Viewing and GLUT Angel 2 6 2 10
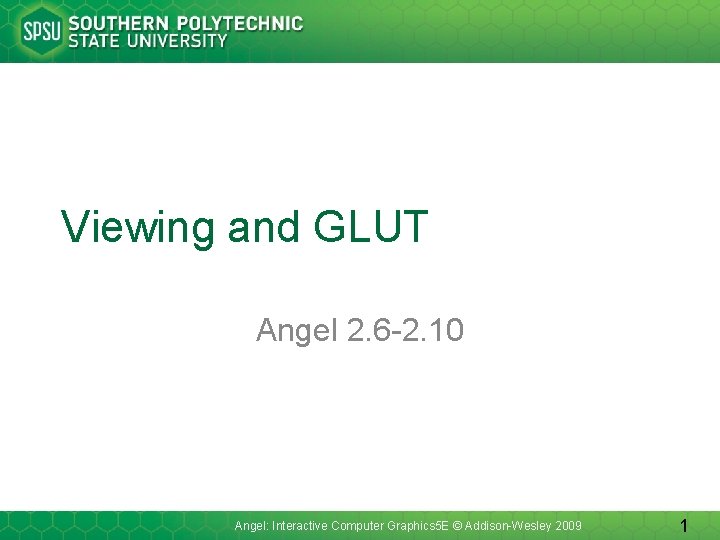
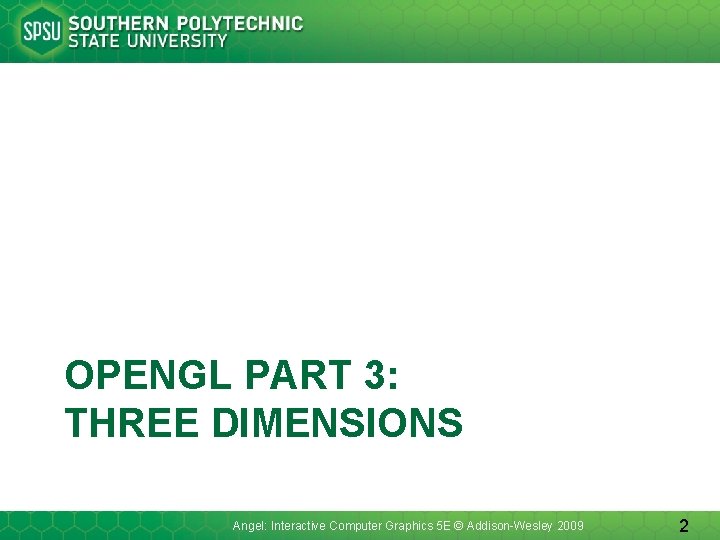
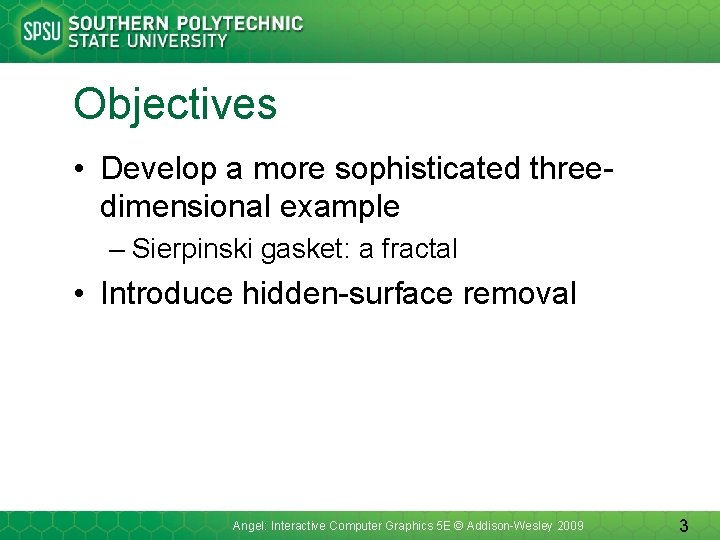
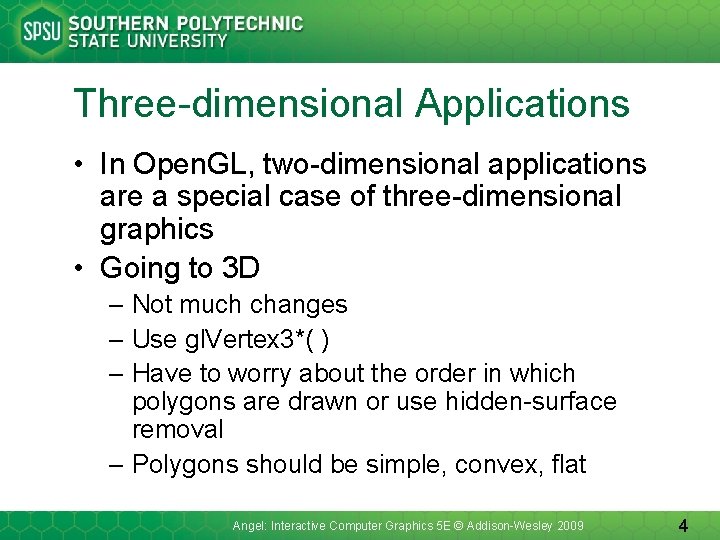
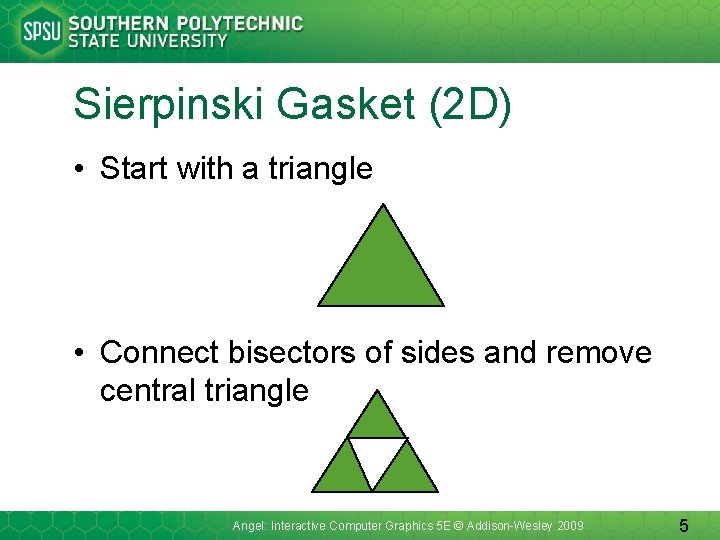
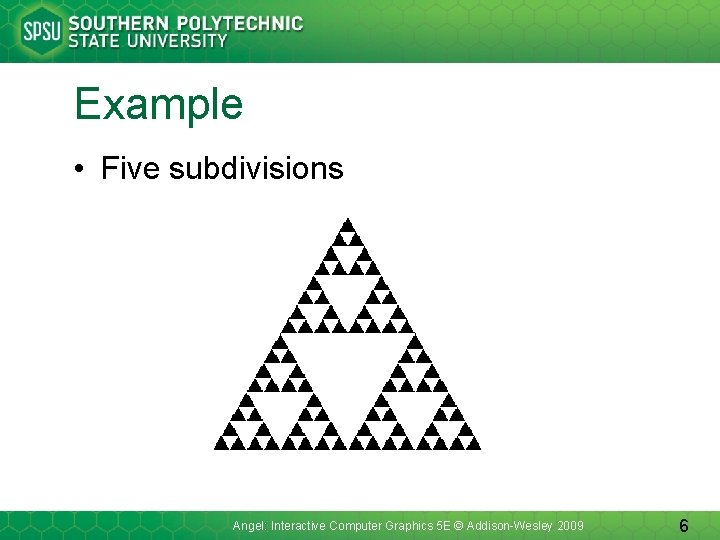
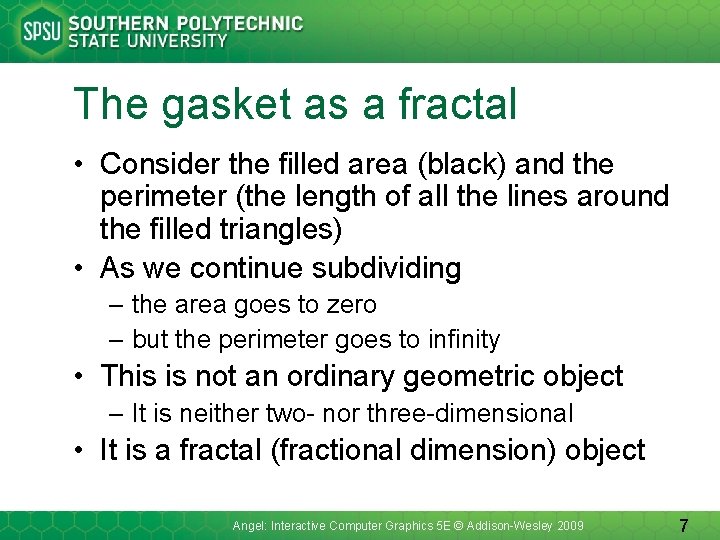
![Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58}, Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58},](https://slidetodoc.com/presentation_image_h2/cb5f87b1a2583a1345228207a6c69945/image-8.jpg)
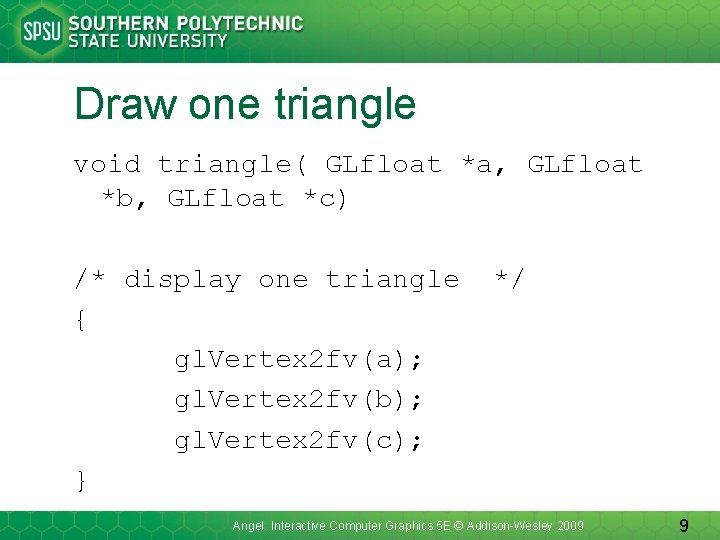
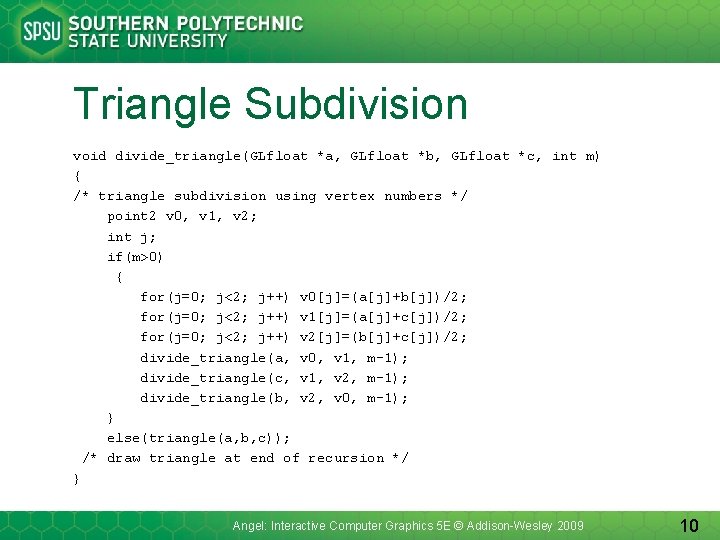
![display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2], display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2],](https://slidetodoc.com/presentation_image_h2/cb5f87b1a2583a1345228207a6c69945/image-11.jpg)
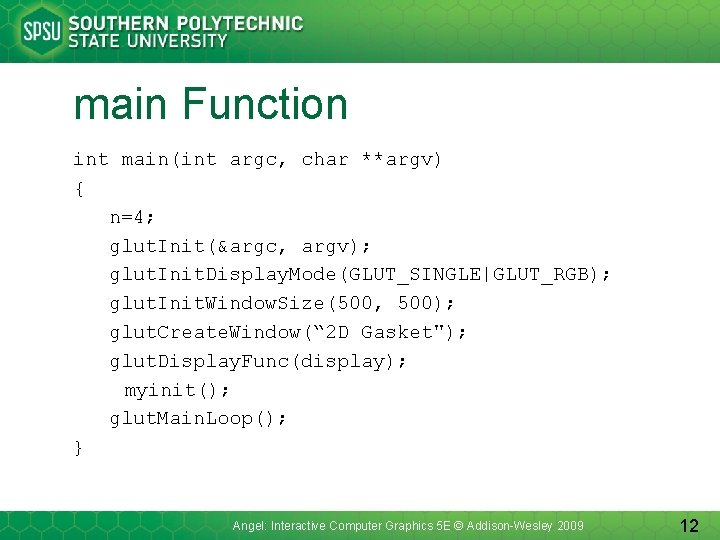
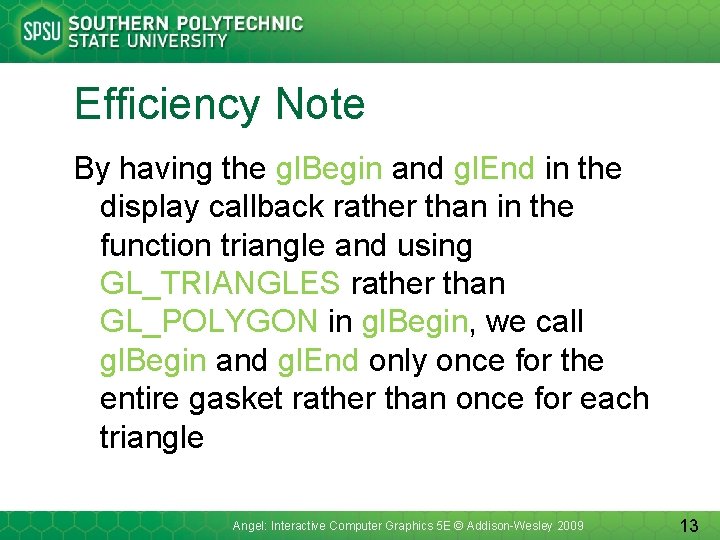
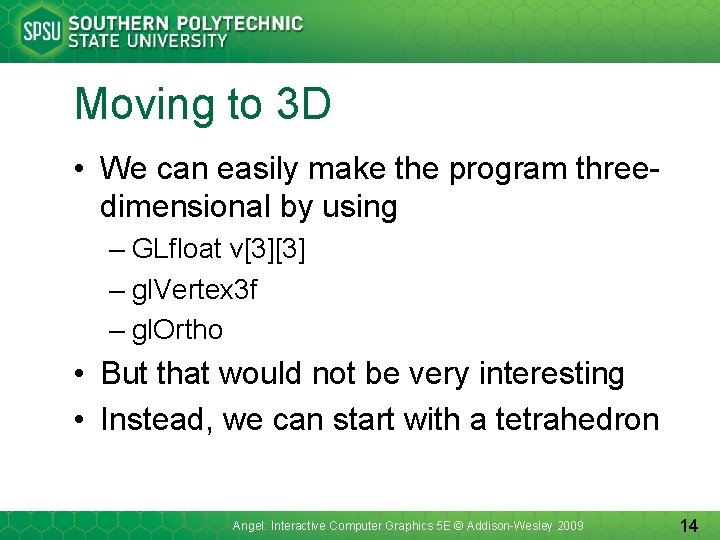
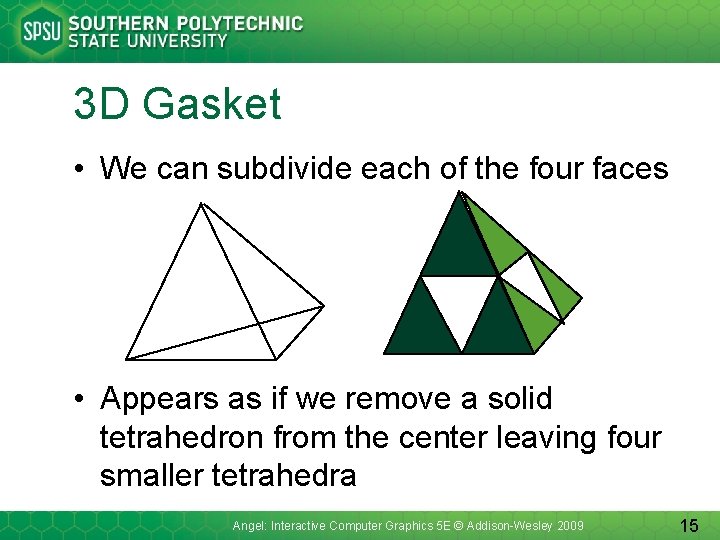
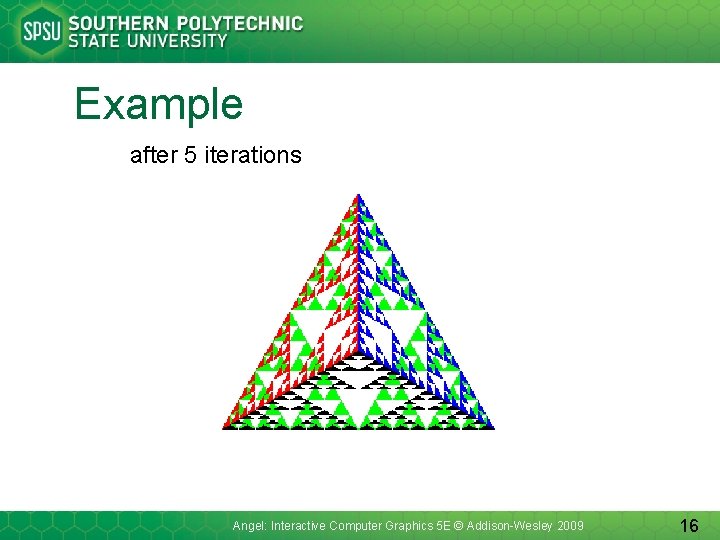
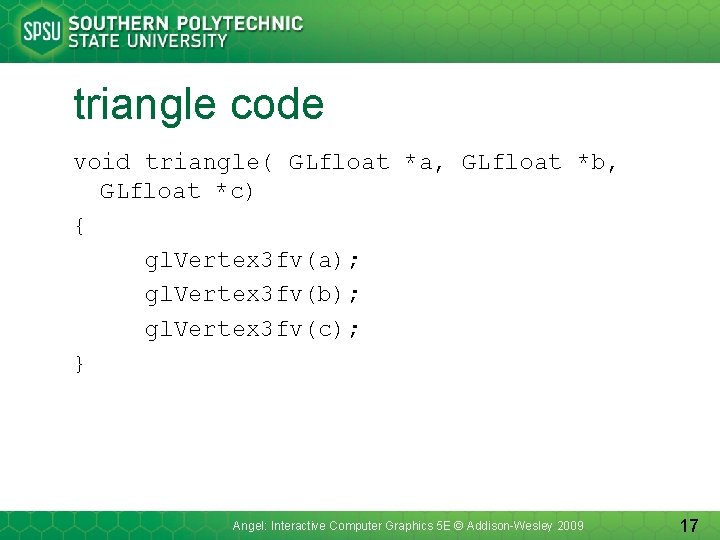
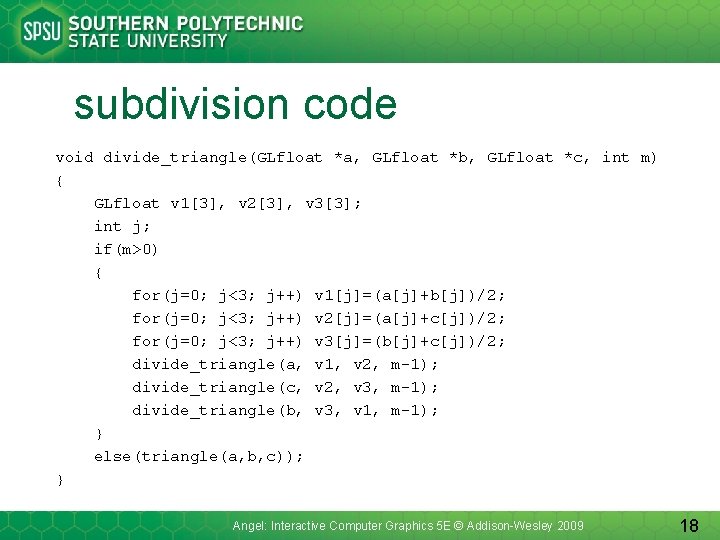
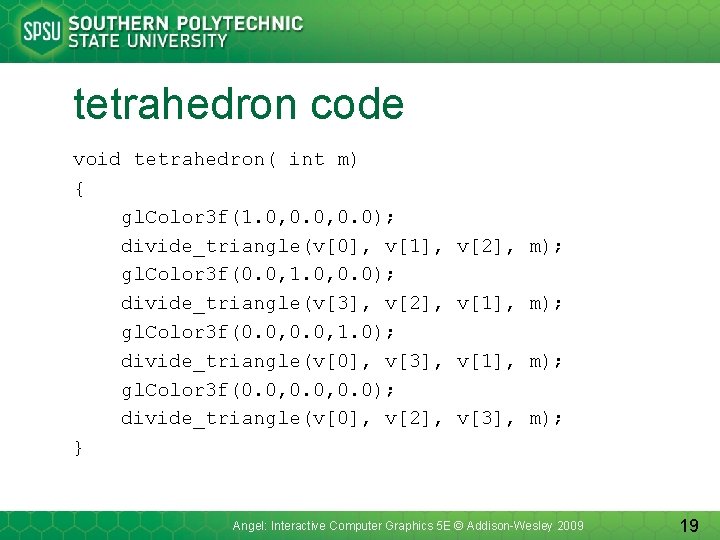
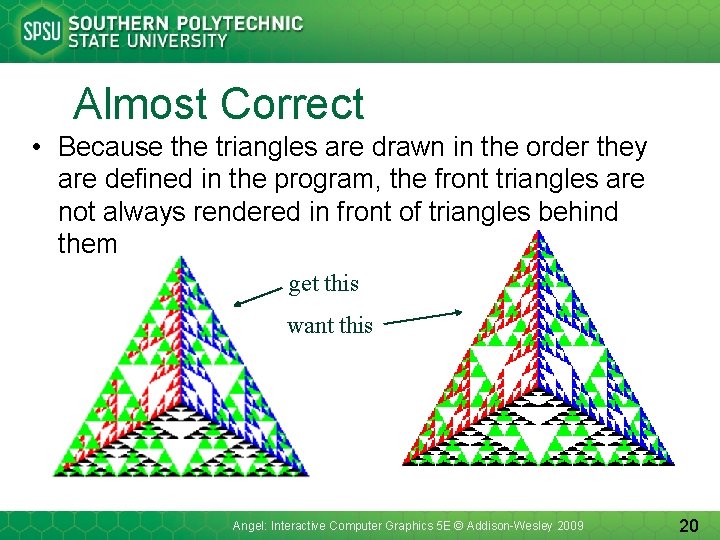
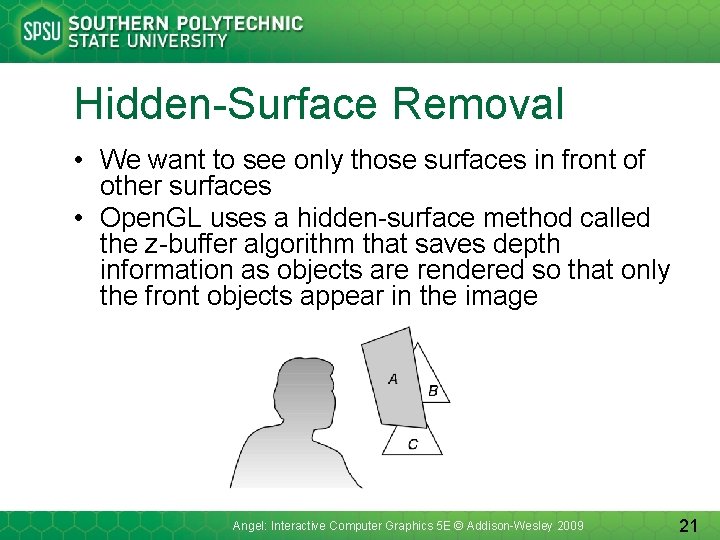
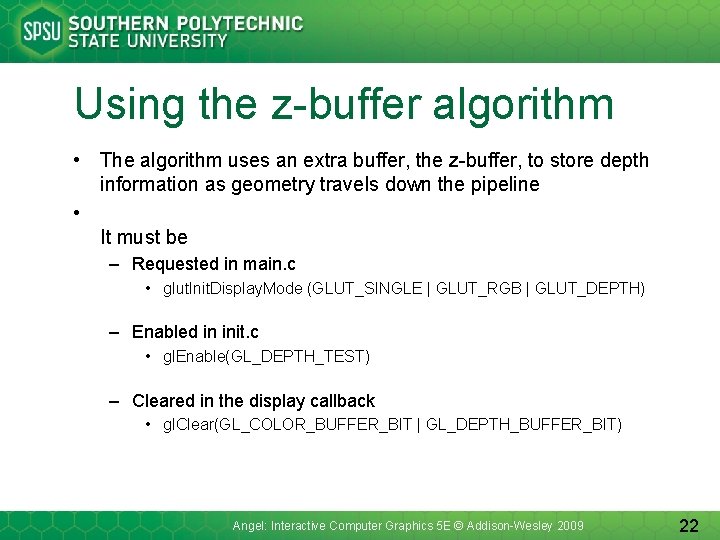
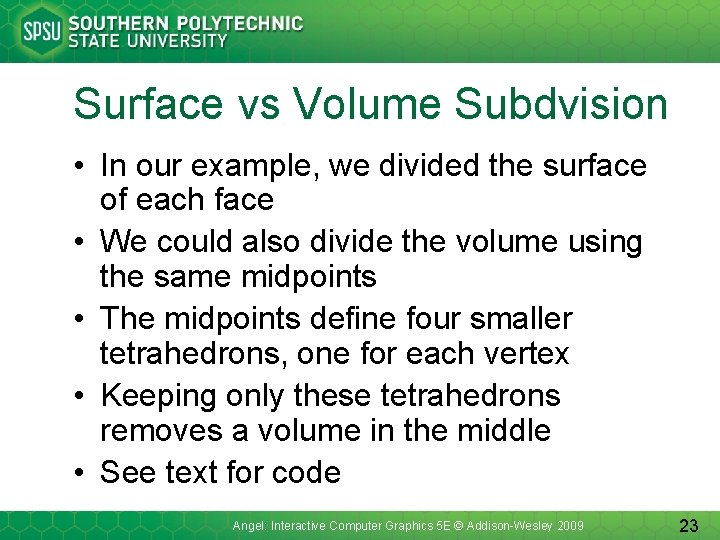
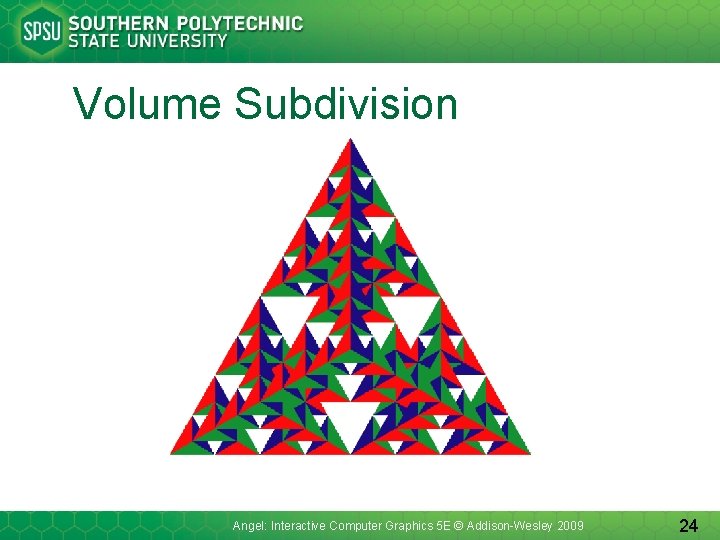
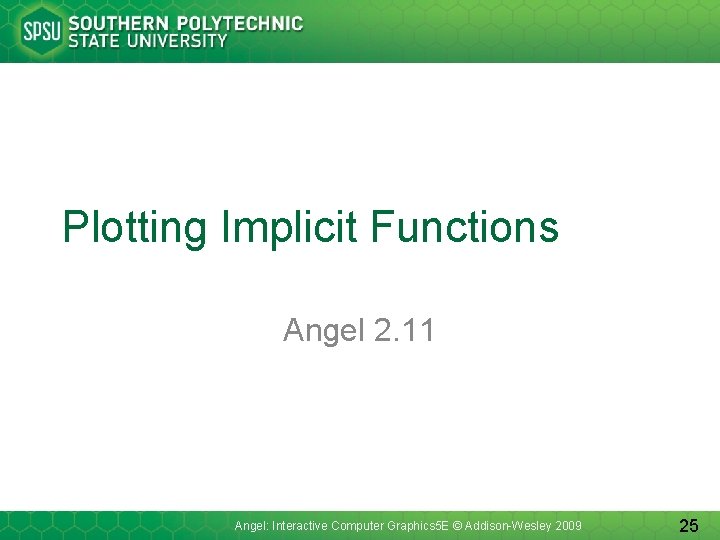
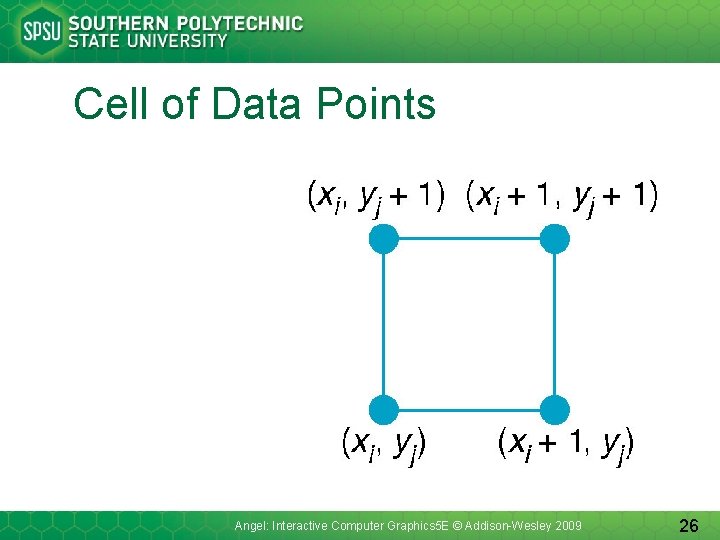
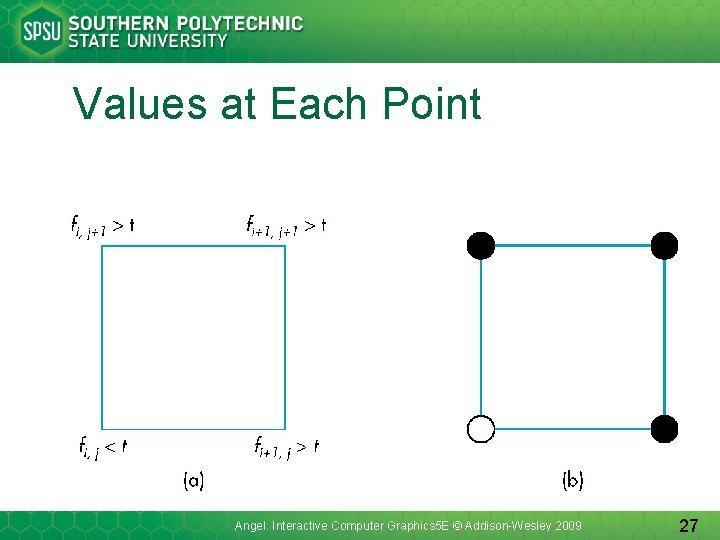
