Arithmetic expressions containing Mathematical functions Arithmetic expressions containing
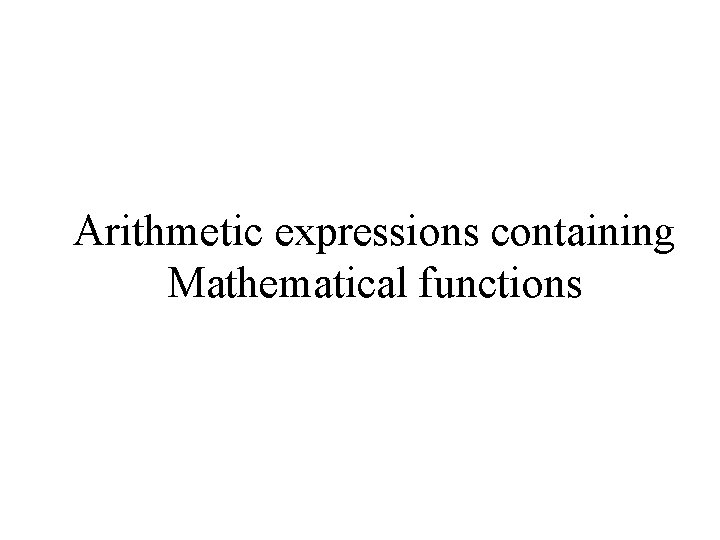
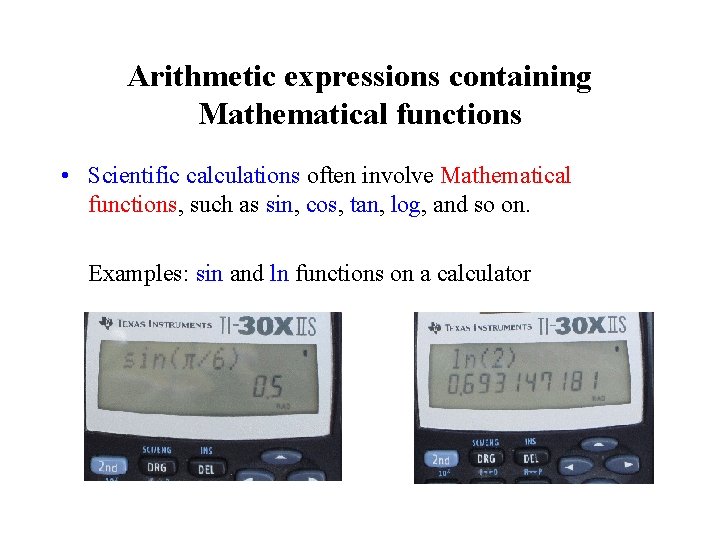
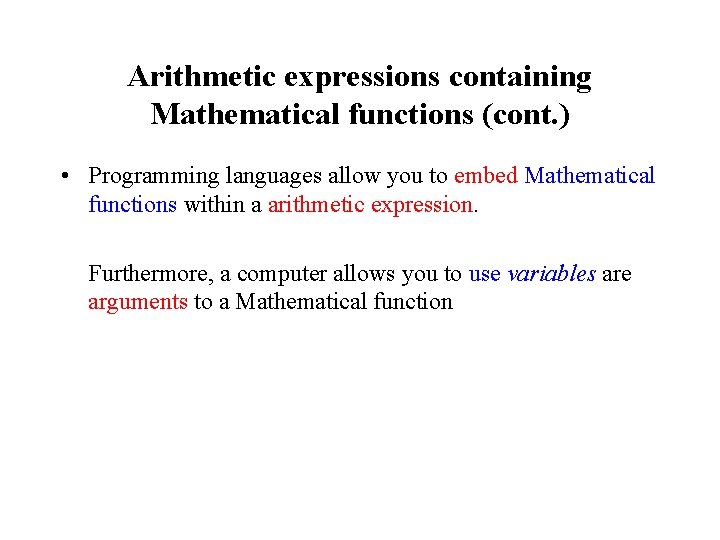
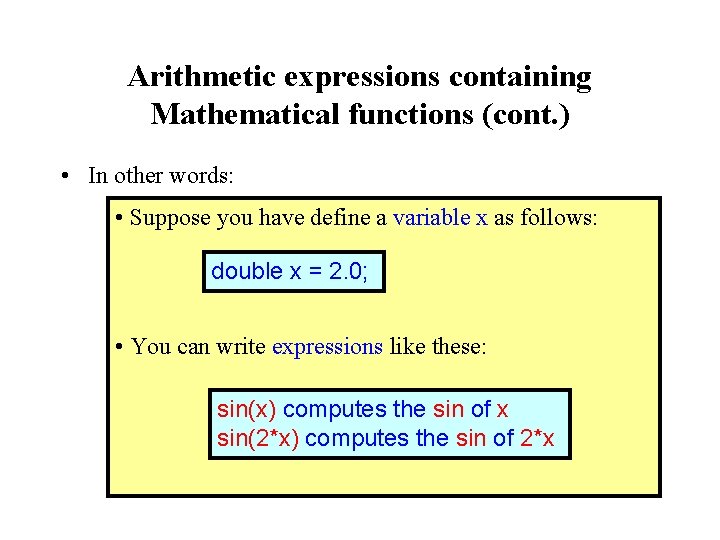
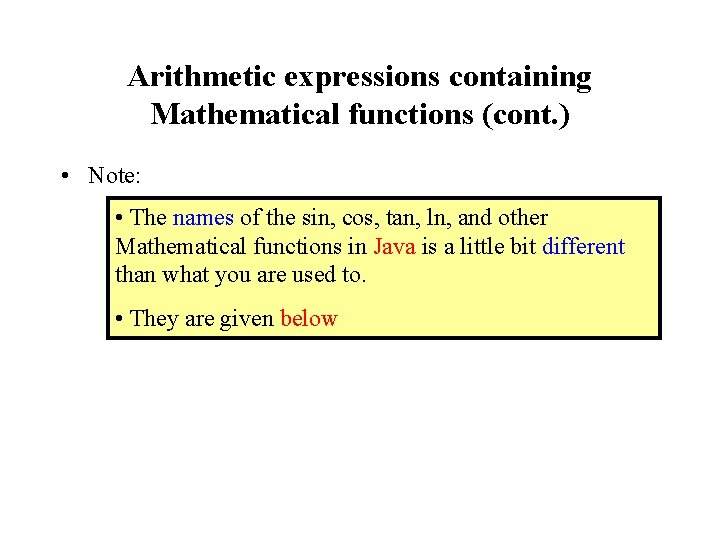
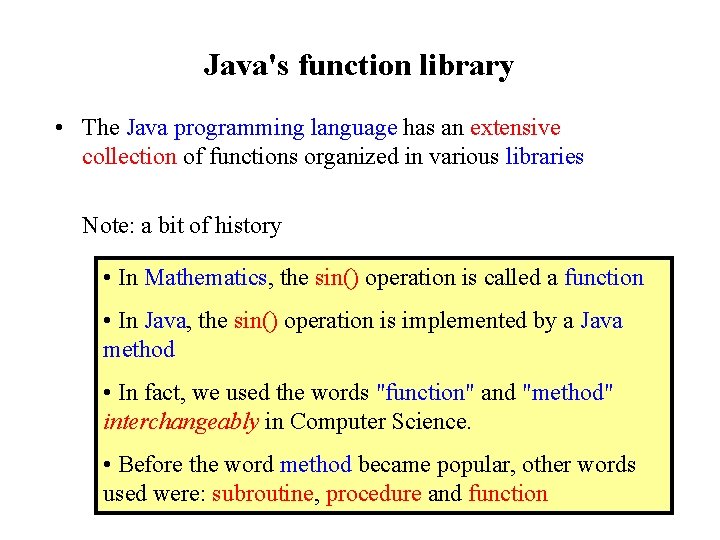
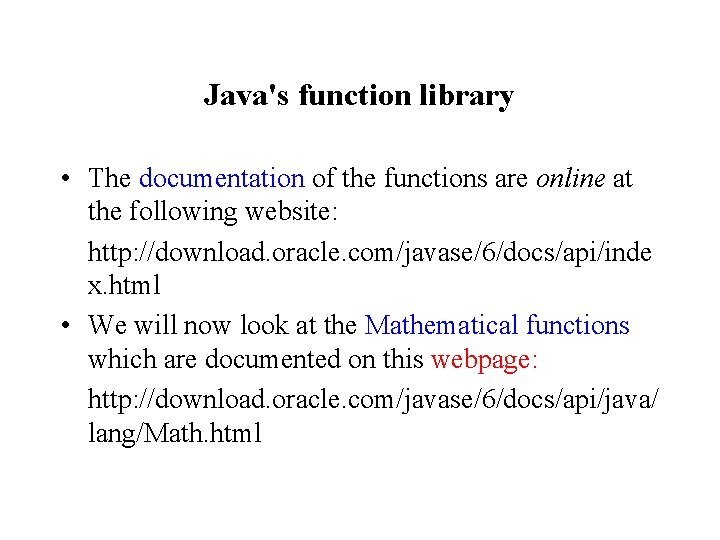
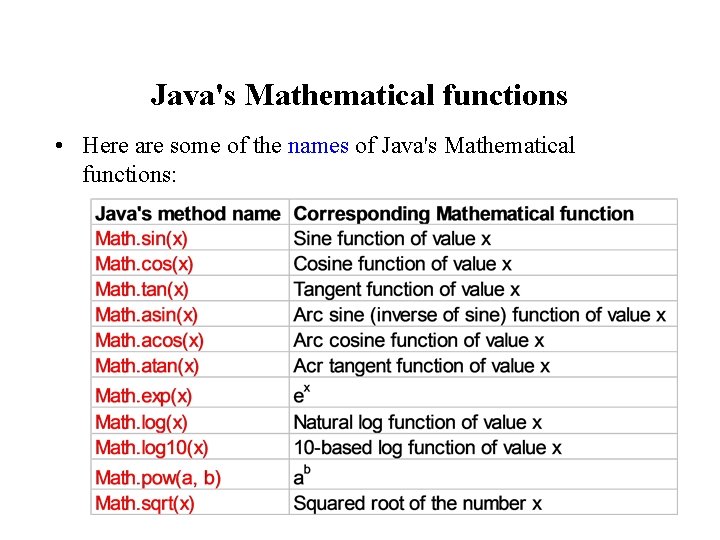
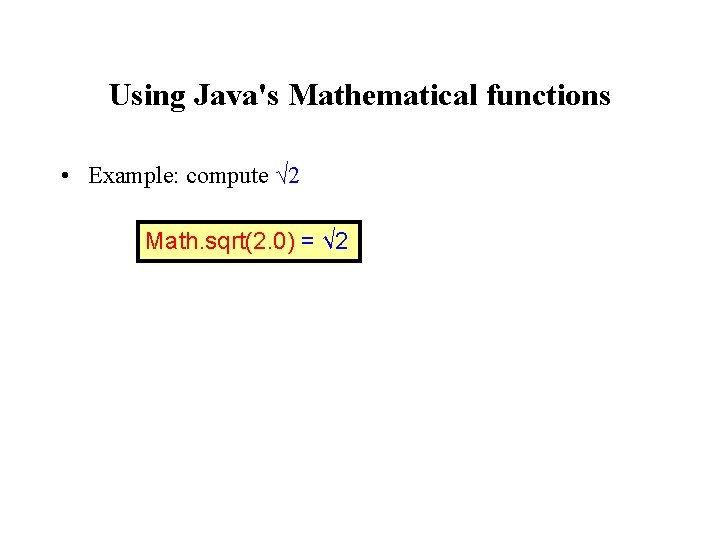
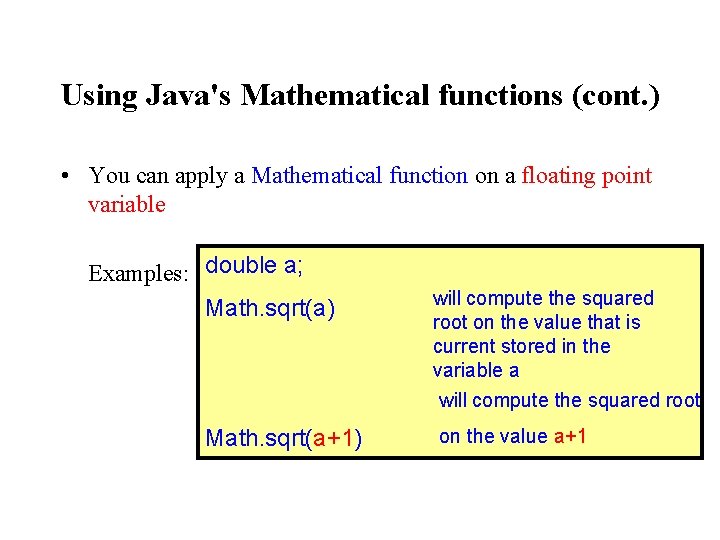
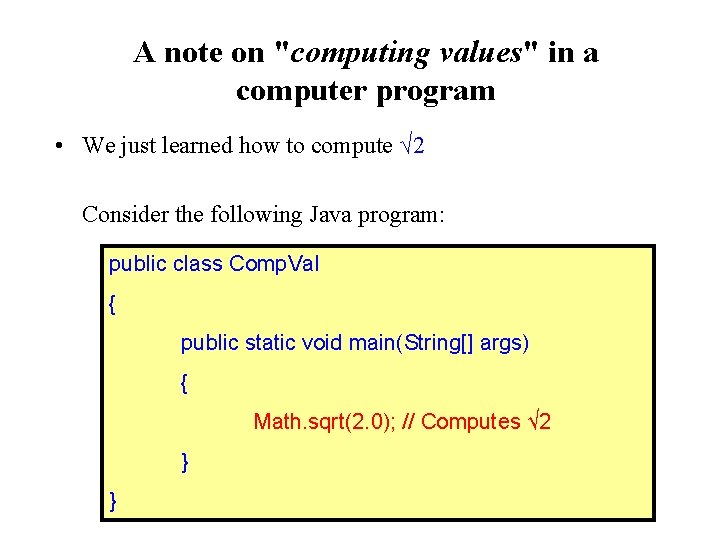
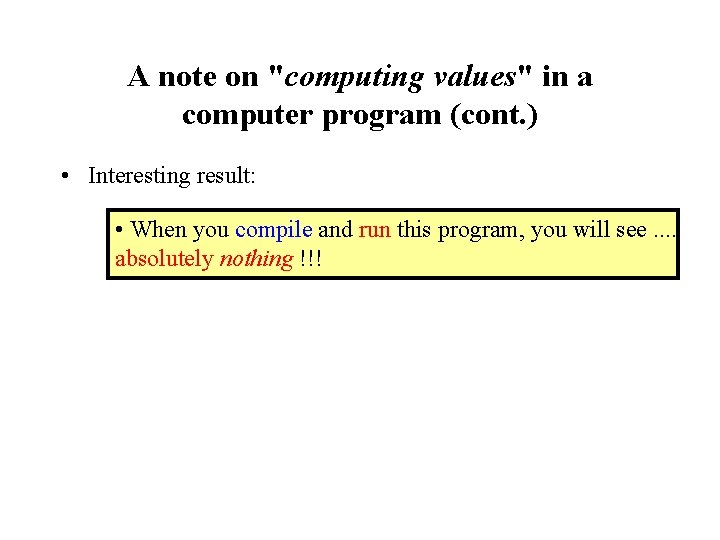
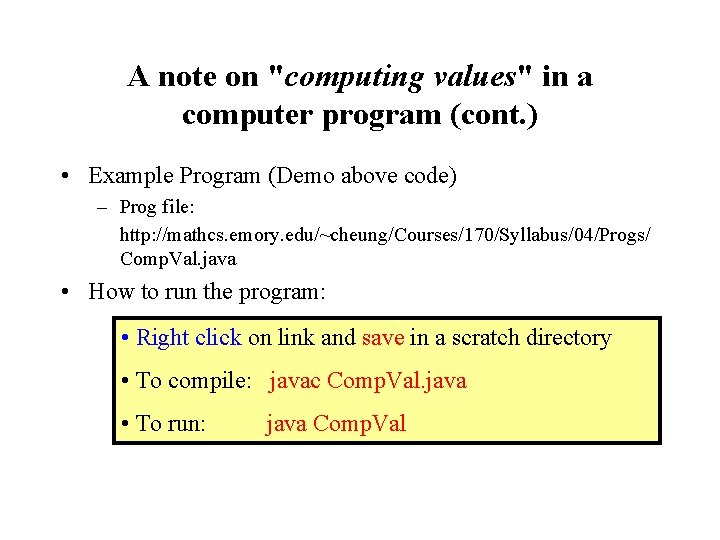
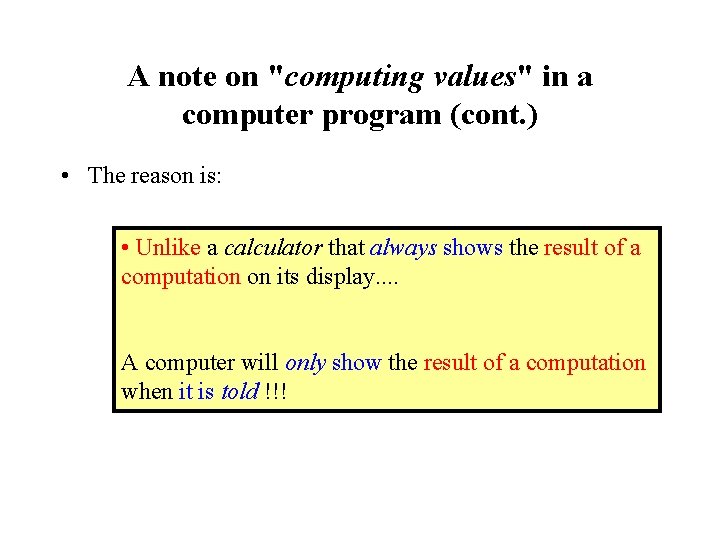
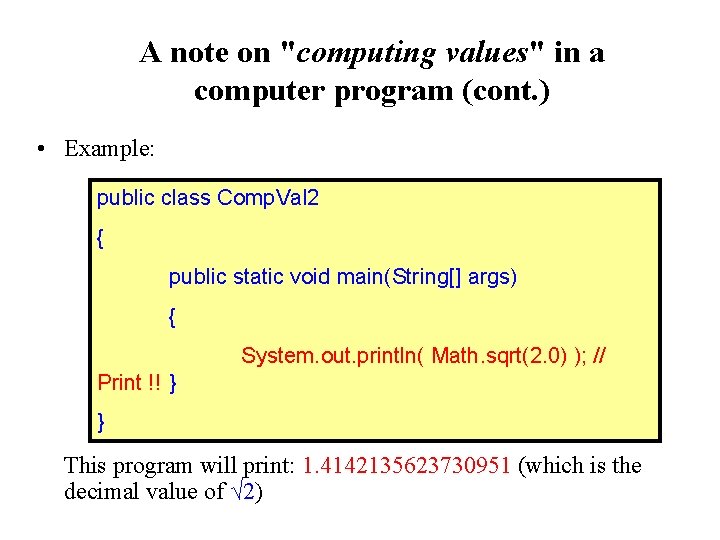
