LOOPS INGE 3016 Algorithms and Computer Programming with
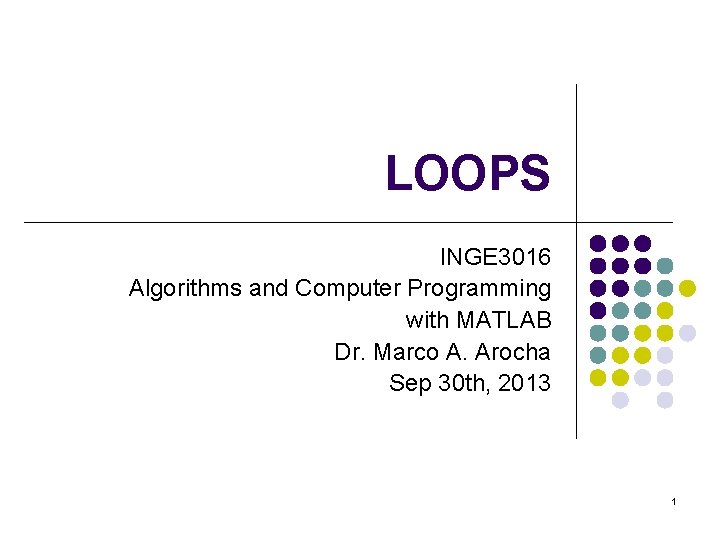
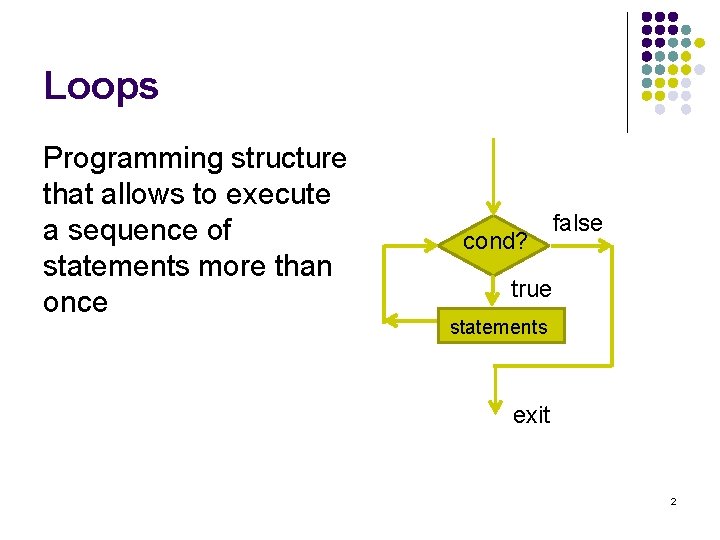
![Loops Two basic syntax forms: for cv=[list of values] while condition statements end cv=control Loops Two basic syntax forms: for cv=[list of values] while condition statements end cv=control](https://slidetodoc.com/presentation_image_h2/93177aa56fa3db444fdf5f6038f1962a/image-3.jpg)
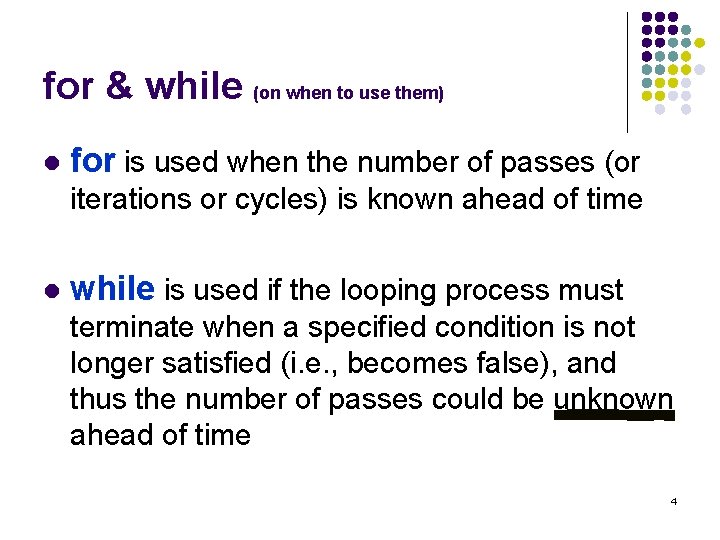
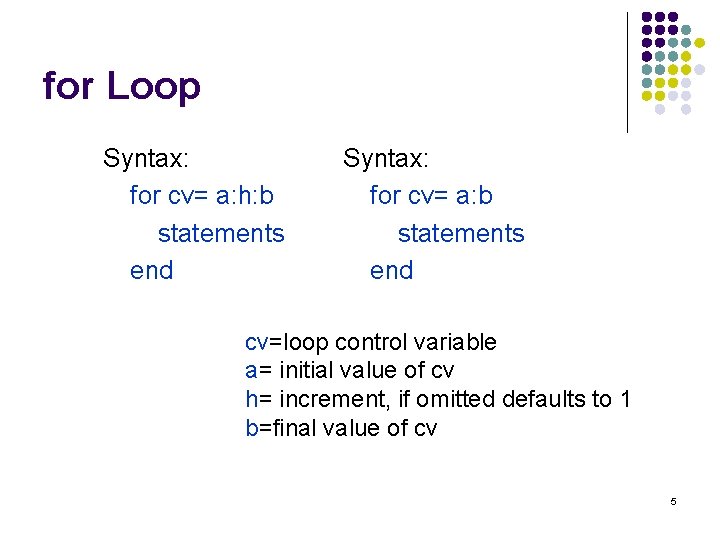
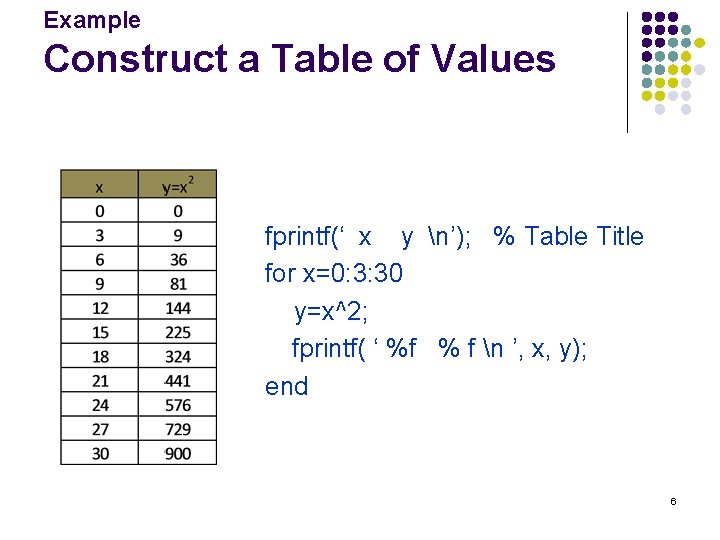
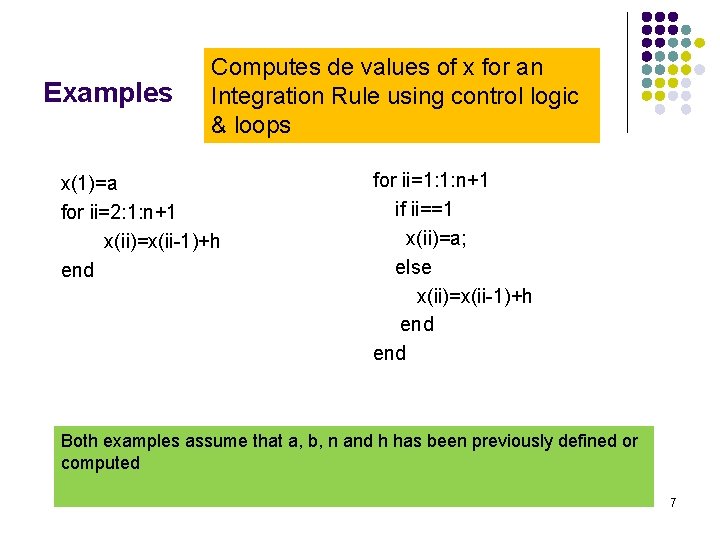
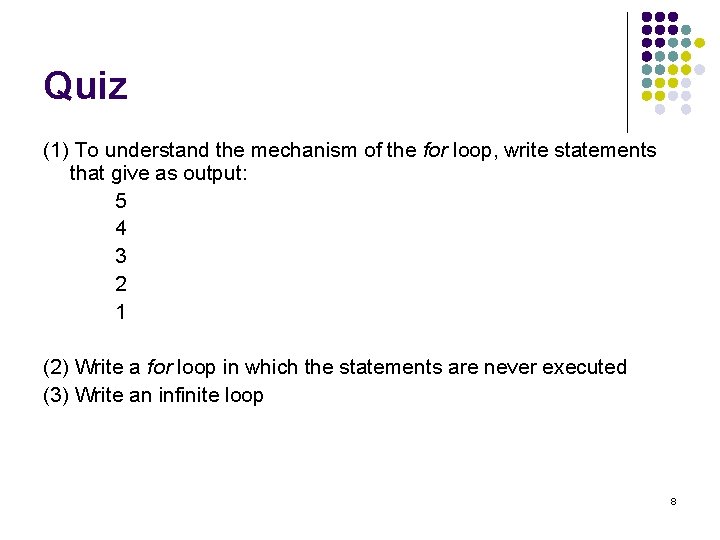
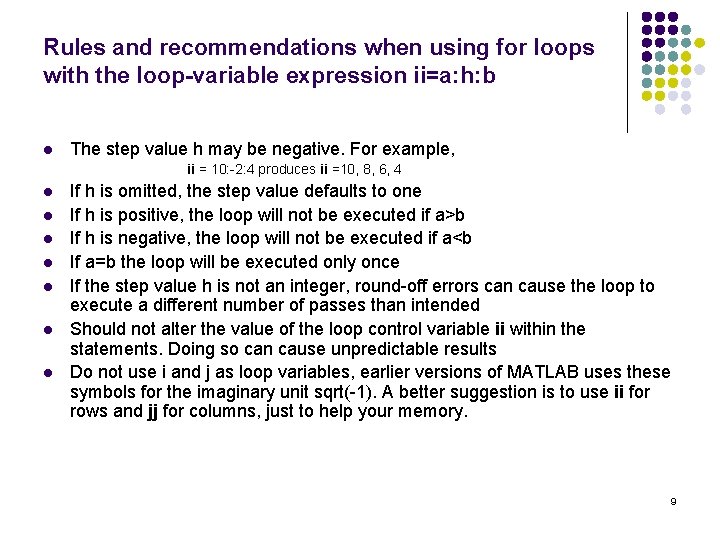
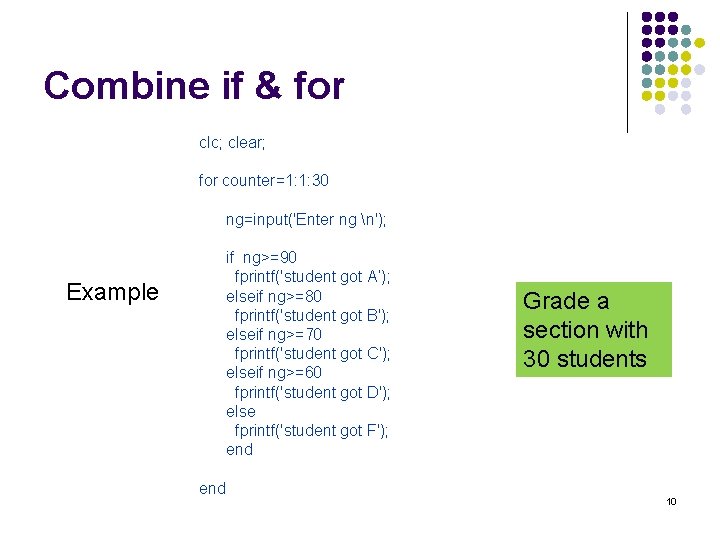
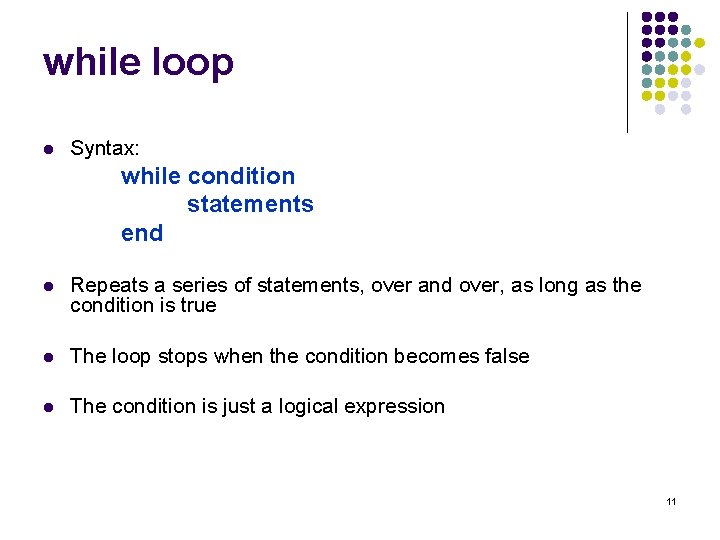
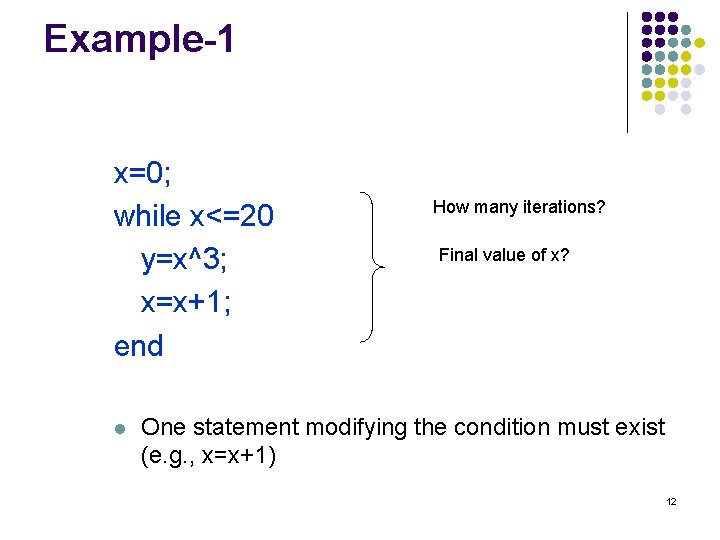
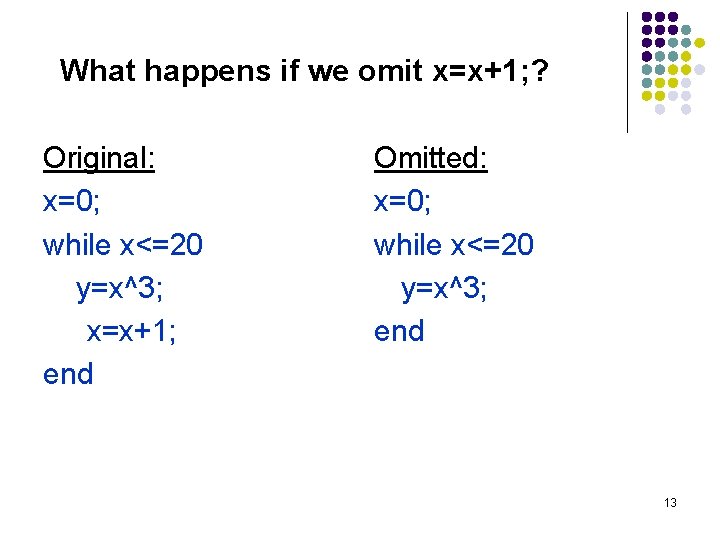
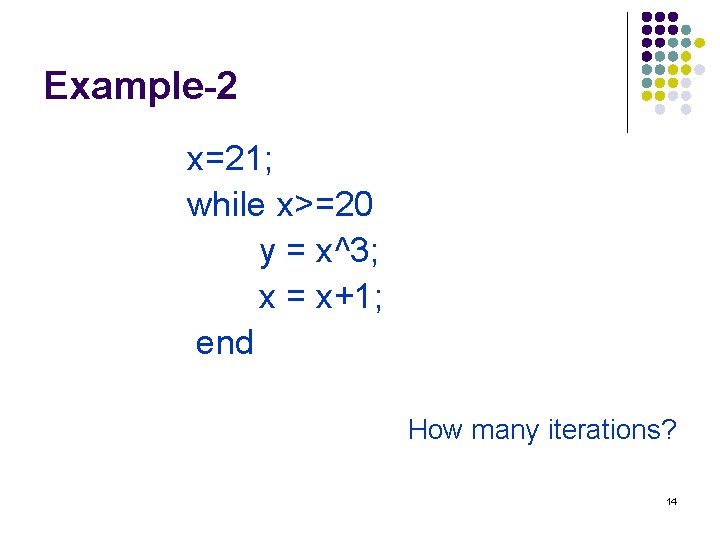
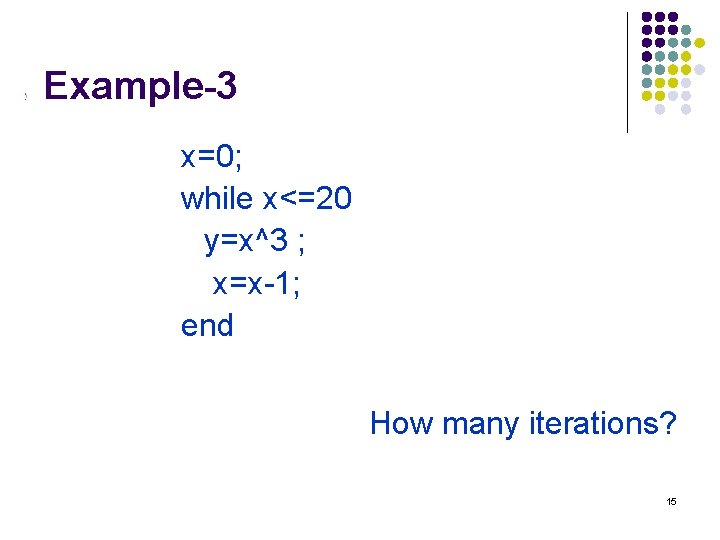
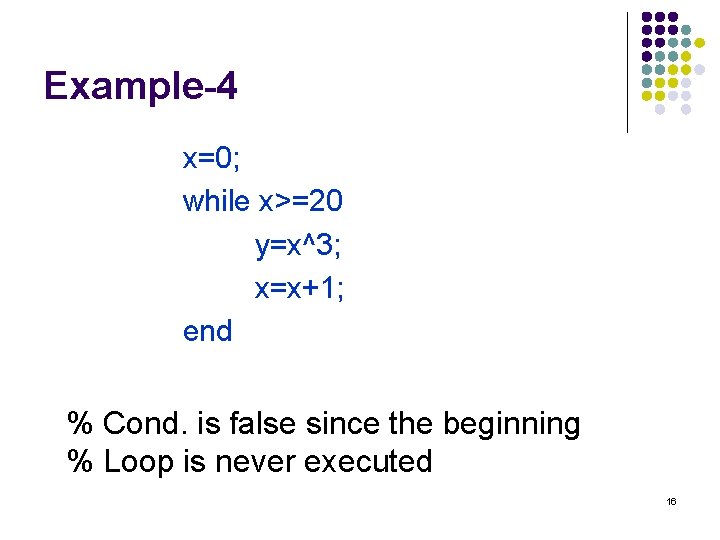
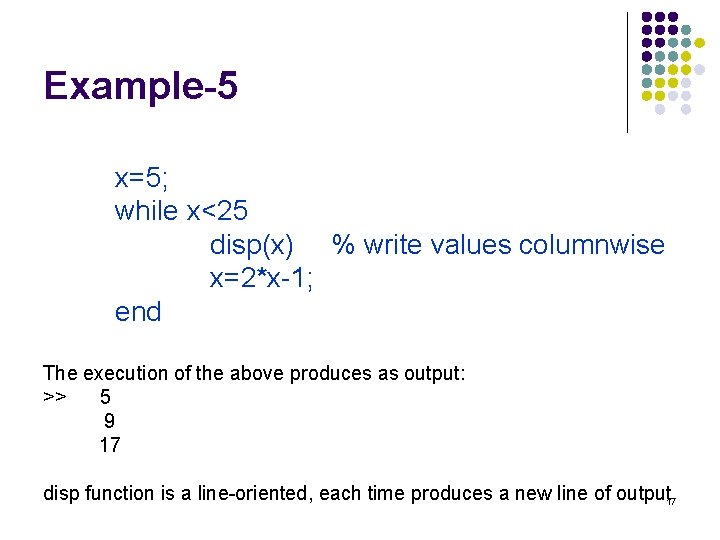
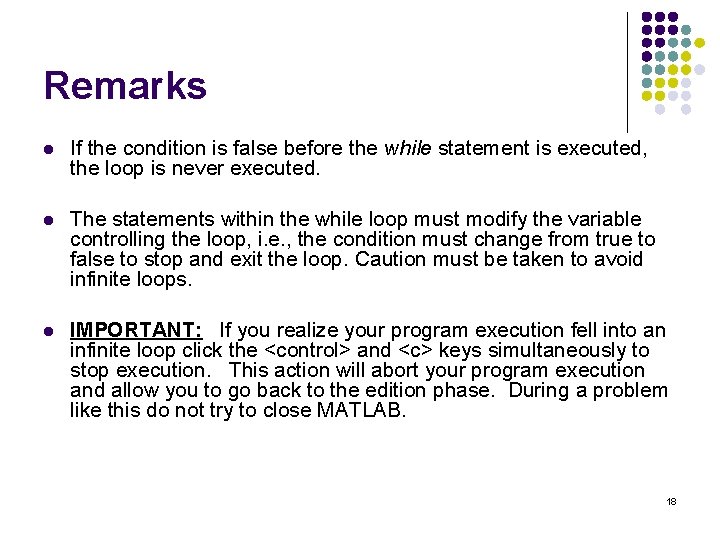
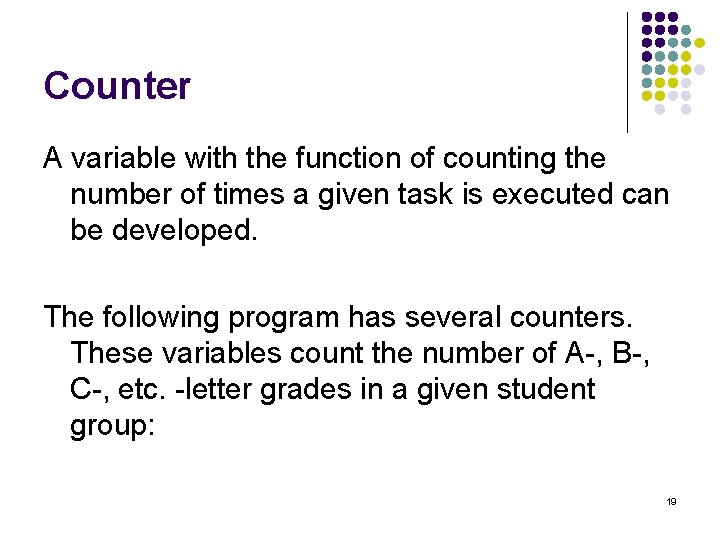
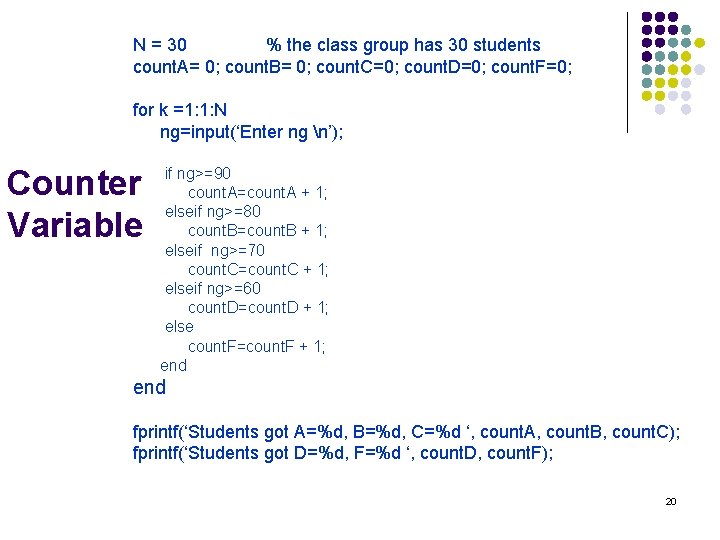
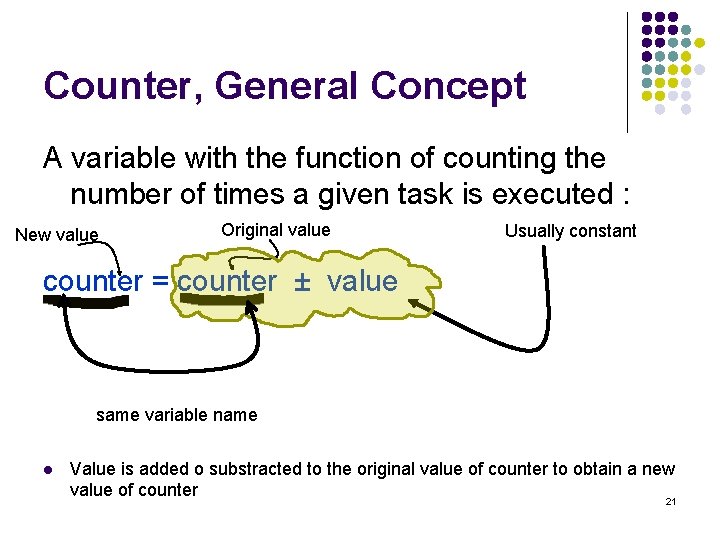
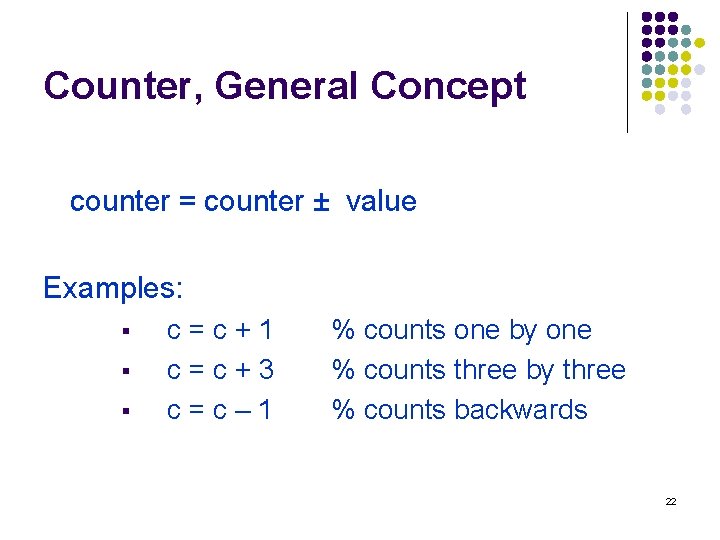
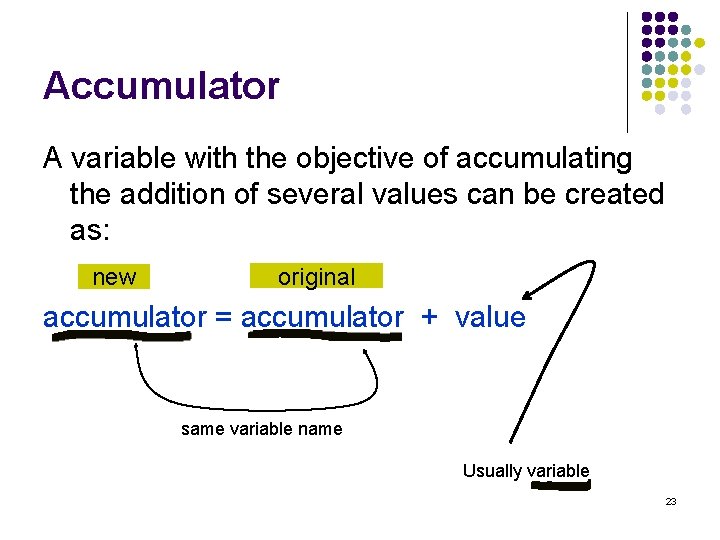
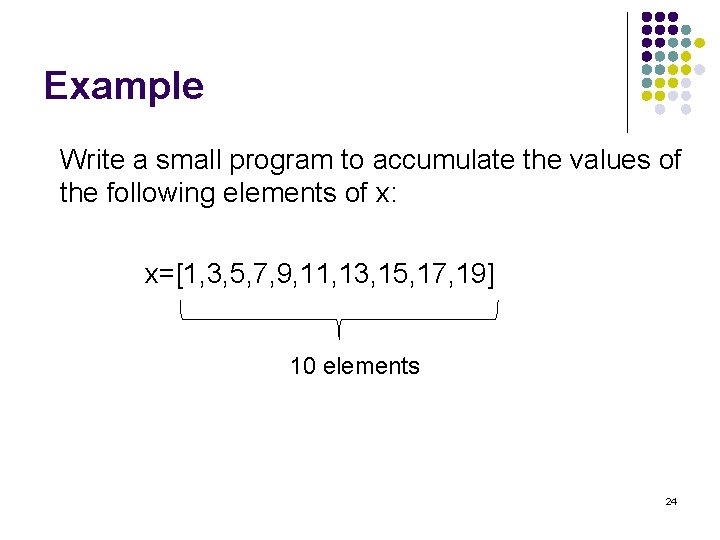
![Solution-1 x= [ 1, 3, 5, 7, 9, 11, 13, 15, 17, 19 ]; Solution-1 x= [ 1, 3, 5, 7, 9, 11, 13, 15, 17, 19 ];](https://slidetodoc.com/presentation_image_h2/93177aa56fa3db444fdf5f6038f1962a/image-25.jpg)
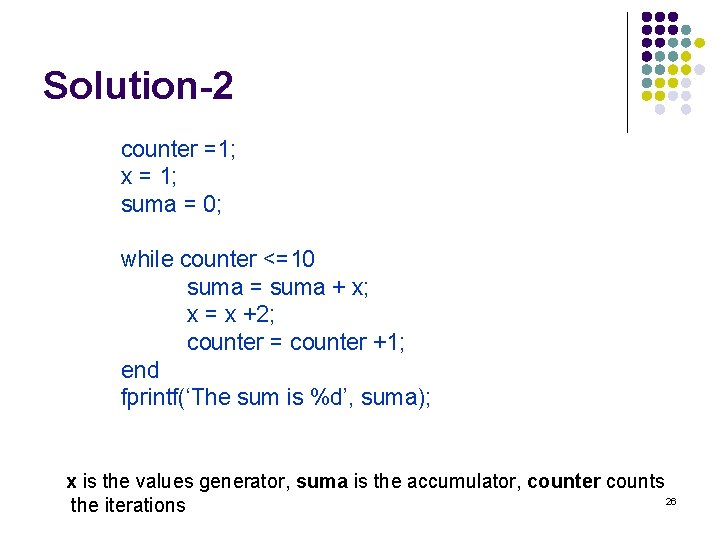
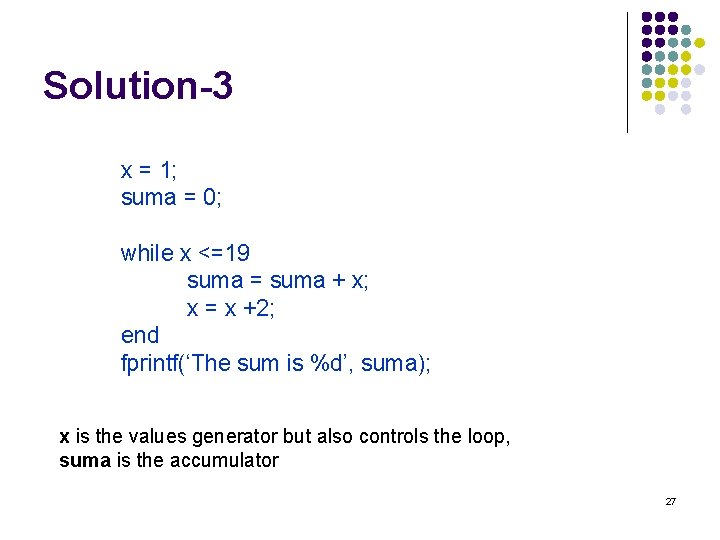
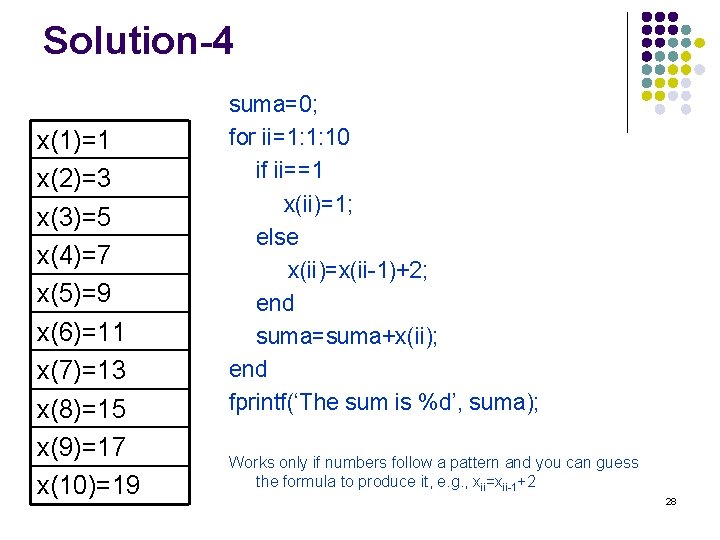
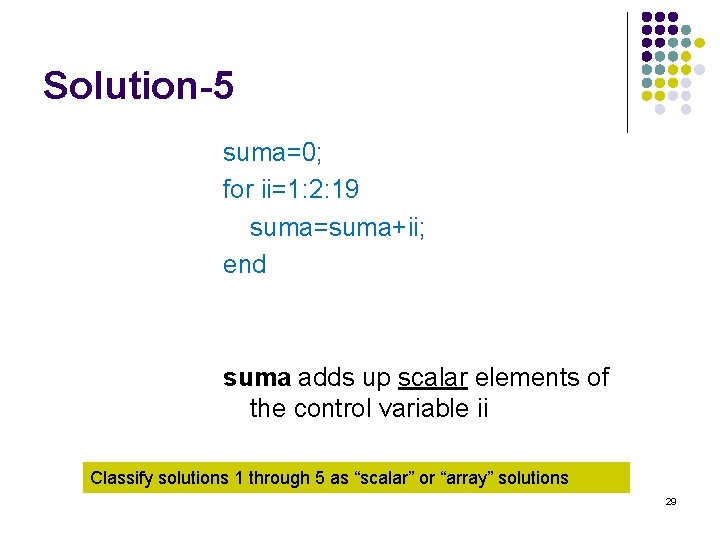
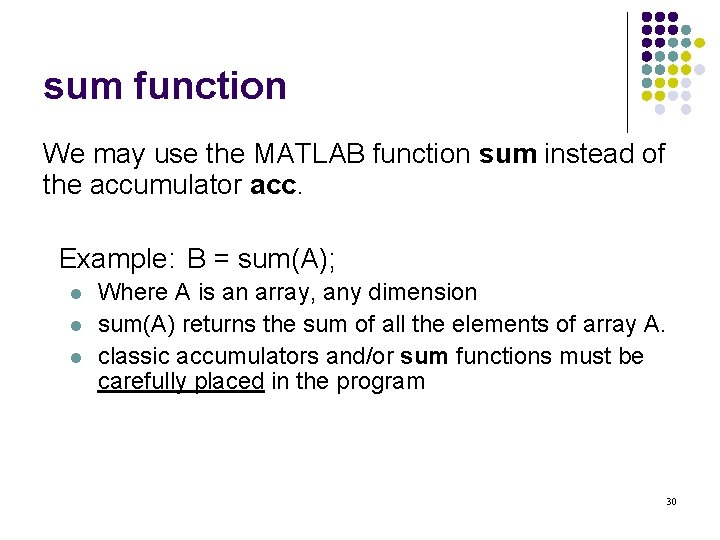
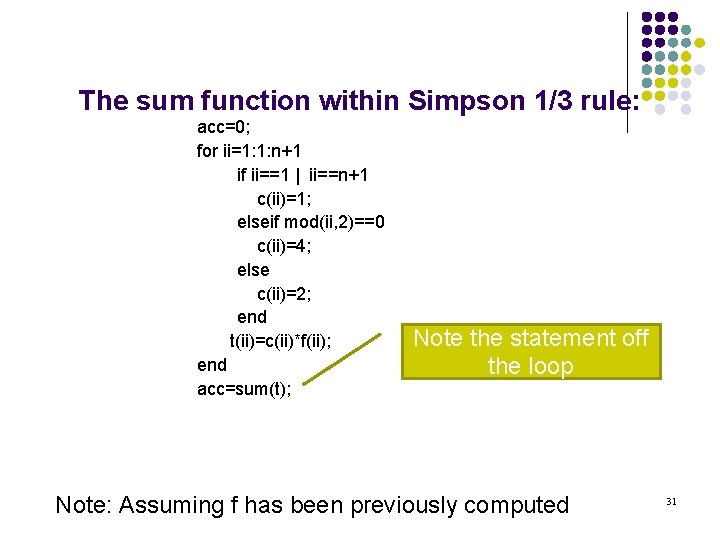
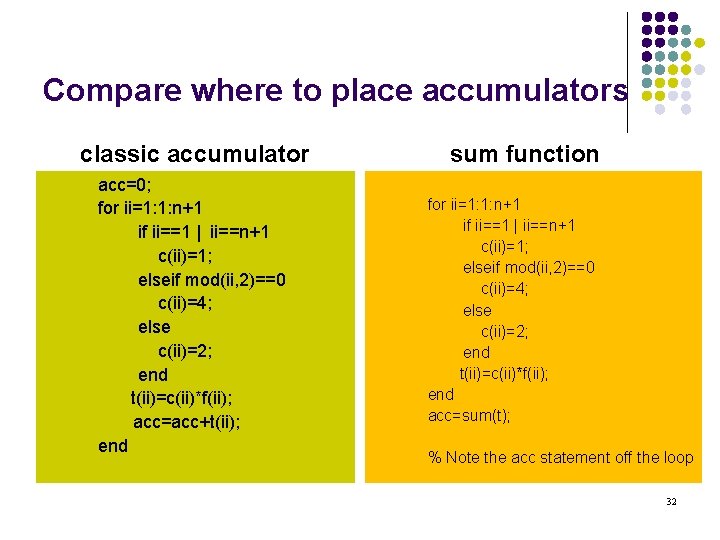
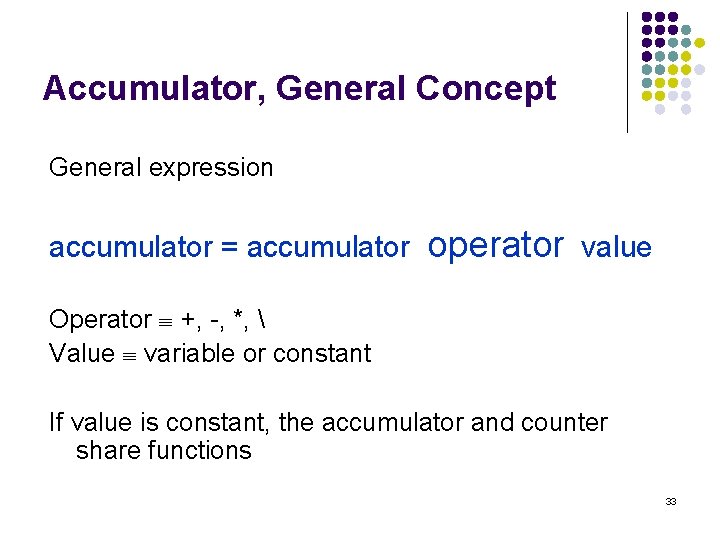
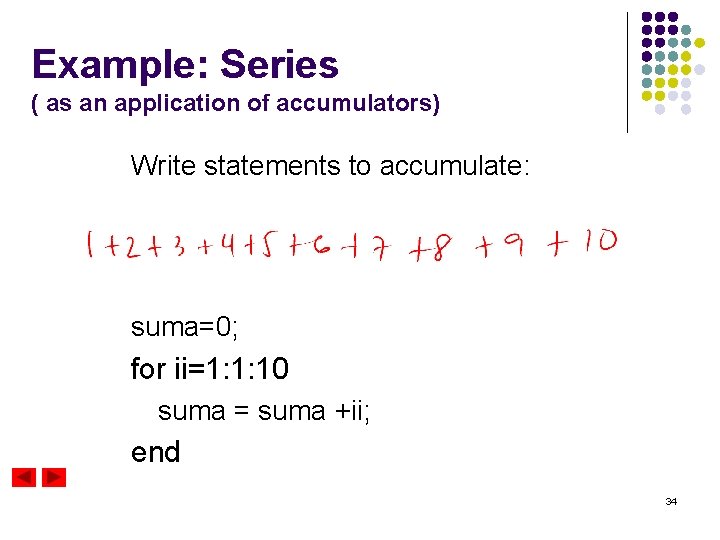
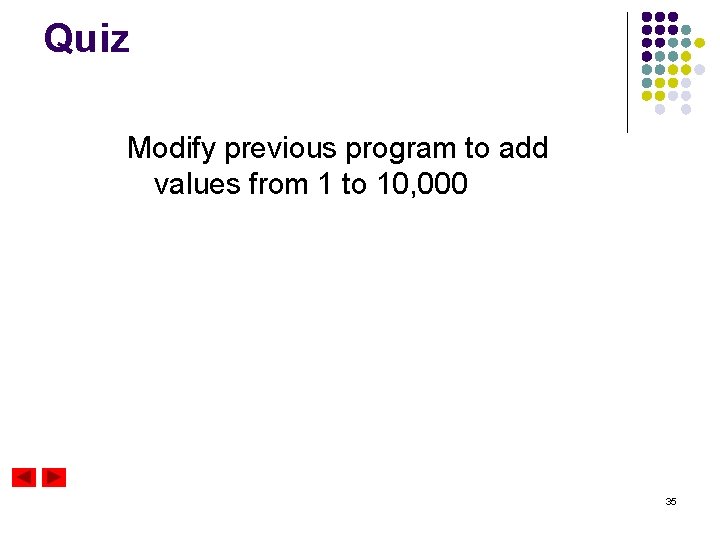
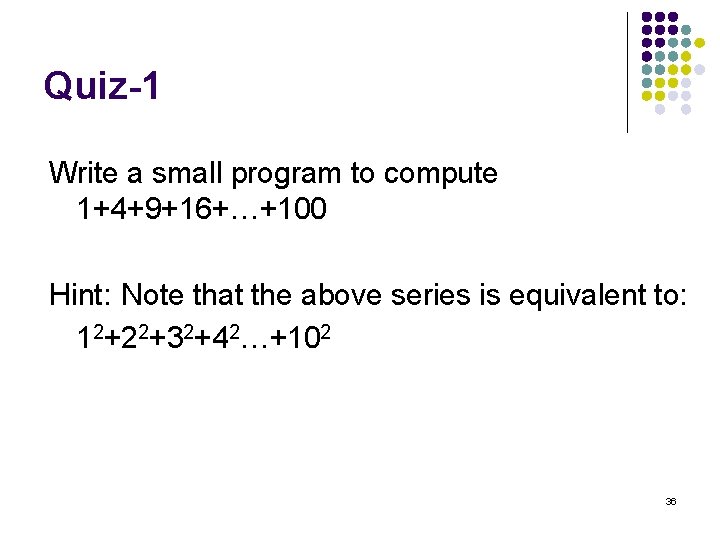
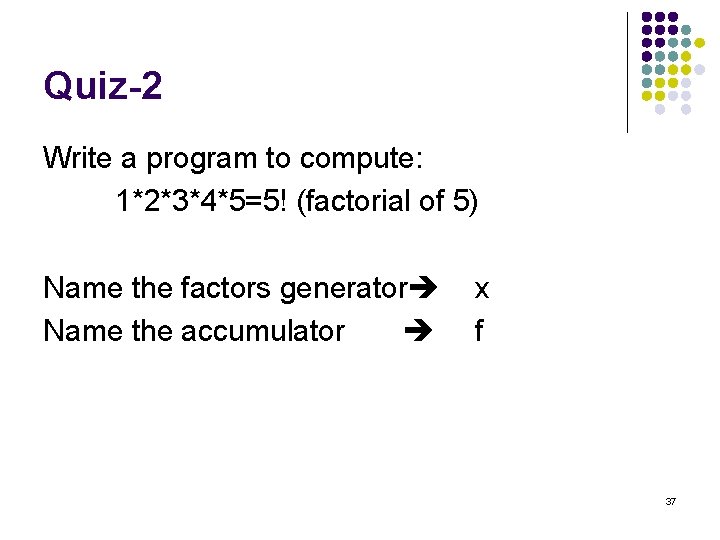
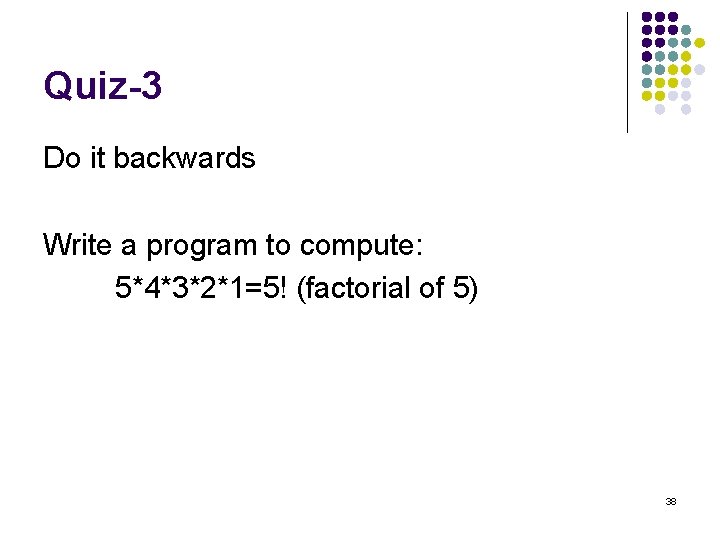
