Programming in C DaleWeemsHeadington Chapter 12 Applied Arrays
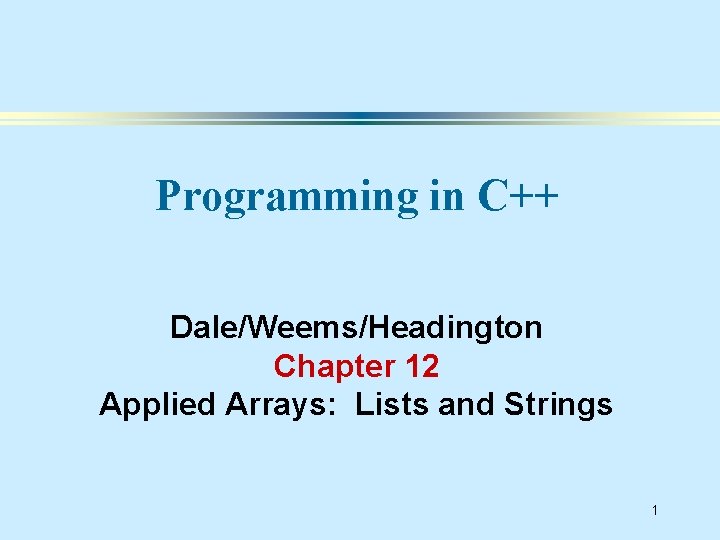
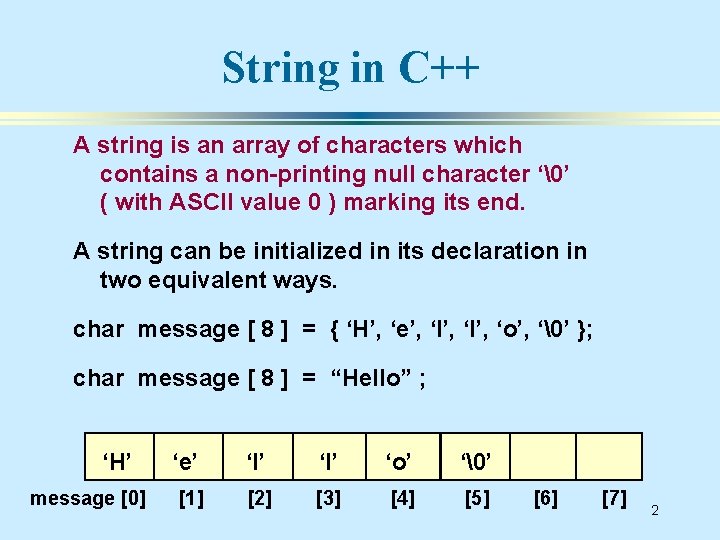
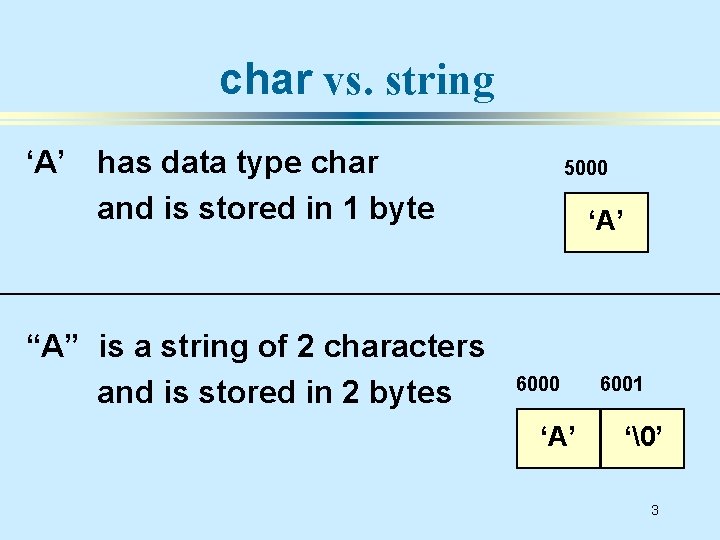
![Recall that. . . char message[8]; // this declaration allocates memory To the compiler, Recall that. . . char message[8]; // this declaration allocates memory To the compiler,](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-4.jpg)
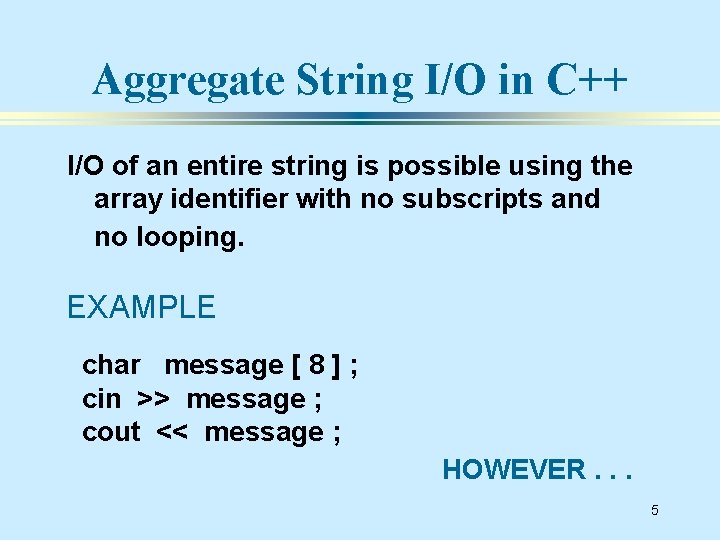
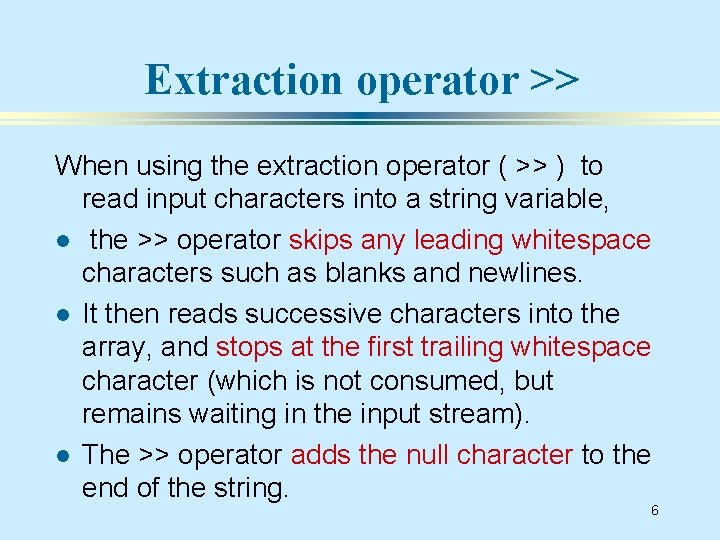
![Example using >> char name [ 5 ] ; cin >> name ; total Example using >> char name [ 5 ] ; cin >> name ; total](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-7.jpg)
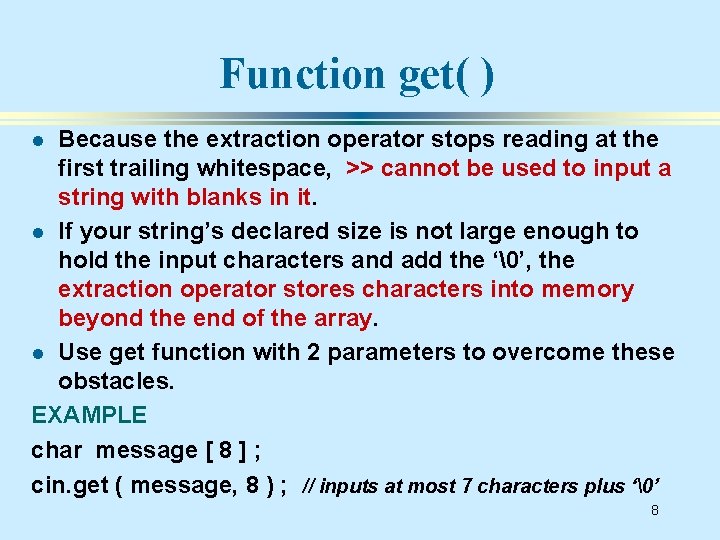
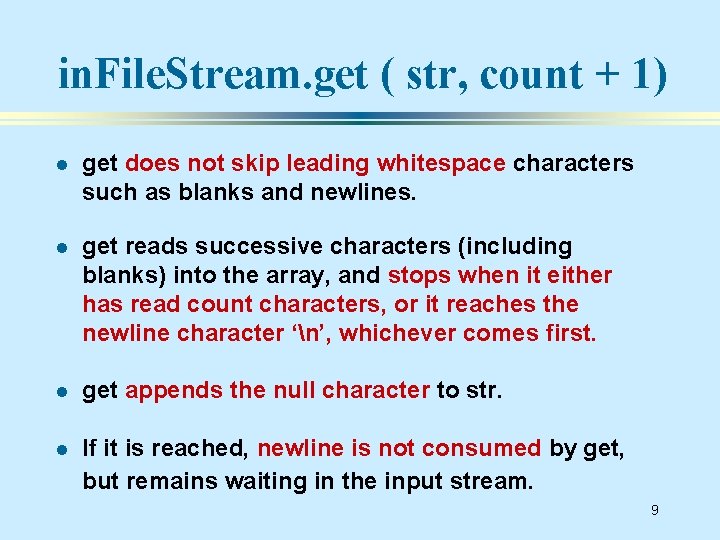
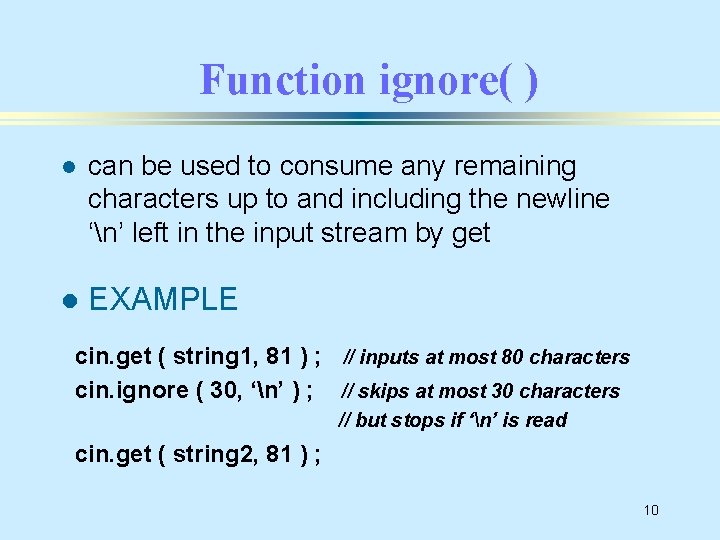
![Another example using get( ) char ch ; char full. Name [ 31 ] Another example using get( ) char ch ; char full. Name [ 31 ]](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-11.jpg)
![String function prototypes in < string. h > int strlen (char str [ ] String function prototypes in < string. h > int strlen (char str [ ]](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-12.jpg)
![# include <string. h>. . . char author [ 21 ] ; int length # include <string. h>. . . char author [ 21 ] ; int length](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-13.jpg)
![char my. Name [ 21 ] = “Huang” ; char your. Name [ 21 char my. Name [ 21 ] = “Huang” ; char your. Name [ 21](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-14.jpg)
![char my. Name [ 21 ] = “Huang” ; char your. Name [ 21 char my. Name [ 21 ] = “Huang” ; char your. Name [ 21](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-15.jpg)
![char my. Name [ 21 ] = “Huang” ; char your. Name [ 21 char my. Name [ 21 ] = “Huang” ; char your. Name [ 21](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-16.jpg)
![char my. Name [ 21 ] = “Huang” ; char your. Name [ 21 char my. Name [ 21 ] = “Huang” ; char your. Name [ 21](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-17.jpg)
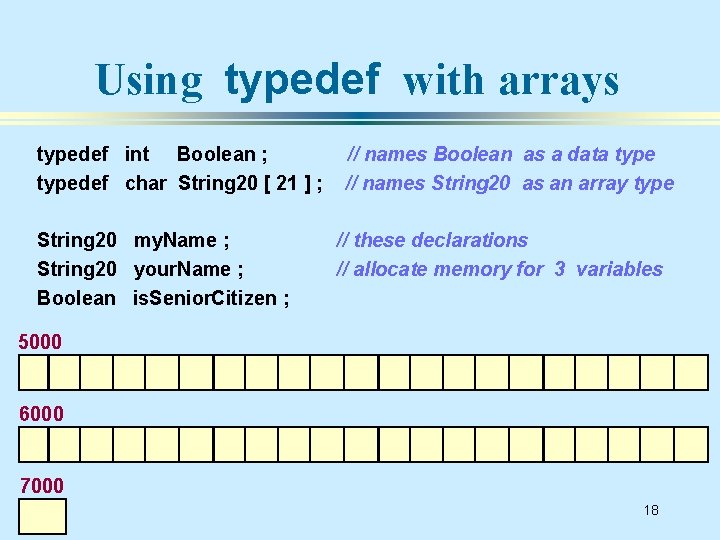
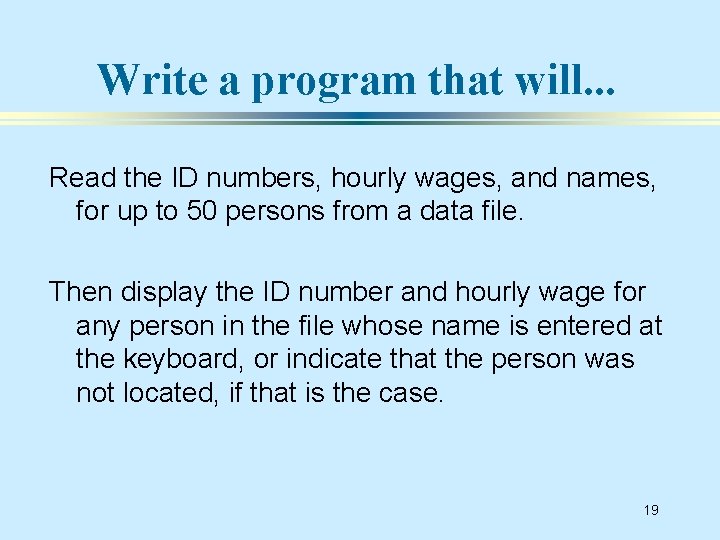
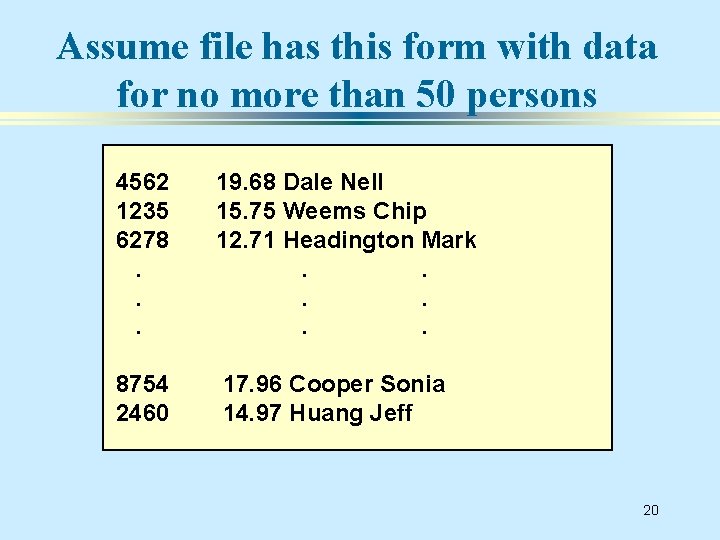
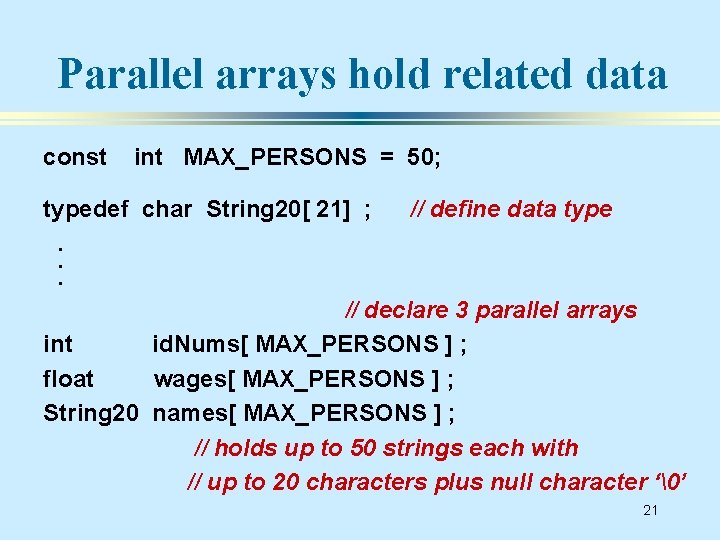
![int id. Nums [ MAX_PERSONS ] ; float wages [ MAX_PERSONS ] ; String int id. Nums [ MAX_PERSONS ] ; float wages [ MAX_PERSONS ] ; String](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-22.jpg)
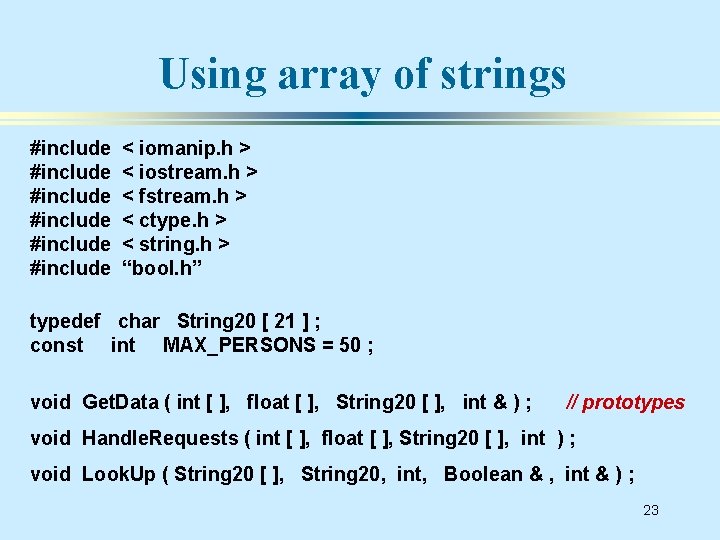
![Main Program int main (void) { int float String 20 int id. Nums [MAX_PERSONS] Main Program int main (void) { int float String 20 int id. Nums [MAX_PERSONS]](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-24.jpg)
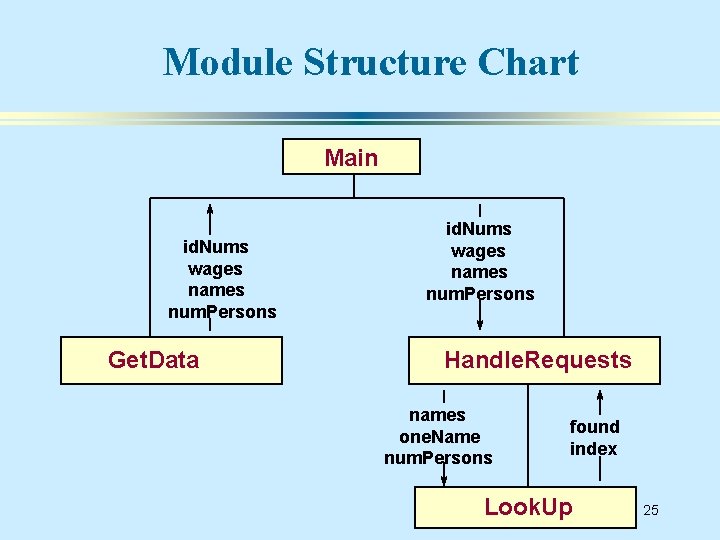
![void Get. Data ( /* out */ int ids[ ] , /* out*/ float void Get. Data ( /* out */ int ids[ ] , /* out*/ float](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-26.jpg)
![void Handle. Requests( const /* in */ int id. Nums[ ], const /* in void Handle. Requests( const /* in */ int id. Nums[ ], const /* in](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-27.jpg)
