CISC 3140 CIS 20 2 Design Implementation of
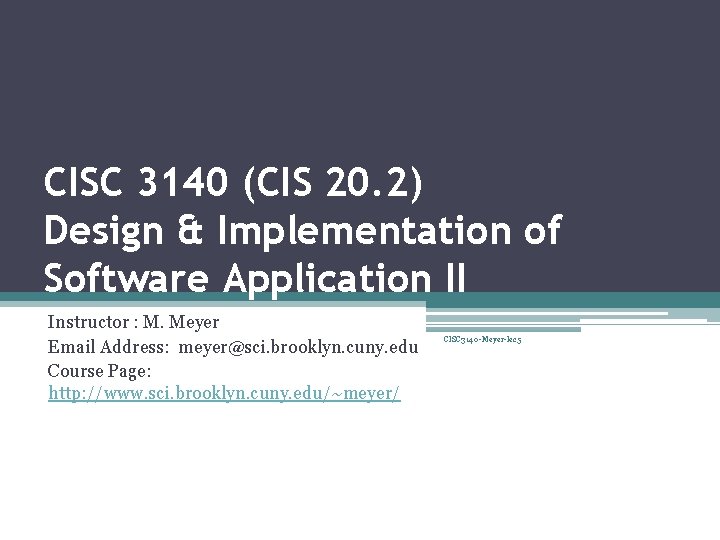
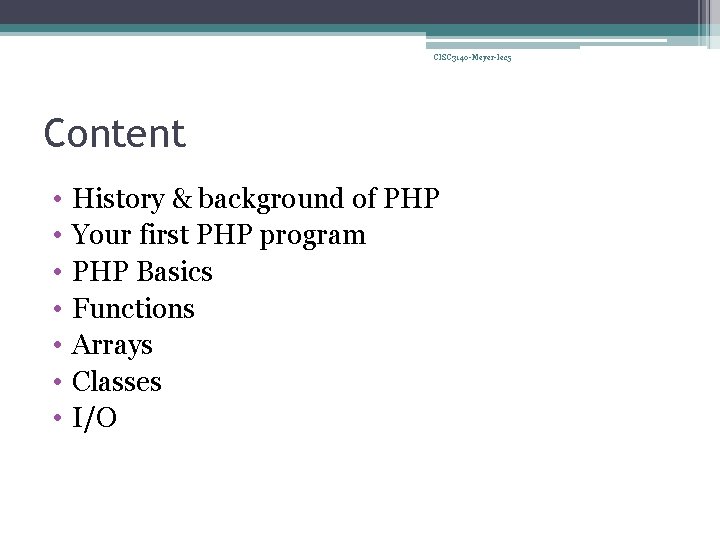
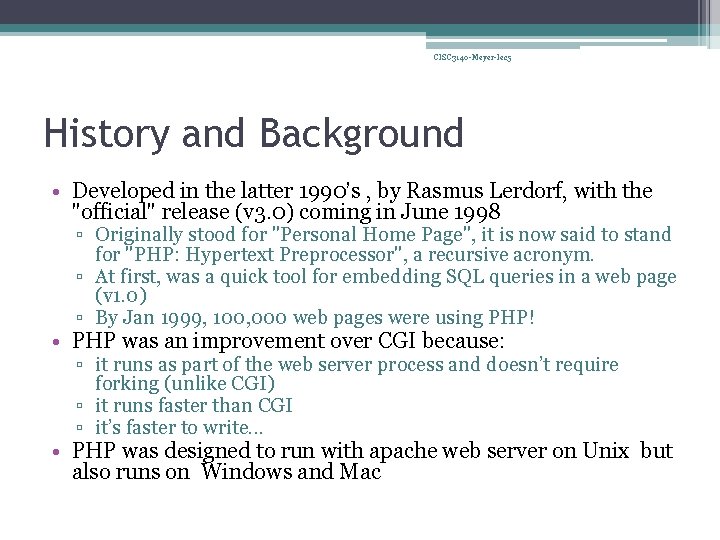
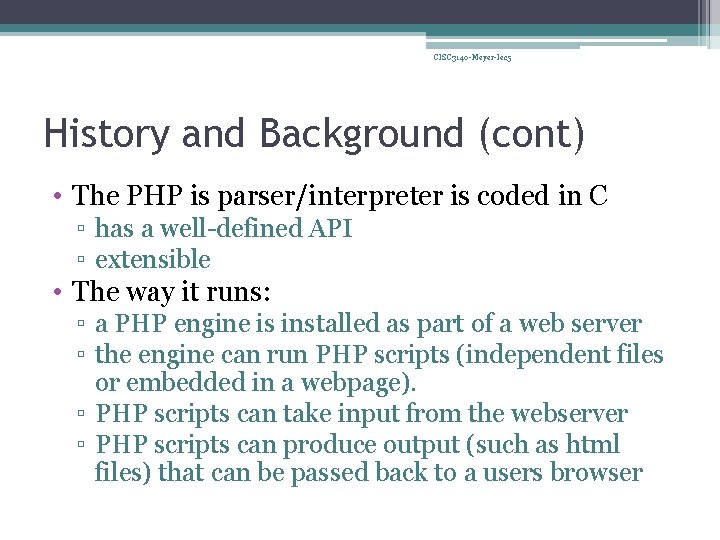
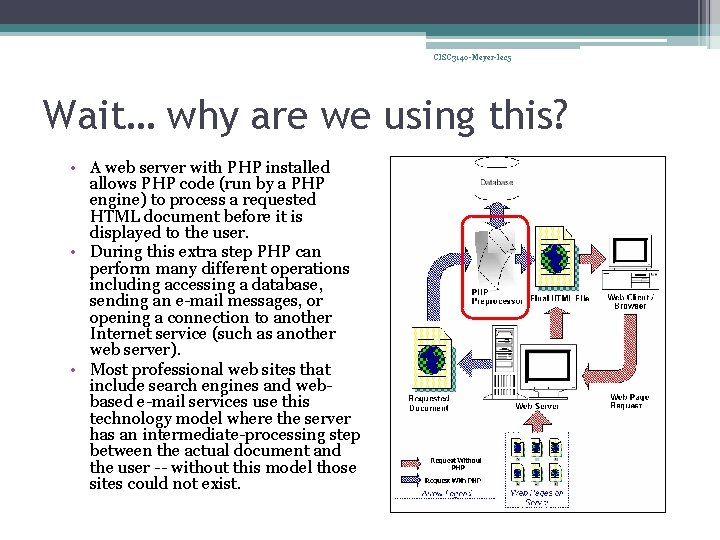
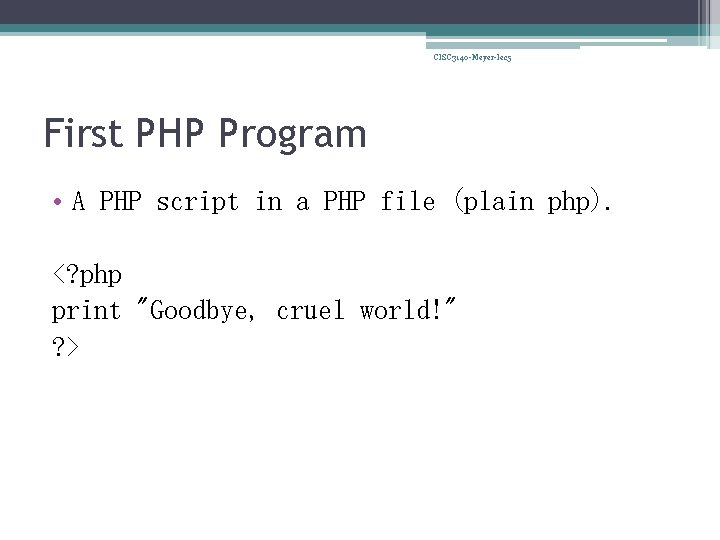
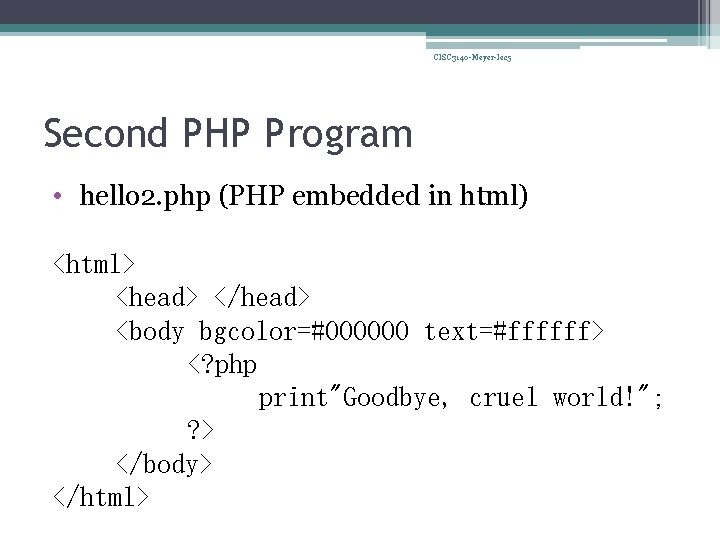
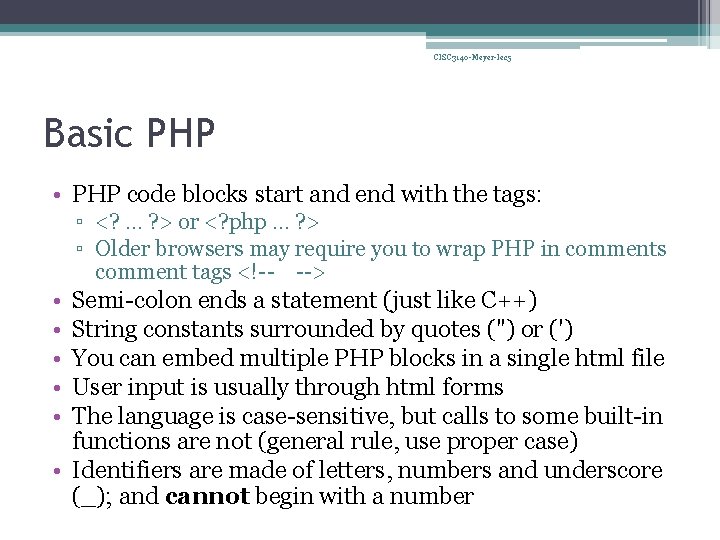
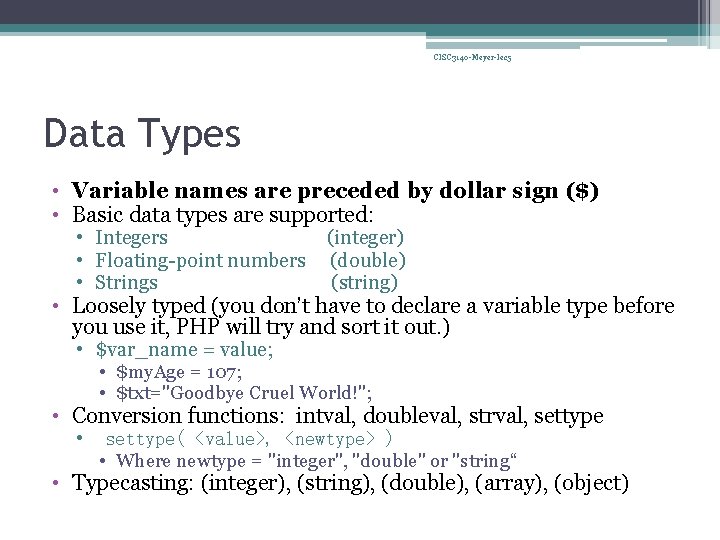
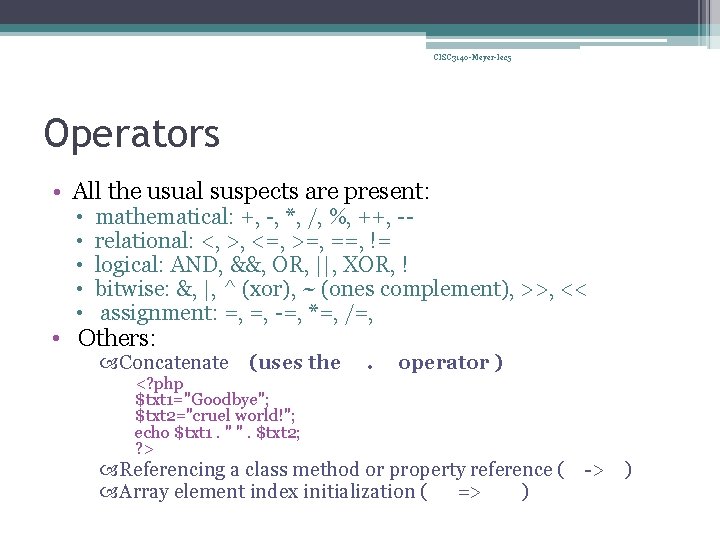
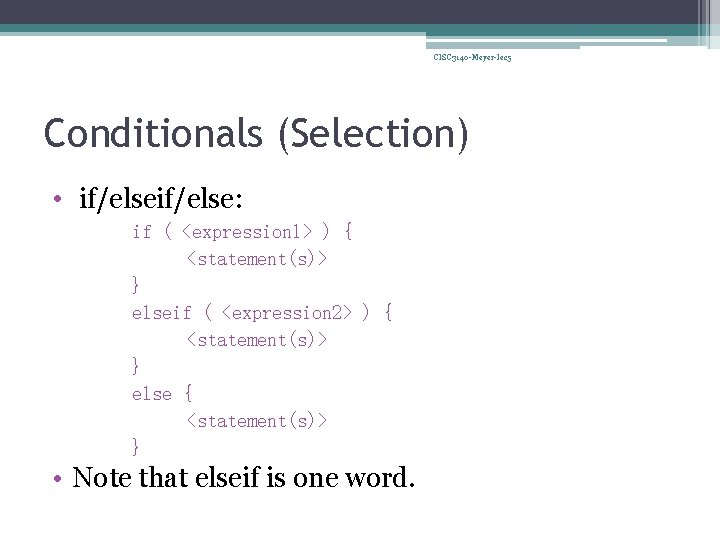
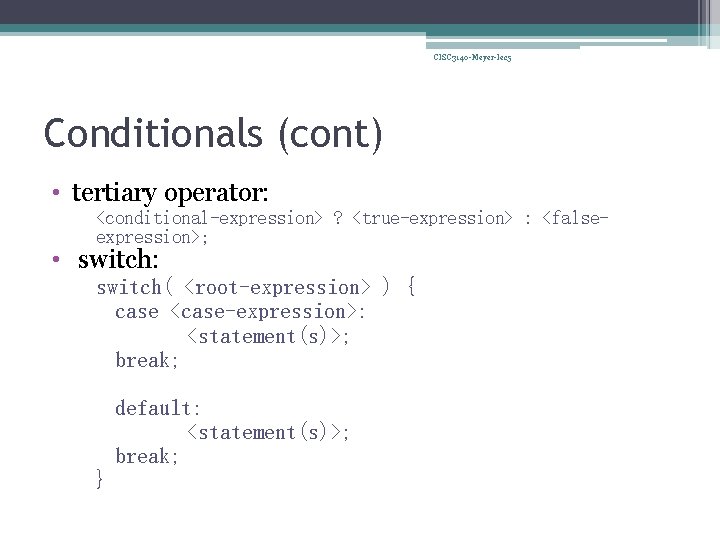
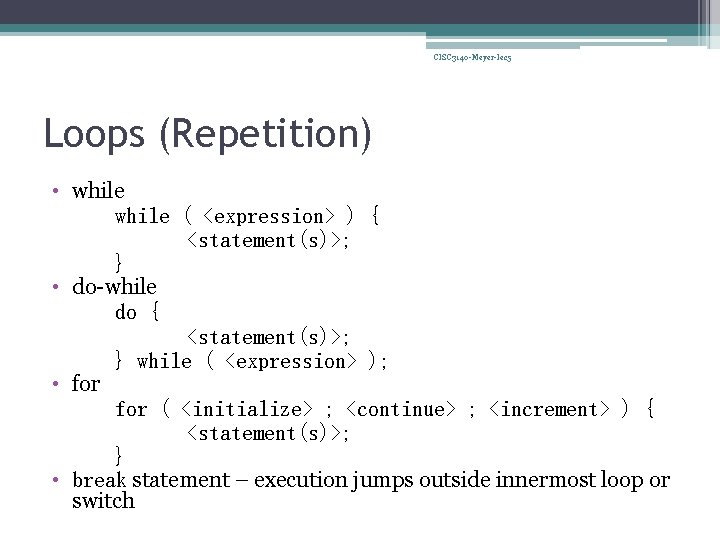
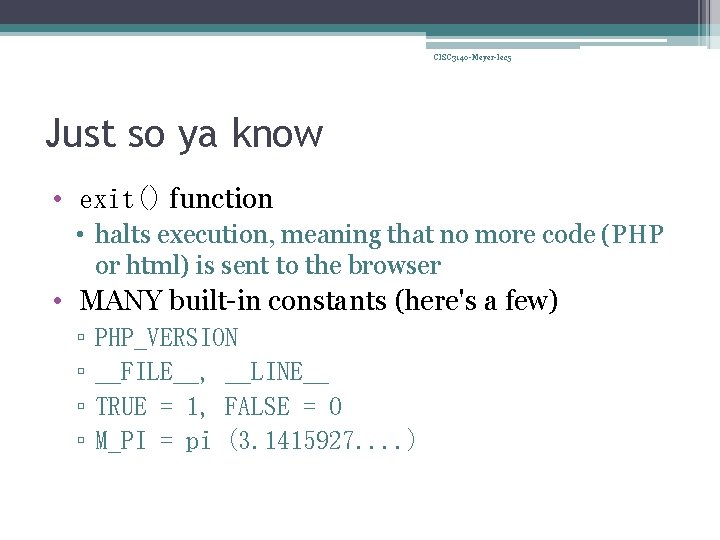
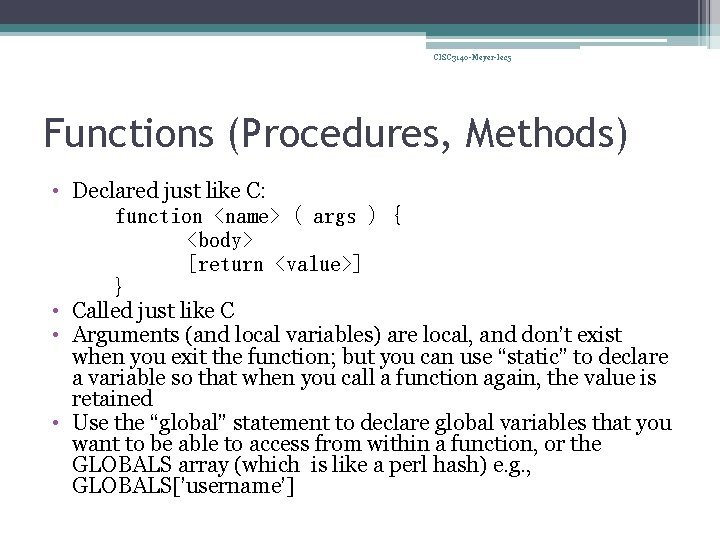
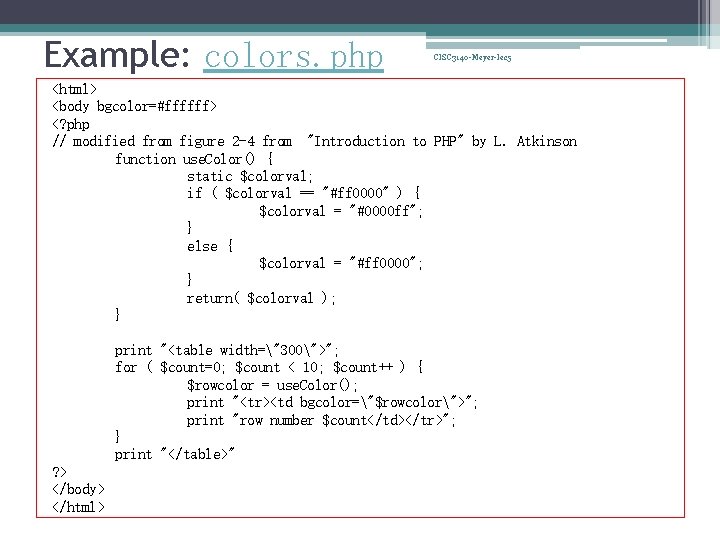
![CISC 3140 -Meyer-lec 5 Arrays (Hurray!) • Indexed using [. . . ] • CISC 3140 -Meyer-lec 5 Arrays (Hurray!) • Indexed using [. . . ] •](https://slidetodoc.com/presentation_image_h2/18c5769f129ee0b22d554ccdd05fb0d1/image-17.jpg)
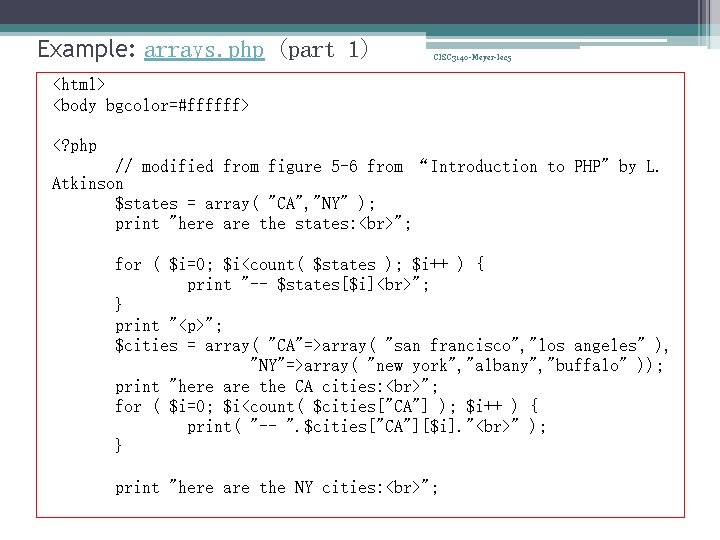