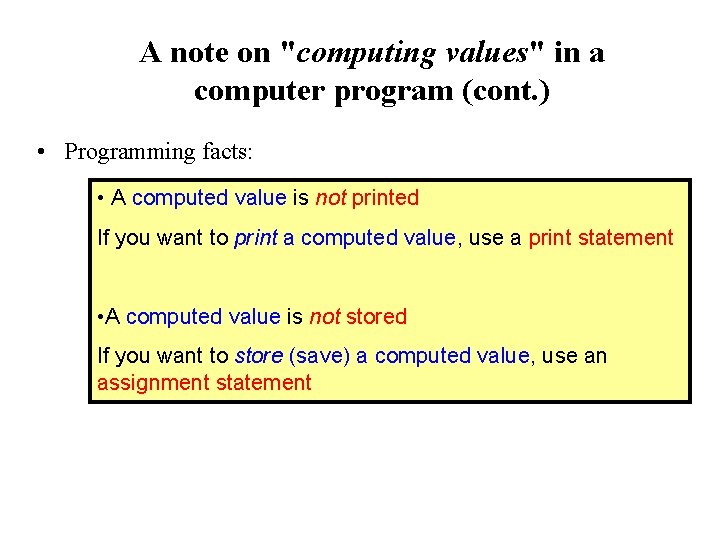
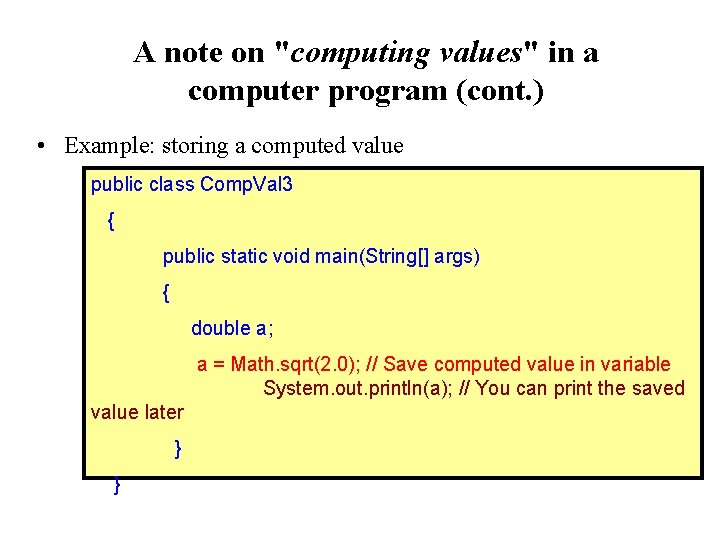
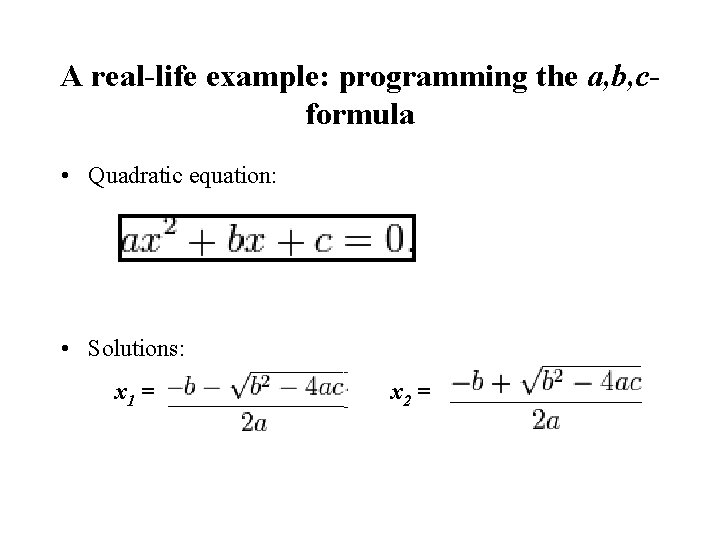
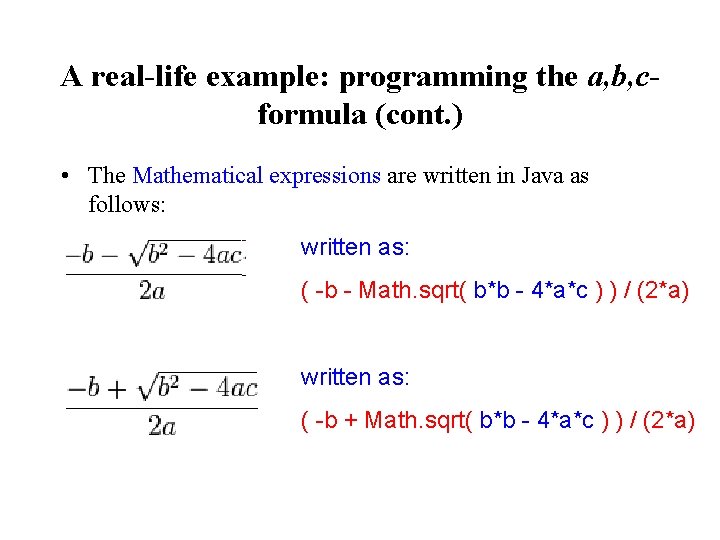
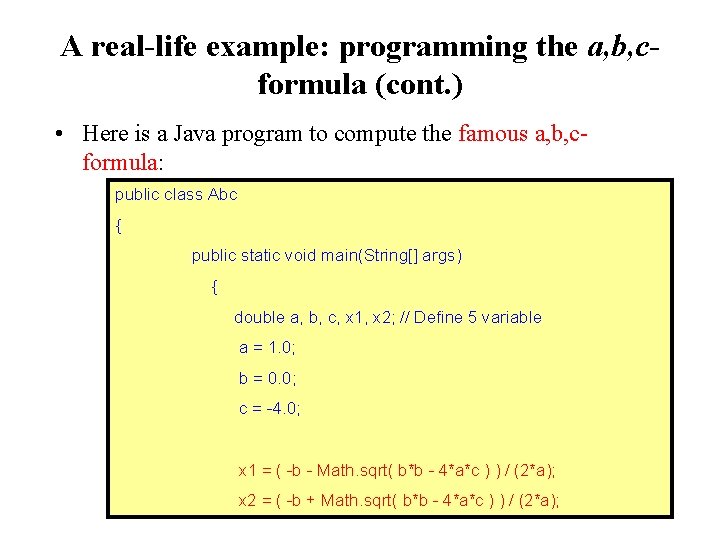
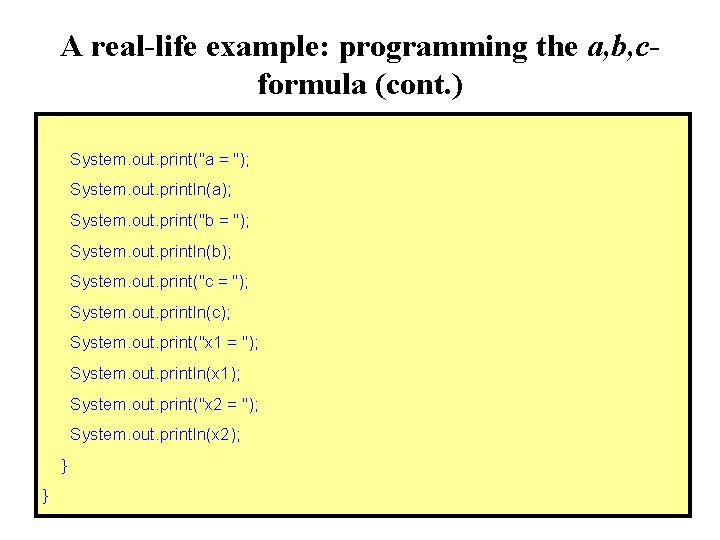
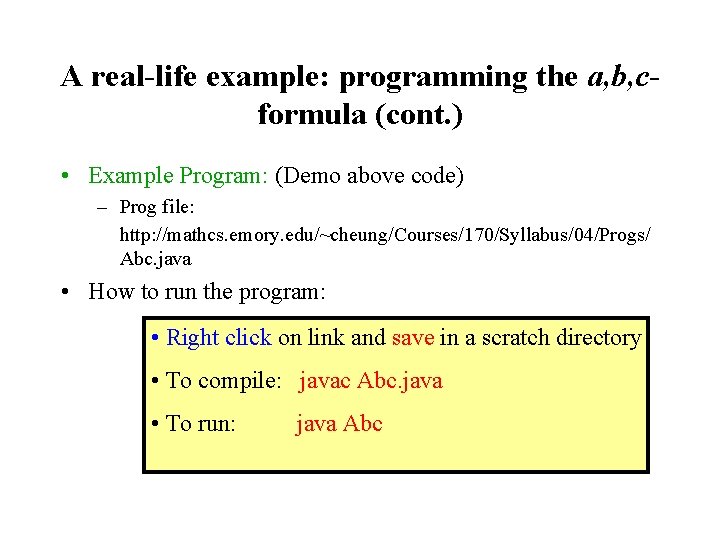
- Slides: 22
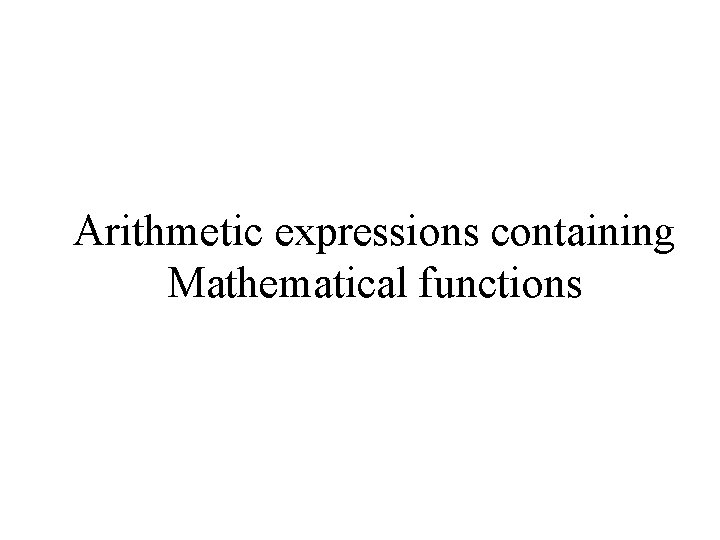
Arithmetic expressions containing Mathematical functions
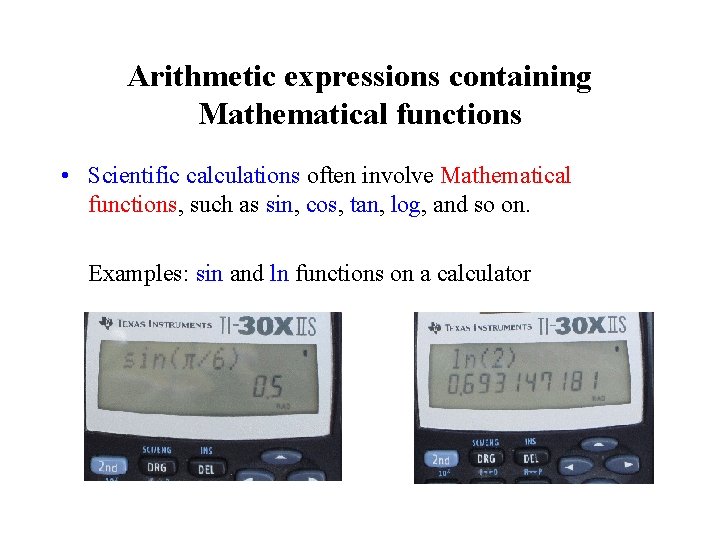
Arithmetic expressions containing Mathematical functions • Scientific calculations often involve Mathematical functions, such as sin, cos, tan, log, and so on. Examples: sin and ln functions on a calculator
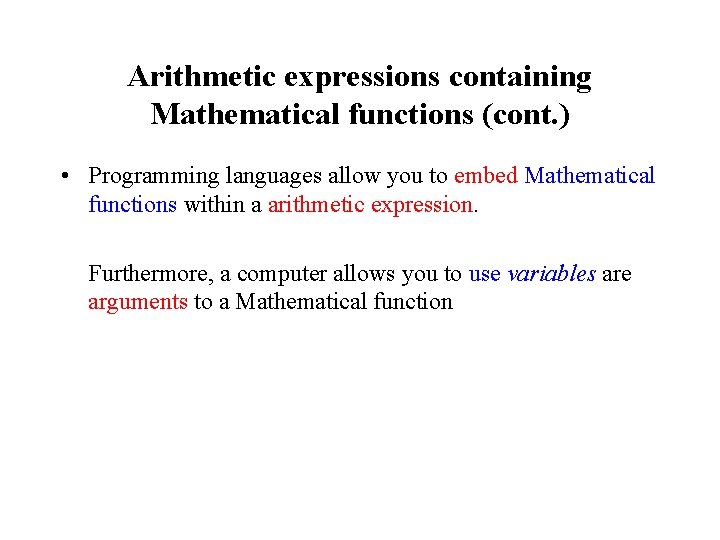