![void Look. Up ( const /* in */ String 20 names [ ], const void Look. Up ( const /* in */ String 20 names [ ], const](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-28.jpg)
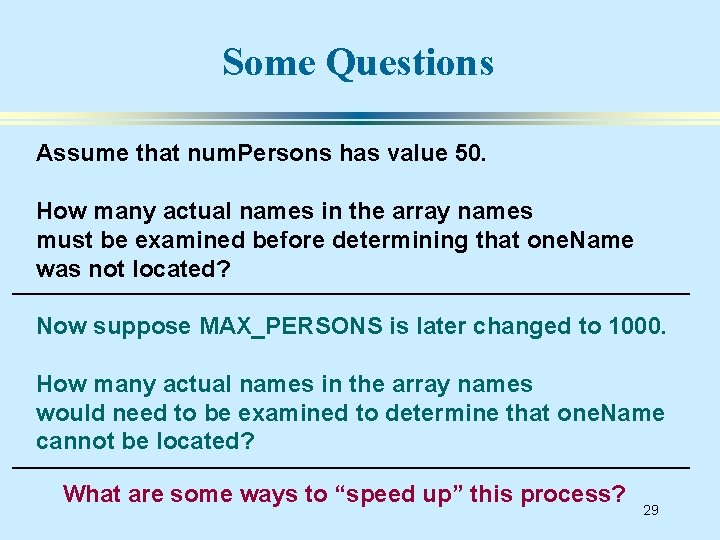
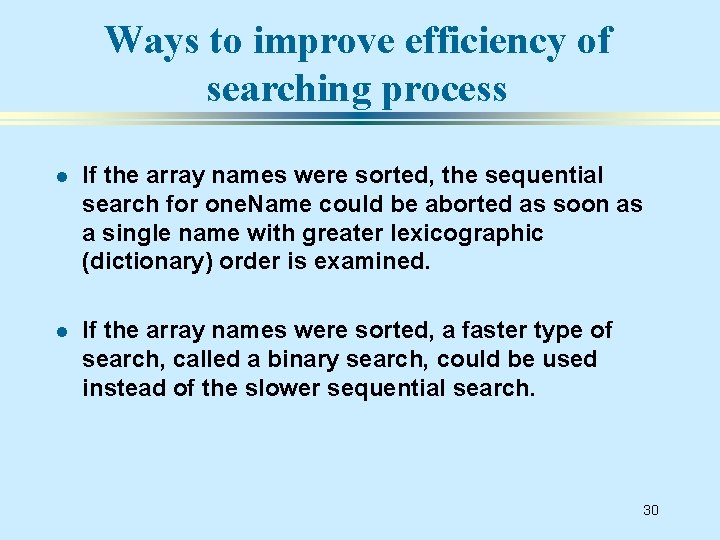
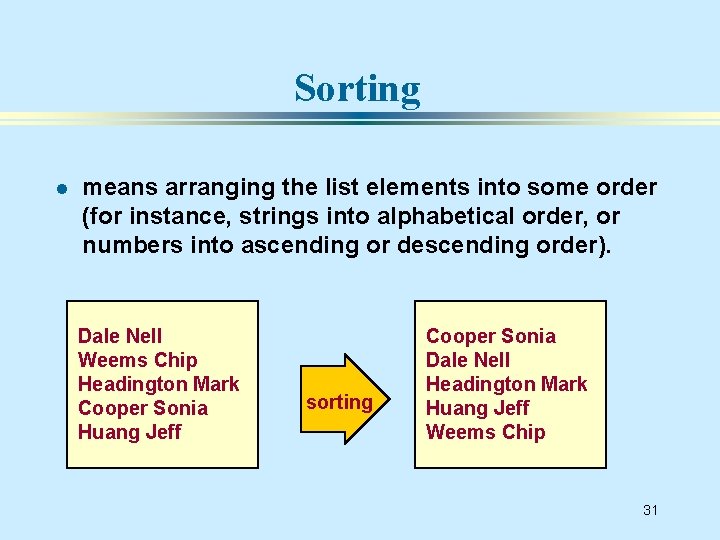
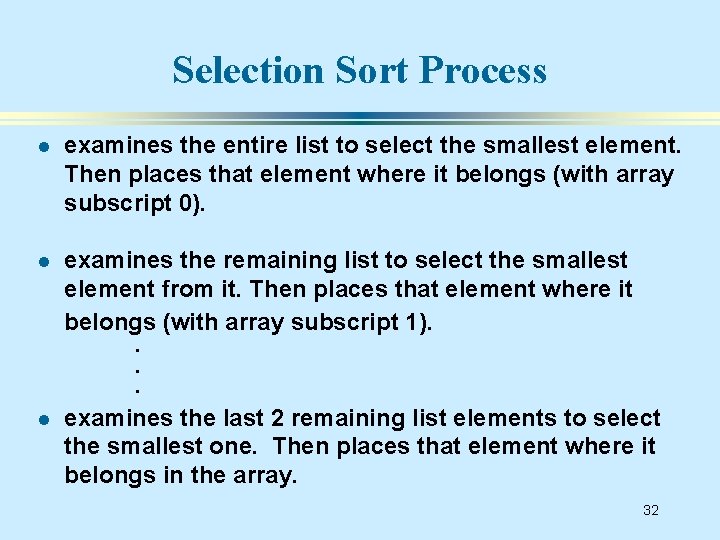
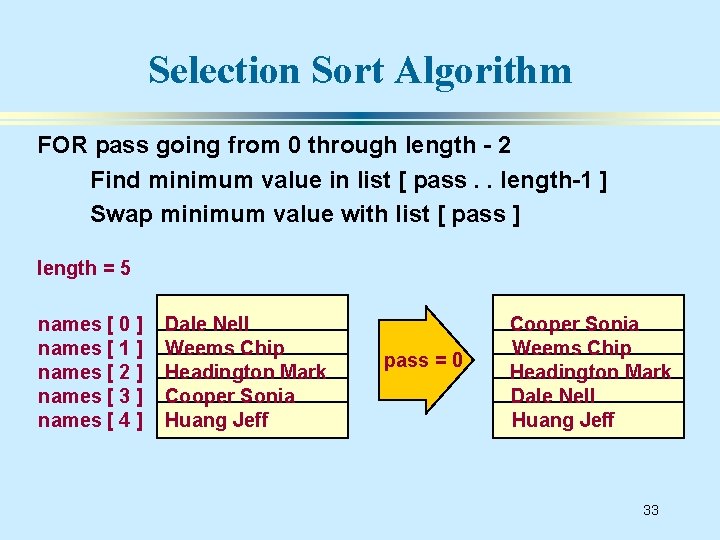
![void Sel. Sort ( /* inout */ String 20 names [ ] , /* void Sel. Sort ( /* inout */ String 20 names [ ] , /*](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-34.jpg)
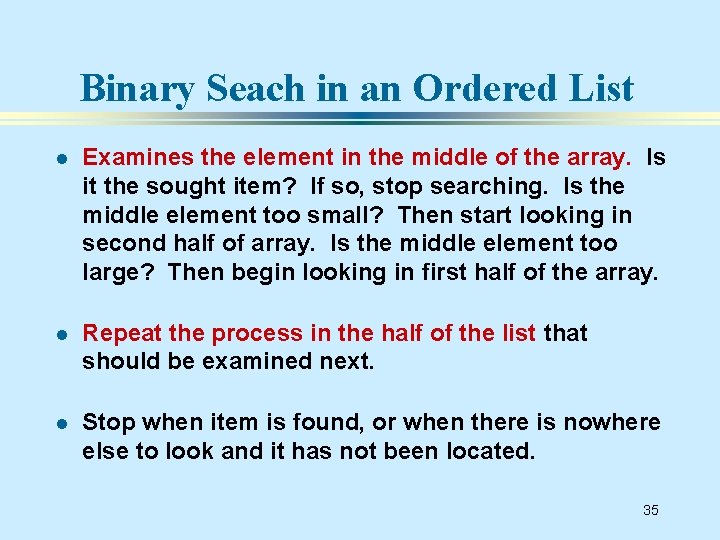
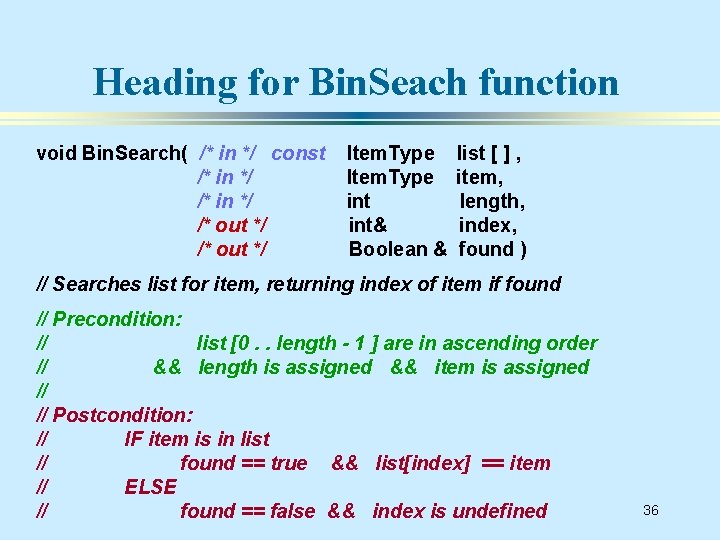
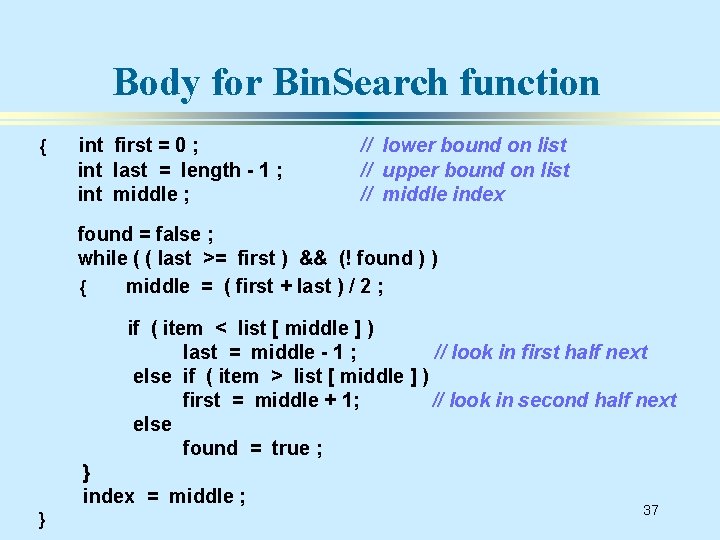
![Trace of Bin. Search function item = 45 15 list[0] 26 38 [1] [2] Trace of Bin. Search function item = 45 15 list[0] 26 38 [1] [2]](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-38.jpg)
![Trace continued item = 45 15 list[0] 26 [1] 38 [2] first, middle 57 Trace continued item = 45 15 list[0] 26 [1] 38 [2] first, middle 57](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-39.jpg)
![Trace concludes item = 45 15 list[0] 26 [1] 38 57 [2] [3] last Trace concludes item = 45 15 list[0] 26 [1] 38 57 [2] [3] last](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-40.jpg)
- Slides: 40
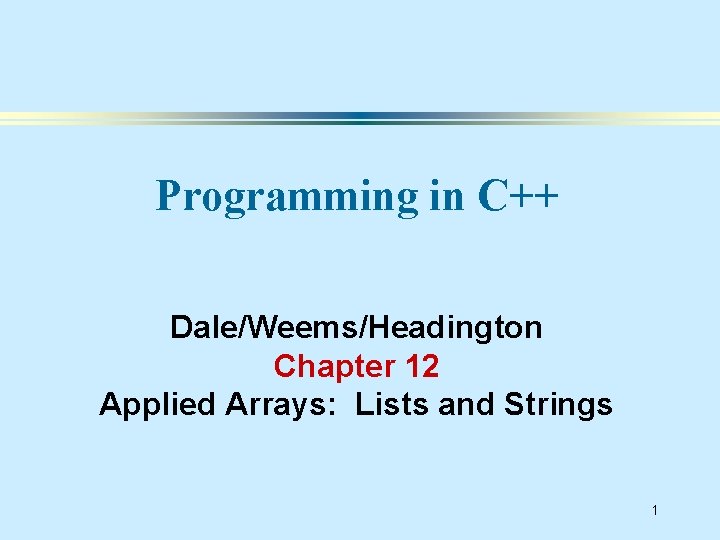
Programming in C++ Dale/Weems/Headington Chapter 12 Applied Arrays: Lists and Strings 1
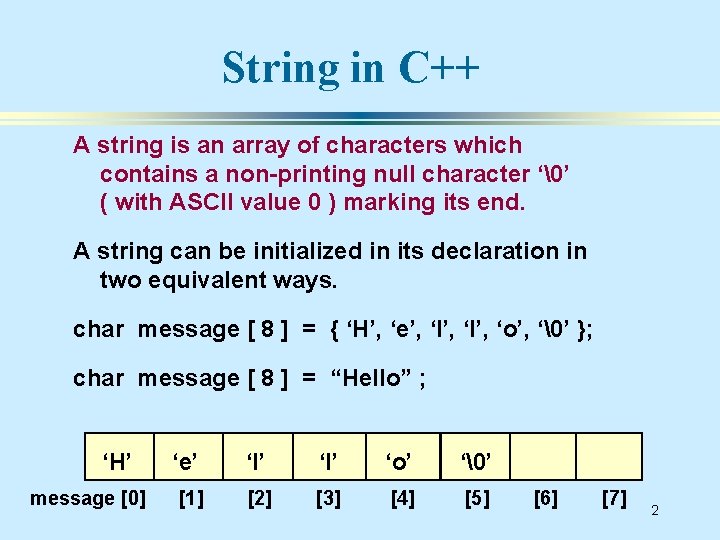
String in C++ A string is an array of characters which contains a non-printing null character ‘ ’ ( with ASCII value 0 ) marking its end. A string can be initialized in its declaration in two equivalent ways. char message [ 8 ] = { ‘H’, ‘e’, ‘l’, ‘o’, ‘ ’ }; char message [ 8 ] = “Hello” ; ‘H’ message [0] ‘e’ ‘l’ ‘o’ ‘ ’ [1] [2] [3] [4] [5] [6] [7] 2
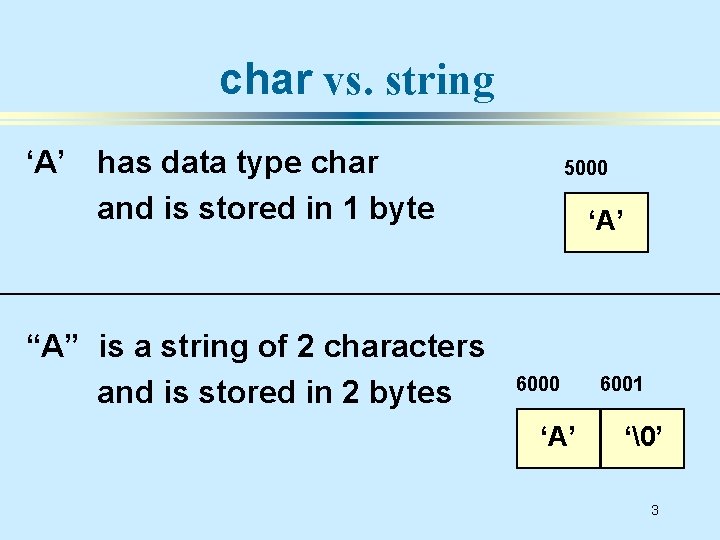
char vs. string ‘A’ has data type char and is stored in 1 byte “A” is a string of 2 characters and is stored in 2 bytes 5000 ‘A’ 6001 ‘ ’ 3
![Recall that char message8 this declaration allocates memory To the compiler Recall that. . . char message[8]; // this declaration allocates memory To the compiler,](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-4.jpg)
Recall that. . . char message[8]; // this declaration allocates memory To the compiler, the value of the identifier message alone is the base address of the array. We say message is a pointer (because its value is an address). It “points” to a memory location. 6000 ‘H’ message [0] ‘e’ ‘l’ ‘o’ ‘ ’ [1] [2] [3] [4] [5] [6] [7] 4
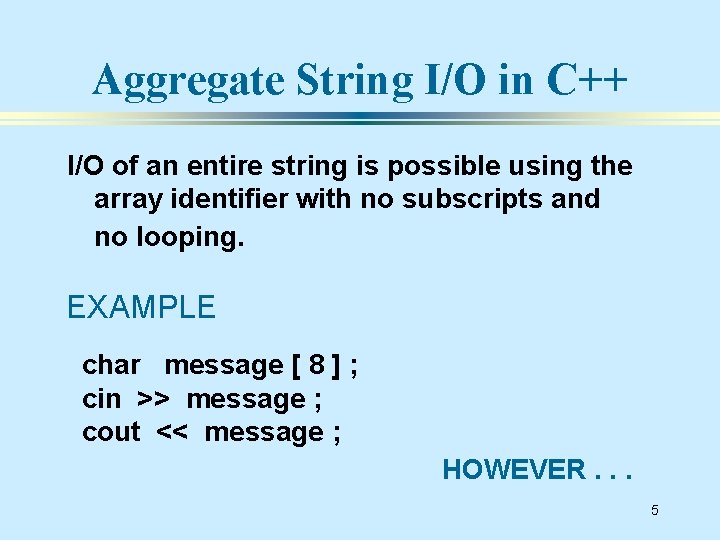
Aggregate String I/O in C++ I/O of an entire string is possible using the array identifier with no subscripts and no looping. EXAMPLE char message [ 8 ] ; cin >> message ; cout << message ; HOWEVER. . . 5
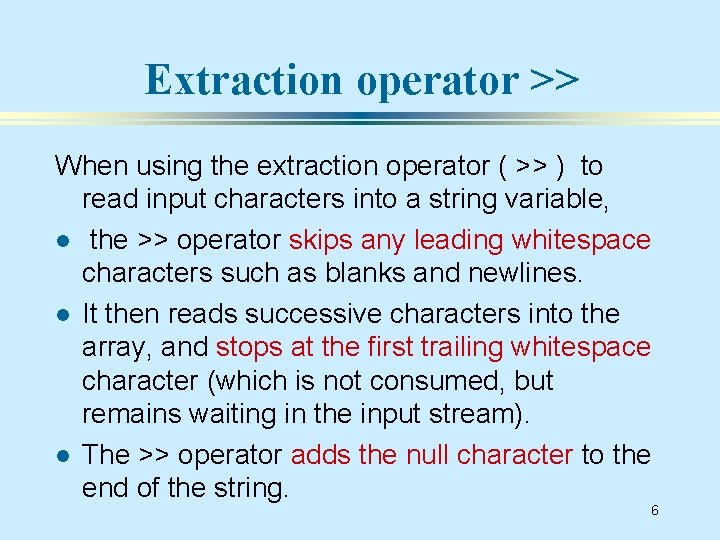
