Stacks Implemented using Linked Lists Damian Gordon Stacks
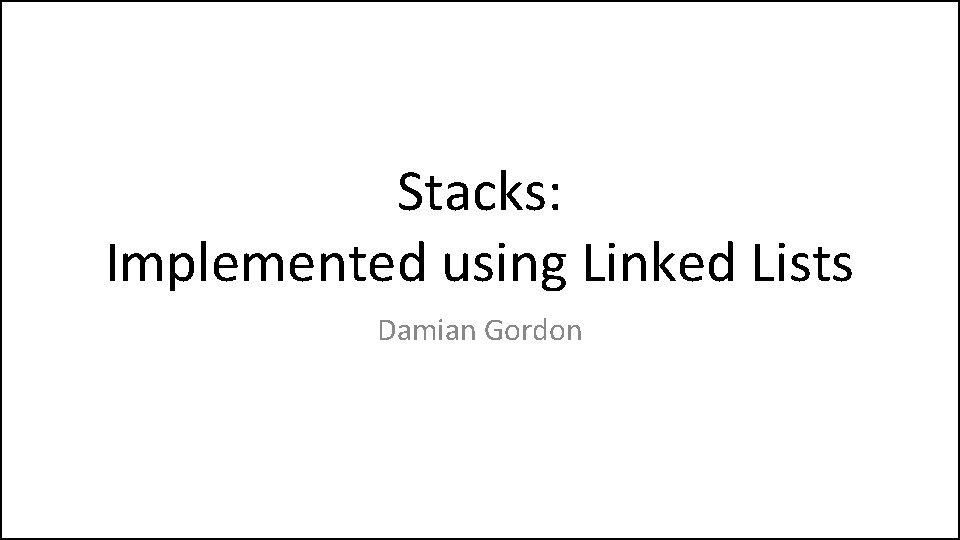
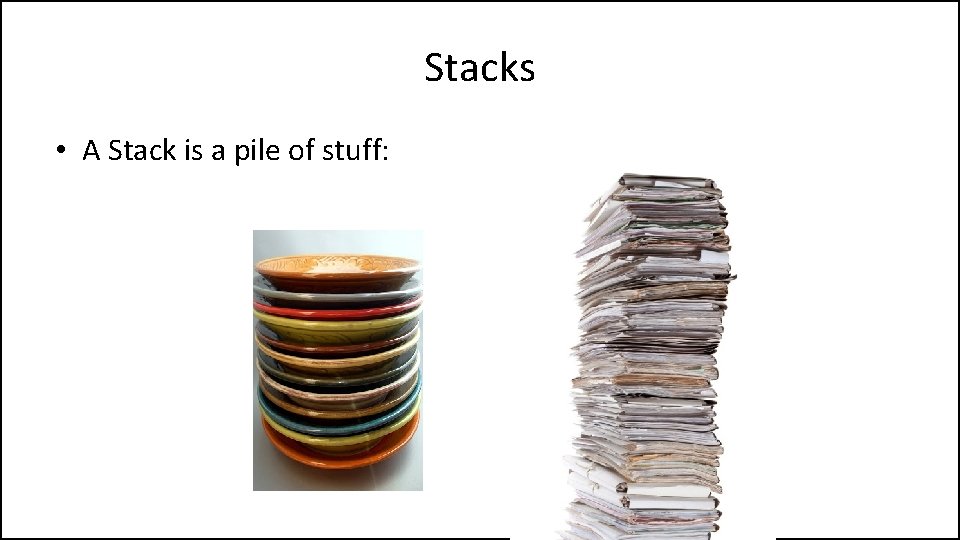
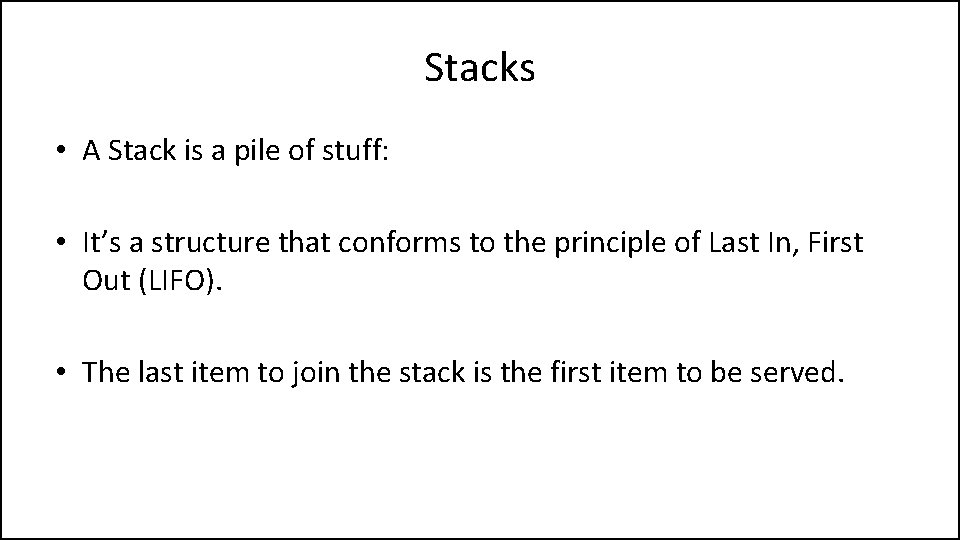
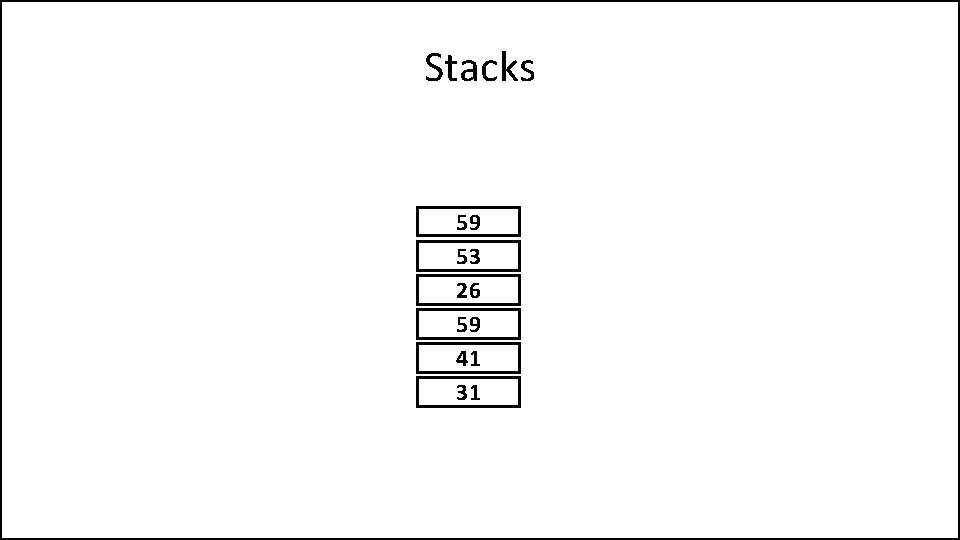
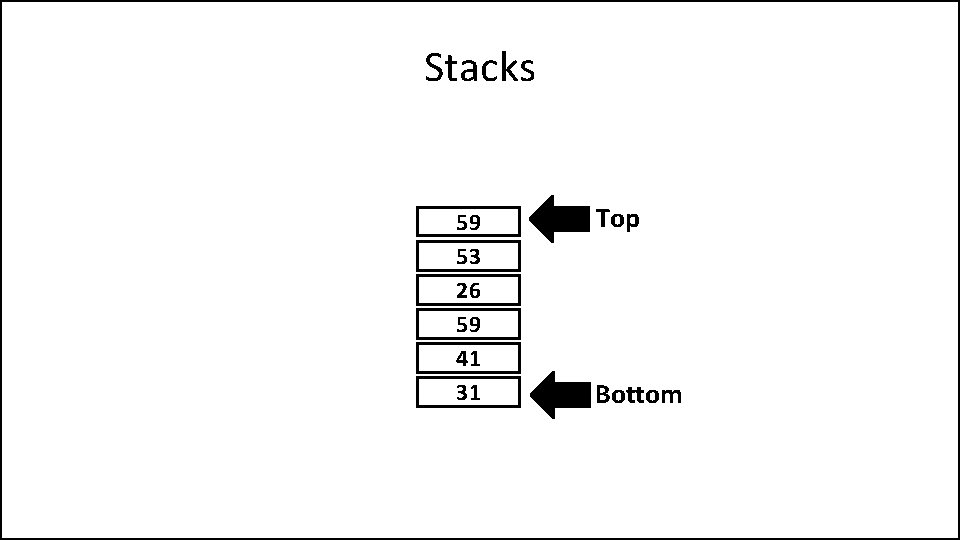
