Wentworth Institute of Technology Engineering Technology WIT COMP
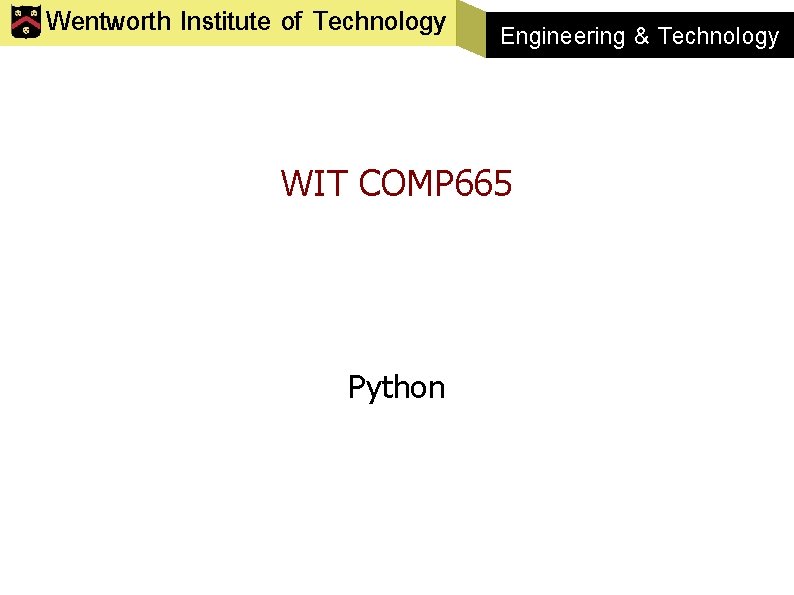
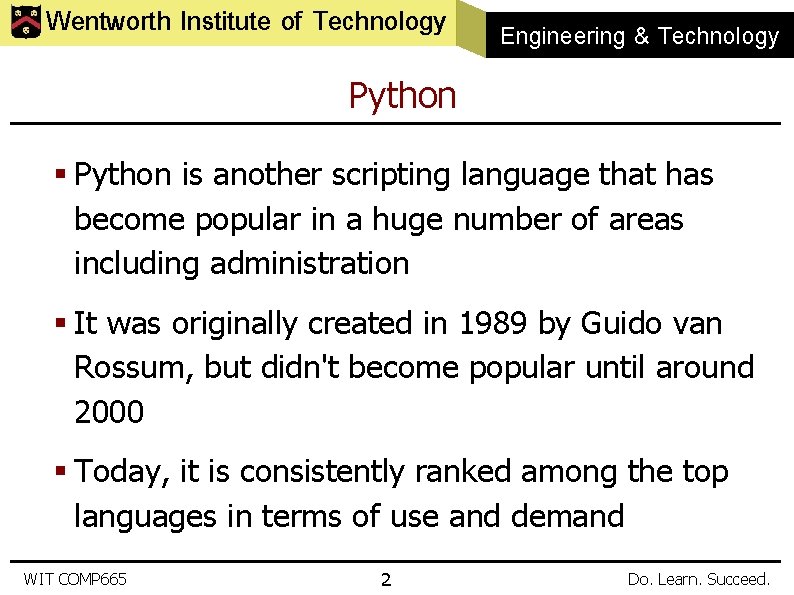
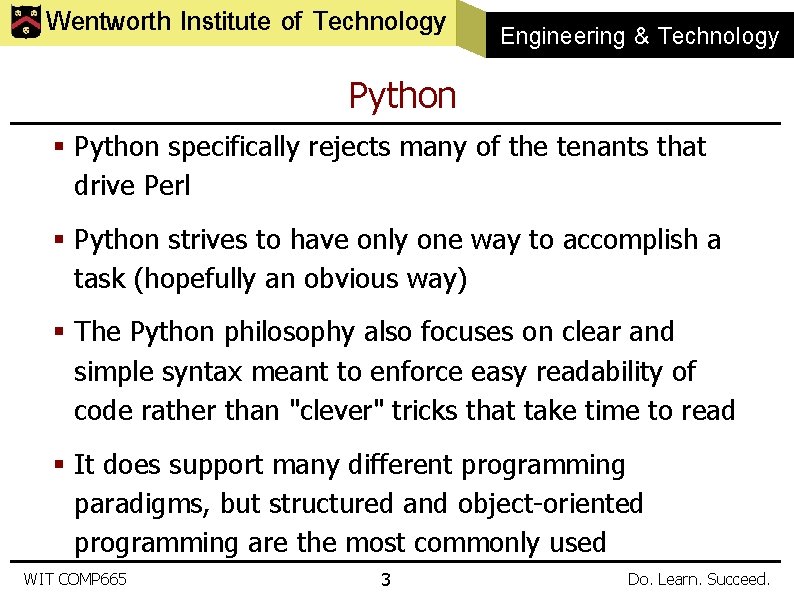
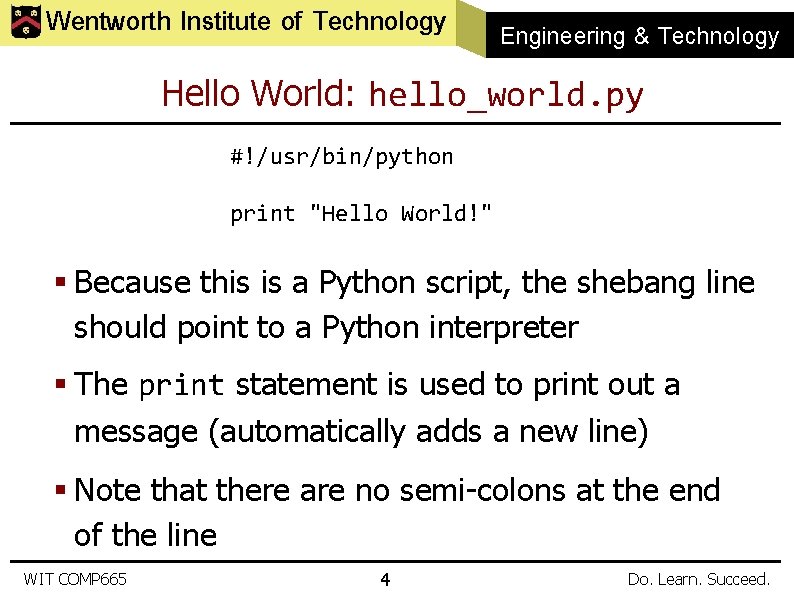
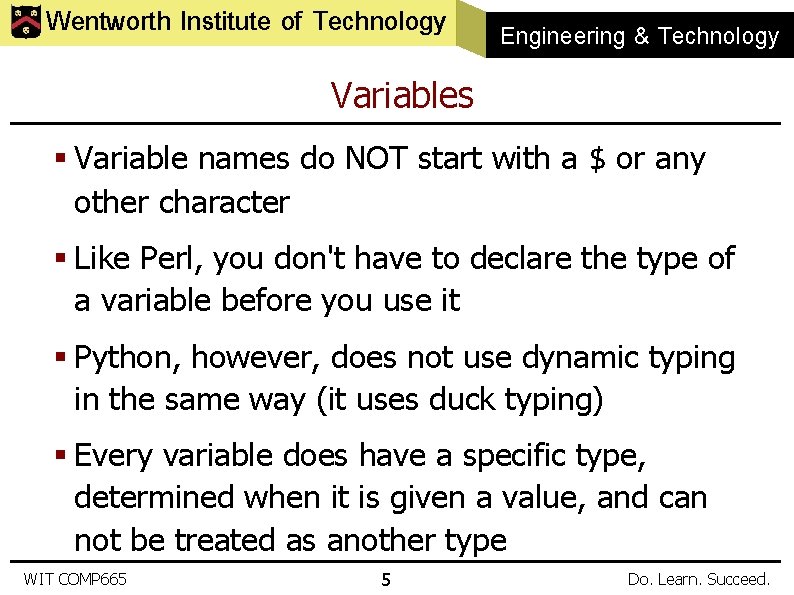
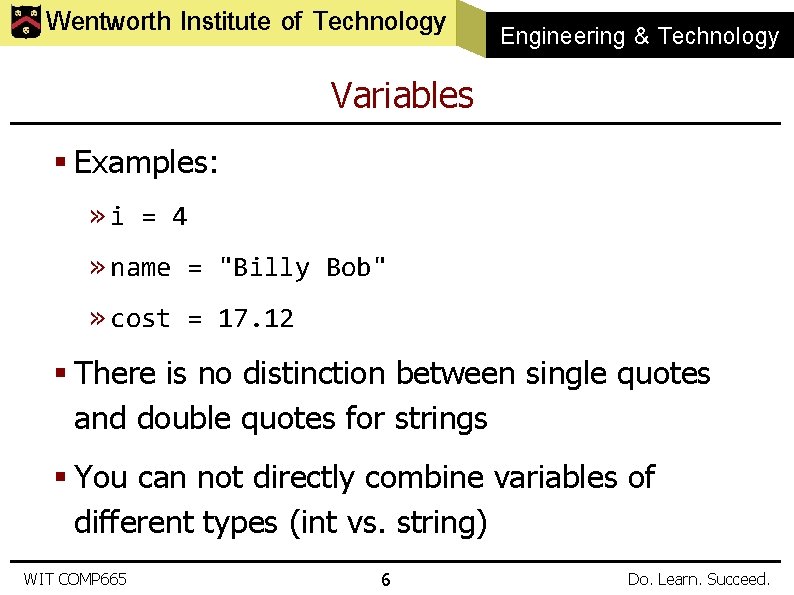
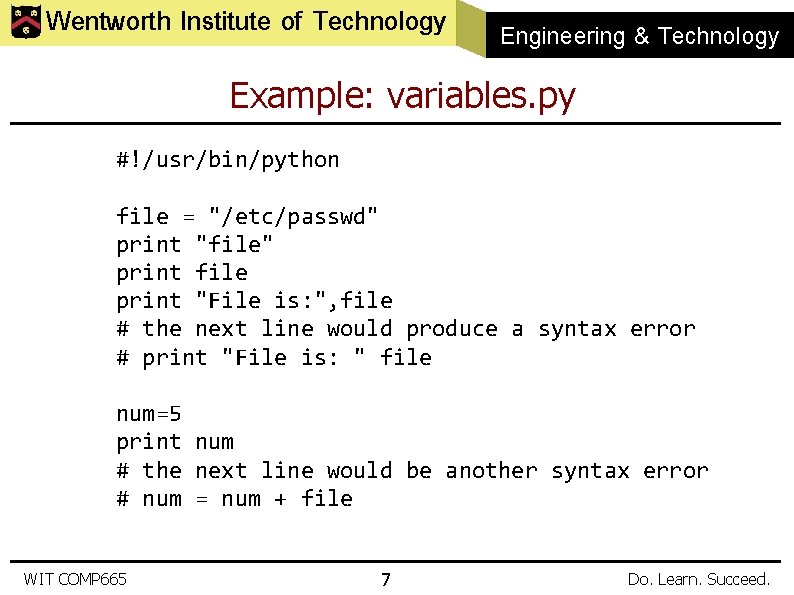
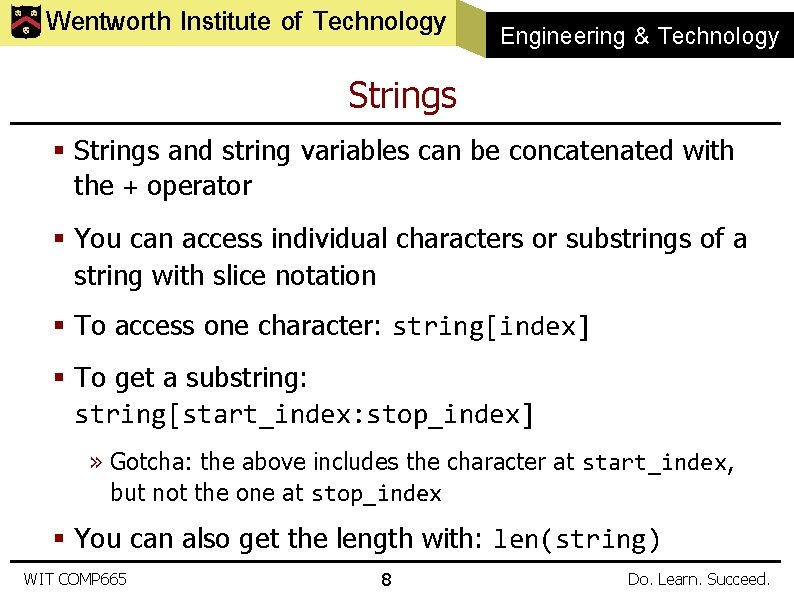
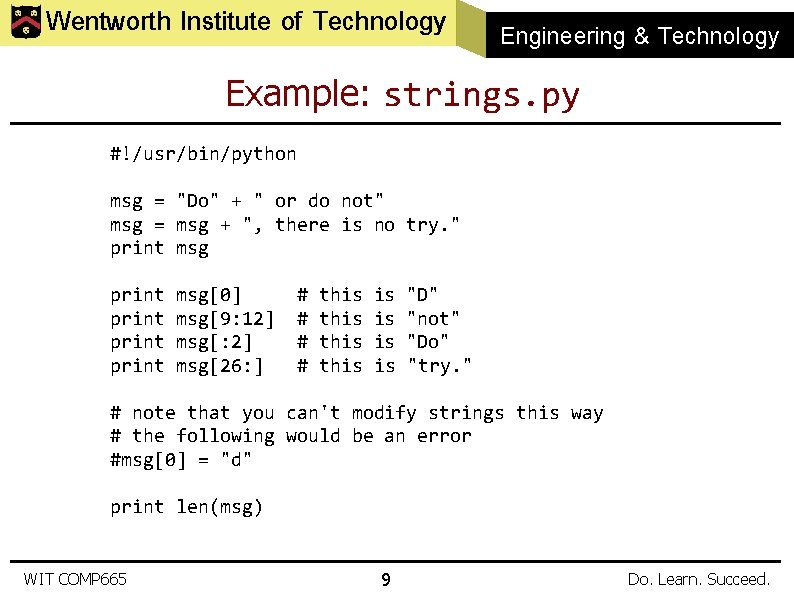
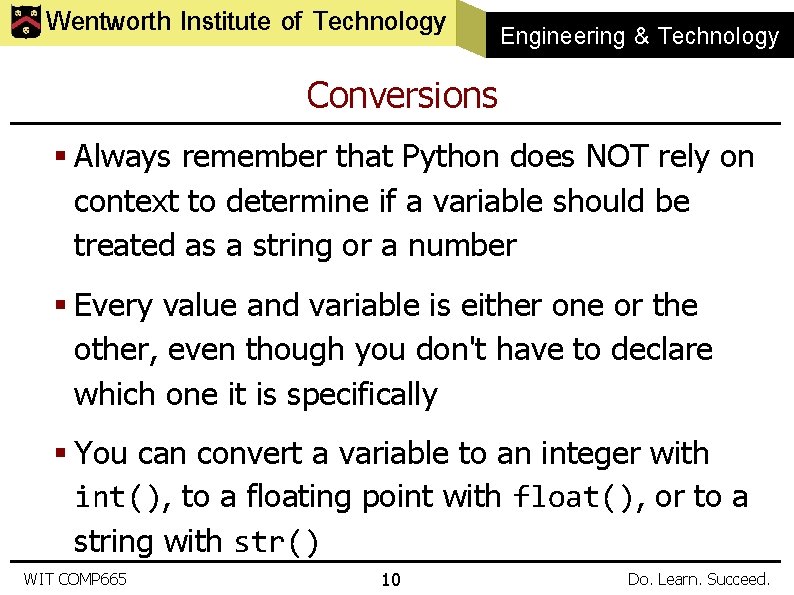
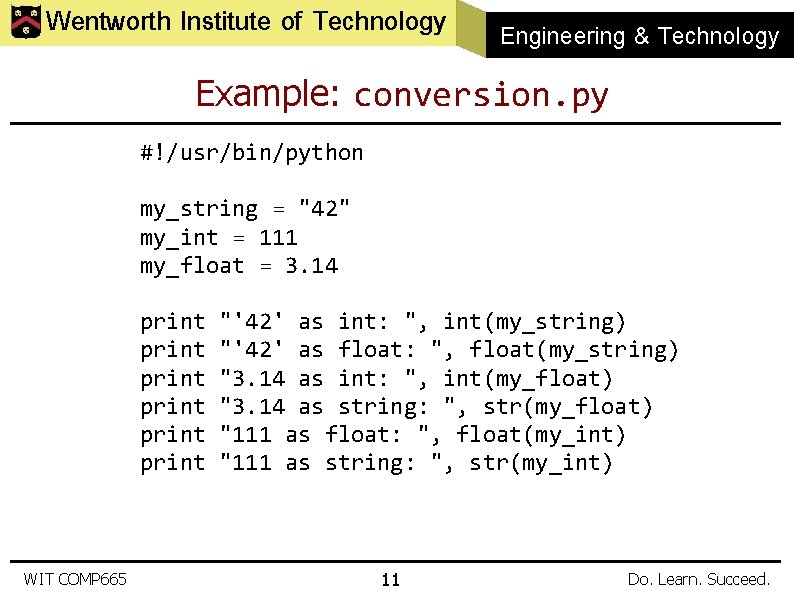
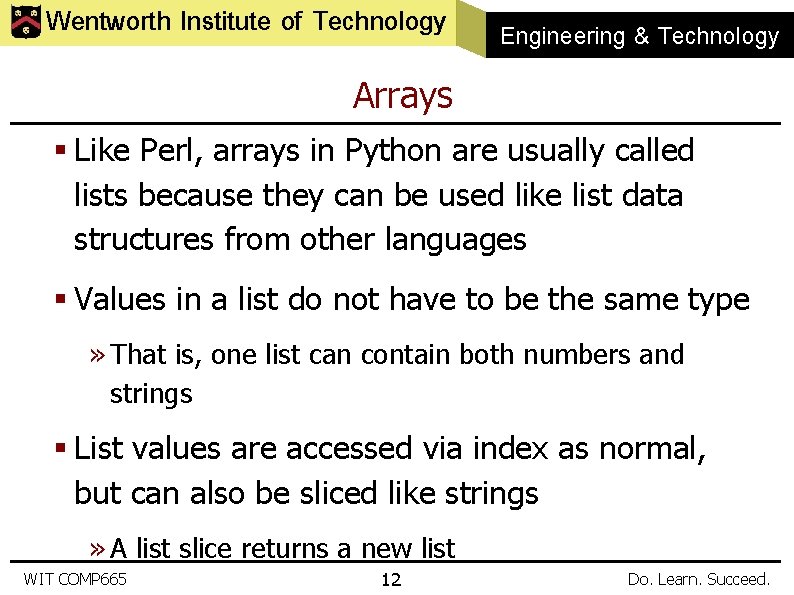
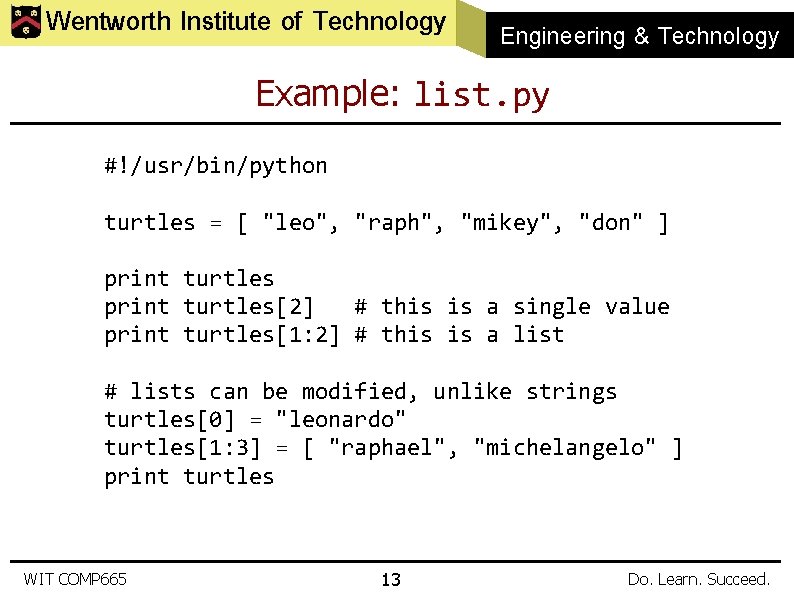
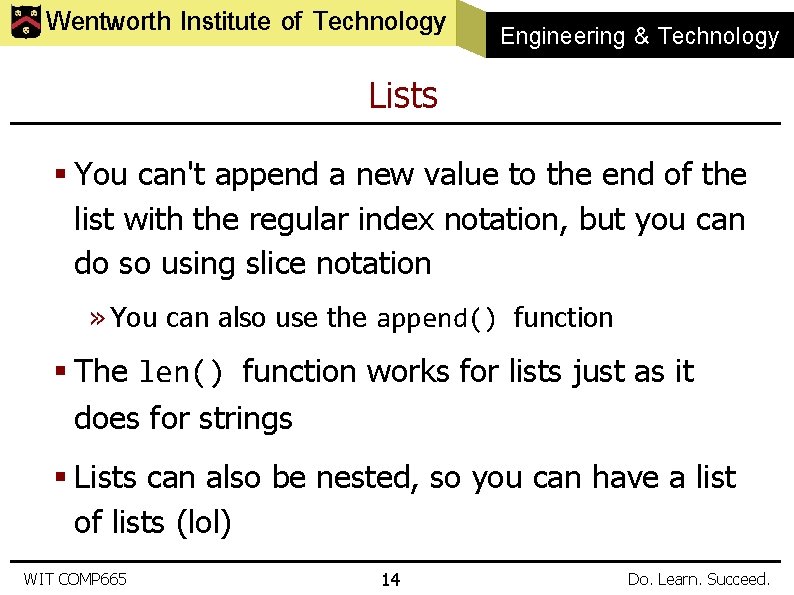
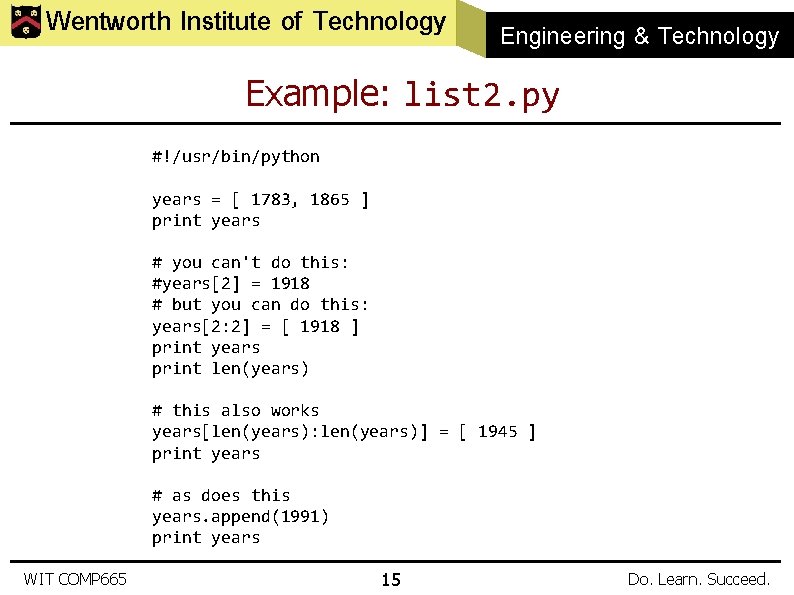
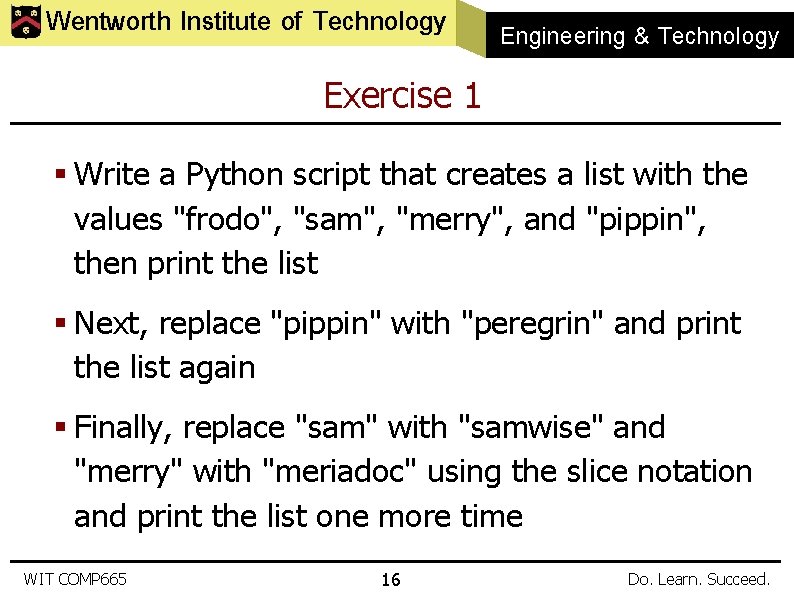
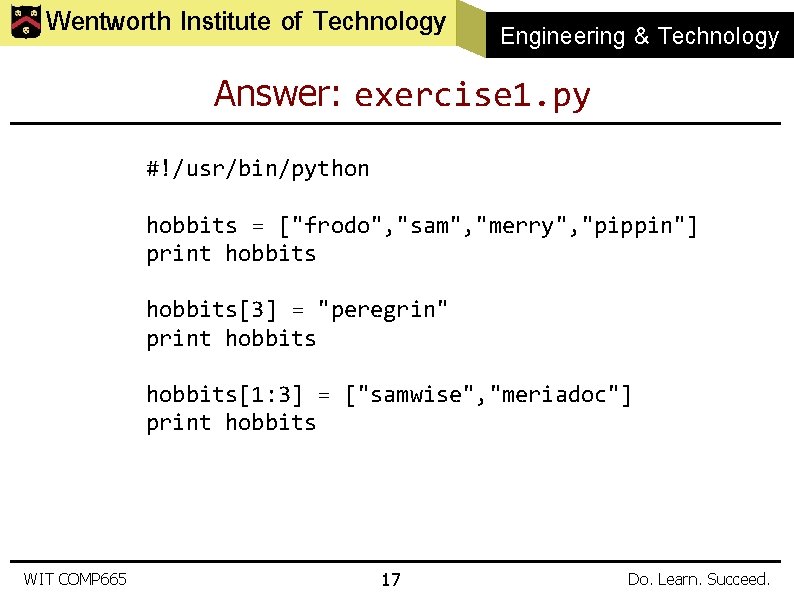
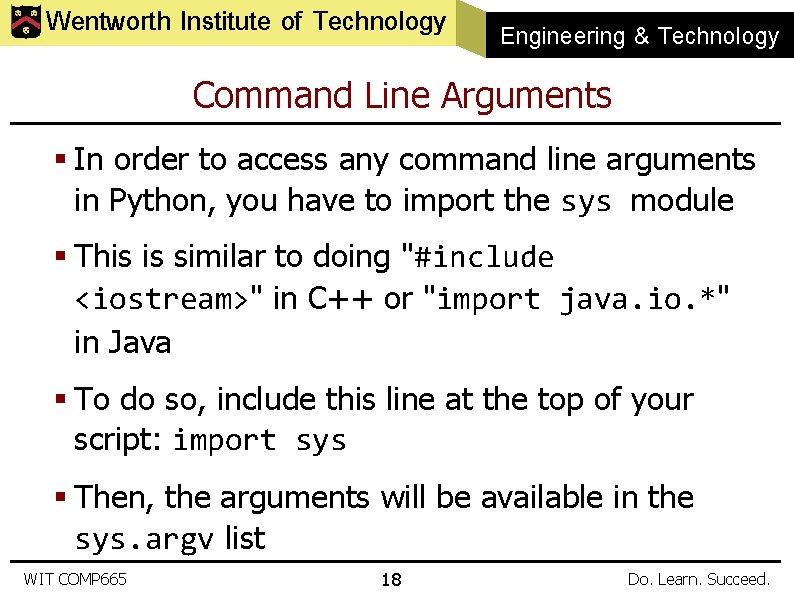
![Wentworth Institute of Technology Engineering & Technology Command Line Arguments § sys. argv[0] is Wentworth Institute of Technology Engineering & Technology Command Line Arguments § sys. argv[0] is](https://slidetodoc.com/presentation_image_h/21c1aba65de38f0c949520080952c8ea/image-19.jpg)
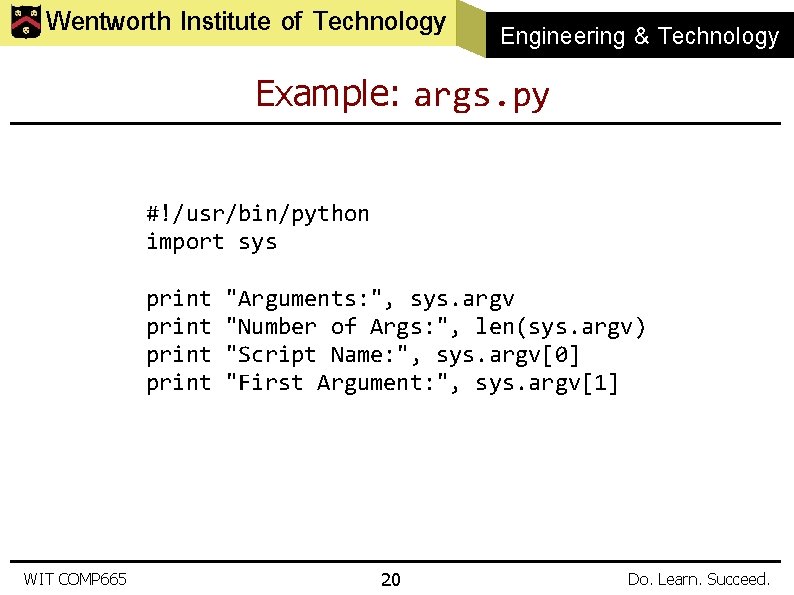
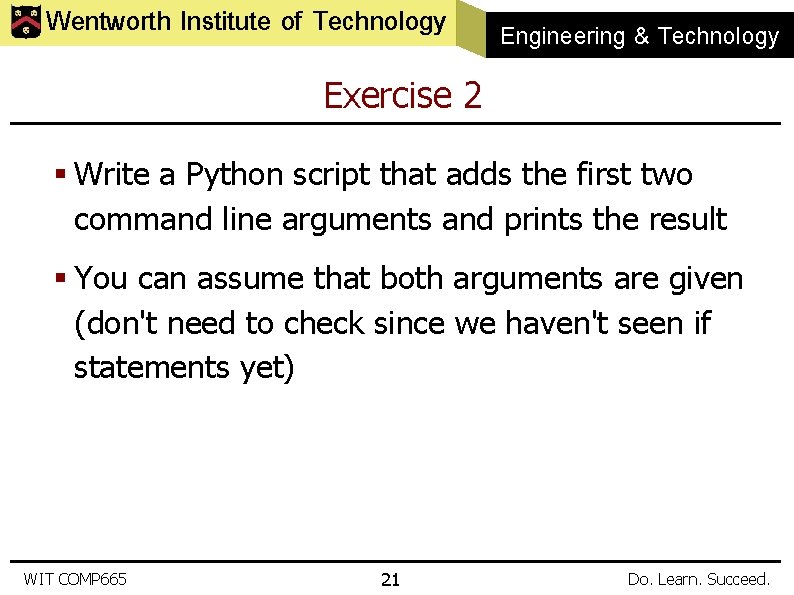
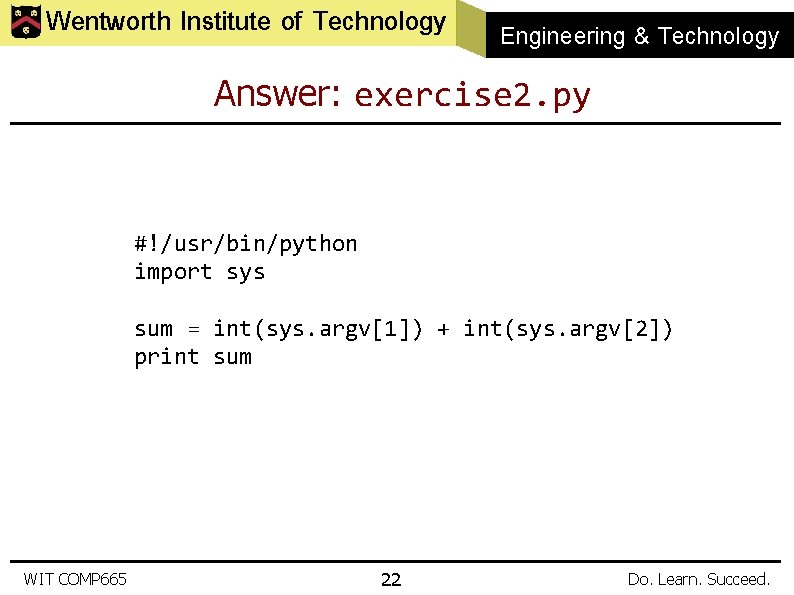
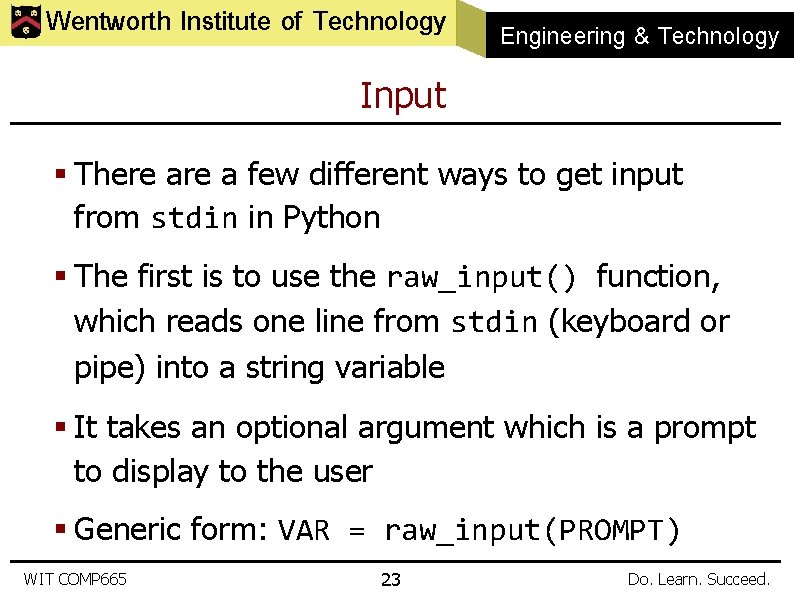
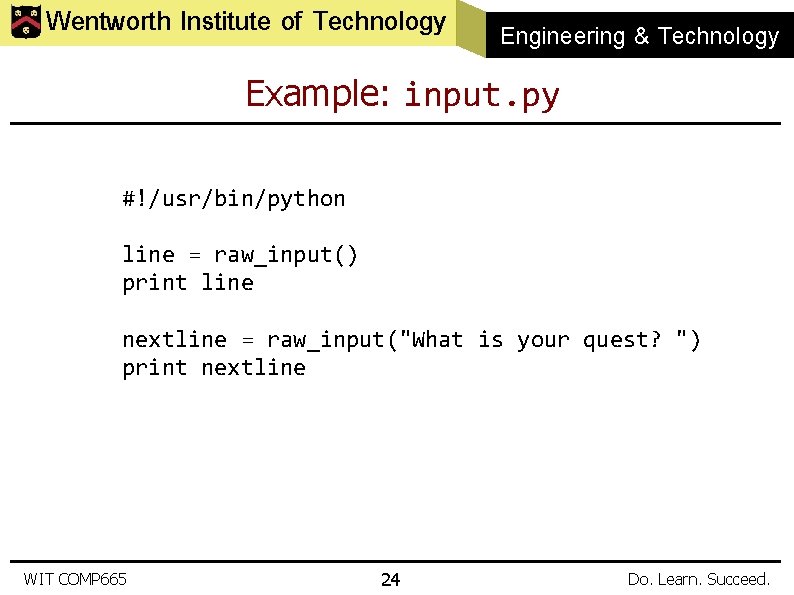
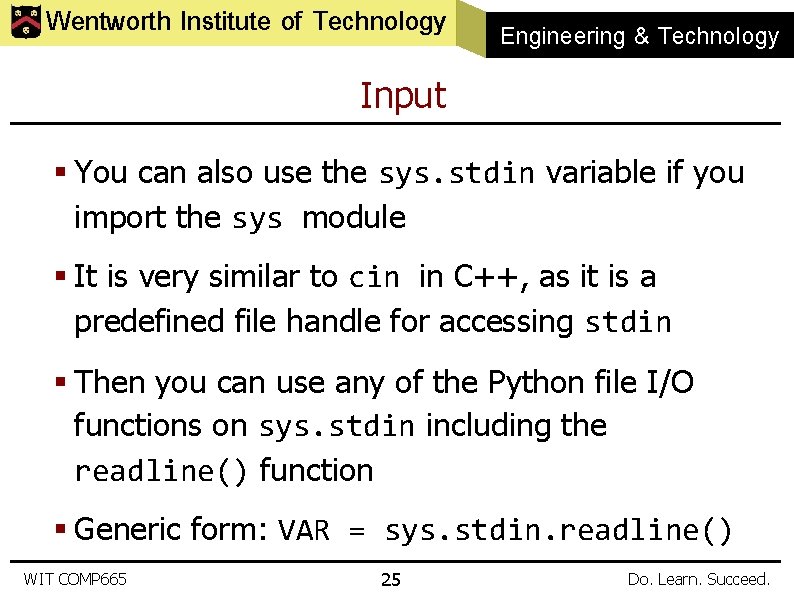
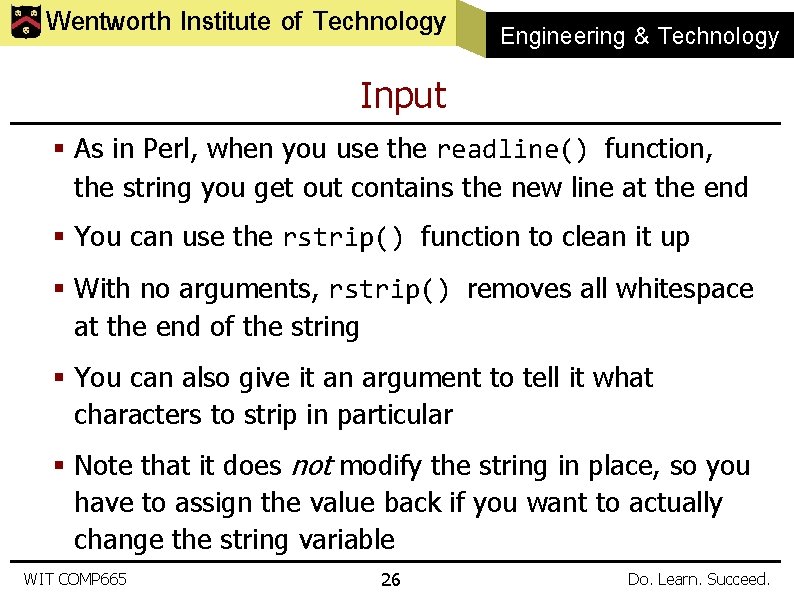
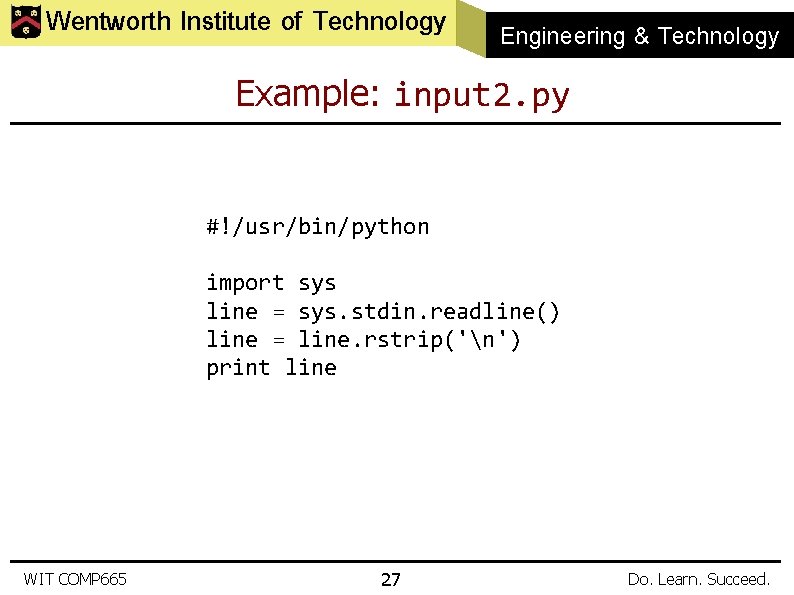
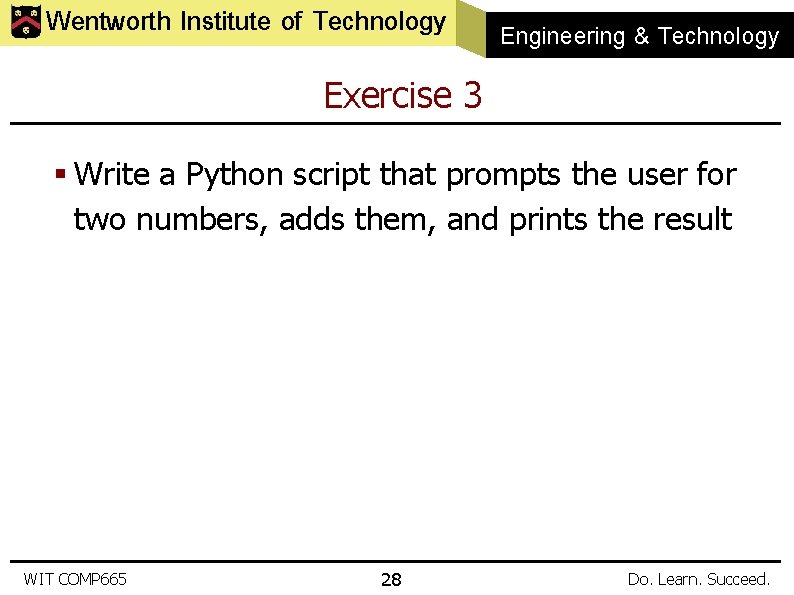
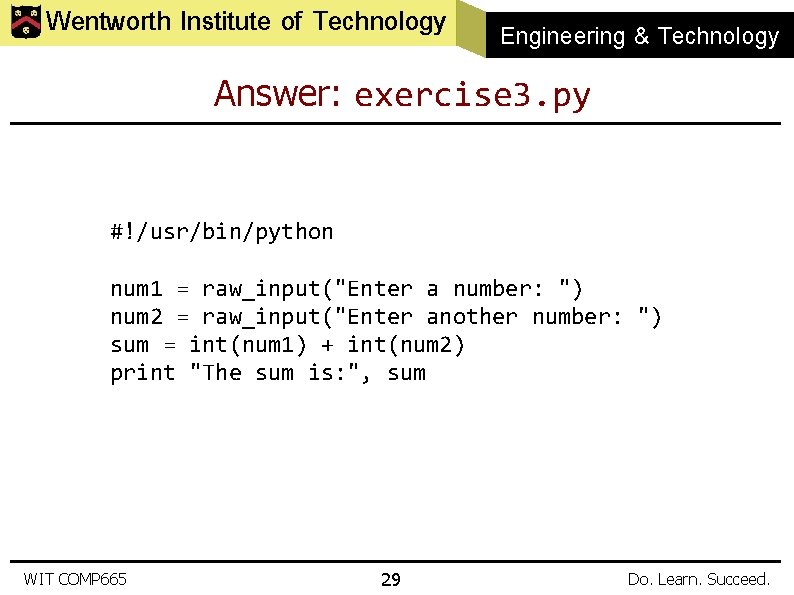
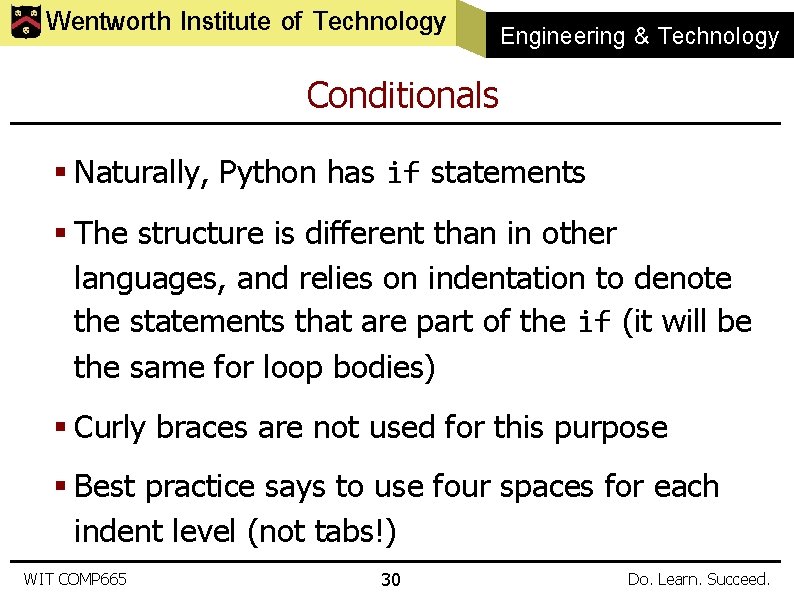
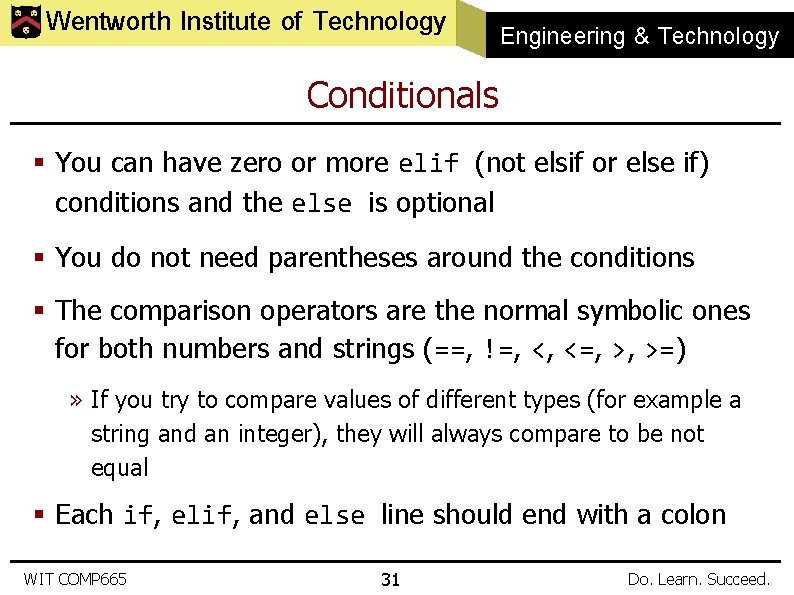
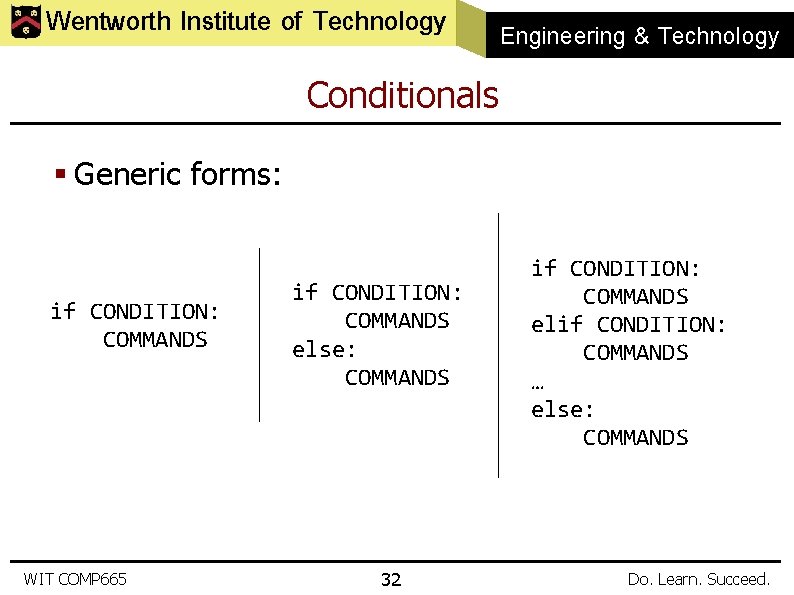
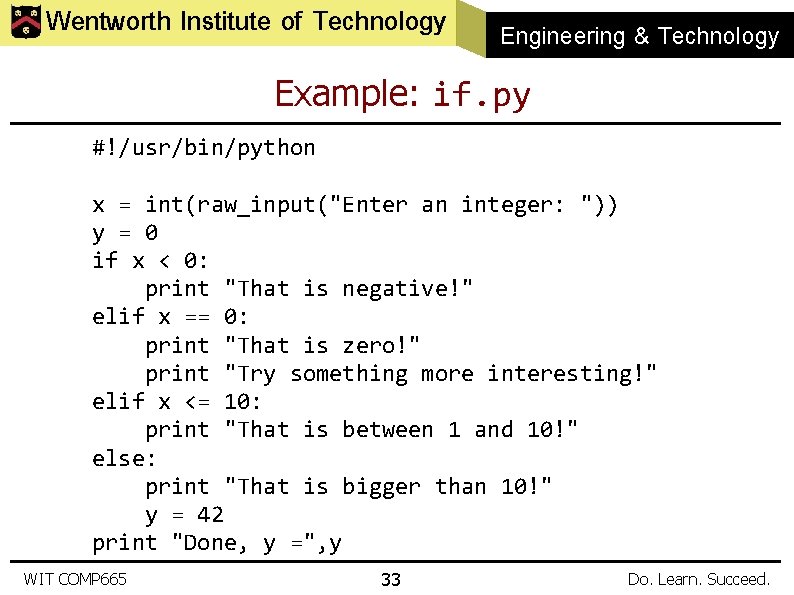
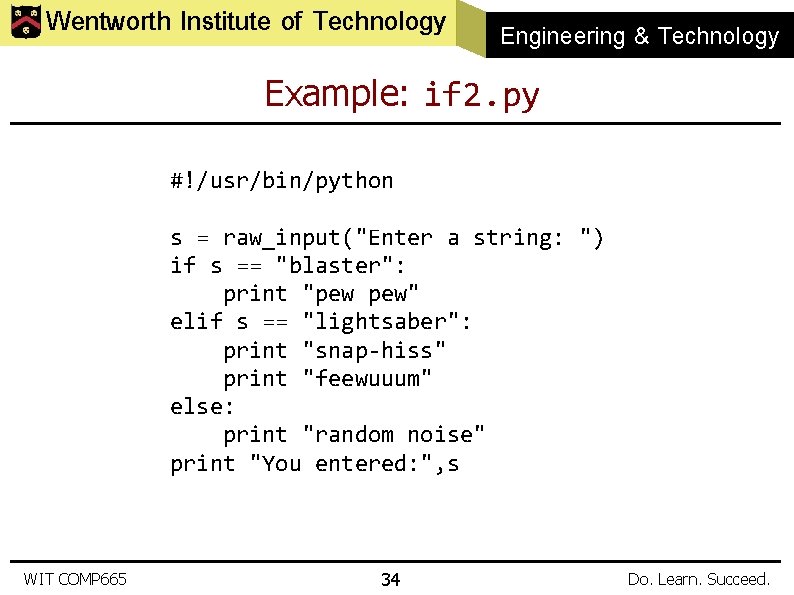
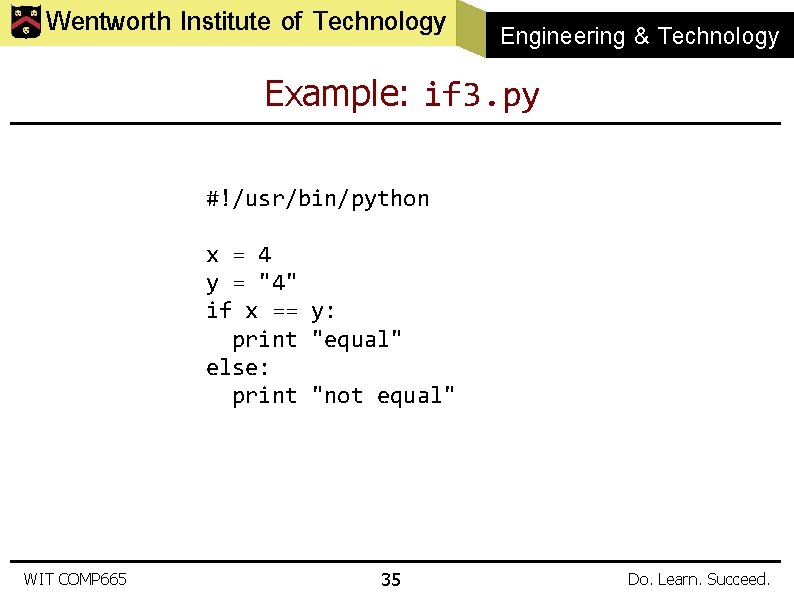
