Advanced Computer Programming Inheritance Sinan AYDIN Inheritance Inheritance
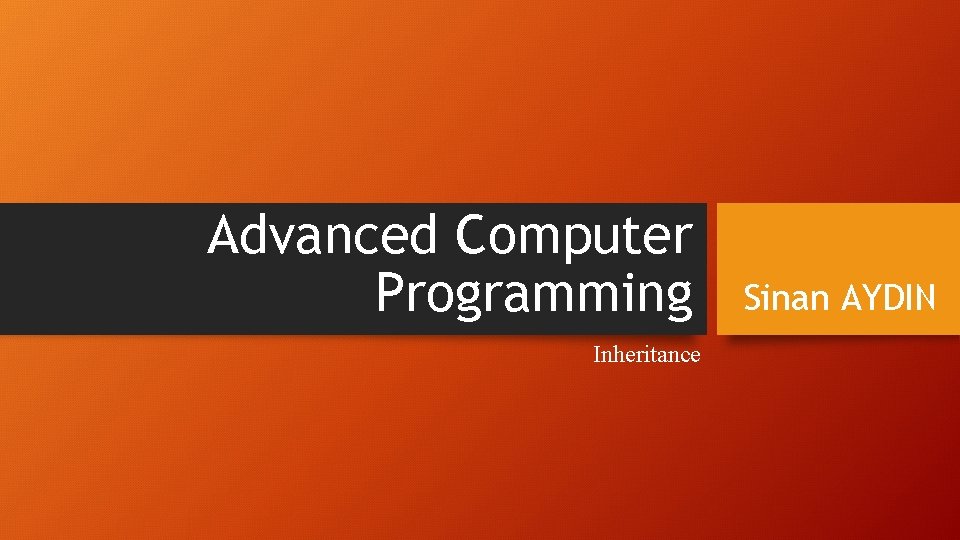
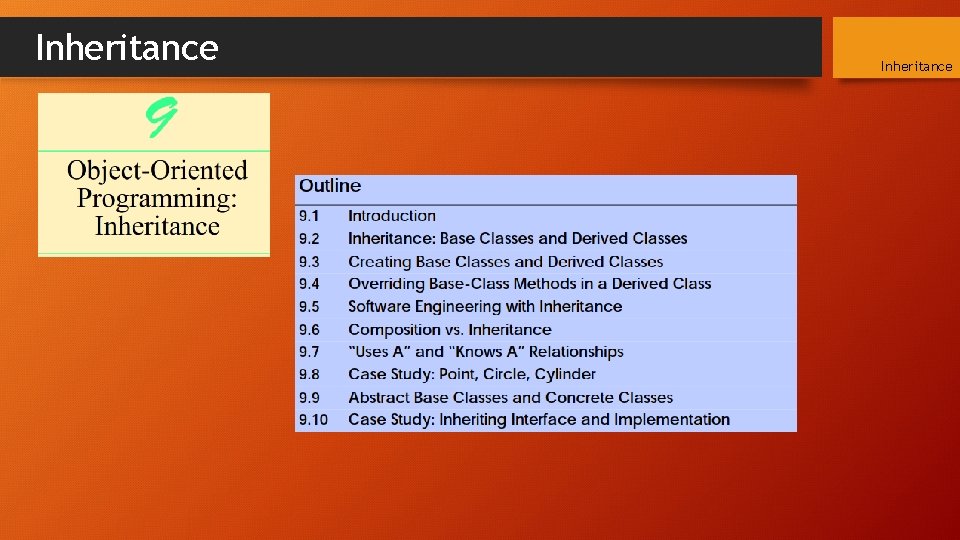
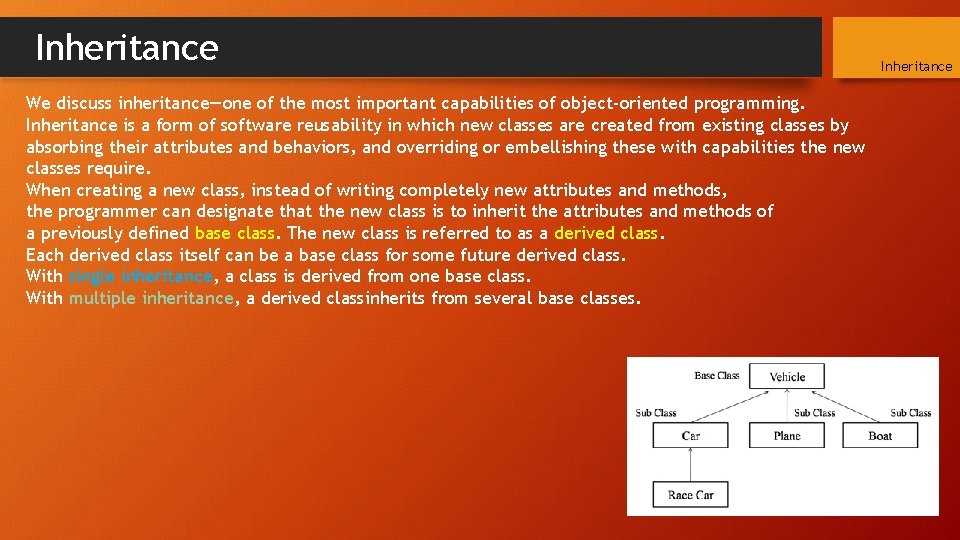
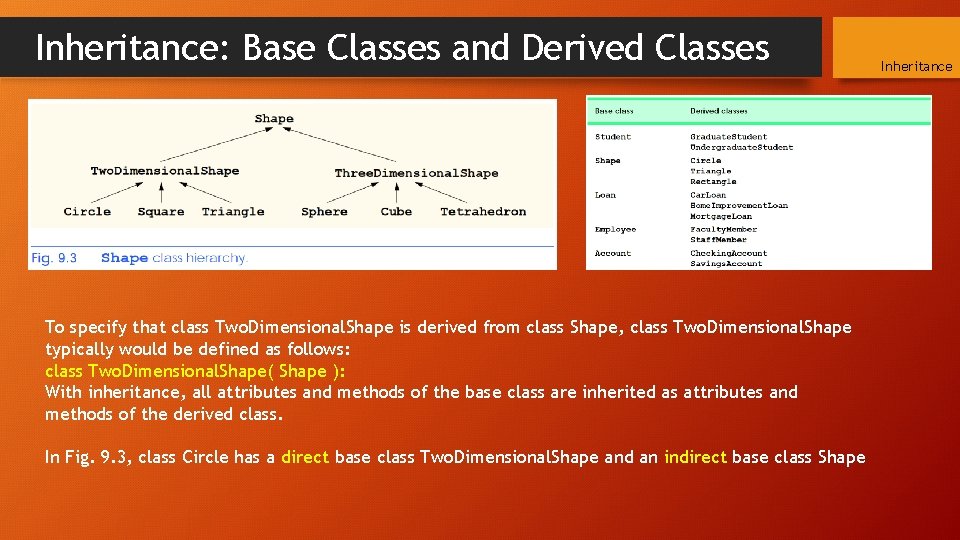
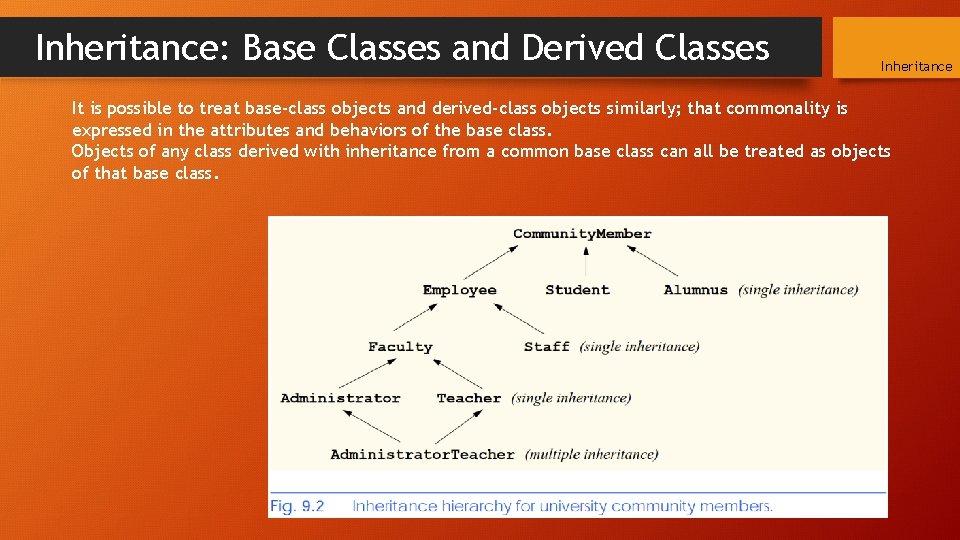
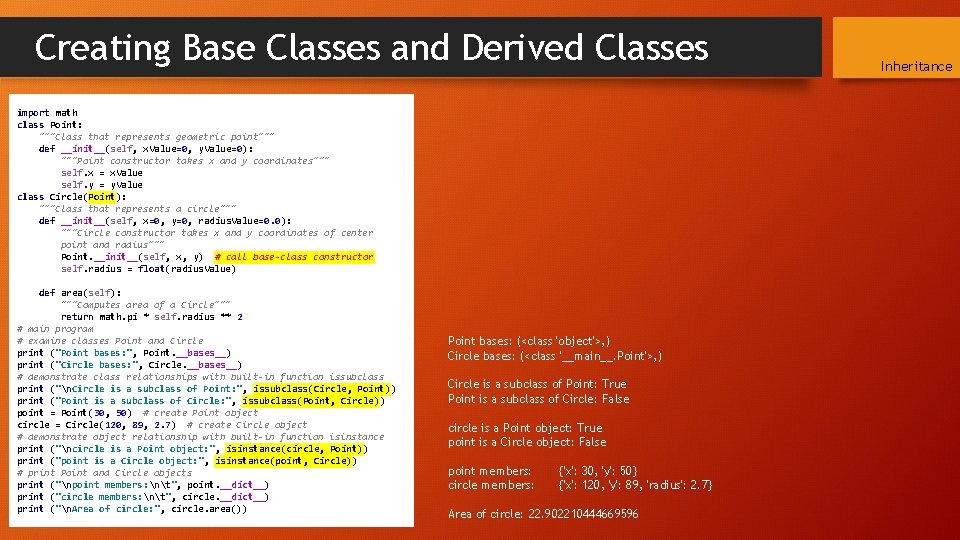
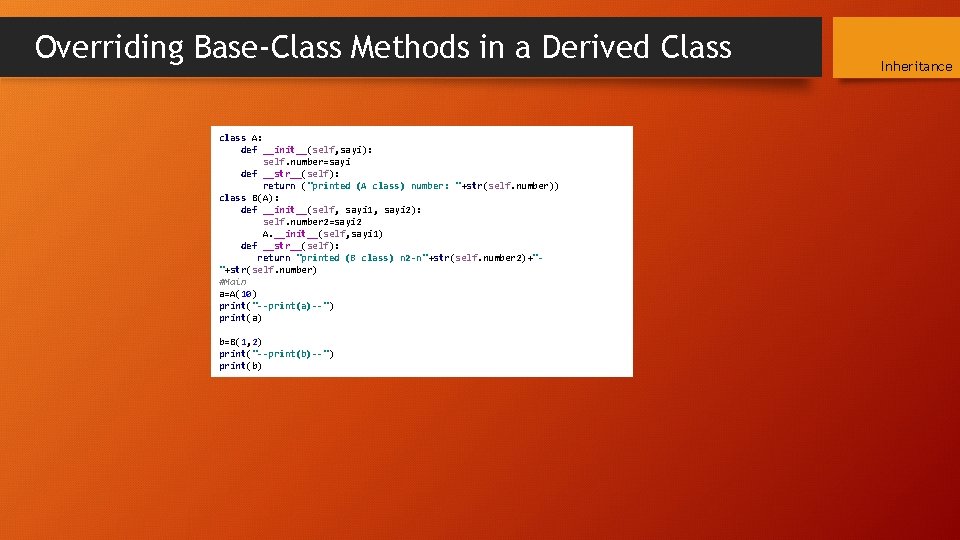
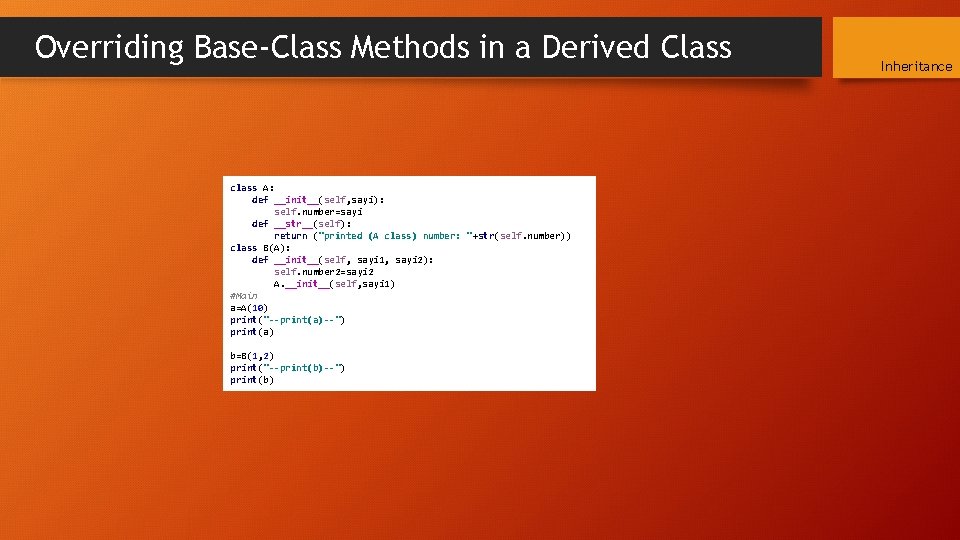
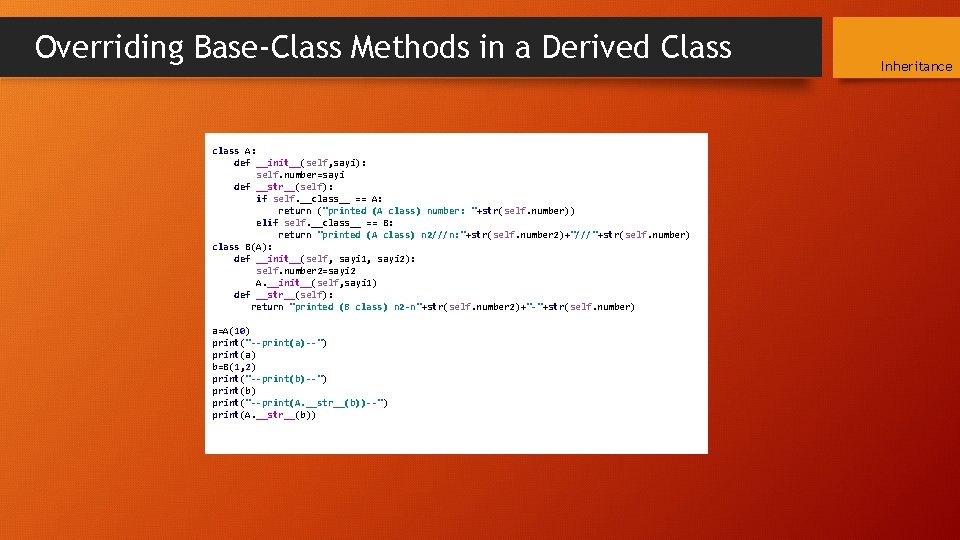
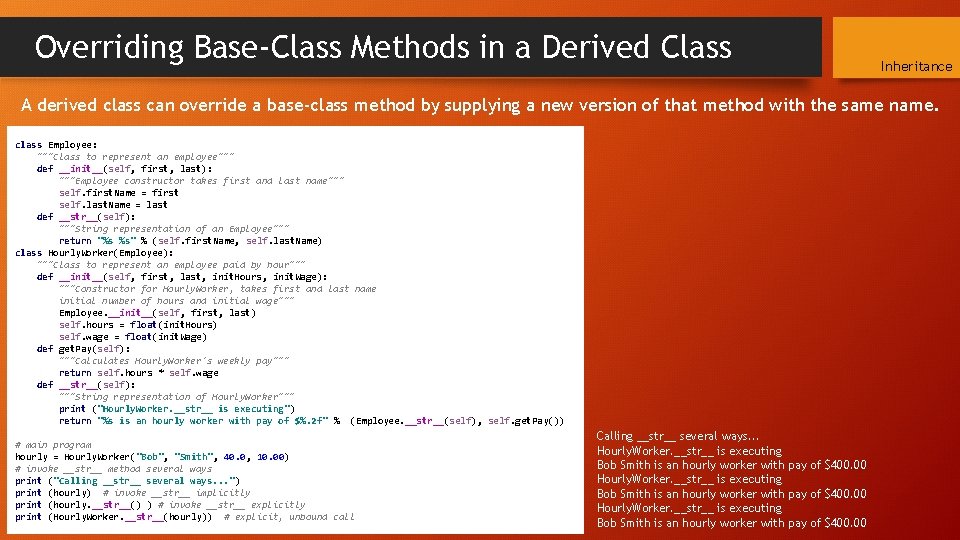
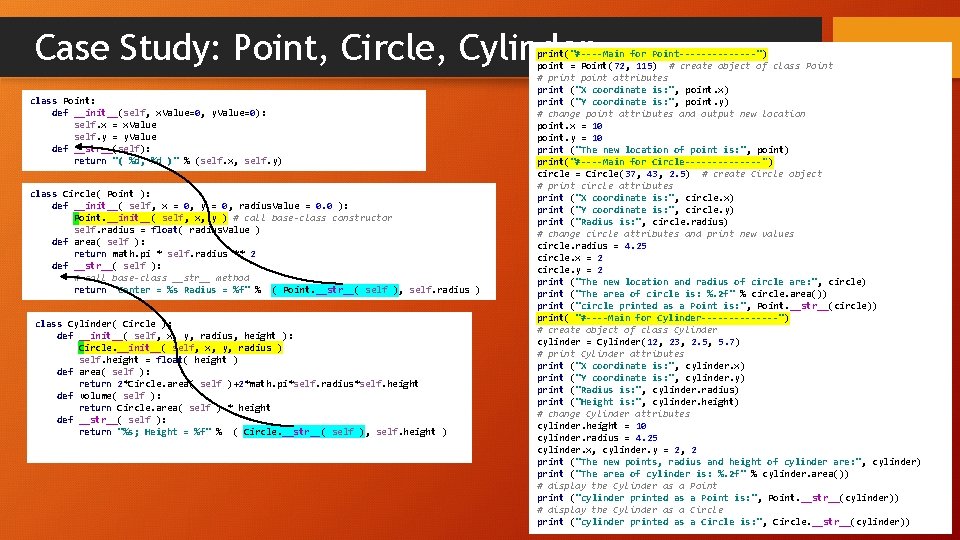
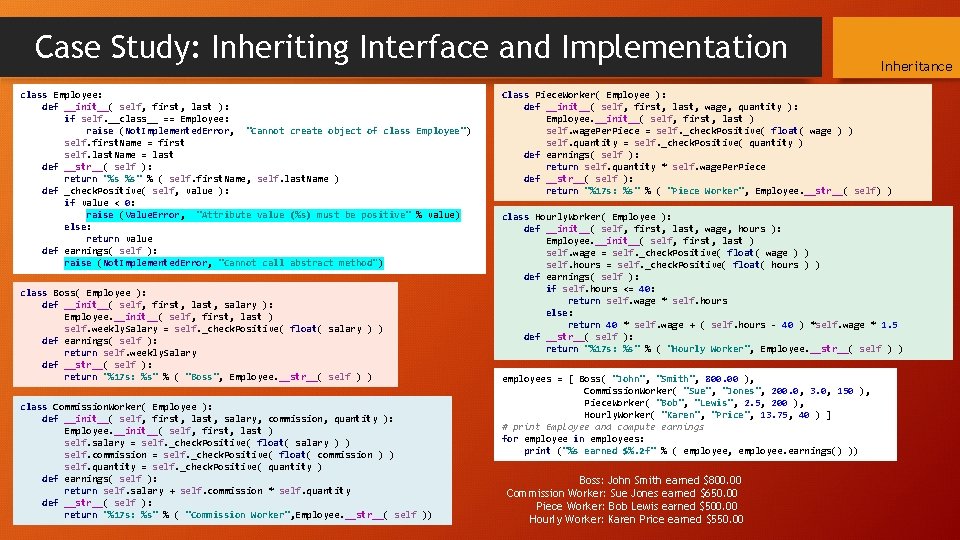
- Slides: 12
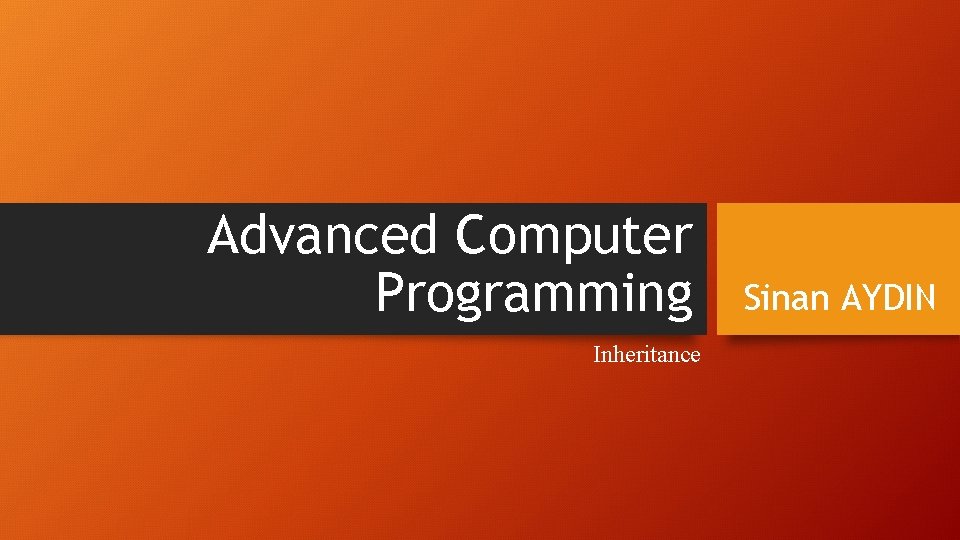
Advanced Computer Programming Inheritance Sinan AYDIN
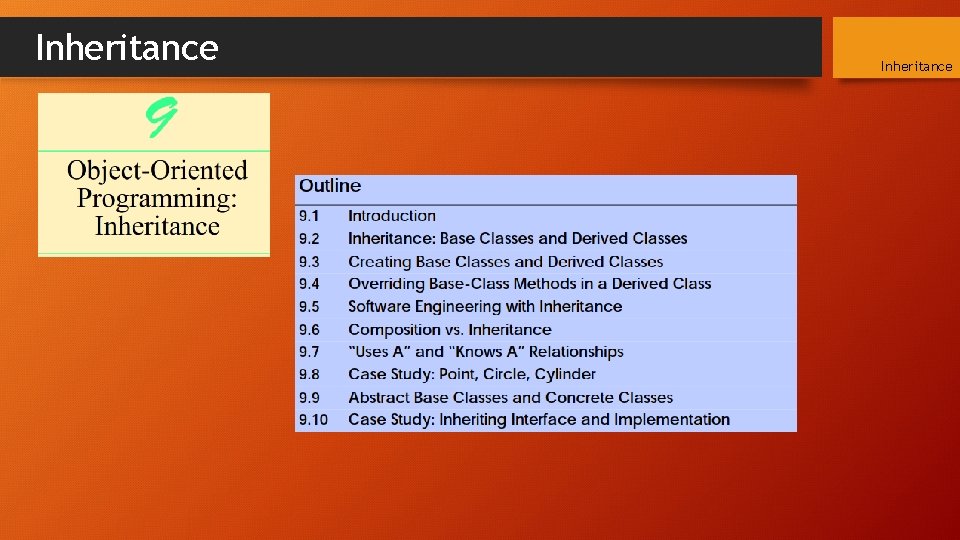
Inheritance
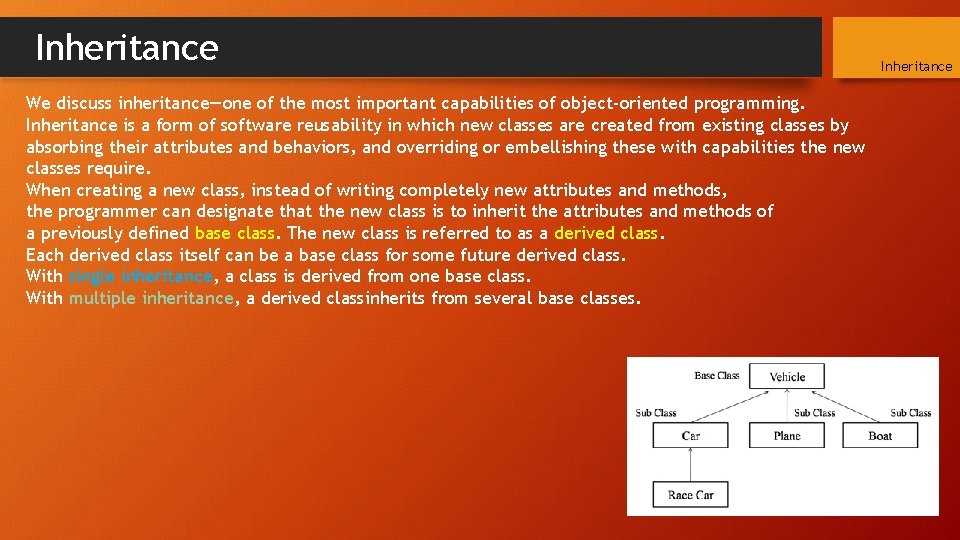
Inheritance We discuss inheritance—one of the most important capabilities of object-oriented programming. Inheritance is a form of software reusability in which new classes are created from existing classes by absorbing their attributes and behaviors, and overriding or embellishing these with capabilities the new classes require. When creating a new class, instead of writing completely new attributes and methods, the programmer can designate that the new class is to inherit the attributes and methods of a previously defined base class. The new class is referred to as a derived class. Each derived class itself can be a base class for some future derived class. With single inheritance, a class is derived from one base class. With multiple inheritance, a derived classinherits from several base classes. Inheritance