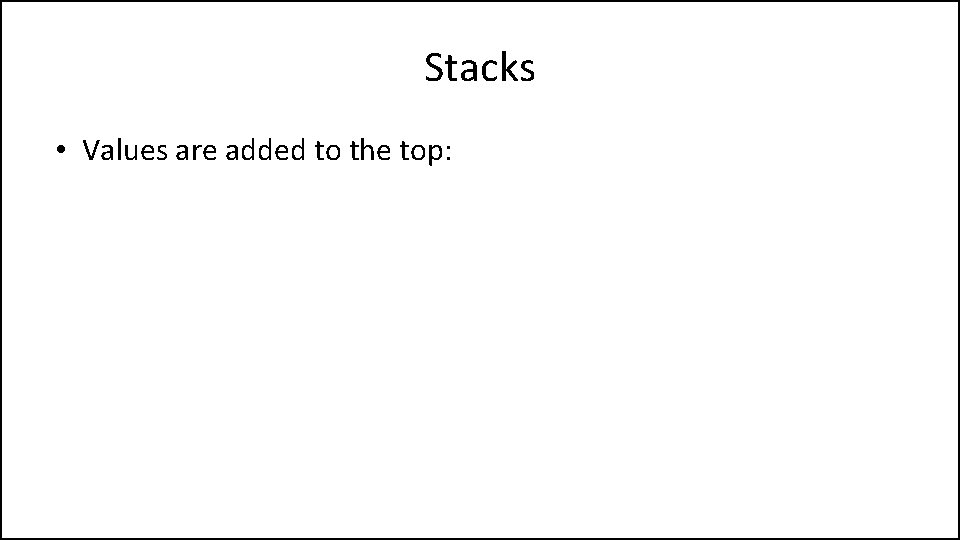
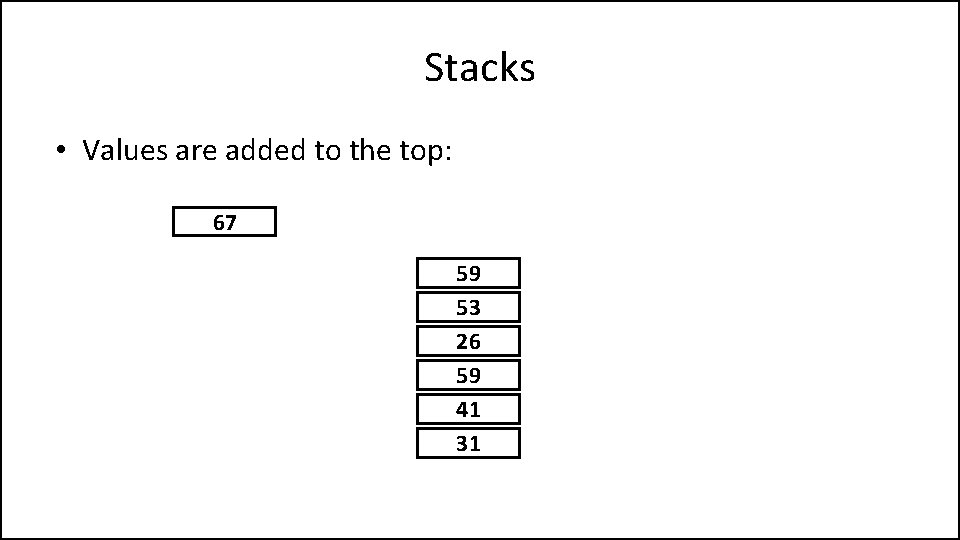
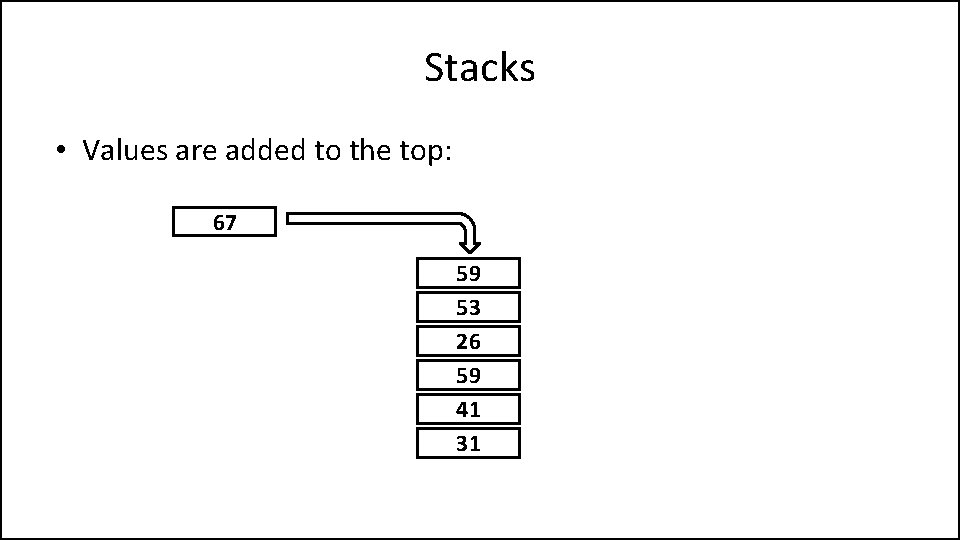
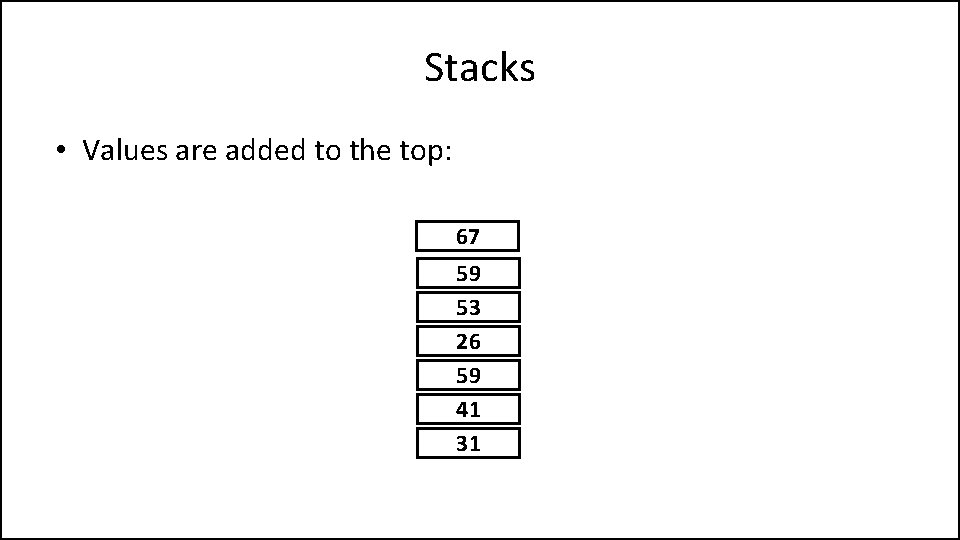
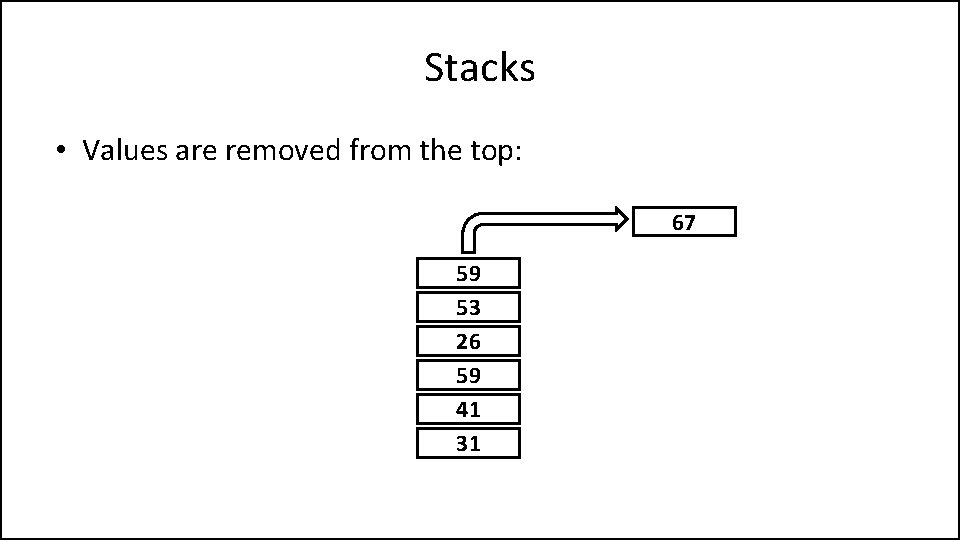
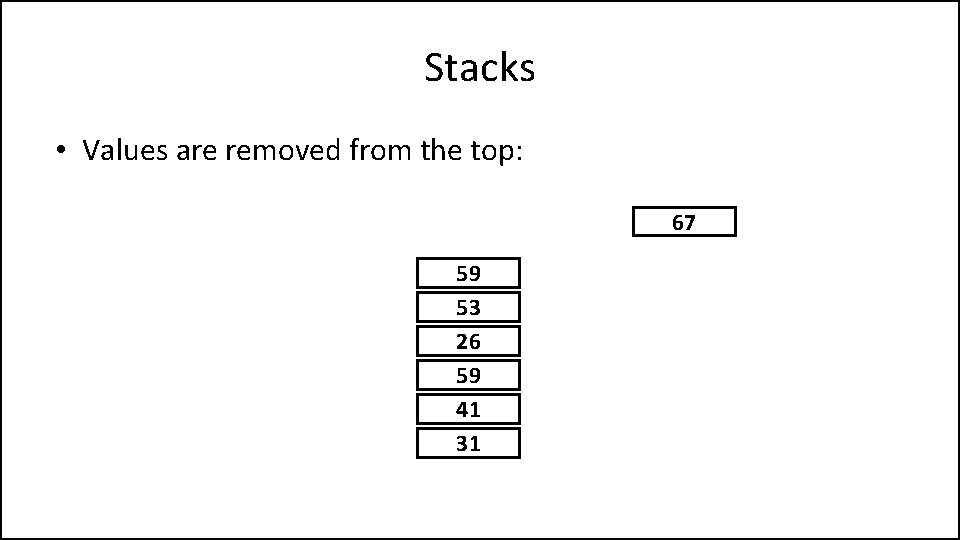
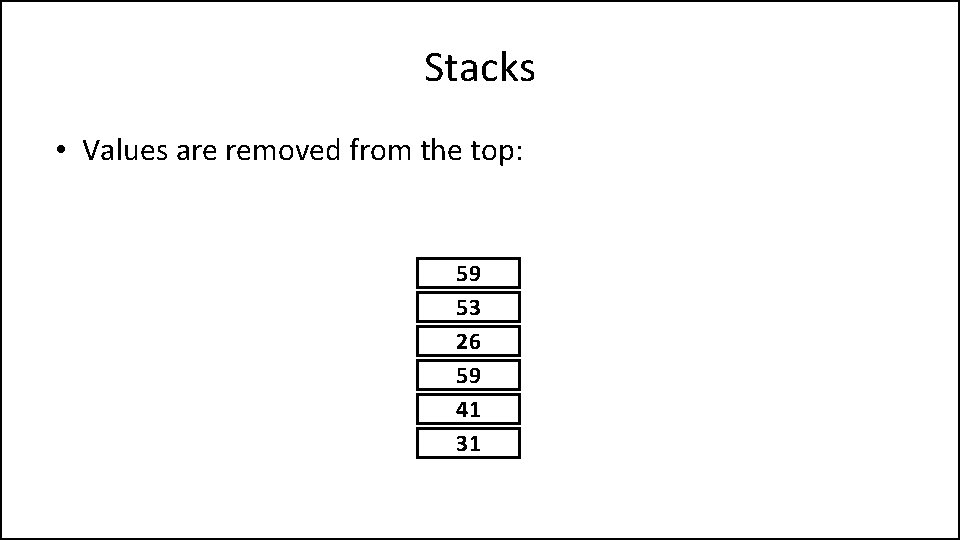
