Introduction to Computer Graphics with Open GLGLUT What
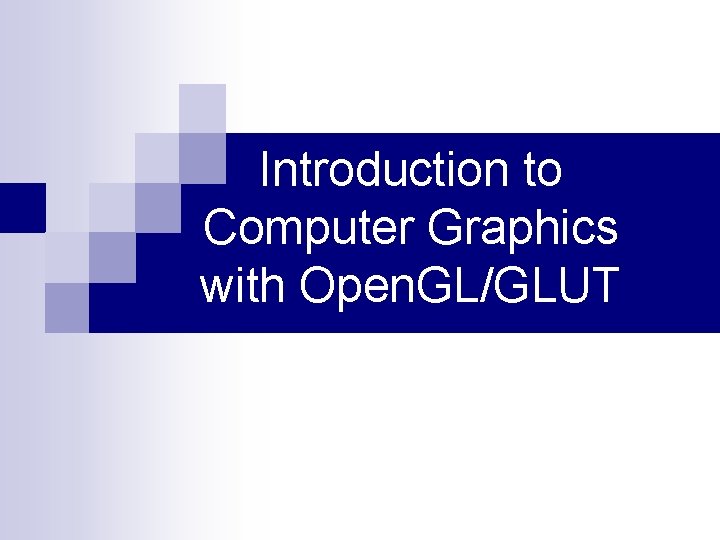
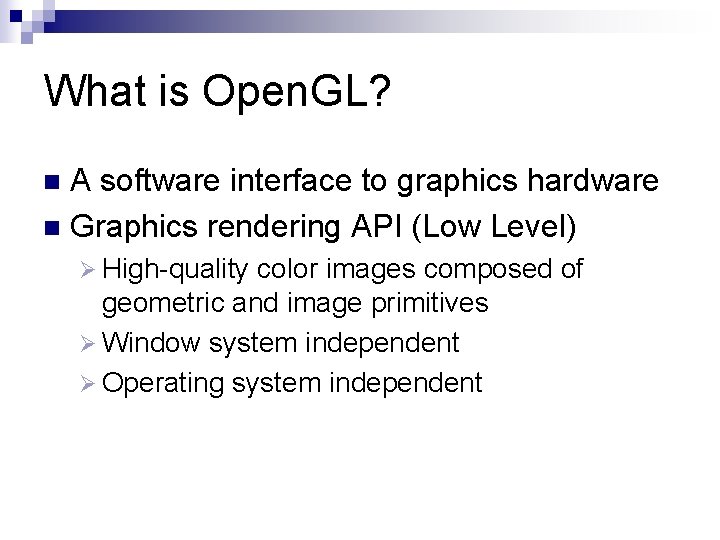
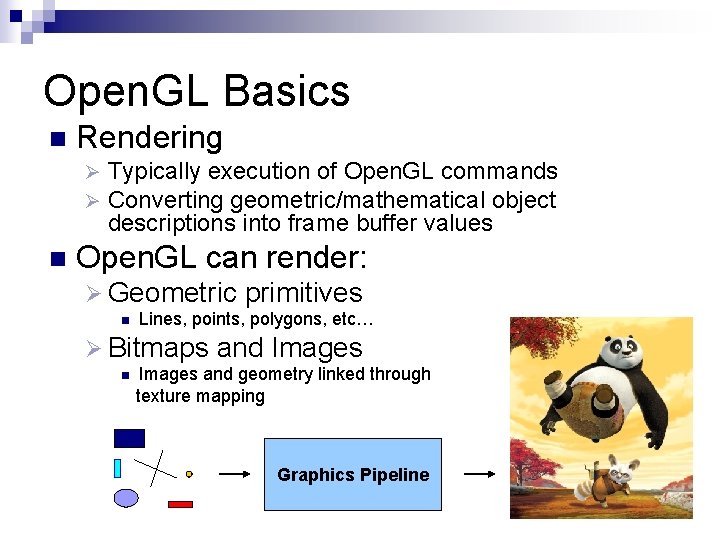
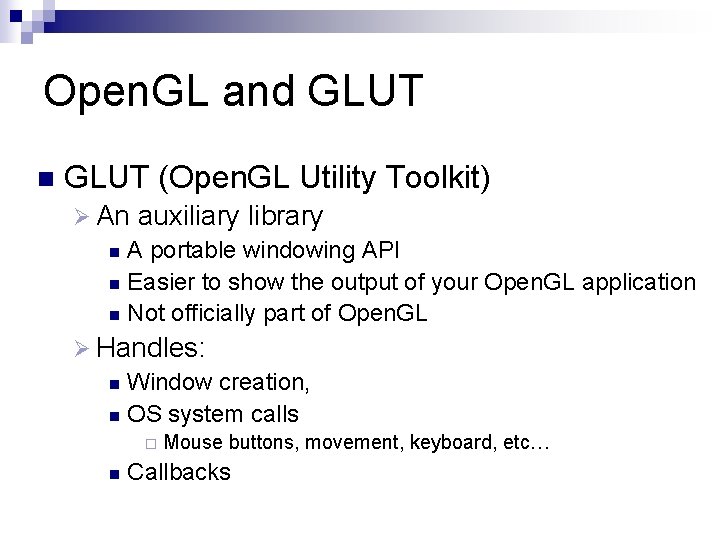
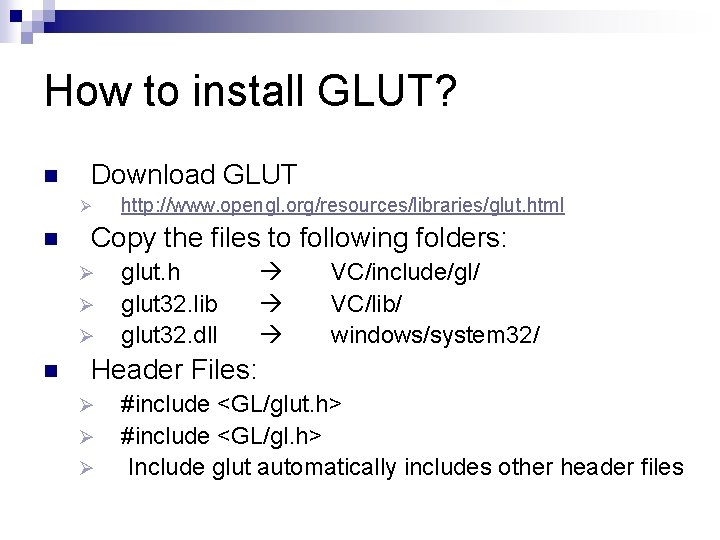
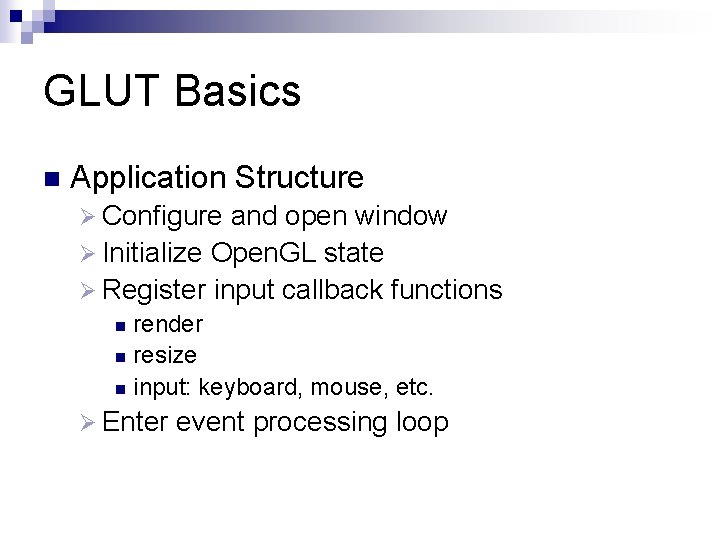
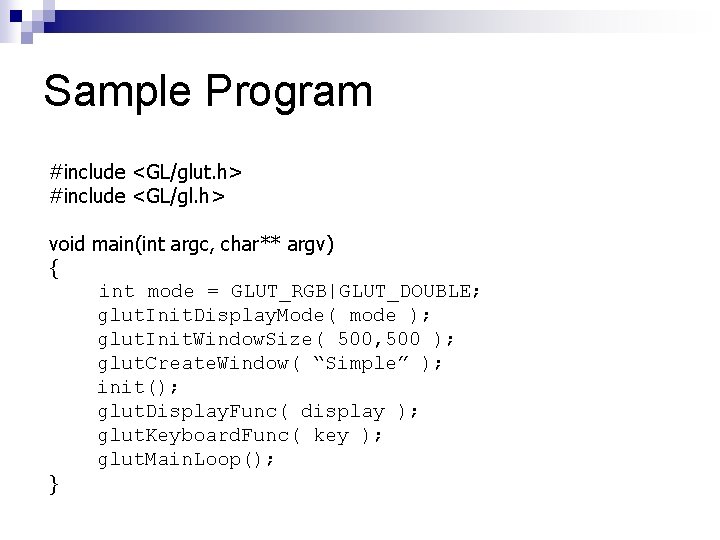
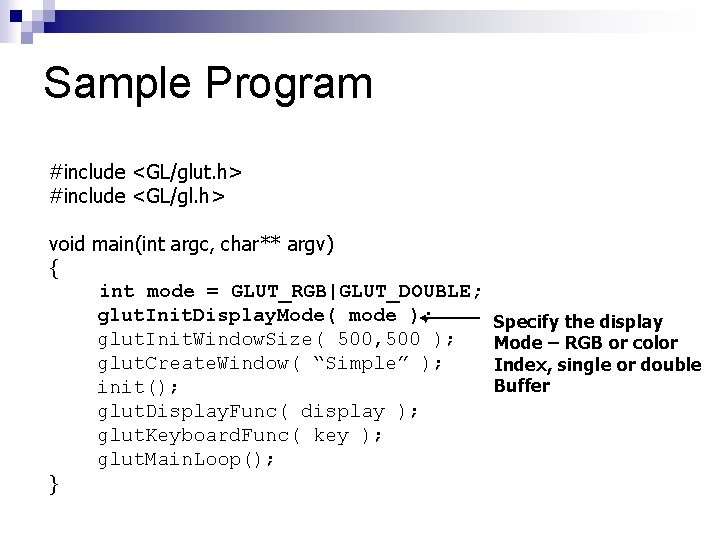
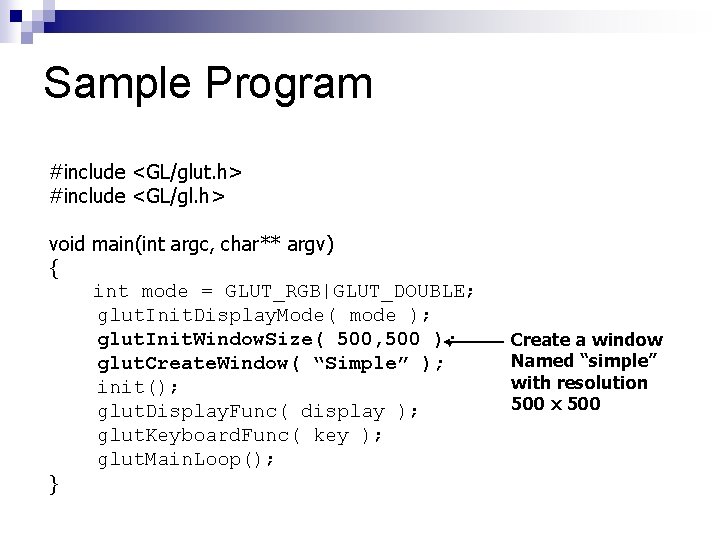
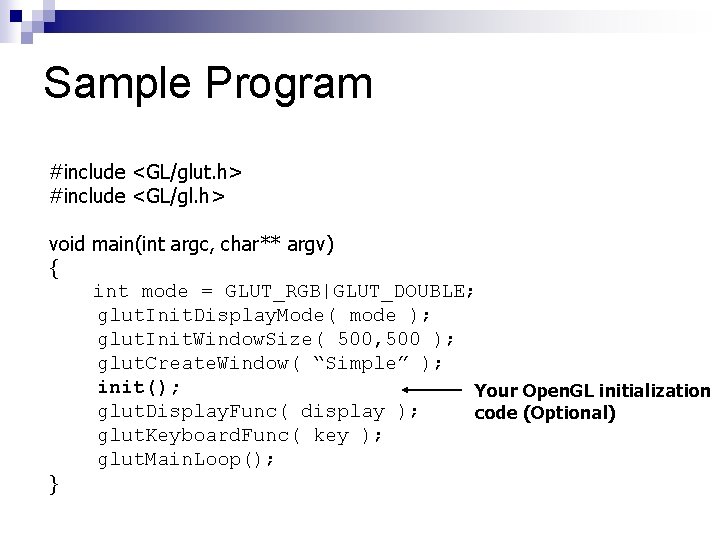
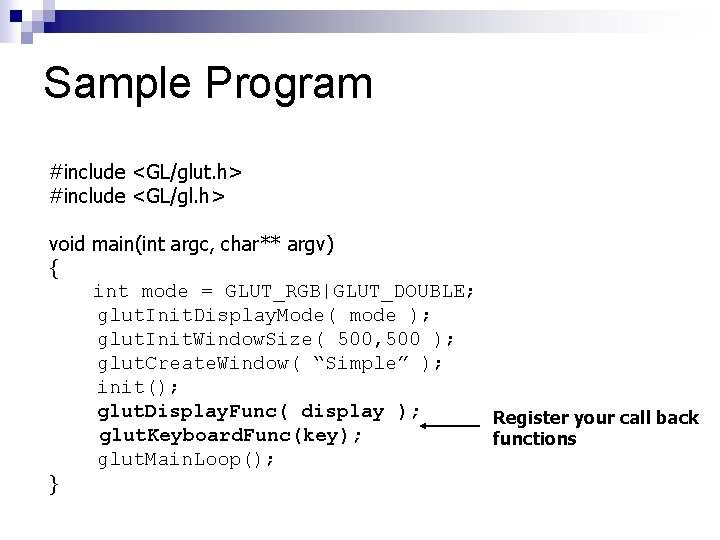
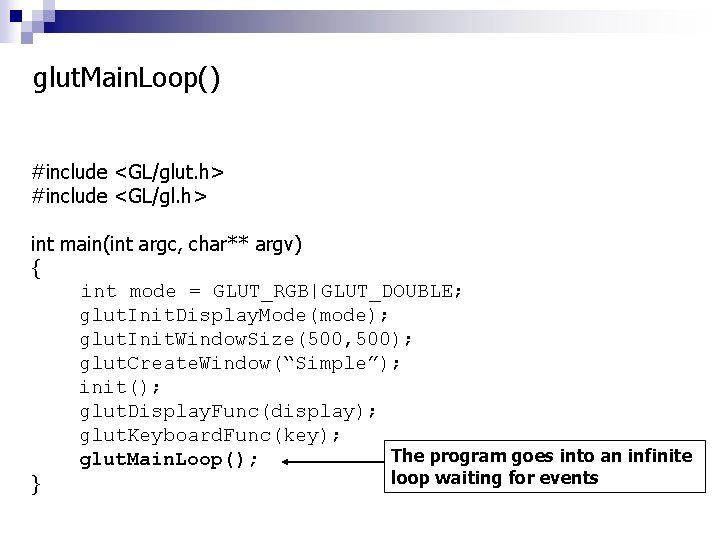
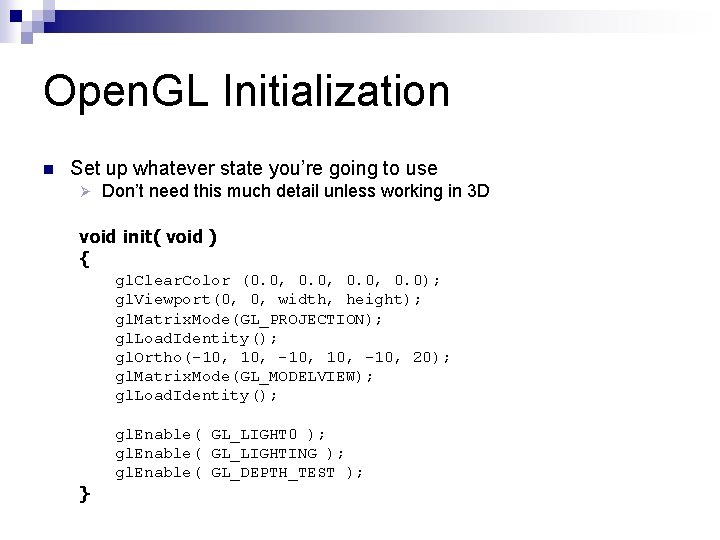
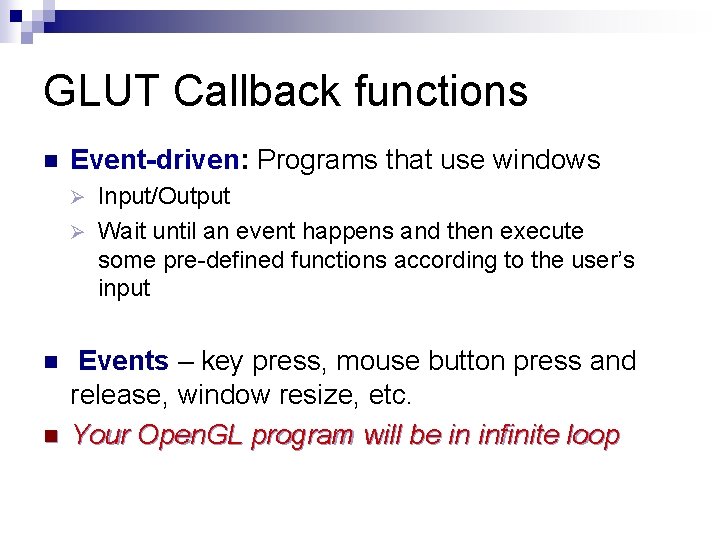
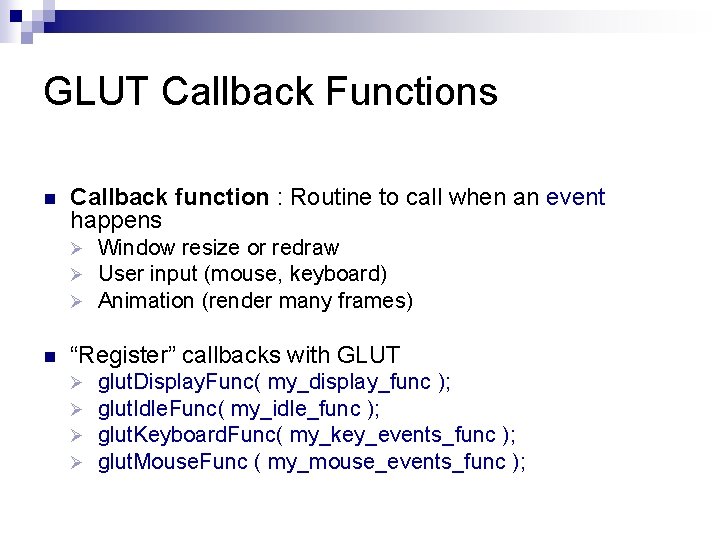
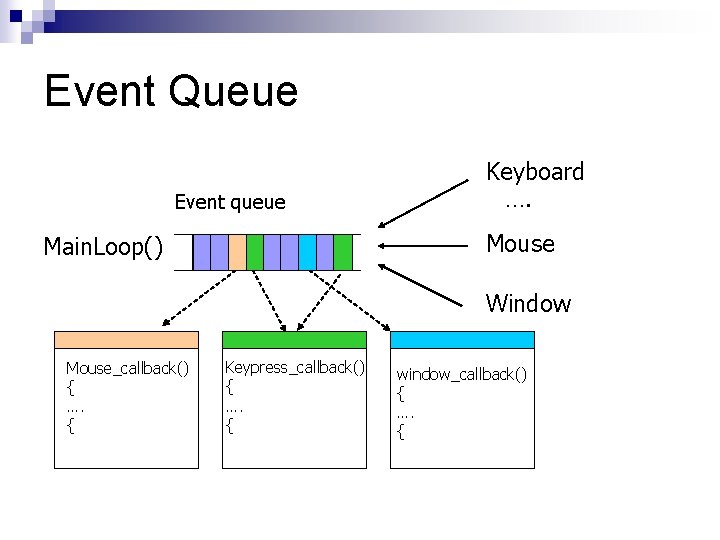
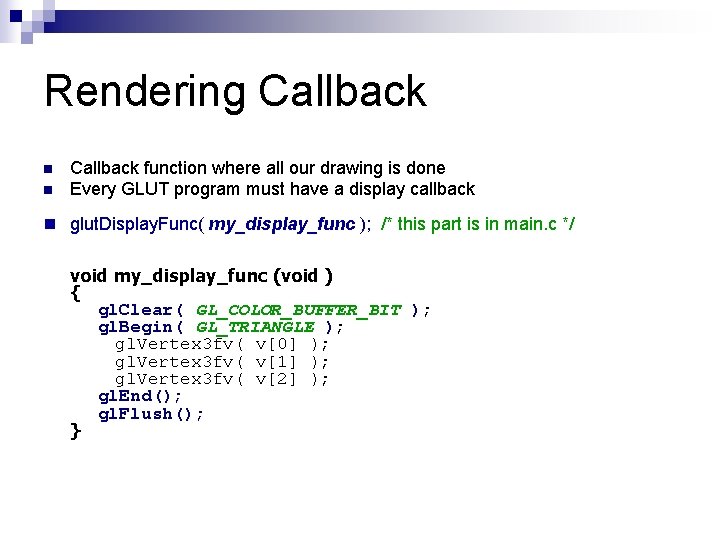
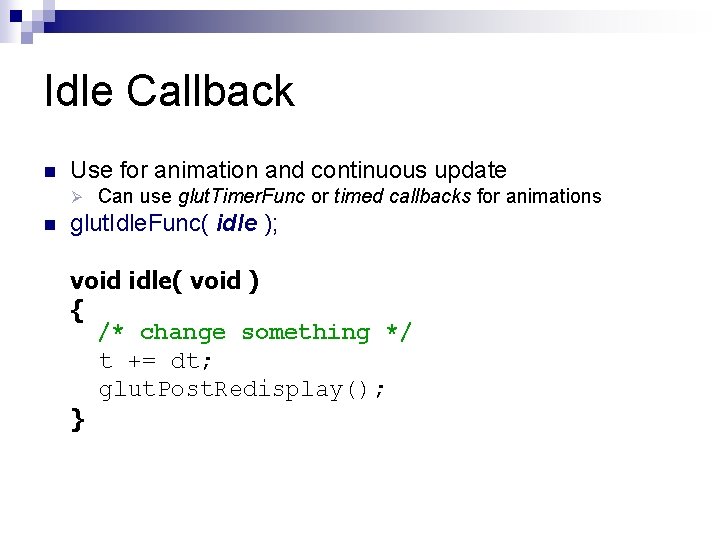
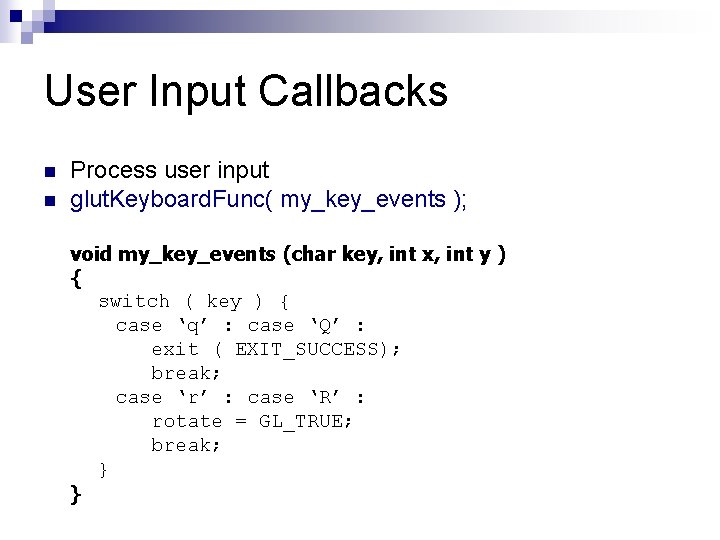
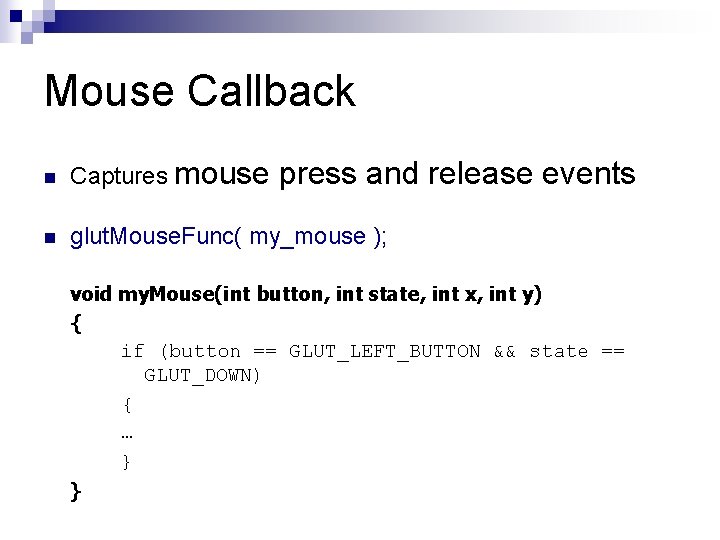
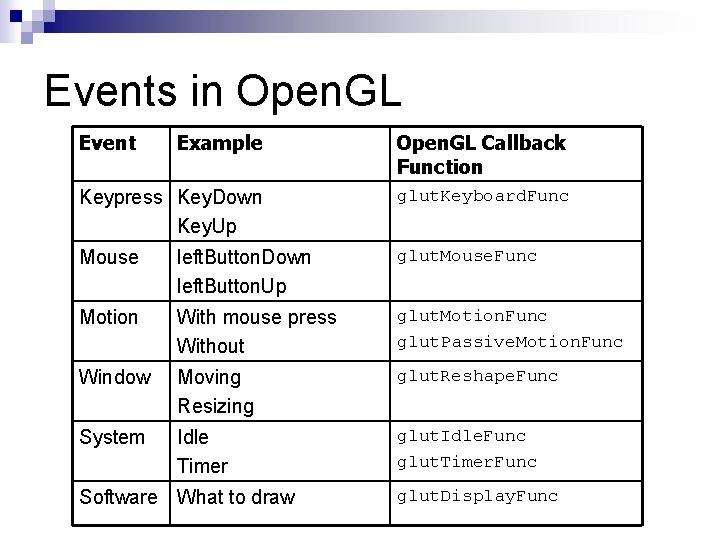
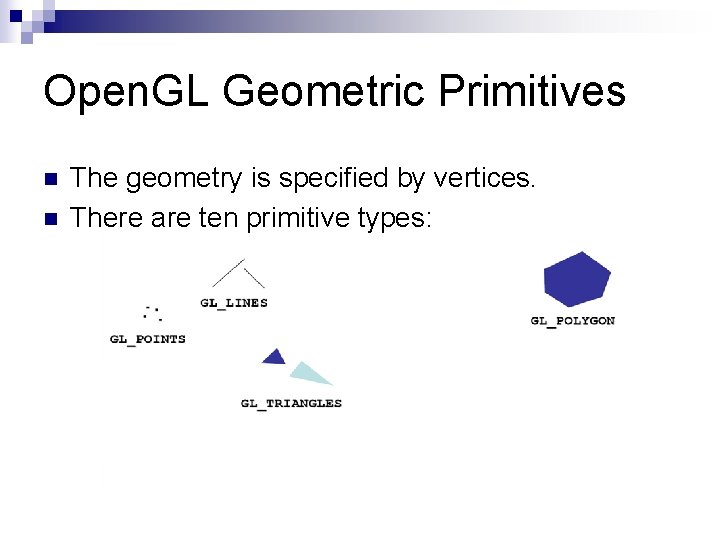
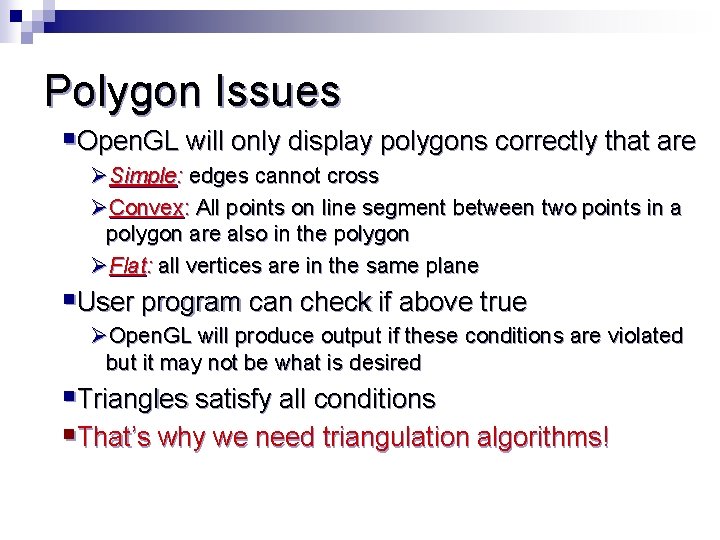
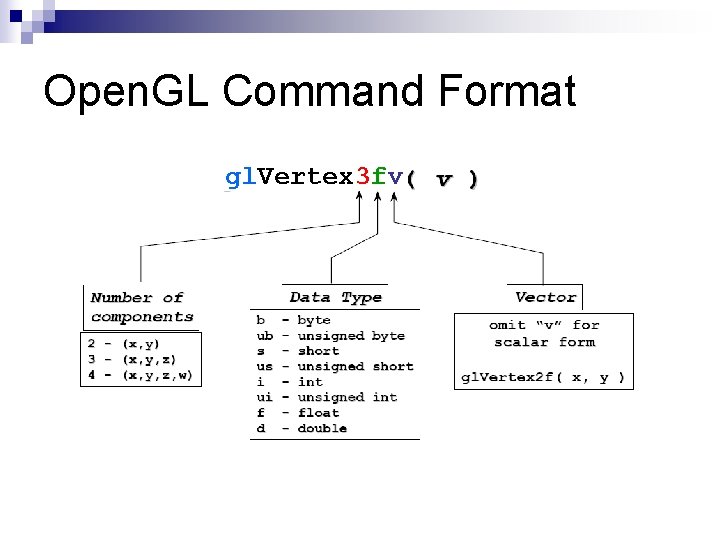
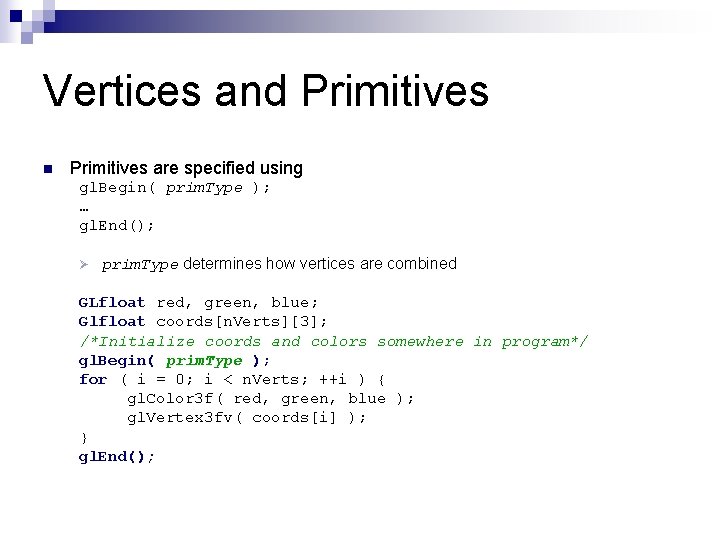
![An Example void draw. Parallelogram( GLfloat color[] ) { gl. Begin( GL_QUADS ); gl. An Example void draw. Parallelogram( GLfloat color[] ) { gl. Begin( GL_QUADS ); gl.](https://slidetodoc.com/presentation_image_h/d1e21a0c6754bd934ff35187fdc530d3/image-26.jpg)
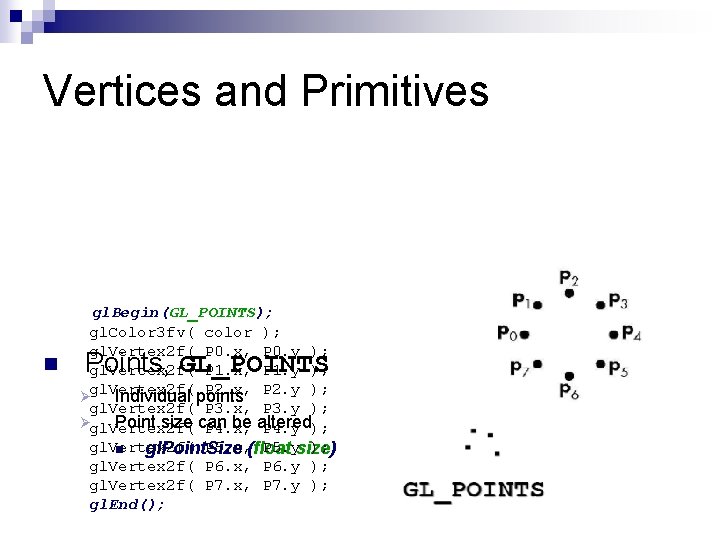
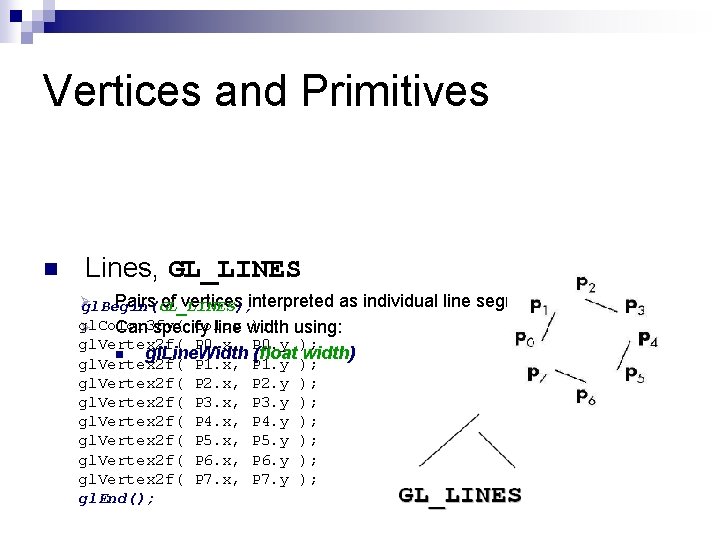
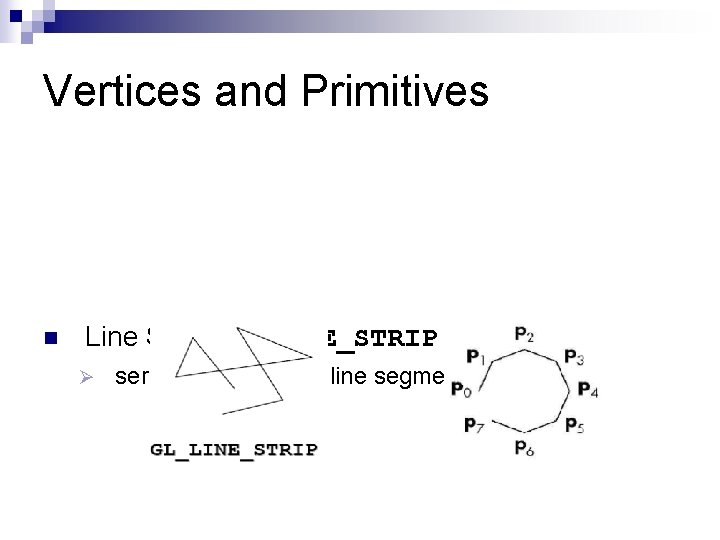
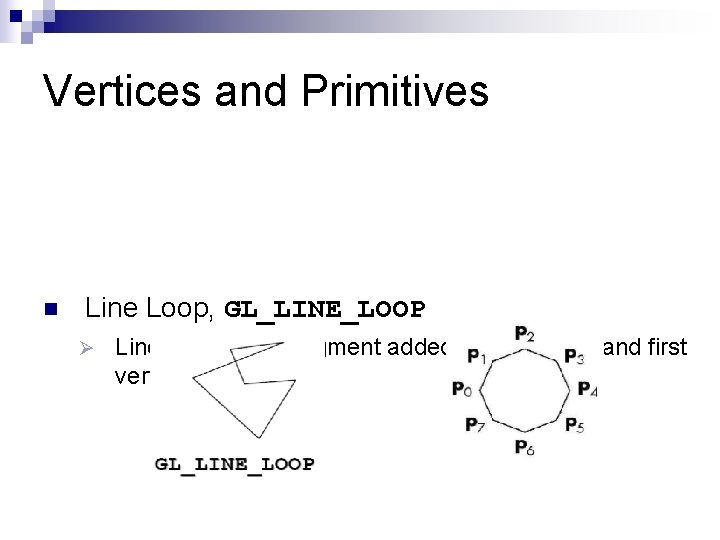
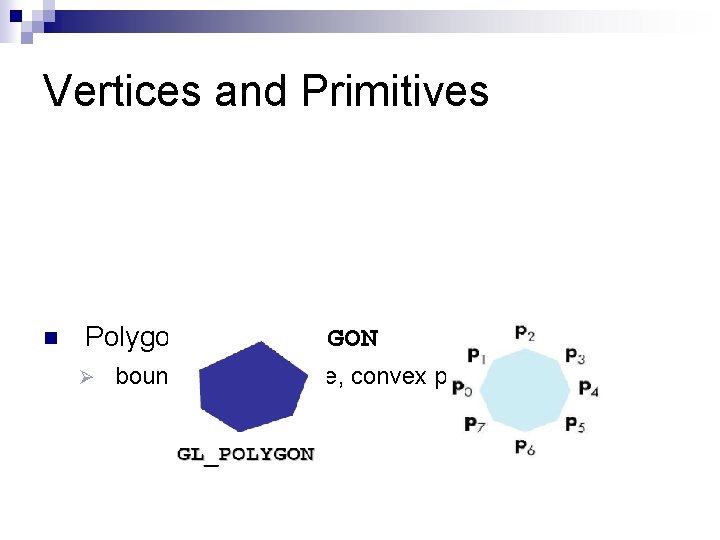
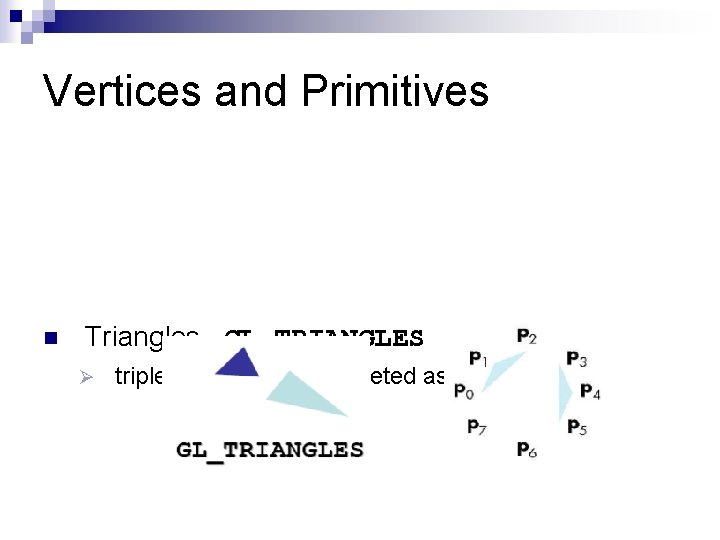
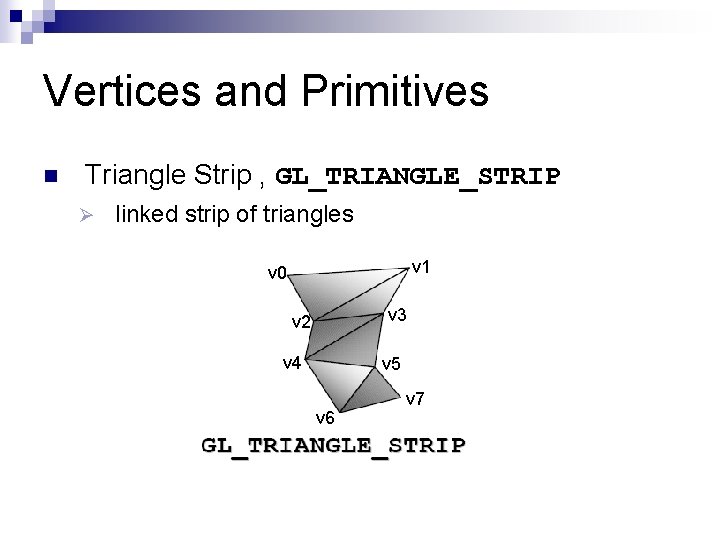
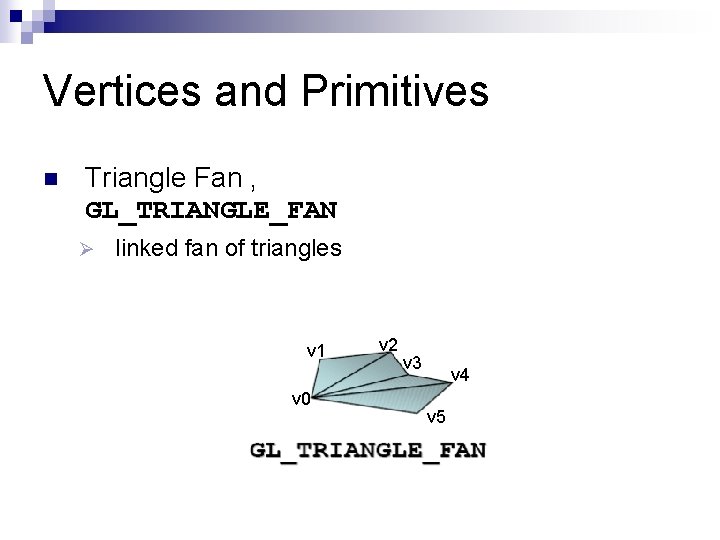
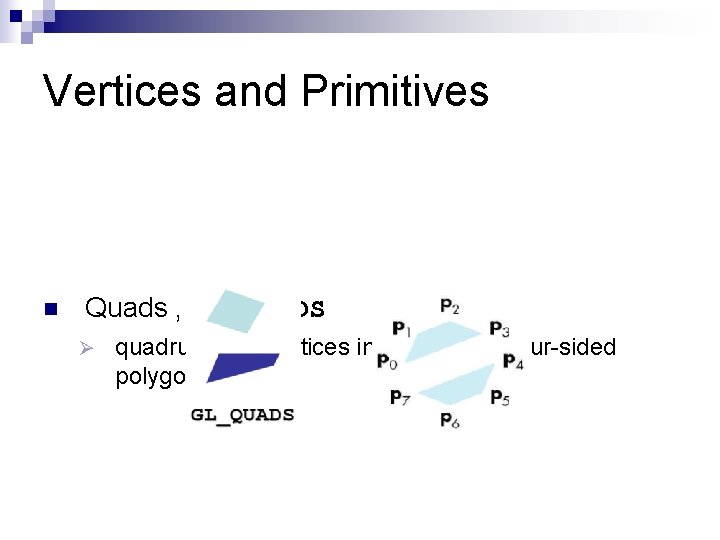
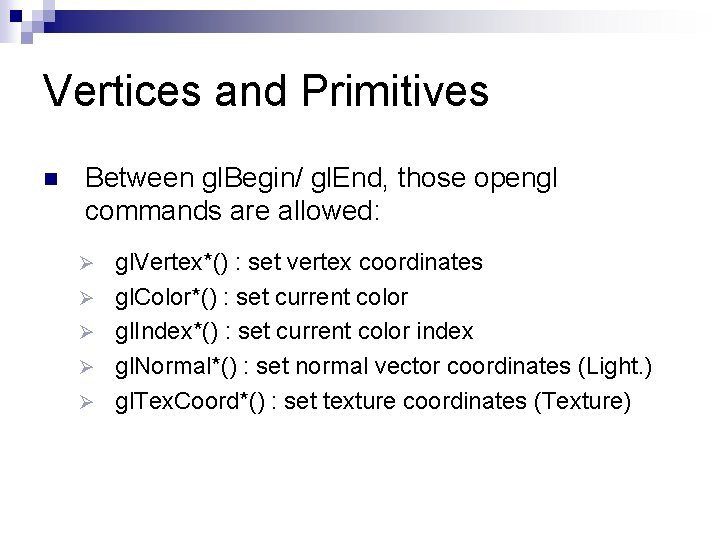
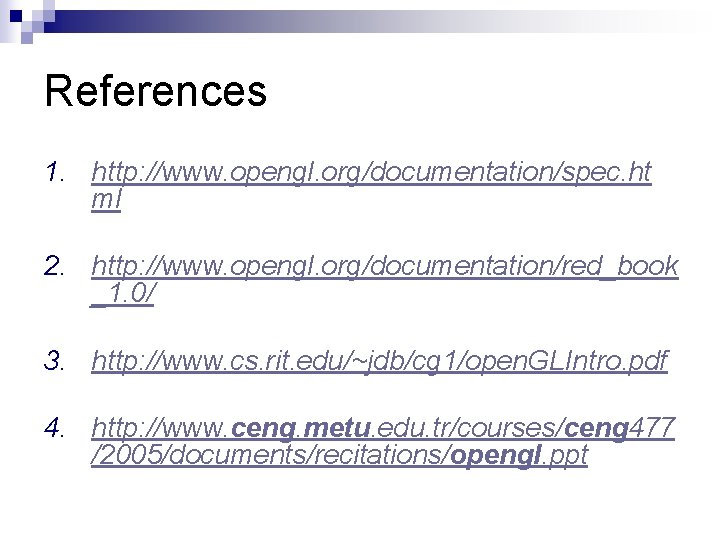
- Slides: 37
