Programming in C DaleWeemsHeadington Chapter 3 Arithmetic Expressions
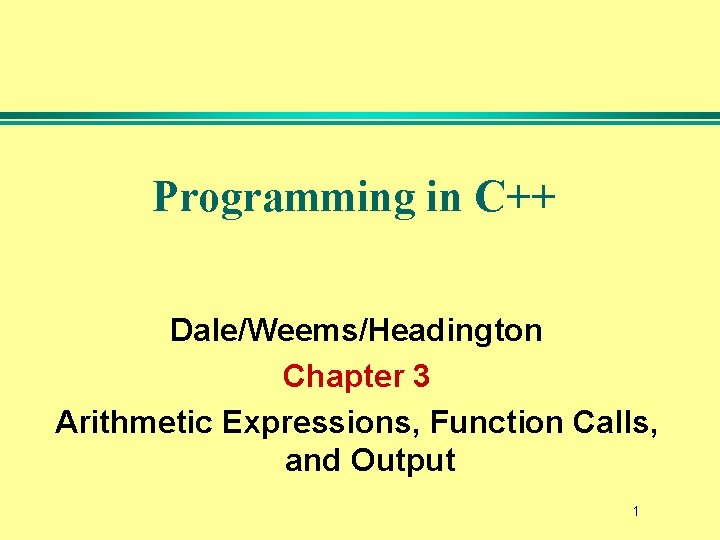
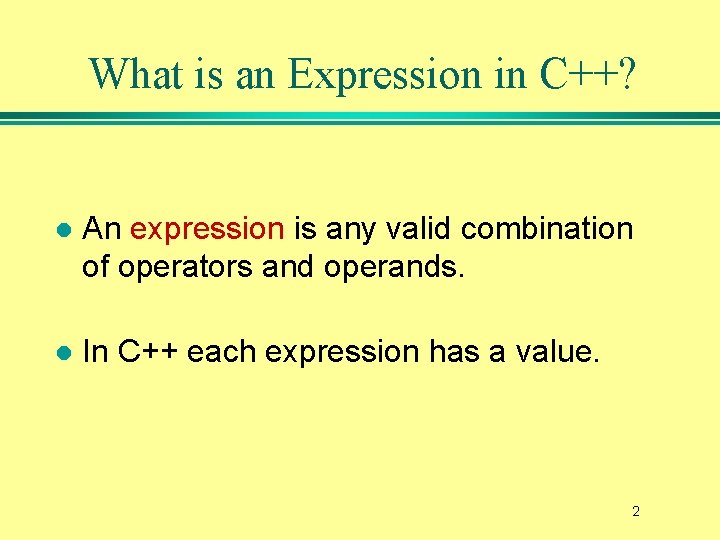
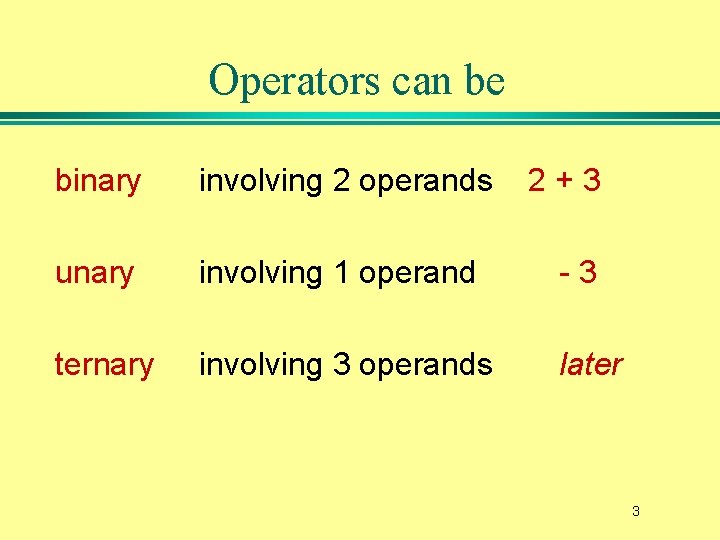
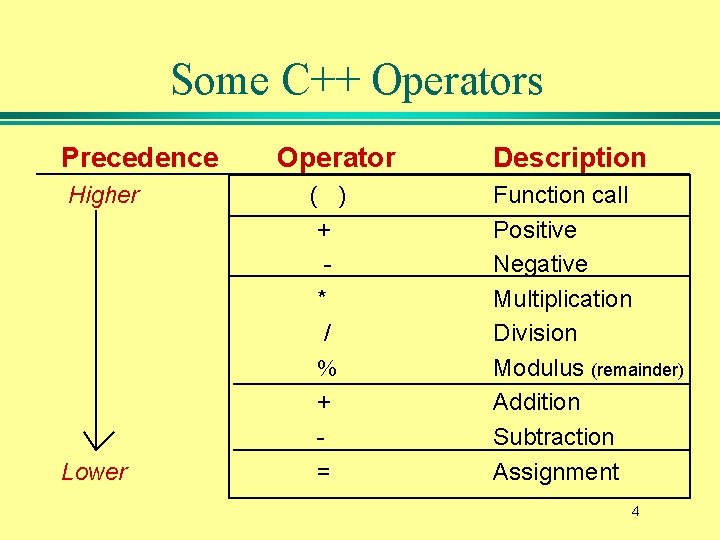
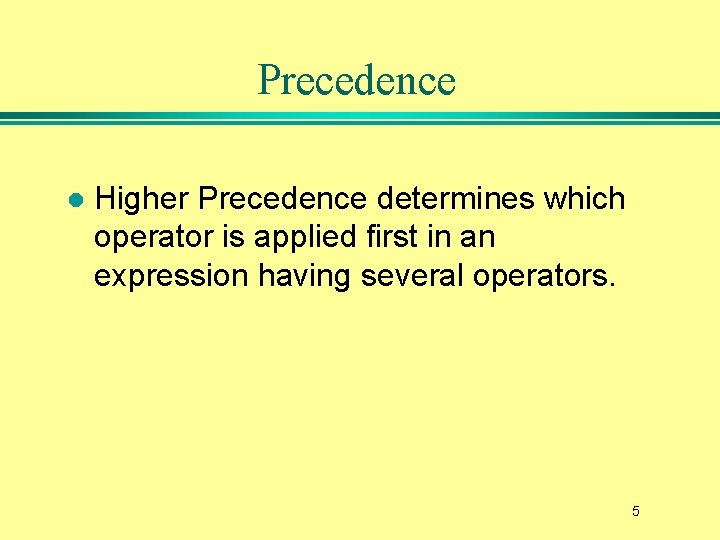
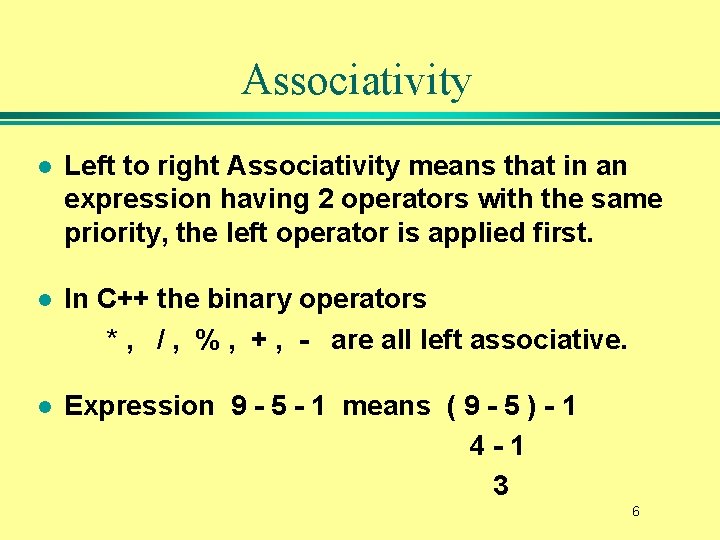
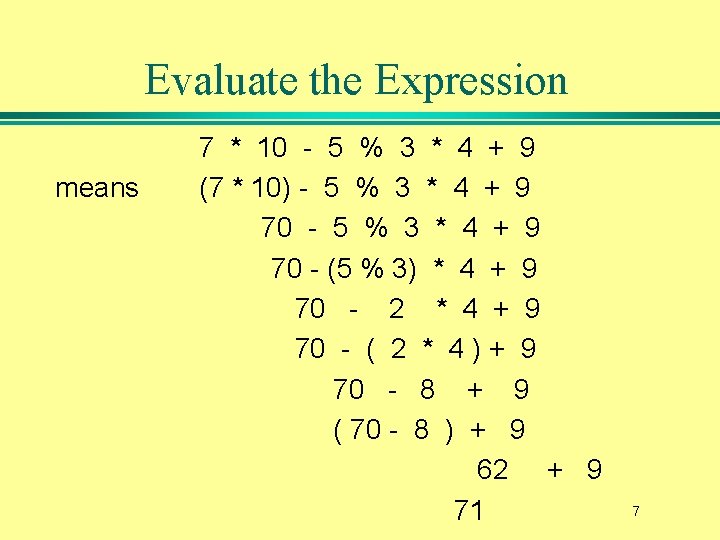
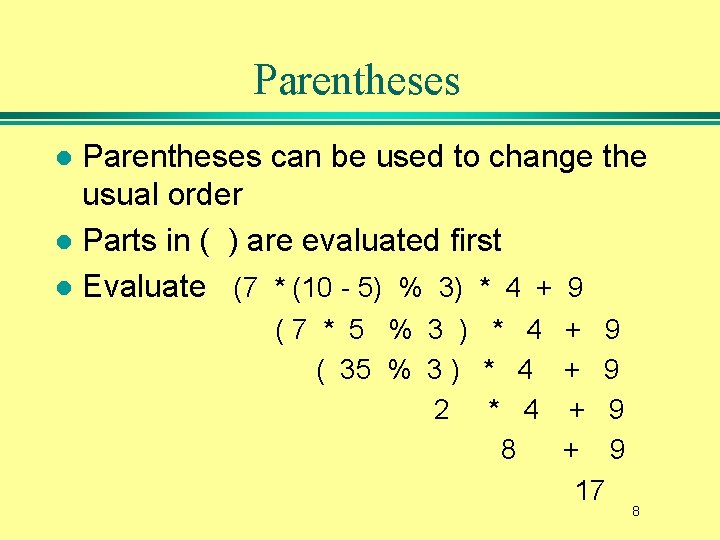
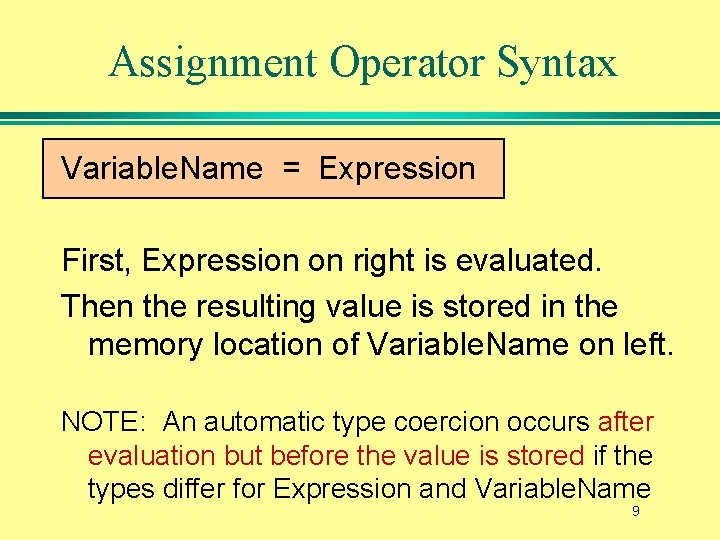
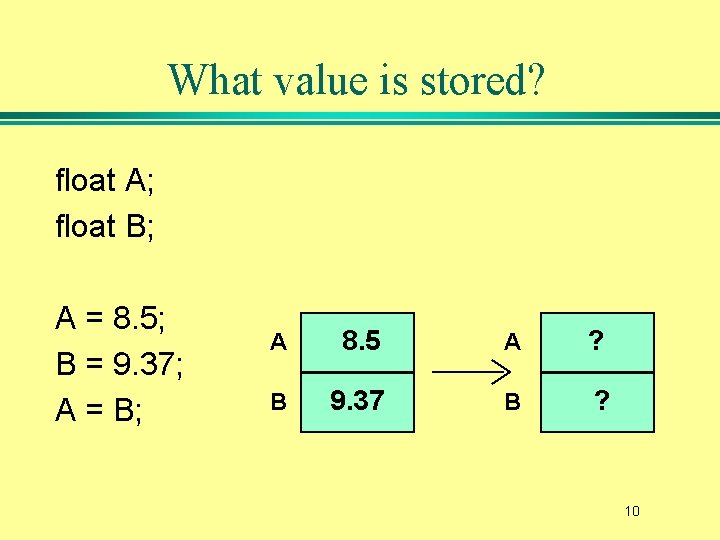
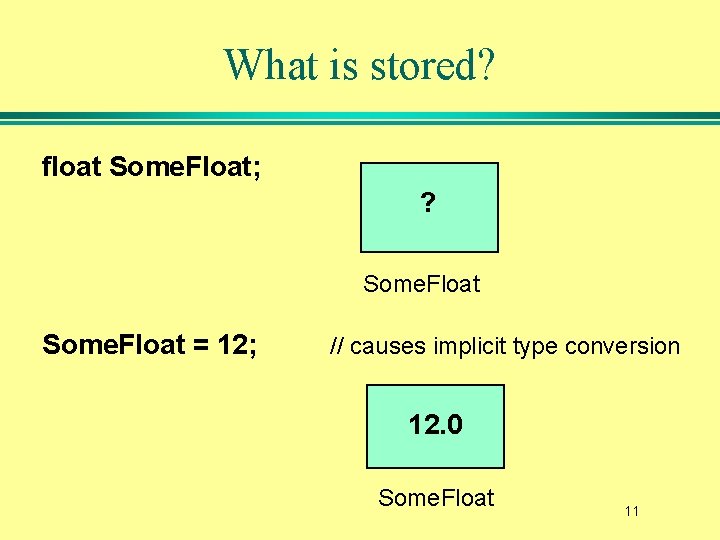
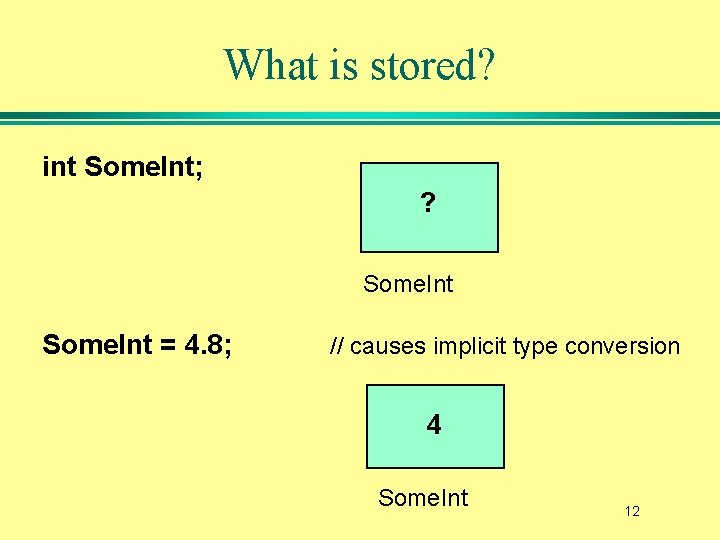
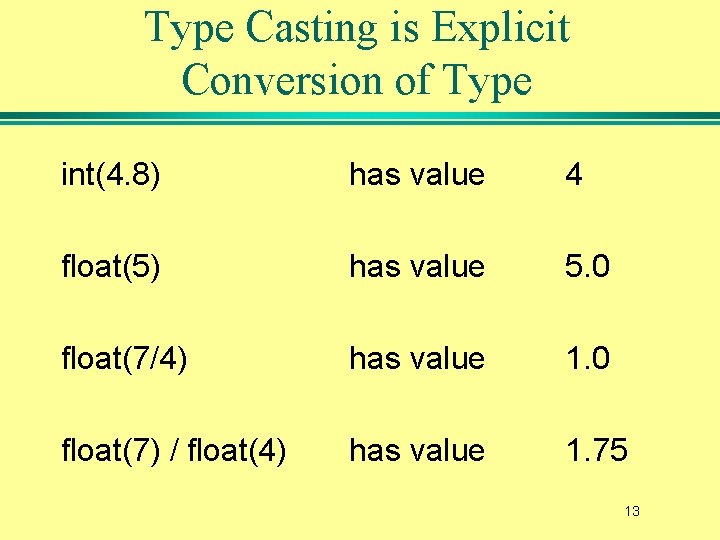
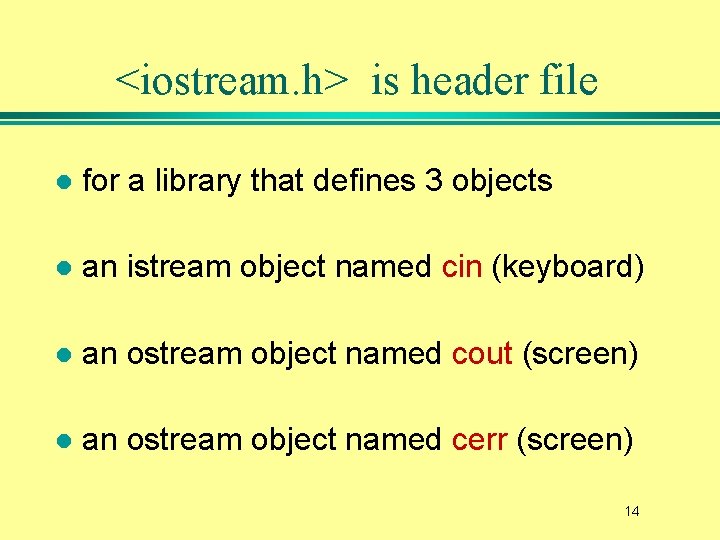
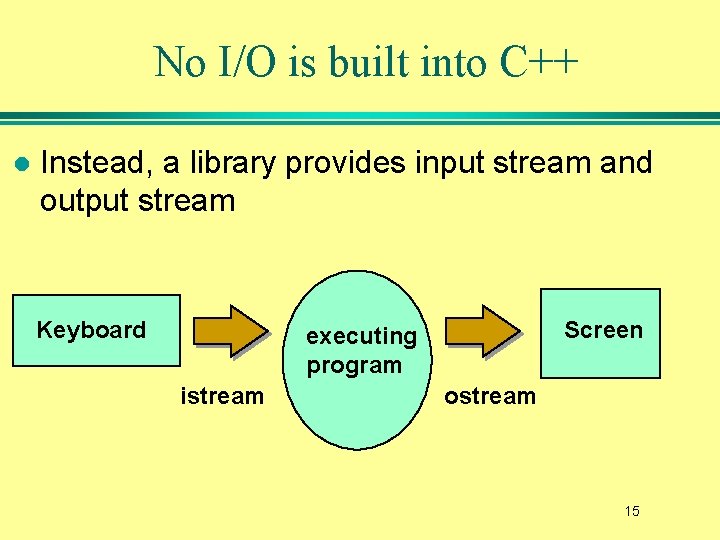
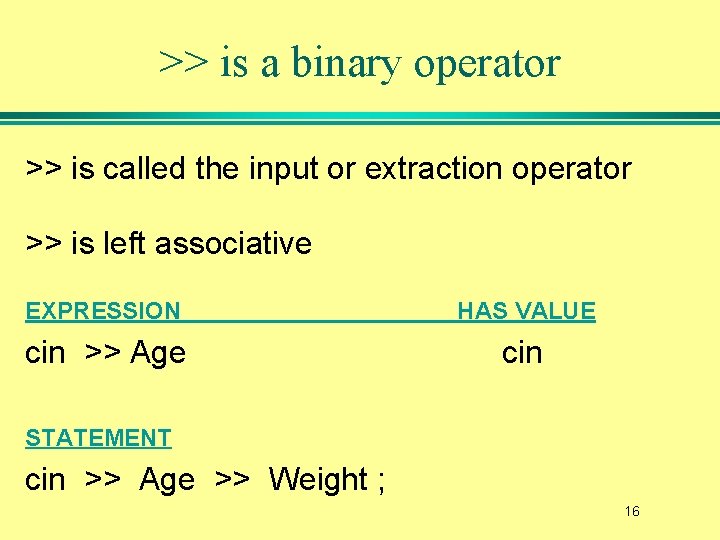
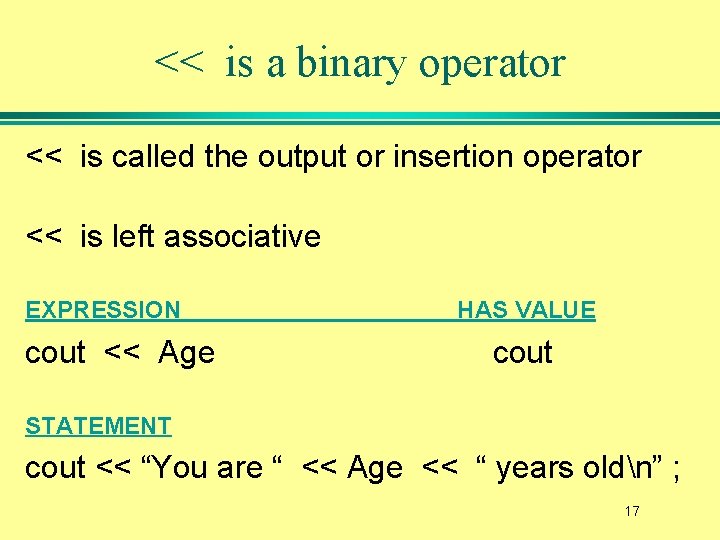
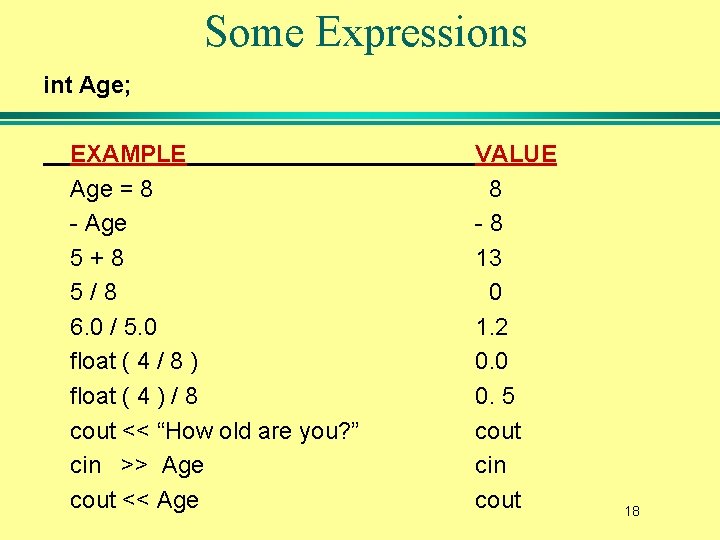
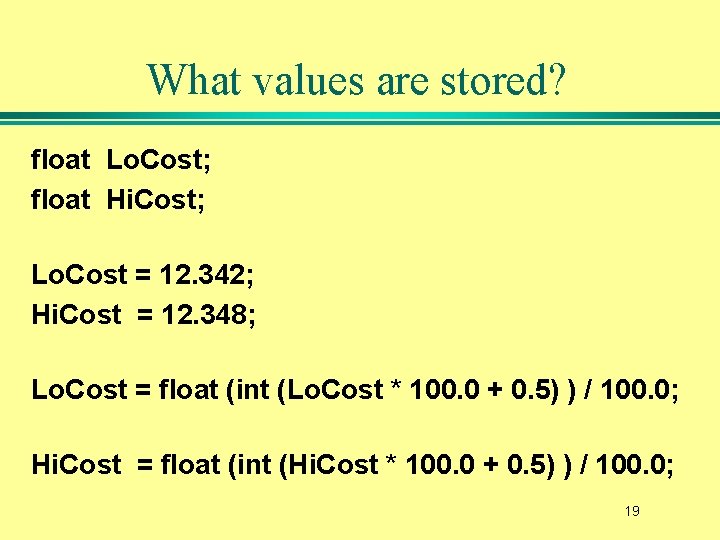
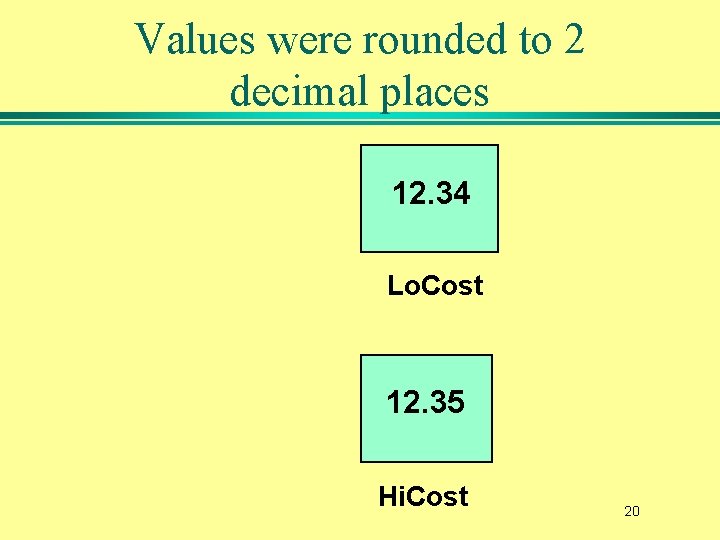
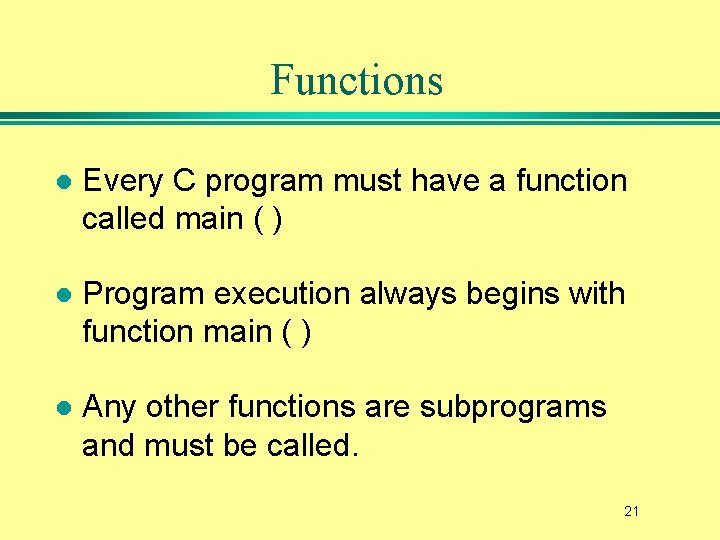
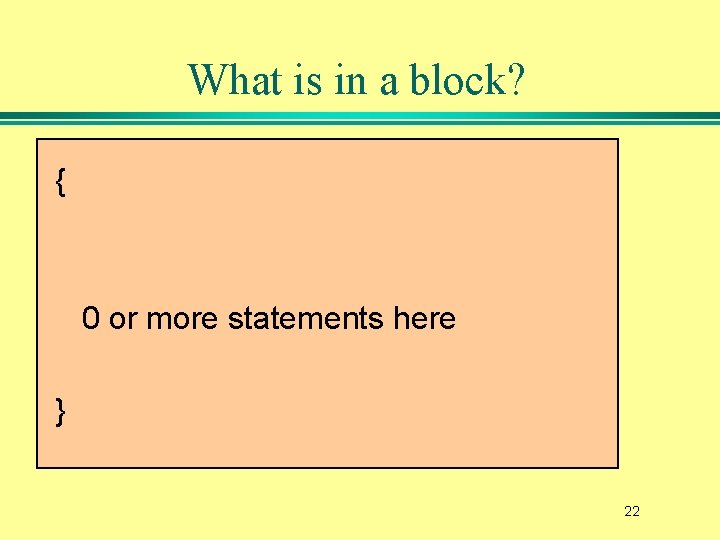
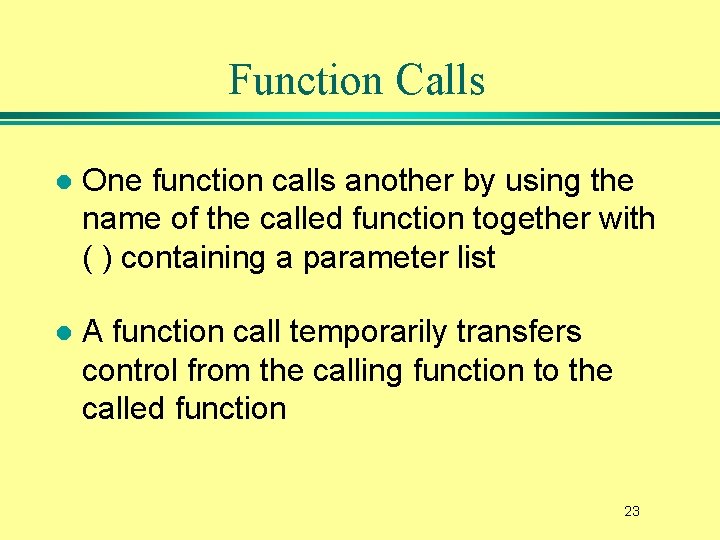
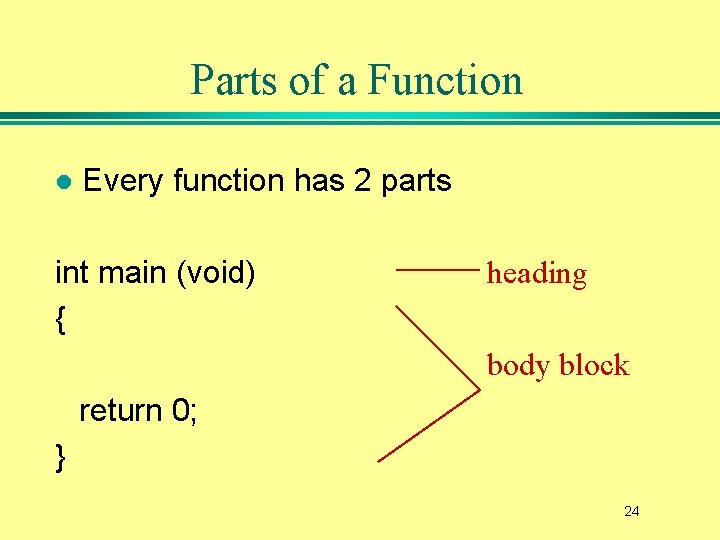
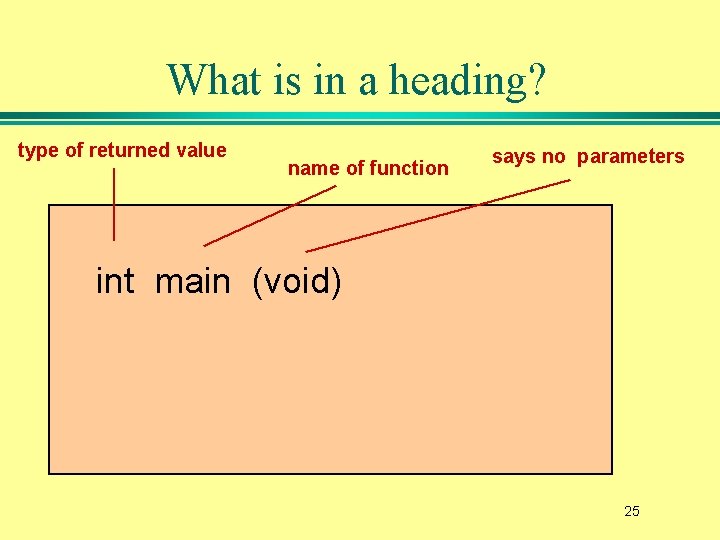
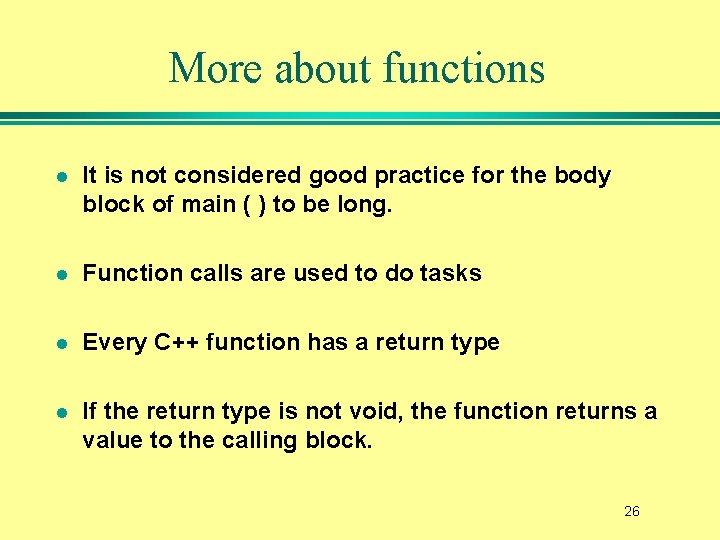
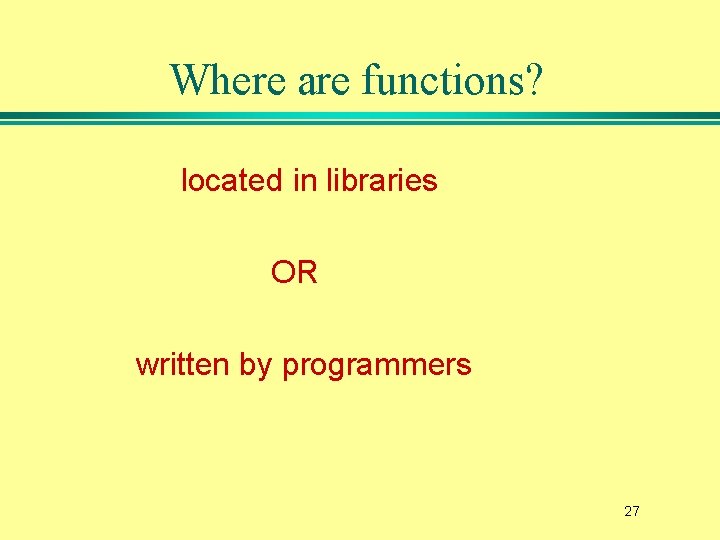
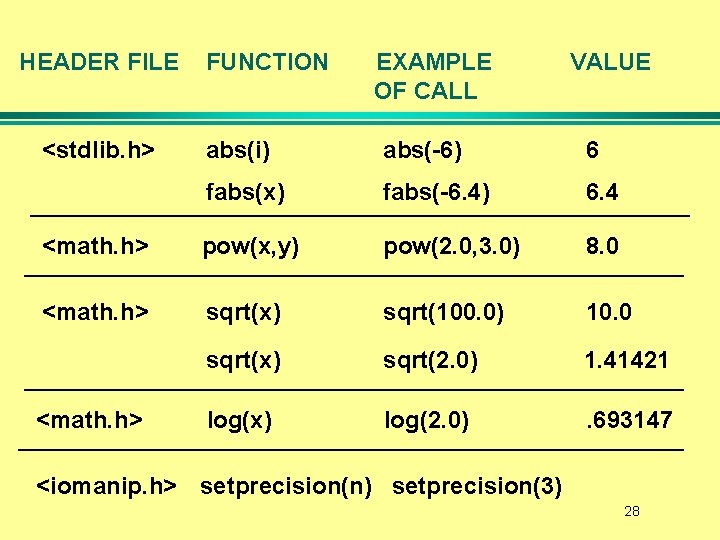
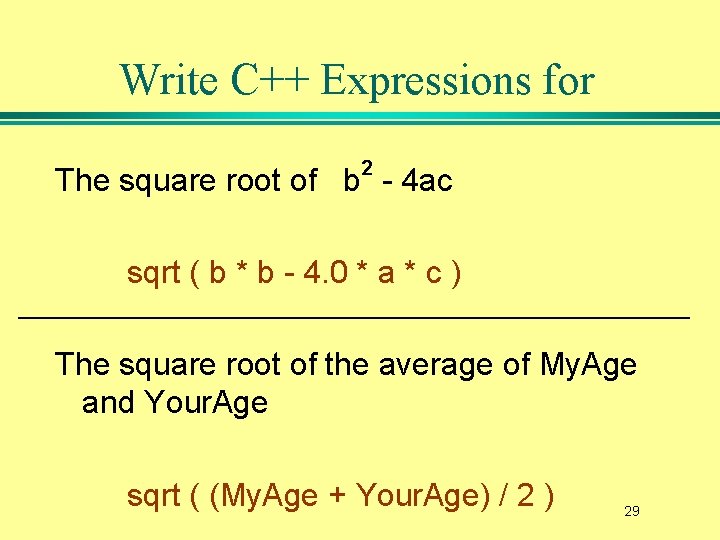
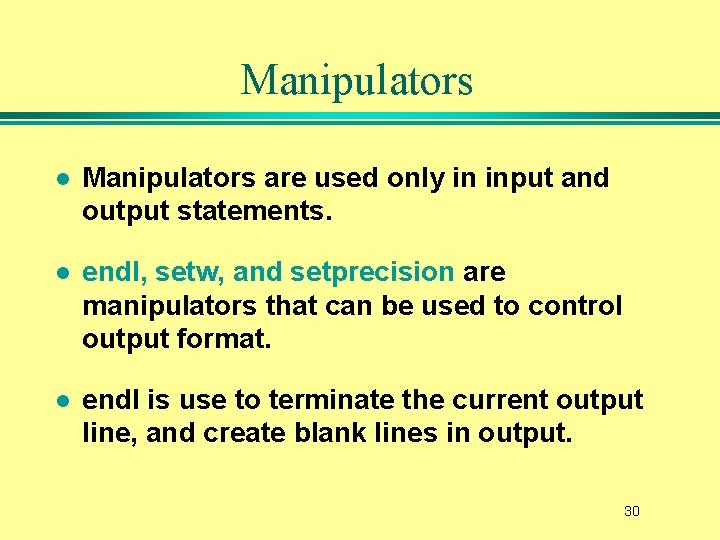
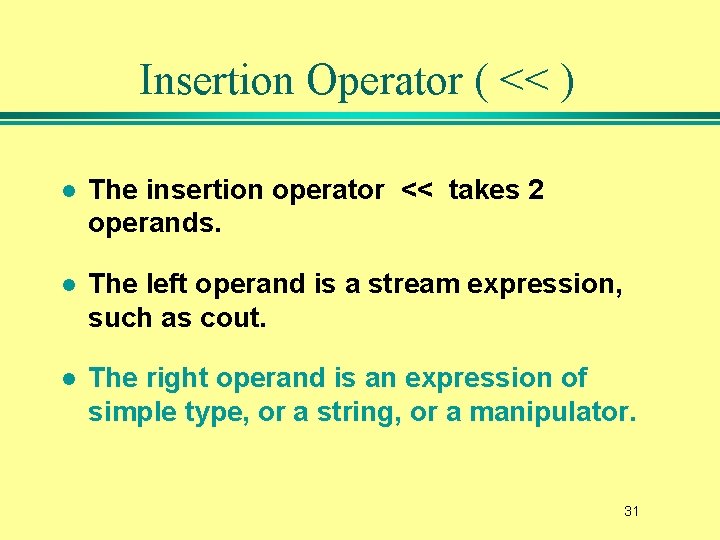
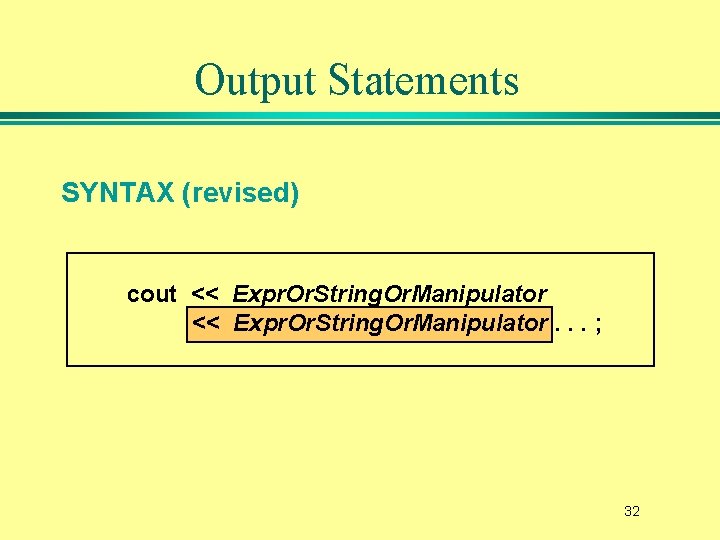
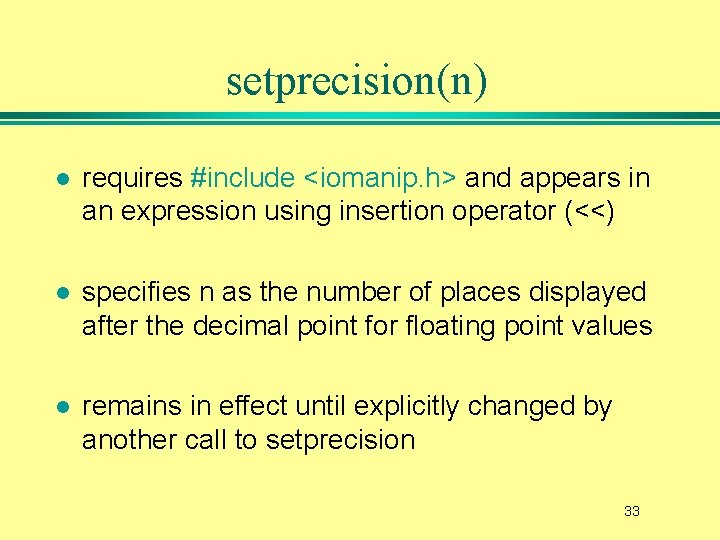
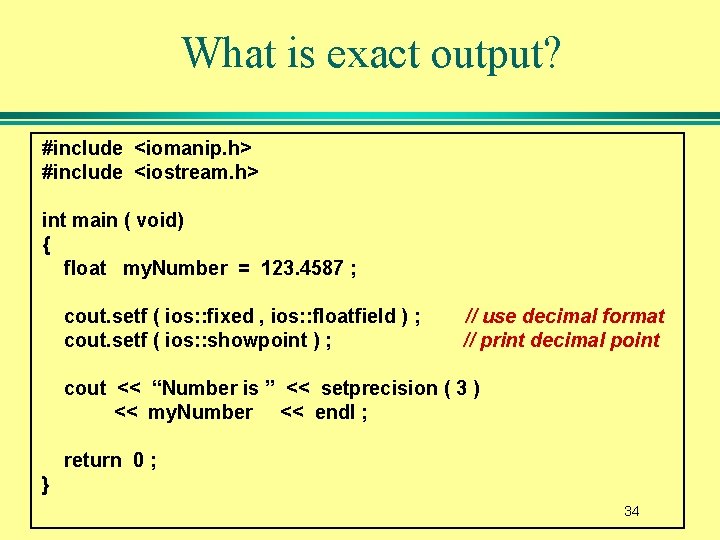
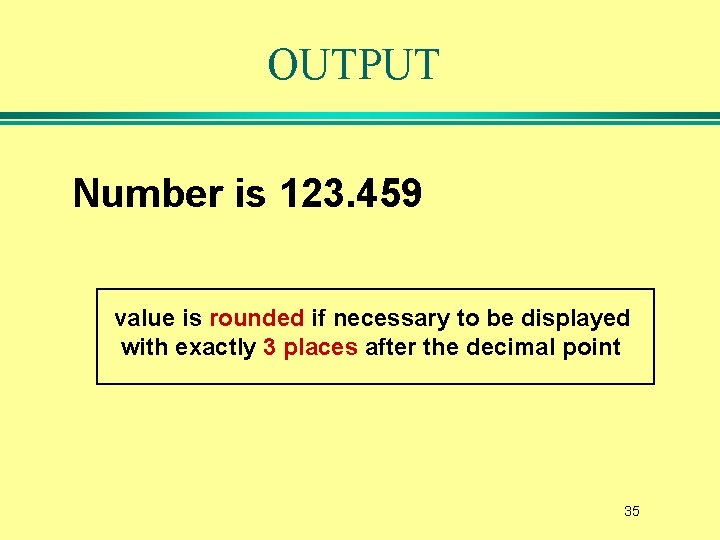
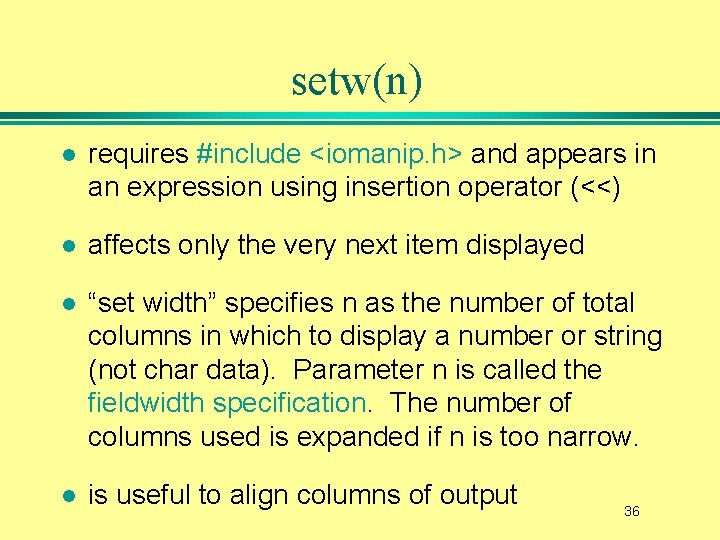
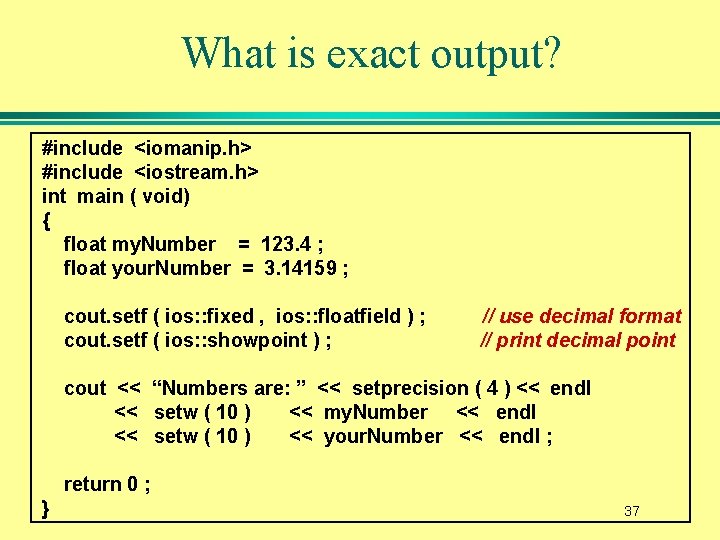
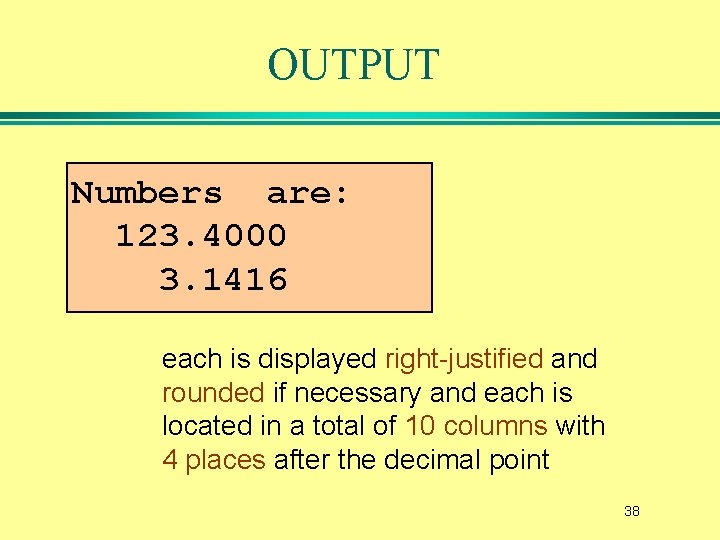
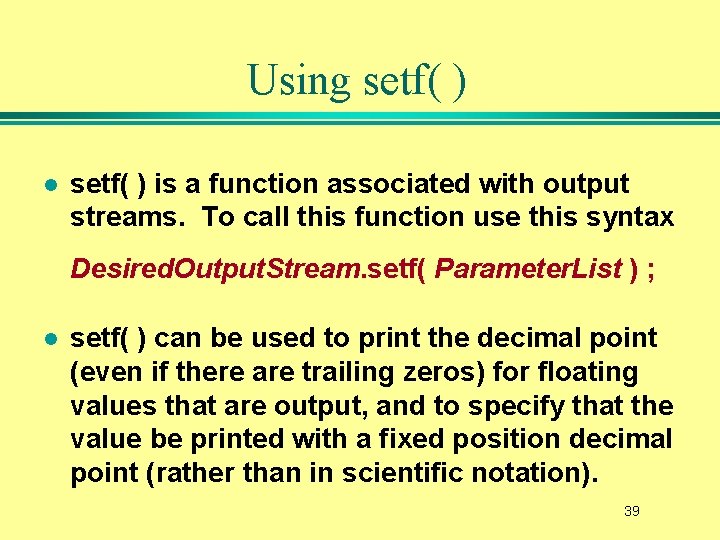
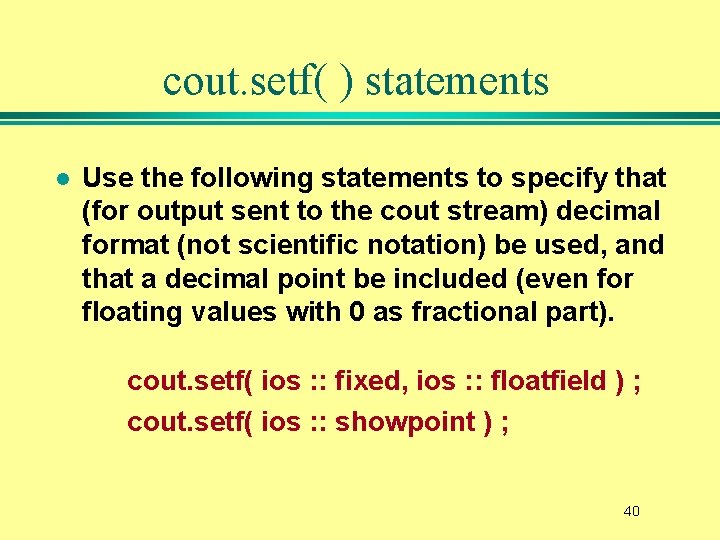
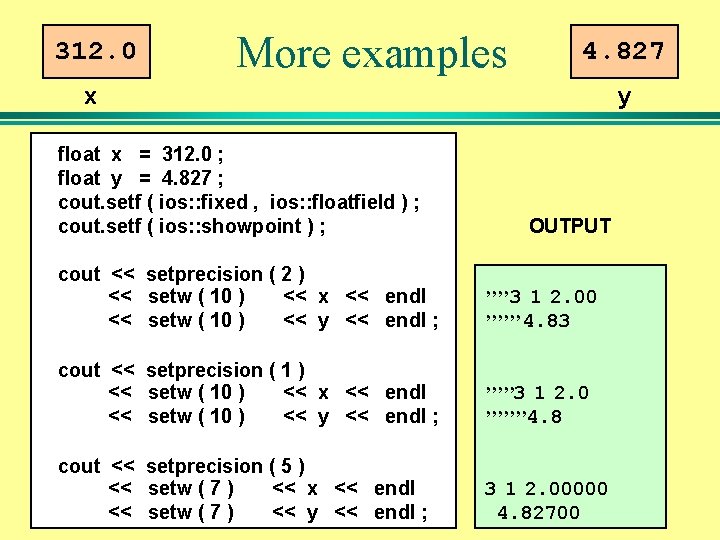
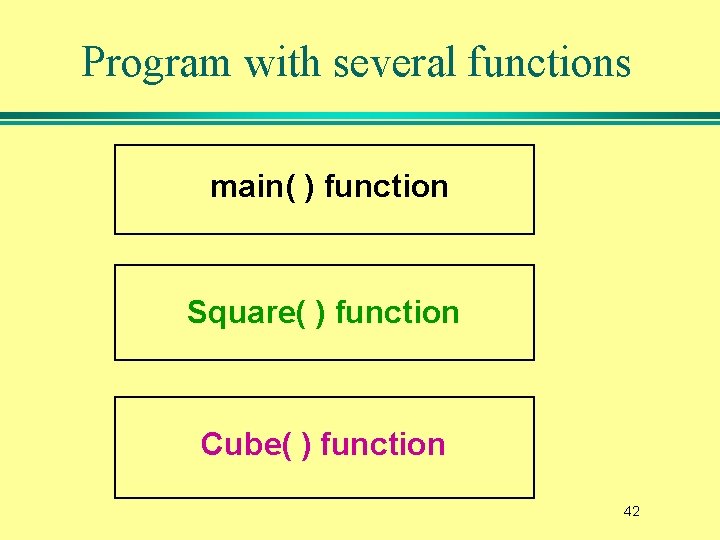
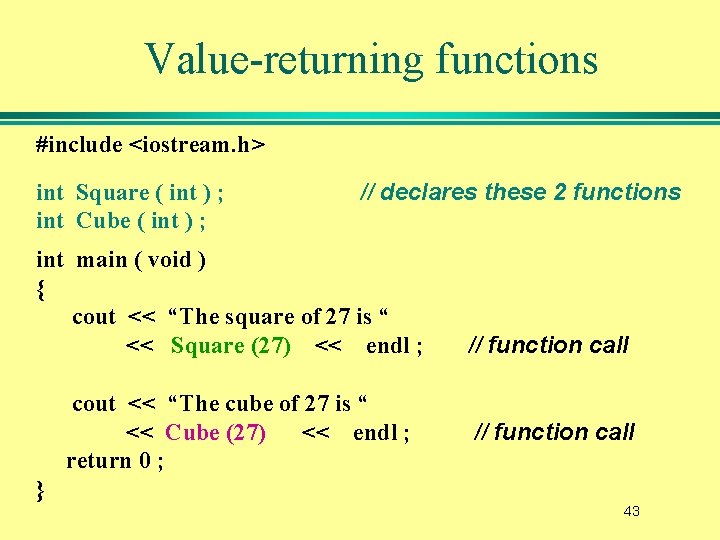