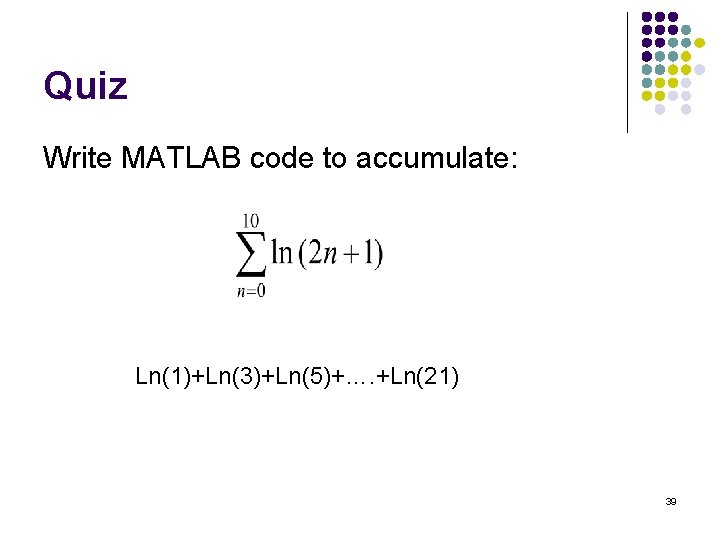
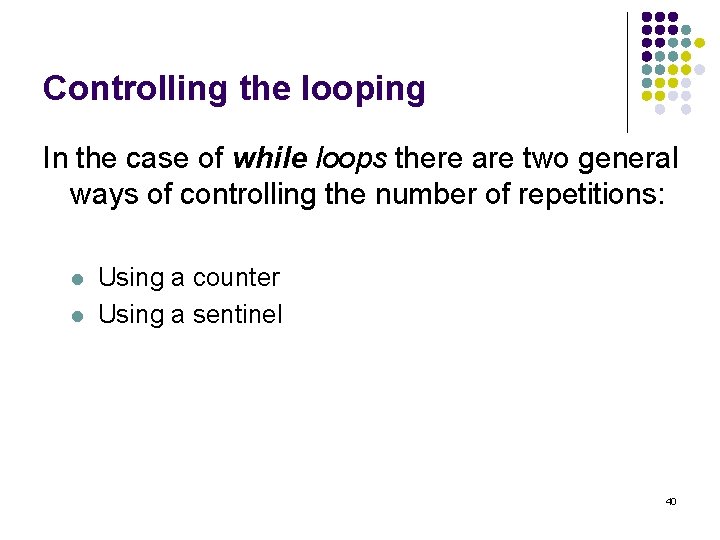
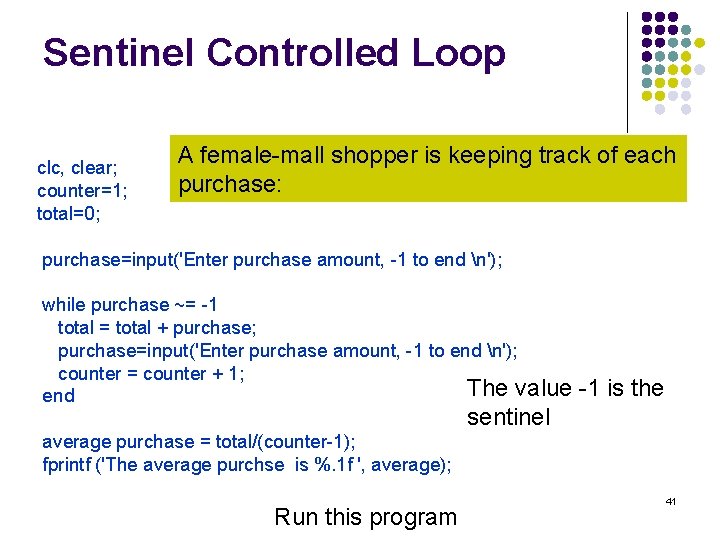
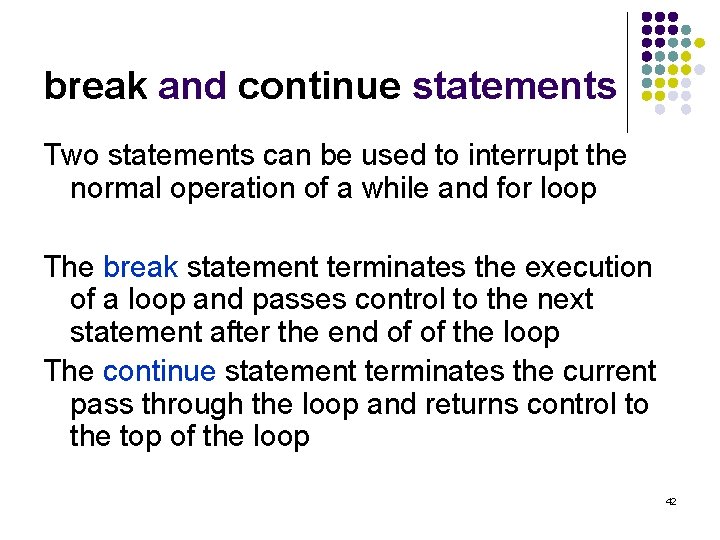
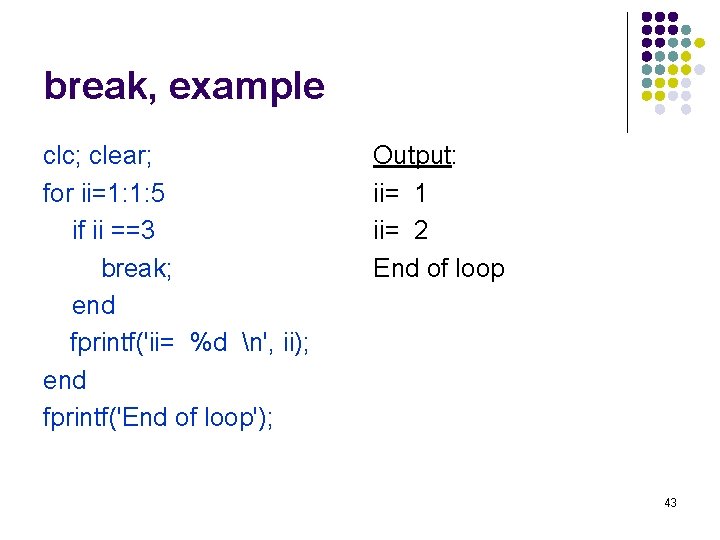
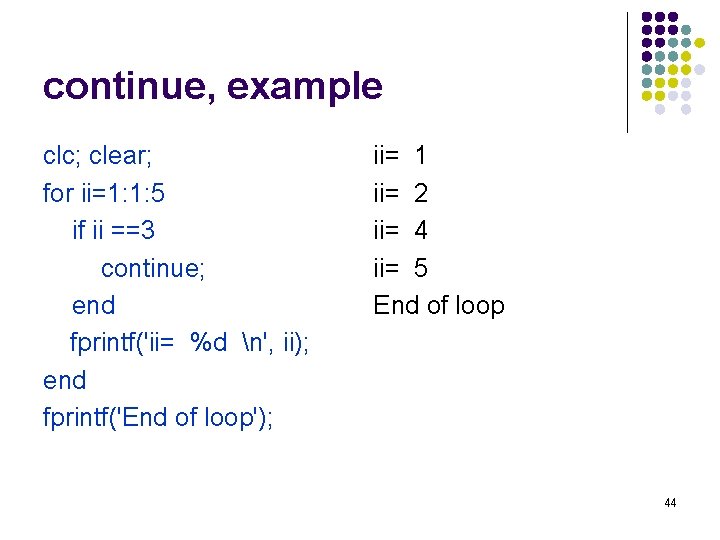
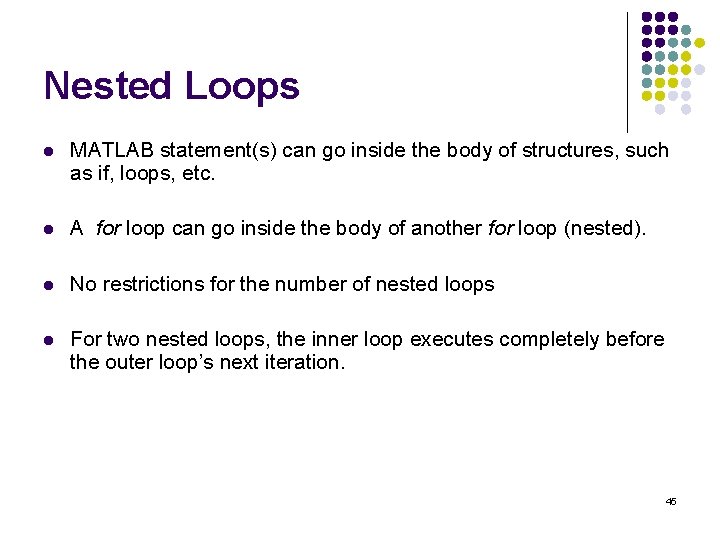
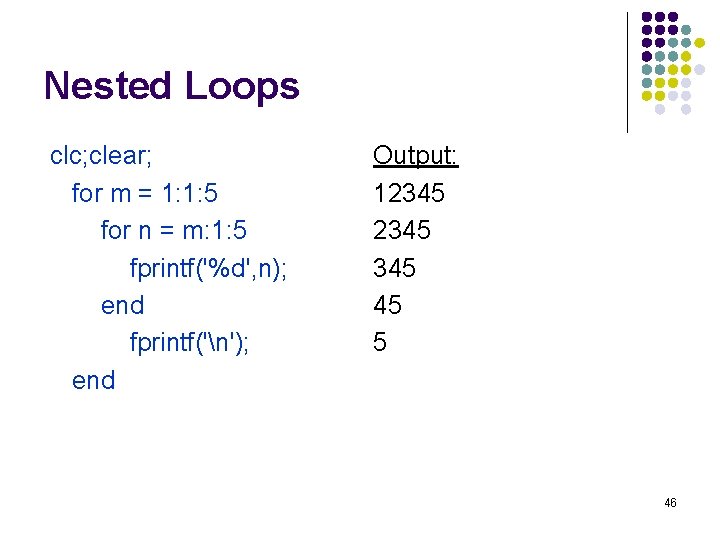
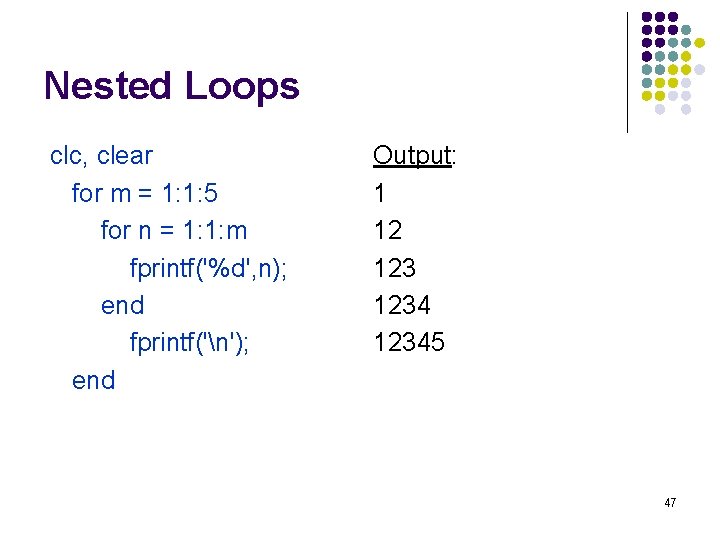
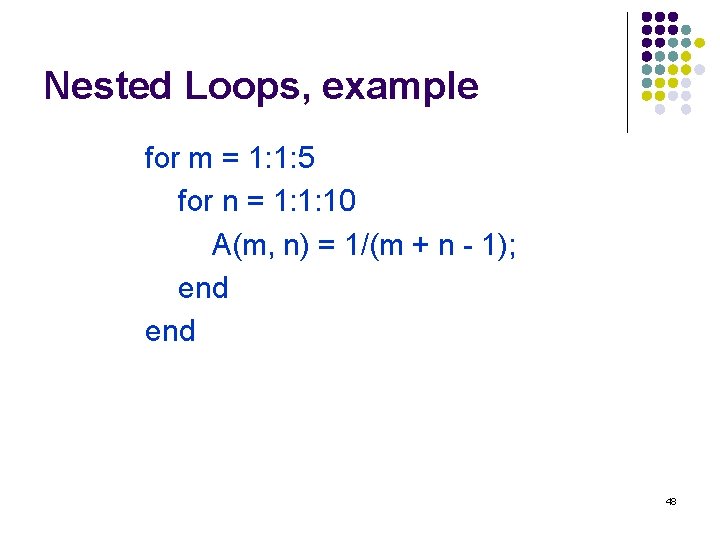
- Slides: 48
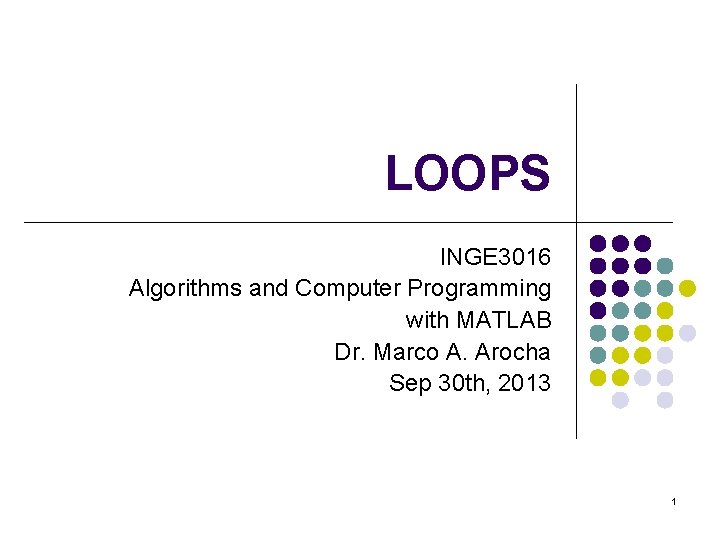
LOOPS INGE 3016 Algorithms and Computer Programming with MATLAB Dr. Marco A. Arocha Sep 30 th, 2013 1
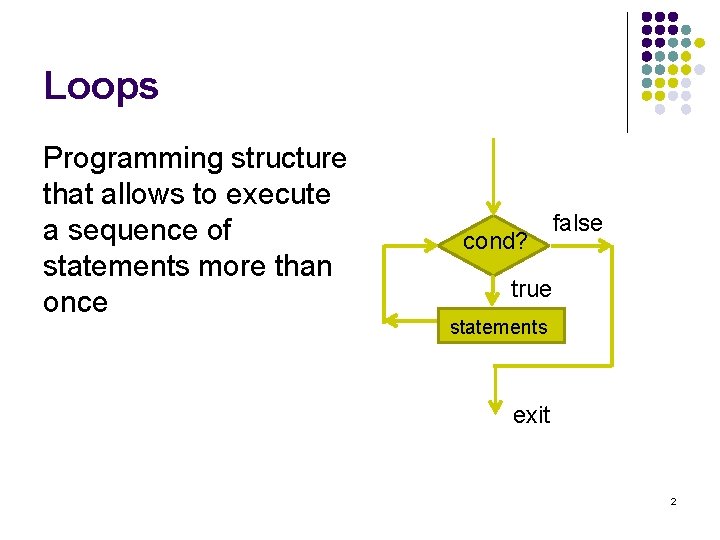
Loops Programming structure that allows to execute a sequence of statements more than once cond? false true statements exit 2
![Loops Two basic syntax forms for cvlist of values while condition statements end cvcontrol Loops Two basic syntax forms: for cv=[list of values] while condition statements end cv=control](https://slidetodoc.com/presentation_image_h2/93177aa56fa3db444fdf5f6038f1962a/image-3.jpg)
Loops Two basic syntax forms: for cv=[list of values] while condition statements end cv=control variable or index variable 3
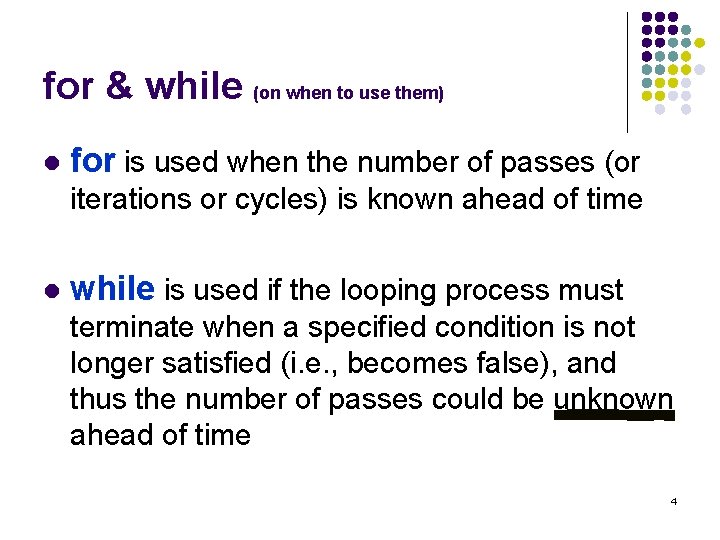
for & while (on when to use them) l for is used when the number of passes (or iterations or cycles) is known ahead of time l while is used if the looping process must terminate when a specified condition is not longer satisfied (i. e. , becomes false), and thus the number of passes could be unknown ahead of time 4
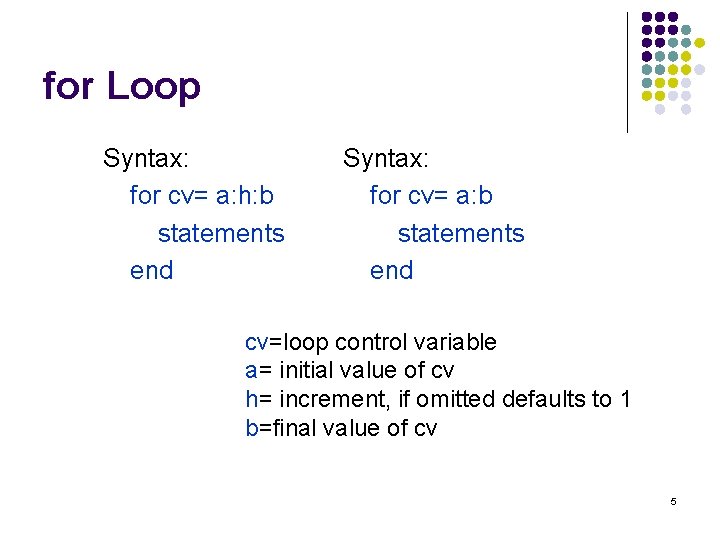
for Loop Syntax: for cv= a: h: b statements end Syntax: for cv= a: b statements end cv=loop control variable a= initial value of cv h= increment, if omitted defaults to 1 b=final value of cv 5
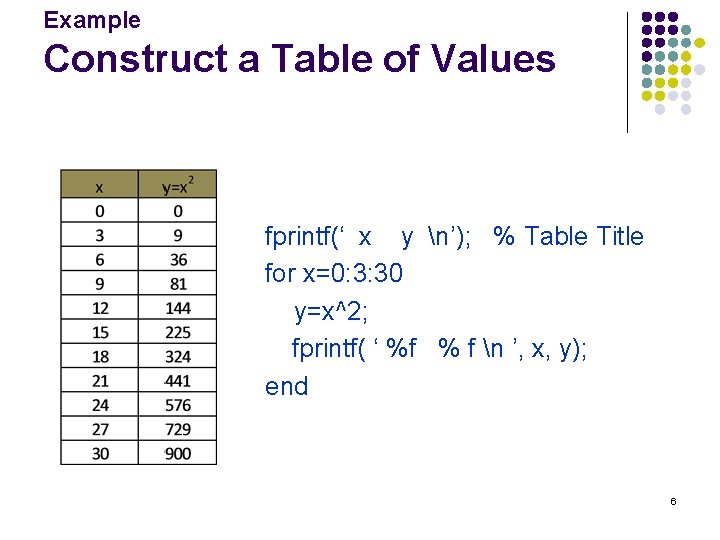
Example Construct a Table of Values fprintf(‘ x y n’); % Table Title for x=0: 3: 30 y=x^2; fprintf( ‘ %f % f n ’, x, y); end 6
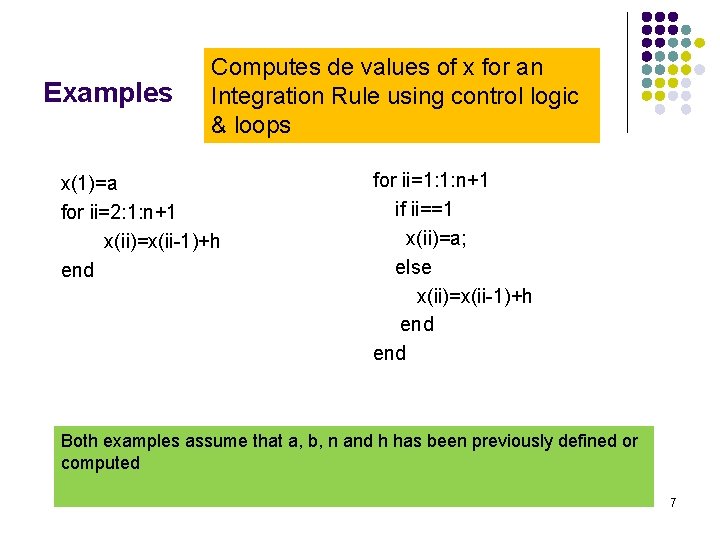