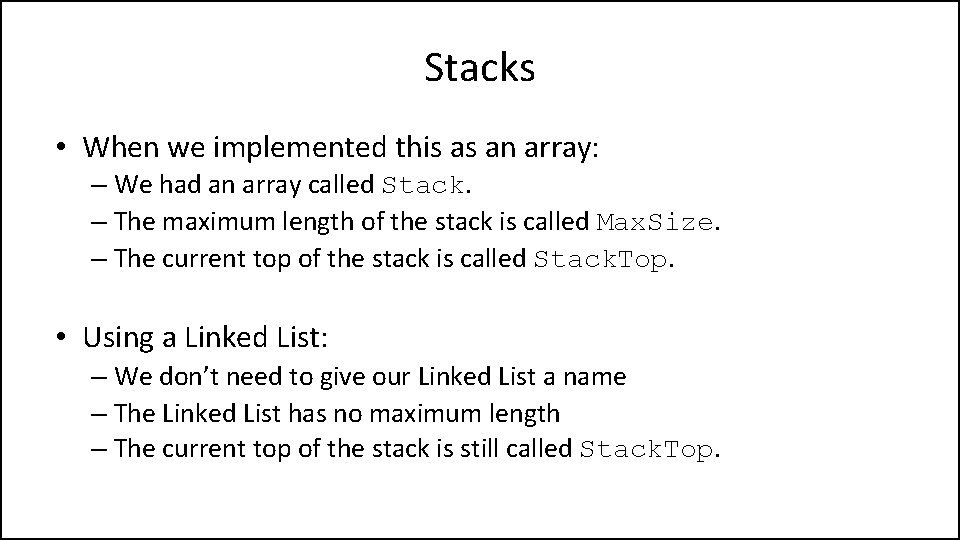
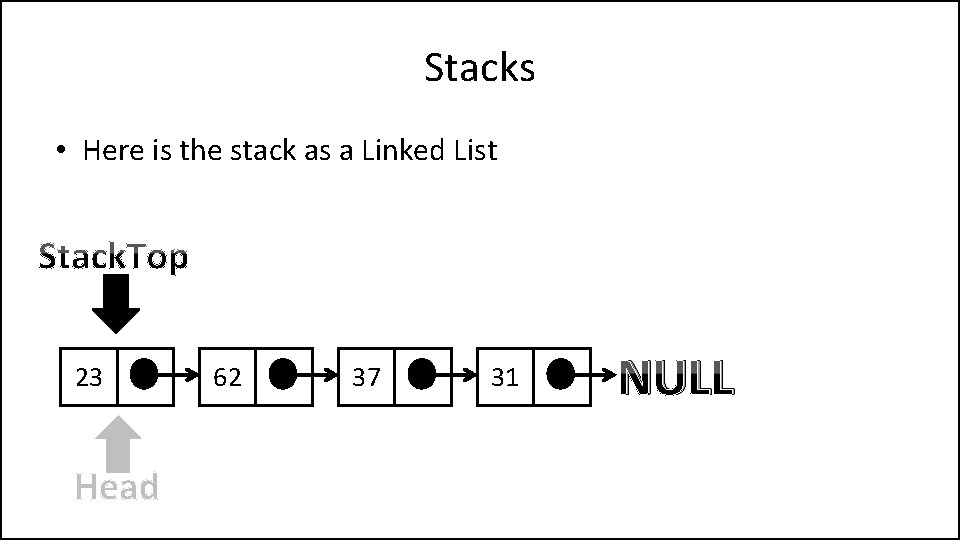
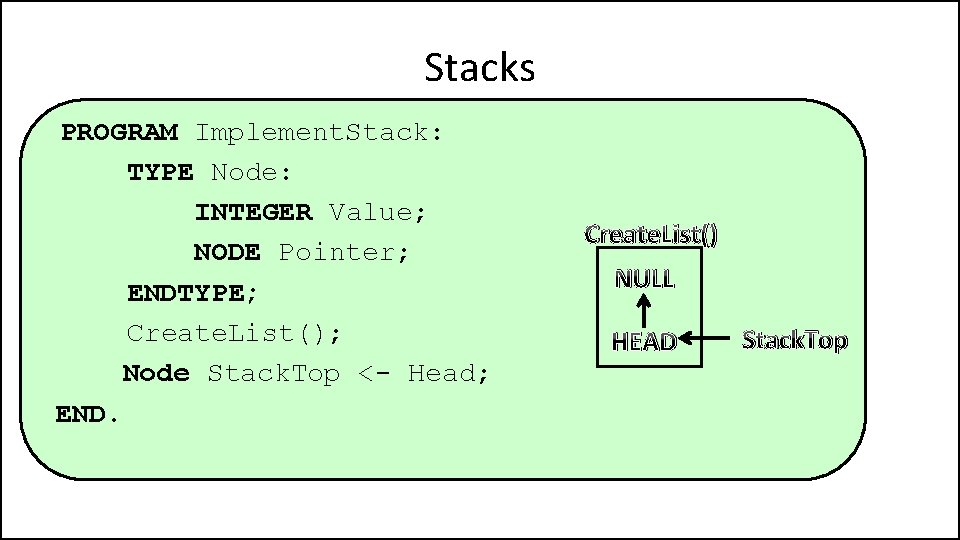
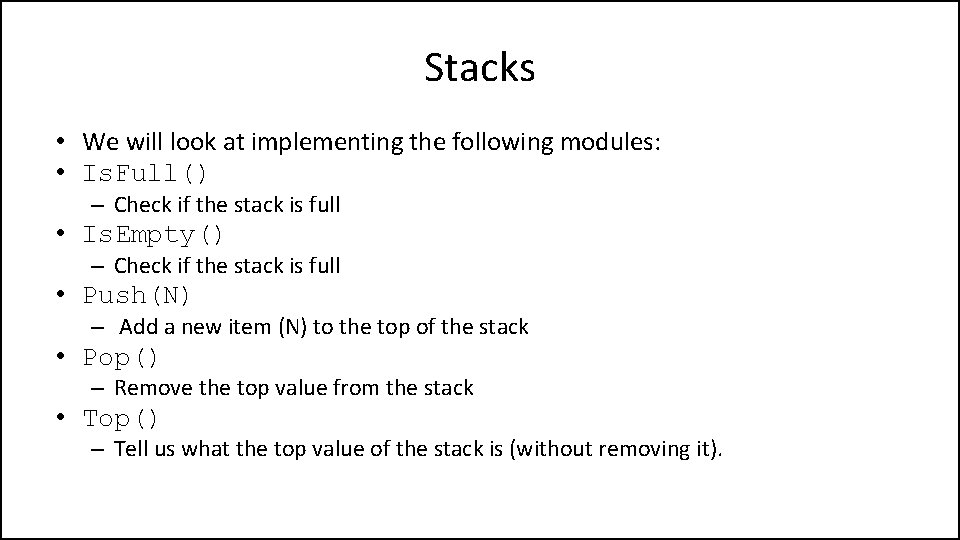
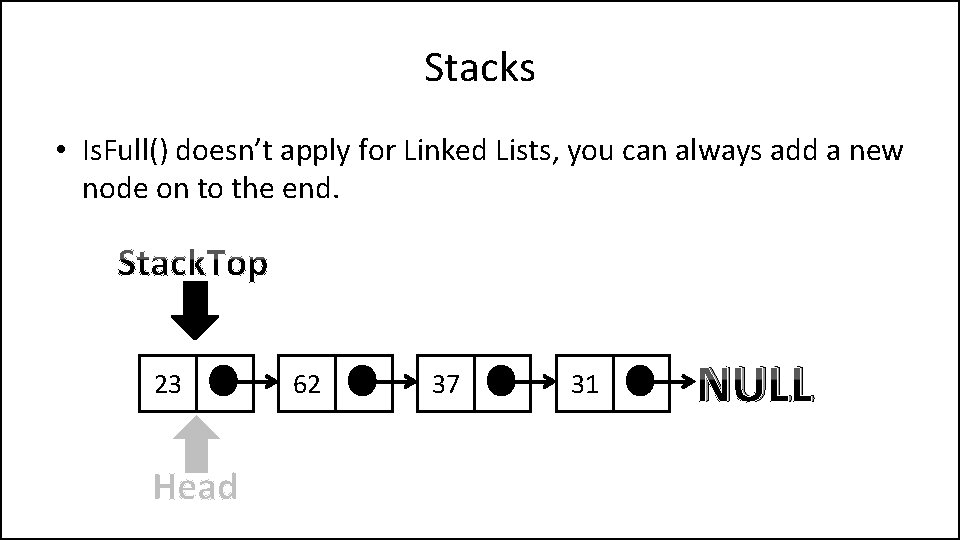
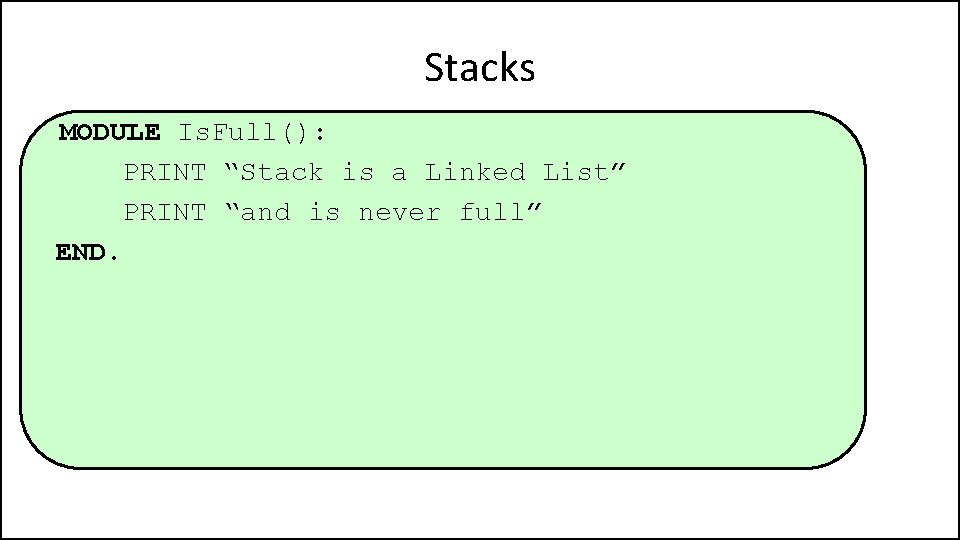
