Linked Lists Damian Gordon Linked Lists Imagine we
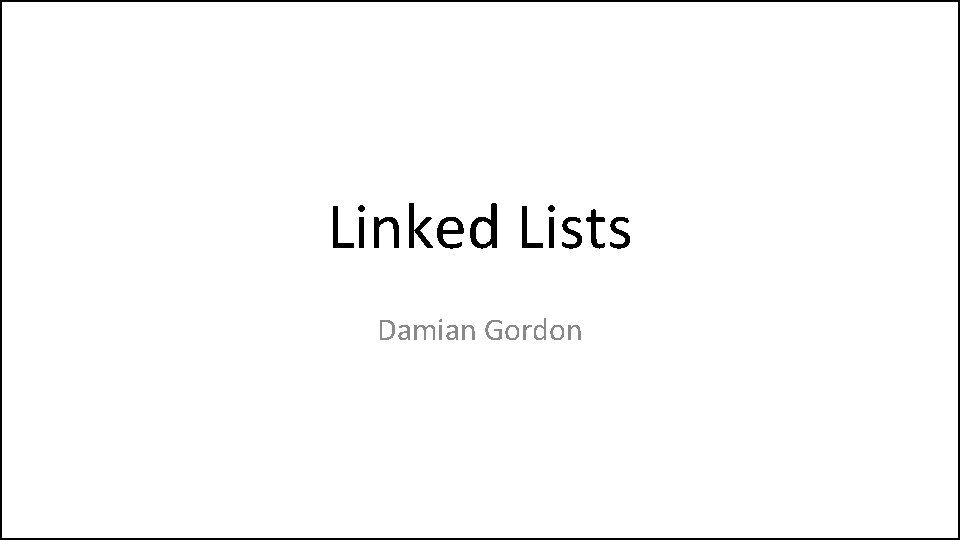
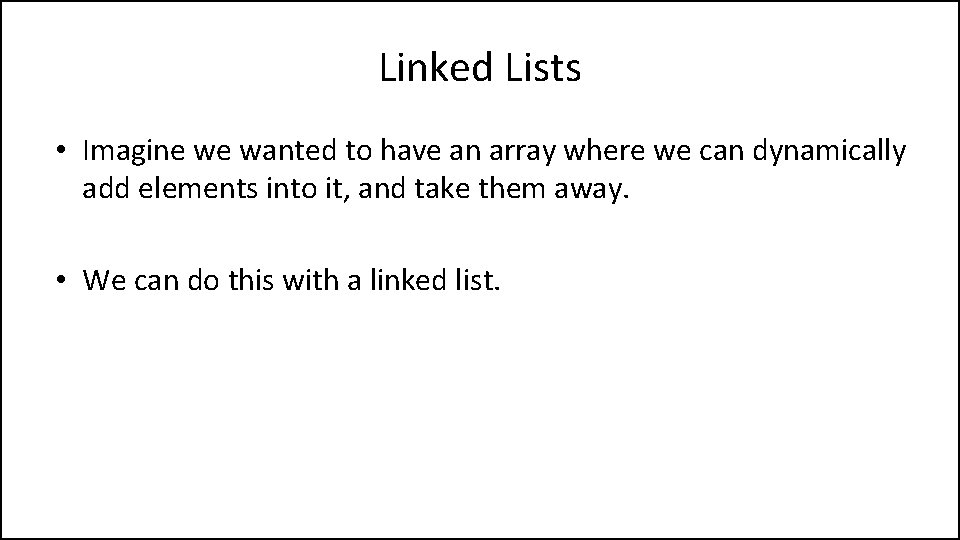
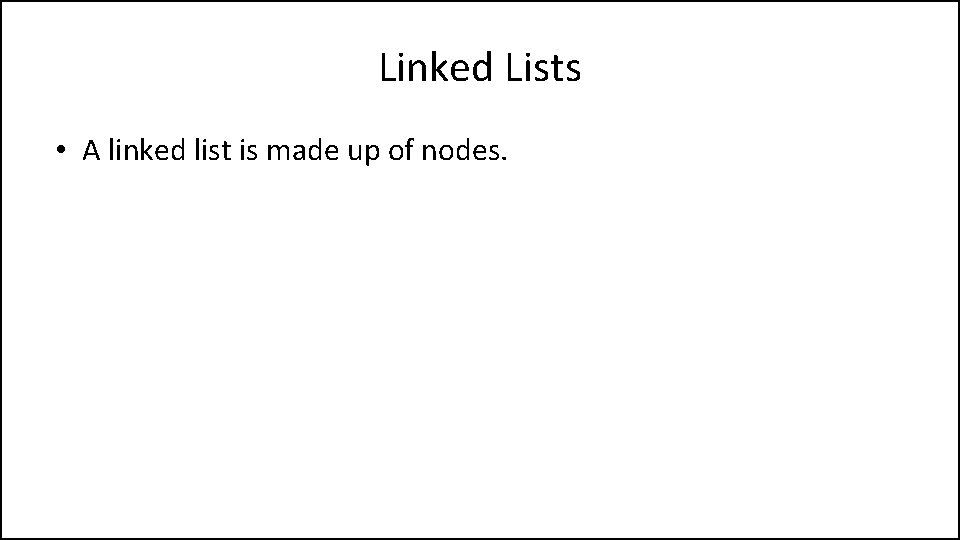
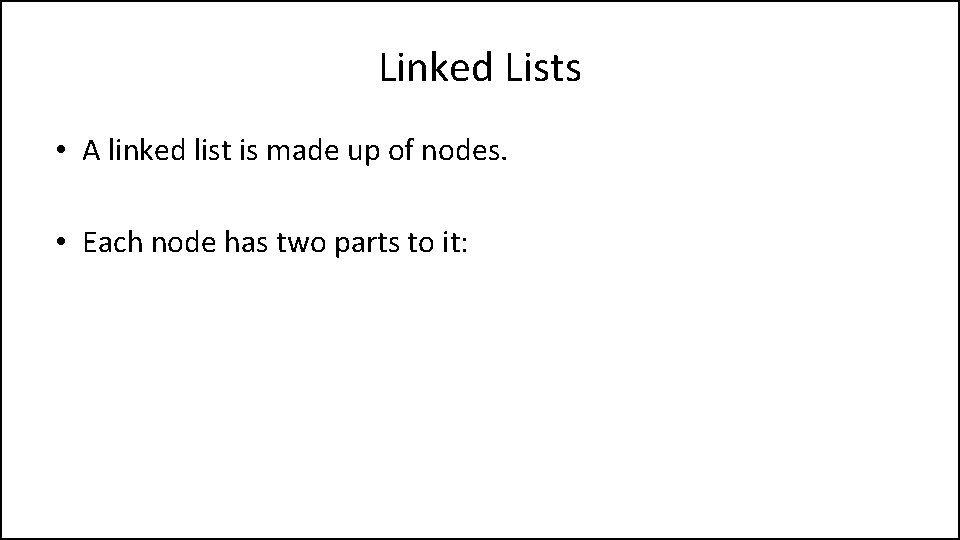
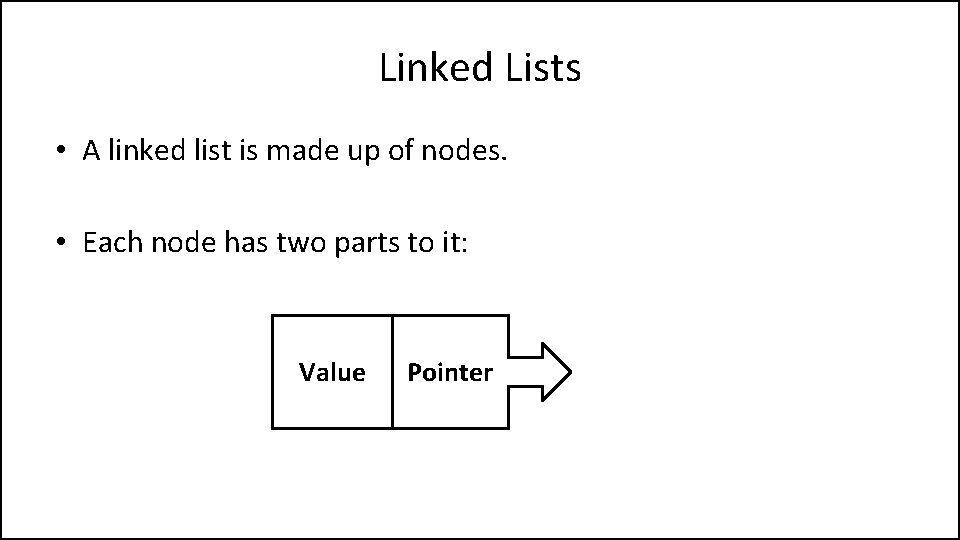
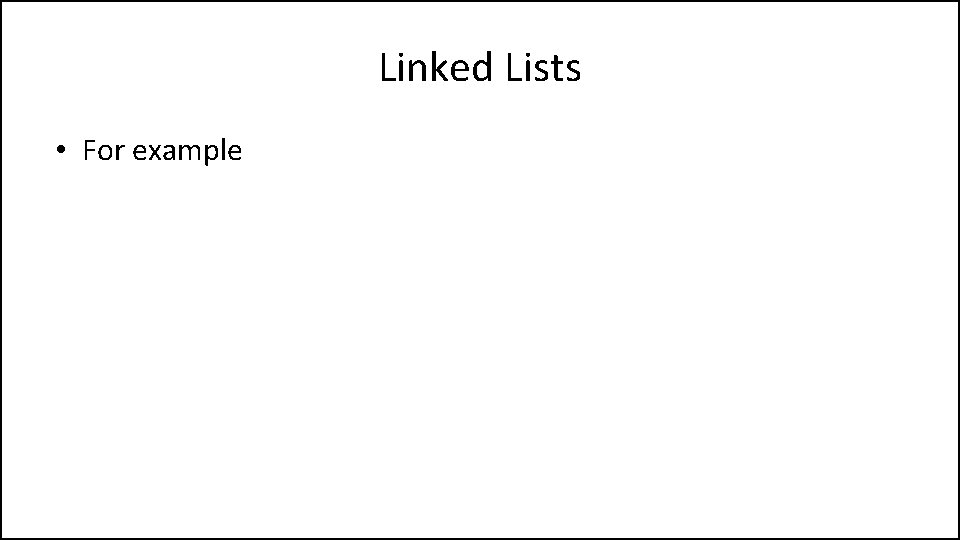
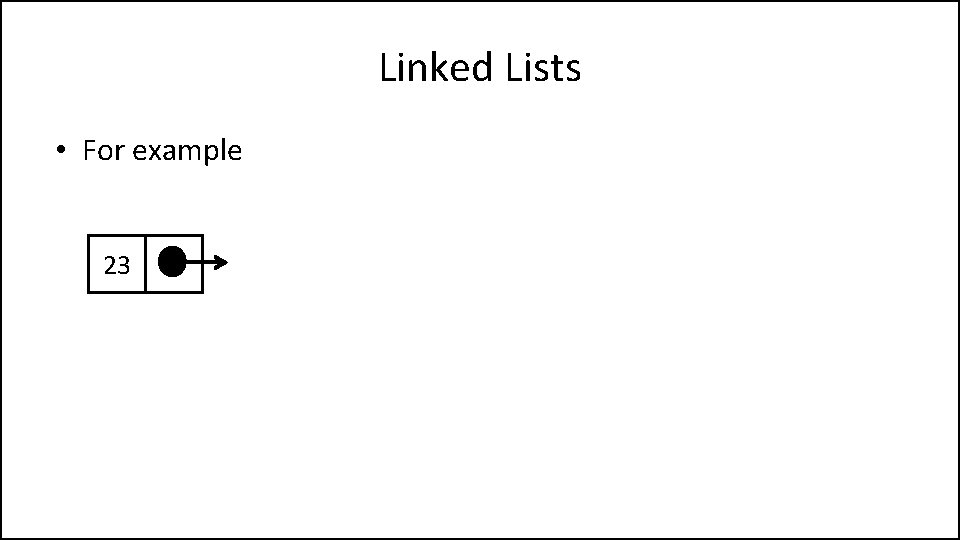
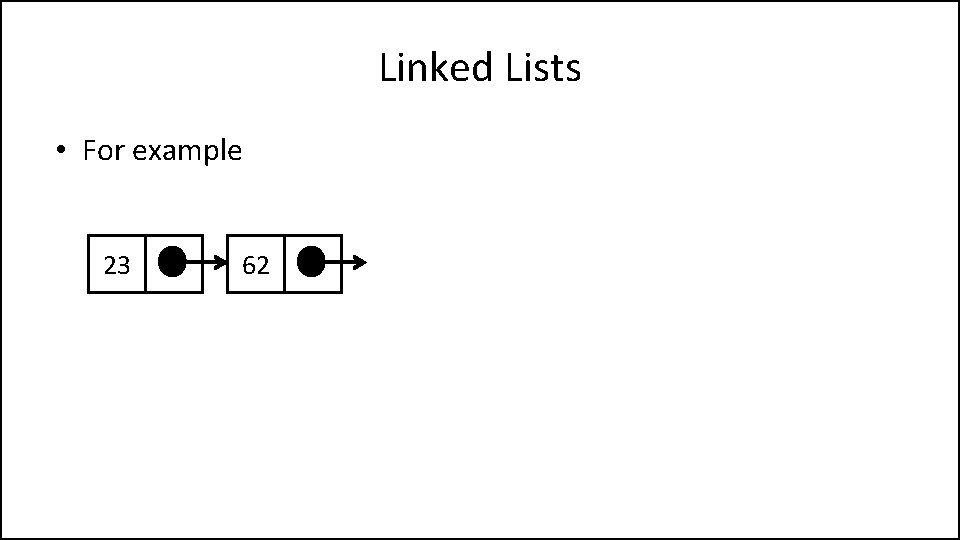
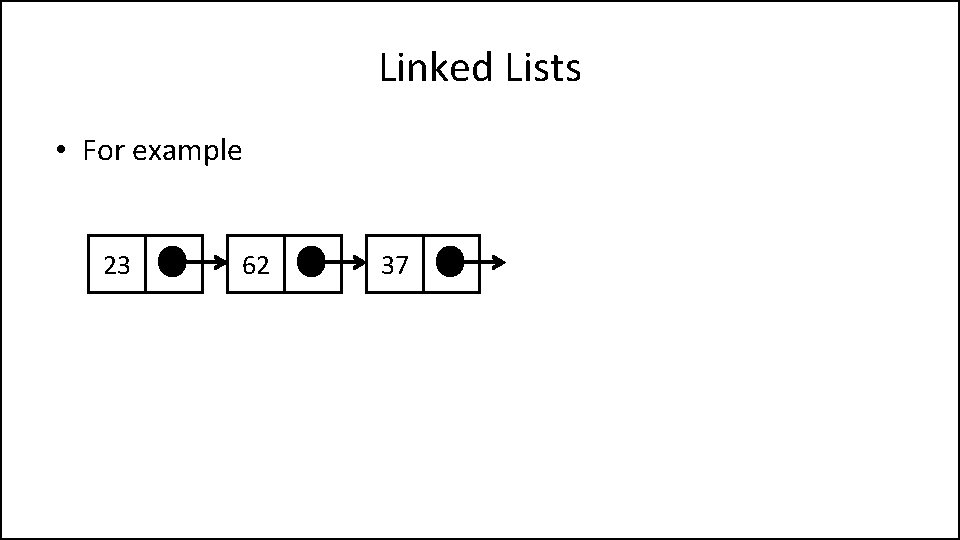
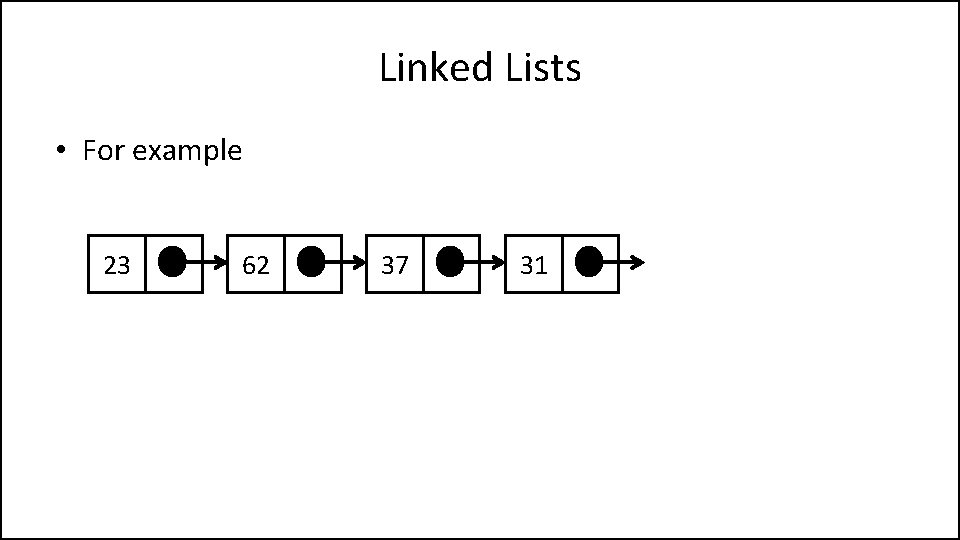
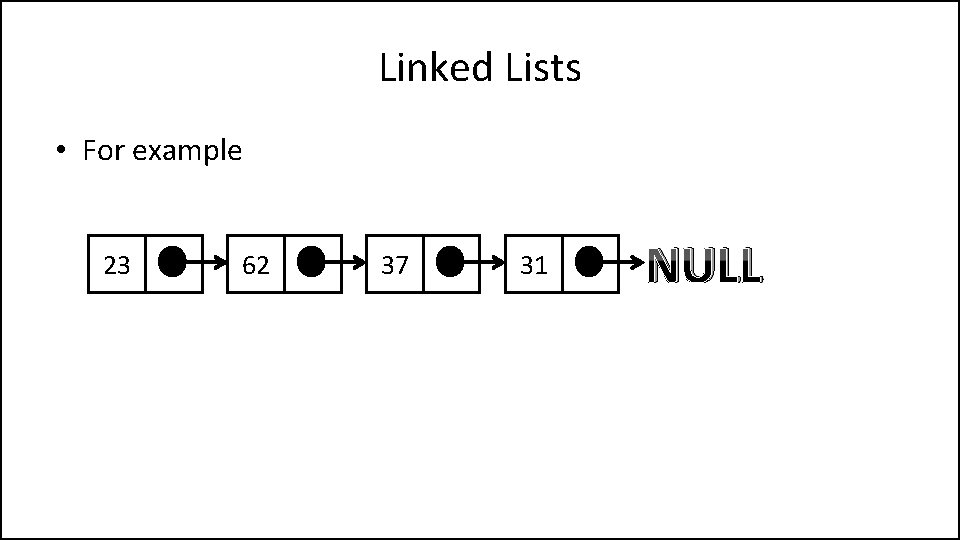
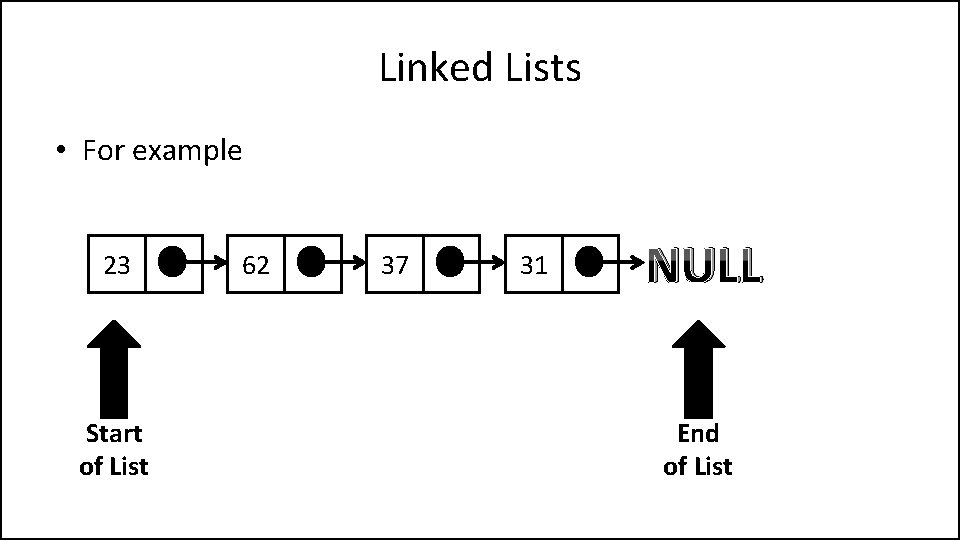
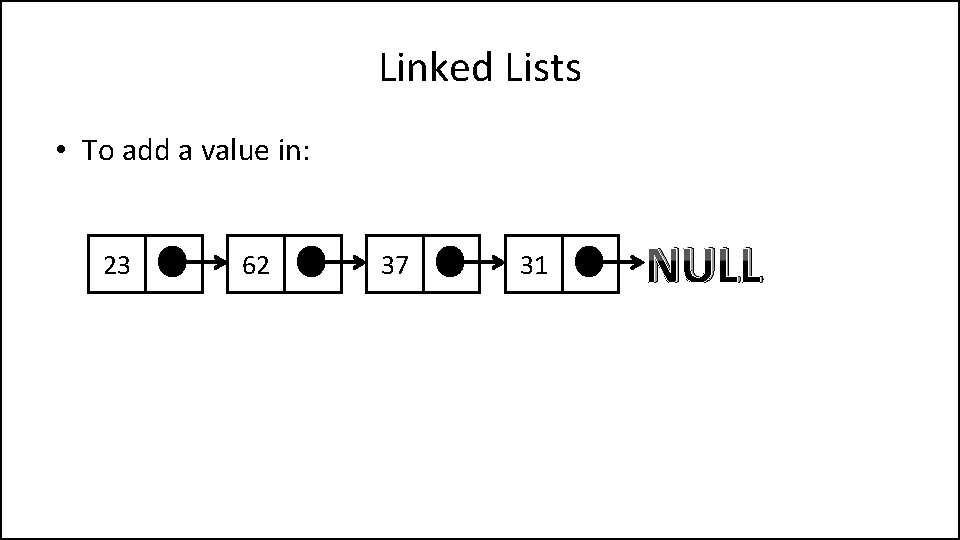
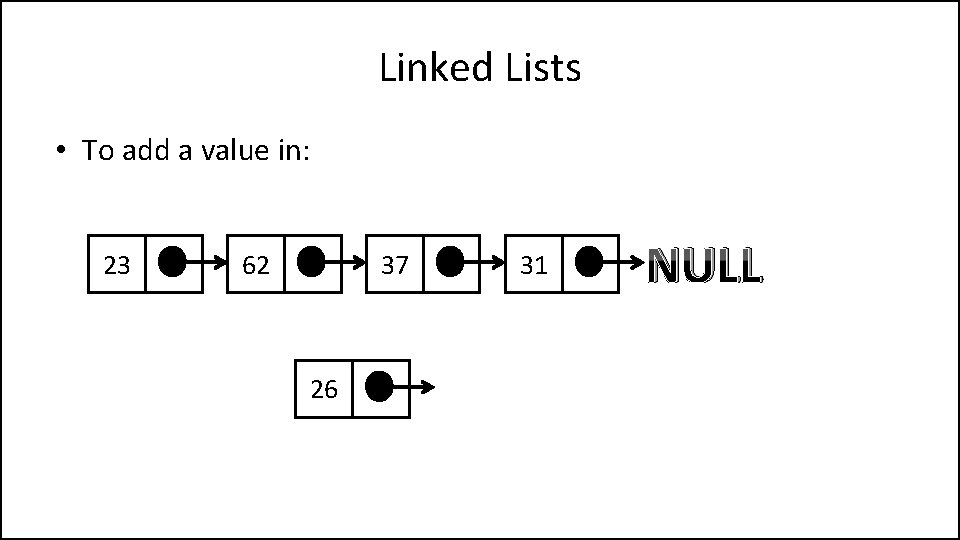
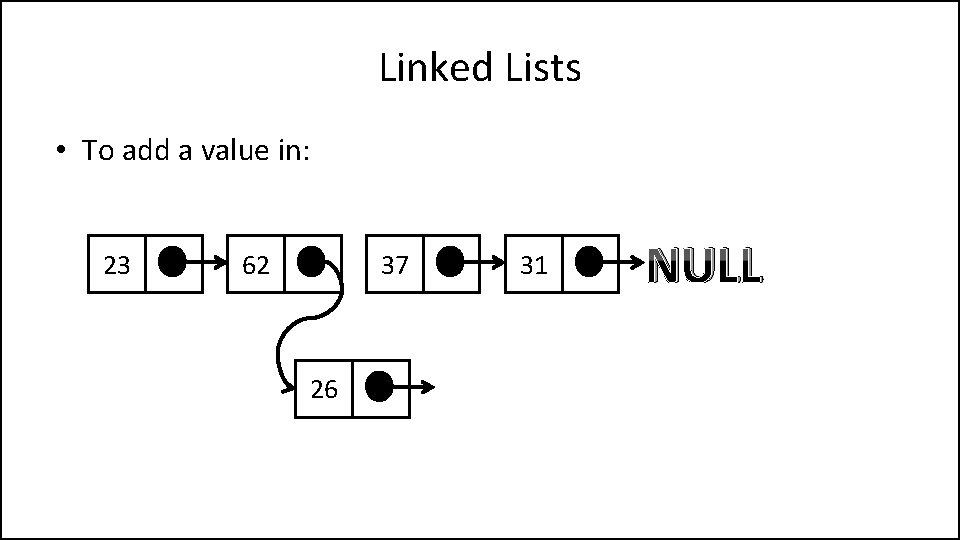
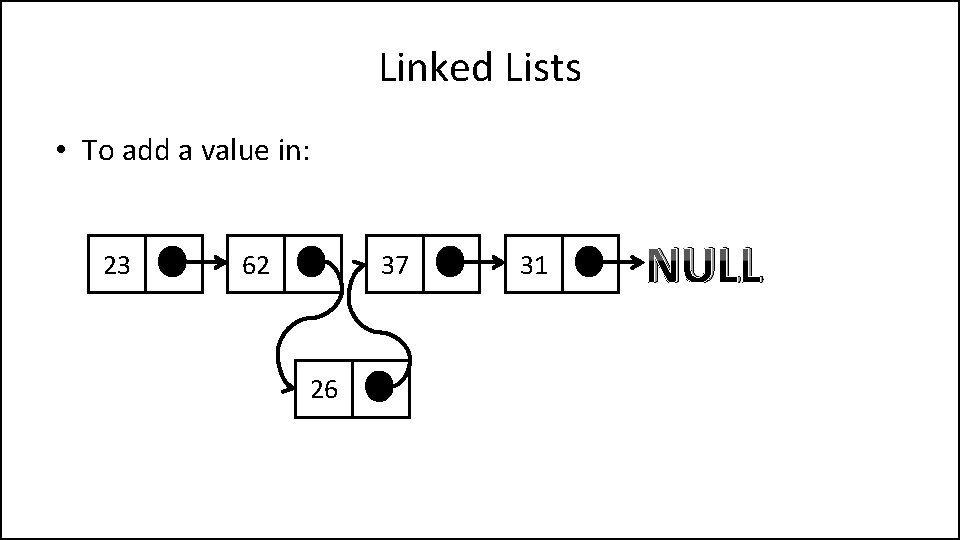
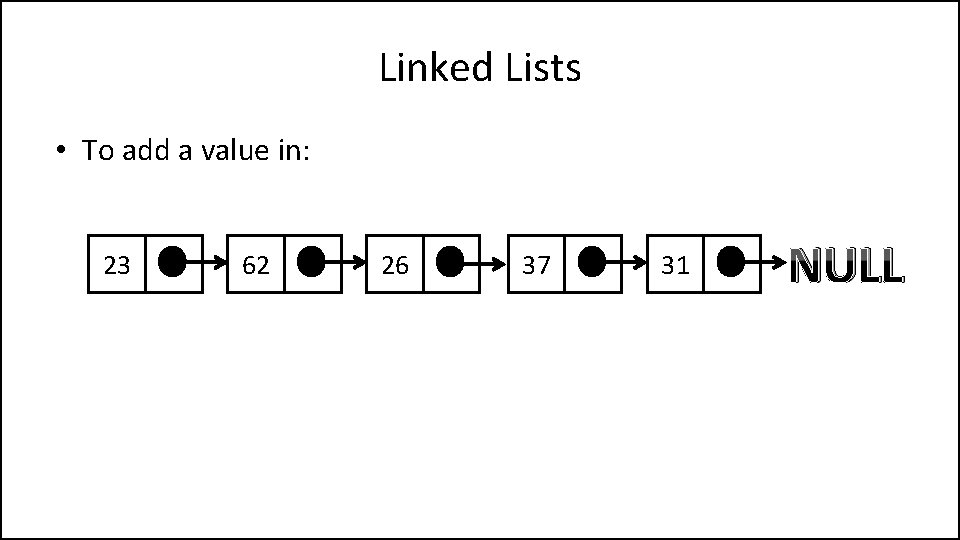
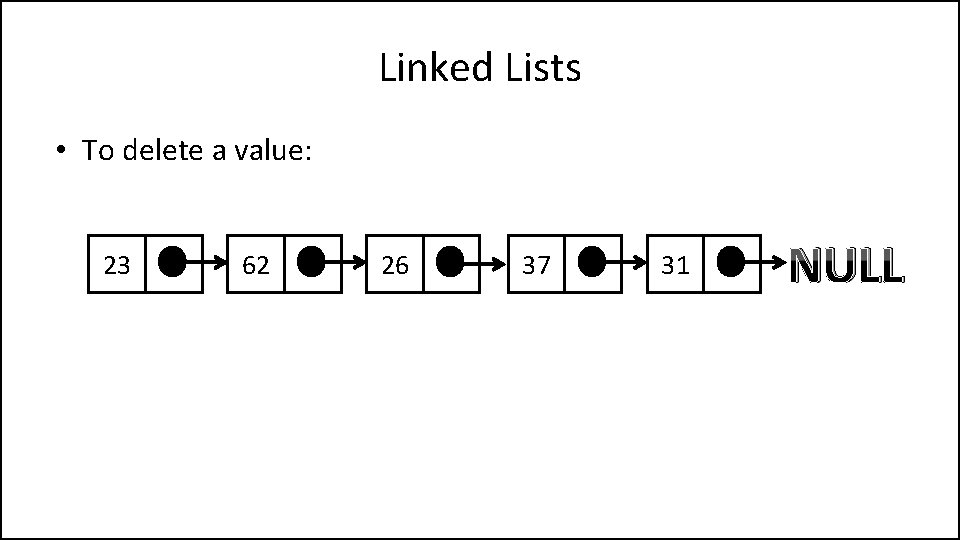
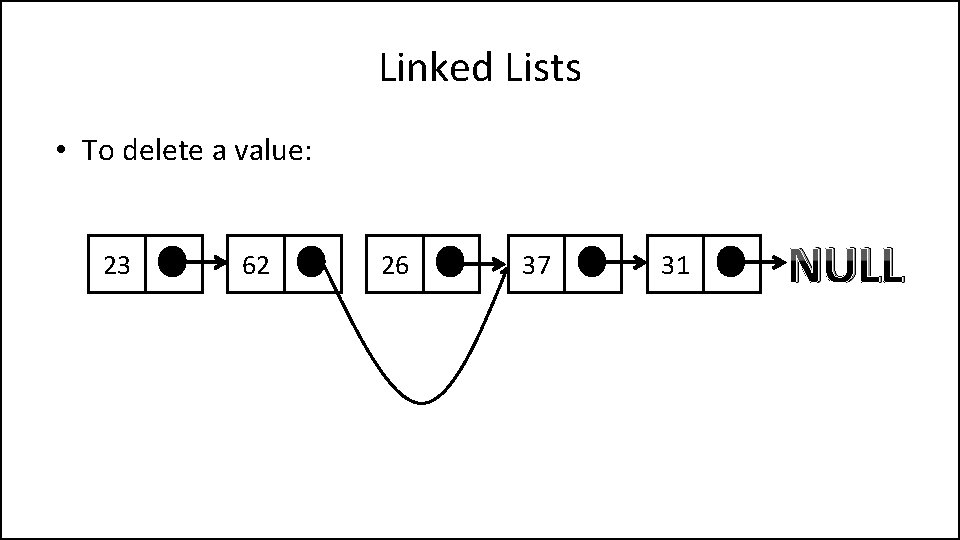
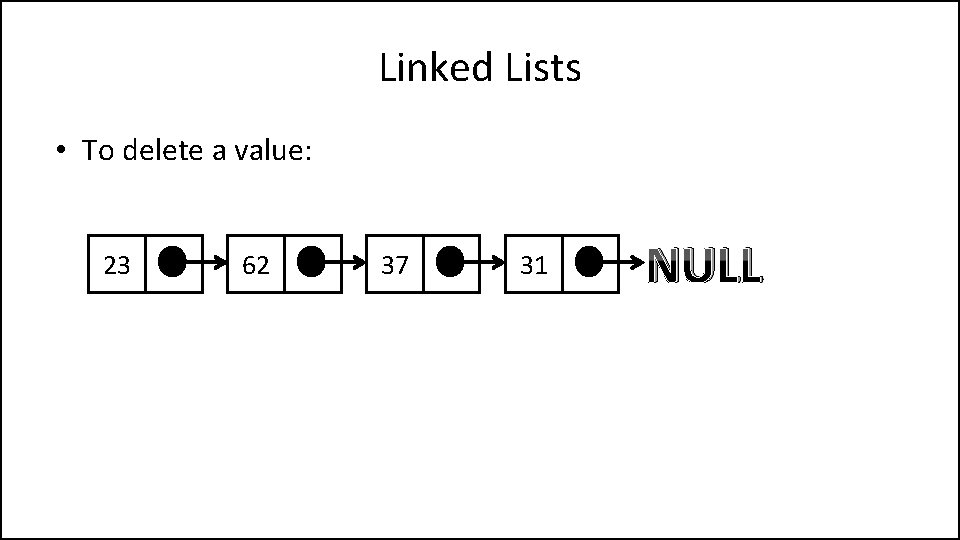
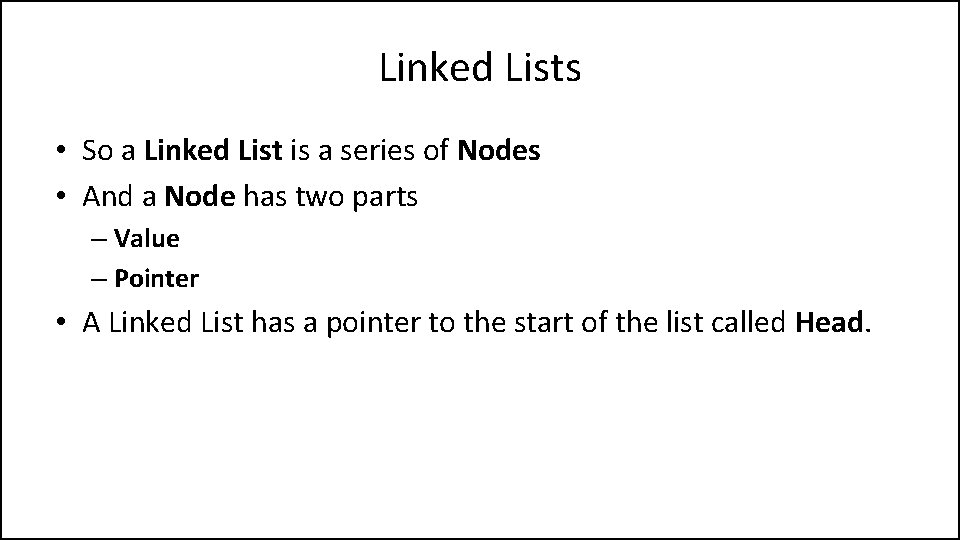