Extraction operator >> When using the extraction operator ( >> ) to read input characters into a string variable, l the >> operator skips any leading whitespace characters such as blanks and newlines. l It then reads successive characters into the array, and stops at the first trailing whitespace character (which is not consumed, but remains waiting in the input stream). l The >> operator adds the null character to the end of the string. 6
![Example using char name 5 cin name total Example using >> char name [ 5 ] ; cin >> name ; total](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-7.jpg)
Example using >> char name [ 5 ] ; cin >> name ; total number of elements in the array Suppose input stream looks like this: Joe 7000 ‘J’ name [0] ‘o’ name [1] ‘e’ ‘ ’ name [2] name [3] null character is added name [4] 7
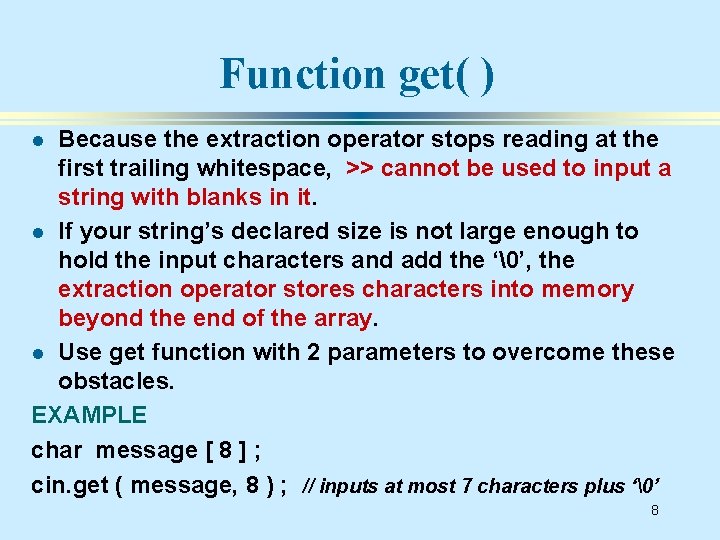
Function get( ) Because the extraction operator stops reading at the first trailing whitespace, >> cannot be used to input a string with blanks in it. l If your string’s declared size is not large enough to hold the input characters and add the ‘ ’, the extraction operator stores characters into memory beyond the end of the array. l Use get function with 2 parameters to overcome these obstacles. EXAMPLE char message [ 8 ] ; cin. get ( message, 8 ) ; // inputs at most 7 characters plus ‘ ’ l 8
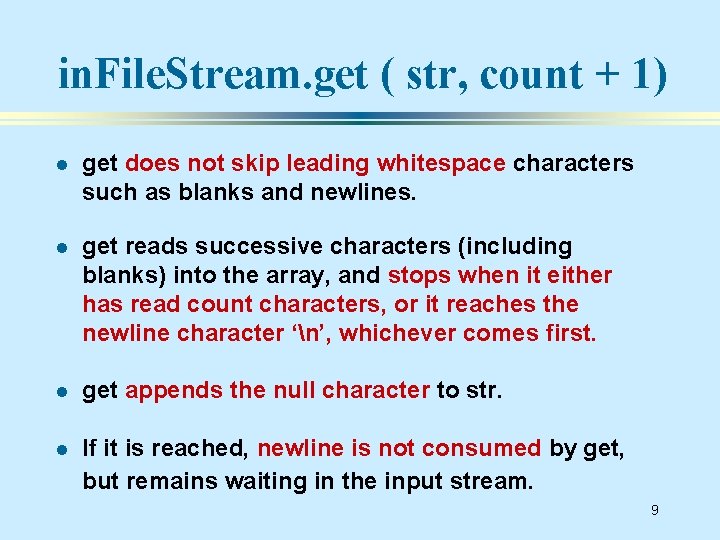
in. File. Stream. get ( str, count + 1) l get does not skip leading whitespace characters such as blanks and newlines. l get reads successive characters (including blanks) into the array, and stops when it either has read count characters, or it reaches the newline character ‘n’, whichever comes first. l get appends the null character to str. l If it is reached, newline is not consumed by get, but remains waiting in the input stream. 9
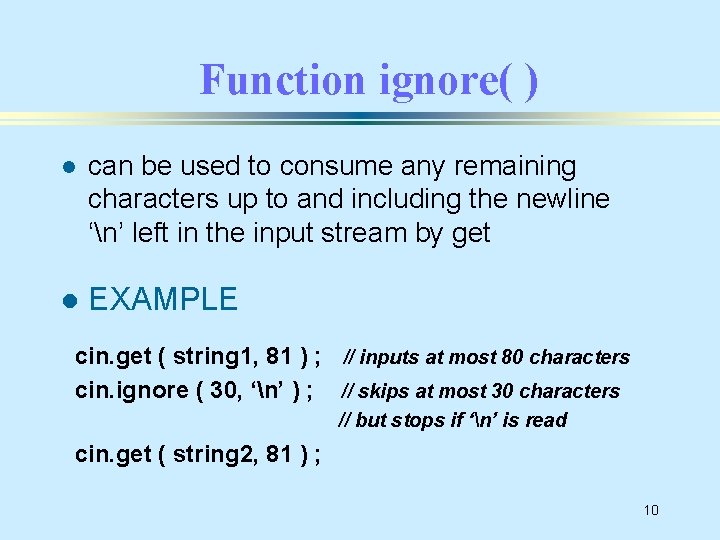
Function ignore( ) l can be used to consume any remaining characters up to and including the newline ‘n’ left in the input stream by get l EXAMPLE cin. get ( string 1, 81 ) ; // inputs at most 80 characters cin. ignore ( 30, ‘n’ ) ; // skips at most 30 characters // but stops if ‘n’ is read cin. get ( string 2, 81 ) ; 10
![Another example using get char ch char full Name 31 Another example using get( ) char ch ; char full. Name [ 31 ]](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-11.jpg)
Another example using get( ) char ch ; char full. Name [ 31 ] ; char address [ 31 ] ; cout << “Enter your full name: “ ; cin. get ( full. Name, 31 ) ; cin. get (ch) ; // to consume the newline cout << “Enter your address: “ ; cin. get ( address, 31 ) ; ‘N’ ‘e’ ‘l’ ‘’ ‘D’ ‘a’ ‘l’ ‘e’ ‘ ’ . . . ‘i‘ ‘n’ ‘’ ‘T’ ‘X’ ‘ ’ . . . full. Name [0] ‘A’ ‘u’ address [0] ‘s’ ‘t’ 11
![String function prototypes in string h int strlen char str String function prototypes in < string. h > int strlen (char str [ ]](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-12.jpg)
String function prototypes in < string. h > int strlen (char str [ ] ); // FCTNVAL == integer length of string str ( not including ‘ ’ ) int strcmp ( char str 1 [ ], char str 2 [ ] ); // FCTNVAL // // == negative, if str 1 precedes str 2 lexicographically == positive, if str 1 follows str 2 lexicographically == 0, if str 1 and str 2 characters same through ‘ ’ char * strcpy ( char to. Str [ ], char from. Str [ ] ); // FCTNVAL == base address of to. Str ( usually ignored ) // POSTCONDITION : characters in string from. Str are copied to // string to. Str, up to and including ‘ ’, 12 // overwriting contents of string to. Str
