Programming in C DaleWeemsHeadington Chapter 11 OneDimensional Arrays
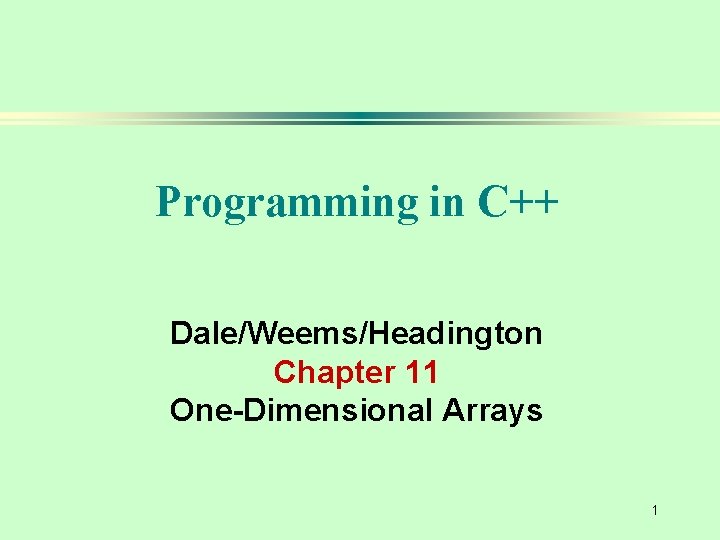
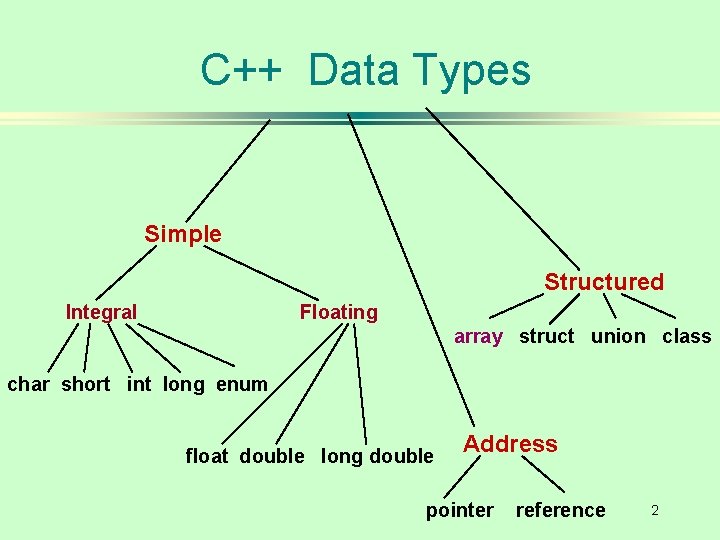
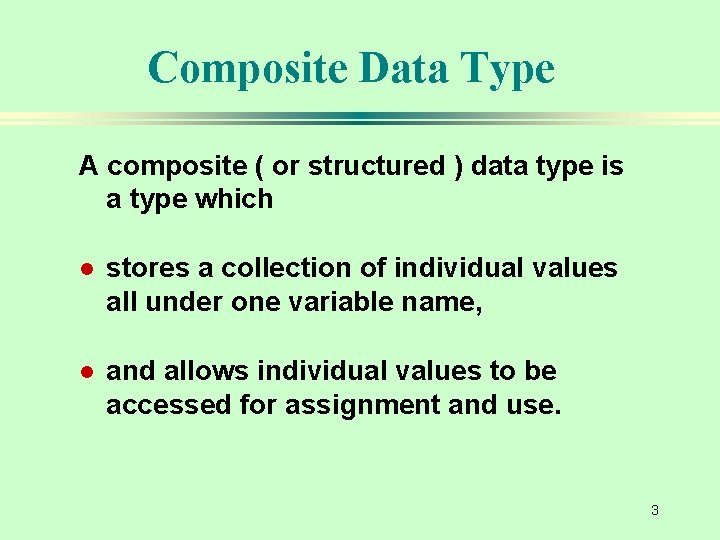
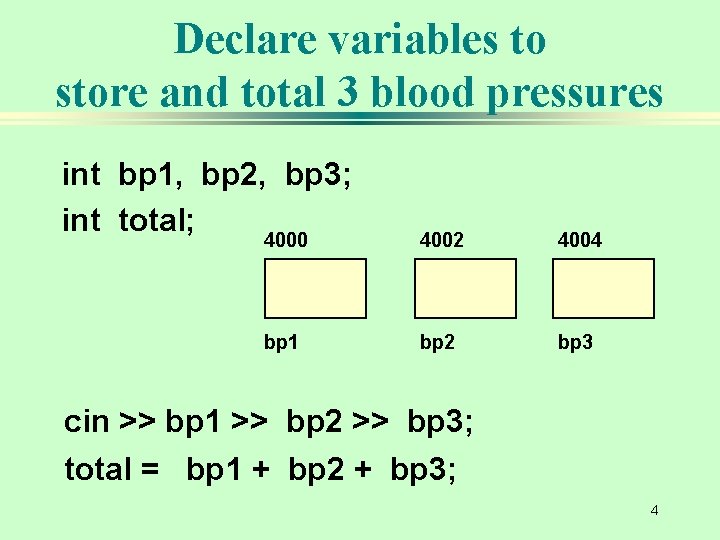
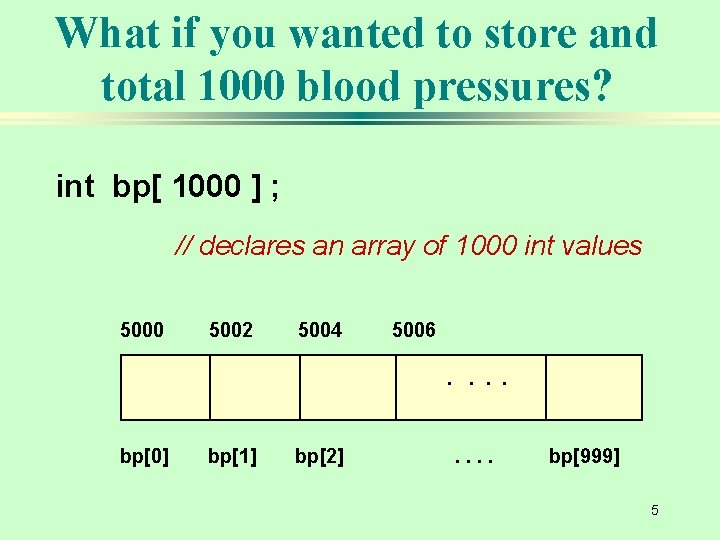
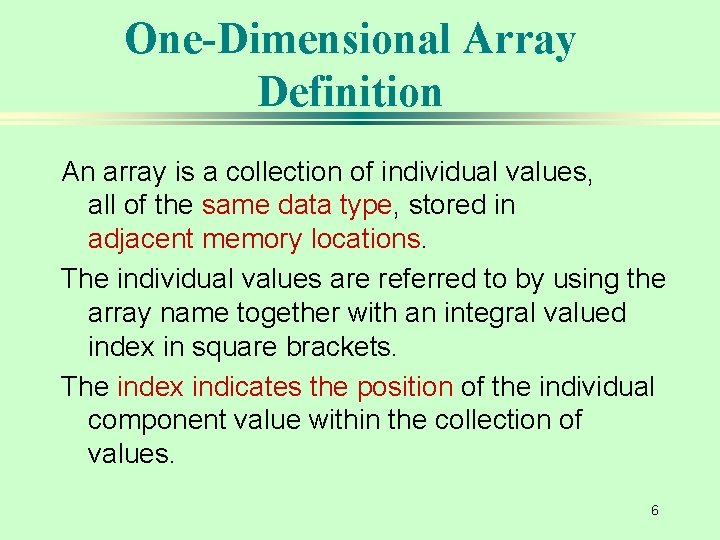
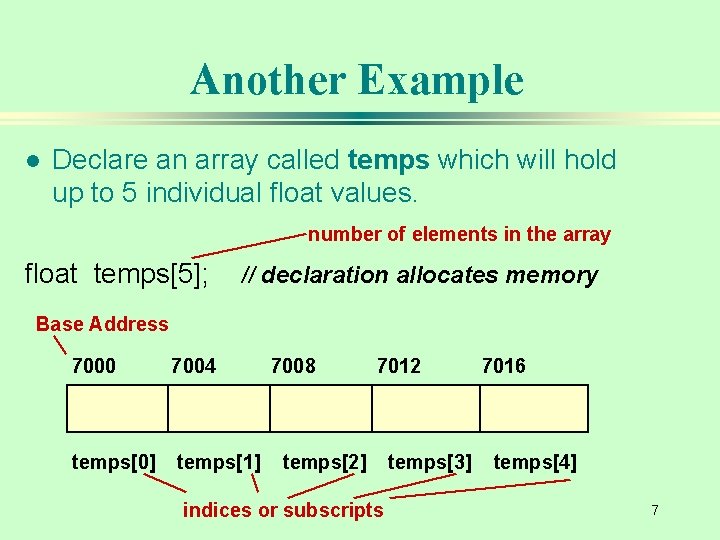
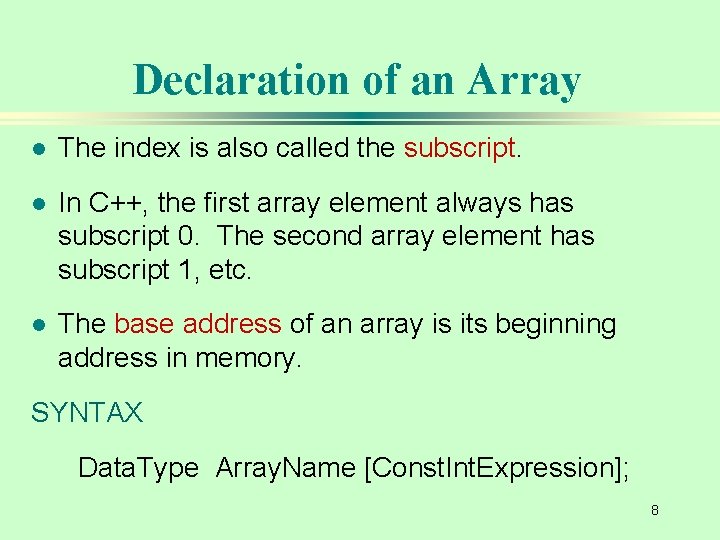
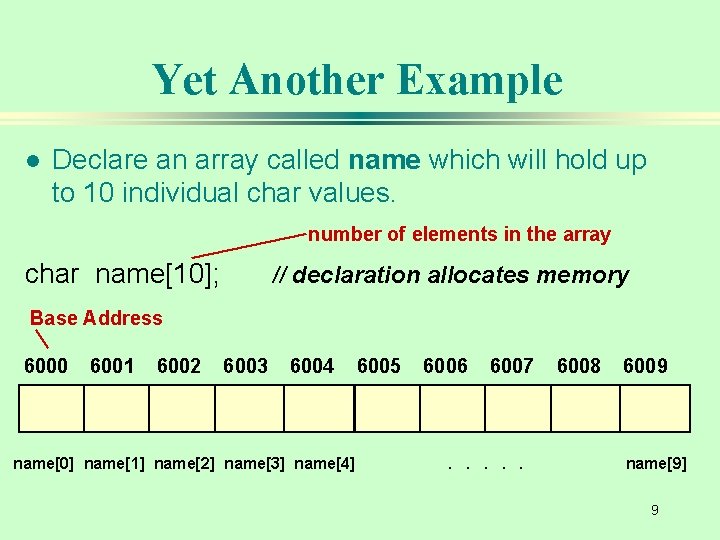
![Assigning values to individual array elements float temps[ 5 ] ; // allocates memory Assigning values to individual array elements float temps[ 5 ] ; // allocates memory](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-10.jpg)
![What values are assigned? float temps[ 5 ] ; int m ; // allocates What values are assigned? float temps[ 5 ] ; int m ; // allocates](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-11.jpg)
![Now what values are printed? float temps[ 5 ] ; // allocates memory for Now what values are printed? float temps[ 5 ] ; // allocates memory for](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-12.jpg)
![Variable subscripts float temps[ 5 ] ; int m = 3 ; . . Variable subscripts float temps[ 5 ] ; int m = 3 ; . .](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-13.jpg)
![A Closer Look at the Compiler float temps[5]; // this declaration allocates memory To A Closer Look at the Compiler float temps[5]; // this declaration allocates memory To](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-14.jpg)
![Initializing in a declaration int ages[ 5 ] = { 40, 13, 20, 19, Initializing in a declaration int ages[ 5 ] = { 40, 13, 20, 19,](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-15.jpg)
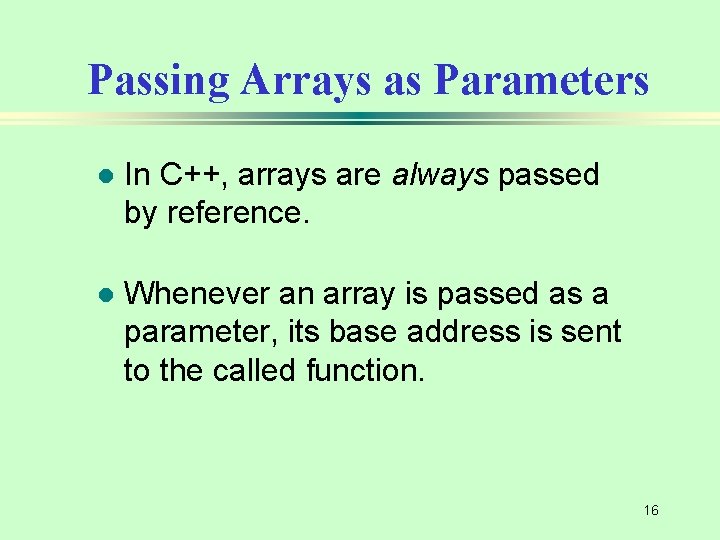
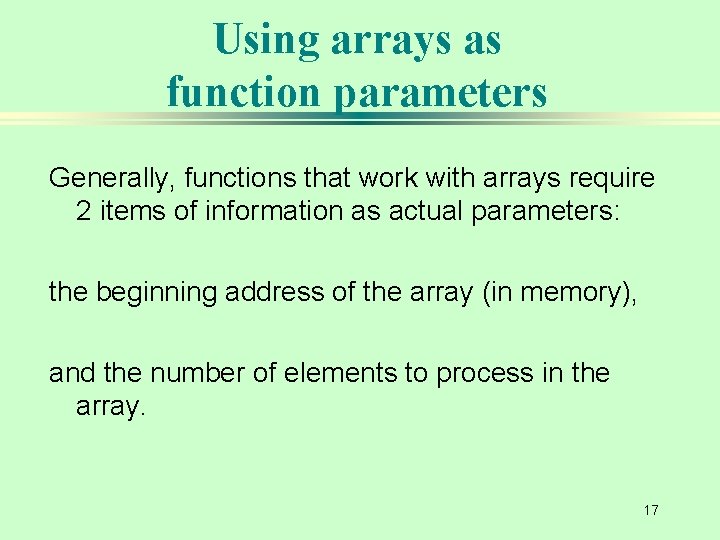
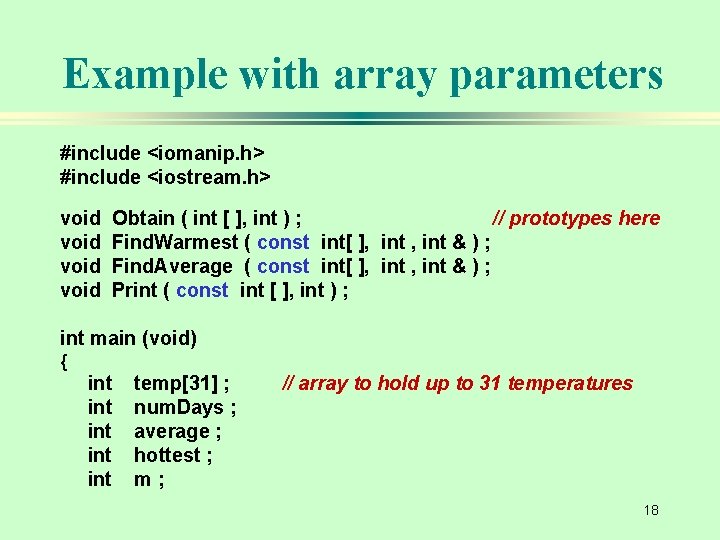
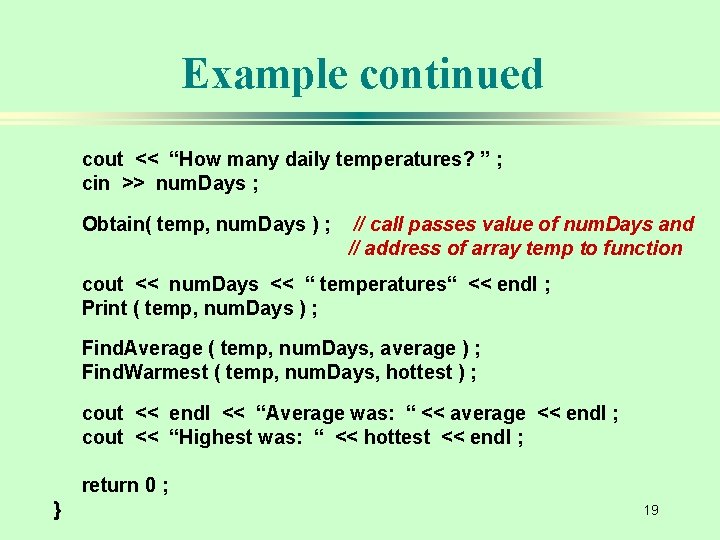
![Memory allocated for array int temp[31] ; // array to hold up to 31 Memory allocated for array int temp[31] ; // array to hold up to 31](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-20.jpg)
![void Obtain ( /* out */ int temp [ ] , /* in */ void Obtain ( /* out */ int temp [ ] , /* in */](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-21.jpg)
![void Print ( /* in */ const int temp [ ] , /* in void Print ( /* in */ const int temp [ ] , /* in](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-22.jpg)
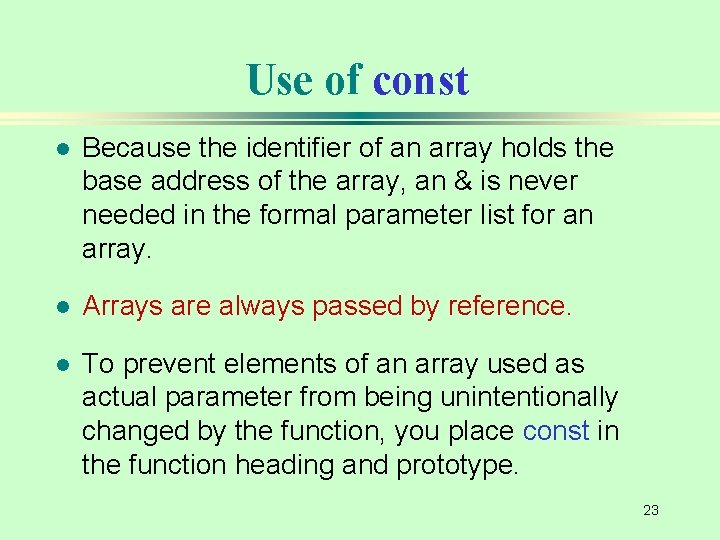
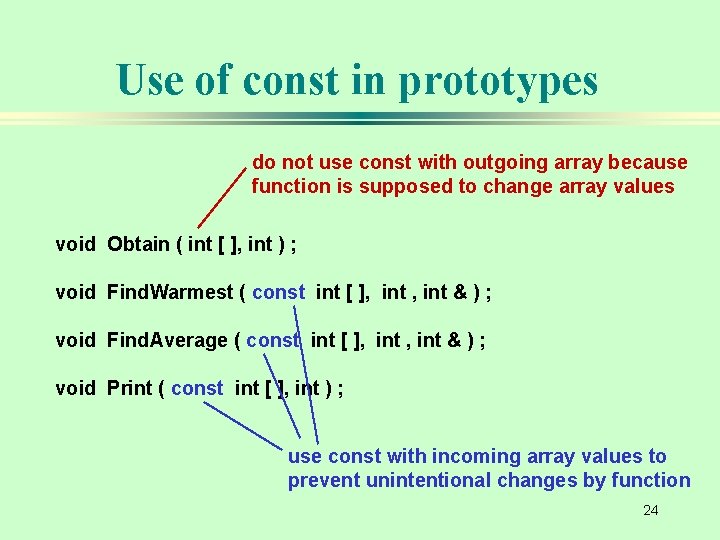