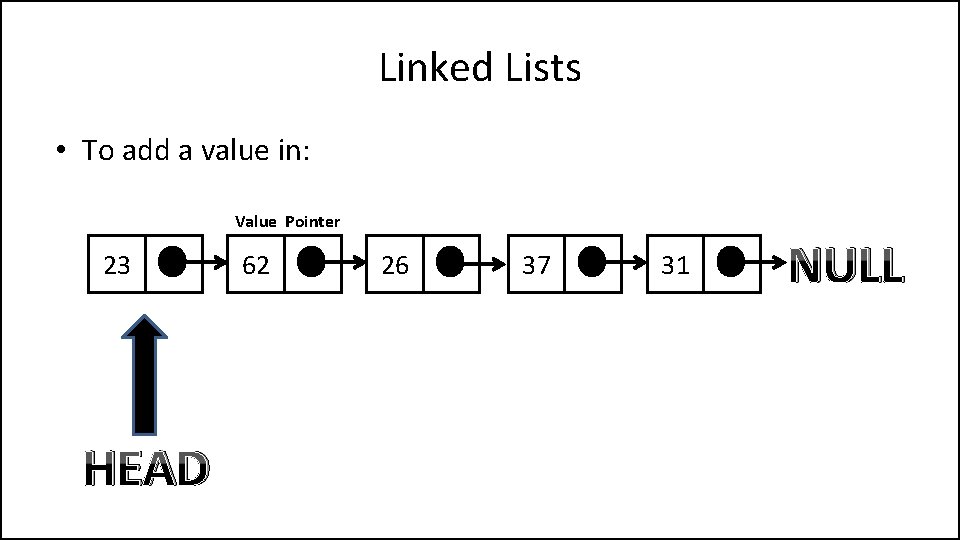
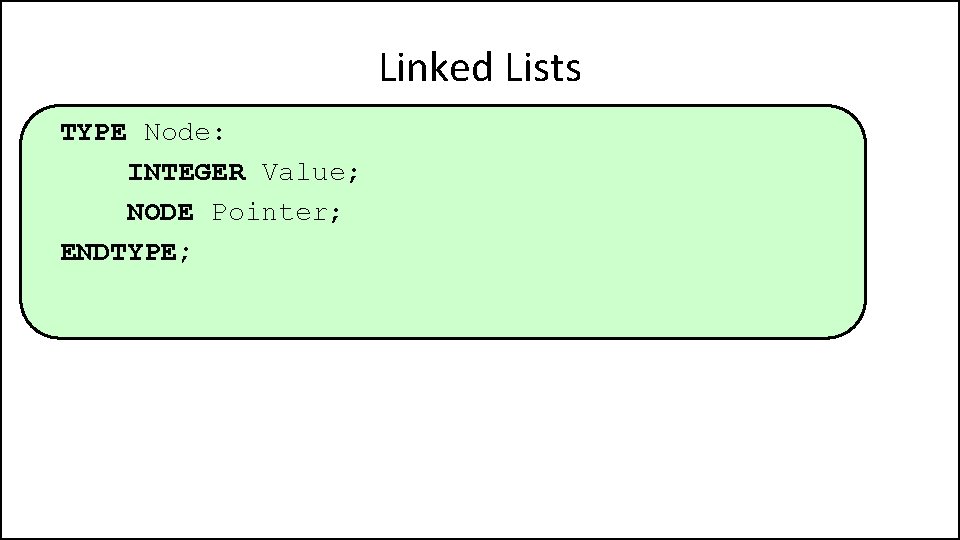
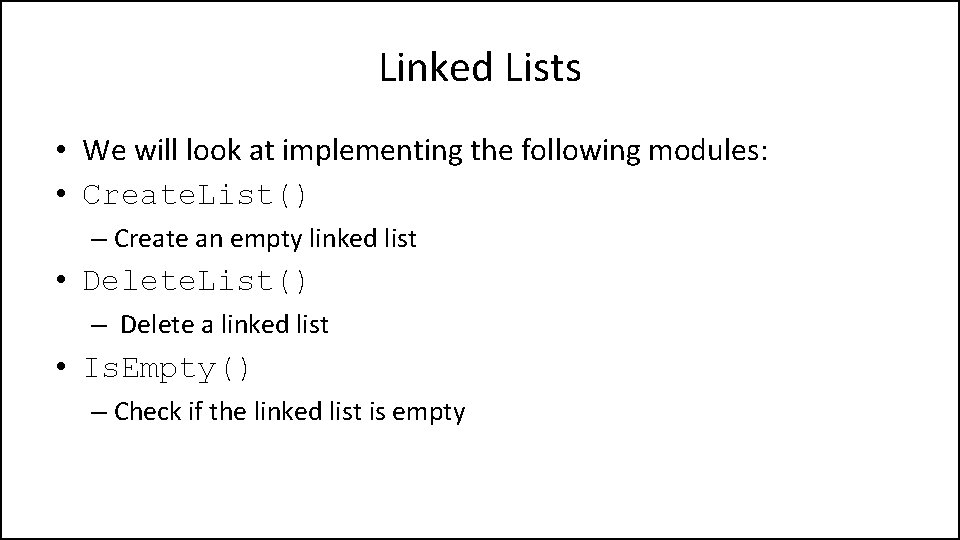
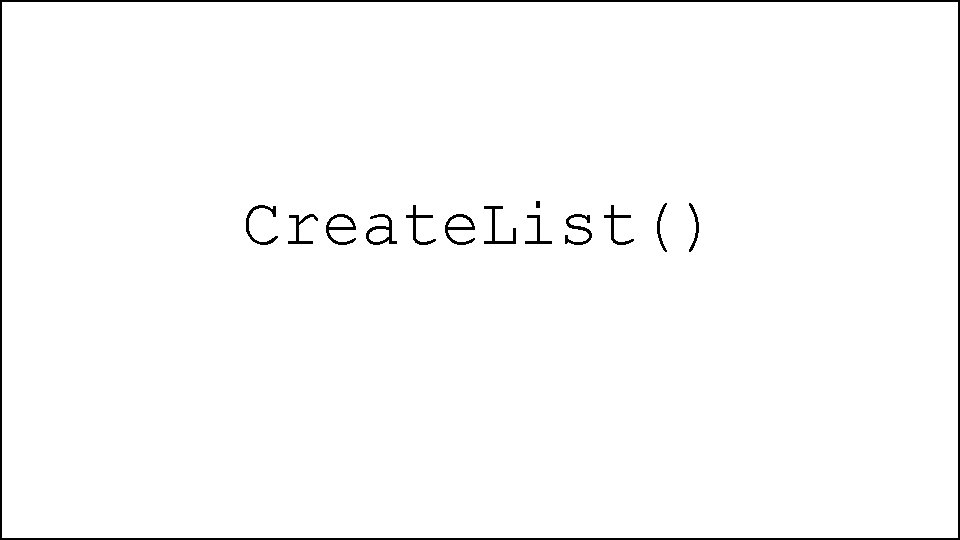
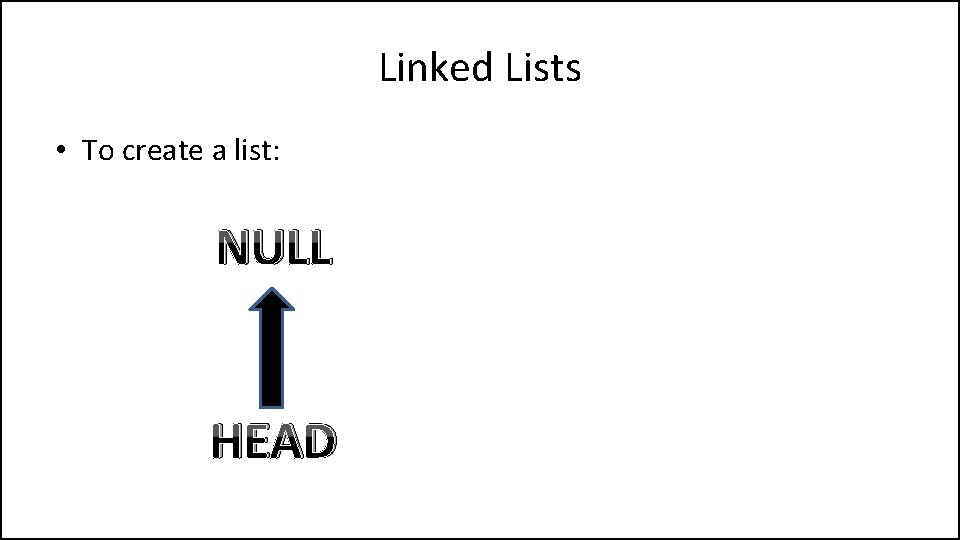
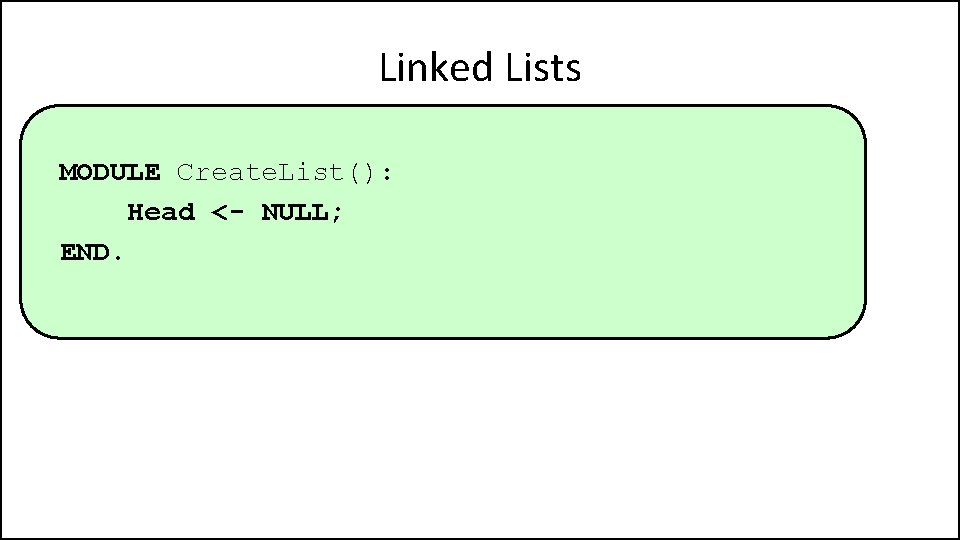
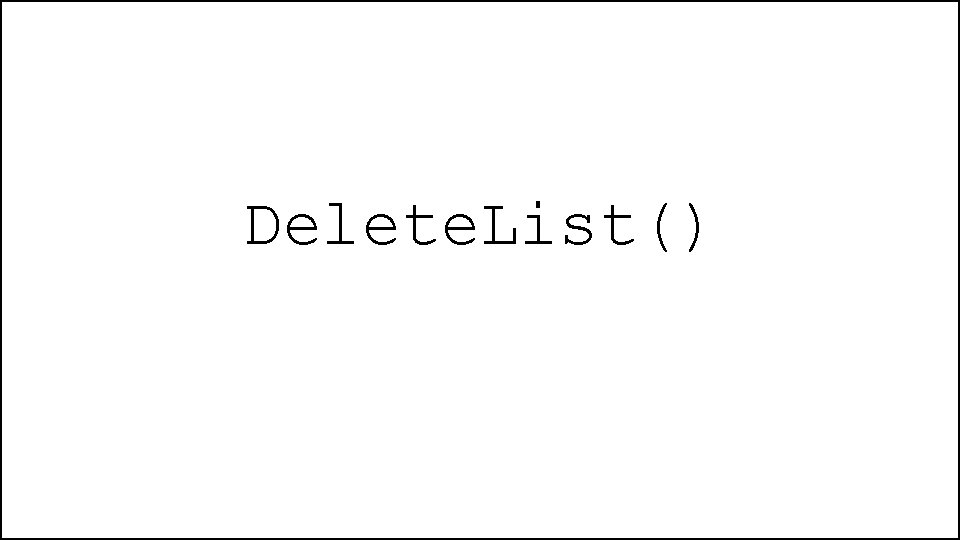
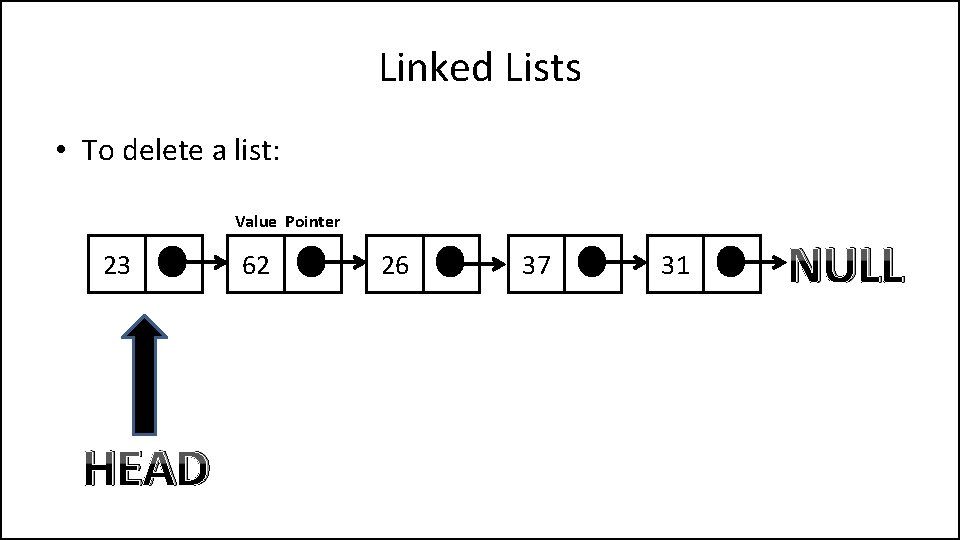
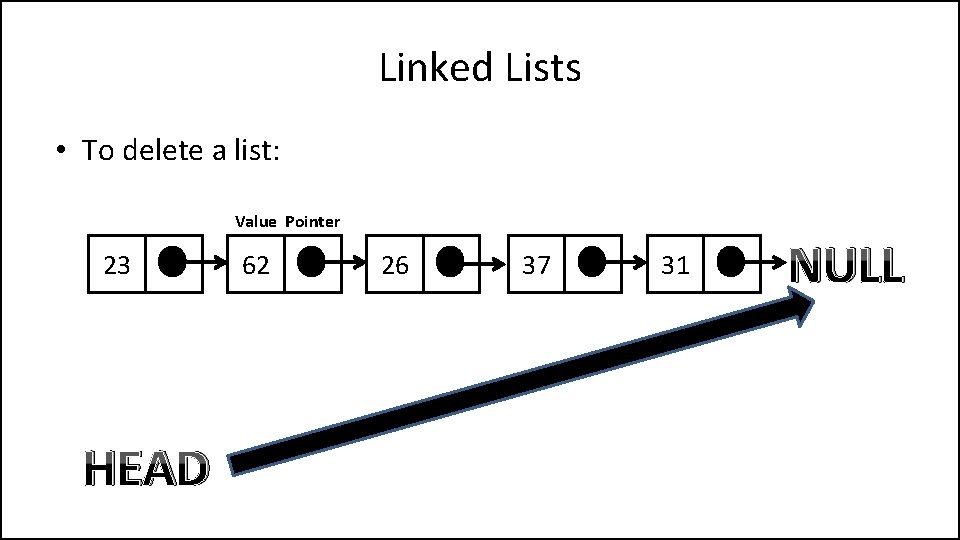
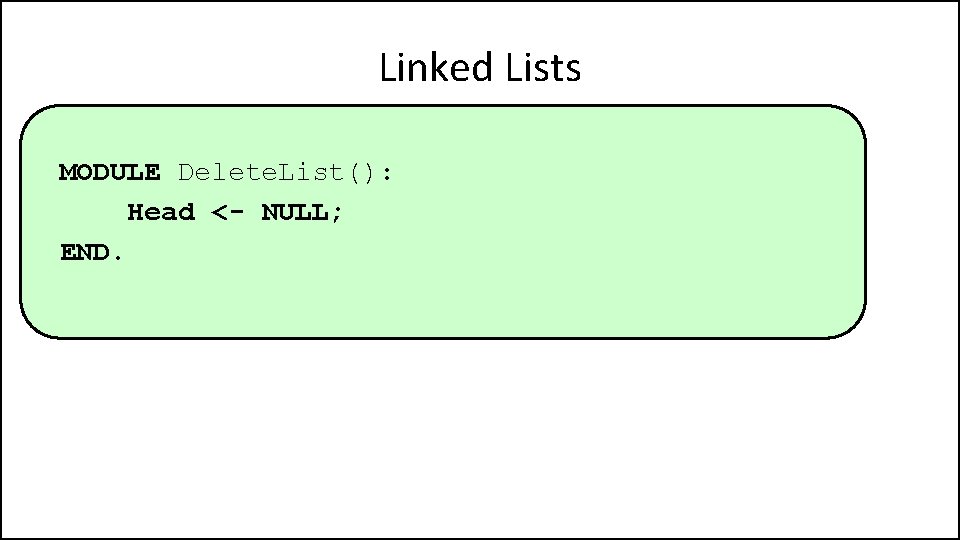
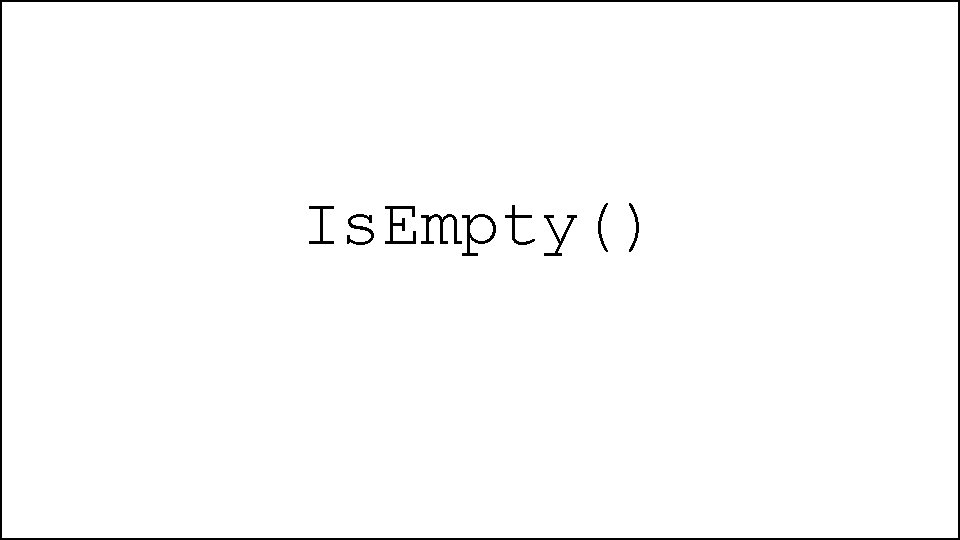
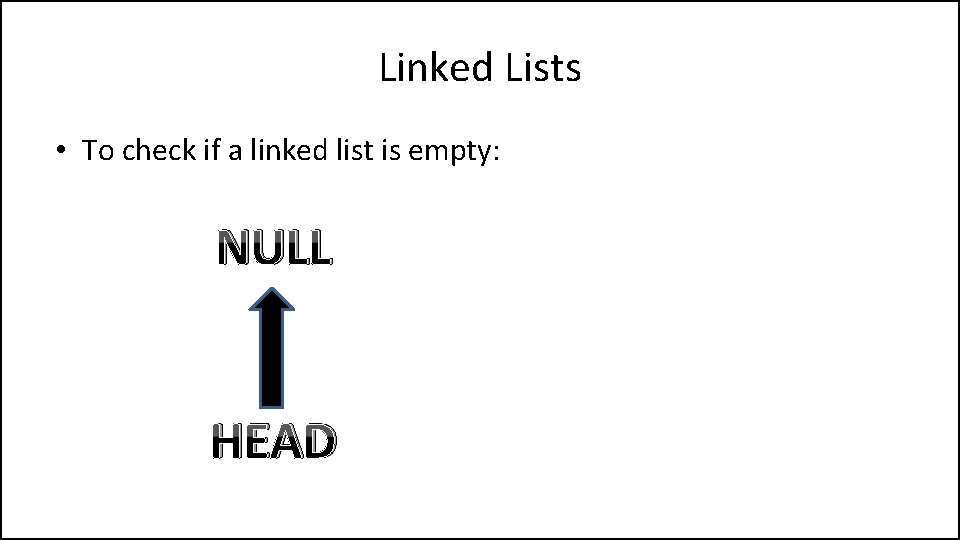
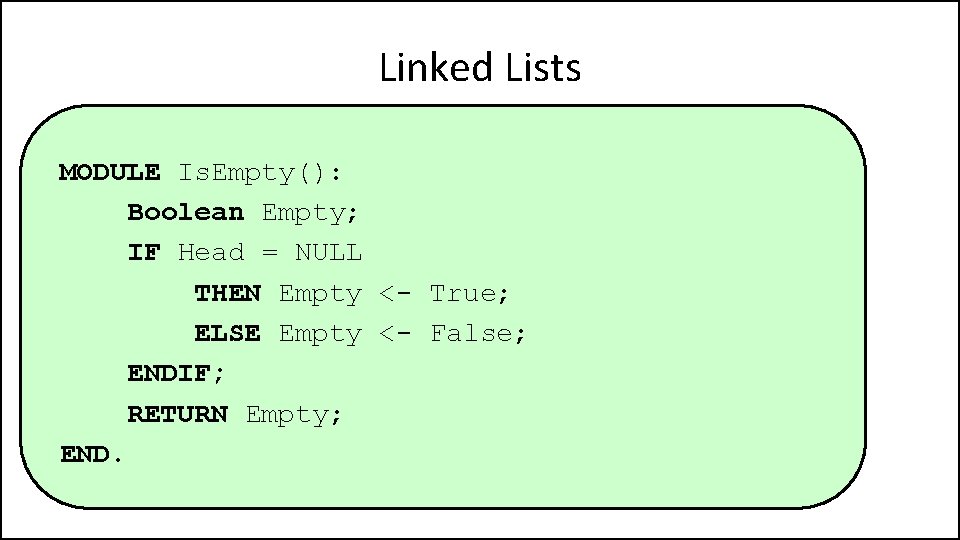
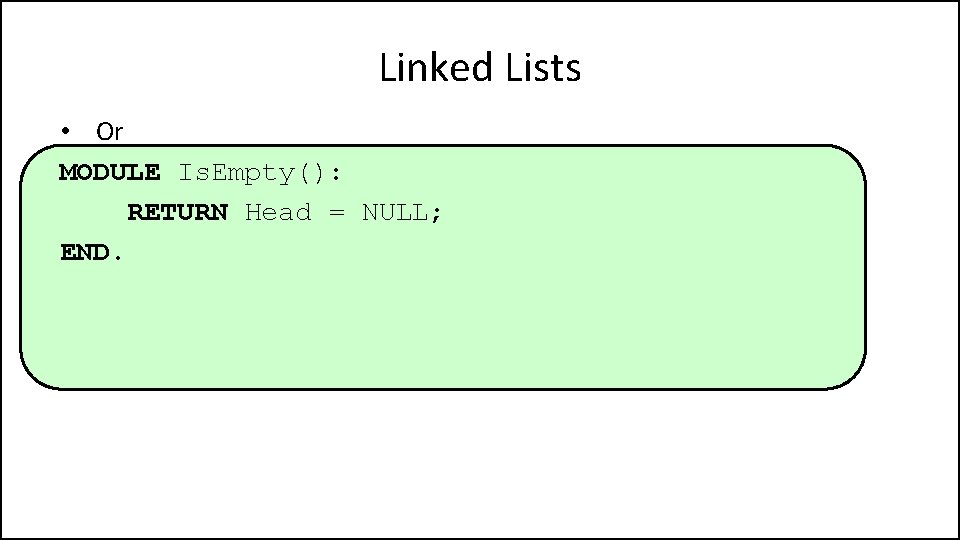
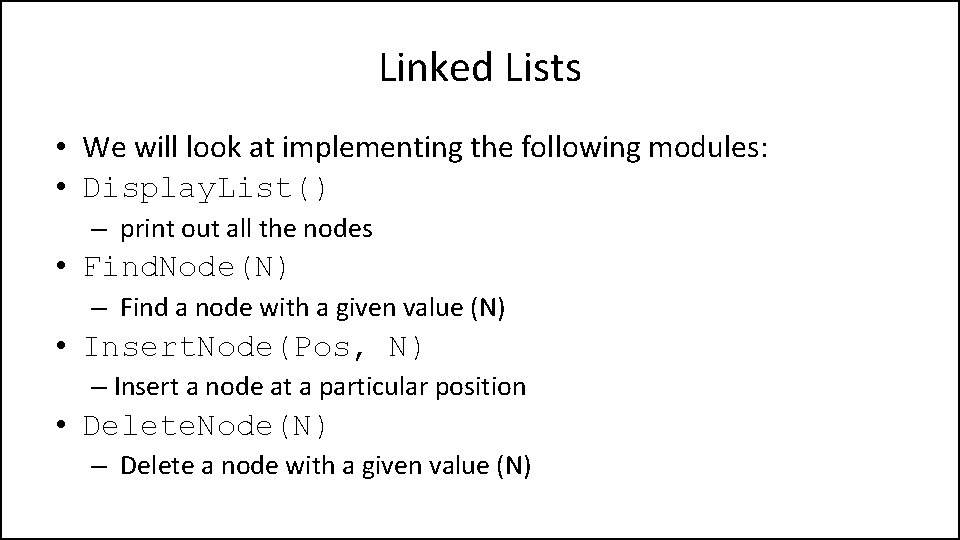
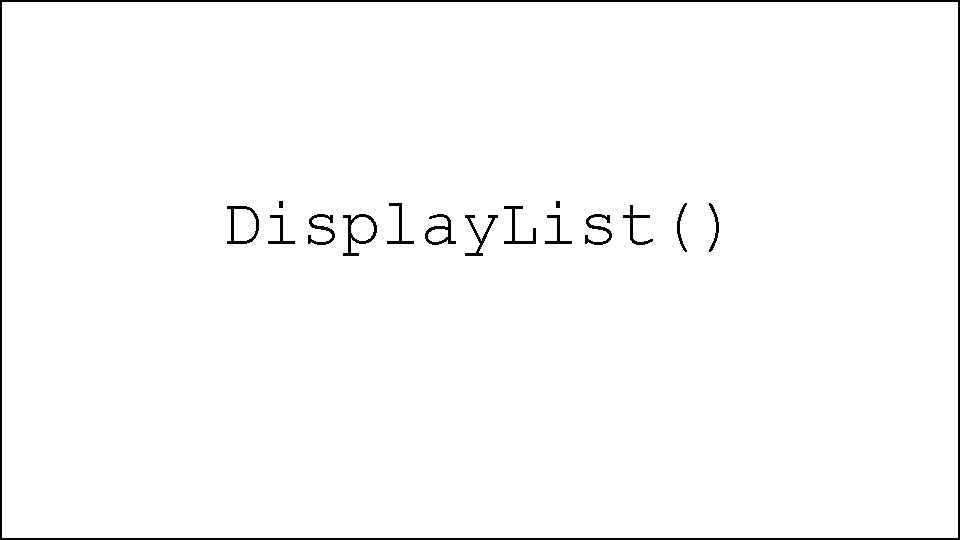
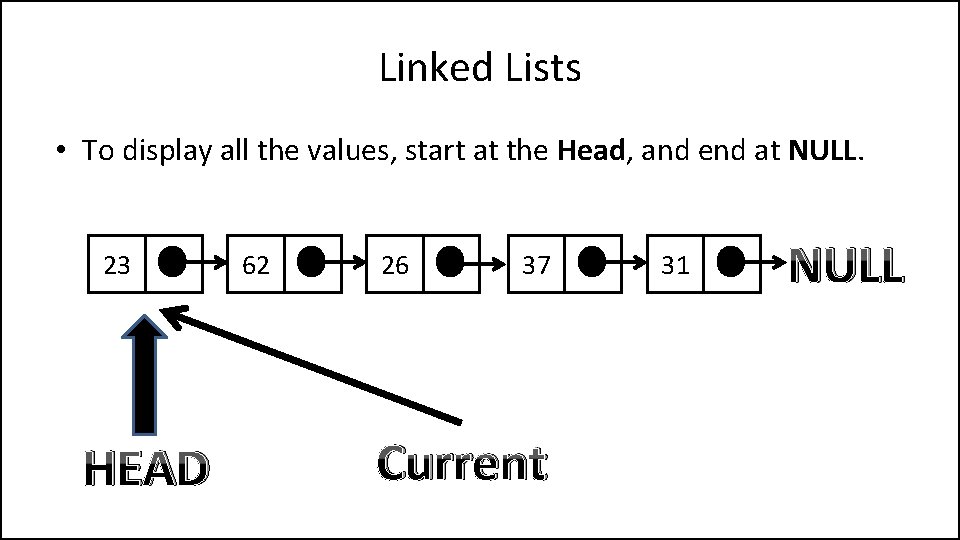
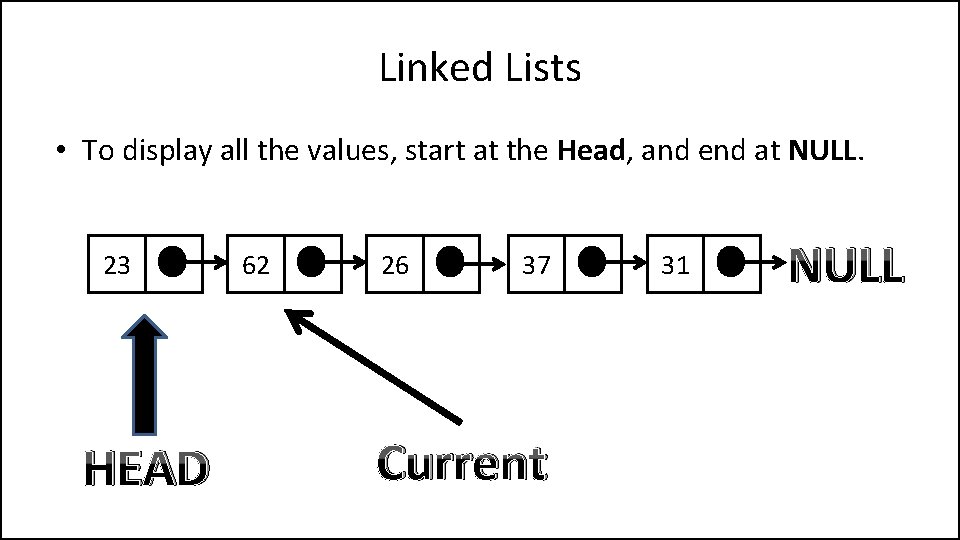
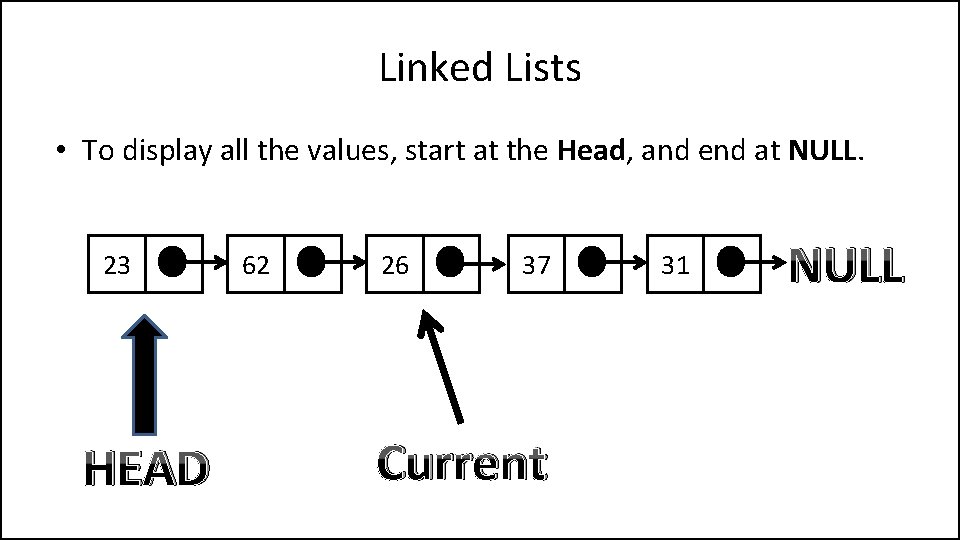
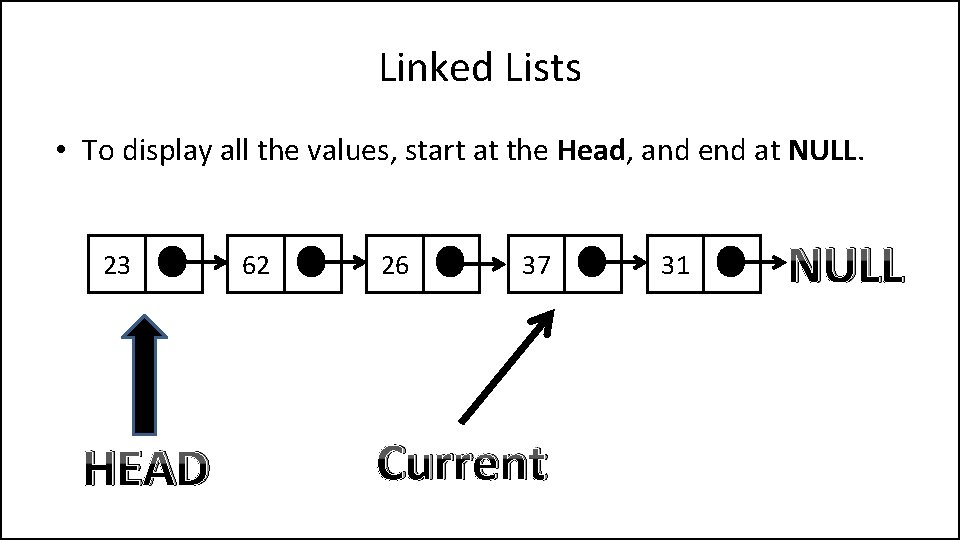
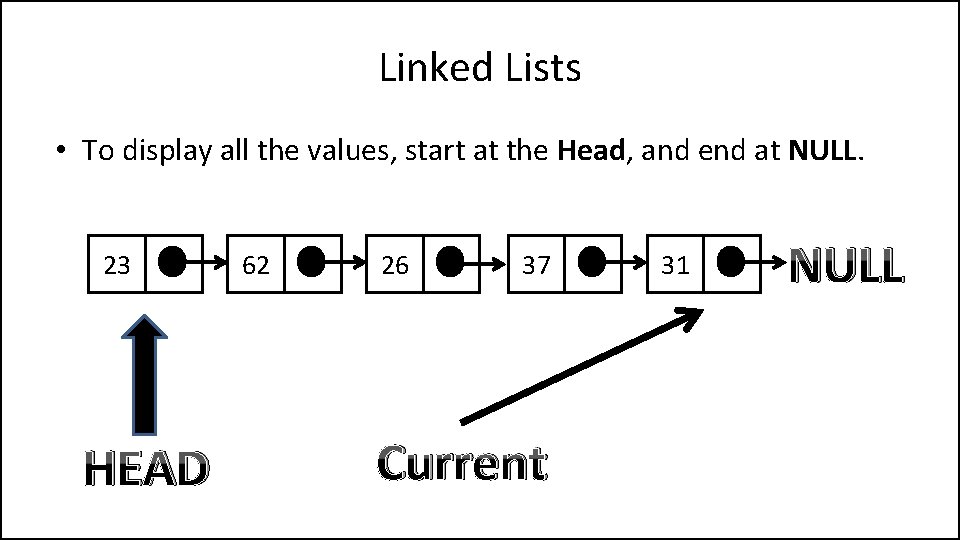
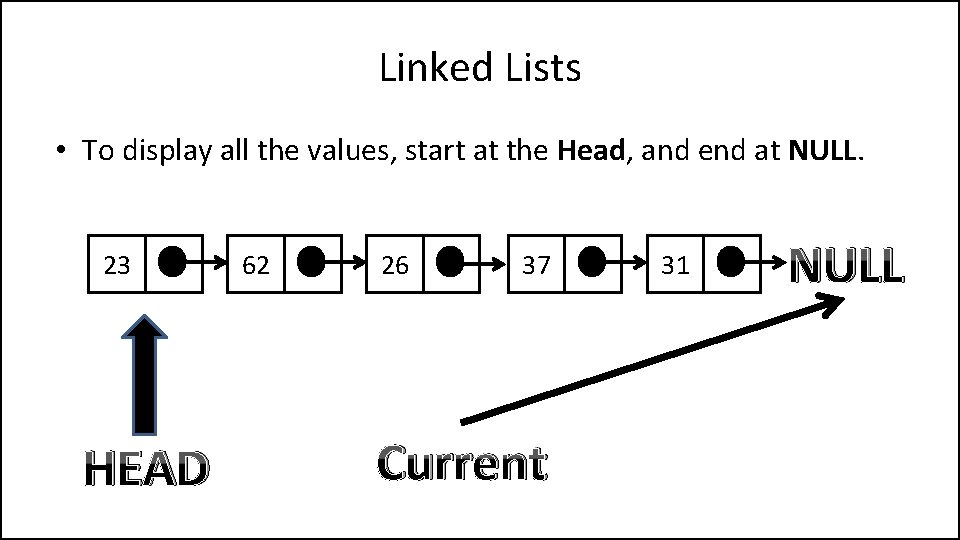
