product <= 100 ) product = 3 * product; System. out. printf (“smallest power of 3 greater than 100 is %dn”, product); Each iteration multiplies product by 3, so product takes on the values 9, 27, 81 and 243 successively. When variable product becomes 243, the while-statement condition—product <= 100—becomes false. Repetition terminates. The final value of product is 243. Program execution continues with the next statement after the while statement.
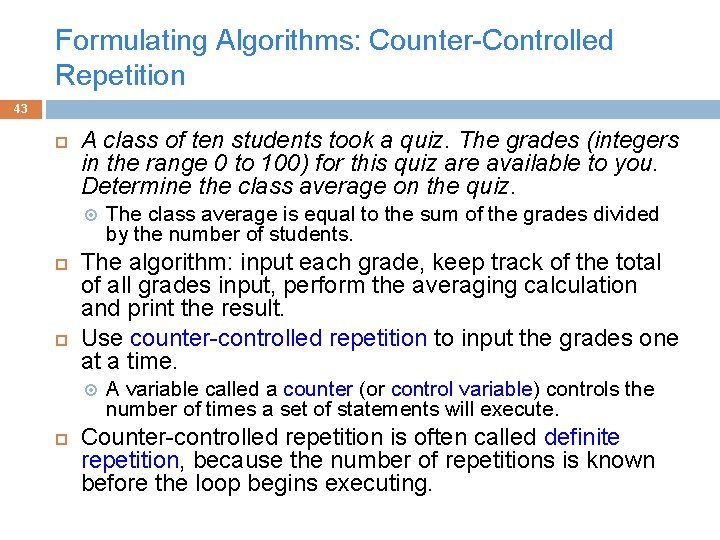
Formulating Algorithms: Counter-Controlled Repetition 43 A class of ten students took a quiz. The grades (integers in the range 0 to 100) for this quiz are available to you. Determine the class average on the quiz. The algorithm: input each grade, keep track of the total of all grades input, perform the averaging calculation and print the result. Use counter-controlled repetition to input the grades one at a time. The class average is equal to the sum of the grades divided by the number of students. A variable called a counter (or control variable) controls the number of times a set of statements will execute. Counter-controlled repetition is often called definite repetition, because the number of repetitions is known before the loop begins executing.
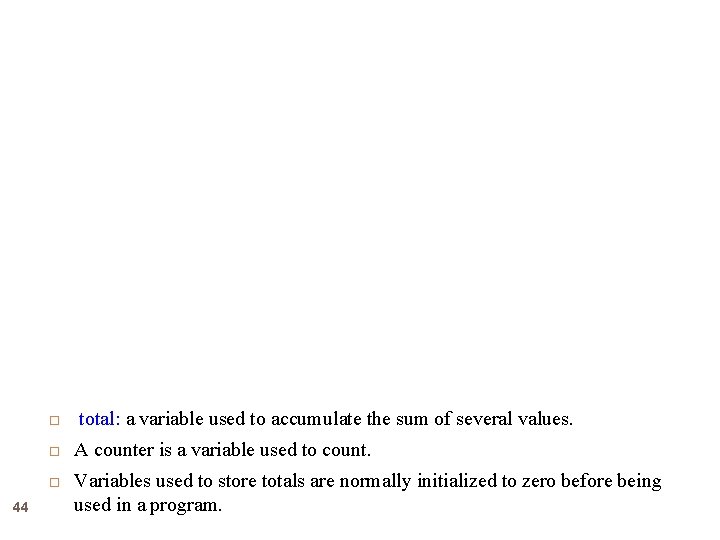
44 total: a variable used to accumulate the sum of several values. A counter is a variable used to count. Variables used to store totals are normally initialized to zero before being used in a program.