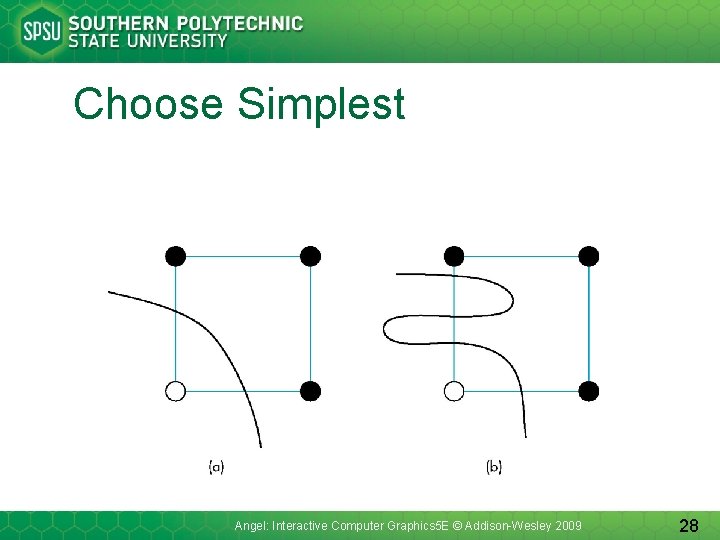
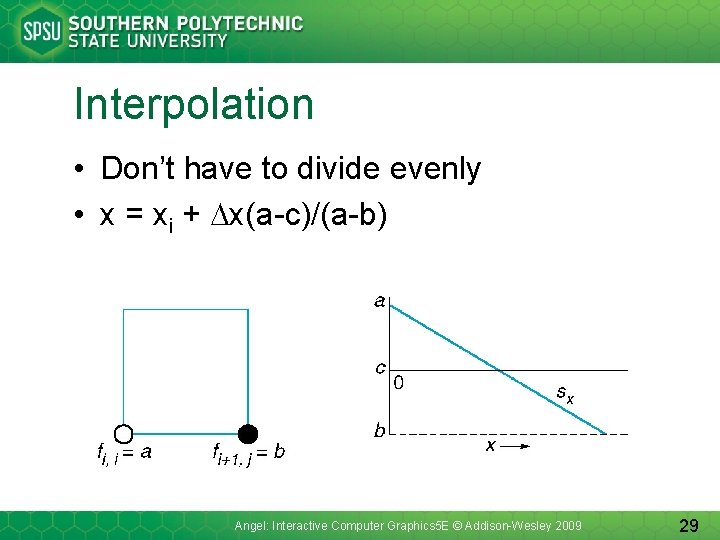
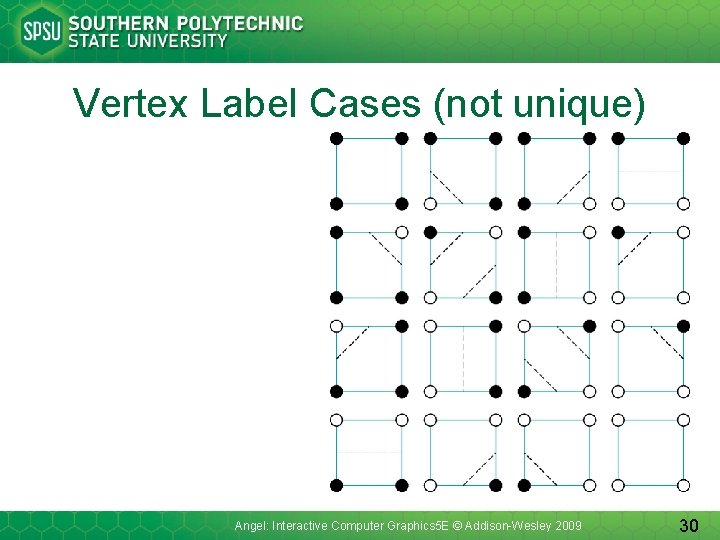
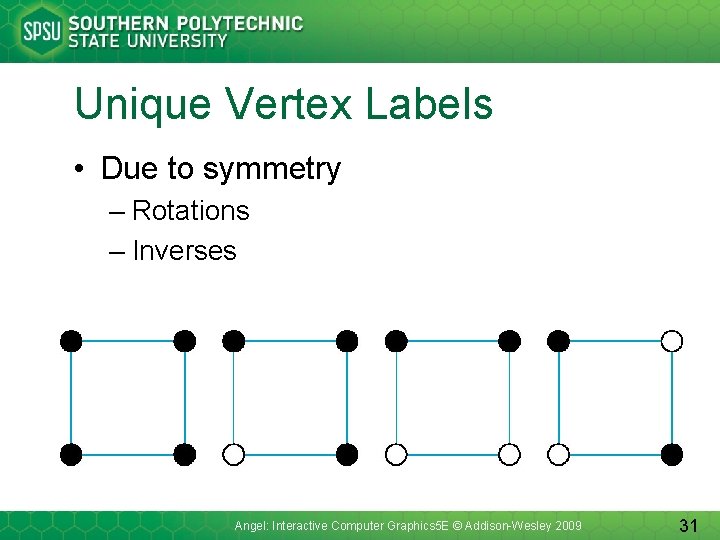
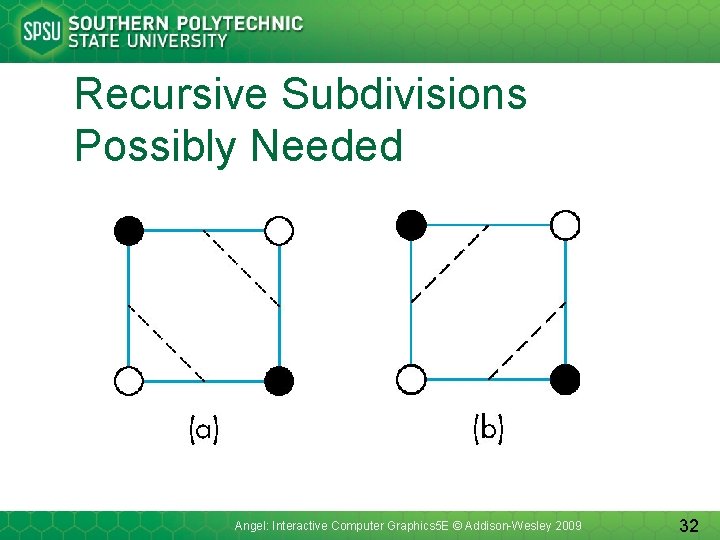
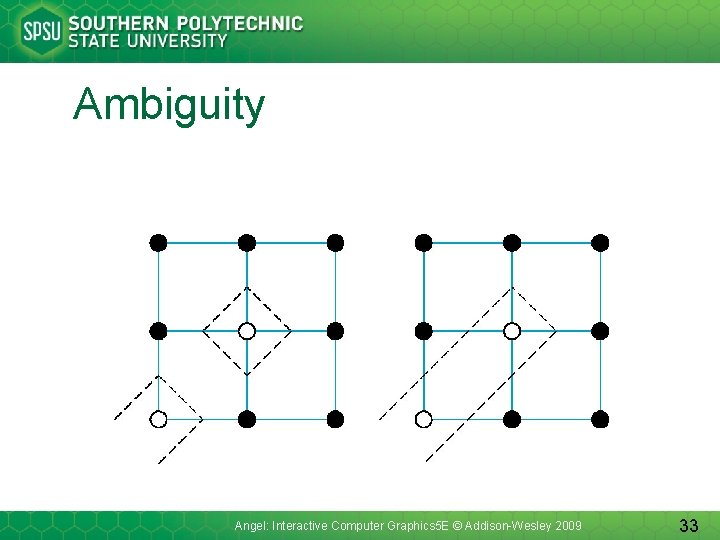
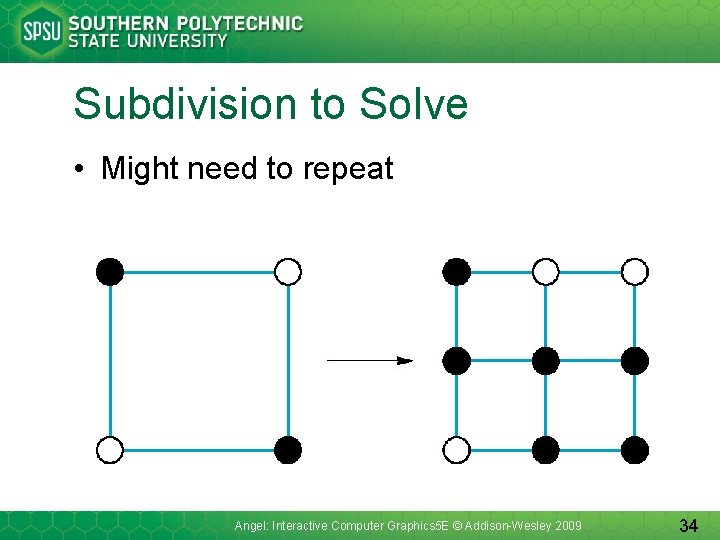
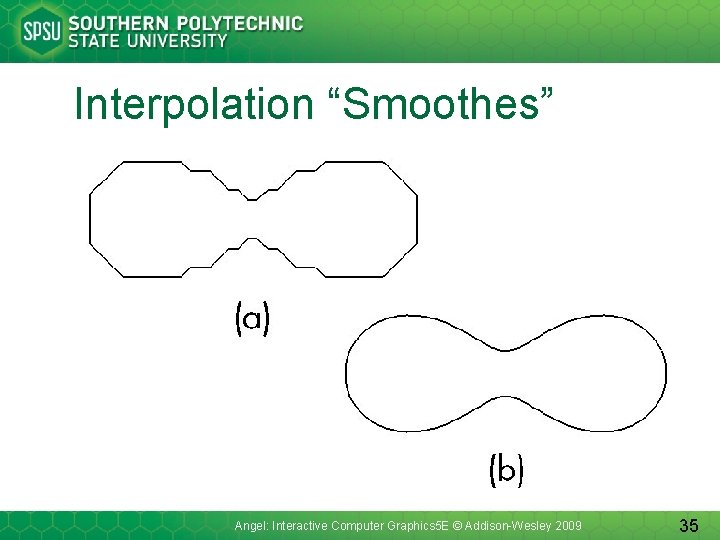
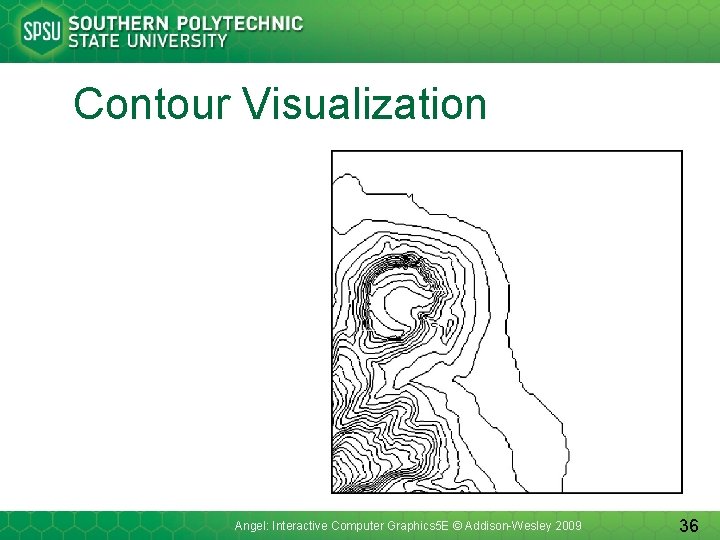
- Slides: 36
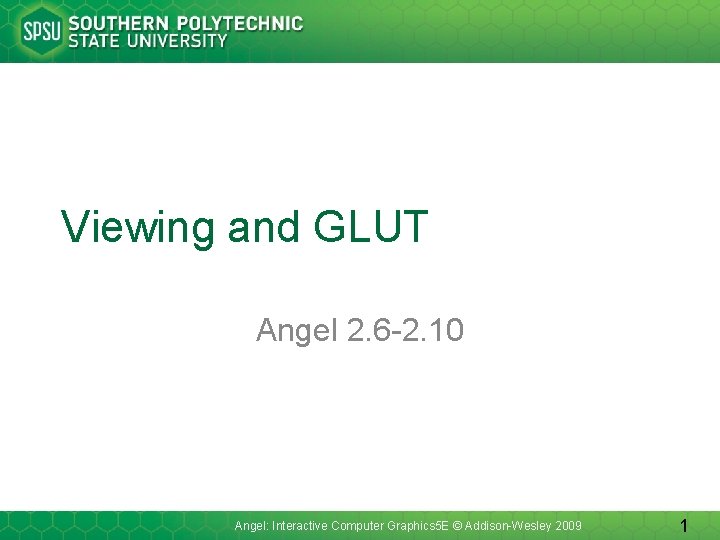
Viewing and GLUT Angel 2. 6 -2. 10 Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 1
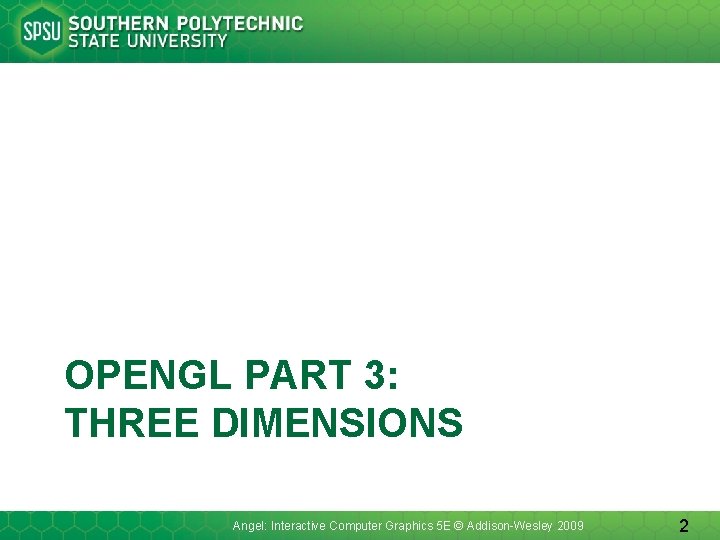
OPENGL PART 3: THREE DIMENSIONS Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 2
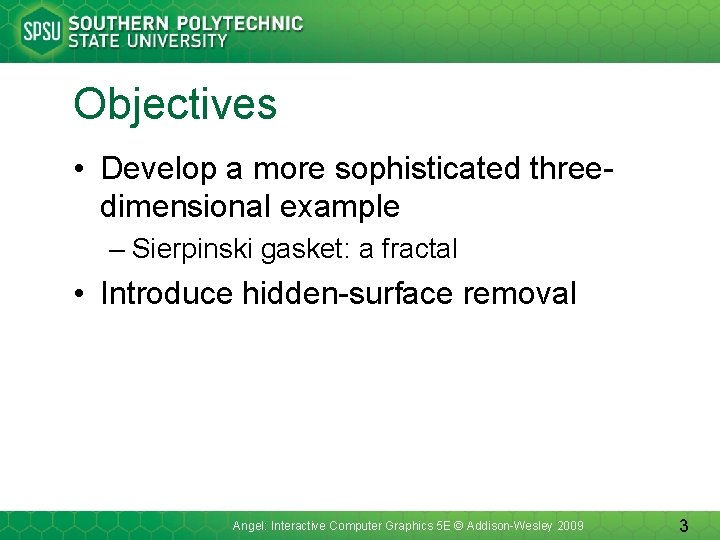
Objectives • Develop a more sophisticated threedimensional example – Sierpinski gasket: a fractal • Introduce hidden-surface removal Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 3
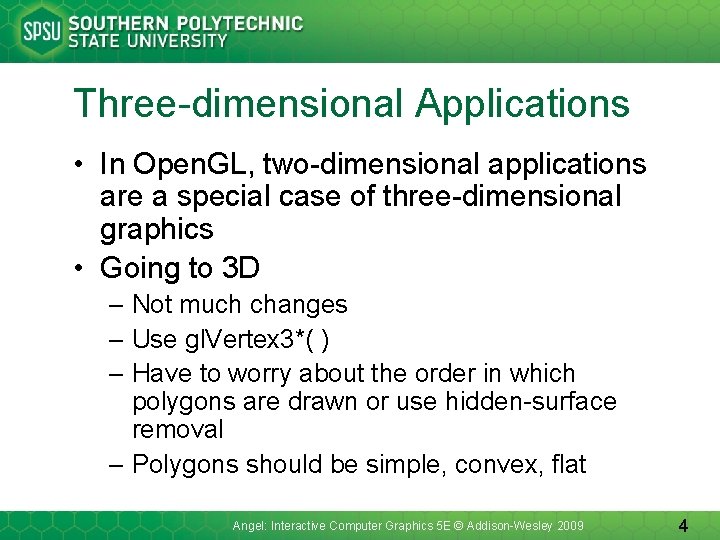
Three-dimensional Applications • In Open. GL, two-dimensional applications are a special case of three-dimensional graphics • Going to 3 D – Not much changes – Use gl. Vertex 3*( ) – Have to worry about the order in which polygons are drawn or use hidden-surface removal – Polygons should be simple, convex, flat Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 4
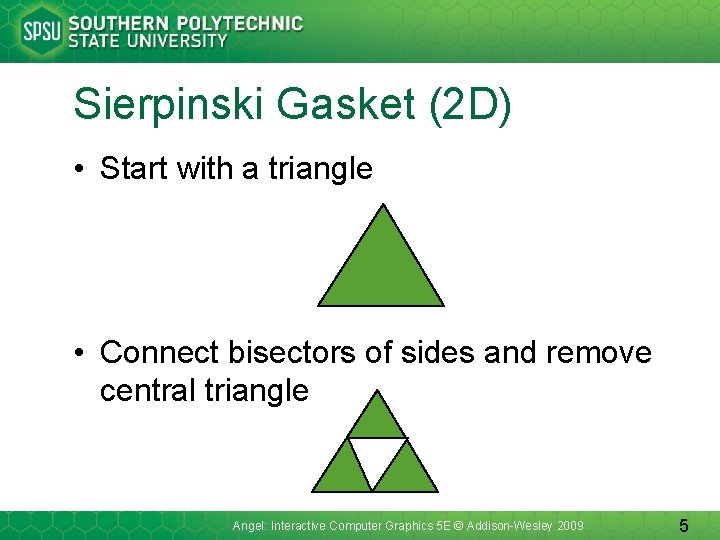
Sierpinski Gasket (2 D) • Start with a triangle • Connect bisectors of sides and remove central triangle Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 5
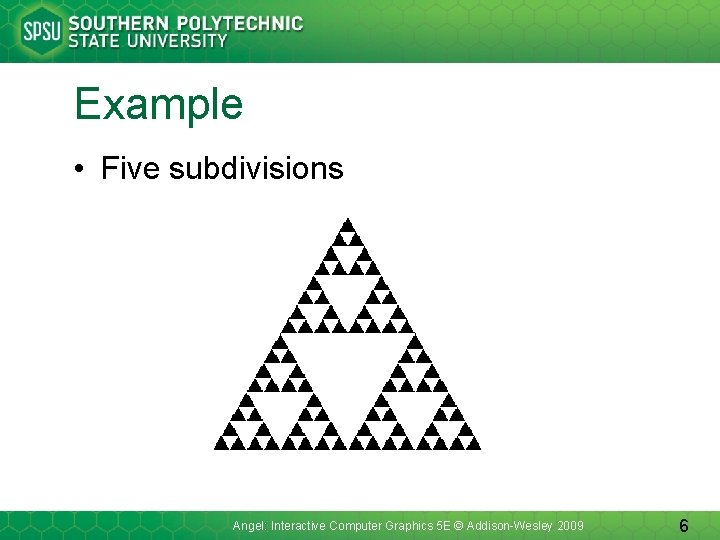
