Programming in C DaleWeemsHeadington Chapter 15 continued Inheritance
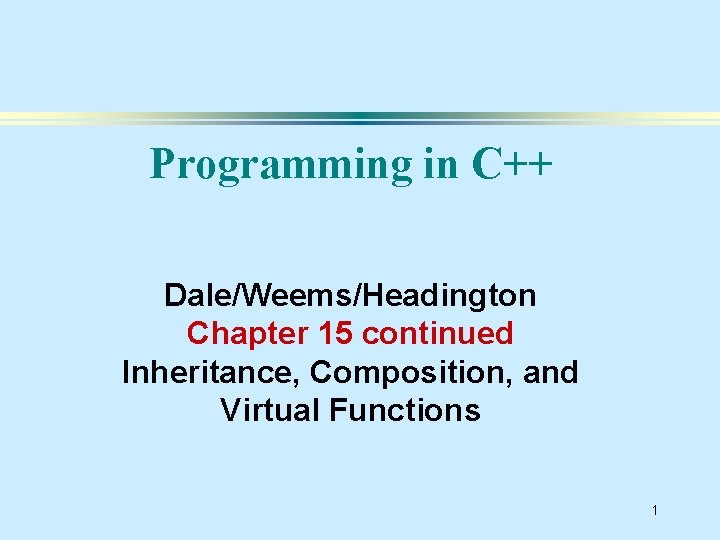
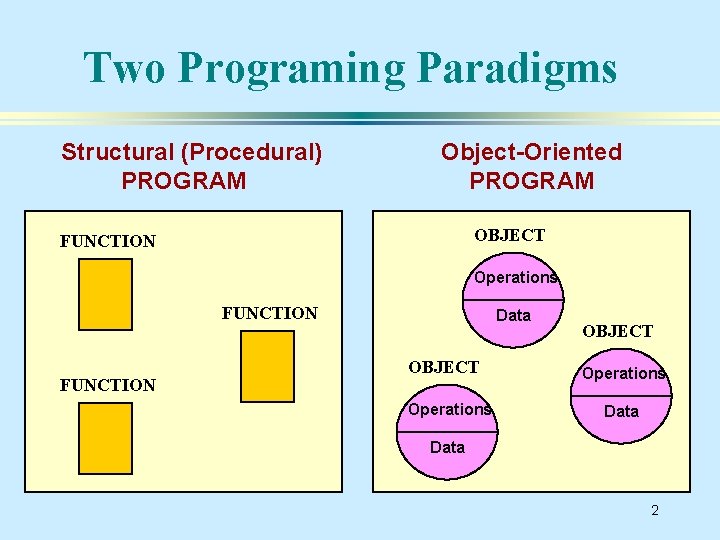
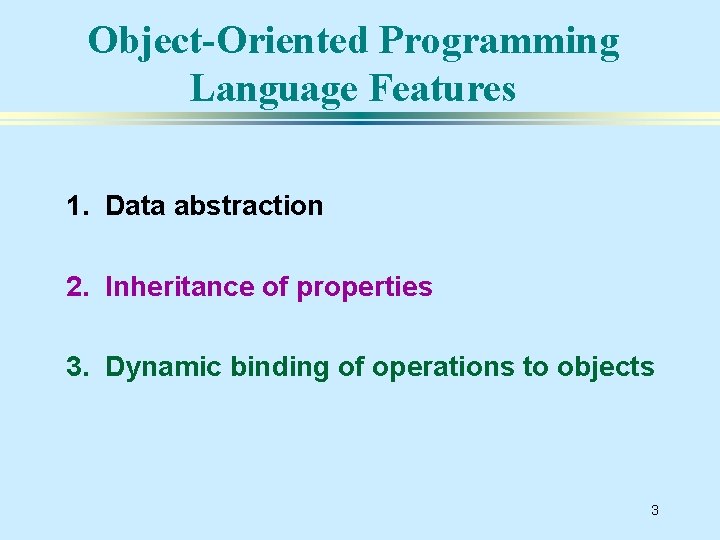
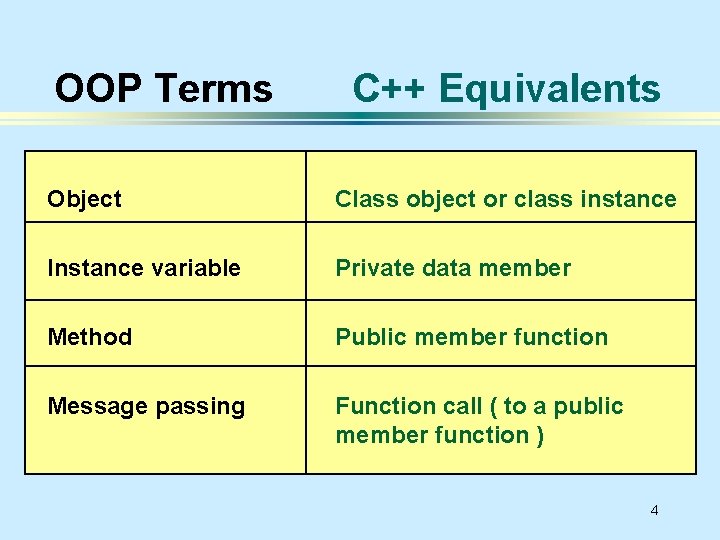
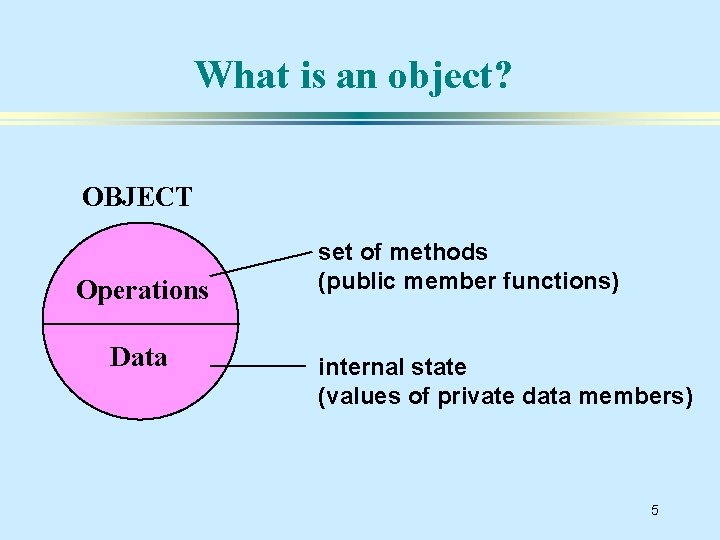
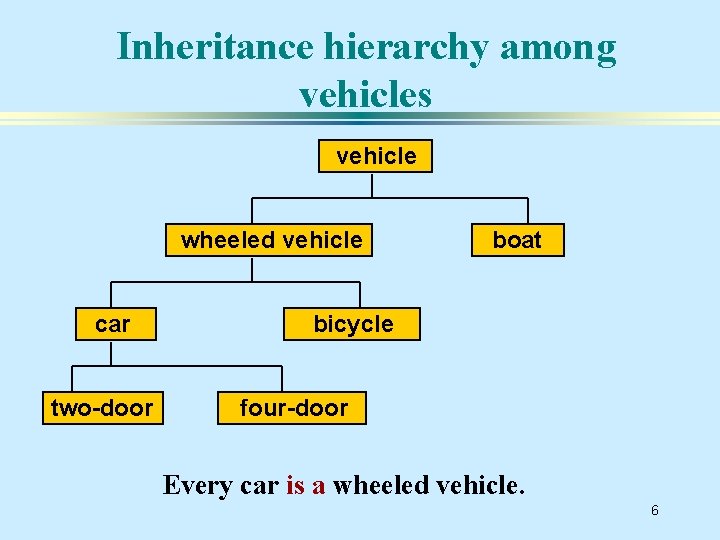
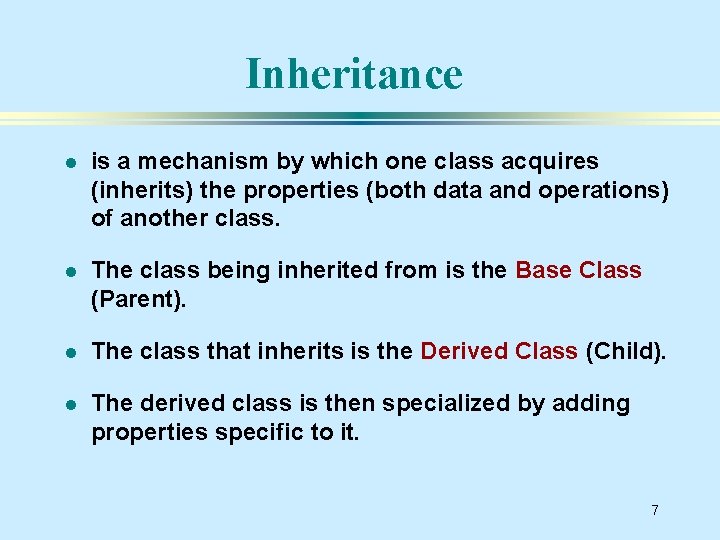
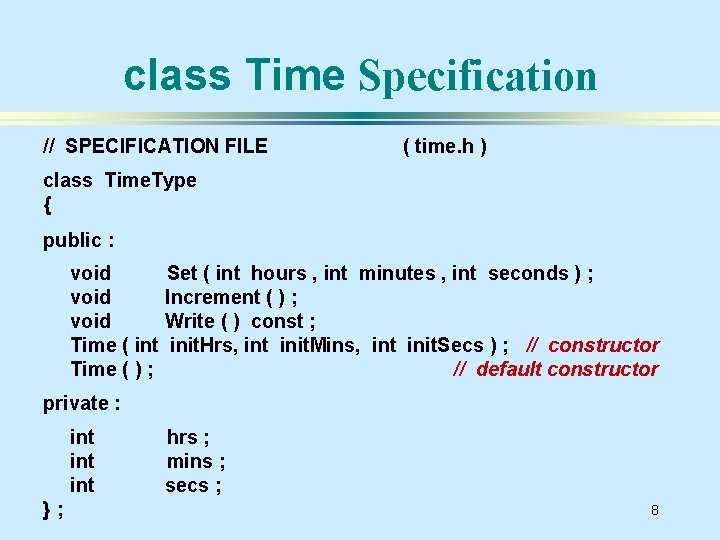
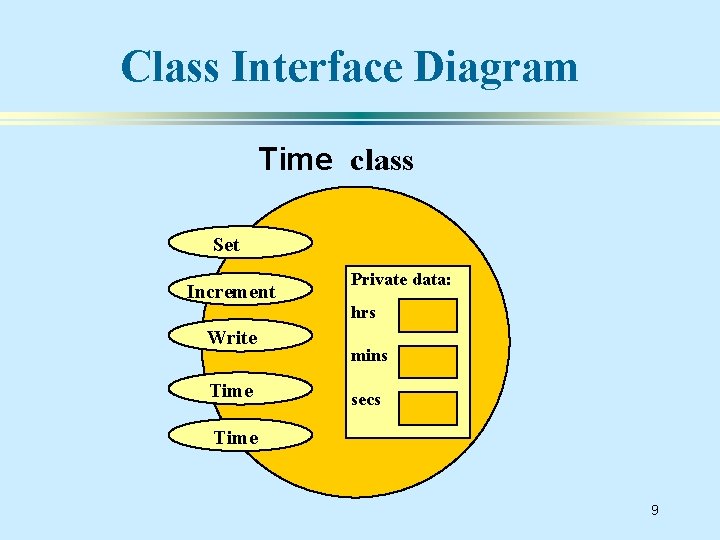
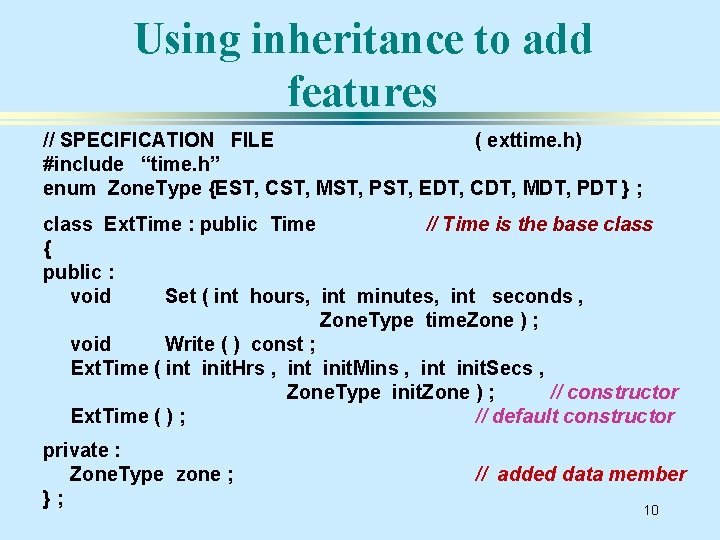
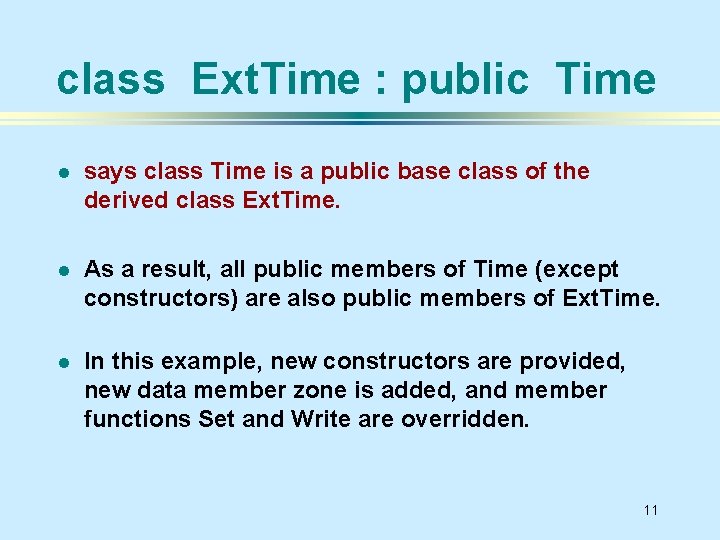
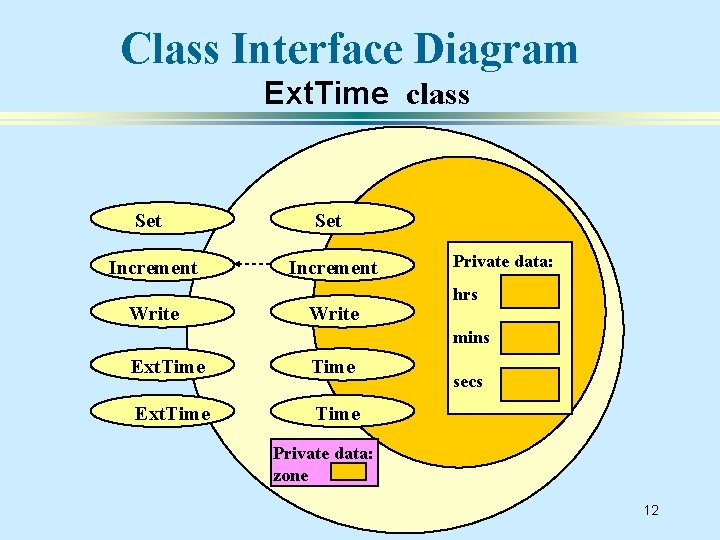
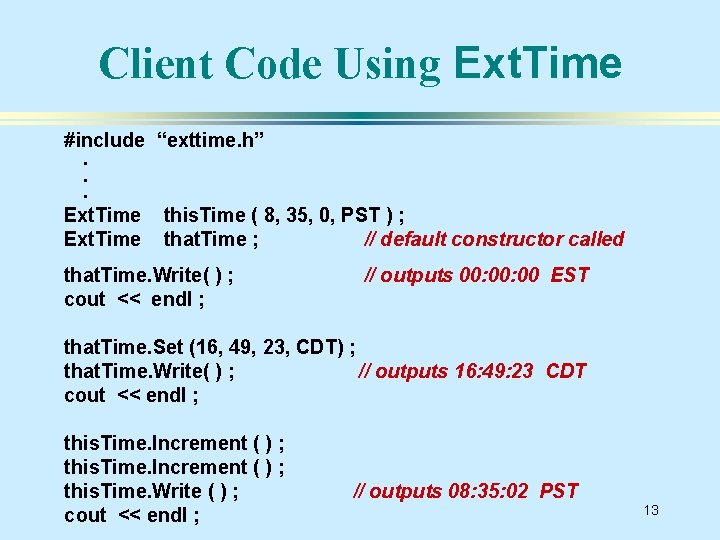
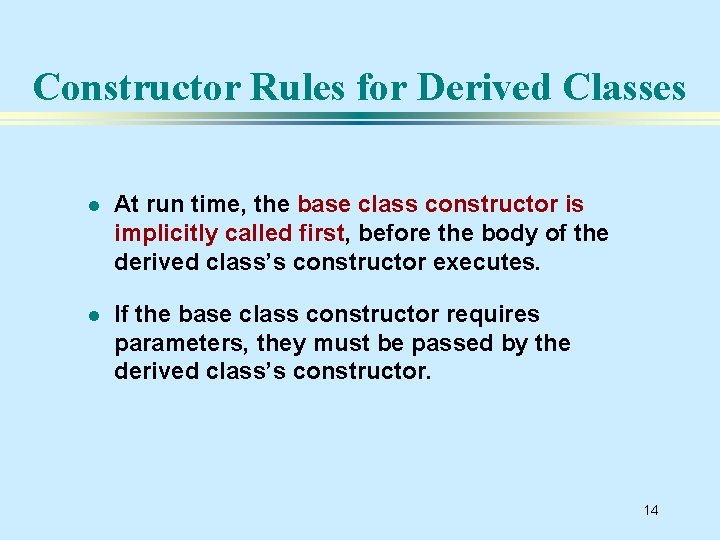
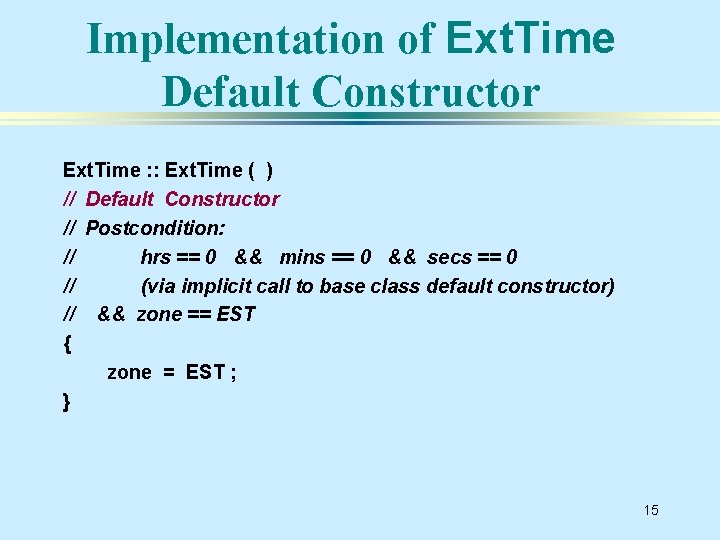
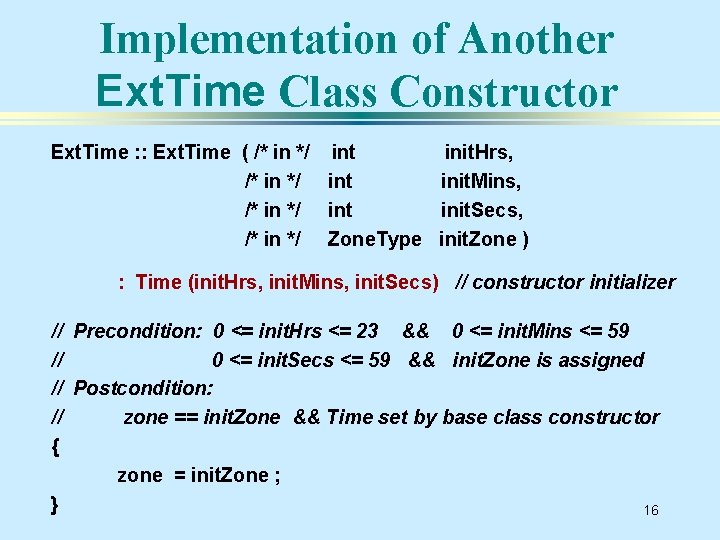
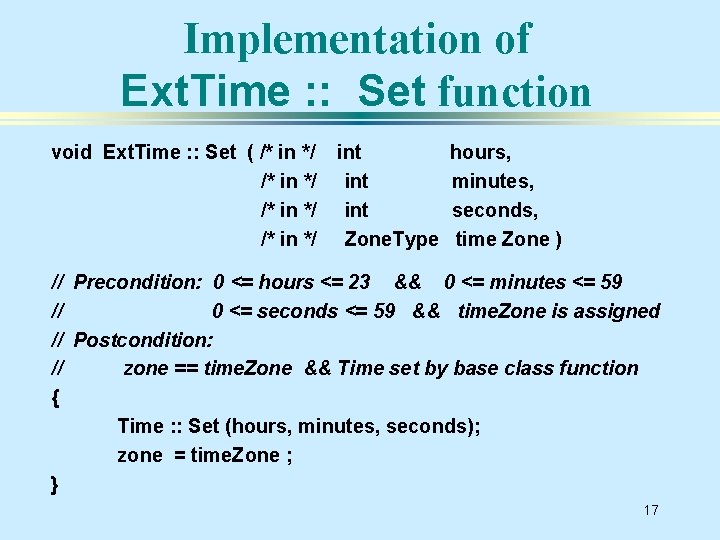
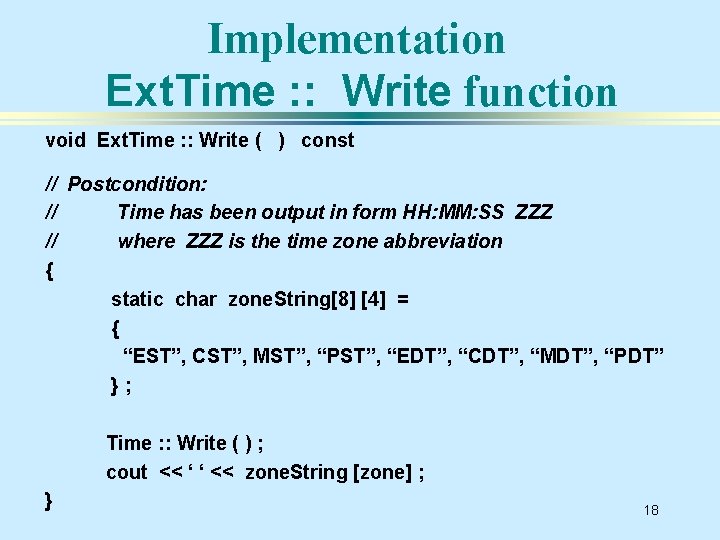
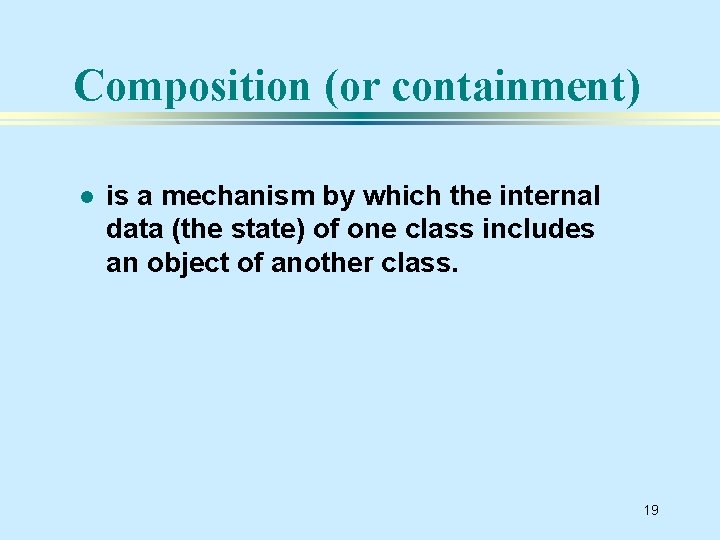
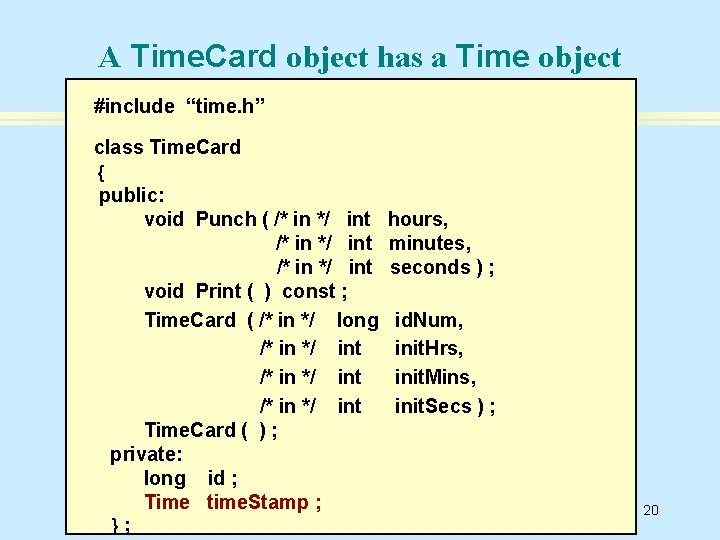
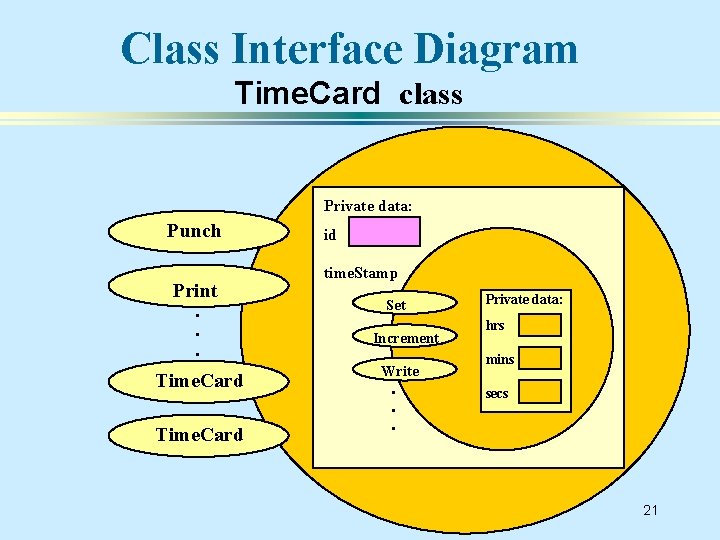
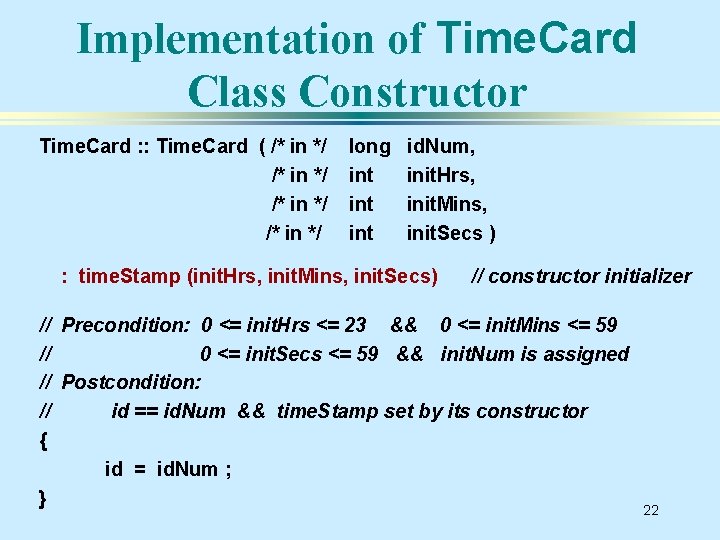
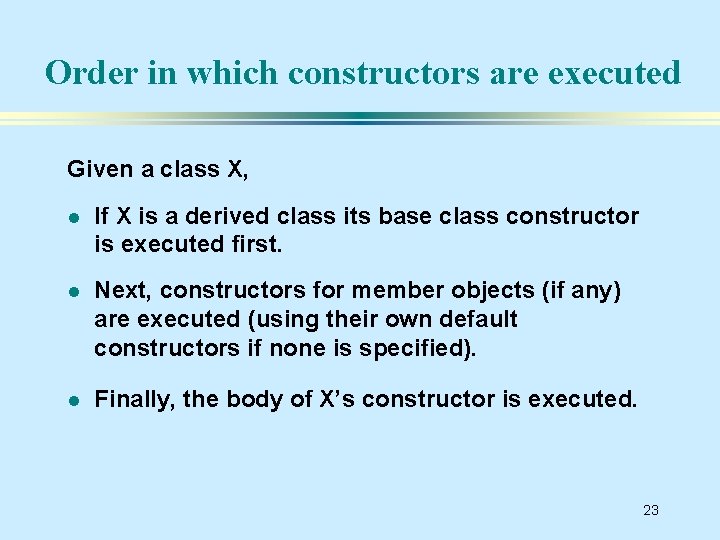
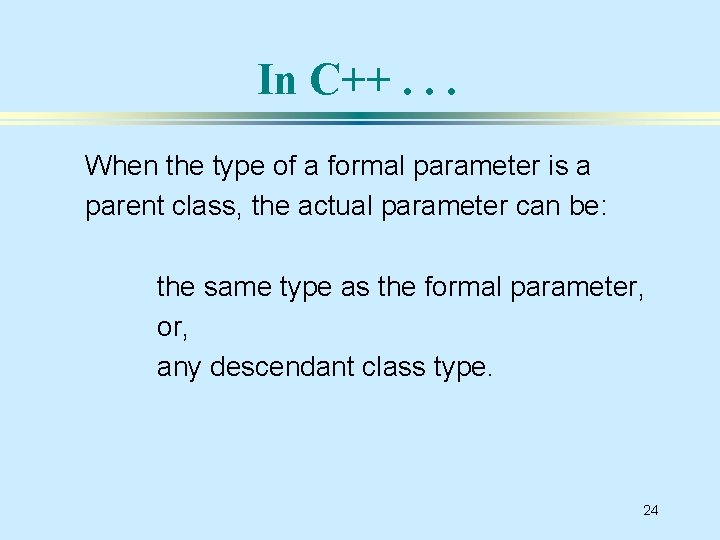
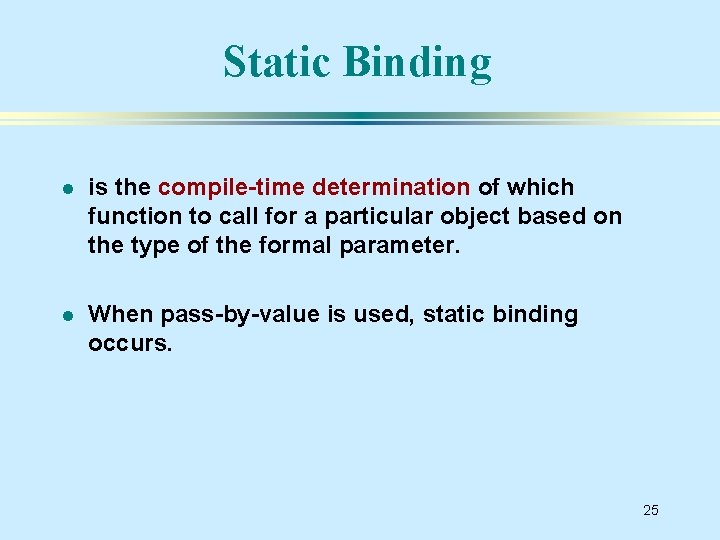
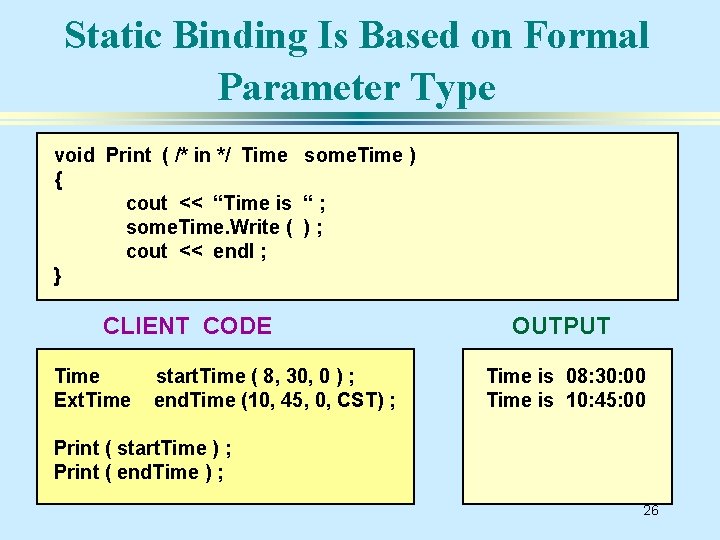
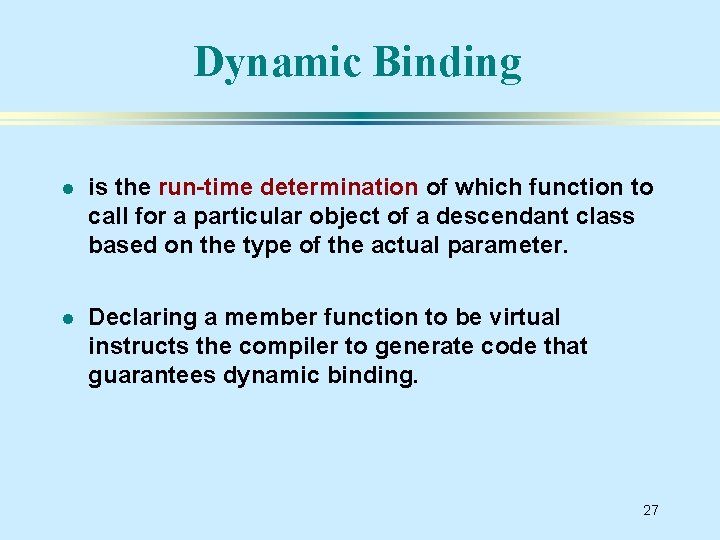
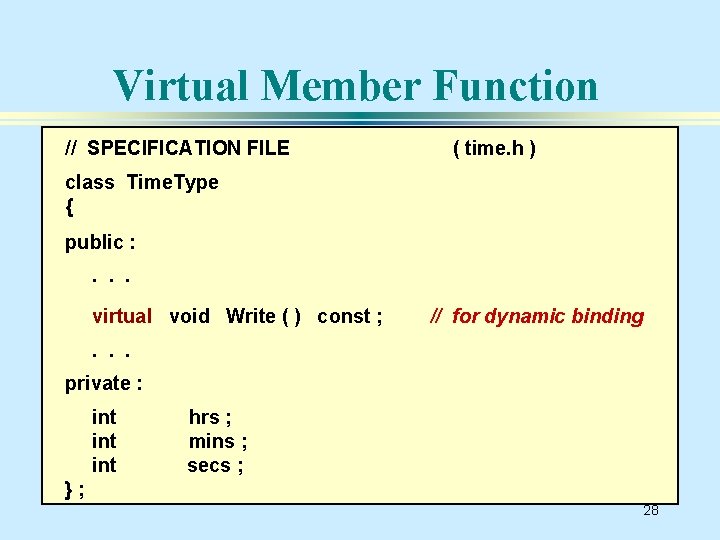
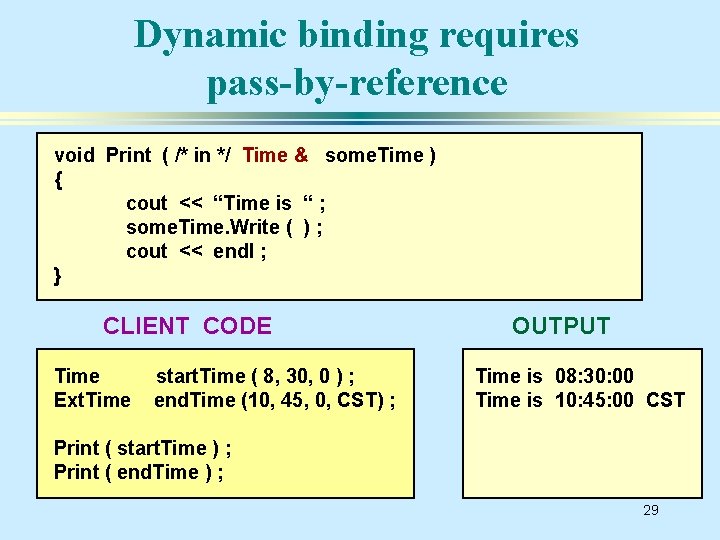
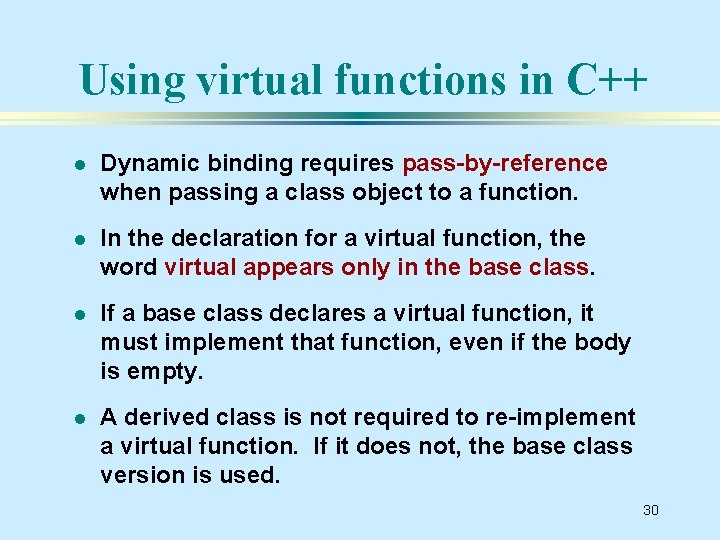
- Slides: 30
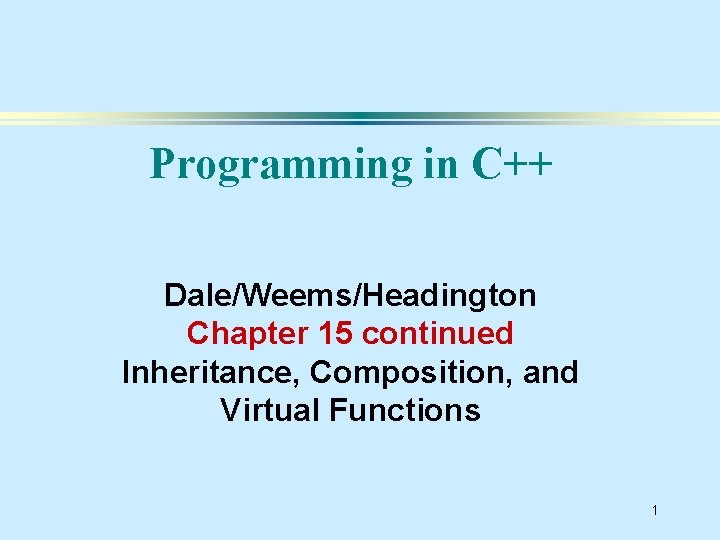
Programming in C++ Dale/Weems/Headington Chapter 15 continued Inheritance, Composition, and Virtual Functions 1