![include string h char author 21 int length # include <string. h>. . . char author [ 21 ] ; int length](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-13.jpg)
# include <string. h>. . . char author [ 21 ] ; int length ; cin. get ( author , 21 ) ; length = strlen ( author ) ; // What is the value of length ? 5000 ‘C’ ‘h’ ‘i’ ‘p’ ‘ ’ ‘W’ ‘e’ ‘m’ ‘s’ ‘ ’. . author [0] 13
![char my Name 21 Huang char your Name 21 char my. Name [ 21 ] = “Huang” ; char your. Name [ 21](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-14.jpg)
char my. Name [ 21 ] = “Huang” ; char your. Name [ 21 ] ; // WHAT IS OUTPUT? cout << “Enter your last name : “ ; cin. get ( your. Name, 21 ) ; if ( strcmp ( my. Name, your. Name ) == 0 ) cout << “We have the same name! “ ; else if ( strcmp ( my. Name, your. Name ) < 0 ) cout << my. Name << “ comes before “ << your. Name ; else if ( strcmp ( my. Name, your. Name ) > 0 ) cout << your. Name << “comes before “ << my. Name ; ‘H’ ‘u’ ‘a’ ‘n’ ‘g’ ‘ ’ . . . my. Name [0] ‘H’ ‘e’ ‘a’ your. Name [0] ‘d’ ‘i‘ ‘n’ ‘ g’ ‘t’ ‘o’ ‘n’ ‘ ’. . . 14
![char my Name 21 Huang char your Name 21 char my. Name [ 21 ] = “Huang” ; char your. Name [ 21](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-15.jpg)
char my. Name [ 21 ] = “Huang” ; char your. Name [ 21 ] ; if ( my. Name == your. Name ) {. // compares addresses only! // That is, 4000 and 6000 here. // DOES NOT COMPARE CONTENTS! . . } 4000 ‘H’ ‘u’ ‘a’ ‘n’ ‘g’ ‘ ’ . . . my. Name [0] 6000 ‘H’ ‘e’ ‘a’ your. Name [0] ‘d’ ‘i‘ ‘n’ ‘ g’ ‘t’ ‘o’ ‘n’ ‘ ’. . . 15
![char my Name 21 Huang char your Name 21 char my. Name [ 21 ] = “Huang” ; char your. Name [ 21](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-16.jpg)
char my. Name [ 21 ] = “Huang” ; char your. Name [ 21 ] ; cin. get ( your. Name, 21 ) ; your. Name = my. Name; // DOES NOT COMPILE! // What is the value of my. Name ? 4000 ‘H’ ‘u’ ‘a’ ‘n’ ‘g’ ‘ ’ . . . my. Name [0] 6000 ‘H’ ‘e’ ‘a’ your. Name [0] ‘d’ ‘i‘ ‘n’ ‘ g’ ‘t’ ‘o’ ‘n’ ‘ ’. . . 16
![char my Name 21 Huang char your Name 21 char my. Name [ 21 ] = “Huang” ; char your. Name [ 21](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-17.jpg)
char my. Name [ 21 ] = “Huang” ; char your. Name [ 21 ] ; cin. get ( your. Name, 21 ) ; strcpy ( your. Name, my. Name ) ; // changes string your. Name // OVERWRITES CONTENTS! 4000 ‘H’ ‘u’ ‘a’ ‘n’ ‘g’ ‘ ’ . . . my. Name [0] 6000 ‘u’ ‘H’ ‘e’ ‘a’ your. Name [0] ‘n’ ‘g’ ‘ ’ ‘d’ ‘n’ ‘ g’ ‘t’ ‘i‘ ‘o’ ‘n’ ‘ ’. . . 17
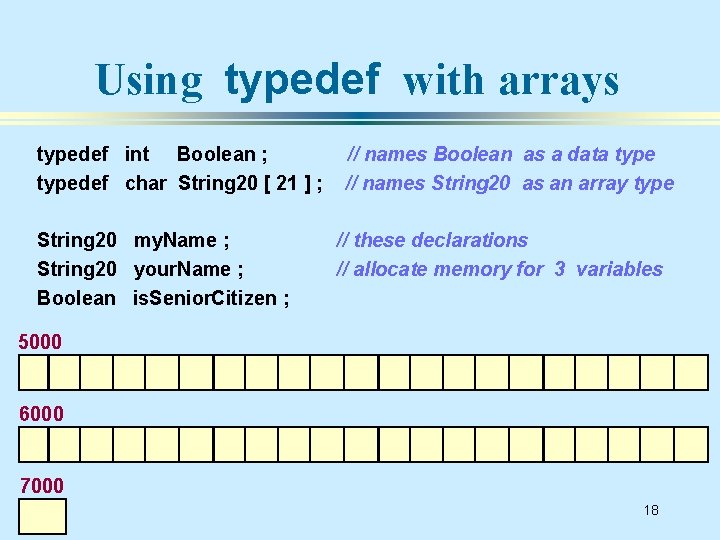
Using typedef with arrays typedef int Boolean ; typedef char String 20 [ 21 ] ; String 20 my. Name ; String 20 your. Name ; Boolean is. Senior. Citizen ; // names Boolean as a data type // names String 20 as an array type // these declarations // allocate memory for 3 variables 5000 6000 7000 18
