Server Side Programming Java Servlets Serverside Programming The
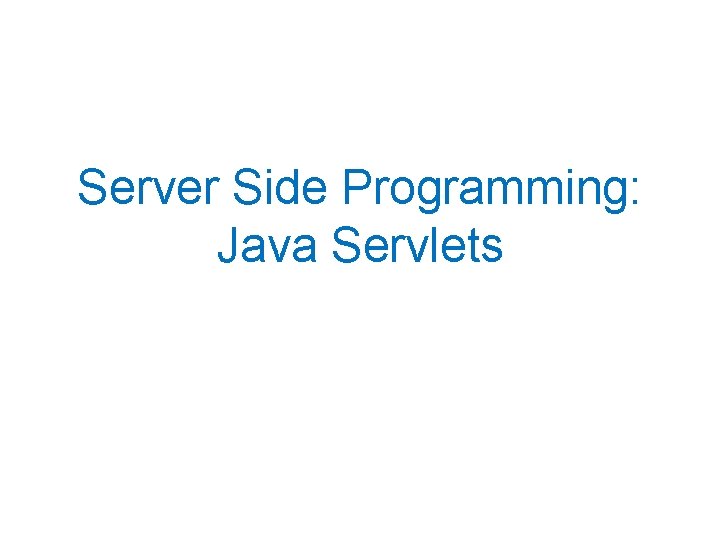
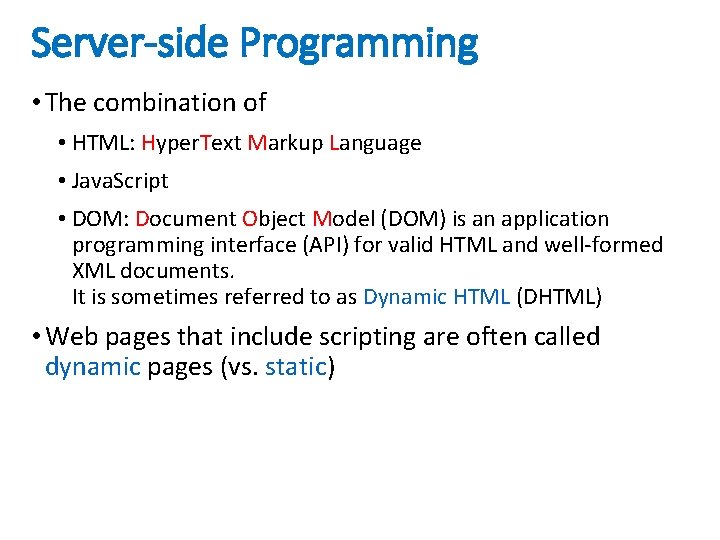
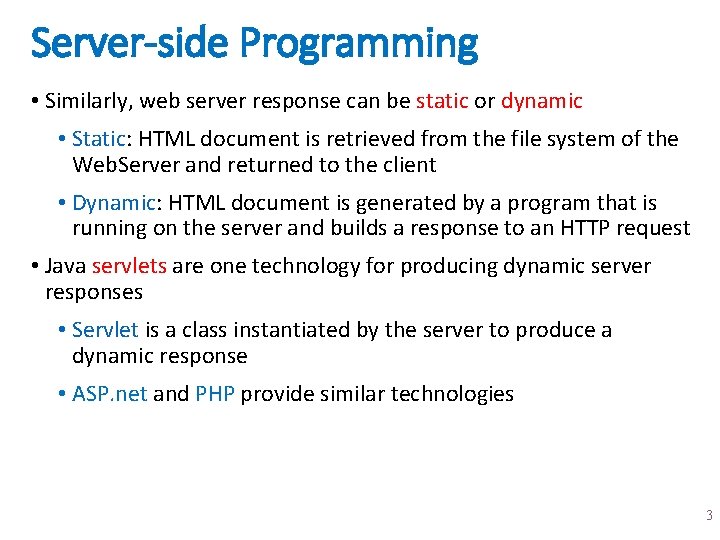
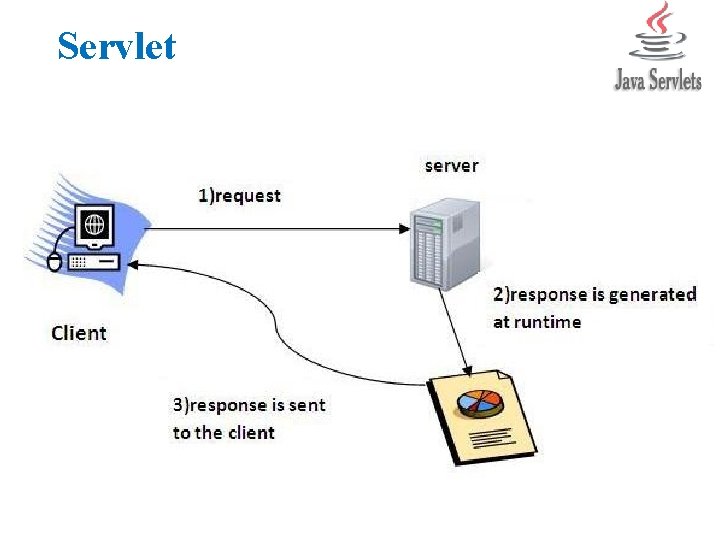
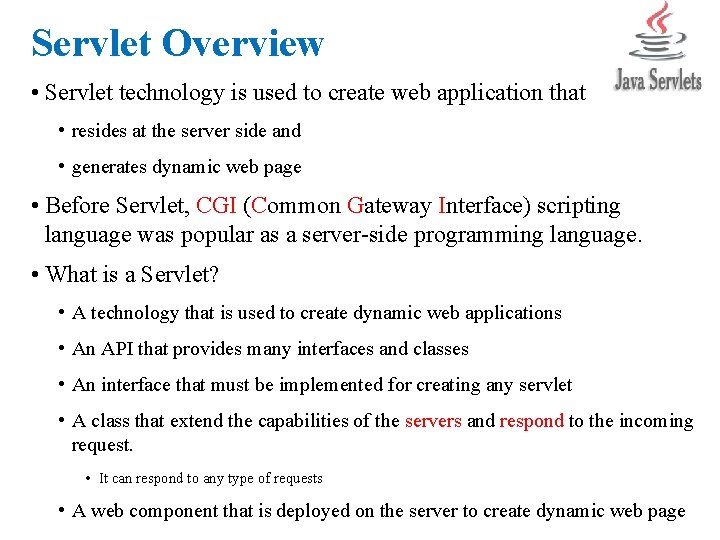
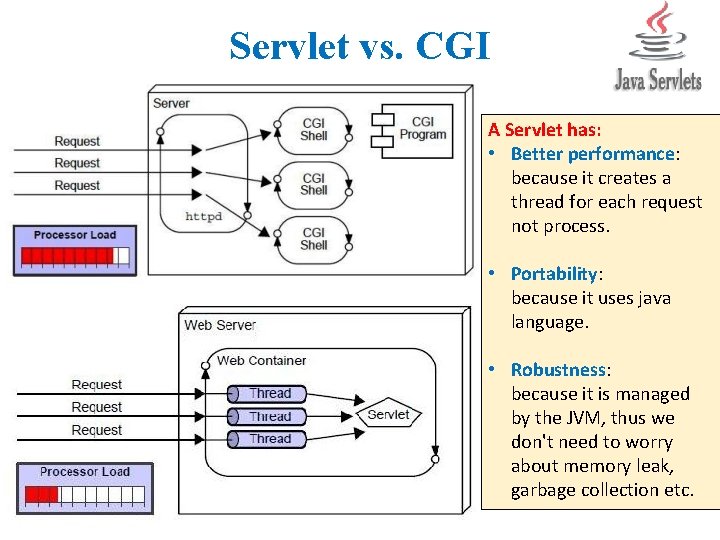
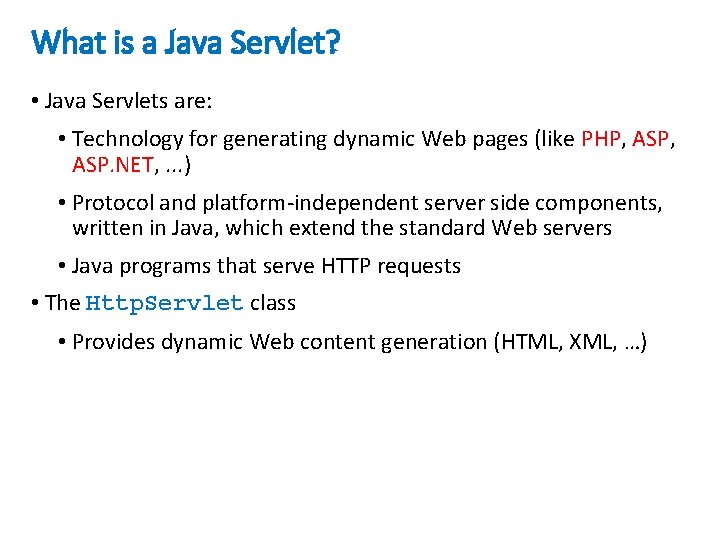
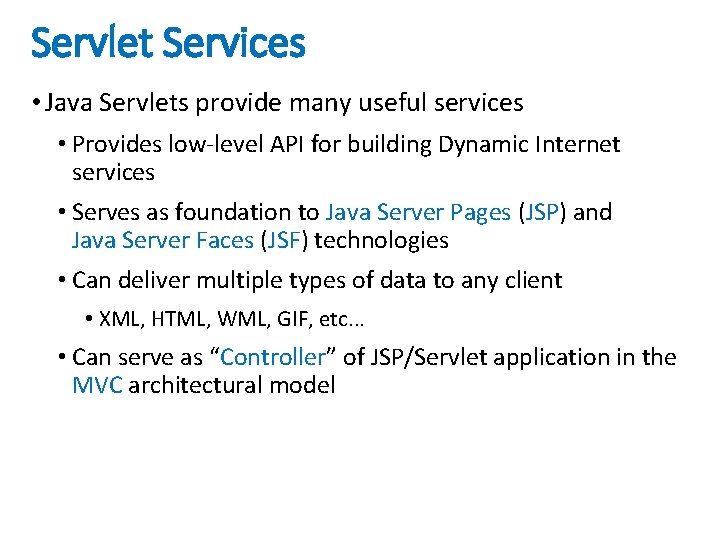
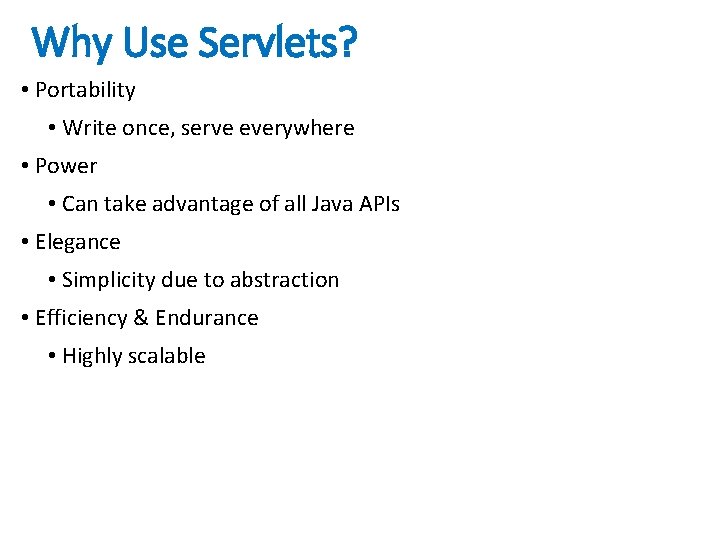
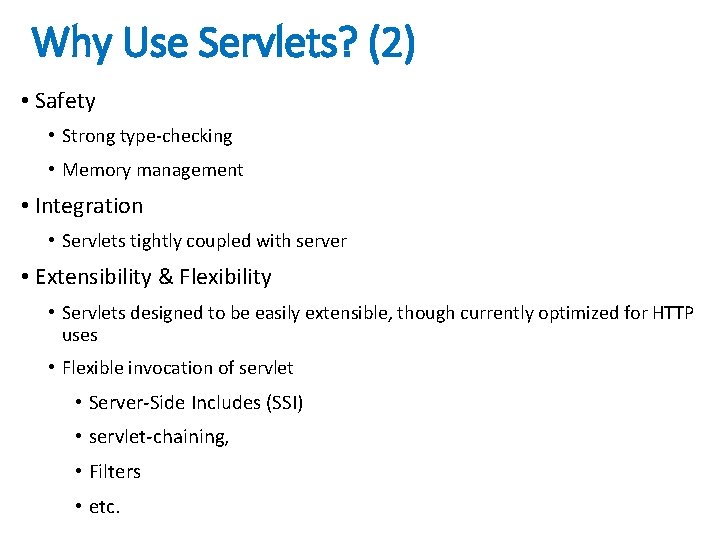
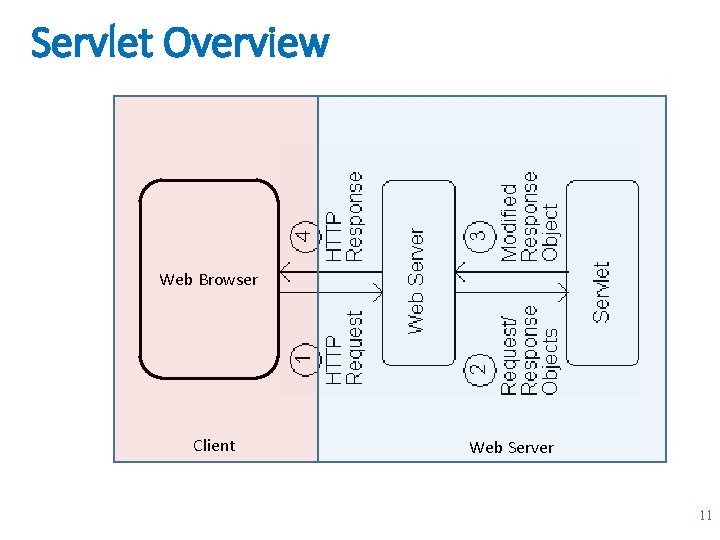
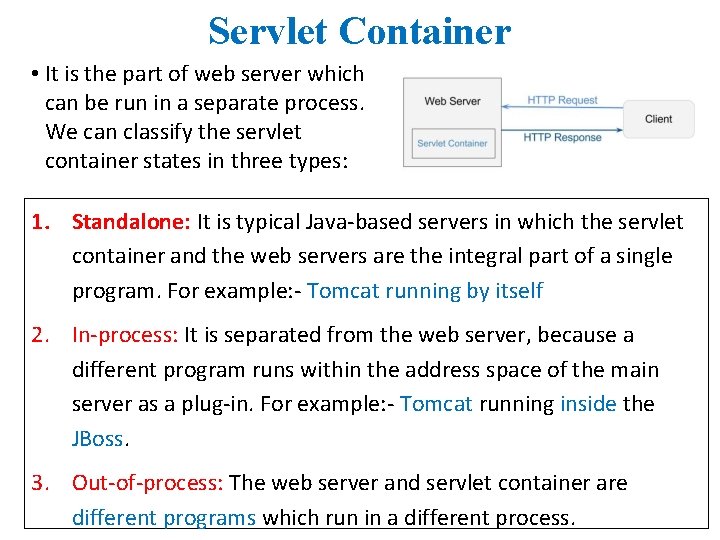
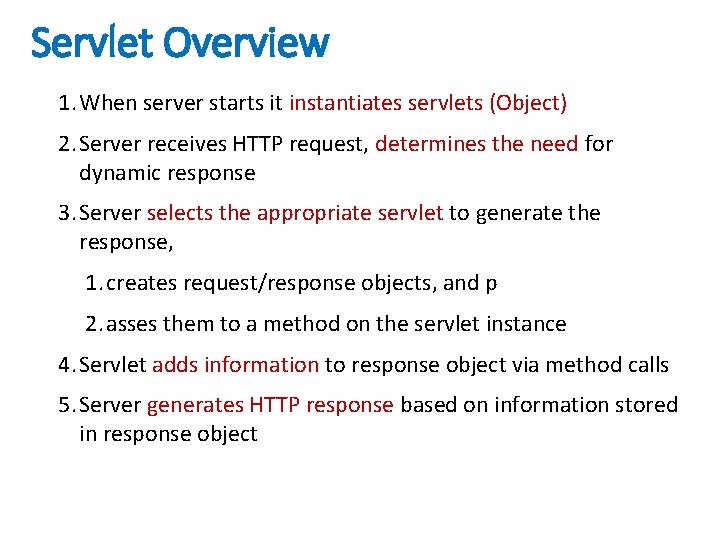
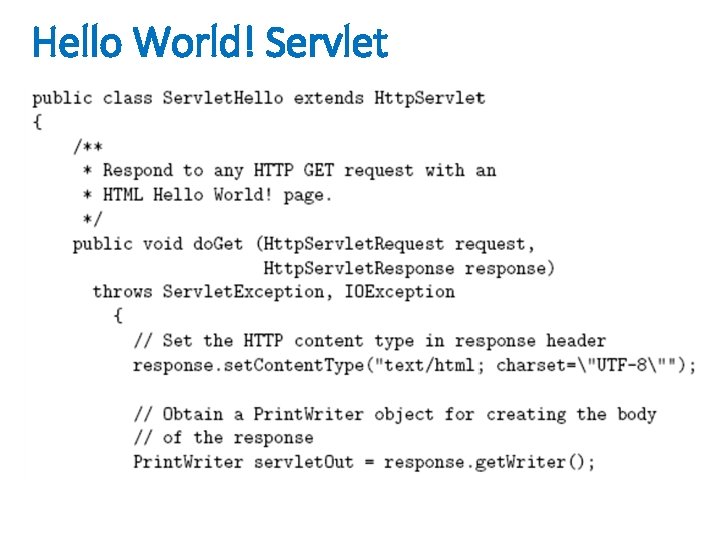
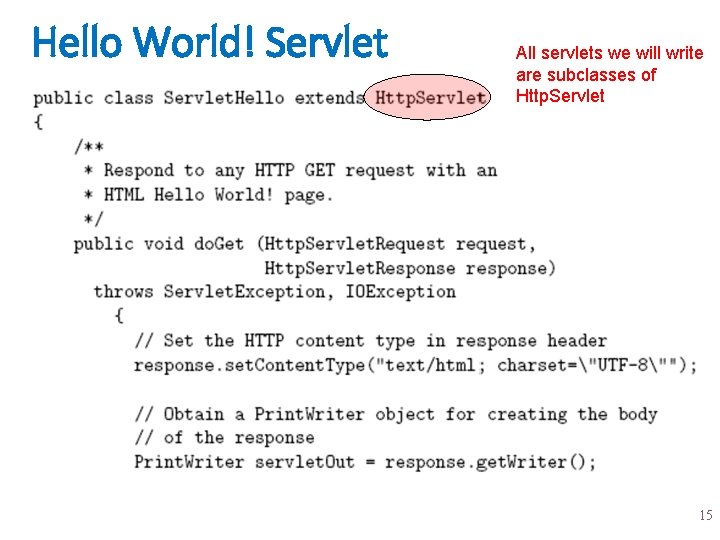
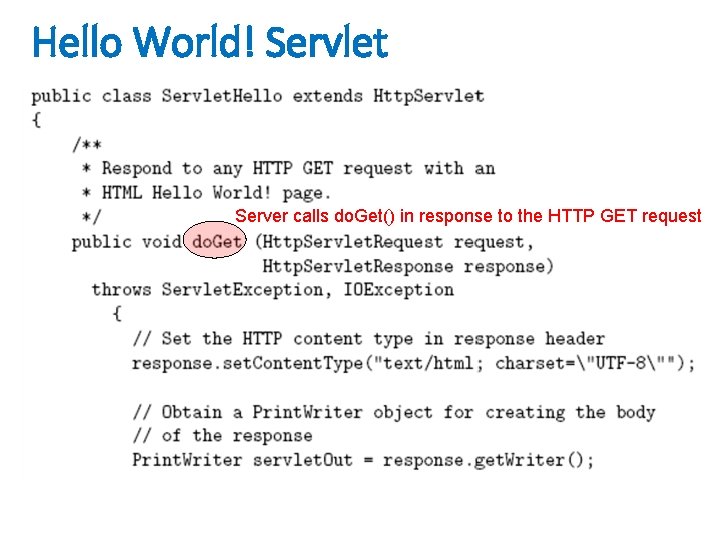
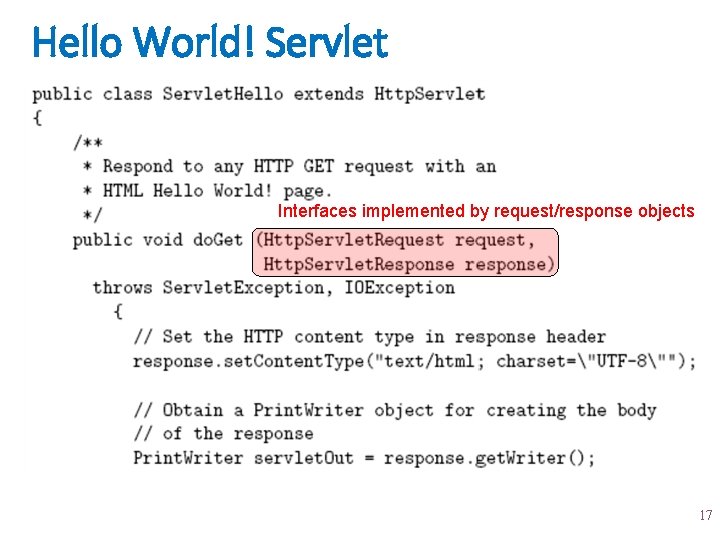
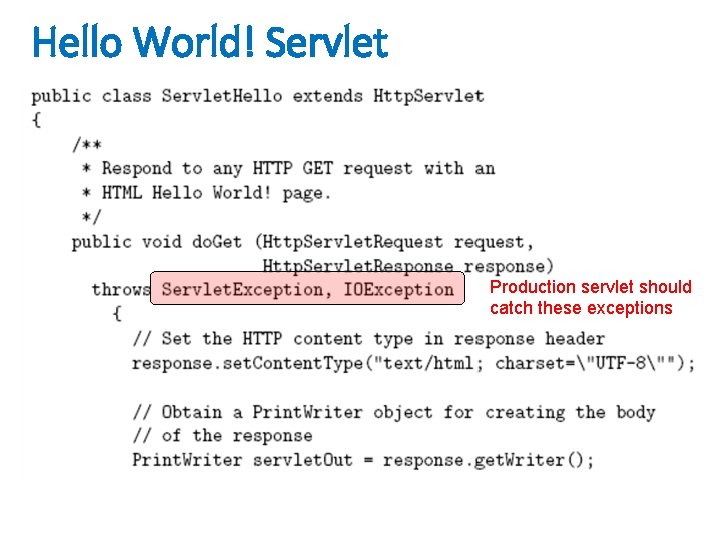
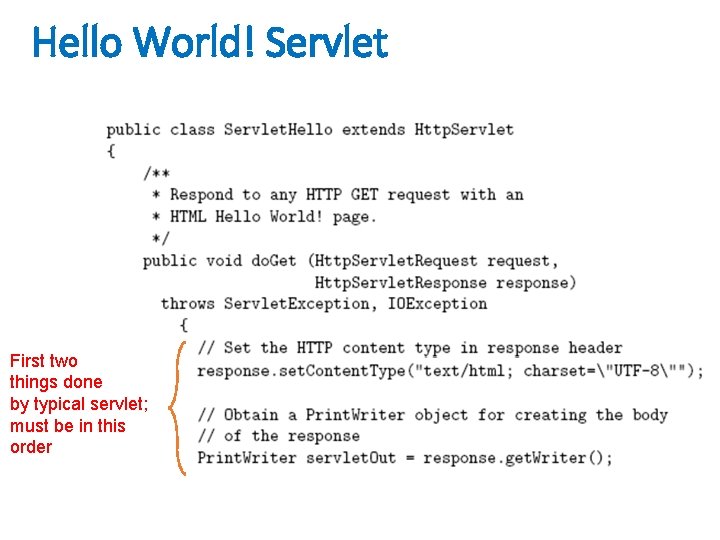
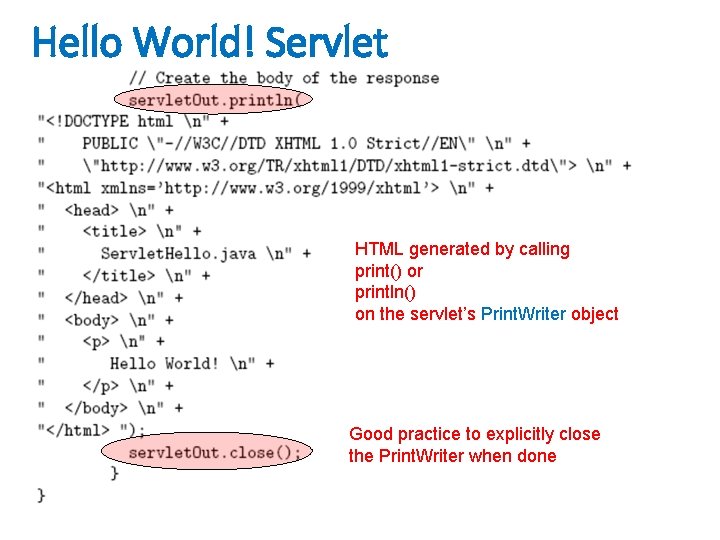
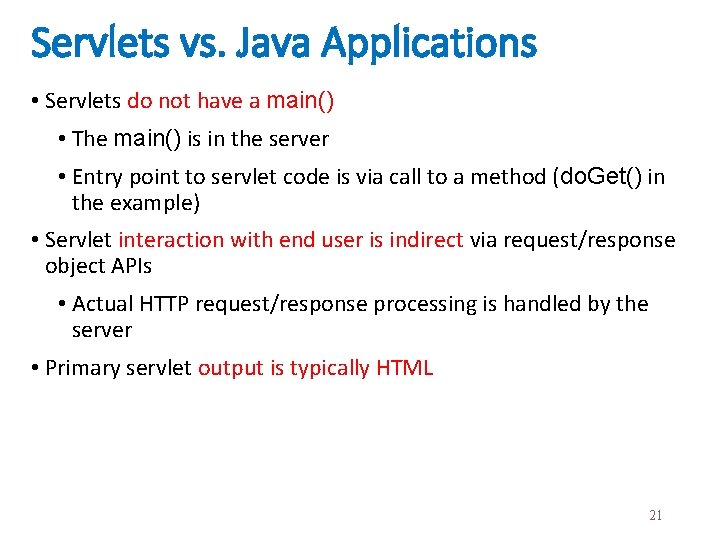
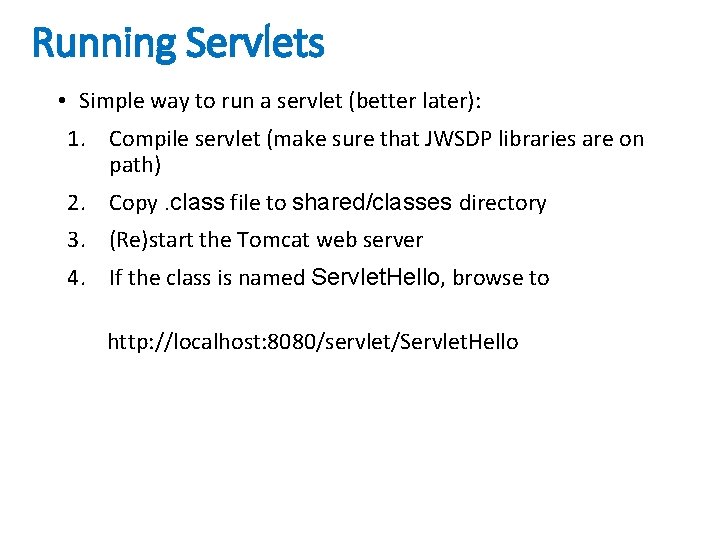
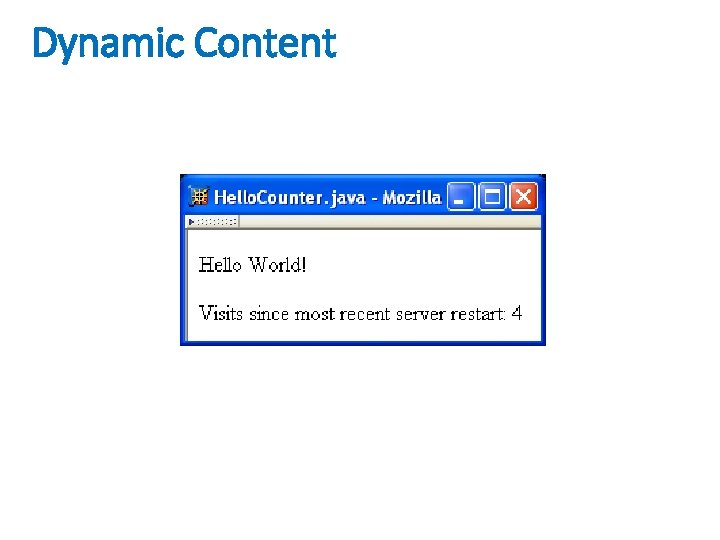
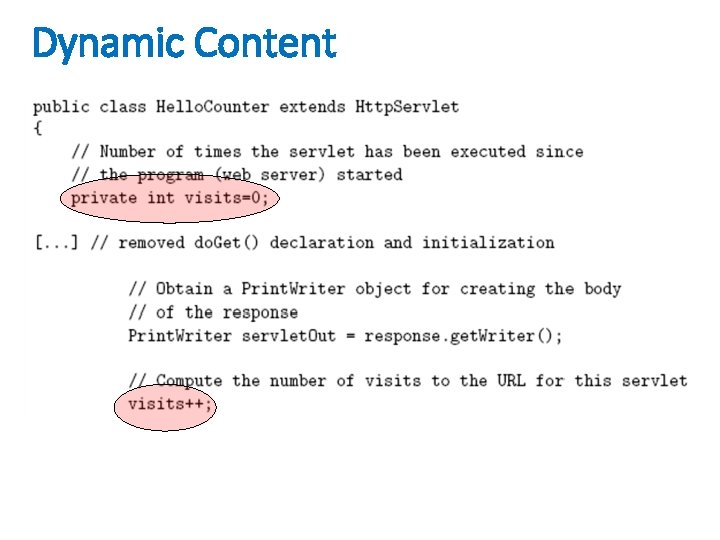
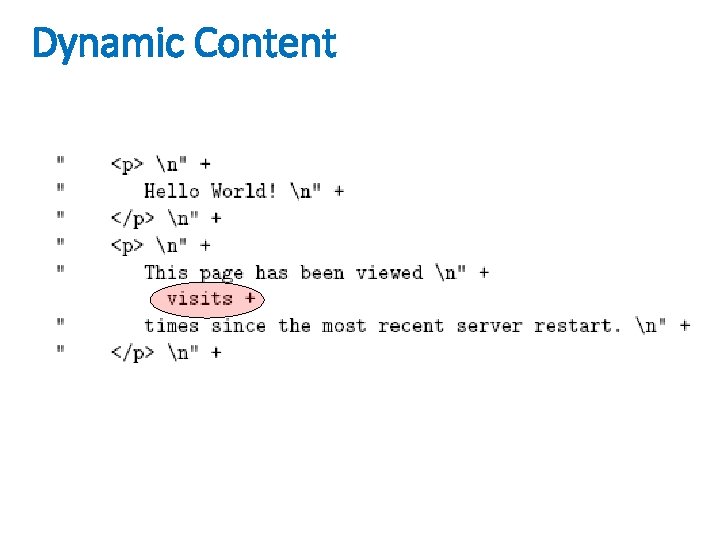
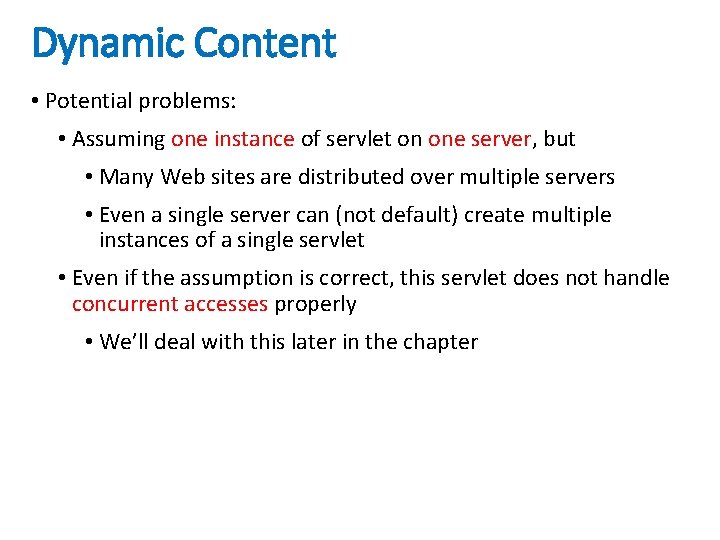