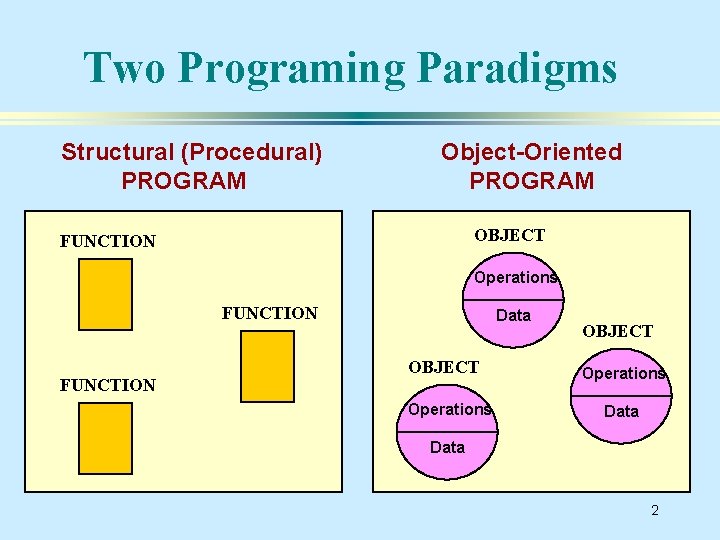
Two Programing Paradigms Structural (Procedural) PROGRAM Object-Oriented PROGRAM OBJECT FUNCTION Operations FUNCTION Data OBJECT Operations Data 2
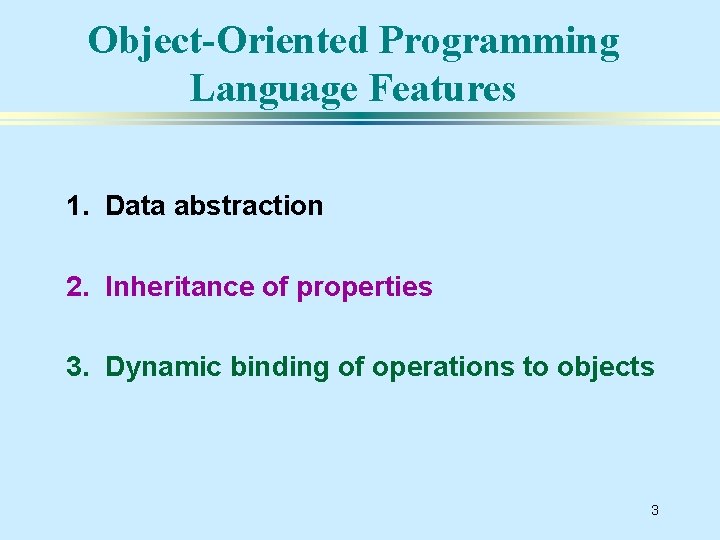
Object-Oriented Programming Language Features 1. Data abstraction 2. Inheritance of properties 3. Dynamic binding of operations to objects 3
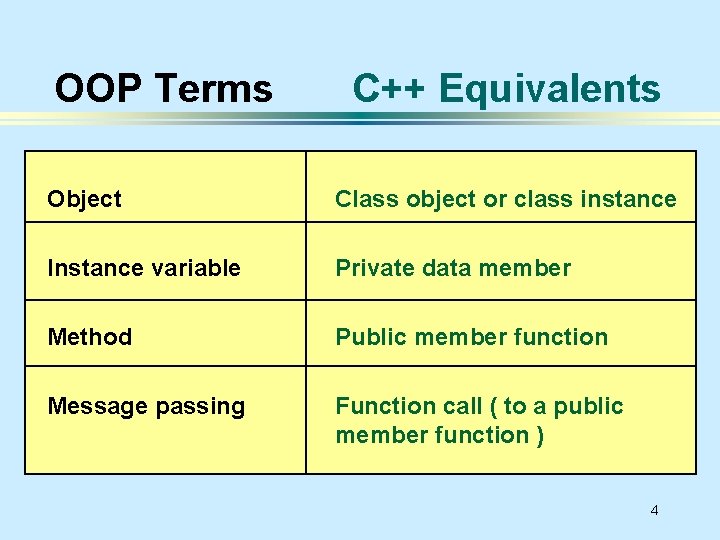
OOP Terms C++ Equivalents Object Class object or class instance Instance variable Private data member Method Public member function Message passing Function call ( to a public member function ) 4
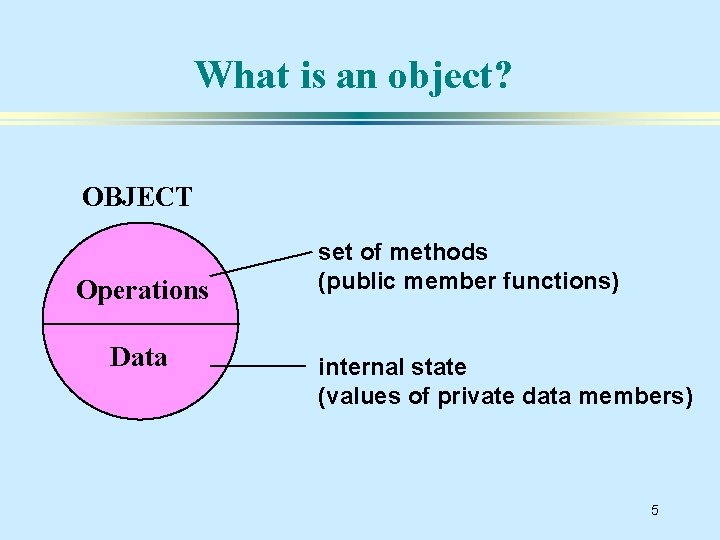
What is an object? OBJECT Operations Data set of methods (public member functions) internal state (values of private data members) 5
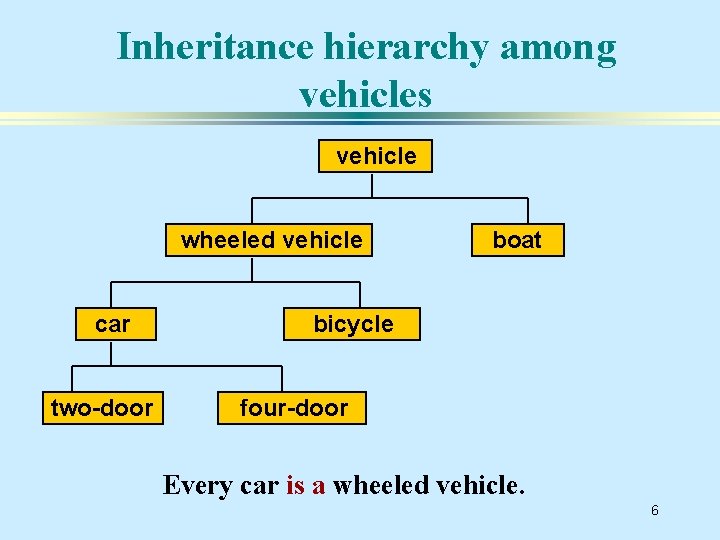
Inheritance hierarchy among vehicles vehicle wheeled vehicle car two-door boat bicycle four-door Every car is a wheeled vehicle. 6
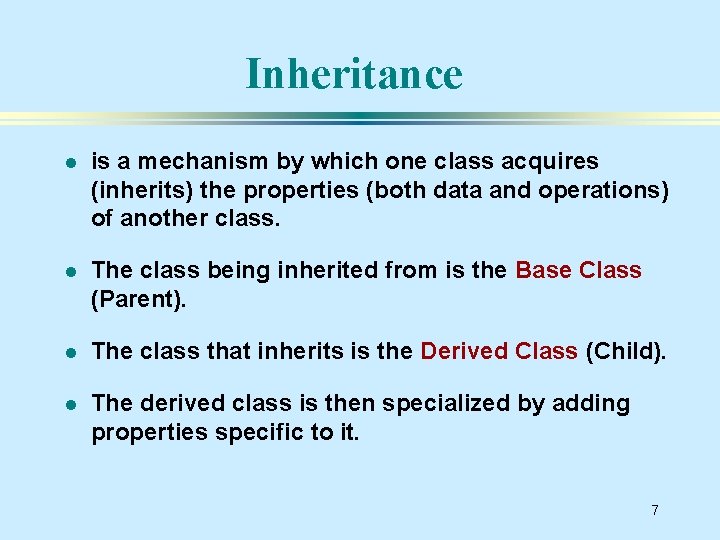
Inheritance l is a mechanism by which one class acquires (inherits) the properties (both data and operations) of another class. l The class being inherited from is the Base Class (Parent). l The class that inherits is the Derived Class (Child). l The derived class is then specialized by adding properties specific to it. 7
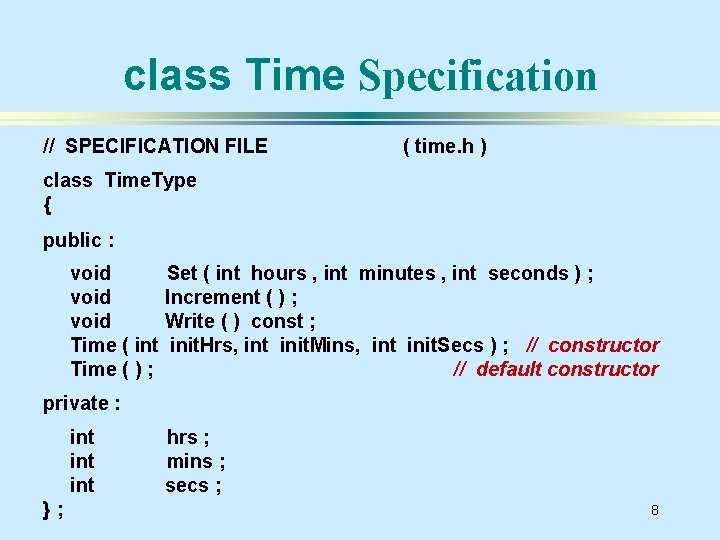