Arithmetic expressions containing Mathematical functions (cont. ) • Programming languages allow you to embed Mathematical functions within a arithmetic expression. Furthermore, a computer allows you to use variables are arguments to a Mathematical function
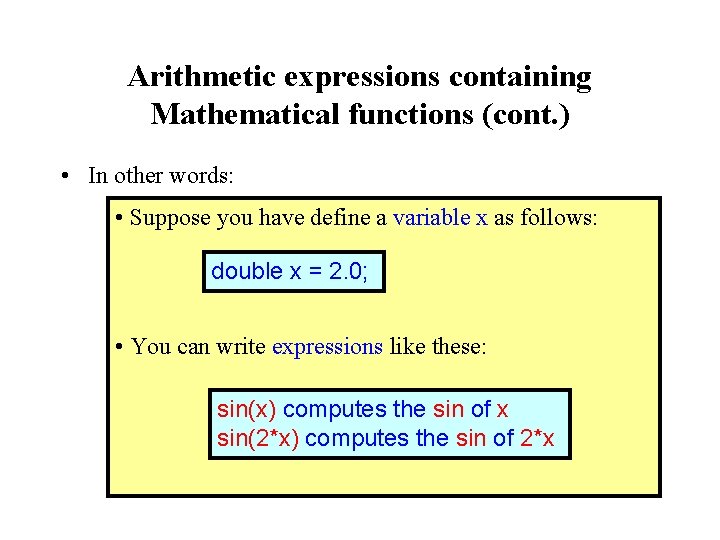
Arithmetic expressions containing Mathematical functions (cont. ) • In other words: • Suppose you have define a variable x as follows: double x = 2. 0; • You can write expressions like these: sin(x) computes the sin of x sin(2*x) computes the sin of 2*x
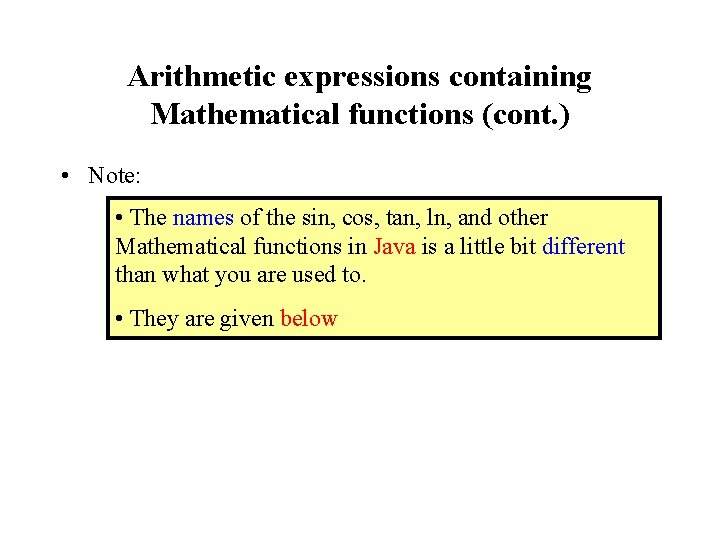