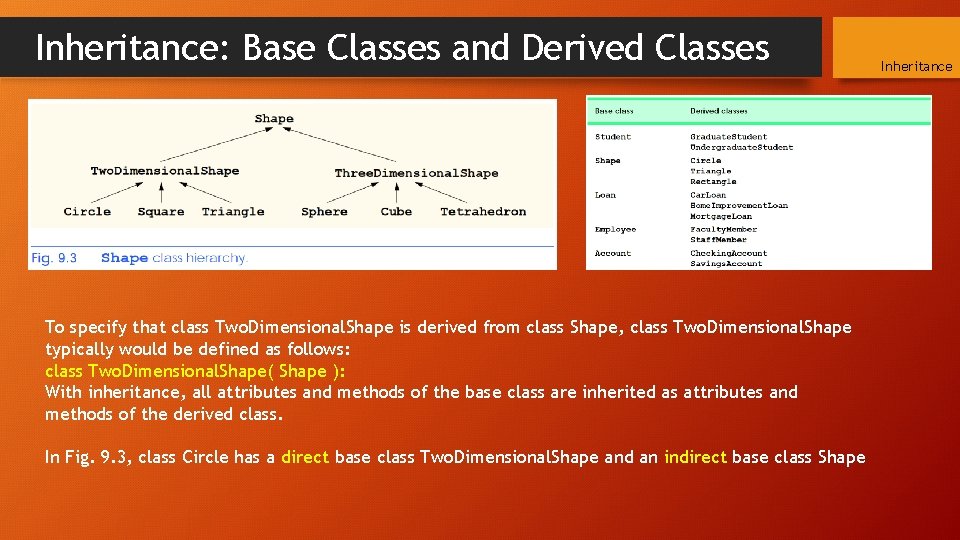
Inheritance: Base Classes and Derived Classes To specify that class Two. Dimensional. Shape is derived from class Shape, class Two. Dimensional. Shape typically would be defined as follows: class Two. Dimensional. Shape( Shape ): With inheritance, all attributes and methods of the base class are inherited as attributes and methods of the derived class. In Fig. 9. 3, class Circle has a direct base class Two. Dimensional. Shape and an indirect base class Shape Inheritance
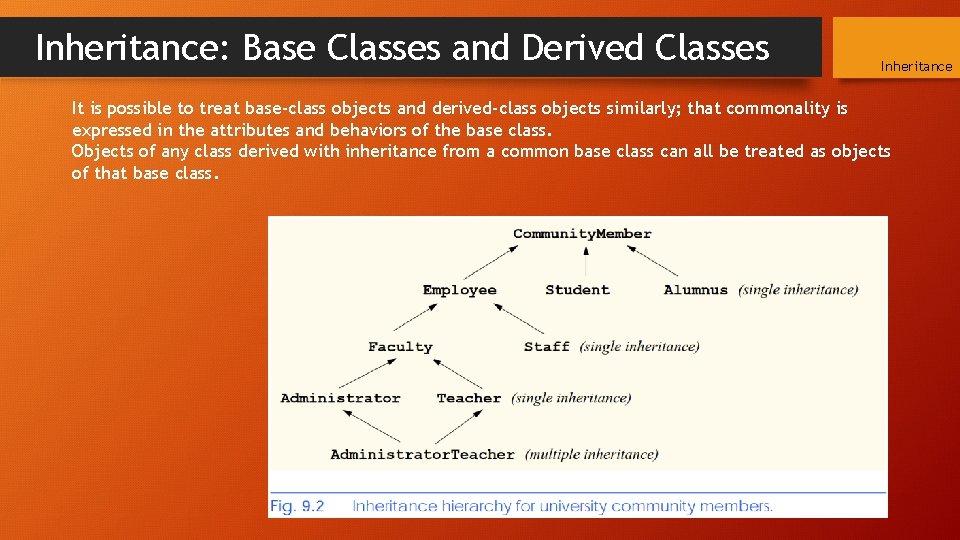
Inheritance: Base Classes and Derived Classes Inheritance It is possible to treat base-class objects and derived-class objects similarly; that commonality is expressed in the attributes and behaviors of the base class. Objects of any class derived with inheritance from a common base class can all be treated as objects of that base class.
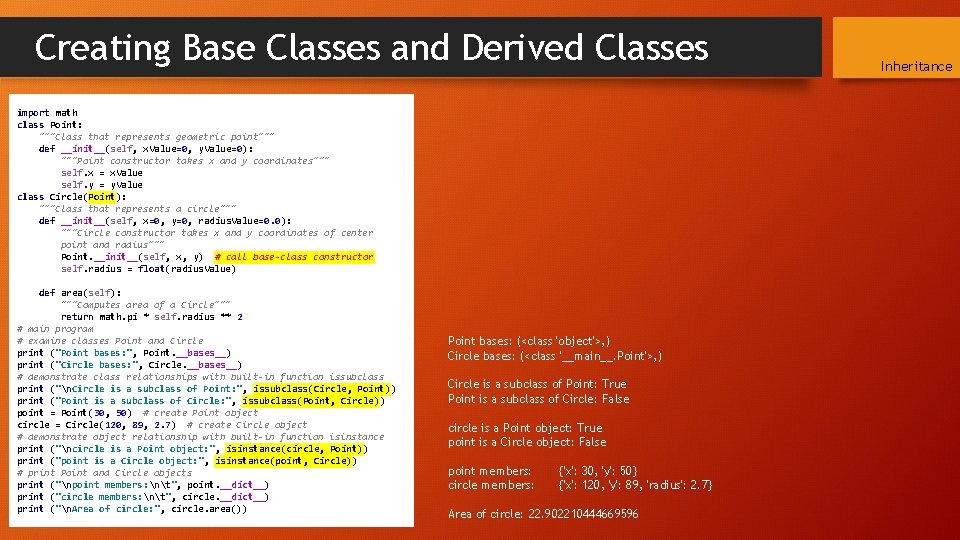
Creating Base Classes and Derived Classes import math class Point: """Class that represents geometric point""" def __init__(self, x. Value=0, y. Value=0): """Point constructor takes x and y coordinates""" self. x = x. Value self. y = y. Value class Circle(Point): """Class that represents a circle""" def __init__(self, x=0, y=0, radius. Value=0. 0): """Circle constructor takes x and y coordinates of center point and radius""" Point. __init__(self, x, y) # call base-class constructor self. radius = float(radius. Value) def area(self): """Computes area of a Circle""" return math. pi * self. radius ** 2 # main program # examine classes Point and Circle print ("Point bases: ", Point. __bases__) print ("Circle bases: ", Circle. __bases__) # demonstrate class relationships with built-in function issubclass print ("n. Circle is a subclass of Point: ", issubclass(Circle, Point)) print ("Point is a subclass of Circle: ", issubclass(Point, Circle)) point = Point(30, 50) # create Point object circle = Circle(120, 89, 2. 7) # create Circle object # demonstrate object relationship with built-in function isinstance print ("ncircle is a Point object: ", isinstance(circle, Point)) print ("point is a Circle object: ", isinstance(point, Circle)) # print Point and Circle objects print ("npoint members: nt", point. __dict__) print ("circle members: nt", circle. __dict__) print ("n. Area of circle: ", circle. area()) Point bases: (<class 'object'>, ) Circle bases: (<class '__main__. Point'>, ) Circle is a subclass of Point: True Point is a subclass of Circle: False circle is a Point object: True point is a Circle object: False point members: circle members: {'x': 30, 'y': 50} {'x': 120, 'y': 89, 'radius': 2. 7} Area of circle: 22. 902210444669596 Inheritance
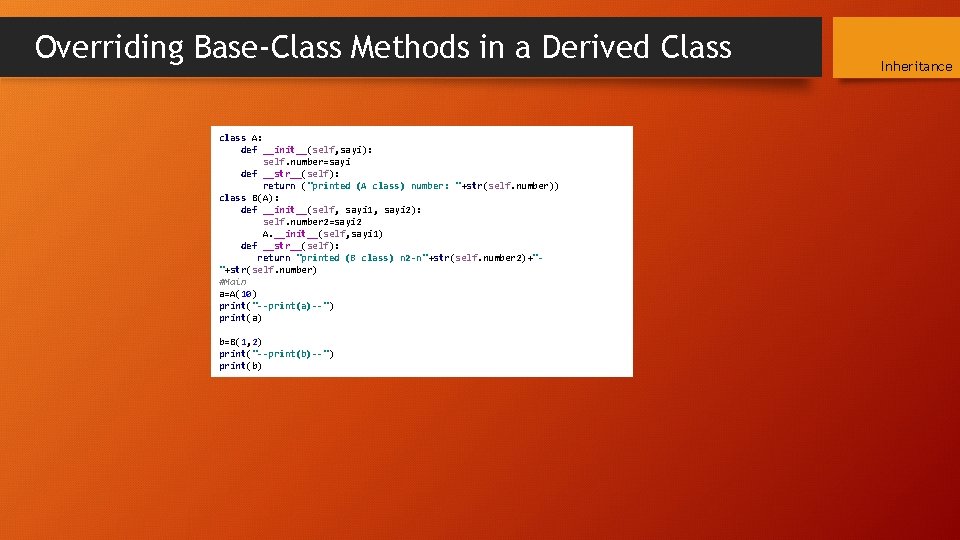
Overriding Base-Class Methods in a Derived Class class A: def __init__(self, sayi): self. number=sayi def __str__(self): return ("printed (A class) number: "+str(self. number)) class B(A): def __init__(self, sayi 1, sayi 2): self. number 2=sayi 2 A. __init__(self, sayi 1) def __str__(self): return "printed (B class) n 2 -n"+str(self. number 2)+""+str(self. number) #Main a=A(10) print("--print(a)--") print(a) b=B(1, 2) print("--print(b)--") print(b) Inheritance