![void Find. Average ( /* in */ const int temp [ ] , /* void Find. Average ( /* in */ const int temp [ ] , /*](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-25.jpg)
![void Find. Warmest ( /* in */ const int temp [ ] , /* void Find. Warmest ( /* in */ const int temp [ ] , /*](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-26.jpg)
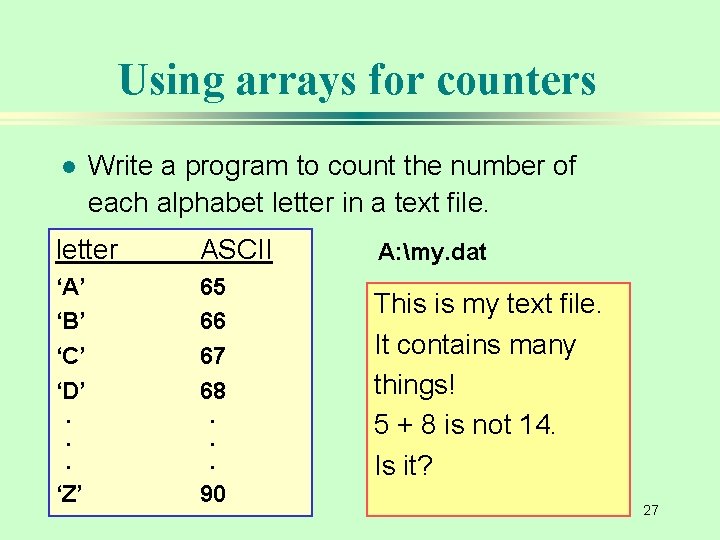
![const int SIZE 91 ; int freq. Count [ SIZE ] ; freq. Count const int SIZE 91 ; int freq. Count [ SIZE ] ; freq. Count](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-28.jpg)
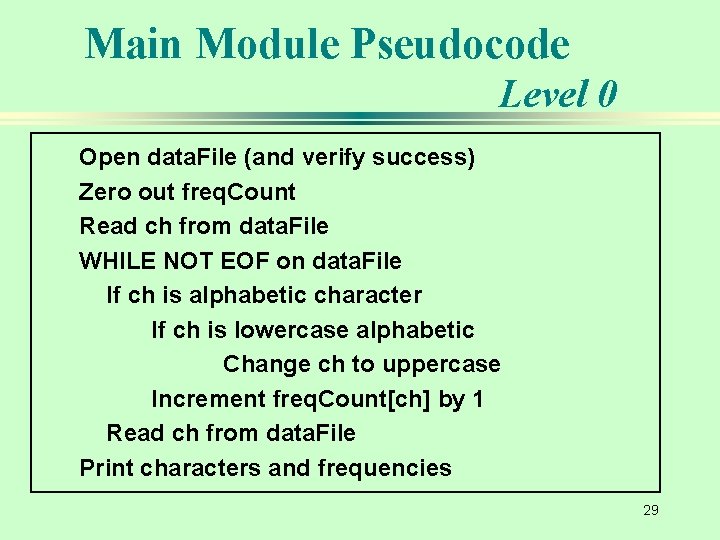
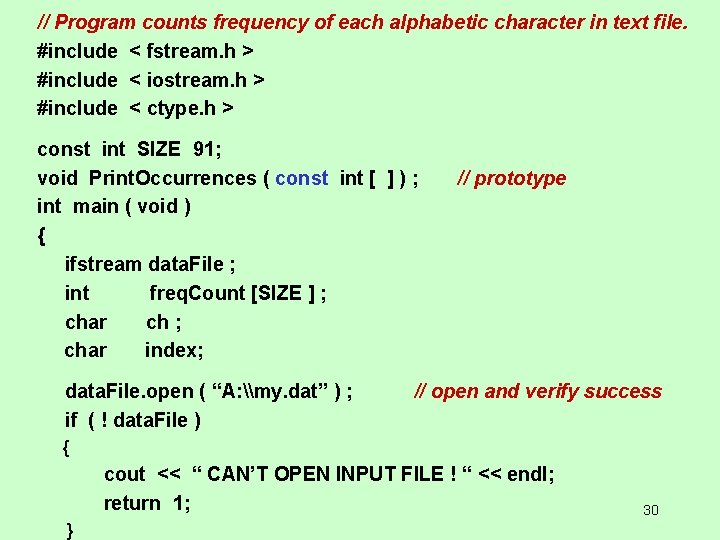
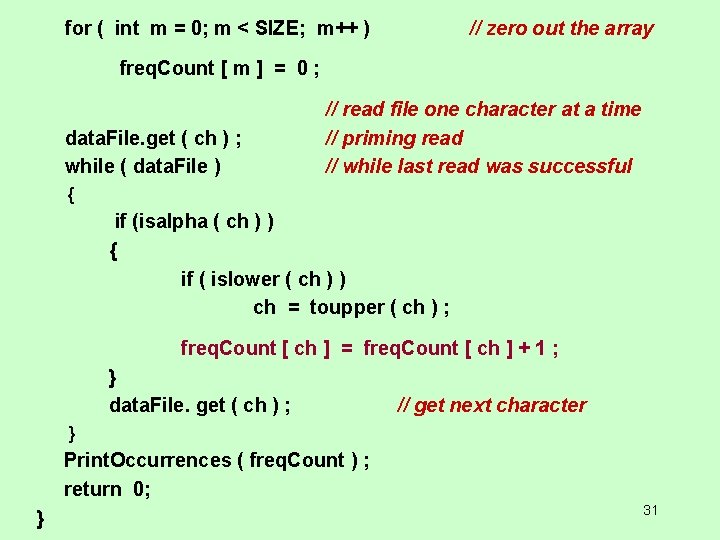
![void Print. Occurrences ( /* in */ const int freq. Count [ ] ) void Print. Occurrences ( /* in */ const int freq. Count [ ] )](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-32.jpg)
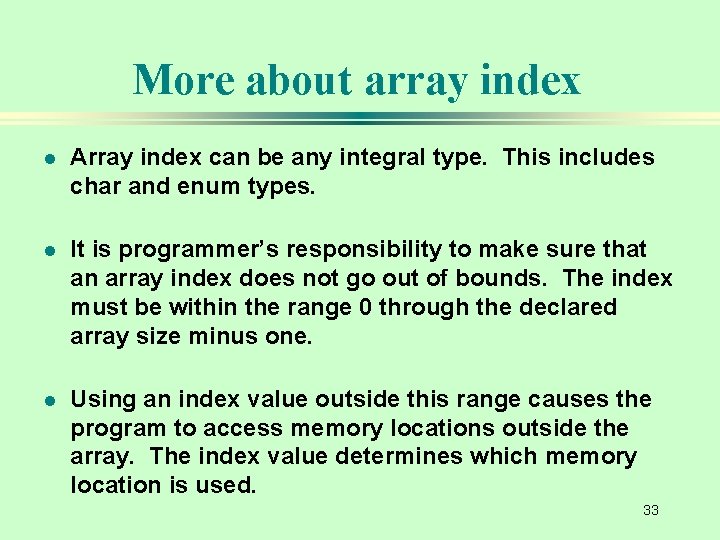
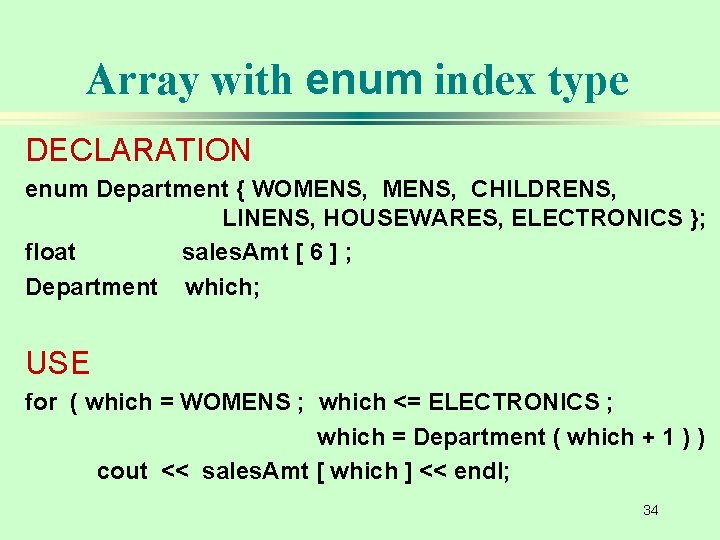
![float sales. Amt [6]; sales. Amt [ WOMENS ] ( i. e. sales. Amt float sales. Amt [6]; sales. Amt [ WOMENS ] ( i. e. sales. Amt](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-35.jpg)
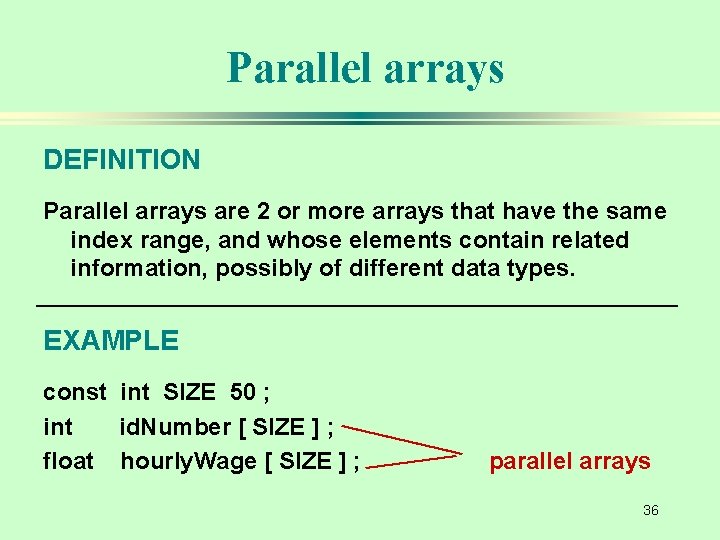
![const int SIZE 50 ; int id. Number [ SIZE ] ; float hourly. const int SIZE 50 ; int id. Number [ SIZE ] ; float hourly.](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-37.jpg)
- Slides: 37
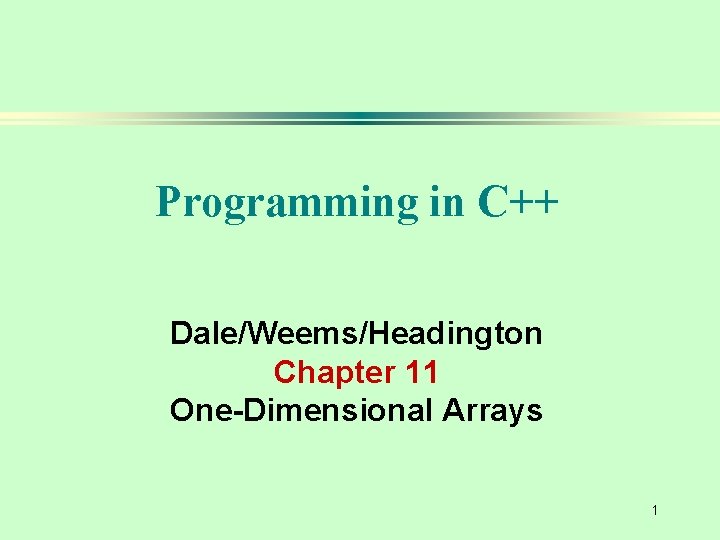
Programming in C++ Dale/Weems/Headington Chapter 11 One-Dimensional Arrays 1
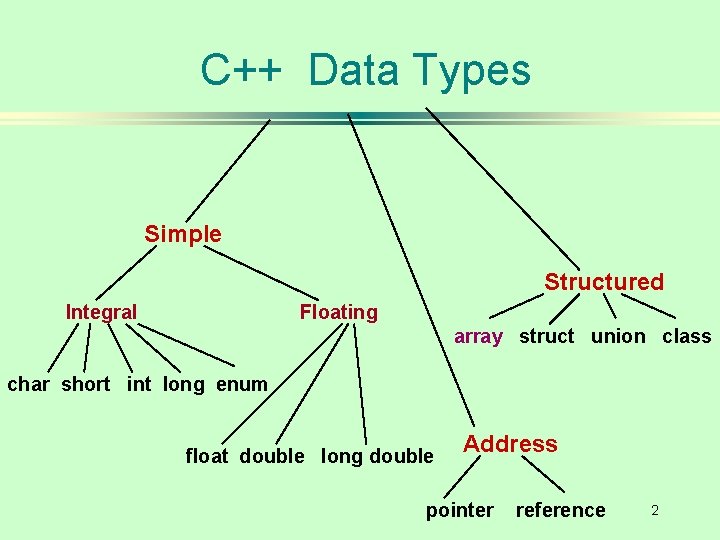
C++ Data Types Simple Structured Integral Floating array struct union class char short int long enum float double long double Address pointer reference 2
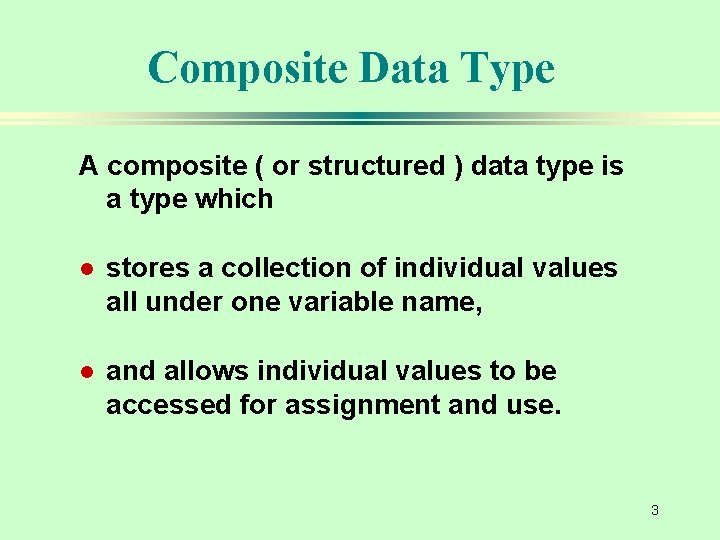
Composite Data Type A composite ( or structured ) data type is a type which l stores a collection of individual values all under one variable name, l and allows individual values to be accessed for assignment and use. 3
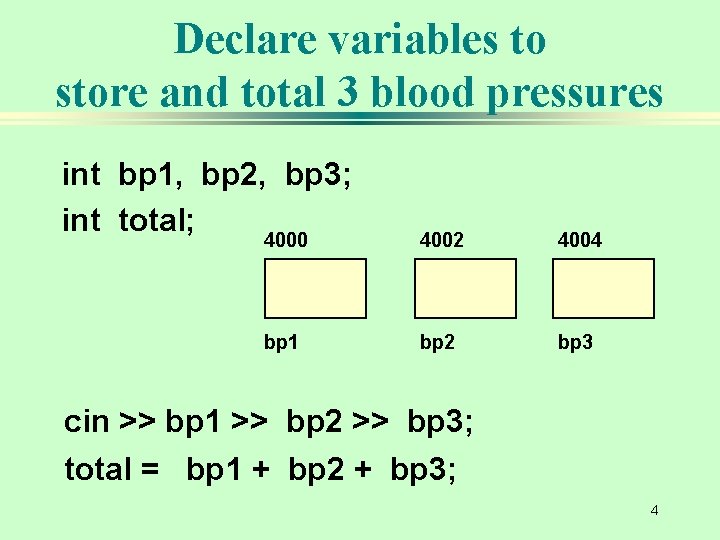
Declare variables to store and total 3 blood pressures int bp 1, bp 2, bp 3; int total; 4000 4002 4004 bp 1 bp 2 bp 3 cin >> bp 1 >> bp 2 >> bp 3; total = bp 1 + bp 2 + bp 3; 4
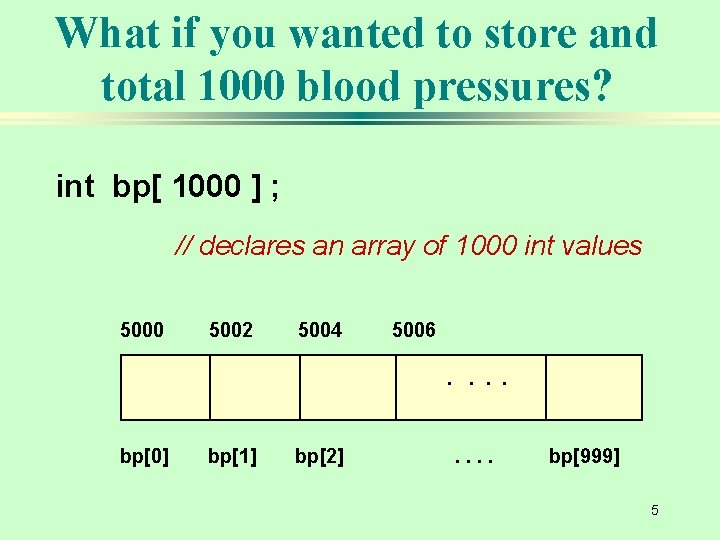
What if you wanted to store and total 1000 blood pressures? int bp[ 1000 ] ; // declares an array of 1000 int values 5000 5002 5004 5006. . bp[0] bp[1] bp[2] . . bp[999] 5
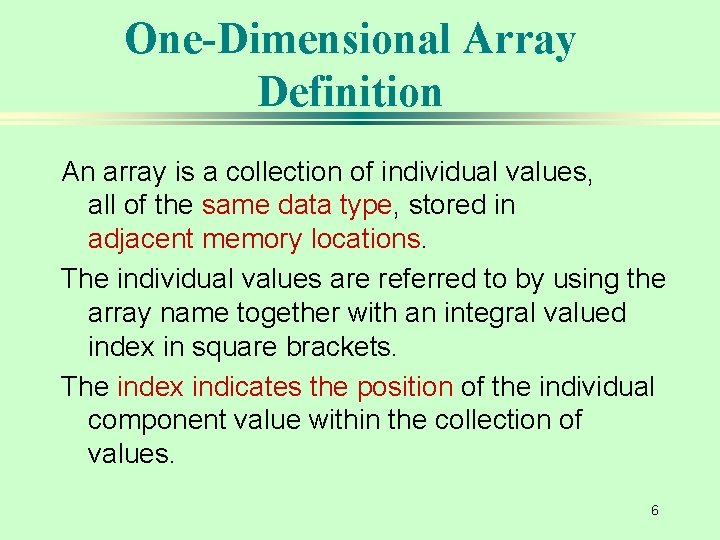