Example • Five subdivisions Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 6
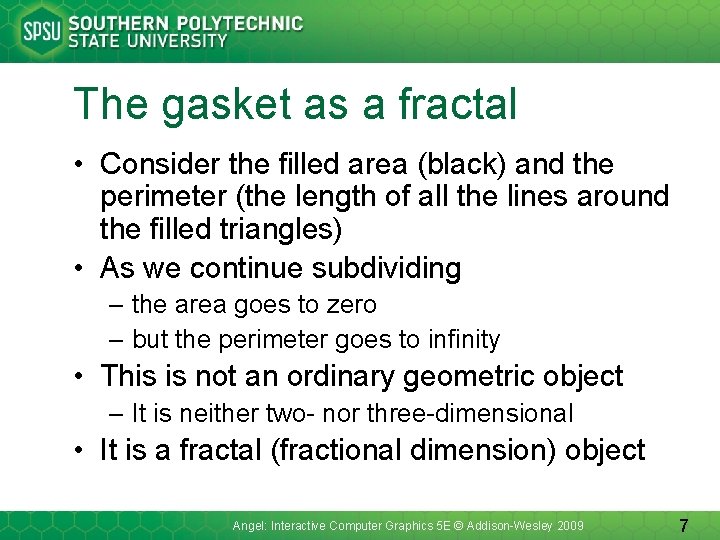
The gasket as a fractal • Consider the filled area (black) and the perimeter (the length of all the lines around the filled triangles) • As we continue subdividing – the area goes to zero – but the perimeter goes to infinity • This is not an ordinary geometric object – It is neither two- nor three-dimensional • It is a fractal (fractional dimension) object Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 7
![Gasket Program include GLglut h initial triangle GLfloat v321 0 0 58 Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58},](https://slidetodoc.com/presentation_image_h2/cb5f87b1a2583a1345228207a6c69945/image-8.jpg)
Gasket Program #include <GL/glut. h> /* initial triangle */ GLfloat v[3][2]={{-1. 0, -0. 58}, {0. 0, 1. 15}}; int n; /* number of recursive steps */ Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 8
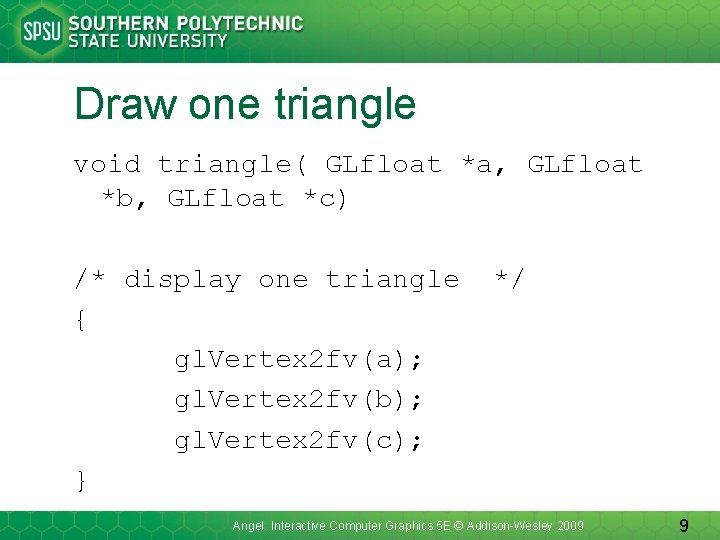
Draw one triangle void triangle( GLfloat *a, GLfloat *b, GLfloat *c) /* display one triangle { gl. Vertex 2 fv(a); gl. Vertex 2 fv(b); gl. Vertex 2 fv(c); } */ Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 9
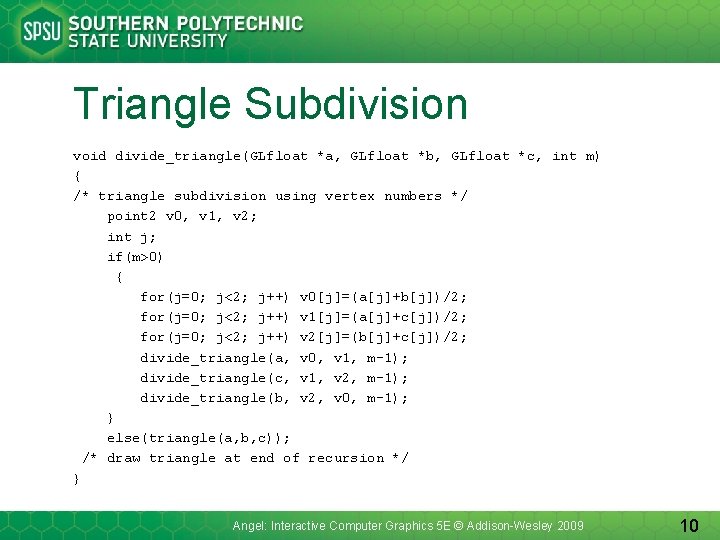
Triangle Subdivision void divide_triangle(GLfloat *a, GLfloat *b, GLfloat *c, int m) { /* triangle subdivision using vertex numbers */ point 2 v 0, v 1, v 2; int j; if(m>0) { for(j=0; j<2; j++) v 0[j]=(a[j]+b[j])/2; for(j=0; j<2; j++) v 