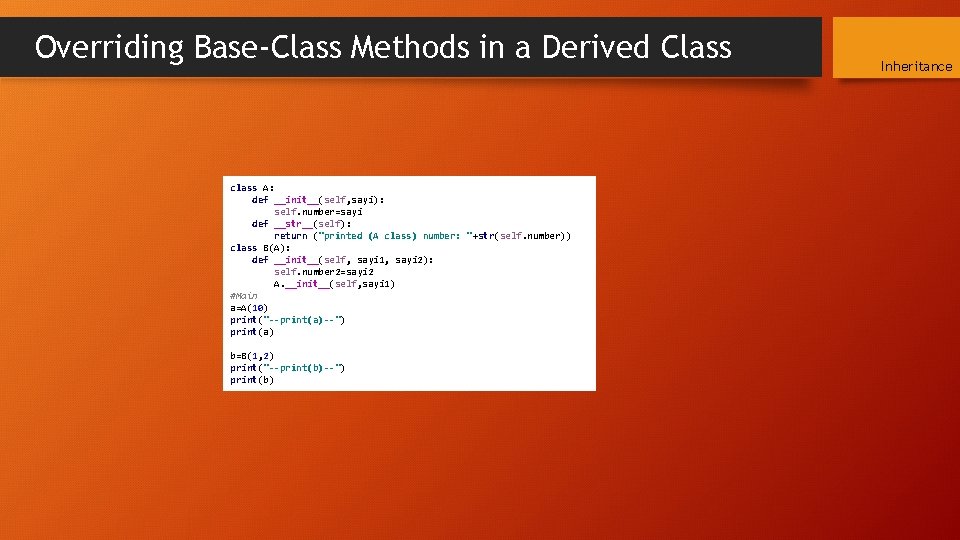
Overriding Base-Class Methods in a Derived Class class A: def __init__(self, sayi): self. number=sayi def __str__(self): return ("printed (A class) number: "+str(self. number)) class B(A): def __init__(self, sayi 1, sayi 2): self. number 2=sayi 2 A. __init__(self, sayi 1) #Main a=A(10) print("--print(a)--") print(a) b=B(1, 2) print("--print(b)--") print(b) Inheritance
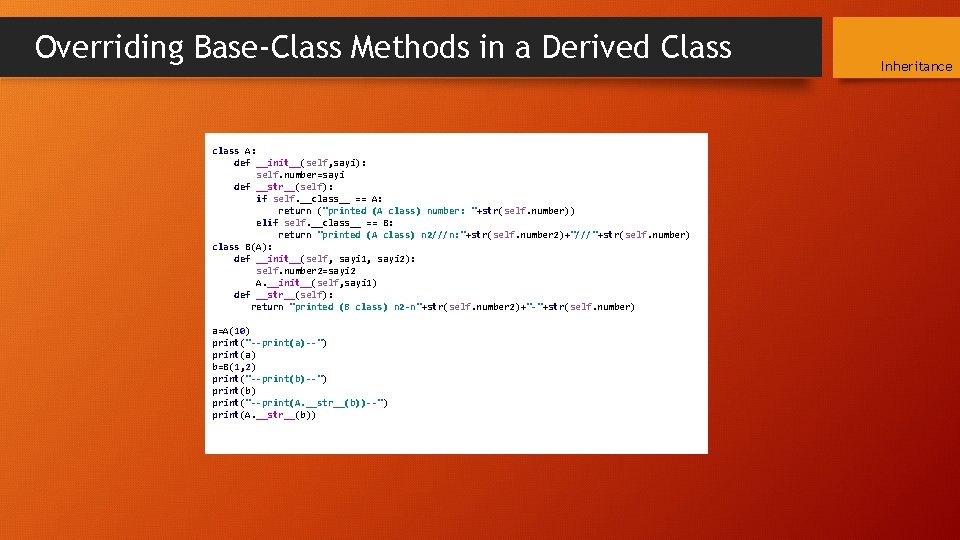
Overriding Base-Class Methods in a Derived Class class A: def __init__(self, sayi): self. number=sayi def __str__(self): if self. __class__ == A: return ("printed (A class) number: "+str(self. number)) elif self. __class__ == B: return "printed (A class) n 2///n: "+str(self. number 2)+"///"+str(self. number) class B(A): def __init__(self, sayi 1, sayi 2): self. number 2=sayi 2 A. __init__(self, sayi 1) def __str__(self): return "printed (B class) n 2 -n"+str(self. number 2)+"-"+str(self. number) a=A(10) print("--print(a)--") print(a) b=B(1, 2) print("--print(b)--") print(b) print("--print(A. __str__(b))--") print(A. __str__(b)) Inheritance
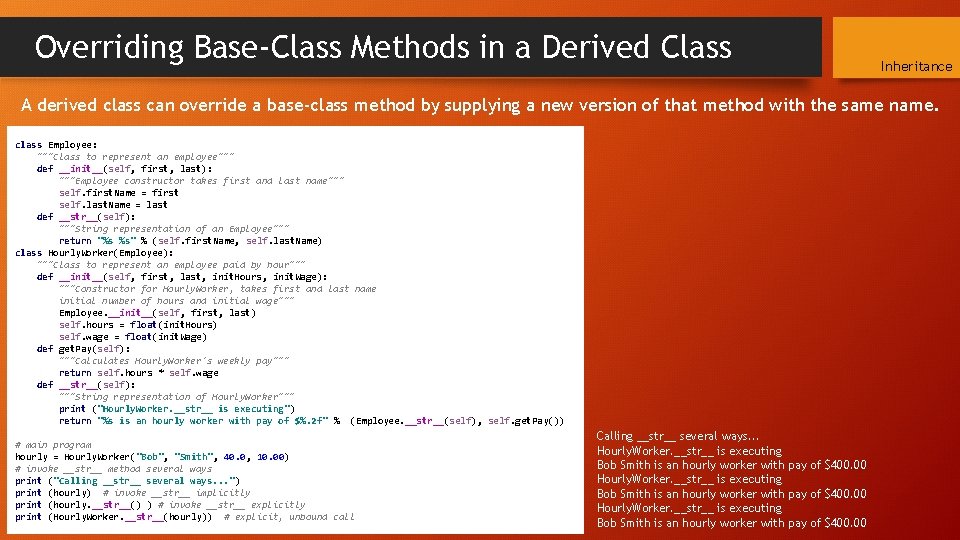
Overriding Base-Class Methods in a Derived Class Inheritance A derived class can override a base-class method by supplying a new version of that method with the same name. class Employee: """Class to represent an employee""" def __init__(self, first, last): """Employee constructor takes first and last name""" self. first. Name = first self. last. Name = last def __str__(self): """String representation of an Employee""" return "%s %s" % (self. first. Name, self. last. Name) class