class Time Specification // SPECIFICATION FILE ( time. h ) class Time. Type { public : void Time ( int Time ( ) ; Set ( int hours , int minutes , int seconds ) ; Increment ( ) ; Write ( ) const ; init. Hrs, int init. Mins, int init. Secs ) ; // constructor // default constructor private : int int }; hrs ; mins ; secs ; 8
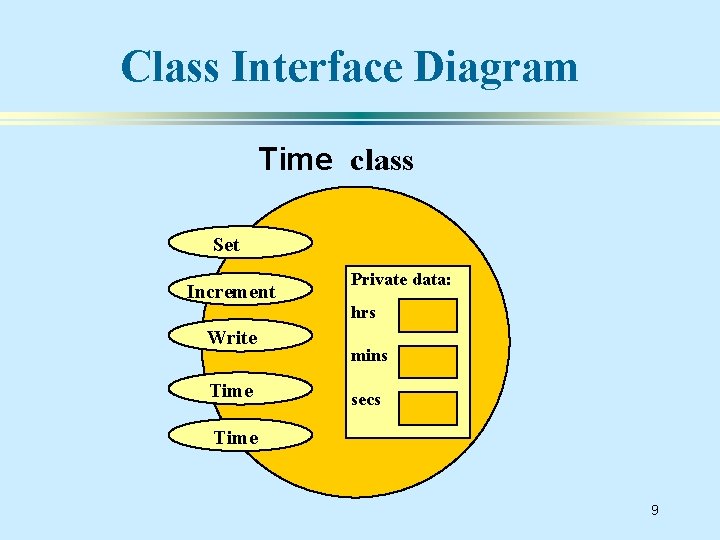
Class Interface Diagram Time class Set Increment Private data: hrs Write Time mins secs Time 9
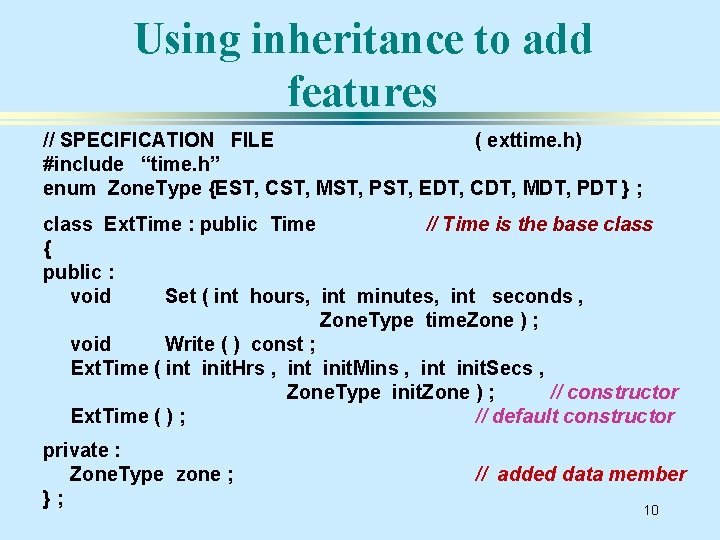
Using inheritance to add features // SPECIFICATION FILE ( exttime. h) #include “time. h” enum Zone. Type {EST, CST, MST, PST, EDT, CDT, MDT, PDT } ; class Ext. Time : public Time // Time is the base class { public : void Set ( int hours, int minutes, int seconds , Zone. Type time. Zone ) ; void Write ( ) const ; Ext. Time ( int init. Hrs , int init. Mins , int init. Secs , Zone. Type init. Zone ) ; // constructor Ext. Time ( ) ; // default constructor private : Zone. Type zone ; }; // added data member 10
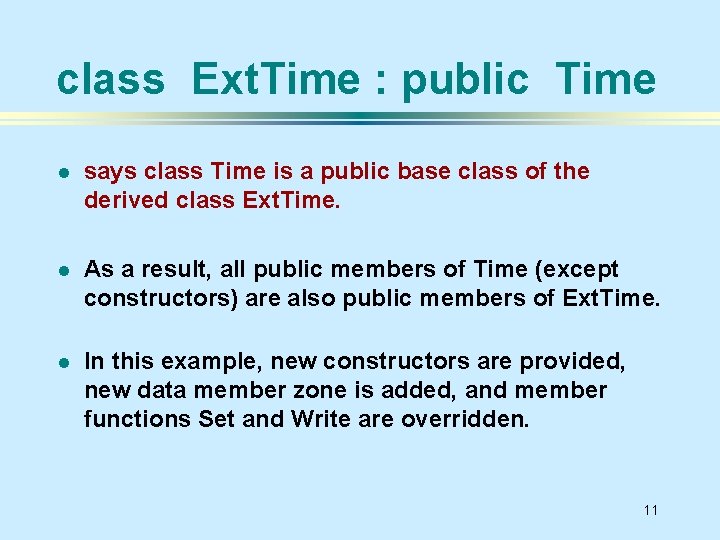
class Ext. Time : public Time l says class Time is a public base class of the derived class Ext. Time. l As a result, all public members of Time (except constructors) are also public members of Ext. Time. l In this example, new constructors are provided, new data member zone is added, and member functions Set and Write are overridden. 11
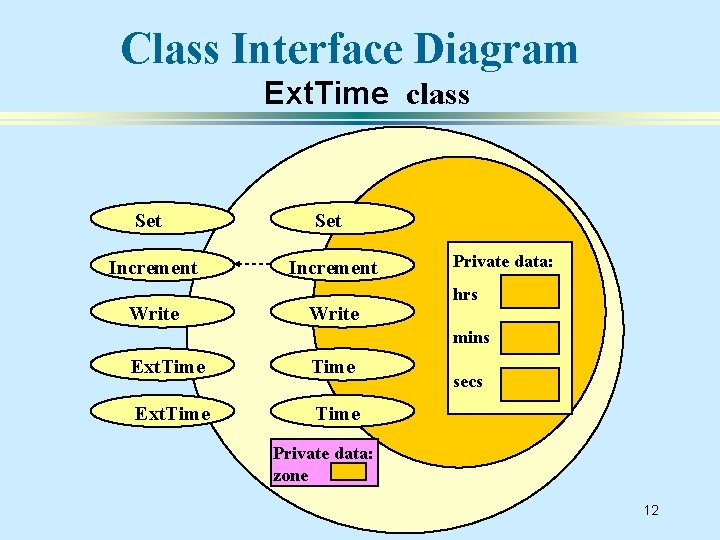
Class Interface Diagram Ext. Time class Set Increment Write Private data: hrs mins Ext. Time secs Private data: zone 12