Examples Computes de values of x for an Integration Rule using control logic & loops x(1)=a for ii=2: 1: n+1 x(ii)=x(ii-1)+h end for ii=1: 1: n+1 if ii==1 x(ii)=a; else x(ii)=x(ii-1)+h end Both examples assume that a, b, n and h has been previously defined or computed 7
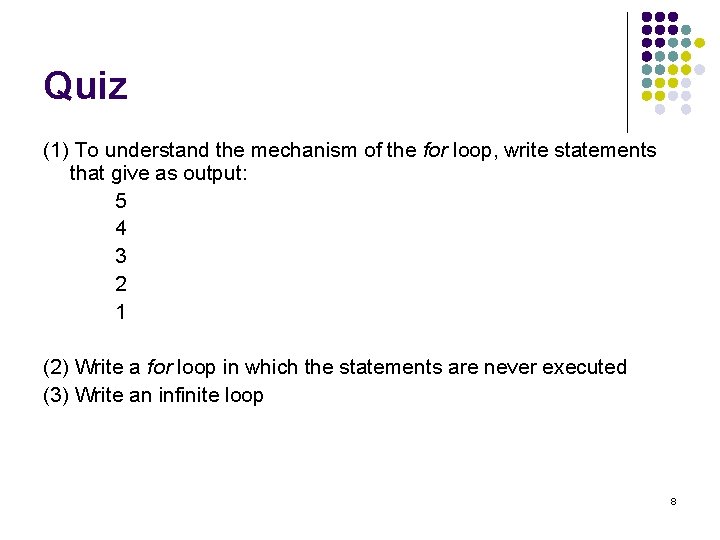
Quiz (1) To understand the mechanism of the for loop, write statements that give as output: 5 4 3 2 1 (2) Write a for loop in which the statements are never executed (3) Write an infinite loop 8
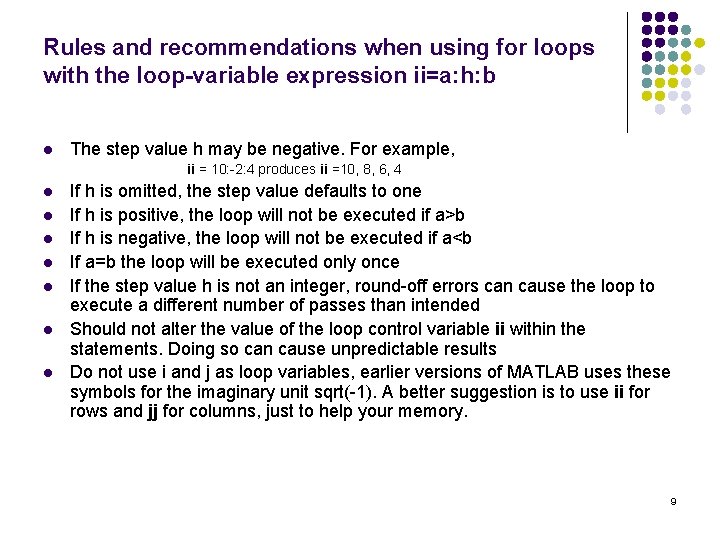
Rules and recommendations when using for loops with the loop-variable expression ii=a: h: b l The step value h may be negative. For example, ii = 10: -2: 4 produces ii =10, 8, 6, 4 l l l l If h is omitted, the step value defaults to one If h is positive, the loop will not be executed if a>b If h is negative, the loop will not be executed if a<b If a=b the loop will be executed only once If the step value h is not an integer, round-off errors can cause the loop to execute a different number of passes than intended Should not alter the value of the loop control variable ii within the statements. Doing so can cause unpredictable results Do not use i and j as loop variables, earlier versions of MATLAB uses these symbols for the imaginary unit sqrt(-1). A better suggestion is to use ii for rows and jj for columns, just to help your memory. 9
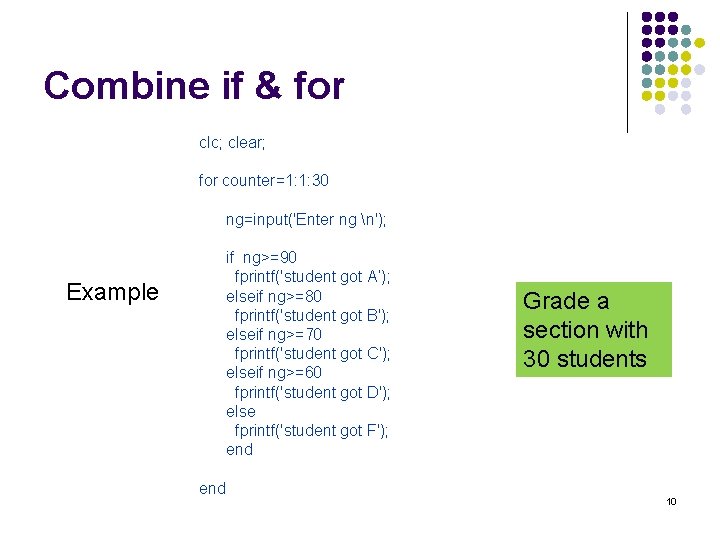
Combine if & for clc; clear; for counter=1: 1: 30 ng=input('Enter ng n'); if ng>=90 fprintf('student got A‘); elseif ng>=80 fprintf('student got B'); elseif ng>=70 fprintf('student got C'); elseif ng>=60 fprintf('student got D'); else fprintf('student got F'); end Example end Grade a section with 30 students 10
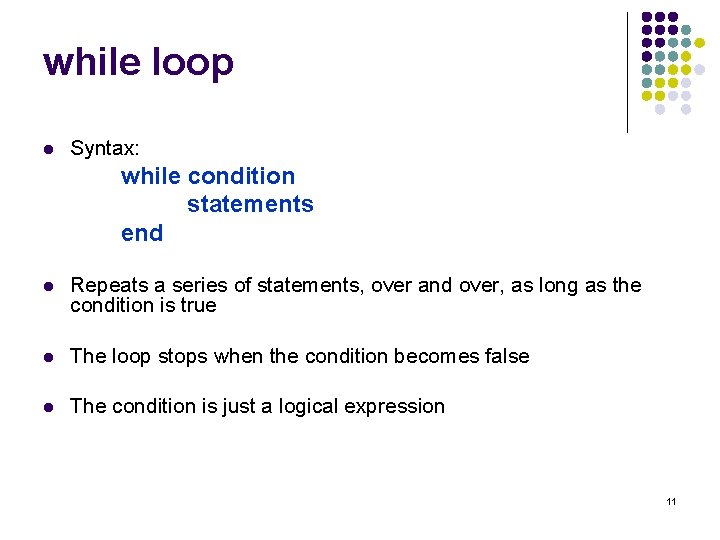
while loop l Syntax: while condition statements end l Repeats a series of statements, over and over, as long as the condition is true l The loop stops when the condition becomes false l The condition is just a logical expression 11
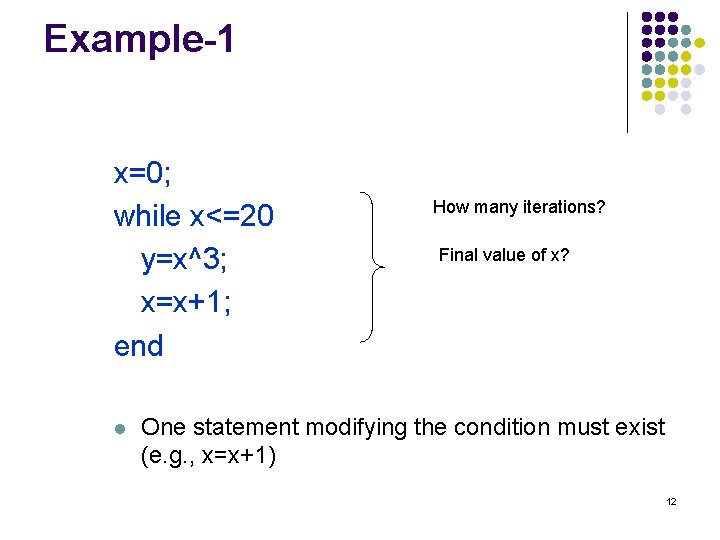