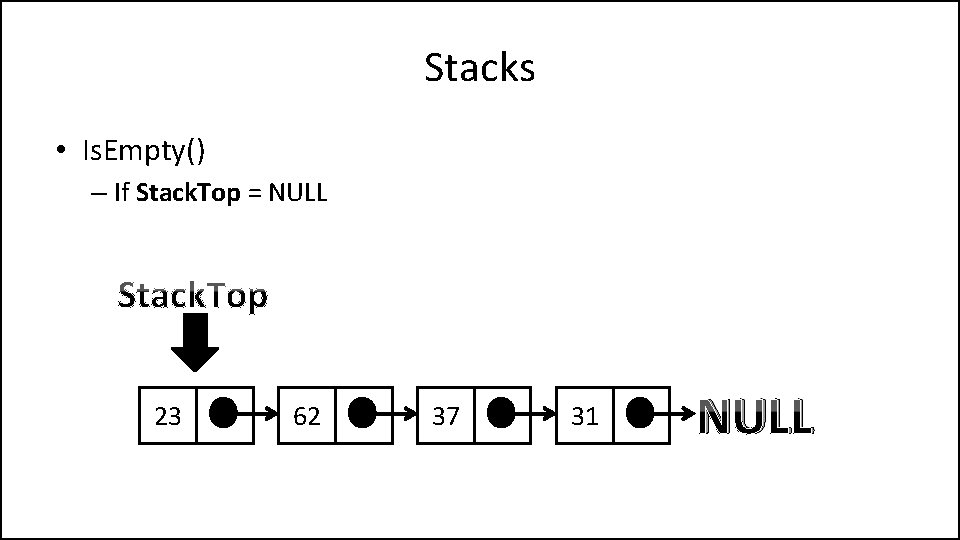
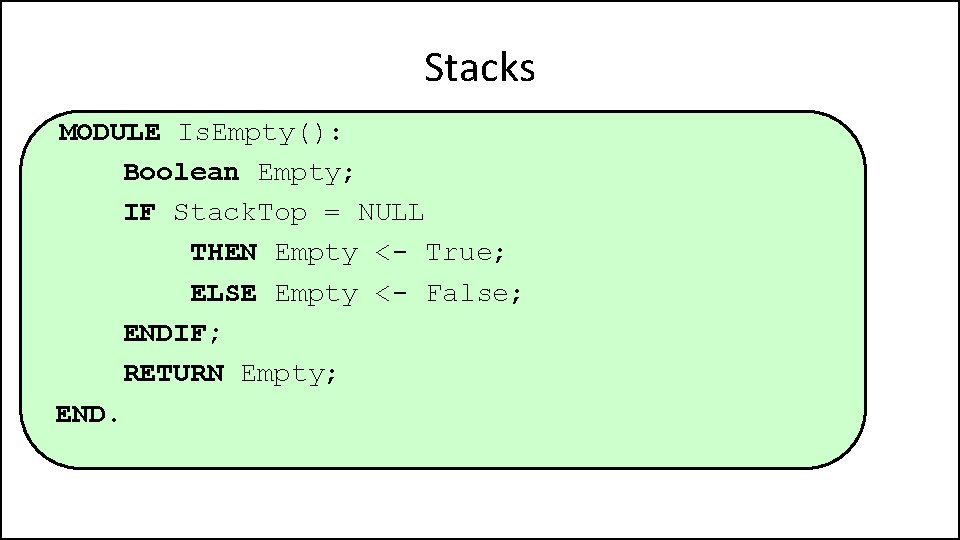
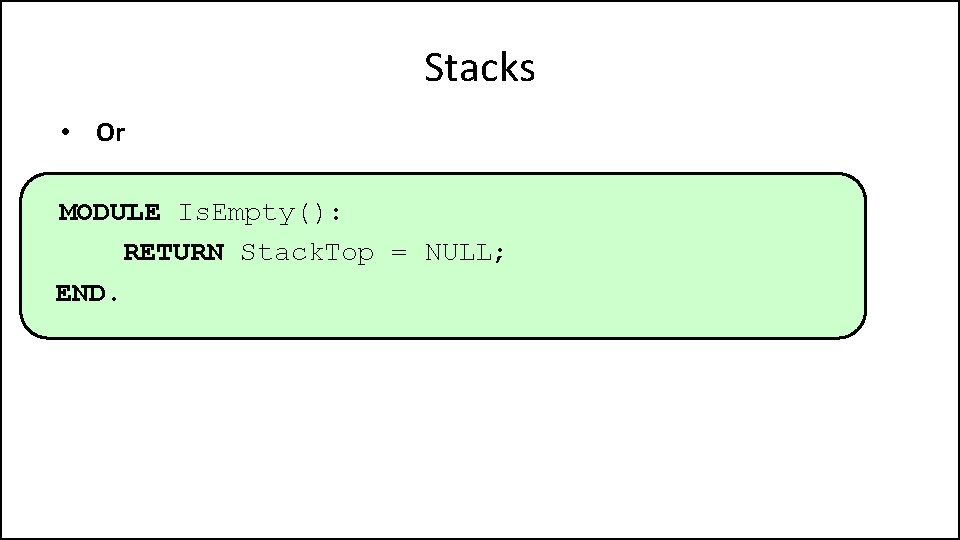
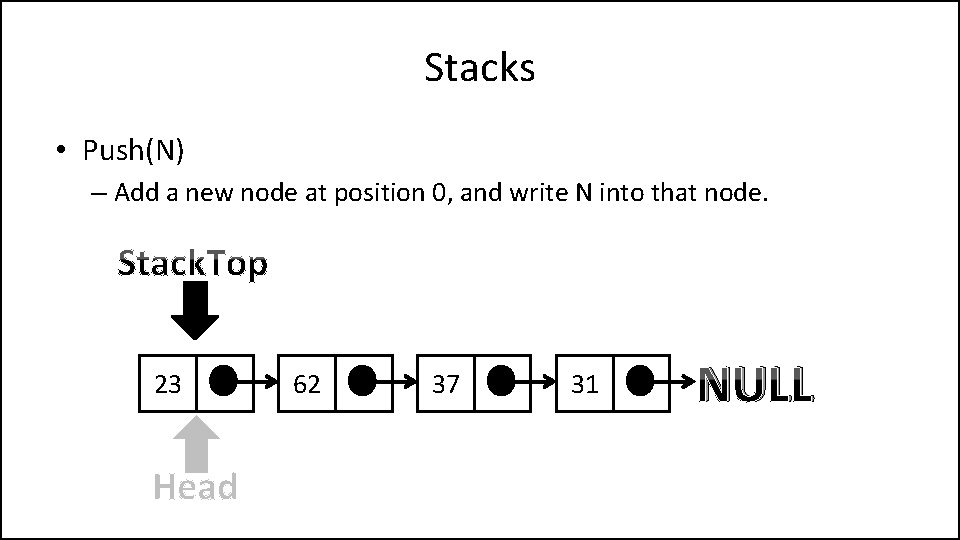
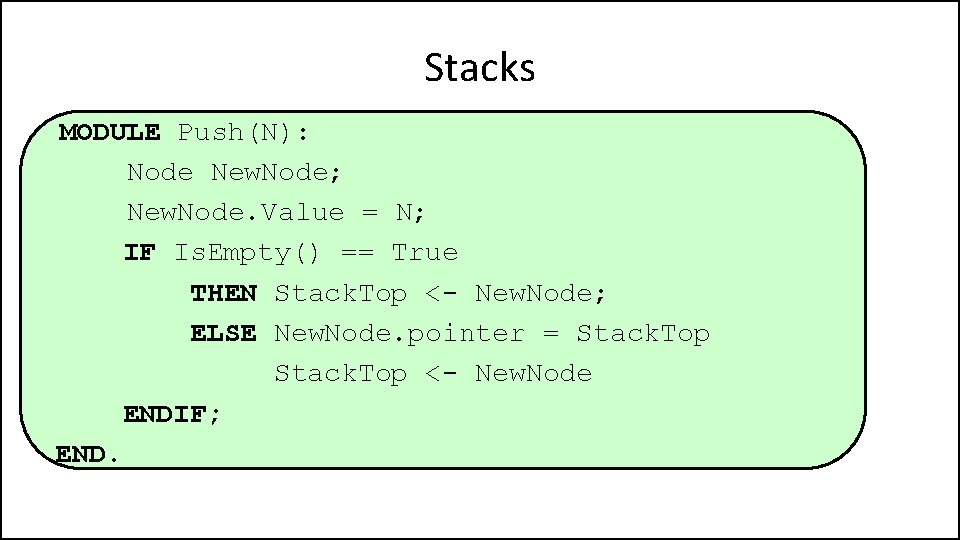
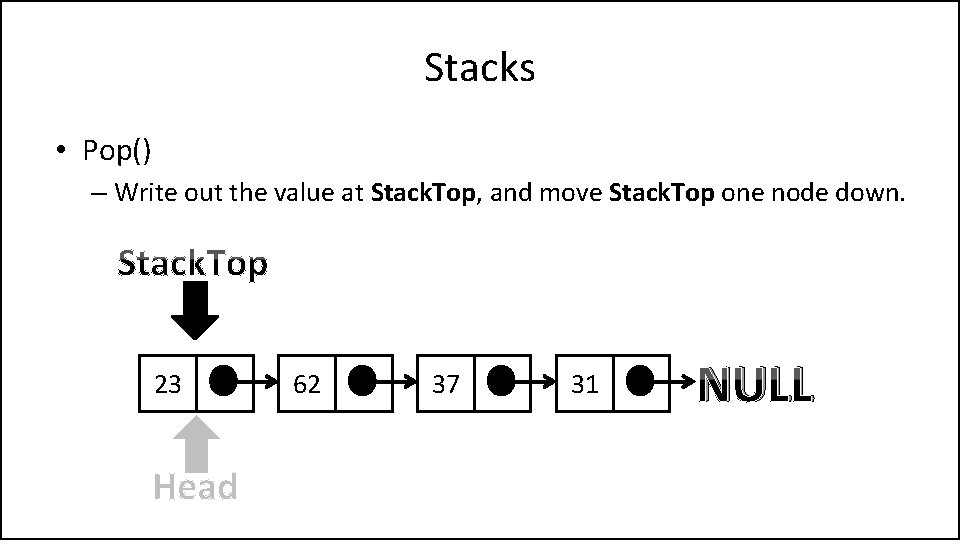
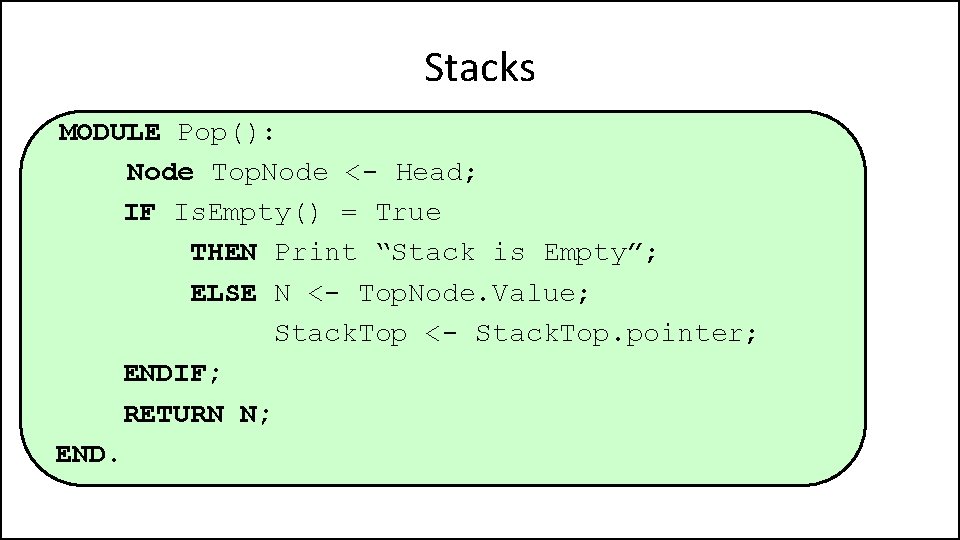