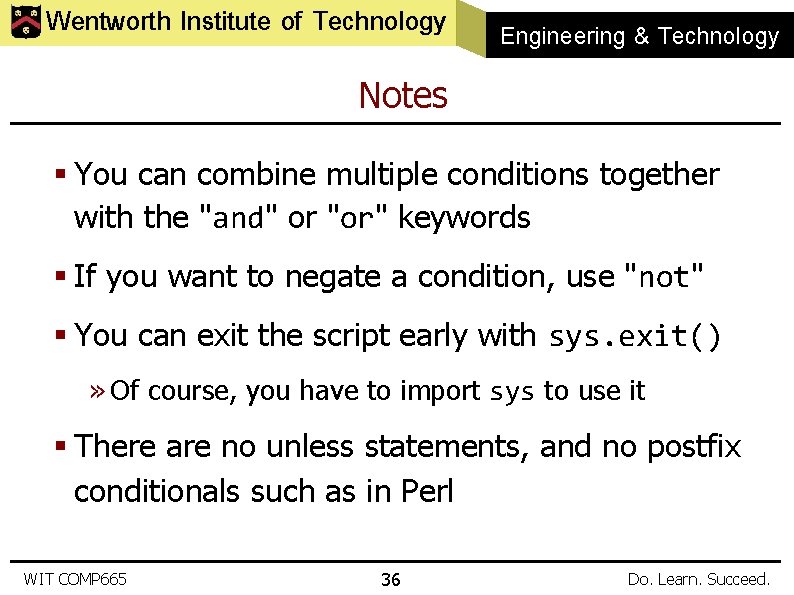
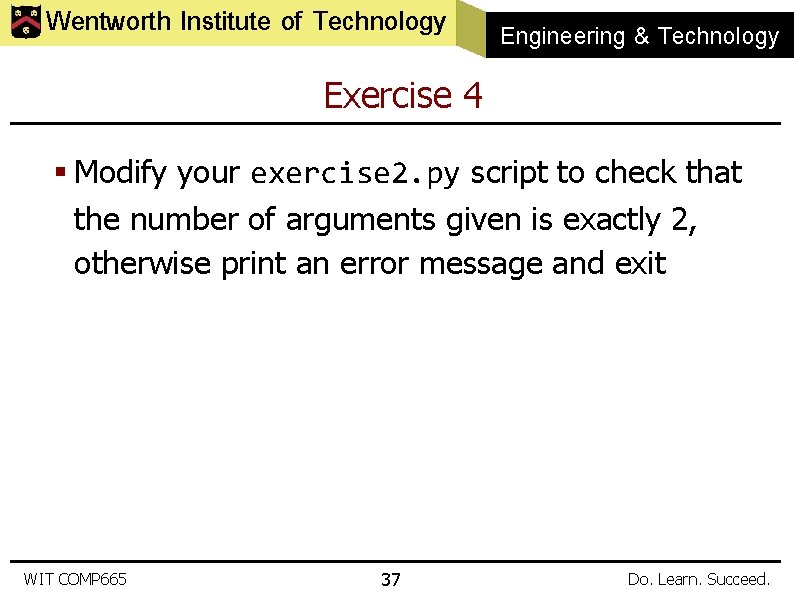
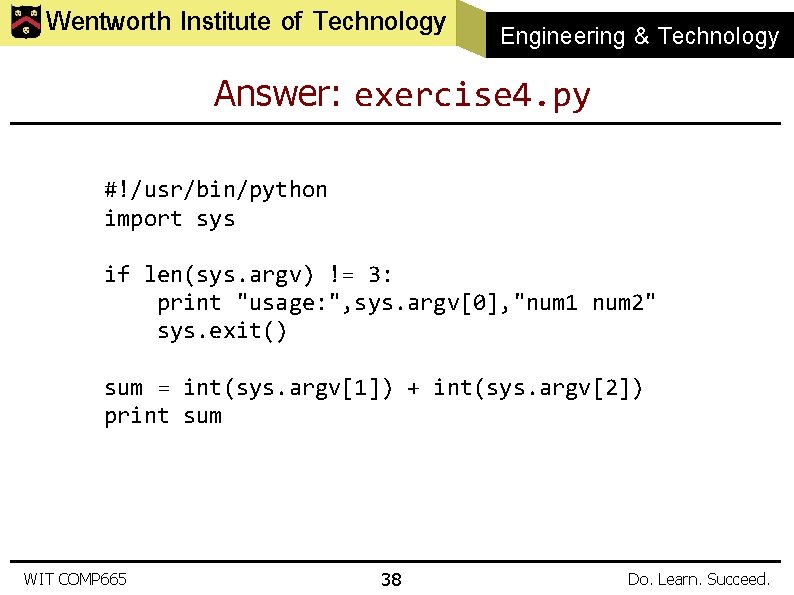
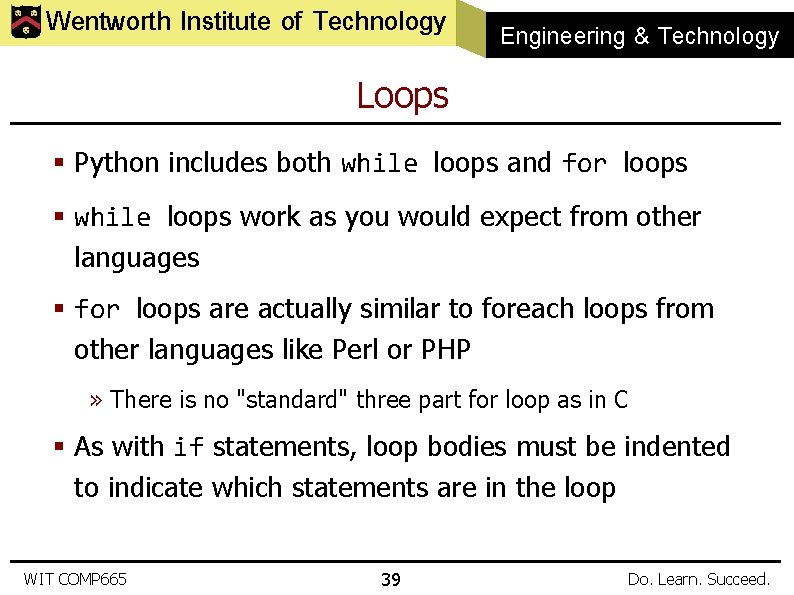
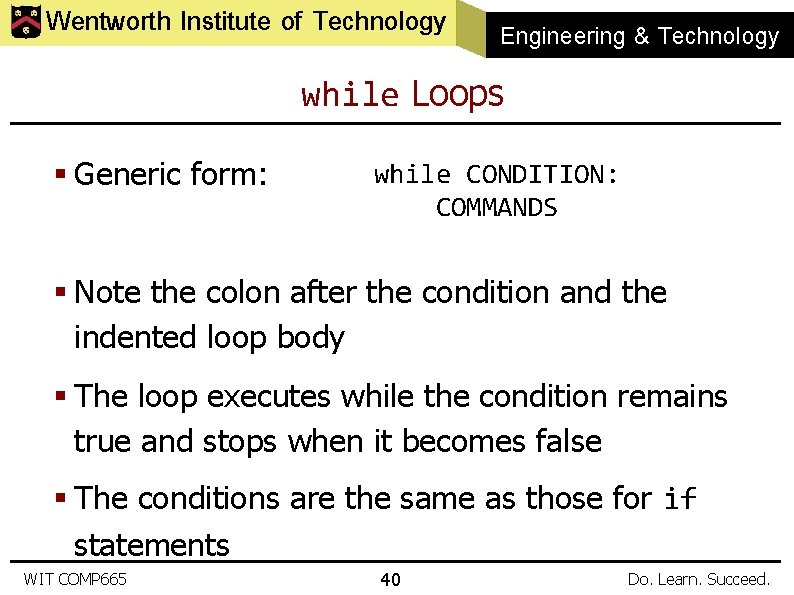
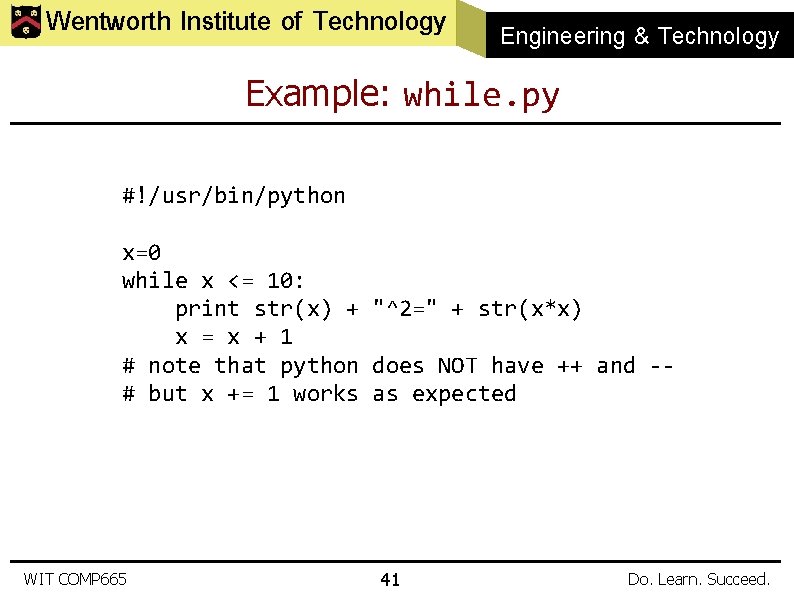
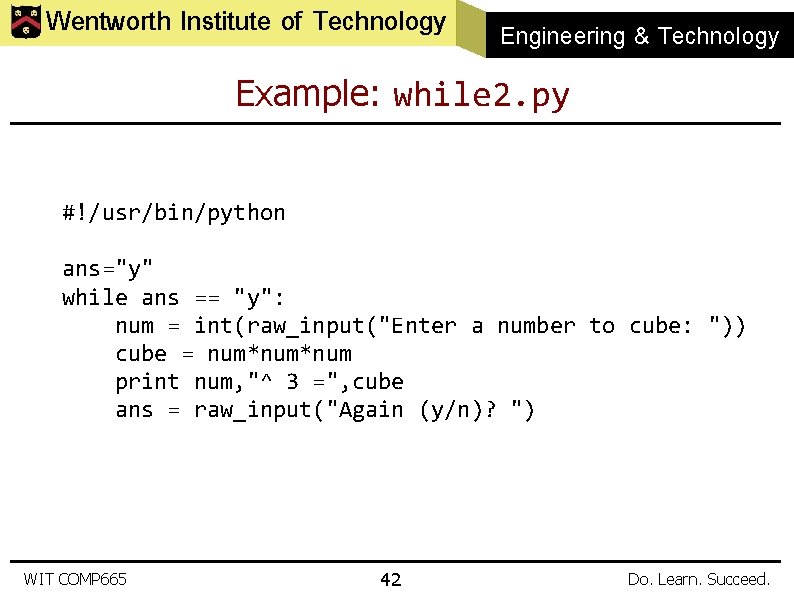
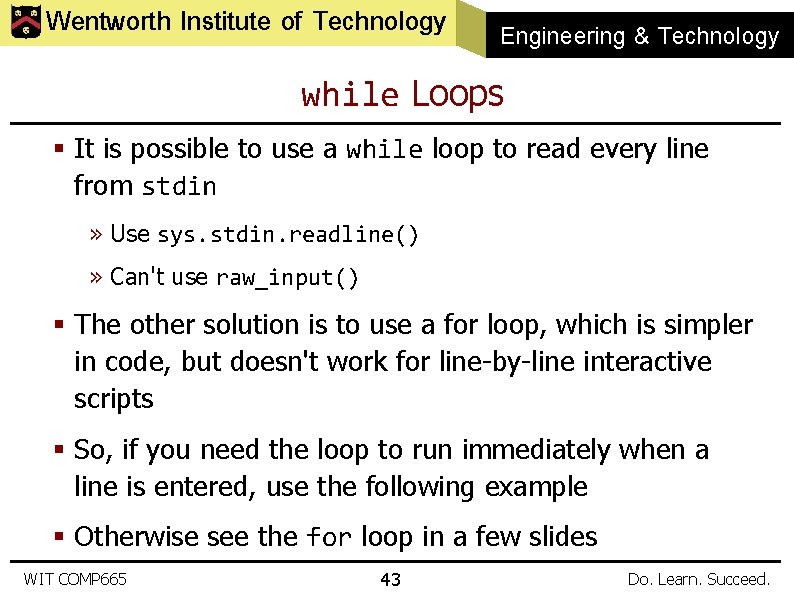
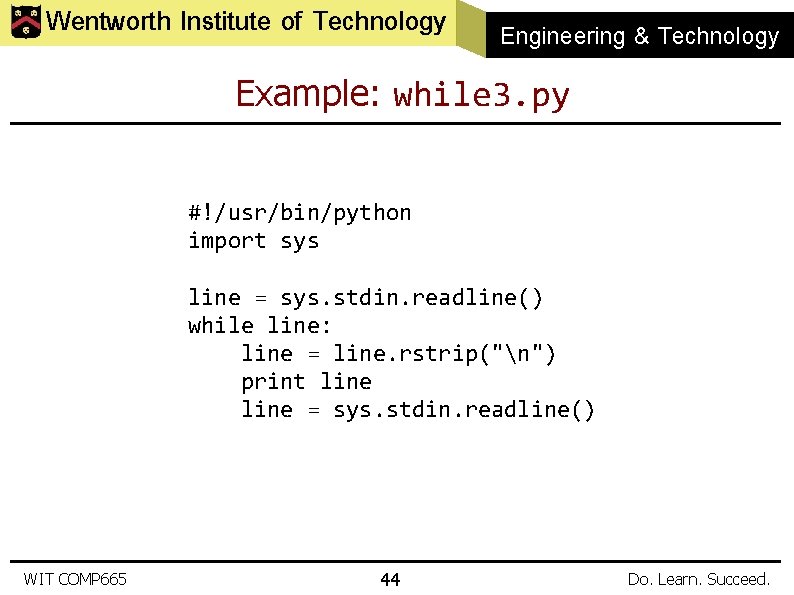
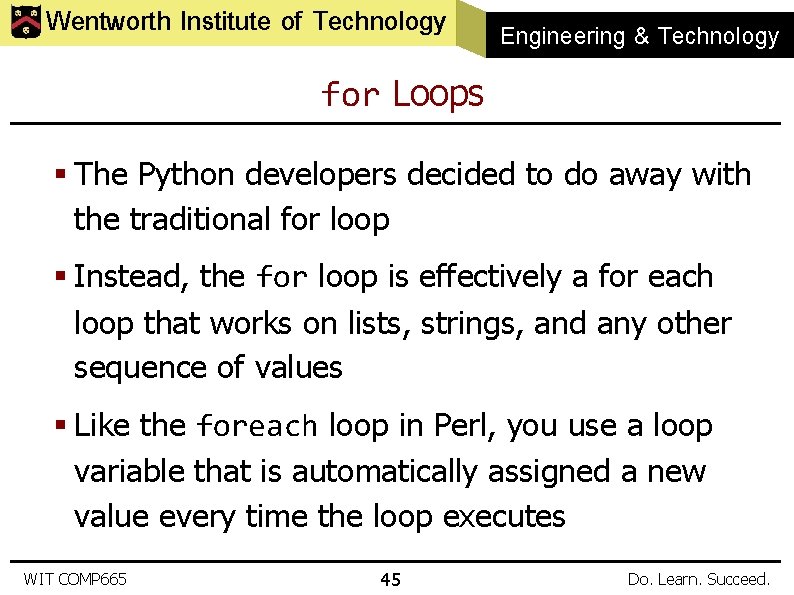
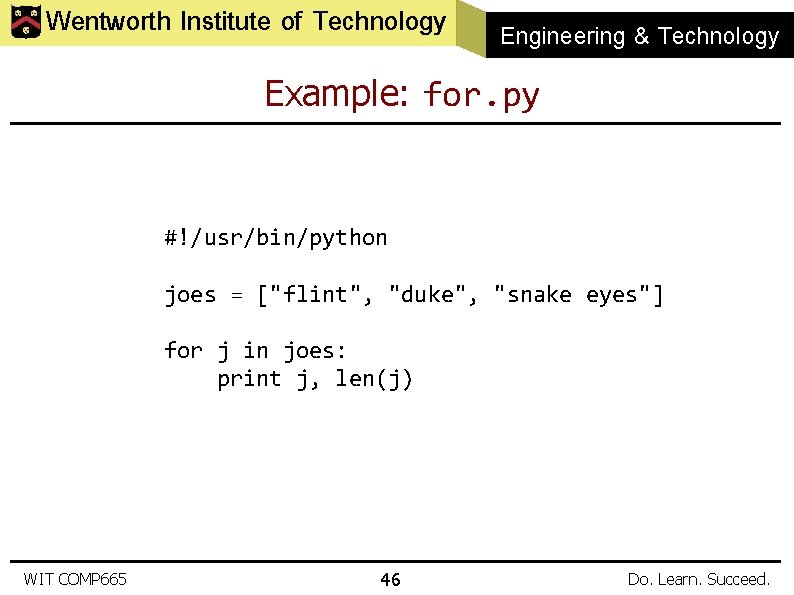
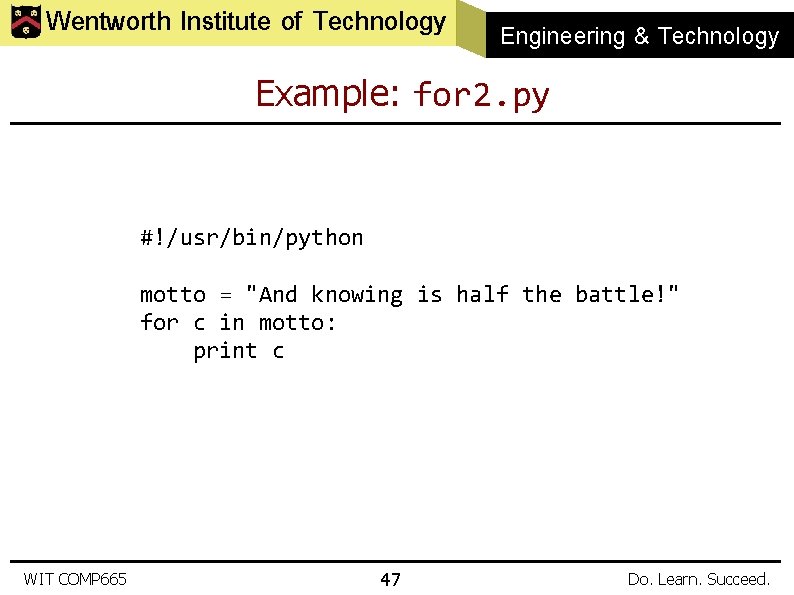
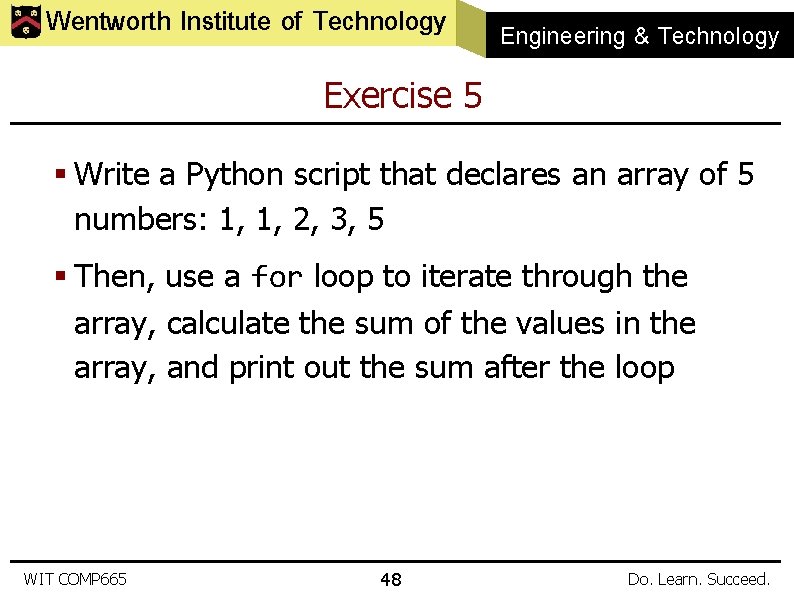
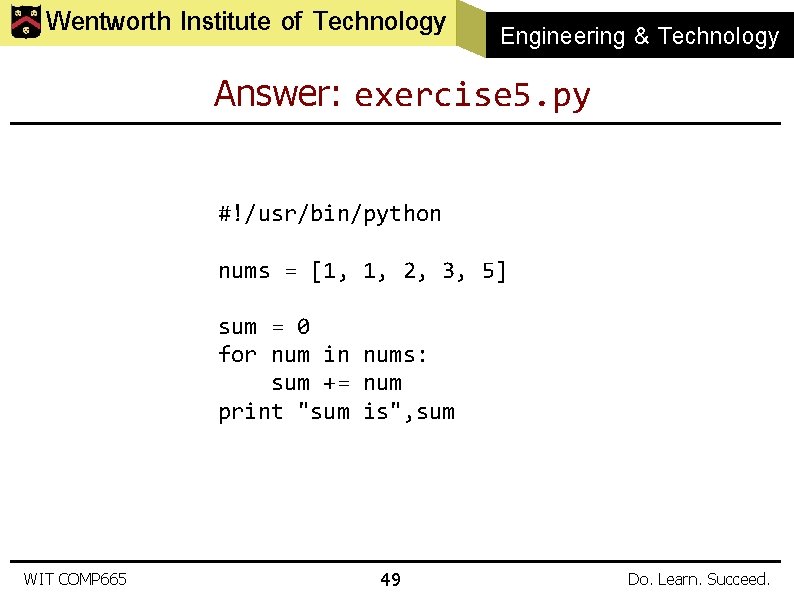
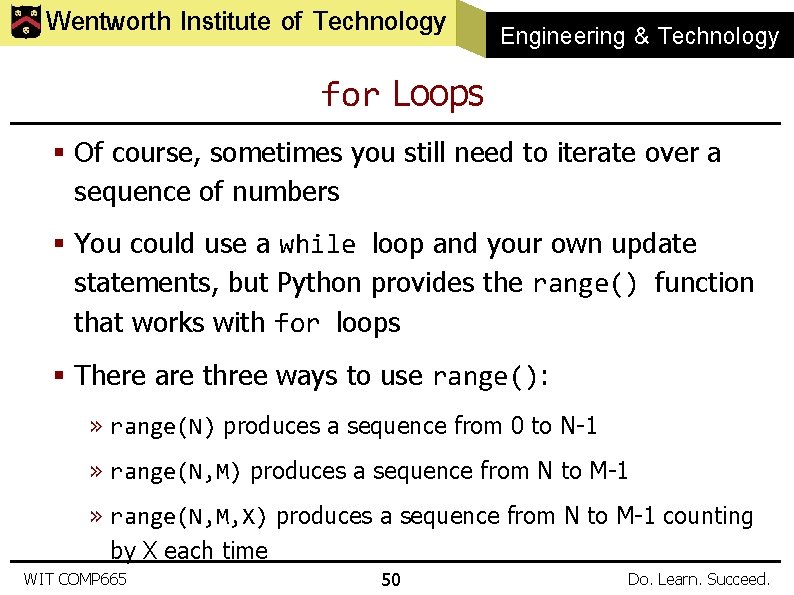
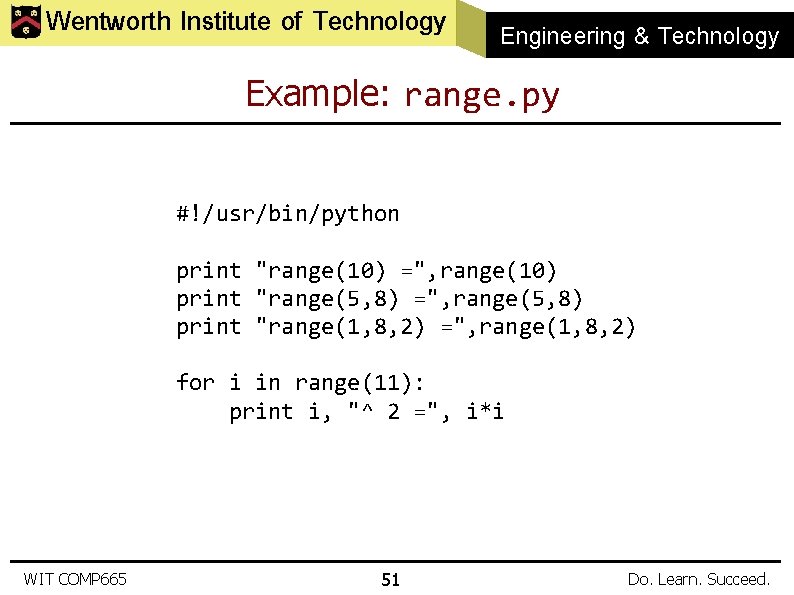
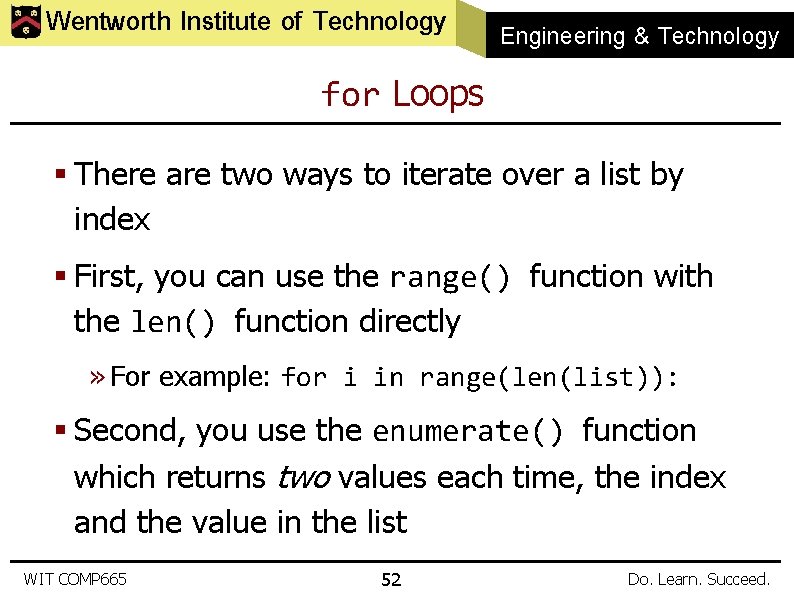
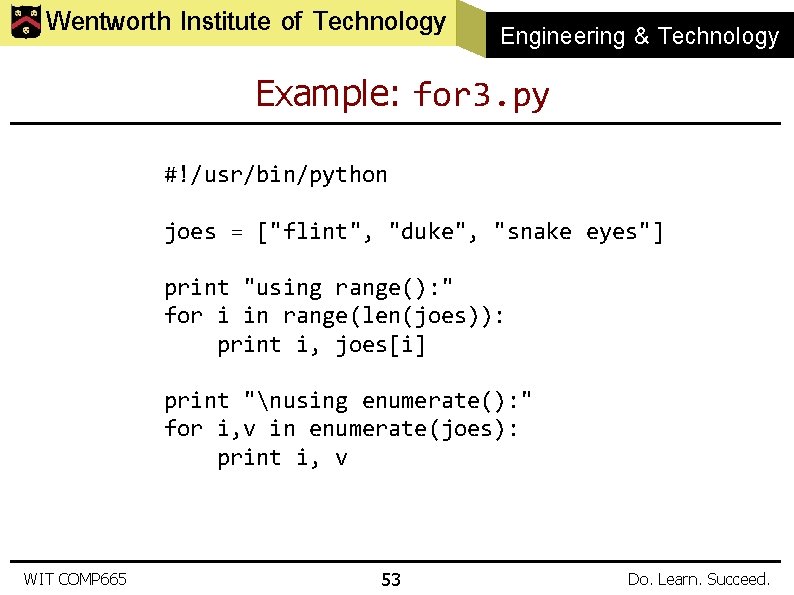
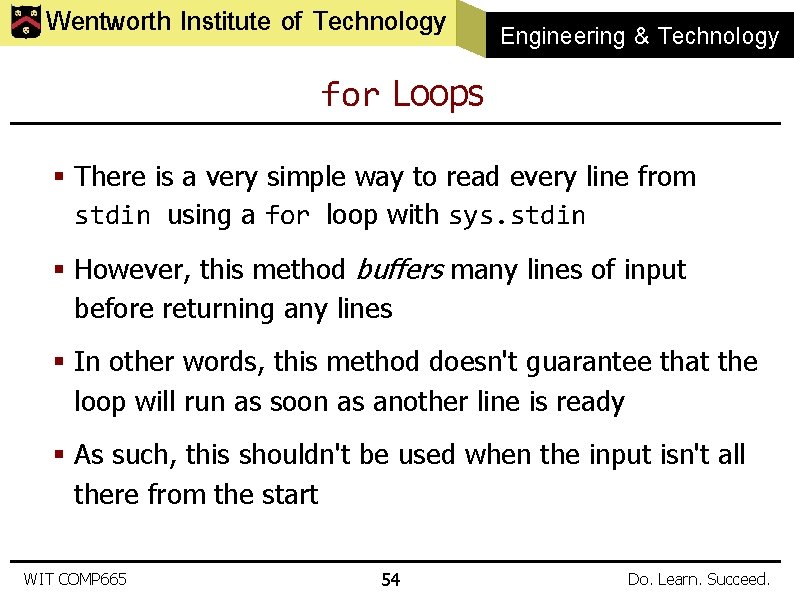
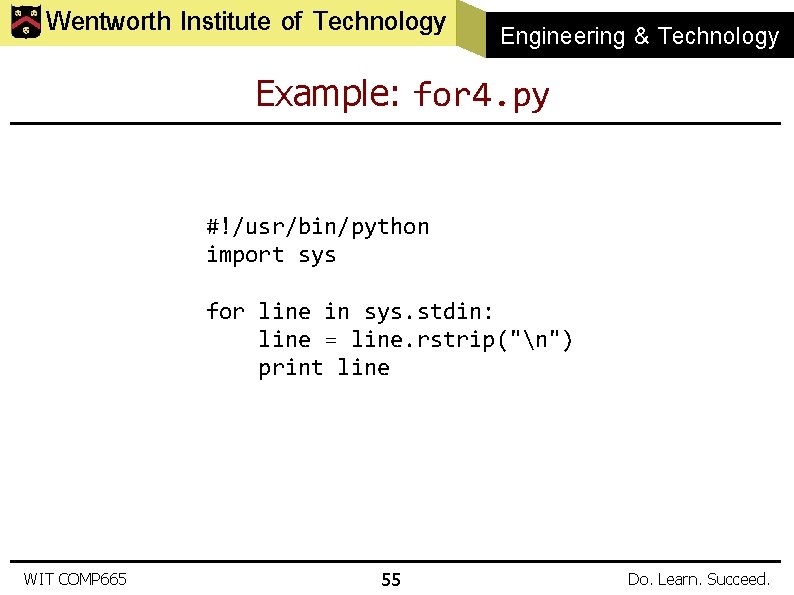
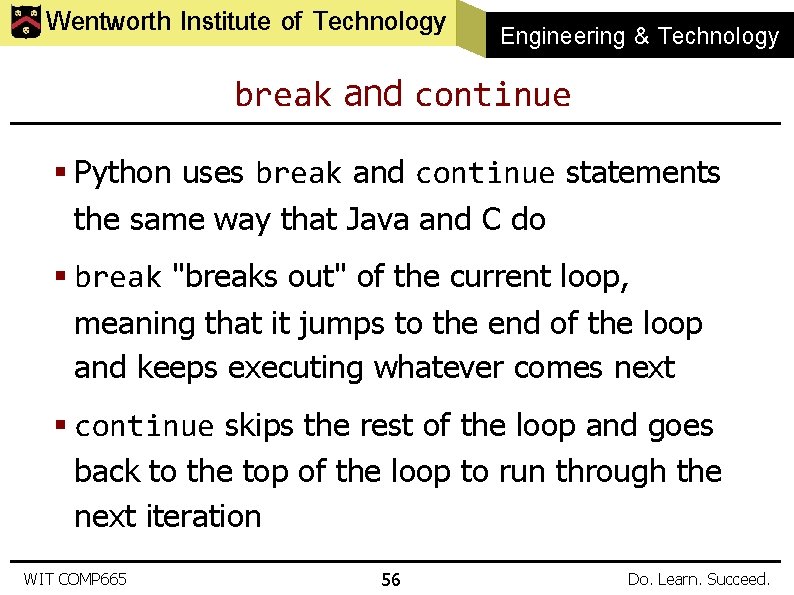
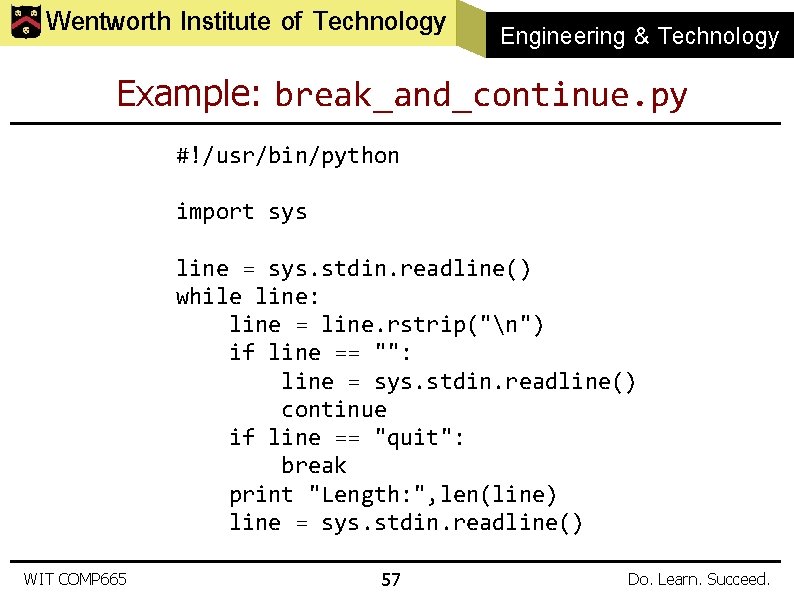
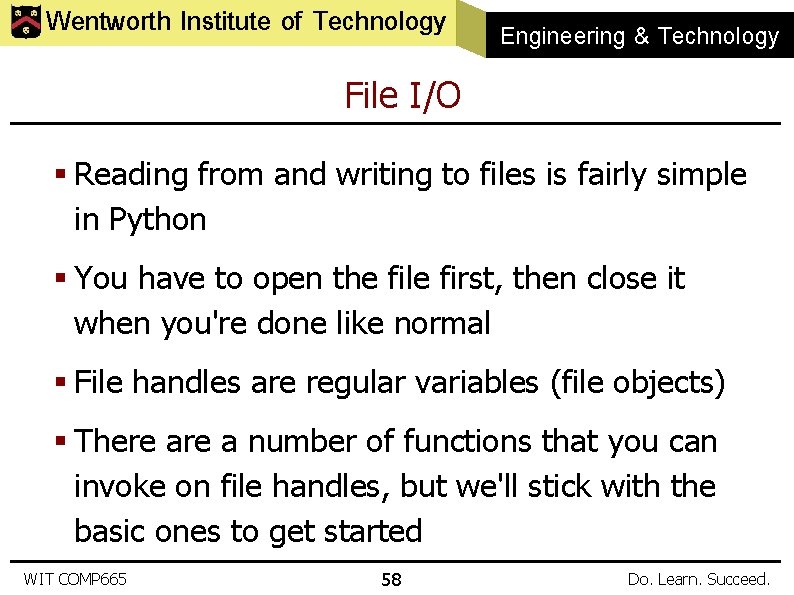
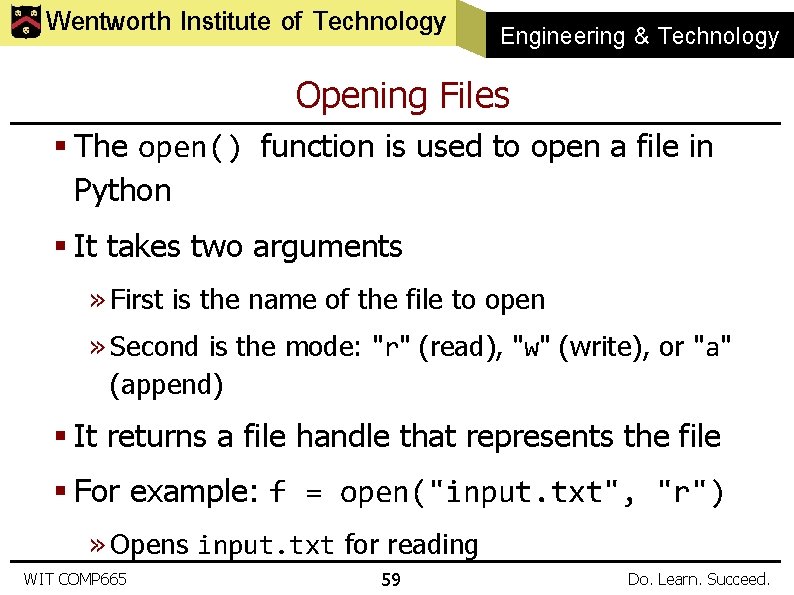
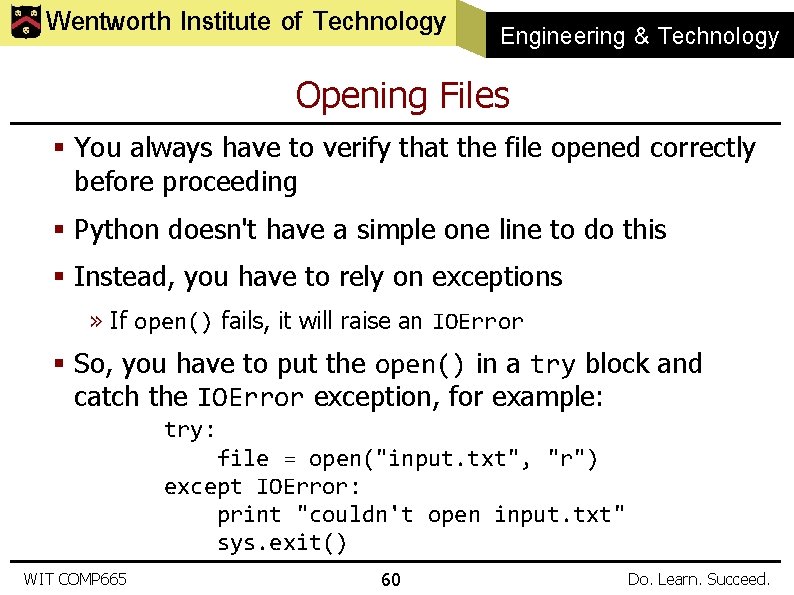
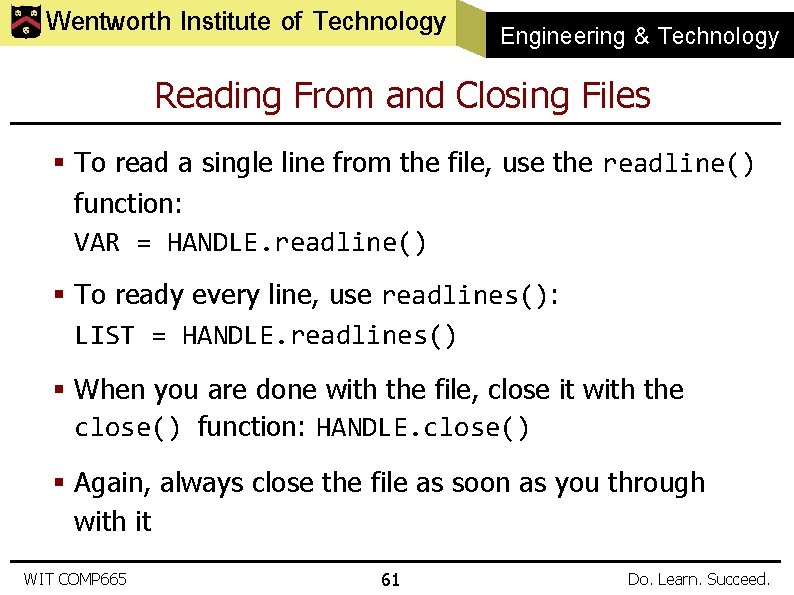
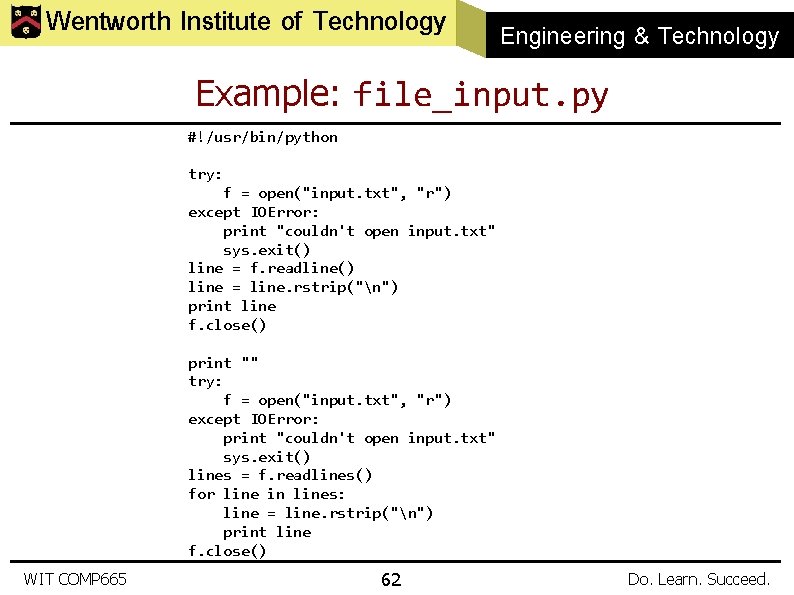
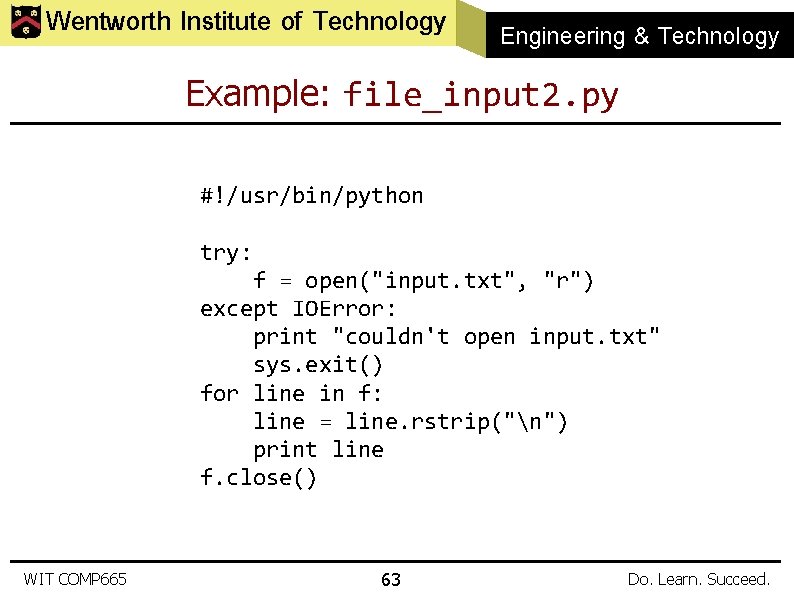
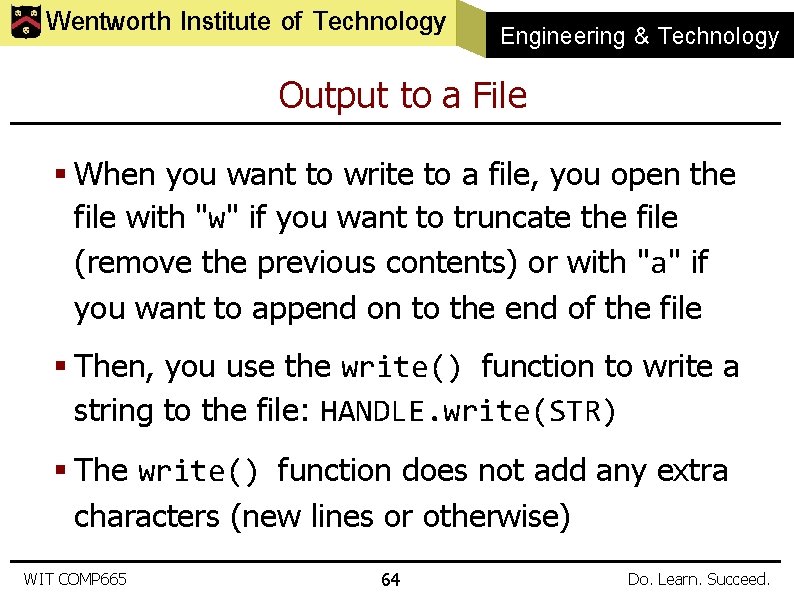
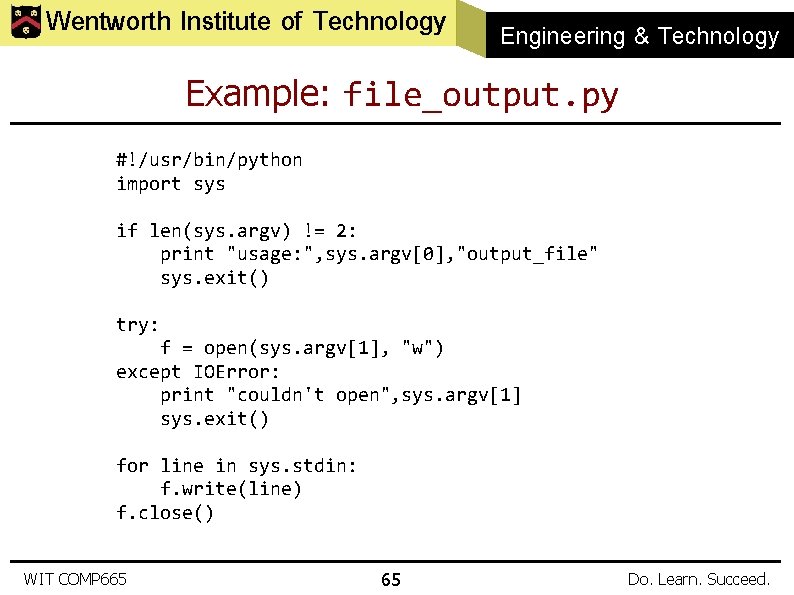
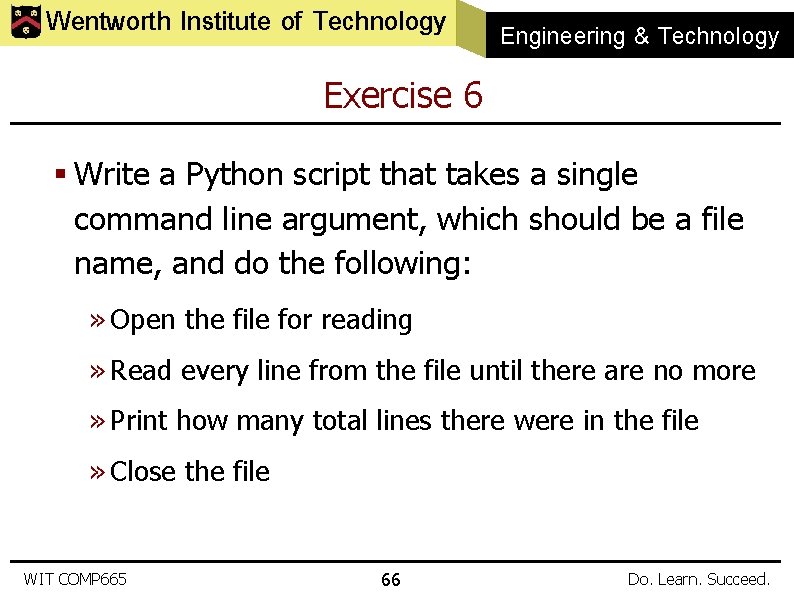
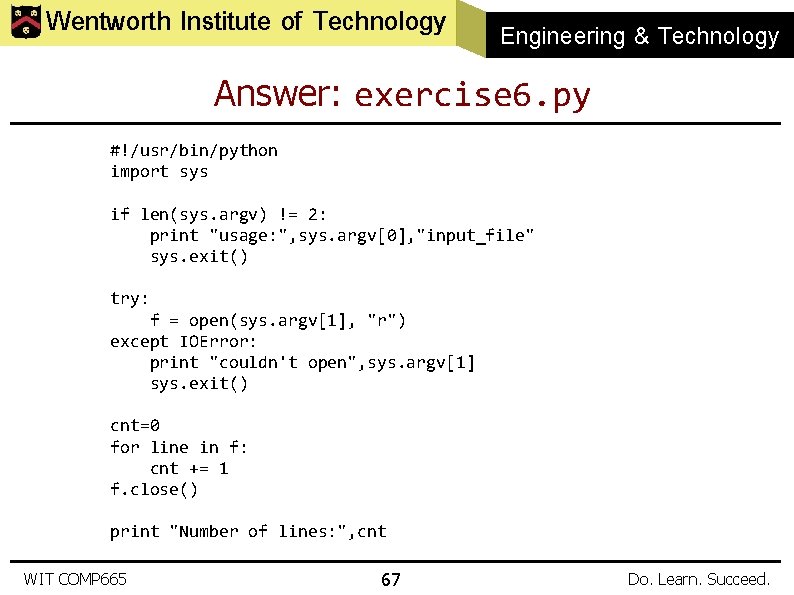
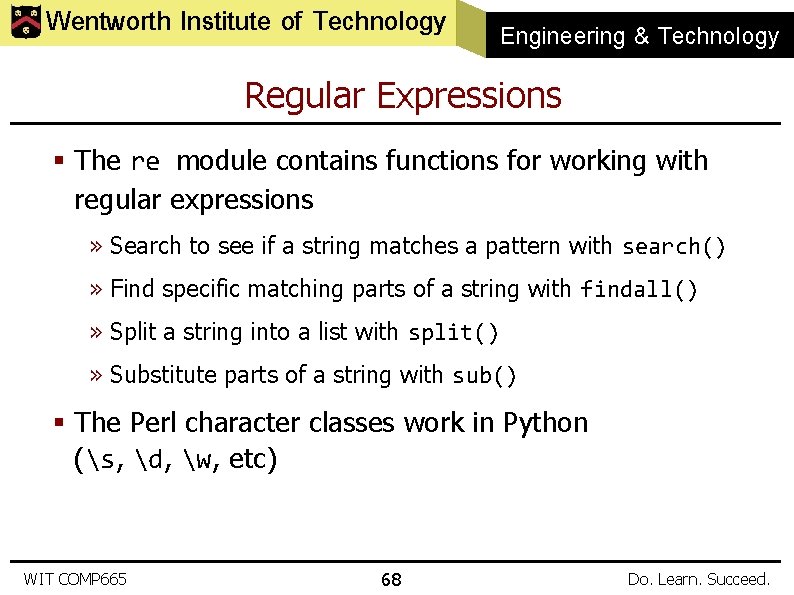
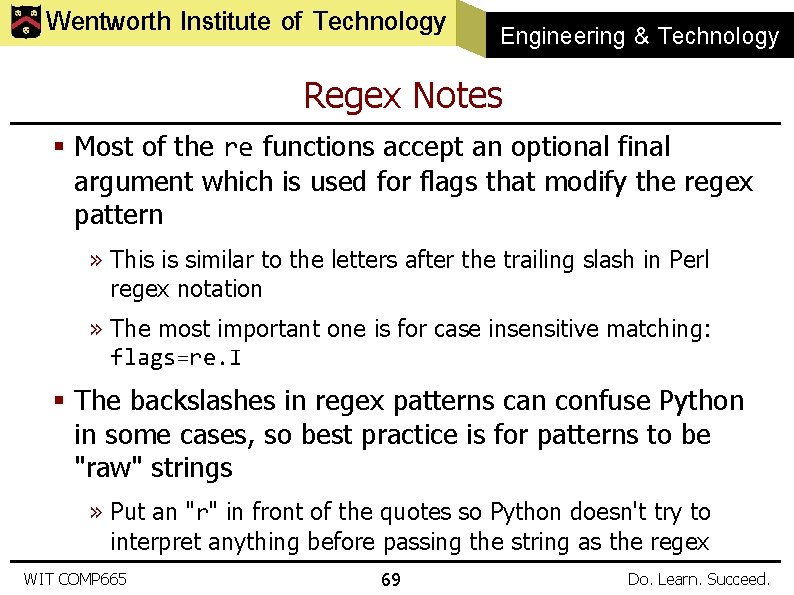
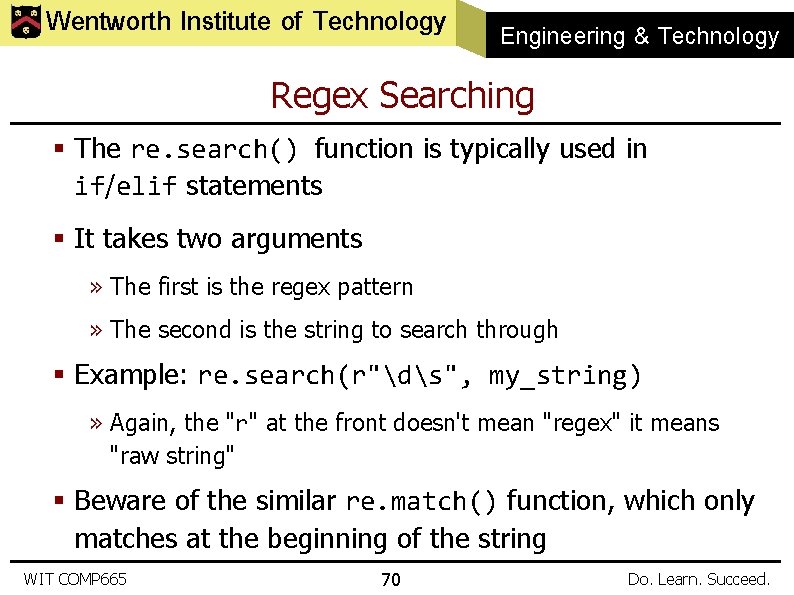
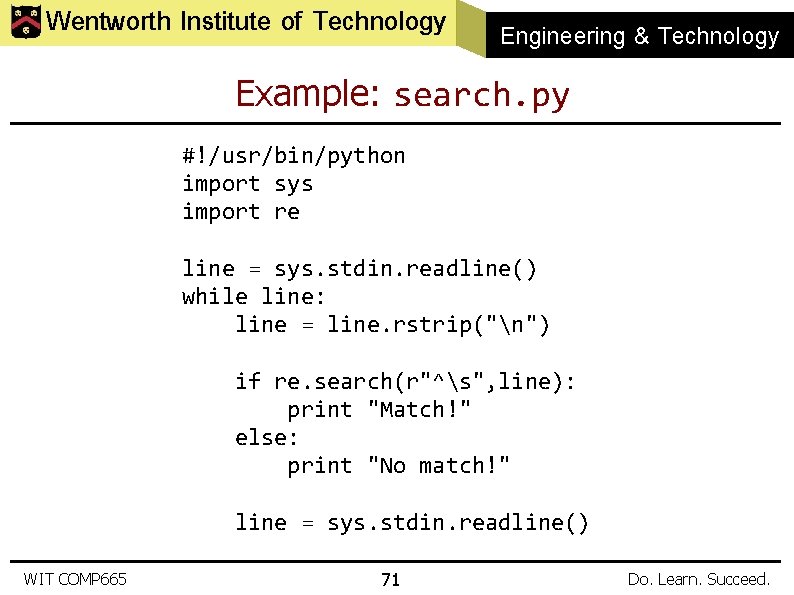
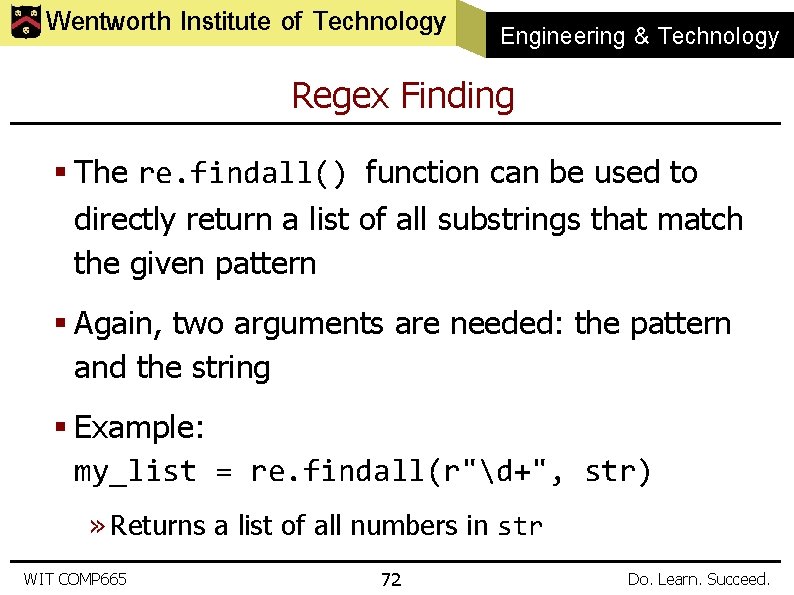
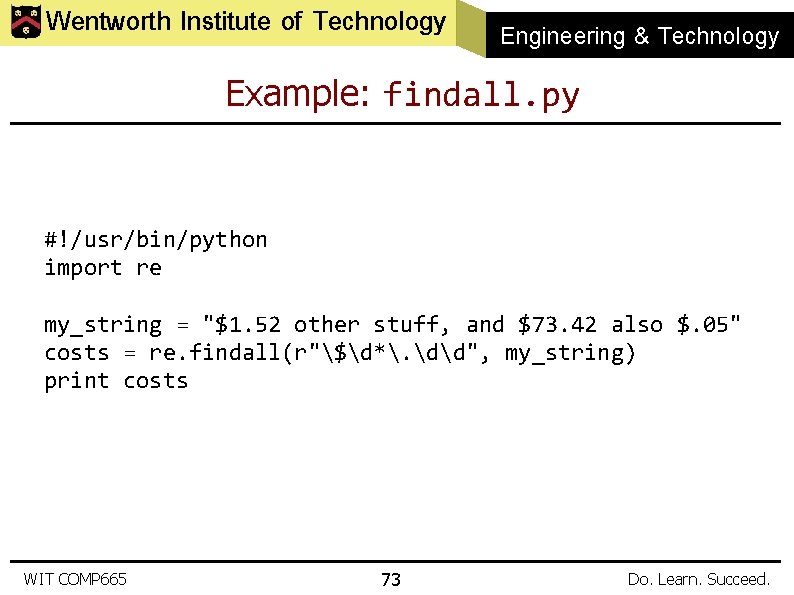
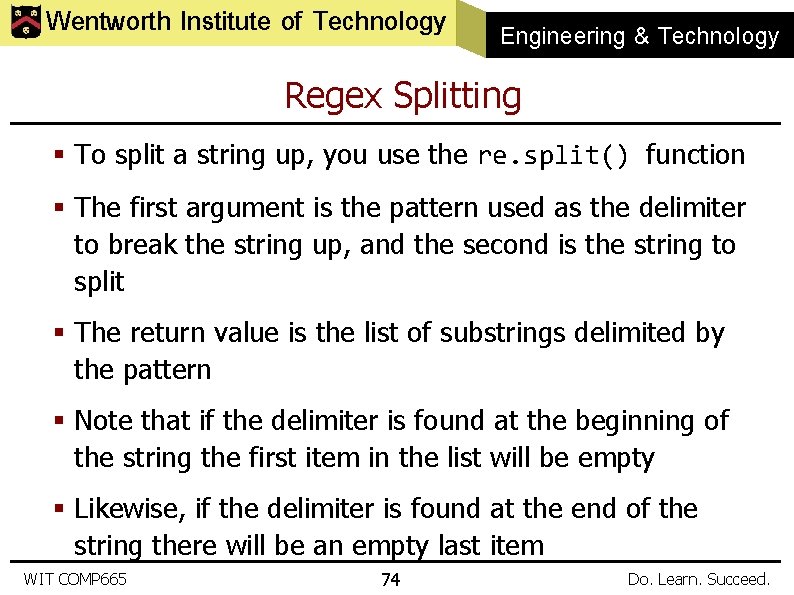
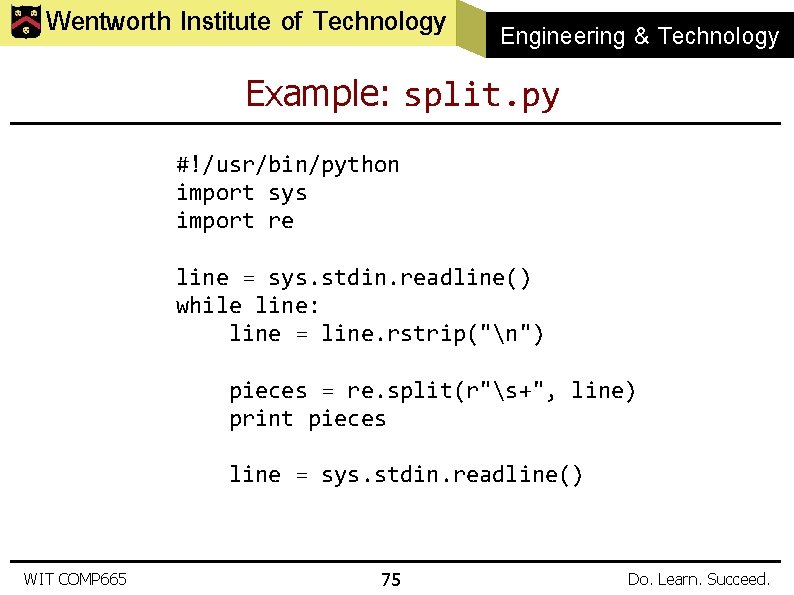
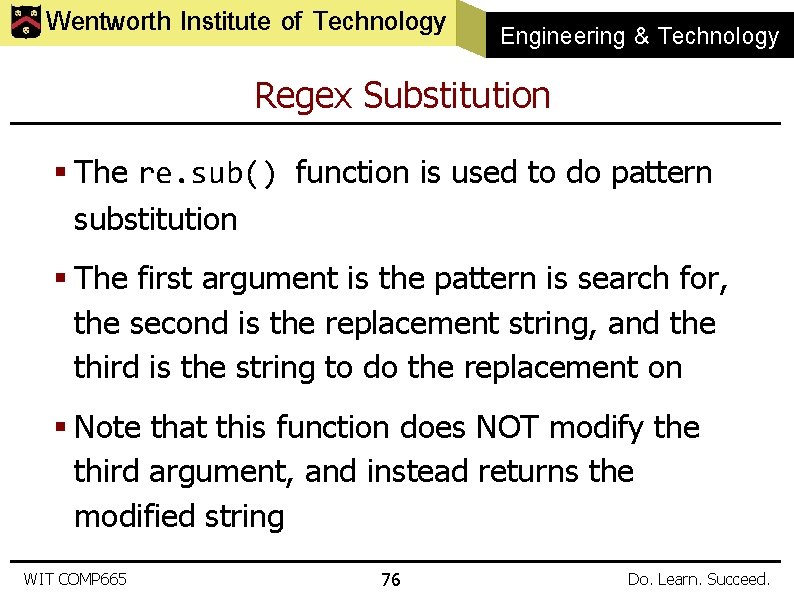
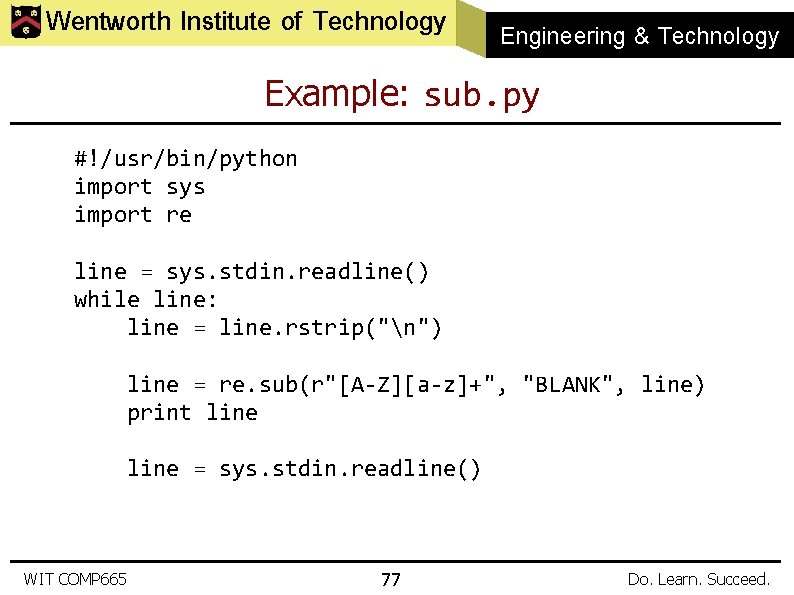
