OneDimensional Array r A onedimensional array is a
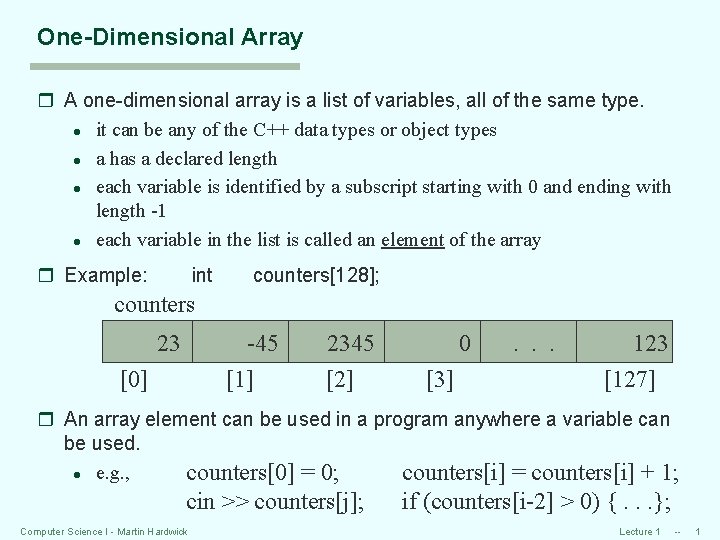
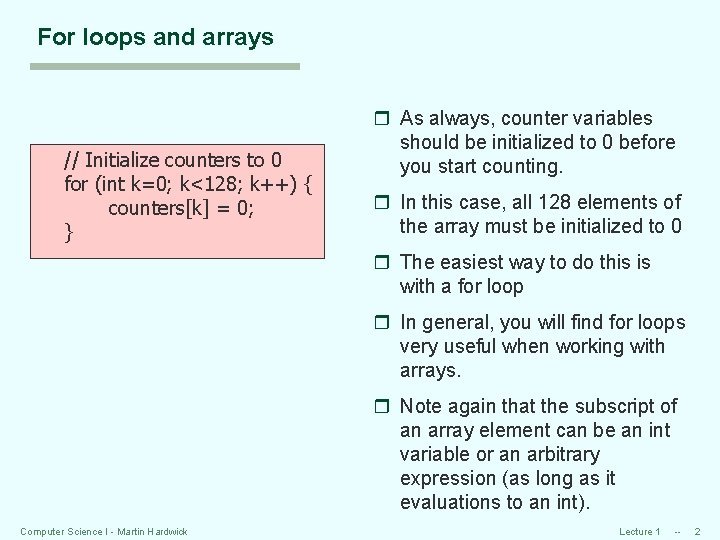
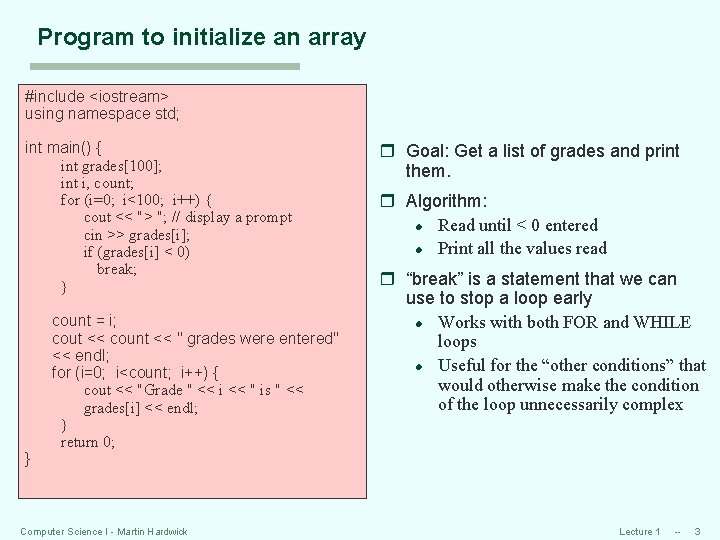
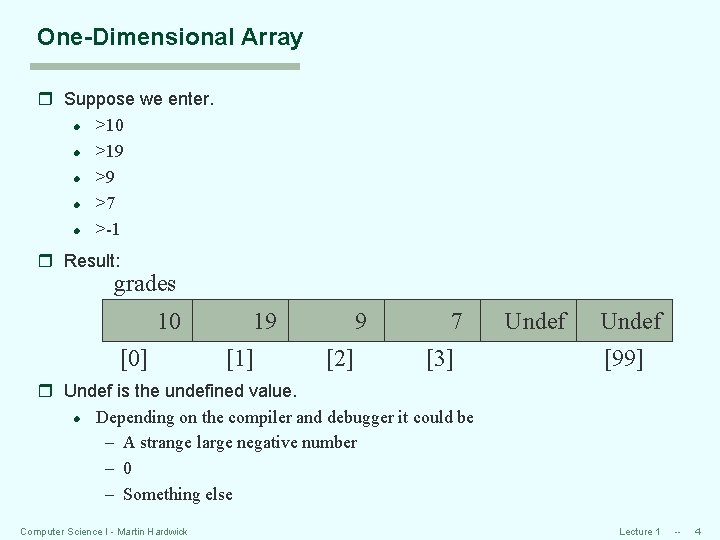
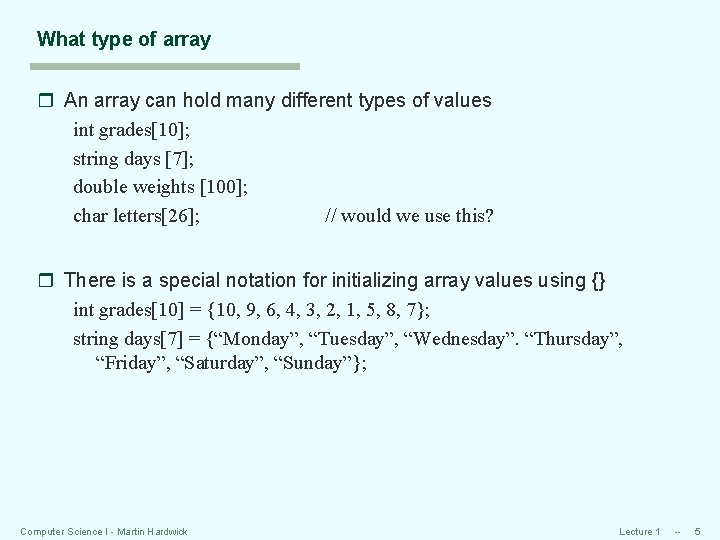
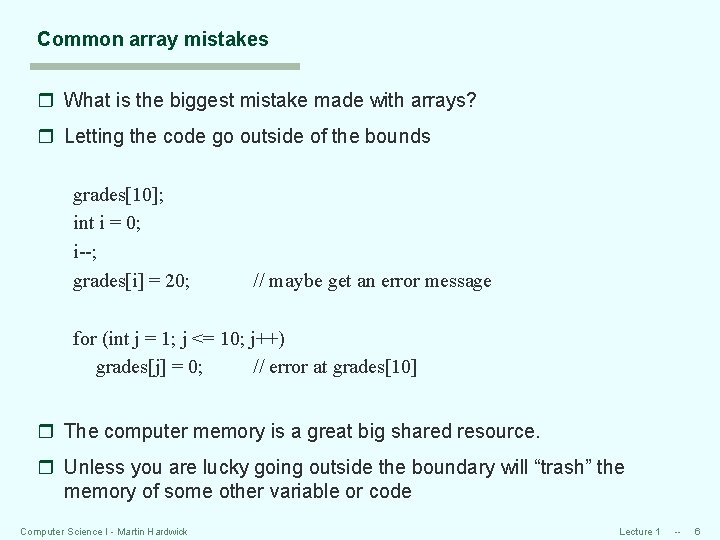
![Computer Main Memory (from lecture 1) If grade[0] starts here . . . 0100101100110101100111000001. Computer Main Memory (from lecture 1) If grade[0] starts here . . . 0100101100110101100111000001.](https://slidetodoc.com/presentation_image_h2/2359916402eae9dfb1cb57b83121874c/image-7.jpg)
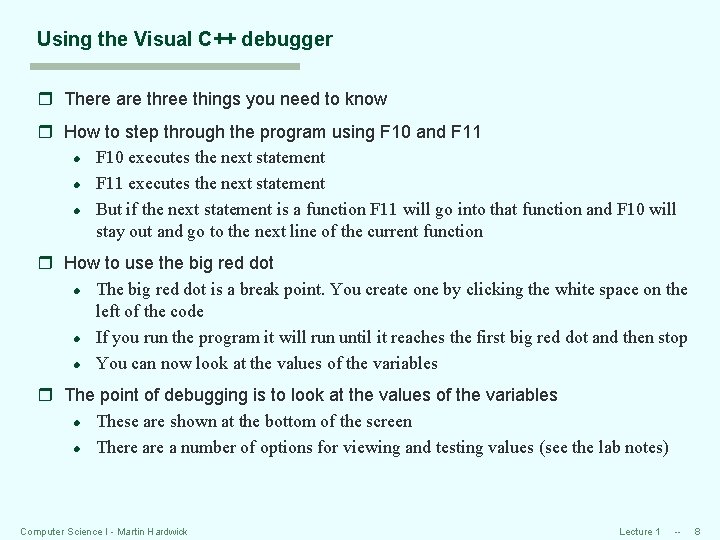
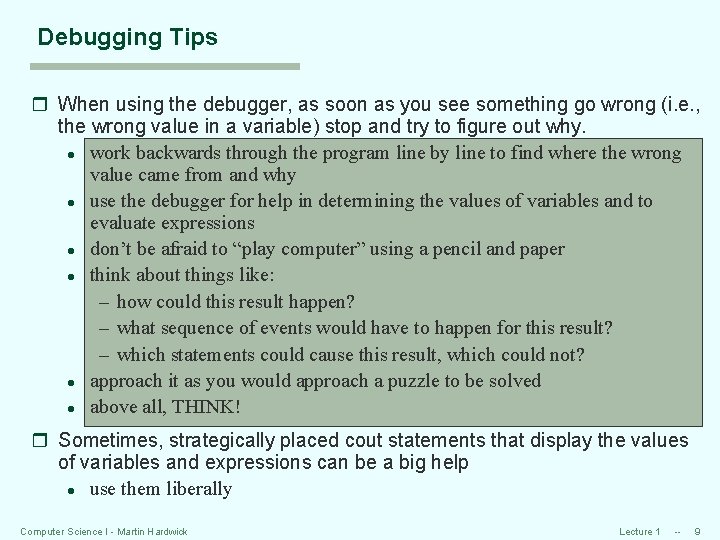
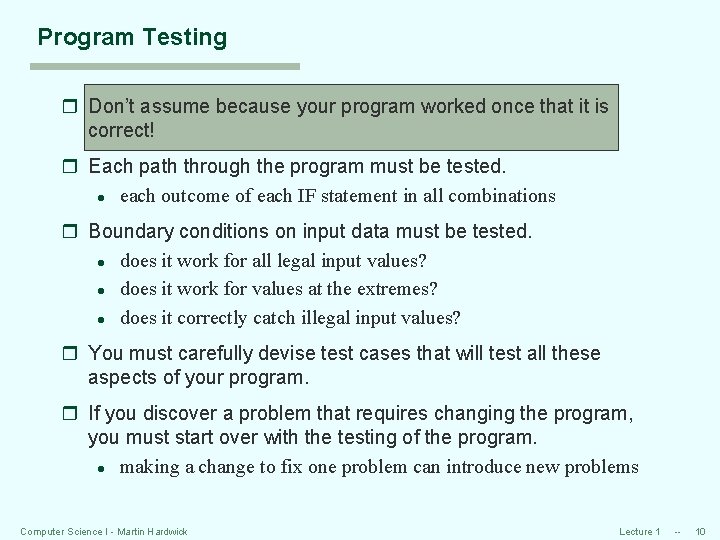
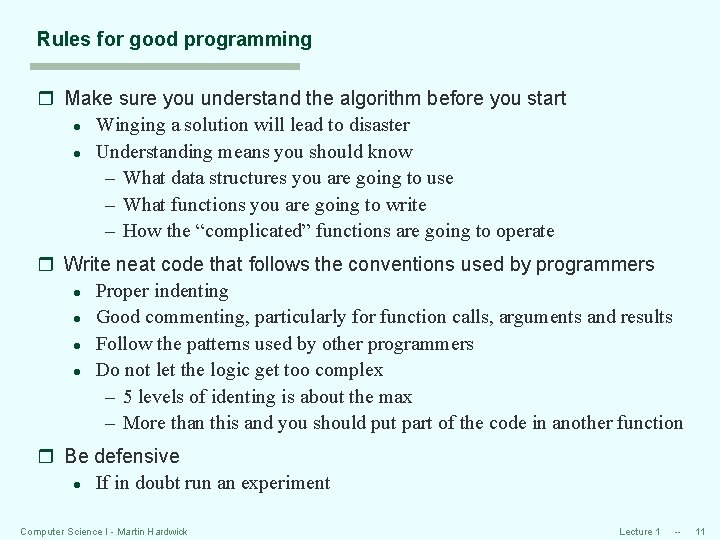
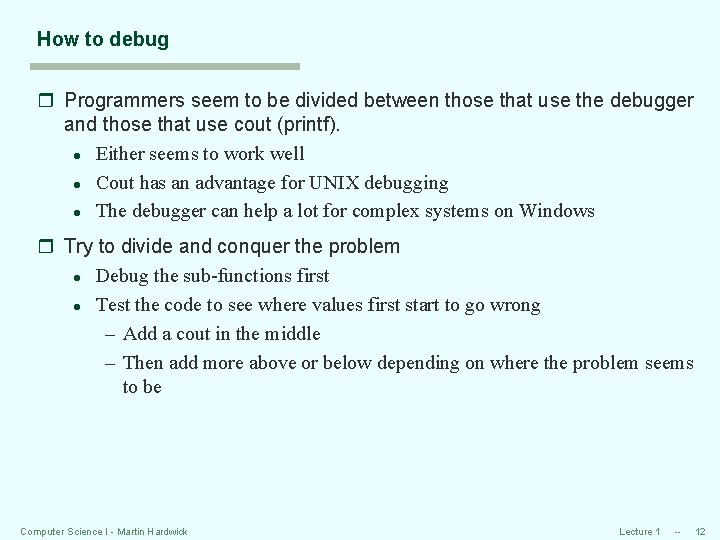
- Slides: 12
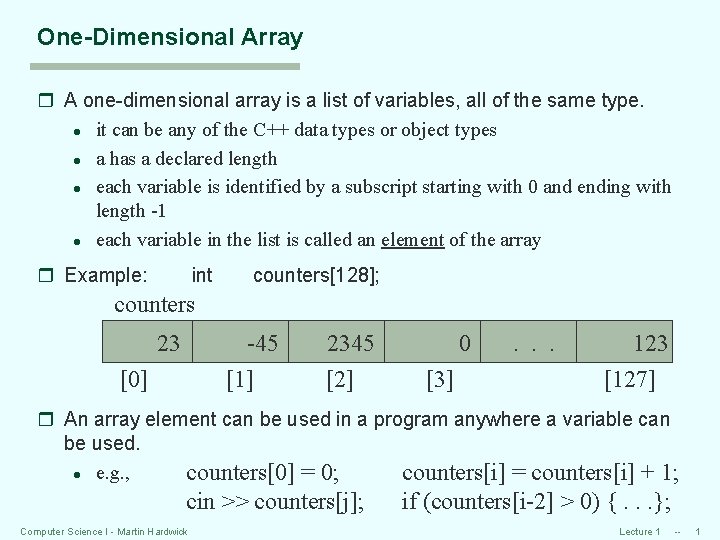
One-Dimensional Array r A one-dimensional array is a list of variables, all of the same type. l it can be any of the C++ data types or object types l a has a declared length l each variable is identified by a subscript starting with 0 and ending with length -1 l each variable in the list is called an element of the array r Example: int counters[128]; counters 23 -45 [0] [1] 2345 [2] 0 [3] . . . 