Hourly. Worker(Employee): """Class to represent an employee paid by hour""" def __init__(self, first, last, init. Hours, init. Wage): """Constructor for Hourly. Worker, takes first and last name initial number of hours and initial wage""" Employee. __init__(self, first, last) self. hours = float(init. Hours) self. wage = float(init. Wage) def get. Pay(self): """Calculates Hourly. Worker's weekly pay""" return self. hours * self. wage def __str__(self): """String representation of Hourly. Worker""" print ("Hourly. Worker. __str__ is executing") return "%s is an hourly worker with pay of $%. 2 f" % (Employee. __str__(self), self. get. Pay()) # main program hourly = Hourly. Worker("Bob", "Smith", 40. 0, 10. 00) # invoke __str__ method several ways print ("Calling __str__ several ways. . . ") print (hourly) # invoke __str__ implicitly print (hourly. __str__() ) # invoke __str__ explicitly print (Hourly. Worker. __str__(hourly)) # explicit, unbound call Calling __str__ several ways. . . Hourly. Worker. __str__ is executing Bob Smith is an hourly worker with pay of $400. 00
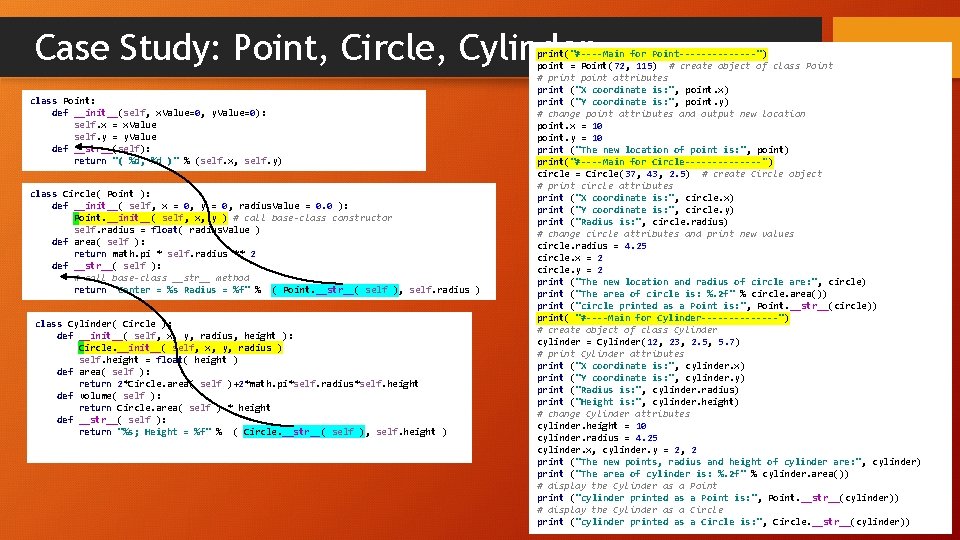
Case Study: Point, Circle, Cylinder class Point: def __init__(self, x. Value=0, y. Value=0): self. x = x. Value self. y = y. Value def __str__(self): return "( %d, %d )" % (self. x, self. y) class Circle( Point ): def __init__( self, x = 0, y = 0, radius. Value = 0. 