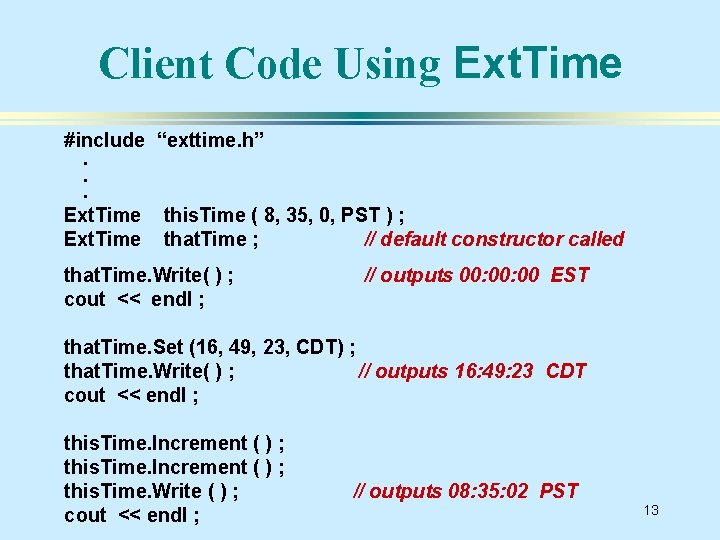
Client Code Using Ext. Time #include “exttime. h”. . . Ext. Time this. Time ( 8, 35, 0, PST ) ; that. Time ; // default constructor called that. Time. Write( ) ; cout << endl ; // outputs 00: 00 EST that. Time. Set (16, 49, 23, CDT) ; that. Time. Write( ) ; // outputs 16: 49: 23 CDT cout << endl ; this. Time. Increment ( ) ; this. Time. Write ( ) ; cout << endl ; // outputs 08: 35: 02 PST 13
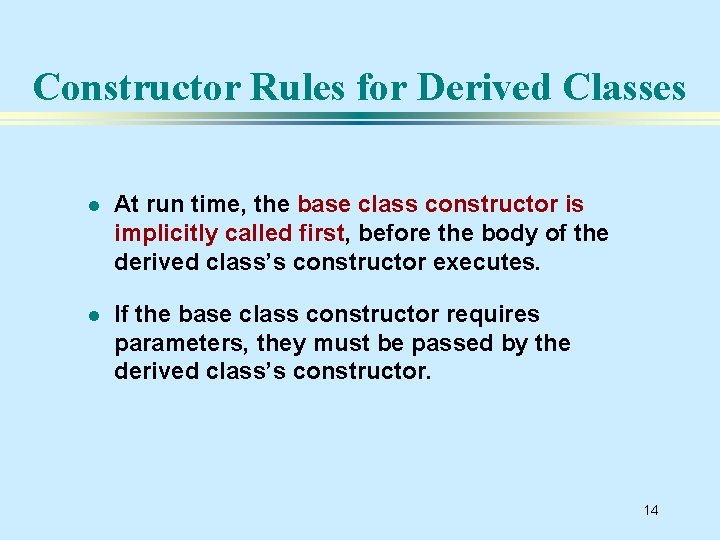
Constructor Rules for Derived Classes l At run time, the base class constructor is implicitly called first, before the body of the derived class’s constructor executes. l If the base class constructor requires parameters, they must be passed by the derived class’s constructor. 14
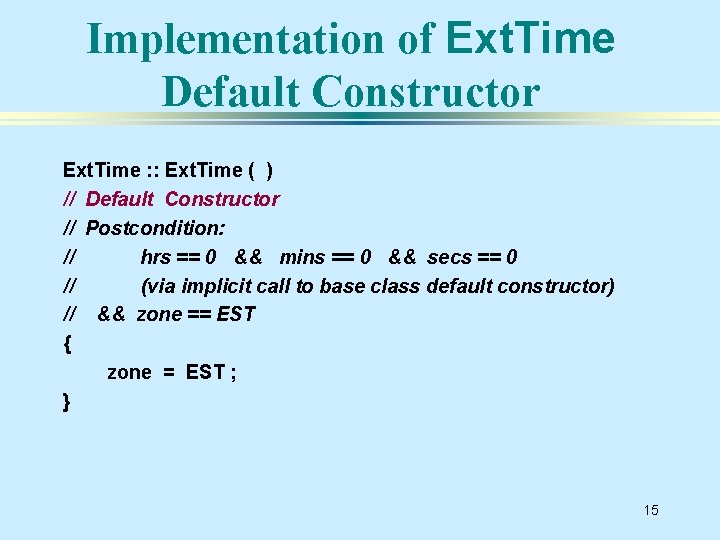
Implementation of Ext. Time Default Constructor Ext. Time : : Ext. Time ( ) // Default Constructor // Postcondition: // hrs == 0 && mins == 0 && secs == 0 // (via implicit call to base class default constructor) // && zone == EST { zone = EST ; } 15
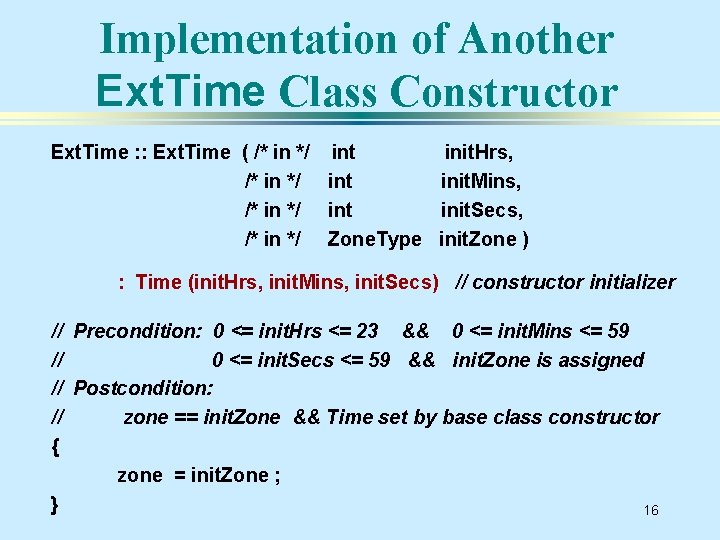
Implementation of Another Ext. Time Class Constructor Ext. Time : : Ext. Time ( /* in */ int init. Hrs, /* in */ int init. Mins, /* in */ int init. Secs, /* in */ Zone. Type init. Zone ) : Time (init. Hrs, init. Mins, init. Secs) // constructor initializer // Precondition: 0 <= init. Hrs <= 23 && 0 <= init. Mins <= 59 // 0 <= init. Secs <= 59 && init. Zone is assigned // Postcondition: // zone == init. Zone && Time set by base class constructor { zone = init. Zone ; } 16