One-Dimensional Array Definition An array is a collection of individual values, all of the same data type, stored in adjacent memory locations. The individual values are referred to by using the array name together with an integral valued index in square brackets. The index indicates the position of the individual component value within the collection of values. 6
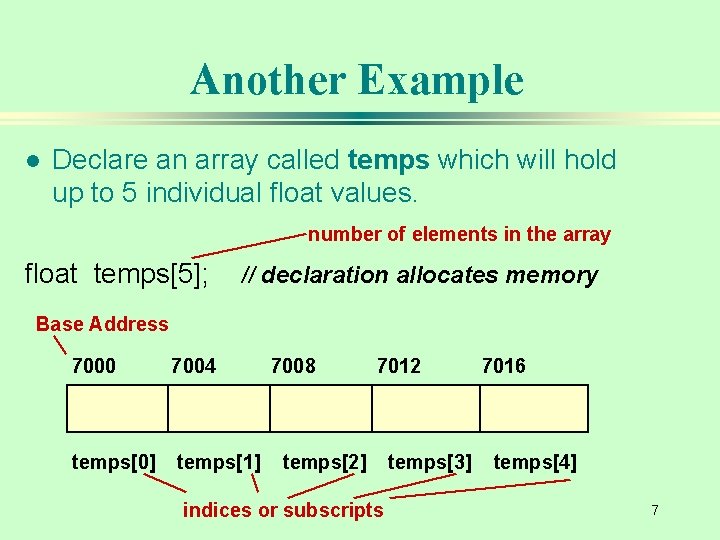
Another Example l Declare an array called temps which will hold up to 5 individual float values. number of elements in the array float temps[5]; // declaration allocates memory Base Address 7000 temps[0] 7004 temps[1] 7008 7012 temps[2] indices or subscripts temps[3] 7016 temps[4] 7
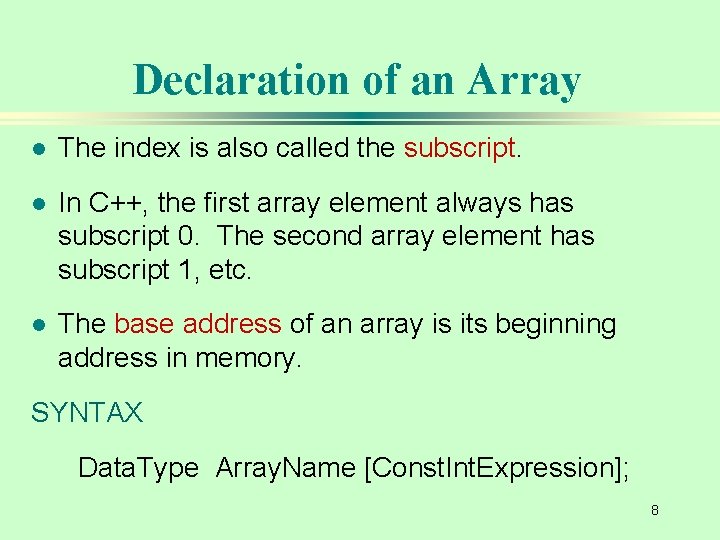
Declaration of an Array l The index is also called the subscript. l In C++, the first array element always has subscript 0. The second array element has subscript 1, etc. l The base address of an array is its beginning address in memory. SYNTAX Data. Type Array. Name [Const. Int. Expression]; 8
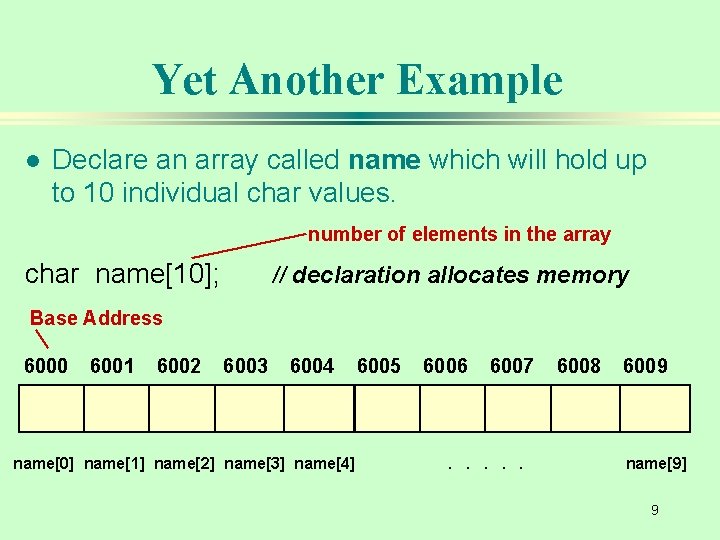
Yet Another Example l Declare an array called name which will hold up to 10 individual char values. number of elements in the array char name[10]; // declaration allocates memory Base Address 6000 6001 6002 6003 6004 name[0] name[1] name[2] name[3] name[4] 6005 6006 6007 . . . 6008 6009 name[9] 9
![Assigning values to individual array elements float temps 5 allocates memory Assigning values to individual array elements float temps[ 5 ] ; // allocates memory](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-10.jpg)
Assigning values to individual array elements float temps[ 5 ] ; // allocates memory for array int m = 4 ; temps[ 2 ] = 98. 6 ; temps[ 3 ] = 101. 2 ; temps[ 0 ] = 99. 4 ; temps[ m ] = temps[ 3 ] / 2. 0 ; temps[ 1 ] = temps[ 3 ] - 1. 2 ; // what value is assigned? 7000 99. 4 temps[0] 7004 7008 ? 98. 6 temps[1] temps[2] 7012 101. 2 temps[3] 7016 50. 6 temps[4] 10
![What values are assigned float temps 5 int m allocates What values are assigned? float temps[ 5 ] ; int m ; // allocates](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-11.jpg)
What values are assigned? float temps[ 5 ] ; int m ; // allocates memory for array for (m = 0; m < 5; m++) { temps[ m ] = 100. 0 + m * 0. 2 ; } 7000 7004 7008 7012 7016 ? ? ? temps[0] temps[1] temps[2] temps[3] temps[4] 11
![Now what values are printed float temps 5 allocates memory for Now what values are printed? float temps[ 5 ] ; // allocates memory for](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-12.jpg)
