Polygon Triangulation Triangulation We already know that any
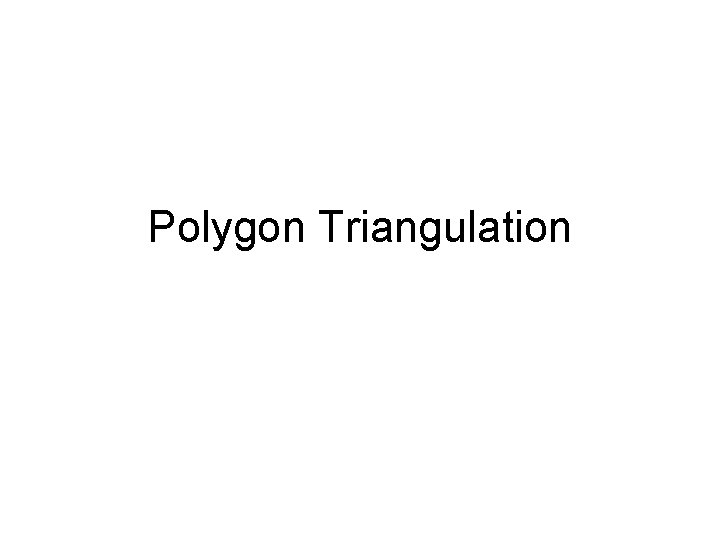
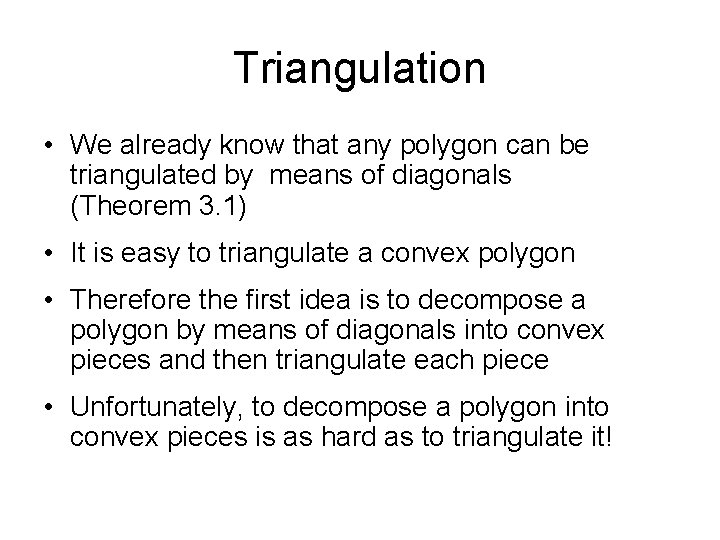
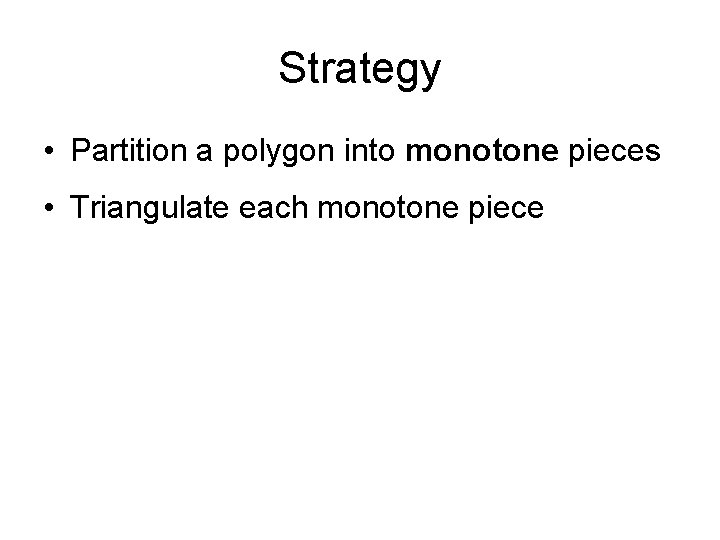
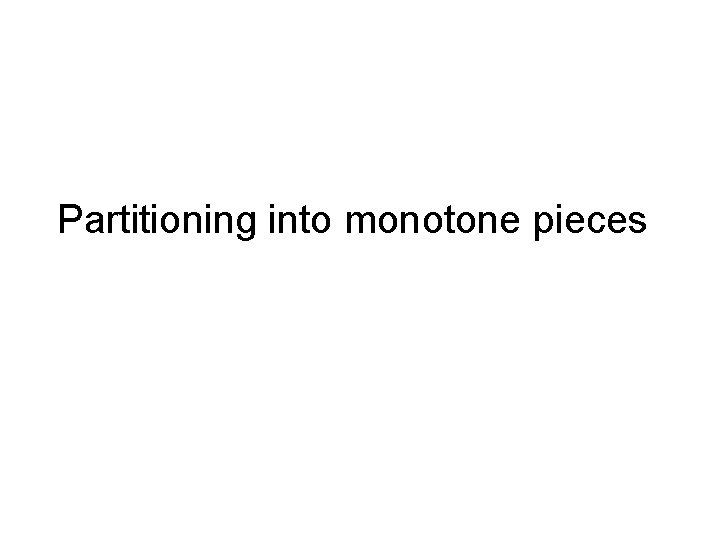
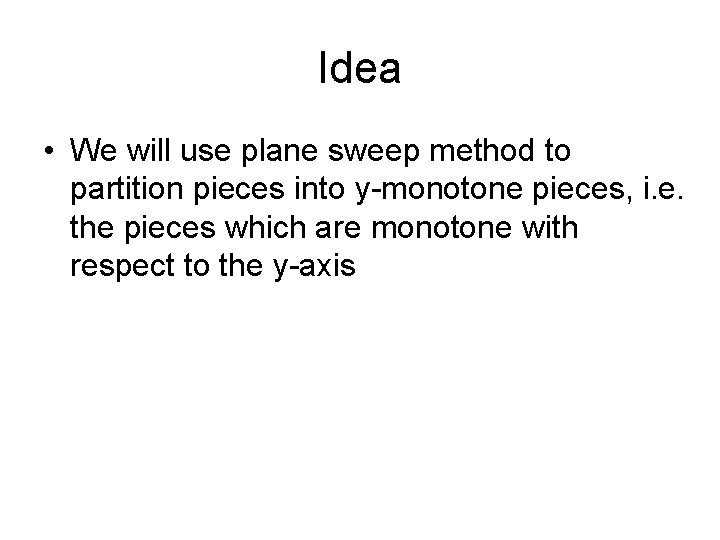
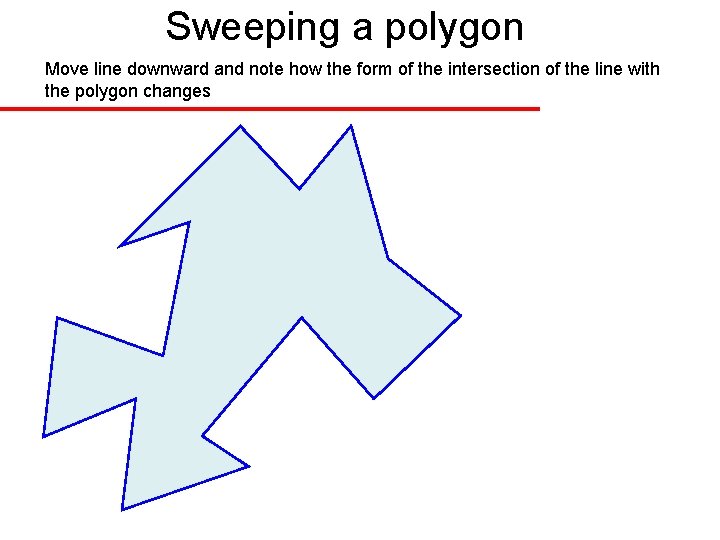
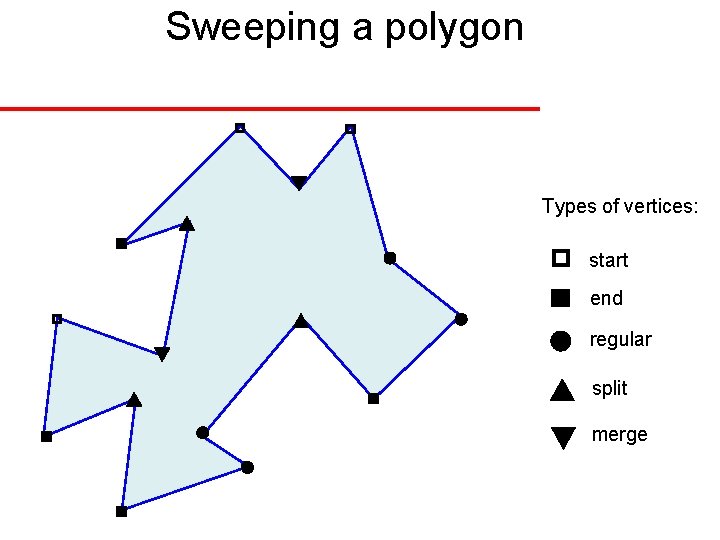
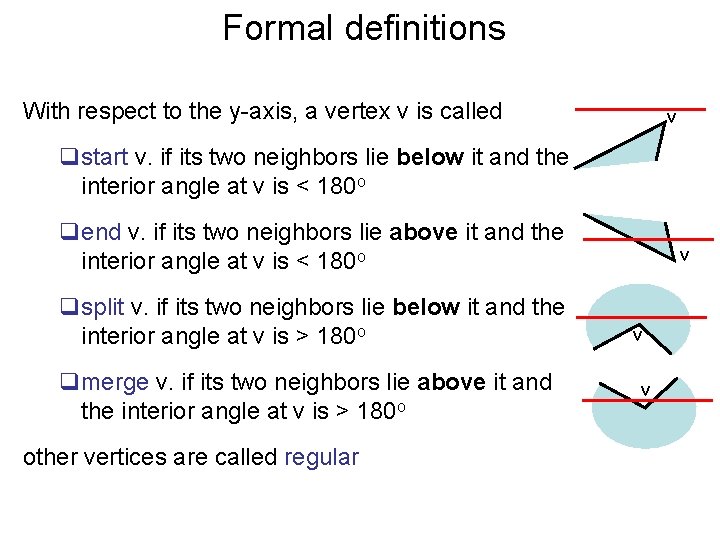
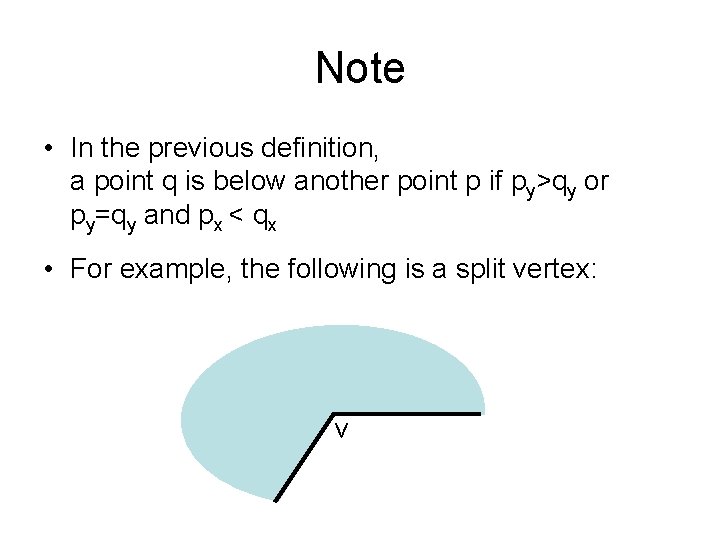
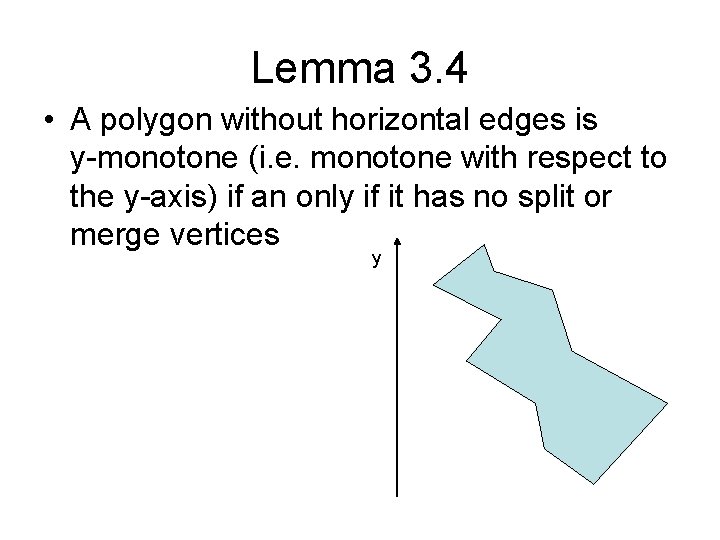
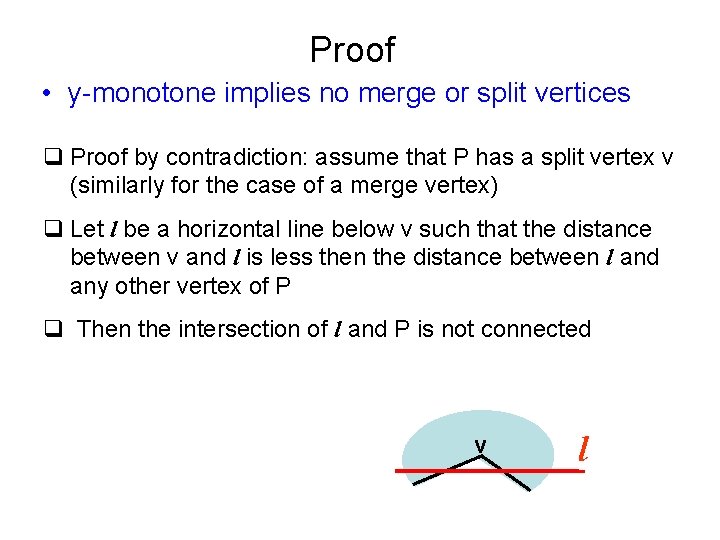