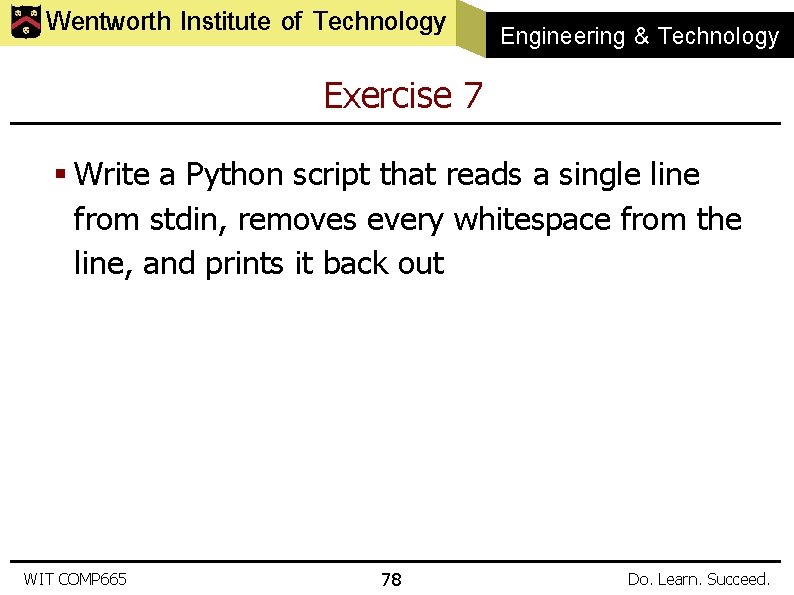
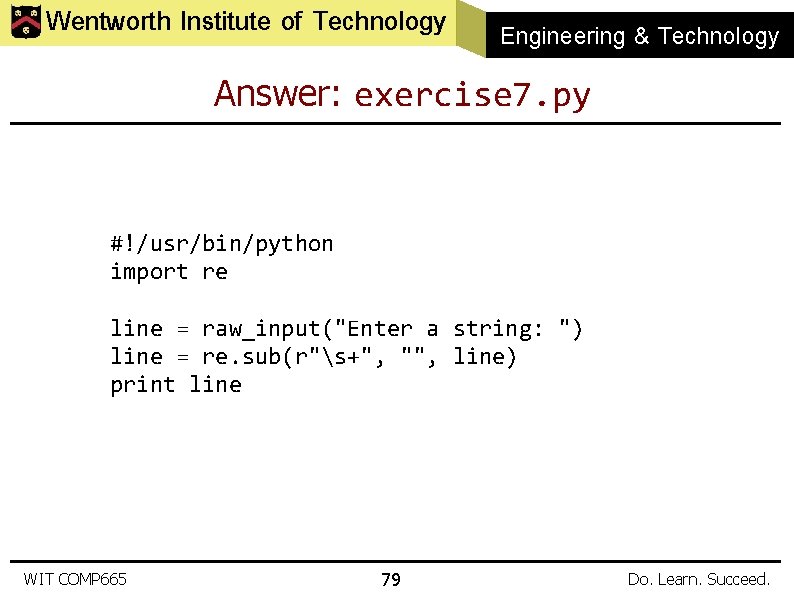
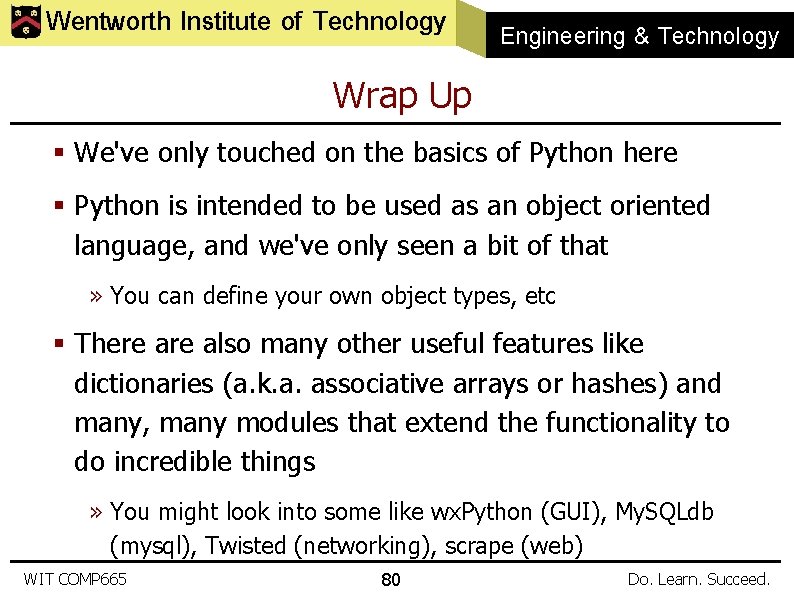
- Slides: 80
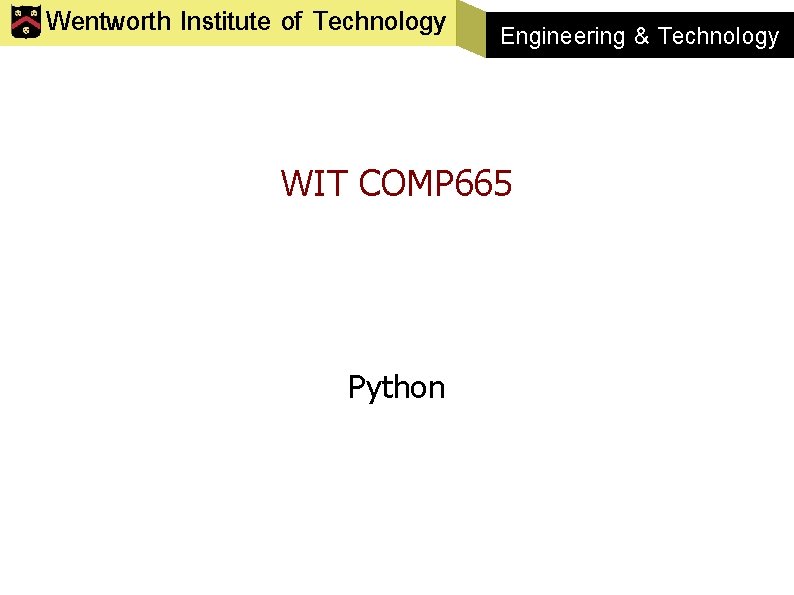
Wentworth Institute of Technology Engineering & Technology WIT COMP 665 Python
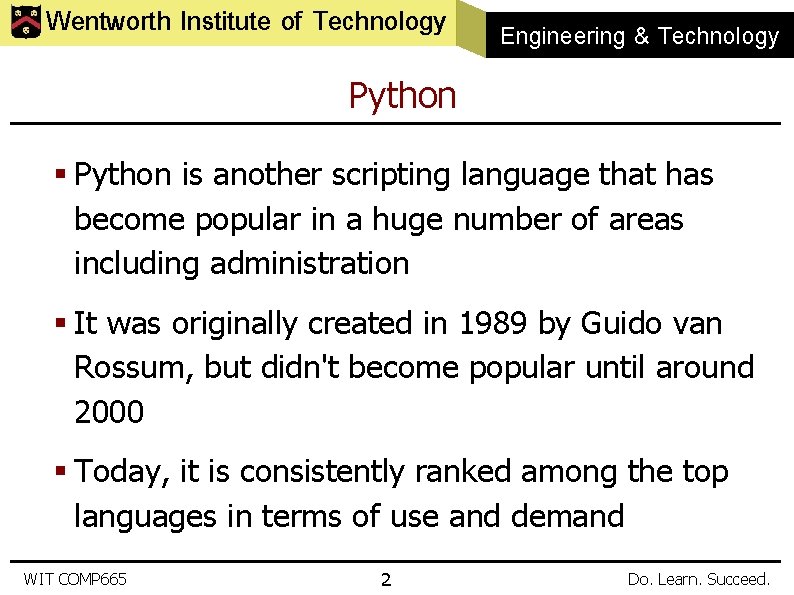
Wentworth Institute of Technology Engineering & Technology Python § Python is another scripting language that has become popular in a huge number of areas including administration § It was originally created in 1989 by Guido van Rossum, but didn't become popular until around 2000 § Today, it is consistently ranked among the top languages in terms of use and demand WIT COMP 665 2 Do. Learn. Succeed.
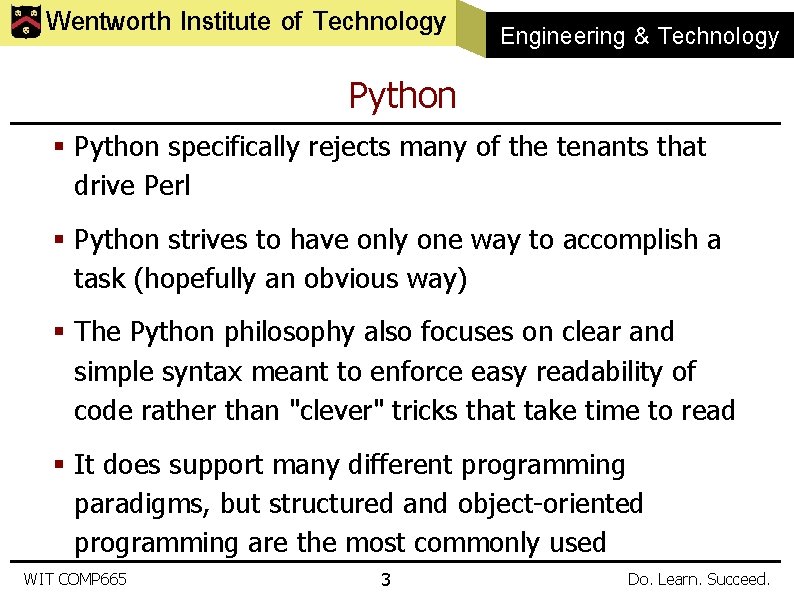
Wentworth Institute of Technology Engineering & Technology Python § Python specifically rejects many of the tenants that drive Perl § Python strives to have only one way to accomplish a task (hopefully an obvious way) § The Python philosophy also focuses on clear and simple syntax meant to enforce easy readability of code rather than "clever" tricks that take time to read § It does support many different programming paradigms, but structured and object-oriented programming are the most commonly used WIT COMP 665 3 Do. Learn. Succeed.
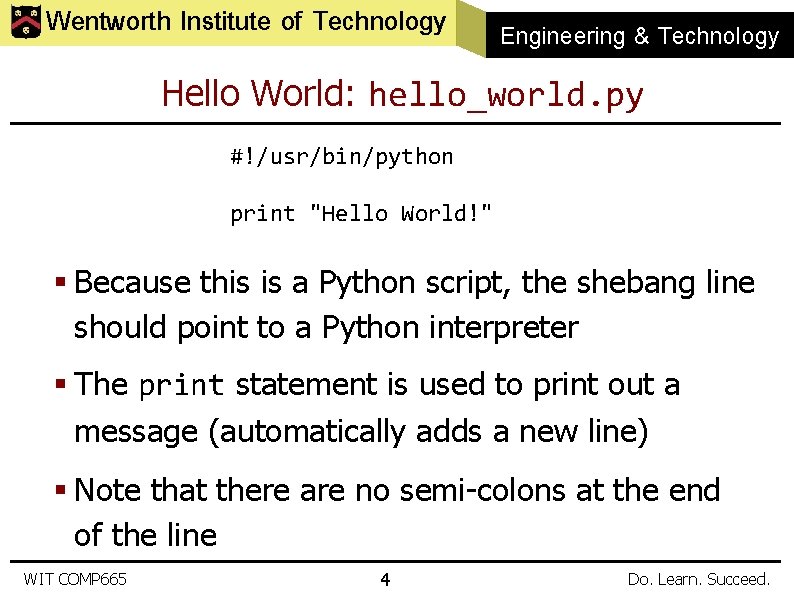
Wentworth Institute of Technology Engineering & Technology Hello World: hello_world. py #!/usr/bin/python print "Hello World!" § Because this is a Python script, the shebang line should point to a Python interpreter § The print statement is used to print out a message (automatically adds a new line) § Note that there are no semi-colons at the end of the line WIT COMP 665 4 Do. Learn. Succeed.
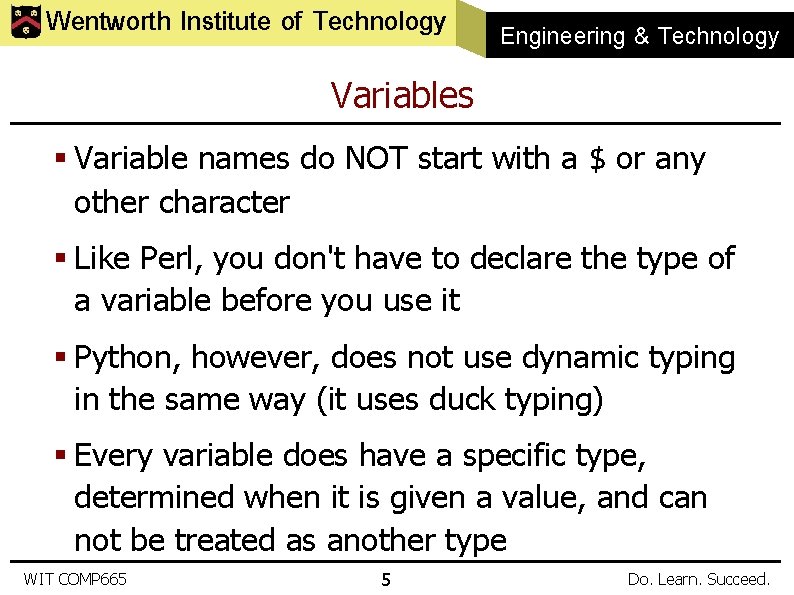