123 [127] r An array element can be used in a program anywhere a variable can be used. l e. g. , counters[0] = 0; counters[i] = counters[i] + 1; cin >> counters[j]; Computer Science I - Martin Hardwick if (counters[i-2] > 0) {. . . }; Lecture 1 -- 1
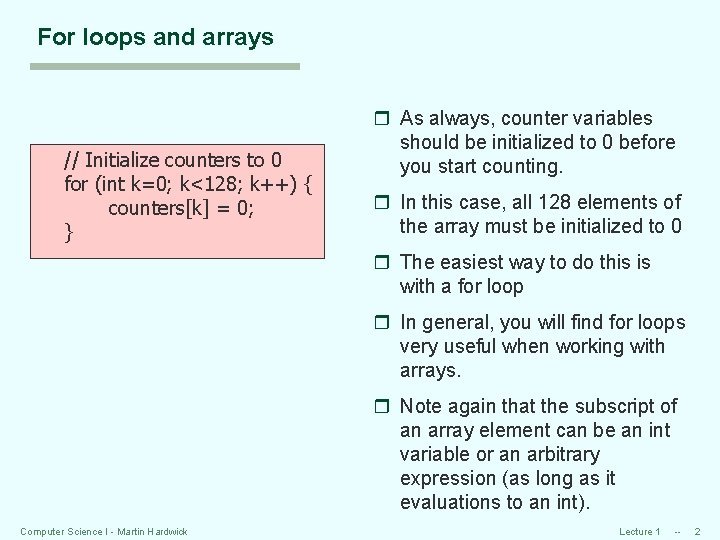
For loops and arrays // Initialize counters to 0 for (int k=0; k<128; k++) { counters[k] = 0; } r As always, counter variables should be initialized to 0 before you start counting. r In this case, all 128 elements of the array must be initialized to 0 r The easiest way to do this is with a for loop r In general, you will find for loops very useful when working with arrays. r Note again that the subscript of an array element can be an int variable or an arbitrary expression (as long as it evaluations to an int). Computer Science I - Martin Hardwick Lecture 1 -- 2
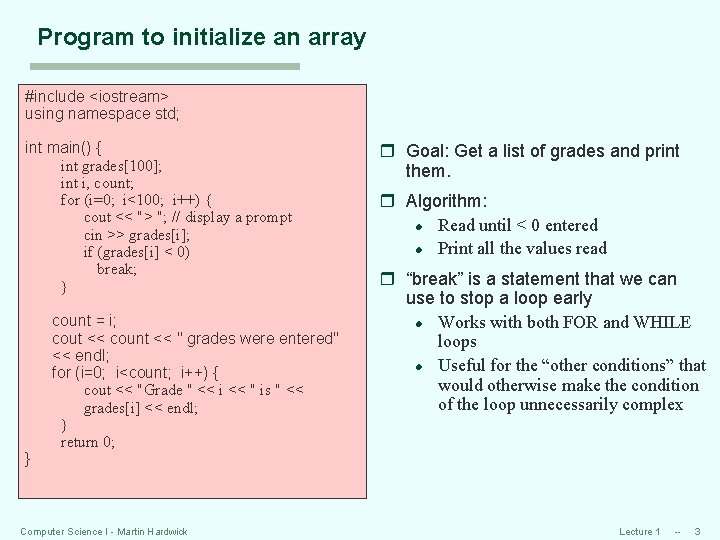