![Example: arrays. php (part 2) CISC 3140 -Meyer-lec 5 for ( $i=0; $i<count( $cities["NY"] Example: arrays. php (part 2) CISC 3140 -Meyer-lec 5 for ( $i=0; $i<count( $cities["NY"]](https://slidetodoc.com/presentation_image_h2/18c5769f129ee0b22d554ccdd05fb0d1/image-19.jpg)
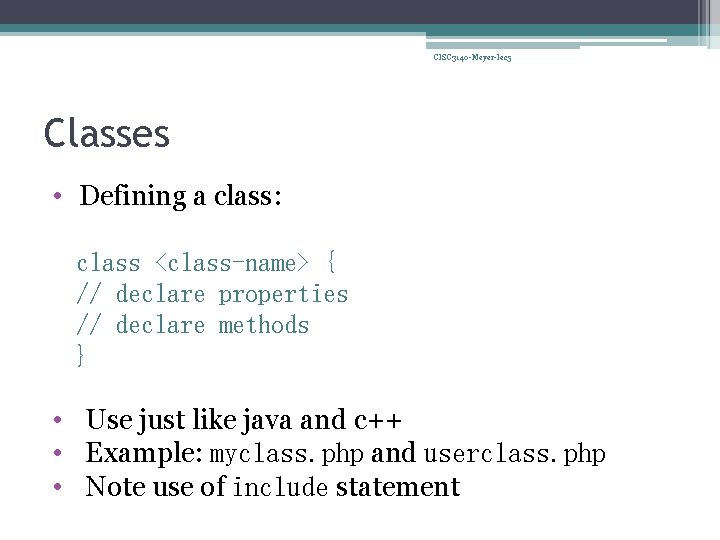
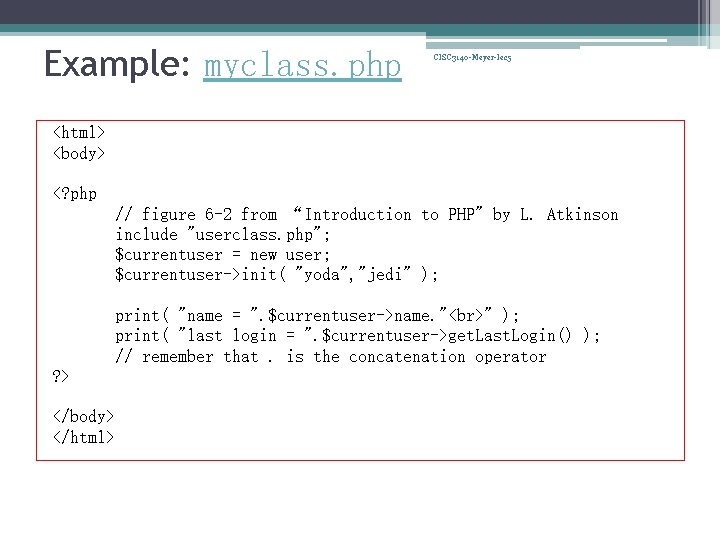
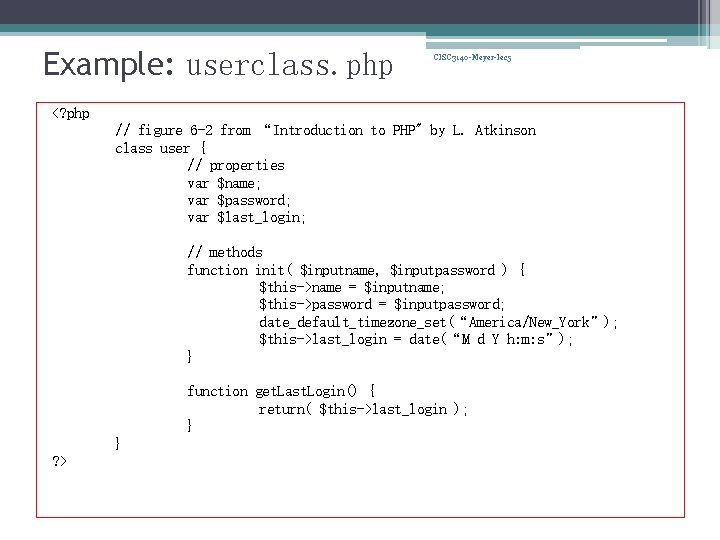
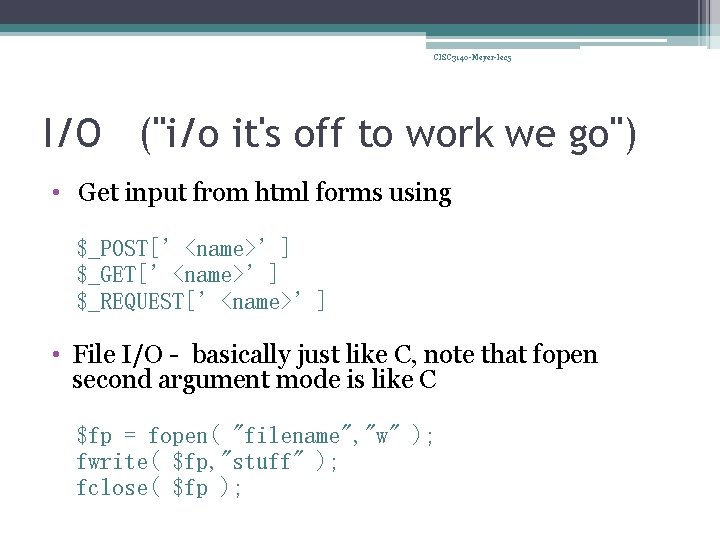
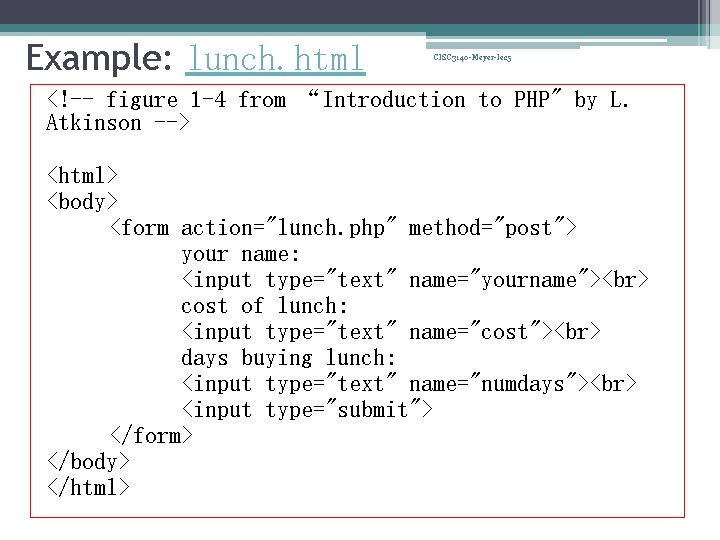
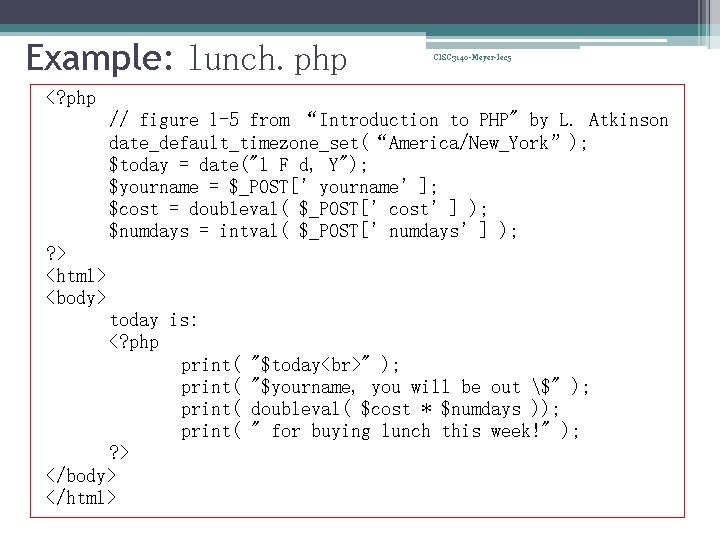
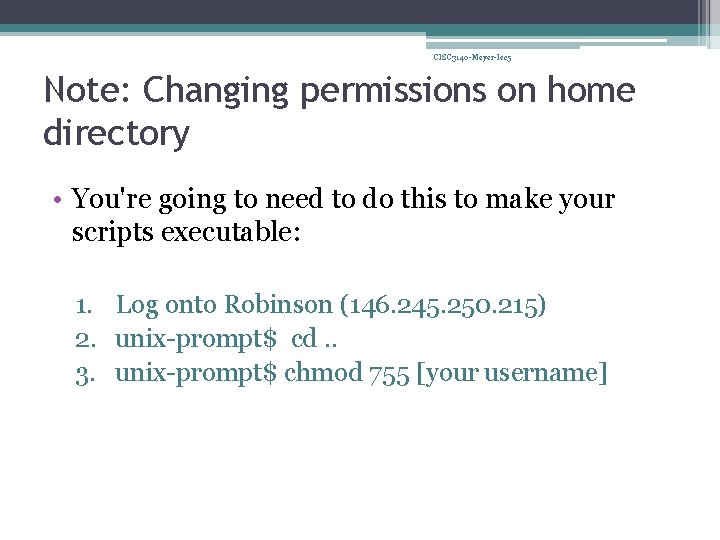
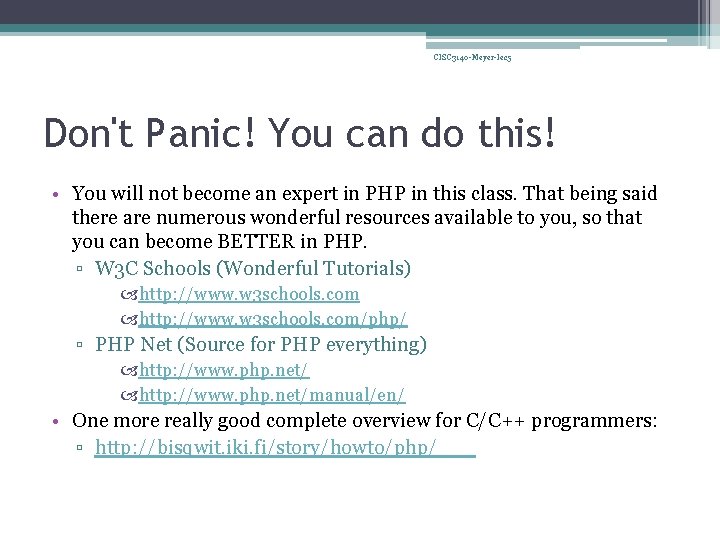
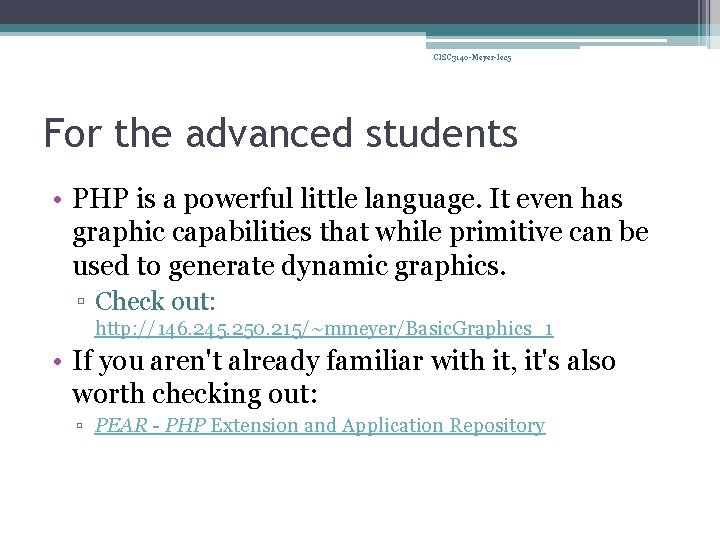
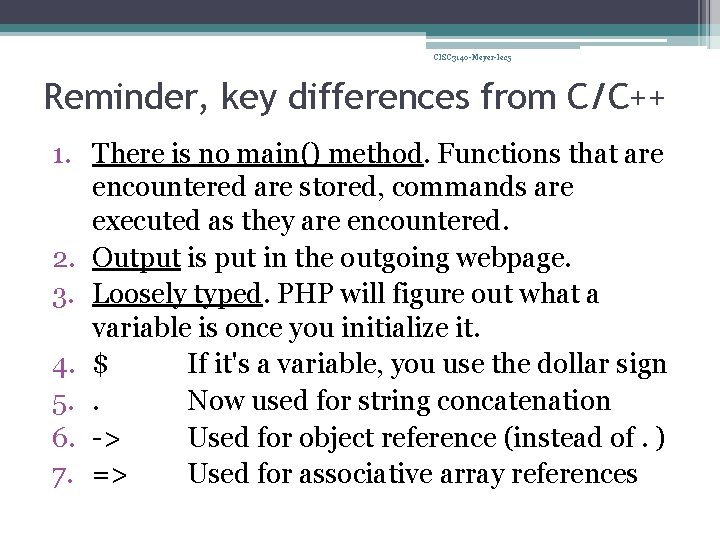
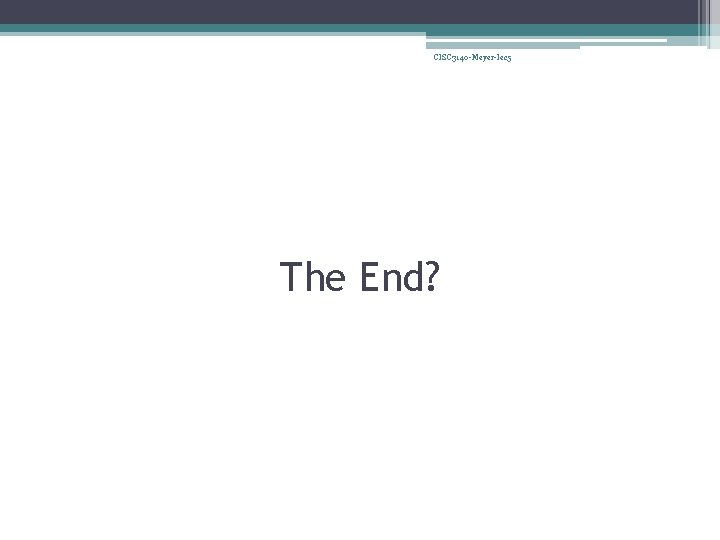
- Slides: 30
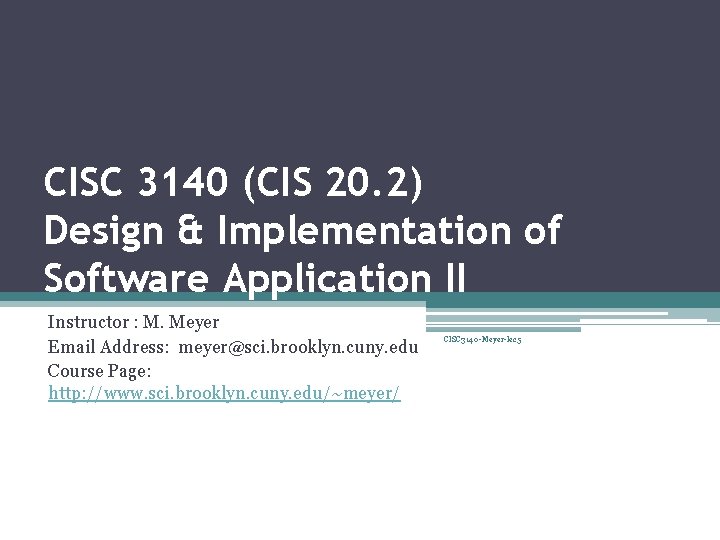
CISC 3140 (CIS 20. 2) Design & Implementation of Software Application II Instructor : M. Meyer Email Address: meyer@sci. brooklyn. cuny. edu Course Page: http: //www. sci. brooklyn. cuny. edu/~meyer/ CISC 3140 -Meyer-lec 5
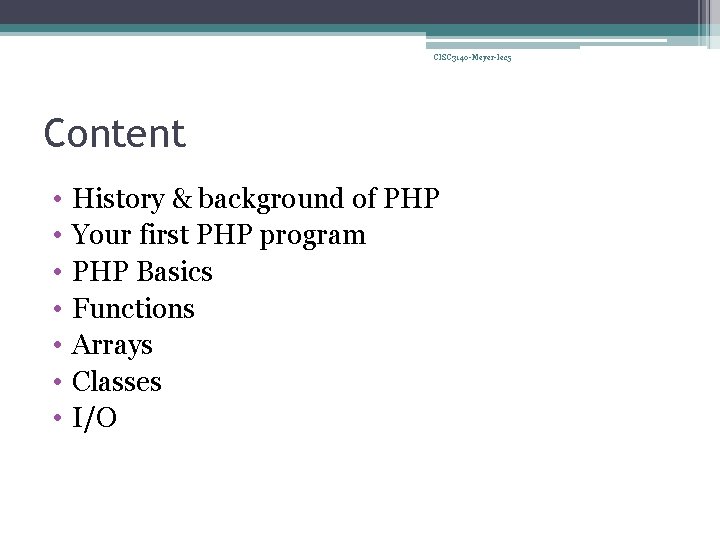