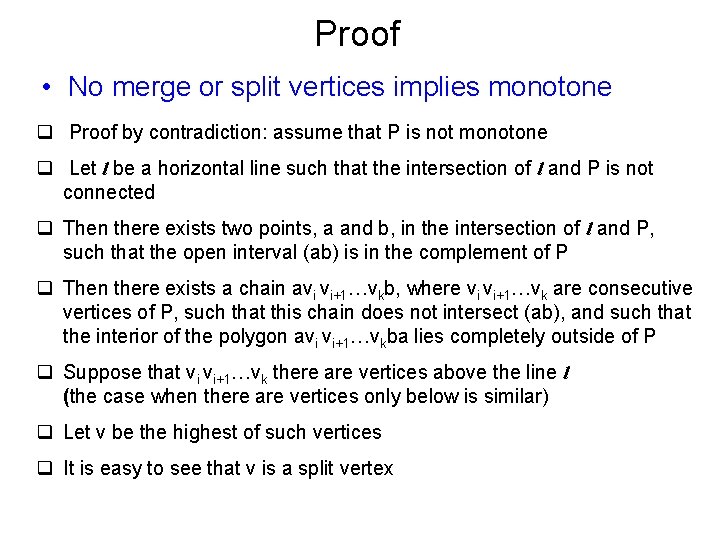
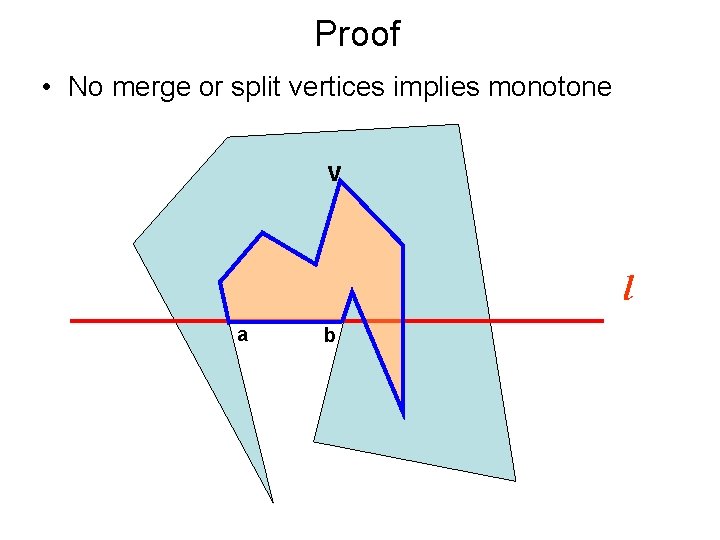
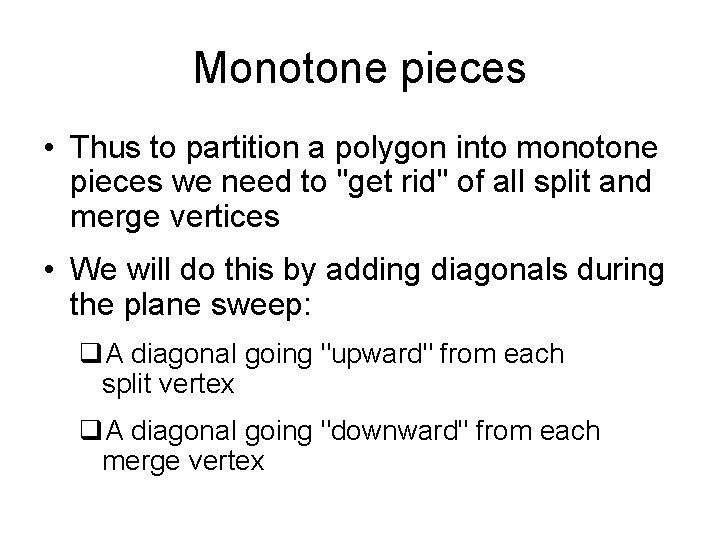
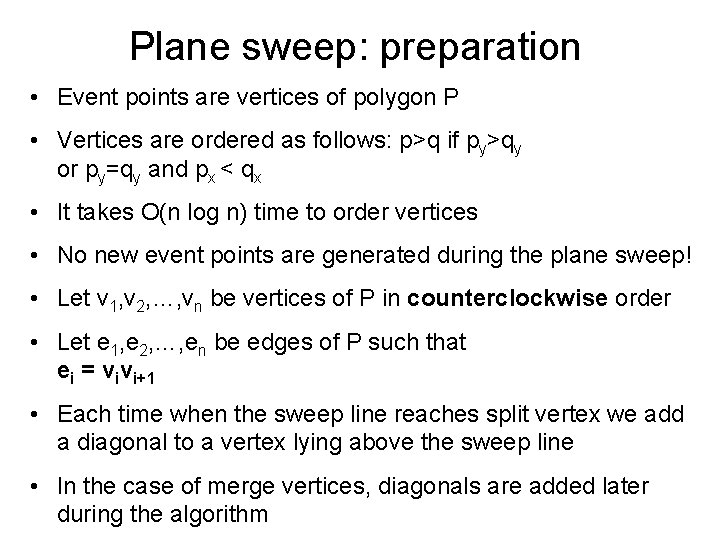
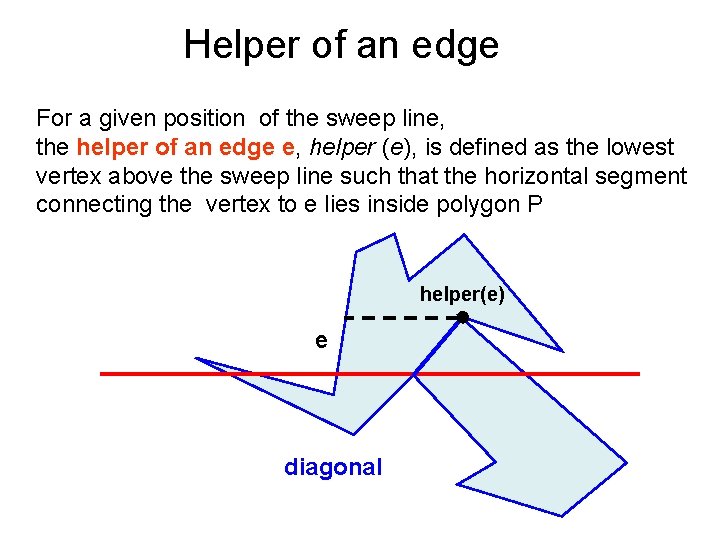
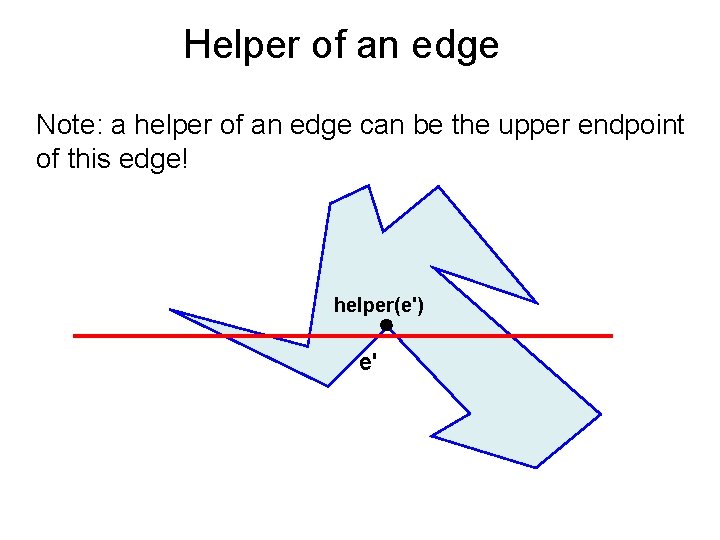
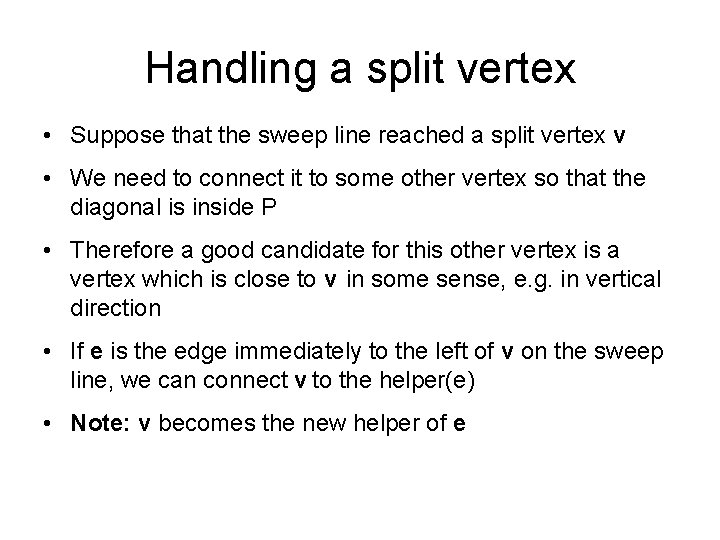
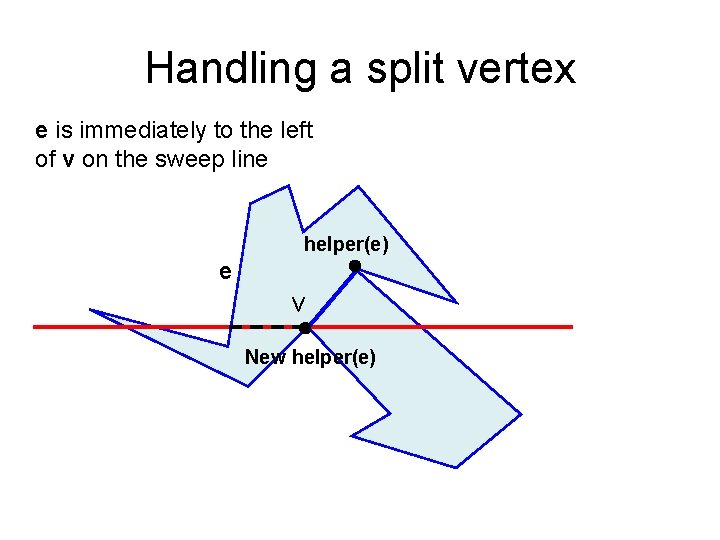
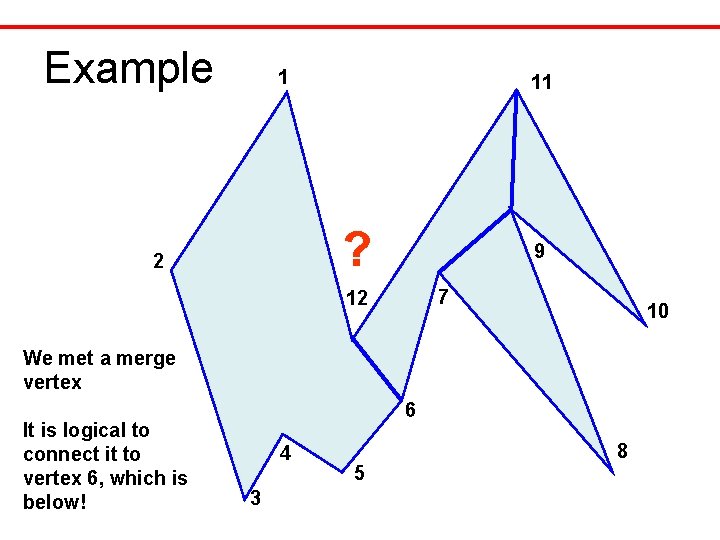
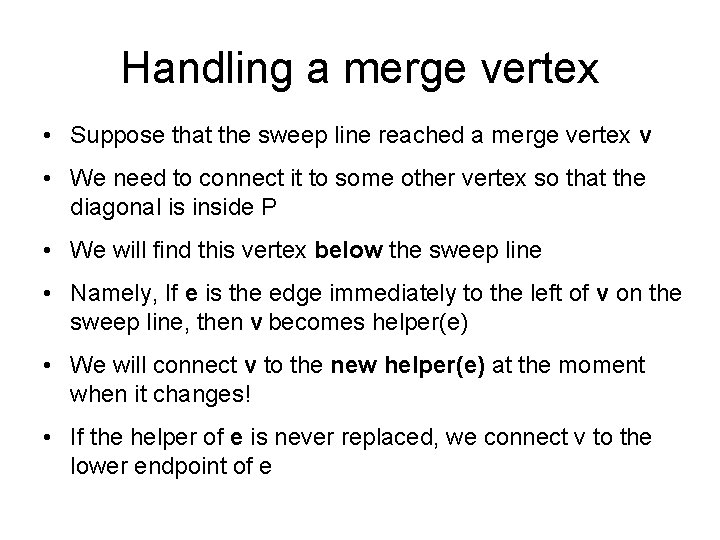
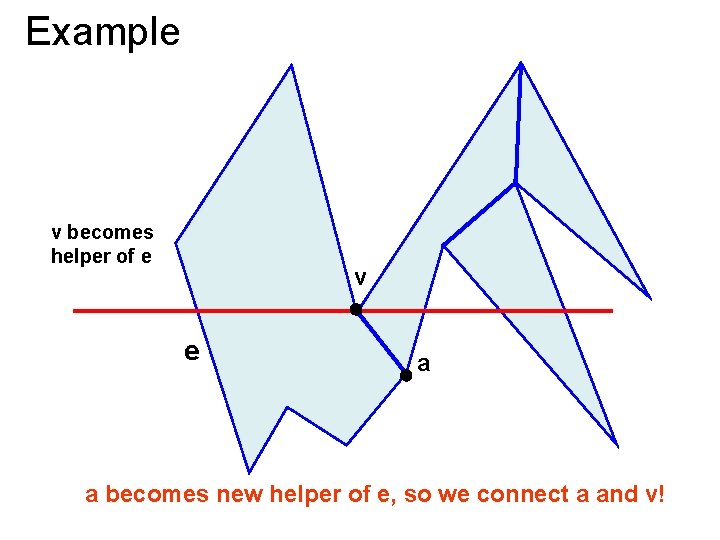
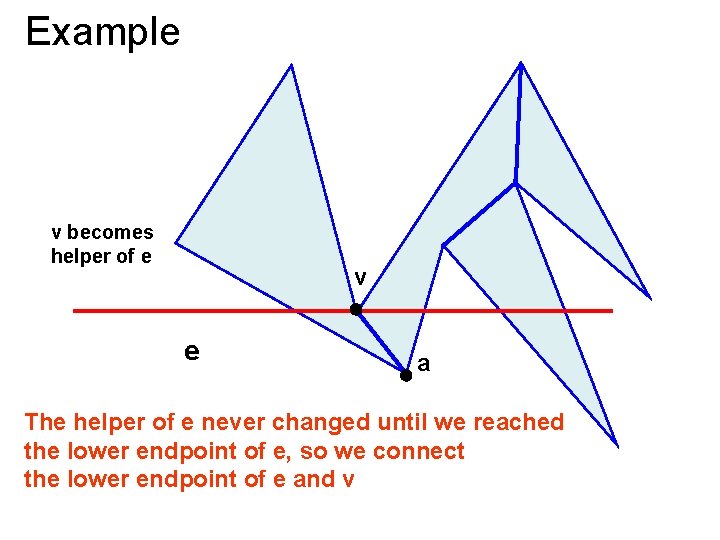
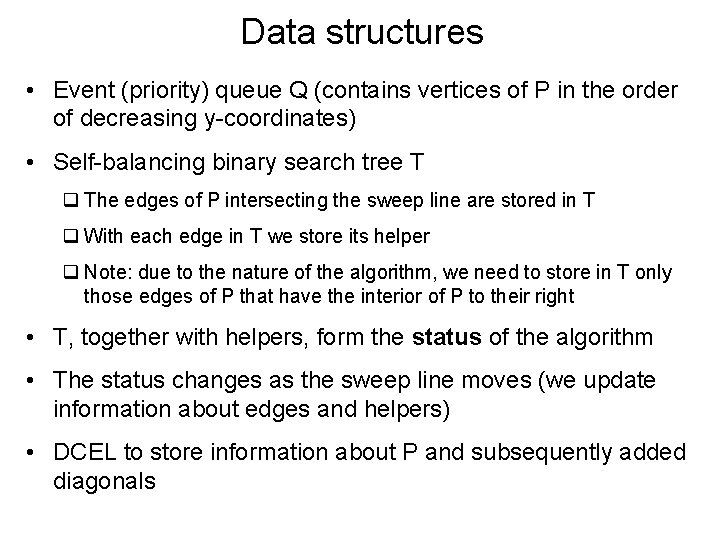