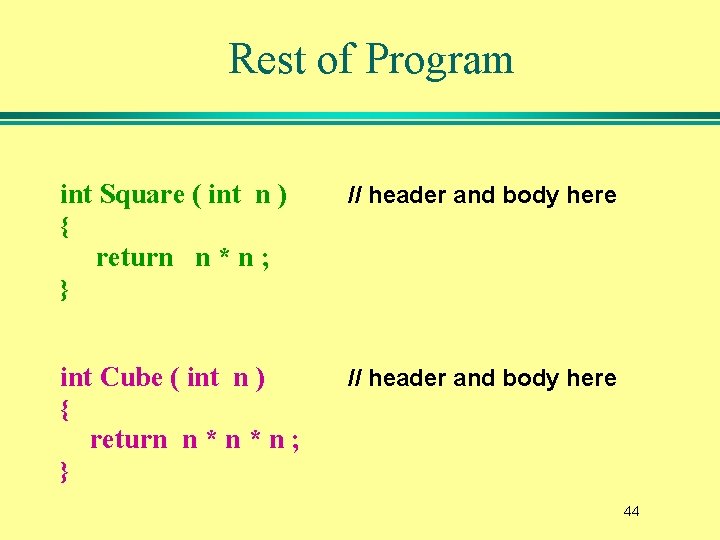
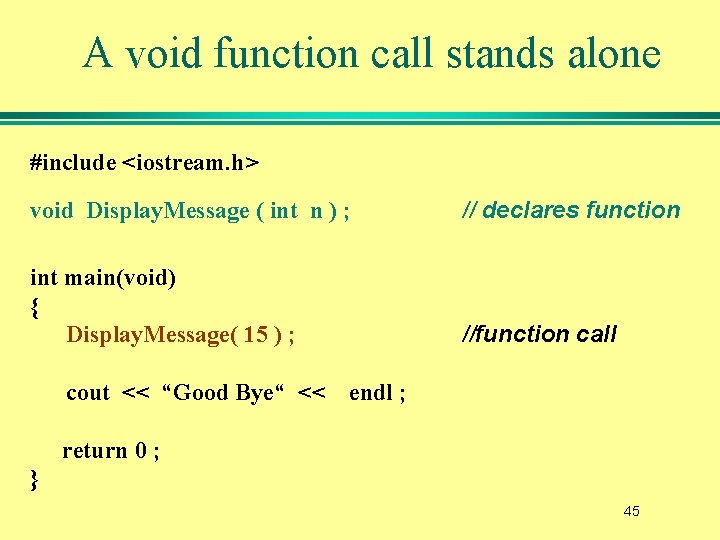
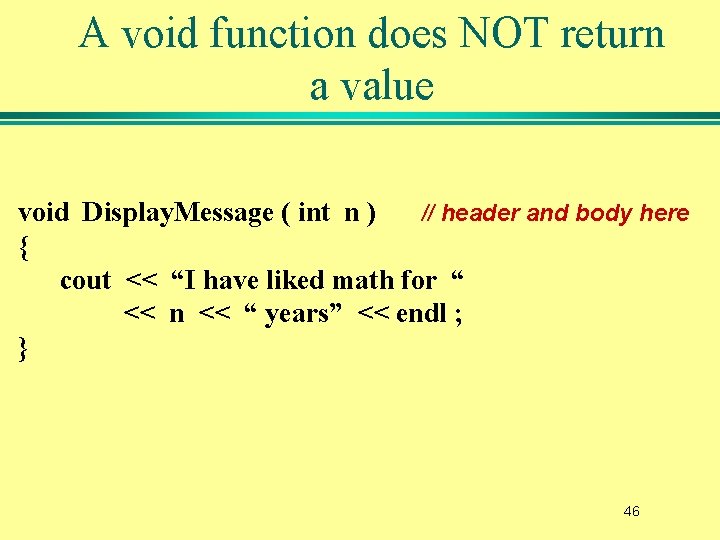
- Slides: 46
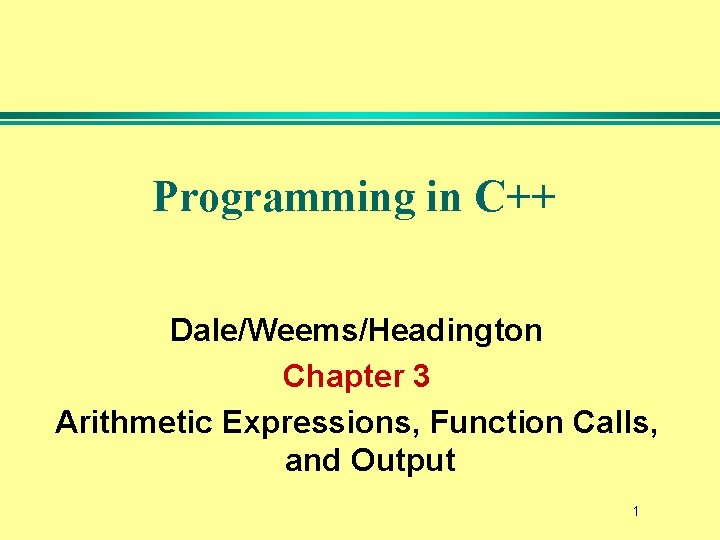
Programming in C++ Dale/Weems/Headington Chapter 3 Arithmetic Expressions, Function Calls, and Output 1
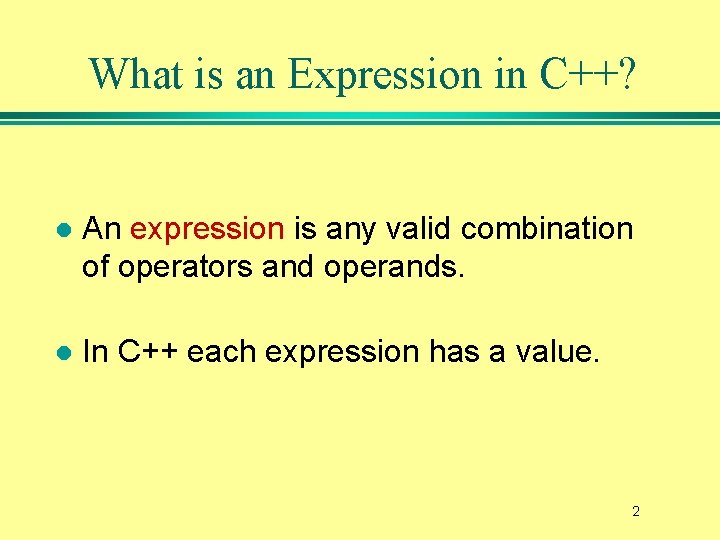
What is an Expression in C++? l An expression is any valid combination of operators and operands. l In C++ each expression has a value. 2
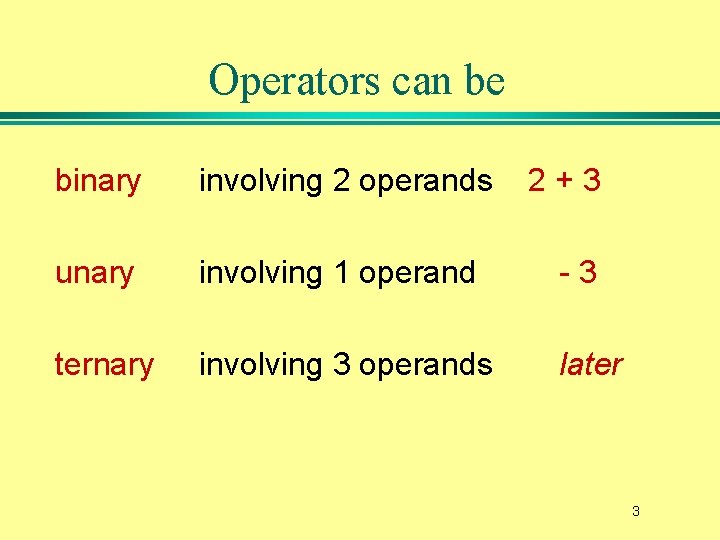
Operators can be binary involving 2 operands 2+3 unary involving 1 operand -3 ternary involving 3 operands later 3
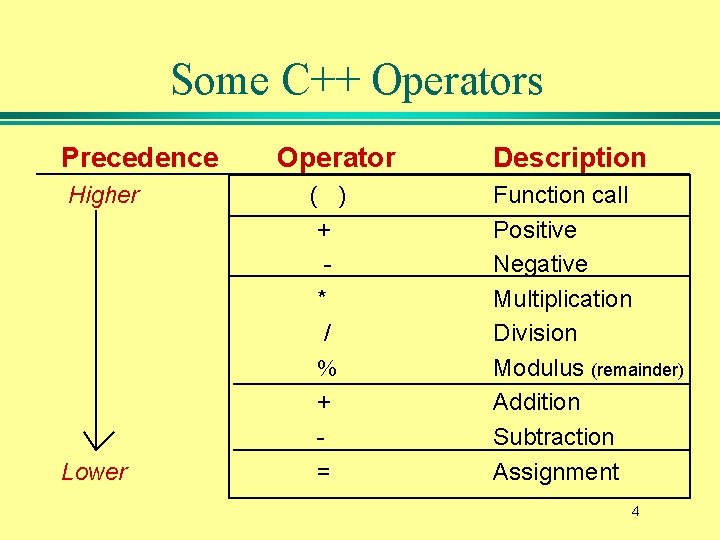
Some C++ Operators Precedence Higher Lower Operator ( ) + * / % + = Description Function call Positive Negative Multiplication Division Modulus (remainder) Addition Subtraction Assignment 4
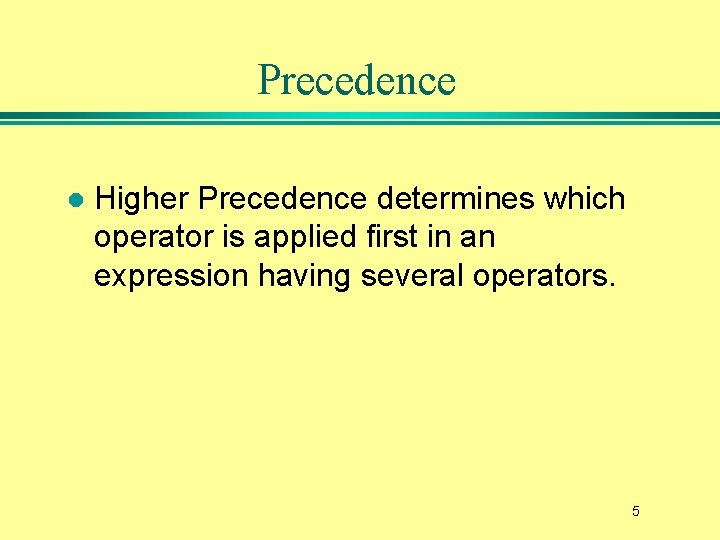
Precedence l Higher Precedence determines which operator is applied first in an expression having several operators. 5
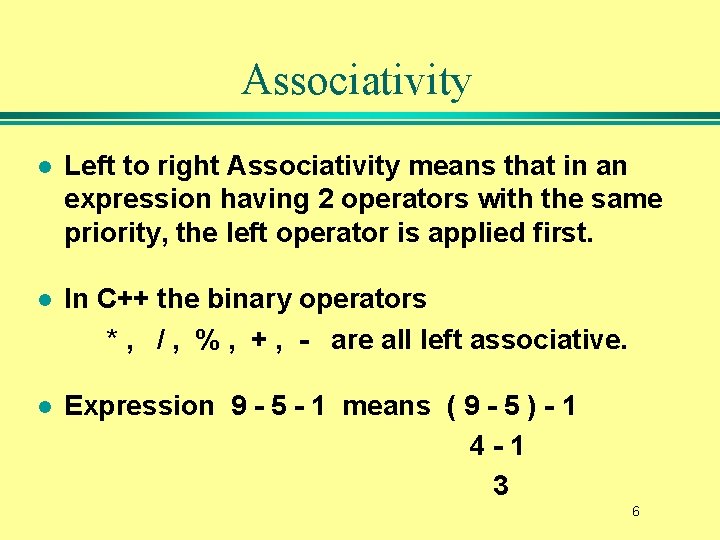
Associativity l Left to right Associativity means that in an expression having 2 operators with the same priority, the left operator is applied first. l In C++ the binary operators * , / , % , + , - are all left associative. l Expression 9 - 5 - 1 means ( 9 - 5 ) - 1 4 -1 3 6
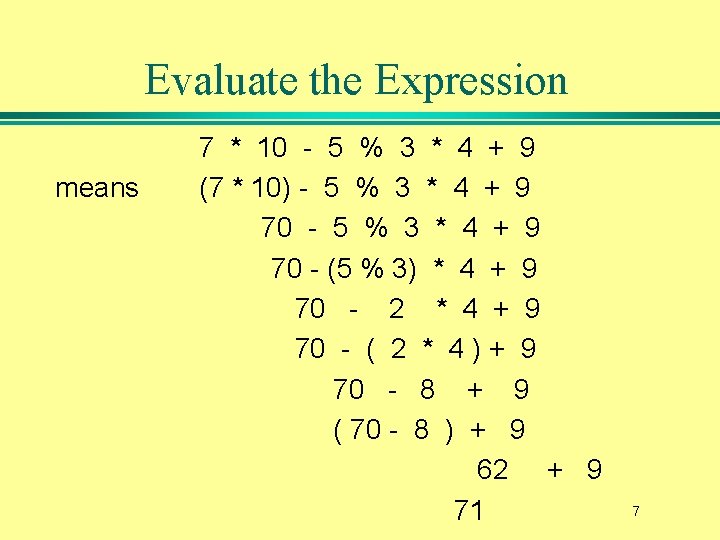
Evaluate the Expression means 7 * 10 - 5 % 3 * 4 + 9 (7 * 10) - 5 % 3 * 4 + 9 70 - (5 % 3) * 4 + 9 70 - 2 * 4 + 9 70 - ( 2 * 4 ) + 9 70 - 8 + 9 ( 70 - 8 ) + 9 62 + 9 71 7
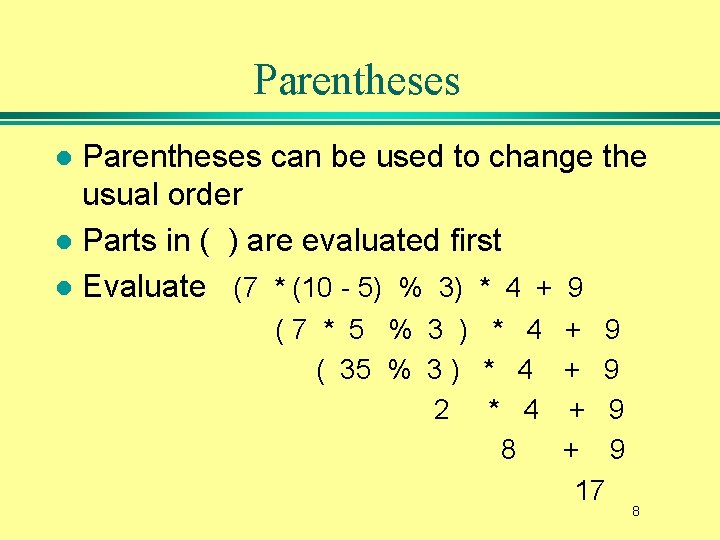
Parentheses can be used to change the usual order l Parts in ( ) are evaluated first l Evaluate (7 * (10 - 5) % 3) * 4 + 9 l (7 * 5 % 3 ) * 4 ( 35 % 3 ) * 4 2 * 4 8 + 9 + 9 17 8
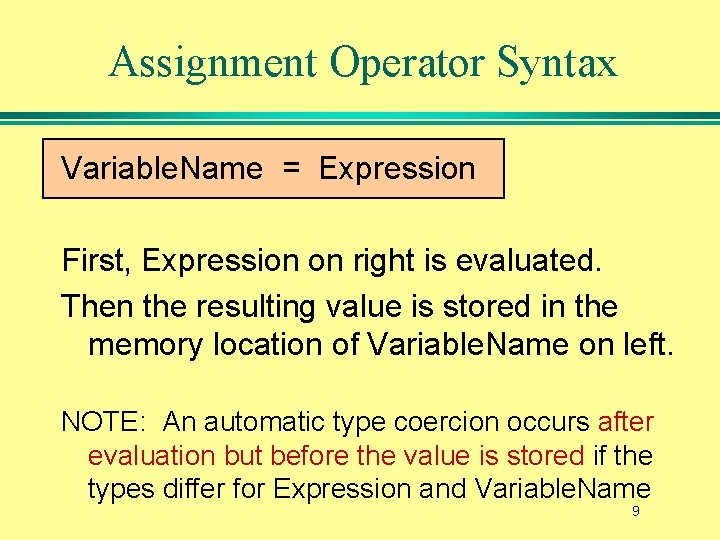