1[j]=(a[j]+c[j])/2; for(j=0; j<2; j++) v 2[j]=(b[j]+c[j])/2; divide_triangle(a, v 0, v 1, m-1); divide_triangle(c, v 1, v 2, m-1); divide_triangle(b, v 2, v 0, m-1); } else(triangle(a, b, c)); /* draw triangle at end of recursion */ } Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 10
![display and init Functions void display gl ClearGLCOLORBUFFERBIT gl BeginGLTRIANGLES dividetrianglev0 v1 v2 display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2],](https://slidetodoc.com/presentation_image_h2/cb5f87b1a2583a1345228207a6c69945/image-11.jpg)
display and init Functions void display() { gl. Clear(GL_COLOR_BUFFER_BIT); gl. Begin(GL_TRIANGLES); divide_triangle(v[0], v[1], v[2], n); gl. End(); gl. Flush(); } void myinit() { gl. Matrix. Mode(GL_PROJECTION); gl. Load. Identity(); glu. Ortho 2 D(-2. 0, 2. 0); gl. Matrix. Mode(GL_MODELVIEW); gl. Clear. Color (1. 0, 1. 0) gl. Color 3 f(0. 0, 0. 0); } Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 11
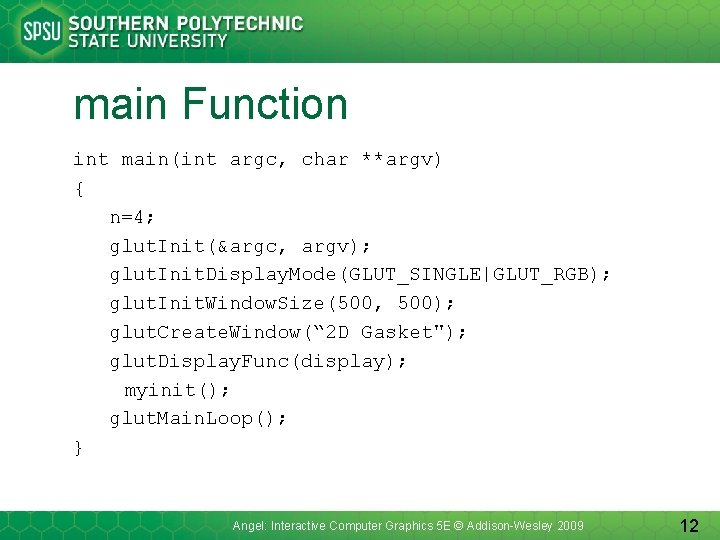
main Function int main(int argc, char **argv) { n=4; glut. Init(&argc, argv); glut. Init. Display. Mode(GLUT_SINGLE|GLUT_RGB); glut. Init. Window. Size(500, 500); glut. Create. Window(“ 2 D Gasket"); glut. Display. Func(display); myinit(); glut. Main. Loop(); } Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 12
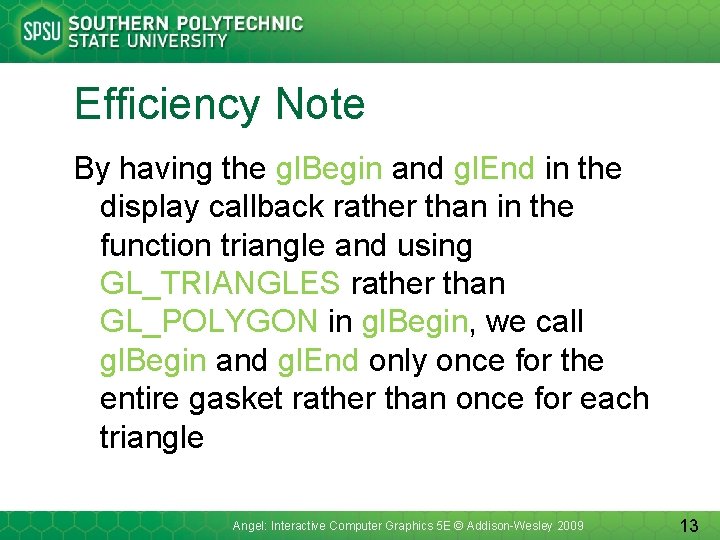