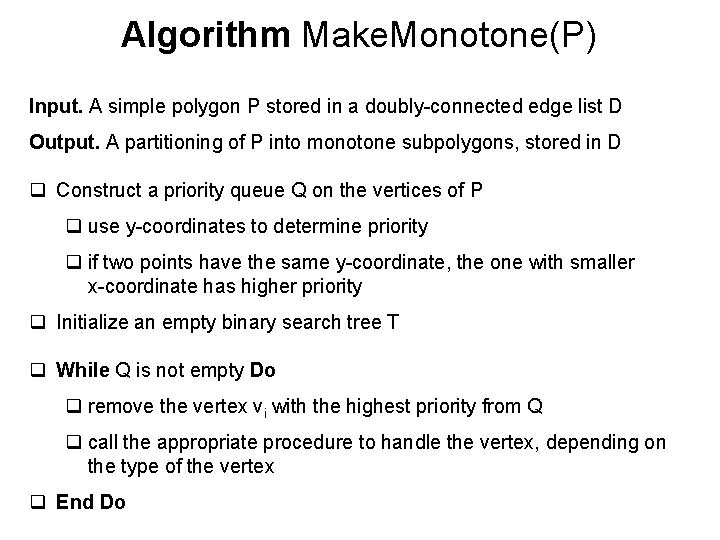
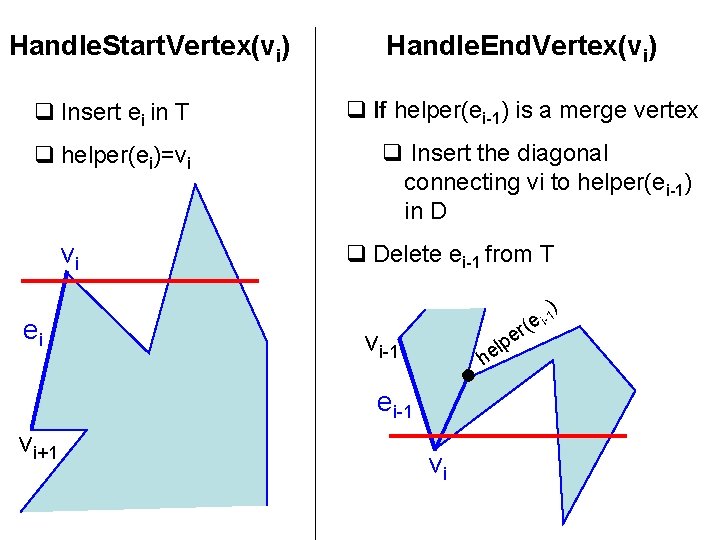
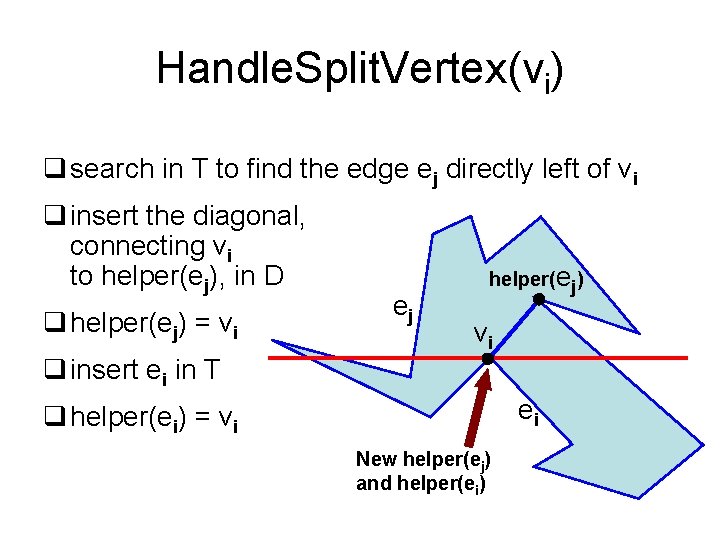
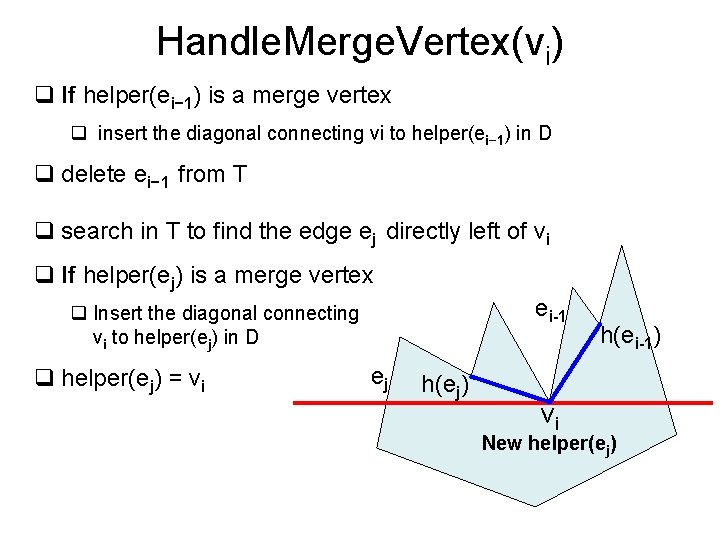
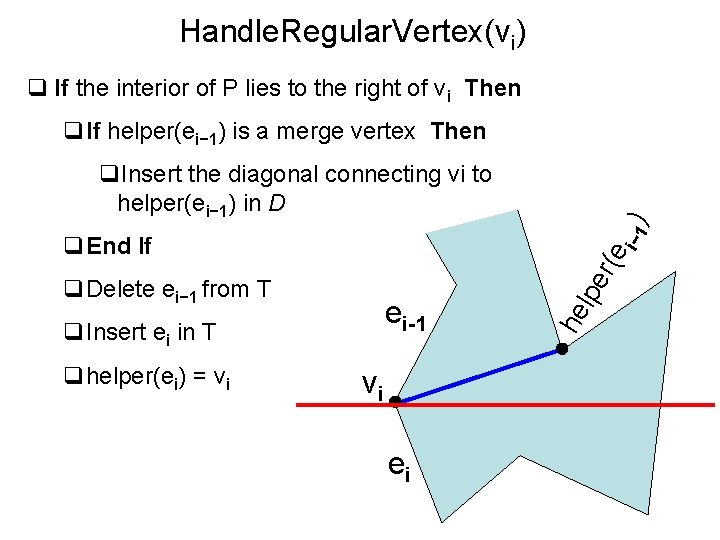
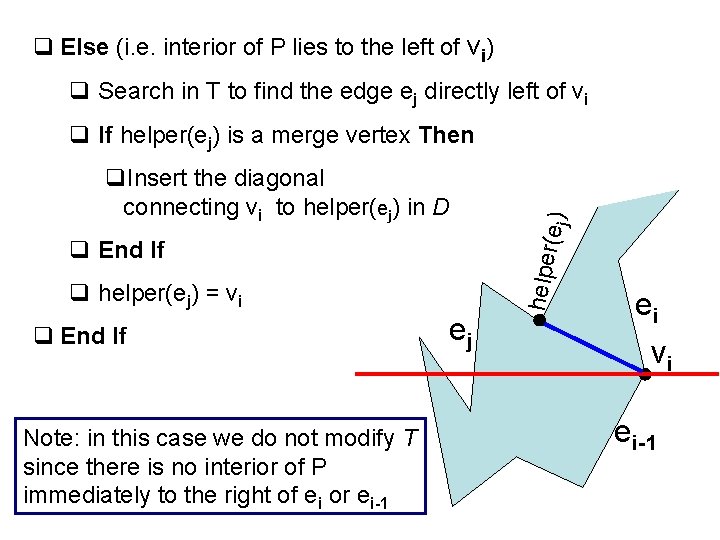
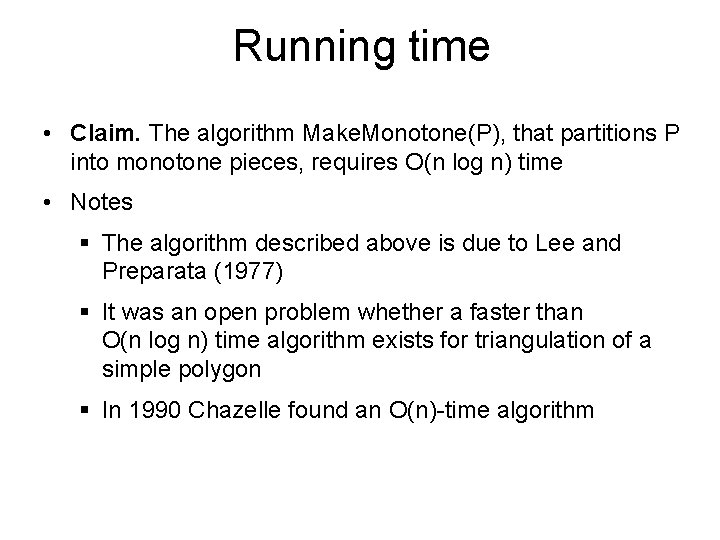
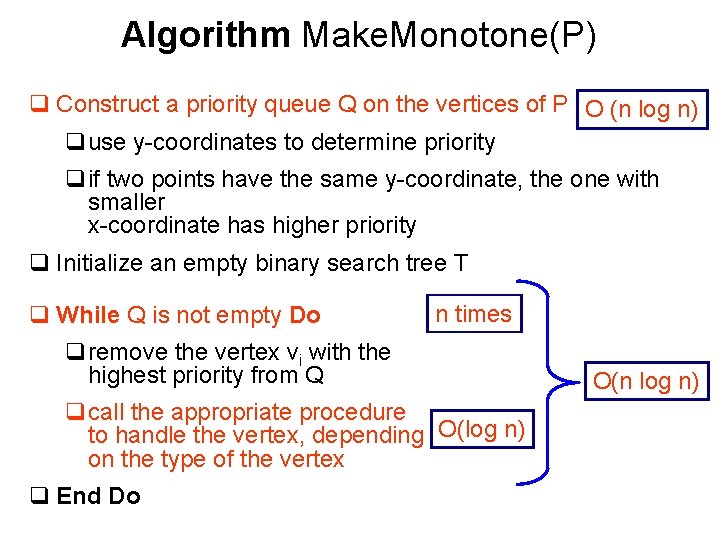
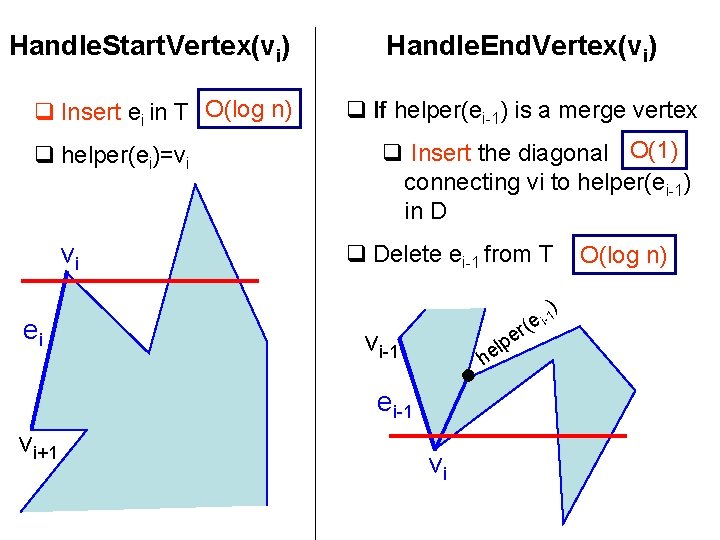
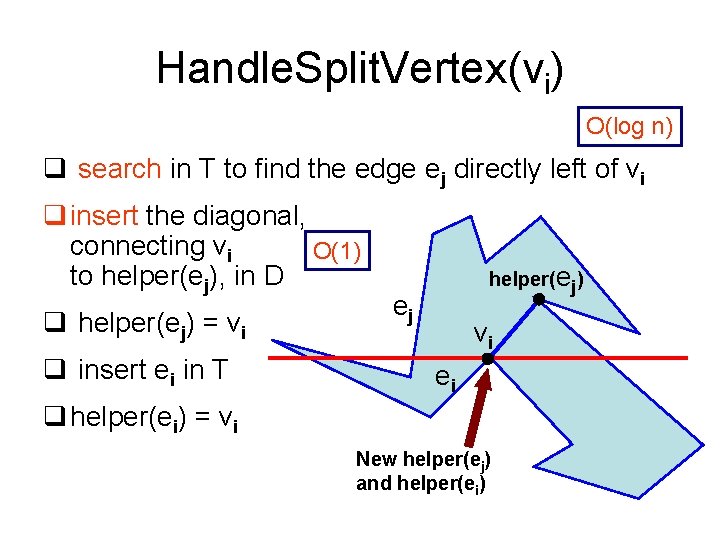
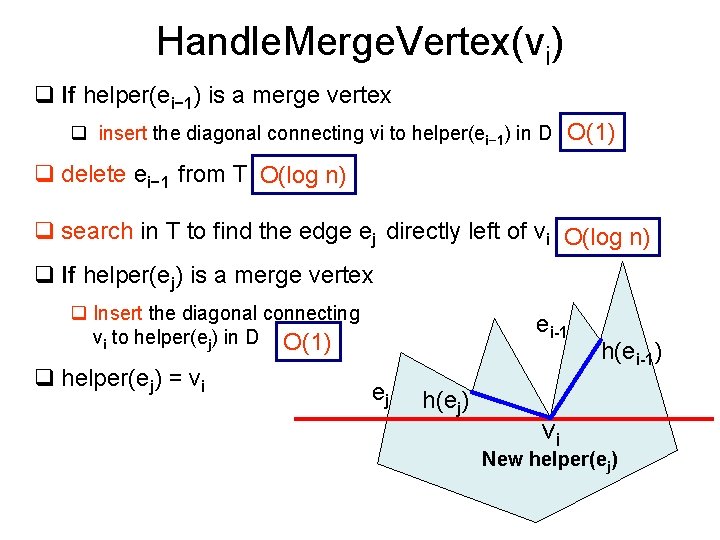
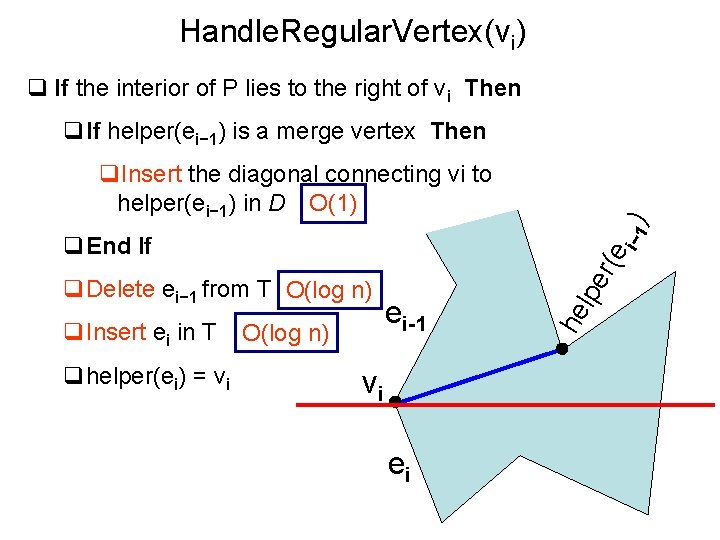