Assignment Operator Syntax Variable. Name = Expression First, Expression on right is evaluated. Then the resulting value is stored in the memory location of Variable. Name on left. NOTE: An automatic type coercion occurs after evaluation but before the value is stored if the types differ for Expression and Variable. Name 9
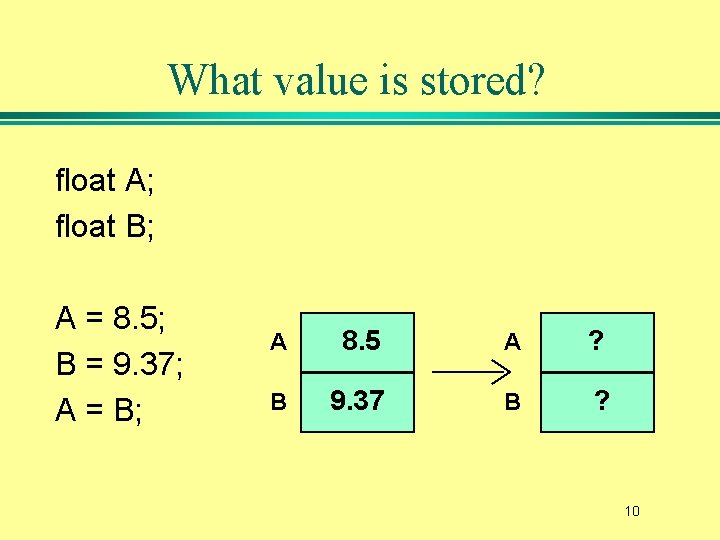
What value is stored? float A; float B; A = 8. 5; B = 9. 37; A = B; A 8. 5 A ? B 9. 37 B ? 10
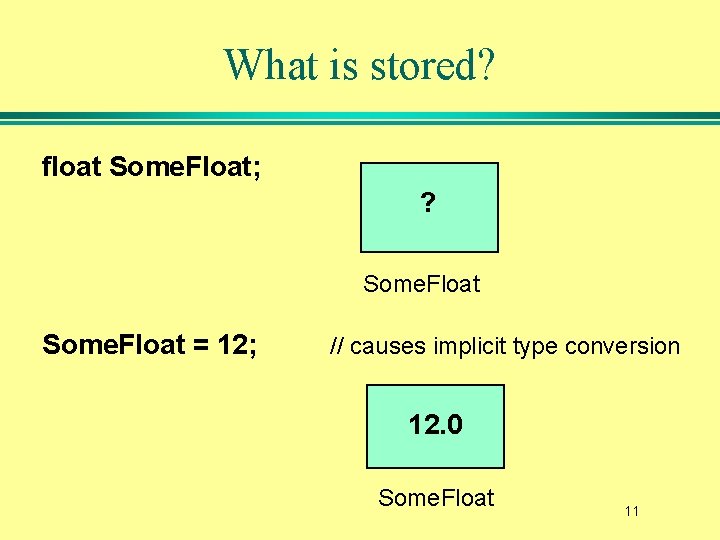
What is stored? float Some. Float; ? Some. Float = 12; // causes implicit type conversion 12. 0 Some. Float 11
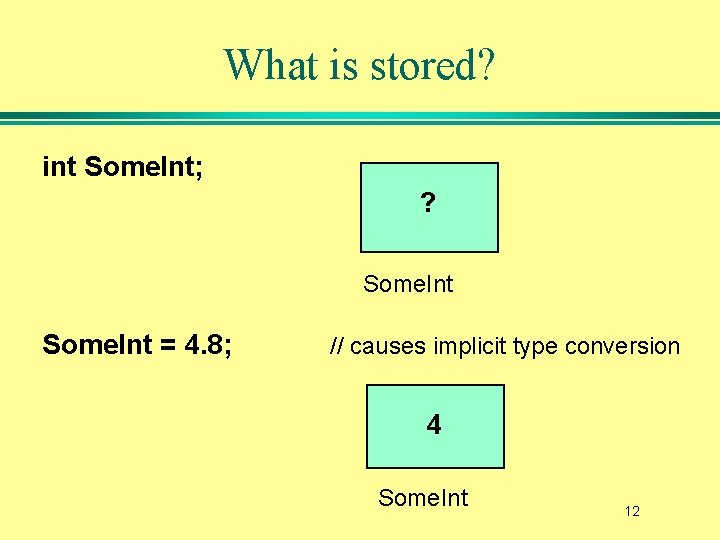
What is stored? int Some. Int; ? Some. Int = 4. 8; // causes implicit type conversion 4 Some. Int 12
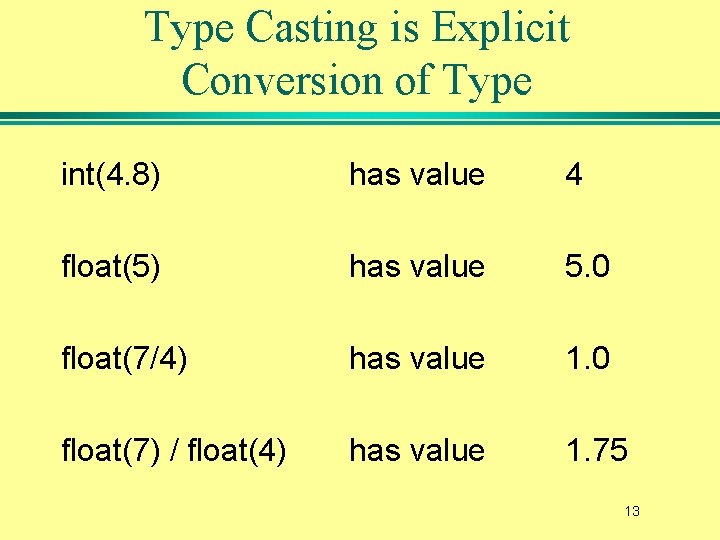
Type Casting is Explicit Conversion of Type int(4. 8) has value 4 float(5) has value 5. 0 float(7/4) has value 1. 0 float(7) / float(4) has value 1. 75 13
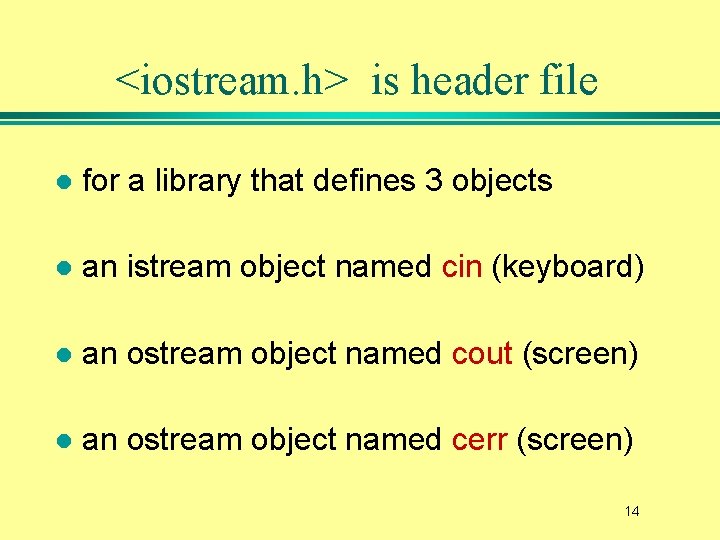
<iostream. h> is header file l for a library that defines 3 objects l an istream object named cin (keyboard) l an ostream object named cout (screen) l an ostream object named cerr (screen) 14
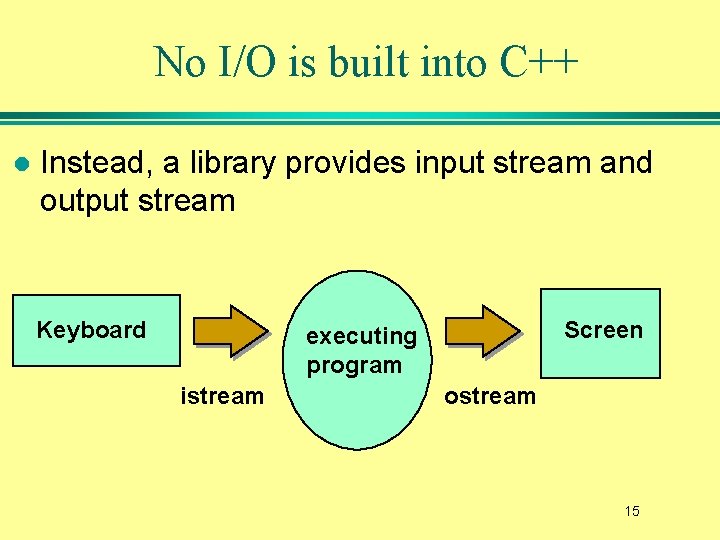
No I/O is built into C++ l Instead, a library provides input stream and output stream Keyboard Screen executing program istream ostream 15
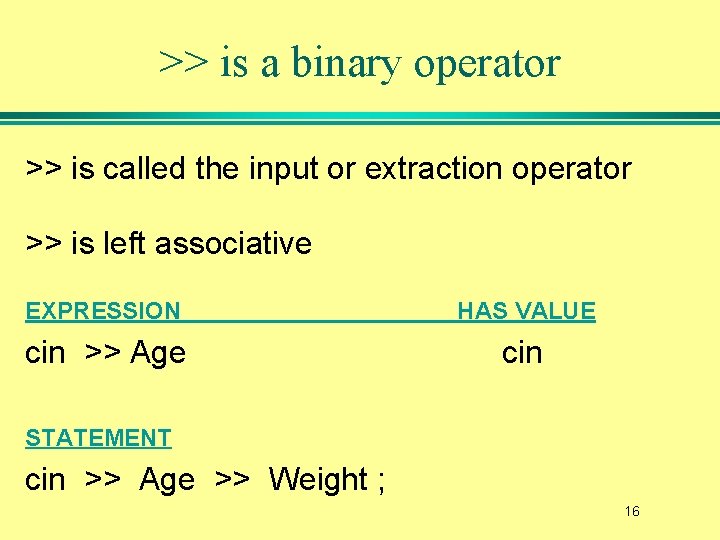
>> is a binary operator >> is called the input or extraction operator >> is left associative EXPRESSION HAS VALUE cin >> Age cin STATEMENT cin >> Age >> Weight ; 16
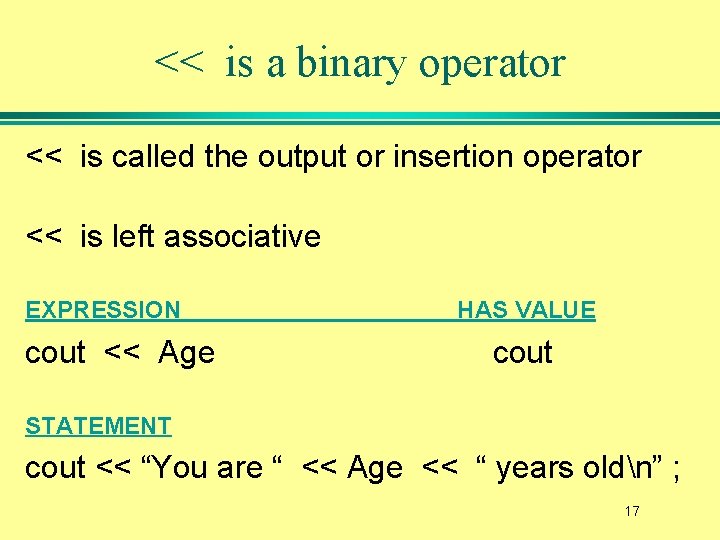
<< is a binary operator << is called the output or insertion operator << is left associative EXPRESSION cout << Age HAS VALUE cout STATEMENT cout << “You are “ << Age << “ years oldn” ; 17
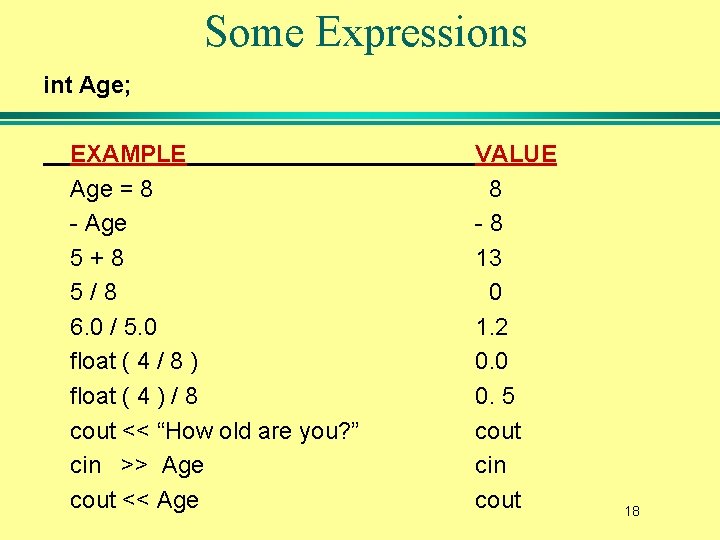