Wentworth Institute of Technology Engineering & Technology Variables § Variable names do NOT start with a $ or any other character § Like Perl, you don't have to declare the type of a variable before you use it § Python, however, does not use dynamic typing in the same way (it uses duck typing) § Every variable does have a specific type, determined when it is given a value, and can not be treated as another type WIT COMP 665 5 Do. Learn. Succeed.
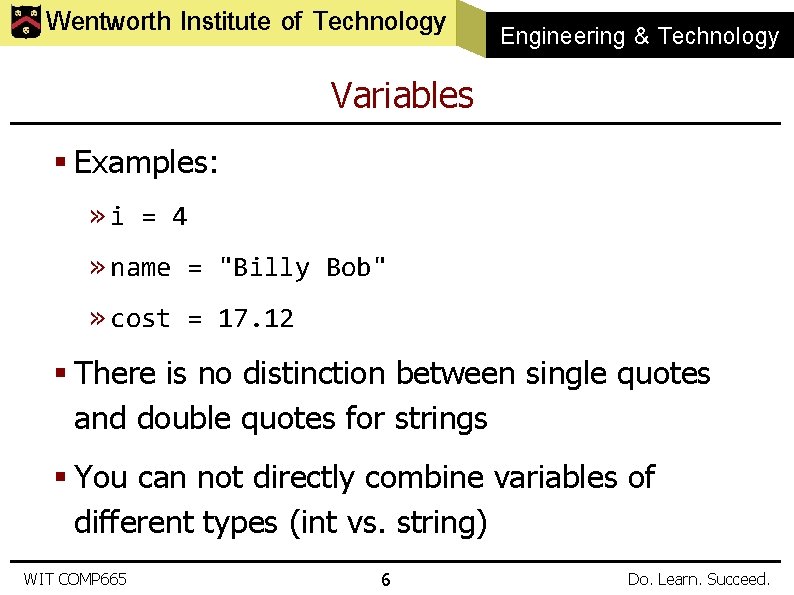
Wentworth Institute of Technology Engineering & Technology Variables § Examples: » i = 4 » name = "Billy Bob" » cost = 17. 12 § There is no distinction between single quotes and double quotes for strings § You can not directly combine variables of different types (int vs. string) WIT COMP 665 6 Do. Learn. Succeed.
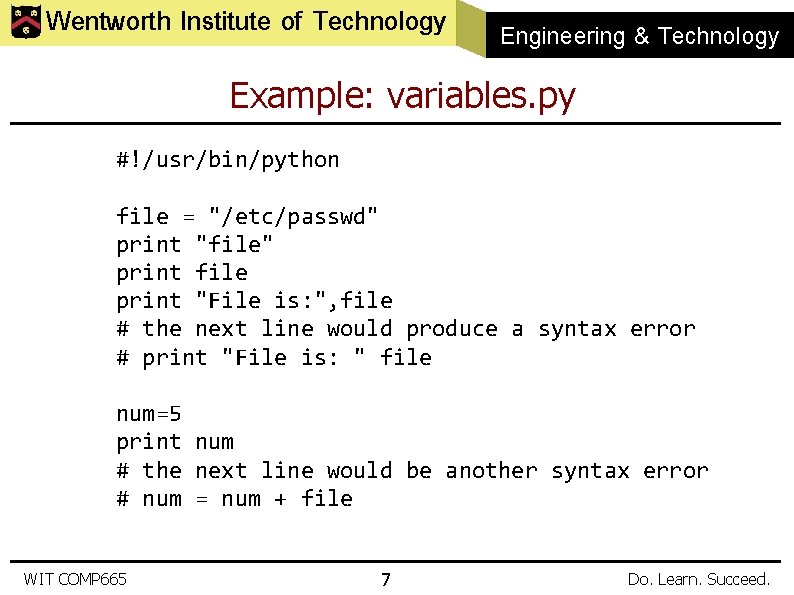
Wentworth Institute of Technology Engineering & Technology Example: variables. py #!/usr/bin/python file = "/etc/passwd" print "file" print file print "File is: ", file # the next line would produce a syntax error # print "File is: " file num=5 print num # the next line would be another syntax error # num = num + file WIT COMP 665 7 Do. Learn. Succeed.
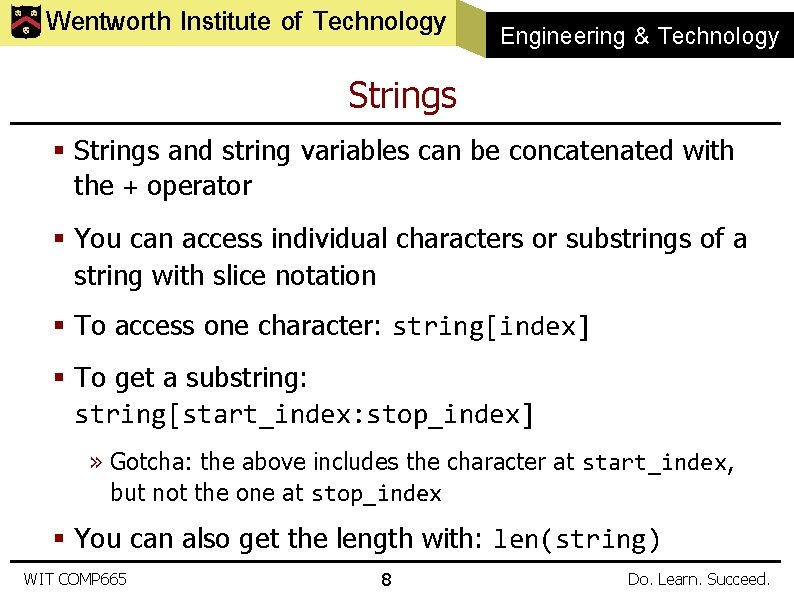
Wentworth Institute of Technology Engineering & Technology Strings § Strings and string variables can be concatenated with the + operator § You can access individual characters or substrings of a string with slice notation § To access one character: string[index] § To get a substring: string[start_index: stop_index] » Gotcha: the above includes the character at start_index, but not the one at stop_index § You can also get the length with: len(string) WIT COMP 665 8 Do. Learn. Succeed.