Program to initialize an array #include <iostream> using namespace std; int main() { int grades[100]; int i, count; for (i=0; i<100; i++) { cout << "> "; // display a prompt cin >> grades[i]; if (grades[i] < 0) break; } } count = i; cout << count << " grades were entered" << endl; for (i=0; i<count; i++) { cout << "Grade " << i << " is " << grades[i] << endl; } return 0; Computer Science I - Martin Hardwick r Goal: Get a list of grades and print them. r Algorithm: l Read until < 0 entered l Print all the values read r “break” is a statement that we can use to stop a loop early l Works with both FOR and WHILE loops l Useful for the “other conditions” that would otherwise make the condition of the loop unnecessarily complex Lecture 1 -- 3
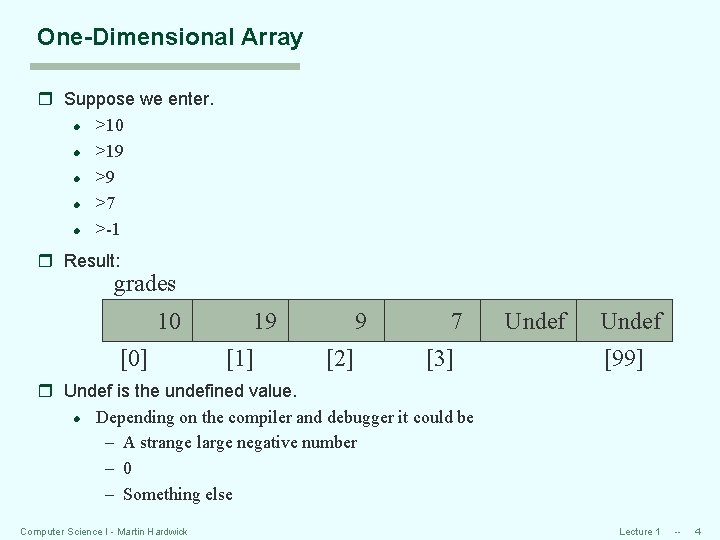
One-Dimensional Array r Suppose we enter. l >10 l >19 l >7 l >-1 r Result: grades 10 [0] 19 [1] 9 [2] 7 [3] Undef [99] r Undef is the undefined value. l Depending on the compiler and debugger it could be – A strange large negative number – 0 – Something else Computer Science I - Martin Hardwick Lecture 1 -- 4
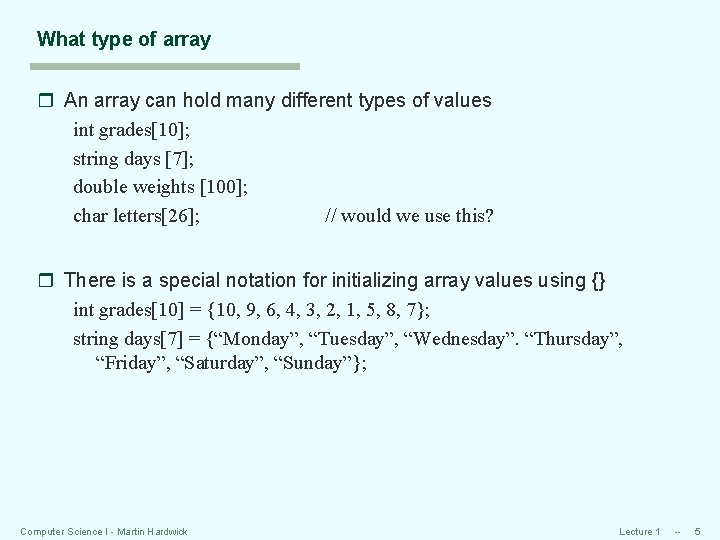