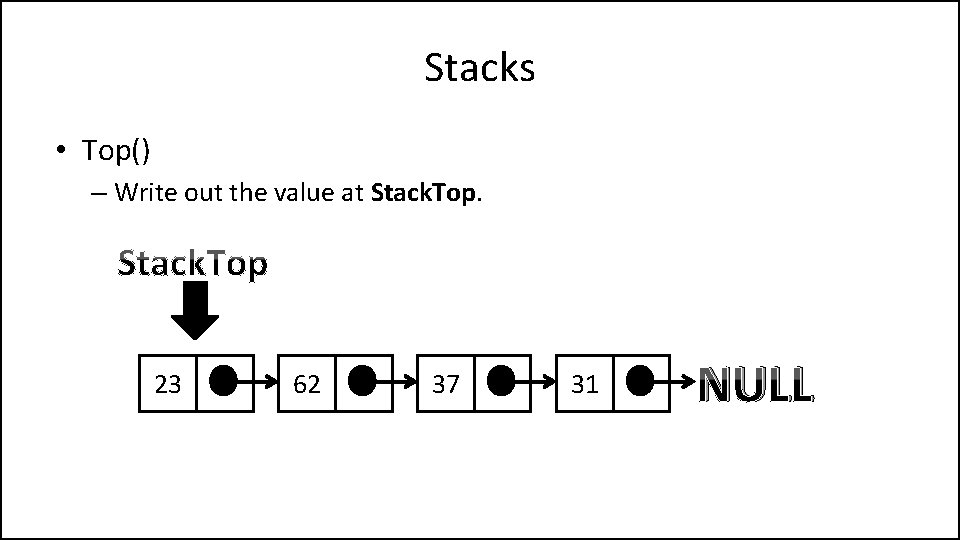
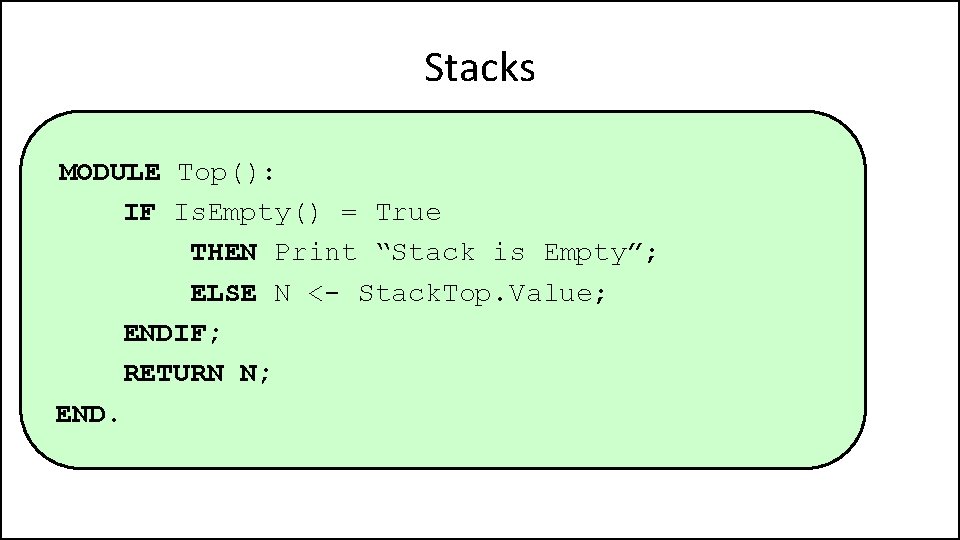
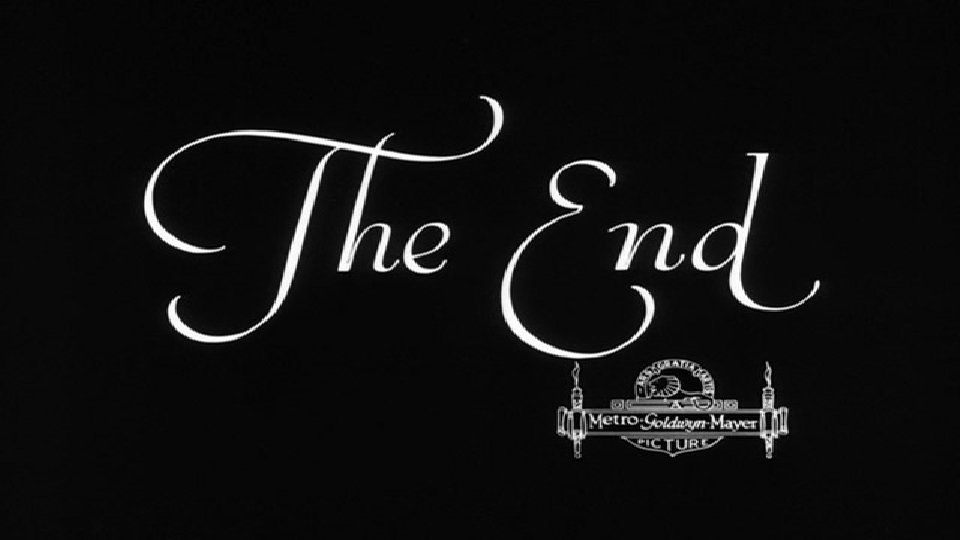
- Slides: 28
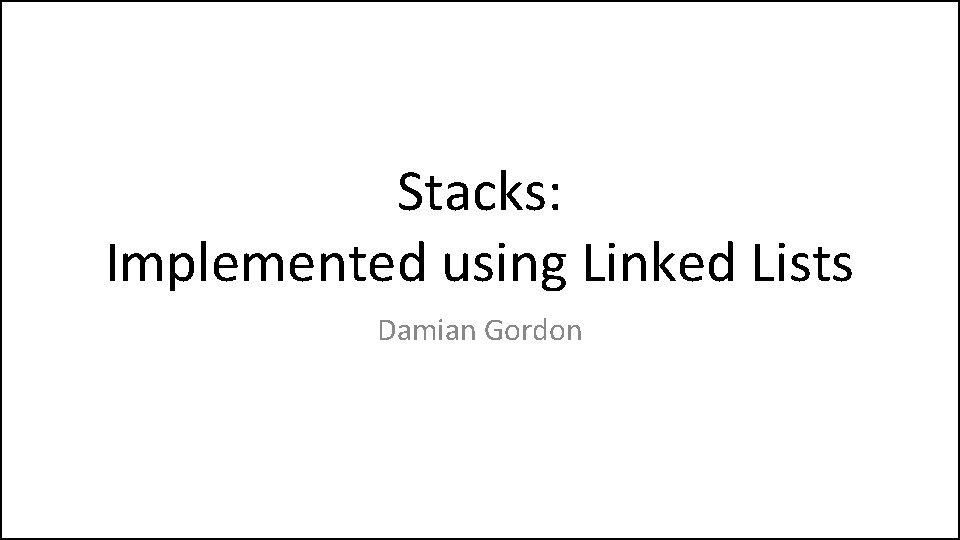
Stacks: Implemented using Linked Lists Damian Gordon
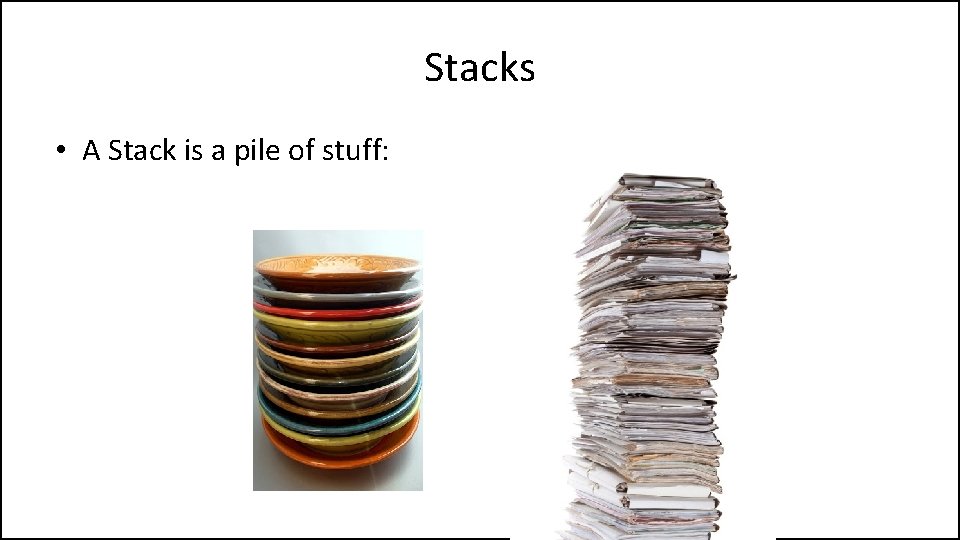