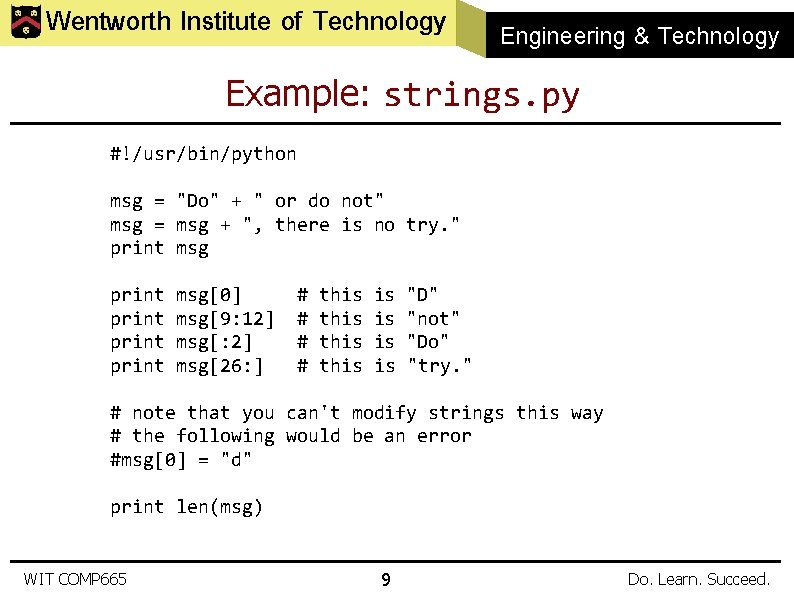
Wentworth Institute of Technology Engineering & Technology Example: strings. py #!/usr/bin/python msg = "Do" + " or do not" msg = msg + ", there is no try. " print msg print msg[0] msg[9: 12] msg[: 2] msg[26: ] # # this is is "D" "not" "Do" "try. " # note that you can't modify strings this way # the following would be an error #msg[0] = "d" print len(msg) WIT COMP 665 9 Do. Learn. Succeed.
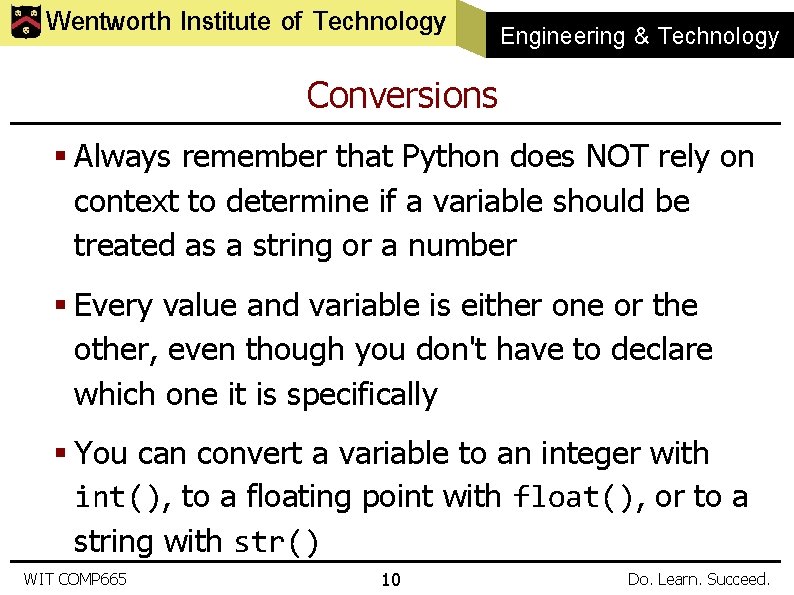
Wentworth Institute of Technology Engineering & Technology Conversions § Always remember that Python does NOT rely on context to determine if a variable should be treated as a string or a number § Every value and variable is either one or the other, even though you don't have to declare which one it is specifically § You can convert a variable to an integer with int(), to a floating point with float(), or to a string with str() WIT COMP 665 10 Do. Learn. Succeed.
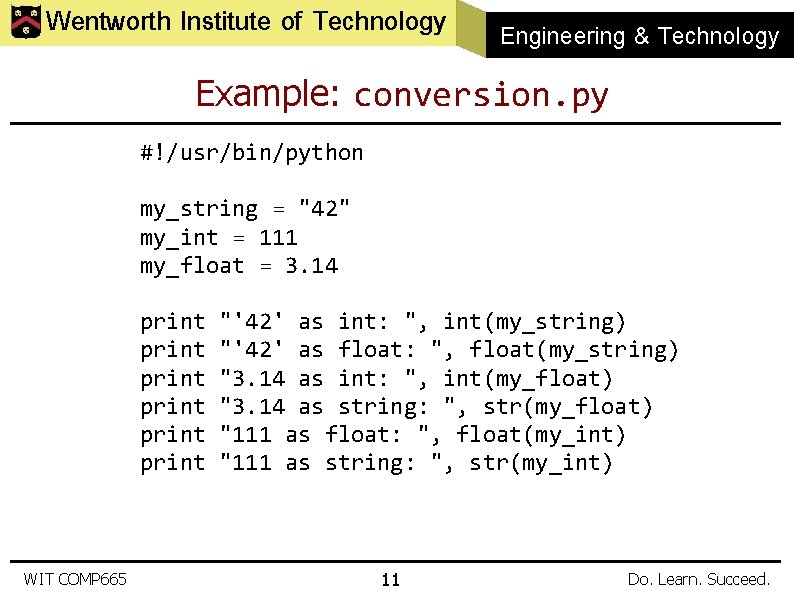
Wentworth Institute of Technology Engineering & Technology Example: conversion. py #!/usr/bin/python my_string = "42" my_int = 111 my_float = 3. 14 print print WIT COMP 665 "'42' as int: ", int(my_string) "'42' as float: ", float(my_string) "3. 14 as int: ", int(my_float) "3. 14 as string: ", str(my_float) "111 as float: ", float(my_int) "111 as string: ", str(my_int) 11 Do. Learn. Succeed.
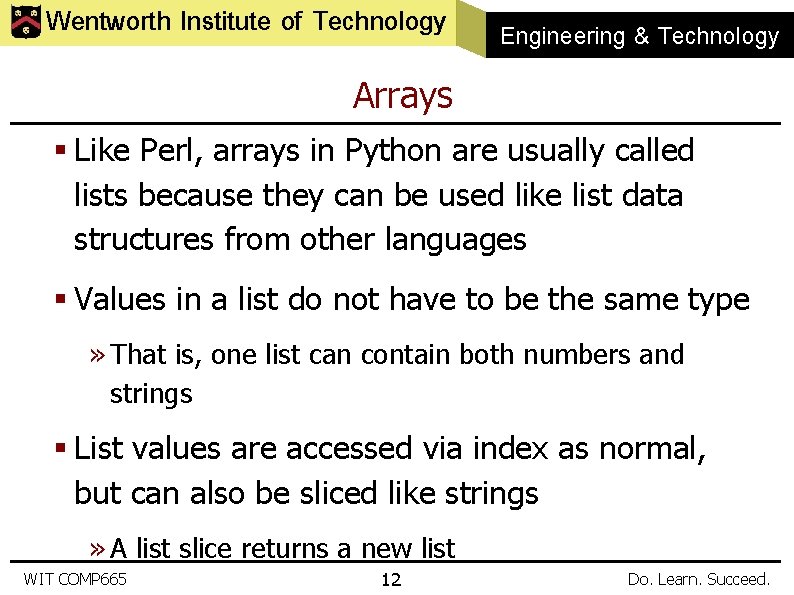
Wentworth Institute of Technology Engineering & Technology Arrays § Like Perl, arrays in Python are usually called lists because they can be used like list data structures from other languages § Values in a list do not have to be the same type » That is, one list can contain both numbers and strings § List values are accessed via index as normal, but can also be sliced like strings » A list slice returns a new list WIT COMP 665 12 Do. Learn. Succeed.
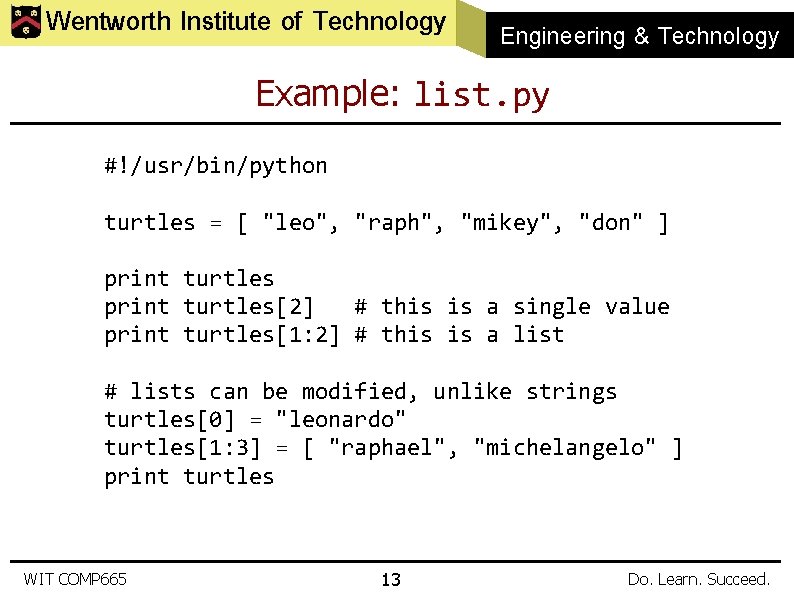
Wentworth Institute of Technology Engineering & Technology Example: list. py #!/usr/bin/python turtles = [ "leo", "raph", "mikey", "don" ] print turtles[2] # this is a single value print turtles[1: 2] # this is a list # lists can be modified, unlike strings turtles[0] = "leonardo" turtles[1: 3] = [ "raphael", "michelangelo" ] print turtles WIT COMP 665 13 Do. Learn. Succeed.
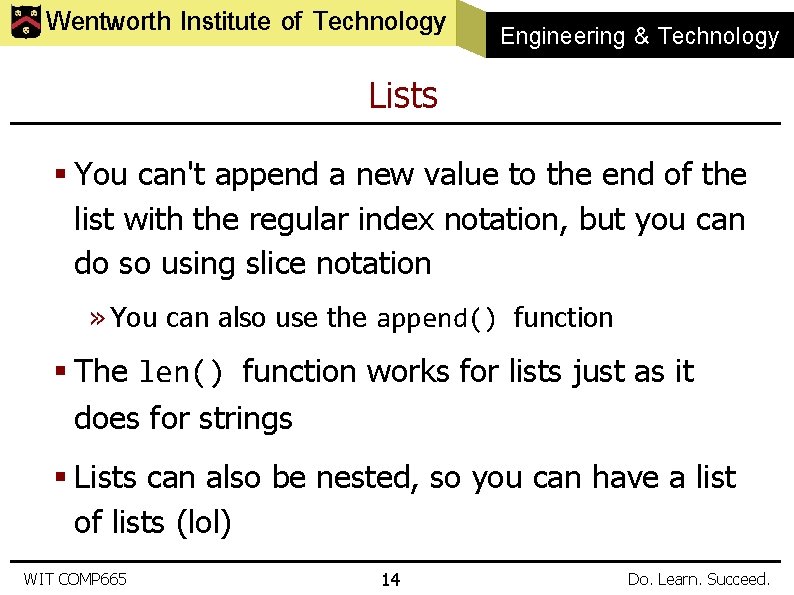
Wentworth Institute of Technology Engineering & Technology Lists § You can't append a new value to the end of the list with the regular index notation, but you can do so using slice notation » You can also use the append() function § The len() function works for lists just as it does for strings § Lists can also be nested, so you can have a list of lists (lol) WIT COMP 665 14 Do. Learn. Succeed.
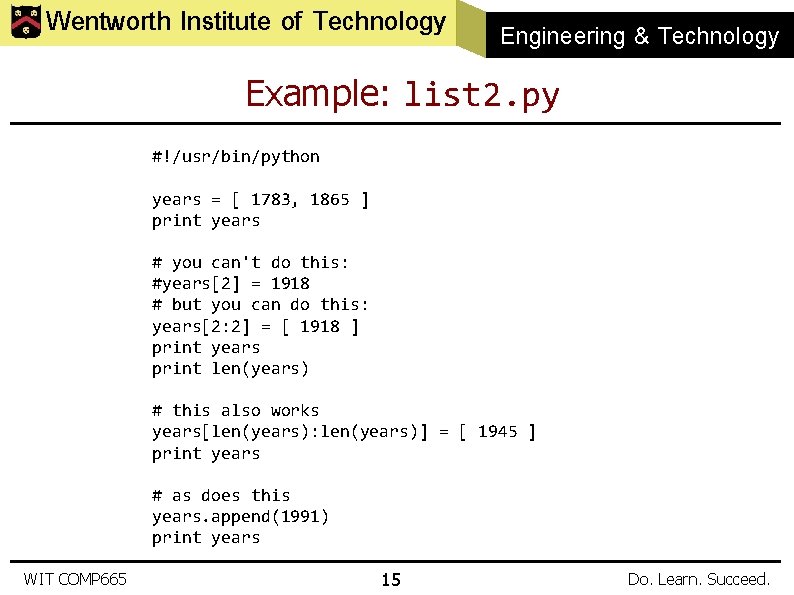
Wentworth Institute of Technology Engineering & Technology Example: list 2. py #!/usr/bin/python years = [ 1783, 1865 ] print years # you can't do this: #years[2] = 1918 # but you can do this: years[2: 2] = [ 1918 ] print years print len(years) # this also works years[len(years): len(years)] = [ 1945 ] print years # as does this years. append(1991) print years WIT COMP 665 15 Do. Learn. Succeed.
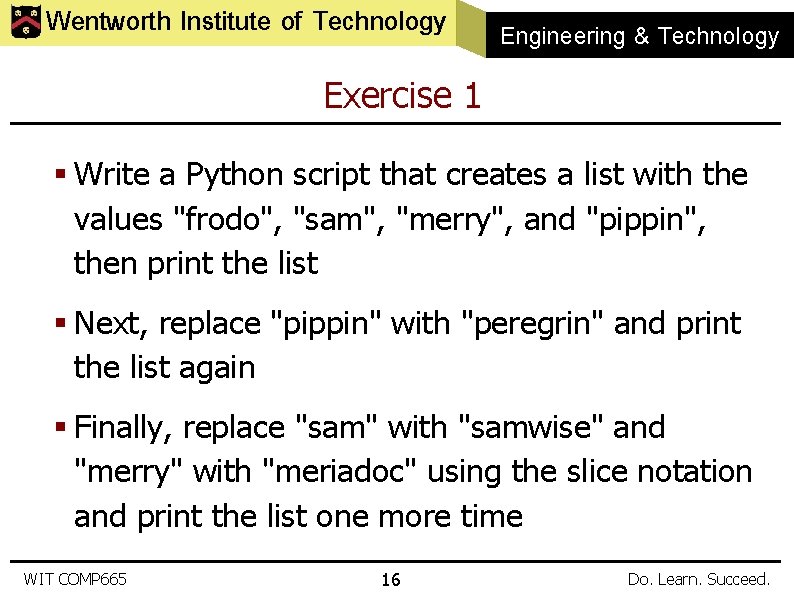
Wentworth Institute of Technology Engineering & Technology Exercise 1 § Write a Python script that creates a list with the values "frodo", "sam", "merry", and "pippin", then print the list § Next, replace "pippin" with "peregrin" and print the list again § Finally, replace "sam" with "samwise" and "merry" with "meriadoc" using the slice notation and print the list one more time WIT COMP 665 16 Do. Learn. Succeed.
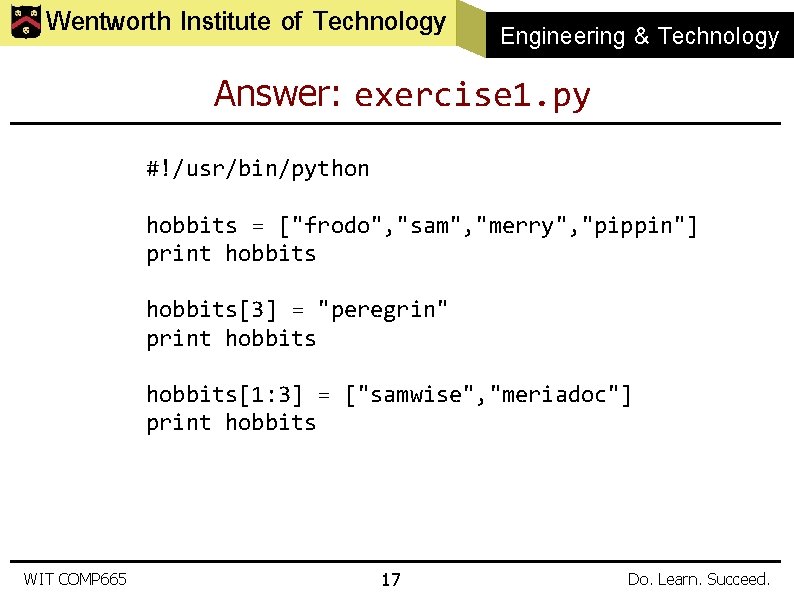
Wentworth Institute of Technology Engineering & Technology Answer: exercise 1. py #!/usr/bin/python hobbits = ["frodo", "sam", "merry", "pippin"] print hobbits[3] = "peregrin" print hobbits[1: 3] = ["samwise", "meriadoc"] print hobbits WIT COMP 665 17 Do. Learn. Succeed.
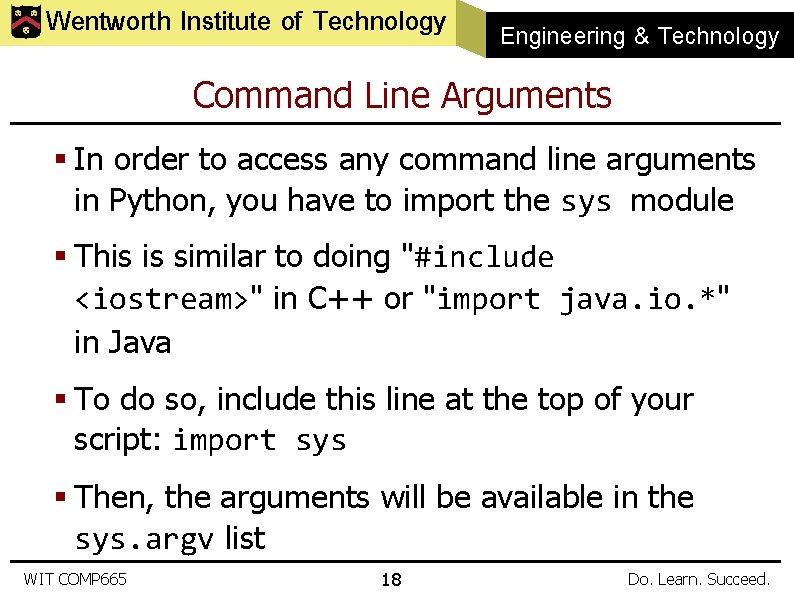
Wentworth Institute of Technology Engineering & Technology Command Line Arguments § In order to access any command line arguments in Python, you have to import the sys module § This is similar to doing "#include <iostream>" in C++ or "import java. io. *" in Java § To do so, include this line at the top of your script: import sys § Then, the arguments will be available in the sys. argv list WIT COMP 665 18 Do. Learn. Succeed.
![Wentworth Institute of Technology Engineering Technology Command Line Arguments sys argv0 is Wentworth Institute of Technology Engineering & Technology Command Line Arguments § sys. argv[0] is](https://slidetodoc.com/presentation_image_h/21c1aba65de38f0c949520080952c8ea/image-19.jpg)
Wentworth Institute of Technology Engineering & Technology Command Line Arguments § sys. argv[0] is the name of the script as it was run on the command line § Then, sys. argv[1] is the first argument, sys. argv[2] is the second argument and so on § Of course, you can use len(sys. argv) to get the number of arguments (including the script itself) § Unlike Perl, if you try to access a list index that doesn't exist, you will get an error § Note that all arguments are treated as strings WIT COMP 665 19 Do. Learn. Succeed.
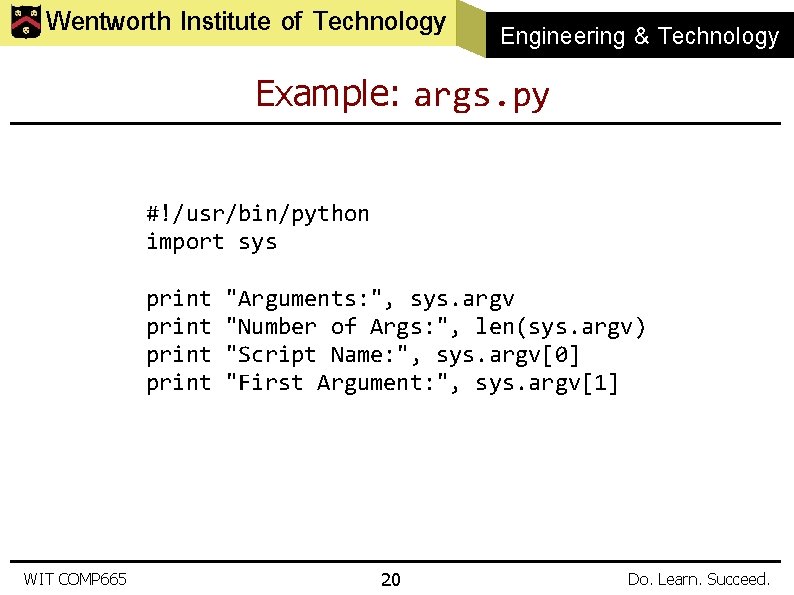
Wentworth Institute of Technology Engineering & Technology Example: args. py #!/usr/bin/python import sys print WIT COMP 665 "Arguments: ", sys. argv "Number of Args: ", len(sys. argv) "Script Name: ", sys. argv[0] "First Argument: ", sys. argv[1] 20 Do. Learn. Succeed.
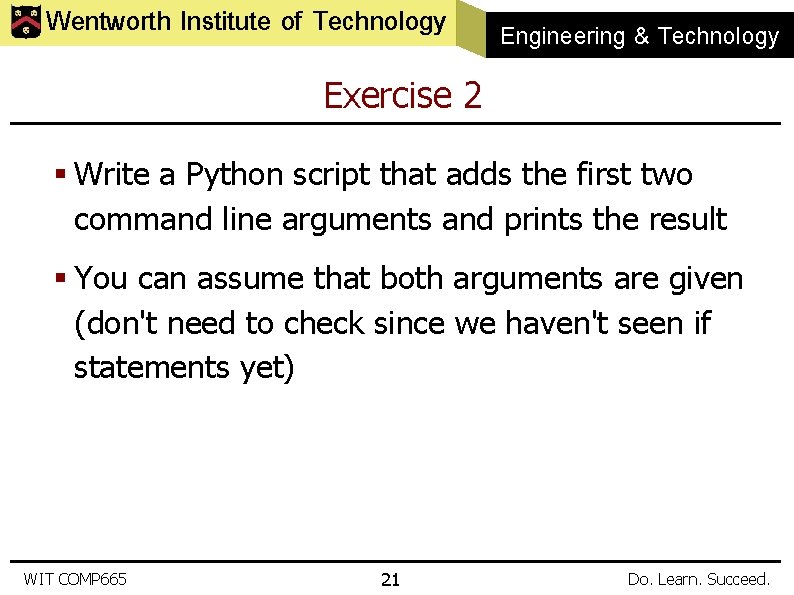
Wentworth Institute of Technology Engineering & Technology Exercise 2 § Write a Python script that adds the first two command line arguments and prints the result § You can assume that both arguments are given (don't need to check since we haven't seen if statements yet) WIT COMP 665 21 Do. Learn. Succeed.
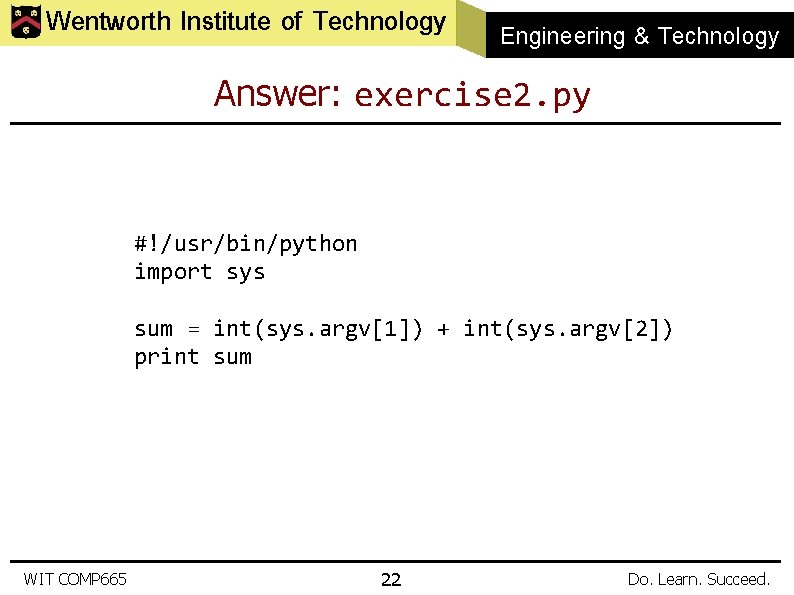
Wentworth Institute of Technology Engineering & Technology Answer: exercise 2. py #!/usr/bin/python import sys sum = int(sys. argv[1]) + int(sys. argv[2]) print sum WIT COMP 665 22 Do. Learn. Succeed.
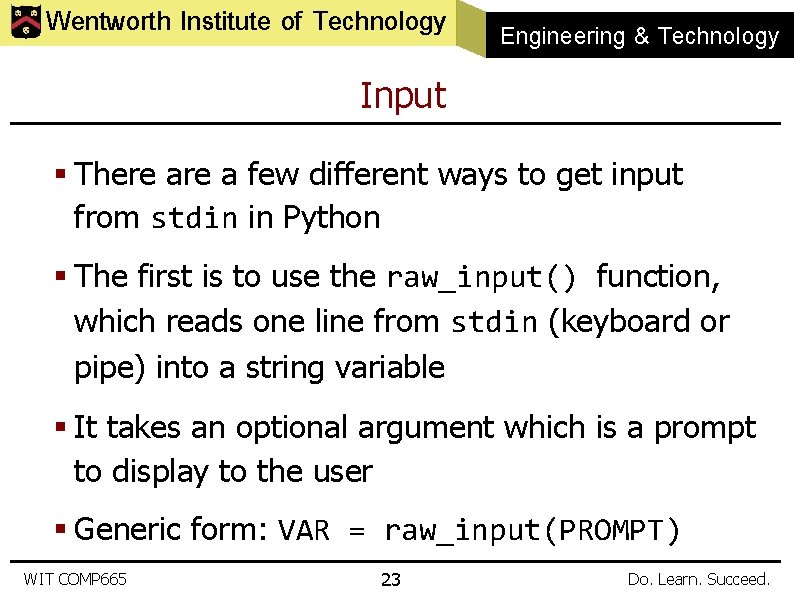
Wentworth Institute of Technology Engineering & Technology Input § There a few different ways to get input from stdin in Python § The first is to use the raw_input() function, which reads one line from stdin (keyboard or pipe) into a string variable § It takes an optional argument which is a prompt to display to the user § Generic form: VAR = raw_input(PROMPT) WIT COMP 665 23 Do. Learn. Succeed.
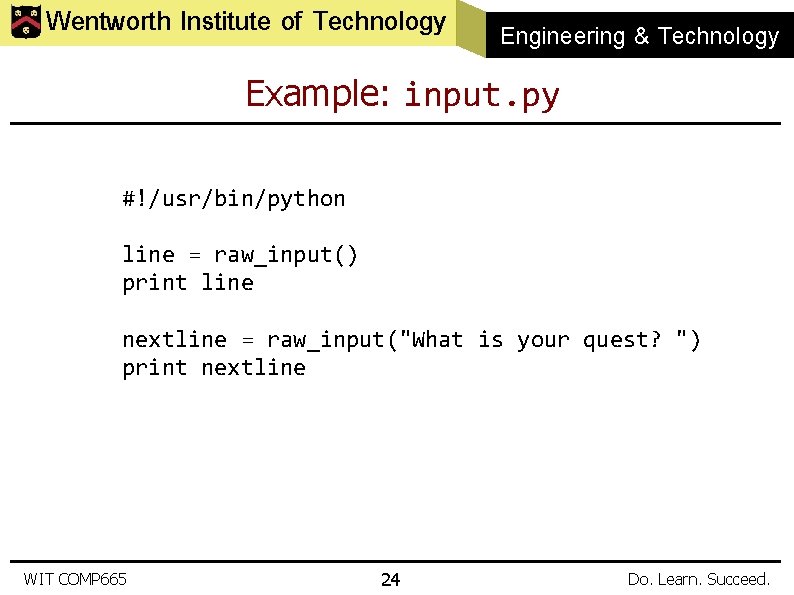
Wentworth Institute of Technology Engineering & Technology Example: input. py #!/usr/bin/python line = raw_input() print line nextline = raw_input("What is your quest? ") print nextline WIT COMP 665 24 Do. Learn. Succeed.
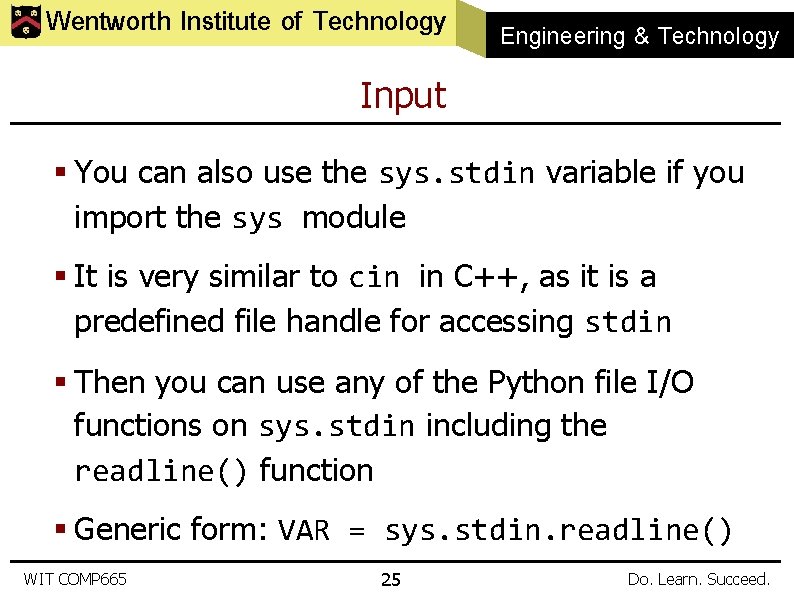
Wentworth Institute of Technology Engineering & Technology Input § You can also use the sys. stdin variable if you import the sys module § It is very similar to cin in C++, as it is a predefined file handle for accessing stdin § Then you can use any of the Python file I/O functions on sys. stdin including the readline() function § Generic form: VAR = sys. stdin. readline() WIT COMP 665 25 Do. Learn. Succeed.
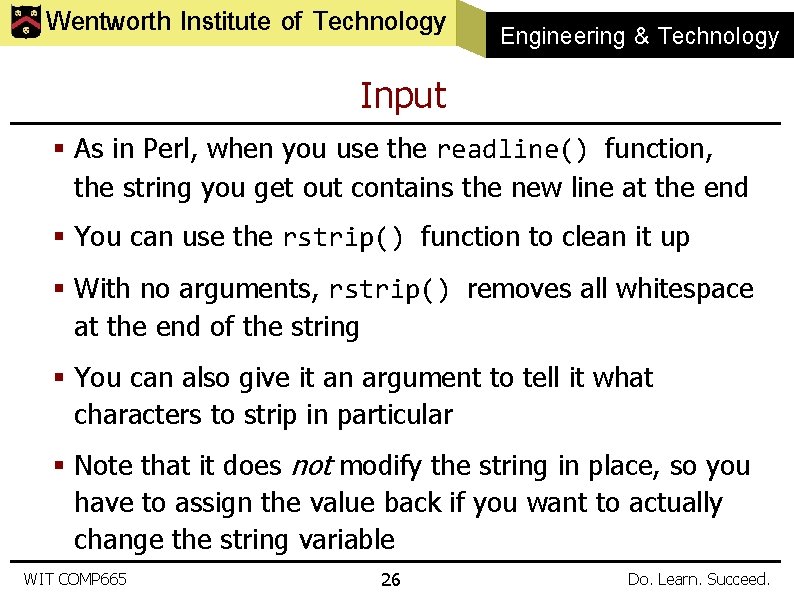
Wentworth Institute of Technology Engineering & Technology Input § As in Perl, when you use the readline() function, the string you get out contains the new line at the end § You can use the rstrip() function to clean it up § With no arguments, rstrip() removes all whitespace at the end of the string § You can also give it an argument to tell it what characters to strip in particular § Note that it does not modify the string in place, so you have to assign the value back if you want to actually change the string variable WIT COMP 665 26 Do. Learn. Succeed.
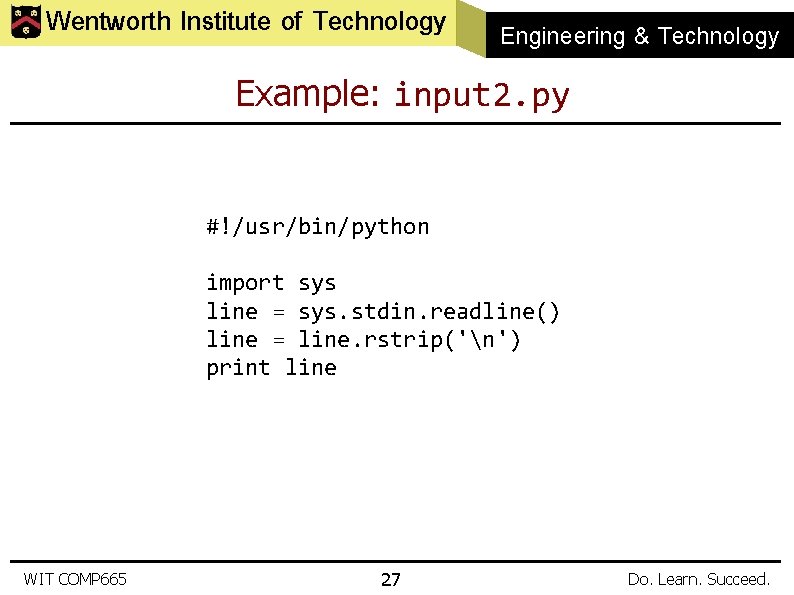
Wentworth Institute of Technology Engineering & Technology Example: input 2. py #!/usr/bin/python import sys line = sys. stdin. readline() line = line. rstrip('n') print line WIT COMP 665 27 Do. Learn. Succeed.