Arithmetic expressions containing Mathematical functions (cont. ) • Note: • The names of the sin, cos, tan, ln, and other Mathematical functions in Java is a little bit different than what you are used to. • They are given below
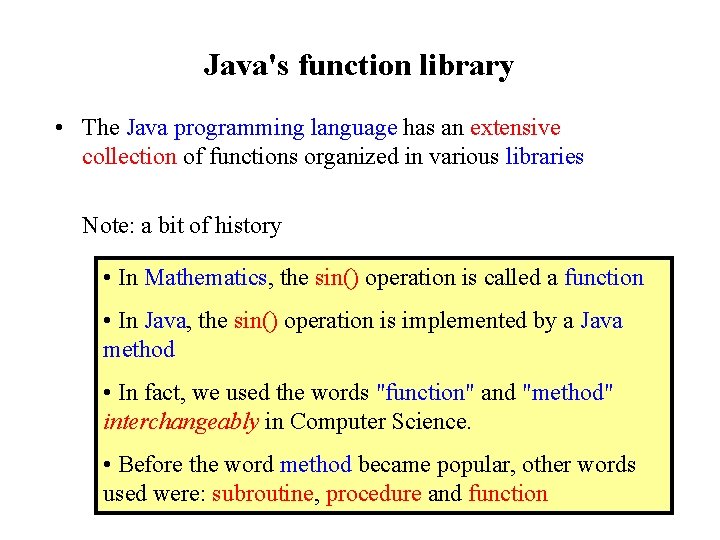
Java's function library • The Java programming language has an extensive collection of functions organized in various libraries Note: a bit of history • In Mathematics, the sin() operation is called a function • In Java, the sin() operation is implemented by a Java method • In fact, we used the words "function" and "method" interchangeably in Computer Science. • Before the word method became popular, other words used were: subroutine, procedure and function
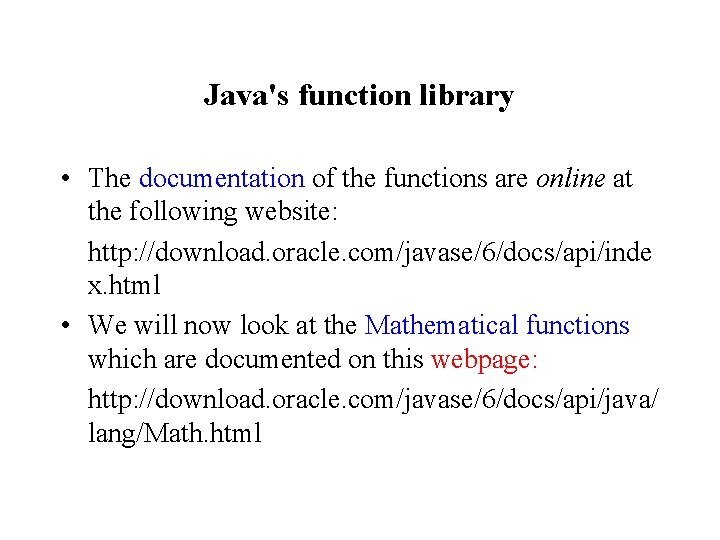