What type of array r An array can hold many different types of values int grades[10]; string days [7]; double weights [100]; char letters[26]; // would we use this? r There is a special notation for initializing array values using {} int grades[10] = {10, 9, 6, 4, 3, 2, 1, 5, 8, 7}; string days[7] = {“Monday”, “Tuesday”, “Wednesday”. “Thursday”, “Friday”, “Saturday”, “Sunday”}; Computer Science I - Martin Hardwick Lecture 1 -- 5
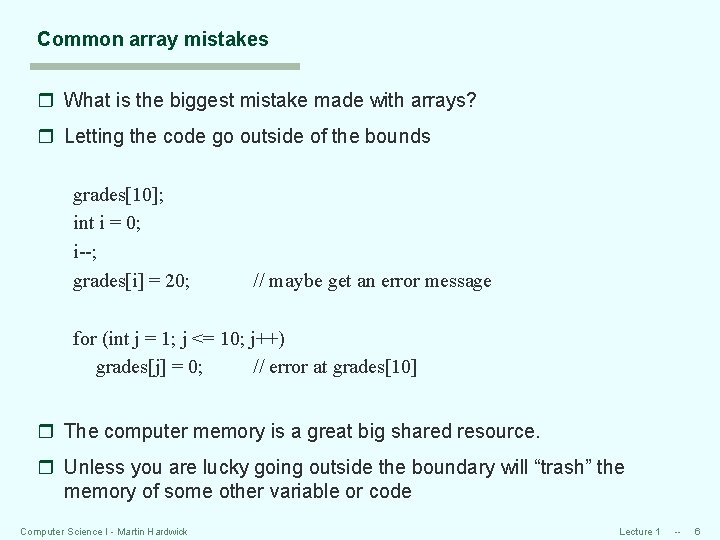
Common array mistakes r What is the biggest mistake made with arrays? r Letting the code go outside of the bounds grades[10]; int i = 0; i--; grades[i] = 20; // maybe get an error message for (int j = 1; j <= 10; j++) grades[j] = 0; // error at grades[10] r The computer memory is a great big shared resource. r Unless you are lucky going outside the boundary will “trash” the memory of some other variable or code Computer Science I - Martin Hardwick Lecture 1 -- 6
![Computer Main Memory from lecture 1 If grade0 starts here 0100101100110101100111000001 Computer Main Memory (from lecture 1) If grade[0] starts here . . . 0100101100110101100111000001.](https://slidetodoc.com/presentation_image_h2/2359916402eae9dfb1cb57b83121874c/image-7.jpg)