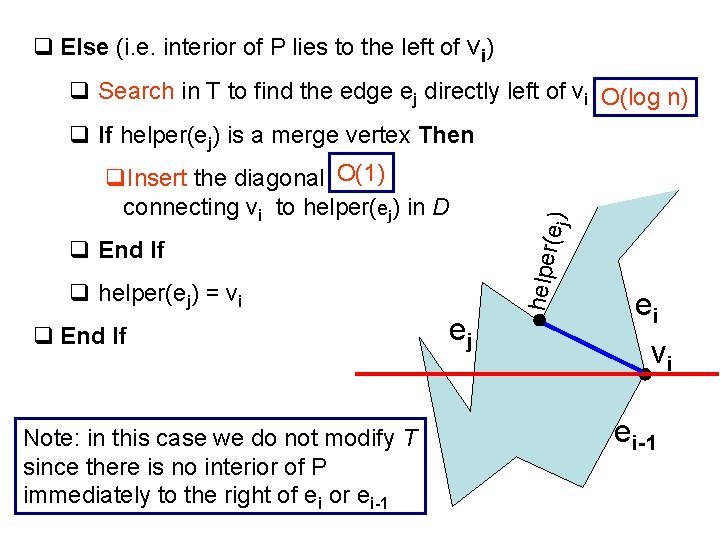
- Slides: 37
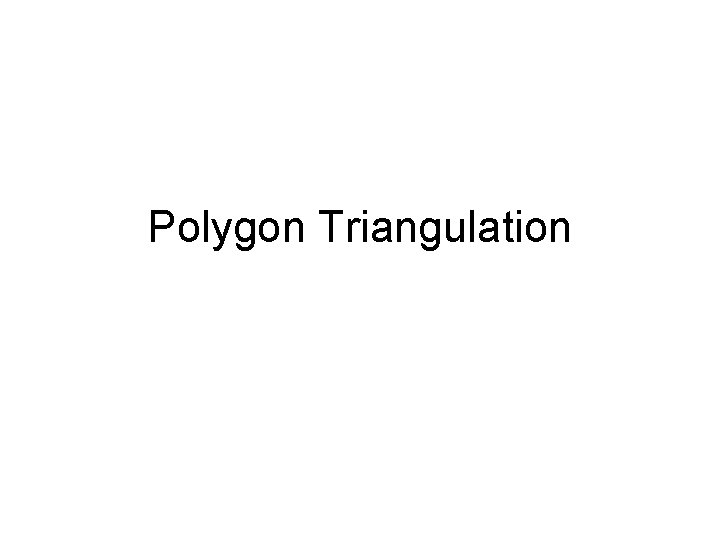
Polygon Triangulation
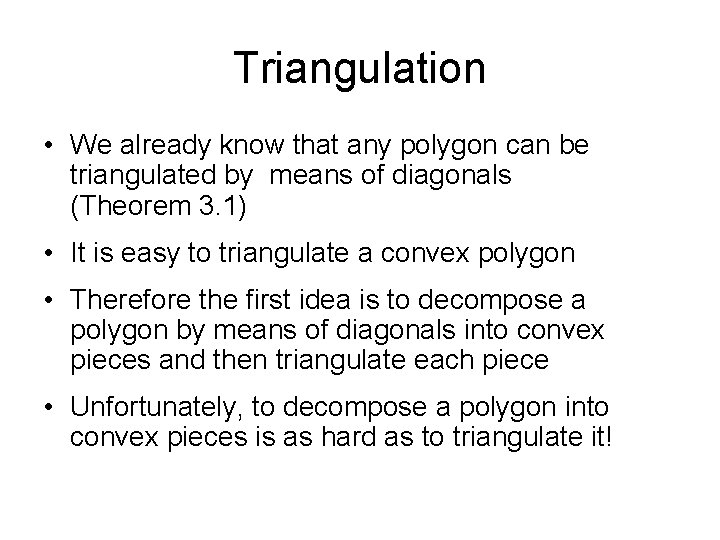
Triangulation • We already know that any polygon can be triangulated by means of diagonals (Theorem 3. 1) • It is easy to triangulate a convex polygon • Therefore the first idea is to decompose a polygon by means of diagonals into convex pieces and then triangulate each piece • Unfortunately, to decompose a polygon into convex pieces is as hard as to triangulate it!