Stacks • A Stack is a pile of stuff:
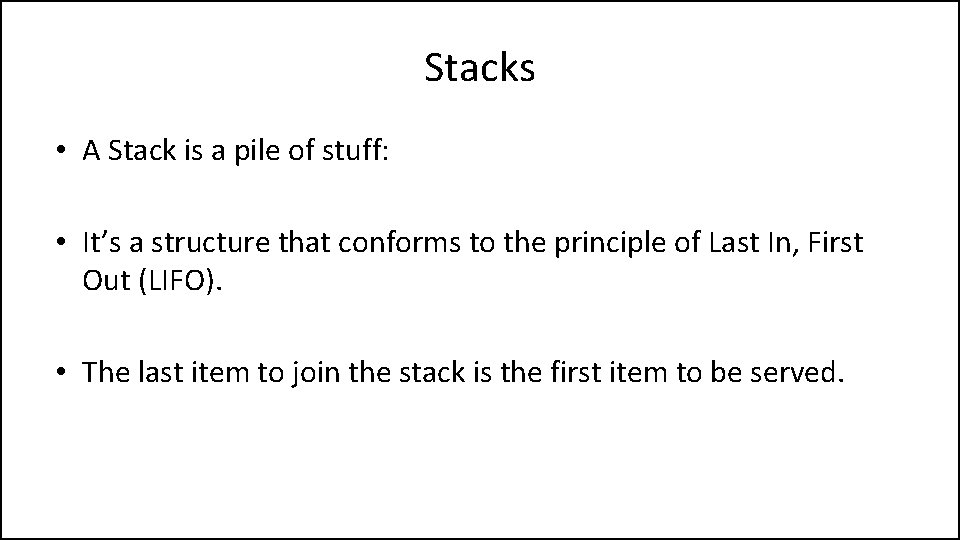
Stacks • A Stack is a pile of stuff: • It’s a structure that conforms to the principle of Last In, First Out (LIFO). • The last item to join the stack is the first item to be served.