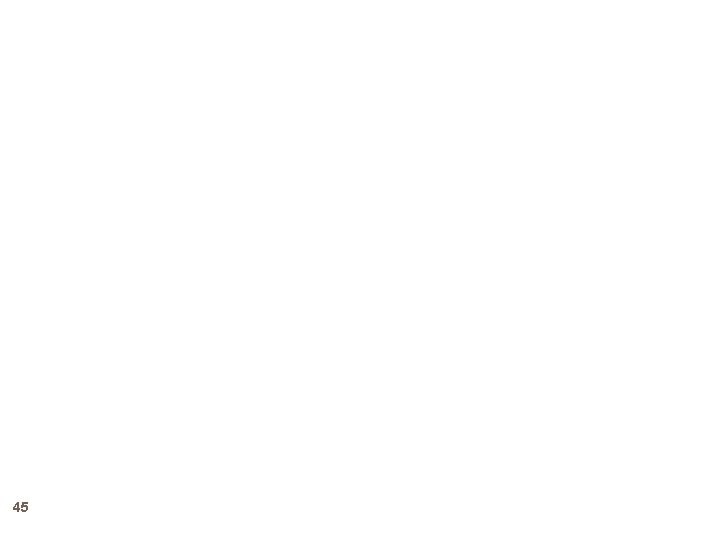
45
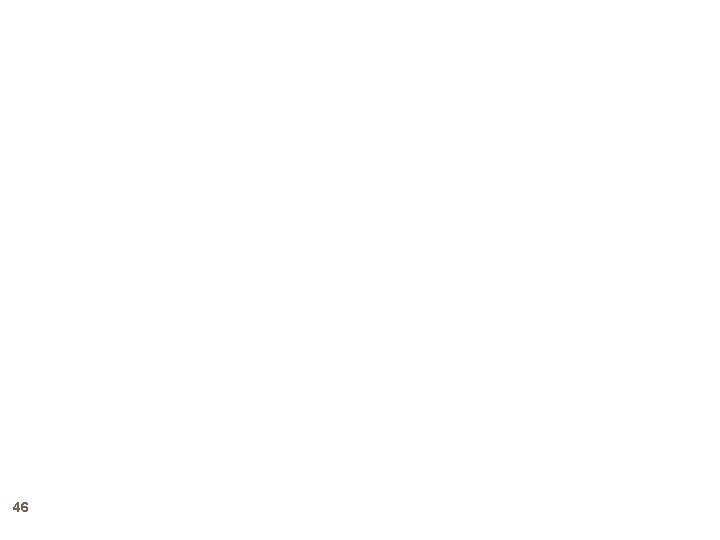
46
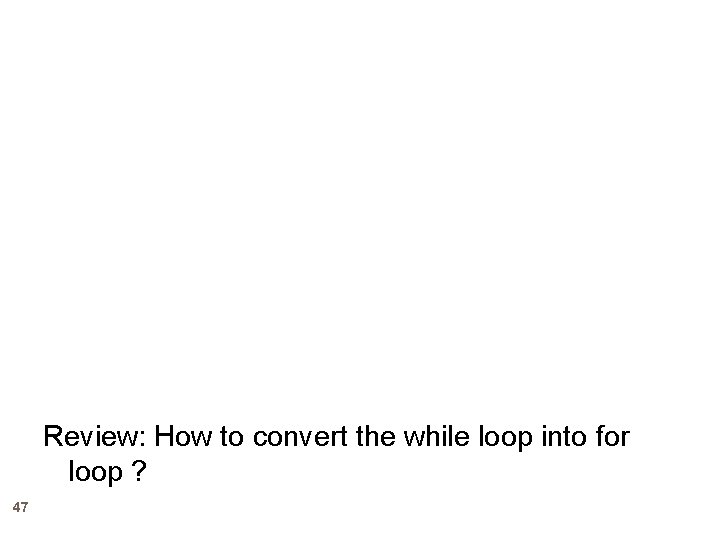
Review: How to convert the while loop into for loop ? 47
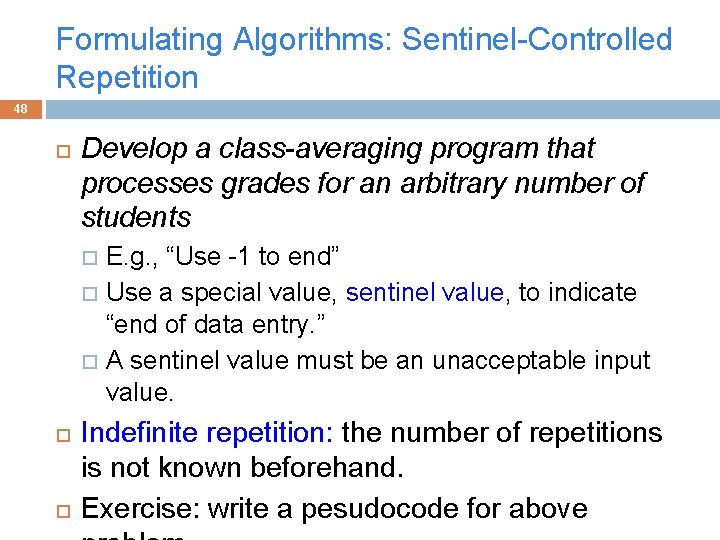
Formulating Algorithms: Sentinel-Controlled Repetition 48 Develop a class-averaging program that processes grades for an arbitrary number of students E. g. , “Use -1 to end” Use a special value, sentinel value, to indicate “end of data entry. ” A sentinel value must be an unacceptable input value. Indefinite repetition: the number of repetitions is not known beforehand. Exercise: write a pesudocode for above
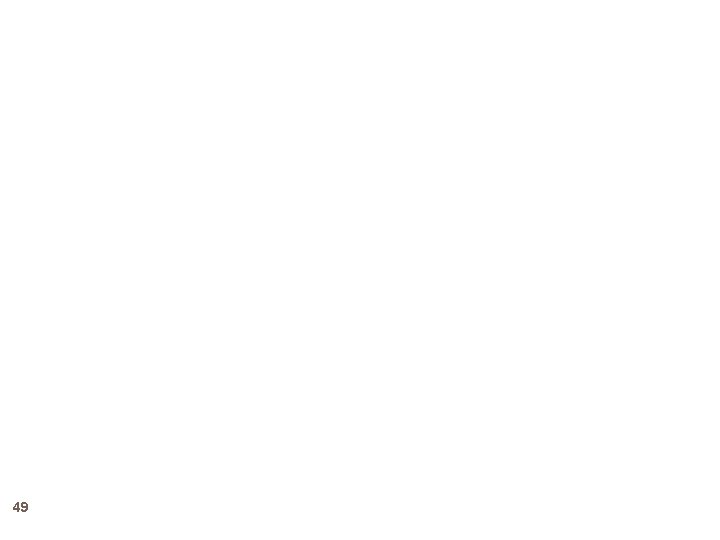
49
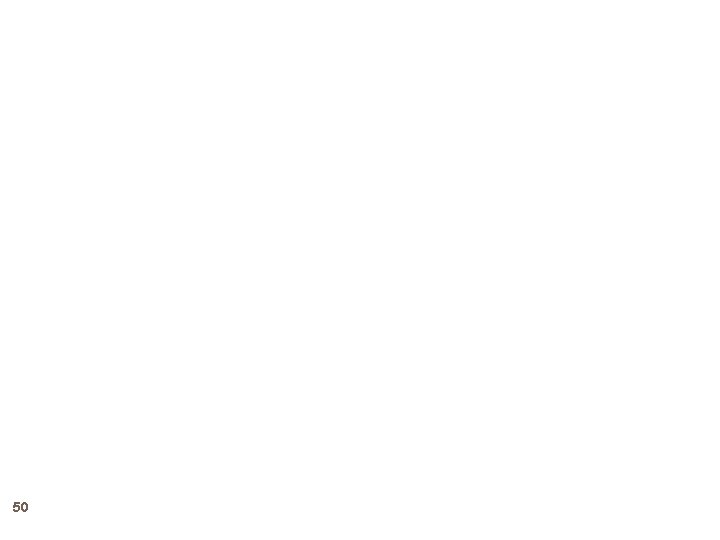
50
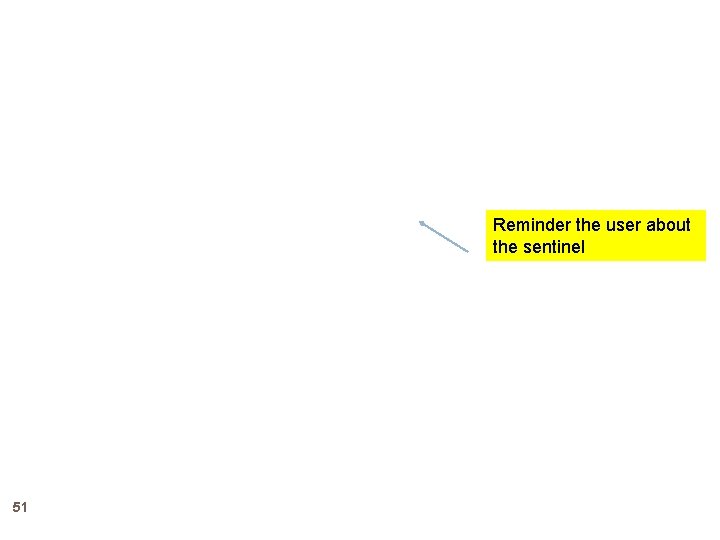
Reminder the user about the sentinel 51