Java's function library • The documentation of the functions are online at the following website: http: //download. oracle. com/javase/6/docs/api/inde x. html • We will now look at the Mathematical functions which are documented on this webpage: http: //download. oracle. com/javase/6/docs/api/java/ lang/Math. html
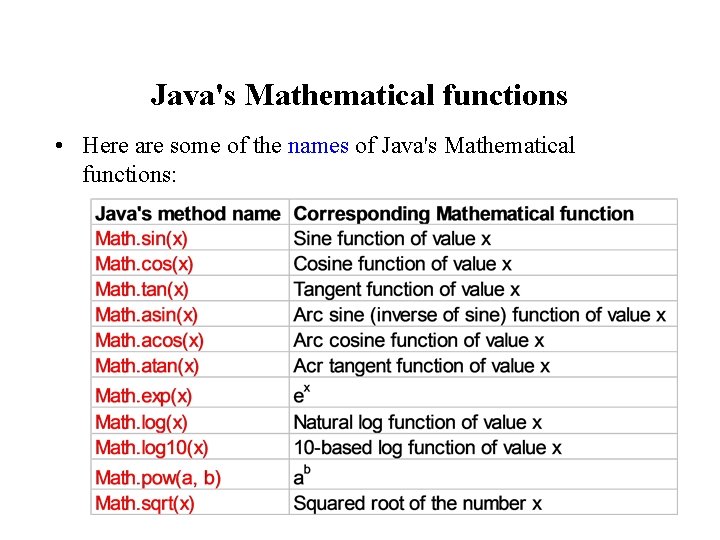
Java's Mathematical functions • Here are some of the names of Java's Mathematical functions:
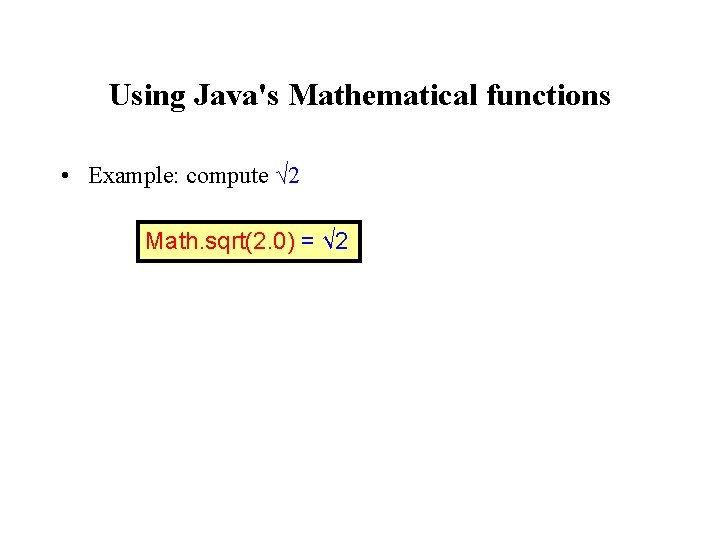
Using Java's Mathematical functions • Example: compute √ 2 Math. sqrt(2. 0) = √ 2
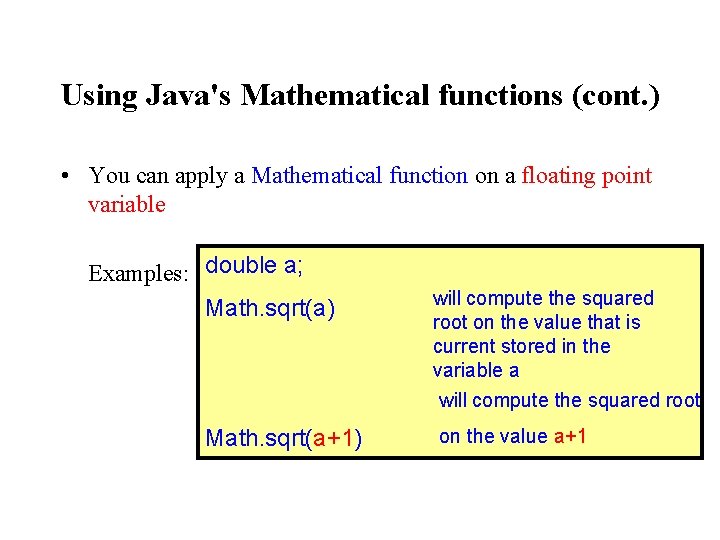