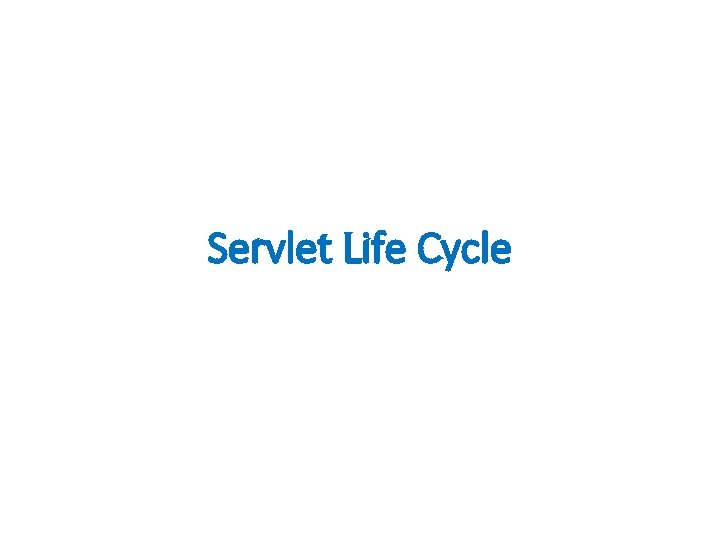
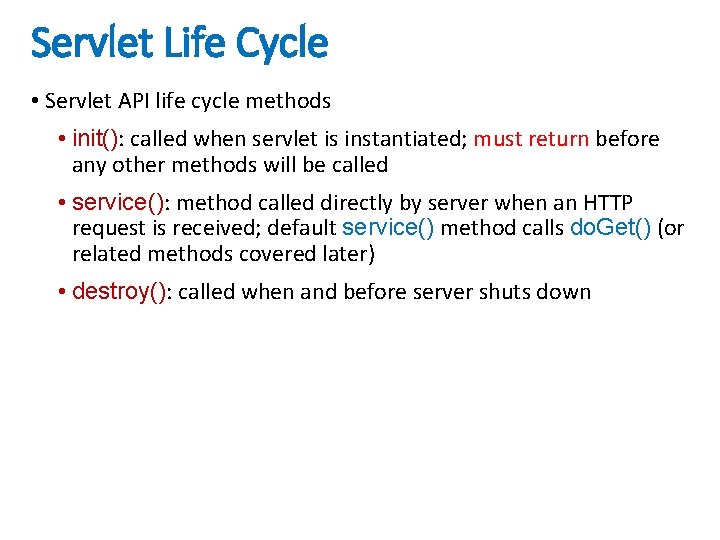
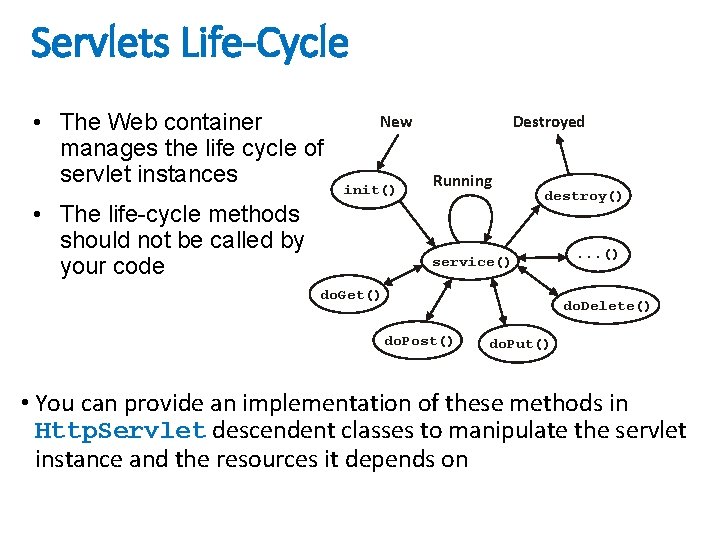
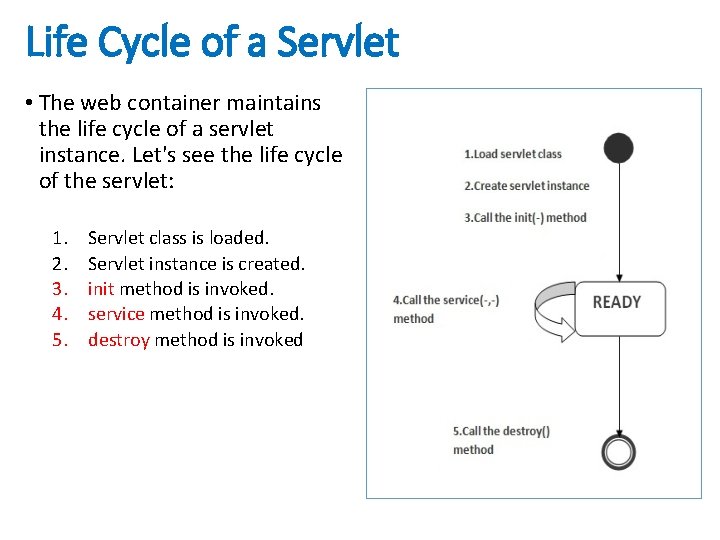
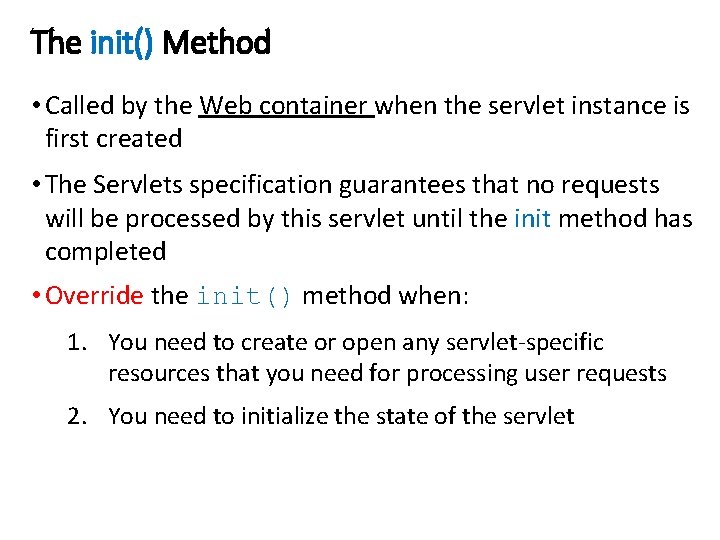
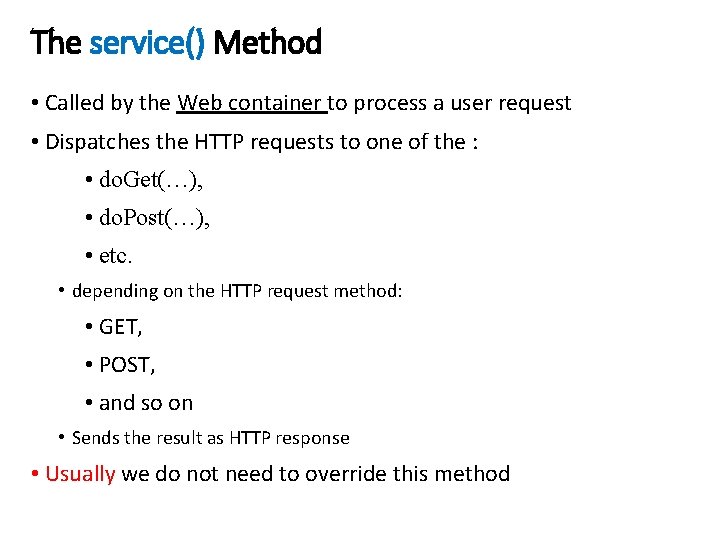
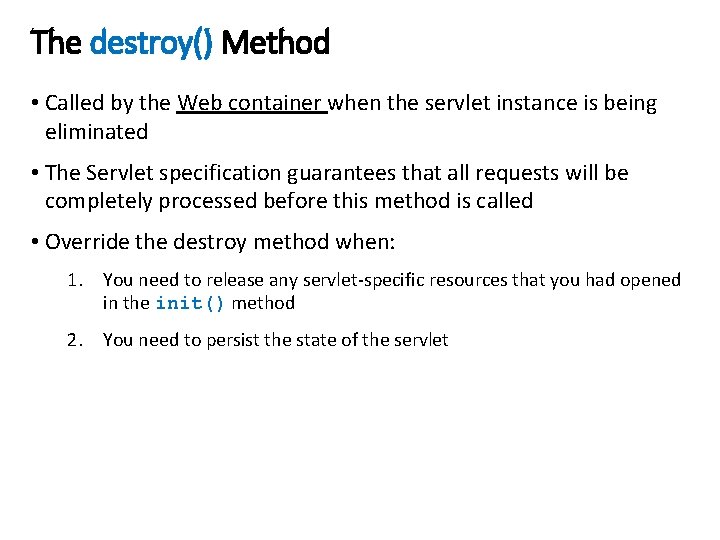
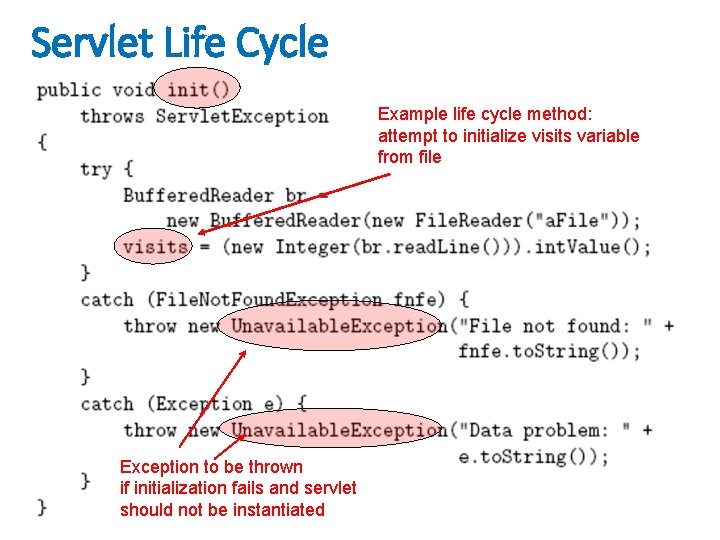
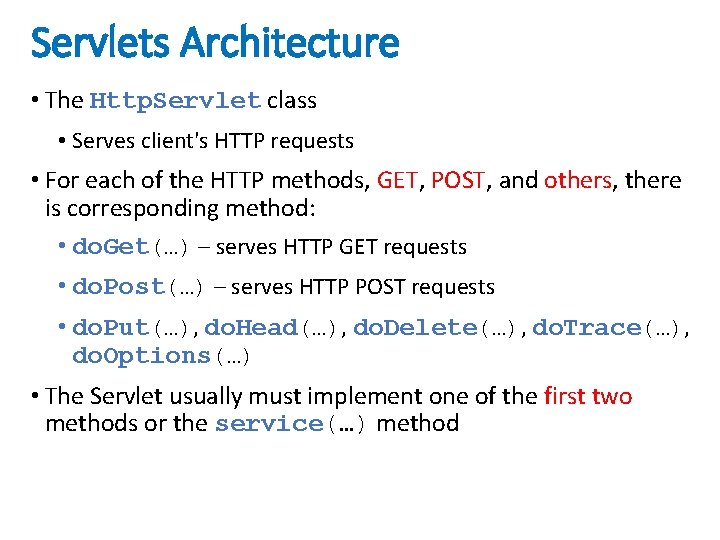
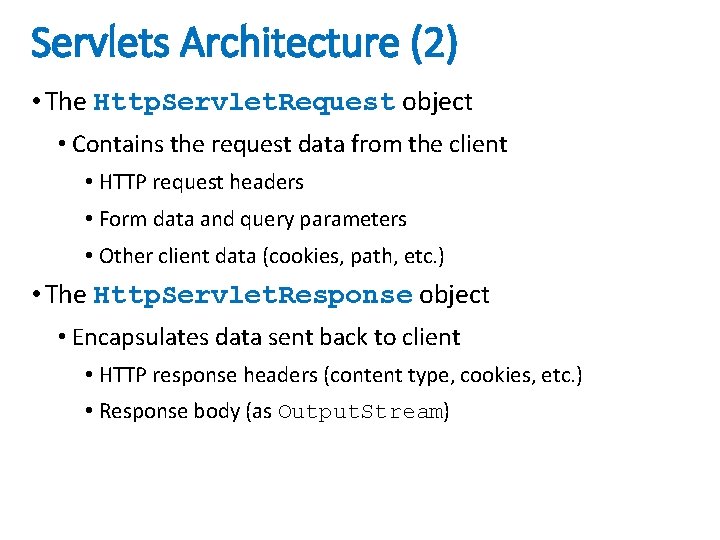
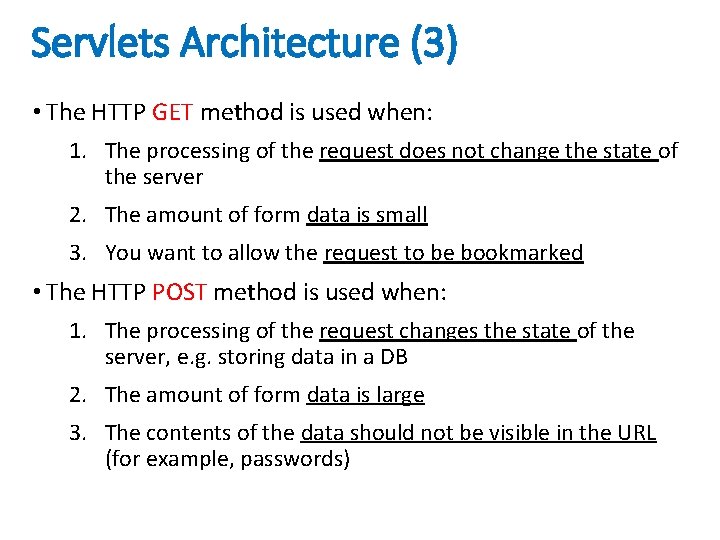
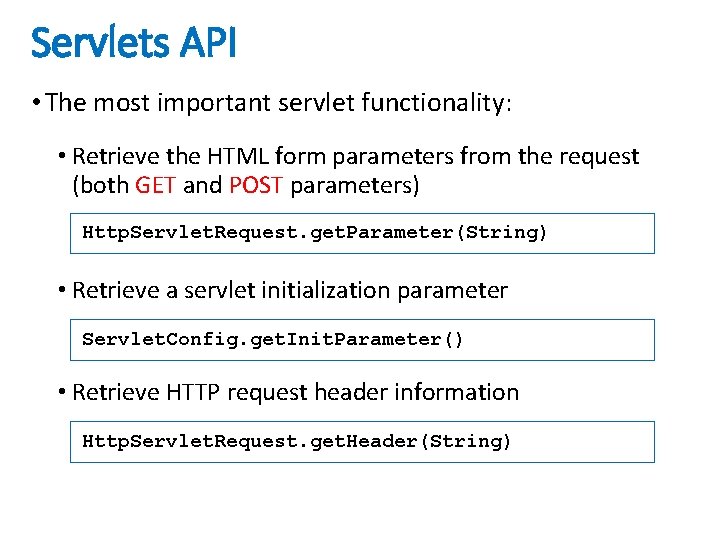
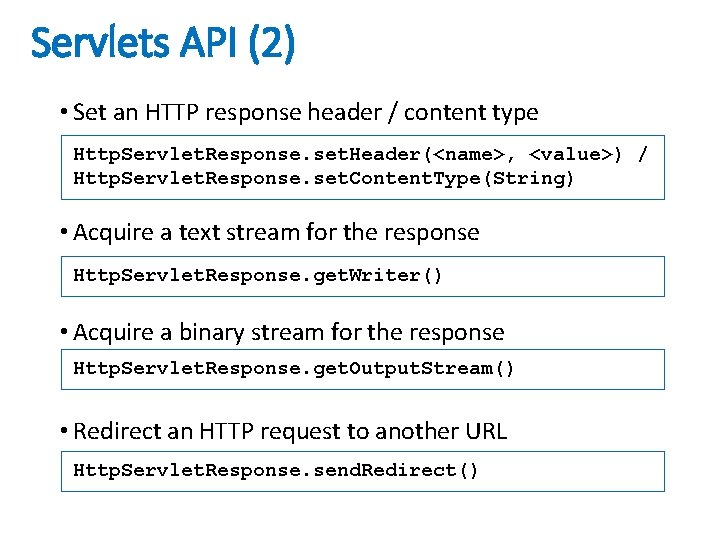
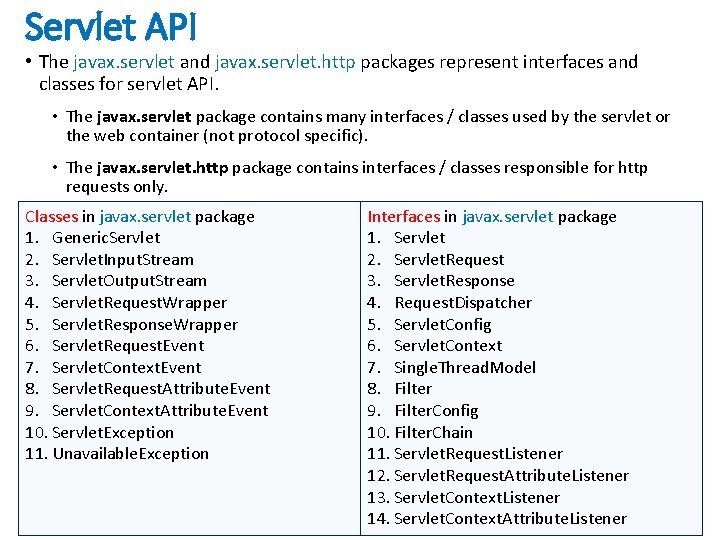
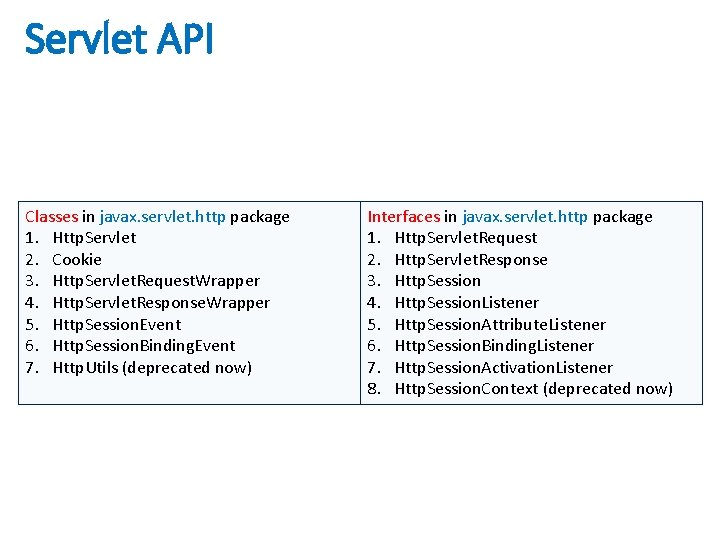
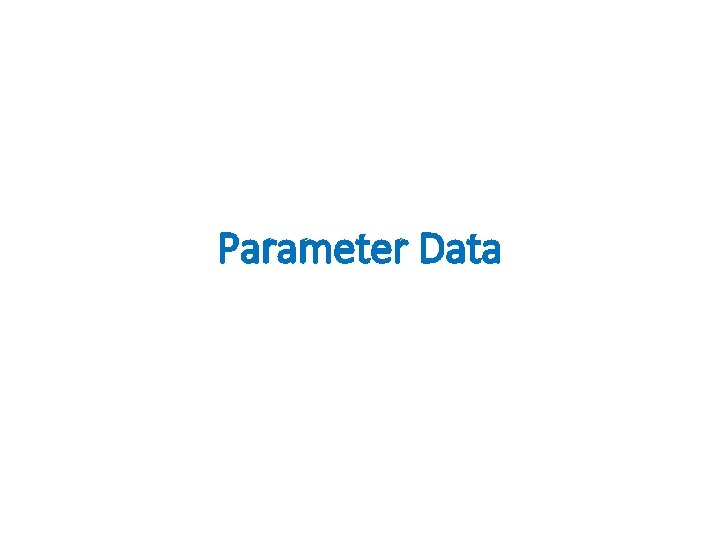
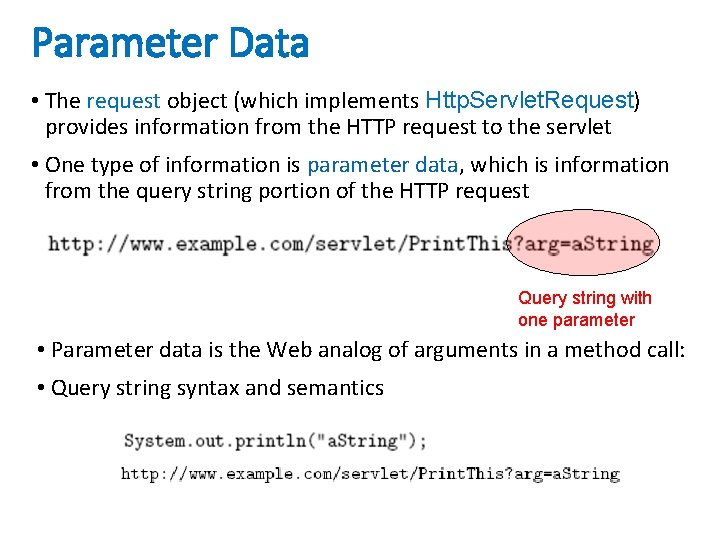
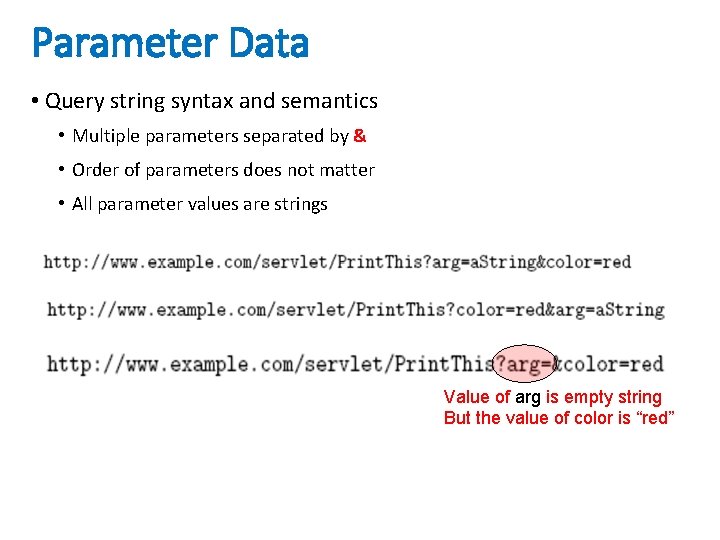
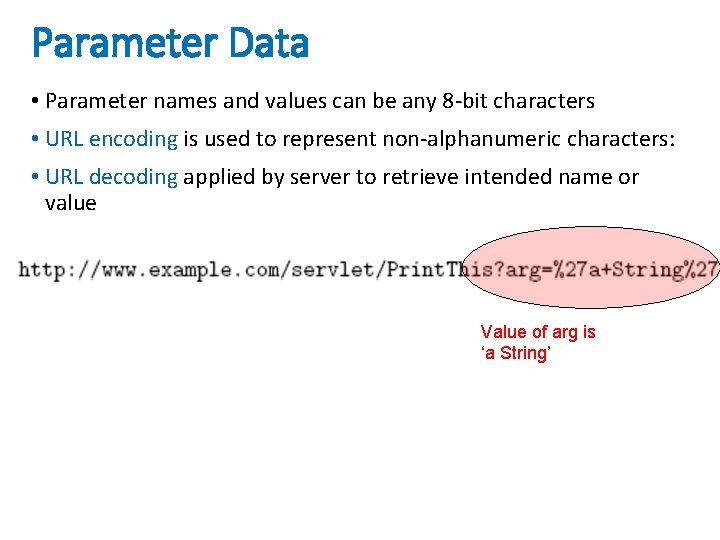
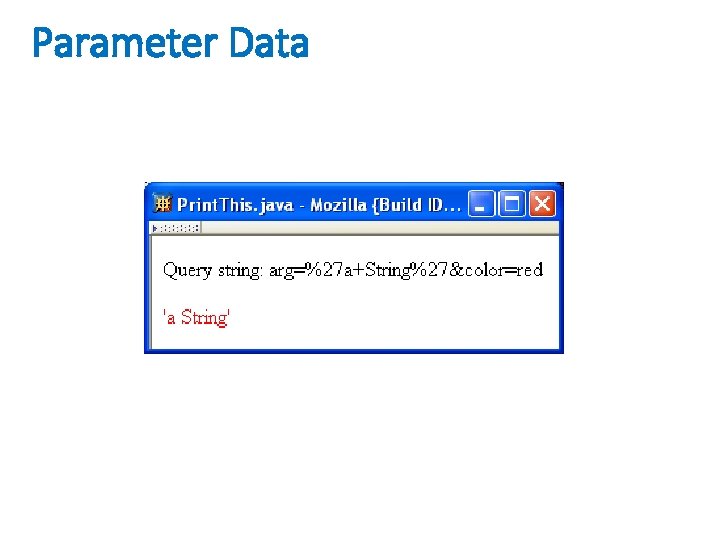
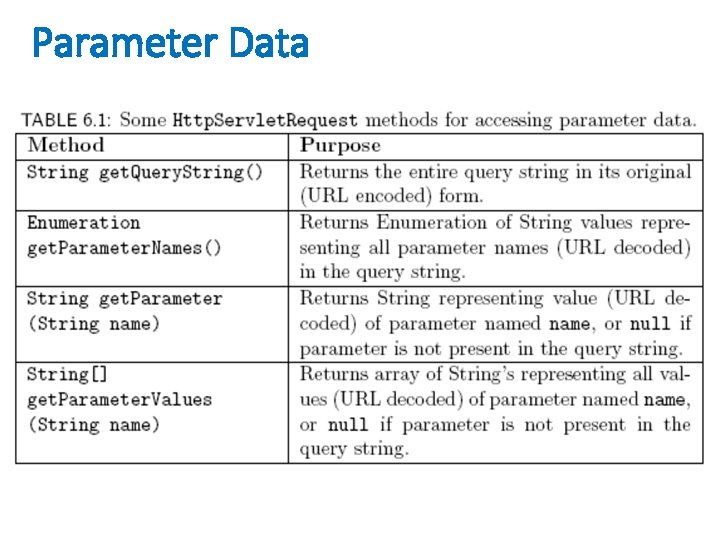
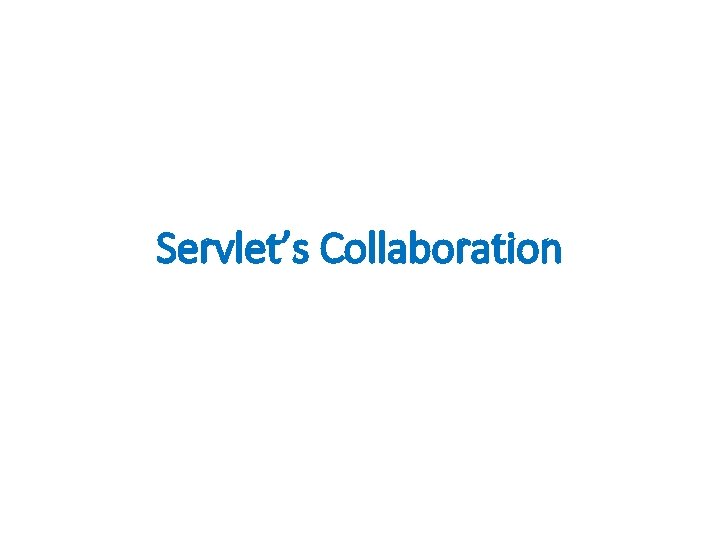
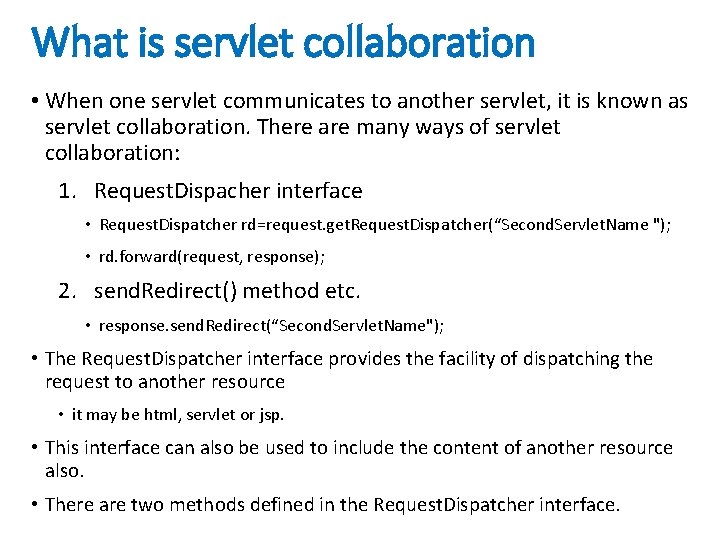
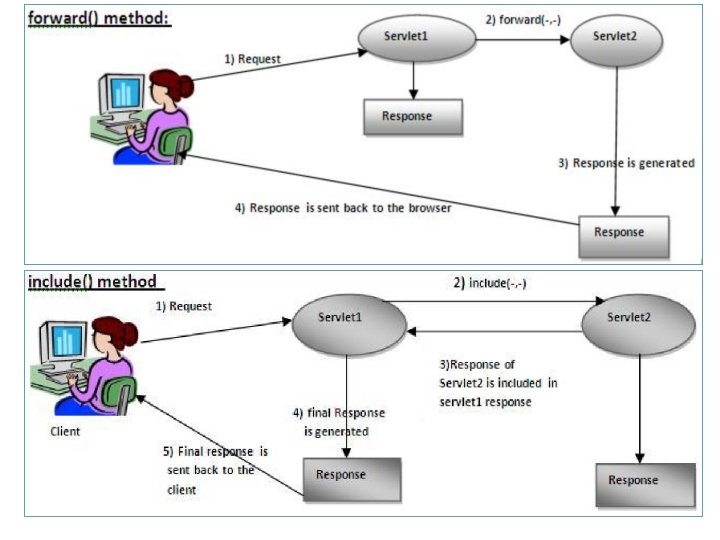
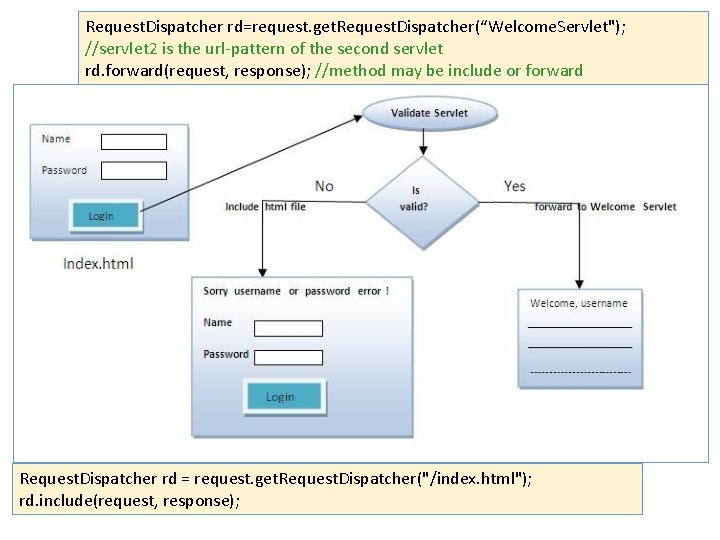
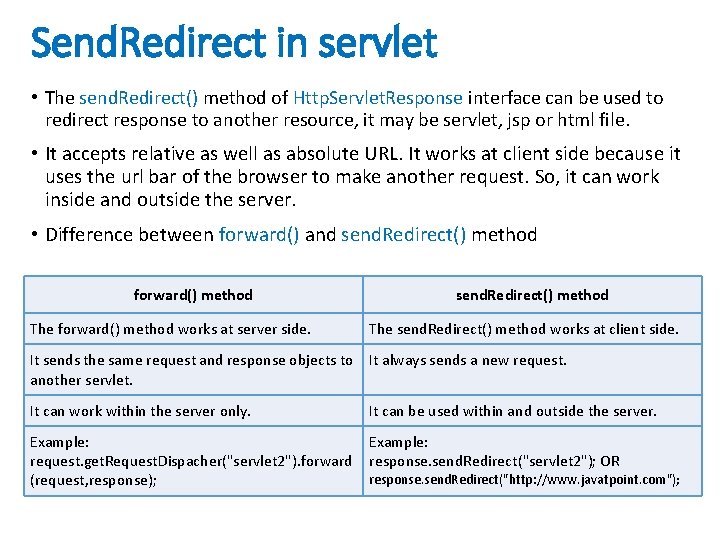
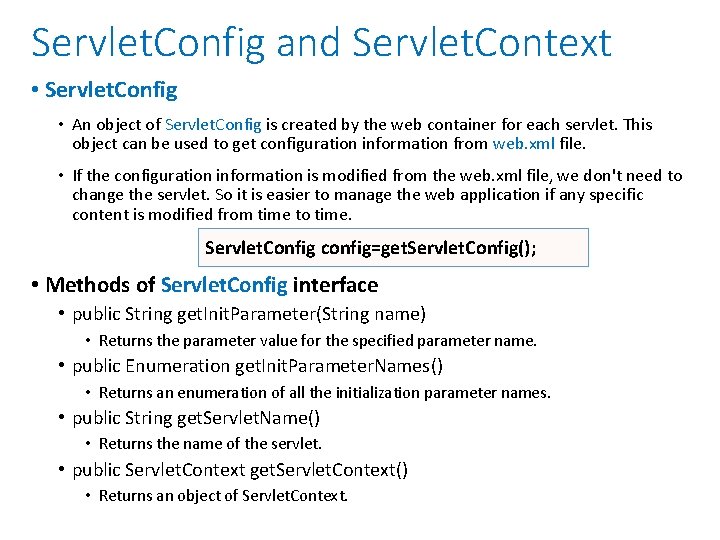