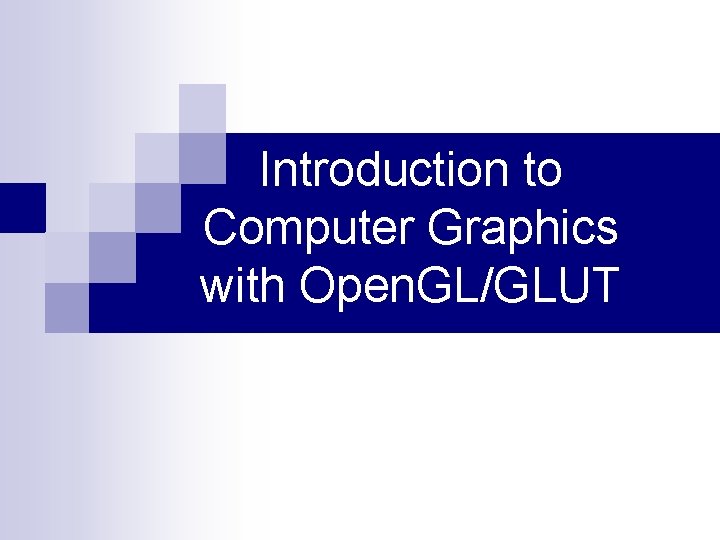
Introduction to Computer Graphics with Open. GL/GLUT
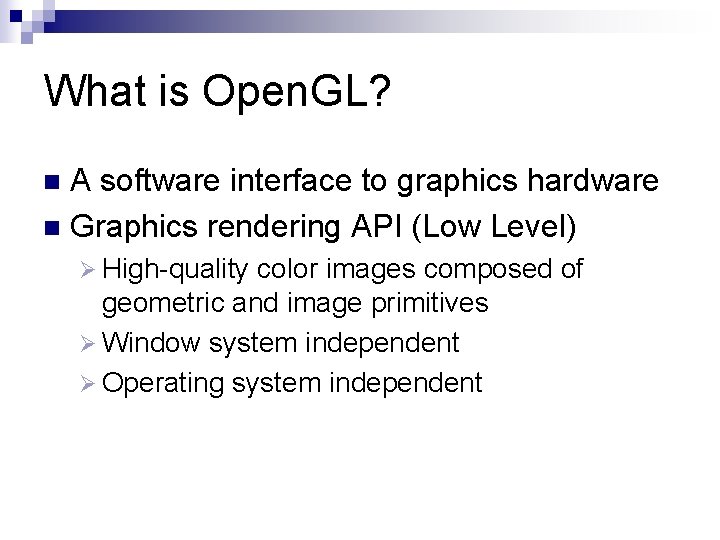
What is Open. GL? A software interface to graphics hardware n Graphics rendering API (Low Level) n Ø High-quality color images composed of geometric and image primitives Ø Window system independent Ø Operating system independent
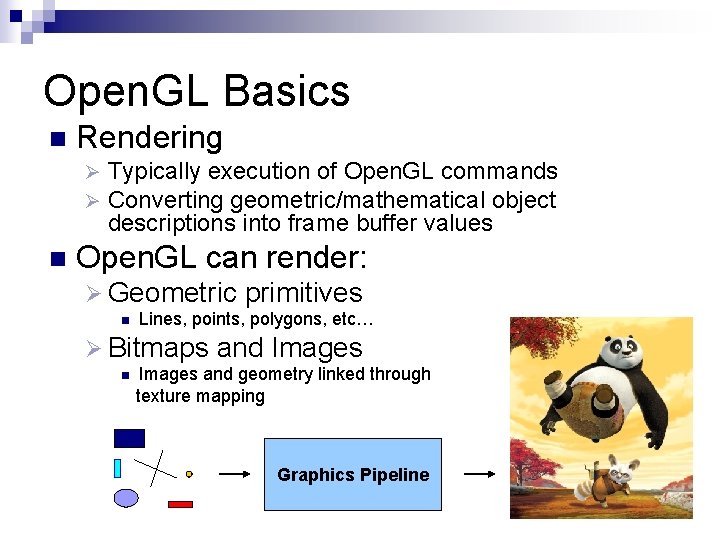
Open. GL Basics n Rendering Ø Ø n Typically execution of Open. GL commands Converting geometric/mathematical object descriptions into frame buffer values Open. GL can render: Ø Geometric n Lines, points, polygons, etc… Ø Bitmaps n primitives and Images and geometry linked through texture mapping Graphics Pipeline
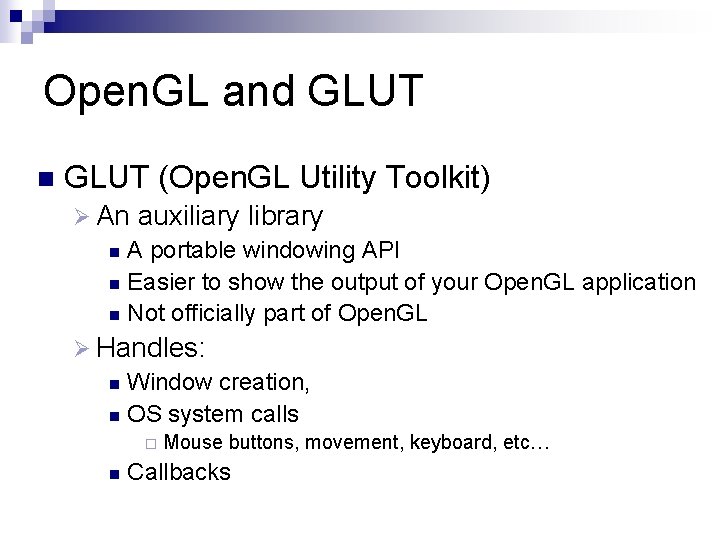
Open. GL and GLUT n GLUT (Open. GL Utility Toolkit) Ø An auxiliary library n A portable windowing API n Easier to show the output of your Open. GL application n Not officially part of Open. GL Ø Handles: n Window creation, n OS system calls ¨ n Mouse buttons, movement, keyboard, etc… Callbacks
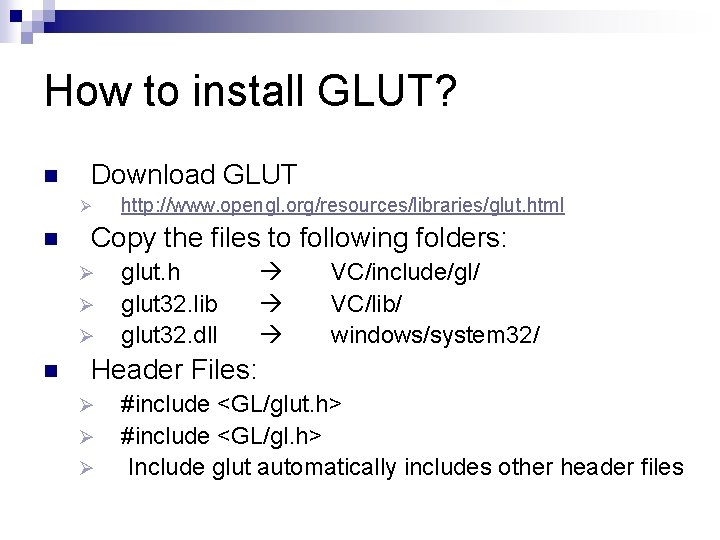
How to install GLUT? n Download GLUT Ø n Copy the files to following folders: Ø Ø Ø n http: //www. opengl. org/resources/libraries/glut. html glut. h glut 32. lib glut 32. dll VC/include/gl/ VC/lib/ windows/system 32/ Header Files: Ø Ø Ø #include <GL/glut. h> #include <GL/gl. h> Include glut automatically includes other header files
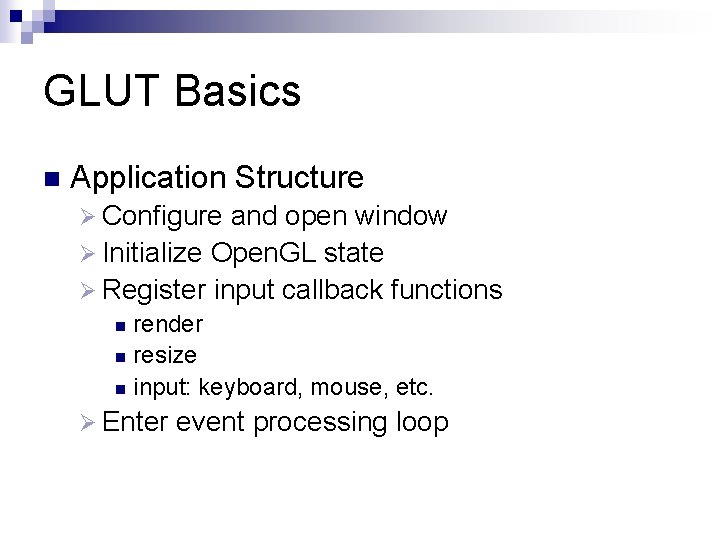
GLUT Basics n Application Structure Ø Configure and open window Ø Initialize Open. GL state Ø Register input callback functions render n resize n input: keyboard, mouse, etc. n Ø Enter event processing loop
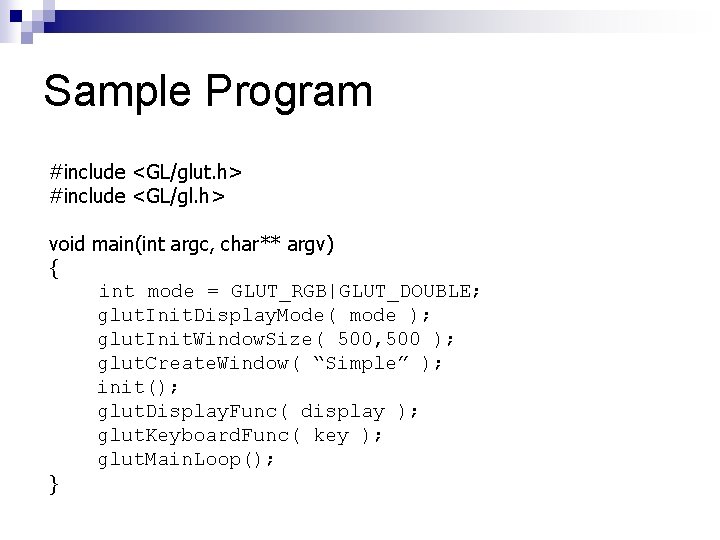