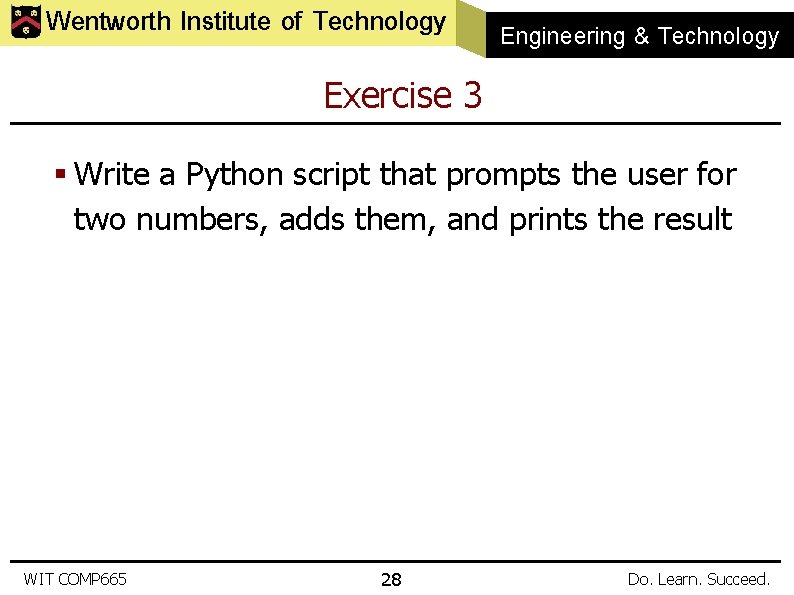
Wentworth Institute of Technology Engineering & Technology Exercise 3 § Write a Python script that prompts the user for two numbers, adds them, and prints the result WIT COMP 665 28 Do. Learn. Succeed.
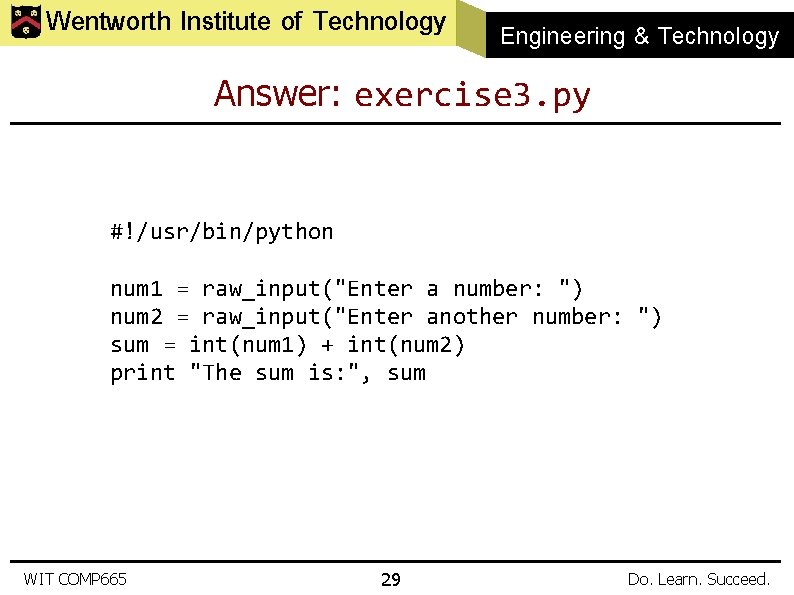
Wentworth Institute of Technology Engineering & Technology Answer: exercise 3. py #!/usr/bin/python num 1 = raw_input("Enter a number: ") num 2 = raw_input("Enter another number: ") sum = int(num 1) + int(num 2) print "The sum is: ", sum WIT COMP 665 29 Do. Learn. Succeed.
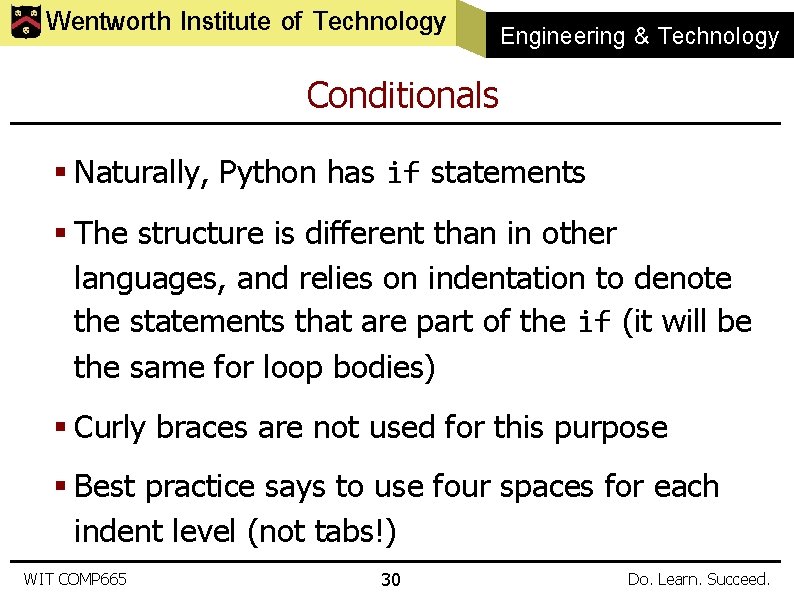
Wentworth Institute of Technology Engineering & Technology Conditionals § Naturally, Python has if statements § The structure is different than in other languages, and relies on indentation to denote the statements that are part of the if (it will be the same for loop bodies) § Curly braces are not used for this purpose § Best practice says to use four spaces for each indent level (not tabs!) WIT COMP 665 30 Do. Learn. Succeed.
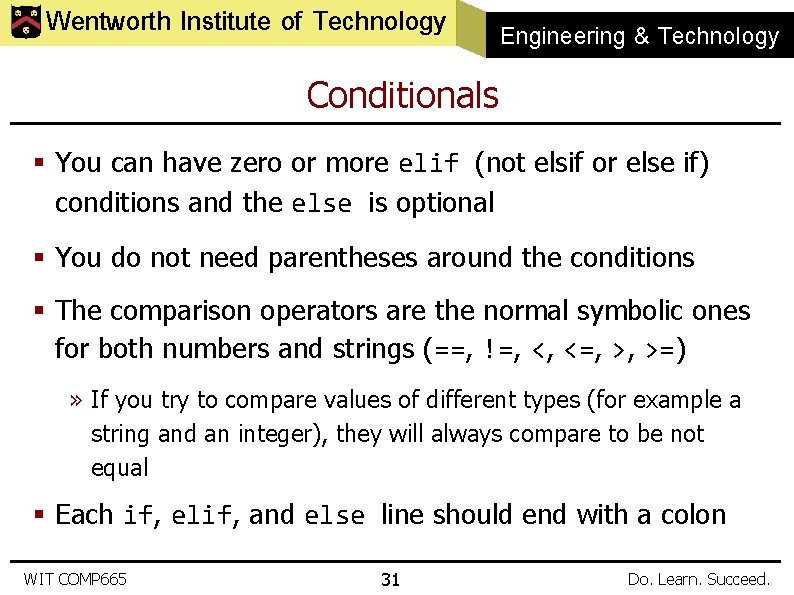
Wentworth Institute of Technology Engineering & Technology Conditionals § You can have zero or more elif (not elsif or else if) conditions and the else is optional § You do not need parentheses around the conditions § The comparison operators are the normal symbolic ones for both numbers and strings (==, !=, <, <=, >, >=) » If you try to compare values of different types (for example a string and an integer), they will always compare to be not equal § Each if, elif, and else line should end with a colon WIT COMP 665 31 Do. Learn. Succeed.
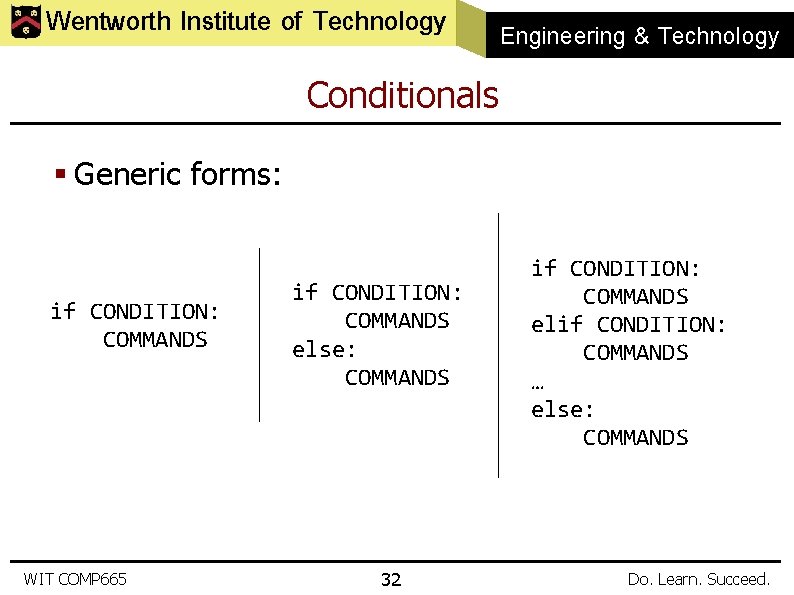
Wentworth Institute of Technology Engineering & Technology Conditionals § Generic forms: if CONDITION: COMMANDS WIT COMP 665 if CONDITION: COMMANDS else: COMMANDS 32 if CONDITION: COMMANDS elif CONDITION: COMMANDS … else: COMMANDS Do. Learn. Succeed.
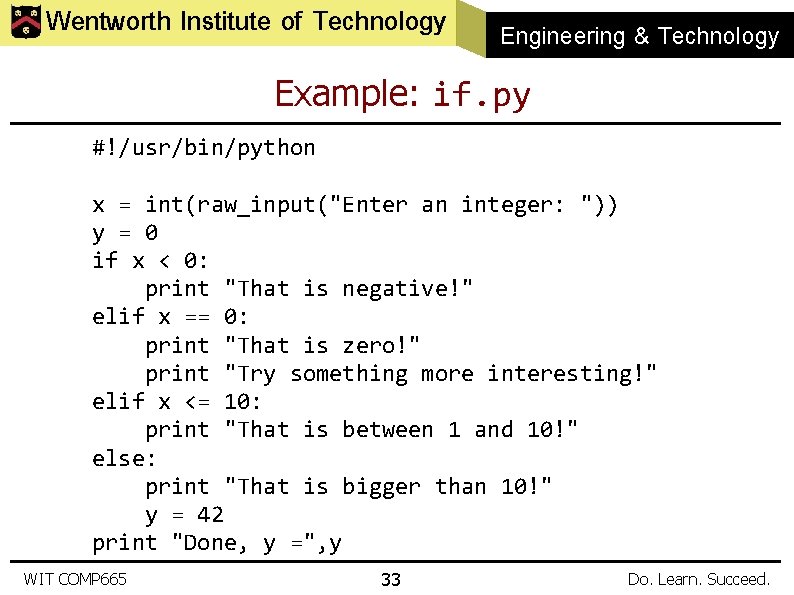
Wentworth Institute of Technology Engineering & Technology Example: if. py #!/usr/bin/python x = int(raw_input("Enter an integer: ")) y = 0 if x < 0: print "That is negative!" elif x == 0: print "That is zero!" print "Try something more interesting!" elif x <= 10: print "That is between 1 and 10!" else: print "That is bigger than 10!" y = 42 print "Done, y =", y WIT COMP 665 33 Do. Learn. Succeed.
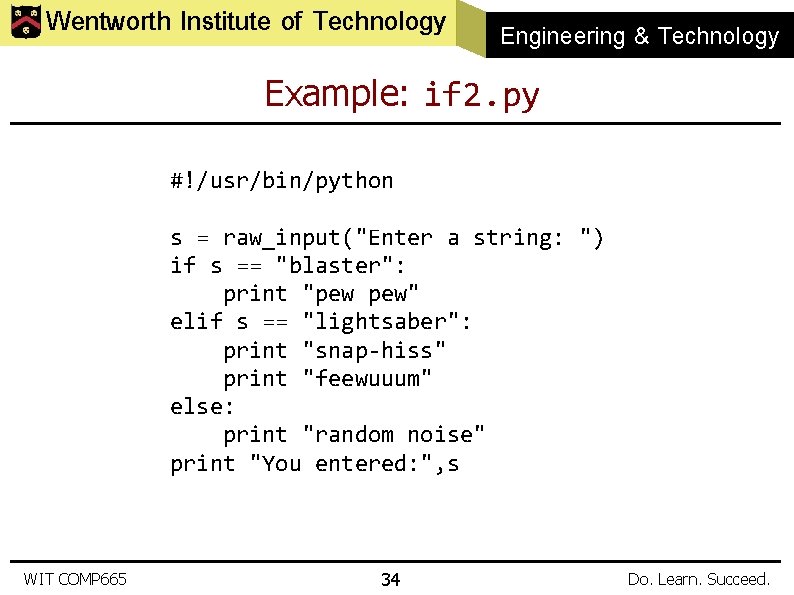
Wentworth Institute of Technology Engineering & Technology Example: if 2. py #!/usr/bin/python s = raw_input("Enter a string: ") if s == "blaster": print "pew pew" elif s == "lightsaber": print "snap-hiss" print "feewuuum" else: print "random noise" print "You entered: ", s WIT COMP 665 34 Do. Learn. Succeed.
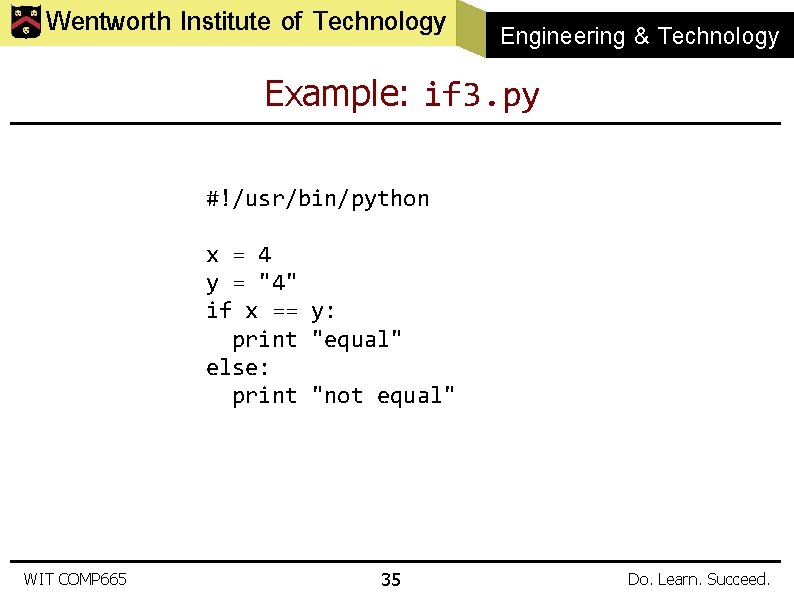
Wentworth Institute of Technology Engineering & Technology Example: if 3. py #!/usr/bin/python x = 4 y = "4" if x == y: print "equal" else: print "not equal" WIT COMP 665 35 Do. Learn. Succeed.
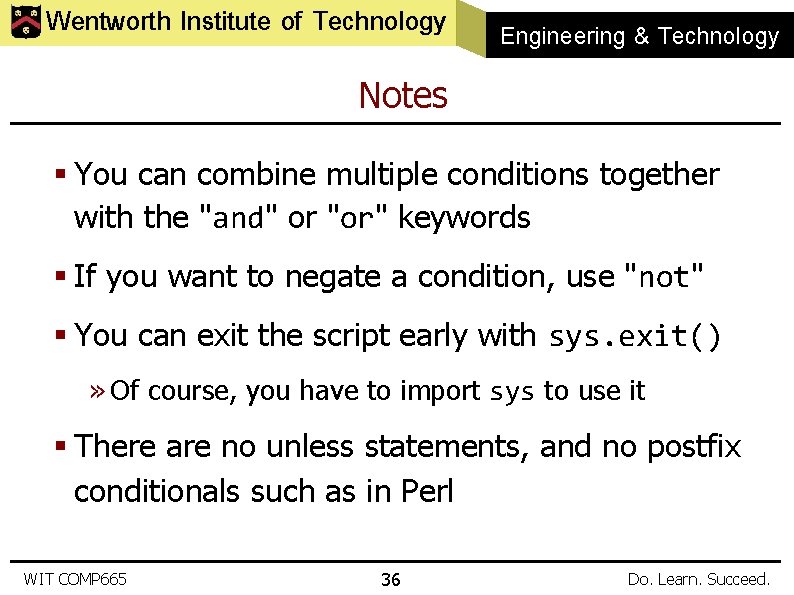
Wentworth Institute of Technology Engineering & Technology Notes § You can combine multiple conditions together with the "and" or "or" keywords § If you want to negate a condition, use "not" § You can exit the script early with sys. exit() » Of course, you have to import sys to use it § There are no unless statements, and no postfix conditionals such as in Perl WIT COMP 665 36 Do. Learn. Succeed.
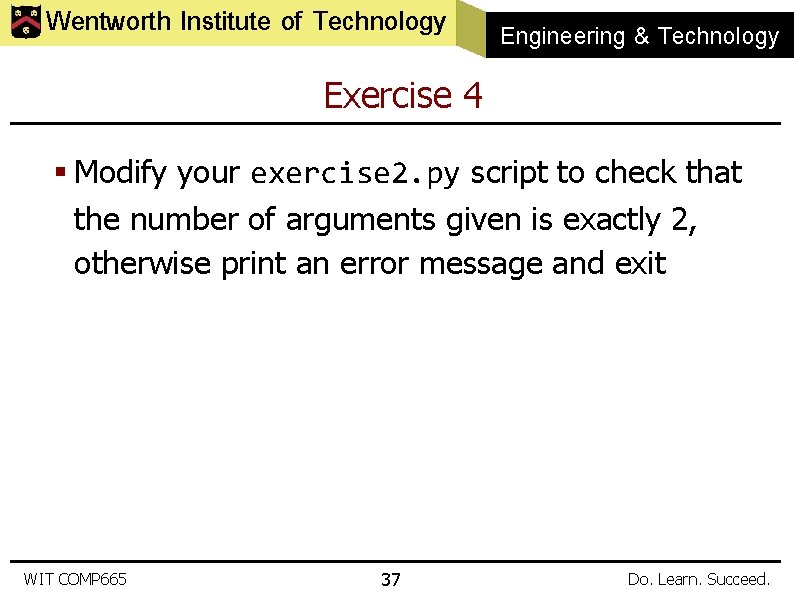
Wentworth Institute of Technology Engineering & Technology Exercise 4 § Modify your exercise 2. py script to check that the number of arguments given is exactly 2, otherwise print an error message and exit WIT COMP 665 37 Do. Learn. Succeed.
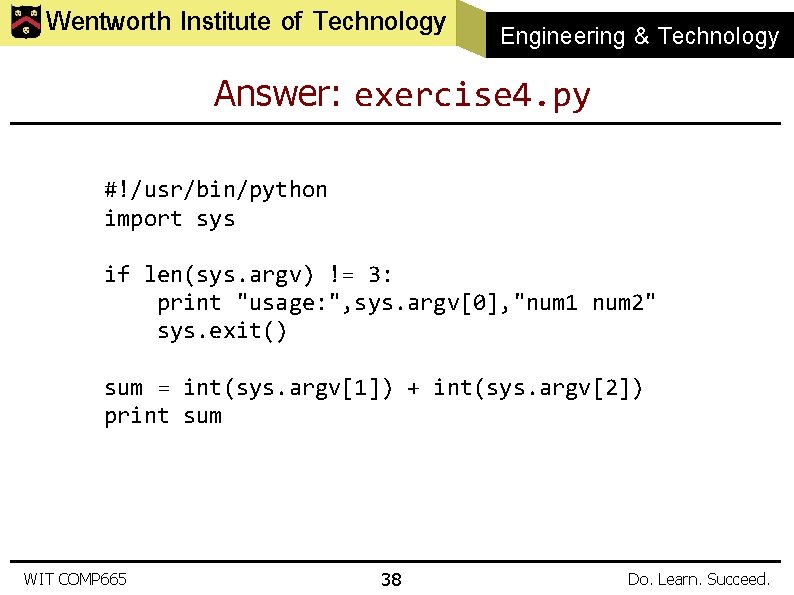
Wentworth Institute of Technology Engineering & Technology Answer: exercise 4. py #!/usr/bin/python import sys if len(sys. argv) != 3: print "usage: ", sys. argv[0], "num 1 num 2" sys. exit() sum = int(sys. argv[1]) + int(sys. argv[2]) print sum WIT COMP 665 38 Do. Learn. Succeed.
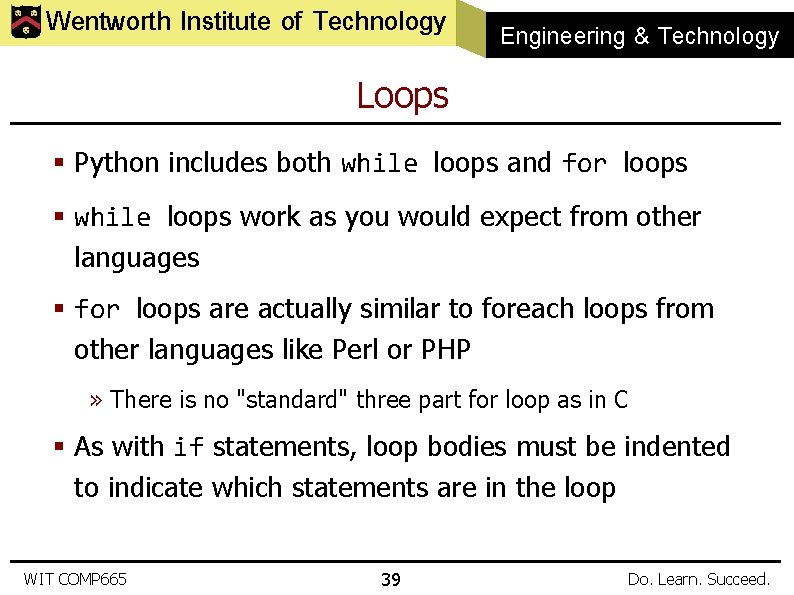
Wentworth Institute of Technology Engineering & Technology Loops § Python includes both while loops and for loops § while loops work as you would expect from other languages § for loops are actually similar to foreach loops from other languages like Perl or PHP » There is no "standard" three part for loop as in C § As with if statements, loop bodies must be indented to indicate which statements are in the loop WIT COMP 665 39 Do. Learn. Succeed.
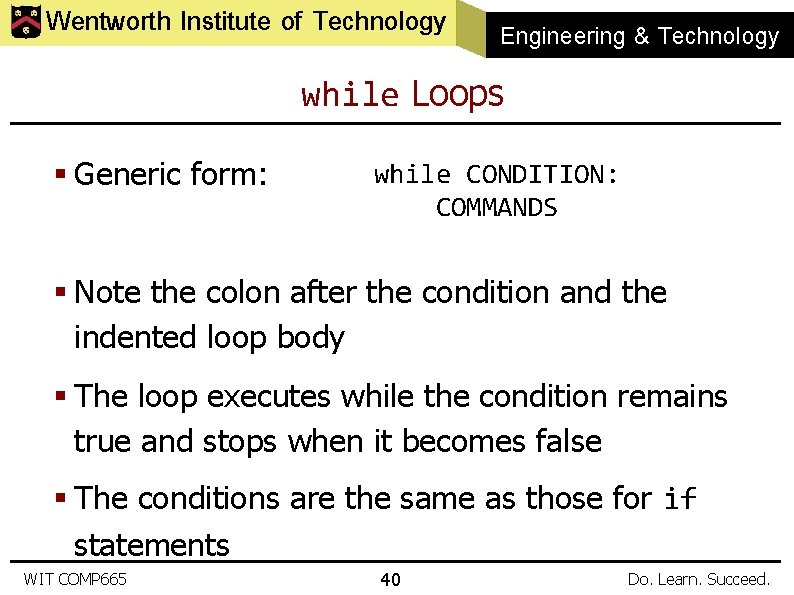
Wentworth Institute of Technology Engineering & Technology while Loops § Generic form: while CONDITION: COMMANDS § Note the colon after the condition and the indented loop body § The loop executes while the condition remains true and stops when it becomes false § The conditions are the same as those for if statements WIT COMP 665 40 Do. Learn. Succeed.
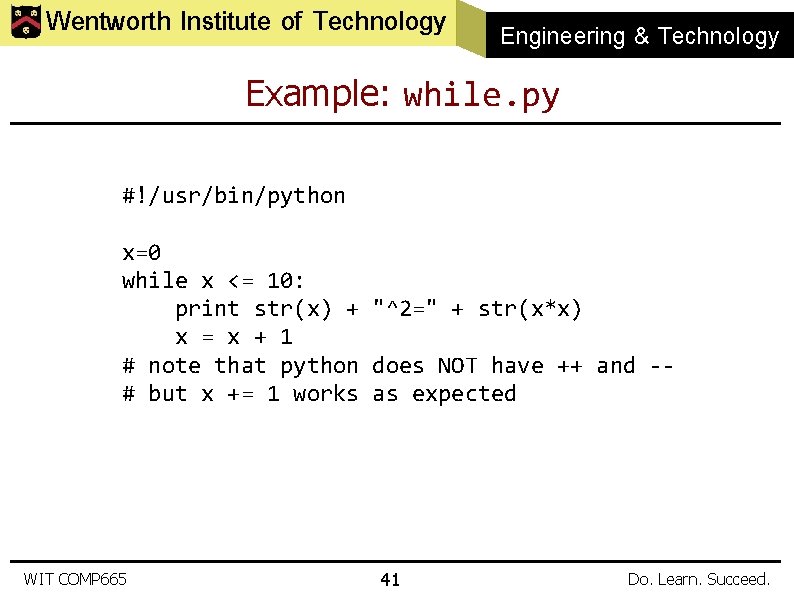
Wentworth Institute of Technology Engineering & Technology Example: while. py #!/usr/bin/python x=0 while x <= 10: print str(x) + "^2=" + str(x*x) x = x + 1 # note that python does NOT have ++ and -# but x += 1 works as expected WIT COMP 665 41 Do. Learn. Succeed.
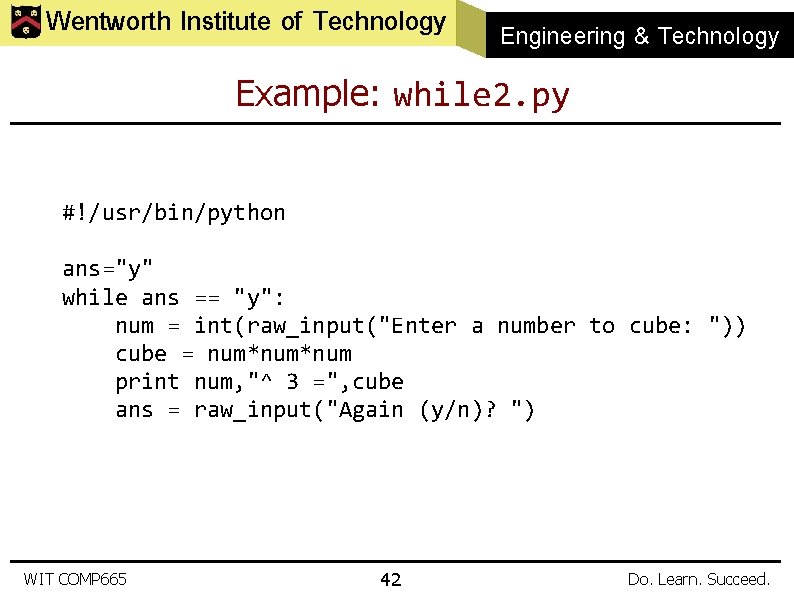
Wentworth Institute of Technology Engineering & Technology Example: while 2. py #!/usr/bin/python ans="y" while ans == "y": num = int(raw_input("Enter a number to cube: ")) cube = num*num print num, "^ 3 =", cube ans = raw_input("Again (y/n)? ") WIT COMP 665 42 Do. Learn. Succeed.
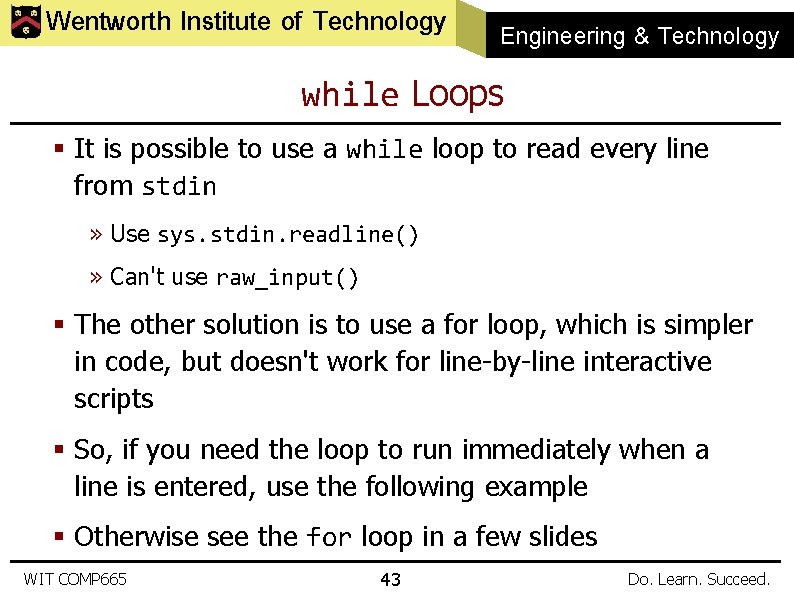
Wentworth Institute of Technology Engineering & Technology while Loops § It is possible to use a while loop to read every line from stdin » Use sys. stdin. readline() » Can't use raw_input() § The other solution is to use a for loop, which is simpler in code, but doesn't work for line-by-line interactive scripts § So, if you need the loop to run immediately when a line is entered, use the following example § Otherwise see the for loop in a few slides WIT COMP 665 43 Do. Learn. Succeed.
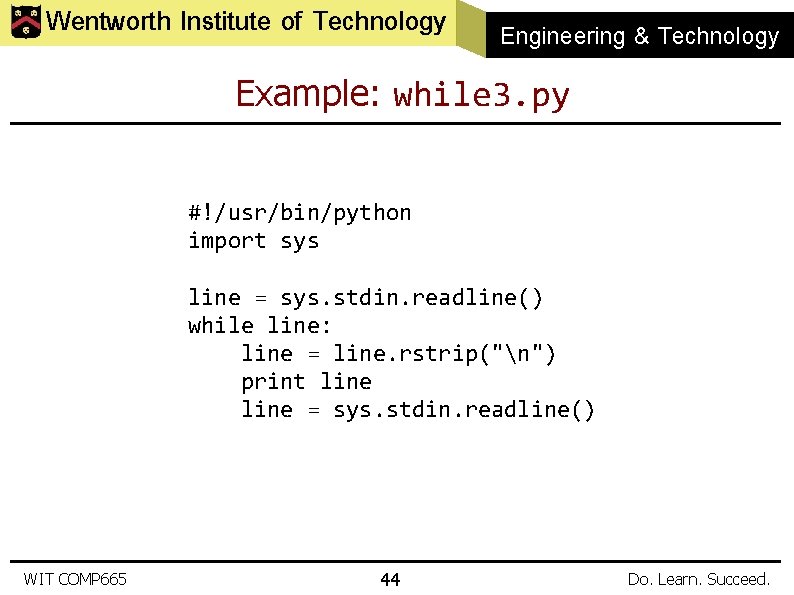
Wentworth Institute of Technology Engineering & Technology Example: while 3. py #!/usr/bin/python import sys line = sys. stdin. readline() while line: line = line. rstrip("n") print line = sys. stdin. readline() WIT COMP 665 44 Do. Learn. Succeed.
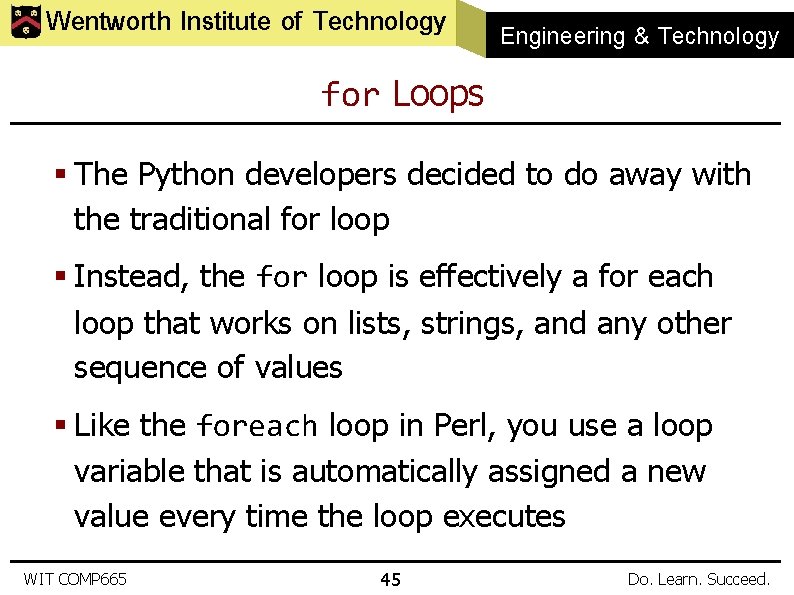
Wentworth Institute of Technology Engineering & Technology for Loops § The Python developers decided to do away with the traditional for loop § Instead, the for loop is effectively a for each loop that works on lists, strings, and any other sequence of values § Like the foreach loop in Perl, you use a loop variable that is automatically assigned a new value every time the loop executes WIT COMP 665 45 Do. Learn. Succeed.