Computer Main Memory (from lecture 1) If grade[0] starts here . . . 0100101100110101100111000001. . . 234 E 16 234 F 16 235016 235116 Then “grade[-1] = 6” will trash four bytes here and If you go a long way outside the bounds e. g grade[-20728291] then you will reach the edge of the memory allowed for your program and there will be a run time error. Computer Science I - Martin Hardwick Lecture 1 -- 7
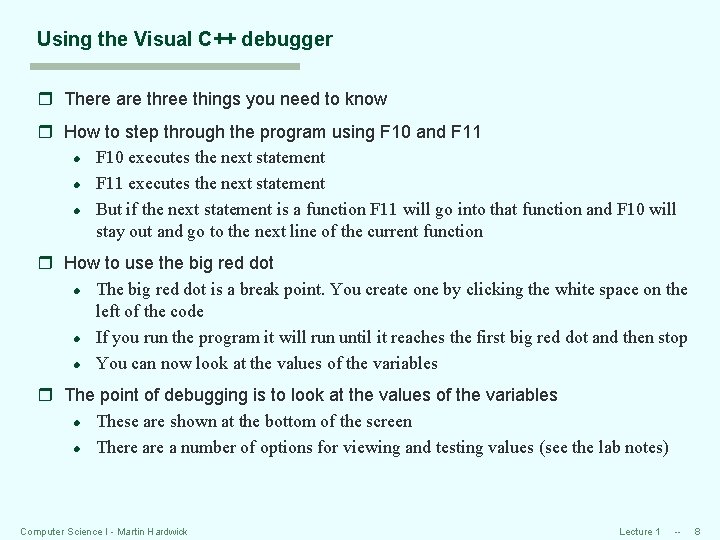
Using the Visual C++ debugger r There are three things you need to know r How to step through the program using F 10 and F 11 l F 10 executes the next statement l F 11 executes the next statement l But if the next statement is a function F 11 will go into that function and F 10 will stay out and go to the next line of the current function r How to use the big red dot l The big red dot is a break point. You create one by clicking the white space on the left of the code l If you run the program it will run until it reaches the first big red dot and then stop l You can now look at the values of the variables r The point of debugging is to look at the values of the variables l These are shown at the bottom of the screen l There a number of options for viewing and testing values (see the lab notes) Computer Science I - Martin Hardwick Lecture 1 -- 8