Sample Program #include <GL/glut. h> #include <GL/gl. h> void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_DOUBLE; glut. Init. Display. Mode( mode ); glut. Init. Window. Size( 500, 500 ); glut. Create. Window( “Simple” ); init(); glut. Display. Func( display ); glut. Keyboard. Func( key ); glut. Main. Loop(); }
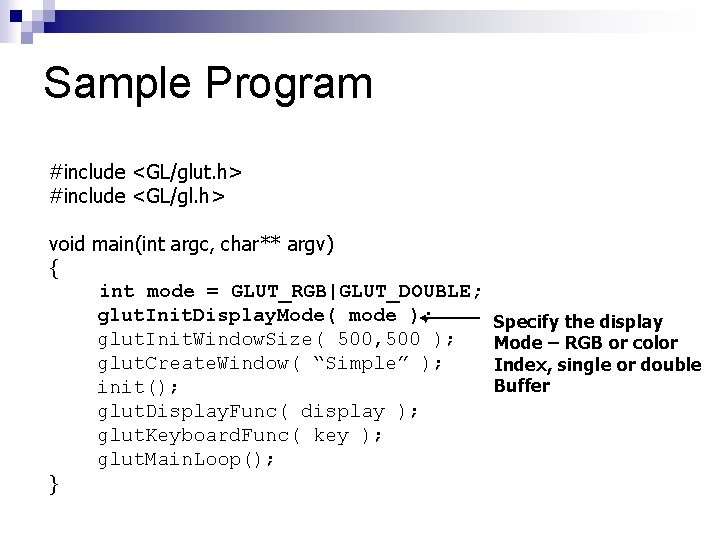
Sample Program #include <GL/glut. h> #include <GL/gl. h> void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_DOUBLE; glut. Init. Display. Mode( mode ); glut. Init. Window. Size( 500, 500 ); glut. Create. Window( “Simple” ); init(); glut. Display. Func( display ); glut. Keyboard. Func( key ); glut. Main. Loop(); } Specify the display Mode – RGB or color Index, single or double Buffer
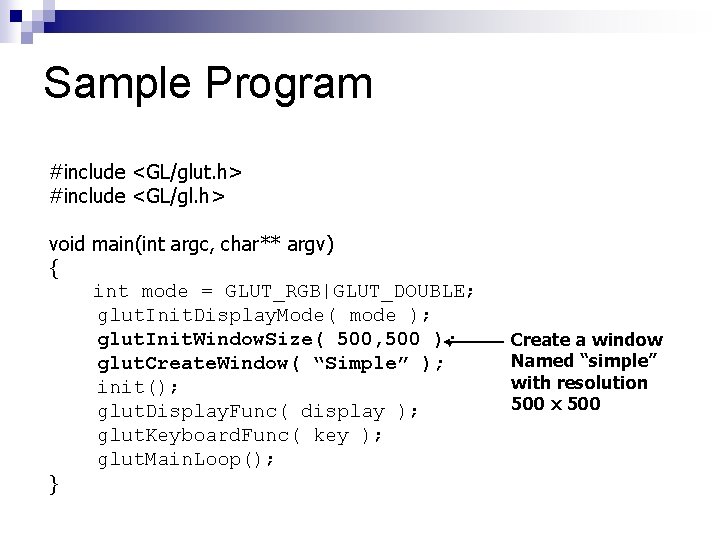
Sample Program #include <GL/glut. h> #include <GL/gl. h> void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_DOUBLE; glut. Init. Display. Mode( mode ); glut. Init. Window. Size( 500, 500 ); glut. Create. Window( “Simple” ); init(); glut. Display. Func( display ); glut. Keyboard. Func( key ); glut. Main. Loop(); } Create a window Named “simple” with resolution 500 x 500
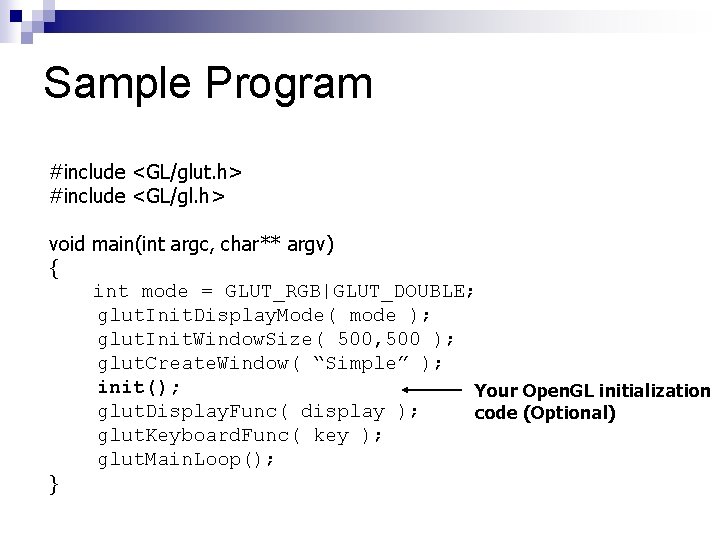
Sample Program #include <GL/glut. h> #include <GL/gl. h> void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_DOUBLE; glut. Init. Display. Mode( mode ); glut. Init. Window. Size( 500, 500 ); glut. Create. Window( “Simple” ); init(); Your Open. GL initialization glut. Display. Func( display ); code (Optional) glut. Keyboard. Func( key ); glut. Main. Loop(); }
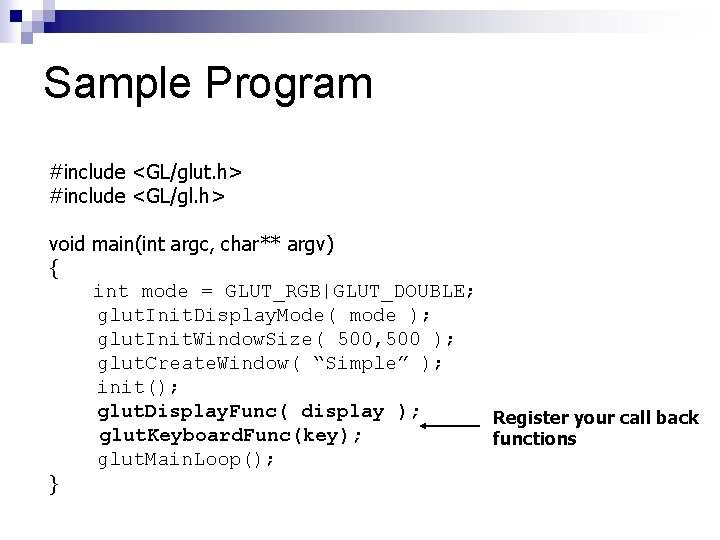
Sample Program #include <GL/glut. h> #include <GL/gl. h> void main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_DOUBLE; glut. Init. Display. Mode( mode ); glut. Init. Window. Size( 500, 500 ); glut. Create. Window( “Simple” ); init(); glut. Display. Func( display ); Register your call back glut. Keyboard. Func(key); functions glut. Main. Loop(); }