Using Java's Mathematical functions (cont. ) • You can apply a Mathematical function on a floating point variable Examples: double a; Math. sqrt(a) Math. sqrt(a+1) will compute the squared root on the value that is current stored in the variable a will compute the squared root on the value a+1
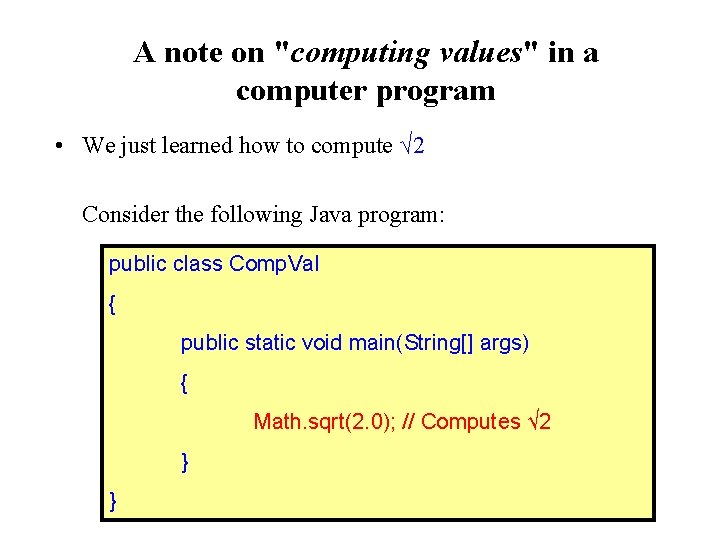
A note on "computing values" in a computer program • We just learned how to compute √ 2 Consider the following Java program: public class Comp. Val { public static void main(String[] args) { Math. sqrt(2. 0); // Computes √ 2 } }
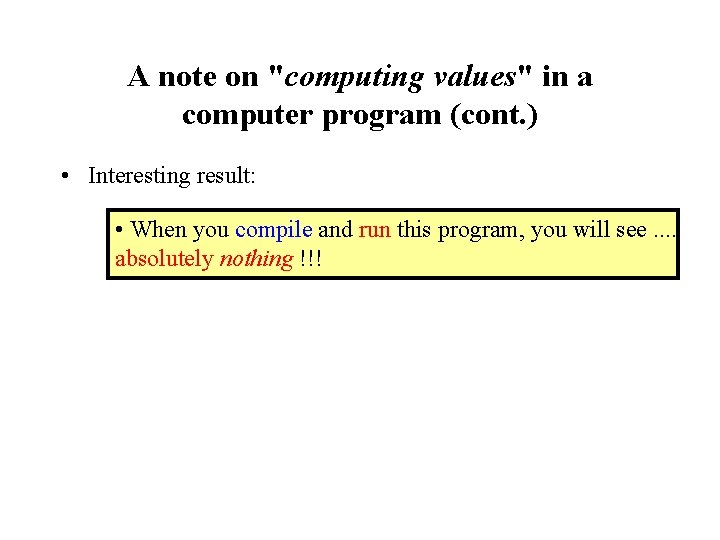
A note on "computing values" in a computer program (cont. ) • Interesting result: • When you compile and run this program, you will see. . absolutely nothing !!!