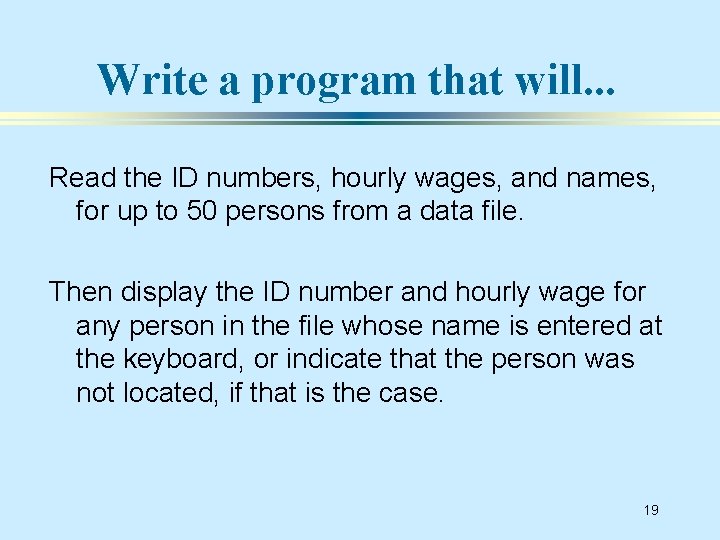
Write a program that will. . . Read the ID numbers, hourly wages, and names, for up to 50 persons from a data file. Then display the ID number and hourly wage for any person in the file whose name is entered at the keyboard, or indicate that the person was not located, if that is the case. 19
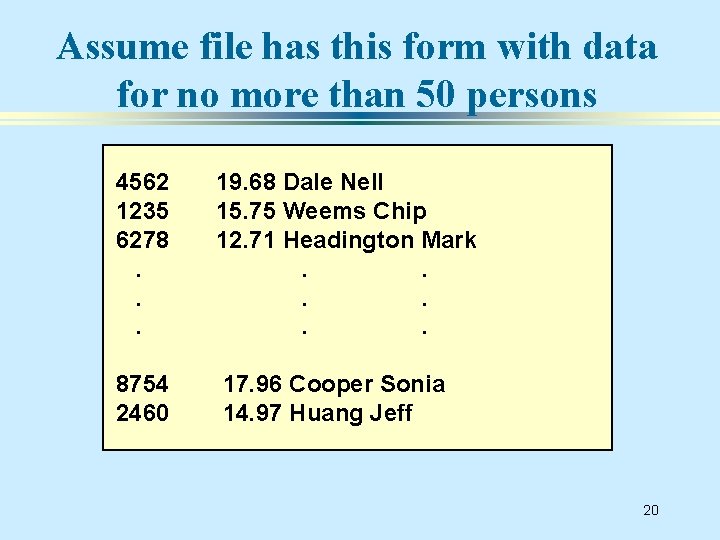
Assume file has this form with data for no more than 50 persons 4562 1235 6278. . . 19. 68 Dale Nell 15. 75 Weems Chip 12. 71 Headington Mark. . . 8754 2460 17. 96 Cooper Sonia 14. 97 Huang Jeff 20
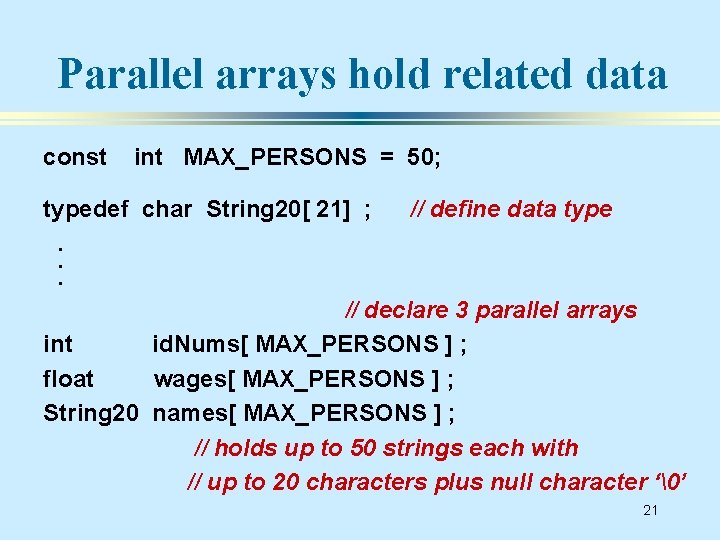
Parallel arrays hold related data const int MAX_PERSONS = 50; typedef char String 20[ 21] ; // define data type . . . // declare 3 parallel arrays int id. Nums[ MAX_PERSONS ] ; float wages[ MAX_PERSONS ] ; String 20 names[ MAX_PERSONS ] ; // holds up to 50 strings each with // up to 20 characters plus null character ‘ ’ 21
![int id Nums MAXPERSONS float wages MAXPERSONS String int id. Nums [ MAX_PERSONS ] ; float wages [ MAX_PERSONS ] ; String](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-22.jpg)
int id. Nums [ MAX_PERSONS ] ; float wages [ MAX_PERSONS ] ; String 20 names [ MAX_PERSONS ] ; // parallel arrays id. Nums[ 0 ] 4562 wages[ 0 ] 19. 68 names[ 0 ] “Dale Nell” id. Nums[ 1 ] 1235 wages[ 1 ] 15. 75 names[ 1 ] “Weems Chip” id. Nums[ 2 ] 6278 wages[ 2 ] 12. 71 names[ 2 ] “Headington Mark” . . . . id. Nums[ 48] 8754 wages[ 48] 17. 96 names[ 48] “Cooper Sonia” id. Nums[ 49] 2460 wages[ 49] 14. 97 names[ 49] “Huang Jeff” 22
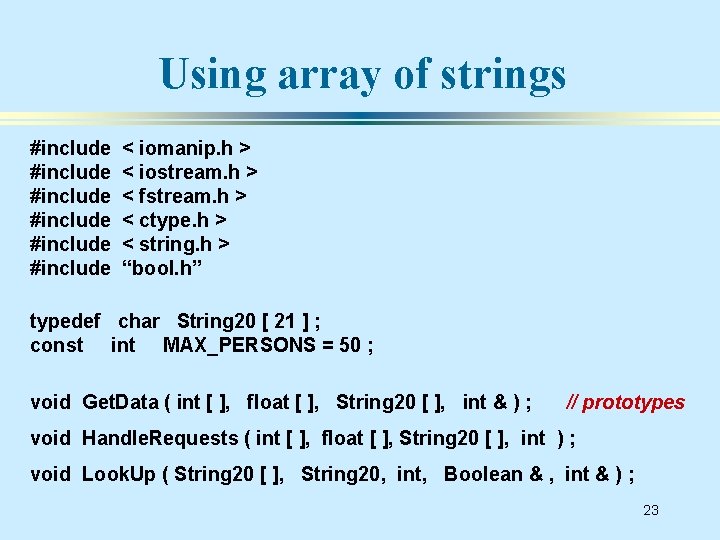
Using array of strings #include #include < iomanip. h > < iostream. h > < fstream. h > < ctype. h > < string. h > “bool. h” typedef char String 20 [ 21 ] ; const int MAX_PERSONS = 50 ; void Get. Data ( int [ ], float [ ], String 20 [ ], int & ) ; // prototypes void Handle. Requests ( int [ ], float [ ], String 20 [ ], int ) ; void Look. Up ( String 20 [ ], String 20, int, Boolean & , int & ) ; 23
![Main Program int main void int float String 20 int id Nums MAXPERSONS Main Program int main (void) { int float String 20 int id. Nums [MAX_PERSONS]](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-24.jpg)
Main Program int main (void) { int float String 20 int id. Nums [MAX_PERSONS] ; // holds up to 50 IDs wages [MAX_PERSONS] ; // holds up to 50 wages names [MAX_PERSONS] ; // holds up to 50 names num. Persons; // number of persons’ information in file Get. Data ( id. Nums, wages, names, num. Persons ) ; Handle. Requests ( id. Nums, wages, names, num. Persons ) ; cout << “End of Program. n”; return 0 ; } 24
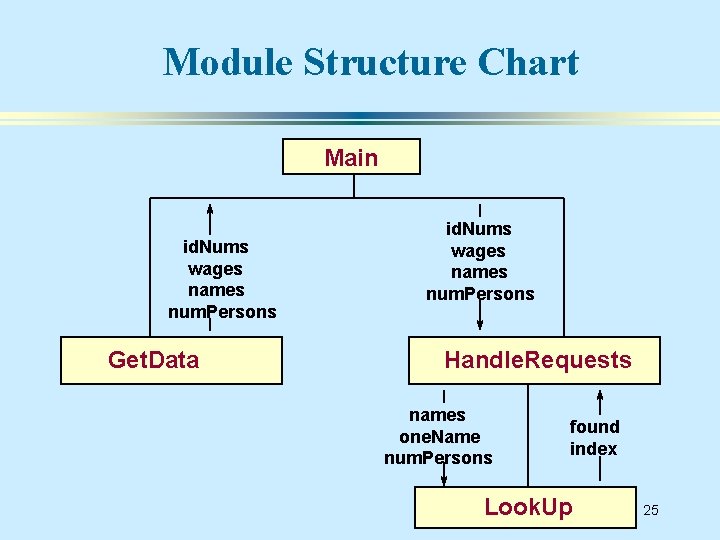
Module Structure Chart Main id. Nums wages names num. Persons Get. Data id. Nums wages names num. Persons Handle. Requests names one. Name num. Persons found index Look. Up 25
![void Get Data out int ids out float void Get. Data ( /* out */ int ids[ ] , /* out*/ float](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-26.jpg)