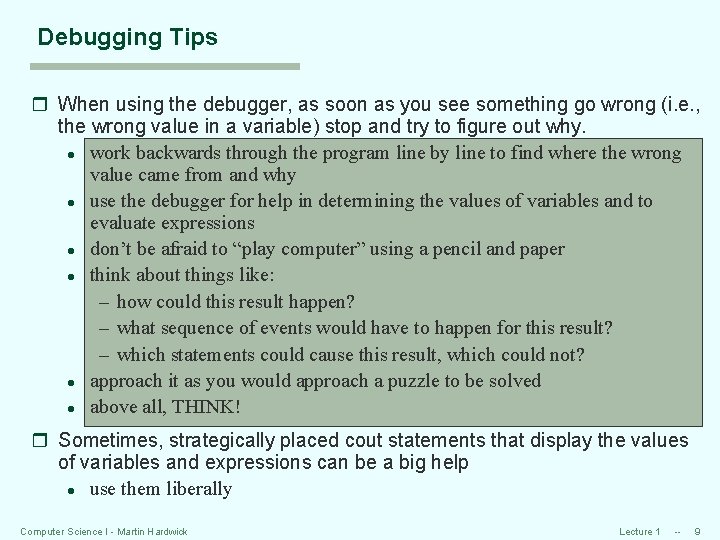
Debugging Tips r When using the debugger, as soon as you see something go wrong (i. e. , the wrong value in a variable) stop and try to figure out why. l work backwards through the program line by line to find where the wrong value came from and why l use the debugger for help in determining the values of variables and to evaluate expressions l don’t be afraid to “play computer” using a pencil and paper l think about things like: – how could this result happen? – what sequence of events would have to happen for this result? – which statements could cause this result, which could not? l approach it as you would approach a puzzle to be solved l above all, THINK! r Sometimes, strategically placed cout statements that display the values of variables and expressions can be a big help l use them liberally Computer Science I - Martin Hardwick Lecture 1 -- 9
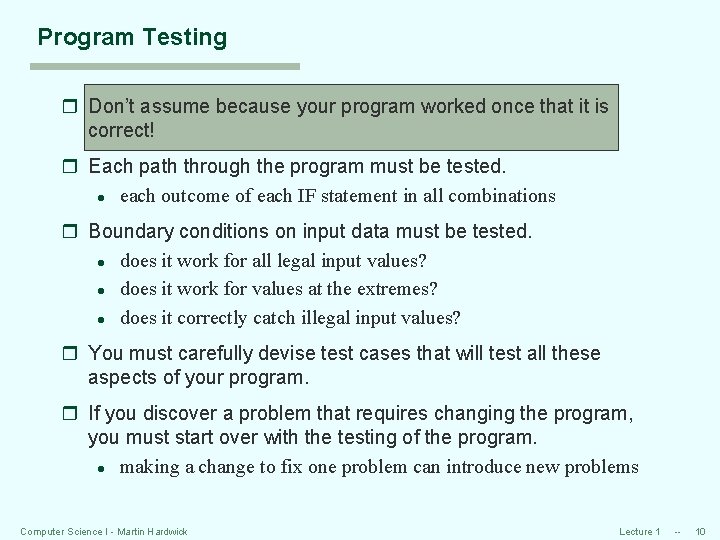