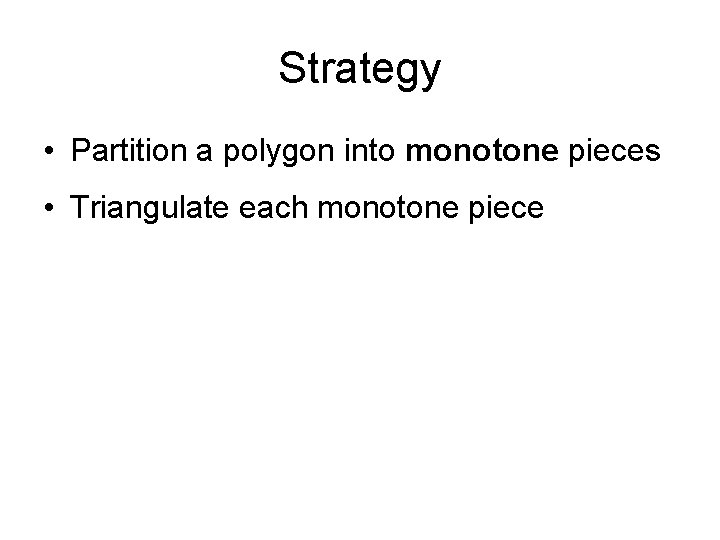
Strategy • Partition a polygon into monotone pieces • Triangulate each monotone piece
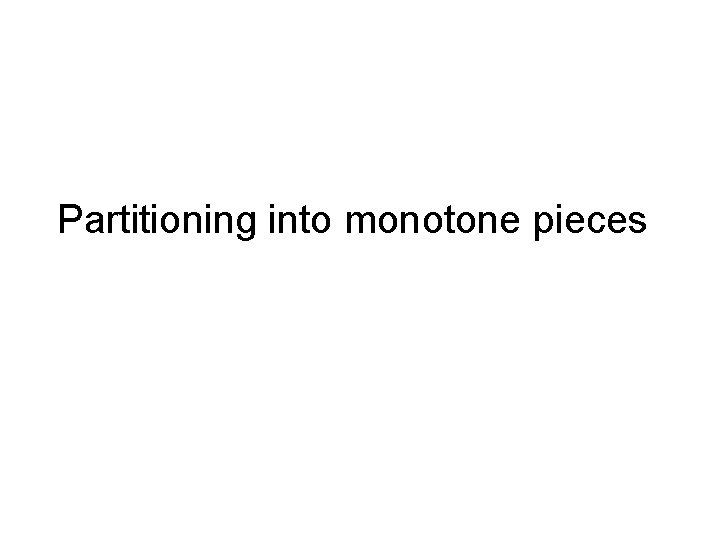
Partitioning into monotone pieces
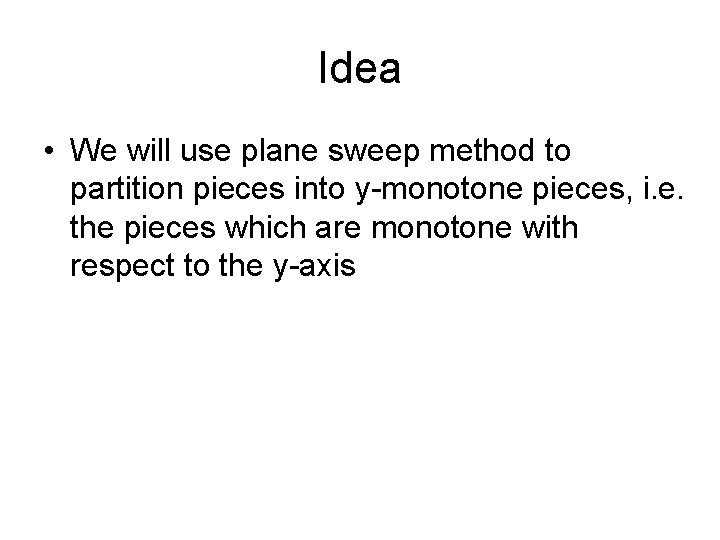
Idea • We will use plane sweep method to partition pieces into y-monotone pieces, i. e. the pieces which are monotone with respect to the y-axis
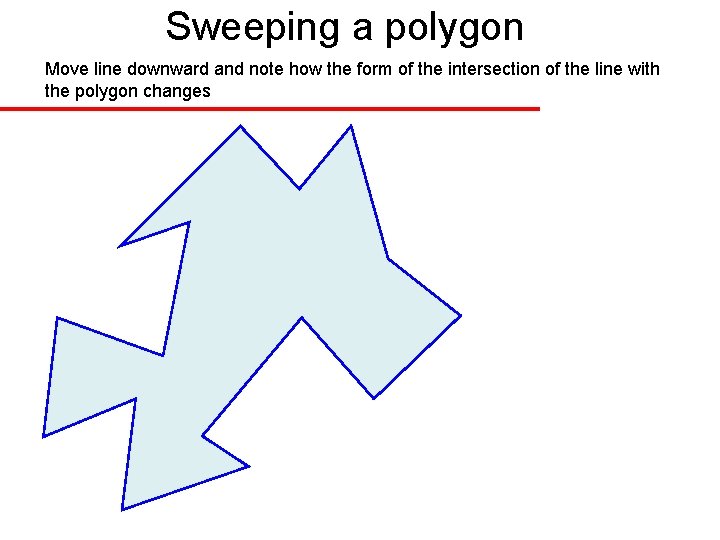
Sweeping a polygon Move line downward and note how the form of the intersection of the line with the polygon changes
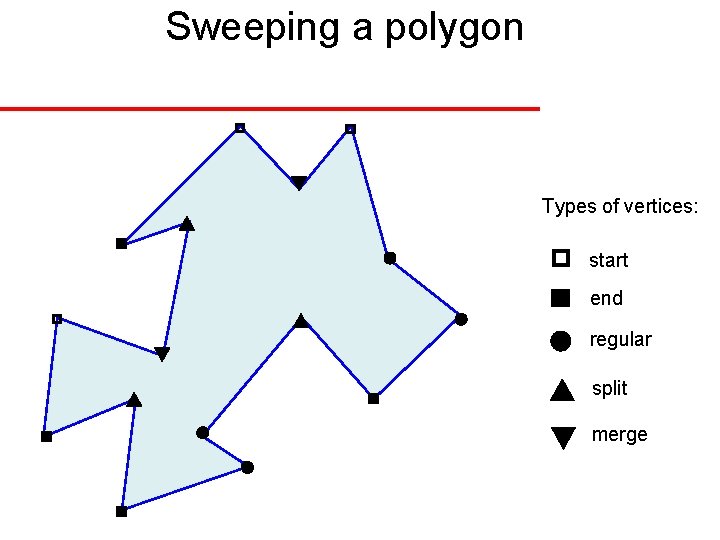
Sweeping a polygon Types of vertices: start end regular split merge
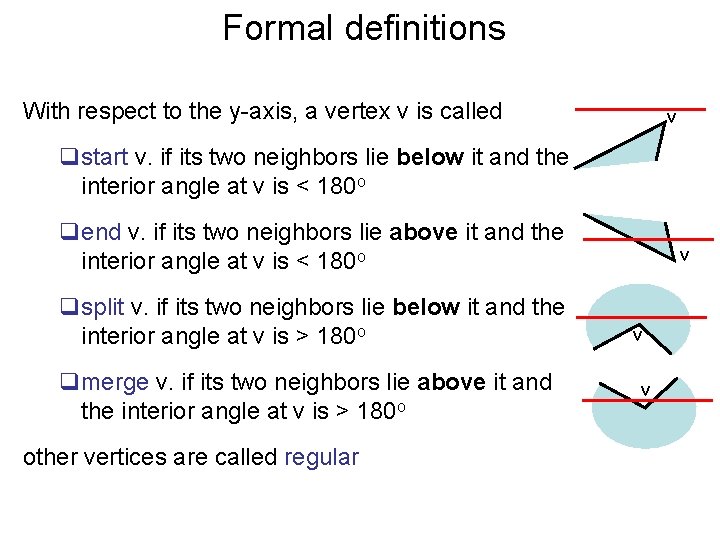
Formal definitions With respect to the y-axis, a vertex v is called v qstart v. if its two neighbors lie below it and the interior angle at v is < 180 o qend v. if its two neighbors lie above it and the interior angle at v is < 180 o qsplit v. if its two neighbors lie below it and the interior angle at v is > 180 o qmerge v. if its two neighbors lie above it and the interior angle at v is > 180 o other vertices are called regular v v v
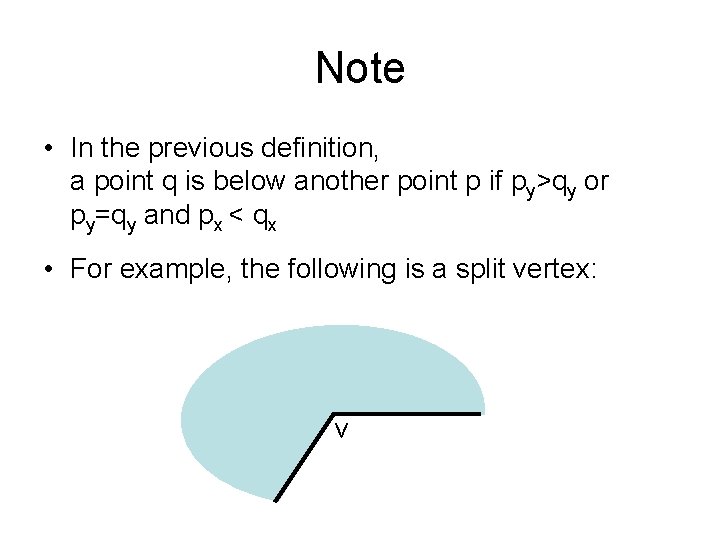
Note • In the previous definition, a point q is below another point p if py>qy or py=qy and px < qx • For example, the following is a split vertex: v
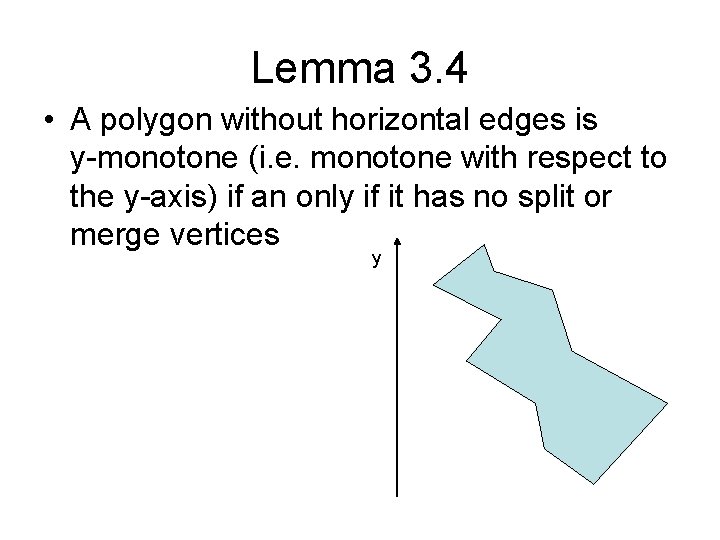
Lemma 3. 4 • A polygon without horizontal edges is y-monotone (i. e. monotone with respect to the y-axis) if an only if it has no split or merge vertices y
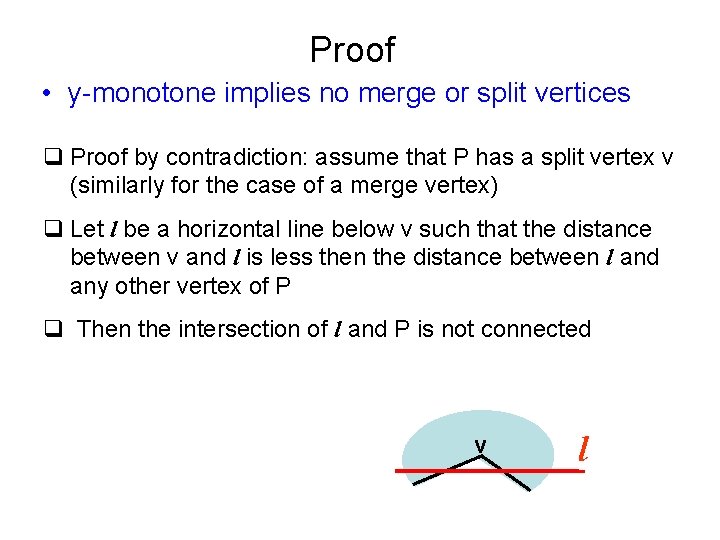
Proof • y-monotone implies no merge or split vertices q Proof by contradiction: assume that P has a split vertex v (similarly for the case of a merge vertex) q Let l be a horizontal line below v such that the distance between v and l is less then the distance between l and any other vertex of P q Then the intersection of l and P is not connected v l
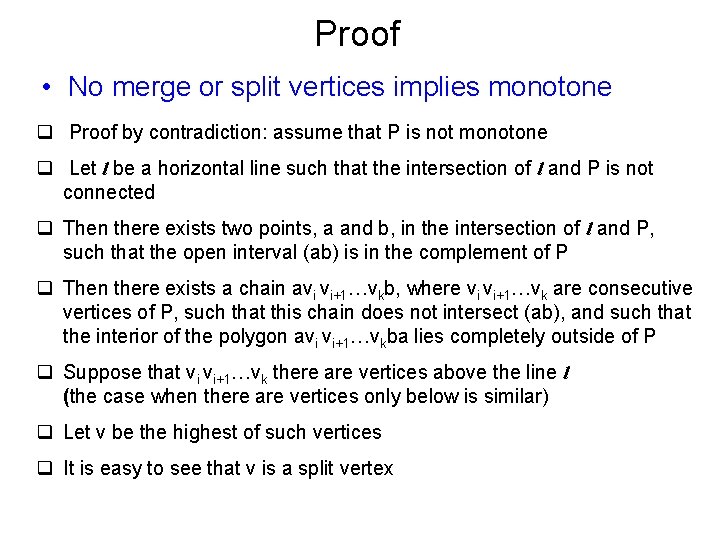
Proof • No merge or split vertices implies monotone q Proof by contradiction: assume that P is not monotone q Let l be a horizontal line such that the intersection of l and P is not connected q Then there exists two points, a and b, in the intersection of l and P, such that the open interval (ab) is in the complement of P q Then there exists a chain avi vi+1…vkb, where vi vi+1…vk are consecutive vertices of P, such that this chain does not intersect (ab), and such that the interior of the polygon avi vi+1…vkba lies completely outside of P q Suppose that vi vi+1…vk there are vertices above the line l (the case when there are vertices only below is similar) q Let v be the highest of such vertices q It is easy to see that v is a split vertex
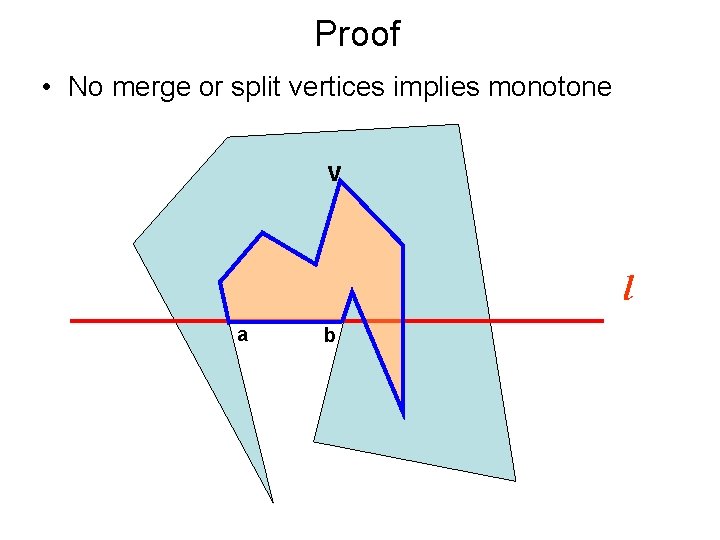