void Get. Data ( /* out */ int ids[ ] , /* out*/ float wages[ ] , /* out */ String 20 names[ ] , /* out */ int & how. Many ) { ifstream my. Infile ; int k= 0; char ch ; // Reads data from data file my. Infile. open (“A: \my. dat”) ; if ( ! my. Infile ) { cout << “File opening error. Program terminated! “ << endl ; exit ( 1 ) ; } my. Infile >> ids[ k ] >> wages [k] ; // get information for first person my. Infile. get(ch) ; // read blank my. Infile. get (names[ k ] , 21) ; my. Infile. ignore(30, ‘n’) ; // consume newline while (my. Infile) // while the last read was successful { k++ ; my. Infile >> ids[ k ] >> wages [k] ; my. Infile. get(ch) ; // read blank my. Infile. get (names[ k ] , 21) ; my. Infile. ignore(30, ‘n’) ; // consume newline } 26 how. Many = k; }
![void Handle Requests const in int id Nums const in void Handle. Requests( const /* in */ int id. Nums[ ], const /* in](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-27.jpg)
void Handle. Requests( const /* in */ int id. Nums[ ], const /* in */ float wages[ ] , const /* in */ String 20 names[ ], /* in */ int num. Persons ) { String 20 int char Boolean do { one. Name ; index ; response; found; // string to hold name of one person // will hold an array index value // user’s response whether to continue // has one. Name been located in array names cout << “Enter name of person to find: ” ; cin. get (one. Name, 21) ; cin. ignore (100, ‘n’); // consume newline Look. Up (names, one. Name, num. Persons, found, index ); if ( found ) cout << one. Name << “ has ID # “ << id. Nums [index] << “ and hourly wage $ “ << wages [index] << endl; else cout << one. Name << “ was not located. “ << endl; cout << “Want to find another (Y/N)? “; cin >> response ; response = toupper ( response ); } while ( response == ‘Y’ ); } 27
![void Look Up const in String 20 names const void Look. Up ( const /* in */ String 20 names [ ], const](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-28.jpg)
void Look. Up ( const /* in */ String 20 names [ ], const /* in */ String 20 one. Name, /* in */ int num. Persons, /* out */ Boolean & found , /* out */ int & index) // Sequential search of unordered array. // POSTCONDITION: // IF one. Name is in names array // found == true && names[index] == one. Name // ELSE // found == false && index == num. Persons { index = 0; found = false; // initialize flag while ( ( ! found ) && ( index < num. Persons ) ) // more to search { if ( strcmp ( one. Name, names[index] ) == 0 ) // match here found = true ; // change flag else index ++ ; } 28 }
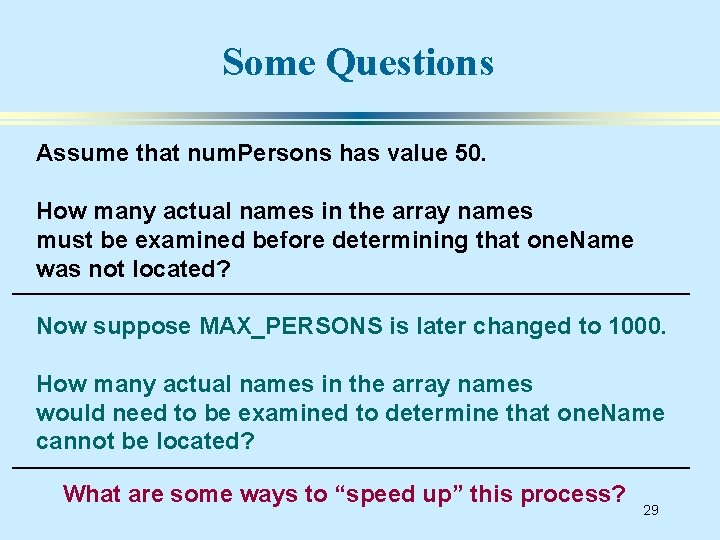
Some Questions Assume that num. Persons has value 50. How many actual names in the array names must be examined before determining that one. Name was not located? Now suppose MAX_PERSONS is later changed to 1000. How many actual names in the array names would need to be examined to determine that one. Name cannot be located? What are some ways to “speed up” this process? 29
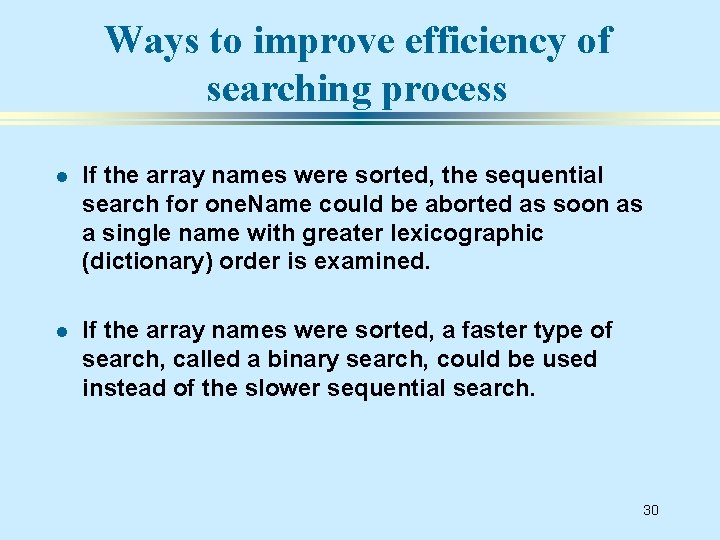