Example-1 x=0; while x<=20 y=x^3; x=x+1; end l How many iterations? Final value of x? One statement modifying the condition must exist (e. g. , x=x+1) 12
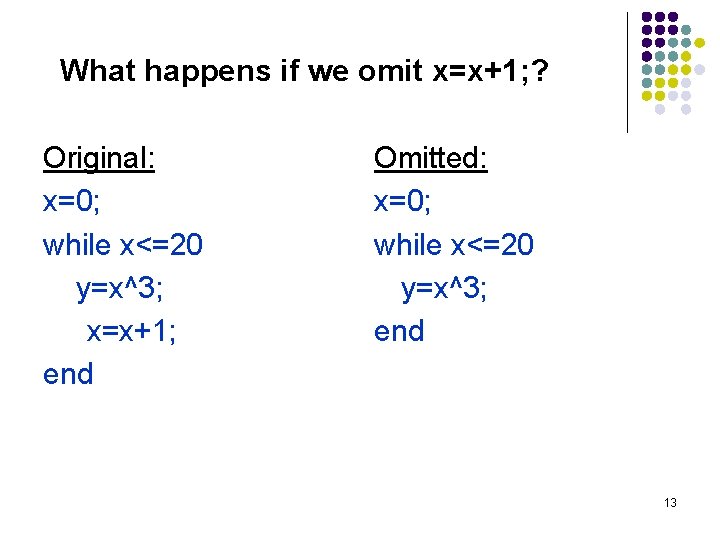
What happens if we omit x=x+1; ? Original: x=0; while x<=20 y=x^3; x=x+1; end Omitted: x=0; while x<=20 y=x^3; end 13
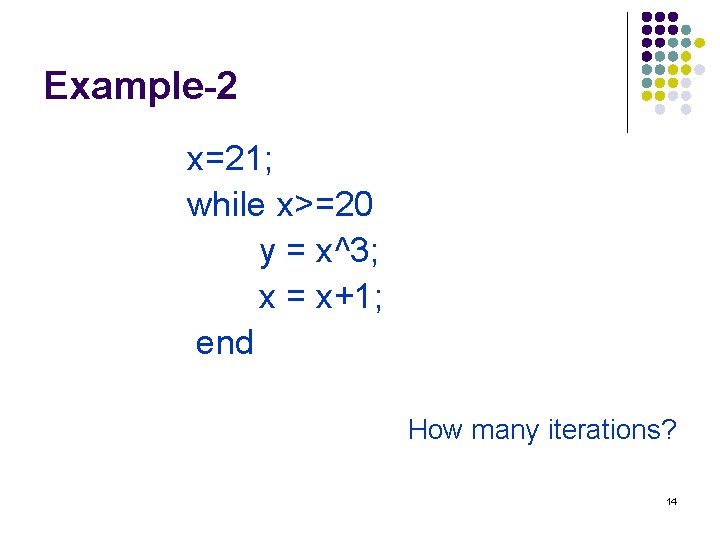
Example-2 x=21; while x>=20 y = x^3; x = x+1; end How many iterations? 14
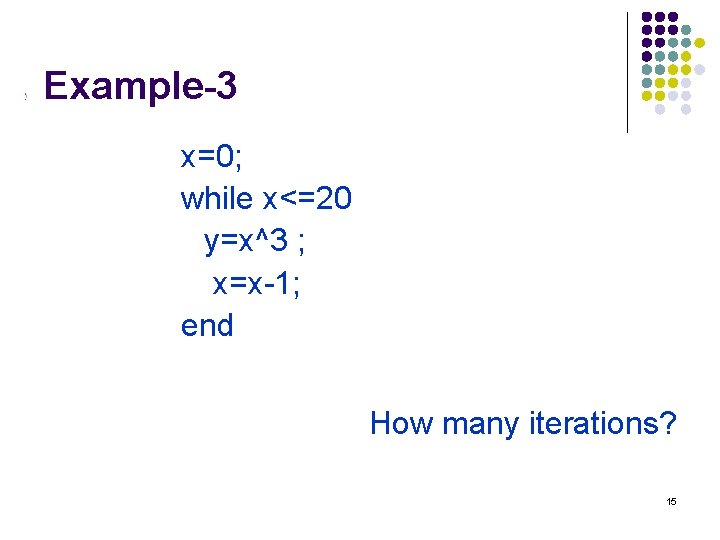
Example-3 x=0; while x<=20 y=x^3 ; x=x-1; end How many iterations? 15
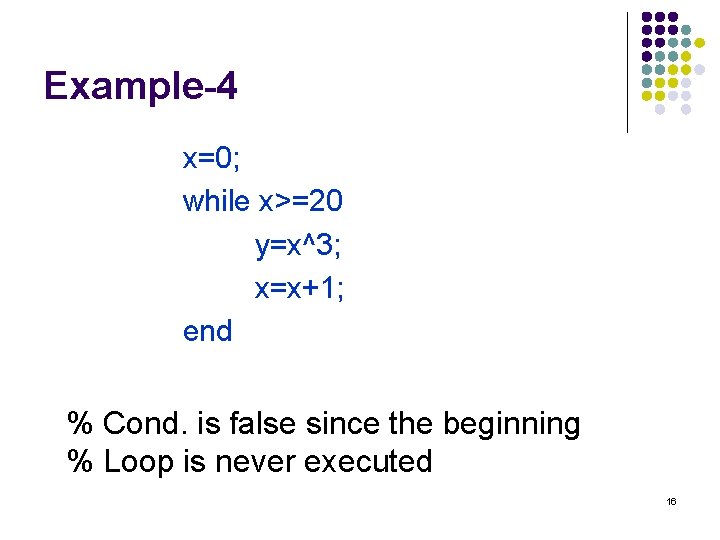
Example-4 x=0; while x>=20 y=x^3; x=x+1; end % Cond. is false since the beginning % Loop is never executed 16
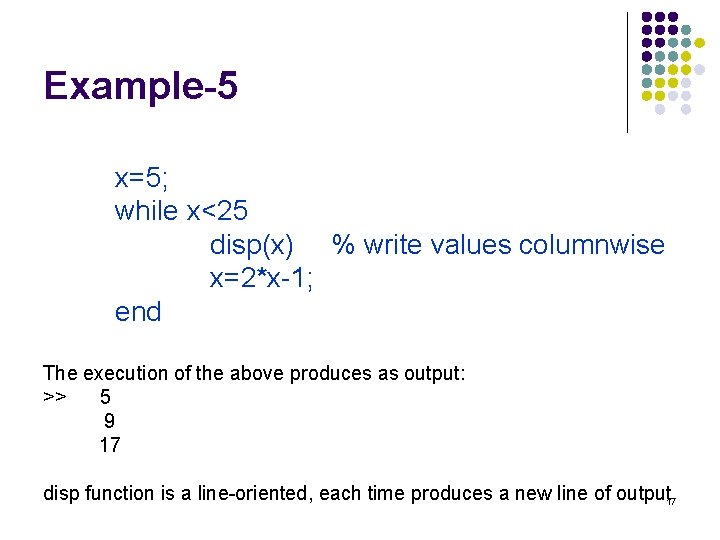
Example-5 x=5; while x<25 disp(x) % write values columnwise x=2*x-1; end The execution of the above produces as output: >> 5 9 17 disp function is a line-oriented, each time produces a new line of output 17
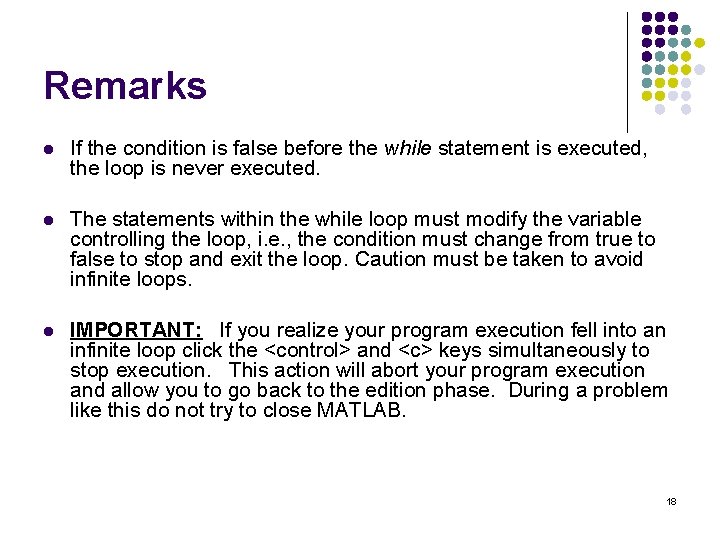
Remarks l If the condition is false before the while statement is executed, the loop is never executed. l The statements within the while loop must modify the variable controlling the loop, i. e. , the condition must change from true to false to stop and exit the loop. Caution must be taken to avoid infinite loops. l IMPORTANT: If you realize your program execution fell into an infinite loop click the <control> and <c> keys simultaneously to stop execution. This action will abort your program execution and allow you to go back to the edition phase. During a problem like this do not try to close MATLAB. 18
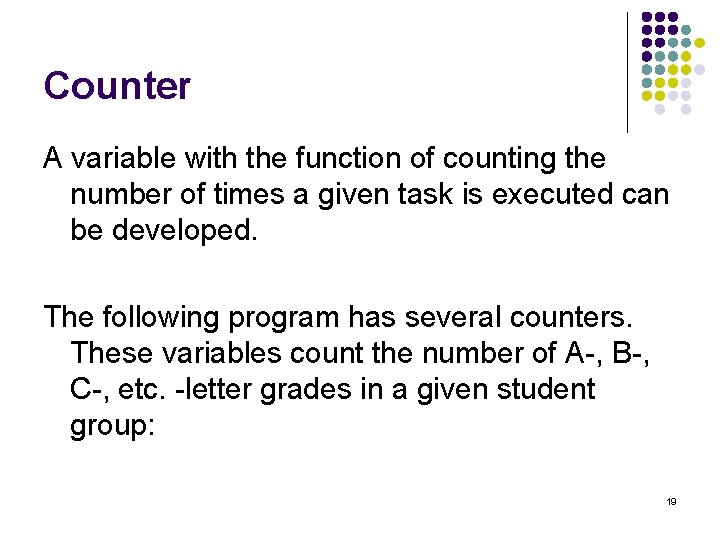
Counter A variable with the function of counting the number of times a given task is executed can be developed. The following program has several counters. These variables count the number of A-, B-, C-, etc. -letter grades in a given student group: 19
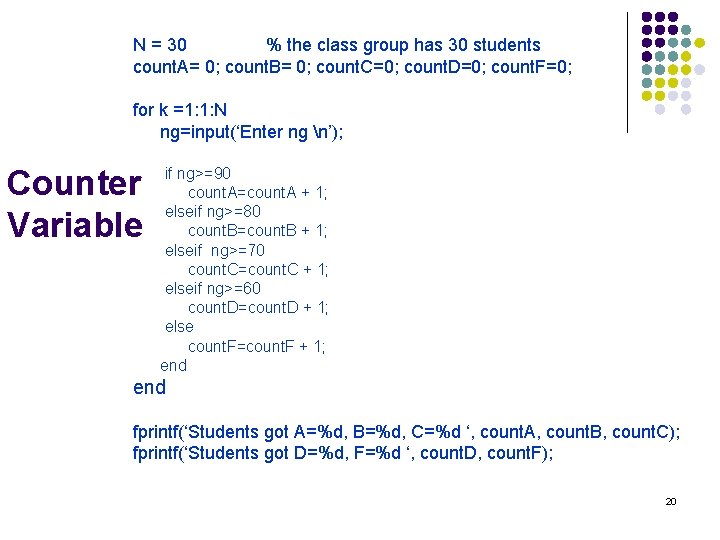