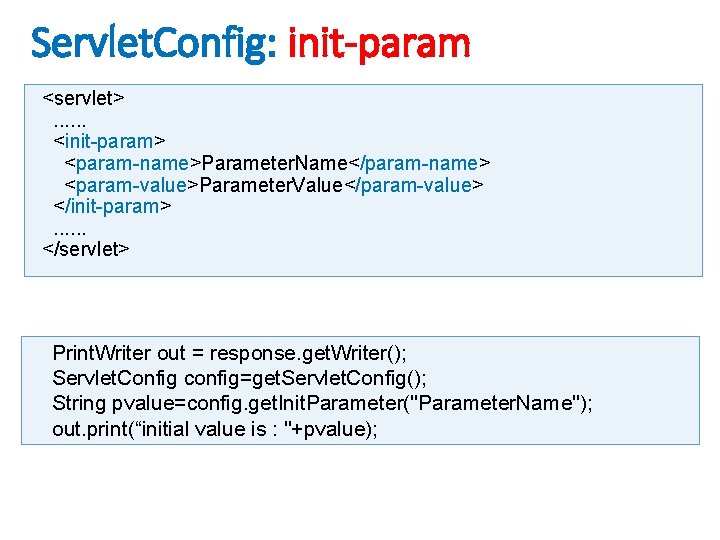
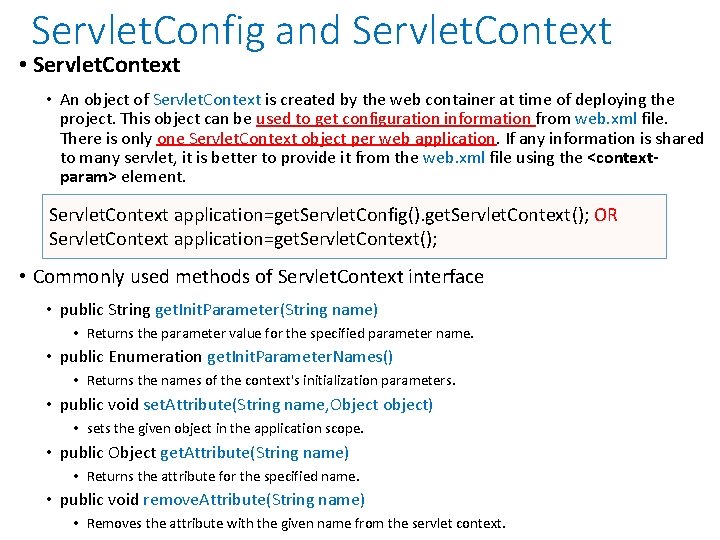
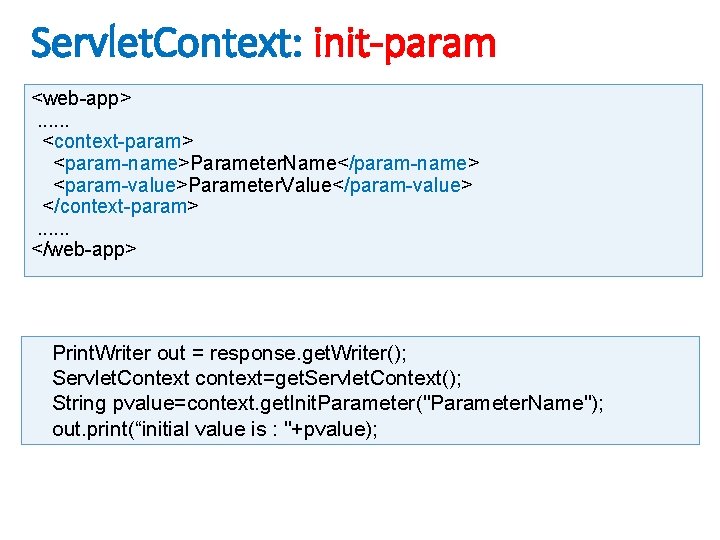
- Slides: 56
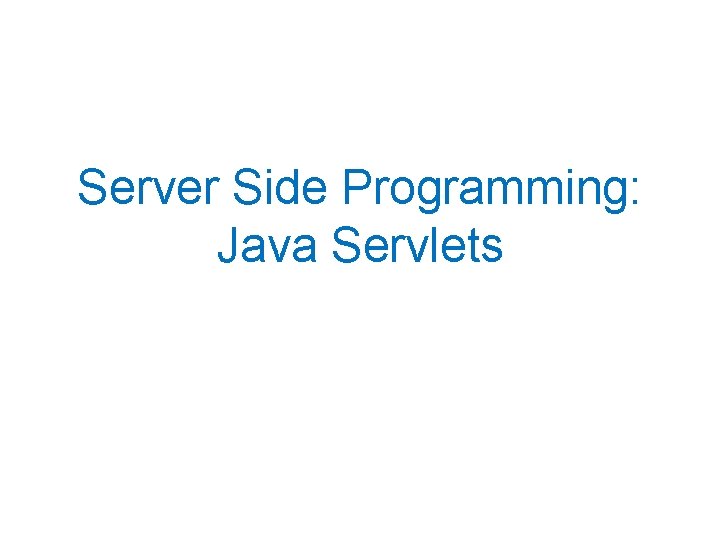
Server Side Programming: Java Servlets
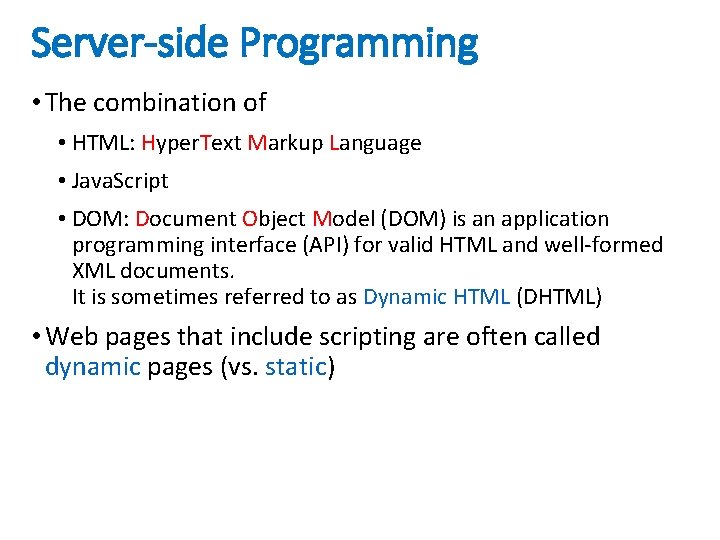
Server-side Programming • The combination of • HTML: Hyper. Text Markup Language • Java. Script • DOM: Document Object Model (DOM) is an application programming interface (API) for valid HTML and well-formed XML documents. It is sometimes referred to as Dynamic HTML (DHTML) • Web pages that include scripting are often called dynamic pages (vs. static)
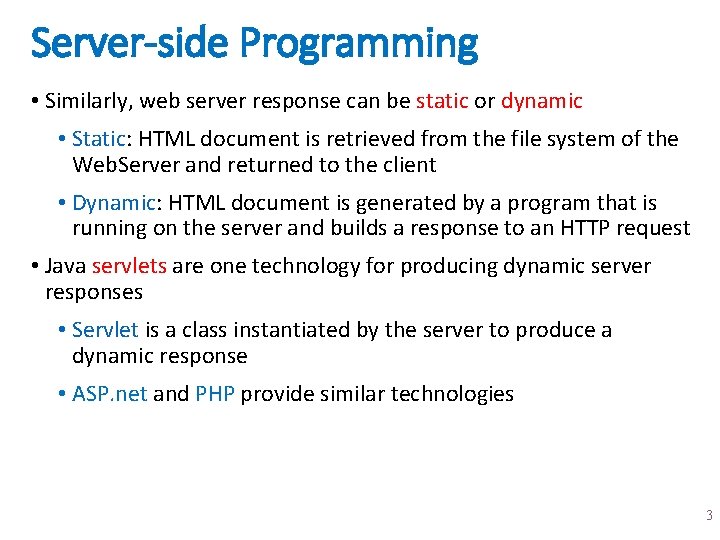
Server-side Programming • Similarly, web server response can be static or dynamic • Static: HTML document is retrieved from the file system of the Web. Server and returned to the client • Dynamic: HTML document is generated by a program that is running on the server and builds a response to an HTTP request • Java servlets are one technology for producing dynamic server responses • Servlet is a class instantiated by the server to produce a dynamic response • ASP. net and PHP provide similar technologies 3