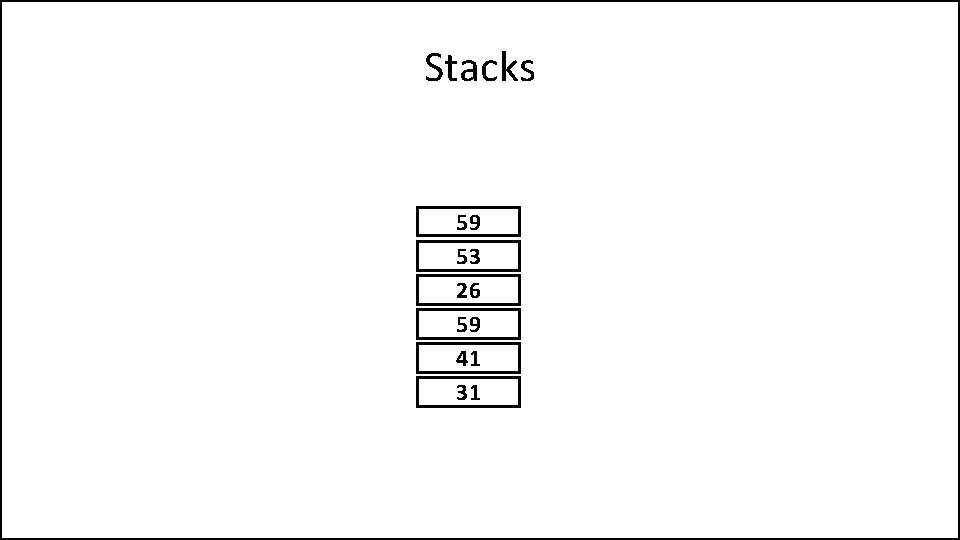
Stacks 59 53 26 59 41 31
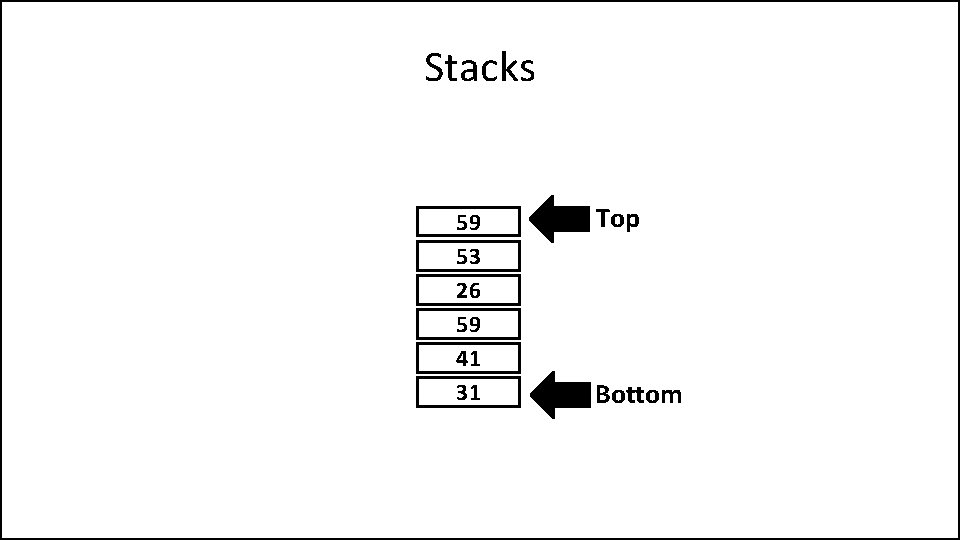
Stacks 59 53 26 59 41 31 Top Bottom
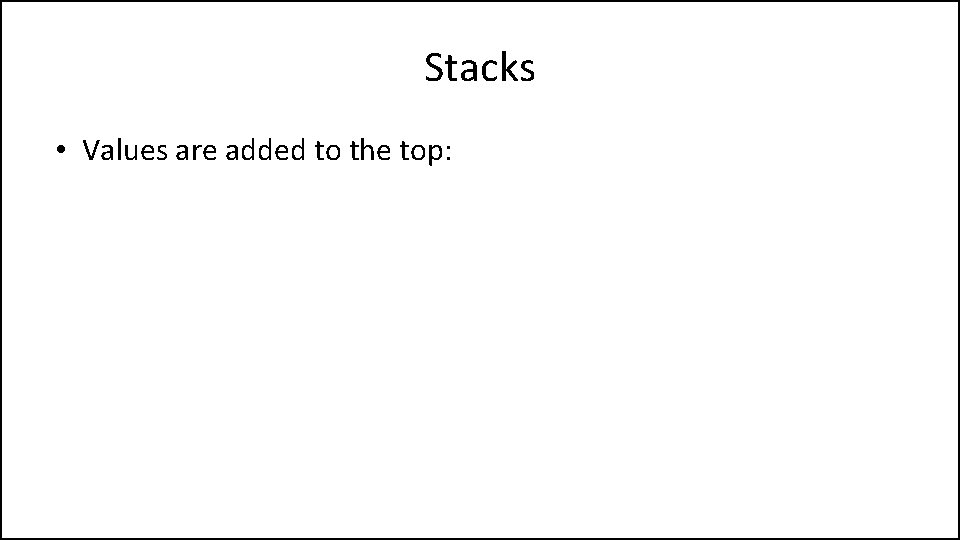
Stacks • Values are added to the top:
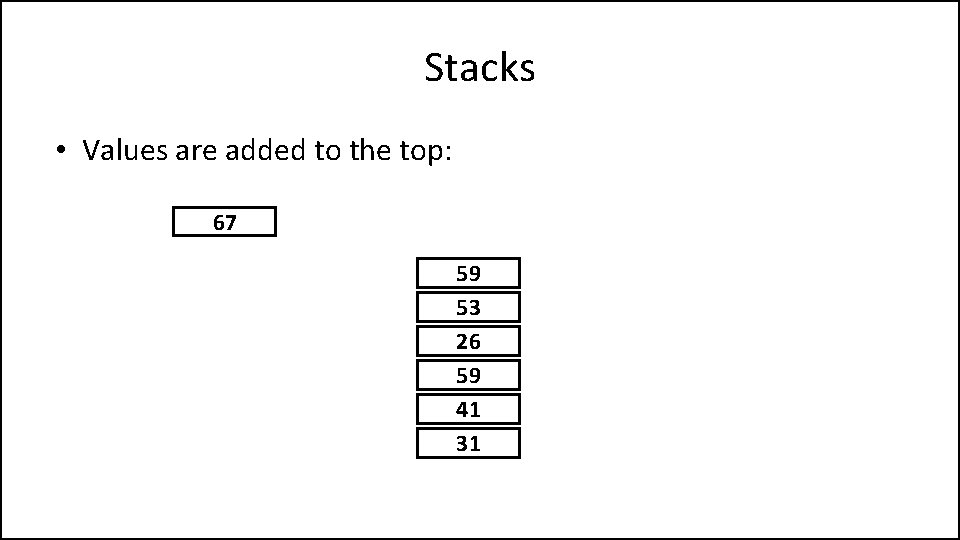
Stacks • Values are added to the top: 67 59 53 26 59 41 31
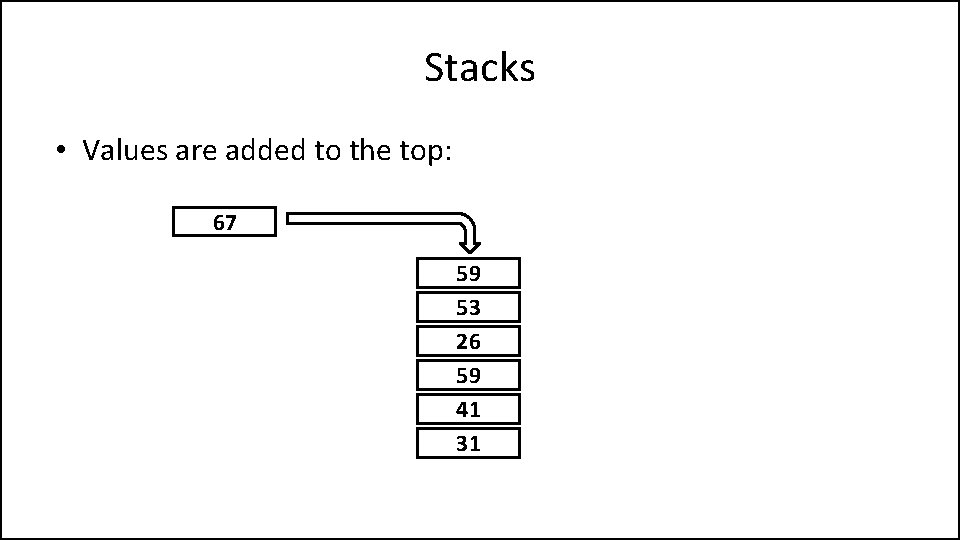
Stacks • Values are added to the top: 67 59 53 26 59 41 31
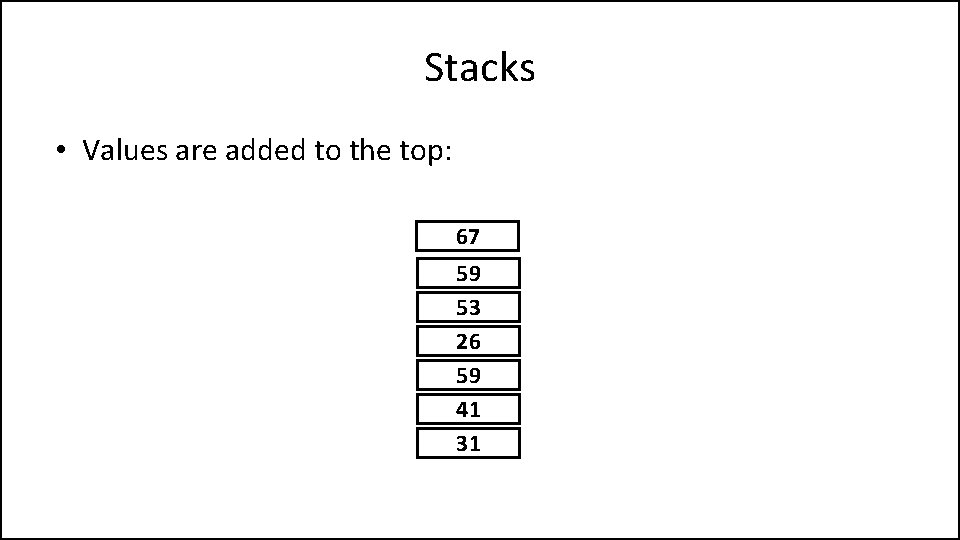
Stacks • Values are added to the top: 67 59 53 26 59 41 31
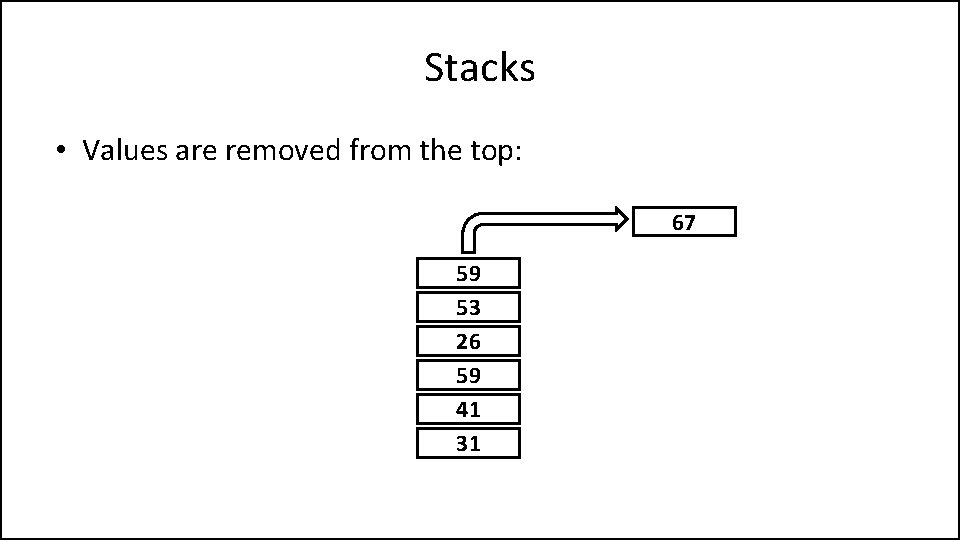
Stacks • Values are removed from the top: 67 59 53 26 59 41 31