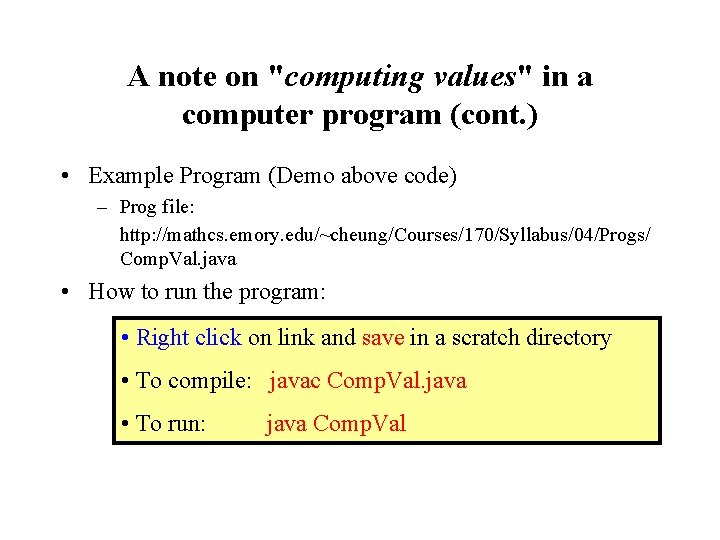
A note on "computing values" in a computer program (cont. ) • Example Program (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/04/Progs/ Comp. Val. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Comp. Val. java • To run: java Comp. Val
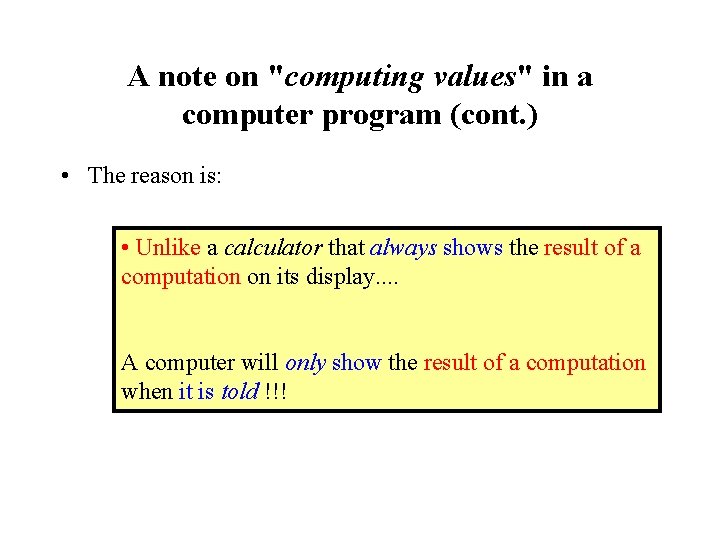
A note on "computing values" in a computer program (cont. ) • The reason is: • Unlike a calculator that always shows the result of a computation on its display. . A computer will only show the result of a computation when it is told !!!
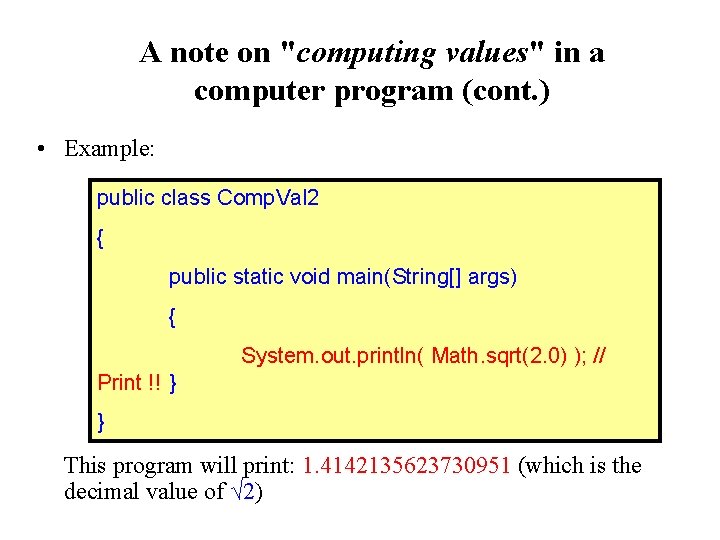
A note on "computing values" in a computer program (cont. ) • Example: public class Comp. Val 2 { public static void main(String[] args) { System. out. println( Math. sqrt(2. 0) ); // Print !! } } This program will print: 1. 4142135623730951 (which is the decimal value of √ 2)
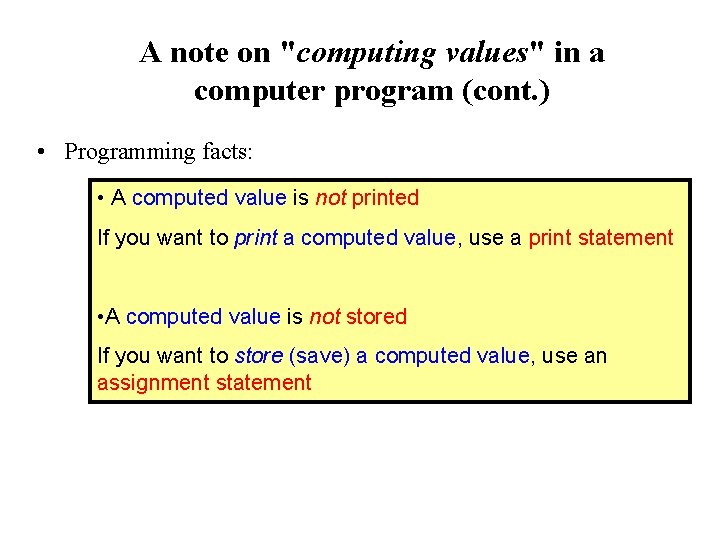
A note on "computing values" in a computer program (cont. ) • Programming facts: • A computed value is not printed If you want to print a computed value, use a print statement • A computed value is not stored If you want to store (save) a computed value, use an assignment statement
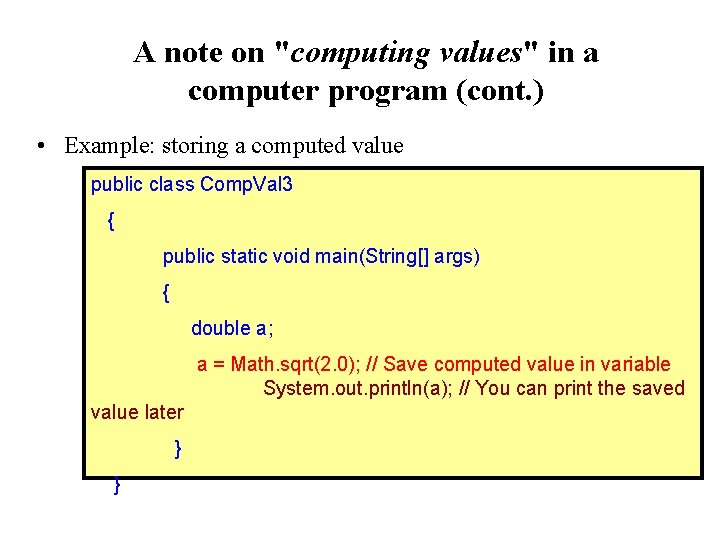
A note on "computing values" in a computer program (cont. ) • Example: storing a computed value public class Comp. Val 3 { public static void main(String[] args) { double a; a = Math. sqrt(2. 0); // Save computed value in variable System. out. println(a); // You can print the saved value later } }
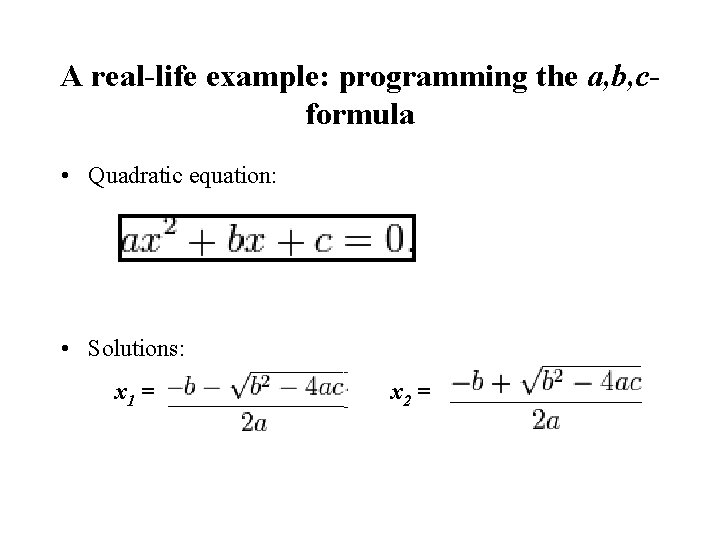
A real-life example: programming the a, b, cformula • Quadratic equation: • Solutions: x 1 = x 2 =
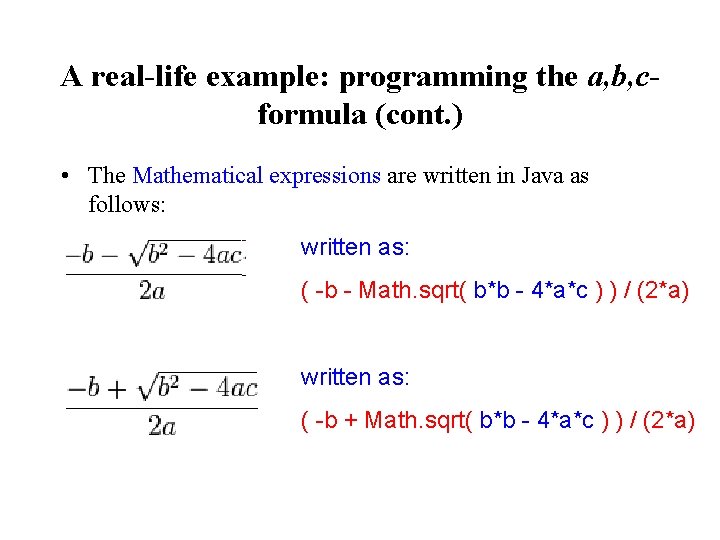
A real-life example: programming the a, b, cformula (cont. ) • The Mathematical expressions are written in Java as follows: written as: ( -b - Math. sqrt( b*b - 4*a*c ) ) / (2*a) written as: ( -b + Math. sqrt( b*b - 4*a*c ) ) / (2*a)