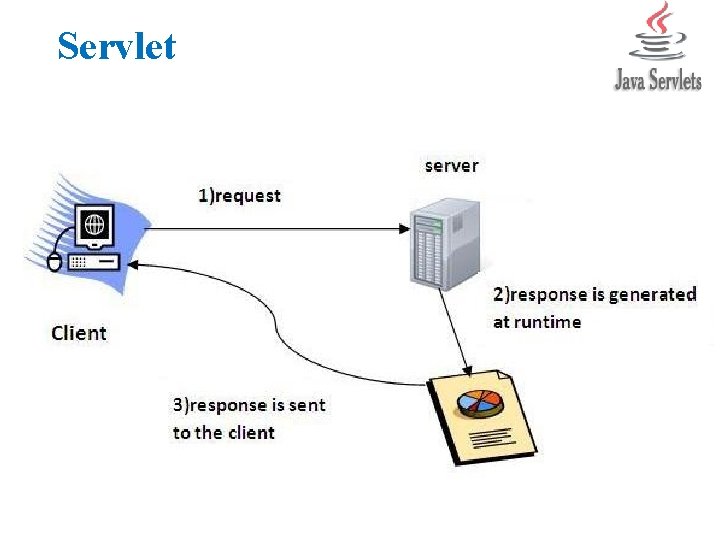
Servlet
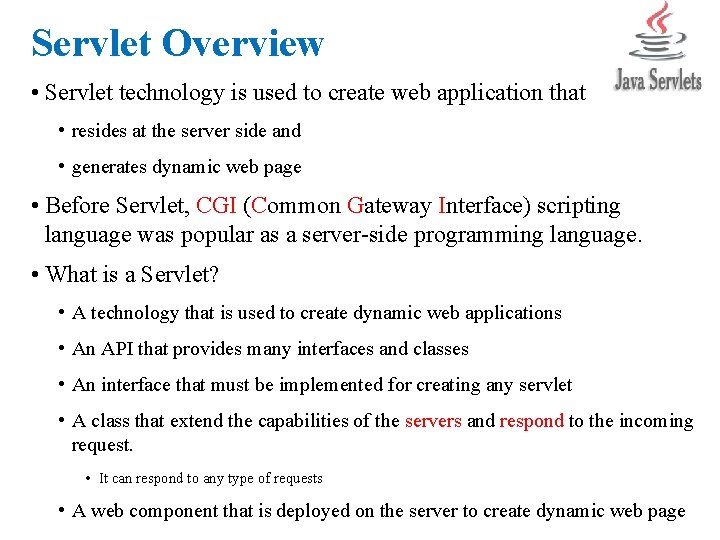
Servlet Overview • Servlet technology is used to create web application that • resides at the server side and • generates dynamic web page • Before Servlet, CGI (Common Gateway Interface) scripting language was popular as a server-side programming language. • What is a Servlet? • A technology that is used to create dynamic web applications • An API that provides many interfaces and classes • An interface that must be implemented for creating any servlet • A class that extend the capabilities of the servers and respond to the incoming request. • It can respond to any type of requests • A web component that is deployed on the server to create dynamic web page
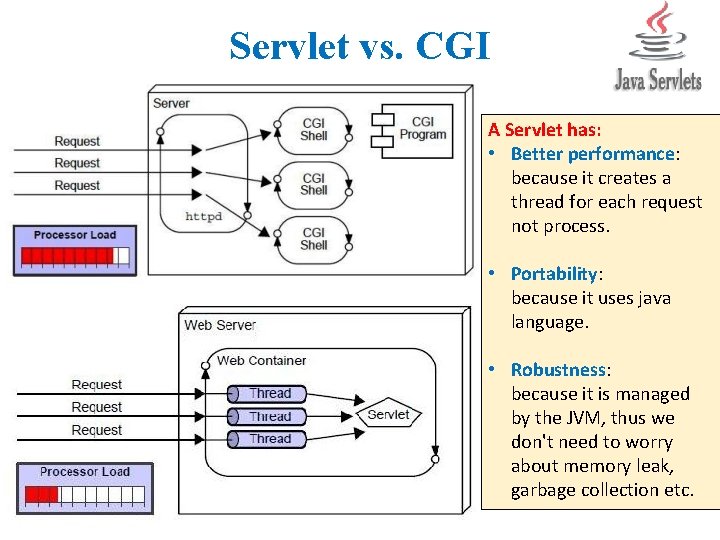
Servlet vs. CGI A Servlet has: • Better performance: because it creates a thread for each request not process. • Portability: because it uses java language. • Robustness: because it is managed by the JVM, thus we don't need to worry about memory leak, garbage collection etc.