N = 30 % the class group has 30 students count. A= 0; count. B= 0; count. C=0; count. D=0; count. F=0; for k =1: 1: N ng=input(‘Enter ng n’); Counter Variable if ng>=90 count. A=count. A + 1; elseif ng>=80 count. B=count. B + 1; elseif ng>=70 count. C=count. C + 1; elseif ng>=60 count. D=count. D + 1; else count. F=count. F + 1; end fprintf(‘Students got A=%d, B=%d, C=%d ‘, count. A, count. B, count. C); fprintf(‘Students got D=%d, F=%d ‘, count. D, count. F); 20
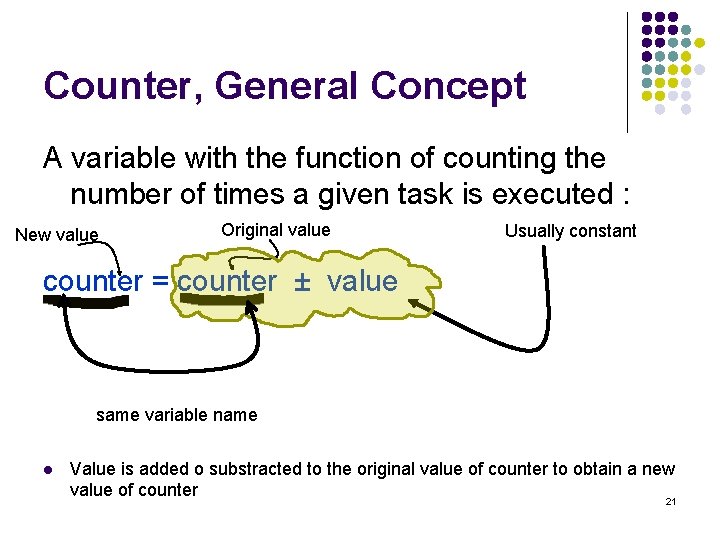
Counter, General Concept A variable with the function of counting the number of times a given task is executed : New value Original value Usually constant counter = counter ± value same variable name l Value is added o substracted to the original value of counter to obtain a new value of counter 21
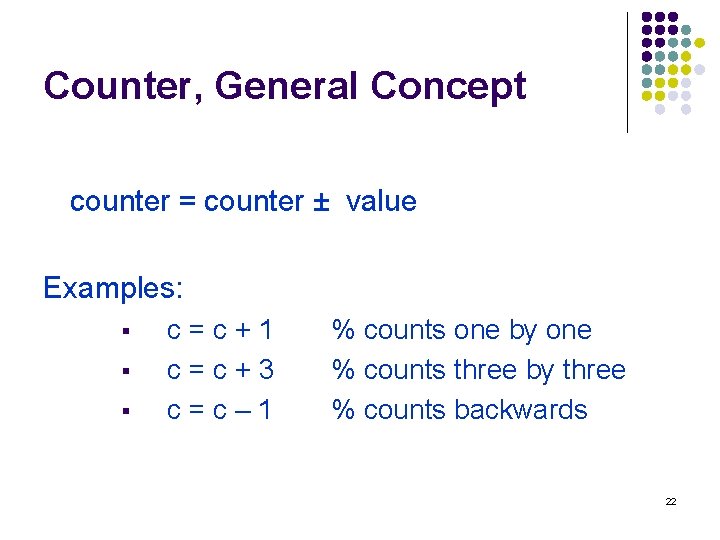
Counter, General Concept counter = counter ± value Examples: § § § c=c+1 c=c+3 c=c– 1 % counts one by one % counts three by three % counts backwards 22
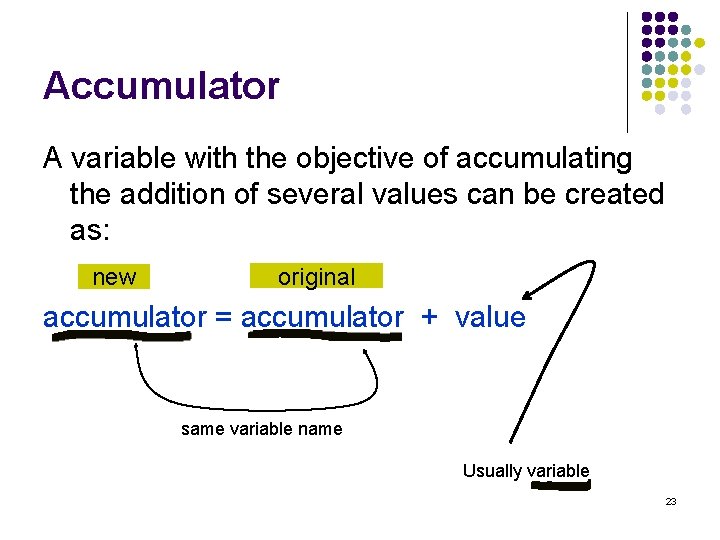
Accumulator A variable with the objective of accumulating the addition of several values can be created as: new original accumulator = accumulator + value same variable name Usually variable 23
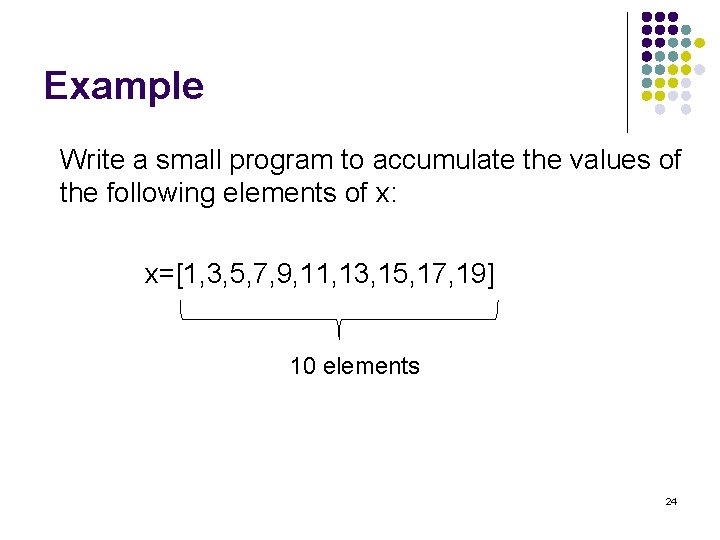
Example Write a small program to accumulate the values of the following elements of x: x=[1, 3, 5, 7, 9, 11, 13, 15, 17, 19] 10 elements 24
![Solution1 x 1 3 5 7 9 11 13 15 17 19 Solution-1 x= [ 1, 3, 5, 7, 9, 11, 13, 15, 17, 19 ];](https://slidetodoc.com/presentation_image_h2/93177aa56fa3db444fdf5f6038f1962a/image-25.jpg)
Solution-1 x= [ 1, 3, 5, 7, 9, 11, 13, 15, 17, 19 ]; suma=0; length(x) for i=1: 1: 10 suma = suma +x( i ); end fprintf(‘Suma= %f n’, suma); 25
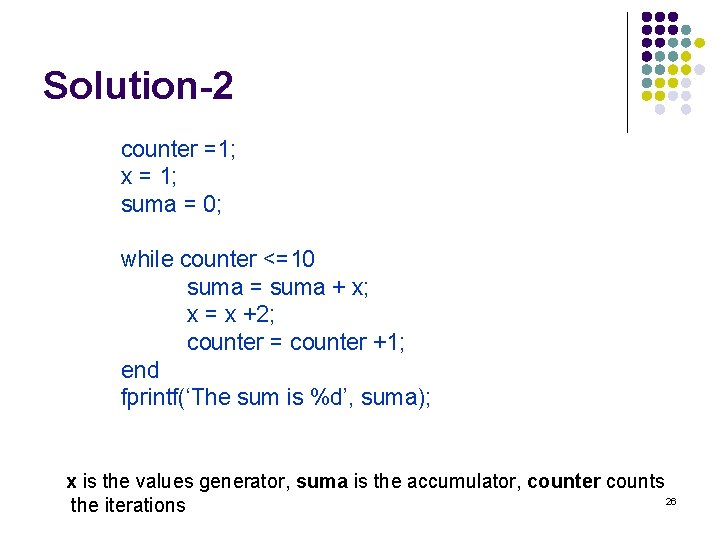
Solution-2 counter =1; x = 1; suma = 0; while counter <=10 suma = suma + x; x = x +2; counter = counter +1; end fprintf(‘The sum is %d’, suma); x is the values generator, suma is the accumulator, counter counts 26 the iterations
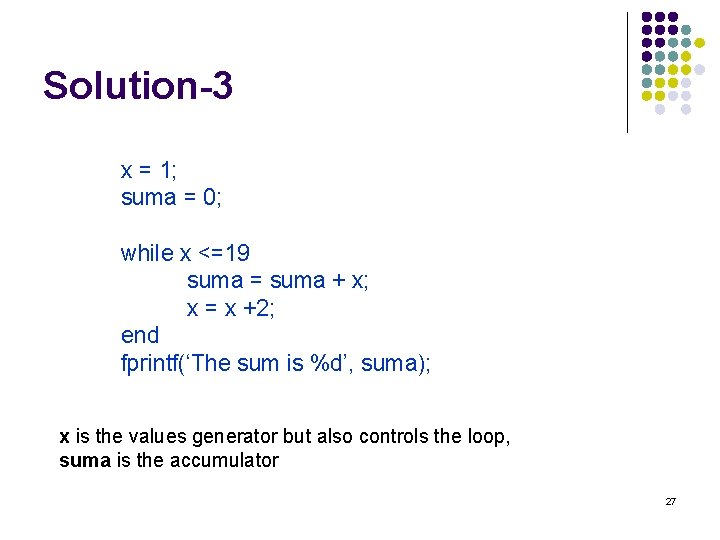
Solution-3 x = 1; suma = 0; while x <=19 suma = suma + x; x = x +2; end fprintf(‘The sum is %d’, suma); x is the values generator but also controls the loop, suma is the accumulator 27