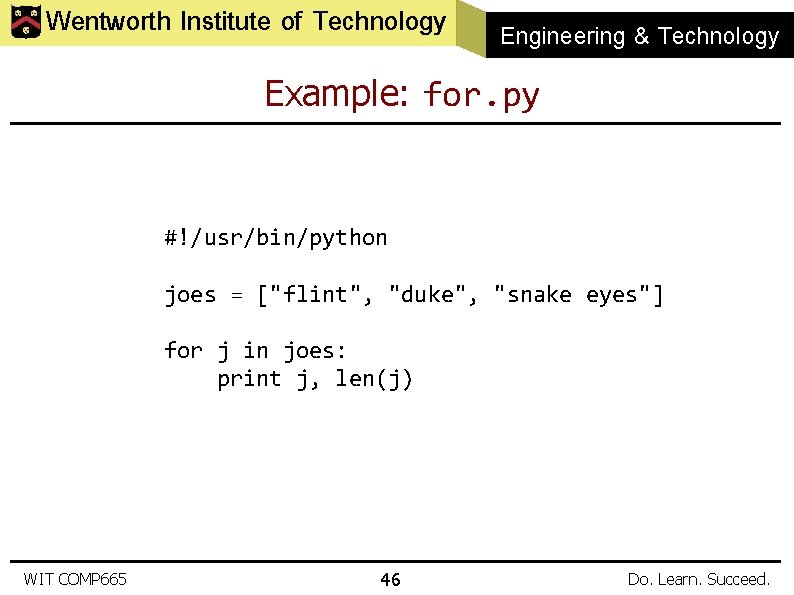
Wentworth Institute of Technology Engineering & Technology Example: for. py #!/usr/bin/python joes = ["flint", "duke", "snake eyes"] for j in joes: print j, len(j) WIT COMP 665 46 Do. Learn. Succeed.
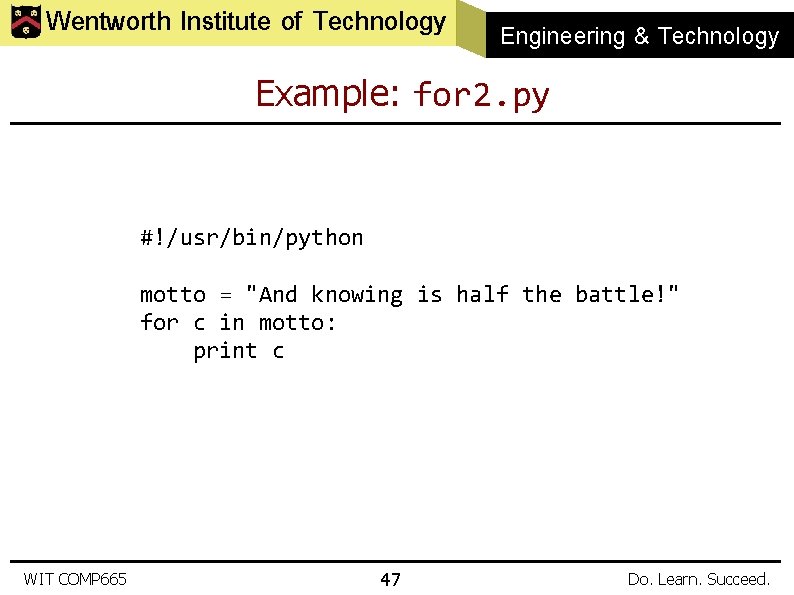
Wentworth Institute of Technology Engineering & Technology Example: for 2. py #!/usr/bin/python motto = "And knowing is half the battle!" for c in motto: print c WIT COMP 665 47 Do. Learn. Succeed.
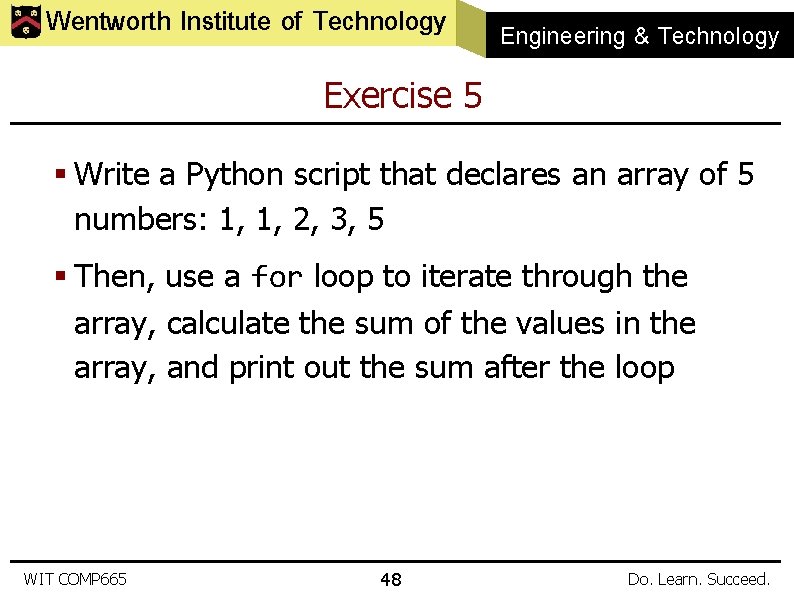
Wentworth Institute of Technology Engineering & Technology Exercise 5 § Write a Python script that declares an array of 5 numbers: 1, 1, 2, 3, 5 § Then, use a for loop to iterate through the array, calculate the sum of the values in the array, and print out the sum after the loop WIT COMP 665 48 Do. Learn. Succeed.
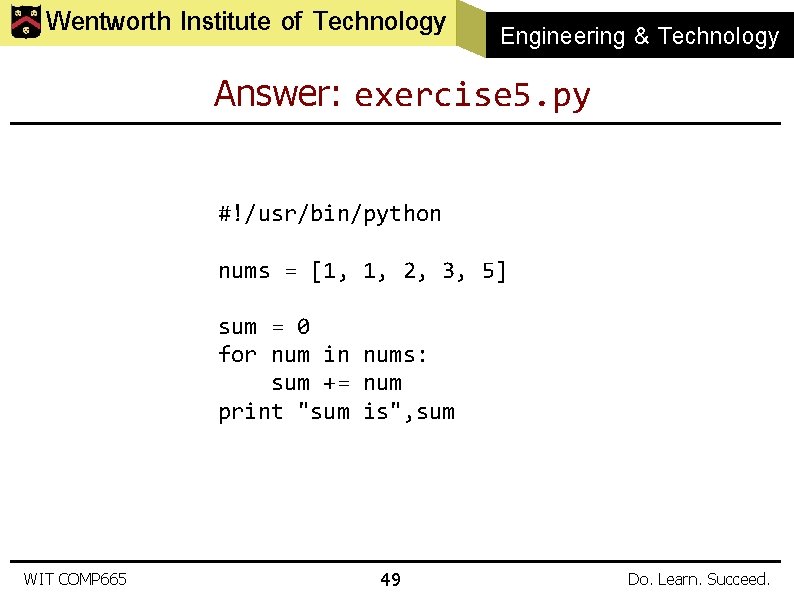
Wentworth Institute of Technology Engineering & Technology Answer: exercise 5. py #!/usr/bin/python nums = [1, 1, 2, 3, 5] sum = 0 for num in nums: sum += num print "sum is", sum WIT COMP 665 49 Do. Learn. Succeed.
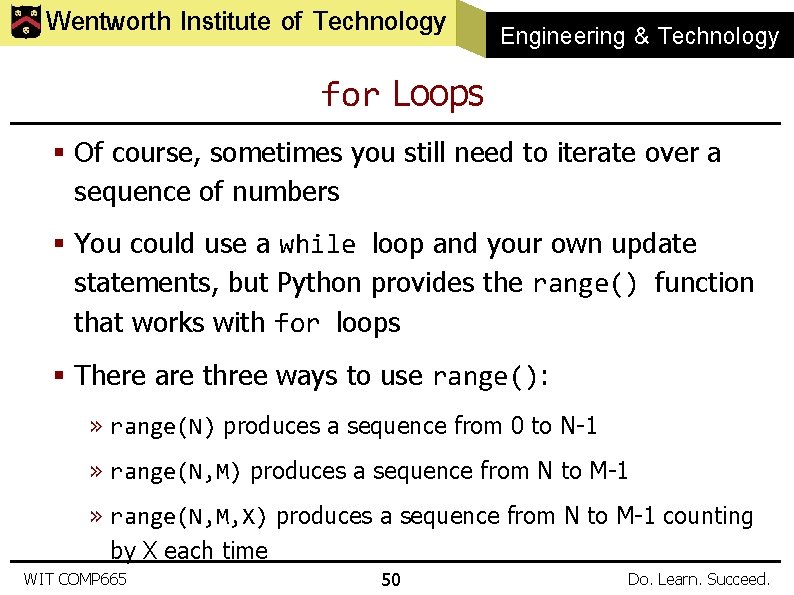
Wentworth Institute of Technology Engineering & Technology for Loops § Of course, sometimes you still need to iterate over a sequence of numbers § You could use a while loop and your own update statements, but Python provides the range() function that works with for loops § There are three ways to use range(): » range(N) produces a sequence from 0 to N-1 » range(N, M) produces a sequence from N to M-1 » range(N, M, X) produces a sequence from N to M-1 counting by X each time WIT COMP 665 50 Do. Learn. Succeed.
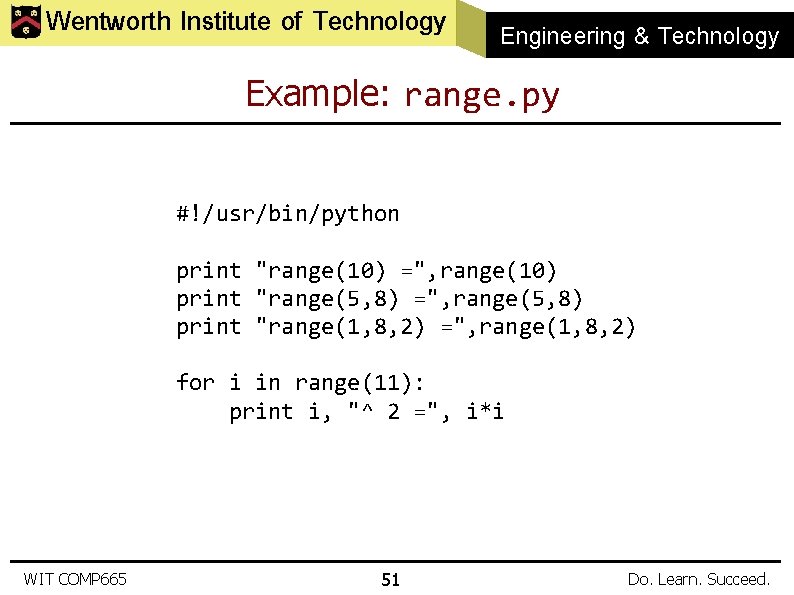
Wentworth Institute of Technology Engineering & Technology Example: range. py #!/usr/bin/python print "range(10) =", range(10) print "range(5, 8) =", range(5, 8) print "range(1, 8, 2) =", range(1, 8, 2) for i in range(11): print i, "^ 2 =", i*i WIT COMP 665 51 Do. Learn. Succeed.
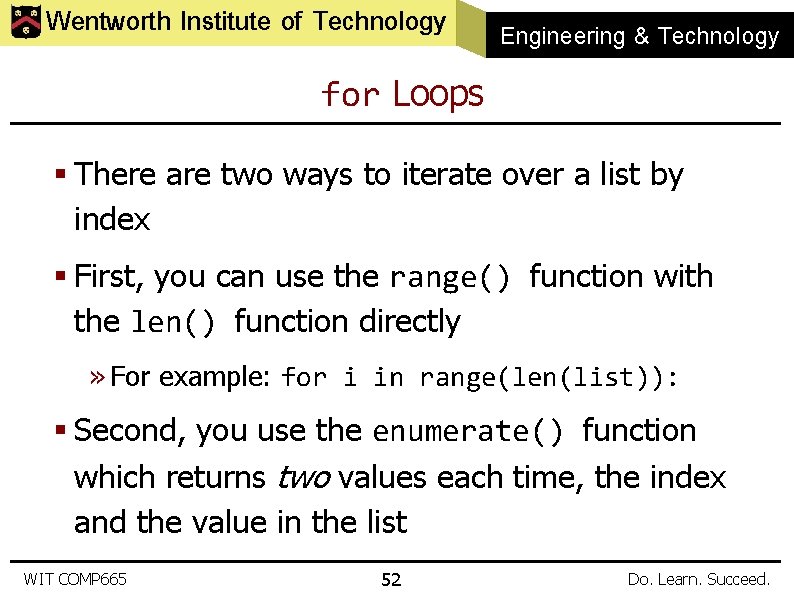
Wentworth Institute of Technology Engineering & Technology for Loops § There are two ways to iterate over a list by index § First, you can use the range() function with the len() function directly » For example: for i in range(len(list)): § Second, you use the enumerate() function which returns two values each time, the index and the value in the list WIT COMP 665 52 Do. Learn. Succeed.
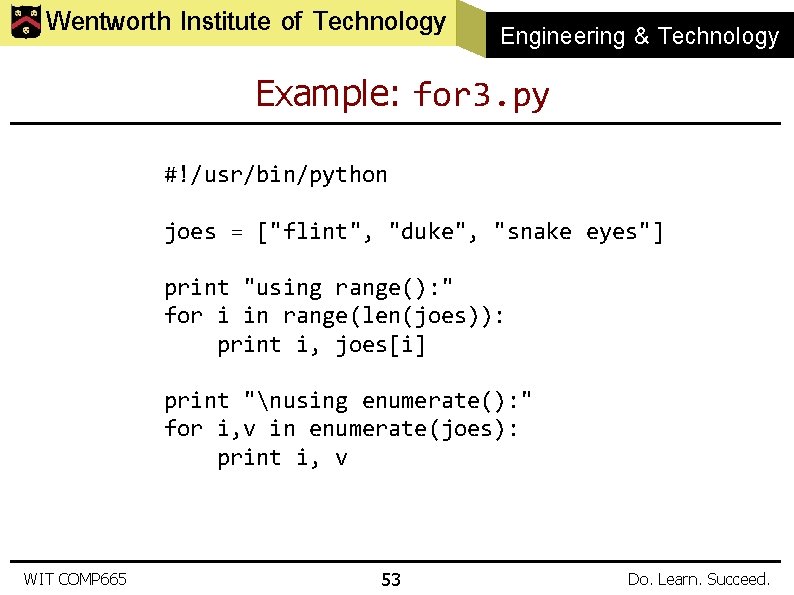
Wentworth Institute of Technology Engineering & Technology Example: for 3. py #!/usr/bin/python joes = ["flint", "duke", "snake eyes"] print "using range(): " for i in range(len(joes)): print i, joes[i] print "nusing enumerate(): " for i, v in enumerate(joes): print i, v WIT COMP 665 53 Do. Learn. Succeed.
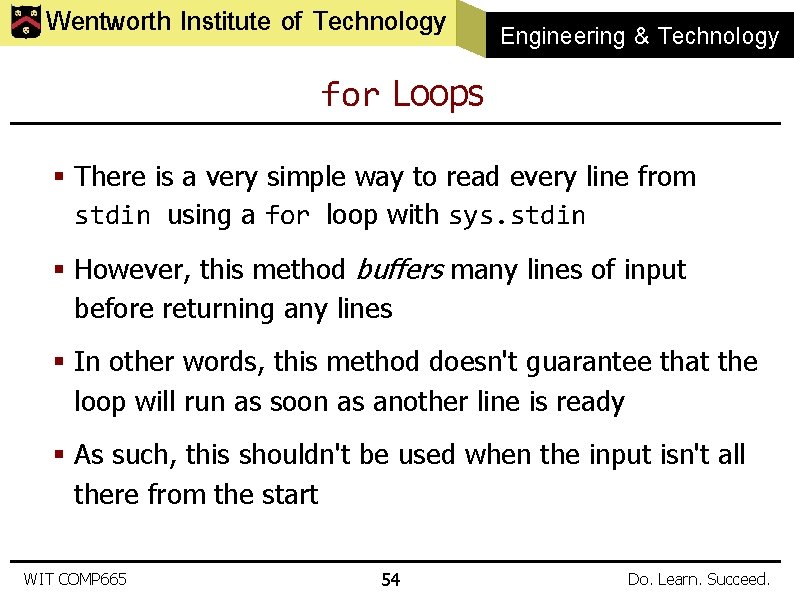
Wentworth Institute of Technology Engineering & Technology for Loops § There is a very simple way to read every line from stdin using a for loop with sys. stdin § However, this method buffers many lines of input before returning any lines § In other words, this method doesn't guarantee that the loop will run as soon as another line is ready § As such, this shouldn't be used when the input isn't all there from the start WIT COMP 665 54 Do. Learn. Succeed.
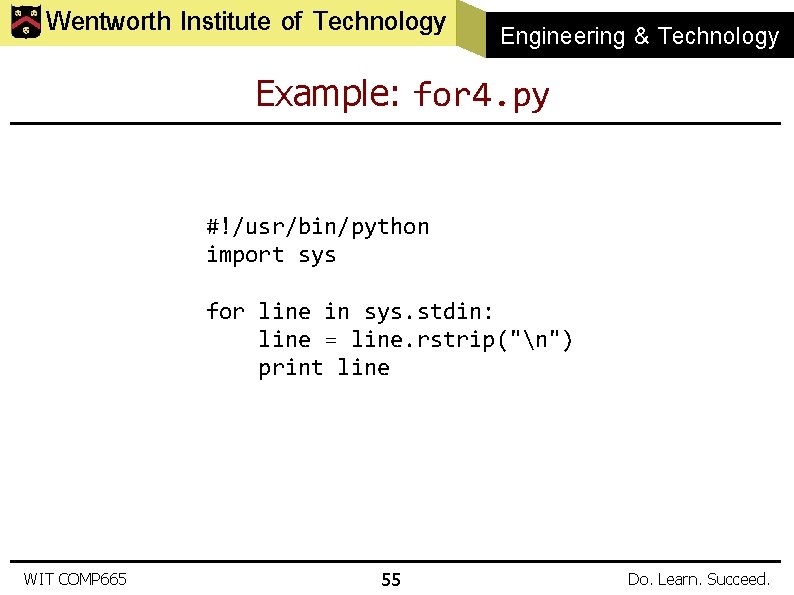
Wentworth Institute of Technology Engineering & Technology Example: for 4. py #!/usr/bin/python import sys for line in sys. stdin: line = line. rstrip("n") print line WIT COMP 665 55 Do. Learn. Succeed.
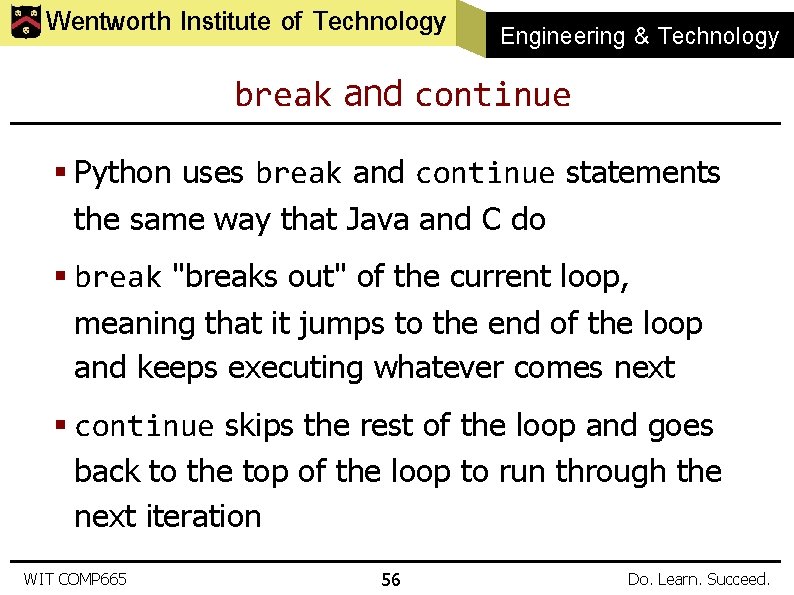
Wentworth Institute of Technology Engineering & Technology break and continue § Python uses break and continue statements the same way that Java and C do § break "breaks out" of the current loop, meaning that it jumps to the end of the loop and keeps executing whatever comes next § continue skips the rest of the loop and goes back to the top of the loop to run through the next iteration WIT COMP 665 56 Do. Learn. Succeed.
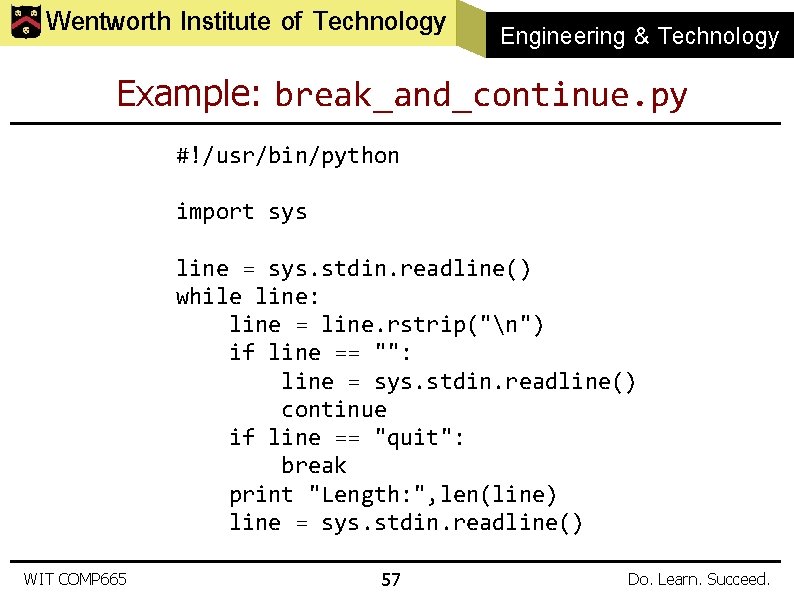
Wentworth Institute of Technology Engineering & Technology Example: break_and_continue. py #!/usr/bin/python import sys line = sys. stdin. readline() while line: line = line. rstrip("n") if line == "": line = sys. stdin. readline() continue if line == "quit": break print "Length: ", len(line) line = sys. stdin. readline() WIT COMP 665 57 Do. Learn. Succeed.
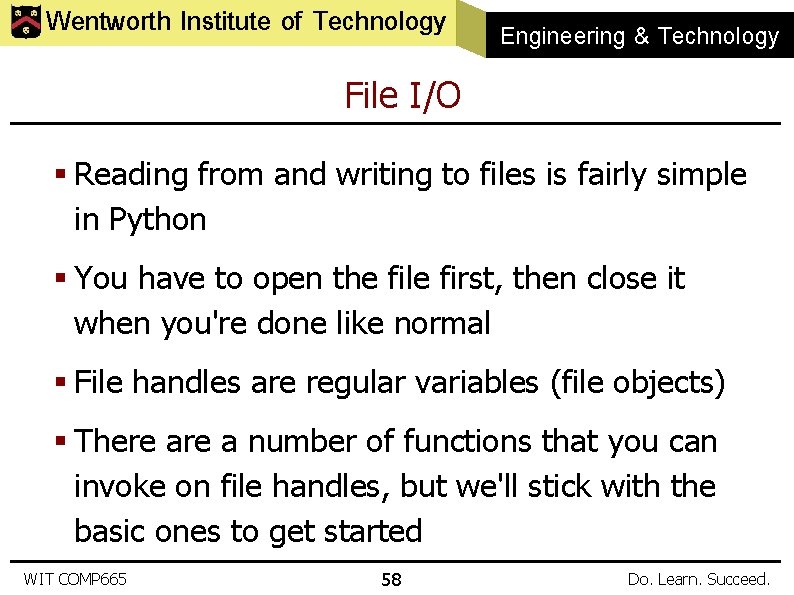
Wentworth Institute of Technology Engineering & Technology File I/O § Reading from and writing to files is fairly simple in Python § You have to open the file first, then close it when you're done like normal § File handles are regular variables (file objects) § There a number of functions that you can invoke on file handles, but we'll stick with the basic ones to get started WIT COMP 665 58 Do. Learn. Succeed.
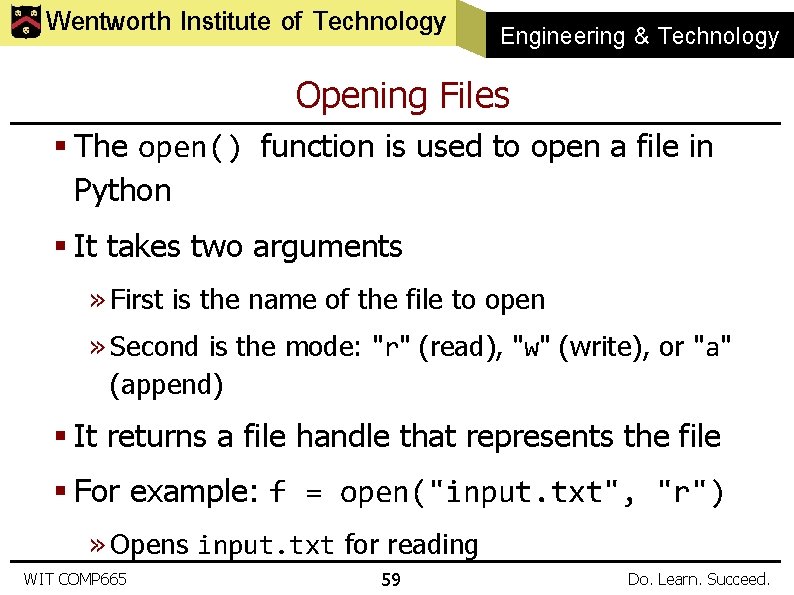
Wentworth Institute of Technology Engineering & Technology Opening Files § The open() function is used to open a file in Python § It takes two arguments » First is the name of the file to open » Second is the mode: "r" (read), "w" (write), or "a" (append) § It returns a file handle that represents the file § For example: f = open("input. txt", "r") » Opens input. txt for reading WIT COMP 665 59 Do. Learn. Succeed.
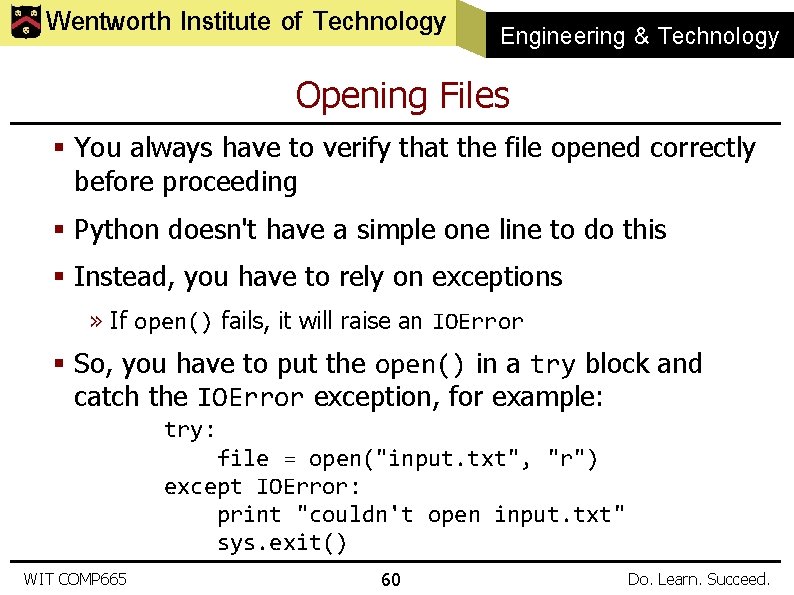
Wentworth Institute of Technology Engineering & Technology Opening Files § You always have to verify that the file opened correctly before proceeding § Python doesn't have a simple one line to do this § Instead, you have to rely on exceptions » If open() fails, it will raise an IOError § So, you have to put the open() in a try block and catch the IOError exception, for example: try: file = open("input. txt", "r") except IOError: print "couldn't open input. txt" sys. exit() WIT COMP 665 60 Do. Learn. Succeed.
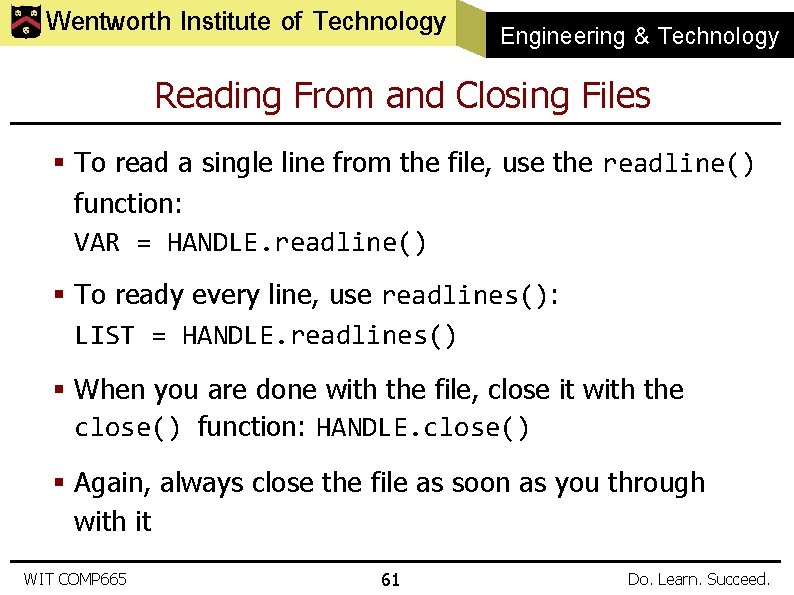
Wentworth Institute of Technology Engineering & Technology Reading From and Closing Files § To read a single line from the file, use the readline() function: VAR = HANDLE. readline() § To ready every line, use readlines(): LIST = HANDLE. readlines() § When you are done with the file, close it with the close() function: HANDLE. close() § Again, always close the file as soon as you through with it WIT COMP 665 61 Do. Learn. Succeed.
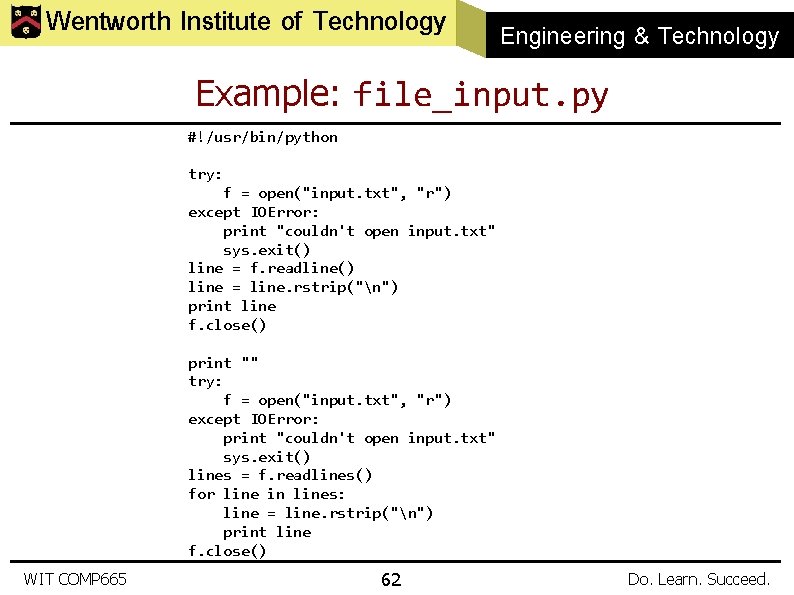
Wentworth Institute of Technology Engineering & Technology Example: file_input. py #!/usr/bin/python try: f = open("input. txt", "r") except IOError: print "couldn't open input. txt" sys. exit() line = f. readline() line = line. rstrip("n") print line f. close() print "" try: f = open("input. txt", "r") except IOError: print "couldn't open input. txt" sys. exit() lines = f. readlines() for line in lines: line = line. rstrip("n") print line f. close() WIT COMP 665 62 Do. Learn. Succeed.
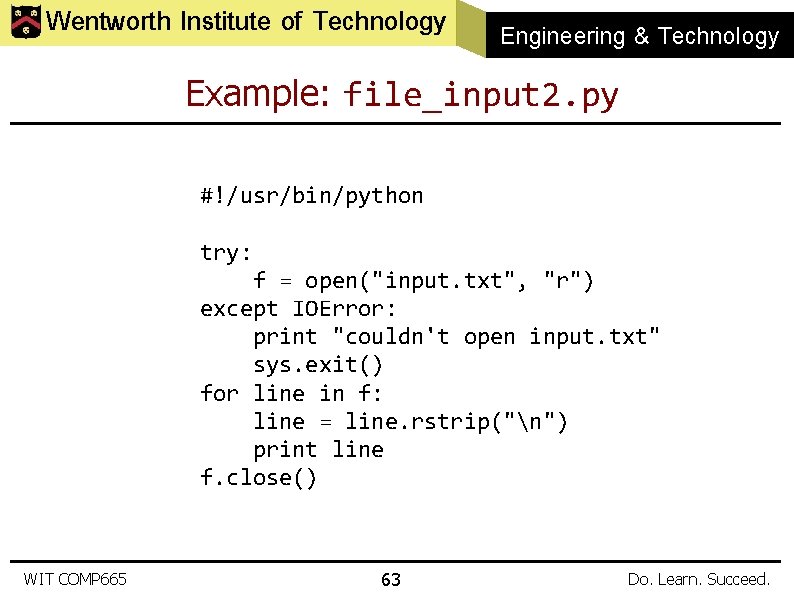
Wentworth Institute of Technology Engineering & Technology Example: file_input 2. py #!/usr/bin/python try: f = open("input. txt", "r") except IOError: print "couldn't open input. txt" sys. exit() for line in f: line = line. rstrip("n") print line f. close() WIT COMP 665 63 Do. Learn. Succeed.