Now what values are printed? float temps[ 5 ] ; // allocates memory for array int m ; . . . for (m = 4; m >= 0; m-- ) { cout << temps[ m ] << endl ; } 7000 100. 0 temps[0] 7004 7008 7012 7016 100. 2 100. 4 100. 6 100. 8 temps[1] temps[2] temps[3] temps[4] 12
![Variable subscripts float temps 5 int m 3 Variable subscripts float temps[ 5 ] ; int m = 3 ; . .](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-13.jpg)
Variable subscripts float temps[ 5 ] ; int m = 3 ; . . . // allocates memory for array What is temps[ m + 1] ? What is temps[ m ] + 1 ? 7000 100. 0 temps[0] 7004 7008 7012 7016 100. 2 100. 4 100. 6 100. 8 temps[1] temps[2] temps[3] temps[4] 13
![A Closer Look at the Compiler float temps5 this declaration allocates memory To A Closer Look at the Compiler float temps[5]; // this declaration allocates memory To](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-14.jpg)
A Closer Look at the Compiler float temps[5]; // this declaration allocates memory To the compiler, the value of the identifier temps alone is the base address of the array. We say temps is a pointer (because its value is an address). It “points” to a memory location. 7000 7004 7008 100. 0 100. 2 100. 4 temps[0] temps[1] temps[2] 7012 7016 100. 8 temps[3] temps[4] 14
![Initializing in a declaration int ages 5 40 13 20 19 Initializing in a declaration int ages[ 5 ] = { 40, 13, 20, 19,](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-15.jpg)
Initializing in a declaration int ages[ 5 ] = { 40, 13, 20, 19, 36 } ; for ( int m = 0; m < 5; m++ ) { cout << ages[ m ] ; } 6000 40 ages[0] 6002 13 ages[1] 6004 6006 20 19 ages[2] ages[3] 6008 36 ages[4] 15
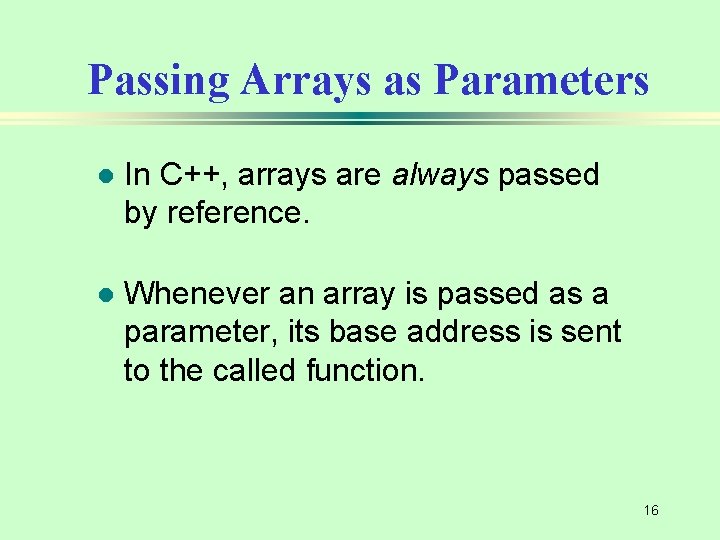
Passing Arrays as Parameters l In C++, arrays are always passed by reference. l Whenever an array is passed as a parameter, its base address is sent to the called function. 16
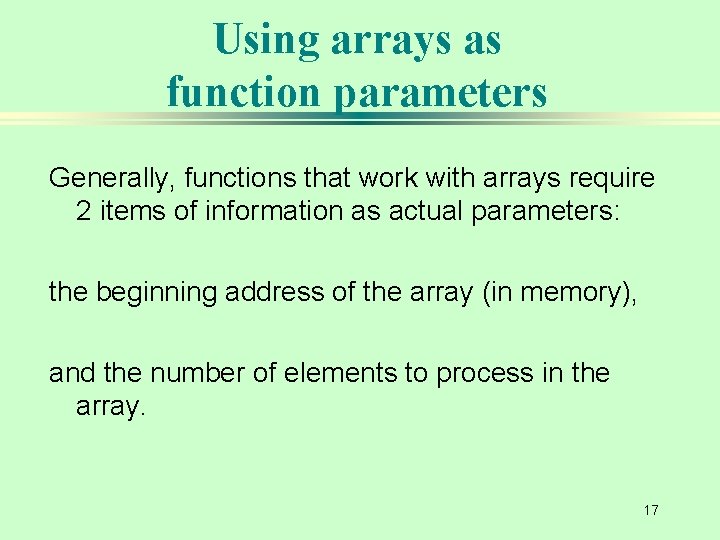
Using arrays as function parameters Generally, functions that work with arrays require 2 items of information as actual parameters: the beginning address of the array (in memory), and the number of elements to process in the array. 17
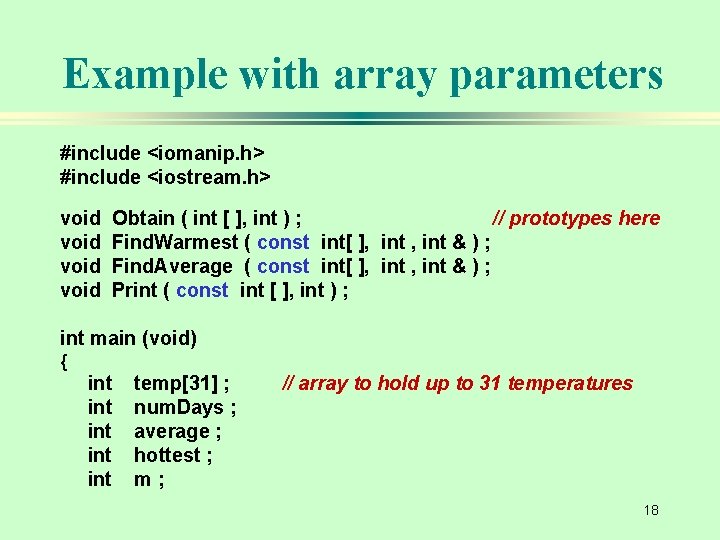
Example with array parameters #include <iomanip. h> #include <iostream. h> void Obtain ( int [ ], int ) ; // prototypes here Find. Warmest ( const int[ ], int & ) ; Find. Average ( const int[ ], int & ) ; Print ( const int [ ], int ) ; int main (void) { int int int temp[31] ; num. Days ; average ; hottest ; m; // array to hold up to 31 temperatures 18