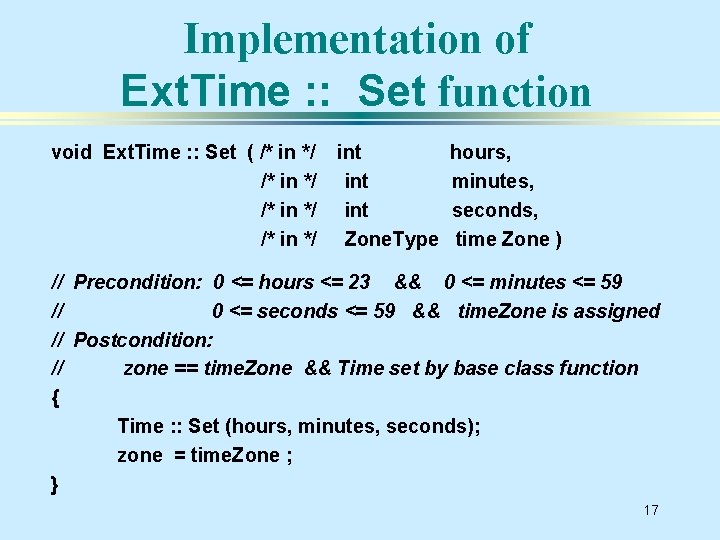
Implementation of Ext. Time : : Set function void Ext. Time : : Set ( /* in */ int int Zone. Type hours, minutes, seconds, time Zone ) // Precondition: 0 <= hours <= 23 && 0 <= minutes <= 59 // 0 <= seconds <= 59 && time. Zone is assigned // Postcondition: // zone == time. Zone && Time set by base class function { Time : : Set (hours, minutes, seconds); zone = time. Zone ; } 17
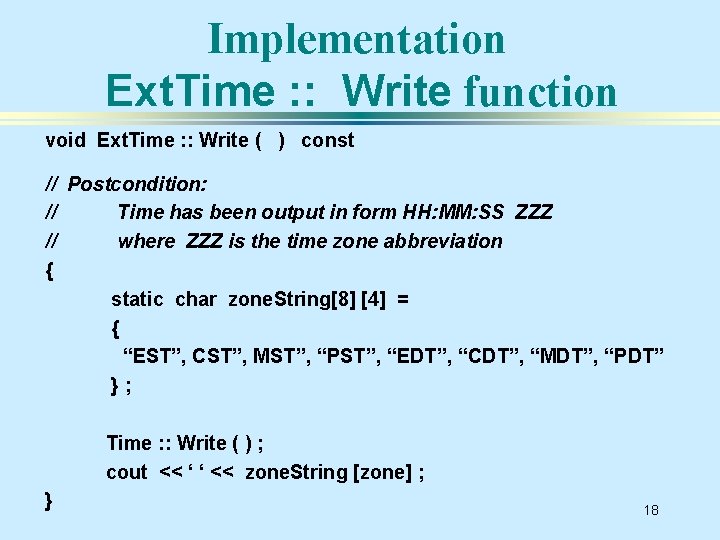
Implementation Ext. Time : : Write function void Ext. Time : : Write ( ) const // Postcondition: // Time has been output in form HH: MM: SS ZZZ // where ZZZ is the time zone abbreviation { static char zone. String[8] [4] = { “EST”, CST”, MST”, “PST”, “EDT”, “CDT”, “MDT”, “PDT” }; Time : : Write ( ) ; cout << ‘ ‘ << zone. String [zone] ; } 18
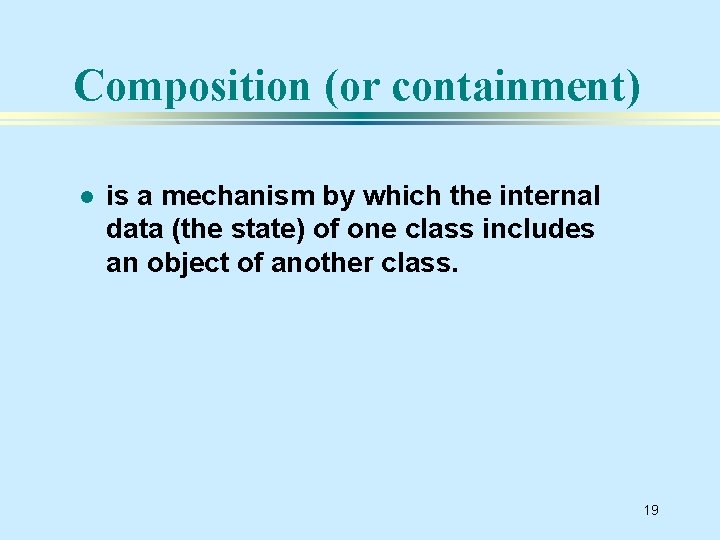
Composition (or containment) l is a mechanism by which the internal data (the state) of one class includes an object of another class. 19
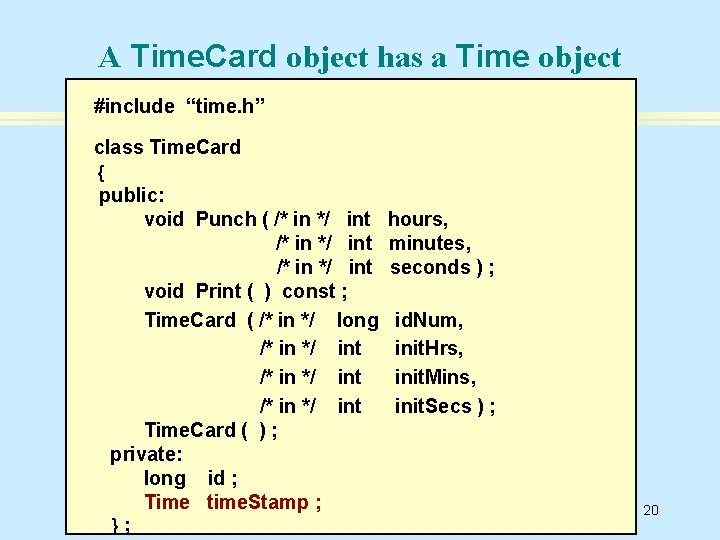
A Time. Card object has a Time object #include “time. h” class Time. Card { public: void Punch ( /* in */ int void Print ( ) const ; Time. Card ( /* in */ long /* in */ int Time. Card ( ) ; private: long id ; Time time. Stamp ; }; hours, minutes, seconds ) ; id. Num, init. Hrs, init. Mins, init. Secs ) ; 20
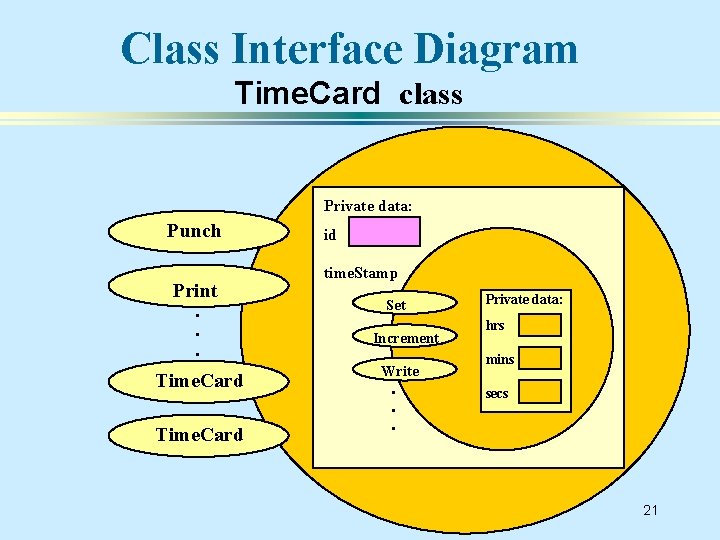