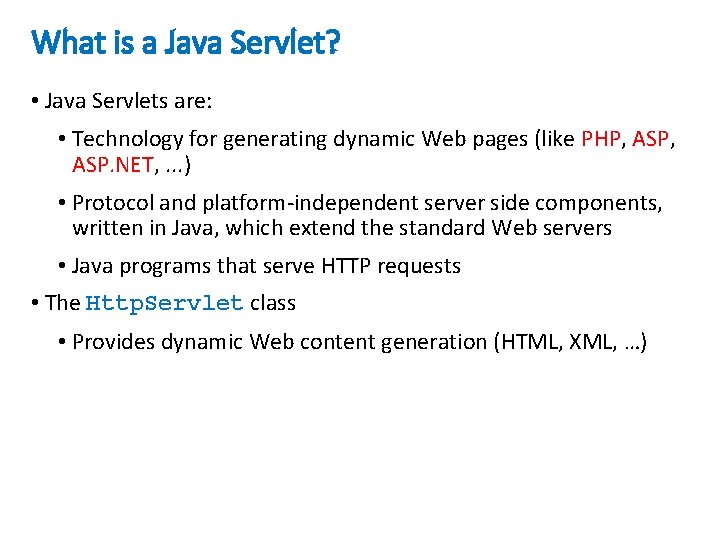
What is a Java Servlet? • Java Servlets are: • Technology for generating dynamic Web pages (like PHP, ASP. NET, . . . ) • Protocol and platform-independent server side components, written in Java, which extend the standard Web servers • Java programs that serve HTTP requests • The Http. Servlet class • Provides dynamic Web content generation (HTML, XML, …)
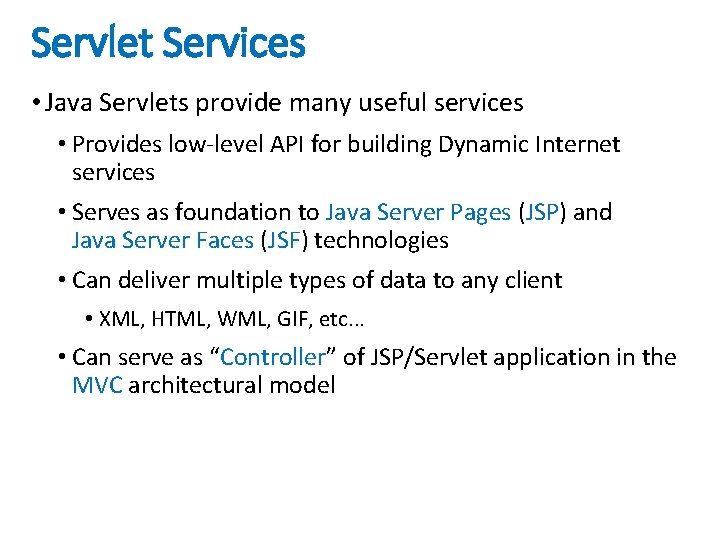
Servlet Services • Java Servlets provide many useful services • Provides low-level API for building Dynamic Internet services • Serves as foundation to Java Server Pages (JSP) and Java Server Faces (JSF) technologies • Can deliver multiple types of data to any client • XML, HTML, WML, GIF, etc. . . • Can serve as “Controller” of JSP/Servlet application in the MVC architectural model
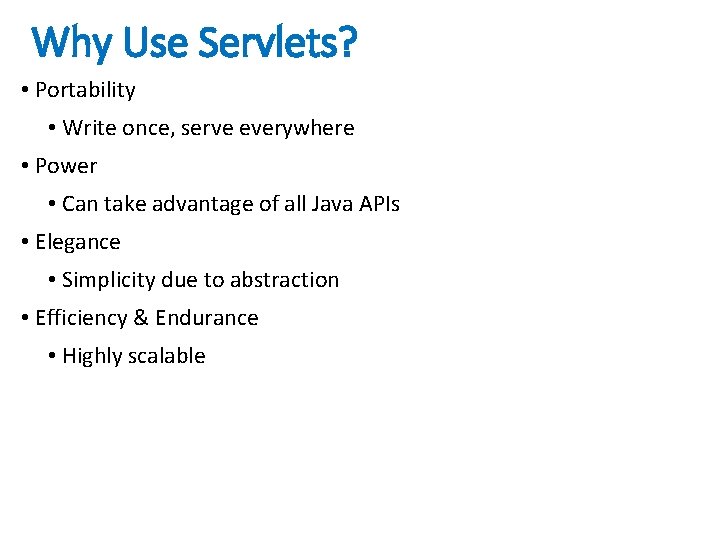
Why Use Servlets? • Portability • Write once, serve everywhere • Power • Can take advantage of all Java APIs • Elegance • Simplicity due to abstraction • Efficiency & Endurance • Highly scalable
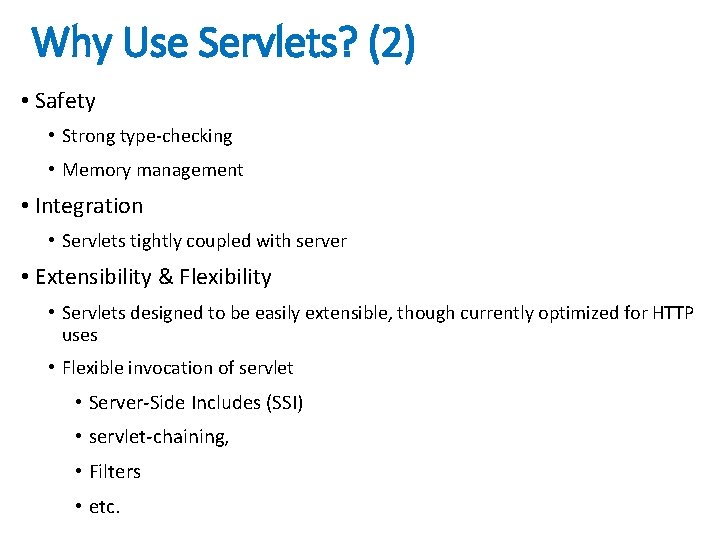
Why Use Servlets? (2) • Safety • Strong type-checking • Memory management • Integration • Servlets tightly coupled with server • Extensibility & Flexibility • Servlets designed to be easily extensible, though currently optimized for HTTP uses • Flexible invocation of servlet • Server-Side Includes (SSI) • servlet-chaining, • Filters • etc.
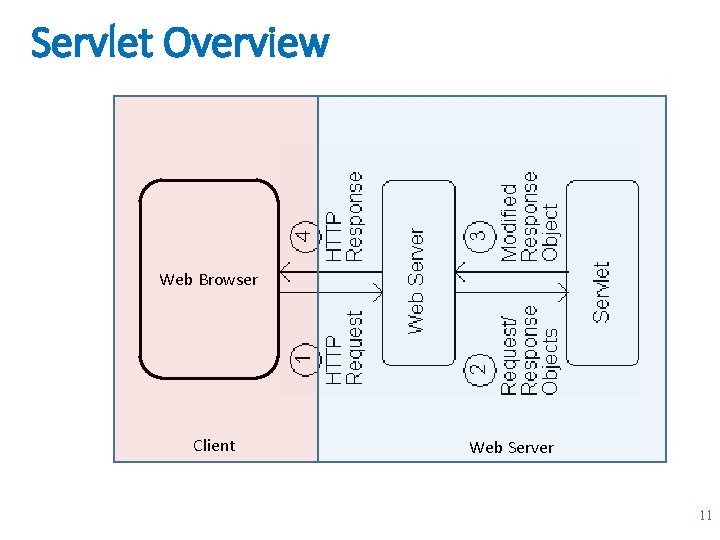
Servlet Overview Web Browser Client Web Server 11
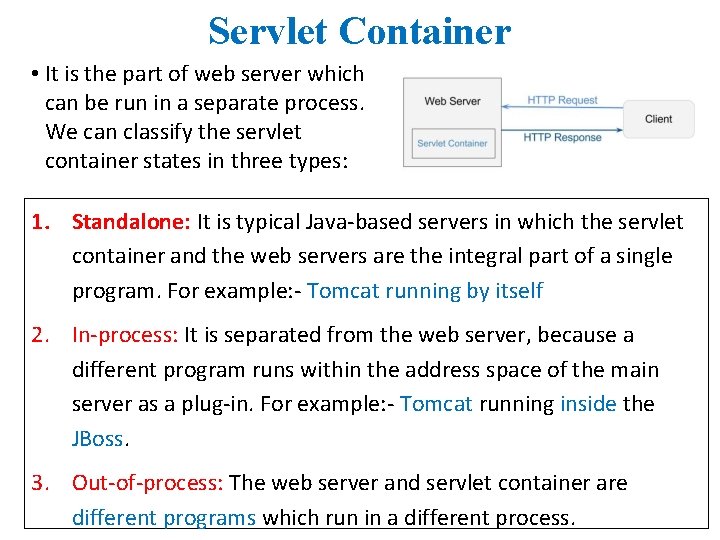
Servlet Container • It is the part of web server which can be run in a separate process. We can classify the servlet container states in three types: 1. Standalone: It is typical Java-based servers in which the servlet container and the web servers are the integral part of a single program. For example: - Tomcat running by itself 2. In-process: It is separated from the web server, because a different program runs within the address space of the main server as a plug-in. For example: - Tomcat running inside the JBoss. 3. Out-of-process: The web server and servlet container are different programs which run in a different process.
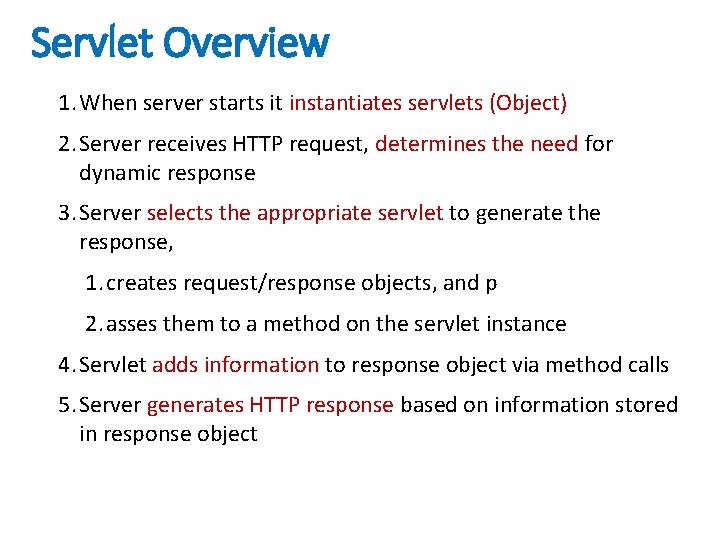
Servlet Overview 1. When server starts it instantiates servlets (Object) 2. Server receives HTTP request, determines the need for dynamic response 3. Server selects the appropriate servlet to generate the response, 1. creates request/response objects, and p 2. asses them to a method on the servlet instance 4. Servlet adds information to response object via method calls 5. Server generates HTTP response based on information stored in response object
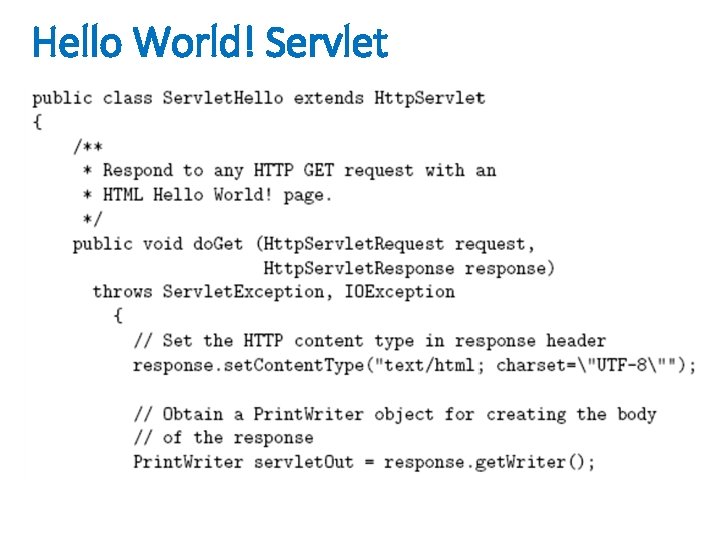
Hello World! Servlet
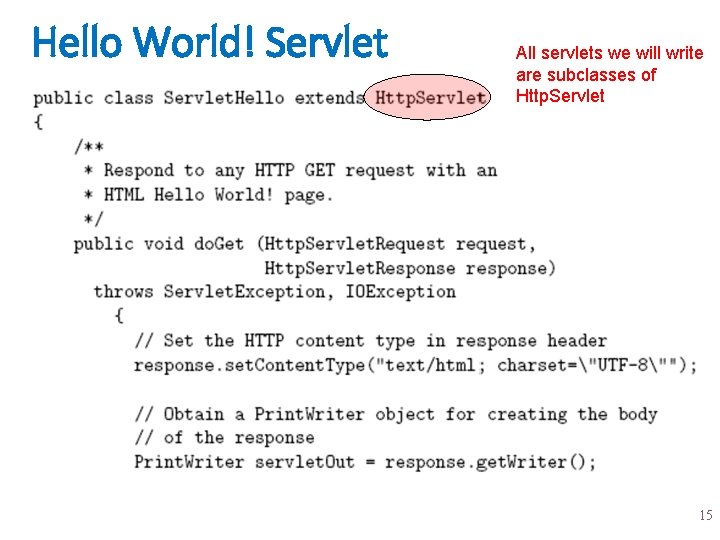
Hello World! Servlet All servlets we will write are subclasses of Http. Servlet 15
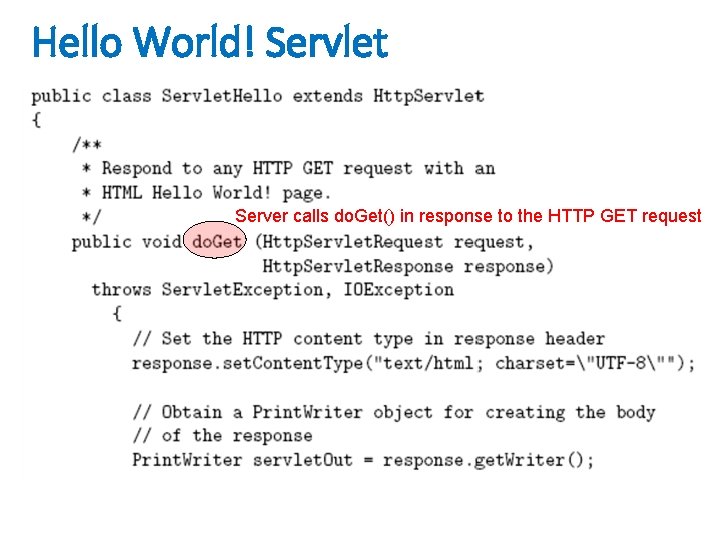
Hello World! Servlet Server calls do. Get() in response to the HTTP GET request
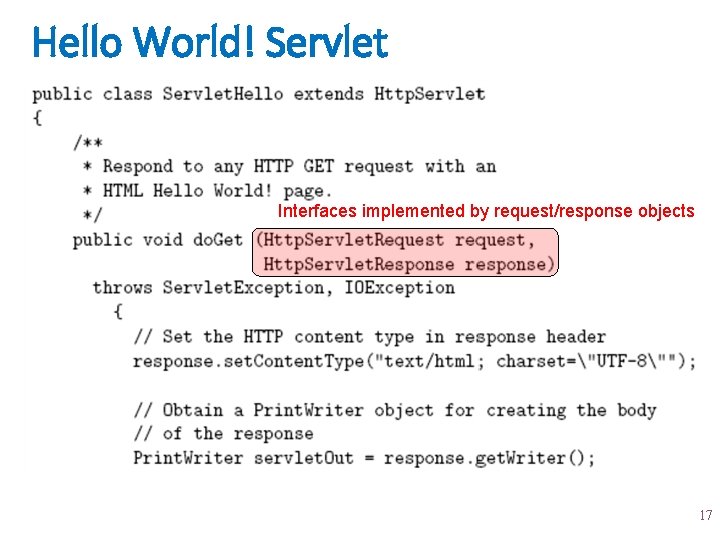
Hello World! Servlet Interfaces implemented by request/response objects 17
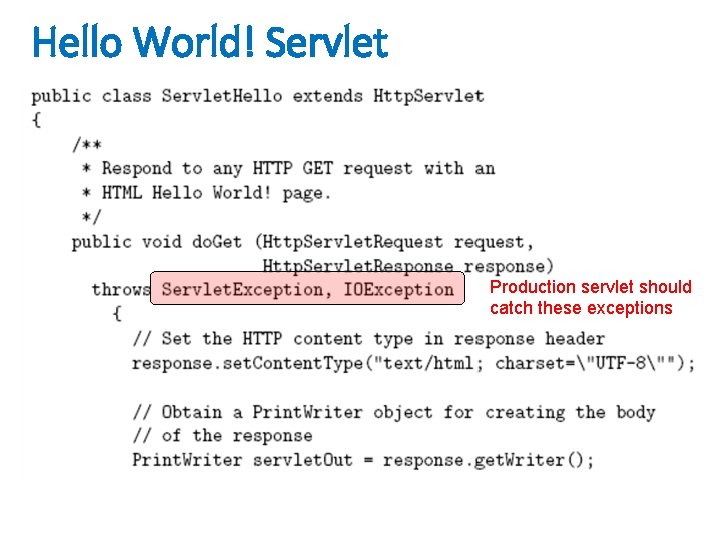
Hello World! Servlet Production servlet should catch these exceptions
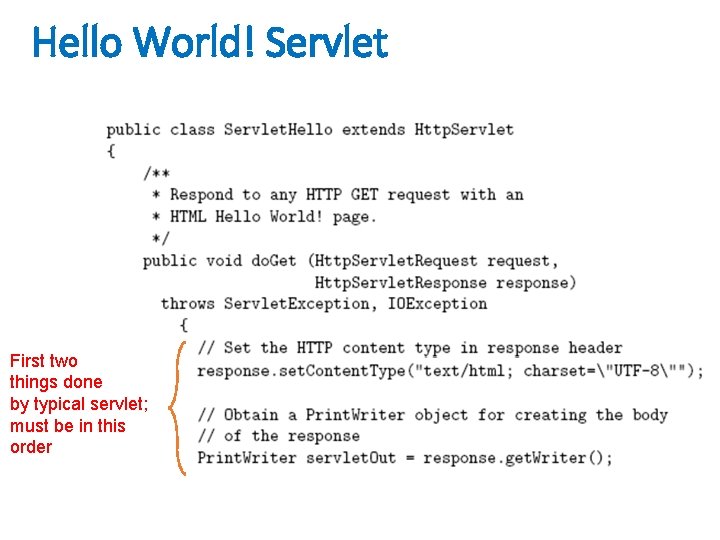
Hello World! Servlet First two things done by typical servlet; must be in this order
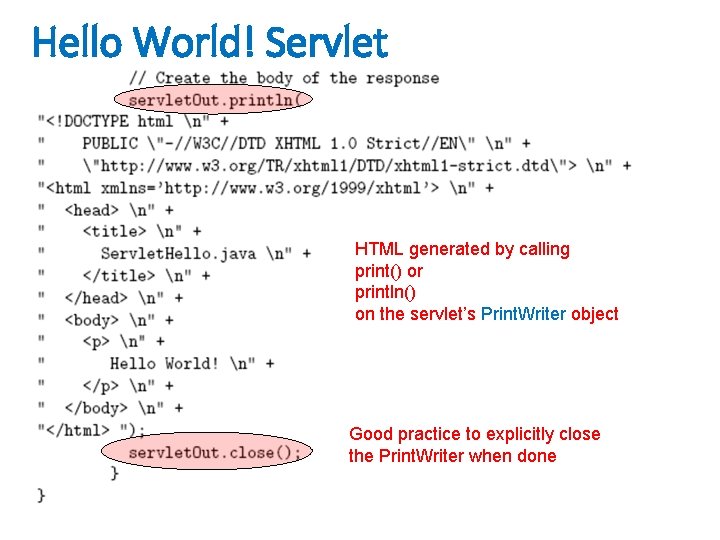
Hello World! Servlet HTML generated by calling print() or println() on the servlet’s Print. Writer object Good practice to explicitly close the Print. Writer when done
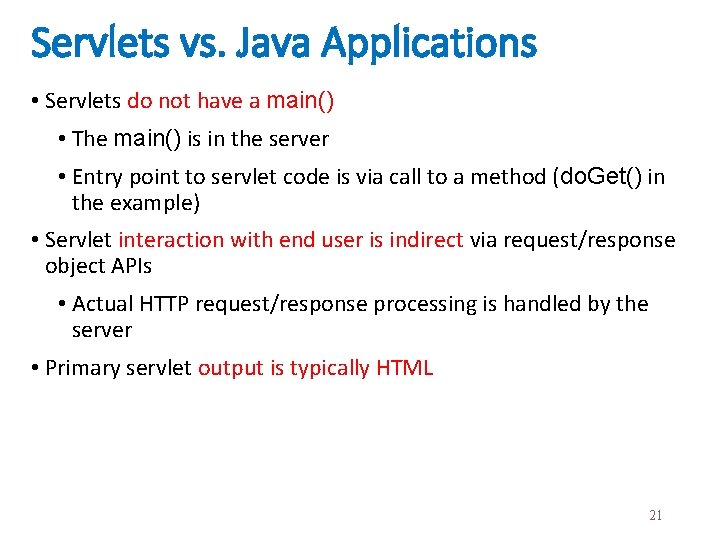
Servlets vs. Java Applications • Servlets do not have a main() • The main() is in the server • Entry point to servlet code is via call to a method (do. Get() in the example) • Servlet interaction with end user is indirect via request/response object APIs • Actual HTTP request/response processing is handled by the server • Primary servlet output is typically HTML 21
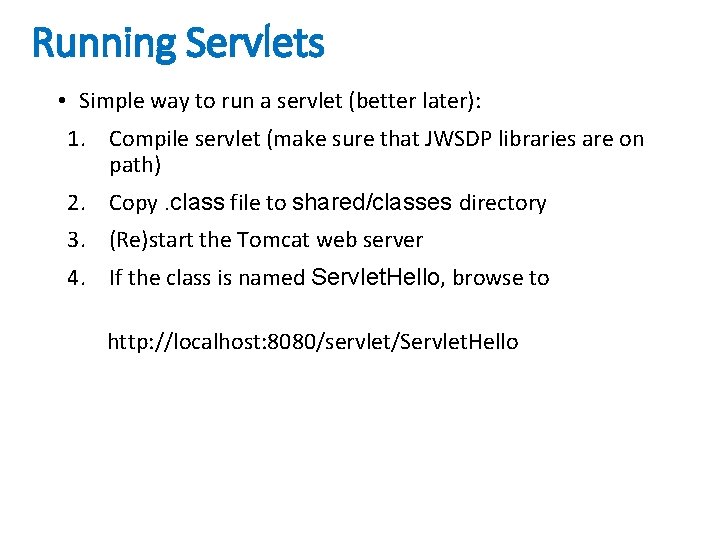
Running Servlets • Simple way to run a servlet (better later): 1. Compile servlet (make sure that JWSDP libraries are on path) 2. Copy. class file to shared/classes directory 3. (Re)start the Tomcat web server 4. If the class is named Servlet. Hello, browse to http: //localhost: 8080/servlet/Servlet. Hello
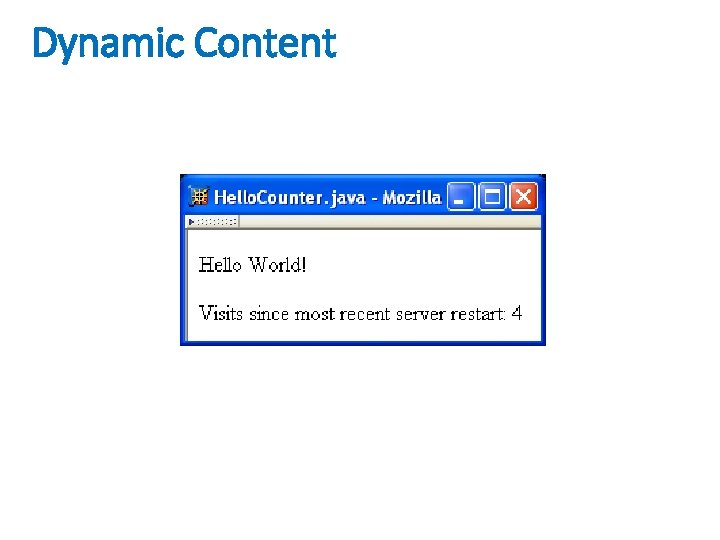
Dynamic Content
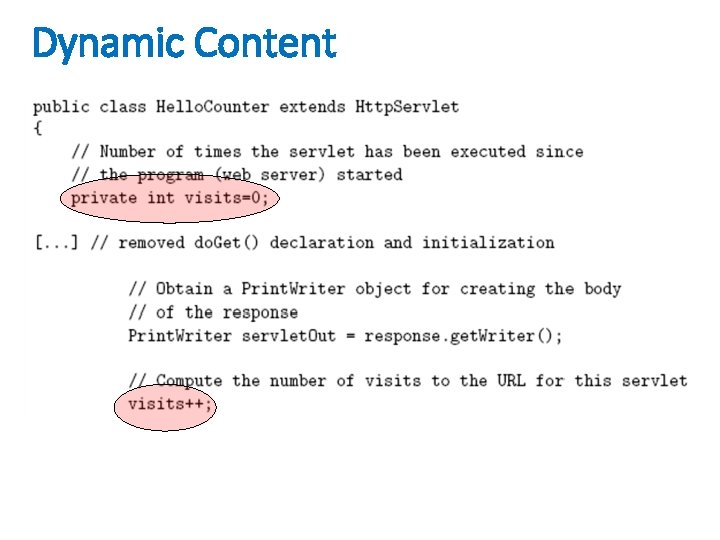
Dynamic Content
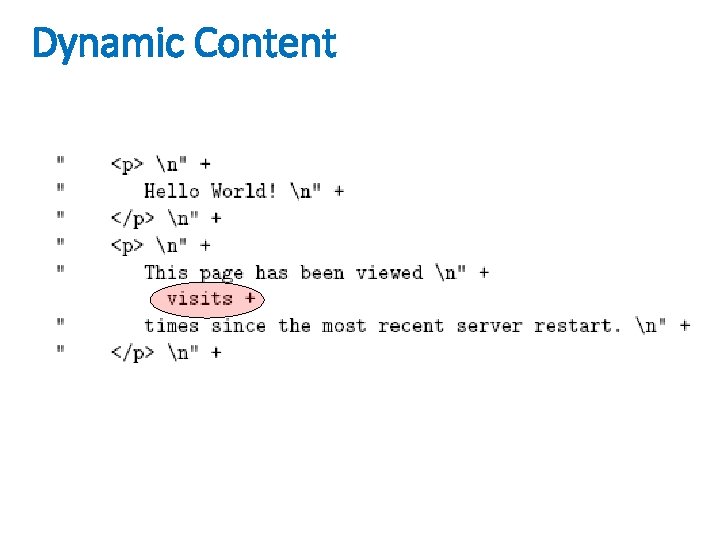
Dynamic Content
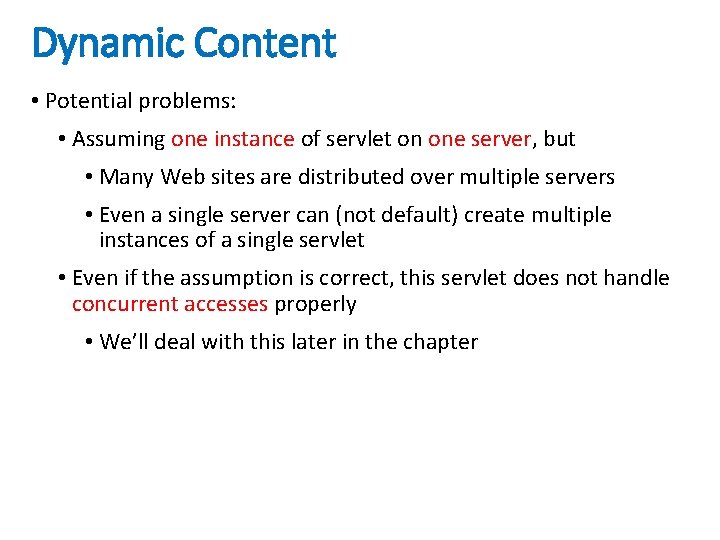
Dynamic Content • Potential problems: • Assuming one instance of servlet on one server, but • Many Web sites are distributed over multiple servers • Even a single server can (not default) create multiple instances of a single servlet • Even if the assumption is correct, this servlet does not handle concurrent accesses properly • We’ll deal with this later in the chapter