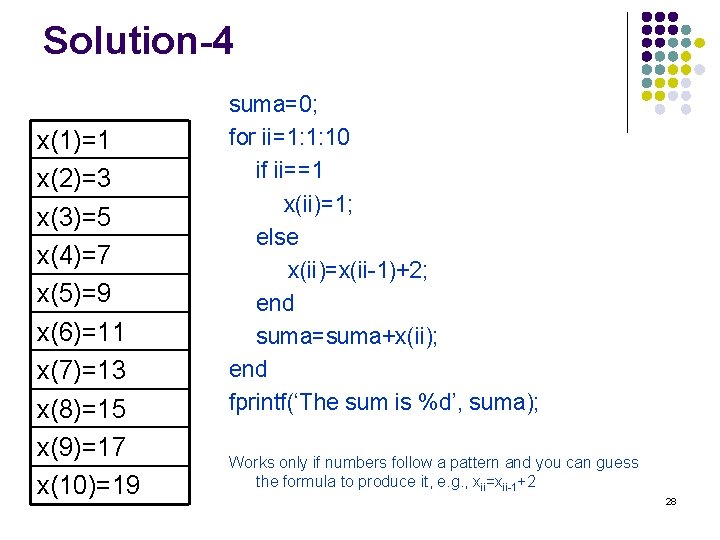
Solution-4 x(1)=1 x(2)=3 x(3)=5 x(4)=7 x(5)=9 x(6)=11 x(7)=13 x(8)=15 x(9)=17 x(10)=19 suma=0; for ii=1: 1: 10 if ii==1 x(ii)=1; else x(ii)=x(ii-1)+2; end suma=suma+x(ii); end fprintf(‘The sum is %d’, suma); Works only if numbers follow a pattern and you can guess the formula to produce it, e. g. , xii=xii-1+2 28
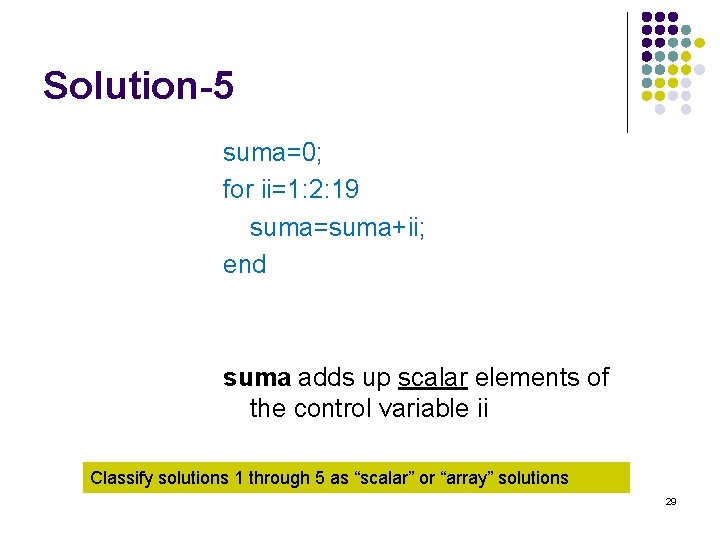
Solution-5 suma=0; for ii=1: 2: 19 suma=suma+ii; end suma adds up scalar elements of the control variable ii Classify solutions 1 through 5 as “scalar” or “array” solutions 29
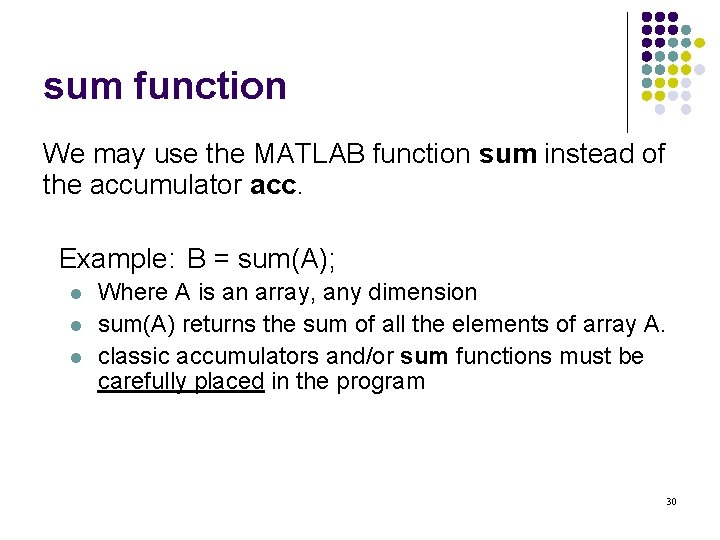
sum function We may use the MATLAB function sum instead of the accumulator acc. Example: B = sum(A); l l l Where A is an array, any dimension sum(A) returns the sum of all the elements of array A. classic accumulators and/or sum functions must be carefully placed in the program 30
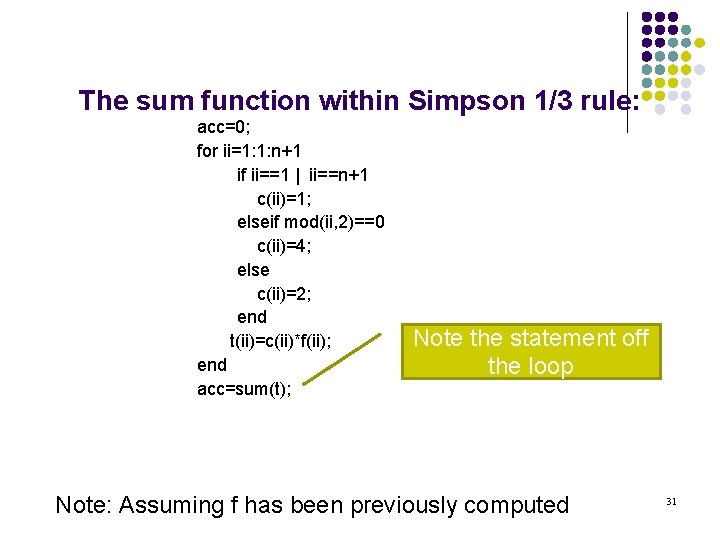
The sum function within Simpson 1/3 rule: acc=0; for ii=1: 1: n+1 if ii==1 | ii==n+1 c(ii)=1; elseif mod(ii, 2)==0 c(ii)=4; else c(ii)=2; end t(ii)=c(ii)*f(ii); end acc=sum(t); Note the statement off the loop Note: Assuming f has been previously computed 31
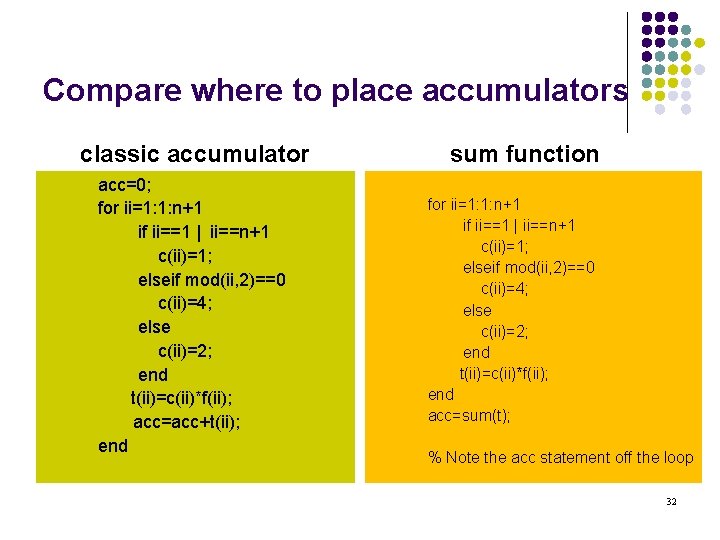
Compare where to place accumulators classic accumulator acc=0; for ii=1: 1: n+1 if ii==1 | ii==n+1 c(ii)=1; elseif mod(ii, 2)==0 c(ii)=4; else c(ii)=2; end t(ii)=c(ii)*f(ii); acc=acc+t(ii); end sum function for ii=1: 1: n+1 if ii==1 | ii==n+1 c(ii)=1; elseif mod(ii, 2)==0 c(ii)=4; else c(ii)=2; end t(ii)=c(ii)*f(ii); end acc=sum(t); % Note the acc statement off the loop 32
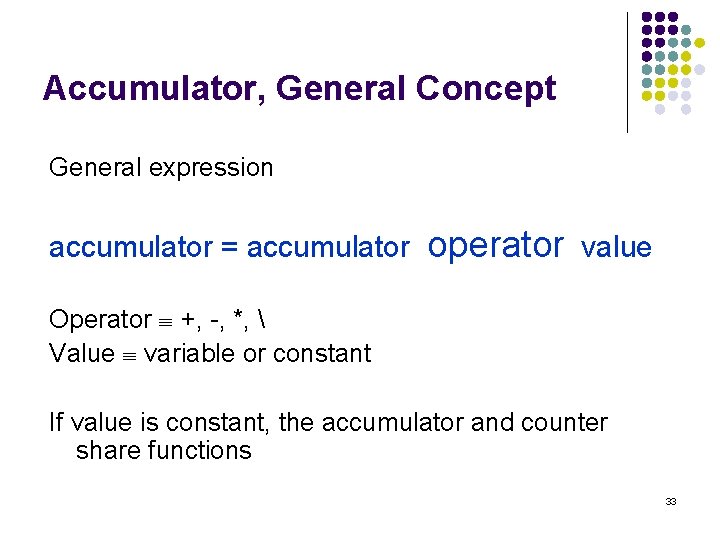
Accumulator, General Concept General expression accumulator = accumulator operator value Operator +, -, *, Value variable or constant If value is constant, the accumulator and counter share functions 33
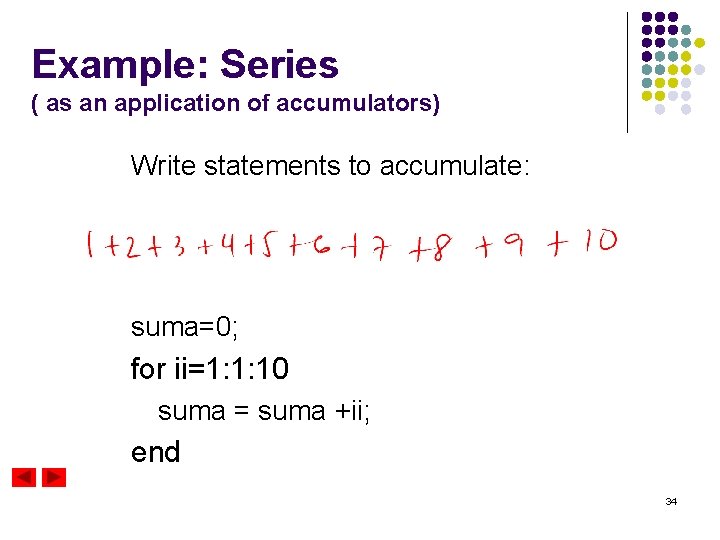