CISC 3140 -Meyer-lec 5 Content • • History & background of PHP Your first PHP program PHP Basics Functions Arrays Classes I/O
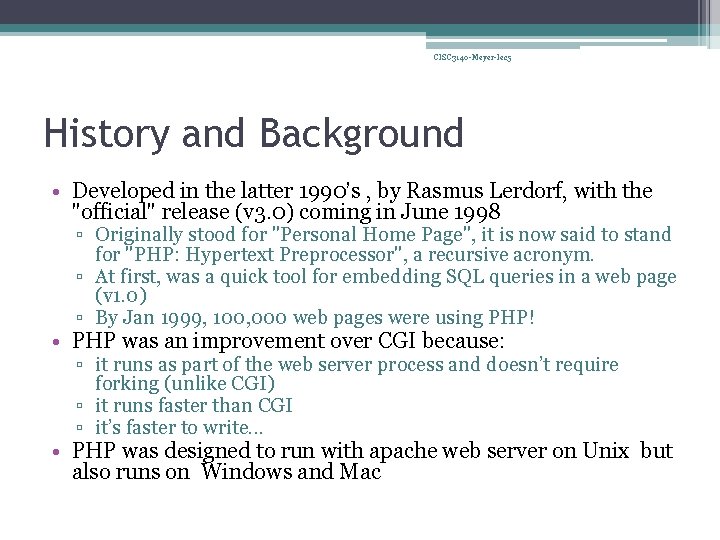
CISC 3140 -Meyer-lec 5 History and Background • Developed in the latter 1990’s , by Rasmus Lerdorf, with the "official" release (v 3. 0) coming in June 1998 ▫ Originally stood for "Personal Home Page", it is now said to stand for "PHP: Hypertext Preprocessor", a recursive acronym. ▫ At first, was a quick tool for embedding SQL queries in a web page (v 1. 0) ▫ By Jan 1999, 100, 000 web pages were using PHP! • PHP was an improvement over CGI because: ▫ it runs as part of the web server process and doesn’t require forking (unlike CGI) ▫ it runs faster than CGI ▫ it’s faster to write. . . • PHP was designed to run with apache web server on Unix but also runs on Windows and Mac
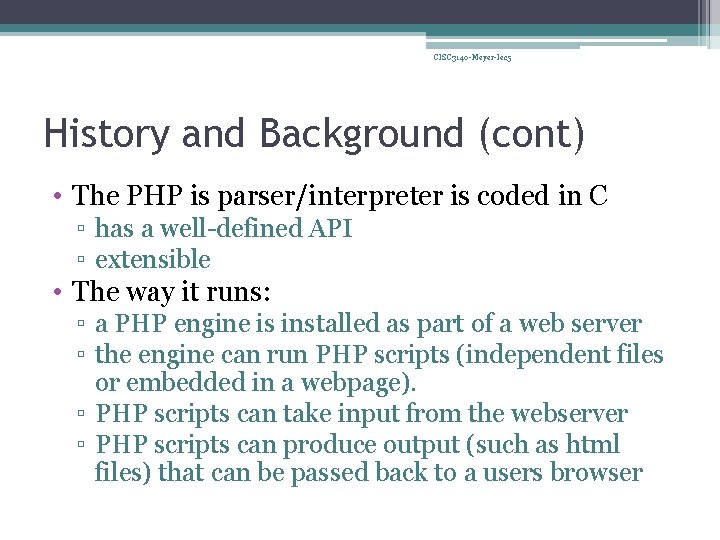