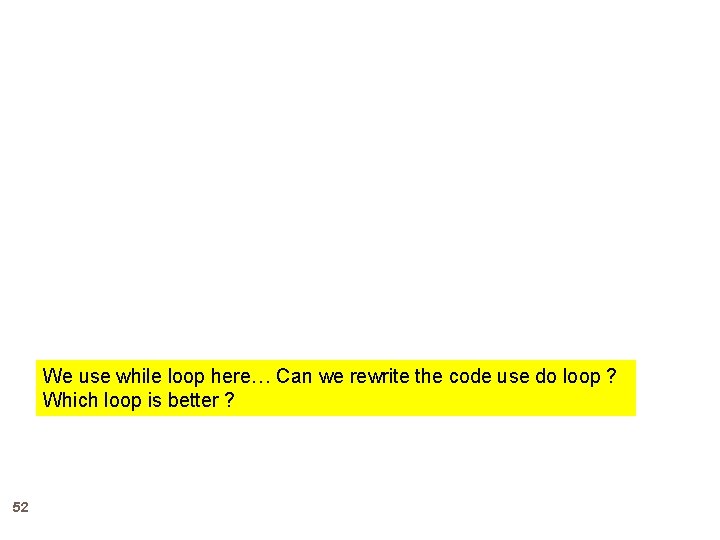
We use while loop here… Can we rewrite the code use do loop ? Which loop is better ? 52
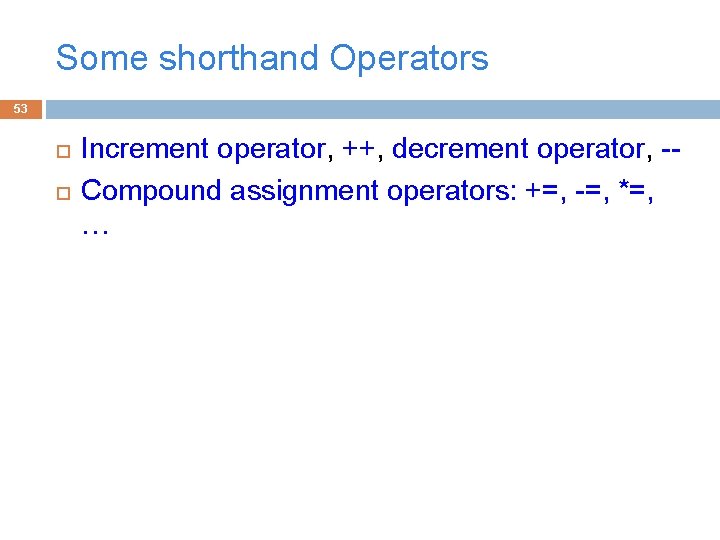
Some shorthand Operators 53 Increment operator, ++, decrement operator, -Compound assignment operators: +=, -=, *=, …
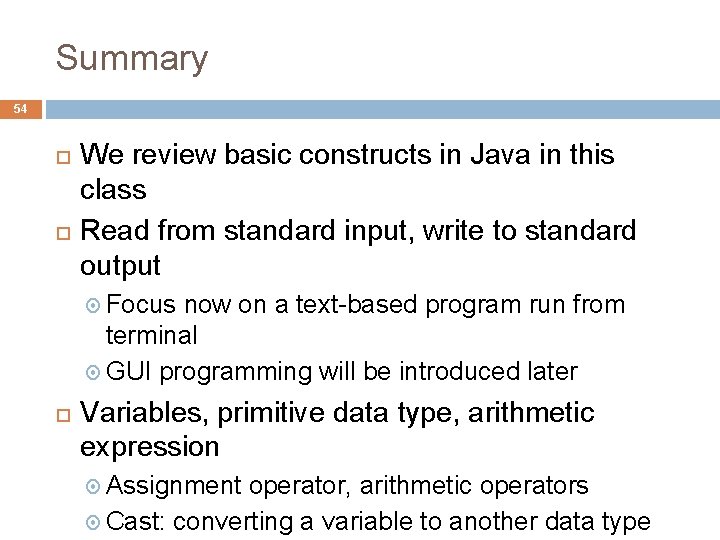
Summary 54 We review basic constructs in Java in this class Read from standard input, write to standard output Focus now on a text-based program run from terminal GUI programming will be introduced later Variables, primitive data type, arithmetic expression Assignment operator, arithmetic operators Cast: converting a variable to another data type
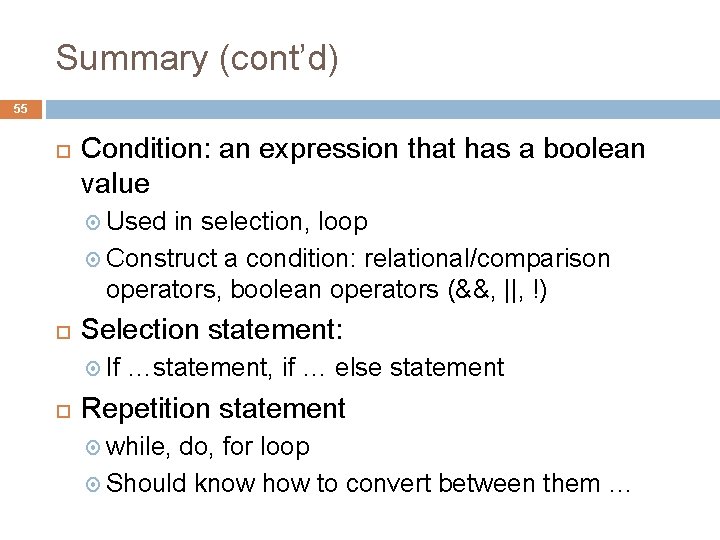