Ways to improve efficiency of searching process l If the array names were sorted, the sequential search for one. Name could be aborted as soon as a single name with greater lexicographic (dictionary) order is examined. l If the array names were sorted, a faster type of search, called a binary search, could be used instead of the slower sequential search. 30
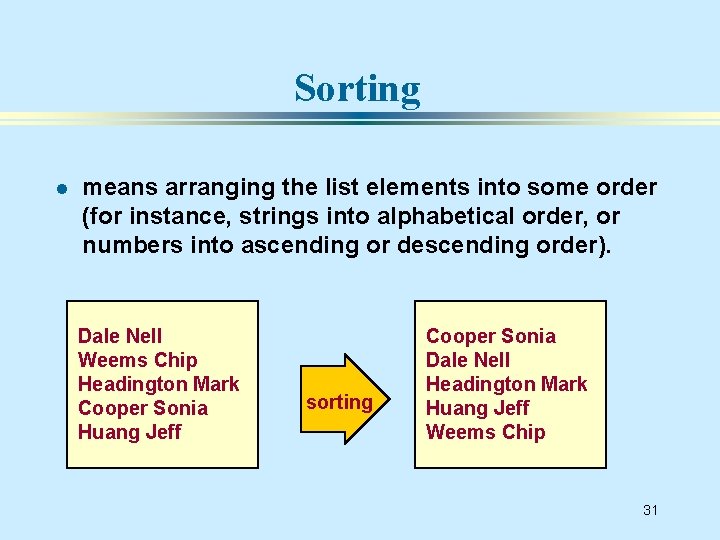
Sorting l means arranging the list elements into some order (for instance, strings into alphabetical order, or numbers into ascending or descending order). Dale Nell Weems Chip Headington Mark Cooper Sonia Huang Jeff sorting Cooper Sonia Dale Nell Headington Mark Huang Jeff Weems Chip 31
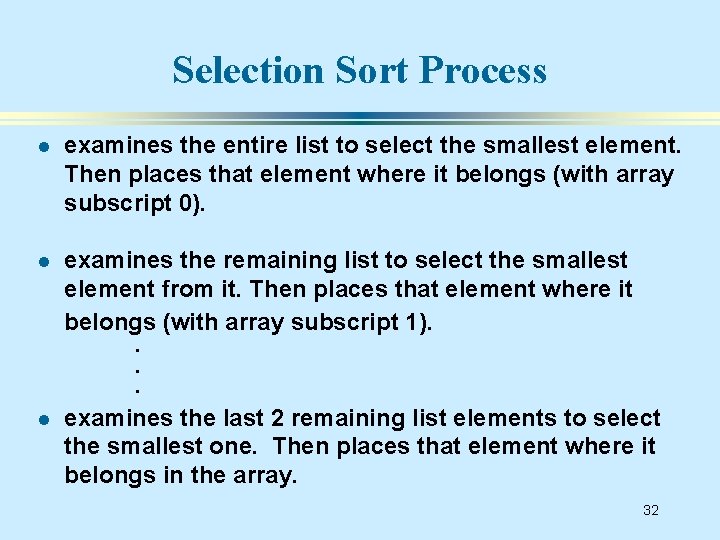
Selection Sort Process l examines the entire list to select the smallest element. Then places that element where it belongs (with array subscript 0). l examines the remaining list to select the smallest element from it. Then places that element where it belongs (with array subscript 1). . l examines the last 2 remaining list elements to select the smallest one. Then places that element where it belongs in the array. 32
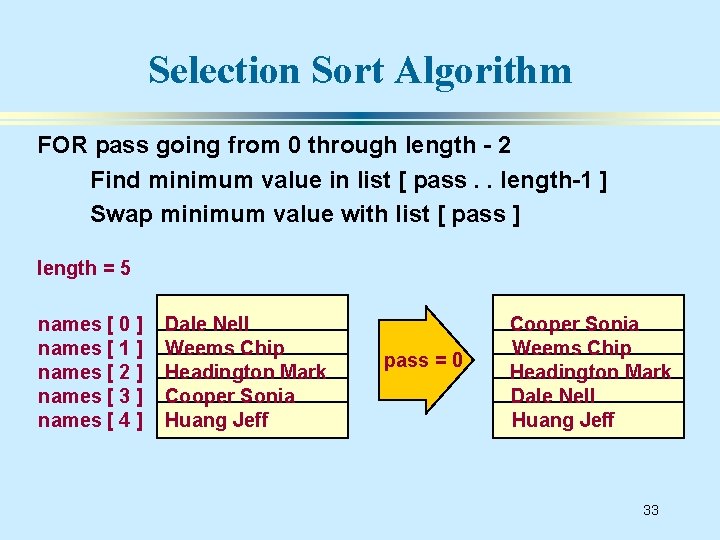
Selection Sort Algorithm FOR pass going from 0 through length - 2 Find minimum value in list [ pass. . length-1 ] Swap minimum value with list [ pass ] length = 5 names [ 0 ] names [ 1 ] names [ 2 ] names [ 3 ] names [ 4 ] Dale Nell Weems Chip Headington Mark Cooper Sonia Huang Jeff pass = 0 Cooper Sonia Weems Chip Headington Mark Dale Nell Huang Jeff 33
![void Sel Sort inout String 20 names void Sel. Sort ( /* inout */ String 20 names [ ] , /*](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-34.jpg)
void Sel. Sort ( /* inout */ String 20 names [ ] , /* in */ int length ) // Selection sorts names into alphabetic order // Preconditions: length <= MAX_PERSONS // && names [0. . length -1 ] are assigned // Postcondition: names [ 0. . length -1 ] are rearranged into order { int pass; int place; int min. Index; String 20 temp; for ( pass = 0 ; pass < length - 1 ; pass++ ) { min. Index = pass; for ( place = pass + 1 ; place < length ; place ++ ) if ( strcmp ( names [ place ] , names [ min. Index ] ) < 0 ) min. Index = place; //swap names[pass] with names[min. Index] strcpy ( temp , names [ min. Index ] ) ; strcpy ( names [ min. Index ] , names [ pass] ) ; strcpy ( names [ pass ] , temp ) ; } } 34
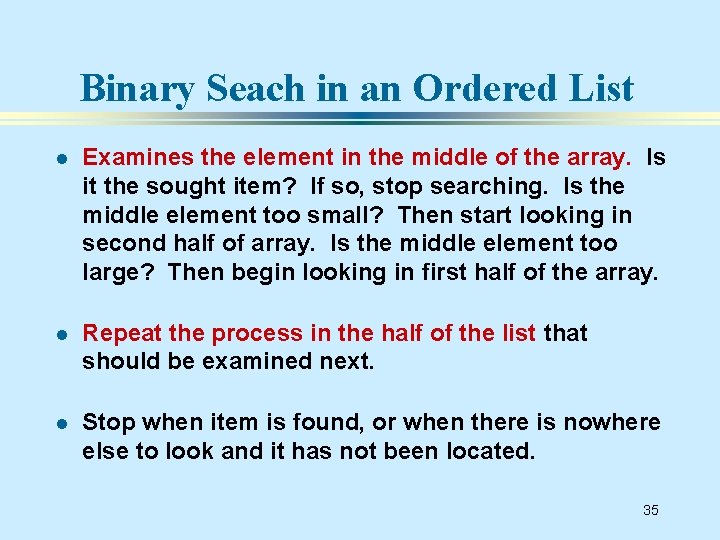
Binary Seach in an Ordered List l Examines the element in the middle of the array. Is it the sought item? If so, stop searching. Is the middle element too small? Then start looking in second half of array. Is the middle element too large? Then begin looking in first half of the array. l Repeat the process in the half of the list that should be examined next. l Stop when item is found, or when there is nowhere else to look and it has not been located. 35
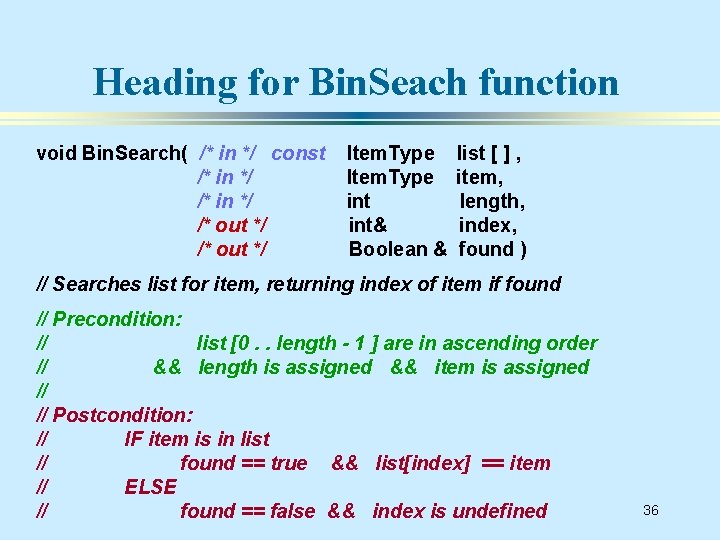
Heading for Bin. Seach function void Bin. Search( /* in */ const /* in */ /* out */ Item. Type int& Boolean & list [ ] , item, length, index, found ) // Searches list for item, returning index of item if found // Precondition: // list [0. . length - 1 ] are in ascending order // && length is assigned && item is assigned // // Postcondition: // IF item is in list // found == true && list[index] == item // ELSE // found == false && index is undefined 36