CISC 3140 -Meyer-lec 5 History and Background (cont) • The PHP is parser/interpreter is coded in C ▫ has a well-defined API ▫ extensible • The way it runs: ▫ a PHP engine is installed as part of a web server ▫ the engine can run PHP scripts (independent files or embedded in a webpage). ▫ PHP scripts can take input from the webserver ▫ PHP scripts can produce output (such as html files) that can be passed back to a users browser
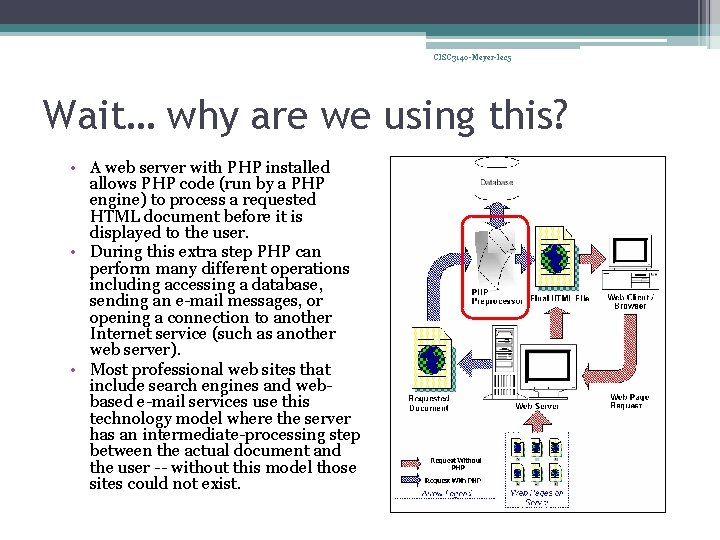
CISC 3140 -Meyer-lec 5 Wait… why are we using this? • A web server with PHP installed allows PHP code (run by a PHP engine) to process a requested HTML document before it is displayed to the user. • During this extra step PHP can perform many different operations including accessing a database, sending an e-mail messages, or opening a connection to another Internet service (such as another web server). • Most professional web sites that include search engines and webbased e-mail services use this technology model where the server has an intermediate-processing step between the actual document and the user -- without this model those sites could not exist.