Summary (cont’d) 55 Condition: an expression that has a boolean value Used in selection, loop Construct a condition: relational/comparison operators, boolean operators (&&, ||, !) Selection statement: If …statement, if … else statement Repetition statement while, do, for loop Should know how to convert between them …
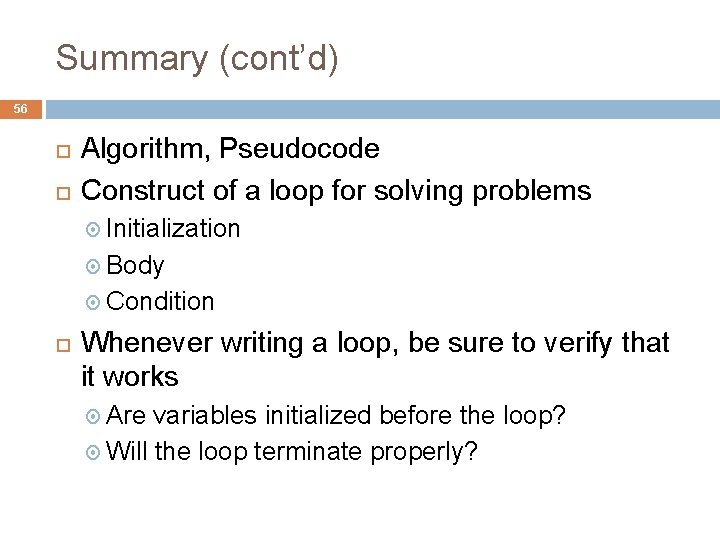
Summary (cont’d) 56 Algorithm, Pseudocode Construct of a loop for solving problems Initialization Body Condition Whenever writing a loop, be sure to verify that it works Are variables initialized before the loop? Will the loop terminate properly?
Xiaolan zhang fordham
Cisc 101
Cisc vs risc architecture
What is cisc
Contoh prosesor risc
Cisc vs risc
Isa cisc
Cisc 1050
Risc e cisc
Cisc pipeline
Pipeline cisc
Cisc risc
Cisc 3140
Risc and cisc difference
Ciri ciri cisc
Risc and cisc difference
Cisc processor examples
Risc vs cisc
Lawrence goetz
Cisc assembly language
Cisc complex instruction set computer
Cisc 3130
Cisc 181
Arduino risc or cisc
Cisc scalar processor
Cisc isa
Cisc cu boulder
Risc vs cisc vs arm
Cisc 235
Risc cisc architecture
Alasan mengapa digunakan risc
Cisc architecture
Arquitectura risc y cisc
Computer organization and architecture definition
Arquitetura risc e cisc
Cisc 235
Cisc 181
Arquitetura risc e cisc
Import java.util.scanner;
Import java.util.*
Swing vs awt
How to import java.util.scanner
Import java.io.file;
Java gcd
Import java.util.random
Import java.io.*
Import java.util.*
Java import java.io.*
Awt adalah
Import java.awt.event.*
Programming language b
Ejb remote vs local
Qirun zhang
Hui zhang cmu
Zuofeng zhang
Zuofeng zhang
Faceflow