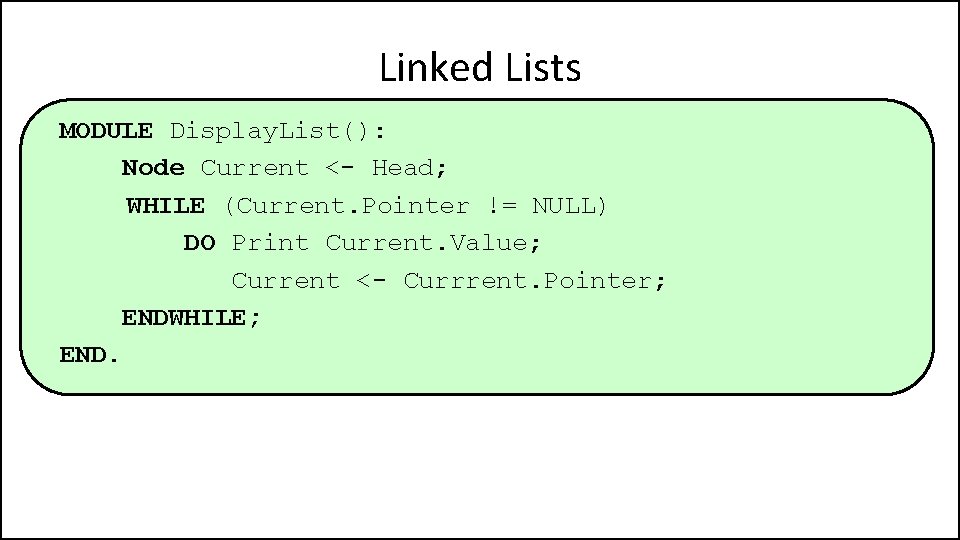
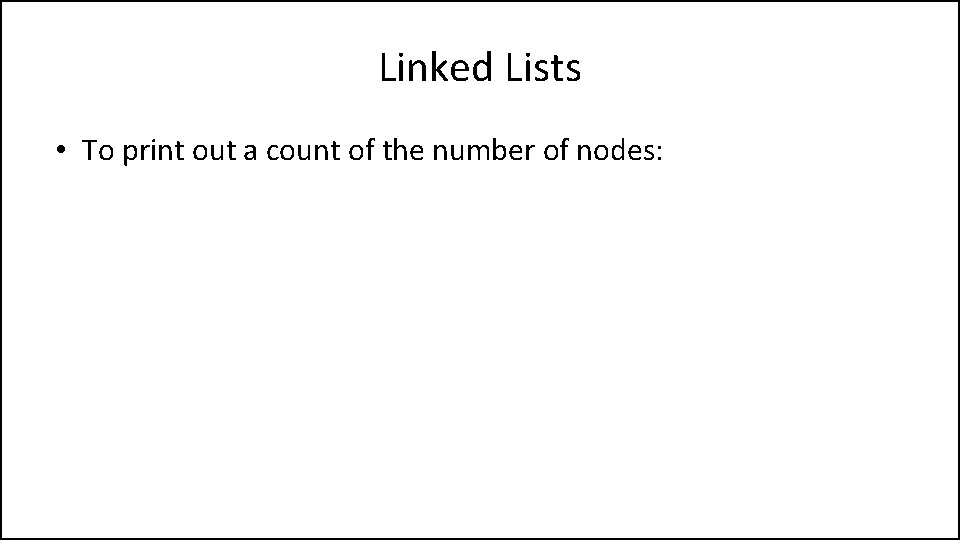
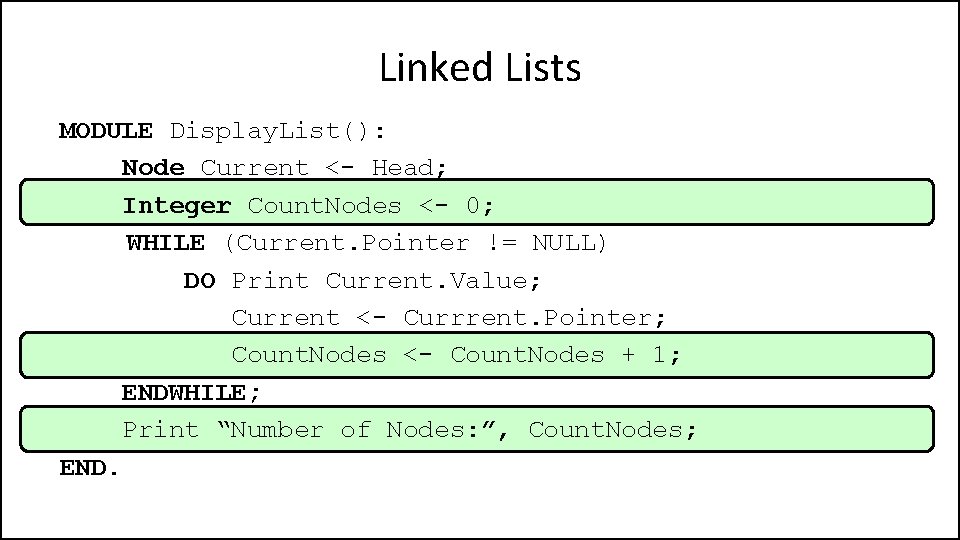
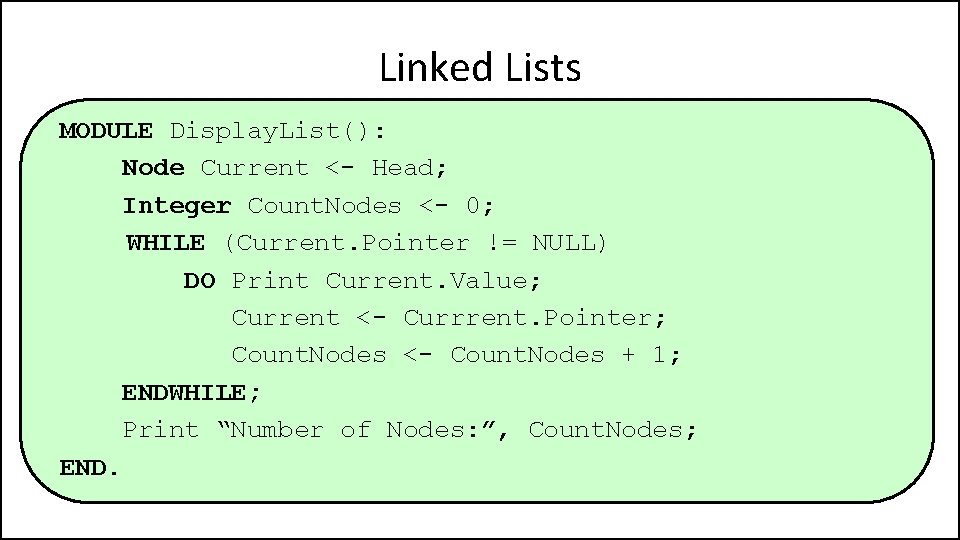
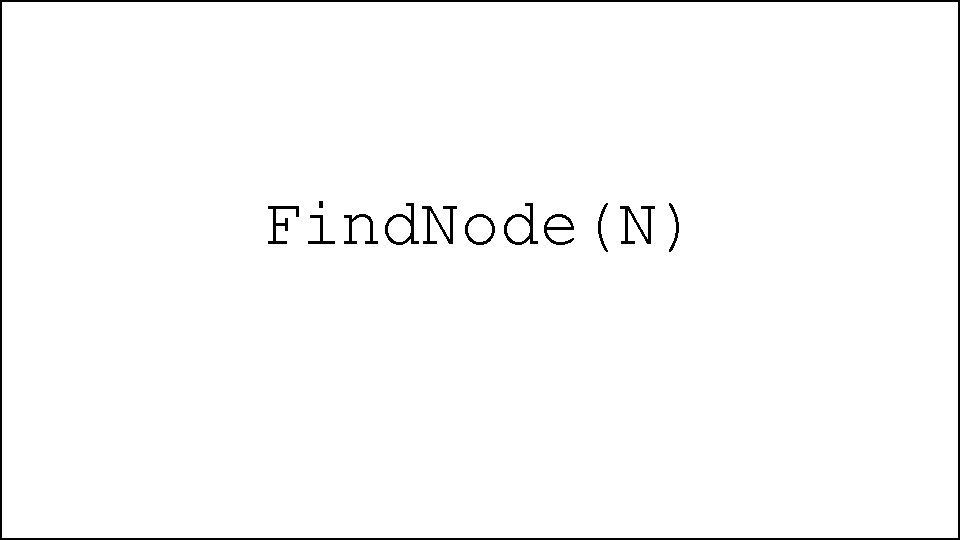
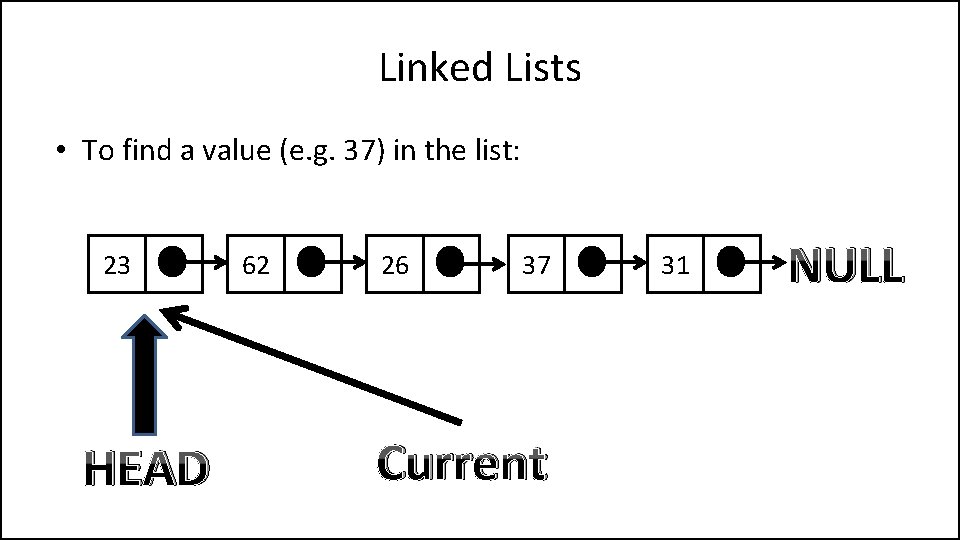
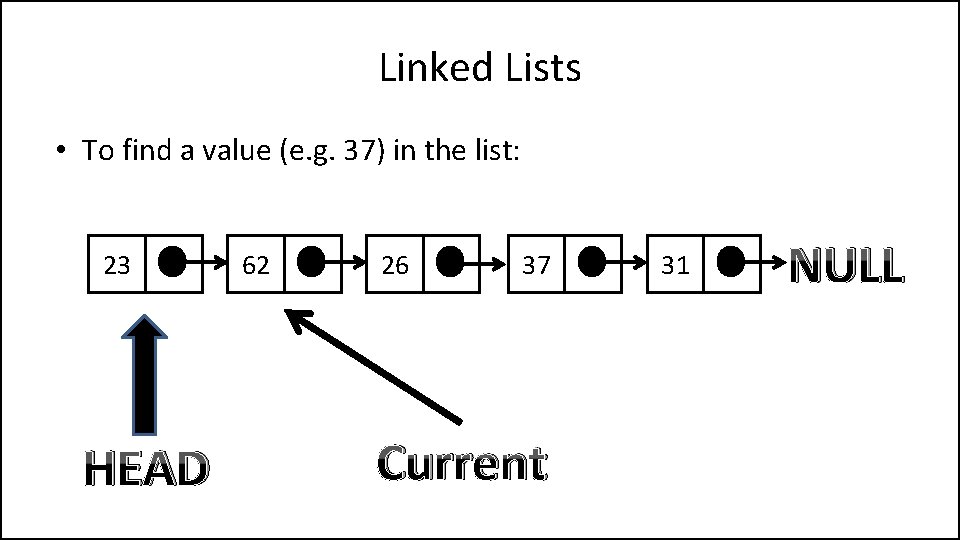
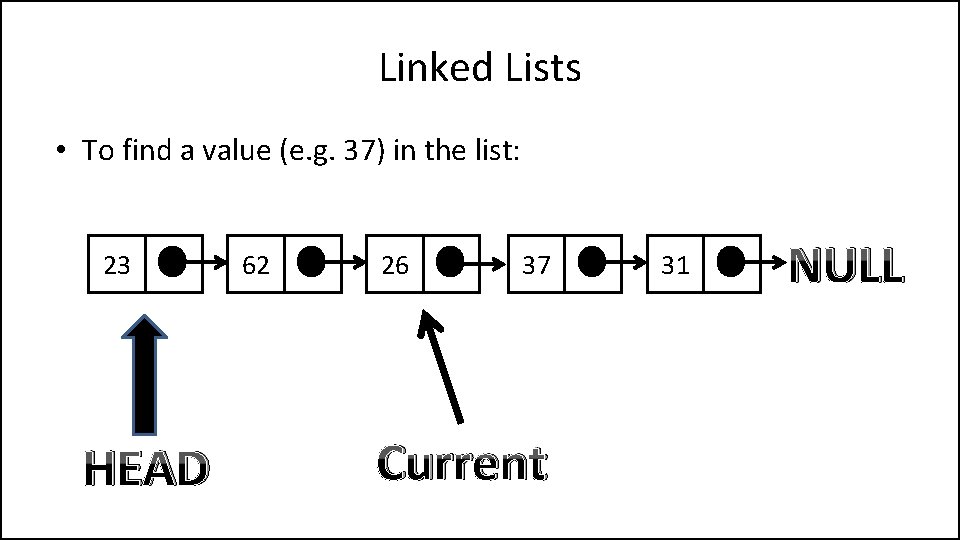
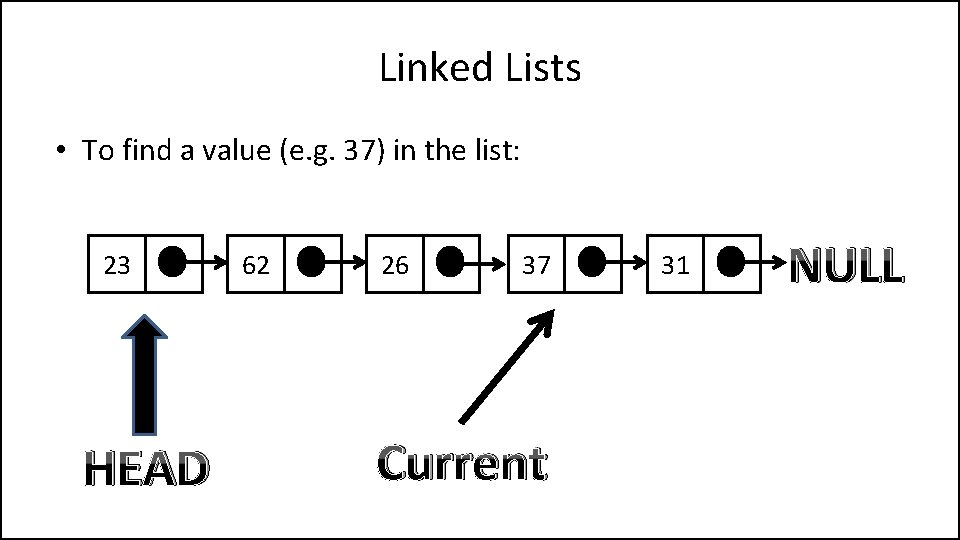
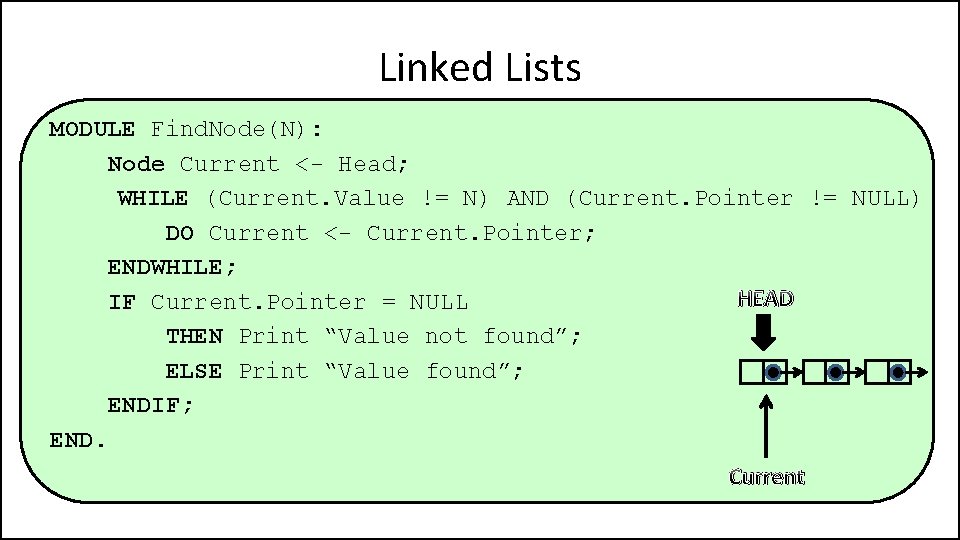
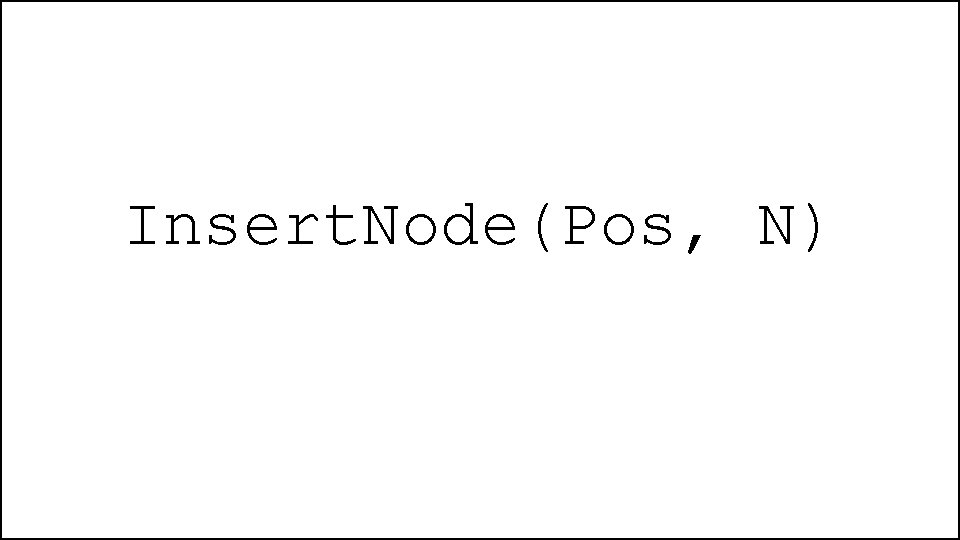
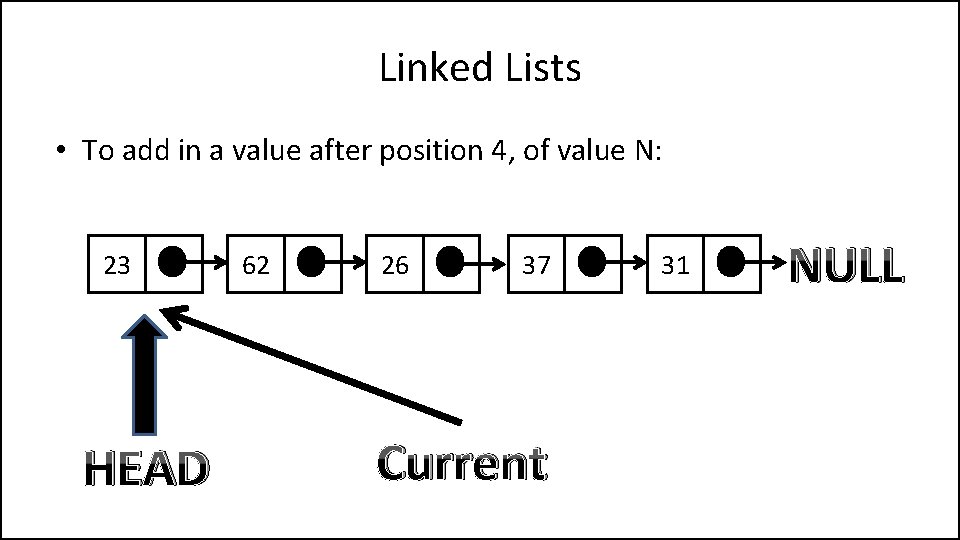
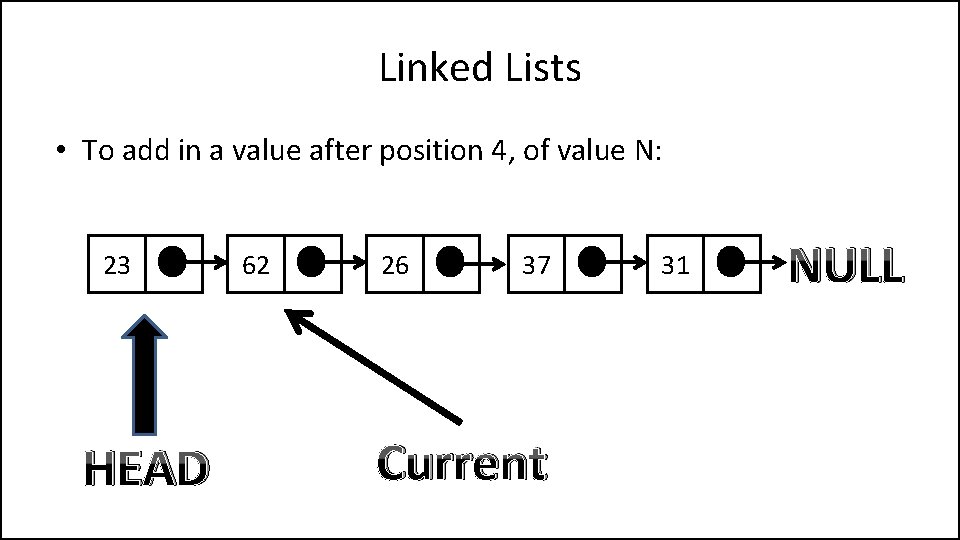
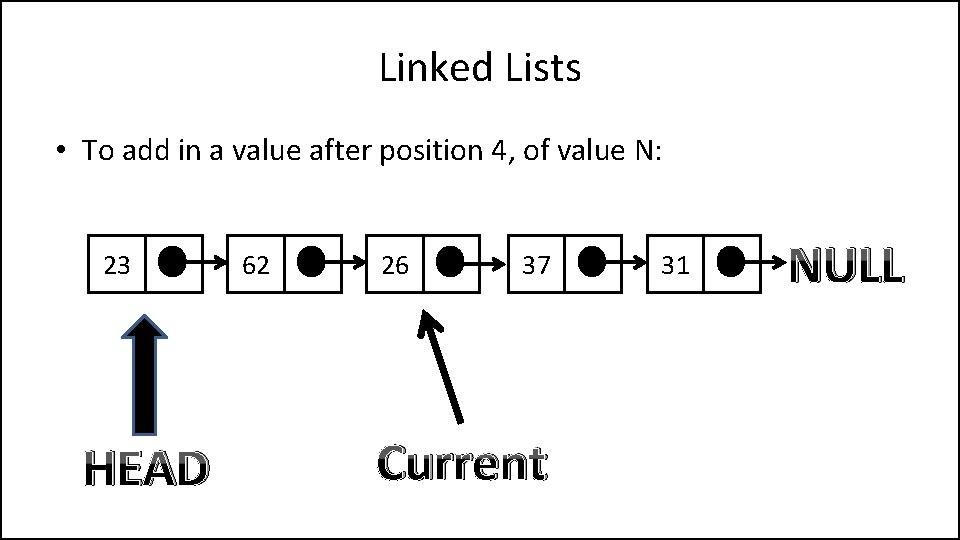
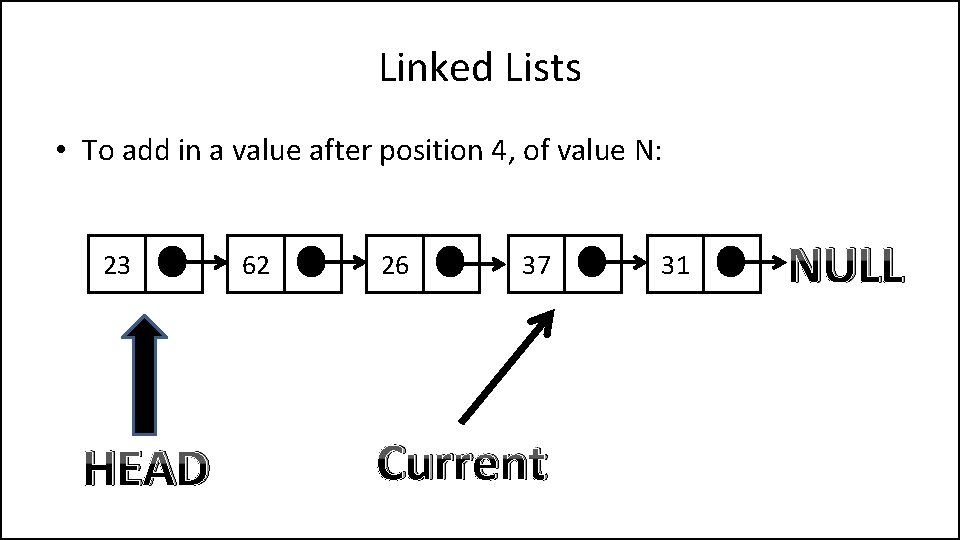
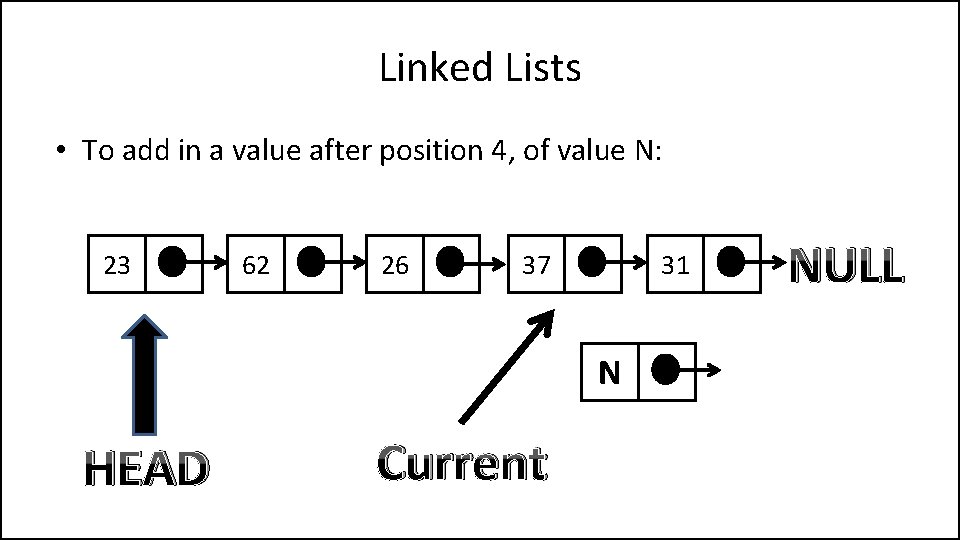
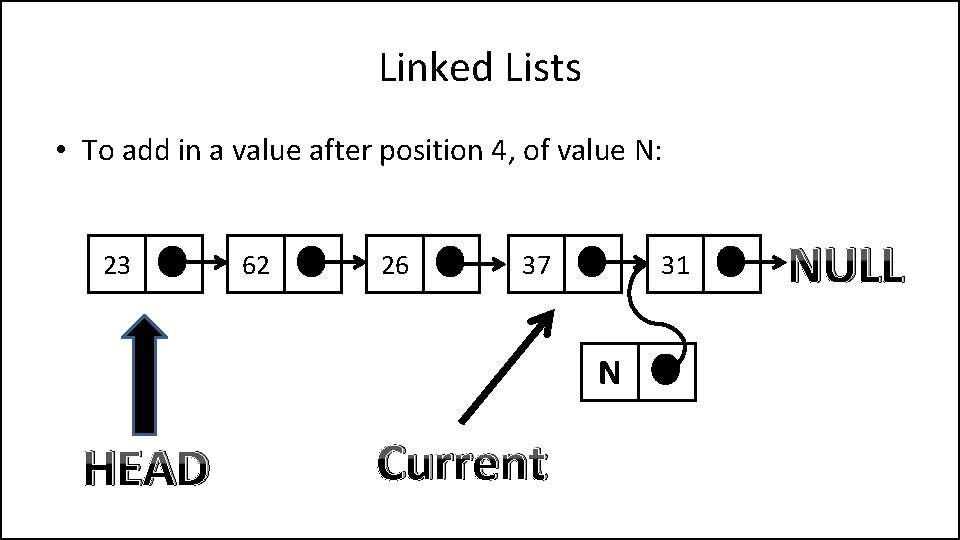
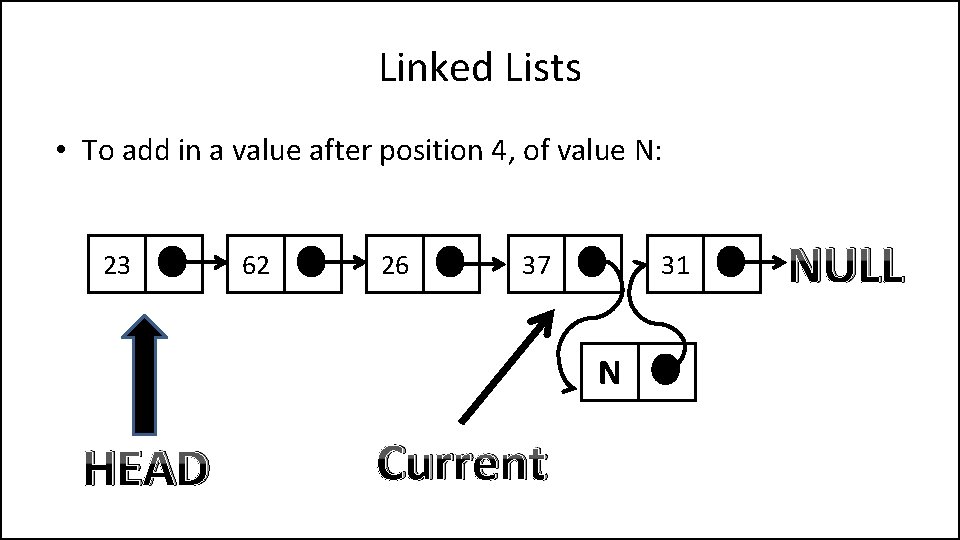
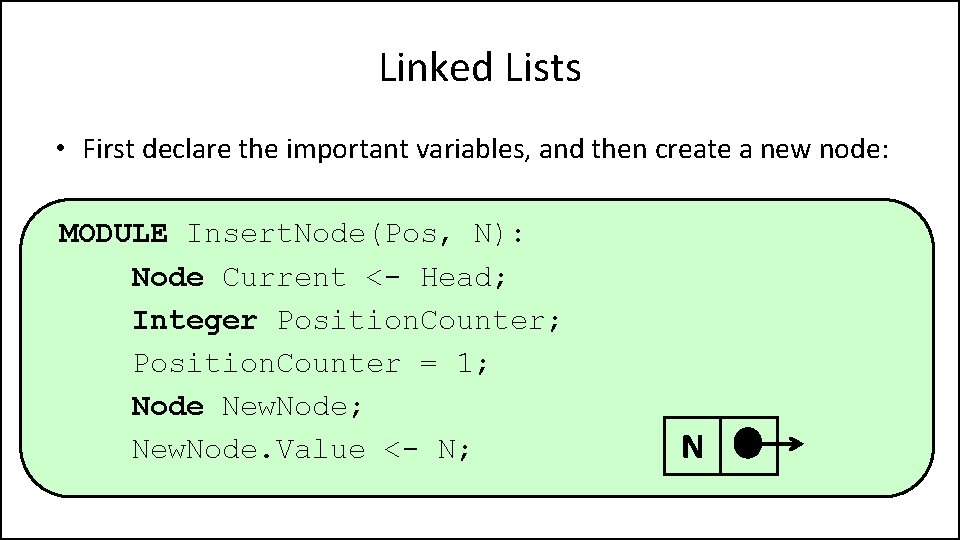
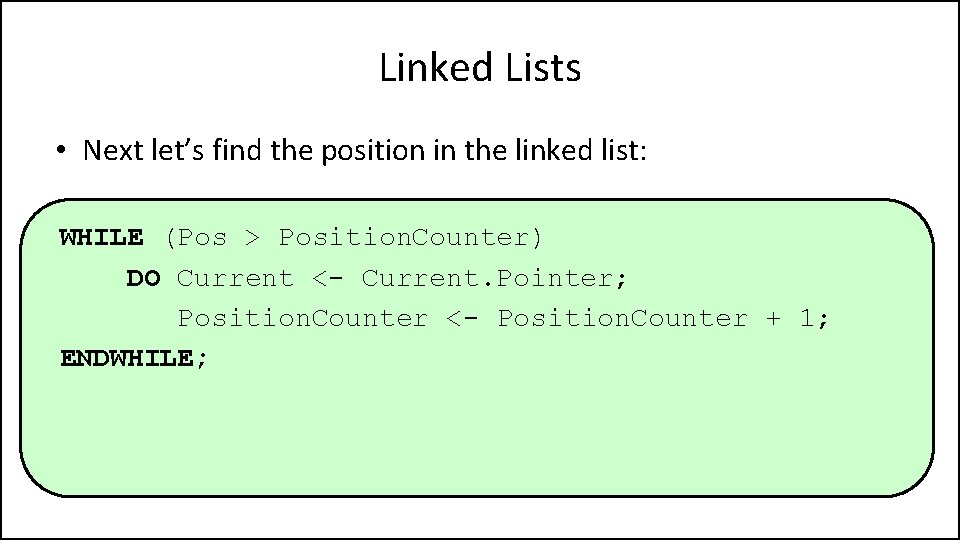
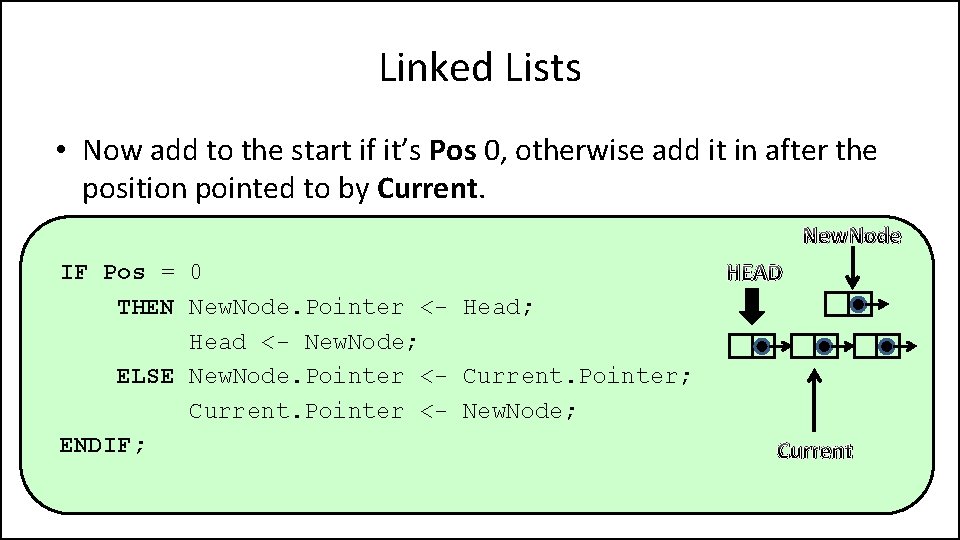
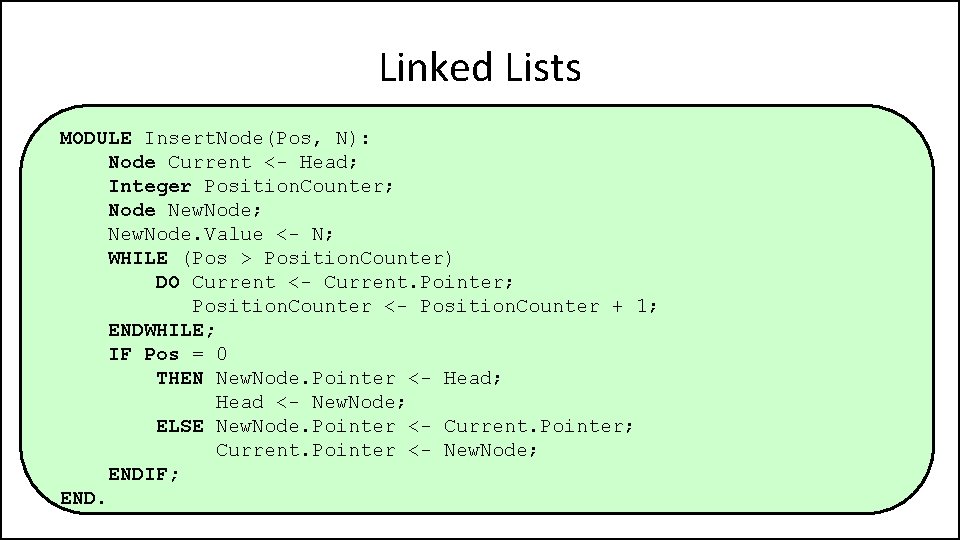
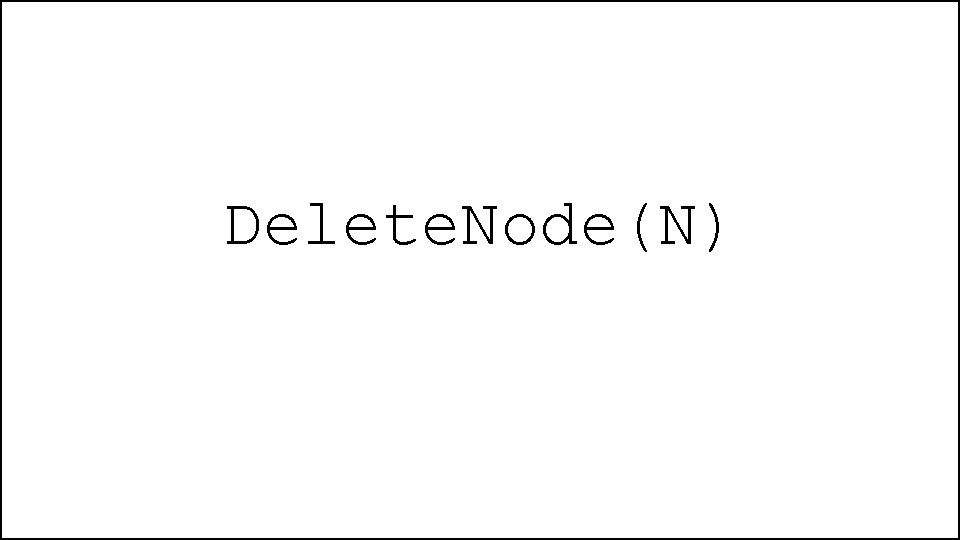