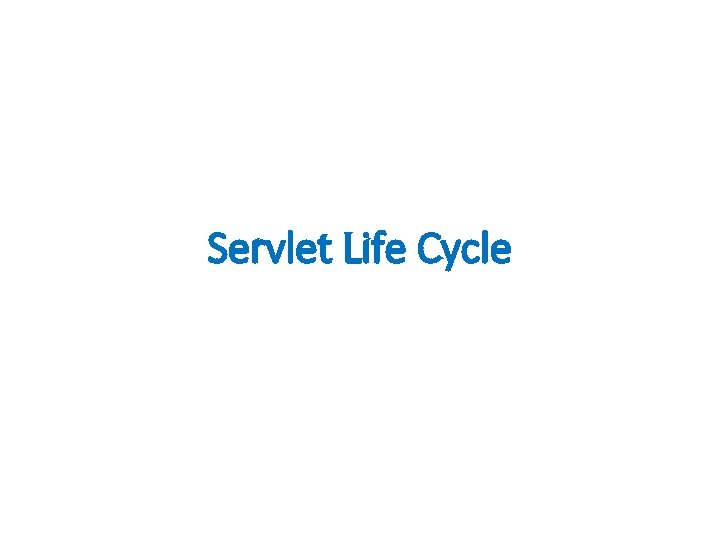
Servlet Life Cycle
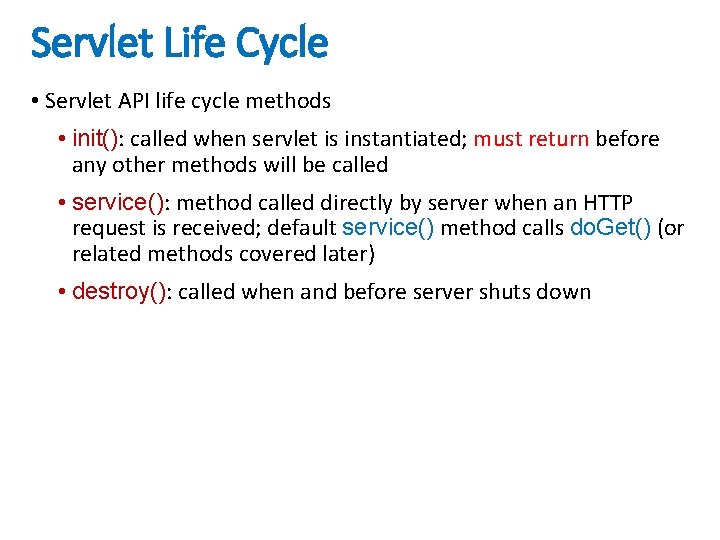
Servlet Life Cycle • Servlet API life cycle methods • init(): called when servlet is instantiated; must return before any other methods will be called • service(): method called directly by server when an HTTP request is received; default service() method calls do. Get() (or related methods covered later) • destroy(): called when and before server shuts down
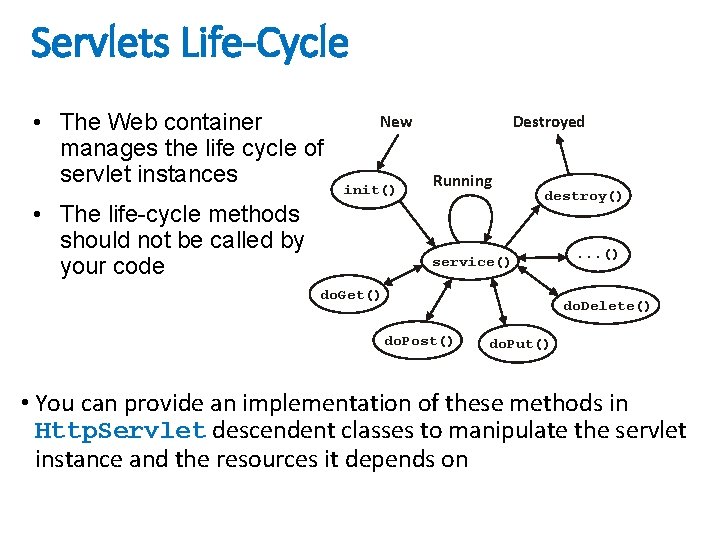
Servlets Life-Cycle • The Web container manages the life cycle of servlet instances New init() • The life-cycle methods should not be called by your code Destroyed Running destroy() service() do. Get() . . . () do. Delete() do. Post() do. Put() • You can provide an implementation of these methods in Http. Servlet descendent classes to manipulate the servlet instance and the resources it depends on
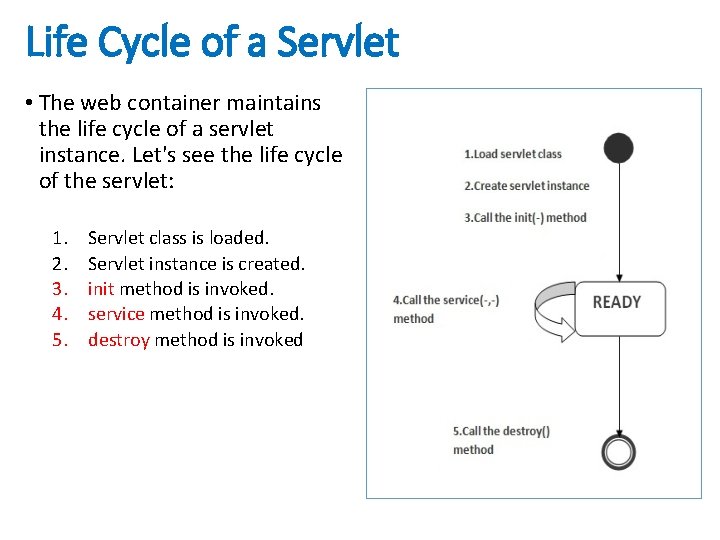
Life Cycle of a Servlet • The web container maintains the life cycle of a servlet instance. Let's see the life cycle of the servlet: 1. 2. 3. 4. 5. Servlet class is loaded. Servlet instance is created. init method is invoked. service method is invoked. destroy method is invoked
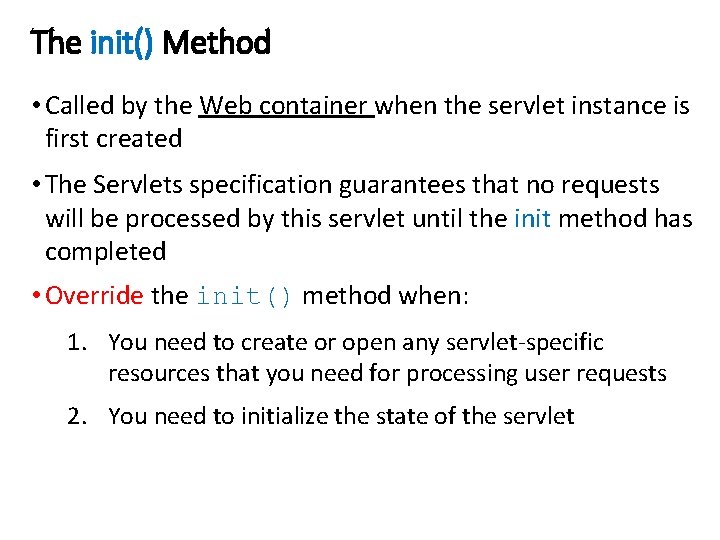
The init() Method • Called by the Web container when the servlet instance is first created • The Servlets specification guarantees that no requests will be processed by this servlet until the init method has completed • Override the init() method when: 1. You need to create or open any servlet-specific resources that you need for processing user requests 2. You need to initialize the state of the servlet
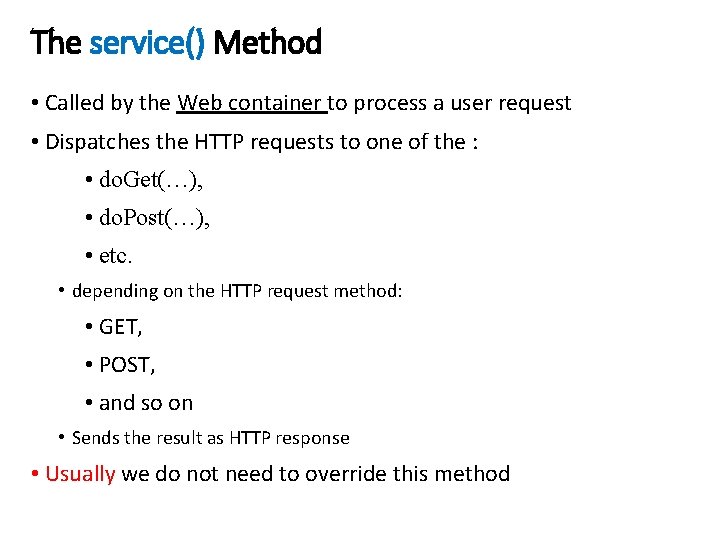
The service() Method • Called by the Web container to process a user request • Dispatches the HTTP requests to one of the : • do. Get(…), • do. Post(…), • etc. • depending on the HTTP request method: • GET, • POST, • and so on • Sends the result as HTTP response • Usually we do not need to override this method
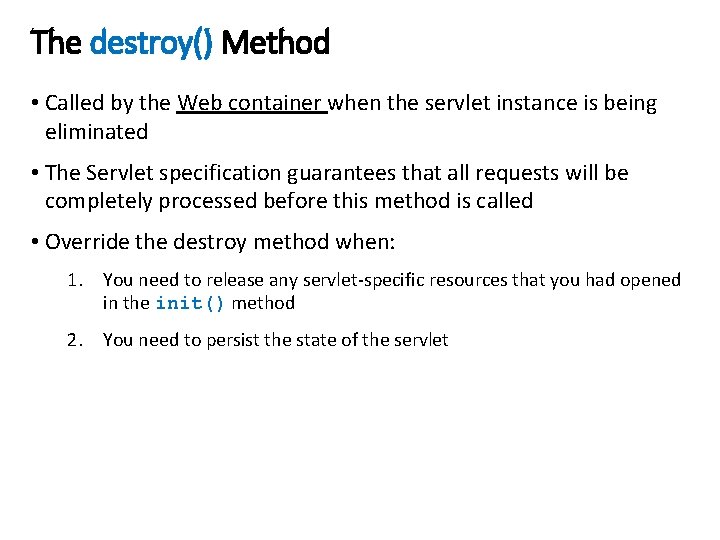
The destroy() Method • Called by the Web container when the servlet instance is being eliminated • The Servlet specification guarantees that all requests will be completely processed before this method is called • Override the destroy method when: 1. You need to release any servlet-specific resources that you had opened in the init() method 2. You need to persist the state of the servlet
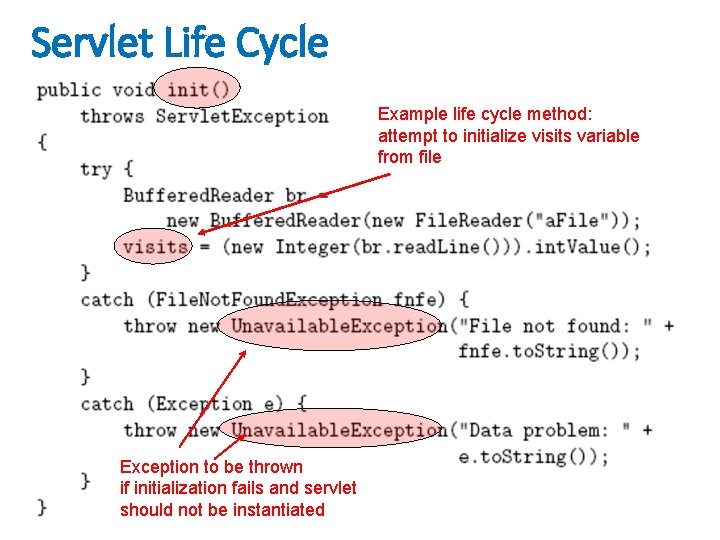
Servlet Life Cycle Example life cycle method: attempt to initialize visits variable from file Exception to be thrown if initialization fails and servlet should not be instantiated
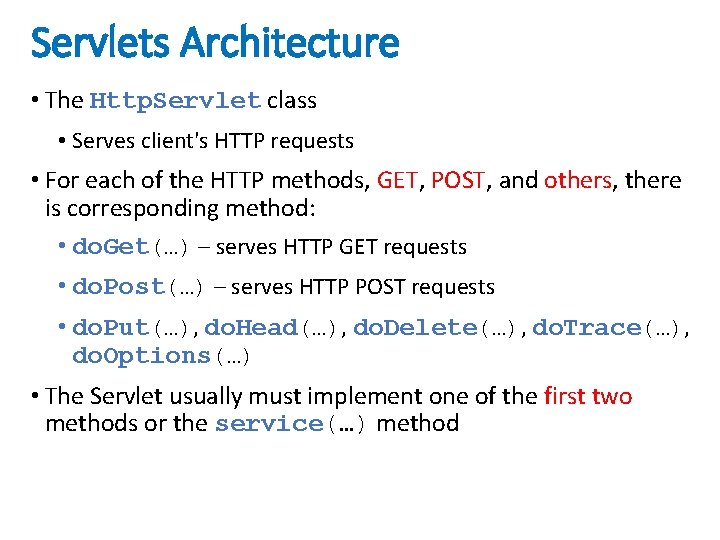
Servlets Architecture • The Http. Servlet class • Serves client's HTTP requests • For each of the HTTP methods, GET, POST, and others, there is corresponding method: • do. Get(…) – serves HTTP GET requests • do. Post(…) – serves HTTP POST requests • do. Put(…), do. Head(…), do. Delete(…), do. Trace(…), do. Options(…) • The Servlet usually must implement one of the first two methods or the service(…) method
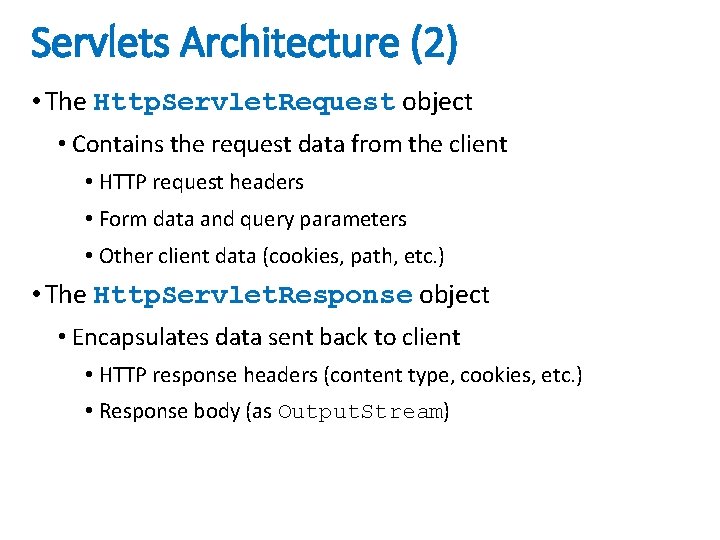
Servlets Architecture (2) • The Http. Servlet. Request object • Contains the request data from the client • HTTP request headers • Form data and query parameters • Other client data (cookies, path, etc. ) • The Http. Servlet. Response object • Encapsulates data sent back to client • HTTP response headers (content type, cookies, etc. ) • Response body (as Output. Stream)
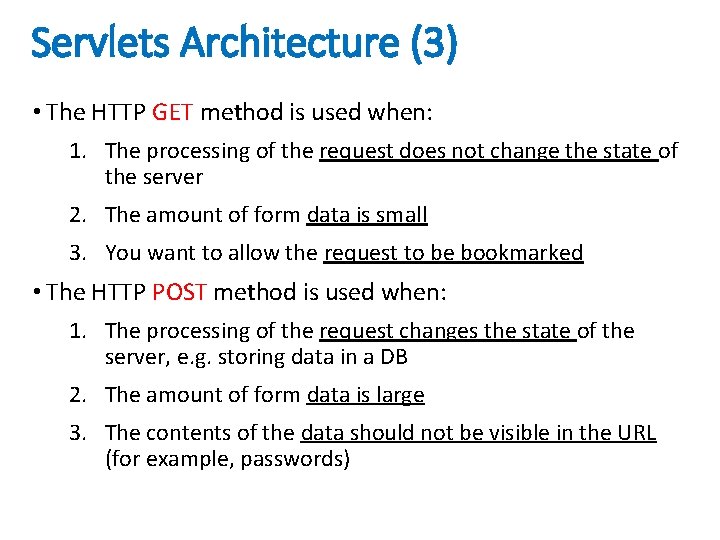
Servlets Architecture (3) • The HTTP GET method is used when: 1. The processing of the request does not change the state of the server 2. The amount of form data is small 3. You want to allow the request to be bookmarked • The HTTP POST method is used when: 1. The processing of the request changes the state of the server, e. g. storing data in a DB 2. The amount of form data is large 3. The contents of the data should not be visible in the URL (for example, passwords)
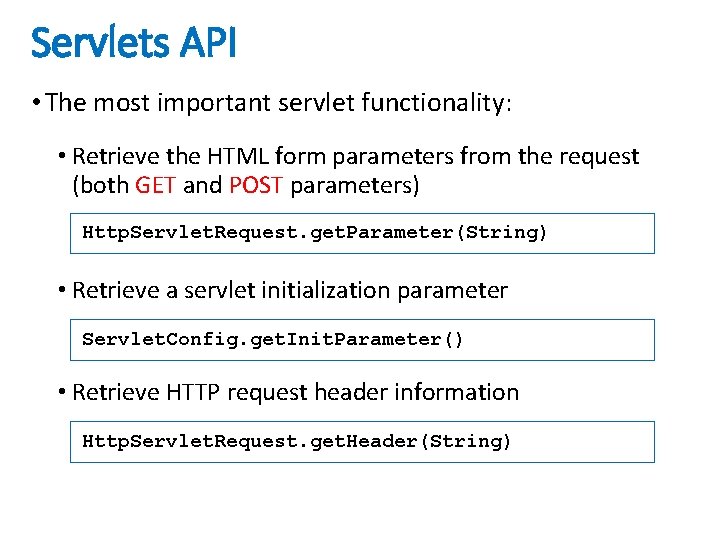
Servlets API • The most important servlet functionality: • Retrieve the HTML form parameters from the request (both GET and POST parameters) Http. Servlet. Request. get. Parameter(String) • Retrieve a servlet initialization parameter Servlet. Config. get. Init. Parameter() • Retrieve HTTP request header information Http. Servlet. Request. get. Header(String)
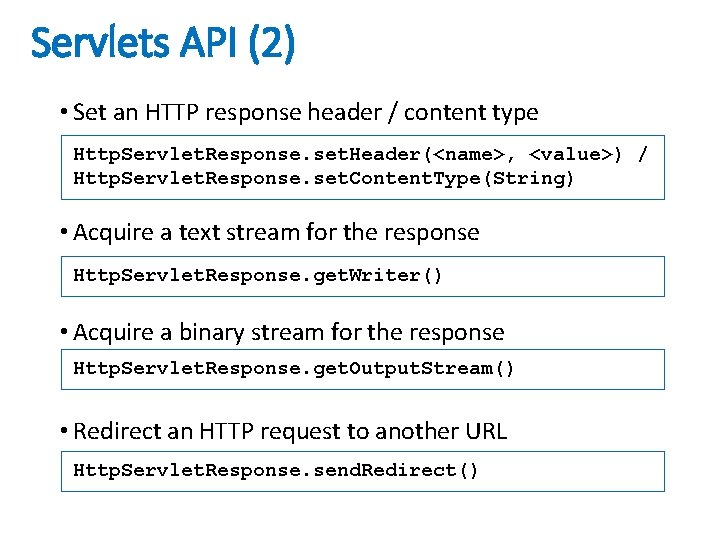