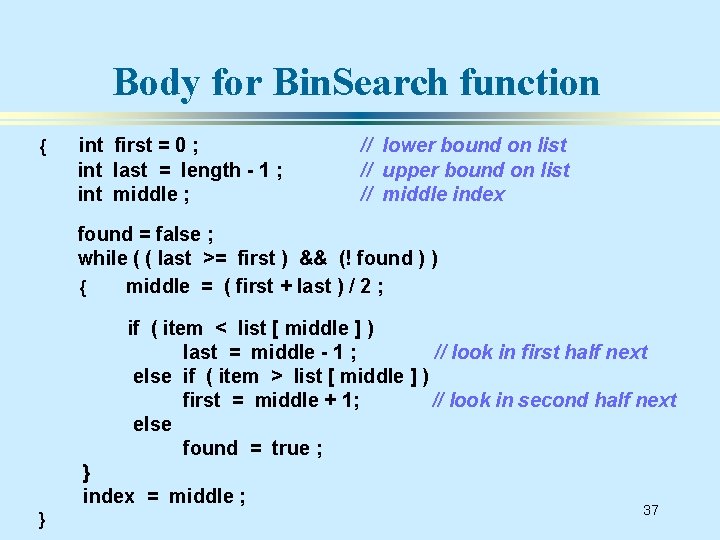
Body for Bin. Search function { int first = 0 ; int last = length - 1 ; int middle ; // lower bound on list // upper bound on list // middle index found = false ; while ( ( last >= first ) && (! found ) ) { middle = ( first + last ) / 2 ; if ( item < list [ middle ] ) last = middle - 1 ; // look in first half next else if ( item > list [ middle ] ) first = middle + 1; // look in second half next else found = true ; } index = middle ; } 37
![Trace of Bin Search function item 45 15 list0 26 38 1 2 Trace of Bin. Search function item = 45 15 list[0] 26 38 [1] [2]](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-38.jpg)
Trace of Bin. Search function item = 45 15 list[0] 26 38 [1] [2] 57 [3] first 62 [4] 78 [5] list[0] first [6] 91 [7] 108 119 [8] [9] middle last item < list [ middle ] 15 84 26 [1] middle 38 [2] 57 [3] 62 [4] last = middle - 1 78 [5] 84 [6] 91 [7] 108 119 [8] [9] last item > list [ middle ] first = middle + 1 38
![Trace continued item 45 15 list0 26 1 38 2 first middle 57 Trace continued item = 45 15 list[0] 26 [1] 38 [2] first, middle 57](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-39.jpg)
Trace continued item = 45 15 list[0] 26 [1] 38 [2] first, middle 57 [3] 62 [4] 78 [5] list[0] 26 [1] 38 [2] [6] 91 [7] 108 119 [8] [9] last item > list [ middle ] 15 84 57 [3] first = middle + 1 62 [4] 78 [5] 84 [6] 91 [7] first, middle, last item < list [ middle ] last = middle - 1 39
![Trace concludes item 45 15 list0 26 1 38 57 2 3 last Trace concludes item = 45 15 list[0] 26 [1] 38 57 [2] [3] last](https://slidetodoc.com/presentation_image/40f211103c84eb3adfc52483b092f2e0/image-40.jpg)
Trace concludes item = 45 15 list[0] 26 [1] 38 57 [2] [3] last first last < first 62 [4] 78 [5] 84 [6] 91 [7] 108 119 [8] [9] found = false 40
Parallel arrays java
Array of arrays c++
Ragged array
潘仁義
C++ parallel arrays
Why do we need arrays?
Dynamic arrays and amortized analysis
Arreglos unidimensionales en java ejemplos
Arreglos bidimensionales en java
Arrays in mips
Polynomial representation using array in c
Array of strings assembly
Global arrays in c
Computer science arrays
Searching and sorting arrays in c++
Arrays visual basic
Python parallel arrays
Advantages and disadvantages of array over linked list
I wonder is it possible
2d array pascal
Declare array in mips
Creating arrays matlab
Array adt
Java partially filled array
Redundancy array of independent disk
Python list of arrays
Arrays
Day 3: arrays
Raid redundant array of independent disks
Small basic array examples
Disadvantages of dynamic array
Microled arrays
Are vectors dynamic arrays
Facts about arrays
Perbedaan linear programming dan integer programming
Greedy vs dynamic
Runtime programming
Integer programming vs linear programming
Definisi linear
Python chapter 5 programming exercises
The zj row in a simplex table for maximization represents