Example: Series ( as an application of accumulators) Write statements to accumulate: suma=0; for ii=1: 1: 10 suma = suma +ii; end 34
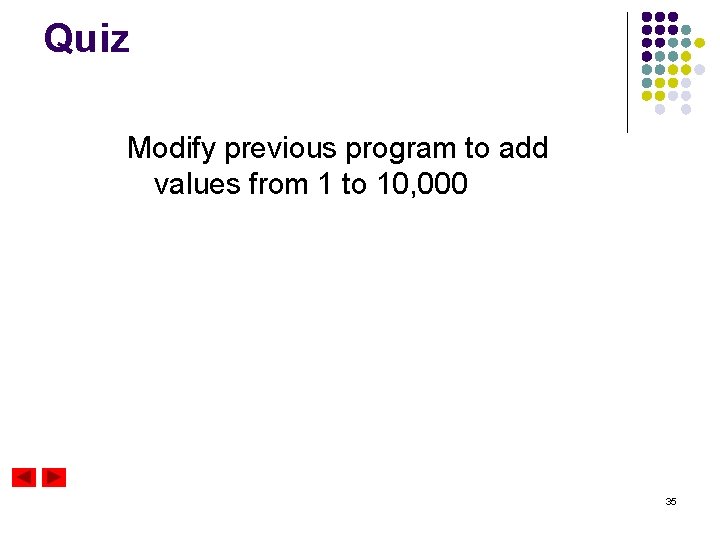
Quiz Modify previous program to add values from 1 to 10, 000 35
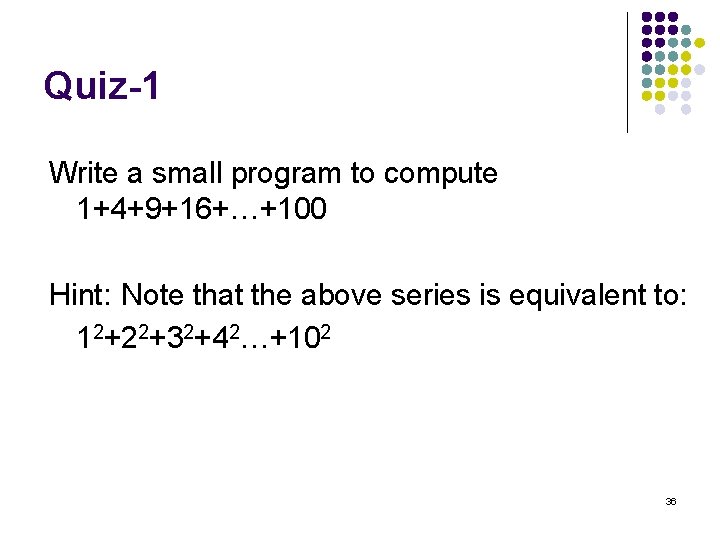
Quiz-1 Write a small program to compute 1+4+9+16+…+100 Hint: Note that the above series is equivalent to: 12+22+32+42…+102 36
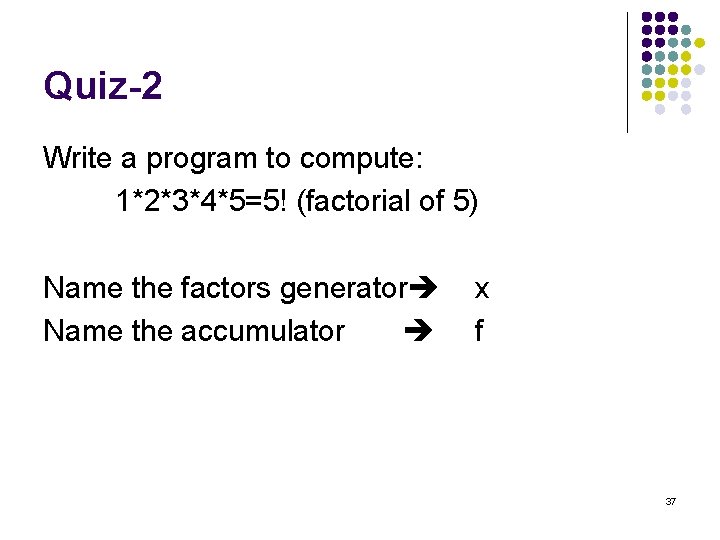
Quiz-2 Write a program to compute: 1*2*3*4*5=5! (factorial of 5) Name the factors generator Name the accumulator x f 37
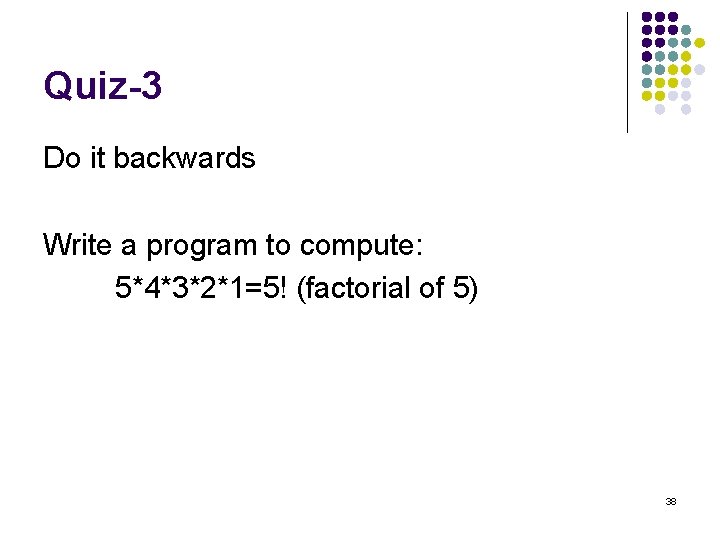
Quiz-3 Do it backwards Write a program to compute: 5*4*3*2*1=5! (factorial of 5) 38
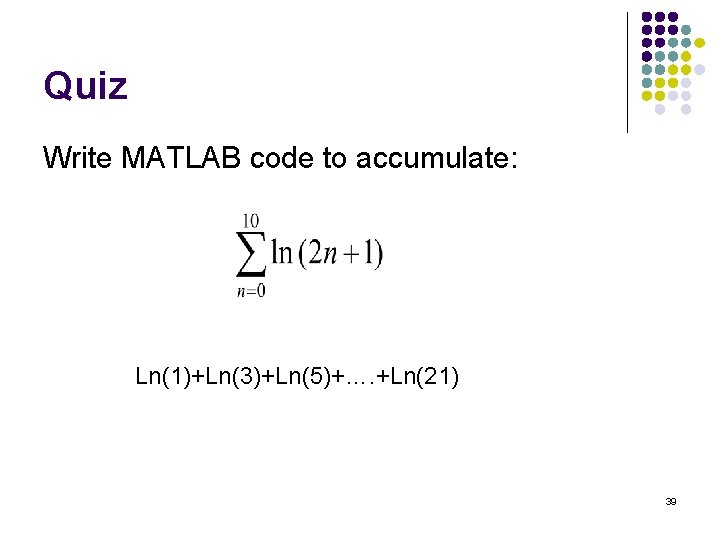
Quiz Write MATLAB code to accumulate: Ln(1)+Ln(3)+Ln(5)+…. +Ln(21) 39
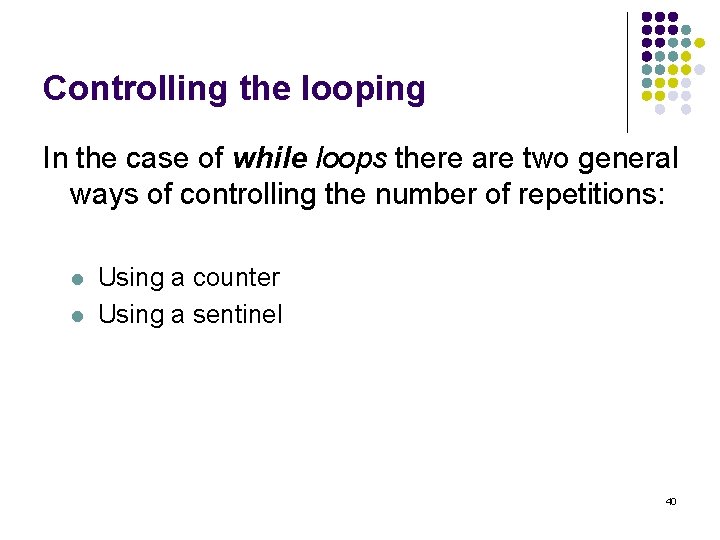
Controlling the looping In the case of while loops there are two general ways of controlling the number of repetitions: l l Using a counter Using a sentinel 40
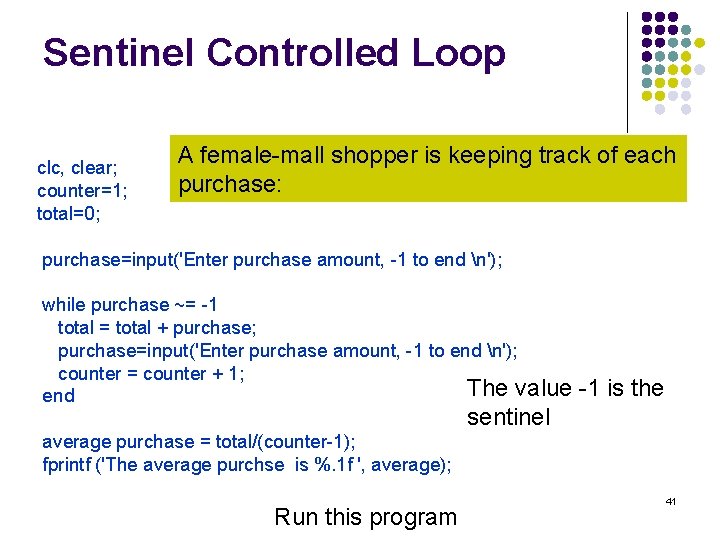
Sentinel Controlled Loop clc, clear; counter=1; total=0; A female-mall shopper is keeping track of each purchase: purchase=input('Enter purchase amount, -1 to end n'); while purchase ~= -1 total = total + purchase; purchase=input('Enter purchase amount, -1 to end n'); counter = counter + 1; The value end -1 is the sentinel average purchase = total/(counter-1); fprintf ('The average purchse is %. 1 f ', average); Run this program 41
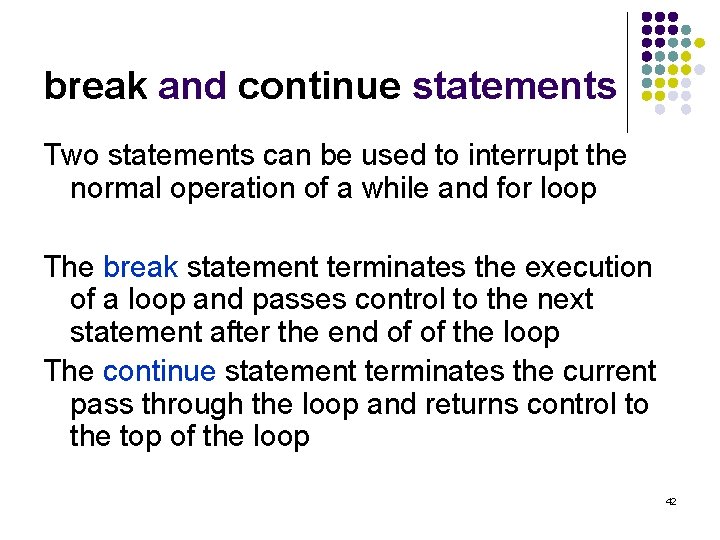
break and continue statements Two statements can be used to interrupt the normal operation of a while and for loop The break statement terminates the execution of a loop and passes control to the next statement after the end of of the loop The continue statement terminates the current pass through the loop and returns control to the top of the loop 42
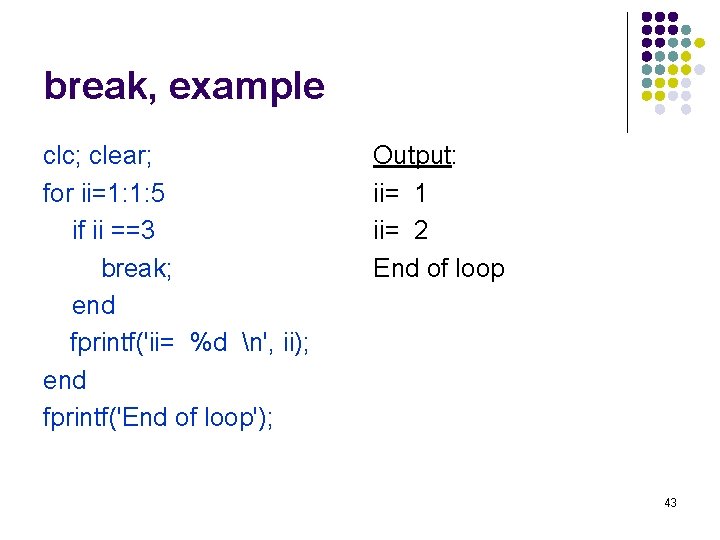
break, example clc; clear; for ii=1: 1: 5 if ii ==3 break; end fprintf('ii= %d n', ii); end fprintf('End of loop'); Output: ii= 1 ii= 2 End of loop 43
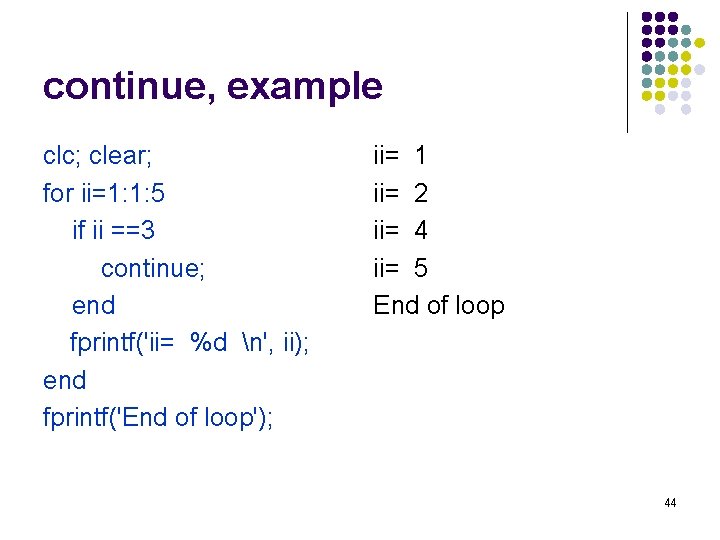