Servlets API (2) • Set an HTTP response header / content type Http. Servlet. Response. set. Header(<name>, <value>) / Http. Servlet. Response. set. Content. Type(String) • Acquire a text stream for the response Http. Servlet. Response. get. Writer() • Acquire a binary stream for the response Http. Servlet. Response. get. Output. Stream() • Redirect an HTTP request to another URL Http. Servlet. Response. send. Redirect()
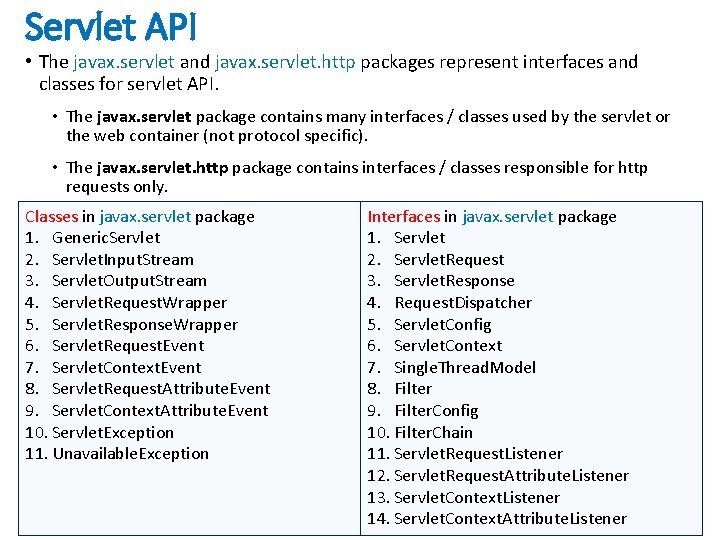
Servlet API • The javax. servlet and javax. servlet. http packages represent interfaces and classes for servlet API. • The javax. servlet package contains many interfaces / classes used by the servlet or the web container (not protocol specific). • The javax. servlet. http package contains interfaces / classes responsible for http requests only. Classes in javax. servlet package 1. Generic. Servlet 2. Servlet. Input. Stream 3. Servlet. Output. Stream 4. Servlet. Request. Wrapper 5. Servlet. Response. Wrapper 6. Servlet. Request. Event 7. Servlet. Context. Event 8. Servlet. Request. Attribute. Event 9. Servlet. Context. Attribute. Event 10. Servlet. Exception 11. Unavailable. Exception Interfaces in javax. servlet package 1. Servlet 2. Servlet. Request 3. Servlet. Response 4. Request. Dispatcher 5. Servlet. Config 6. Servlet. Context 7. Single. Thread. Model 8. Filter 9. Filter. Config 10. Filter. Chain 11. Servlet. Request. Listener 12. Servlet. Request. Attribute. Listener 13. Servlet. Context. Listener 14. Servlet. Context. Attribute. Listener
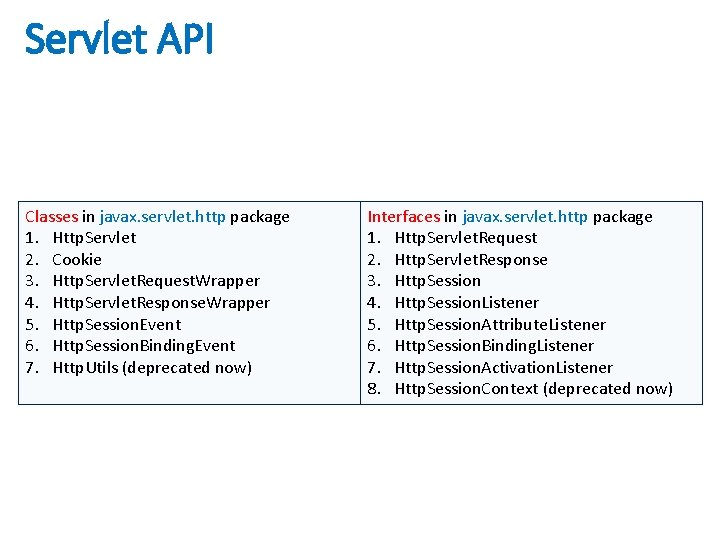
Servlet API Classes in javax. servlet. http package 1. Http. Servlet 2. Cookie 3. Http. Servlet. Request. Wrapper 4. Http. Servlet. Response. Wrapper 5. Http. Session. Event 6. Http. Session. Binding. Event 7. Http. Utils (deprecated now) Interfaces in javax. servlet. http package 1. Http. Servlet. Request 2. Http. Servlet. Response 3. Http. Session 4. Http. Session. Listener 5. Http. Session. Attribute. Listener 6. Http. Session. Binding. Listener 7. Http. Session. Activation. Listener 8. Http. Session. Context (deprecated now)
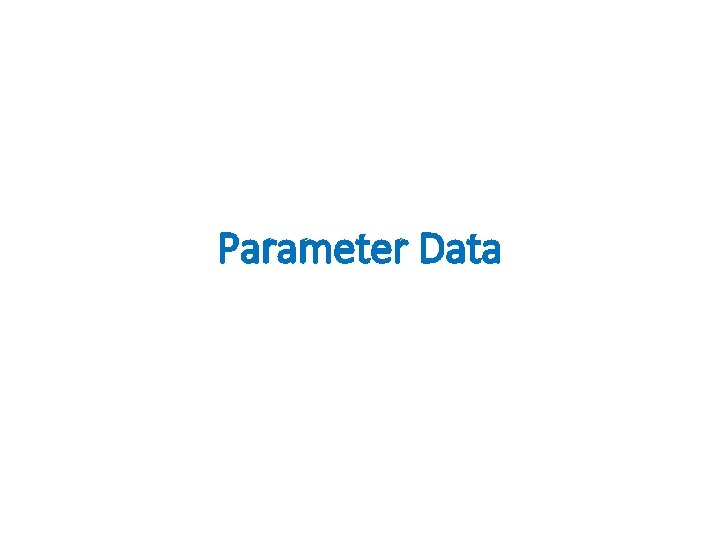
Parameter Data
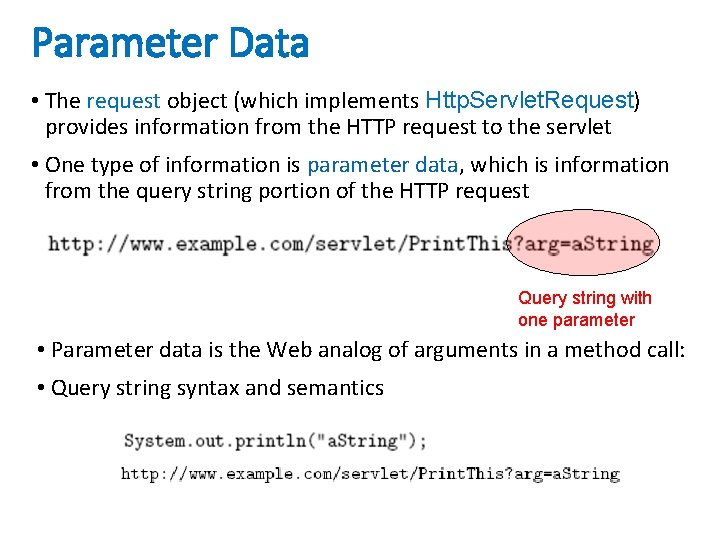
Parameter Data • The request object (which implements Http. Servlet. Request) provides information from the HTTP request to the servlet • One type of information is parameter data, which is information from the query string portion of the HTTP request Query string with one parameter • Parameter data is the Web analog of arguments in a method call: • Query string syntax and semantics
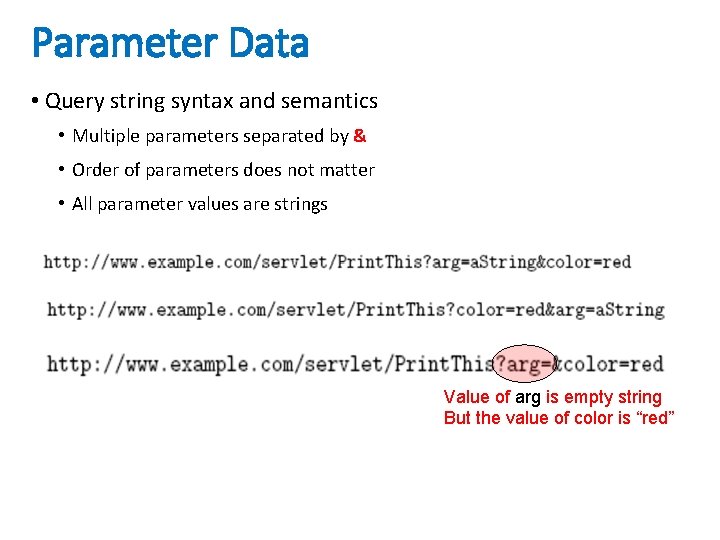
Parameter Data • Query string syntax and semantics • Multiple parameters separated by & • Order of parameters does not matter • All parameter values are strings Value of arg is empty string But the value of color is “red”
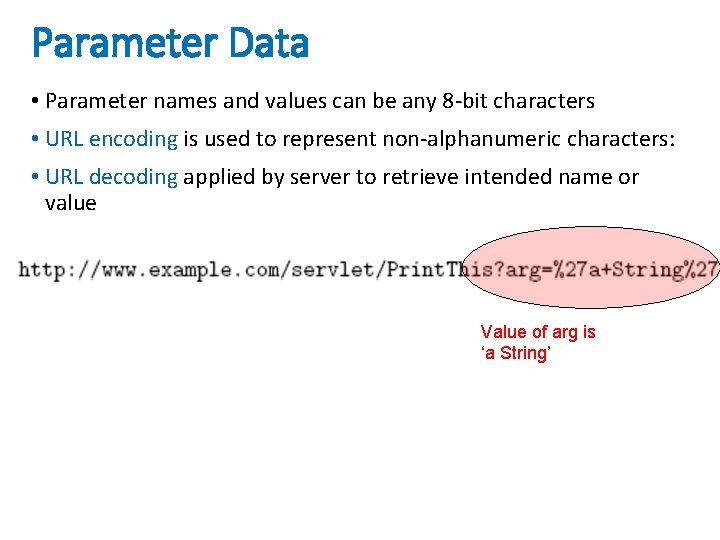
Parameter Data • Parameter names and values can be any 8 -bit characters • URL encoding is used to represent non-alphanumeric characters: • URL decoding applied by server to retrieve intended name or value Value of arg is ‘a String’
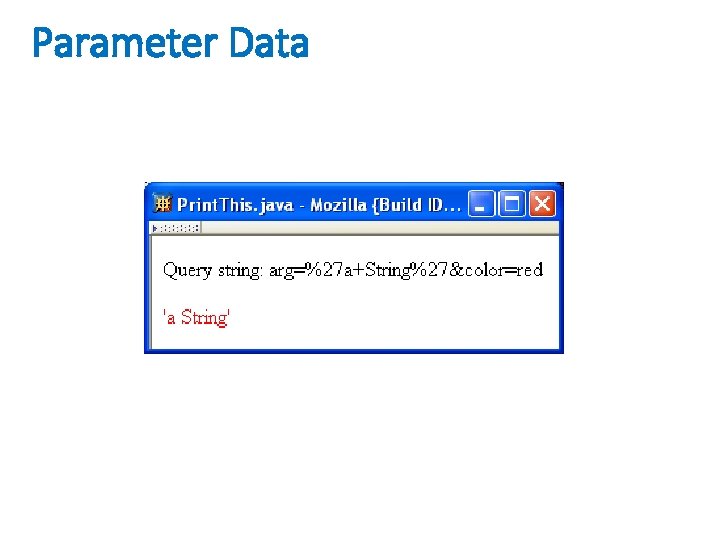
Parameter Data
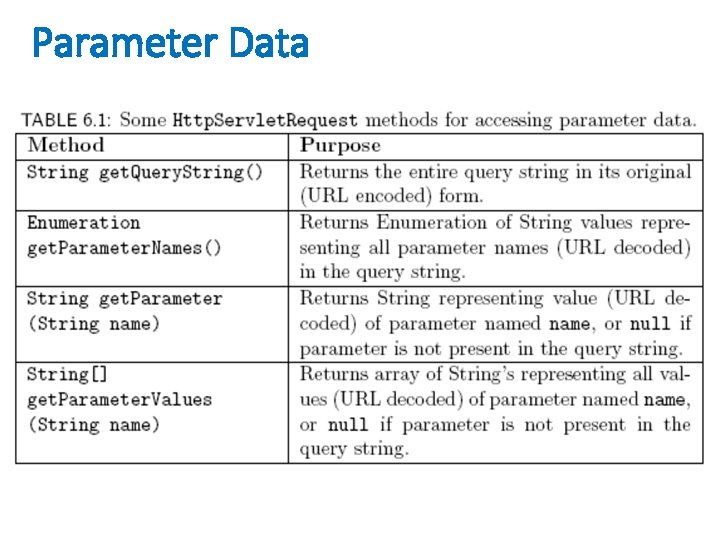
Parameter Data
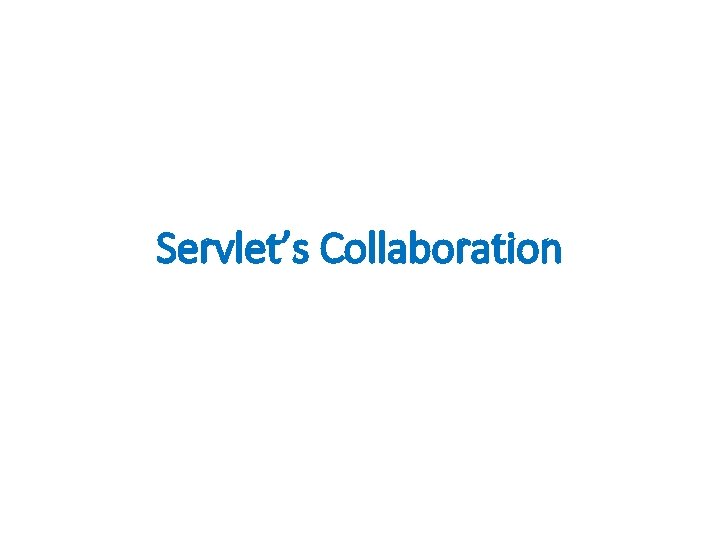
Servlet’s Collaboration
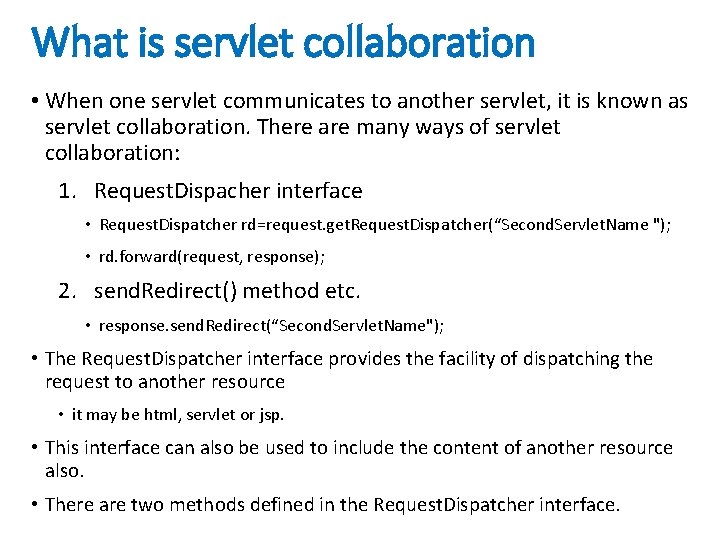
What is servlet collaboration • When one servlet communicates to another servlet, it is known as servlet collaboration. There are many ways of servlet collaboration: 1. Request. Dispacher interface • Request. Dispatcher rd=request. get. Request. Dispatcher(“Second. Servlet. Name "); • rd. forward(request, response); 2. send. Redirect() method etc. • response. send. Redirect(“Second. Servlet. Name"); • The Request. Dispatcher interface provides the facility of dispatching the request to another resource • it may be html, servlet or jsp. • This interface can also be used to include the content of another resource also. • There are two methods defined in the Request. Dispatcher interface.
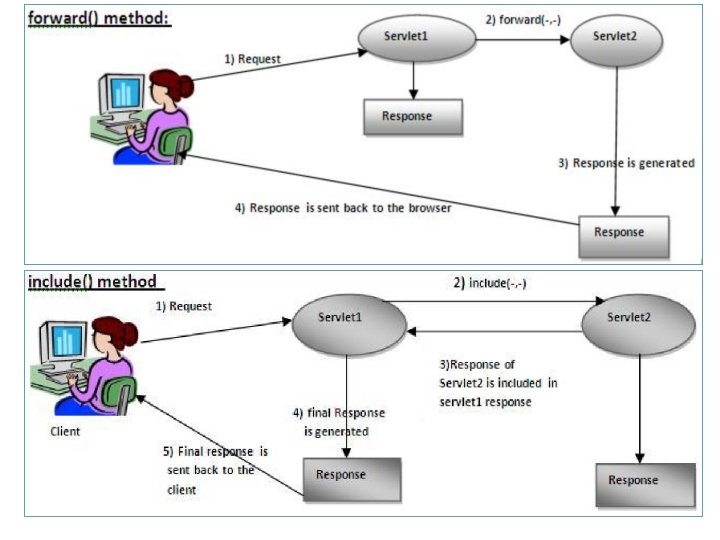
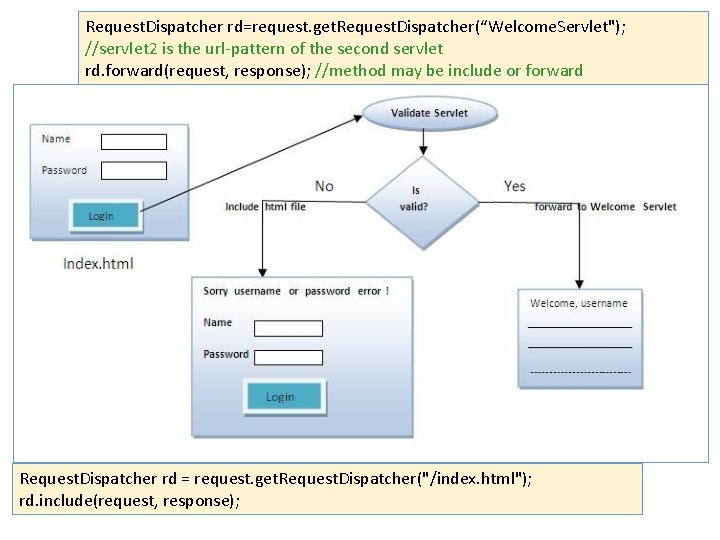
Request. Dispatcher rd=request. get. Request. Dispatcher(“Welcome. Servlet"); //servlet 2 is the url-pattern of the second servlet rd. forward(request, response); //method may be include or forward Request. Dispatcher rd = request. get. Request. Dispatcher("/index. html"); rd. include(request, response);
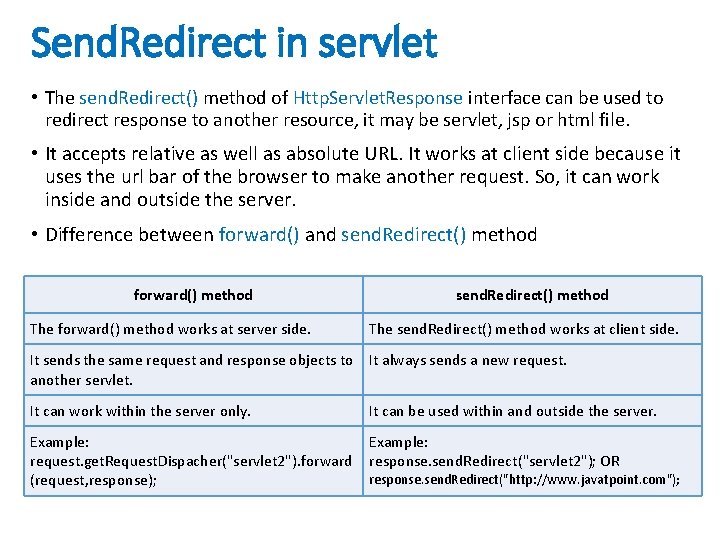
Send. Redirect in servlet • The send. Redirect() method of Http. Servlet. Response interface can be used to redirect response to another resource, it may be servlet, jsp or html file. • It accepts relative as well as absolute URL. It works at client side because it uses the url bar of the browser to make another request. So, it can work inside and outside the server. • Difference between forward() and send. Redirect() method forward() method The forward() method works at server side. send. Redirect() method The send. Redirect() method works at client side. It sends the same request and response objects to It always sends a new request. another servlet. It can work within the server only. It can be used within and outside the server. Example: request. get. Request. Dispacher("servlet 2"). forward (request, response); Example: response. send. Redirect("servlet 2"); OR response. send. Redirect("http: //www. javatpoint. com");