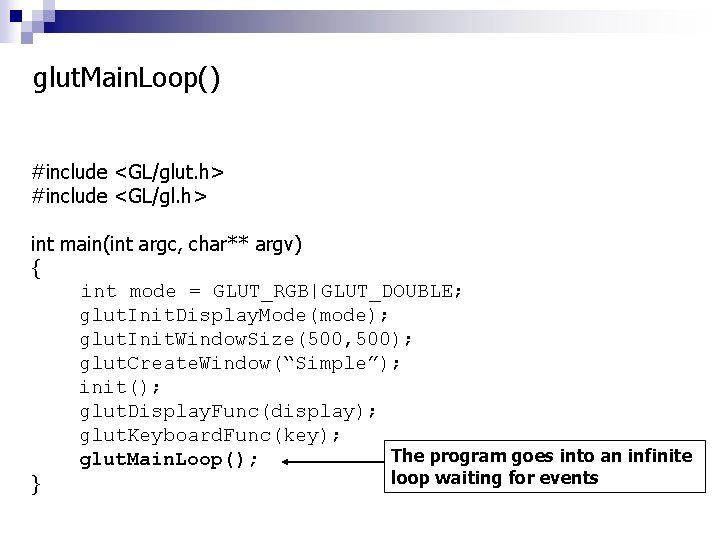
glut. Main. Loop() #include <GL/glut. h> #include <GL/gl. h> int main(int argc, char** argv) { int mode = GLUT_RGB|GLUT_DOUBLE; glut. Init. Display. Mode(mode); glut. Init. Window. Size(500, 500); glut. Create. Window(“Simple”); init(); glut. Display. Func(display); glut. Keyboard. Func(key); The program goes into an infinite glut. Main. Loop(); loop waiting for events }
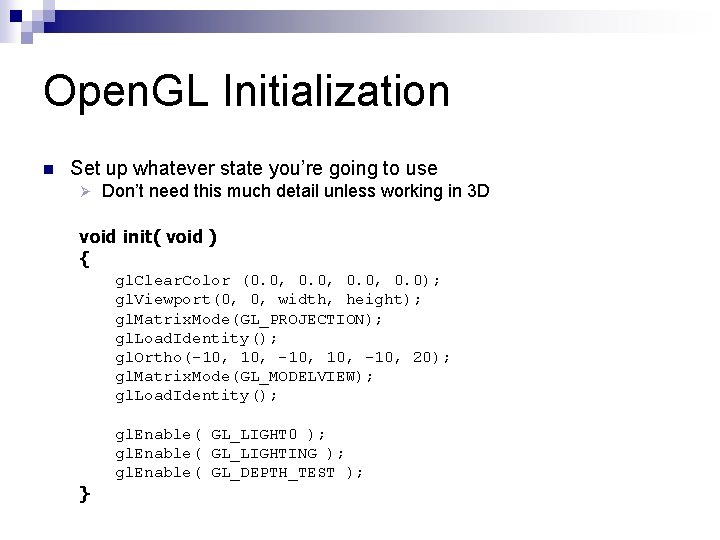
Open. GL Initialization n Set up whatever state you’re going to use Ø Don’t need this much detail unless working in 3 D void init( void ) { gl. Clear. Color (0. 0, 0. 0); gl. Viewport(0, 0, width, height); gl. Matrix. Mode(GL_PROJECTION); gl. Load. Identity(); gl. Ortho(-10, 10, -10, 20); gl. Matrix. Mode(GL_MODELVIEW); gl. Load. Identity(); } gl. Enable( GL_LIGHT 0 ); gl. Enable( GL_LIGHTING ); gl. Enable( GL_DEPTH_TEST );
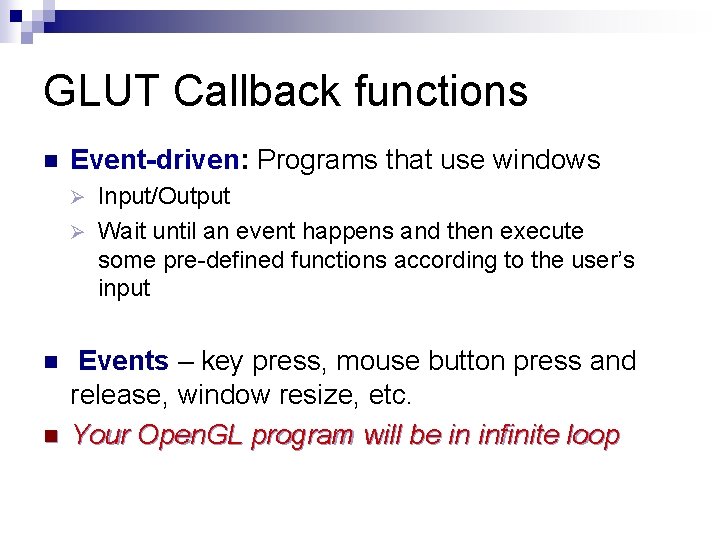
GLUT Callback functions n Event-driven: Programs that use windows Input/Output Ø Wait until an event happens and then execute some pre-defined functions according to the user’s input Ø n n Events – key press, mouse button press and release, window resize, etc. Your Open. GL program will be in infinite loop
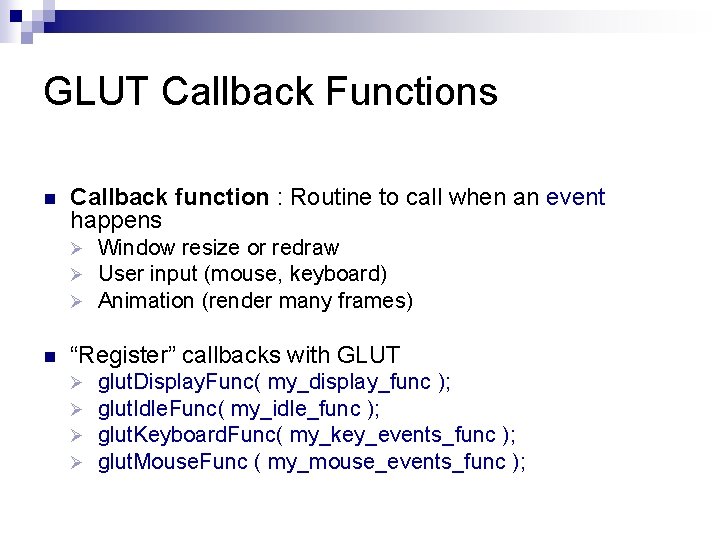
GLUT Callback Functions n Callback function : Routine to call when an event happens Ø Ø Ø n Window resize or redraw User input (mouse, keyboard) Animation (render many frames) “Register” callbacks with GLUT Ø Ø glut. Display. Func( my_display_func ); glut. Idle. Func( my_idle_func ); glut. Keyboard. Func( my_key_events_func ); glut. Mouse. Func ( my_mouse_events_func );
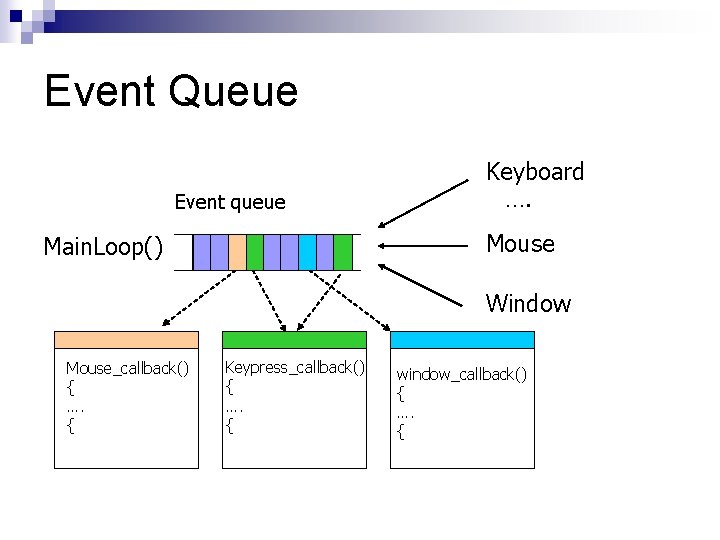