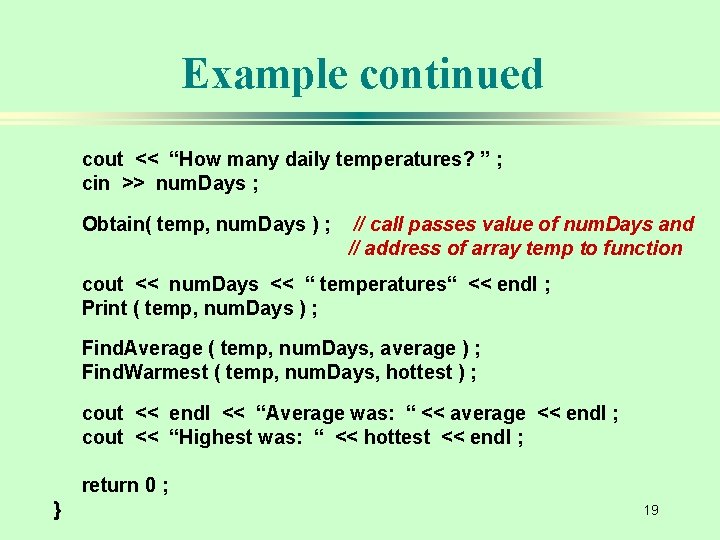
Example continued cout << “How many daily temperatures? ” ; cin >> num. Days ; Obtain( temp, num. Days ) ; // call passes value of num. Days and // address of array temp to function cout << num. Days << “ temperatures“ << endl ; Print ( temp, num. Days ) ; Find. Average ( temp, num. Days, average ) ; Find. Warmest ( temp, num. Days, hottest ) ; cout << endl << “Average was: “ << average << endl ; cout << “Highest was: “ << hottest << endl ; return 0 ; } 19
![Memory allocated for array int temp31 array to hold up to 31 Memory allocated for array int temp[31] ; // array to hold up to 31](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-20.jpg)
Memory allocated for array int temp[31] ; // array to hold up to 31 temperatures Base Address 6000 50 65 70 62 68 temp[0] temp[1] temp[2] temp[3] temp[4] . . . temp[30] 20
![void Obtain out int temp in void Obtain ( /* out */ int temp [ ] , /* in */](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-21.jpg)
void Obtain ( /* out */ int temp [ ] , /* in */ int number ) // Has user enter number temperature values at keyboard // Precondition: // number is assigned && number > 0 // Postcondition: // temp [ 0. . number -1 ] are assigned { int m; for ( m = 0 ; m < number; m++ ) { cout << “Enter a temperature : “ ; cin >> temp [m] ; } } 21
![void Print in const int temp in void Print ( /* in */ const int temp [ ] , /* in](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-22.jpg)
void Print ( /* in */ const int temp [ ] , /* in */ int number ) // Prints number temperature values to screen // Precondition: // number is assigned && number > 0 // temp [0. . number -1 ] are assigned // Postcondition: // temp [ 0. . number -1 ] have been printed 5 to a line { int m; cout << “You entered: “ ; for ( m = 0 ; m < number; m++ ) { if ( m % 5 == 0 ) cout << endl ; cout << setw(7) << temp [m] ; } } 22
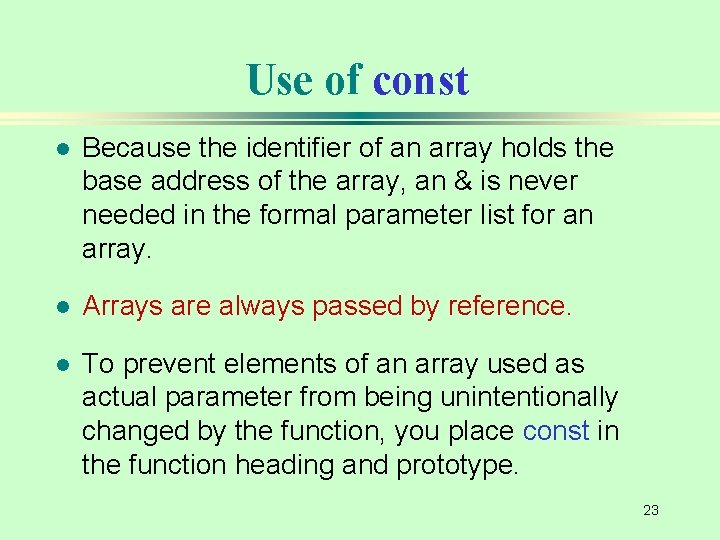
Use of const l Because the identifier of an array holds the base address of the array, an & is never needed in the formal parameter list for an array. l Arrays are always passed by reference. l To prevent elements of an array used as actual parameter from being unintentionally changed by the function, you place const in the function heading and prototype. 23
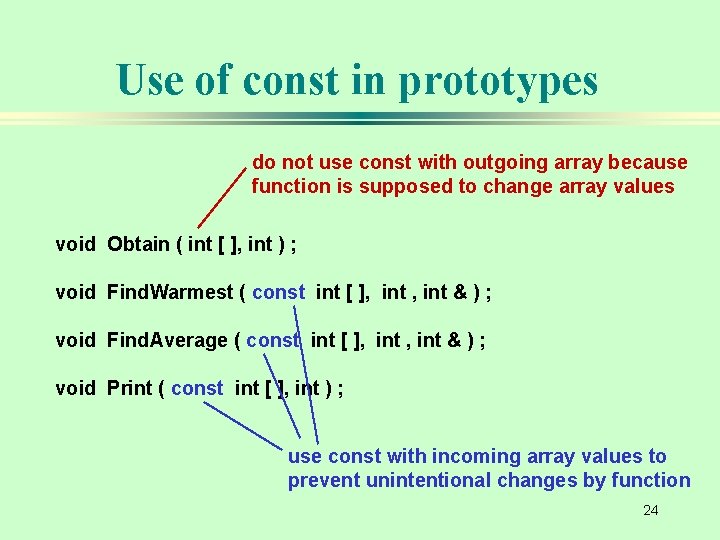