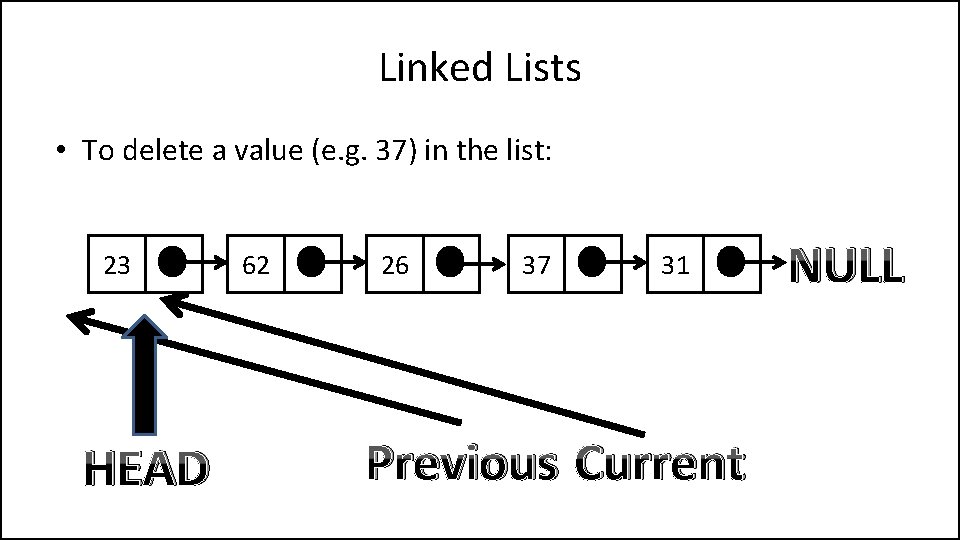
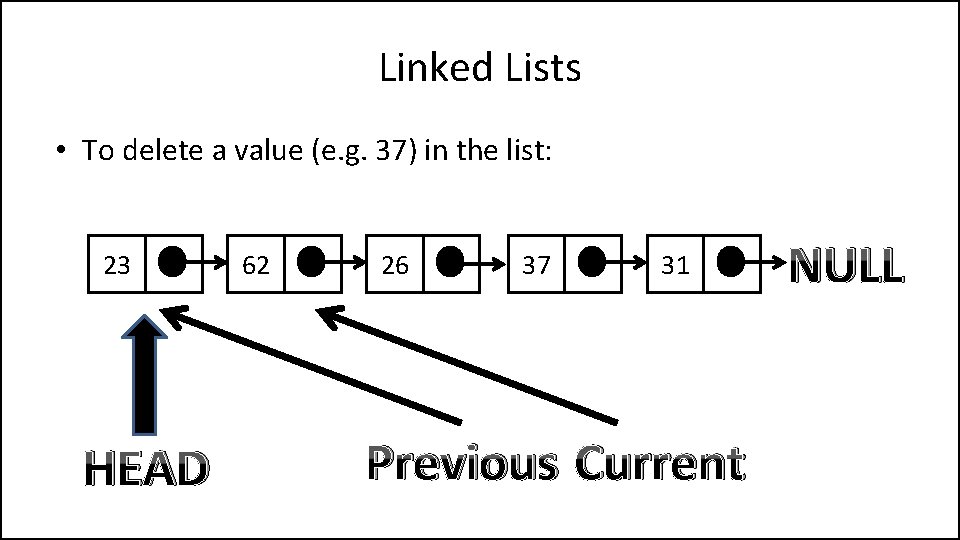
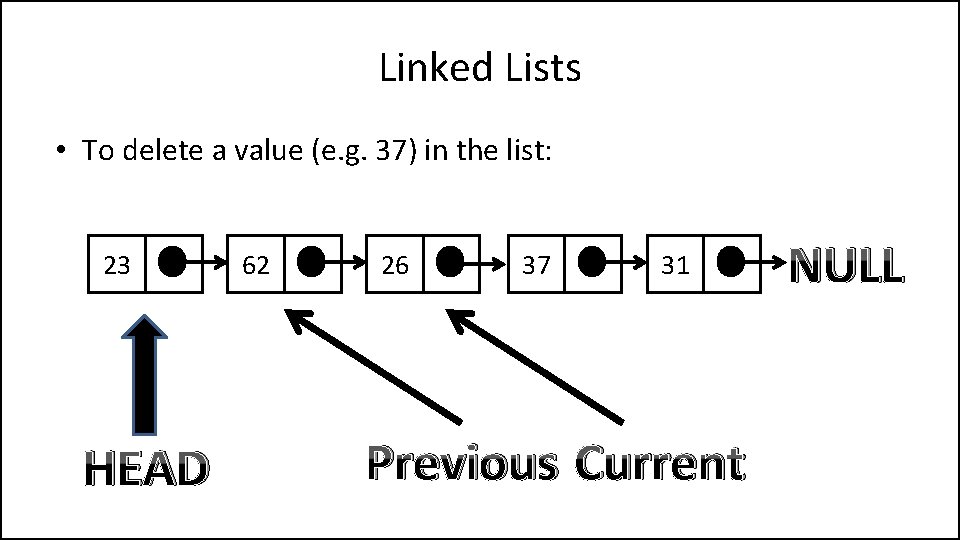
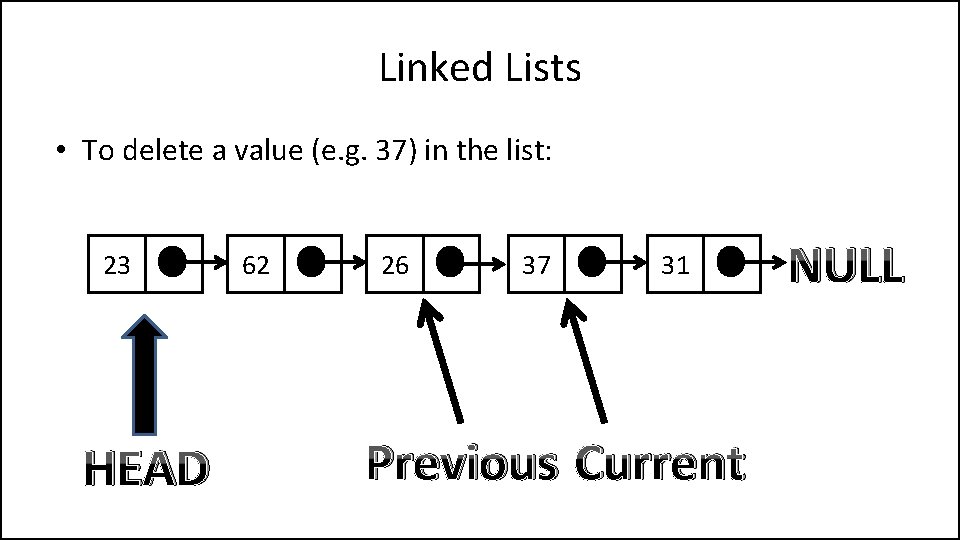
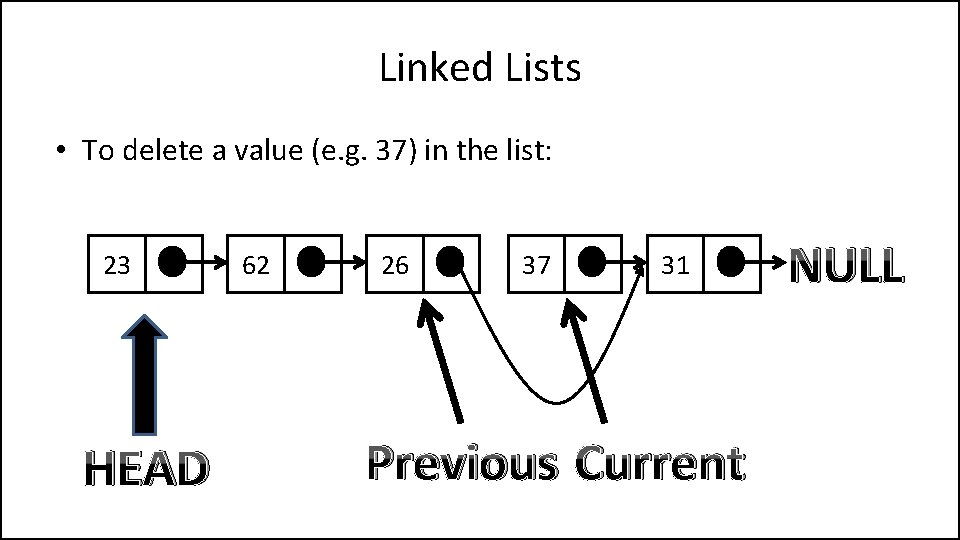
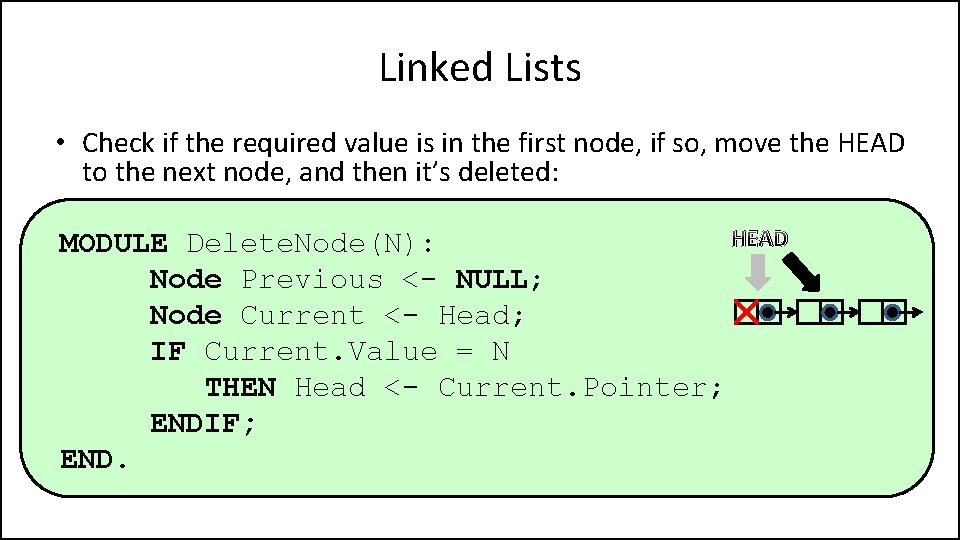
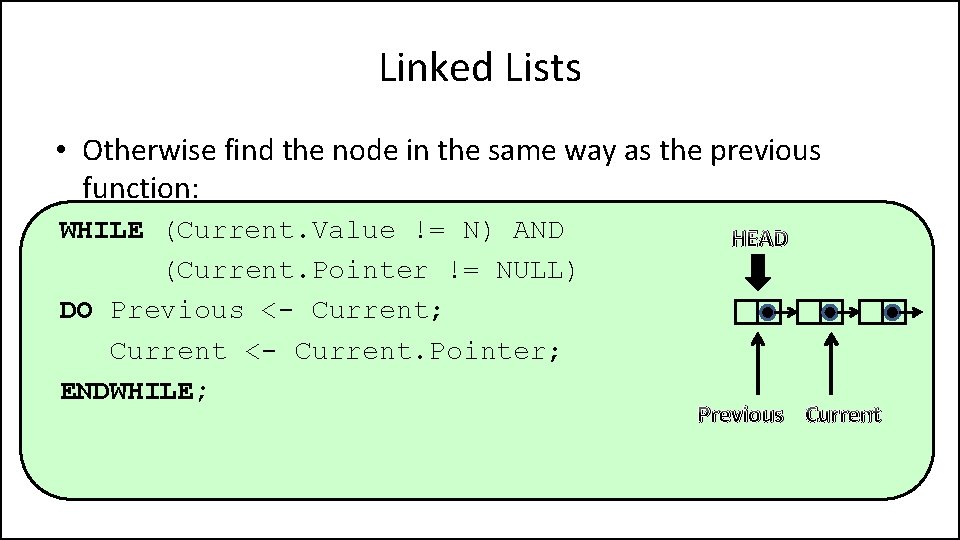
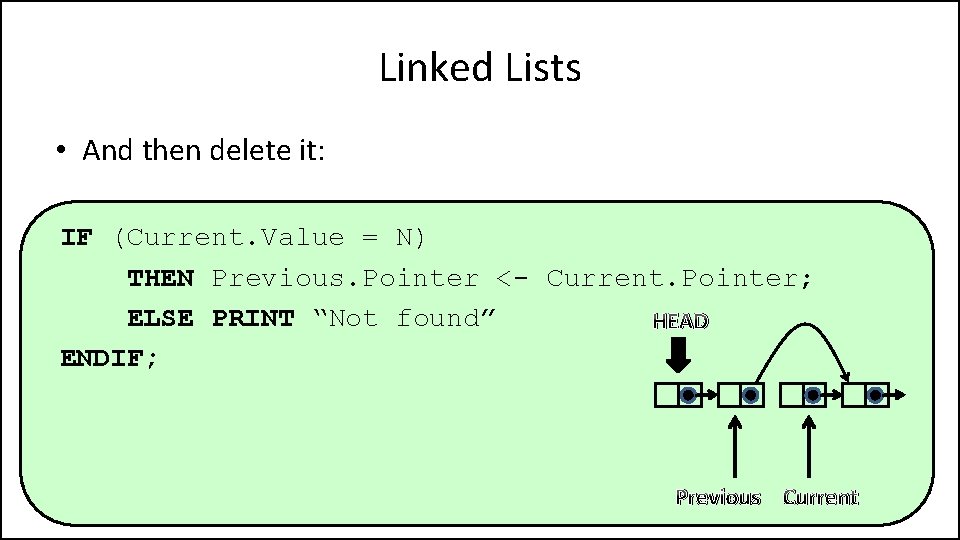
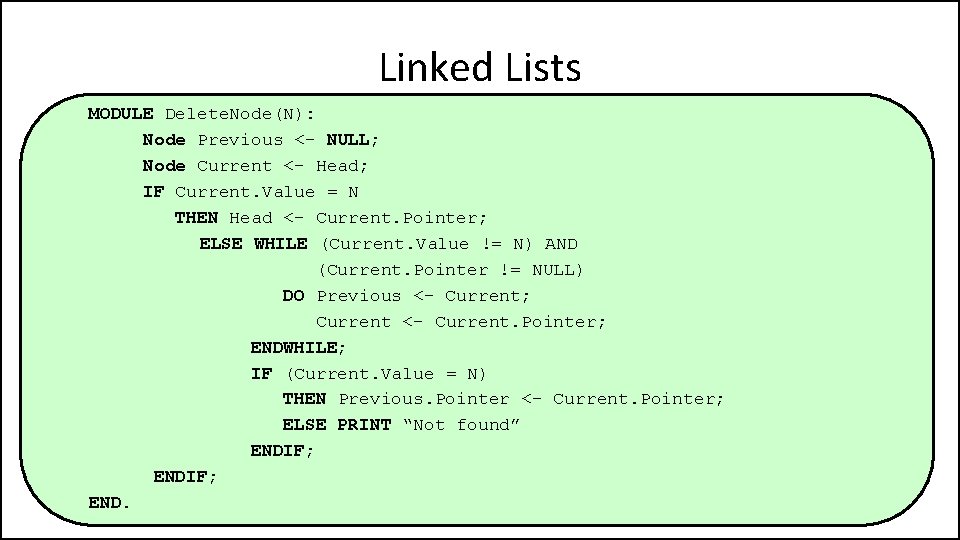
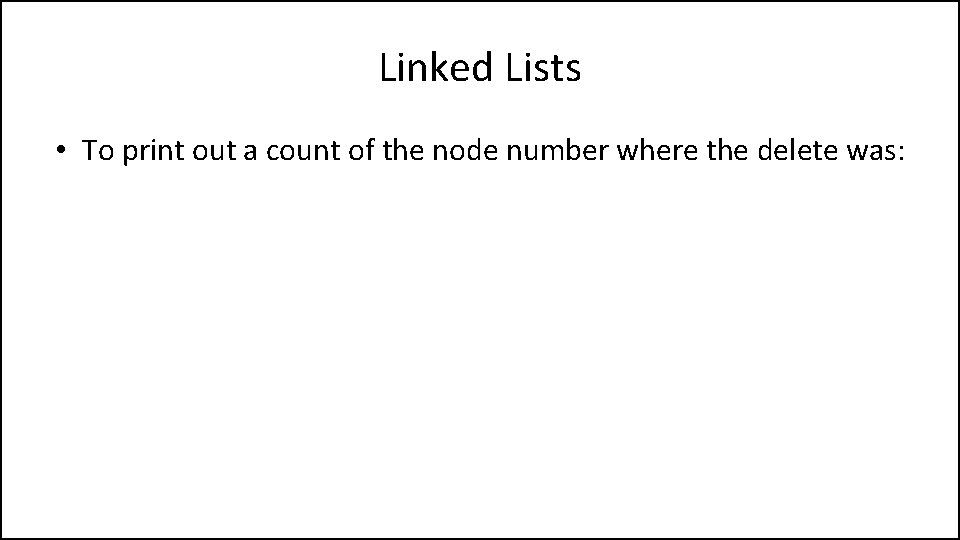
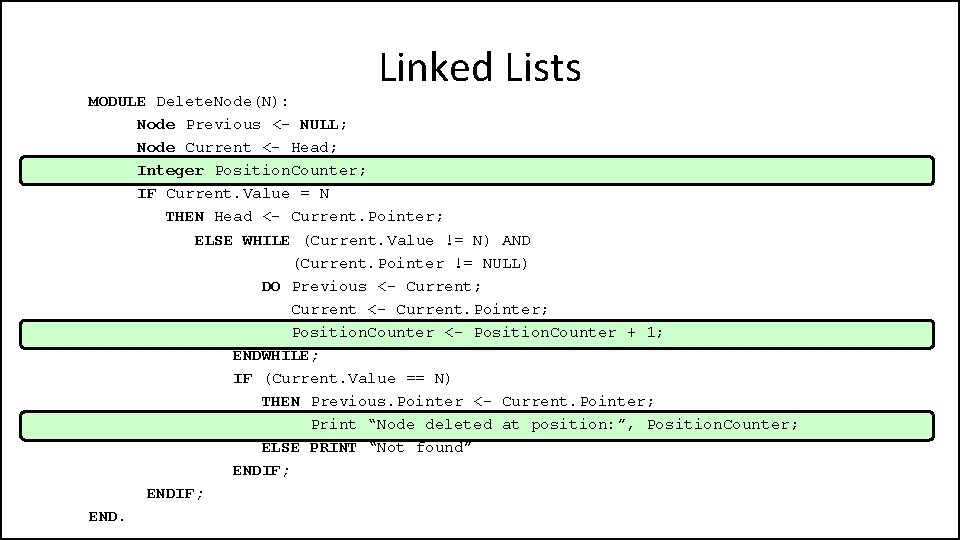
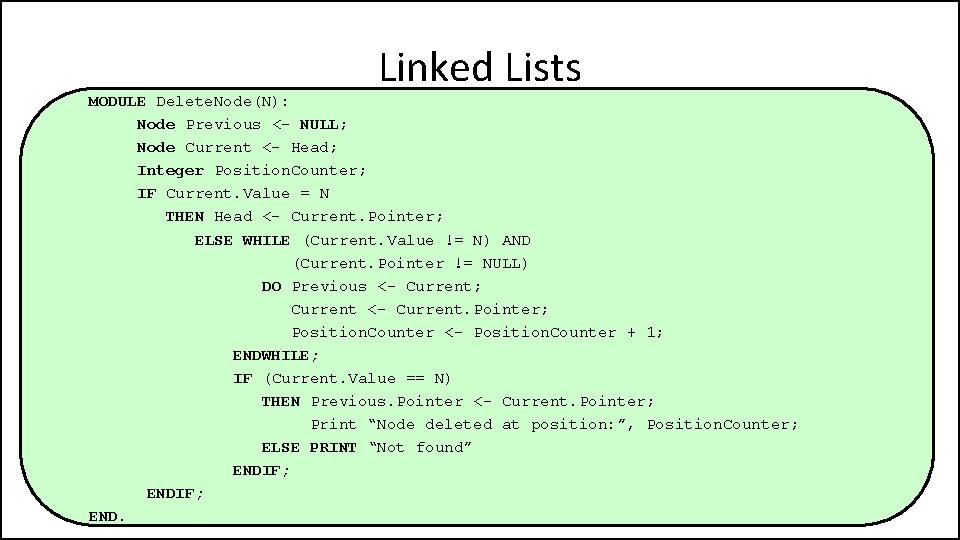
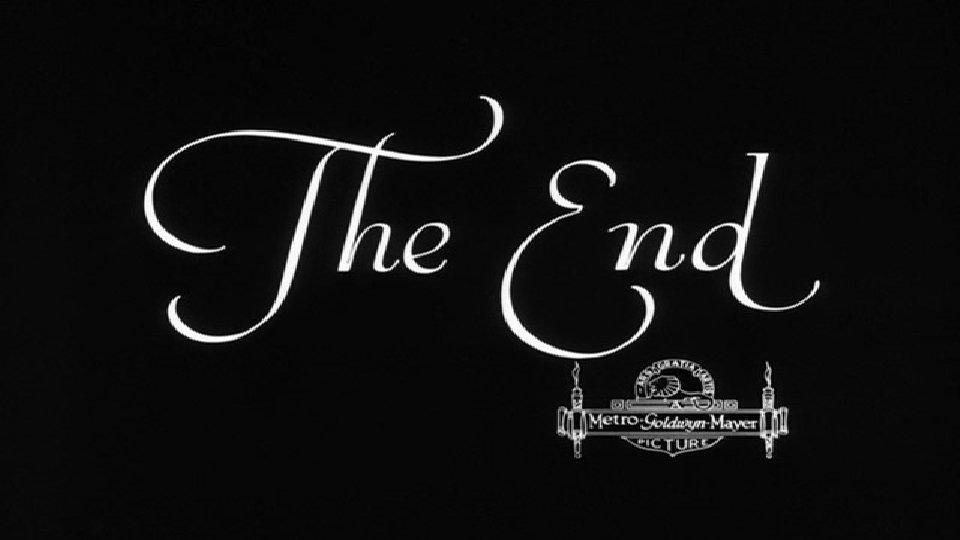
- Slides: 79
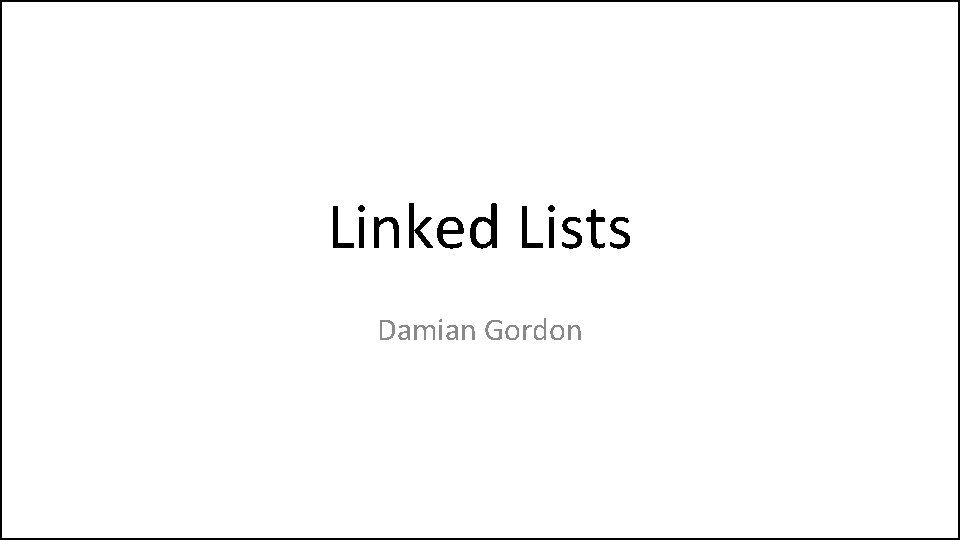
Linked Lists Damian Gordon
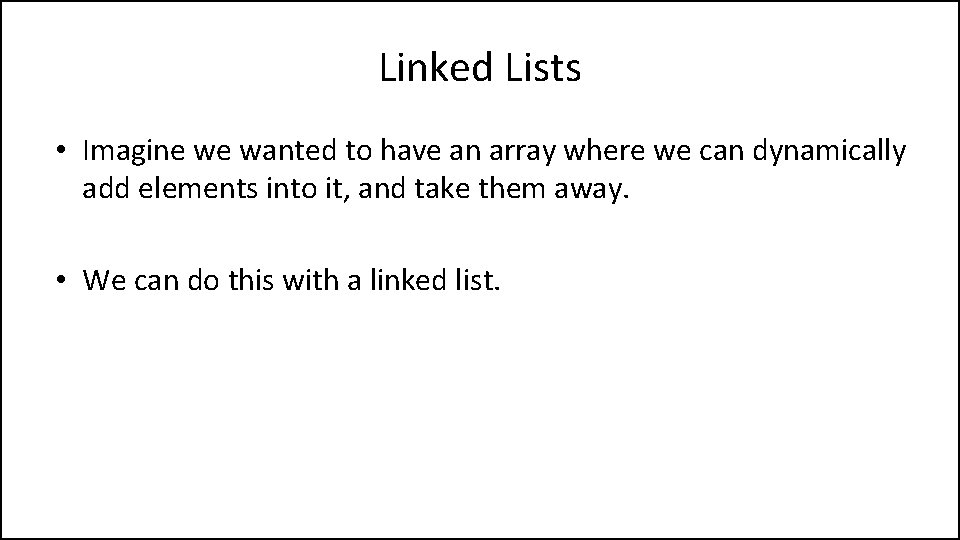
Linked Lists • Imagine we wanted to have an array where we can dynamically add elements into it, and take them away. • We can do this with a linked list.
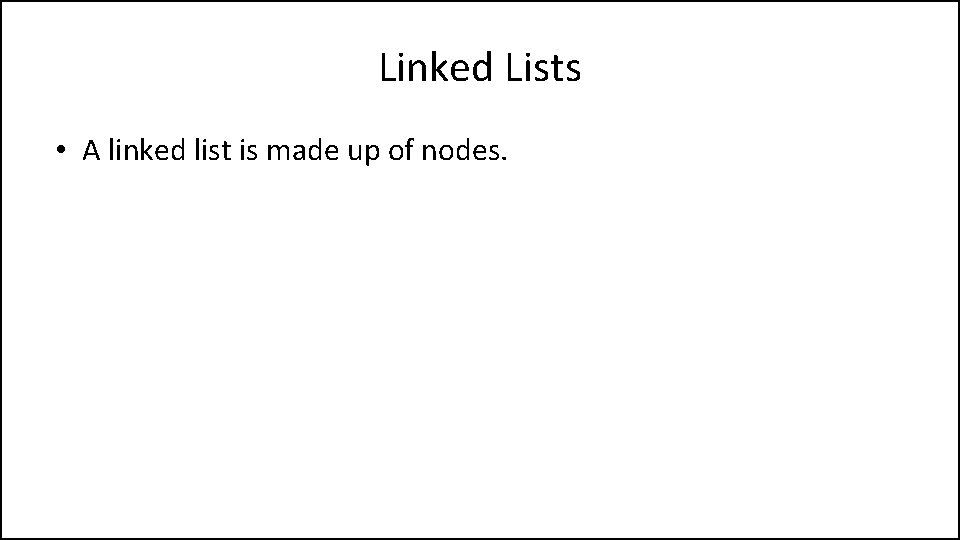
Linked Lists • A linked list is made up of nodes.