Proof • No merge or split vertices implies monotone v l a b
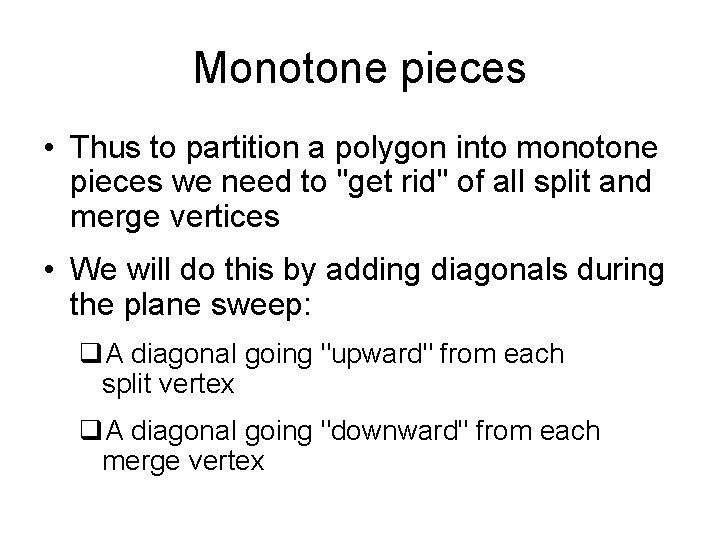
Monotone pieces • Thus to partition a polygon into monotone pieces we need to "get rid" of all split and merge vertices • We will do this by adding diagonals during the plane sweep: q. A diagonal going "upward" from each split vertex q. A diagonal going "downward" from each merge vertex
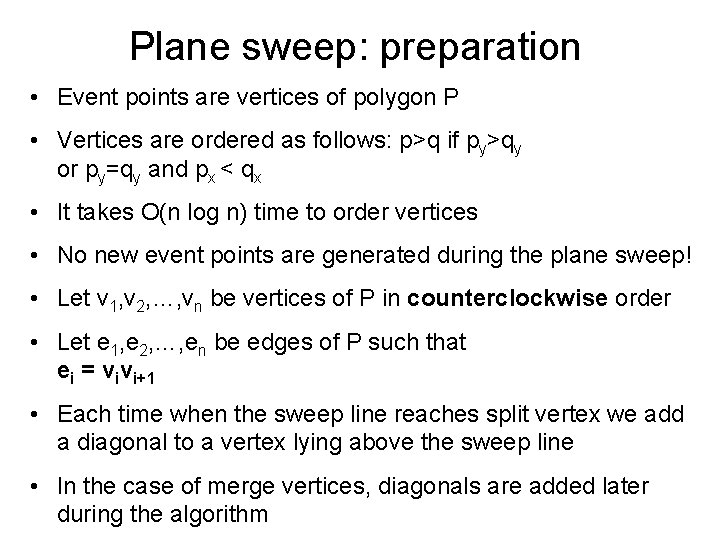
Plane sweep: preparation • Event points are vertices of polygon P • Vertices are ordered as follows: p>q if py>qy or py=qy and px < qx • It takes O(n log n) time to order vertices • No new event points are generated during the plane sweep! • Let v 1, v 2, …, vn be vertices of P in counterclockwise order • Let e 1, e 2, …, en be edges of P such that ei = vivi+1 • Each time when the sweep line reaches split vertex we add a diagonal to a vertex lying above the sweep line • In the case of merge vertices, diagonals are added later during the algorithm
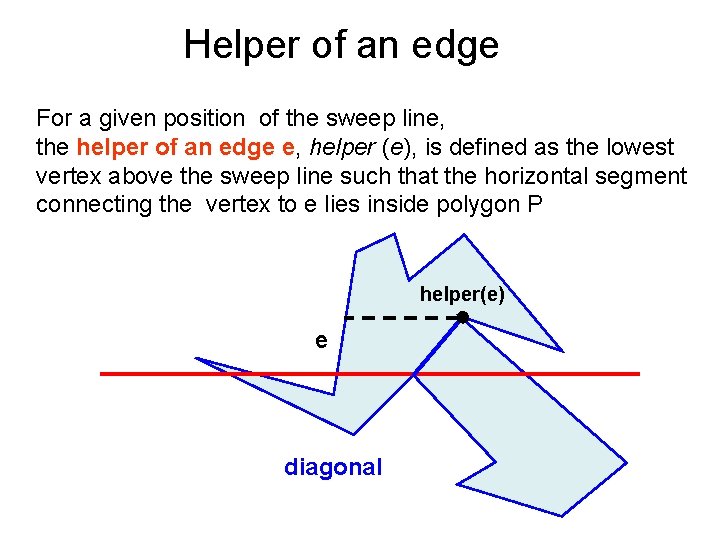
Helper of an edge For a given position of the sweep line, the helper of an edge e, helper (e), is defined as the lowest vertex above the sweep line such that the horizontal segment connecting the vertex to e lies inside polygon P helper(e) e diagonal
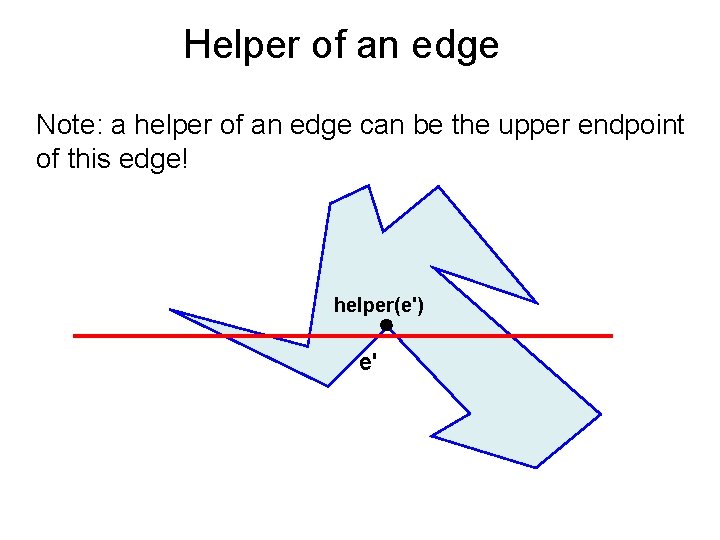
Helper of an edge Note: a helper of an edge can be the upper endpoint of this edge! helper(e') e'
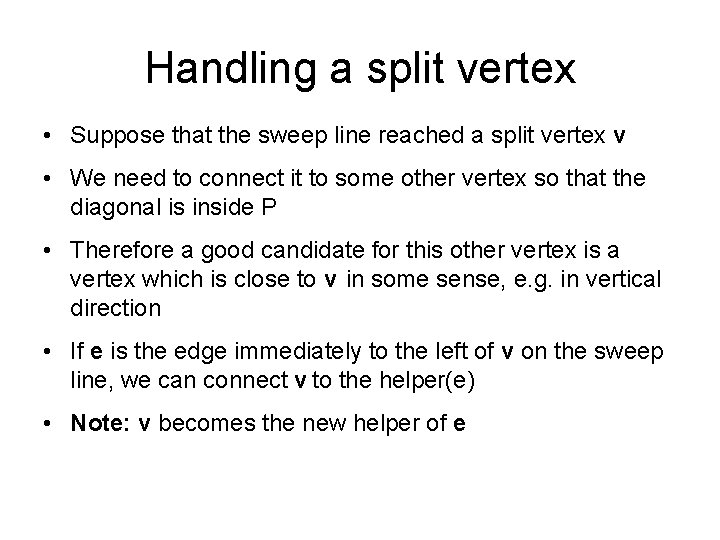
Handling a split vertex • Suppose that the sweep line reached a split vertex v • We need to connect it to some other vertex so that the diagonal is inside P • Therefore a good candidate for this other vertex is a vertex which is close to v in some sense, e. g. in vertical direction • If e is the edge immediately to the left of v on the sweep line, we can connect v to the helper(e) • Note: v becomes the new helper of e
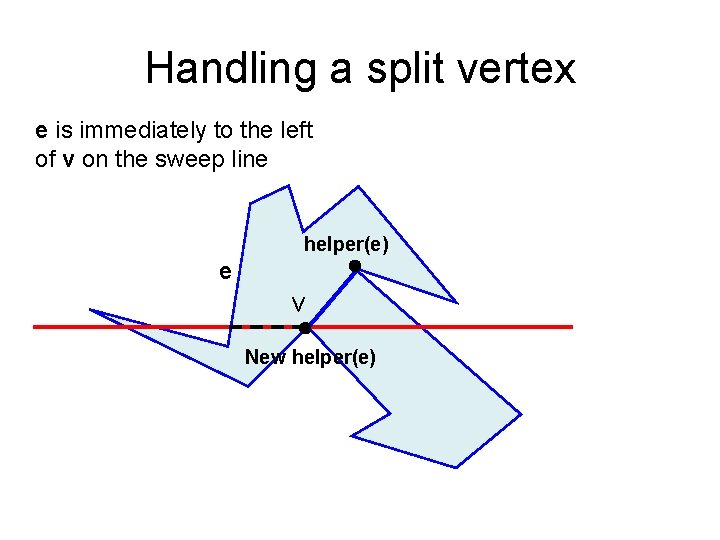
Handling a split vertex e is immediately to the left of v on the sweep line helper(e) e v New helper(e)
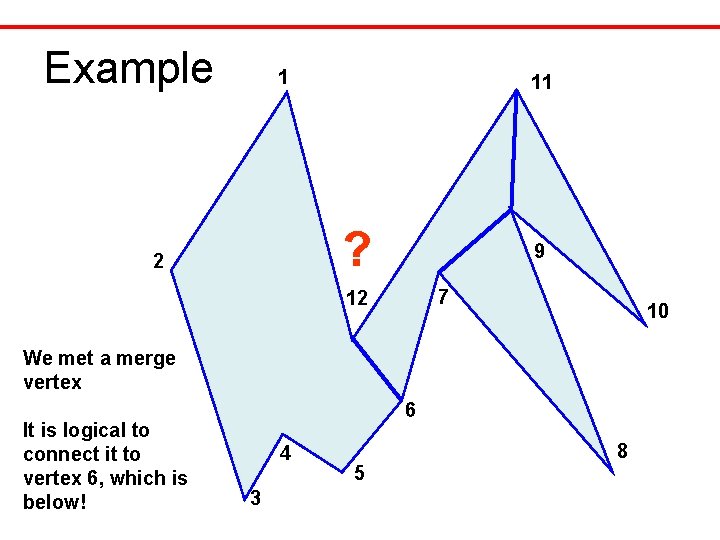
Example 1 11 ? 2 9 7 12 10 We met a merge vertex It is logical to connect it to vertex 6, which is below! 6 4 3 5 8
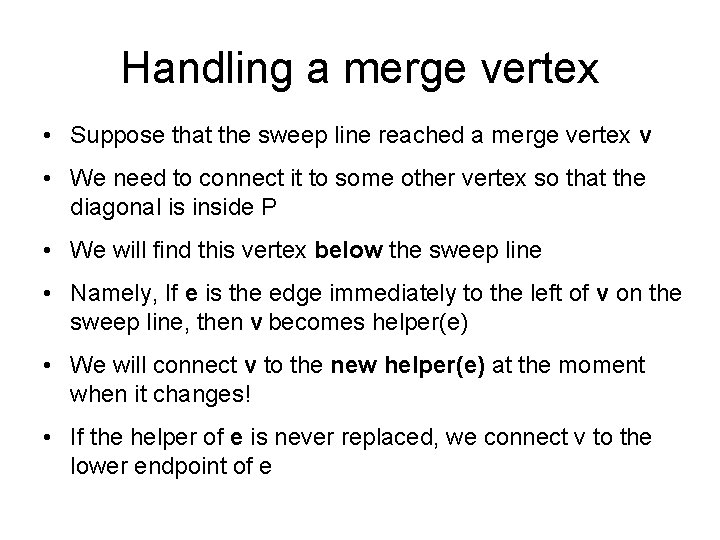
Handling a merge vertex • Suppose that the sweep line reached a merge vertex v • We need to connect it to some other vertex so that the diagonal is inside P • We will find this vertex below the sweep line • Namely, If e is the edge immediately to the left of v on the sweep line, then v becomes helper(e) • We will connect v to the new helper(e) at the moment when it changes! • If the helper of e is never replaced, we connect v to the lower endpoint of e
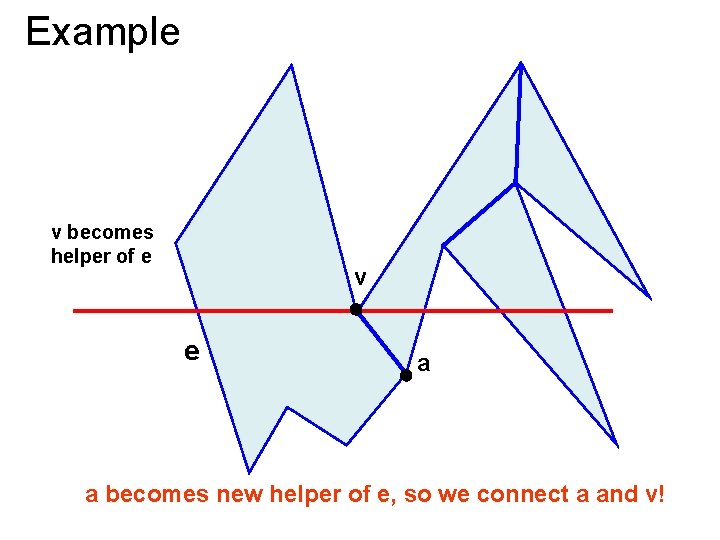
Example v becomes helper of e v e a a becomes new helper of e, so we connect a and v!