Class Interface Diagram Time. Card class Private data: Punch Print. . . Time. Card id time. Stamp Set Increment Write. . . Private data: hrs mins secs 21
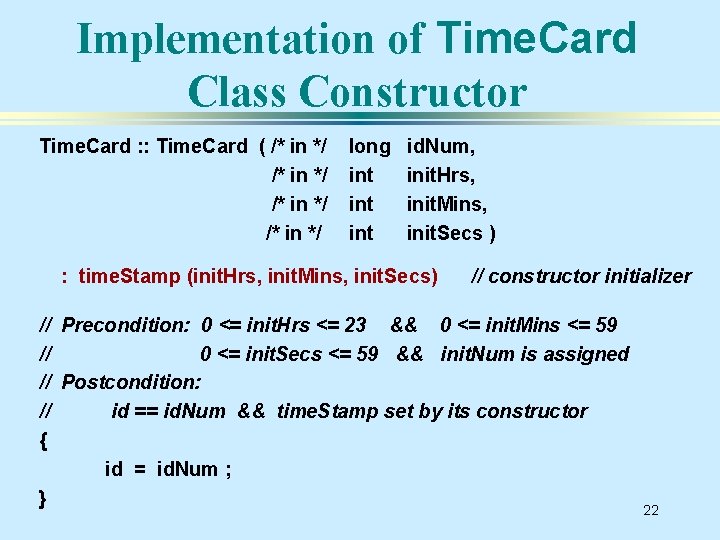
Implementation of Time. Card Class Constructor Time. Card : : Time. Card ( /* in */ long int int id. Num, init. Hrs, init. Mins, init. Secs ) : time. Stamp (init. Hrs, init. Mins, init. Secs) // constructor initializer // Precondition: 0 <= init. Hrs <= 23 && 0 <= init. Mins <= 59 // 0 <= init. Secs <= 59 && init. Num is assigned // Postcondition: // id == id. Num && time. Stamp set by its constructor { id = id. Num ; } 22
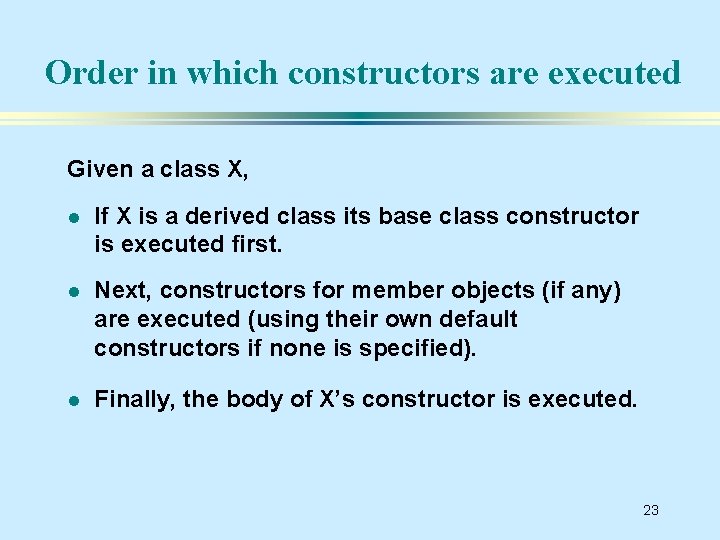
Order in which constructors are executed Given a class X, l If X is a derived class its base class constructor is executed first. l Next, constructors for member objects (if any) are executed (using their own default constructors if none is specified). l Finally, the body of X’s constructor is executed. 23
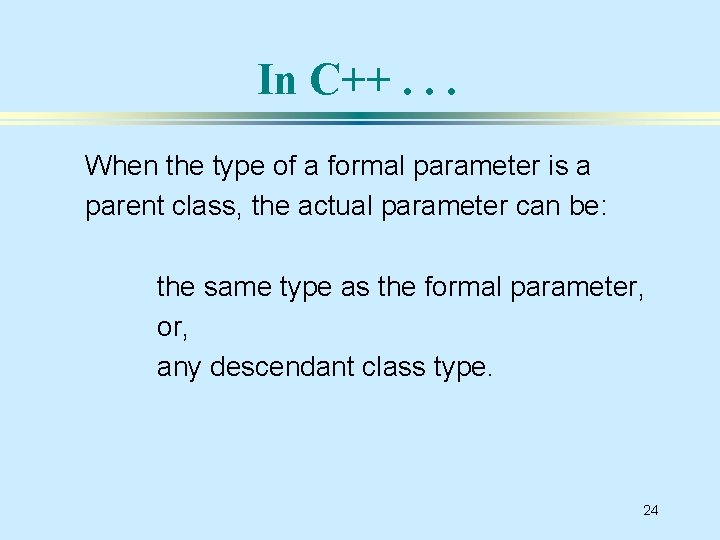
In C++. . . When the type of a formal parameter is a parent class, the actual parameter can be: the same type as the formal parameter, or, any descendant class type. 24
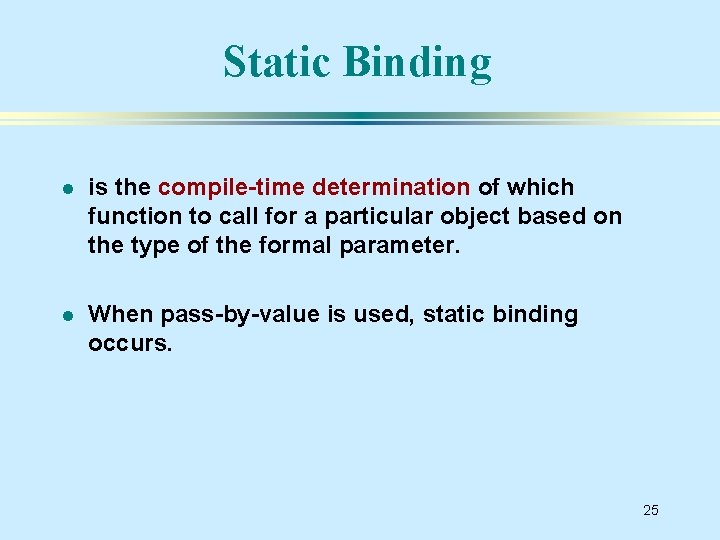
Static Binding l is the compile-time determination of which function to call for a particular object based on the type of the formal parameter. l When pass-by-value is used, static binding occurs. 25
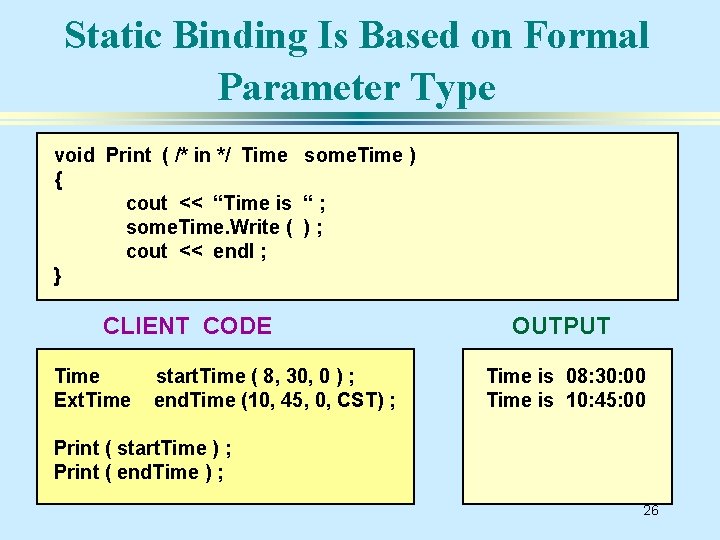