Event Queue Event queue Keyboard …. Mouse Main. Loop() Window Mouse_callback() { …. { Keypress_callback() { …. { window_callback() { …. {
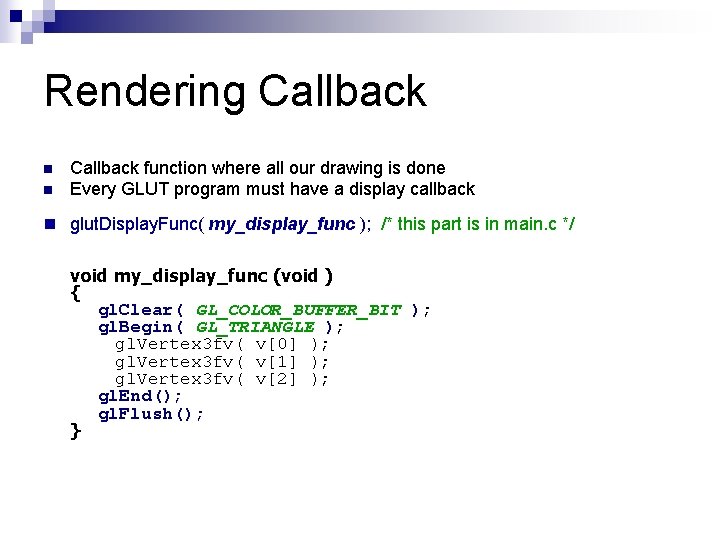
Rendering Callback n n Callback function where all our drawing is done Every GLUT program must have a display callback n glut. Display. Func( my_display_func ); /* this part is in main. c */ void my_display_func (void ) { gl. Clear( GL_COLOR_BUFFER_BIT ); gl. Begin( GL_TRIANGLE ); gl. Vertex 3 fv( v[0] ); gl. Vertex 3 fv( v[1] ); gl. Vertex 3 fv( v[2] ); gl. End(); gl. Flush(); }
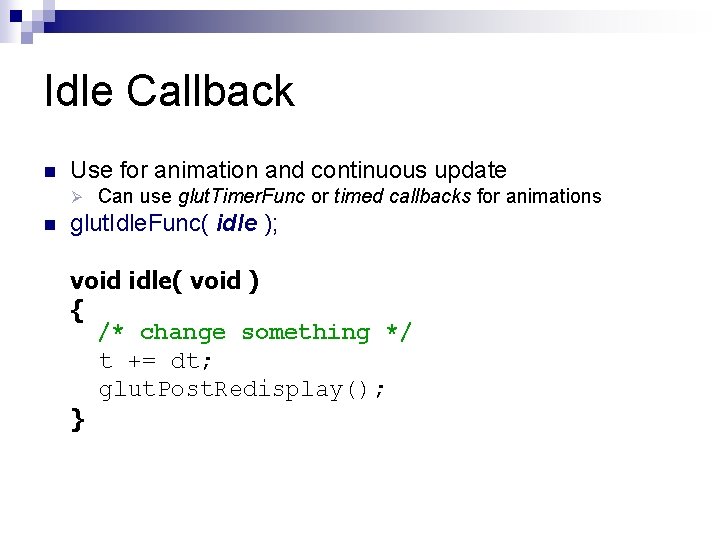
Idle Callback n Use for animation and continuous update Ø n Can use glut. Timer. Func or timed callbacks for animations glut. Idle. Func( idle ); void idle( void ) { /* change something */ t += dt; glut. Post. Redisplay(); }
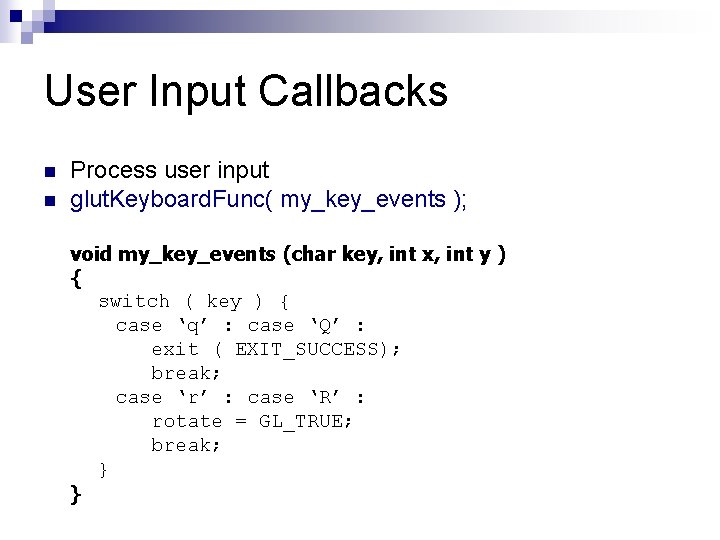
User Input Callbacks n n Process user input glut. Keyboard. Func( my_key_events ); void my_key_events (char key, int x, int y ) { switch ( key ) { case ‘q’ : case ‘Q’ : exit ( EXIT_SUCCESS); break; case ‘r’ : case ‘R’ : rotate = GL_TRUE; break; } }
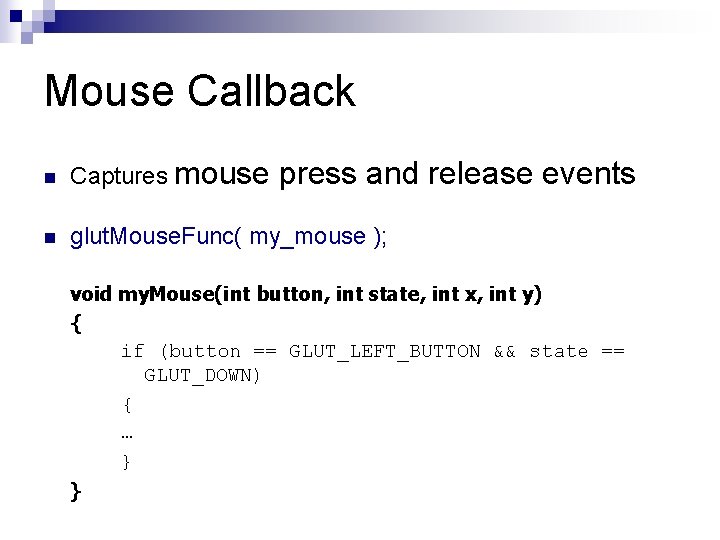
Mouse Callback n Captures mouse press and release events n glut. Mouse. Func( my_mouse ); void my. Mouse(int button, int state, int x, int y) { if (button == GLUT_LEFT_BUTTON && state == GLUT_DOWN) { … } }
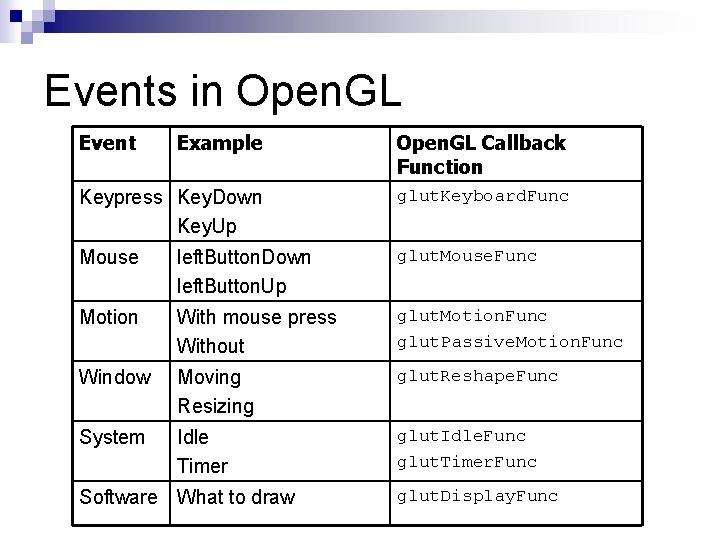
Events in Open. GL Event Example Open. GL Callback Function Keypress Key. Down Key. Up glut. Keyboard. Func Mouse left. Button. Down left. Button. Up glut. Mouse. Func Motion With mouse press Without glut. Motion. Func glut. Passive. Motion. Func Window Moving Resizing glut. Reshape. Func System Idle Timer glut. Idle. Func glut. Timer. Func Software What to draw glut. Display. Func
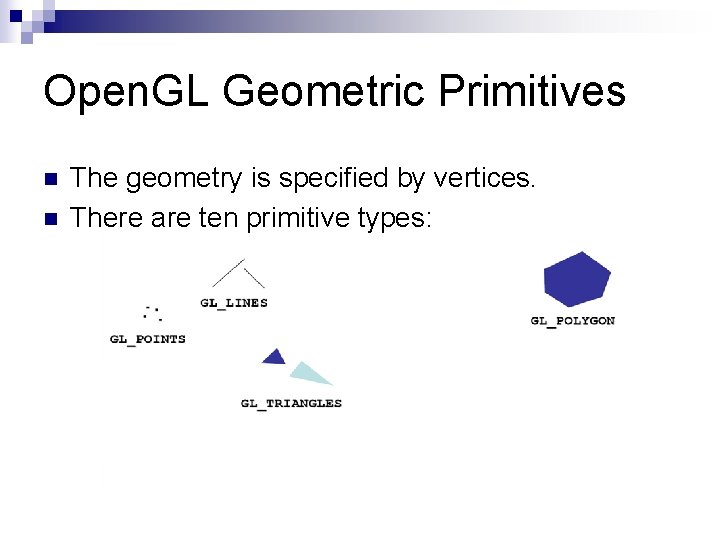