Efficiency Note By having the gl. Begin and gl. End in the display callback rather than in the function triangle and using GL_TRIANGLES rather than GL_POLYGON in gl. Begin, we call gl. Begin and gl. End only once for the entire gasket rather than once for each triangle Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 13
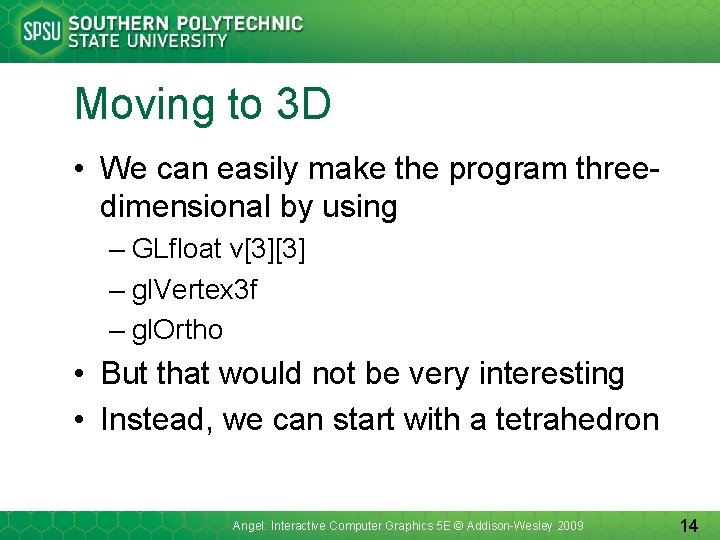
Moving to 3 D • We can easily make the program threedimensional by using – GLfloat v[3][3] – gl. Vertex 3 f – gl. Ortho • But that would not be very interesting • Instead, we can start with a tetrahedron Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 14
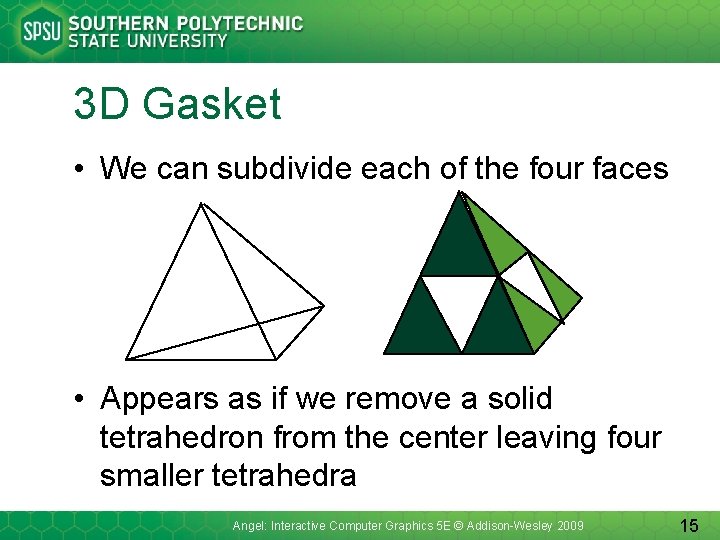
3 D Gasket • We can subdivide each of the four faces • Appears as if we remove a solid tetrahedron from the center leaving four smaller tetrahedra Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 15
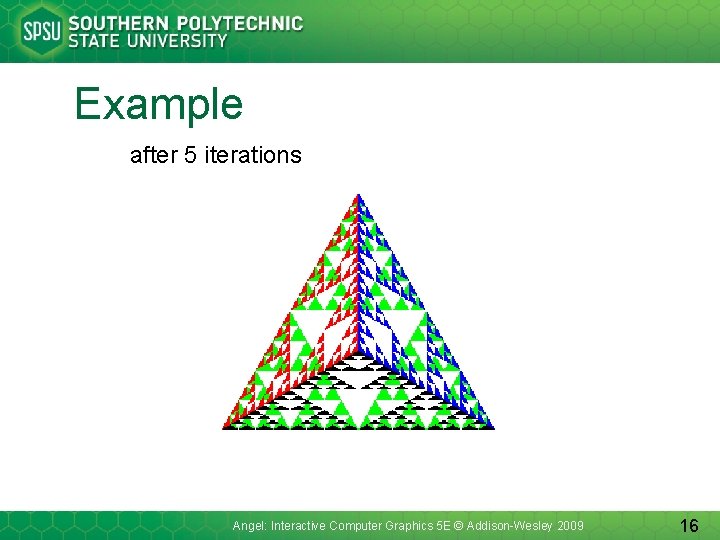
Example after 5 iterations Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 16
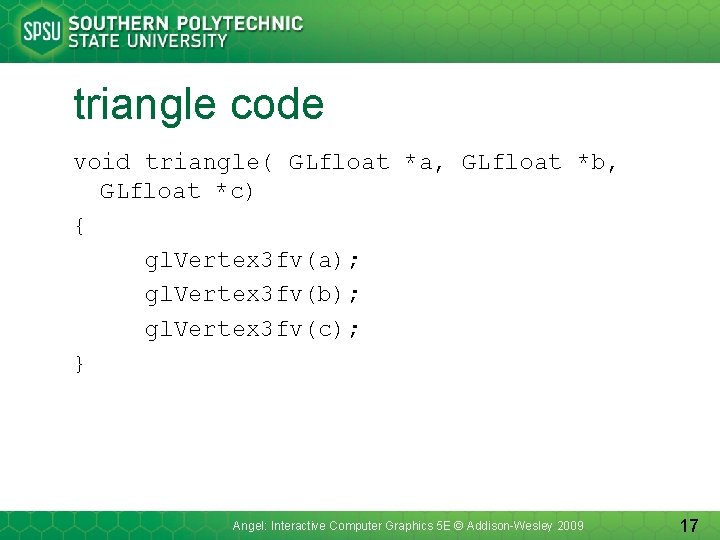
triangle code void triangle( GLfloat *a, GLfloat *b, GLfloat *c) { gl. Vertex 3 fv(a); gl. Vertex 3 fv(b); gl. Vertex 3 fv(c); } Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 17
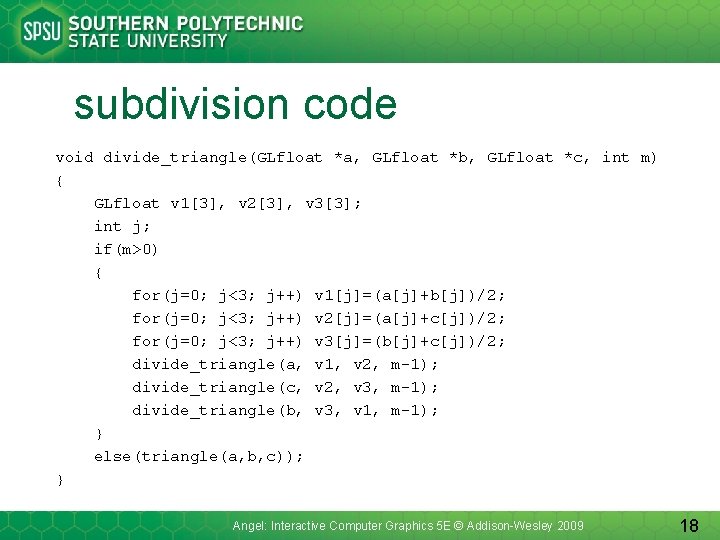
subdivision code void divide_triangle(GLfloat *a, GLfloat *b, GLfloat *c, int m) { GLfloat v 1[3], v 2[3], v 3[3]; int j; if(m>0) { for(j=0; j<3; j++) v 1[j]=(a[j]+b[j])/2; for(j=0; j<3; j++) v 2[j]=(a[j]+c[j])/2; for(j=0; j<3; j++) v 3[j]=(b[j]+c[j])/2; divide_triangle(a, v 1, v 2, m-1); divide_triangle(c, v 2, v 3, m-1); divide_triangle(b, v 3, v 1, m-1); } else(triangle(a, b, c)); } Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 18