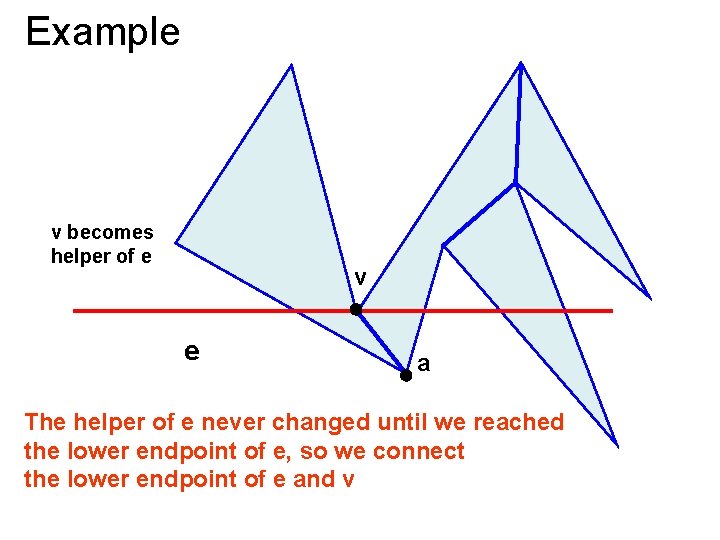
Example v becomes helper of e v e a The helper of e never changed until we reached the lower endpoint of e, so we connect the lower endpoint of e and v
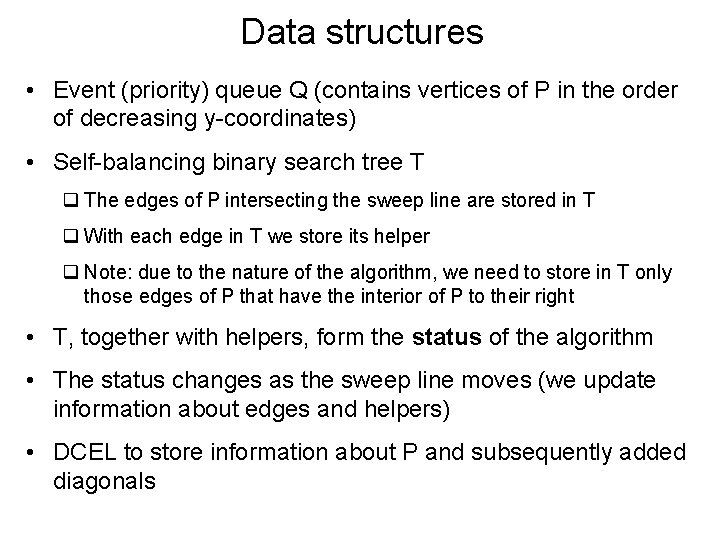
Data structures • Event (priority) queue Q (contains vertices of P in the order of decreasing y-coordinates) • Self-balancing binary search tree T q The edges of P intersecting the sweep line are stored in T q With each edge in T we store its helper q Note: due to the nature of the algorithm, we need to store in T only those edges of P that have the interior of P to their right • T, together with helpers, form the status of the algorithm • The status changes as the sweep line moves (we update information about edges and helpers) • DCEL to store information about P and subsequently added diagonals
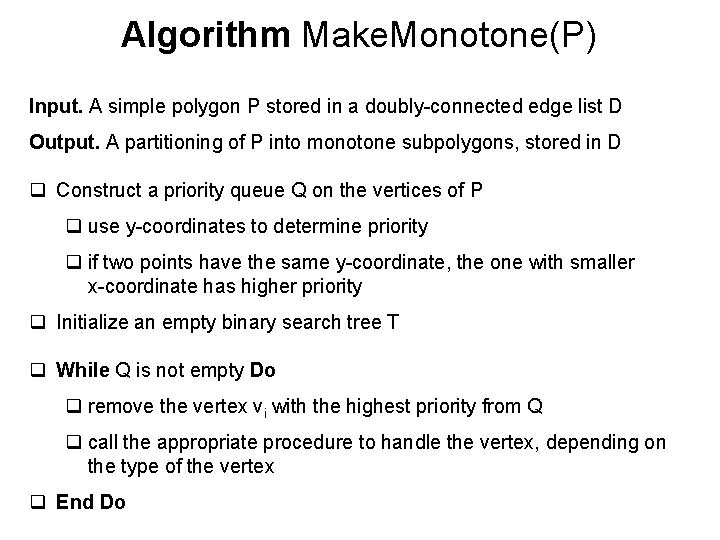
Algorithm Make. Monotone(P) Input. A simple polygon P stored in a doubly-connected edge list D Output. A partitioning of P into monotone subpolygons, stored in D q Construct a priority queue Q on the vertices of P q use y-coordinates to determine priority q if two points have the same y-coordinate, the one with smaller x-coordinate has higher priority q Initialize an empty binary search tree T q While Q is not empty Do q remove the vertex vi with the highest priority from Q q call the appropriate procedure to handle the vertex, depending on the type of the vertex q End Do
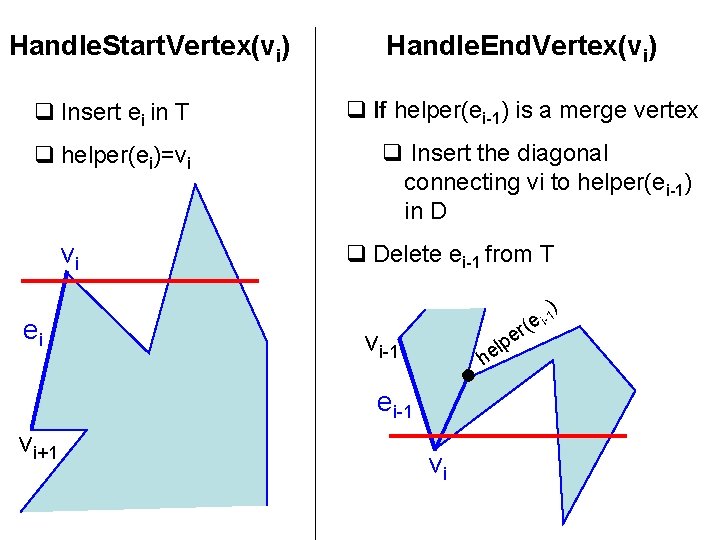
Handle. Start. Vertex(vi) Handle. End. Vertex(vi) q Insert ei in T q If helper(ei-1) is a merge vertex q helper(ei)=vi q Insert the diagonal connecting vi to helper(ei-1) in D vi ei q Delete ei-1 from T ( r e p l vi-1 he ei-1 vi+1 vi ) e i-1
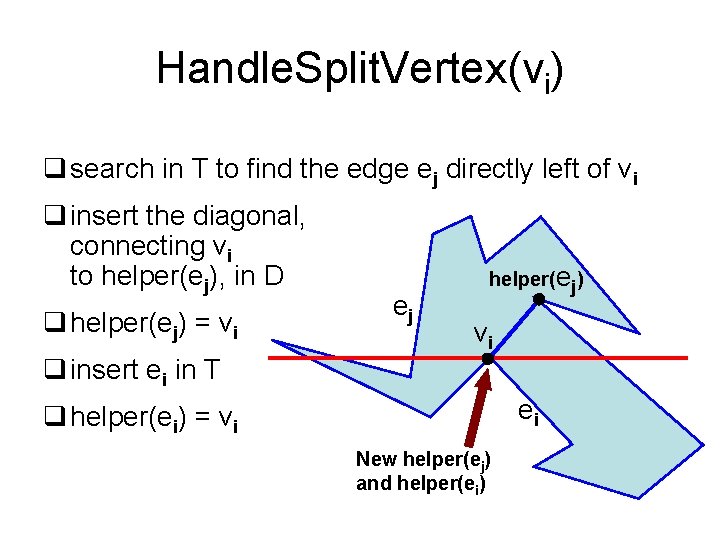
Handle. Split. Vertex(vi) q search in T to find the edge ej directly left of vi q insert the diagonal, connecting vi to helper(ej), in D q helper(ej) = vi ej helper(ej) vi q insert ei in T ei q helper(ei) = vi New helper(ej) and helper(ei)
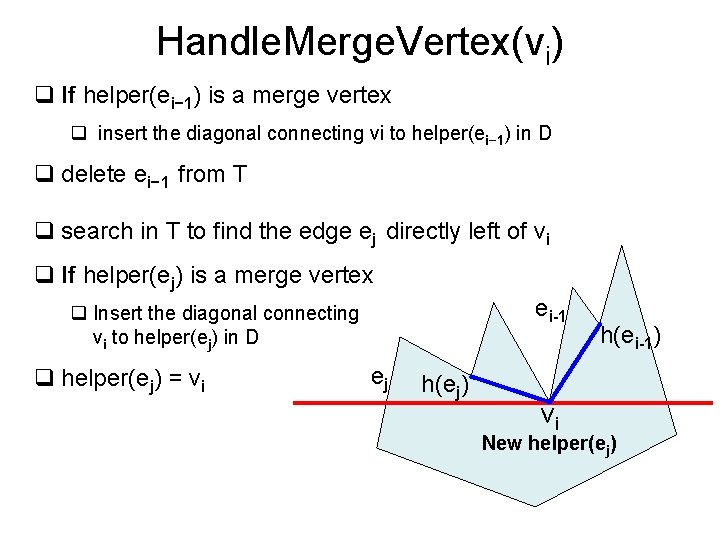
Handle. Merge. Vertex(vi) q If helper(ei− 1) is a merge vertex q insert the diagonal connecting vi to helper(ei− 1) in D q delete ei− 1 from T q search in T to find the edge ej directly left of vi q If helper(ej) is a merge vertex ei-1 q Insert the diagonal connecting vi to helper(ej) in D q helper(ej) = vi ej h(ej) vi h(ei-1) New helper(ej)
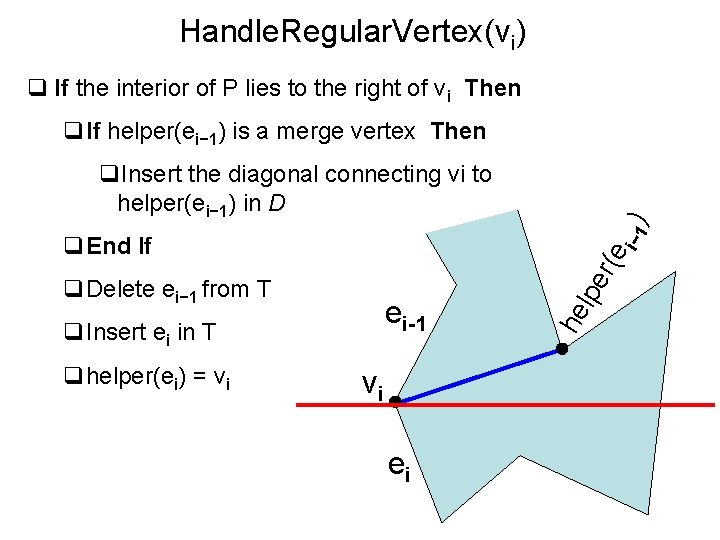
Handle. Regular. Vertex(vi) q If the interior of P lies to the right of vi Then q. If helper(ei− 1) is a merge vertex Then ei-1 q. Insert ei in T qhelper(ei) = vi vi ei he q. Delete ei− 1 from T lpe r(e q. End If i− 1 ) q. Insert the diagonal connecting vi to helper(ei− 1) in D
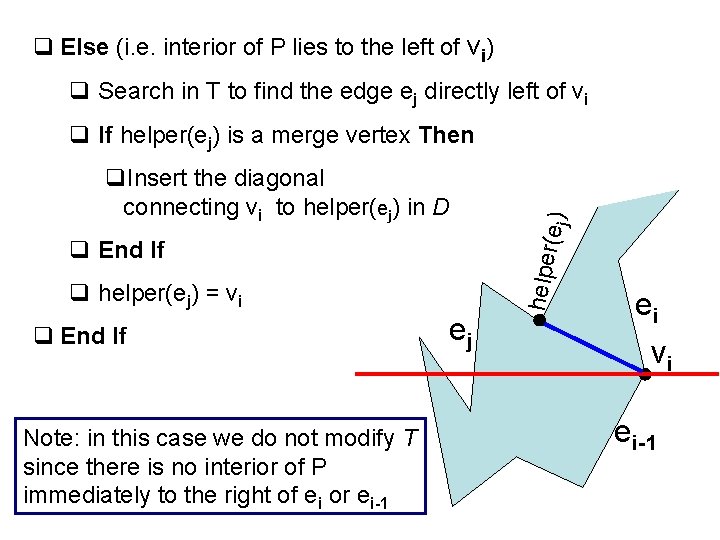
q Else (i. e. interior of P lies to the left of vi) q Search in T to find the edge ej directly left of vi q End If q helper(ej) = vi q End If Note: in this case we do not modify T since there is no interior of P immediately to the right of ei or ei-1 ej helpe q. Insert the diagonal connecting vi to helper(ej) in D r(ej ) q If helper(ej) is a merge vertex Then ei vi ei-1
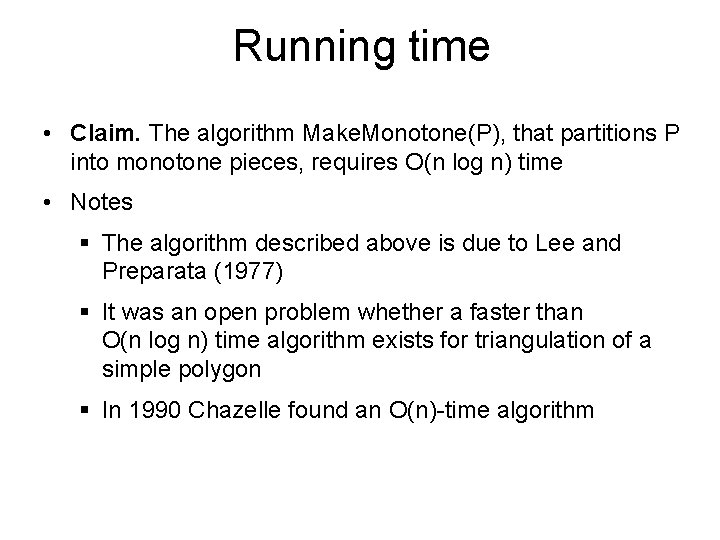
Running time • Claim. The algorithm Make. Monotone(P), that partitions P into monotone pieces, requires O(n log n) time • Notes § The algorithm described above is due to Lee and Preparata (1977) § It was an open problem whether a faster than O(n log n) time algorithm exists for triangulation of a simple polygon § In 1990 Chazelle found an O(n)-time algorithm