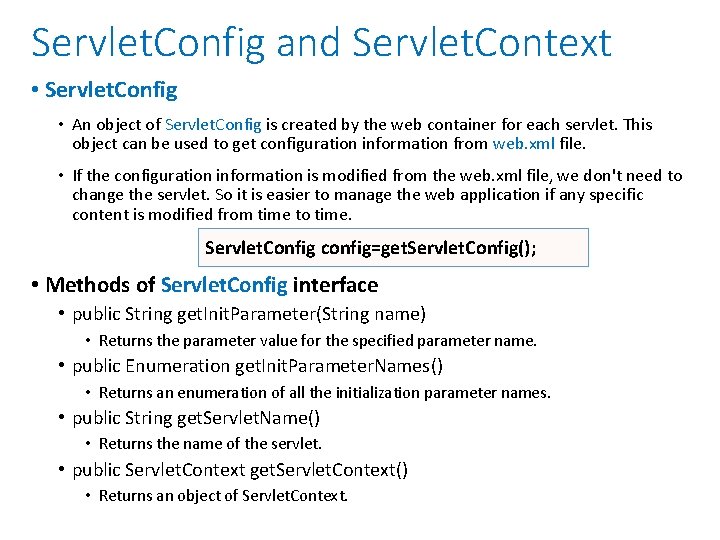
Servlet. Config and Servlet. Context • Servlet. Config • An object of Servlet. Config is created by the web container for each servlet. This object can be used to get configuration information from web. xml file. • If the configuration information is modified from the web. xml file, we don't need to change the servlet. So it is easier to manage the web application if any specific content is modified from time to time. Servlet. Config config=get. Servlet. Config(); • Methods of Servlet. Config interface • public String get. Init. Parameter(String name) • Returns the parameter value for the specified parameter name. • public Enumeration get. Init. Parameter. Names() • Returns an enumeration of all the initialization parameter names. • public String get. Servlet. Name() • Returns the name of the servlet. • public Servlet. Context get. Servlet. Context() • Returns an object of Servlet. Context.
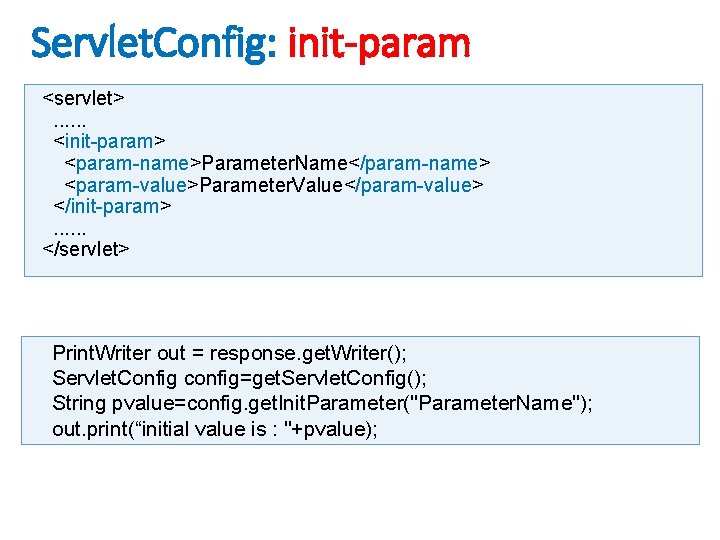
Servlet. Config: init-param <servlet>. . . <init-param> <param-name>Parameter. Name</param-name> <param-value>Parameter. Value</param-value> </init-param>. . . </servlet> Print. Writer out = response. get. Writer(); Servlet. Config config=get. Servlet. Config(); String pvalue=config. get. Init. Parameter("Parameter. Name"); out. print(“initial value is : "+pvalue);
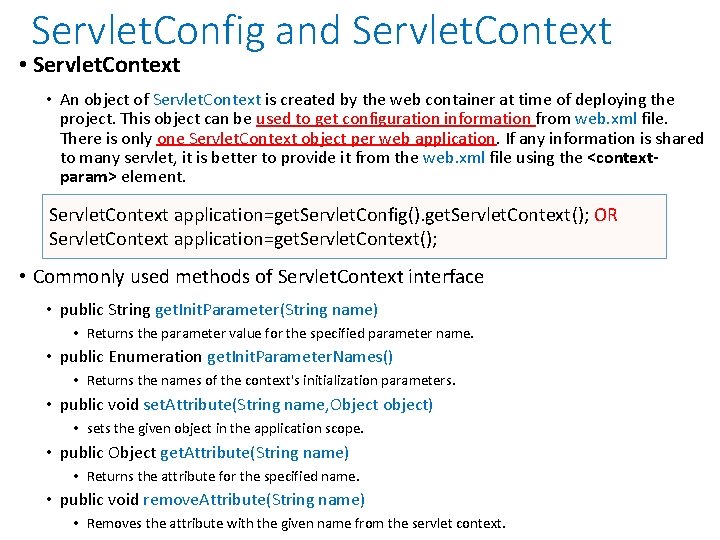
Servlet. Config and Servlet. Context • An object of Servlet. Context is created by the web container at time of deploying the project. This object can be used to get configuration information from web. xml file. There is only one Servlet. Context object per web application. If any information is shared to many servlet, it is better to provide it from the web. xml file using the <contextparam> element. Servlet. Context application=get. Servlet. Config(). get. Servlet. Context(); OR Servlet. Context application=get. Servlet. Context(); • Commonly used methods of Servlet. Context interface • public String get. Init. Parameter(String name) • Returns the parameter value for the specified parameter name. • public Enumeration get. Init. Parameter. Names() • Returns the names of the context's initialization parameters. • public void set. Attribute(String name, Object object) • sets the given object in the application scope. • public Object get. Attribute(String name) • Returns the attribute for the specified name. • public void remove. Attribute(String name) • Removes the attribute with the given name from the servlet context.
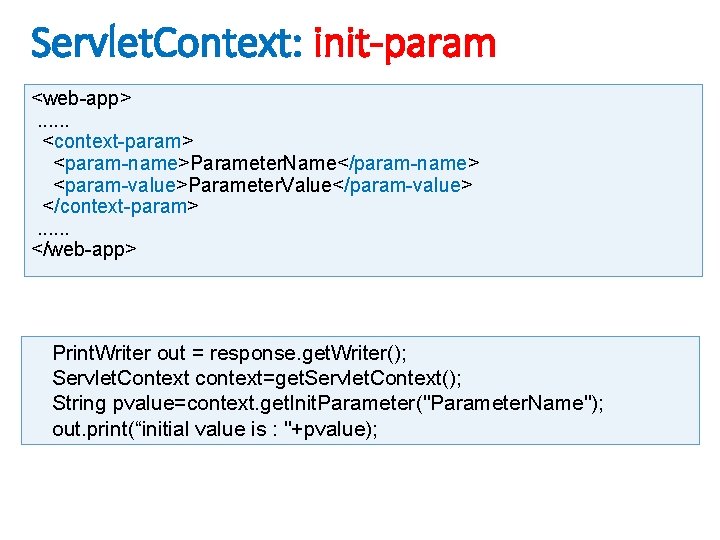
Servlet. Context: init-param <web-app>. . . <context-param> <param-name>Parameter. Name</param-name> <param-value>Parameter. Value</param-value> </context-param>. . . </web-app> Print. Writer out = response. get. Writer(); Servlet. Context context=get. Servlet. Context(); String pvalue=context. get. Init. Parameter("Parameter. Name"); out. print(“initial value is : "+pvalue);
Serverside scripts
Sss similarity theorem
Similarity theorem examples
Side side side similarity
Aa similarity theorem
What is server side scripting language
Coreservlets
Servlets notes
Introduction to server side programming
Perfect competition side by side graphs
Tea uil side by side
M&a process timeline
Glass will break first on the weaker side, the side:
Side angle side theorem
Two wheels roll side by side
Single bevel weld symbol
A regular hexagonal lamina has equal sides
The incisal guidance angle varies directly with.
Platzbedarf side by side melkstand
Side by side stuff
Sin inverse -1
Immediate bennett shift
Videocon side by side refrigerator
Red side blue side
Adobe audience manager server side forwarding
Php server side includes
Server-side technologies
Azure server side encryption
What is node js server side javascript
Server side
Java server pages tutorial
Pircbot
Java irc server
Android udp client example
Java.rmi.server.codebase
Lập trình socket giao tiếp tcp client/server java
Knock knock server java
Jsf sample
Java server pages
Java server pages
Jsp o que é
Tcp echo client
Hình ảnh bộ gõ cơ thể búng tay
Frameset trong html5
Bổ thể
Tỉ lệ cơ thể trẻ em
Chó sói
Tư thế worm breton là gì
Chúa yêu trần thế
Các môn thể thao bắt đầu bằng tiếng bóng
Thế nào là hệ số cao nhất
Các châu lục và đại dương trên thế giới
Công thức tiính động năng
Trời xanh đây là của chúng ta thể thơ
Mật thư anh em như thể tay chân
Làm thế nào để 102-1=99
độ dài liên kết