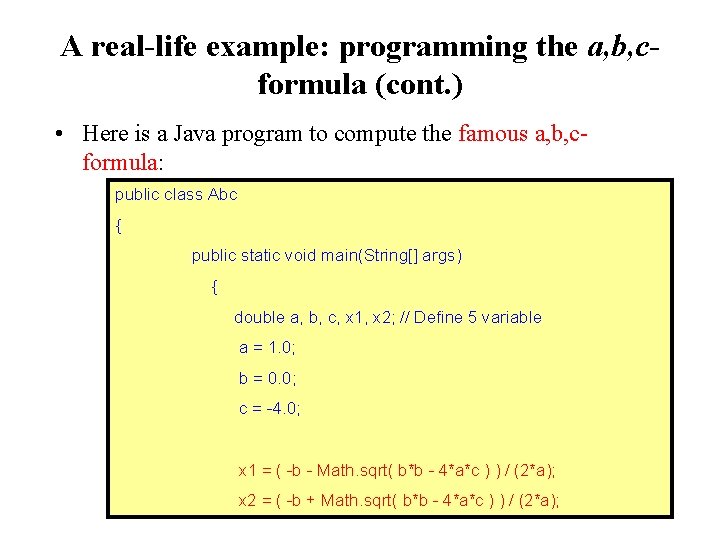
A real-life example: programming the a, b, cformula (cont. ) • Here is a Java program to compute the famous a, b, cformula: public class Abc { public static void main(String[] args) { double a, b, c, x 1, x 2; // Define 5 variable a = 1. 0; b = 0. 0; c = -4. 0; x 1 = ( -b - Math. sqrt( b*b - 4*a*c ) ) / (2*a); x 2 = ( -b + Math. sqrt( b*b - 4*a*c ) ) / (2*a);
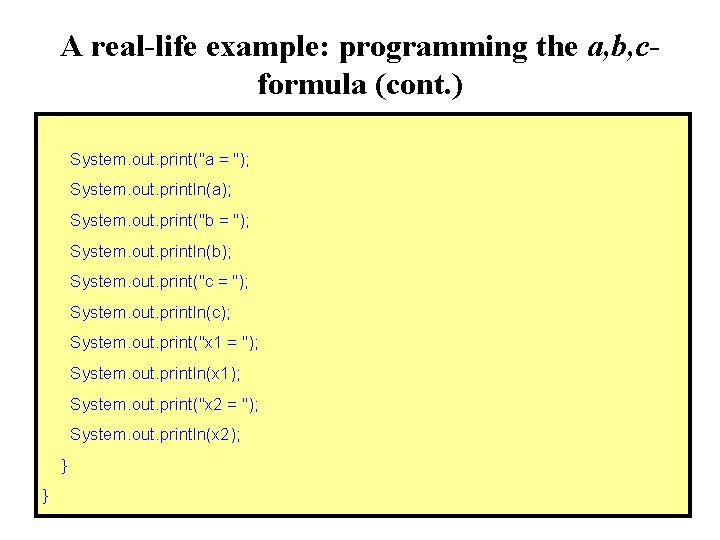
A real-life example: programming the a, b, cformula (cont. ) System. out. print("a = "); System. out. println(a); System. out. print("b = "); System. out. println(b); System. out. print("c = "); System. out. println(c); System. out. print("x 1 = "); System. out. println(x 1); System. out. print("x 2 = "); System. out. println(x 2); } }
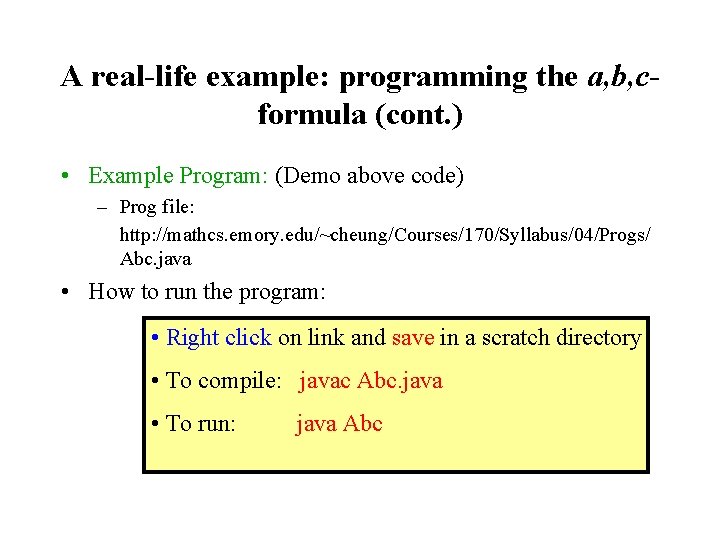
A real-life example: programming the a, b, cformula (cont. ) • Example Program: (Demo above code) – Prog file: http: //mathcs. emory. edu/~cheung/Courses/170/Syllabus/04/Progs/ Abc. java • How to run the program: • Right click on link and save in a scratch directory • To compile: javac Abc. java • To run: java Abc
Arithmetic expressions containing a null value evaluate to
Arithmetic expressions containing a null value evaluate to
Non mathematical economics
Solving linear rationals with lcm
Write variable expressions for arithmetic sequences
Pointer expressions
Math scanner
Real world phenomena
Linear functions as mathematical models
Linear functions as mathematical models
Evaluating an arithmetic combination of functions
Rational expressions and functions
7-3 lesson quiz
Absolute value of x as a piecewise function
Evaluating functions
Evaluating functions and operations on functions
This sewing machine part checks the length of the stitches
Alcohols containing cppp nucleus
Middle meatus drainage
Noun phrase containing relative clause
The subsidiary ledger containing vendor accounts.
Phosphorus containing foods
Simple cubic unit cell