Static Binding Is Based on Formal Parameter Type void Print ( /* in */ Time some. Time ) { cout << “Time is “ ; some. Time. Write ( ) ; cout << endl ; } CLIENT CODE Time Ext. Time start. Time ( 8, 30, 0 ) ; end. Time (10, 45, 0, CST) ; OUTPUT Time is 08: 30: 00 Time is 10: 45: 00 Print ( start. Time ) ; Print ( end. Time ) ; 26
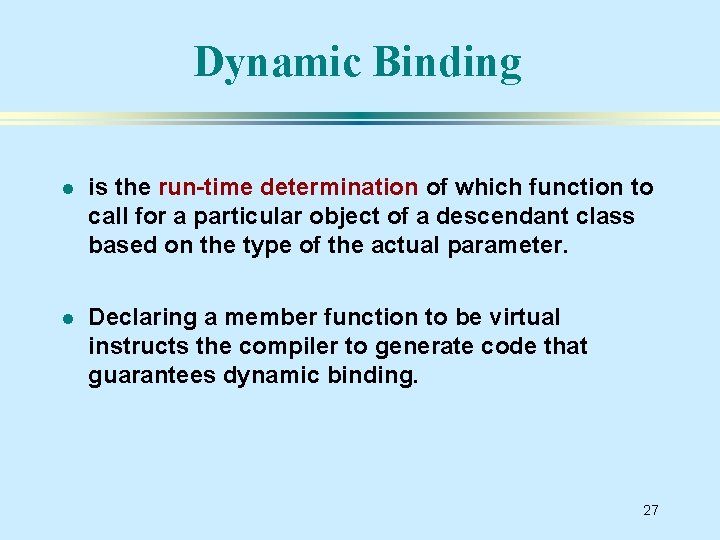
Dynamic Binding l is the run-time determination of which function to call for a particular object of a descendant class based on the type of the actual parameter. l Declaring a member function to be virtual instructs the compiler to generate code that guarantees dynamic binding. 27
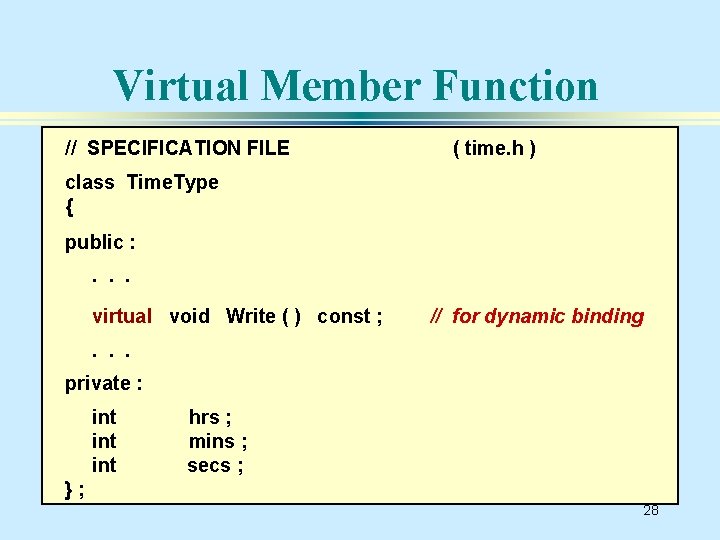
Virtual Member Function // SPECIFICATION FILE ( time. h ) class Time. Type { public : . . . virtual void Write ( ) const ; // for dynamic binding . . . private : int int hrs ; mins ; secs ; }; 28
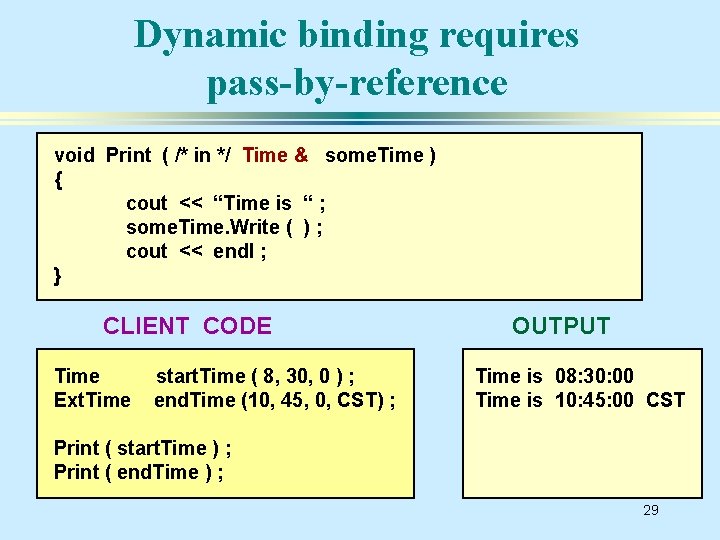
Dynamic binding requires pass-by-reference void Print ( /* in */ Time & some. Time ) { cout << “Time is “ ; some. Time. Write ( ) ; cout << endl ; } CLIENT CODE Time Ext. Time start. Time ( 8, 30, 0 ) ; end. Time (10, 45, 0, CST) ; OUTPUT Time is 08: 30: 00 Time is 10: 45: 00 CST Print ( start. Time ) ; Print ( end. Time ) ; 29
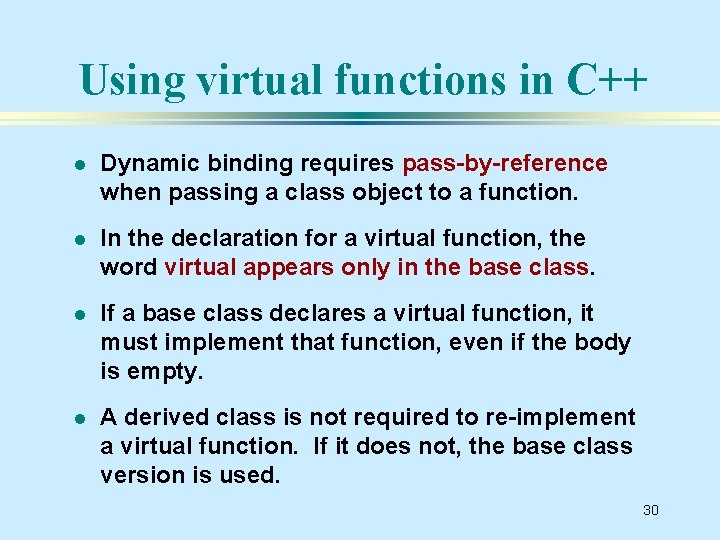