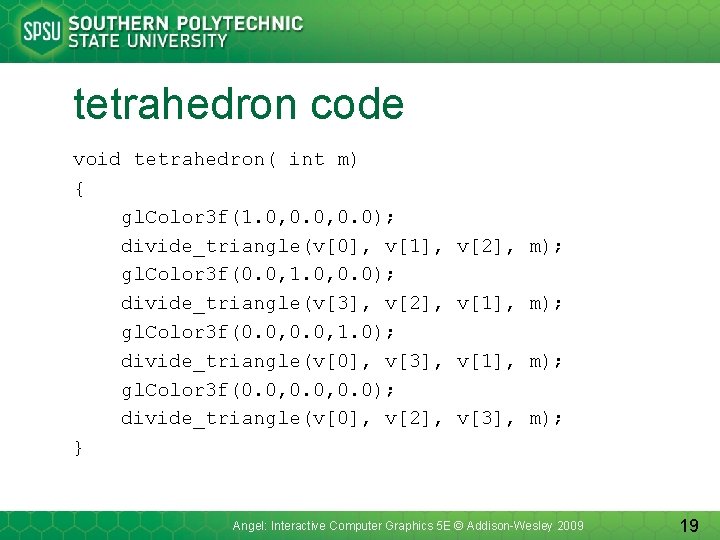
tetrahedron code void tetrahedron( int m) { gl. Color 3 f(1. 0, 0. 0); divide_triangle(v[0], v[1], gl. Color 3 f(0. 0, 1. 0, 0. 0); divide_triangle(v[3], v[2], gl. Color 3 f(0. 0, 1. 0); divide_triangle(v[0], v[3], gl. Color 3 f(0. 0, 0. 0); divide_triangle(v[0], v[2], } v[2], m); v[1], m); v[3], m); Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 19
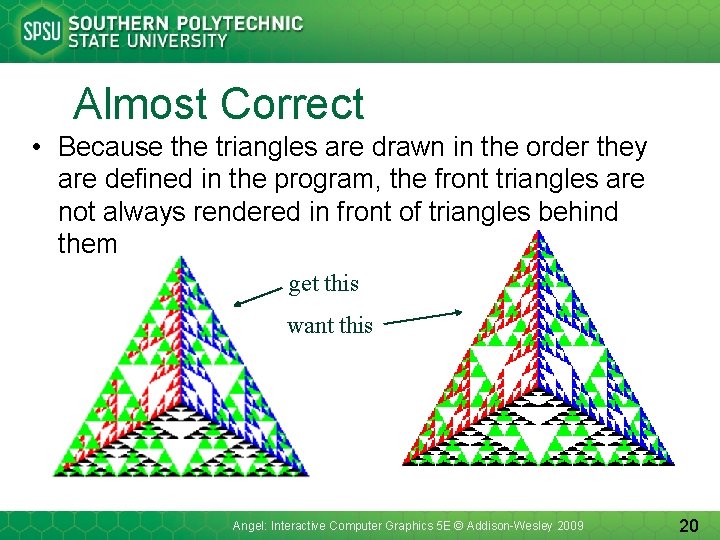
Almost Correct • Because the triangles are drawn in the order they are defined in the program, the front triangles are not always rendered in front of triangles behind them get this want this Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 20
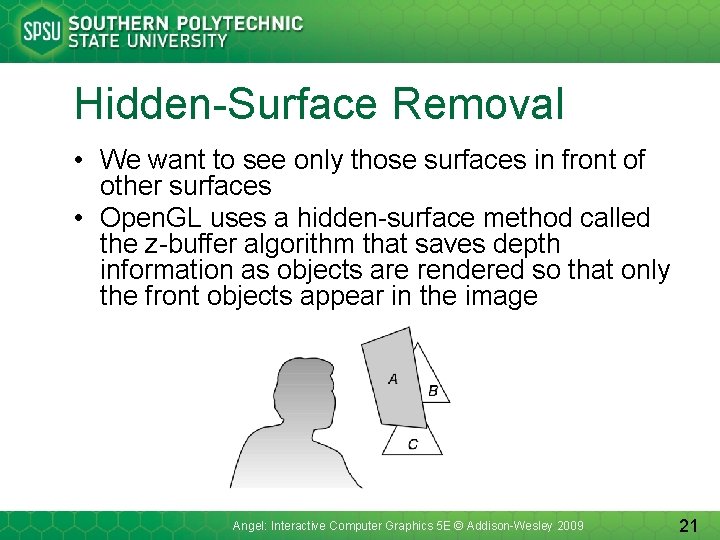
Hidden-Surface Removal • We want to see only those surfaces in front of other surfaces • Open. GL uses a hidden-surface method called the z-buffer algorithm that saves depth information as objects are rendered so that only the front objects appear in the image Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 21
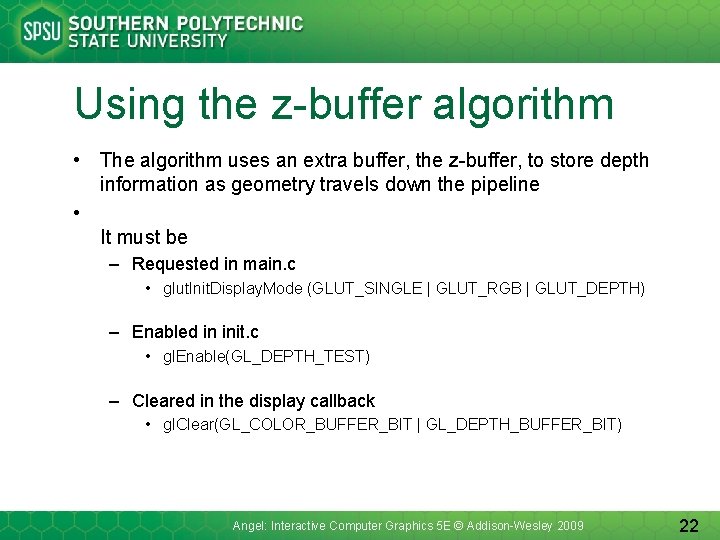
Using the z-buffer algorithm • The algorithm uses an extra buffer, the z-buffer, to store depth information as geometry travels down the pipeline • It must be – Requested in main. c • glut. Init. Display. Mode (GLUT_SINGLE | GLUT_RGB | GLUT_DEPTH) – Enabled in init. c • gl. Enable(GL_DEPTH_TEST) – Cleared in the display callback • gl. Clear(GL_COLOR_BUFFER_BIT | GL_DEPTH_BUFFER_BIT) Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 22