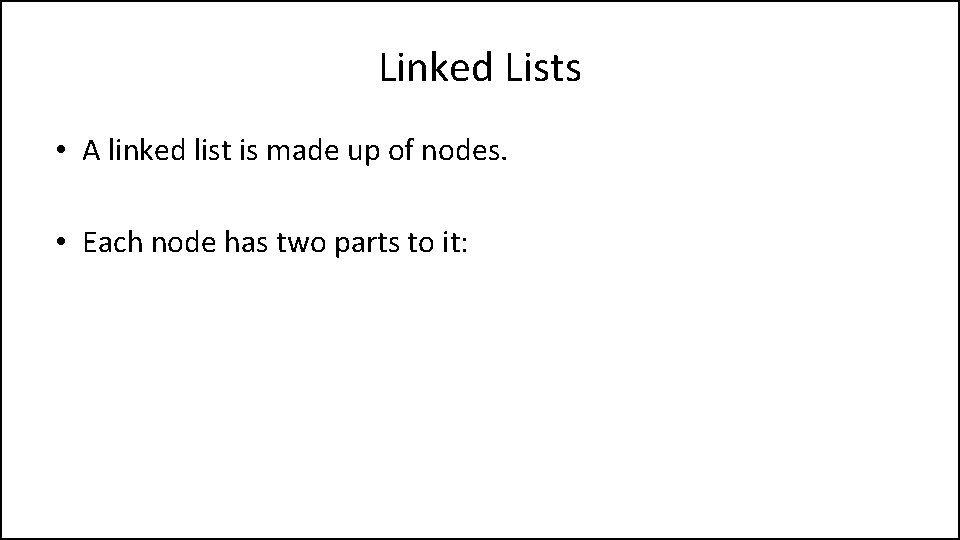
Linked Lists • A linked list is made up of nodes. • Each node has two parts to it:
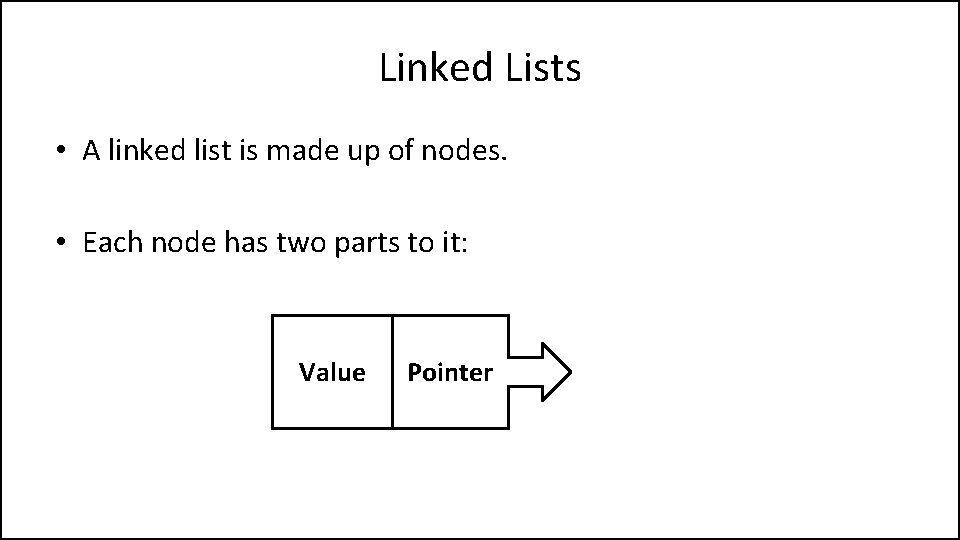
Linked Lists • A linked list is made up of nodes. • Each node has two parts to it: Value Pointer
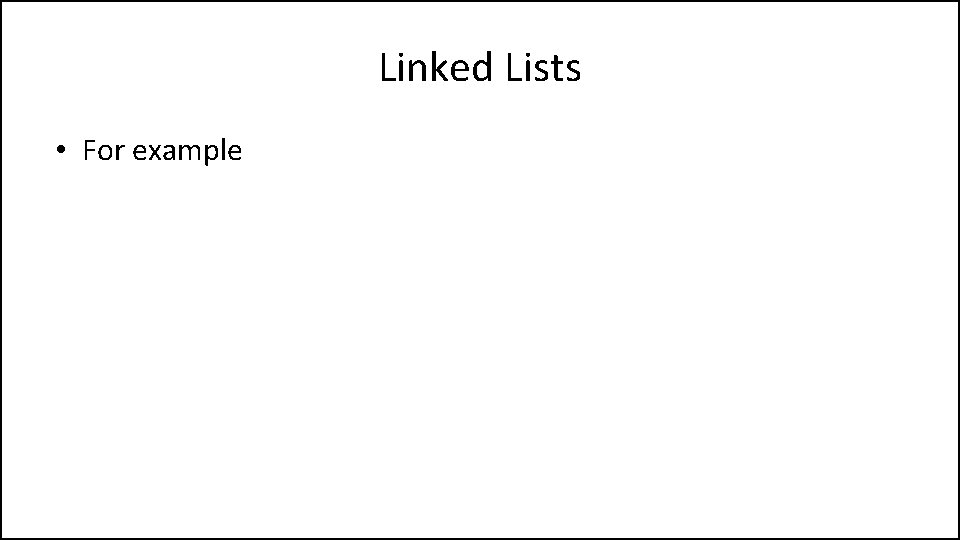
Linked Lists • For example
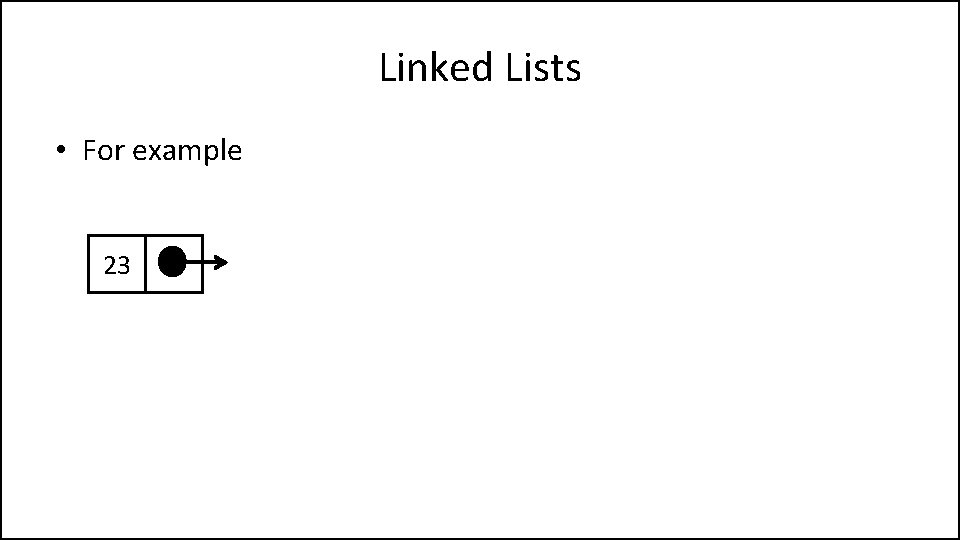
Linked Lists • For example 23
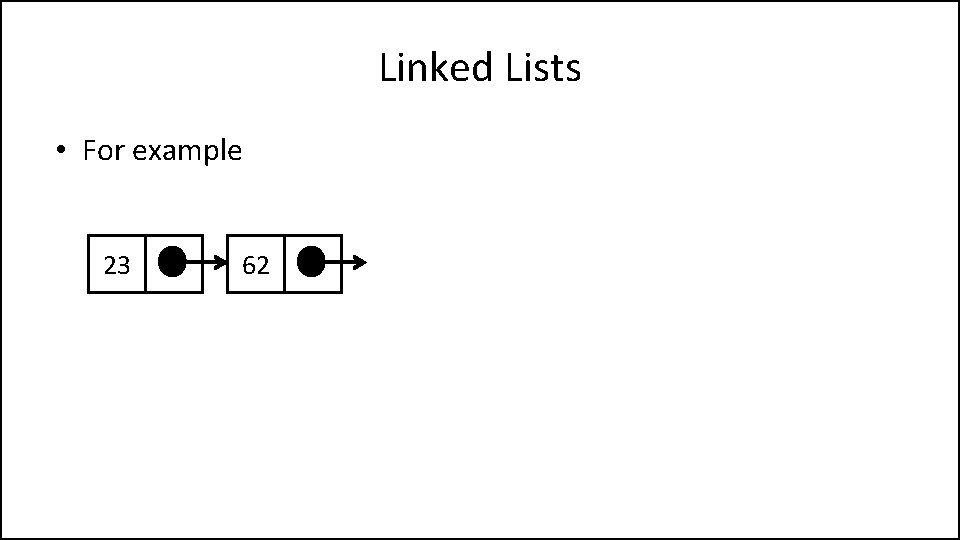
Linked Lists • For example 23 62
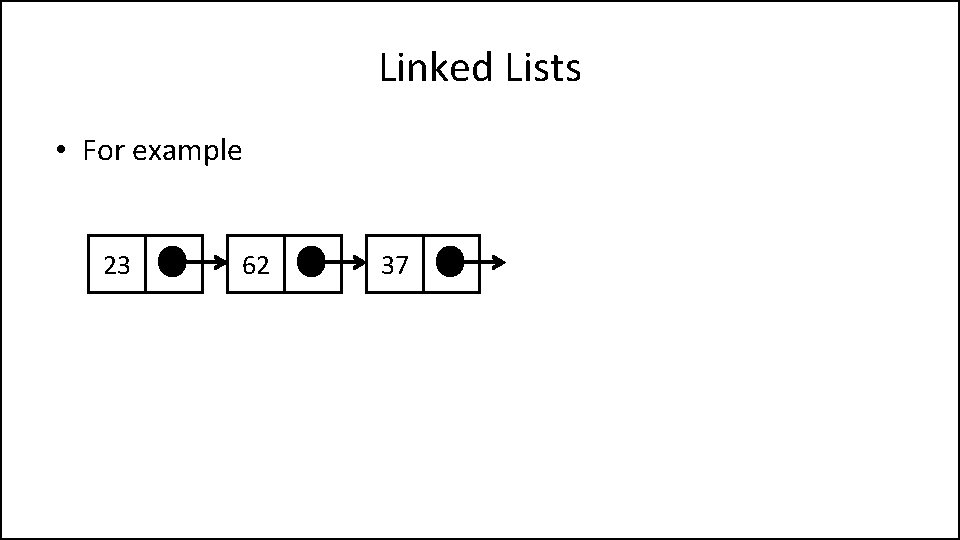
Linked Lists • For example 23 62 37
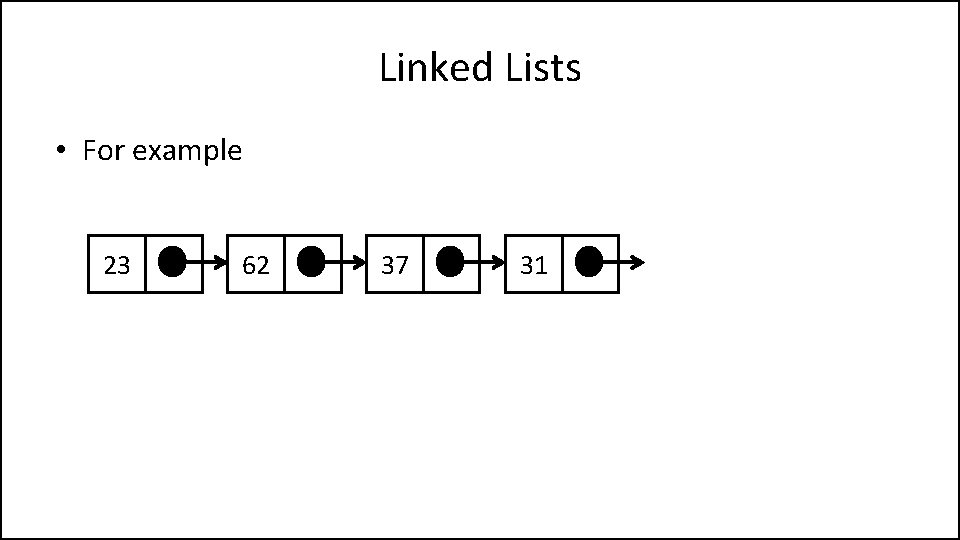
Linked Lists • For example 23 62 37 31
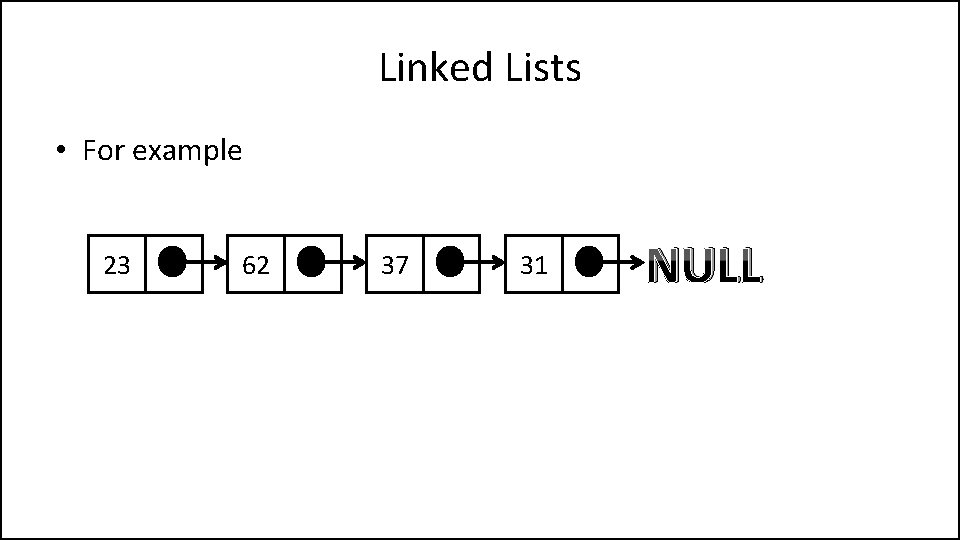
Linked Lists • For example 23 62 37 31 NULL
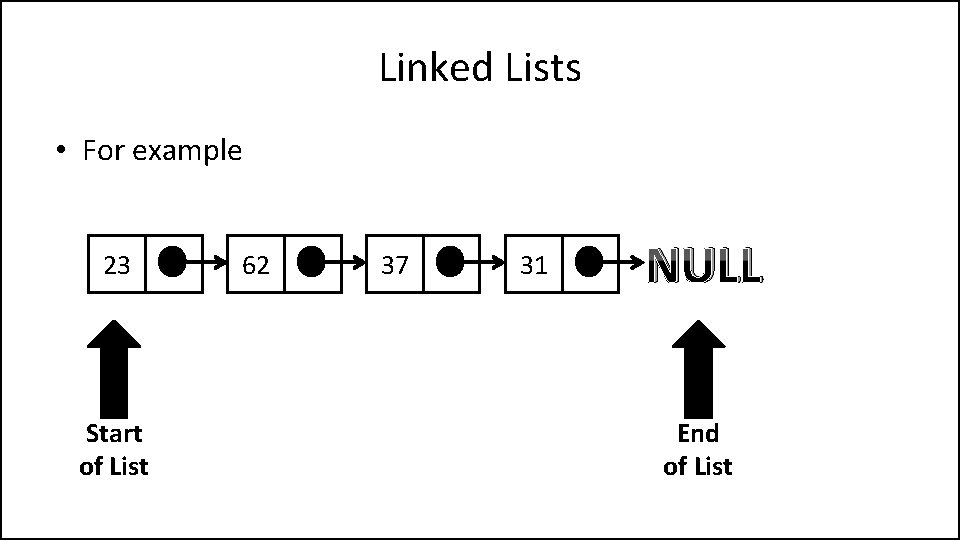
Linked Lists • For example 23 Start of List 62 37 31 NULL End of List
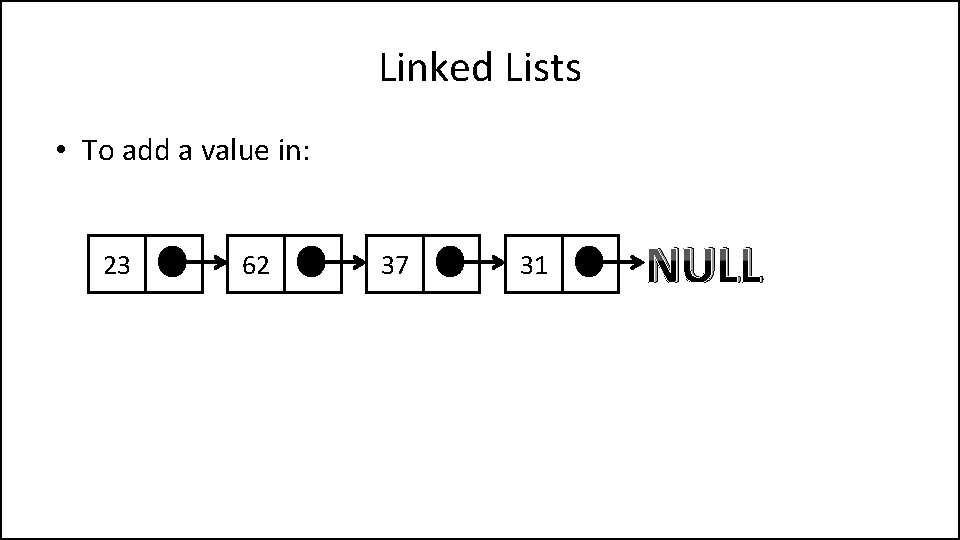
Linked Lists • To add a value in: 23 62 37 31 NULL
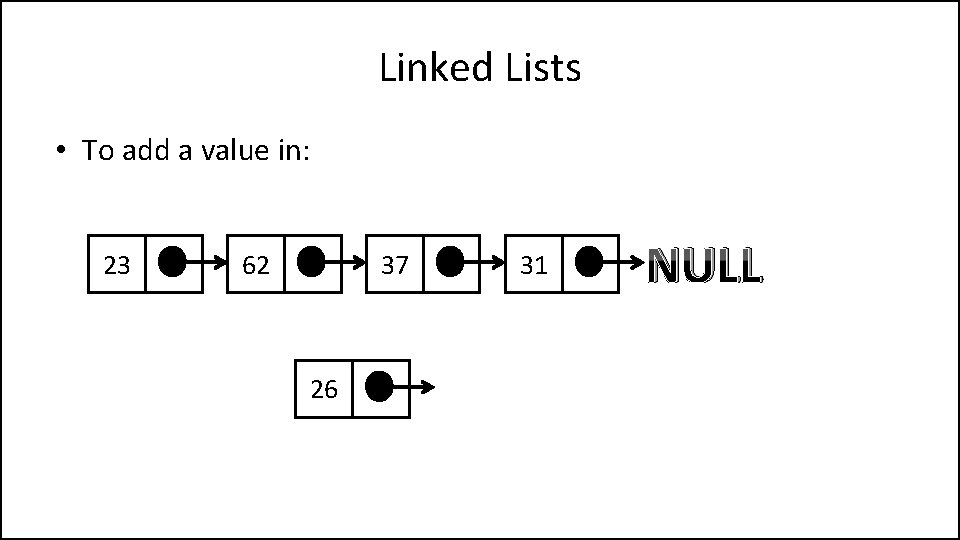
Linked Lists • To add a value in: 23 62 37 26 31 NULL
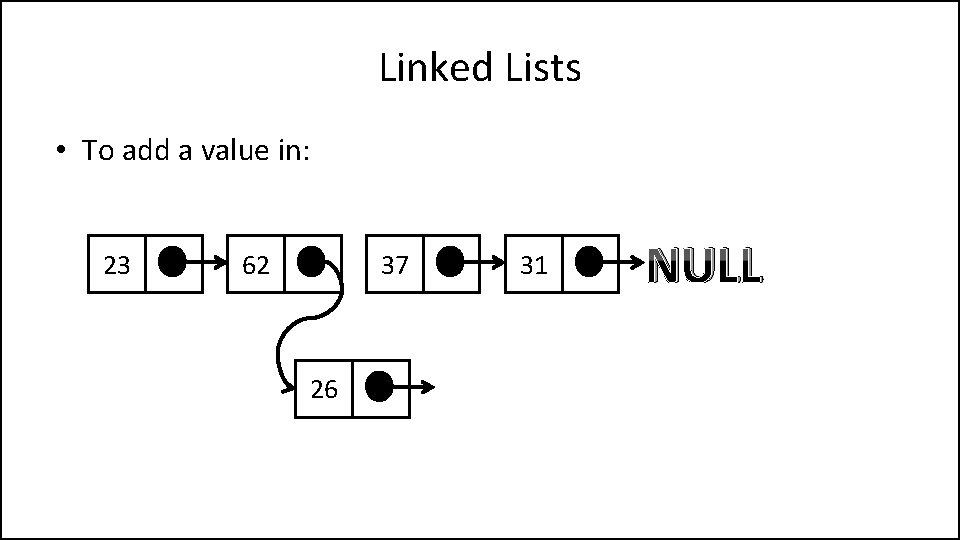
Linked Lists • To add a value in: 23 62 37 26 31 NULL
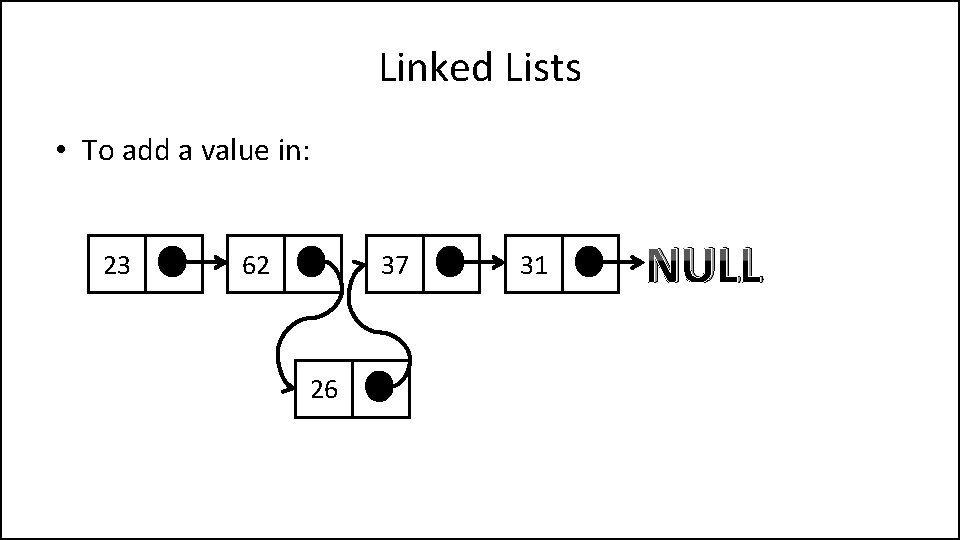
Linked Lists • To add a value in: 23 62 37 26 31 NULL
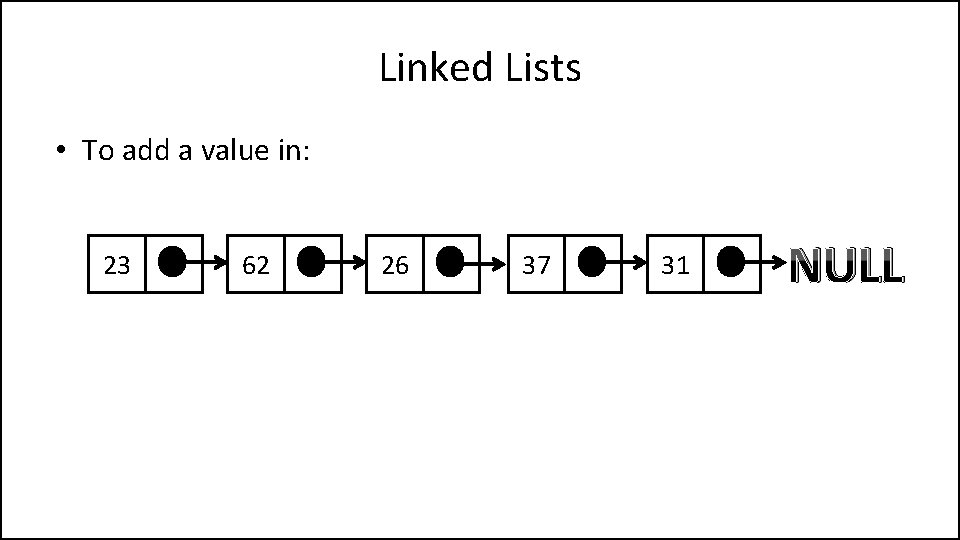
Linked Lists • To add a value in: 23 62 26 37 31 NULL
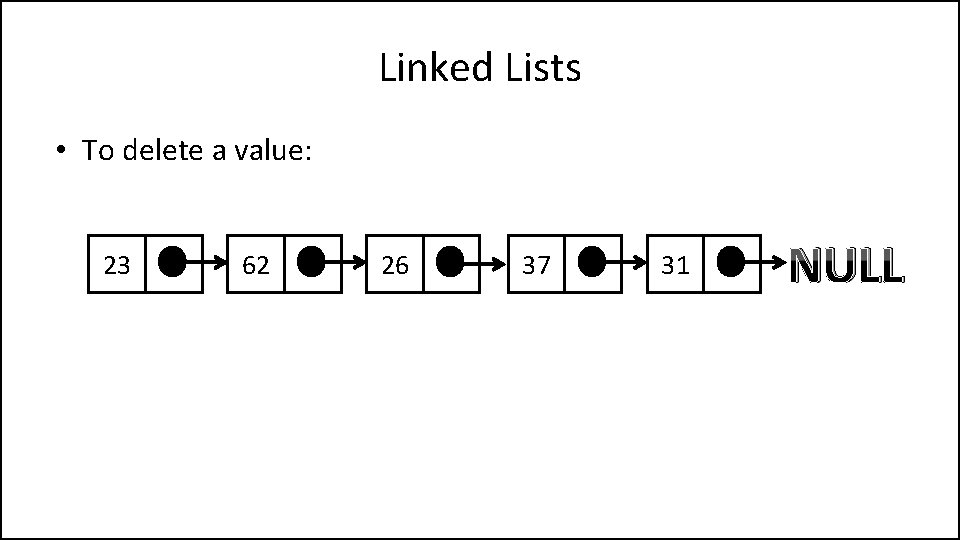
Linked Lists • To delete a value: 23 62 26 37 31 NULL
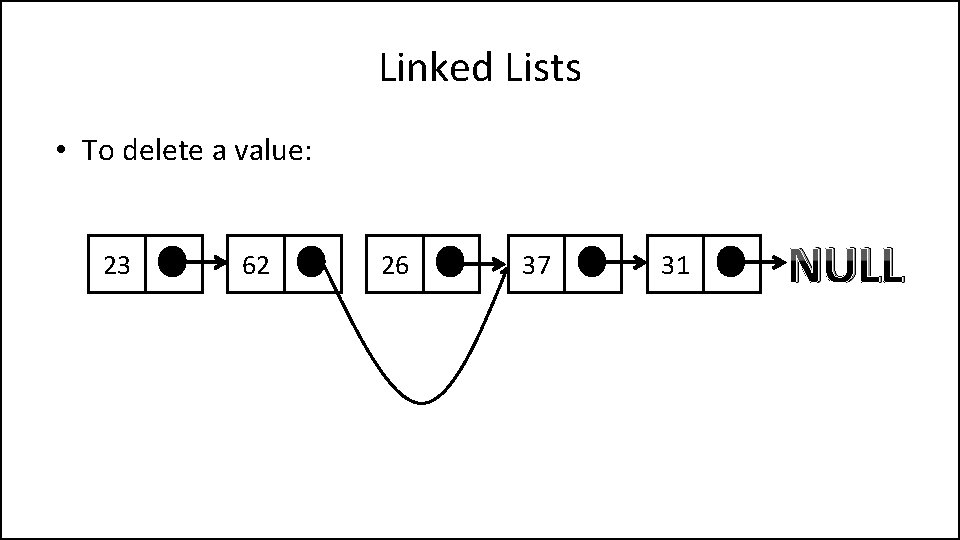
Linked Lists • To delete a value: 23 62 26 37 31 NULL
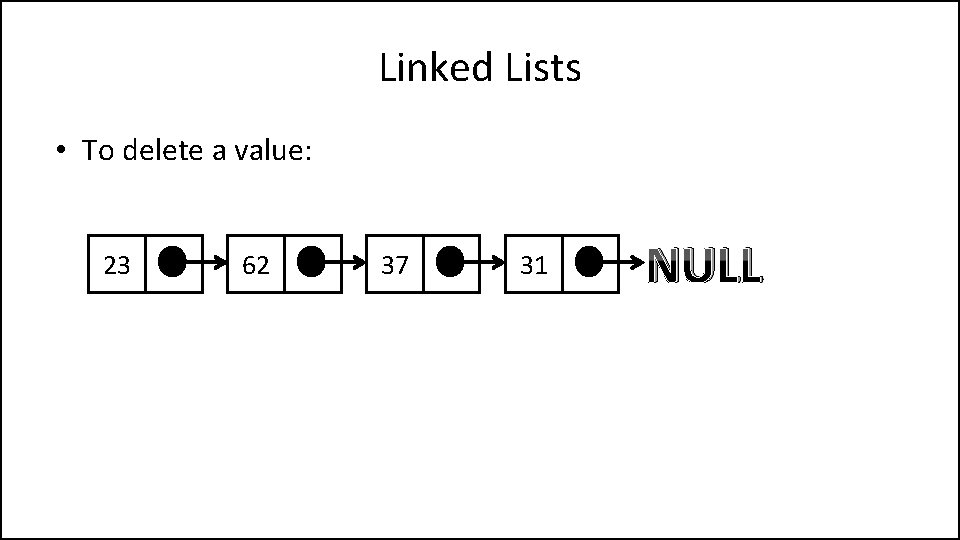
Linked Lists • To delete a value: 23 62 37 31 NULL
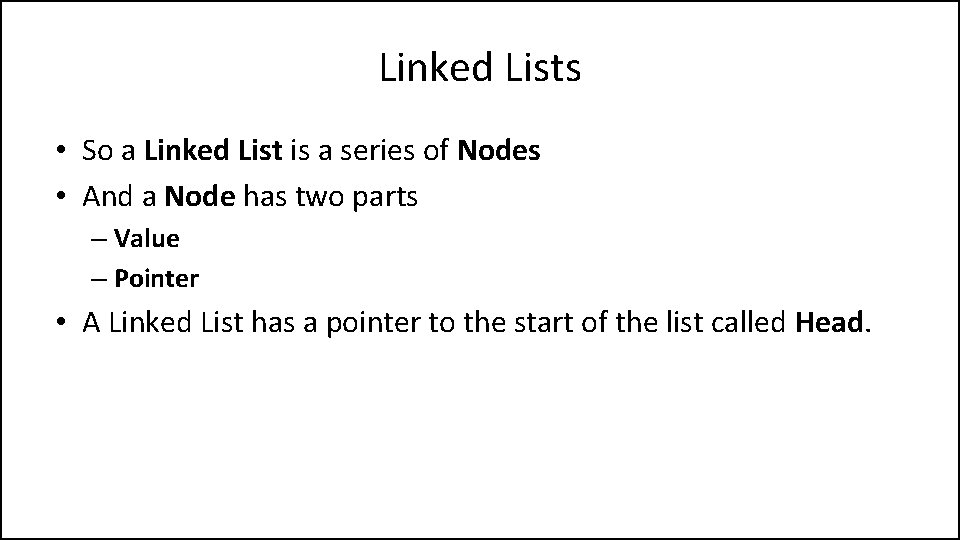
Linked Lists • So a Linked List is a series of Nodes • And a Node has two parts – Value – Pointer • A Linked List has a pointer to the start of the list called Head.