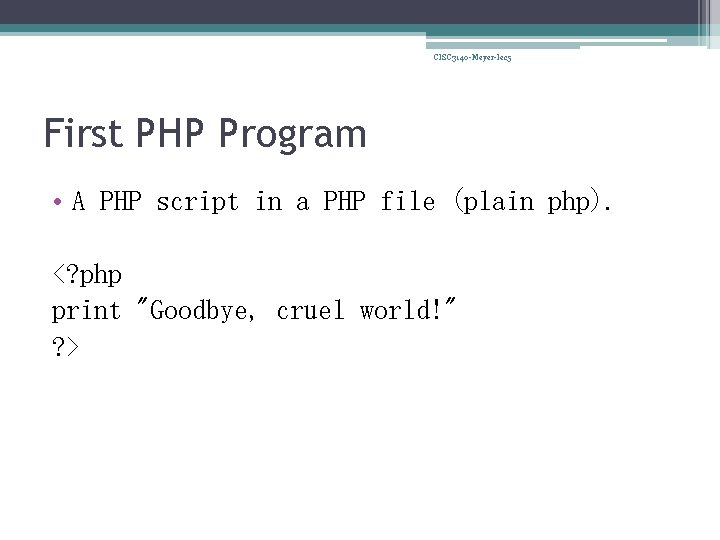
CISC 3140 -Meyer-lec 5 First PHP Program • A PHP script in a PHP file (plain php). <? php print "Goodbye, cruel world!" ? >
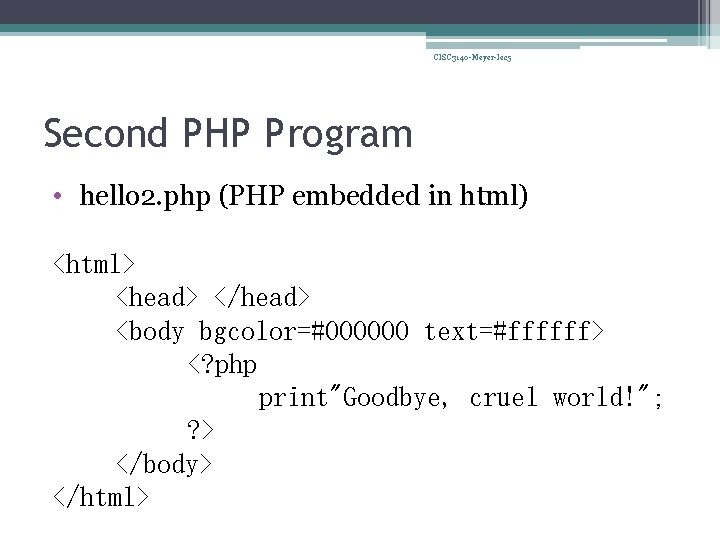
CISC 3140 -Meyer-lec 5 Second PHP Program • hello 2. php (PHP embedded in html) <html> <head> </head> <body bgcolor=#000000 text=#ffffff> <? php print"Goodbye, cruel world!"; ? > </body> </html>
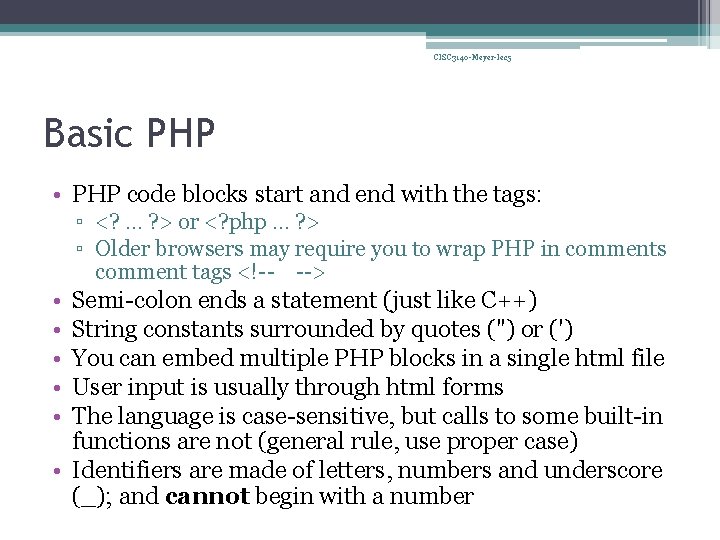
CISC 3140 -Meyer-lec 5 Basic PHP • PHP code blocks start and end with the tags: ▫ <? . . . ? > or <? php. . . ? > ▫ Older browsers may require you to wrap PHP in comments comment tags <!-- --> • • • Semi-colon ends a statement (just like C++) String constants surrounded by quotes (") or (') You can embed multiple PHP blocks in a single html file User input is usually through html forms The language is case-sensitive, but calls to some built-in functions are not (general rule, use proper case) • Identifiers are made of letters, numbers and underscore (_); and cannot begin with a number
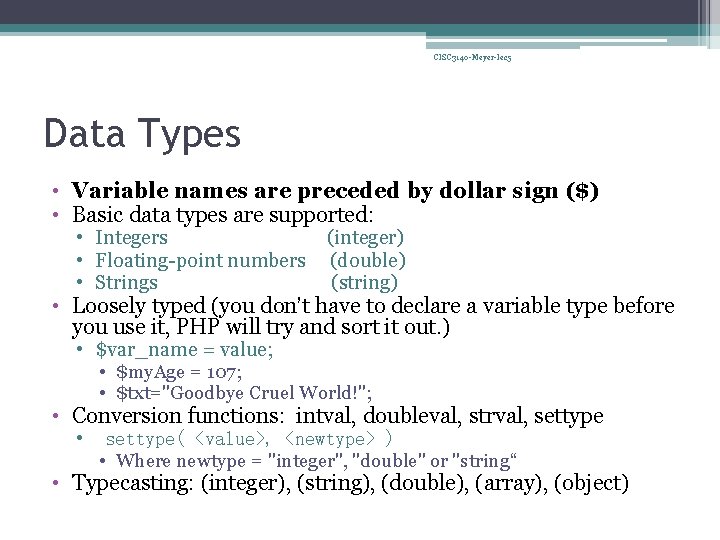
CISC 3140 -Meyer-lec 5 Data Types • Variable names are preceded by dollar sign ($) • Basic data types are supported: • Integers • Floating-point numbers • Strings (integer) (double) (string) • Loosely typed (you don’t have to declare a variable type before you use it, PHP will try and sort it out. ) • $var_name = value; • $my. Age = 107; • $txt="Goodbye Cruel World!"; • Conversion functions: intval, doubleval, strval, settype • settype( <value>, <newtype> ) • Where newtype = "integer", "double" or "string“ • Typecasting: (integer), (string), (double), (array), (object)
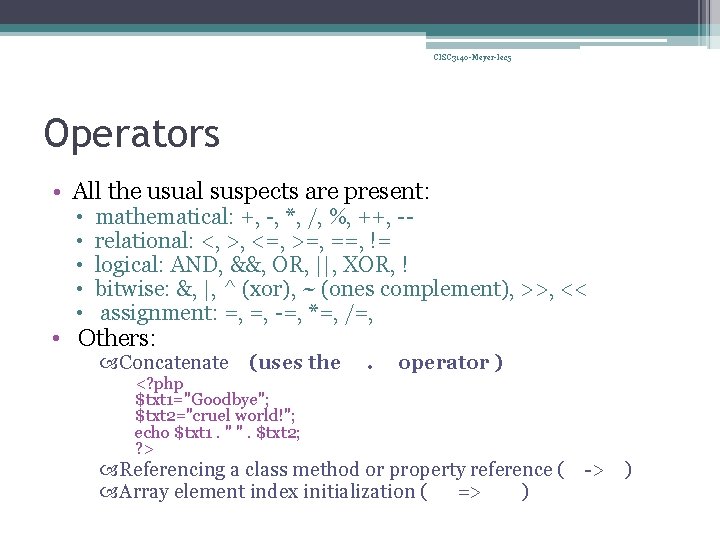
CISC 3140 -Meyer-lec 5 Operators • All the usual suspects are present: • • • mathematical: +, -, *, /, %, ++, -relational: <, >, <=, >=, ==, != logical: AND, &&, OR, ||, XOR, ! bitwise: &, |, ^ (xor), ~ (ones complement), >>, << assignment: =, =, -=, *=, /=, • Others: Concatenate (uses the <? php $txt 1="Goodbye"; $txt 2="cruel world!"; echo $txt 1. " ". $txt 2; ? > . operator ) Referencing a class method or property reference ( -> ) Array element index initialization ( => )
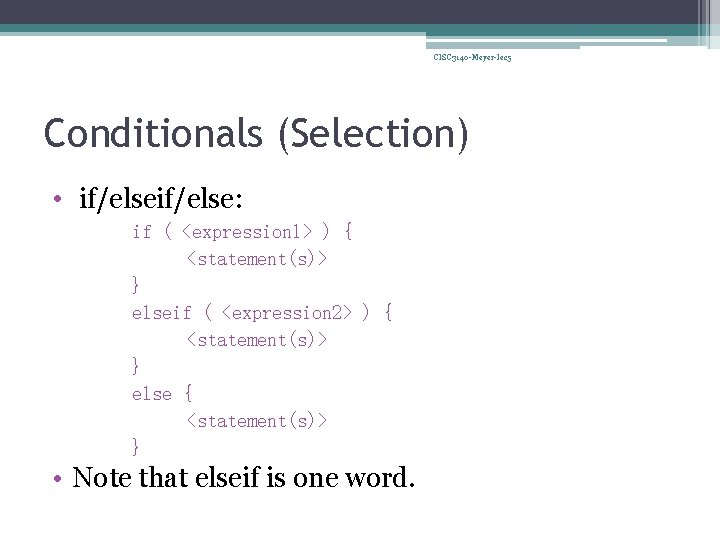
CISC 3140 -Meyer-lec 5 Conditionals (Selection) • if/else: if ( <expression 1> ) { <statement(s)> } elseif ( <expression 2> ) { <statement(s)> } else { <statement(s)> } • Note that elseif is one word.