Program Testing r Don’t assume because your program worked once that it is correct! r Each path through the program must be tested. l each outcome of each IF statement in all combinations r Boundary conditions on input data must be tested. l does it work for all legal input values? l does it work for values at the extremes? l does it correctly catch illegal input values? r You must carefully devise test cases that will test all these aspects of your program. r If you discover a problem that requires changing the program, you must start over with the testing of the program. l making a change to fix one problem can introduce new problems Computer Science I - Martin Hardwick Lecture 1 -- 10
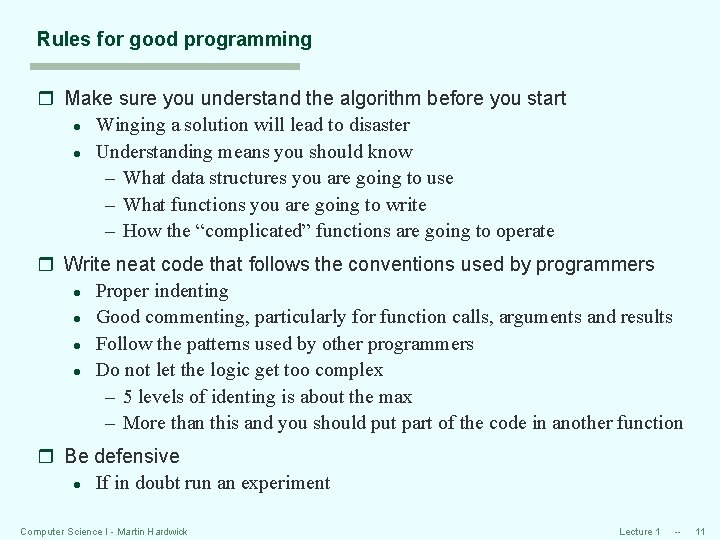
Rules for good programming r Make sure you understand the algorithm before you start l Winging a solution will lead to disaster l Understanding means you should know – What data structures you are going to use – What functions you are going to write – How the “complicated” functions are going to operate r Write neat code that follows the conventions used by programmers l Proper indenting l Good commenting, particularly for function calls, arguments and results l Follow the patterns used by other programmers l Do not let the logic get too complex – 5 levels