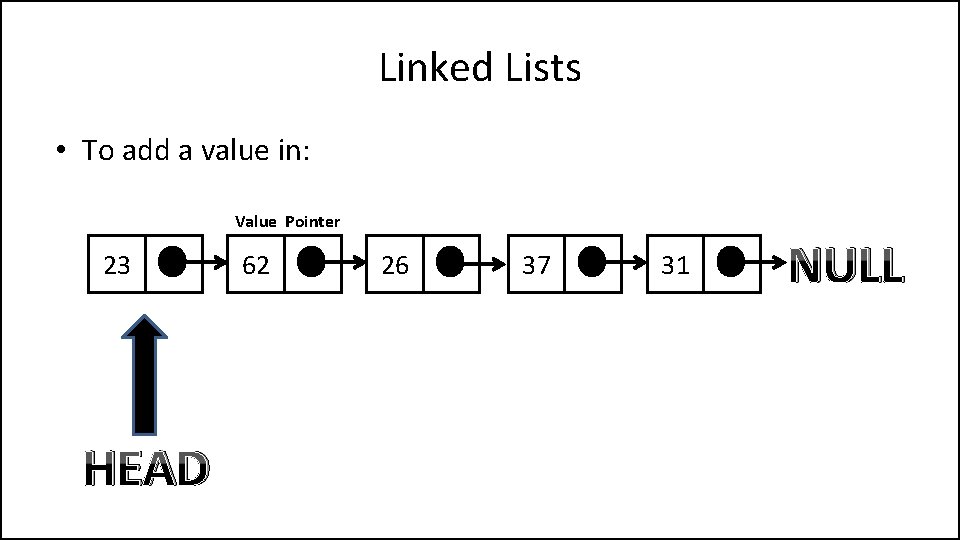
Linked Lists • To add a value in: Value Pointer 23 HEAD 62 26 37 31 NULL
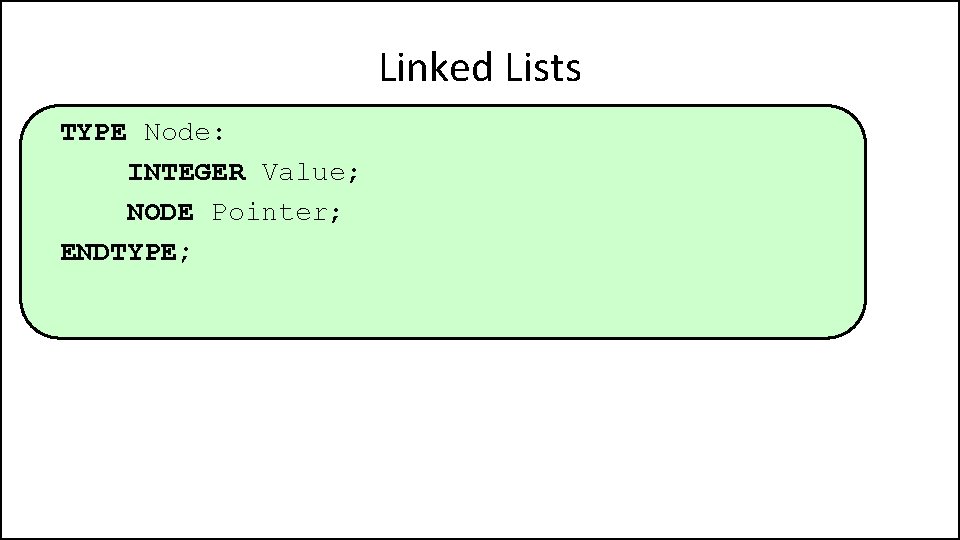
Linked Lists TYPE Node: INTEGER Value; NODE Pointer; ENDTYPE;
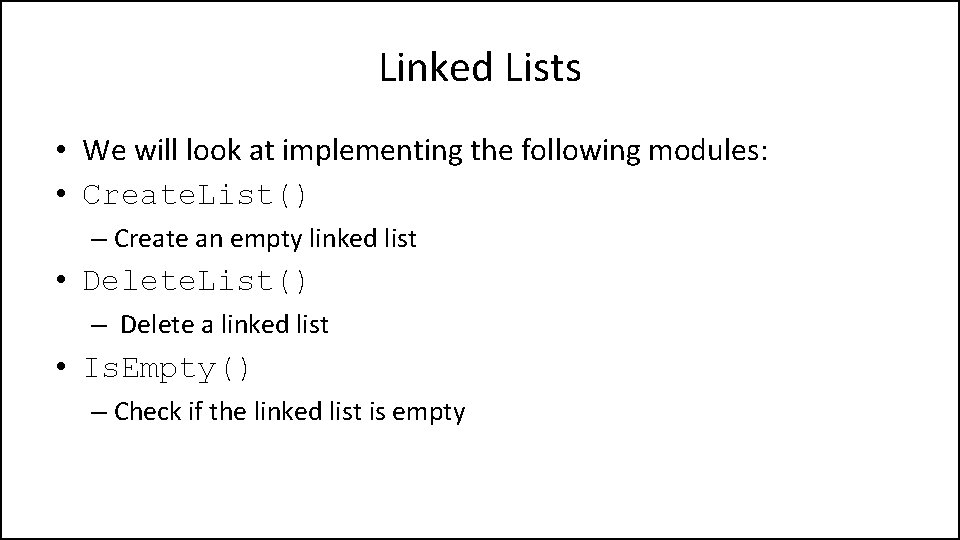
Linked Lists • We will look at implementing the following modules: • Create. List() – Create an empty linked list • Delete. List() – Delete a linked list • Is. Empty() – Check if the linked list is empty
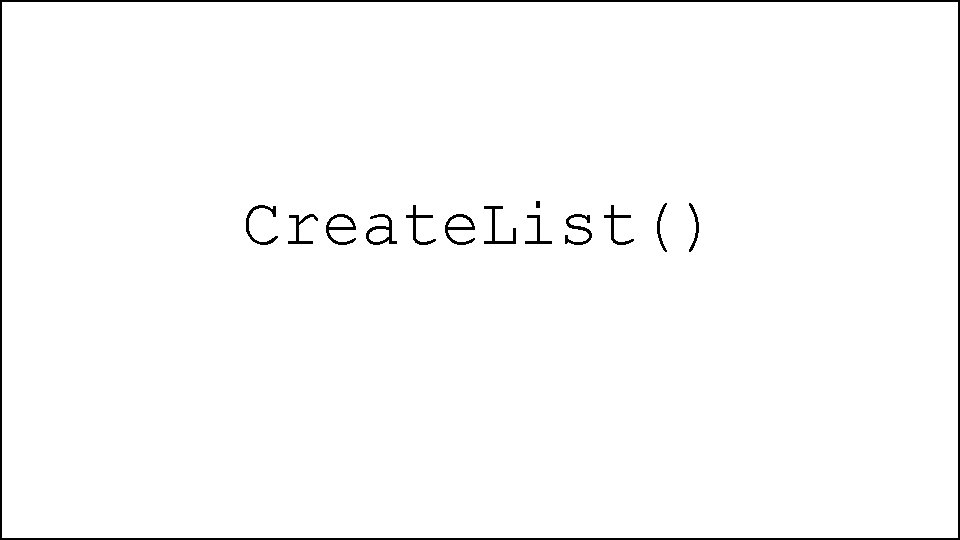
Create. List()
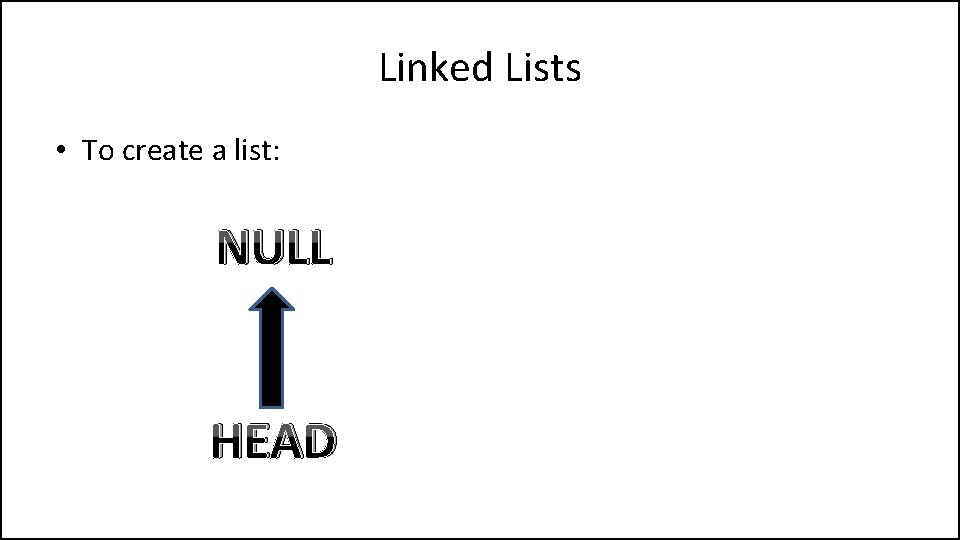
Linked Lists • To create a list: NULL HEAD
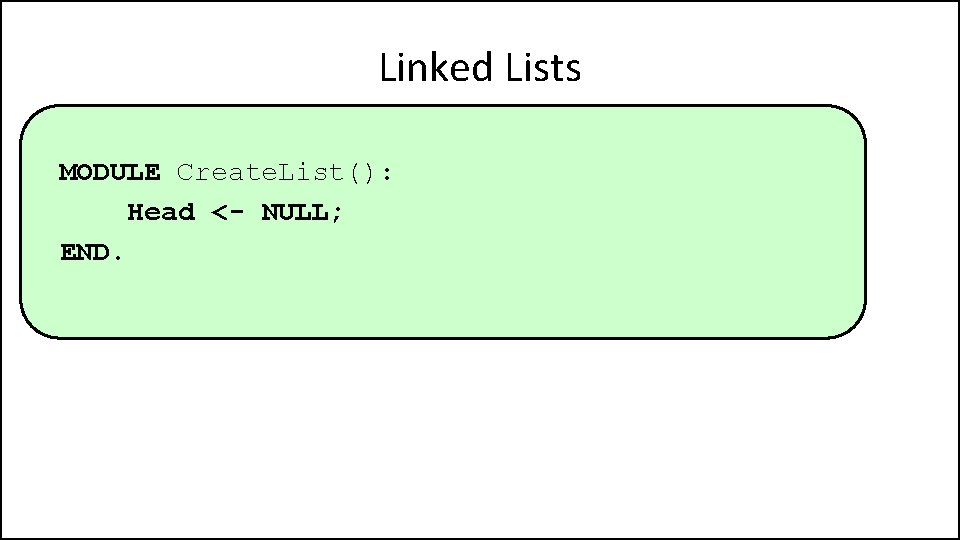
Linked Lists MODULE Create. List(): Head <- NULL; END.
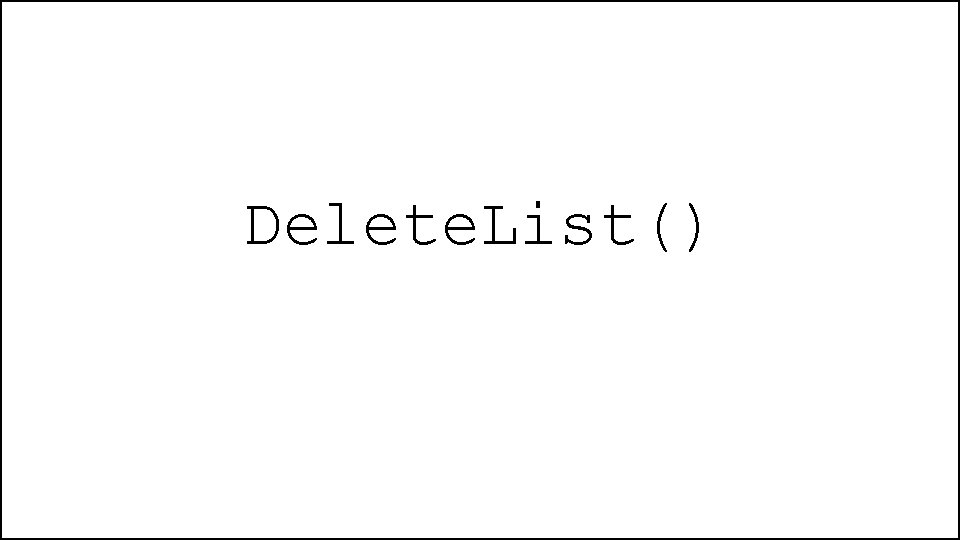
Delete. List()
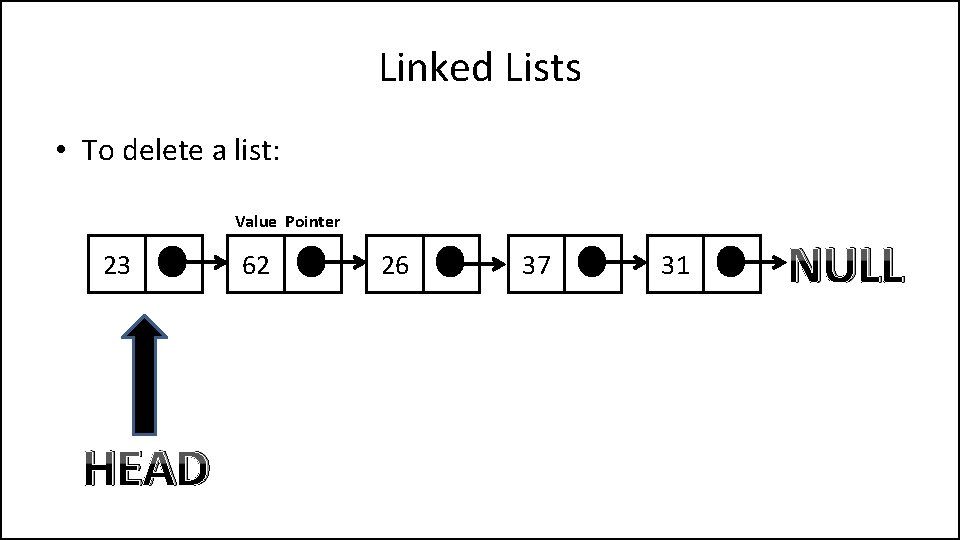
Linked Lists • To delete a list: Value Pointer 23 HEAD 62 26 37 31 NULL
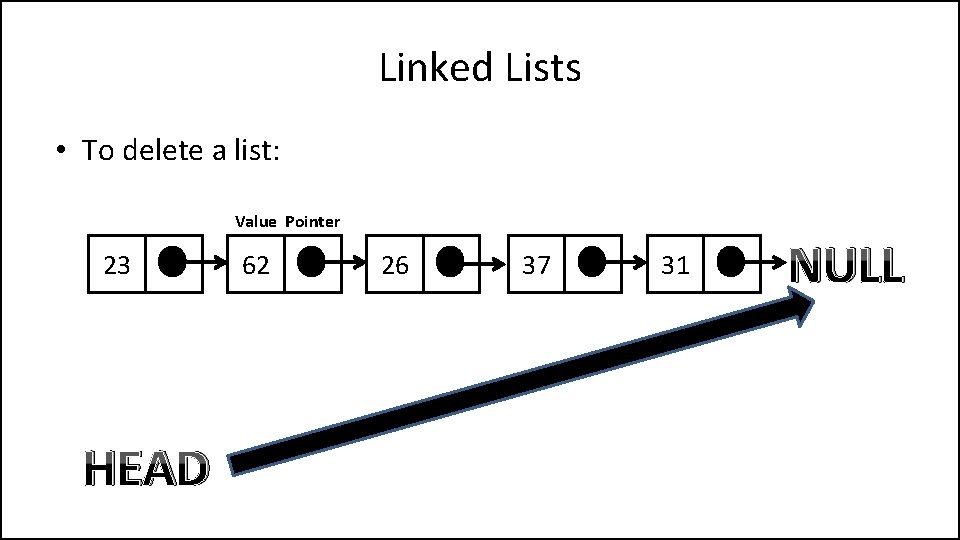
Linked Lists • To delete a list: Value Pointer 23 HEAD 62 26 37 31 NULL
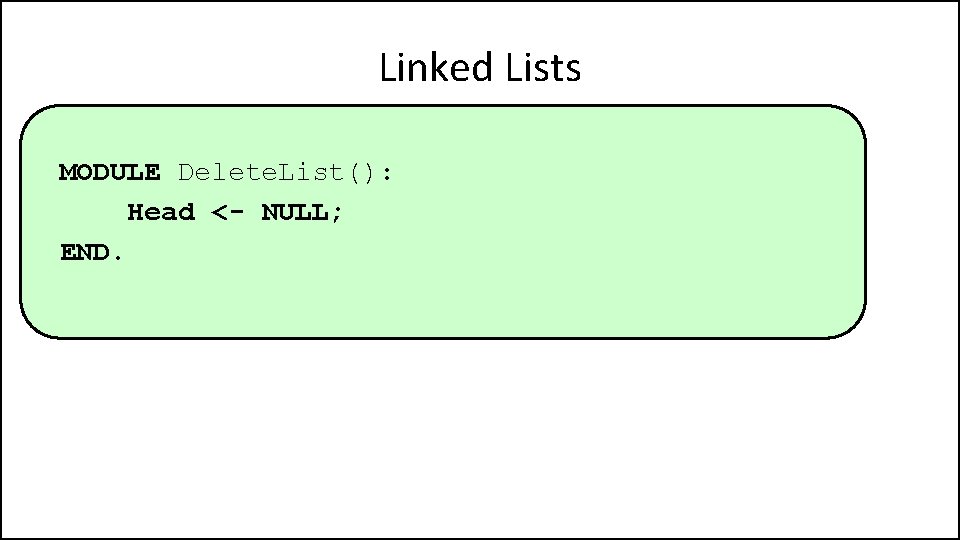
Linked Lists MODULE Delete. List(): Head <- NULL; END.
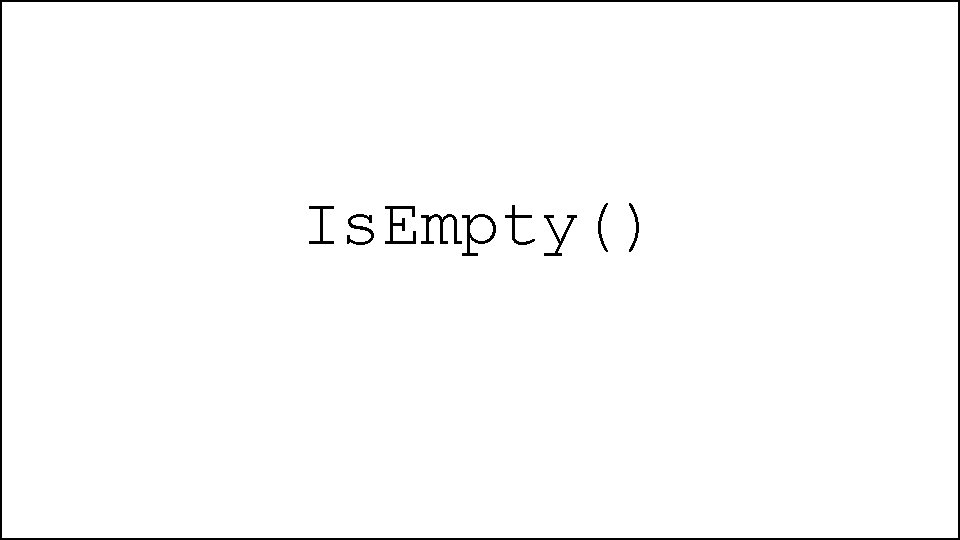
Is. Empty()
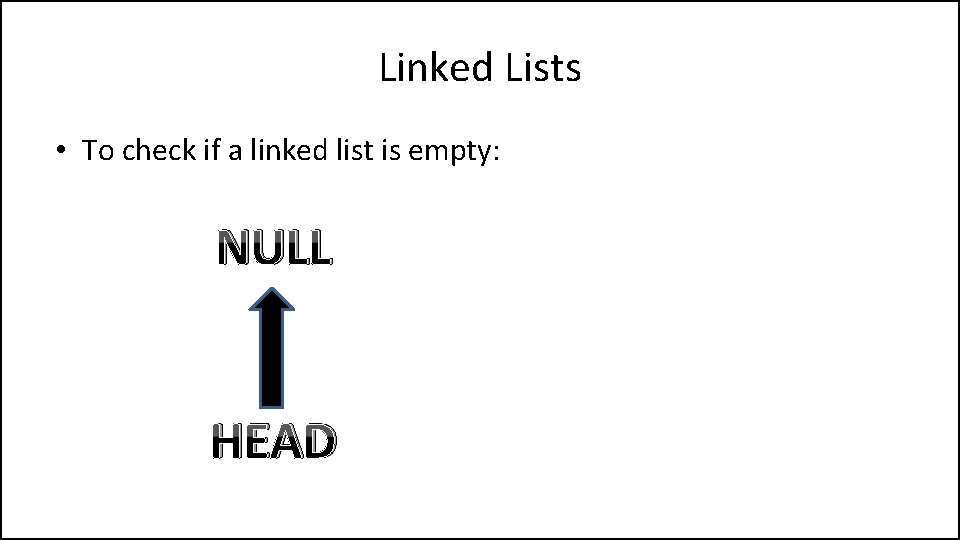
Linked Lists • To check if a linked list is empty: NULL HEAD
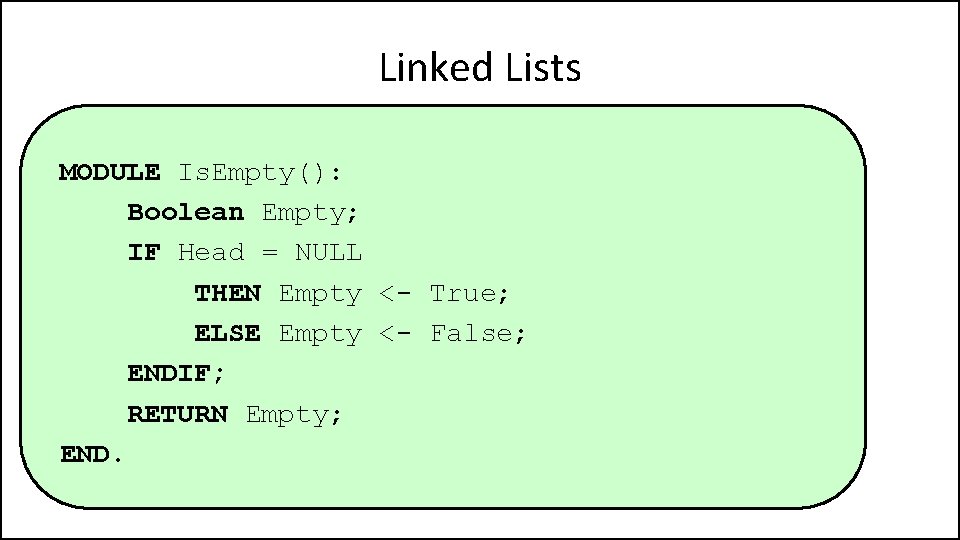
Linked Lists MODULE Is. Empty(): Boolean Empty; IF Head = NULL THEN Empty <- True; ELSE Empty <- False; ENDIF; RETURN Empty; END.
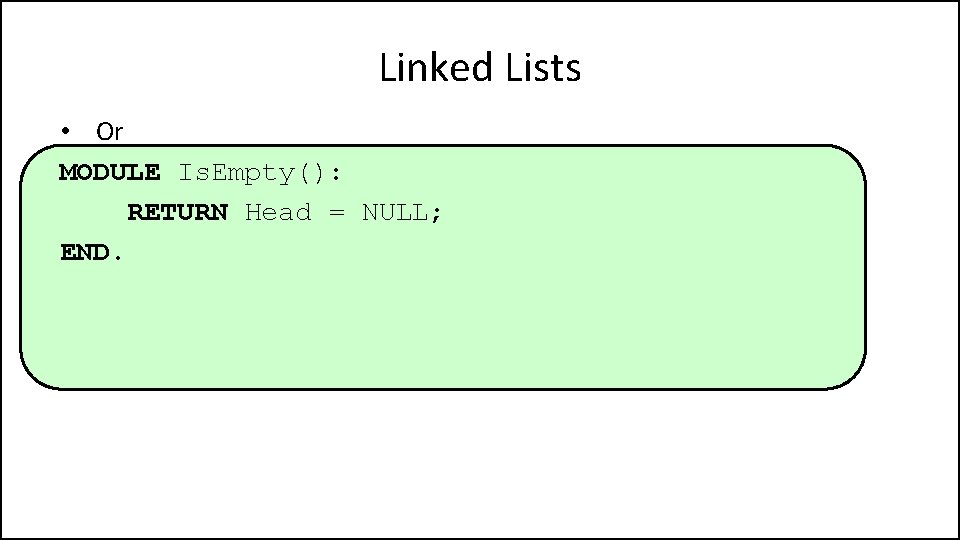
Linked Lists • Or MODULE Is. Empty(): RETURN Head = NULL; END.
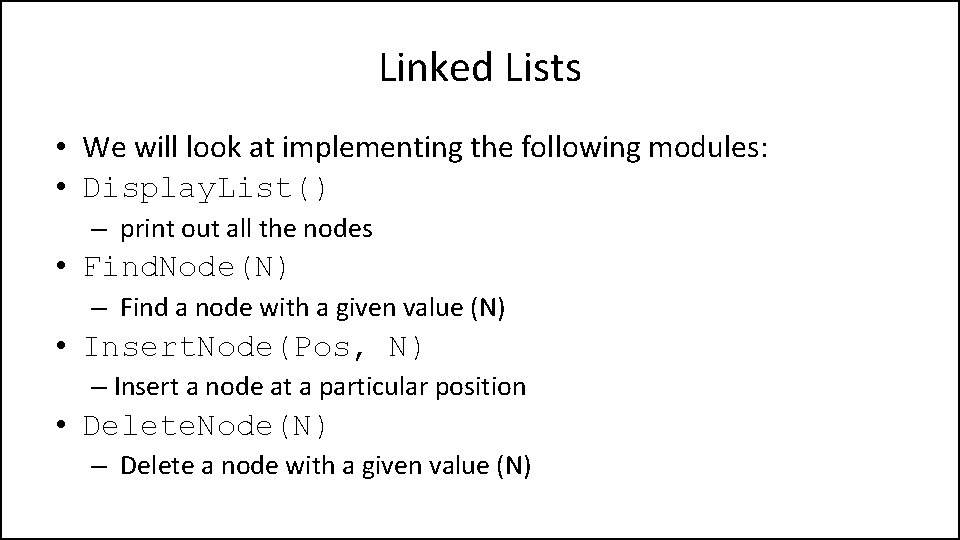
Linked Lists • We will look at implementing the following modules: • Display. List() – print out all the nodes • Find. Node(N) – Find a node with a given value (N) • Insert. Node(Pos, N) – Insert a node at a particular position • Delete. Node(N) – Delete a node with a given value (N)
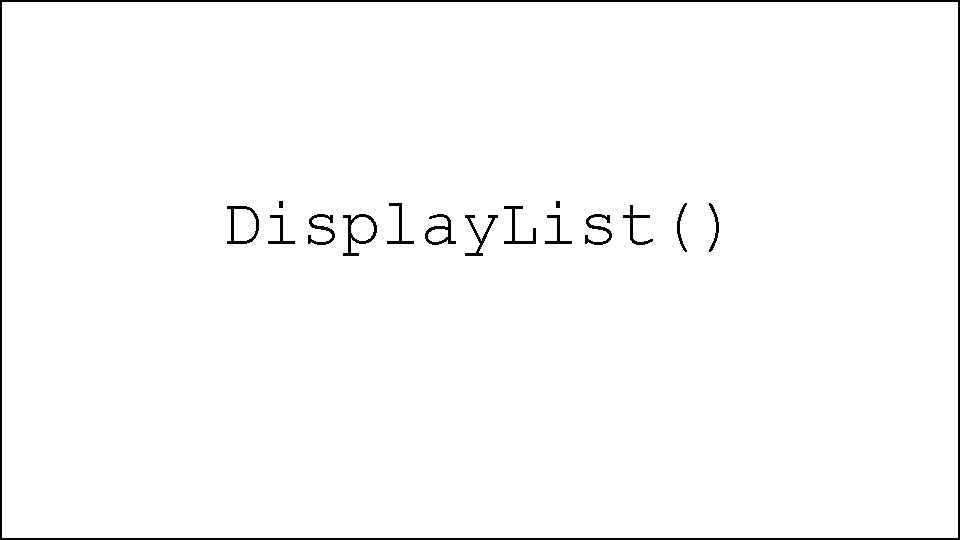
Display. List()
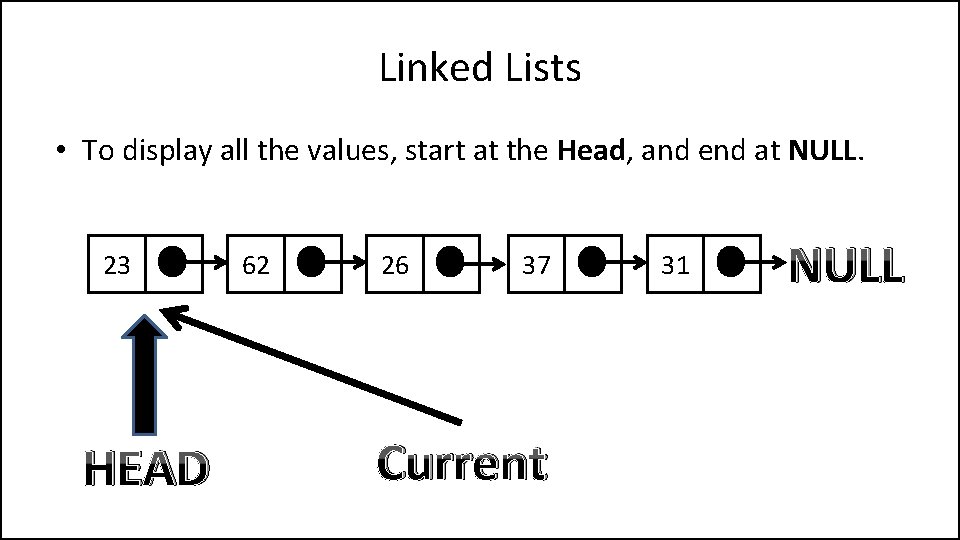
Linked Lists • To display all the values, start at the Head, and end at NULL. 23 HEAD 62 26 37 Current 31 NULL
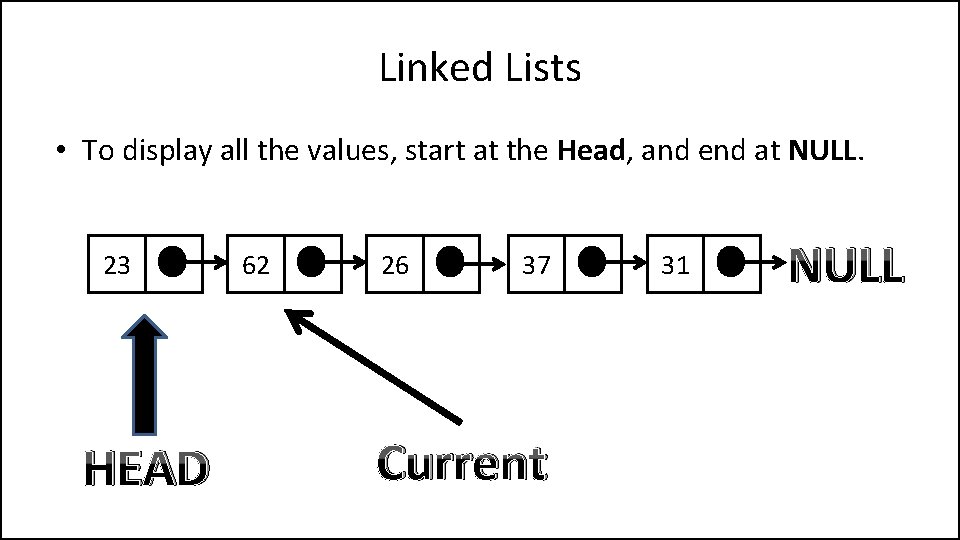
Linked Lists • To display all the values, start at the Head, and end at NULL. 23 HEAD 62 26 37 Current 31 NULL
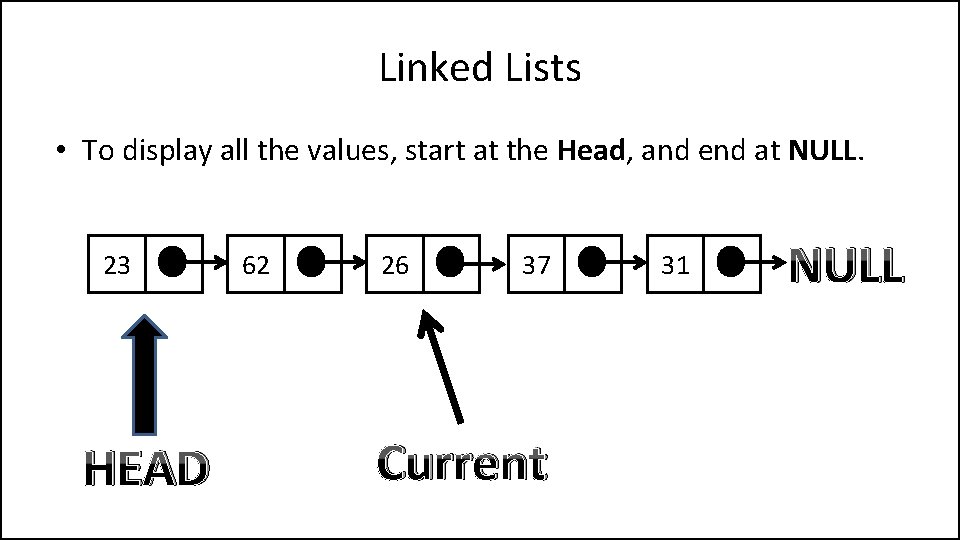
Linked Lists • To display all the values, start at the Head, and end at NULL. 23 HEAD 62 26 37 Current 31 NULL
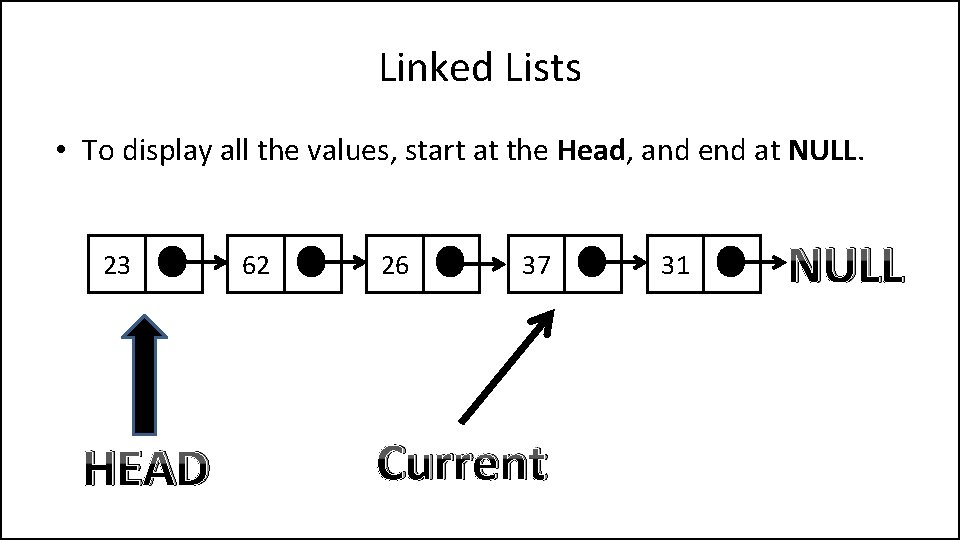
Linked Lists • To display all the values, start at the Head, and end at NULL. 23 HEAD 62 26 37 Current 31 NULL
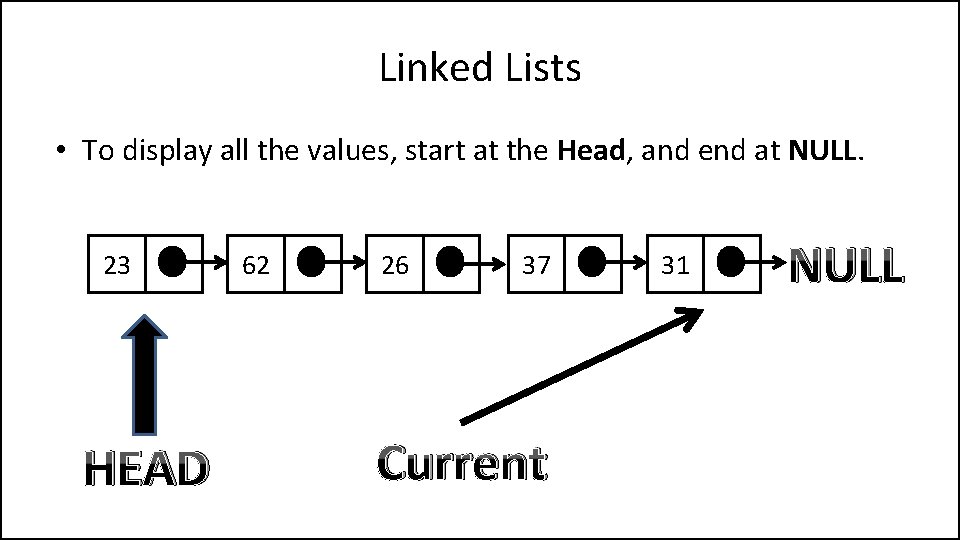
Linked Lists • To display all the values, start at the Head, and end at NULL. 23 HEAD 62 26 37 Current 31 NULL
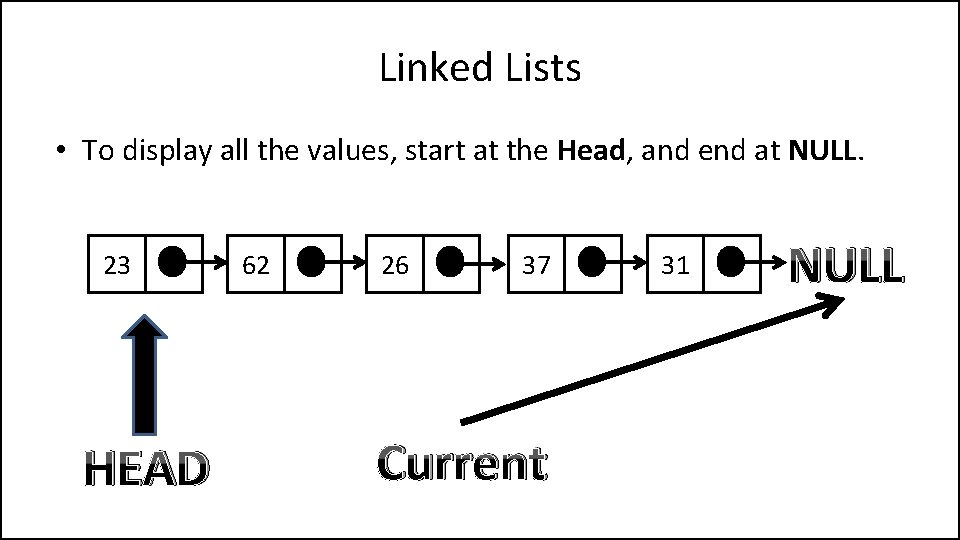
Linked Lists • To display all the values, start at the Head, and end at NULL. 23 HEAD 62 26 37 Current 31 NULL
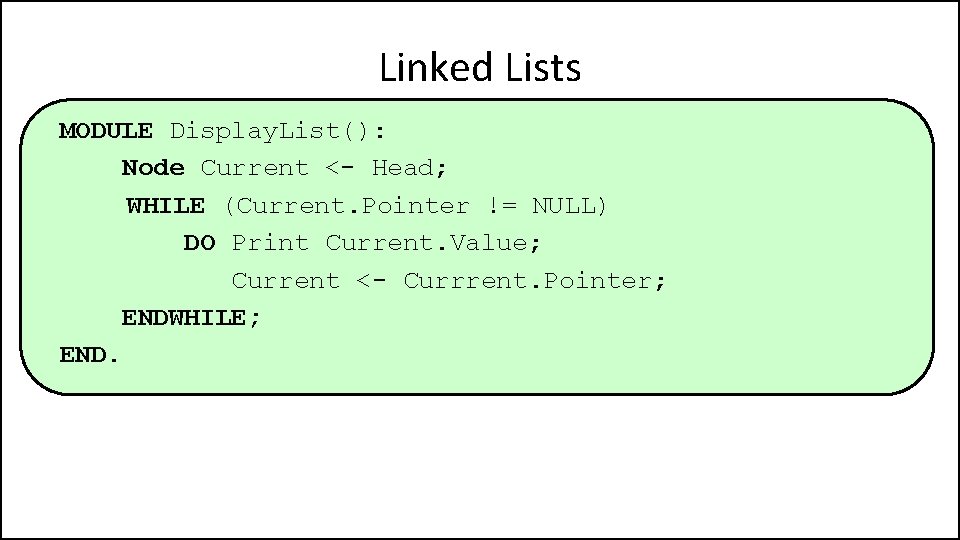
Linked Lists MODULE Display. List(): Node Current <- Head; WHILE (Current. Pointer != NULL) DO Print Current. Value; Current <- Currrent. Pointer; ENDWHILE; END.