Using virtual functions in C++ l Dynamic binding requires pass-by-reference when passing a class object to a function. l In the declaration for a virtual function, the word virtual appears only in the base class. l If a base class declares a virtual function, it must implement that function, even if the body is empty. l A derived class is not required to re-implement a virtual function. If it does not, the base class version is used. 30
Chapter 8 section 3 cellular respiration continued
Perbedaan linear programming dan integer programming
Greedy vs dynamic
System programming vs application programming
Integer programming vs linear programming
Programing adalah
Chapter 11 section 2: complex patterns of inheritance
Chapter 16 the molecular basis of inheritance
Chromosomal theory of inheritance
Chapter 11 complex inheritance and human heredity test
Chapter 9 patterns of inheritance
Chapter 16 molecular basis of inheritance
Chapter 15 the chromosomal basis of inheritance
The chromosomal basis of inheritance chapter 15
Chapter 15 the chromosomal basis of inheritance
Chapter 11 section 1 basic patterns of human inheritance
Chapter 11 section 1 basic patterns of human inheritance
Bioflix dna replication
Chapter 9 patterns of inheritance
Chapter 15: the chromosomal basis of inheritance
Romeo and juliet act 2 prologue meaning
Summary of act 4 and 5 romeo and juliet
Indirect object pronouns p 199
Abbreviation of continued
Signal words example
Table continued
Continued abbreviation
Continuous
Demonstrative adjectives continued answers
Tu 1 of 1 montres tes photos? (us)
Professional adjectives