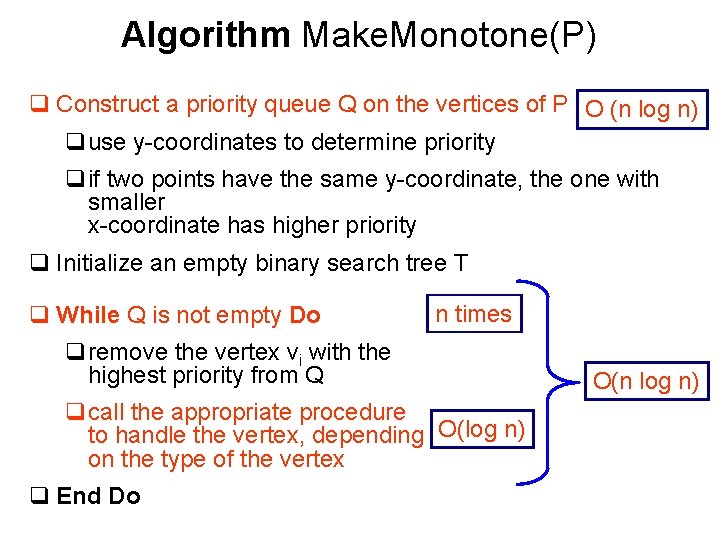
Algorithm Make. Monotone(P) q Construct a priority queue Q on the vertices of P O (n log n) quse y-coordinates to determine priority qif two points have the same y-coordinate, the one with smaller x-coordinate has higher priority q Initialize an empty binary search tree T q While Q is not empty Do n times qremove the vertex vi with the highest priority from Q qcall the appropriate procedure to handle the vertex, depending O(log n) on the type of the vertex q End Do O(n log n)
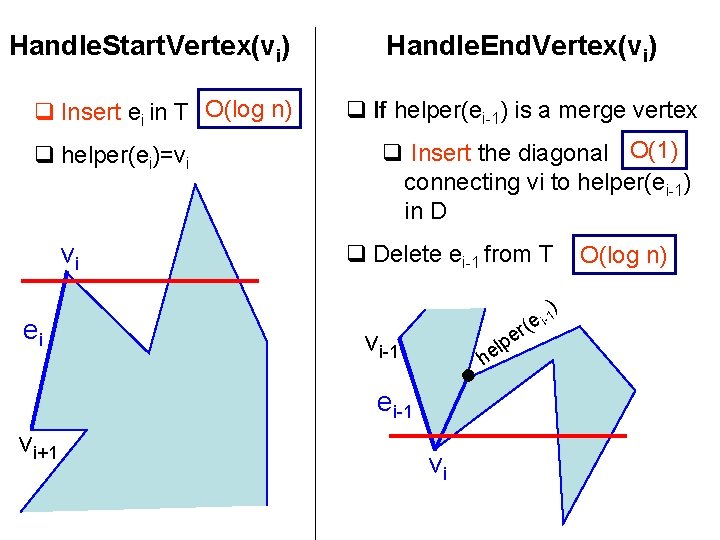
Handle. Start. Vertex(vi) q Insert ei in T O(log n) q helper(ei)=vi vi ei Handle. End. Vertex(vi) q If helper(ei-1) is a merge vertex q Insert the diagonal O(1) connecting vi to helper(ei-1) in D q Delete ei-1 from T vi-1 he ei-1 vi+1 vi ) e i-1 ( r e p l O(log n)
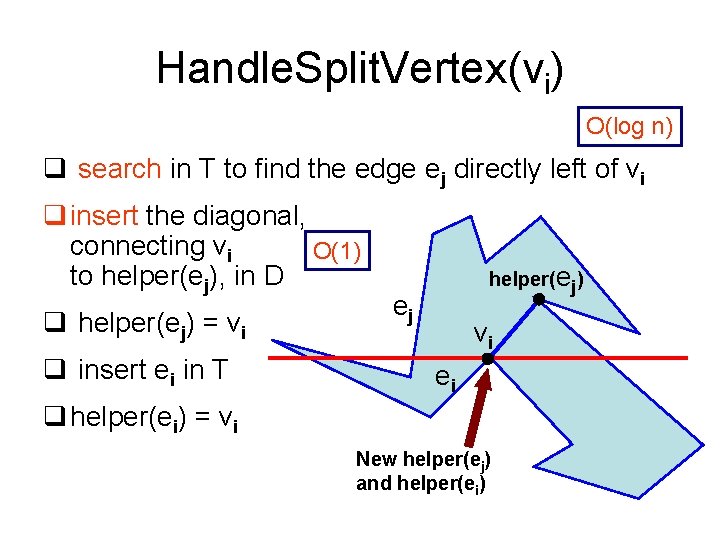
Handle. Split. Vertex(vi) O(log n) q search in T to find the edge ej directly left of vi q insert the diagonal, connecting vi O(1) to helper(ej), in D q helper(ej) = vi q insert ei in T helper(ej) ej vi ei q helper(ei) = vi New helper(ej) and helper(ei)
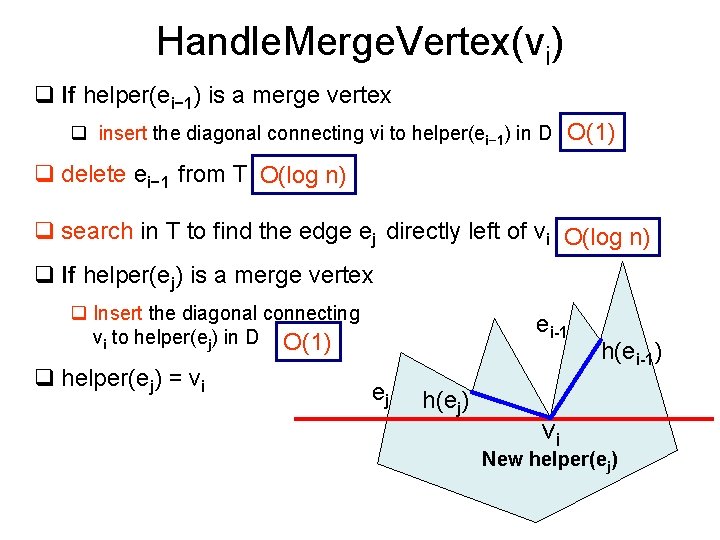
Handle. Merge. Vertex(vi) q If helper(ei− 1) is a merge vertex q insert the diagonal connecting vi to helper(ei− 1) in D O(1) q delete ei− 1 from T O(log n) q search in T to find the edge ej directly left of vi O(log n) q If helper(ej) is a merge vertex q Insert the diagonal connecting vi to helper(ej) in D O(1) q helper(ej) = vi ei-1 ej h(ej) vi h(ei-1) New helper(ej)
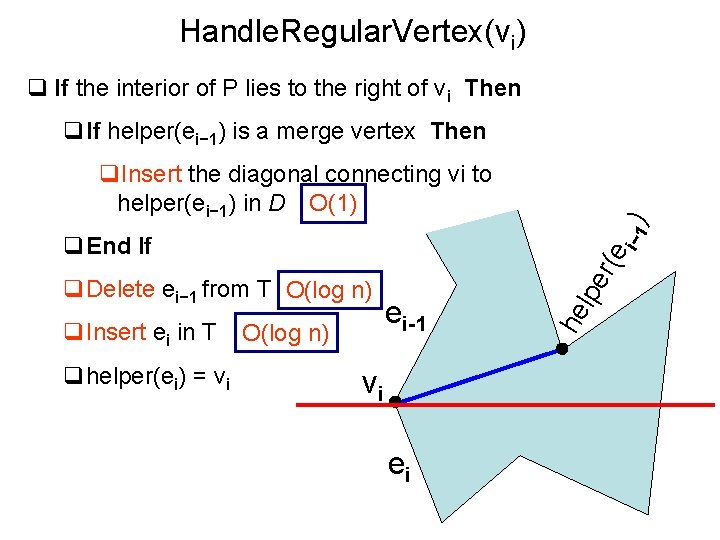
Handle. Regular. Vertex(vi) q If the interior of P lies to the right of vi Then q. If helper(ei− 1) is a merge vertex Then q. Insert ei in T qhelper(ei) = vi O(log n) vi ei lpe ei-1 he q. Delete ei− 1 from T O(log n) r(e q. End If i− 1 ) q. Insert the diagonal connecting vi to helper(ei− 1) in D O(1)
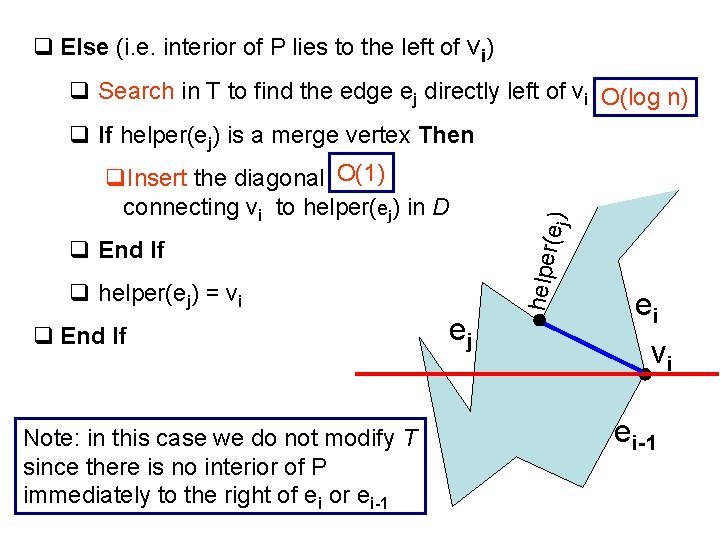
q Else (i. e. interior of P lies to the left of vi) q Search in T to find the edge ej directly left of vi O(log n) q End If q helper(ej) = vi q End If Note: in this case we do not modify T since there is no interior of P immediately to the right of ei or ei-1 ej helpe q. Insert the diagonal O(1) connecting vi to helper(ej) in D r(ej ) q If helper(ej) is a merge vertex Then ei vi ei-1
Talk en futuro
Monotone polygon triangulation
You already know future
How much do you already know about
What do we already know
Starter which muscles do you already know
What do you already know about rainforests?
What do you already know about volcanoes?
Lupe y guillermo mucho en la clase de arte
Lets see what you know
"as you already know"
Lets see what you already know
What do we already know
Parabola in standard form
Pronunciation poem i take it you already know
Lets see what you already know
Not polygon
Tôn thất thuyết là ai
Ngoại tâm thu thất chùm đôi
Walmart thất bại ở nhật
Gây tê cơ vuông thắt lưng
Block nhĩ thất độ 1
Tìm độ lớn thật của tam giác abc
Sau thất bại ở hồ điển triệt
Thể thơ truyền thống
Con hãy đưa tay khi thấy người vấp ngã
Thơ thất ngôn tứ tuyệt đường luật
3-sided
Parallelogram properties
I know who goes before me
"know history know self"
Normalizing flow
Informational probes adalah
There isn't any
Any to any connectivity
Triangulation police
Methodological triangulation
Triangulation of epicenter