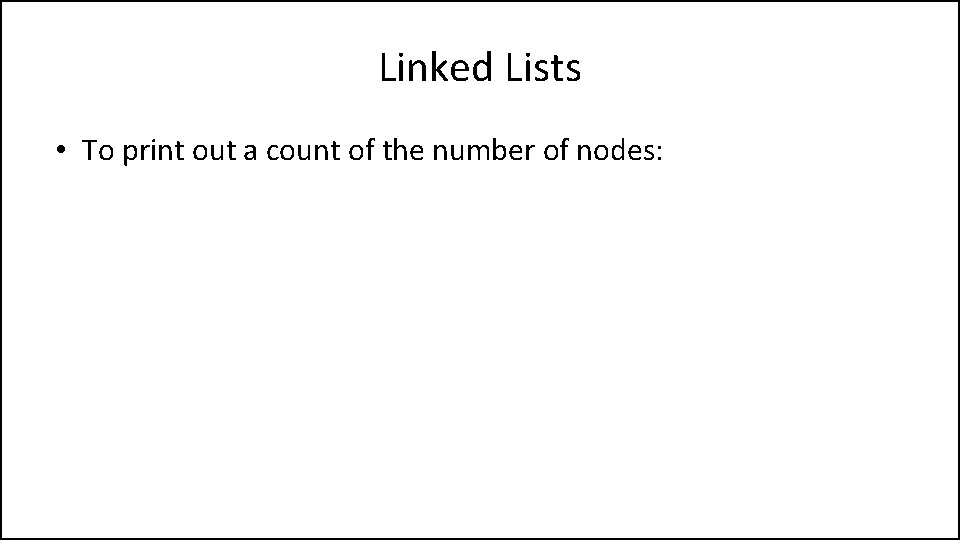
Linked Lists • To print out a count of the number of nodes:
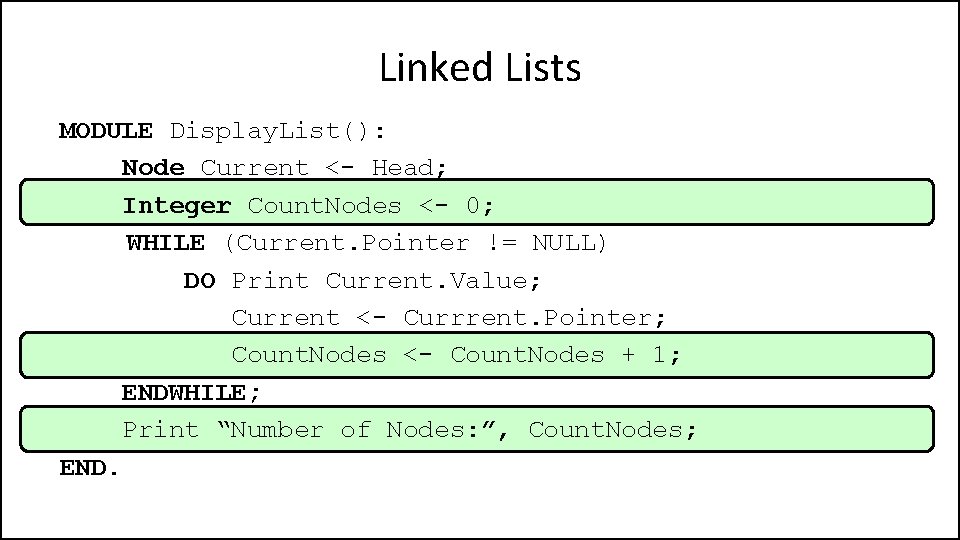
Linked Lists MODULE Display. List(): Node Current <- Head; Integer Count. Nodes <- 0; WHILE (Current. Pointer != NULL) DO Print Current. Value; Current <- Currrent. Pointer; Count. Nodes <- Count. Nodes + 1; ENDWHILE; Print “Number of Nodes: ”, Count. Nodes; END.
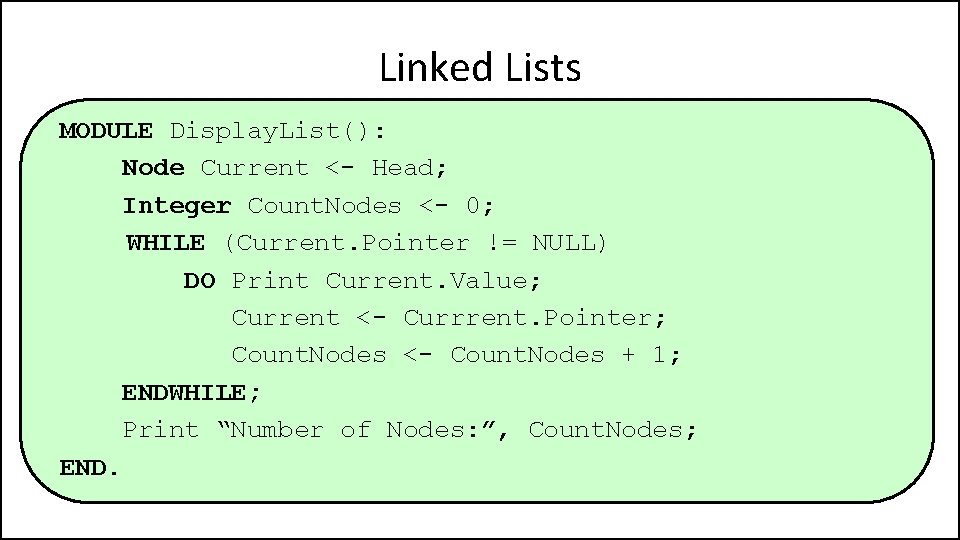
Linked Lists MODULE Display. List(): Node Current <- Head; Integer Count. Nodes <- 0; WHILE (Current. Pointer != NULL) DO Print Current. Value; Current <- Currrent. Pointer; Count. Nodes <- Count. Nodes + 1; ENDWHILE; Print “Number of Nodes: ”, Count. Nodes; END.
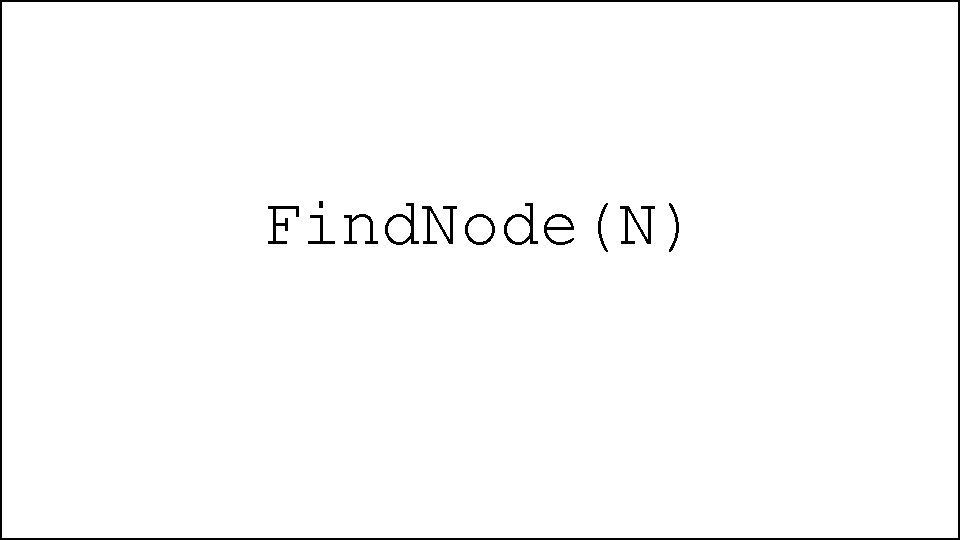
Find. Node(N)
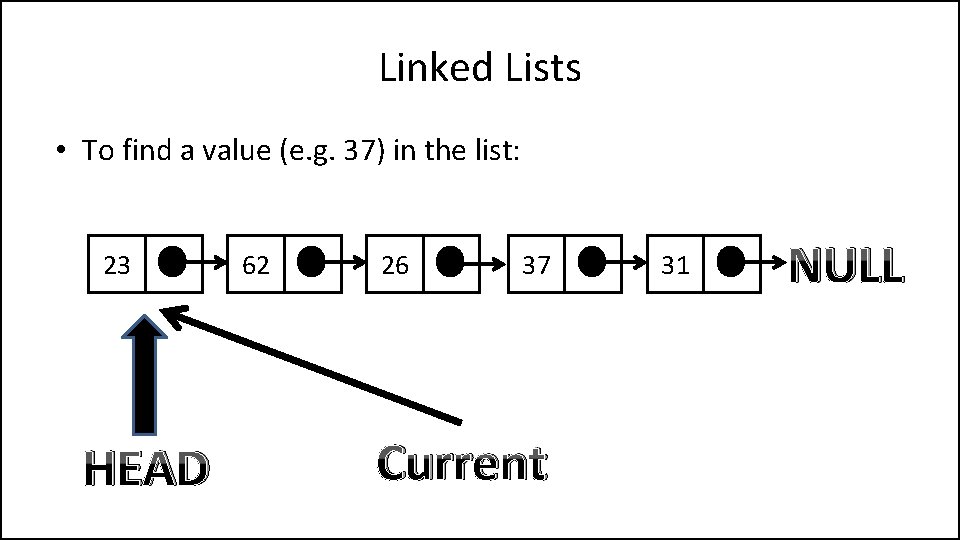
Linked Lists • To find a value (e. g. 37) in the list: 23 HEAD 62 26 37 Current 31 NULL
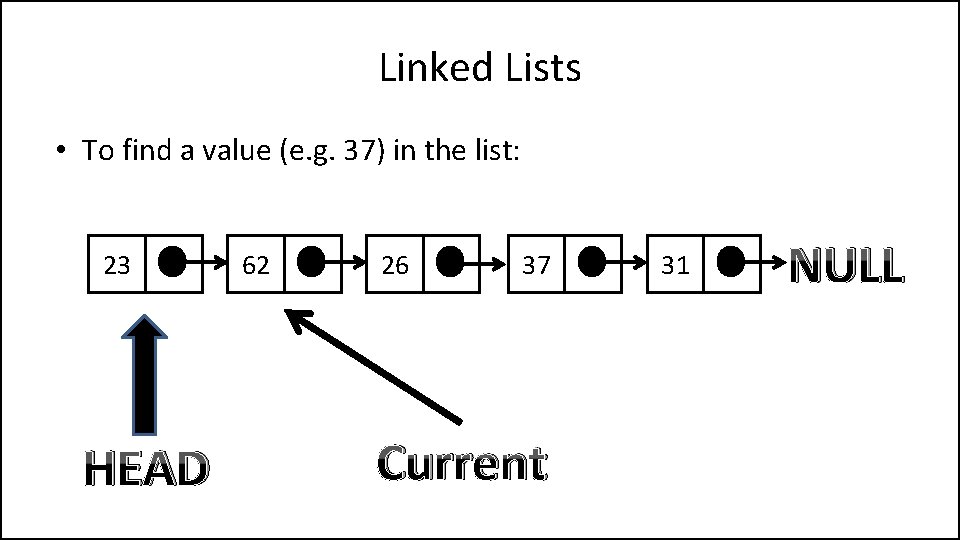
Linked Lists • To find a value (e. g. 37) in the list: 23 HEAD 62 26 37 Current 31 NULL
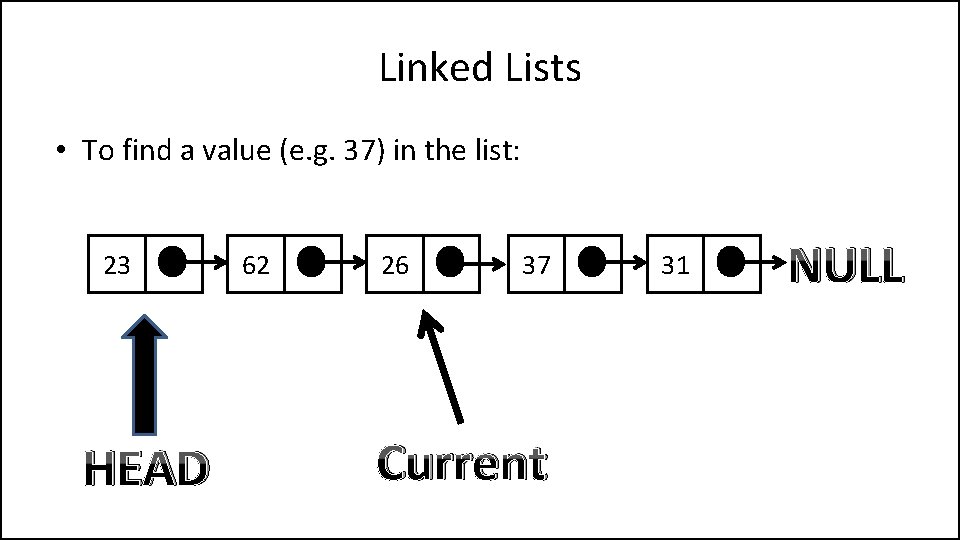
Linked Lists • To find a value (e. g. 37) in the list: 23 HEAD 62 26 37 Current 31 NULL
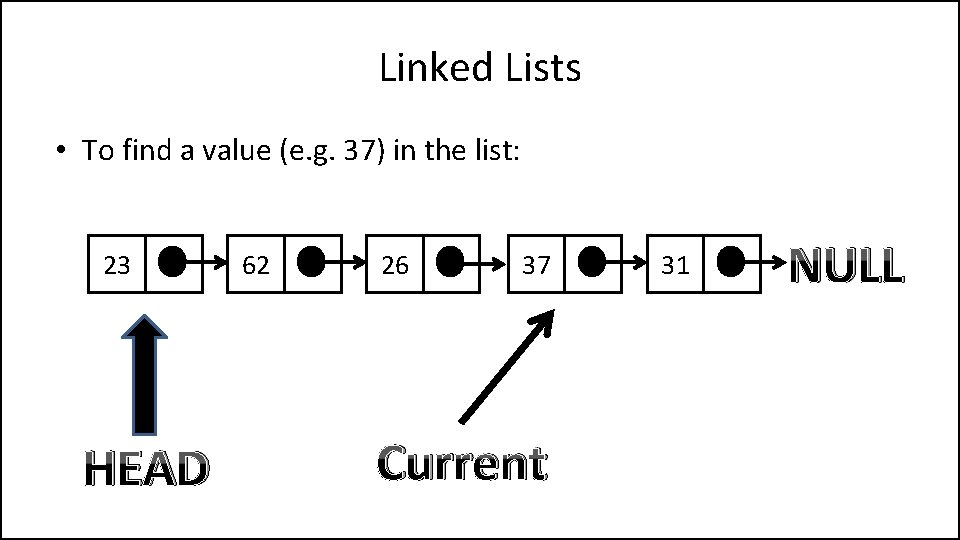
Linked Lists • To find a value (e. g. 37) in the list: 23 HEAD 62 26 37 Current 31 NULL
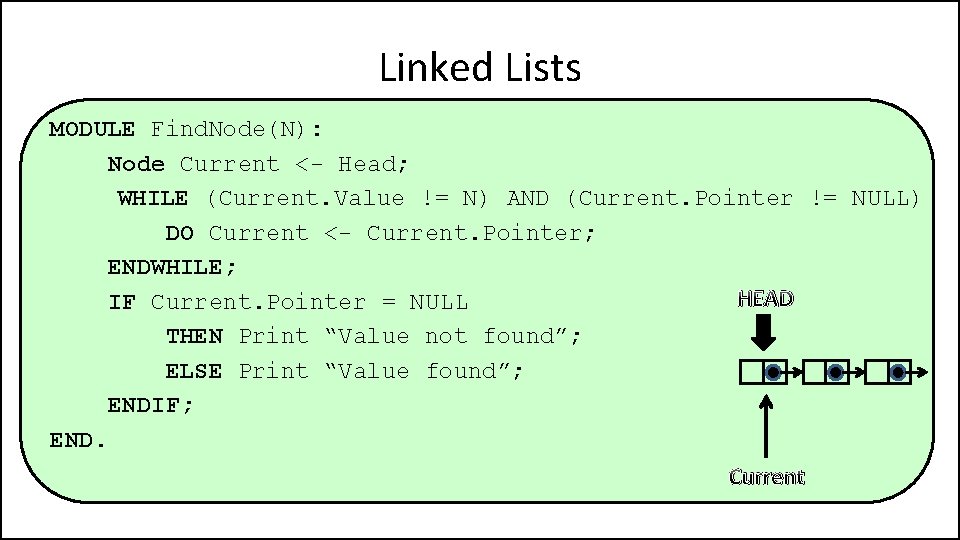
Linked Lists MODULE Find. Node(N): Node Current <- Head; WHILE (Current. Value != N) AND (Current. Pointer != NULL) DO Current <- Current. Pointer; ENDWHILE; HEAD IF Current. Pointer = NULL THEN Print “Value not found”; ELSE Print “Value found”; ENDIF; END. Current
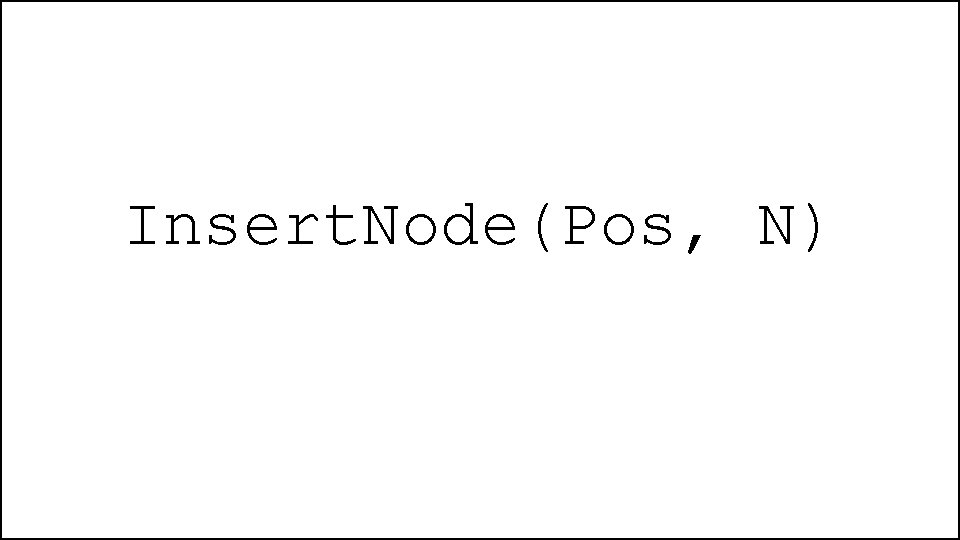
Insert. Node(Pos, N)
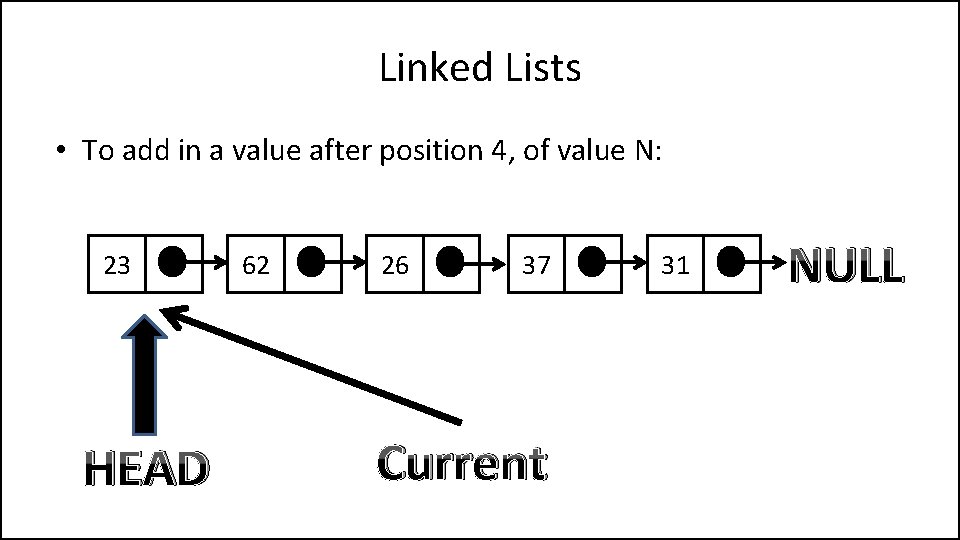
Linked Lists • To add in a value after position 4, of value N: 23 HEAD 62 26 37 Current 31 NULL
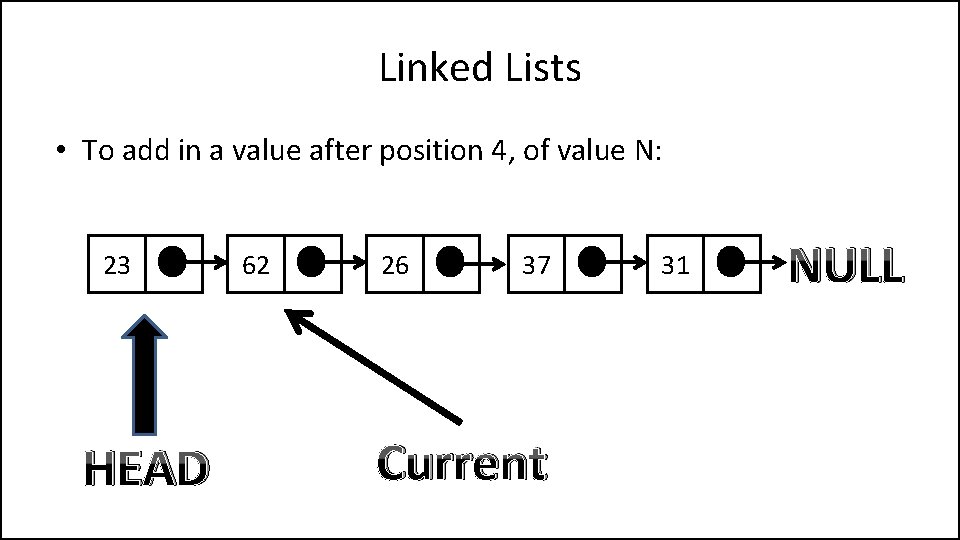
Linked Lists • To add in a value after position 4, of value N: 23 HEAD 62 26 37 Current 31 NULL
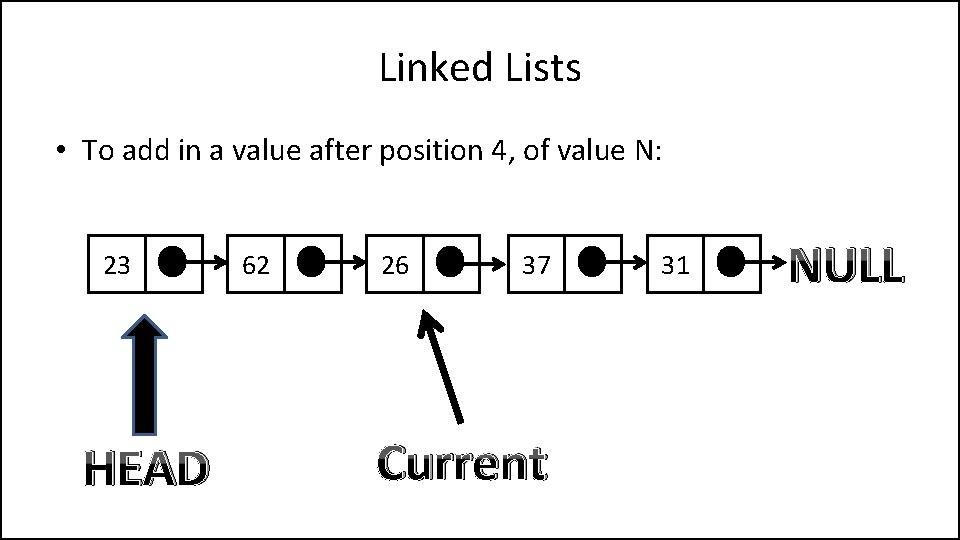
Linked Lists • To add in a value after position 4, of value N: 23 HEAD 62 26 37 Current 31 NULL
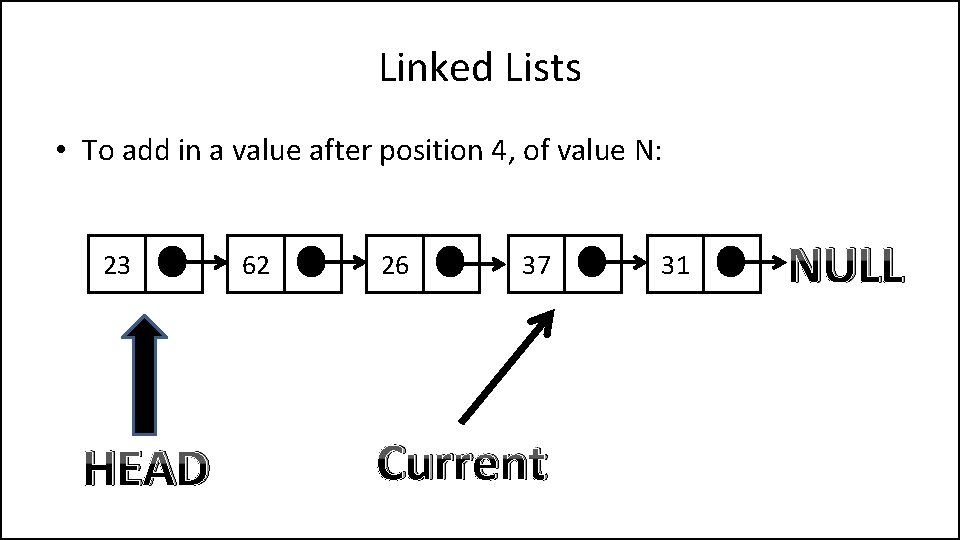
Linked Lists • To add in a value after position 4, of value N: 23 HEAD 62 26 37 Current 31 NULL
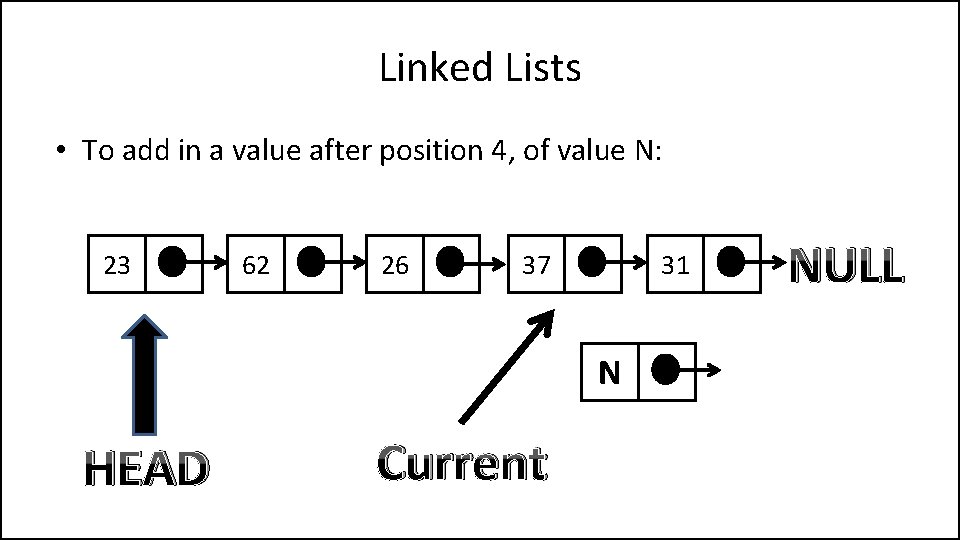
Linked Lists • To add in a value after position 4, of value N: 23 62 26 37 31 N HEAD Current NULL
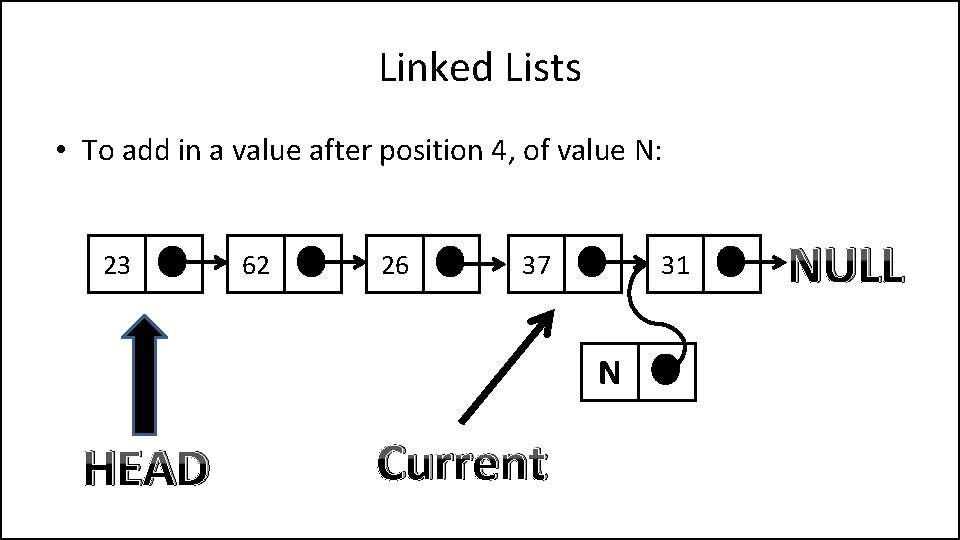
Linked Lists • To add in a value after position 4, of value N: 23 62 26 37 31 N HEAD Current NULL
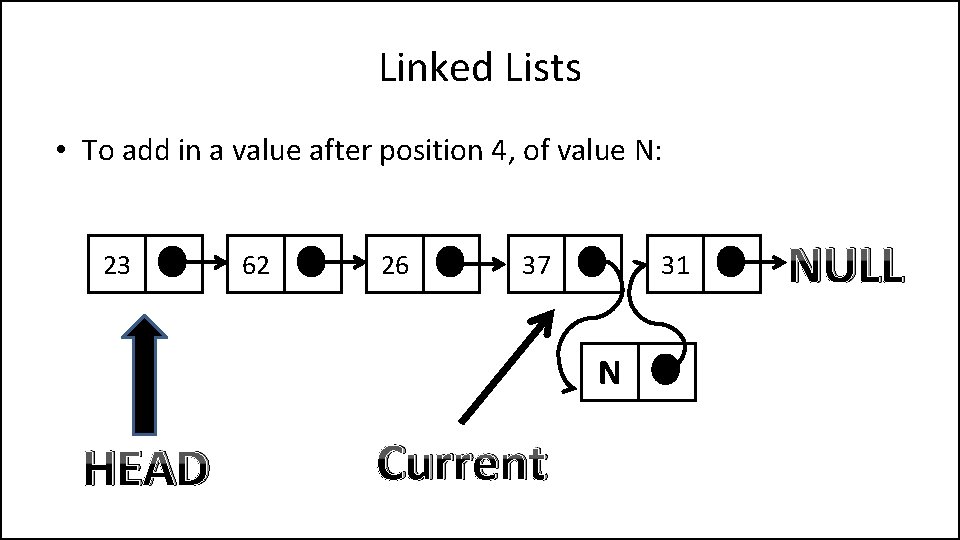
Linked Lists • To add in a value after position 4, of value N: 23 62 26 37 31 N HEAD Current NULL
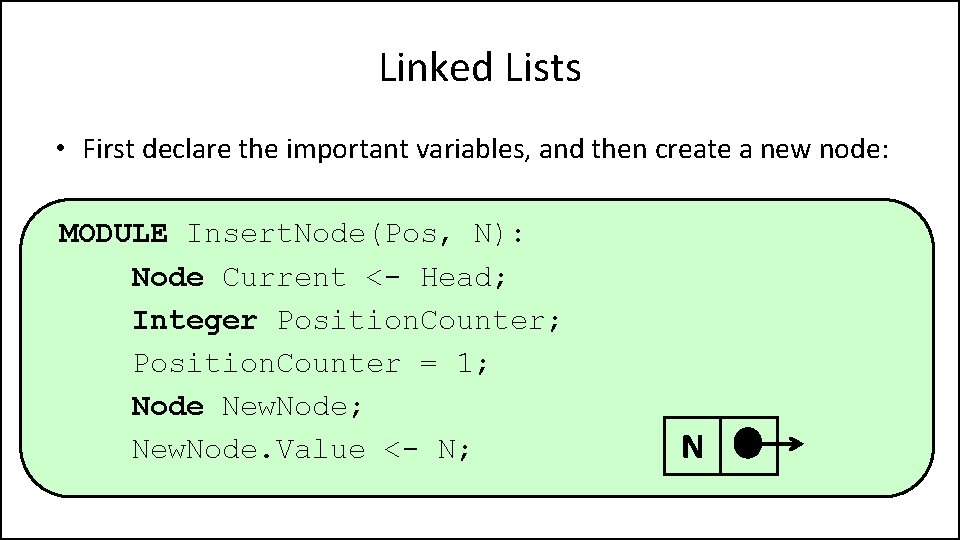
Linked Lists • First declare the important variables, and then create a new node: MODULE Insert. Node(Pos, N): Node Current <- Head; Integer Position. Counter; Position. Counter = 1; Node New. Node; New. Node. Value <- N; N
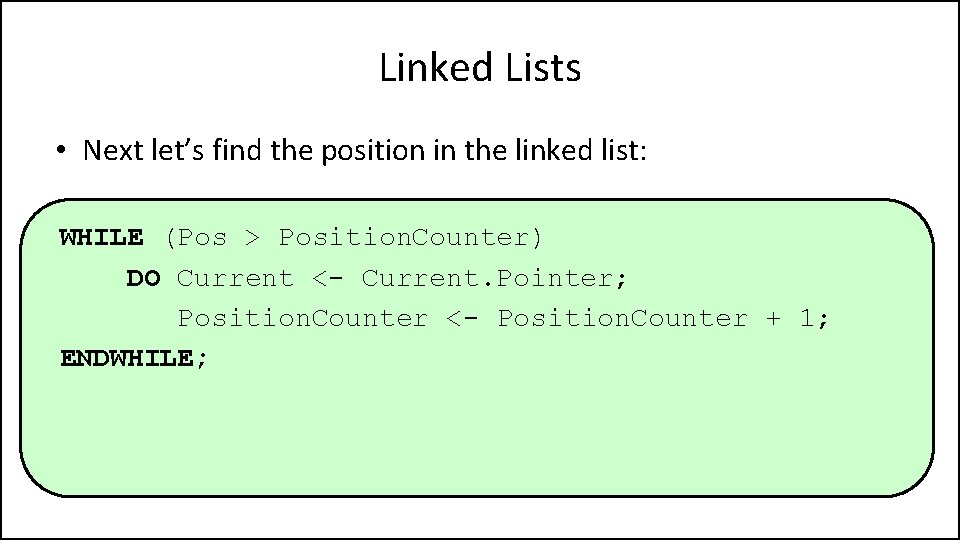
Linked Lists • Next let’s find the position in the linked list: WHILE (Pos > Position. Counter) DO Current <- Current. Pointer; Position. Counter <- Position. Counter + 1; ENDWHILE;
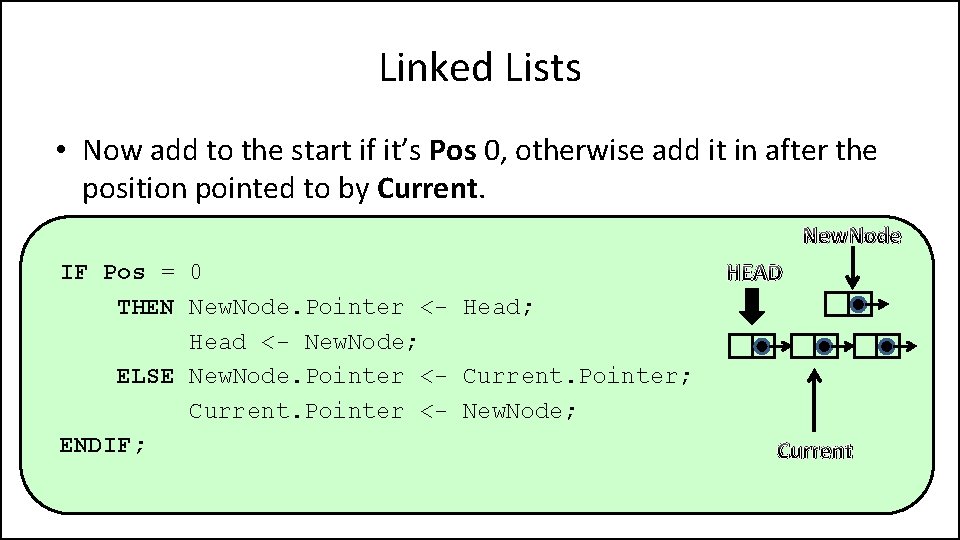
Linked Lists • Now add to the start if it’s Pos 0, otherwise add it in after the position pointed to by Current. New. Node IF Pos = 0 THEN New. Node. Pointer <- Head; Head <- New. Node; ELSE New. Node. Pointer <- Current. Pointer; Current. Pointer <- New. Node; ENDIF; HEAD Current
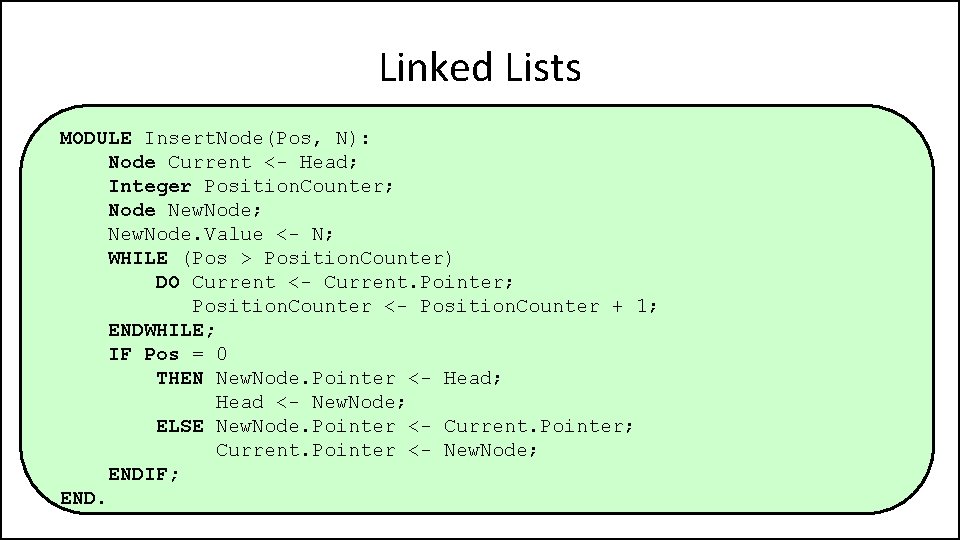
Linked Lists MODULE Insert. Node(Pos, N): Node Current <- Head; Integer Position. Counter; Node New. Node; New. Node. Value <- N; WHILE (Pos > Position. Counter) DO Current <- Current. Pointer; Position. Counter <- Position. Counter + 1; ENDWHILE; IF Pos = 0 THEN New. Node. Pointer <- Head; Head <- New. Node; ELSE New. Node. Pointer <- Current. Pointer; Current. Pointer <- New. Node; ENDIF; END.