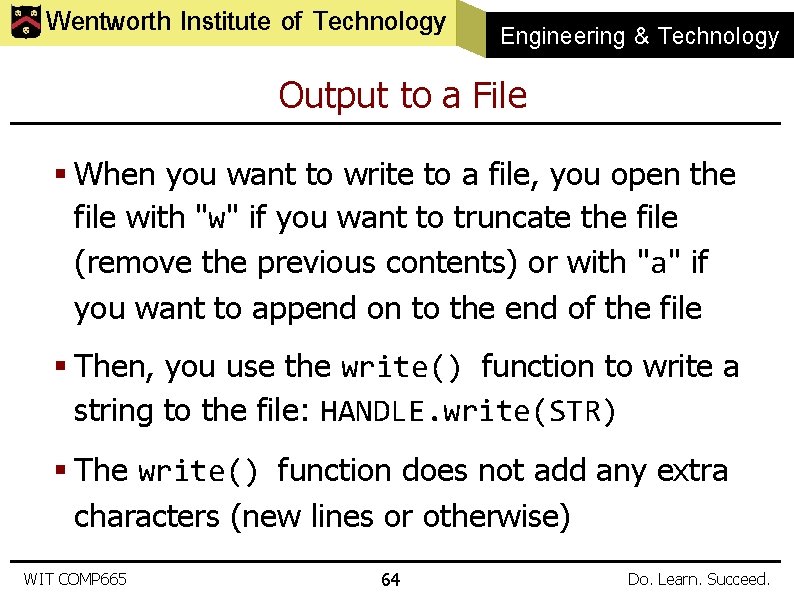
Wentworth Institute of Technology Engineering & Technology Output to a File § When you want to write to a file, you open the file with "w" if you want to truncate the file (remove the previous contents) or with "a" if you want to append on to the end of the file § Then, you use the write() function to write a string to the file: HANDLE. write(STR) § The write() function does not add any extra characters (new lines or otherwise) WIT COMP 665 64 Do. Learn. Succeed.
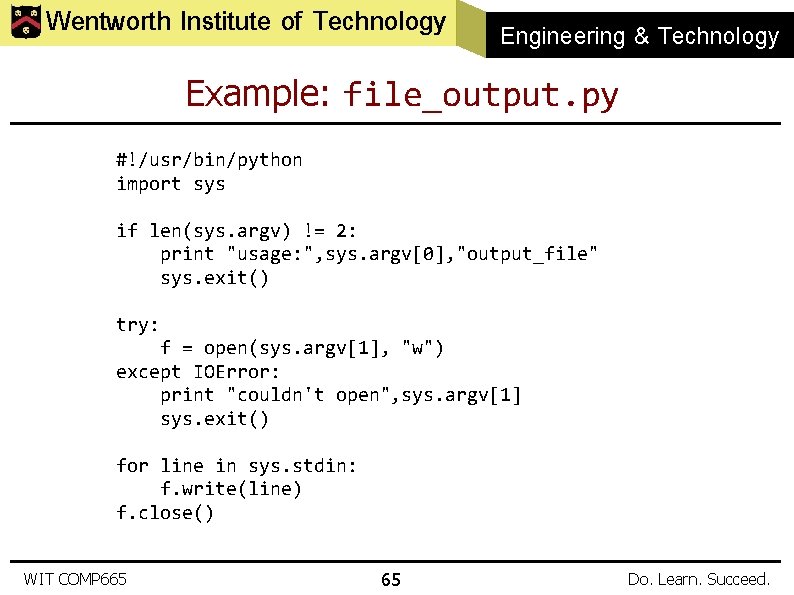
Wentworth Institute of Technology Engineering & Technology Example: file_output. py #!/usr/bin/python import sys if len(sys. argv) != 2: print "usage: ", sys. argv[0], "output_file" sys. exit() try: f = open(sys. argv[1], "w") except IOError: print "couldn't open", sys. argv[1] sys. exit() for line in sys. stdin: f. write(line) f. close() WIT COMP 665 65 Do. Learn. Succeed.
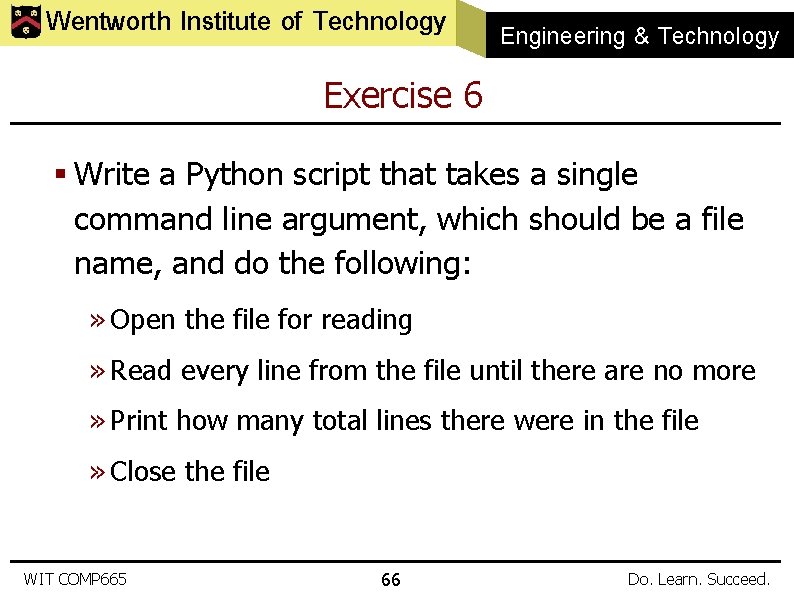
Wentworth Institute of Technology Engineering & Technology Exercise 6 § Write a Python script that takes a single command line argument, which should be a file name, and do the following: » Open the file for reading » Read every line from the file until there are no more » Print how many total lines there were in the file » Close the file WIT COMP 665 66 Do. Learn. Succeed.
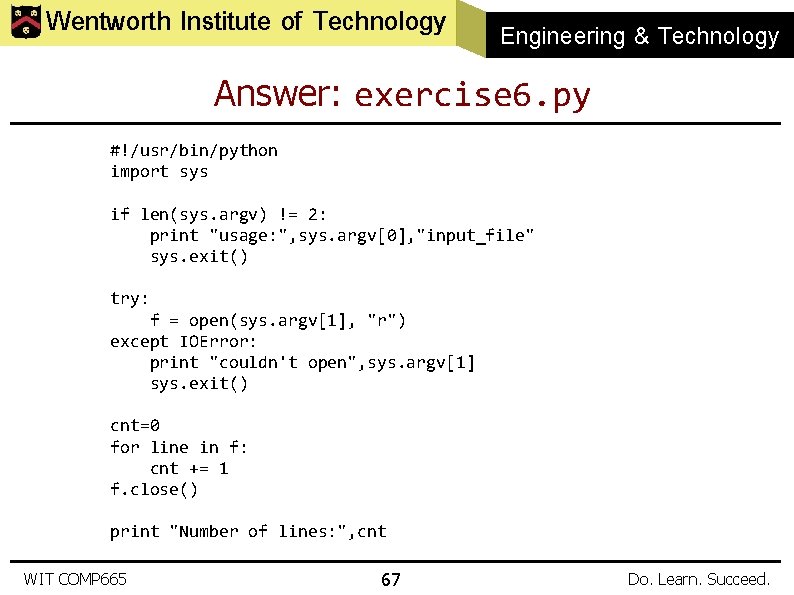
Wentworth Institute of Technology Engineering & Technology Answer: exercise 6. py #!/usr/bin/python import sys if len(sys. argv) != 2: print "usage: ", sys. argv[0], "input_file" sys. exit() try: f = open(sys. argv[1], "r") except IOError: print "couldn't open", sys. argv[1] sys. exit() cnt=0 for line in f: cnt += 1 f. close() print "Number of lines: ", cnt WIT COMP 665 67 Do. Learn. Succeed.
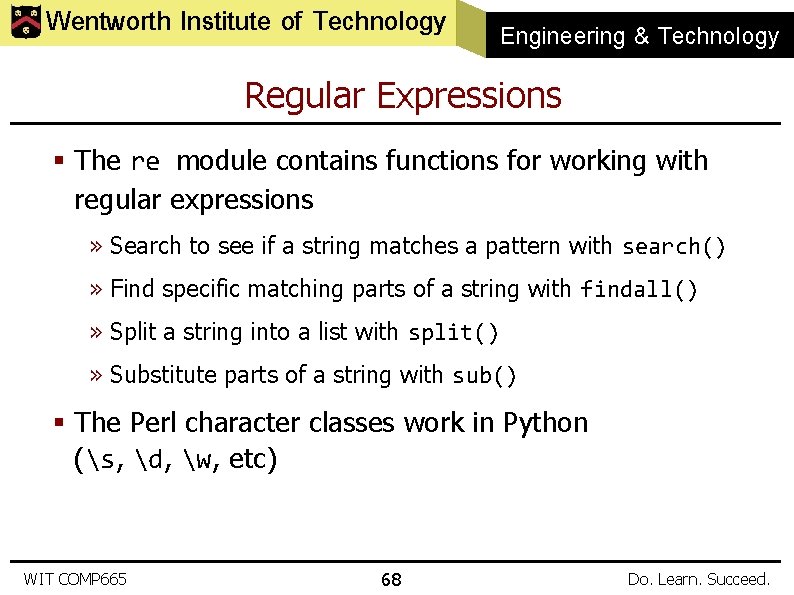
Wentworth Institute of Technology Engineering & Technology Regular Expressions § The re module contains functions for working with regular expressions » Search to see if a string matches a pattern with search() » Find specific matching parts of a string with findall() » Split a string into a list with split() » Substitute parts of a string with sub() § The Perl character classes work in Python (s, d, w, etc) WIT COMP 665 68 Do. Learn. Succeed.
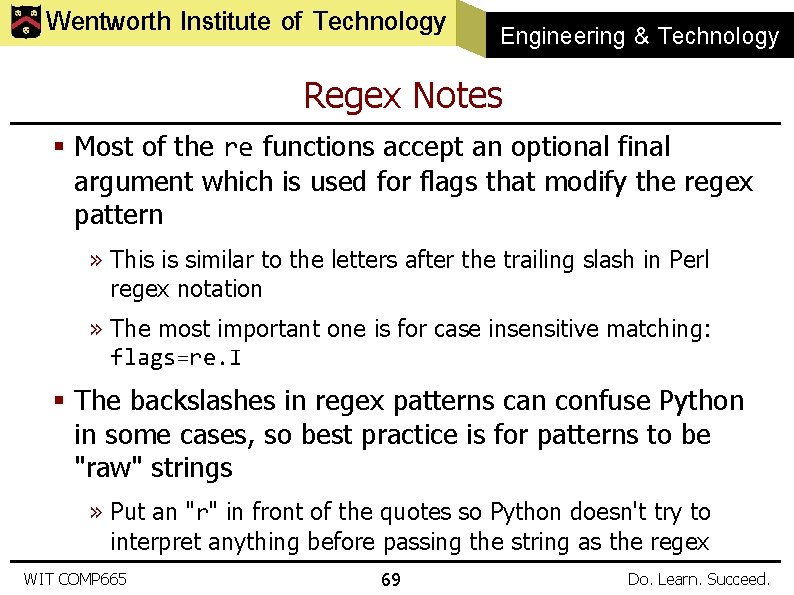
Wentworth Institute of Technology Engineering & Technology Regex Notes § Most of the re functions accept an optional final argument which is used for flags that modify the regex pattern » This is similar to the letters after the trailing slash in Perl regex notation » The most important one is for case insensitive matching: flags=re. I § The backslashes in regex patterns can confuse Python in some cases, so best practice is for patterns to be "raw" strings » Put an "r" in front of the quotes so Python doesn't try to interpret anything before passing the string as the regex WIT COMP 665 69 Do. Learn. Succeed.
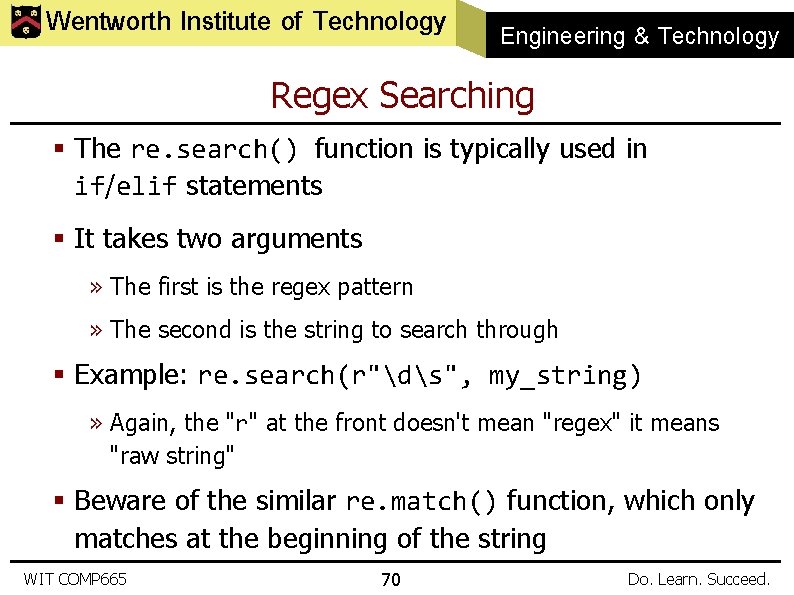
Wentworth Institute of Technology Engineering & Technology Regex Searching § The re. search() function is typically used in if/elif statements § It takes two arguments » The first is the regex pattern » The second is the string to search through § Example: re. search(r"ds", my_string) » Again, the "r" at the front doesn't mean "regex" it means "raw string" § Beware of the similar re. match() function, which only matches at the beginning of the string WIT COMP 665 70 Do. Learn. Succeed.
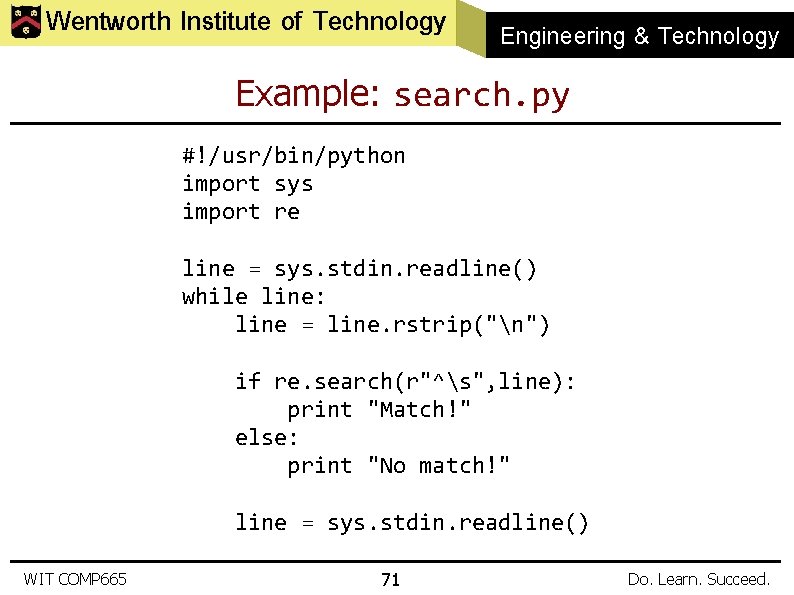
Wentworth Institute of Technology Engineering & Technology Example: search. py #!/usr/bin/python import sys import re line = sys. stdin. readline() while line: line = line. rstrip("n") if re. search(r"^s", line): print "Match!" else: print "No match!" line = sys. stdin. readline() WIT COMP 665 71 Do. Learn. Succeed.
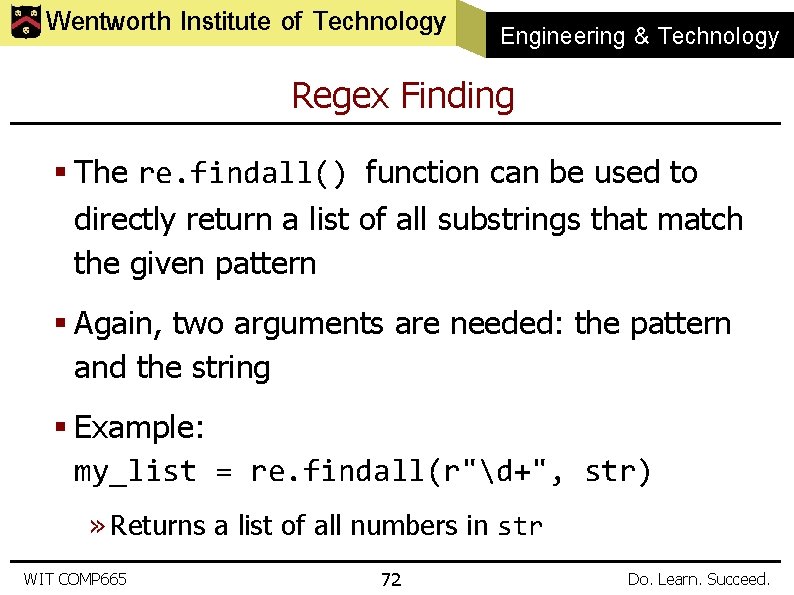
Wentworth Institute of Technology Engineering & Technology Regex Finding § The re. findall() function can be used to directly return a list of all substrings that match the given pattern § Again, two arguments are needed: the pattern and the string § Example: my_list = re. findall(r"d+", str) » Returns a list of all numbers in str WIT COMP 665 72 Do. Learn. Succeed.
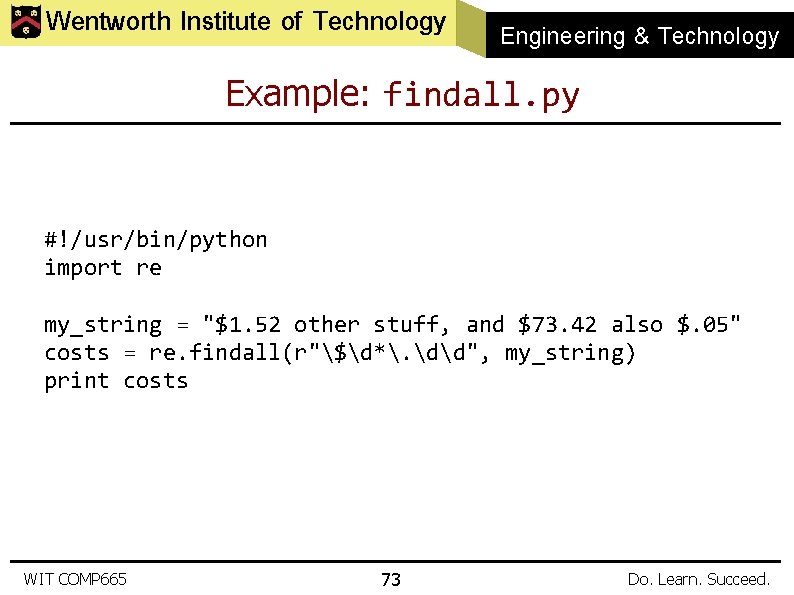
Wentworth Institute of Technology Engineering & Technology Example: findall. py #!/usr/bin/python import re my_string = "$1. 52 other stuff, and $73. 42 also $. 05" costs = re. findall(r"$d*. dd", my_string) print costs WIT COMP 665 73 Do. Learn. Succeed.
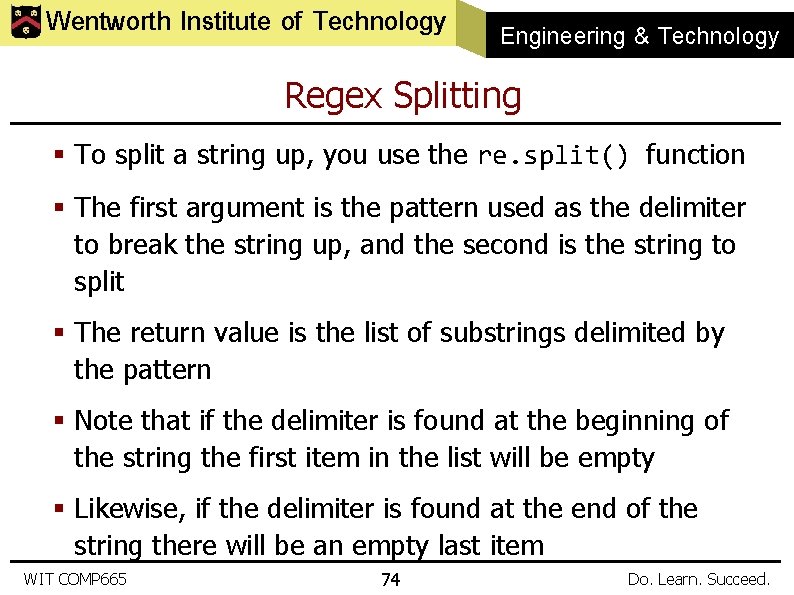
Wentworth Institute of Technology Engineering & Technology Regex Splitting § To split a string up, you use the re. split() function § The first argument is the pattern used as the delimiter to break the string up, and the second is the string to split § The return value is the list of substrings delimited by the pattern § Note that if the delimiter is found at the beginning of the string the first item in the list will be empty § Likewise, if the delimiter is found at the end of the string there will be an empty last item WIT COMP 665 74 Do. Learn. Succeed.
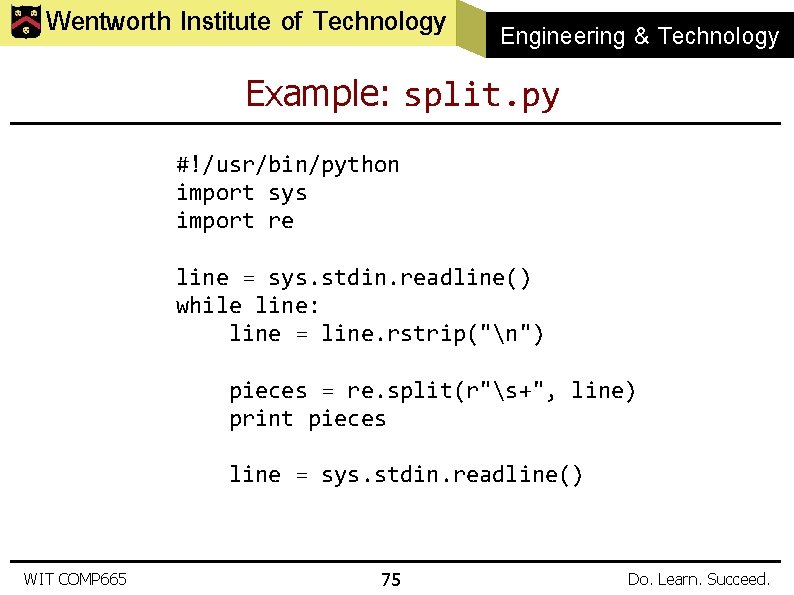
Wentworth Institute of Technology Engineering & Technology Example: split. py #!/usr/bin/python import sys import re line = sys. stdin. readline() while line: line = line. rstrip("n") pieces = re. split(r"s+", line) print pieces line = sys. stdin. readline() WIT COMP 665 75 Do. Learn. Succeed.
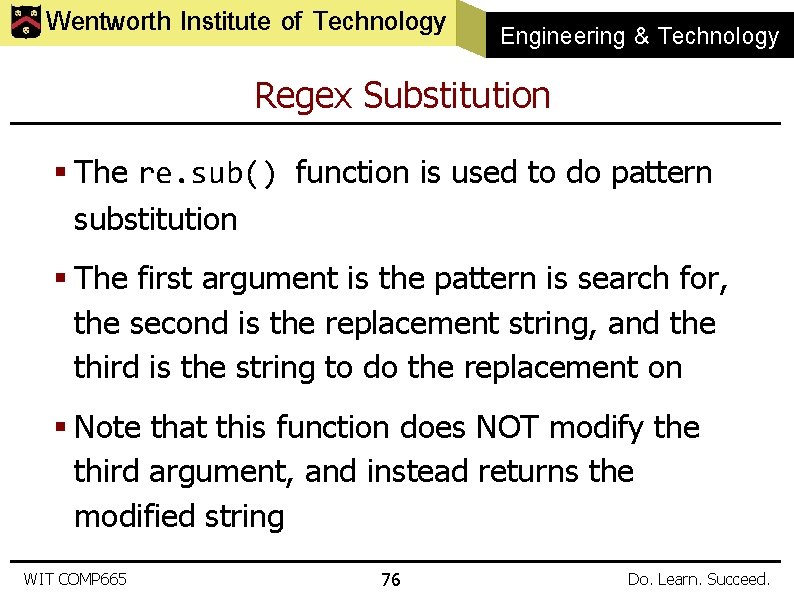
Wentworth Institute of Technology Engineering & Technology Regex Substitution § The re. sub() function is used to do pattern substitution § The first argument is the pattern is search for, the second is the replacement string, and the third is the string to do the replacement on § Note that this function does NOT modify the third argument, and instead returns the modified string WIT COMP 665 76 Do. Learn. Succeed.
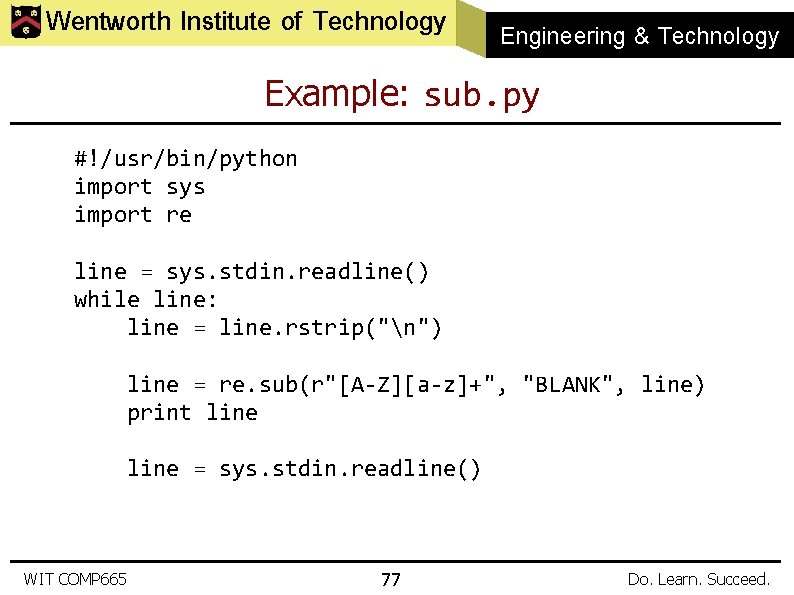
Wentworth Institute of Technology Engineering & Technology Example: sub. py #!/usr/bin/python import sys import re line = sys. stdin. readline() while line: line = line. rstrip("n") line = re. sub(r"[A-Z][a-z]+", "BLANK", line) print line = sys. stdin. readline() WIT COMP 665 77 Do. Learn. Succeed.
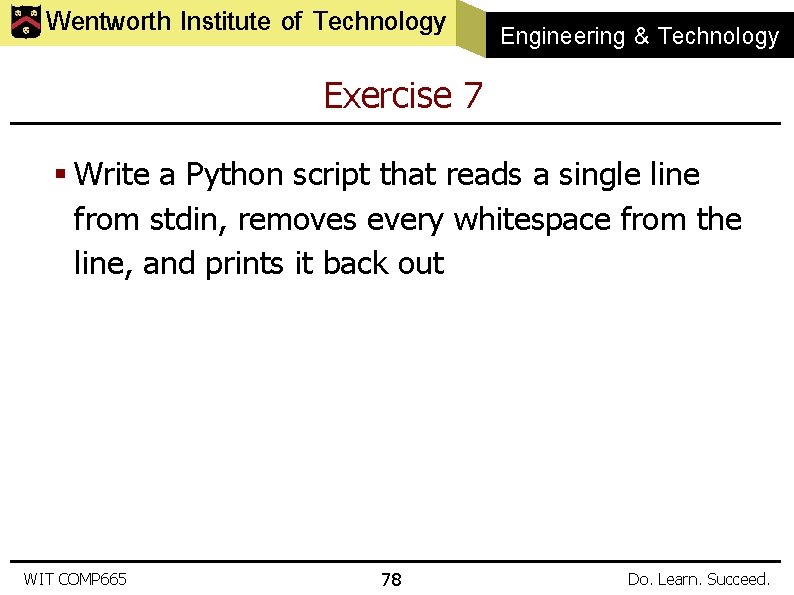
Wentworth Institute of Technology Engineering & Technology Exercise 7 § Write a Python script that reads a single line from stdin, removes every whitespace from the line, and prints it back out WIT COMP 665 78 Do. Learn. Succeed.
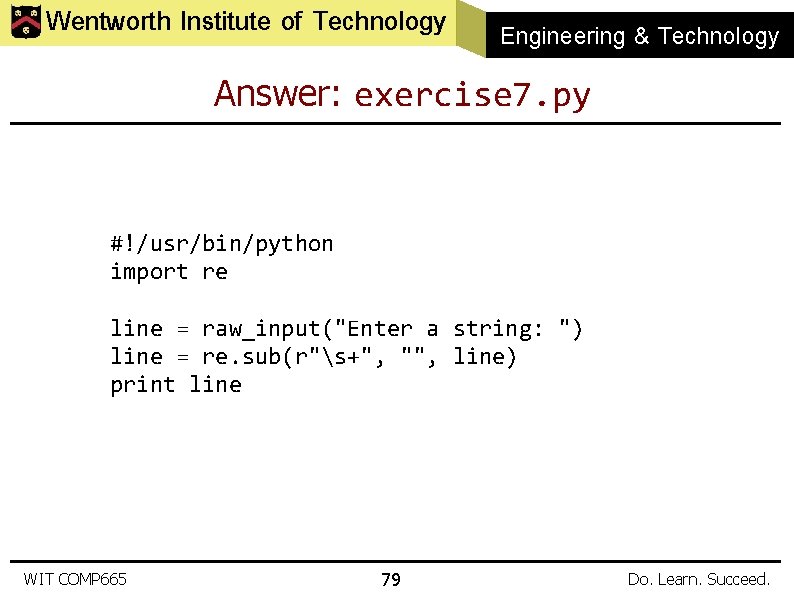
Wentworth Institute of Technology Engineering & Technology Answer: exercise 7. py #!/usr/bin/python import re line = raw_input("Enter a string: ") line = re. sub(r"s+", "", line) print line WIT COMP 665 79 Do. Learn. Succeed.
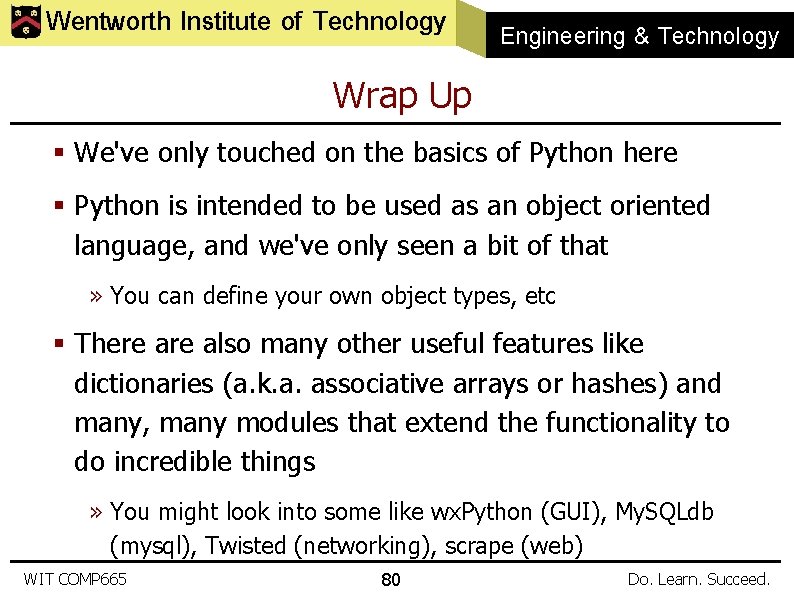
Wentworth Institute of Technology Engineering & Technology Wrap Up § We've only touched on the basics of Python here § Python is intended to be used as an object oriented language, and we've only seen a bit of that » You can define your own object types, etc § There also many other useful features like dictionaries (a. k. a. associative arrays or hashes) and many, many modules that extend the functionality to do incredible things » You might look into some like wx. Python (GUI), My. SQLdb (mysql), Twisted (networking), scrape (web) WIT COMP 665 80 Do. Learn. Succeed.
Scala di wentworth
Wentworth cheswell
Batu lempung
Grain size sorting formula
Wit source technology
Vidhyadeep institute of engineering and technology
Stevens institute of technology mechanical engineering
Mandava institute of engineering and technology
Stevens institute of technology mechanical engineering
Laconic spartan
Cumulatie medicatie betekenis
Wat word n strofe met drie reels genoem
Intensiewe vorm vir koud
Dian's wit
Pandora's box and loo-wit the fire keeper
Examples of irony in pride and prejudice
Dominante waarde methode
John de wit uu
Volrym
Zelfzorgbehoeften
Wit and humor propaganda
Propaganda techniques
De wit venhorst
Wit gele kruis
Forgetfulness potion
Graduate institute of electronics engineering
Worcester polytechnic institute chemical engineering
Grenoble institute of technology
Rensselaer polytechnic institute electrical engineering
Rensselaer polytechnic institute biomedical engineering
El shorouk academy
Electronic engineering polytechnic institute of surabaya
Ntu
Graduate institute of electronics engineering
Graduate institute of electronics engineering
Graduate institute of electronics engineering
Calc institute of technology
Waterford institute of technology vacancies
Ulsan national institute of science and technology
National assistive technology research institute
Masdar institute of science and technology
Madhav institute of technology and science
Gas technology institute
Aperture stop
Dbs institute of technology
Materials technology institute
Download r for windows
Vocational education uae
Sri lanka institute of information technology
Sagar institute of research and technology
Prajnanananda institute of technology
National institute of standards and technology
Monohakobi
Kigali institute of science and technology
Netherlands maritime institute of technology (nmit)
Stevens institute of technology
Kth royal institute of technology notable alumni
Iist pune
Brno institute of technology
Sri lanka institute of information technology affiliations
Shri dadaji institute of technology and science
Prof ram meghe institute of technology and research
Monohakobi technology institute
Illinois institute of technology toefl requirement
Swami vivekanand institute of technology and management
Theory of probability in surveying
It gopeshwar
Saigon institute of technology
Hong kong institute of technology
Dublin institute of technology college of business
National institute of agricultural technology
Communication research
Corporate institute of science and technology bhopal
Addis ababa university institute of technology
What is system in software engineering
Forward engineering in software engineering
Dicapine
Engineering elegant systems: theory of systems engineering
Forward and reverse engineering
Fcc office of engineering and technology
Thakur college of engineering and technology