Some Expressions int Age; EXAMPLE Age = 8 - Age 5+8 5/8 6. 0 / 5. 0 float ( 4 / 8 ) float ( 4 ) / 8 cout << “How old are you? ” cin >> Age cout << Age VALUE 8 -8 13 0 1. 2 0. 0 0. 5 cout cin cout 18
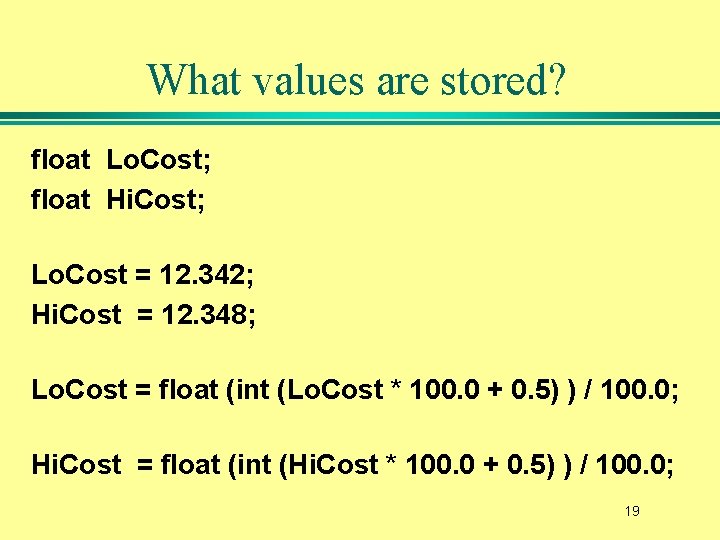
What values are stored? float Lo. Cost; float Hi. Cost; Lo. Cost = 12. 342; Hi. Cost = 12. 348; Lo. Cost = float (int (Lo. Cost * 100. 0 + 0. 5) ) / 100. 0; Hi. Cost = float (int (Hi. Cost * 100. 0 + 0. 5) ) / 100. 0; 19
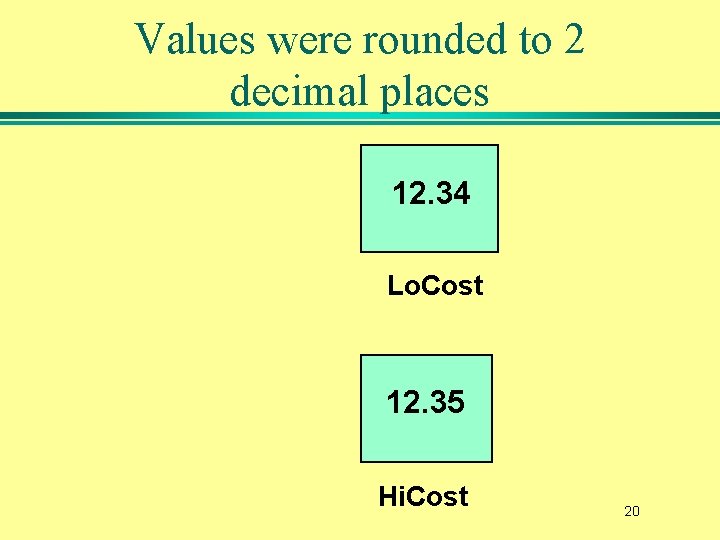
Values were rounded to 2 decimal places 12. 34 Lo. Cost 12. 35 Hi. Cost 20
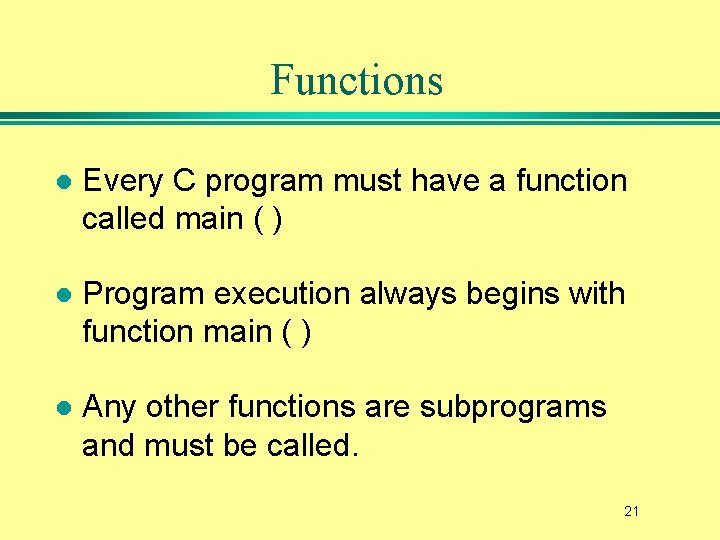
Functions l Every C program must have a function called main ( ) l Program execution always begins with function main ( ) l Any other functions are subprograms and must be called. 21
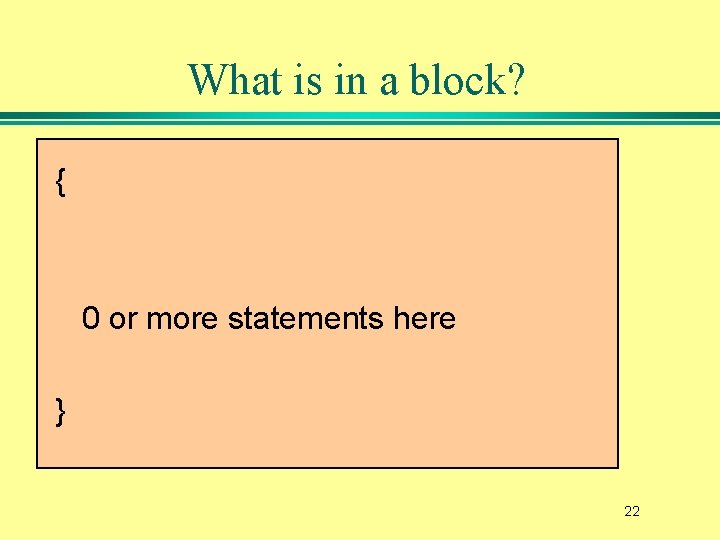
What is in a block? { 0 or more statements here } 22
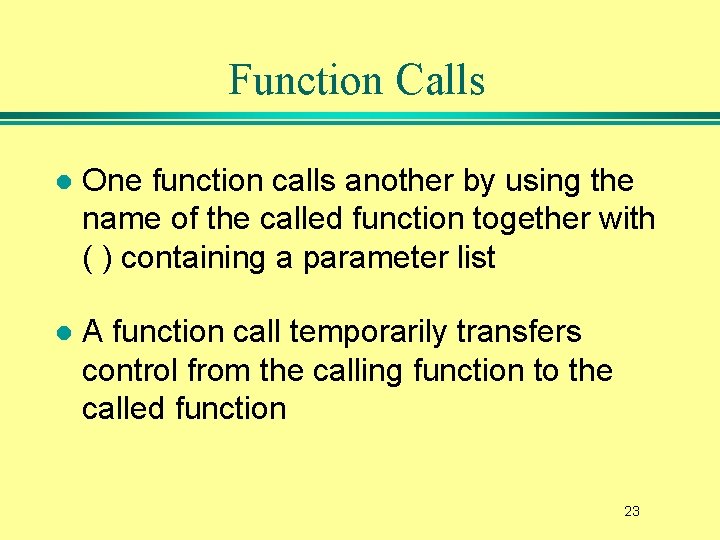
Function Calls l One function calls another by using the name of the called function together with ( ) containing a parameter list l A function call temporarily transfers control from the calling function to the called function 23
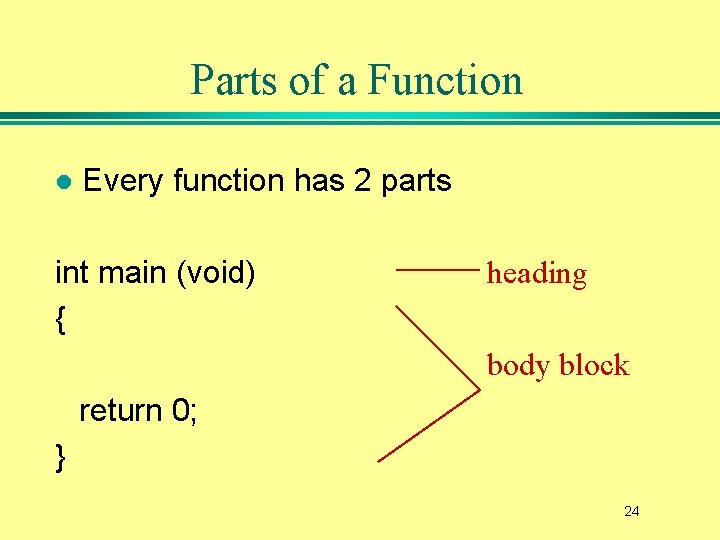
Parts of a Function l Every function has 2 parts int main (void) { heading body block return 0; } 24
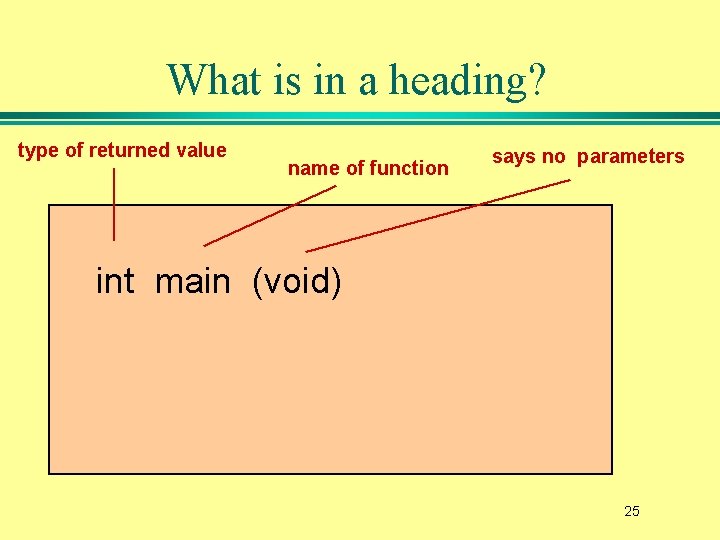
What is in a heading? type of returned value name of function says no parameters int main (void) 25
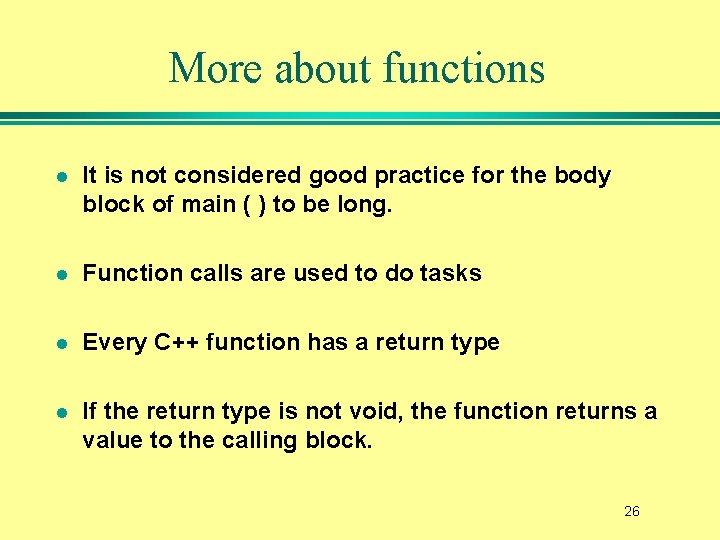
More about functions l It is not considered good practice for the body block of main ( ) to be long. l Function calls are used to do tasks l Every C++ function has a return type l If the return type is not void, the function returns a value to the calling block. 26
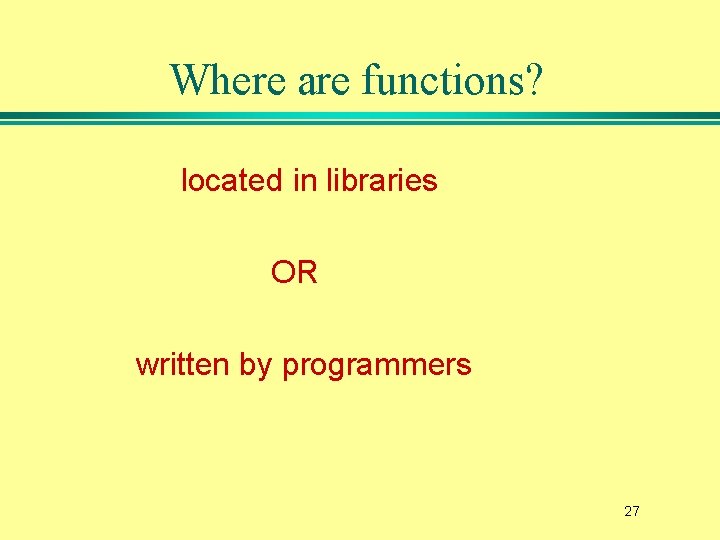
Where are functions? located in libraries OR written by programmers 27