Open. GL Geometric Primitives n n The geometry is specified by vertices. There are ten primitive types:
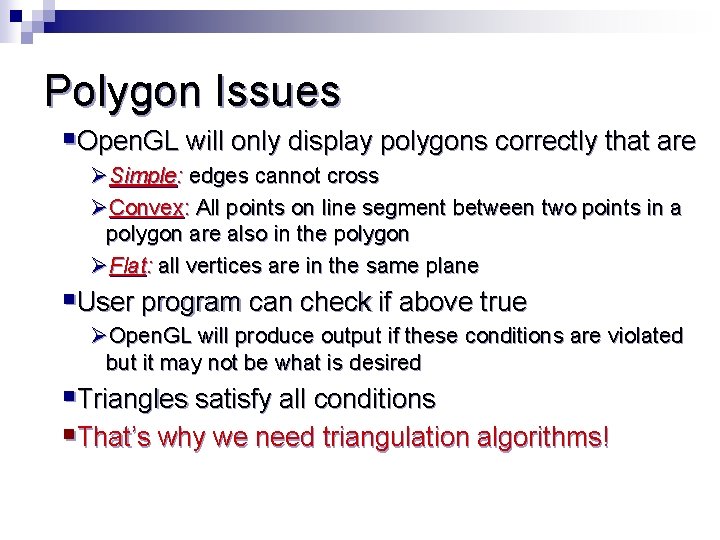
Polygon Issues §Open. GL will only display polygons correctly that are ØSimple: edges cannot cross ØConvex: All points on line segment between two points in a polygon are also in the polygon ØFlat: all vertices are in the same plane §User program can check if above true ØOpen. GL will produce output if these conditions are violated but it may not be what is desired §Triangles satisfy all conditions §That’s why we need triangulation algorithms!
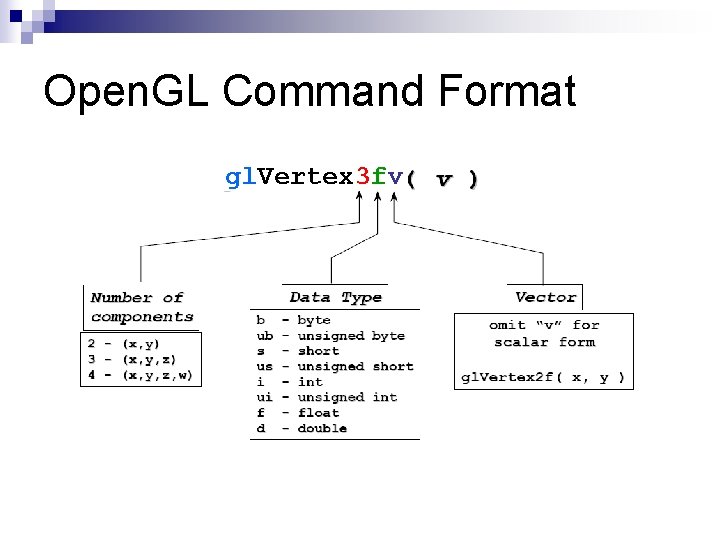
Open. GL Command Format gl. Vertex 3 fv
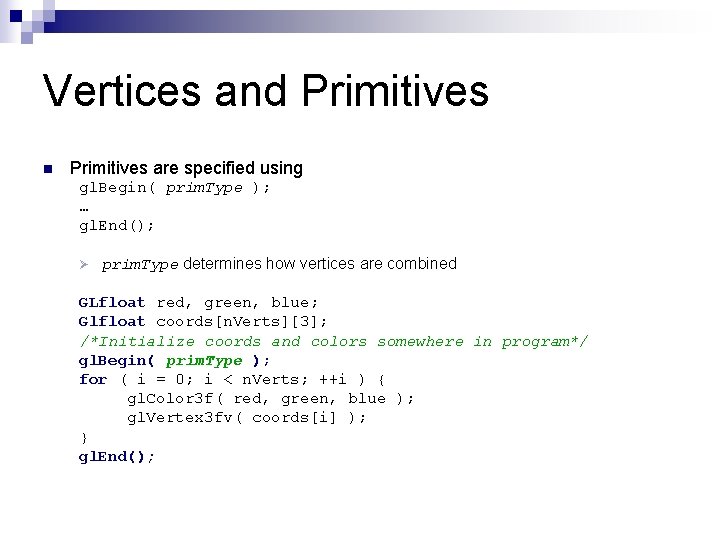
Vertices and Primitives n Primitives are specified using gl. Begin( prim. Type ); … gl. End(); Ø prim. Type determines how vertices are combined GLfloat red, green, blue; Glfloat coords[n. Verts][3]; /*Initialize coords and colors somewhere in program*/ gl. Begin( prim. Type ); for ( i = 0; i < n. Verts; ++i ) { gl. Color 3 f( red, green, blue ); gl. Vertex 3 fv( coords[i] ); } gl. End();
![An Example void draw Parallelogram GLfloat color gl Begin GLQUADS gl An Example void draw. Parallelogram( GLfloat color[] ) { gl. Begin( GL_QUADS ); gl.](https://slidetodoc.com/presentation_image_h/d1e21a0c6754bd934ff35187fdc530d3/image-26.jpg)
An Example void draw. Parallelogram( GLfloat color[] ) { gl. Begin( GL_QUADS ); gl. Color 3 fv( color ); gl. Vertex 2 f( 0. 0, 0. 0 ); gl. Vertex 2 f( 1. 5, 1. 118 ); gl. Vertex 2 f( 0. 5, 1. 118 ); gl. End(); }
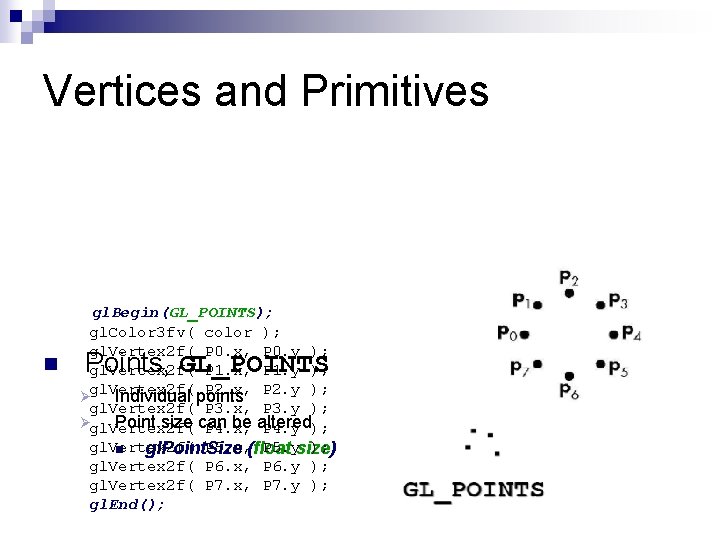
Vertices and Primitives n gl. Begin(GL_POINTS); gl. Color 3 fv( color ); gl. Vertex 2 f( P 0. x, P 0. y ); gl. Vertex 2 f( P 1. x, P 1. y ); gl. Vertex 2 f( P 2. x, P 2. y ); Ø Individual points gl. Vertex 2 f( P 3. x, P 3. y ); Øgl. Vertex 2 f( Point size can be altered P 4. x, P 4. y ); gl. Vertex 2 f( P 5. x, (float P 5. ysize) ); n gl. Point. Size gl. Vertex 2 f( P 6. x, P 6. y ); gl. Vertex 2 f( P 7. x, P 7. y ); gl. End(); Points, GL_POINTS