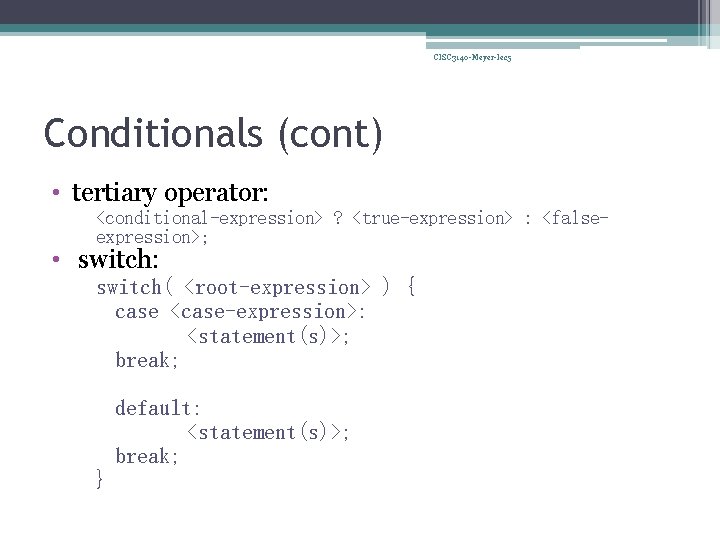
CISC 3140 -Meyer-lec 5 Conditionals (cont) • tertiary operator: <conditional-expression> ? <true-expression> : <falseexpression>; • switch: switch( <root-expression> ) { case <case-expression>: <statement(s)>; break; default: <statement(s)>; break; }
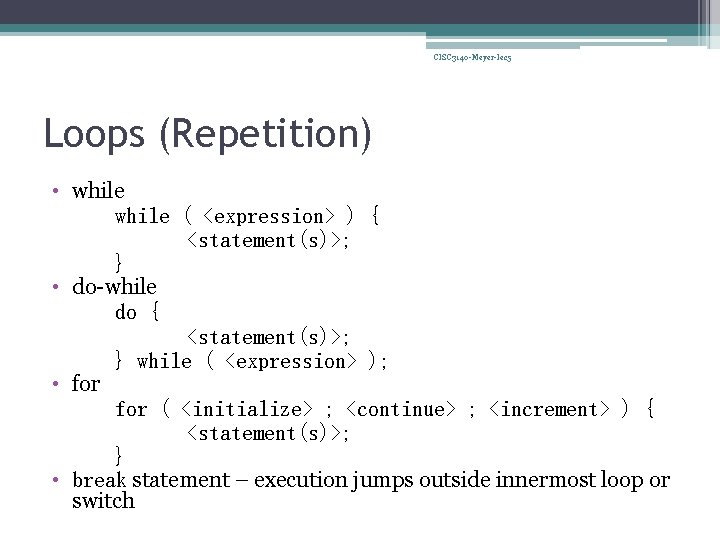
CISC 3140 -Meyer-lec 5 Loops (Repetition) • while ( <expression> ) { <statement(s)>; } • do-while do { <statement(s)>; } while ( <expression> ); • for ( <initialize> ; <continue> ; <increment> ) { <statement(s)>; } • break statement – execution jumps outside innermost loop or switch
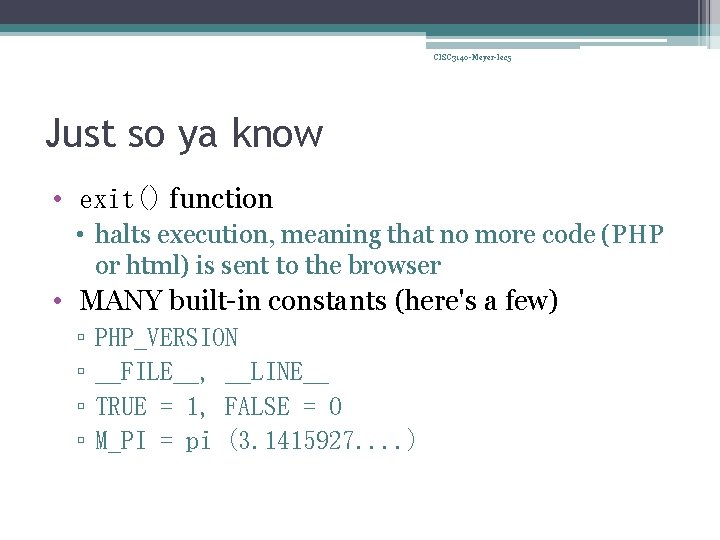
CISC 3140 -Meyer-lec 5 Just so ya know • exit() function • halts execution, meaning that no more code (PHP or html) is sent to the browser • MANY built-in constants (here's a few) ▫ ▫ PHP_VERSION __FILE__, __LINE__ TRUE = 1, FALSE = 0 M_PI = pi (3. 1415927. . )
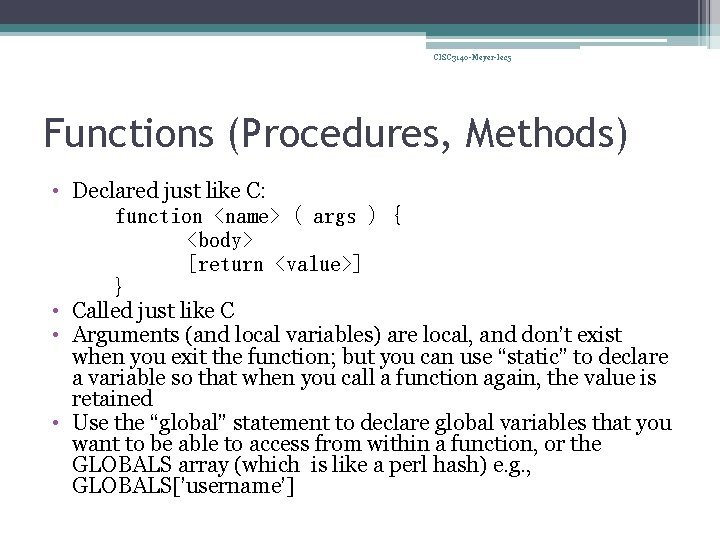
CISC 3140 -Meyer-lec 5 Functions (Procedures, Methods) • Declared just like C: function <name> ( args ) { <body> [return <value>] } • Called just like C • Arguments (and local variables) are local, and don’t exist when you exit the function; but you can use “static” to declare a variable so that when you call a function again, the value is retained • Use the “global” statement to declare global variables that you want to be able to access from within a function, or the GLOBALS array (which is like a perl hash) e. g. , GLOBALS[’username’]
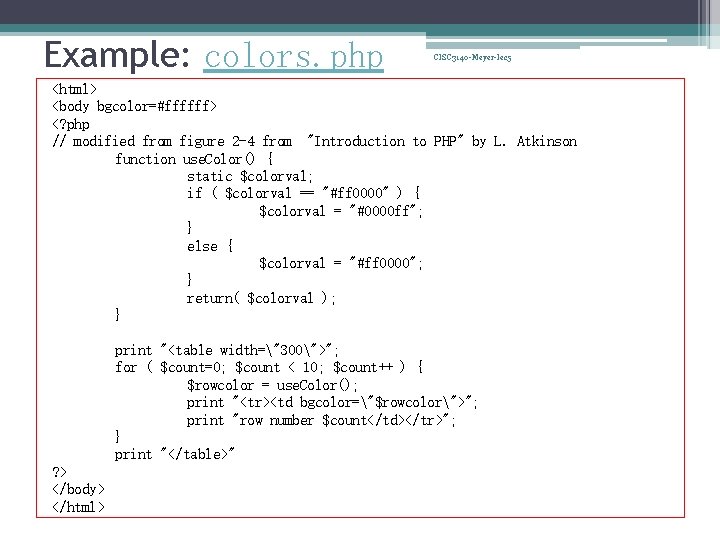
Example: colors. php CISC 3140 -Meyer-lec 5 <html> <body bgcolor=#ffffff> <? php // modified from figure 2 -4 from "Introduction to PHP" by L. Atkinson function use. Color() { static $colorval; if ( $colorval == "#ff 0000" ) { $colorval = "#0000 ff"; } else { $colorval = "#ff 0000"; } return( $colorval ); } print "<table width="300">"; for ( $count=0; $count < 10; $count++ ) { $rowcolor = use. Color(); print "<tr><td bgcolor="$rowcolor">"; print "row number $count</td></tr>"; } print "</table>" ? > </body> </html>
![CISC 3140 Meyerlec 5 Arrays Hurray Indexed using CISC 3140 -Meyer-lec 5 Arrays (Hurray!) • Indexed using [. . . ] •](https://slidetodoc.com/presentation_image_h2/18c5769f129ee0b22d554ccdd05fb0d1/image-17.jpg)
CISC 3140 -Meyer-lec 5 Arrays (Hurray!) • Indexed using [. . . ] • Indices can be integers or strings (like a Perl hash, or MAP class) • When strings are the Indices , it’s called an “associative array” • array() function can be used to initialize an array i. e. $var = array( value 0, value 1, . . . ); • Use the => operator to define the index: ▫ $var = array( 1=>value 1, value 2, . . . ); ▫ $var = array( "a"=>value 1, "b"=>value 2, . . . ); • Multidimensional arrays allowed as well