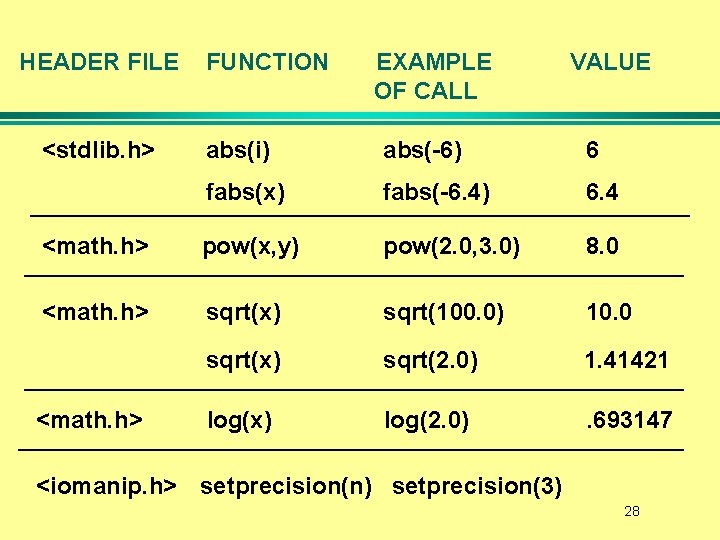
HEADER FILE <stdlib. h> FUNCTION EXAMPLE OF CALL VALUE abs(i) abs(-6) 6 fabs(x) fabs(-6. 4) 6. 4 <math. h> pow(x, y) pow(2. 0, 3. 0) 8. 0 <math. h> sqrt(x) sqrt(100. 0) 10. 0 sqrt(x) sqrt(2. 0) 1. 41421 log(x) log(2. 0) . 693147 <math. h> <iomanip. h> setprecision(n) setprecision(3) 28
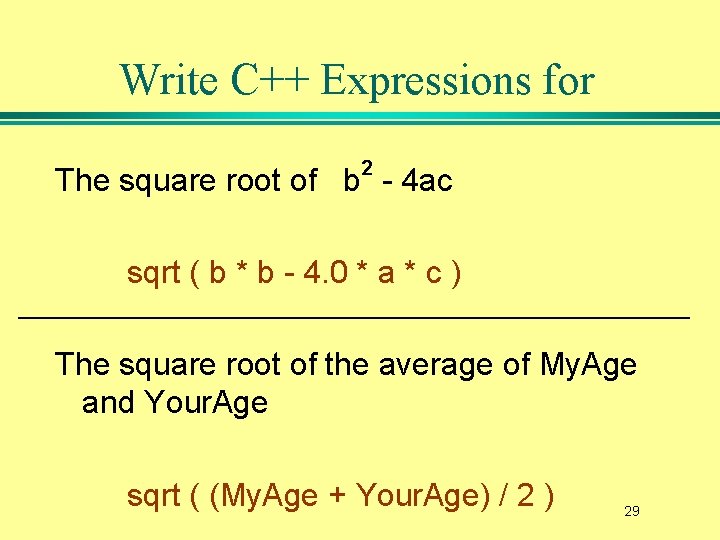
Write C++ Expressions for 2 The square root of b - 4 ac sqrt ( b * b - 4. 0 * a * c ) The square root of the average of My. Age and Your. Age sqrt ( (My. Age + Your. Age) / 2 ) 29
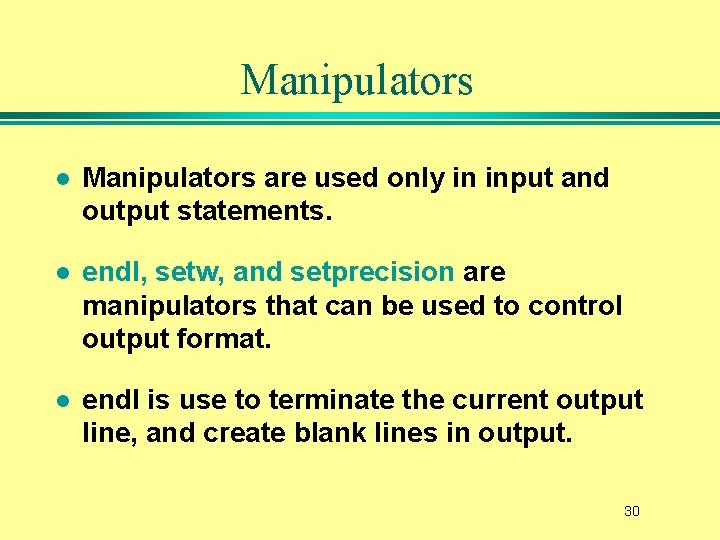
Manipulators l Manipulators are used only in input and output statements. l endl, setw, and setprecision are manipulators that can be used to control output format. l endl is use to terminate the current output line, and create blank lines in output. 30
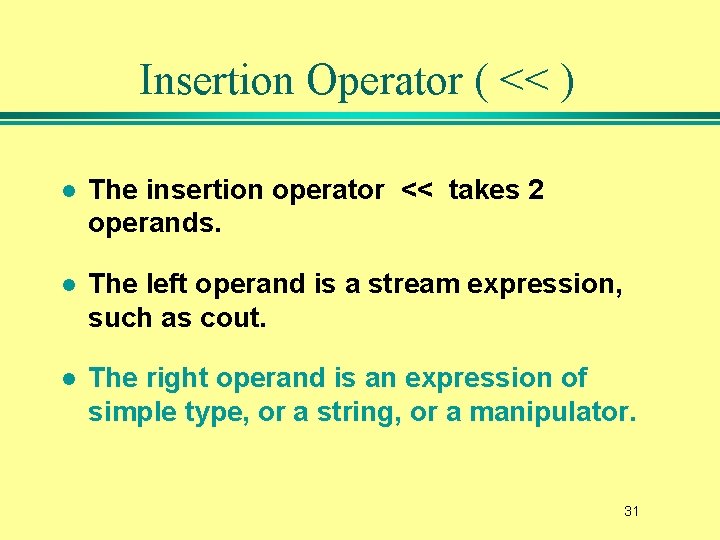
Insertion Operator ( << ) l The insertion operator << takes 2 operands. l The left operand is a stream expression, such as cout. l The right operand is an expression of simple type, or a string, or a manipulator. 31
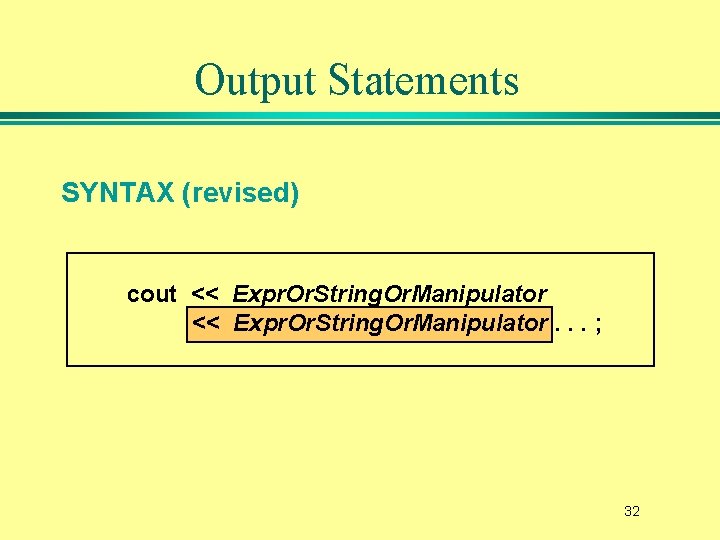
Output Statements SYNTAX (revised) cout << Expr. Or. String. Or. Manipulator. . . ; 32
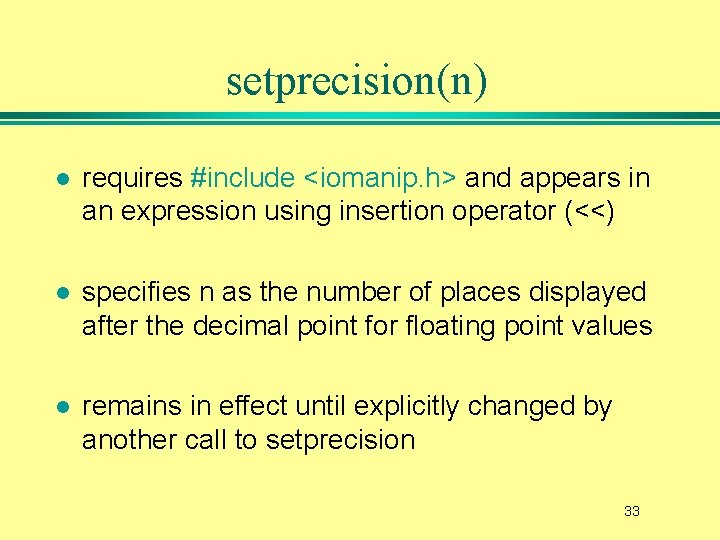
setprecision(n) l requires #include <iomanip. h> and appears in an expression using insertion operator (<<) l specifies n as the number of places displayed after the decimal point for floating point values l remains in effect until explicitly changed by another call to setprecision 33
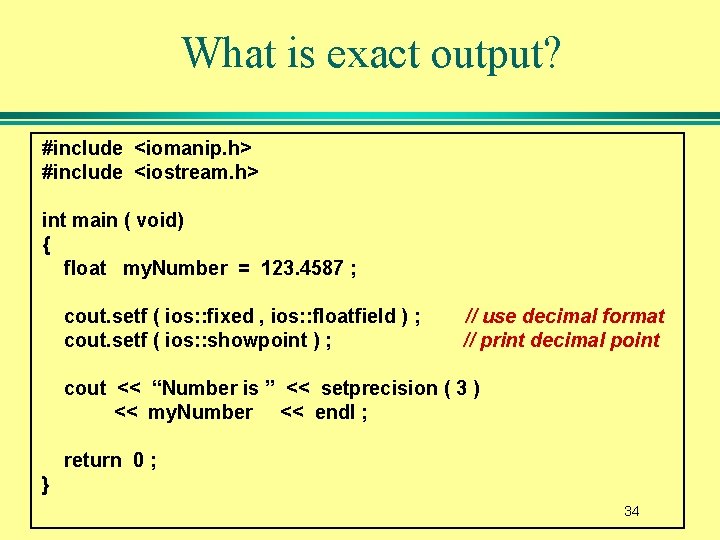
What is exact output? #include <iomanip. h> #include <iostream. h> int main ( void) { float my. Number = 123. 4587 ; cout. setf ( ios: : fixed , ios: : floatfield ) ; cout. setf ( ios: : showpoint ) ; // use decimal format // print decimal point cout << “Number is ” << setprecision ( 3 ) << my. Number << endl ; return 0 ; } 34