Use of const in prototypes do not use const with outgoing array because function is supposed to change array values void Obtain ( int [ ], int ) ; void Find. Warmest ( const int [ ], int & ) ; void Find. Average ( const int [ ], int & ) ; void Print ( const int [ ], int ) ; use const with incoming array values to prevent unintentional changes by function 24
![void Find Average in const int temp void Find. Average ( /* in */ const int temp [ ] , /*](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-25.jpg)
void Find. Average ( /* in */ const int temp [ ] , /* in */ int number , /* out */ int & avg ) // Determines average of temp[0. . number-1] // Precondition: // number is assigned && number > 0 // temp [0. . number -1 ] are assigned // Postcondition: // avg == arithmetic average of temp[0. . number-1] { int m; int total = 0; for ( m = 0 ; m < number; m++ ) { total = total + temp [m] ; } avg = int (float (total) / float (number) +. 5) ; } 25
![void Find Warmest in const int temp void Find. Warmest ( /* in */ const int temp [ ] , /*](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-26.jpg)
void Find. Warmest ( /* in */ const int temp [ ] , /* in */ int number , /* out */ int & largest ) // Determines largest of temp[0. . number-1] // Precondition: // number is assigned && number > 0 // temp [0. . number -1 ] are assigned // Postcondition: // largest== largest value in temp[0. . number-1] { int m; largest = temp[0] ; // initialize largest to first element // then compare with other elements for ( m = 0 ; m < number; m++ ) { if ( temp [m] > largest ) largest = temp[m] ; } } 26
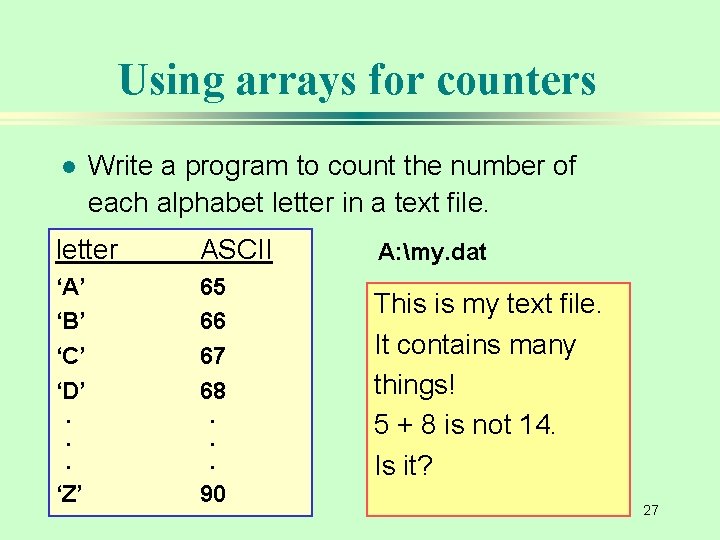
Using arrays for counters l Write a program to count the number of each alphabet letter in a text file. letter ASCII ‘A’ ‘B’ ‘C’ ‘D’ 65 66 67 68 . . . ‘Z’ 90 A: my. dat This is my text file. It contains many things! 5 + 8 is not 14. Is it? 27
![const int SIZE 91 int freq Count SIZE freq Count const int SIZE 91 ; int freq. Count [ SIZE ] ; freq. Count](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-28.jpg)
const int SIZE 91 ; int freq. Count [ SIZE ] ; freq. Count [ 0 ] 0 freq. Count [ 1] 0 freq. Count [ 65 ] 2 counts ‘A’ and ‘a’ freq. Count [ 66 ] 0 counts ‘B’ and ‘b’ . . . unused . . . freq. Count [ 89] 1 counts ‘ Y’ and ‘y’ freq. Count [ 90 ] 0 counts ‘Z’ and ‘z’ 28
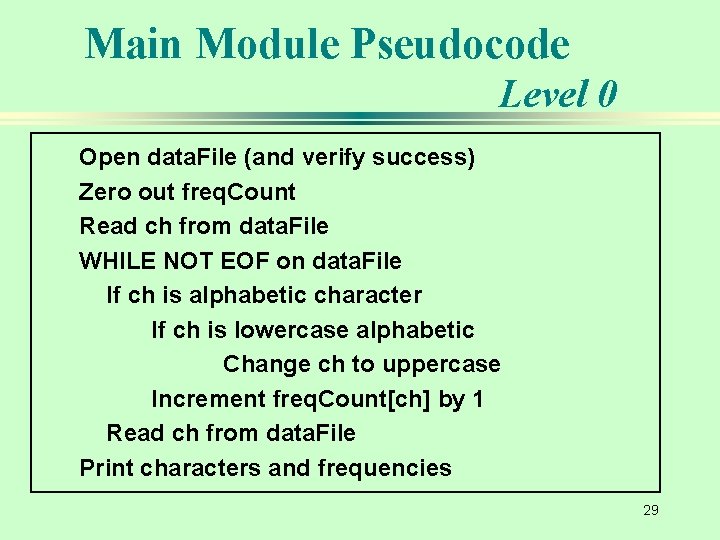