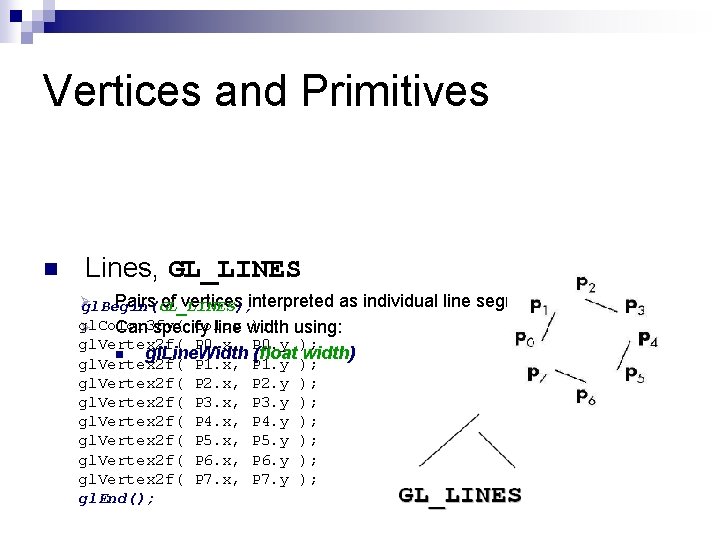
Vertices and Primitives n Lines, GL_LINES Ø Pairs of vertices interpreted gl. Begin(GL_LINES); as individual line segments gl. Color 3 fv( color ); using: Ø Can specify line width gl. Vertex 2 f( P 0. x, P 0. y ); n gl. Line. Width (float width) gl. Vertex 2 f( gl. End(); P 1. x, P 2. x, P 3. x, P 4. x, P 5. x, P 6. x, P 7. x, P 1. y P 2. y P 3. y P 4. y P 5. y P 6. y P 7. y ); );
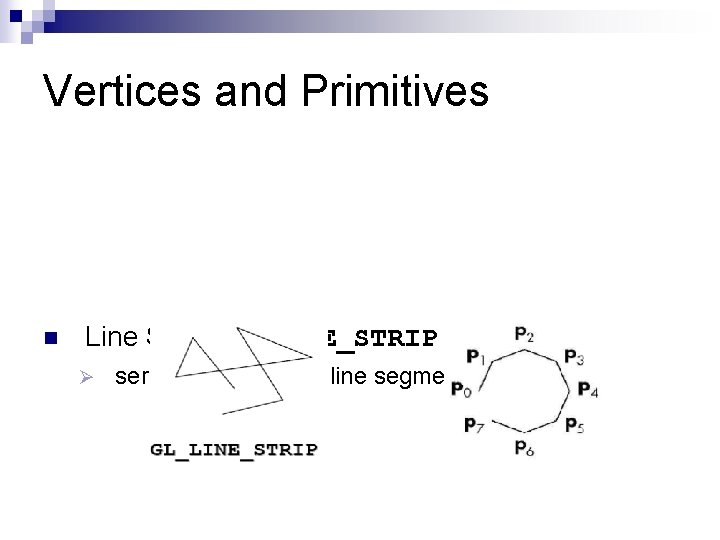
Vertices and Primitives n Line Strip, GL_LINE_STRIP Ø series of connected line segments
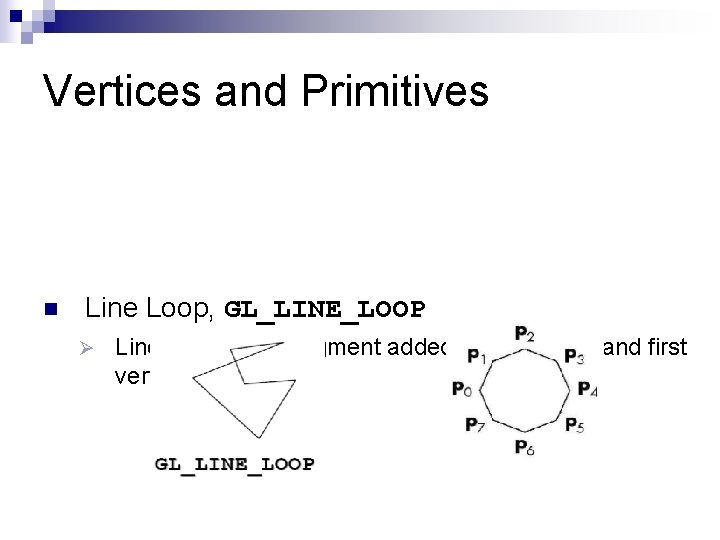
Vertices and Primitives n Line Loop, GL_LINE_LOOP Ø Line strip with a segment added between last and first vertices
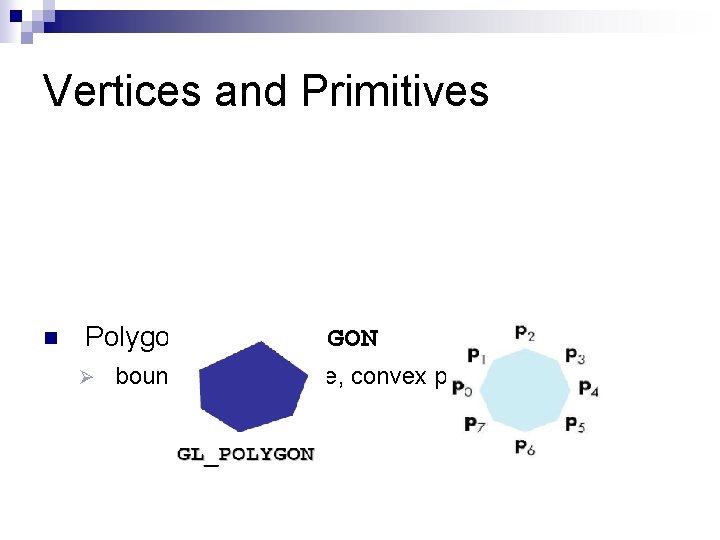
Vertices and Primitives n Polygon , GL_POLYGON Ø boundary of a simple, convex polygon
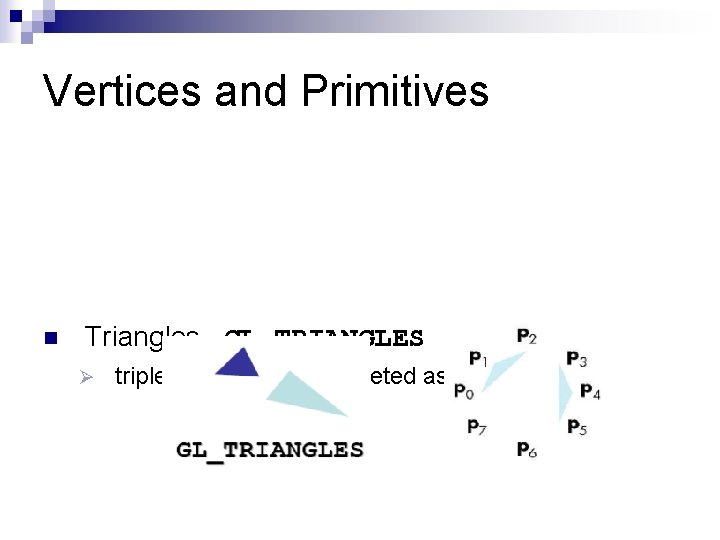
Vertices and Primitives n Triangles , GL_TRIANGLES Ø triples of vertices interpreted as triangles
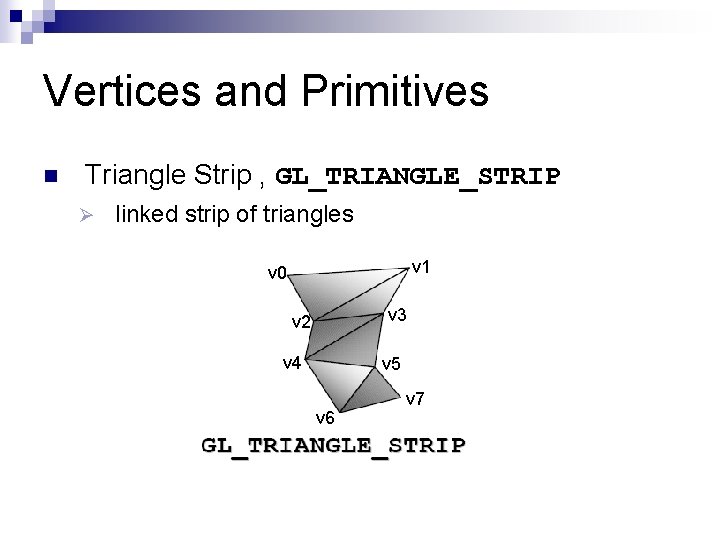
Vertices and Primitives n Triangle Strip , GL_TRIANGLE_STRIP Ø linked strip of triangles v 1 v 0 v 3 v 2 v 4 v 5 v 6 v 7
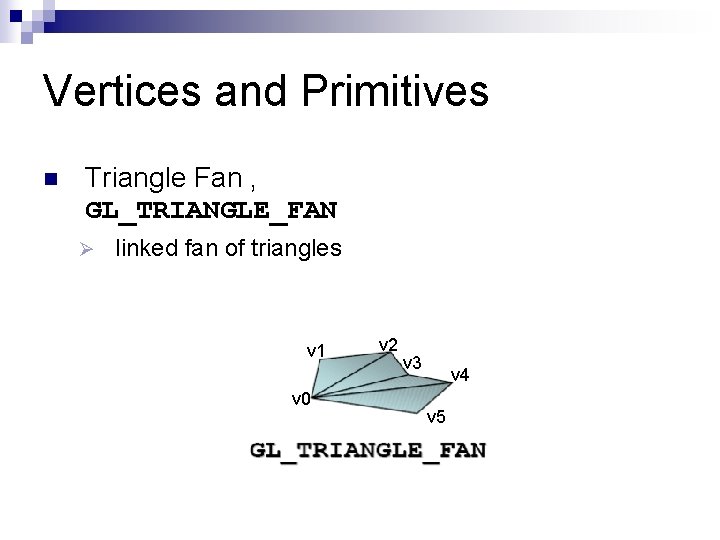
Vertices and Primitives n Triangle Fan , GL_TRIANGLE_FAN Ø linked fan of triangles v 1 v 0 v 2 v 3 v 4 v 5
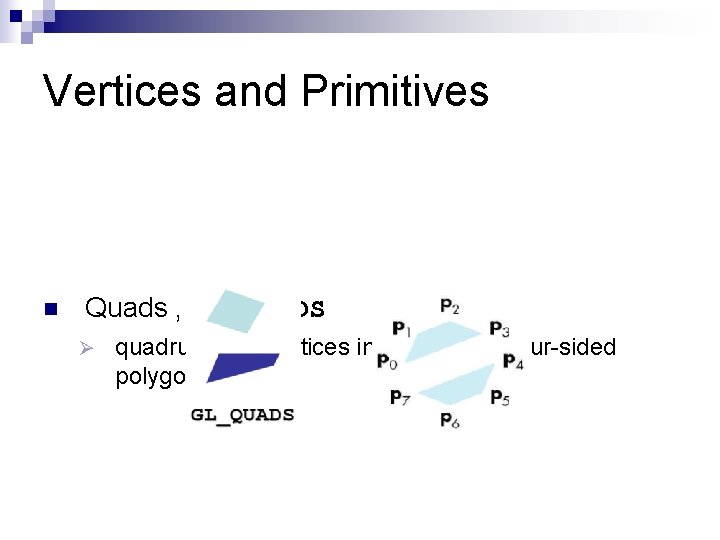
Vertices and Primitives n Quads , GL_QUADS Ø quadruples of vertices interpreted as four-sided polygons
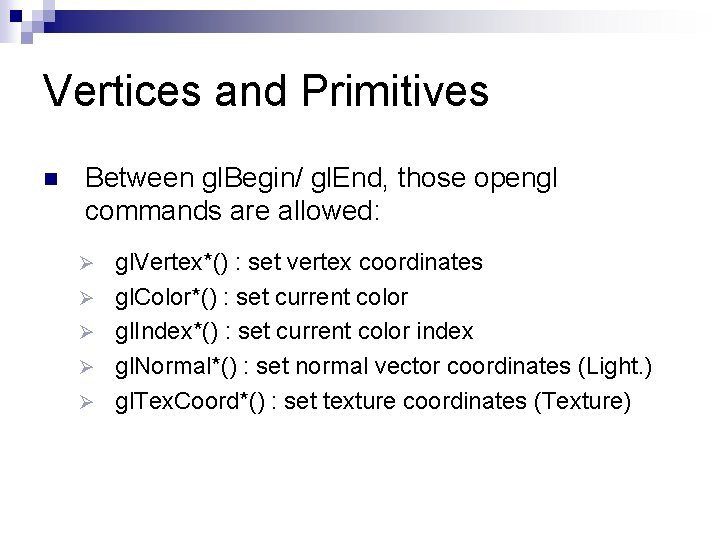