0 ): Point. __init__( self, x, y ) # call base-class constructor self. radius = float( radius. Value ) def area( self ): return math. pi * self. radius ** 2 def __str__( self ): # call base-class __str__ method return "Center = %s Radius = %f" % ( Point. __str__( self ), self. radius ) class Cylinder( Circle ): def __init__( self, x, y, radius, height ): Circle. __init__( self, x, y, radius ) self. height = float( height ) def area( self ): return 2*Circle. area( self )+2*math. pi*self. radius*self. height def volume( self ): return Circle. area( self ) * height def __str__( self ): return "%s; Height = %f" % ( Circle. __str__( self ), self. height ) print("#----Main for Point-------") point = Point(72, 115) # create object of class Point Inheritance # print point attributes print ("X coordinate is: ", point. x) print ("Y coordinate is: ", point. y) # change point attributes and output new location point. x = 10 point. y = 10 print ("The new location of point is: ", point) print("#----Main for Circle-------") circle = Circle(37, 43, 2. 5) # create Circle object # print circle attributes print ("X coordinate is: ", circle. x) print ("Y coordinate is: ", circle. y) print ("Radius is: ", circle. radius) # change circle attributes and print new values circle. radius = 4. 25 circle. x = 2 circle. y = 2 print ("The new location and radius of circle are: ", circle) print ("The area of circle is: %. 2 f" % circle. area()) print ("circle printed as a Point is: ", Point. __str__(circle)) print( "#----Main for Cylinder-------") # create object of class Cylinder cylinder = Cylinder(12, 23, 2. 5, 5. 7) # print Cylinder attributes print ("X coordinate is: ", cylinder. x) print ("Y coordinate is: ", cylinder. y) print ("Radius is: ", cylinder. radius) print ("Height is: ", cylinder. height) # change Cylinder attributes cylinder. height = 10 cylinder. radius = 4. 