of identing is about the max – More than this and you should put part of the code in another function r Be defensive l If in doubt run an experiment Computer Science I - Martin Hardwick Lecture 1 -- 11
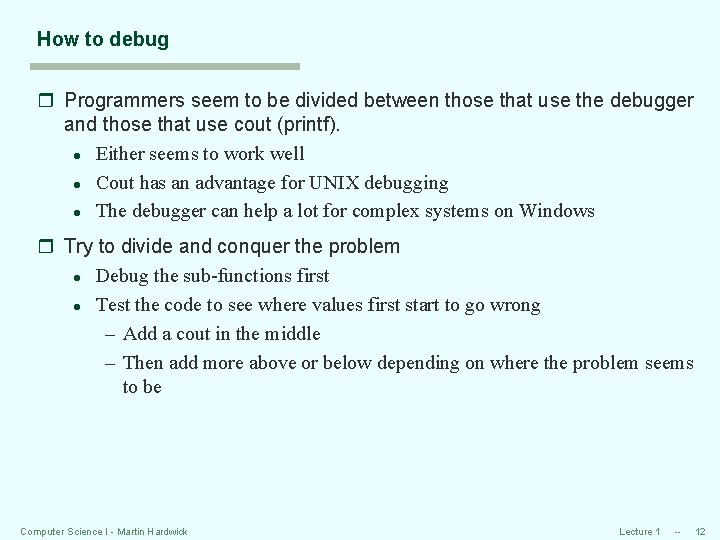
How to debug r Programmers seem to be divided between those that use the debugger and those that use cout (printf). l Either seems to work well l Cout has an advantage for UNIX debugging l The debugger can help a lot for complex systems on Windows r Try to divide and conquer the problem l Debug the sub-functions first l Test the code to see where values first start to go wrong – Add a cout in the middle – Then add more above or below depending on where the problem seems to be Computer Science I - Martin Hardwick Lecture 1 -- 12
Photovoltaic array maximum power point tracking array
Array dimensi 3
Apa itu menginisialisasi
Jagged array
Suatu array dikatakan upper triangular jika
Associative array vs indexed array
Array 2 dimension
Pin grid array
Broadside array and endfire array difference
Contoh studi kasus stack
Lunar laser ranging retroreflector array
Array adt
Array element is accessed using