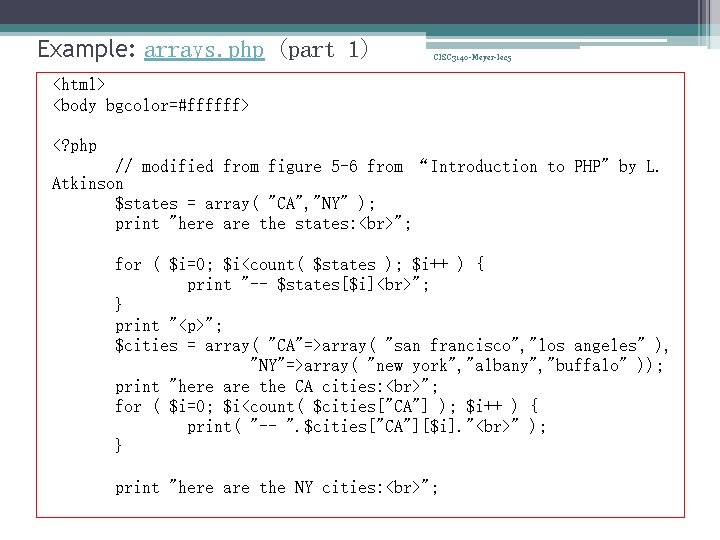
Example: arrays. php (part 1) CISC 3140 -Meyer-lec 5 <html> <body bgcolor=#ffffff> <? php // modified from figure 5 -6 from “Introduction to PHP" by L. Atkinson $states = array( "CA", "NY" ); print "here are the states: "; for ( $i=0; $i<count( $states ); $i++ ) { print "-- $states[$i] "; } print "<p>"; $cities = array( "CA"=>array( "san francisco", "los angeles" ), "NY"=>array( "new york", "albany", "buffalo" )); print "here are the CA cities: "; for ( $i=0; $i<count( $cities["CA"] ); $i++ ) { print( "-- ". $cities["CA"][$i]. " " ); } print "here are the NY cities: ";
![Example arrays php part 2 CISC 3140 Meyerlec 5 for i0 icount citiesNY Example: arrays. php (part 2) CISC 3140 -Meyer-lec 5 for ( $i=0; $i<count( $cities["NY"]](https://slidetodoc.com/presentation_image_h2/18c5769f129ee0b22d554ccdd05fb0d1/image-19.jpg)
Example: arrays. php (part 2) CISC 3140 -Meyer-lec 5 for ( $i=0; $i<count( $cities["NY"] ); $i++ ) { print( "-- ". $cities["NY"][$i]. " " ); } print "<p>"; $states[] = "MA"; print "now here are the states: "; for ( $i=0; $i<count( $states ); $i++ ) { print "-- $states[$i] "; } $cities[] = "MA"; $cities["MA"][] = "boston"; print "here are the MA cities: "; for ( $i=0; $i<count( $cities["MA"] ); $i++ ) { print( "-- ". $cities["MA"][$i]. " " ); } ? > </body> </html>
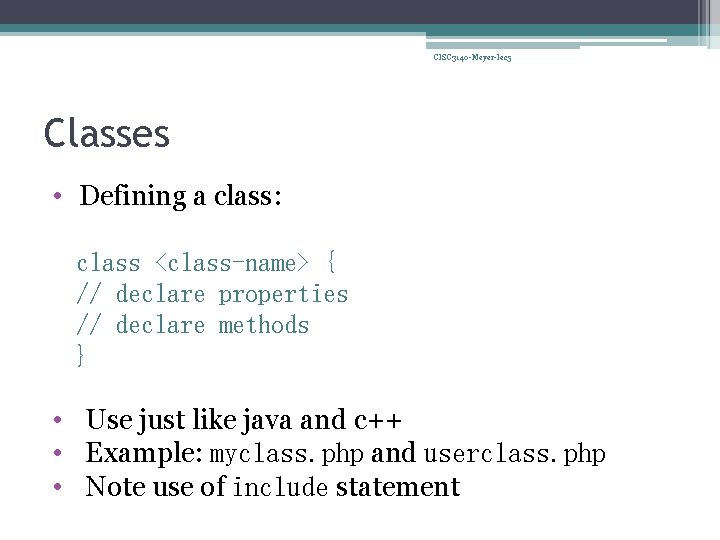
CISC 3140 -Meyer-lec 5 Classes • Defining a class: class <class-name> { // declare properties // declare methods } • Use just like java and c++ • Example: myclass. php and userclass. php • Note use of include statement
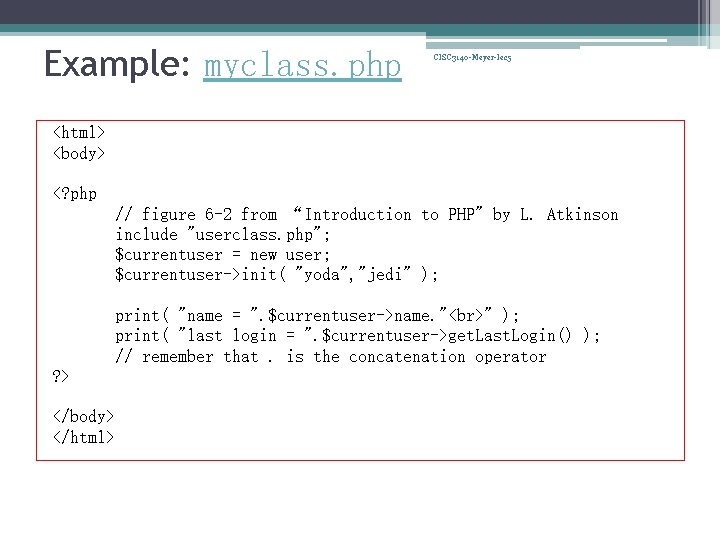
Example: myclass. php CISC 3140 -Meyer-lec 5 <html> <body> <? php // figure 6 -2 from “Introduction to PHP" by L. Atkinson include "userclass. php"; $currentuser = new user; $currentuser->init( "yoda", "jedi" ); print( "name = ". $currentuser->name. " " ); print( "last login = ". $currentuser->get. Last. Login() ); // remember that. is the concatenation operator ? > </body> </html>
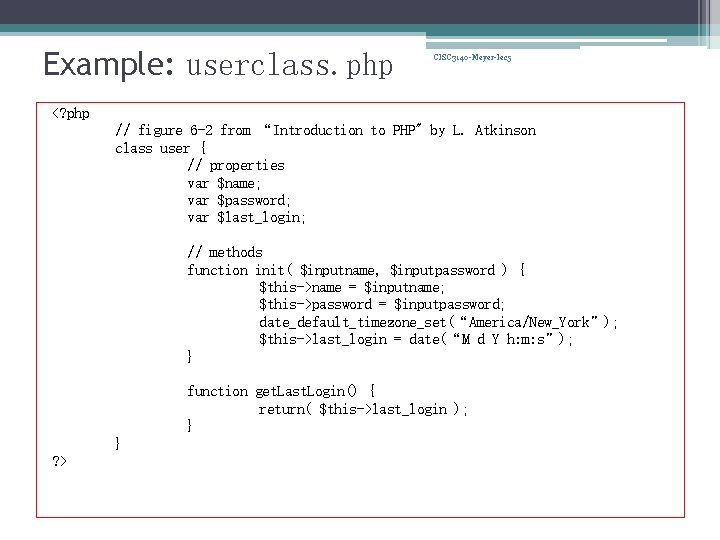
Example: userclass. php CISC 3140 -Meyer-lec 5 <? php // figure 6 -2 from “Introduction to PHP" by L. Atkinson class user { // properties var $name; var $password; var $last_login; // methods function init( $inputname, $inputpassword ) { $this->name = $inputname; $this->password = $inputpassword; date_default_timezone_set(“America/New_York”); $this->last_login = date(“M d Y h: m: s”); } function get. Last. Login() { return( $this->last_login ); } } ? >
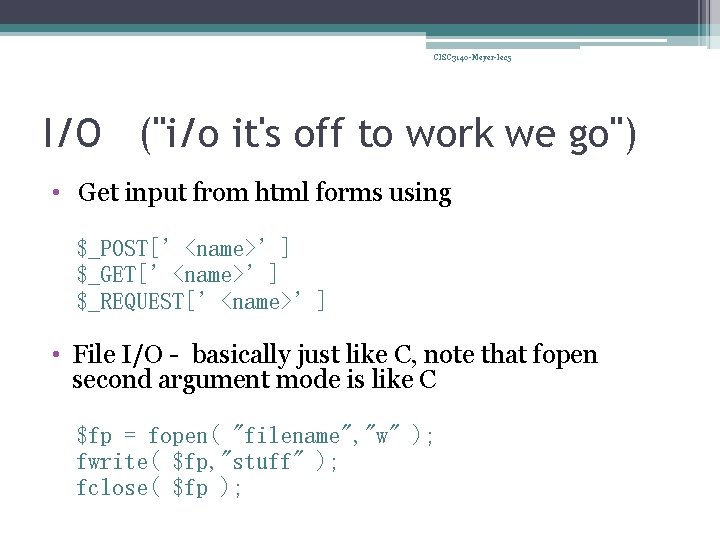
CISC 3140 -Meyer-lec 5 I/O ("i/o it's off to work we go") • Get input from html forms using $_POST[’<name>’] $_GET[’<name>’] $_REQUEST[’<name>’] • File I/O - basically just like C, note that fopen second argument mode is like C $fp = fopen( "filename", "w" ); fwrite( $fp, "stuff" ); fclose( $fp );