25 cylinder. x, cylinder. y = 2, 2 print ("The new points, radius and height of cylinder are: ", cylinder) print ("The area of cylinder is: %. 2 f" % cylinder. area()) # display the Cylinder as a Point print ("cylinder printed as a Point is: ", Point. __str__(cylinder)) # display the Cylinder as a Circle print ("cylinder printed as a Circle is: ", Circle. __str__(cylinder))
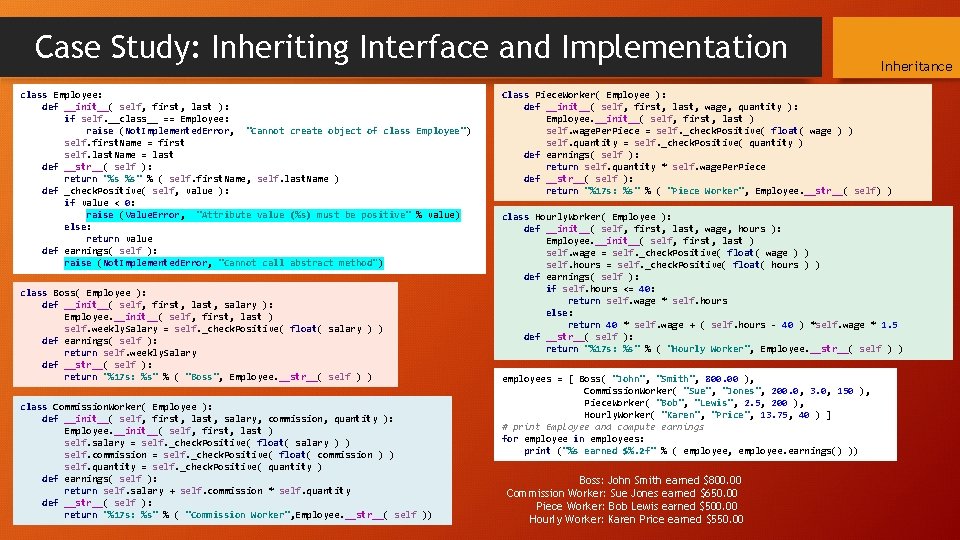
Case Study: Inheriting Interface and Implementation class Employee: def __init__( self, first, last ): if self. __class__ == Employee: raise (Not. Implemented. Error, "Cannot create object of class Employee") self. first. Name = first self. last. Name = last def __str__( self ): return "%s %s" % ( self. first. Name, self. last. Name ) def _check. Positive( self, value ): if value < 0: raise (Value. Error, "Attribute value (%s) must be positive" % value) else: return value def earnings( self ): raise (Not. Implemented. Error, "Cannot call abstract method") class Boss( Employee ): def __init__( self, first, last, salary ): Employee. __init__( self, first, last ) self. weekly. Salary = self. _check. Positive( float( salary ) ) def earnings( self ): return self. weekly. Salary def __str__( self ): return "%17 s: %s" % ( "Boss", Employee. __str__( self ) ) class Commission. Worker( Employee ): def __init__( self, first, last, salary, commission, quantity ): Employee. __init__( self, first, last ) self. salary = self. _check. Positive( float( salary ) ) self. commission = self. _check. Positive( float( commission ) ) self. quantity = self. _check. Positive( quantity ) def earnings( self ): return self. salary + self. commission * self. quantity def __str__( self ): return "%17 s: %s" % ( "Commission Worker", Employee. __str__( self )) Inheritance Class Piece. Worker( Employee ): def __init__( self, first, last, wage, quantity ): Employee. __init__( self, first, last ) self. wage. Per. Piece = self. _check. Positive( float( wage ) ) self. quantity = self. _check. Positive( quantity ) def earnings( self ): return self. quantity * self. wage. Per. Piece def __str__( self ): return "%17 s: %s" % ( "Piece Worker", Employee. __str__( self) ) class Hourly. Worker( Employee ): def __init__( self, first, last, wage, hours ): Employee. __init__( self, first, last ) self. wage = self. _check. Positive( float( wage ) ) self. hours = self. _check. Positive( float( hours ) ) def earnings( self ): if self. hours <= 40: return self. wage * self. hours else: return 40 * self. wage + ( self. hours - 40 ) *self. wage * 1. 5 def __str__( self ): return "%17 s: %s" % ( "Hourly Worker", Employee. __str__( self ) ) employees = [ Boss( "John", "Smith", 800. 00 ), Commission. Worker( "Sue", "Jones", 200. 0, 3. 0, 150 ), Piece. Worker( "Bob", "Lewis", 2. 5, 200 ), Hourly. Worker( "Karen", "Price", 13. 75, 40 ) ] # print Employee and compute earnings for employee in employees: print ("%s earned $%. 2 f" % ( employee, employee. earnings() )) Boss: John Smith earned $800. 00 Commission Worker: Sue Jones earned $650. 00 Piece Worker: Bob Lewis earned $500. 00 Hourly Worker: Karen Price earned $550. 00