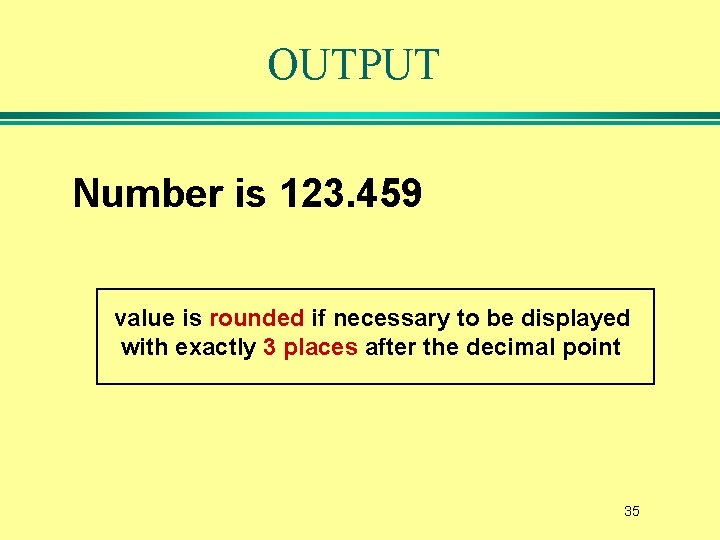
OUTPUT Number is 123. 459 value is rounded if necessary to be displayed with exactly 3 places after the decimal point 35
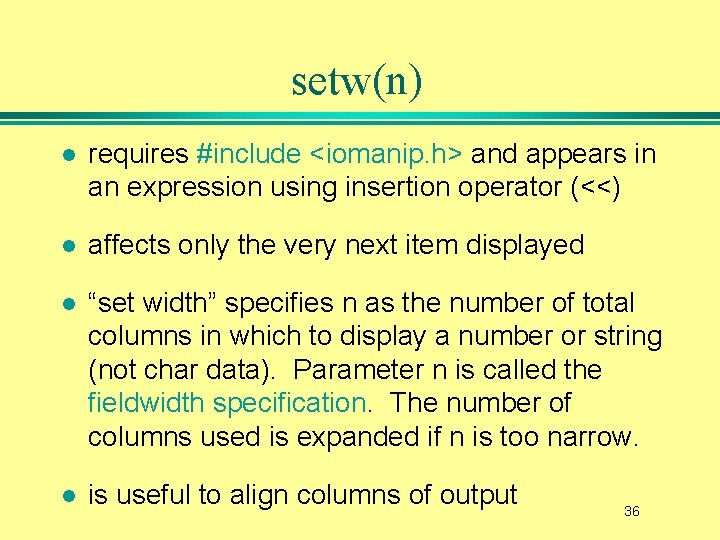
setw(n) l requires #include <iomanip. h> and appears in an expression using insertion operator (<<) l affects only the very next item displayed l “set width” specifies n as the number of total columns in which to display a number or string (not char data). Parameter n is called the fieldwidth specification. The number of columns used is expanded if n is too narrow. l is useful to align columns of output 36
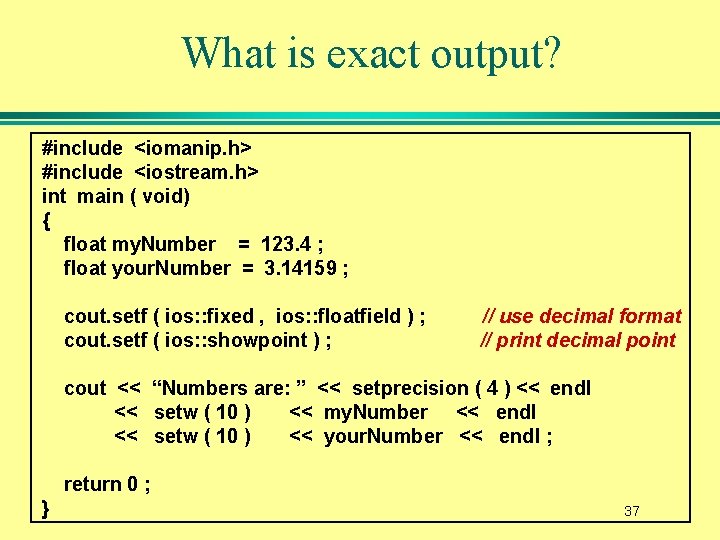
What is exact output? #include <iomanip. h> #include <iostream. h> int main ( void) { float my. Number = 123. 4 ; float your. Number = 3. 14159 ; cout. setf ( ios: : fixed , ios: : floatfield ) ; cout. setf ( ios: : showpoint ) ; // use decimal format // print decimal point cout << “Numbers are: ” << setprecision ( 4 ) << endl << setw ( 10 ) << my. Number << endl << setw ( 10 ) << your. Number << endl ; return 0 ; } 37
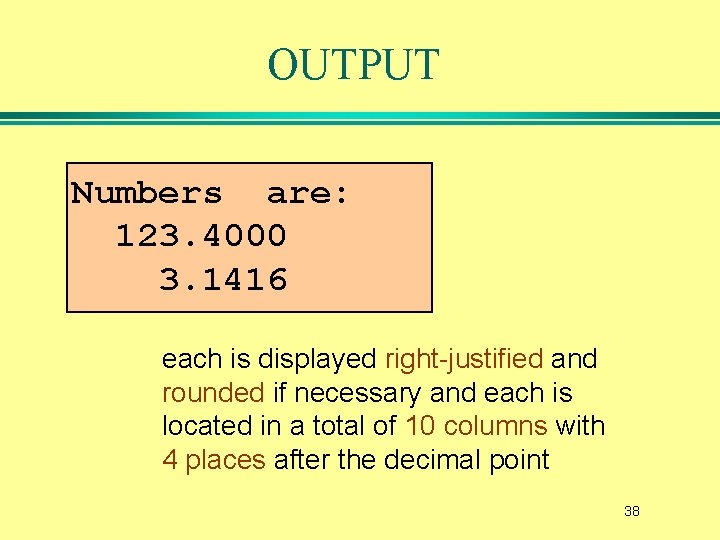
OUTPUT Numbers are: 123. 4000 3. 1416 each is displayed right-justified and rounded if necessary and each is located in a total of 10 columns with 4 places after the decimal point 38
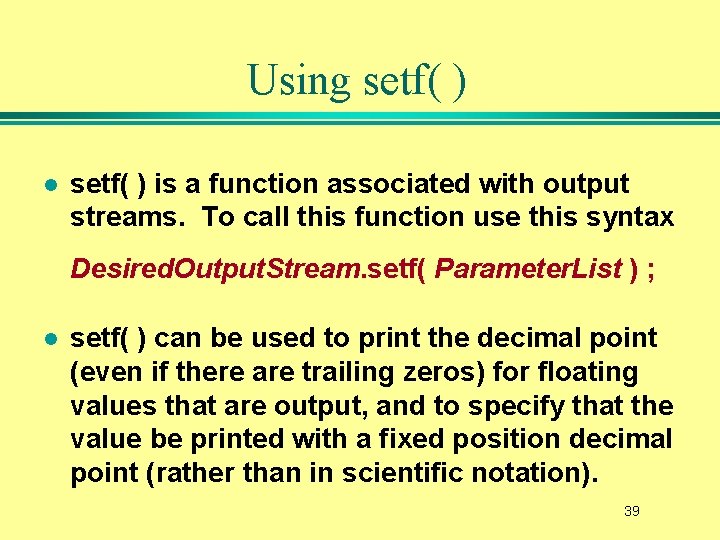
Using setf( ) l setf( ) is a function associated with output streams. To call this function use this syntax Desired. Output. Stream. setf( Parameter. List ) ; l setf( ) can be used to print the decimal point (even if there are trailing zeros) for floating values that are output, and to specify that the value be printed with a fixed position decimal point (rather than in scientific notation). 39
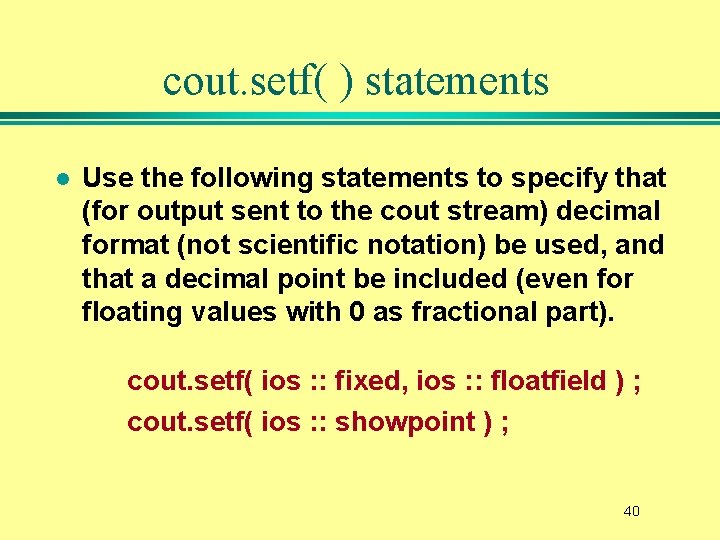