Main Module Pseudocode Level 0 Open data. File (and verify success) Zero out freq. Count Read ch from data. File WHILE NOT EOF on data. File If ch is alphabetic character If ch is lowercase alphabetic Change ch to uppercase Increment freq. Count[ch] by 1 Read ch from data. File Print characters and frequencies 29
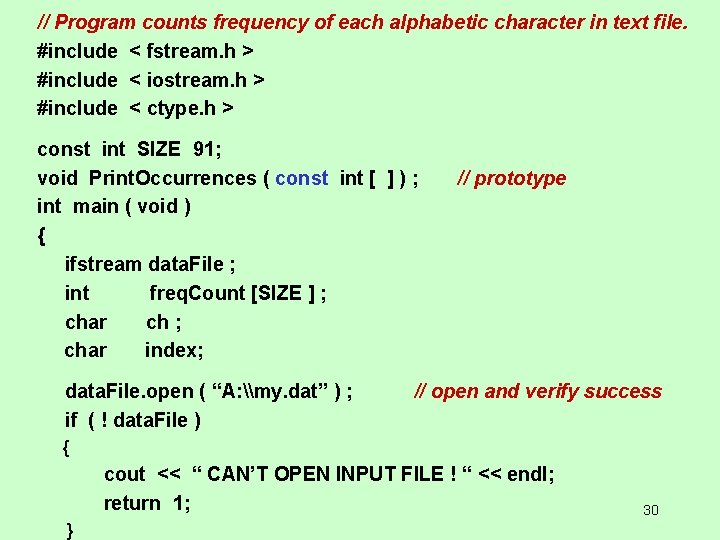
// Program counts frequency of each alphabetic character in text file. #include < fstream. h > #include < iostream. h > #include < ctype. h > const int SIZE 91; void Print. Occurrences ( const int [ ] ) ; int main ( void ) { ifstream data. File ; int freq. Count [SIZE ] ; char ch ; char index; data. File. open ( “A: \my. dat” ) ; if ( ! data. File ) // prototype // open and verify success { cout << “ CAN’T OPEN INPUT FILE ! “ << endl; return 1; } 30
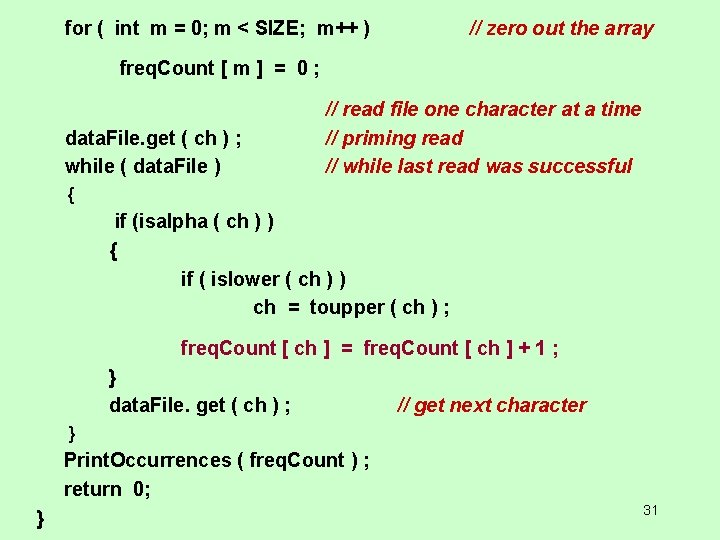
for ( int m = 0; m < SIZE; m++ ) // zero out the array freq. Count [ m ] = 0 ; data. File. get ( ch ) ; while ( data. File ) // read file one character at a time // priming read // while last read was successful { if (isalpha ( ch ) ) { if ( islower ( ch ) ) ch = toupper ( ch ) ; freq. Count [ ch ] = freq. Count [ ch ] + 1 ; } data. File. get ( ch ) ; // get next character } Print. Occurrences ( freq. Count ) ; return 0; } 31
![void Print Occurrences in const int freq Count void Print. Occurrences ( /* in */ const int freq. Count [ ] )](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-32.jpg)
void Print. Occurrences ( /* in */ const int freq. Count [ ] ) // Prints each alphabet character and its frequency // Precondition: // freq. Count [ ‘A’. . ‘Z’ ] are assigned // Postcondition: // freq. Count [ ‘A’. . ‘Z’ ] have been printed { char index ; cout << “File contained “ << endl ; cout << “LETTER OCCURRENCES” << endl ; for ( index = ‘A’ ; index < = ‘Z’ ; index ++ ) { cout << setw ( 4 ) << index << setw ( 10 ) << freq. Count [ index ] << endl ; } } 32
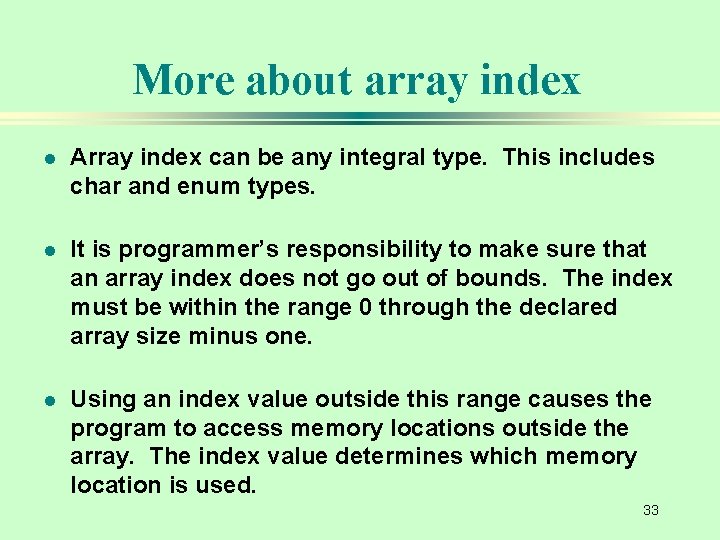
More about array index l Array index can be any integral type. This includes char and enum types. l It is programmer’s responsibility to make sure that an array index does not go out of bounds. The index must be within the range 0 through the declared array size minus one. l Using an index value outside this range causes the program to access memory locations outside the array. The index value determines which memory location is used. 33
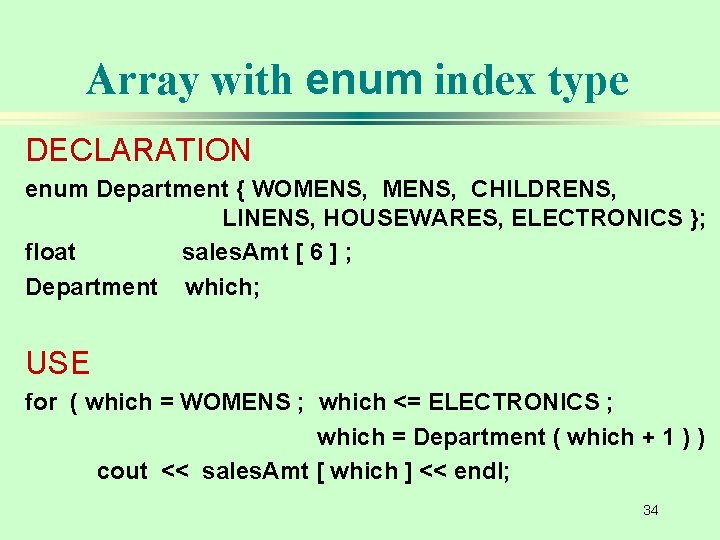
Array with enum index type DECLARATION enum Department { WOMENS, CHILDRENS, LINENS, HOUSEWARES, ELECTRONICS }; float sales. Amt [ 6 ] ; Department which; USE for ( which = WOMENS ; which <= ELECTRONICS ; which = Department ( which + 1 ) ) cout << sales. Amt [ which ] << endl; 34