Vertices and Primitives n Between gl. Begin/ gl. End, those opengl commands are allowed: Ø Ø Ø gl. Vertex*() : set vertex coordinates gl. Color*() : set current color gl. Index*() : set current color index gl. Normal*() : set normal vector coordinates (Light. ) gl. Tex. Coord*() : set texture coordinates (Texture)
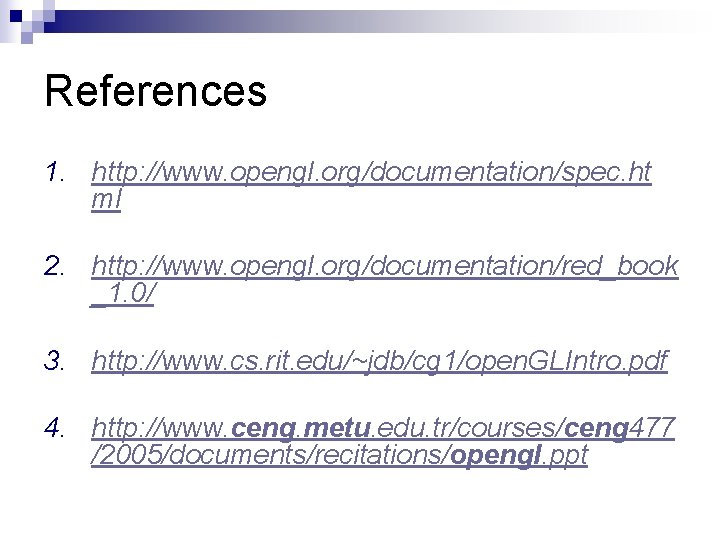
References 1. http: //www. opengl. org/documentation/spec. ht ml 2. http: //www. opengl. org/documentation/red_book _1. 0/ 3. http: //www. cs. rit. edu/~jdb/cg 1/open. GLIntro. pdf 4. http: //www. ceng. metu. edu. tr/courses/ceng 477 /2005/documents/recitations/opengl. ppt
Graphic monitor and workstation in computer graphics
Introduction to computer graphics - ppt
Open innovation open science open to the world
Computer graphics ppt
Open graphics library
Crt in computer graphics
Types of projection in computer graphics
Types of display devices in computer graphics
Two dimensional viewing
Shear transformation in computer graphics
Shader computer graphics
Scan converting circle in computer graphics
Computer graphics
Two region filling algorithms are
Character generation methods in computer graphics
Polygon fill algorithm
Random scan system
Computer graphics
Algorithm to draw a line in computer graphics
Cs 418 interactive computer graphics
Glcreatebuffer
Hidden surface removal in computer graphics
Achromatic light in computer graphics
Logical classification of input devices
Uniform scaling in computer graphics
Uniform scaling in computer graphics
Orthogonal projection in computer graphics
Logical input devices in computer graphics
Sierpinski gasket in computer graphics
Points and lines in computer graphics ppt
Dda algorithm in computer graphics
What is window and viewport in computer graphics
Keyframe animation in computer graphics
Solid examples
Scan conversion in computer graphics
Boundary fill algorithm in computer graphics
Utah teapot vertex data
Thick primitives in computer graphics