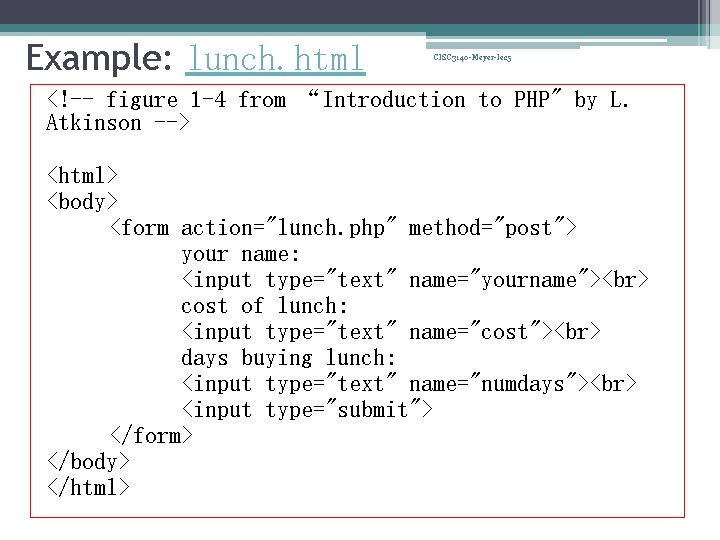
Example: lunch. html CISC 3140 -Meyer-lec 5 <!-- figure 1 -4 from “Introduction to PHP" by L. Atkinson --> <html> <body> <form action="lunch. php" method="post"> your name: <input type="text" name="yourname"> cost of lunch: <input type="text" name="cost"> days buying lunch: <input type="text" name="numdays"> <input type="submit"> </form> </body> </html>
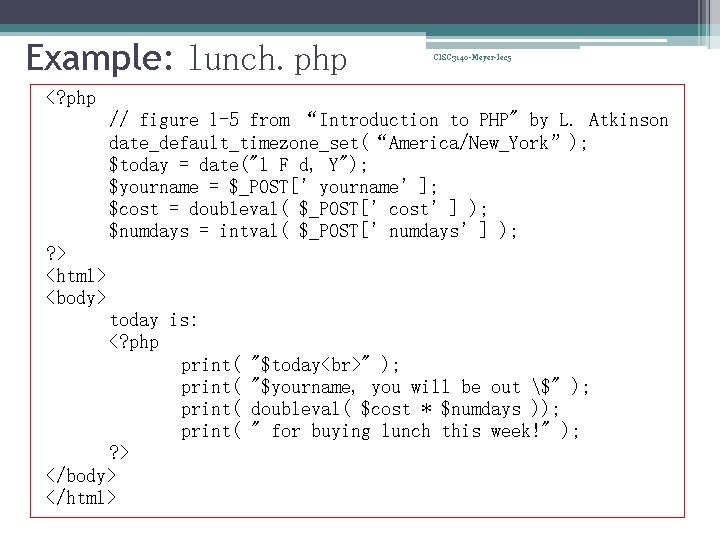
Example: lunch. php CISC 3140 -Meyer-lec 5 <? php // figure 1 -5 from “Introduction to PHP" by L. Atkinson date_default_timezone_set(“America/New_York”); $today = date("l F d, Y"); $yourname = $_POST[’yourname’]; $cost = doubleval( $_POST[’cost’] ); $numdays = intval( $_POST[’numdays’] ); ? > <html> <body> today is: <? php print( ? > </body> </html> "$today " ); "$yourname, you will be out $" ); doubleval( $cost * $numdays )); " for buying lunch this week!" );
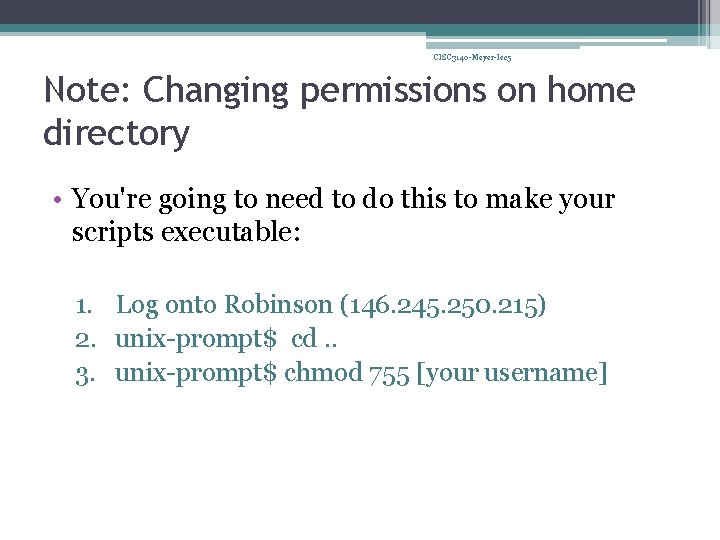
CISC 3140 -Meyer-lec 5 Note: Changing permissions on home directory • You're going to need to do this to make your scripts executable: 1. Log onto Robinson (146. 245. 250. 215) 2. unix-prompt$ cd. . 3. unix-prompt$ chmod 755 [your username]
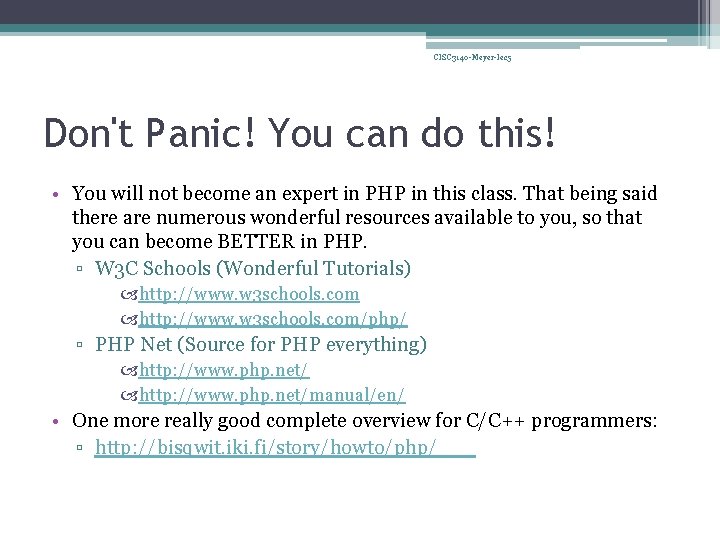
CISC 3140 -Meyer-lec 5 Don't Panic! You can do this! • You will not become an expert in PHP in this class. That being said there are numerous wonderful resources available to you, so that you can become BETTER in PHP. ▫ W 3 C Schools (Wonderful Tutorials) http: //www. w 3 schools. com/php/ ▫ PHP Net (Source for PHP everything) http: //www. php. net/manual/en/ • One more really good complete overview for C/C++ programmers: ▫ http: //bisqwit. iki. fi/story/howto/php/
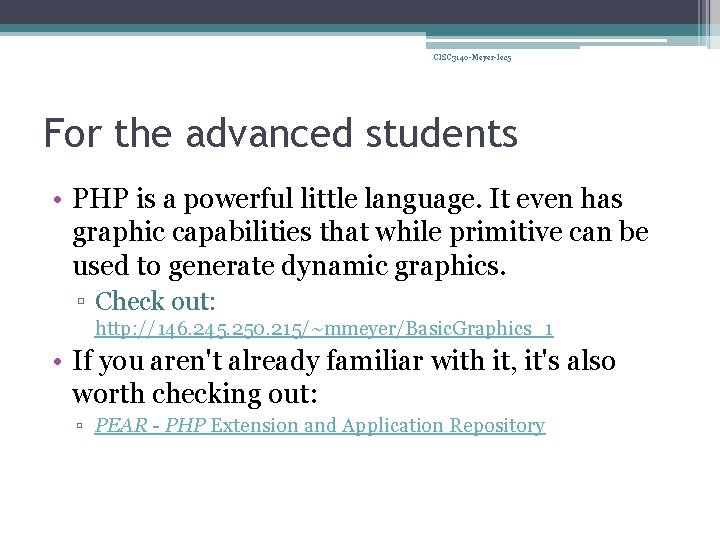
CISC 3140 -Meyer-lec 5 For the advanced students • PHP is a powerful little language. It even has graphic capabilities that while primitive can be used to generate dynamic graphics. ▫ Check out: http: //146. 245. 250. 215/~mmeyer/Basic. Graphics_1 • If you aren't already familiar with it, it's also worth checking out: ▫ PEAR - PHP Extension and Application Repository
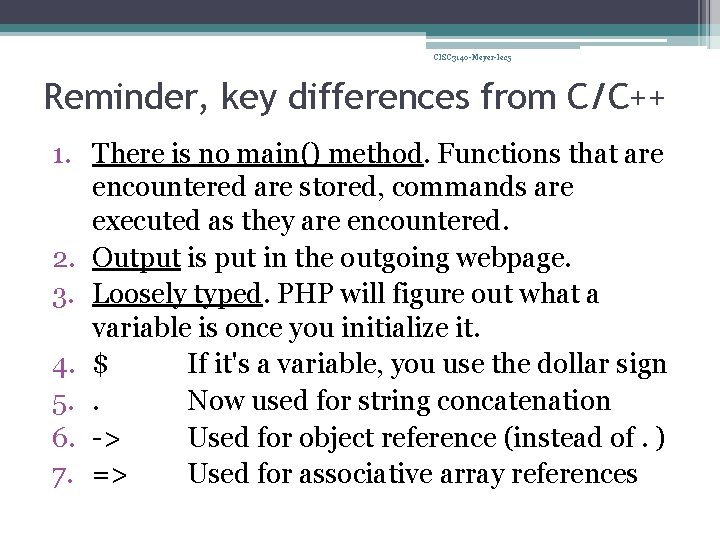
CISC 3140 -Meyer-lec 5 Reminder, key differences from C/C++ 1. There is no main() method. Functions that are encountered are stored, commands are executed as they are encountered. 2. Output is put in the outgoing webpage. 3. Loosely typed. PHP will figure out what a variable is once you initialize it. 4. $ If it's a variable, you use the dollar sign 5. . Now used for string concatenation 6. -> Used for object reference (instead of. ) 7. => Used for associative array references
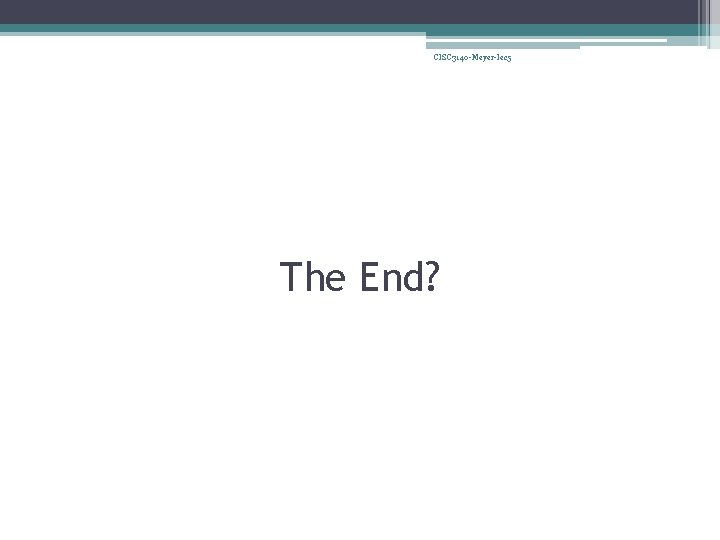
CISC 3140 -Meyer-lec 5 The End?