![float sales Amt 6 sales Amt WOMENS i e sales Amt float sales. Amt [6]; sales. Amt [ WOMENS ] ( i. e. sales. Amt](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-35.jpg)
float sales. Amt [6]; sales. Amt [ WOMENS ] ( i. e. sales. Amt [ 0 ] ) sales. Amt [ MENS] ( i. e. sales. Amt [ 1 ] ) sales. Amt [ CHILDRENS ] ( i. e. sales. Amt [ 2 ] ) sales. Amt [ LINENS ] ( i. e. sales. Amt [ 3 ] ) sales. Amt [ HOUSEWARES ] ( i. e. sales. Amt [ 4 ] ) sales. Amt [ ELECTRONICS ] ( i. e. sales. Amt [ 5 ] ) 35
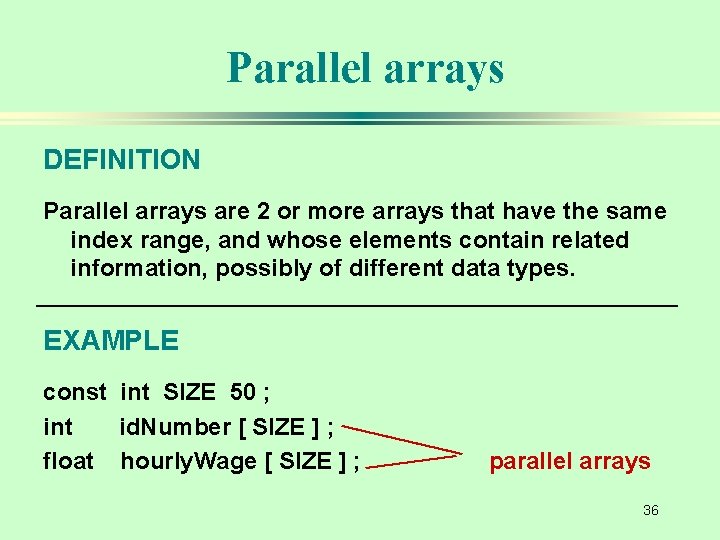
Parallel arrays DEFINITION Parallel arrays are 2 or more arrays that have the same index range, and whose elements contain related information, possibly of different data types. EXAMPLE const int SIZE 50 ; int id. Number [ SIZE ] ; float hourly. Wage [ SIZE ] ; parallel arrays 36
![const int SIZE 50 int id Number SIZE float hourly const int SIZE 50 ; int id. Number [ SIZE ] ; float hourly.](https://slidetodoc.com/presentation_image/af8e7050f9deb298def01c922544b8c0/image-37.jpg)
const int SIZE 50 ; int id. Number [ SIZE ] ; float hourly. Wage [ SIZE ] ; // parallel arrays hold // related information id. Number [ 0 ] 4562 hourly. Wage [ 0 ] 9. 68 id. Number [ 1 ] 1235 hourly. Wage [ 1 ] 45. 75 id. Number [ 2 ] 6278 hourly. Wage [ 2 ] 12. 71 . . . id. Number [ 48 ] 8754 hourly. Wage [ 48 ] 67. 96 id. Number [ 49 ] 2460 hourly. Wage [ 49 ] 8. 97 37
Parallel arrays java
Array of arrays c++
Java array operations
Partially filled arrays
C++ parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis
Arreglos unidimensionales ejemplos
Arreglos bidimensionales java
Arrays in mips
Polynomial representation using arrays
Arrays in arm assembly
Global arrays in c
Computer science arrays
Searching and sorting arrays in c++
Arrays visual basic
Python parallel arrays
Advantages and disadvantages of arrays
How many arrays in 24
Arrays in pascal
Declare array in mips
Creating arrays matlab
Array adt
Partially filled array java
Redundancy array of independent disk
Python list of arrays
Arrays
Day 3: arrays
Raid redundant array of independent disks
Small basic array examples
Advantages of static memory allocation
Microled arrays
Are vectors dynamic arrays
Facts about arrays
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
Runtime programming
Integer programming vs linear programming