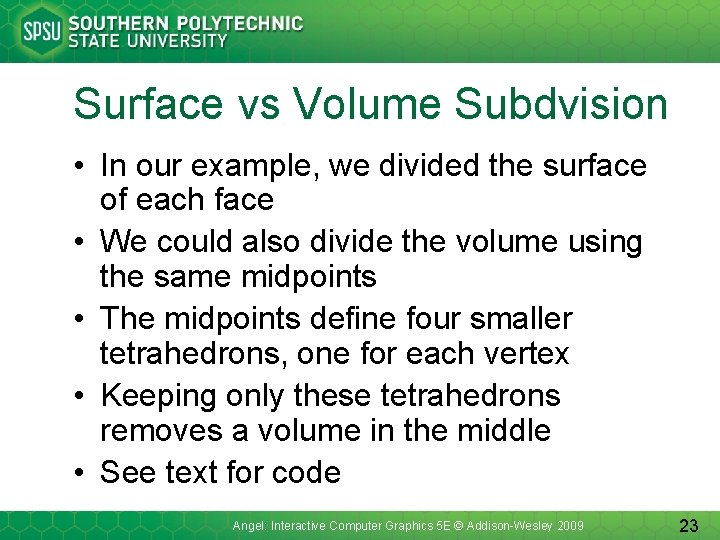
Surface vs Volume Subdvision • In our example, we divided the surface of each face • We could also divide the volume using the same midpoints • The midpoints define four smaller tetrahedrons, one for each vertex • Keeping only these tetrahedrons removes a volume in the middle • See text for code Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 23
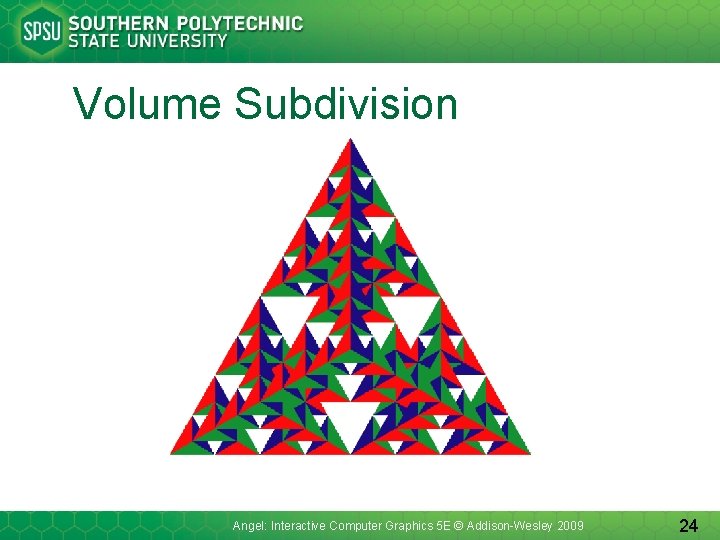
Volume Subdivision Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 24
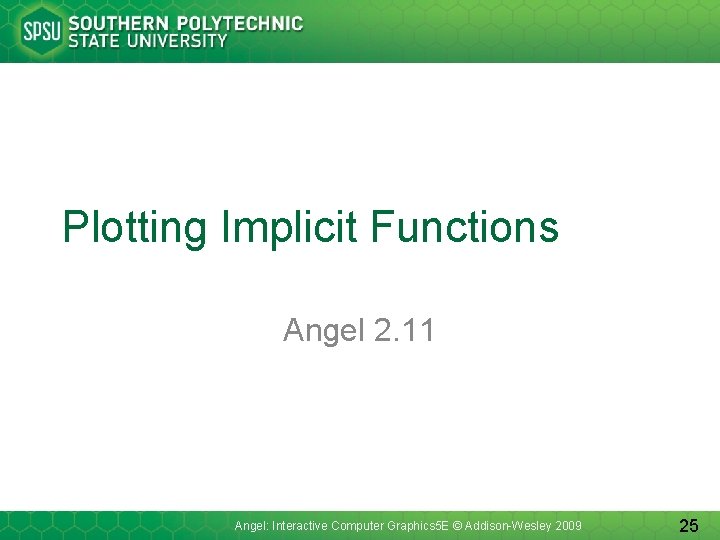
Plotting Implicit Functions Angel 2. 11 Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 25
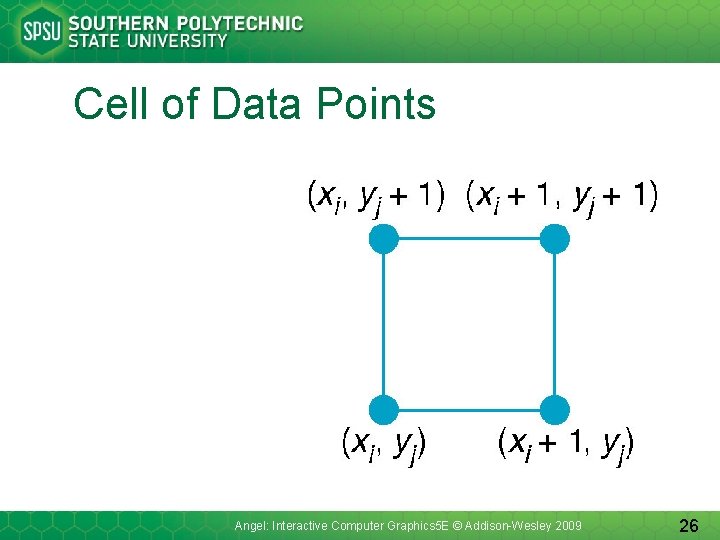
Cell of Data Points Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 26
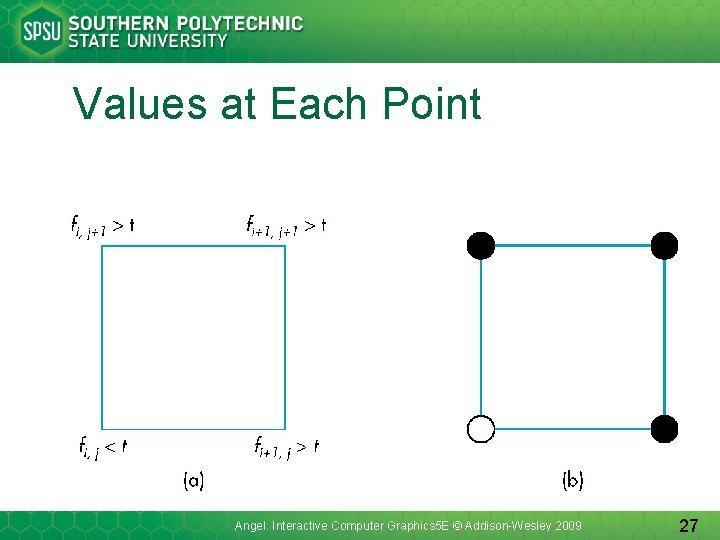
Values at Each Point Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 27
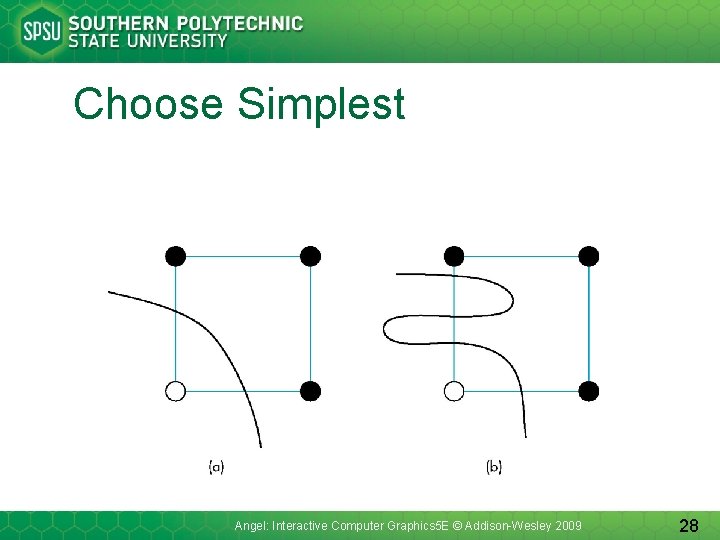
Choose Simplest Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 28
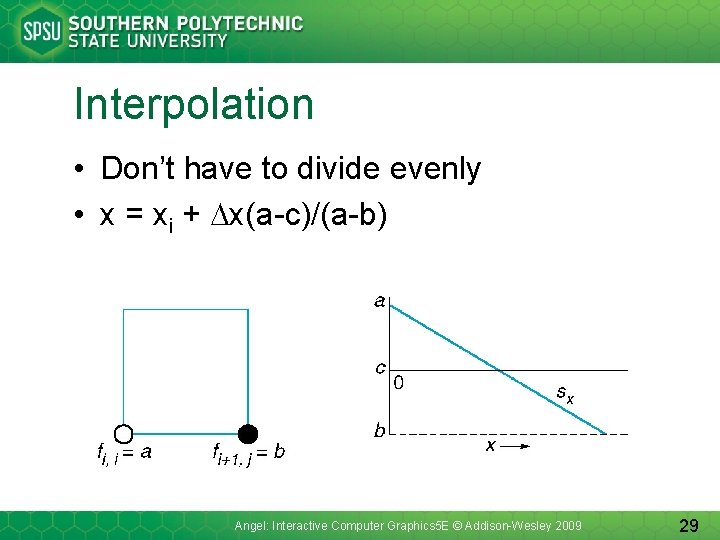
Interpolation • Don’t have to divide evenly • x = xi + x(a-c)/(a-b) Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 29
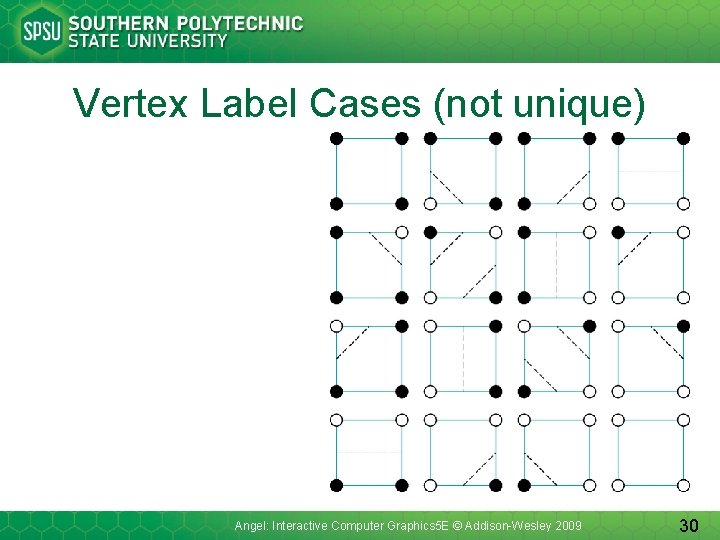
Vertex Label Cases (not unique) Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 30
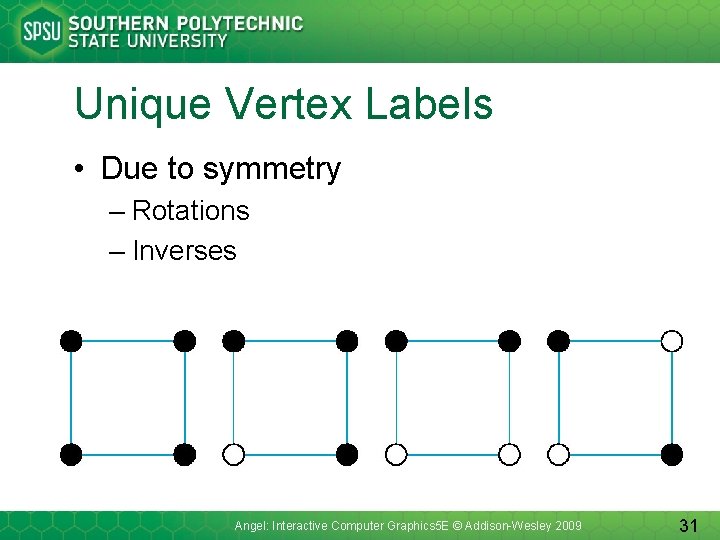
Unique Vertex Labels • Due to symmetry – Rotations – Inverses Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 31