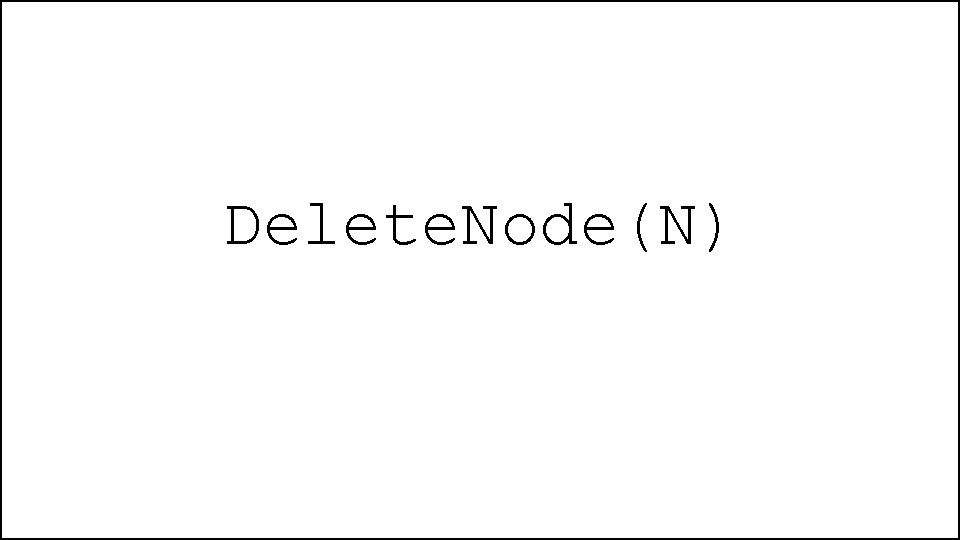
Delete. Node(N)
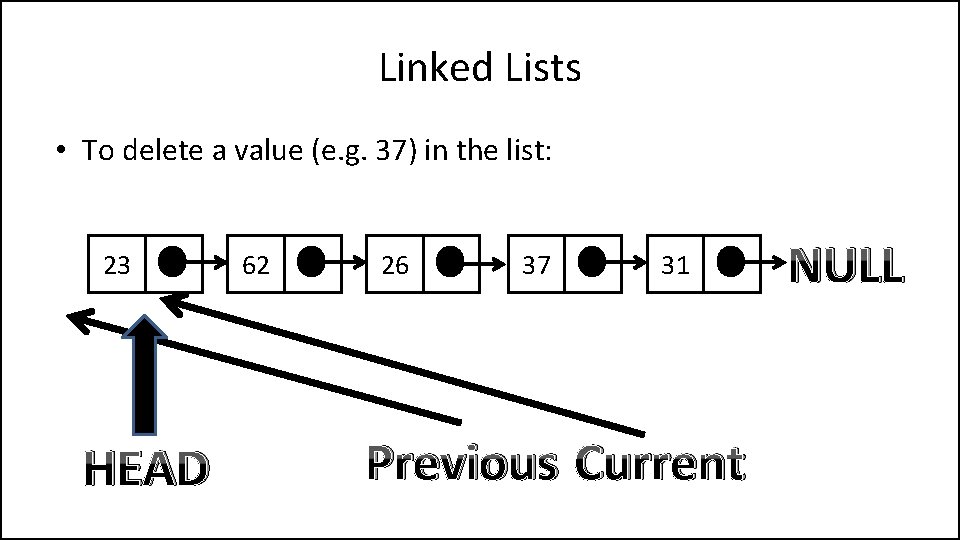
Linked Lists • To delete a value (e. g. 37) in the list: 23 HEAD 62 26 37 31 Previous Current NULL
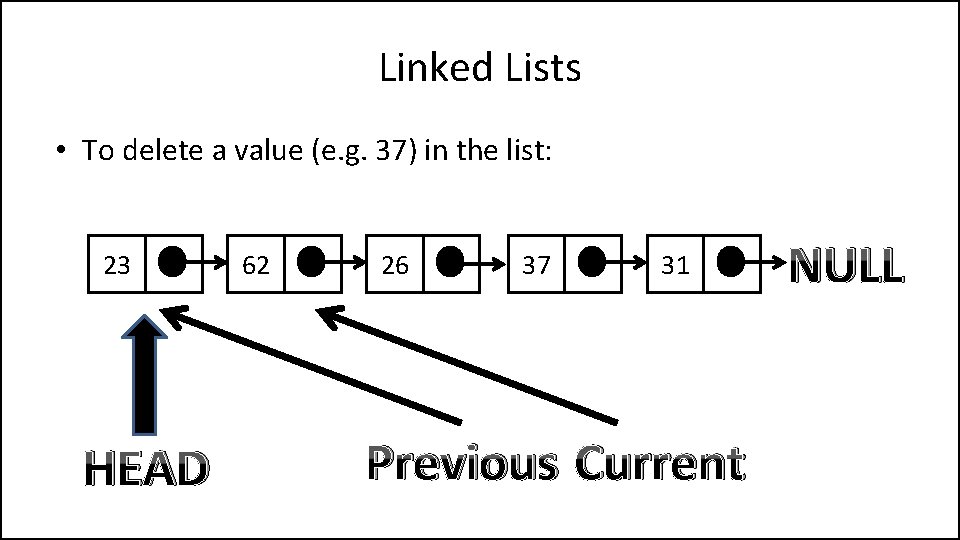
Linked Lists • To delete a value (e. g. 37) in the list: 23 HEAD 62 26 37 31 Previous Current NULL
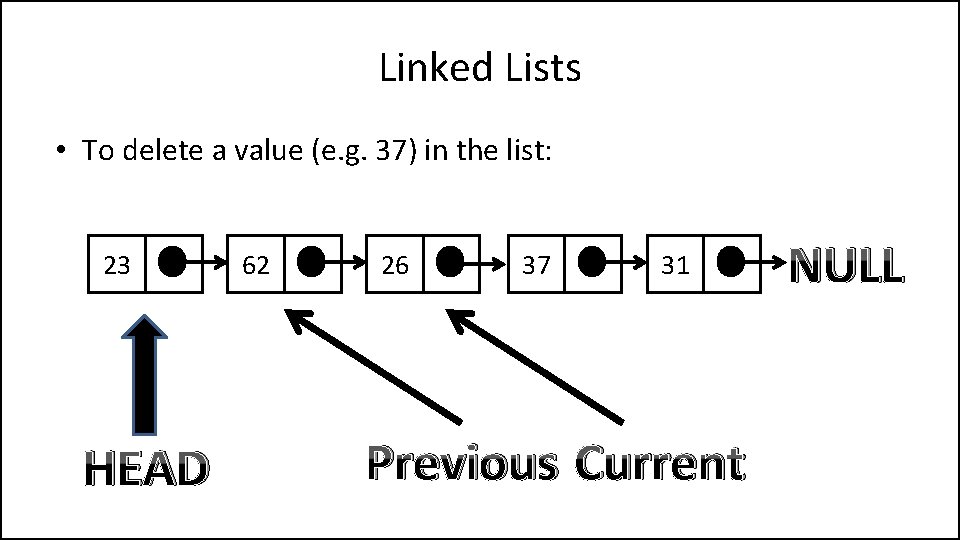
Linked Lists • To delete a value (e. g. 37) in the list: 23 HEAD 62 26 37 31 Previous Current NULL
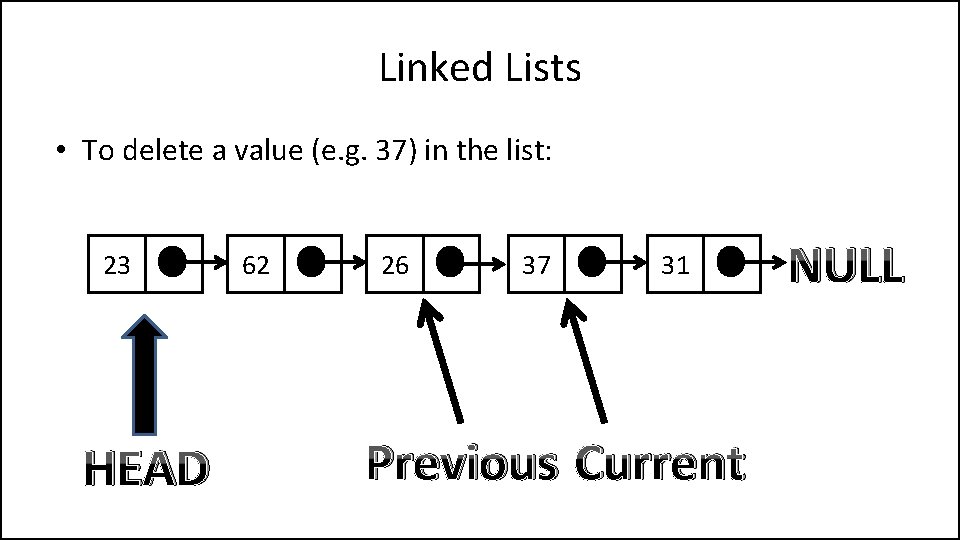
Linked Lists • To delete a value (e. g. 37) in the list: 23 HEAD 62 26 37 31 Previous Current NULL
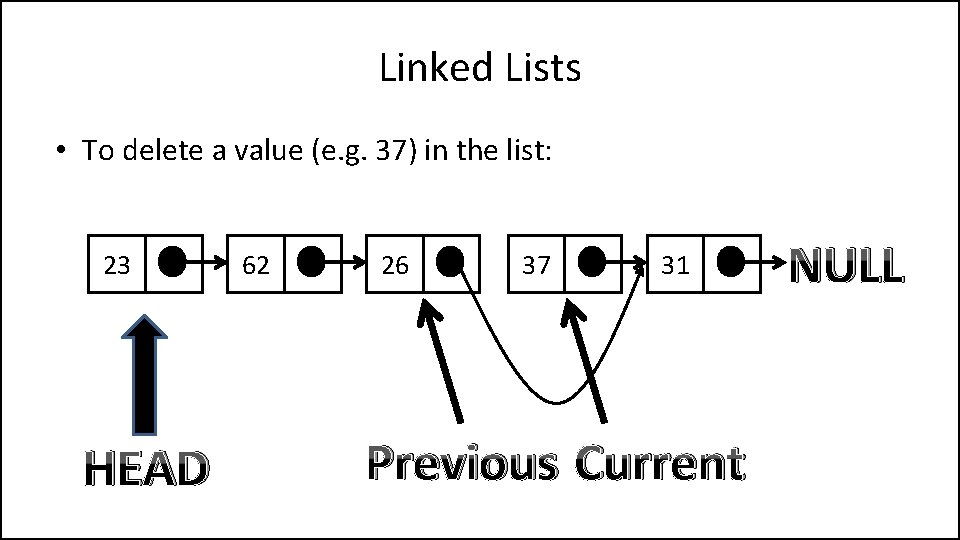
Linked Lists • To delete a value (e. g. 37) in the list: 23 HEAD 62 26 37 31 Previous Current NULL
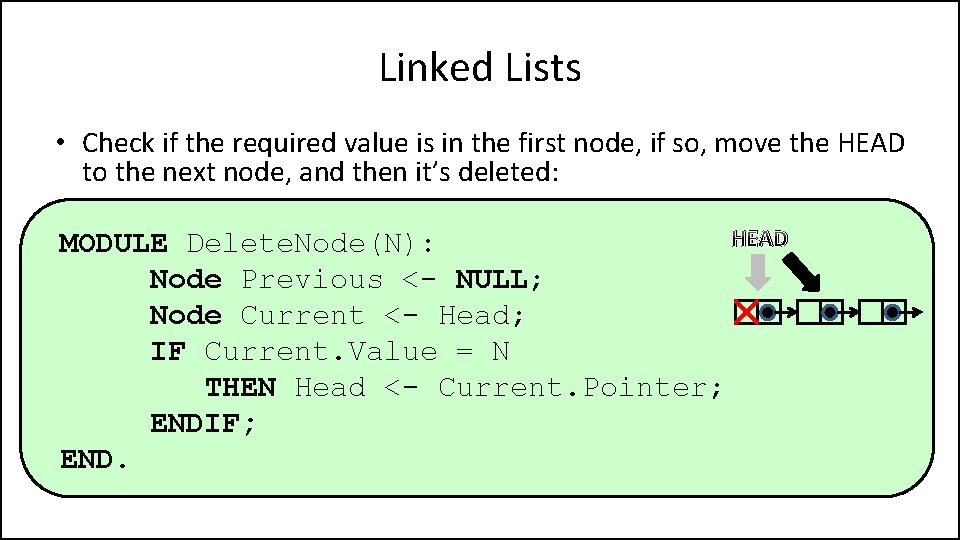
Linked Lists • Check if the required value is in the first node, if so, move the HEAD to the next node, and then it’s deleted: HEAD MODULE Delete. Node(N): Node Previous <- NULL; Node Current <- Head; IF Current. Value = N THEN Head <- Current. Pointer; ENDIF; END.
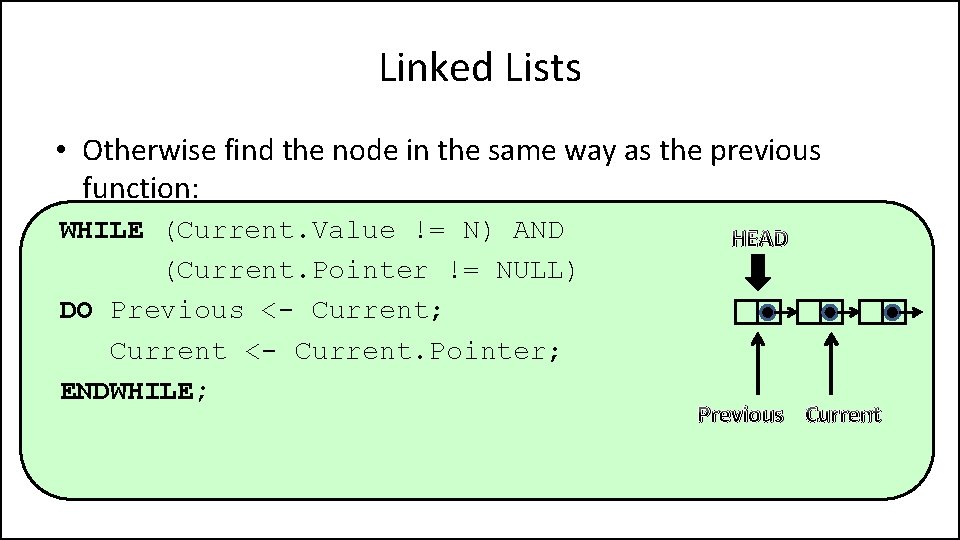
Linked Lists • Otherwise find the node in the same way as the previous function: WHILE (Current. Value != N) AND (Current. Pointer != NULL) DO Previous <- Current; Current <- Current. Pointer; ENDWHILE; HEAD Previous Current
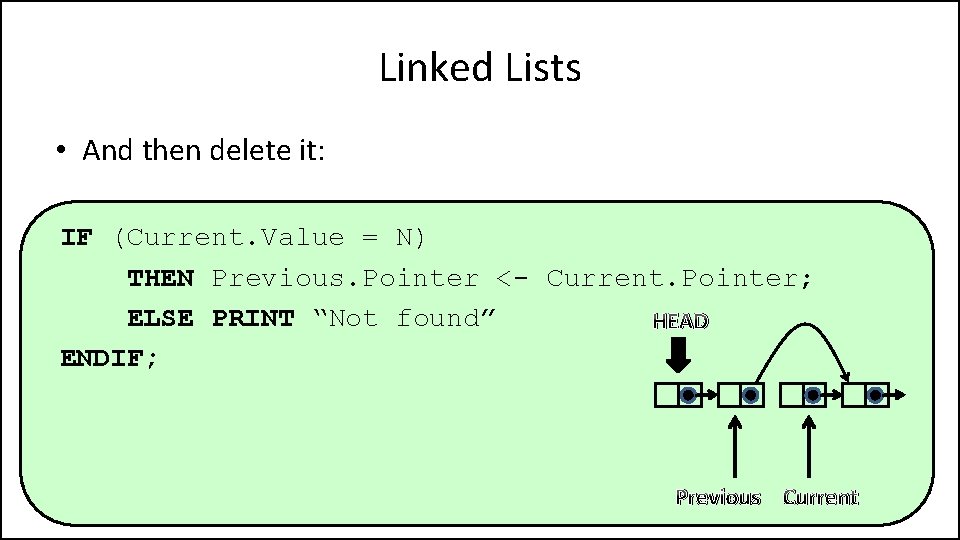
Linked Lists • And then delete it: IF (Current. Value = N) THEN Previous. Pointer <- Current. Pointer; ELSE PRINT “Not found” HEAD ENDIF; Previous Current
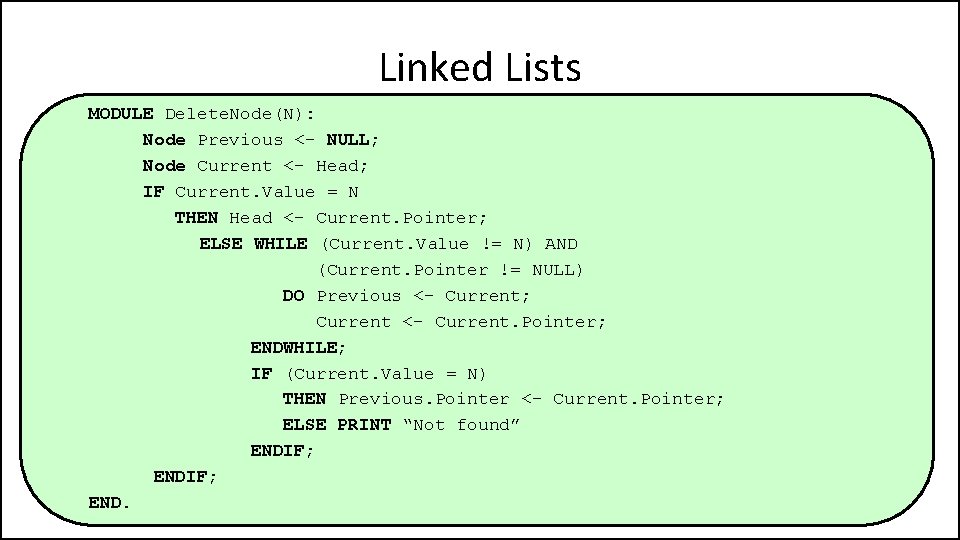
Linked Lists MODULE Delete. Node(N): Node Previous <- NULL; Node Current <- Head; IF Current. Value = N THEN Head <- Current. Pointer; ELSE WHILE (Current. Value != N) AND (Current. Pointer != NULL) DO Previous <- Current; Current <- Current. Pointer; ENDWHILE; IF (Current. Value = N) THEN Previous. Pointer <- Current. Pointer; ELSE PRINT “Not found” ENDIF; END.
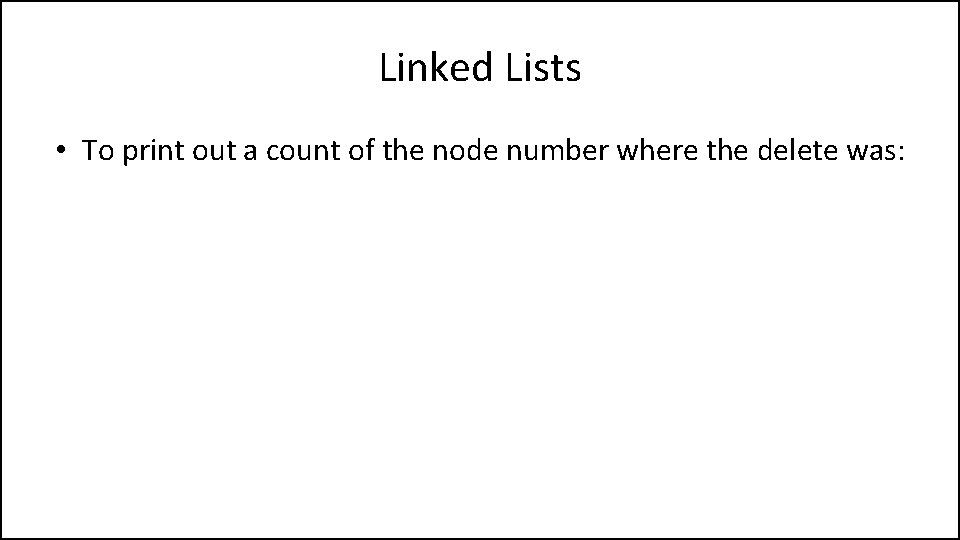
Linked Lists • To print out a count of the node number where the delete was:
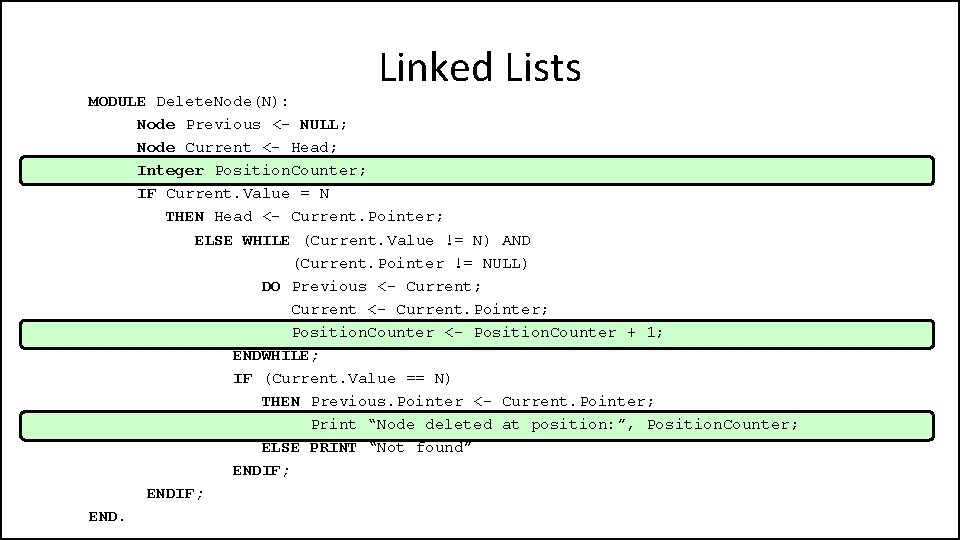
Linked Lists MODULE Delete. Node(N): Node Previous <- NULL; Node Current <- Head; Integer Position. Counter; IF Current. Value = N THEN Head <- Current. Pointer; ELSE WHILE (Current. Value != N) AND (Current. Pointer != NULL) DO Previous <- Current; Current <- Current. Pointer; Position. Counter <- Position. Counter + 1; ENDWHILE; IF (Current. Value == N) THEN Previous. Pointer <- Current. Pointer; Print “Node deleted at position: ”, Position. Counter; ELSE PRINT “Not found” ENDIF; END.
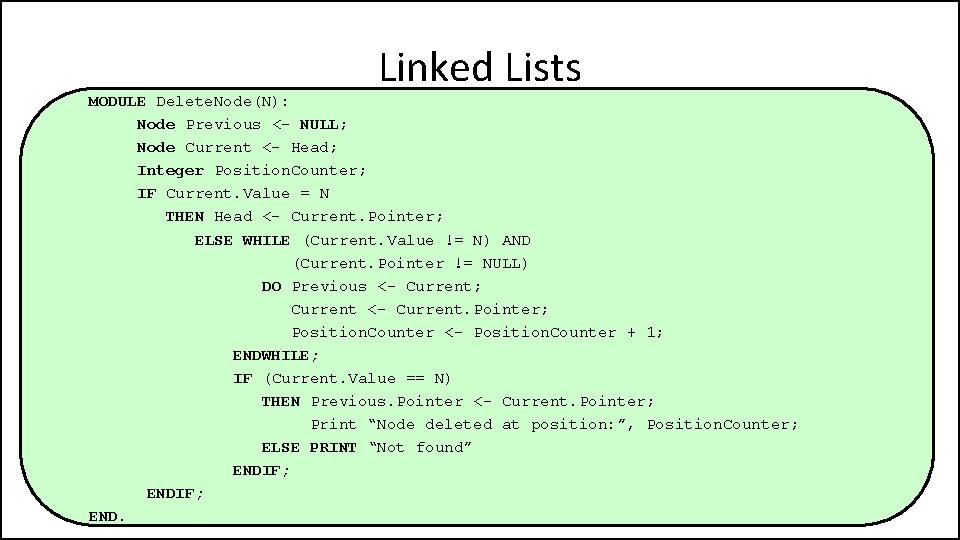
Linked Lists MODULE Delete. Node(N): Node Previous <- NULL; Node Current <- Head; Integer Position. Counter; IF Current. Value = N THEN Head <- Current. Pointer; ELSE WHILE (Current. Value != N) AND (Current. Pointer != NULL) DO Previous <- Current; Current <- Current. Pointer; Position. Counter <- Position. Counter + 1; ENDWHILE; IF (Current. Value == N) THEN Previous. Pointer <- Current. Pointer; Print “Node deleted at position: ”, Position. Counter; ELSE PRINT “Not found” ENDIF; END.
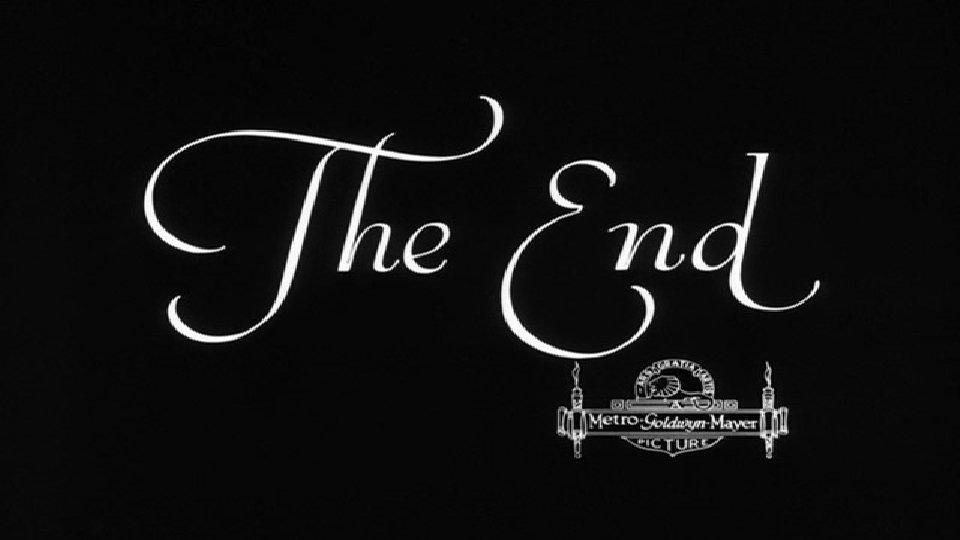
etc.
The story behind this picture
Damian gordon
Index.of.secret
Damian gordon
Singly linked list vs doubly linked list
Singly linked list vs doubly linked list
Single linked list adalah yang paling dari semua varian
Damian heywood
Fray damian massanet picture
Damian urbańczyk partnerka
Damian sturzaker
666 rule presentation
Money money money team
Damian urbańczyk wikipedia
Damian clancy
Melissa damian
Padre damian de veuster 2215 vitacura
Padre damian de veuster 2215 vitacura
Iru academy
Damian topolski
Damian krysztofik
Christophe damian
Damian marli
Padre damian de veuster 2215
Padre damian de veuster 2215
Damian forbes
Damian mac
Damian folch
Damian czudek
Python and gate
Damian harding
Padre damian de veuster 2215 vitacura
Sound waves spelling word lists
Political wish lists
Wish lists year
Python plot list of lists
Blockly c
Wish lists year
Parallel structure example
Words their way test
Clwords
Ear words
Political lists new
Lists of tuples python
What is semicolon
"new bookmarking lists 2018"
Python list methods
Who wrote this
Au words list
Ucl library reading lists
Qri word lists
Lisp lists
Lesson 3 lists practice
Www.diigo.com sign up
Mexica tribute lists ap world history
Prolog head tail
Resource list edinburgh
Swot analysis customer service example
No nonsense spelling lists
Java types of lists
Imagine that you have a bag containing 10 marbles
Imagine yourself as a living house chapter
Horror looks you right between the eyes figurative language
Imagine logo
These are photos from your photo album
Pickit images
Skull imagine
I can hardly imagine
Compare contrast athens and sparta
Well imagine that
Imagine n
Imagine + gerund
Analyse imagine john lennon
Imagine it's your birthday
Imagine you are studying english
Imagine ho
Imagine charter school hemet
Hindawi
Toamna frunzele colinda
Hot face