cout. setf( ) statements l Use the following statements to specify that (for output sent to the cout stream) decimal format (not scientific notation) be used, and that a decimal point be included (even for floating values with 0 as fractional part). cout. setf( ios : : fixed, ios : : floatfield ) ; cout. setf( ios : : showpoint ) ; 40
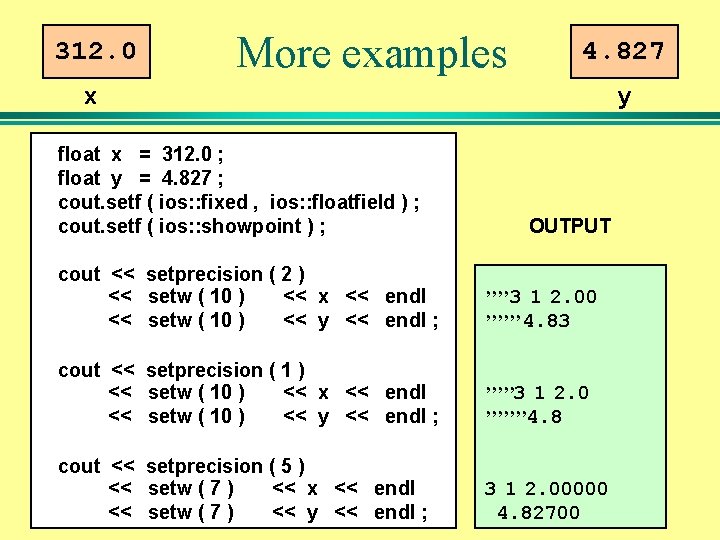
312. 0 More examples 4. 827 x float x = 312. 0 ; float y = 4. 827 ; cout. setf ( ios: : fixed , ios: : floatfield ) ; cout. setf ( ios: : showpoint ) ; y OUTPUT cout << setprecision ( 2 ) << setw ( 10 ) << x << endl << setw ( 10 ) << y << endl ; ’’’’ 3 1 2. 00 ’’’’’’ 4. 83 cout << setprecision ( 1 ) << setw ( 10 ) << x << endl << setw ( 10 ) << y << endl ; ’’’’’ 3 1 2. 0 ’’’’’’’ 4. 8 cout << setprecision ( 5 ) << setw ( 7 ) << x << endl << setw ( 7 ) << y << endl ; 3 1 2. 00000 4. 82700 41
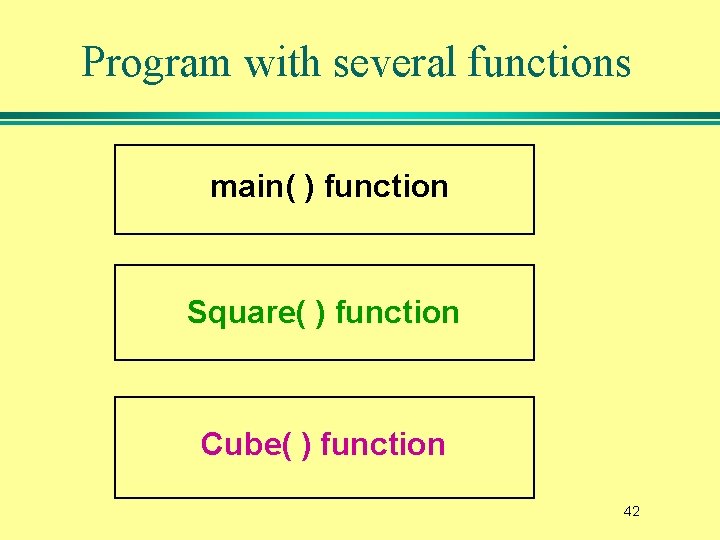
Program with several functions main( ) function Square( ) function Cube( ) function 42
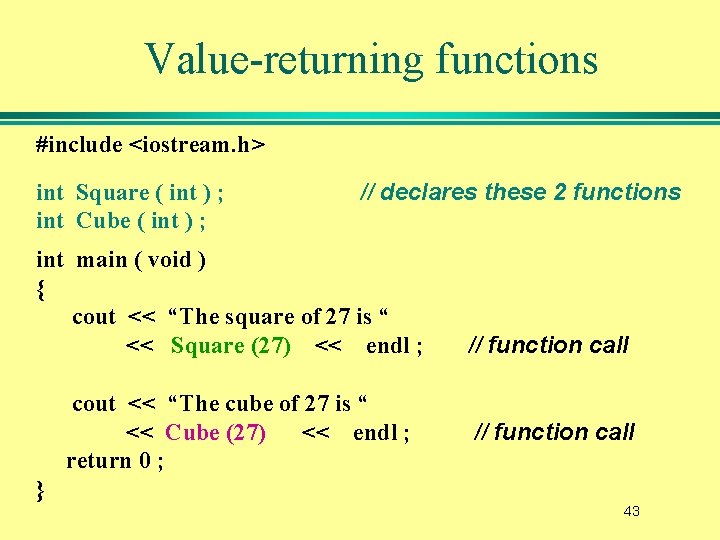
Value-returning functions #include <iostream. h> int Square ( int ) ; int Cube ( int ) ; // declares these 2 functions int main ( void ) { cout << “The square of 27 is “ << Square (27) << endl ; cout << “The cube of 27 is “ << Cube (27) << endl ; return 0 ; // function call } 43
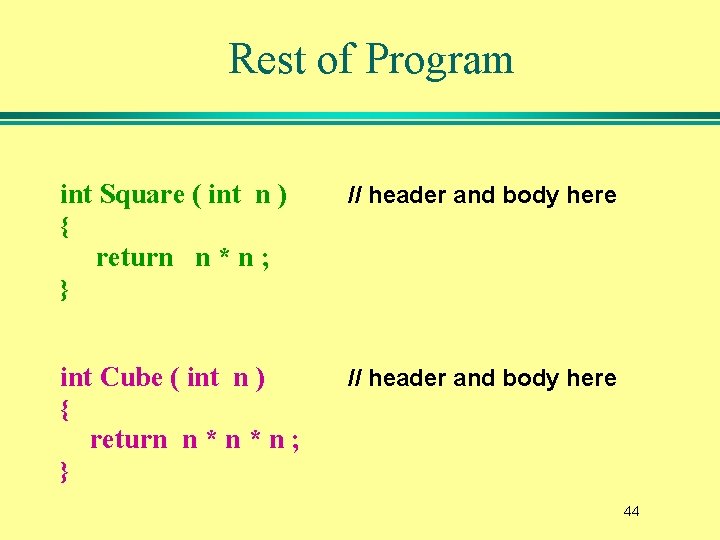
Rest of Program int Square ( int n ) // header and body here { return n * n ; } int Cube ( int n ) // header and body here { return n * n ; } 44
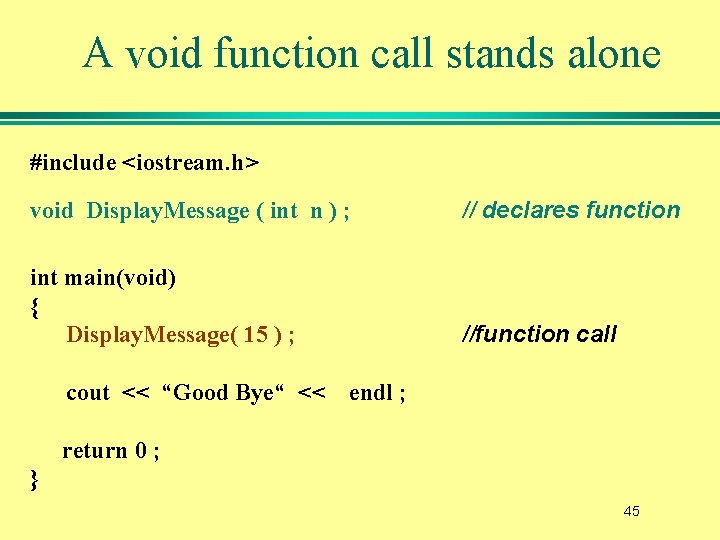
A void function call stands alone #include <iostream. h> void Display. Message ( int n ) ; // declares function int main(void) { Display. Message( 15 ) ; //function call cout << “Good Bye“ << endl ; return 0 ; } 45
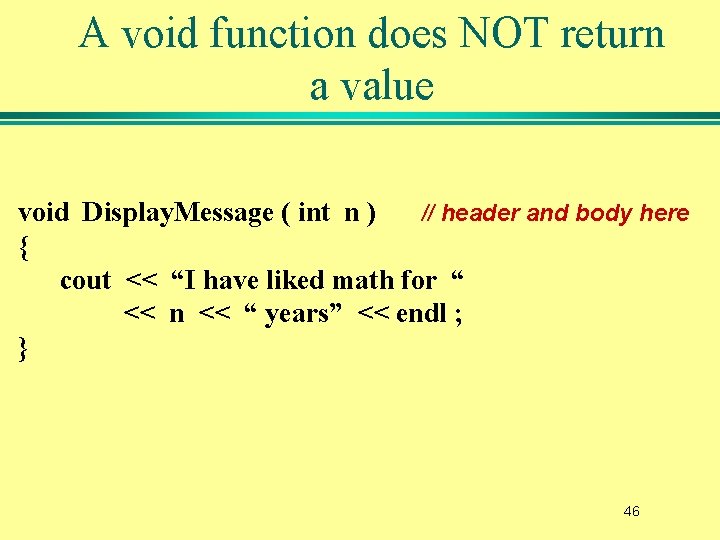