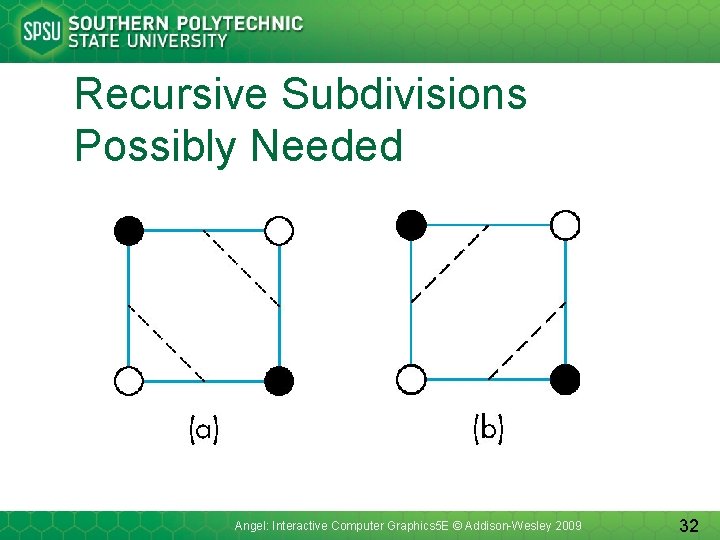
Recursive Subdivisions Possibly Needed Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 32
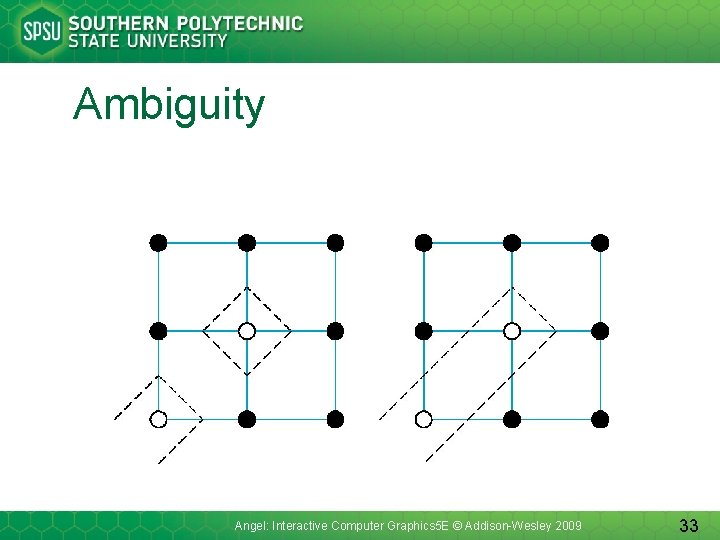
Ambiguity Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 33
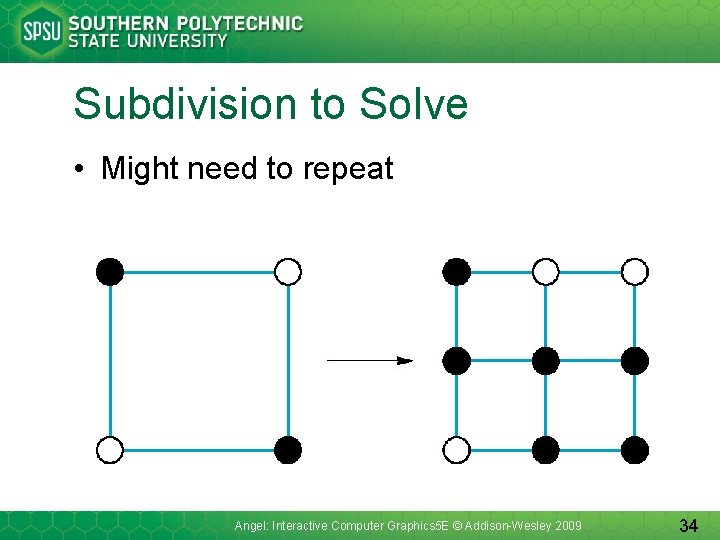
Subdivision to Solve • Might need to repeat Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 34
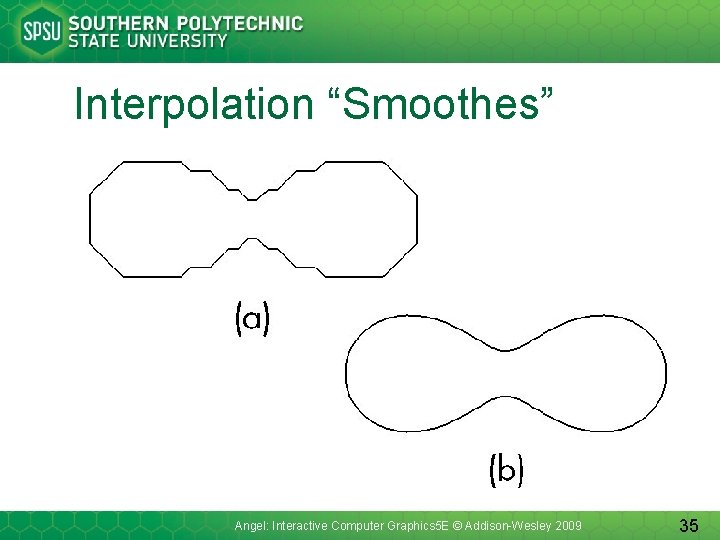
Interpolation “Smoothes” Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 35
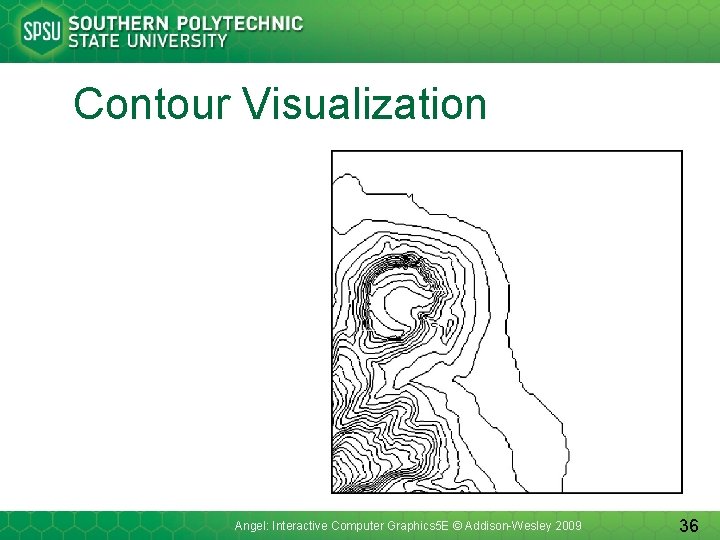
Contour Visualization Angel: Interactive Computer Graphics 5 E © Addison-Wesley 2009 36
Viewing angel
Claim of cause examples
Sectional drawing examples
Is film a text
Occupational therapy assessments for low vision
Slit diffraction
Thank you for viewing my presentation
Steps in viewing
Konsep viewing 3d adalah
Define viewing pipeline
Viewing coordinate sering juga disebut
Module 19 visual organization and interpretation
Constant intensity shading
Types of projection in computer graphics
Lcd working principle ppt
What is window and viewport in computer graphics
Exterior clipping
What process occurs
The ipde process is an organized system of
Install glut
Managing customer information to gain customer insights
G;4g6
Glut solid cone
Glut
Glut display function
Glut draw text
Glut keyboard
Glut objects
Protezoik
Kata polyhedral bersal dari kata?
Opengl.com
Display device
Glutwire
Glut init
Glut is ours
Gl_line_loop example
¿qué es la homeostasis y ejemplos