continue, example clc; clear; for ii=1: 1: 5 if ii ==3 continue; end fprintf('ii= %d n', ii); end fprintf('End of loop'); ii= 1 ii= 2 ii= 4 ii= 5 End of loop 44
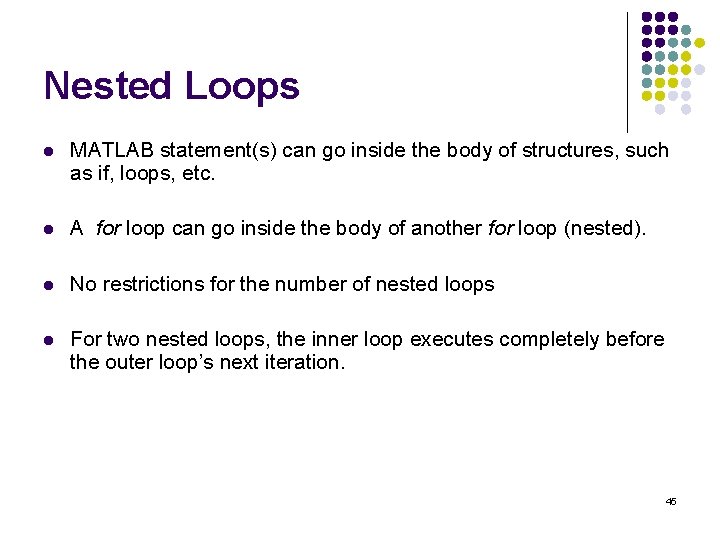
Nested Loops l MATLAB statement(s) can go inside the body of structures, such as if, loops, etc. l A for loop can go inside the body of another for loop (nested). l No restrictions for the number of nested loops l For two nested loops, the inner loop executes completely before the outer loop’s next iteration. 45
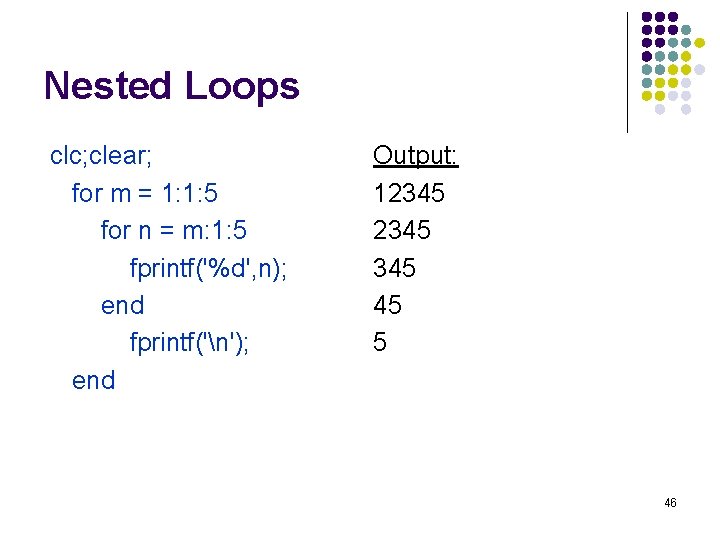
Nested Loops clc; clear; for m = 1: 1: 5 for n = m: 1: 5 fprintf('%d', n); end fprintf('n'); end Output: 12345 345 45 5 46
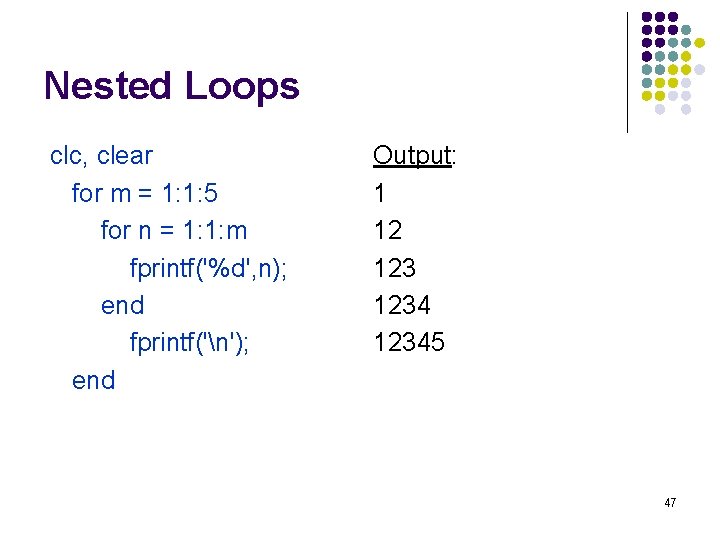
Nested Loops clc, clear for m = 1: 1: 5 for n = 1: 1: m fprintf('%d', n); end fprintf('n'); end Output: 1 12 12345 47
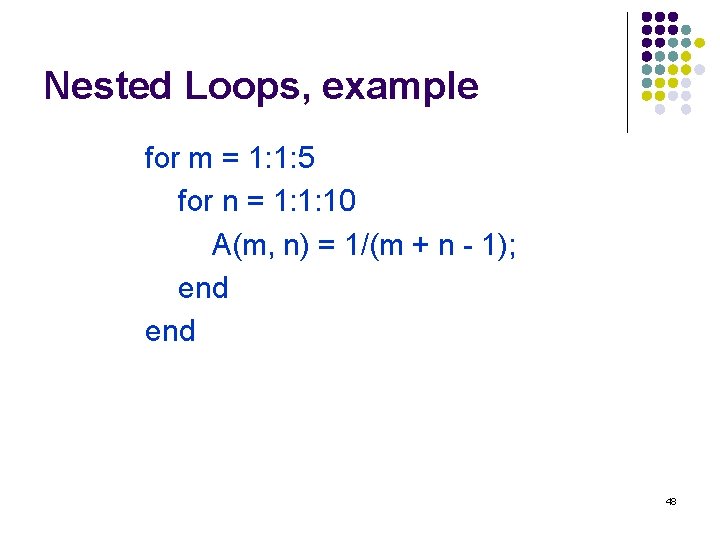
Nested Loops, example for m = 1: 1: 5 for n = 1: 1: 10 A(m, n) = 1/(m + n - 1); end 48
Computational thinking algorithms and programming
Synchronization algorithms and concurrent programming
Dot product rules
Fftooo
Algorithms in computer networks
Computer graphics line drawing algorithm
Inge van wijk
Inge lammers
Inge van boxtel
Inge-bert täljedal
Leif inge tjelta
Signalstoffer
Inge s. fomsgaard
Inge meichsner
Inge amundsen
Gorik stevens
Marika eidmann
Inge steen
Inge hill
Inge trijssenaar
Fitinge
Inge lehmann
Inge lahtmets
Inge lindsaar
Inge harmsen
Heidi paju
Inge van vilsteren
Inge holsbeeks
Inge kjær andersen
Inge lammers
Ulla lehmann
Cb ingé
Inge holsbeeks
Inge thoelen
While loops and if-else structures
Relation between loop, branch and node is given by
Loops and graphics in small basic
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
What is system program
Linear vs integer programming
Definisi integer
Concepts, techniques and models of computer programming
Flair furniture company linear programming
Fef 200-1200
Sun means
Matlab nested loops
Types of loops in matlab
Dame meaning in baa black sheep