A void function does NOT return a value void Display. Message ( int n ) // header and body here { cout << “I have liked math for “ << n << “ years” << endl ; } 46
Write variable expressions for arithmetic sequences
Pointer expressions
Arithmetic expressions containing a null value evaluate to
Arithmetic expressions containing a null value evaluate to
Perbedaan linear programming dan integer programming
Greedy vs dynamic
Runtime programming
Integer programming vs linear programming
Programing adalah
Chapter 9 rational expressions and equations answers
Lesson 2 the distributive property
Chapter 1 expressions equations and inequalities
Chapter 1 expressions equations and inequalities
Python chapter 5
The zj row in a simplex table for maximization represents
Headless horn clause
Chapter 16 programming language
Linear programming models: graphical and computer methods
Computer programming chapter 1
Chapter 1 introduction to computers and programming
Chapter 1 introduction to computers and programming
History of python
Chapter 8 linear programming applications solutions
Chapter 2 elementary programming
Chapter 16 programming language
Chapter 1 introduction to computers and programming
10-2 arithmetic sequences and series
How to write recursive formula for arithmetic sequence
Two's complement
Calculations using balanced equations are called
Sum notation
The sequence
Arithmetic explicit formula
Proof of arithmetic series formula
Sum of arithmetic progression formula
Partial sum formula
Geometric and arithmetic sequences
Fermat's little theorem problems with solutions
Selective set operation with example
Recursive sequence formula
Ap and gp solved problems
Unifunction and multifunction pipeline
Positional number system in computer
What does arithmetic density tell us
Exponential vs geometric growth
Vektor dot
Arithmetic right shift