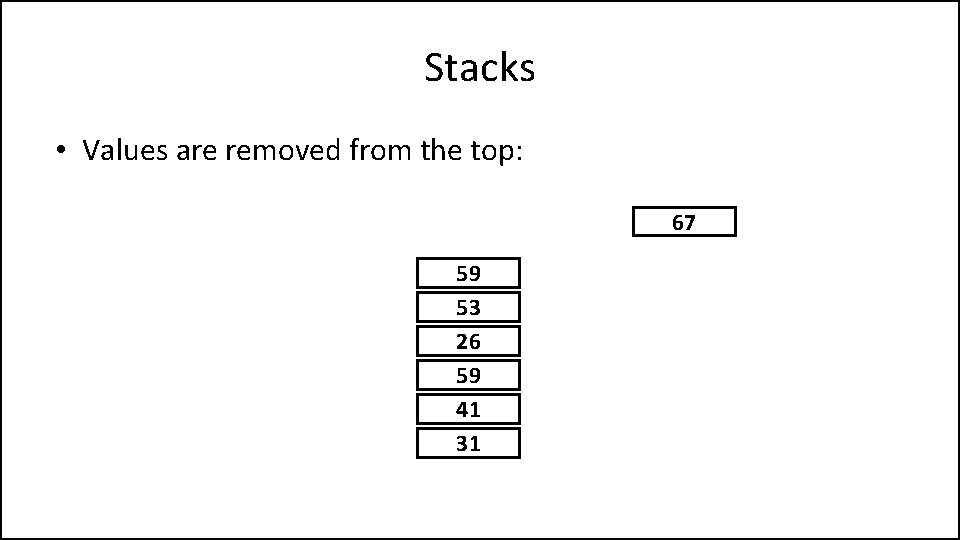
Stacks • Values are removed from the top: 67 59 53 26 59 41 31
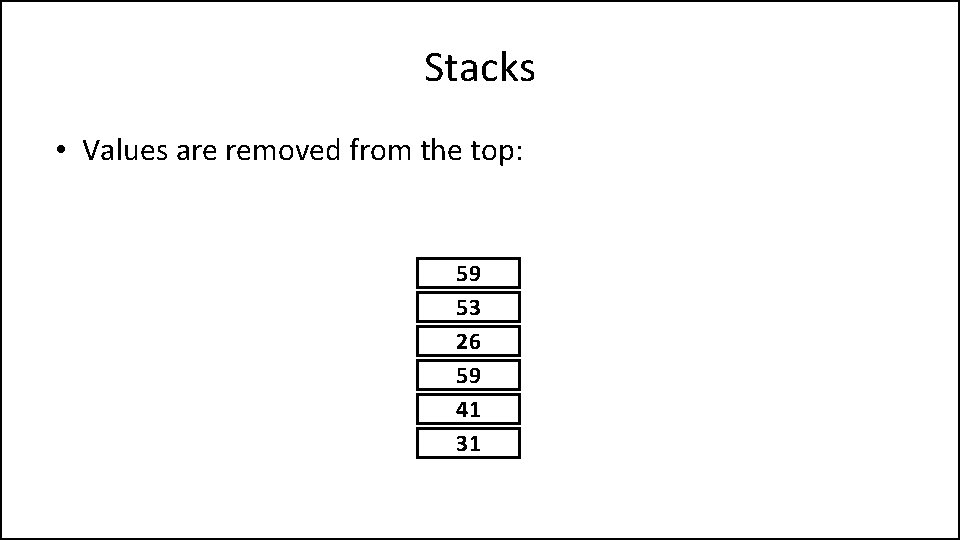
Stacks • Values are removed from the top: 59 53 26 59 41 31
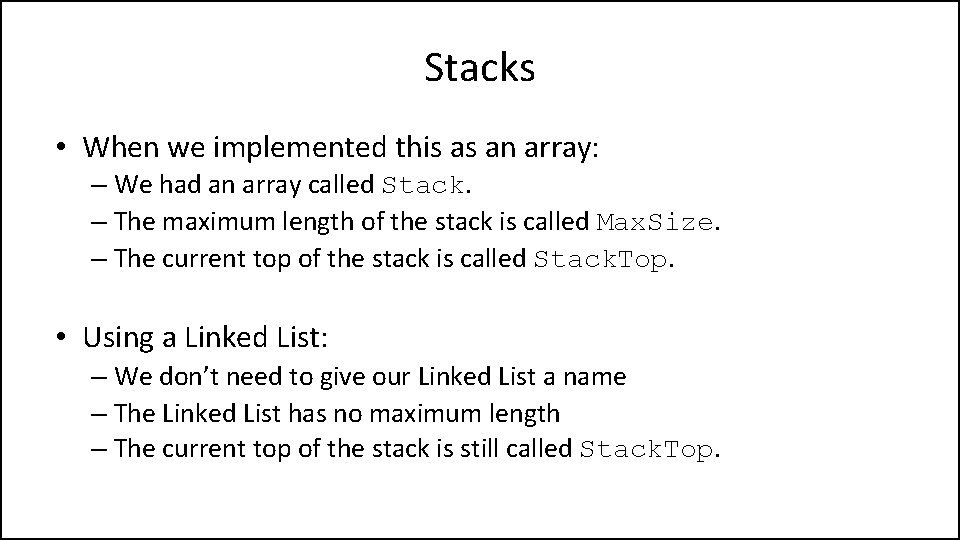
Stacks • When we implemented this as an array: – We had an array called Stack. – The maximum length of the stack is called Max. Size. – The current top of the stack is called Stack. Top. • Using a Linked List: – We don’t need to give our Linked List a name – The Linked List has no maximum length – The current top of the stack is still called Stack. Top.
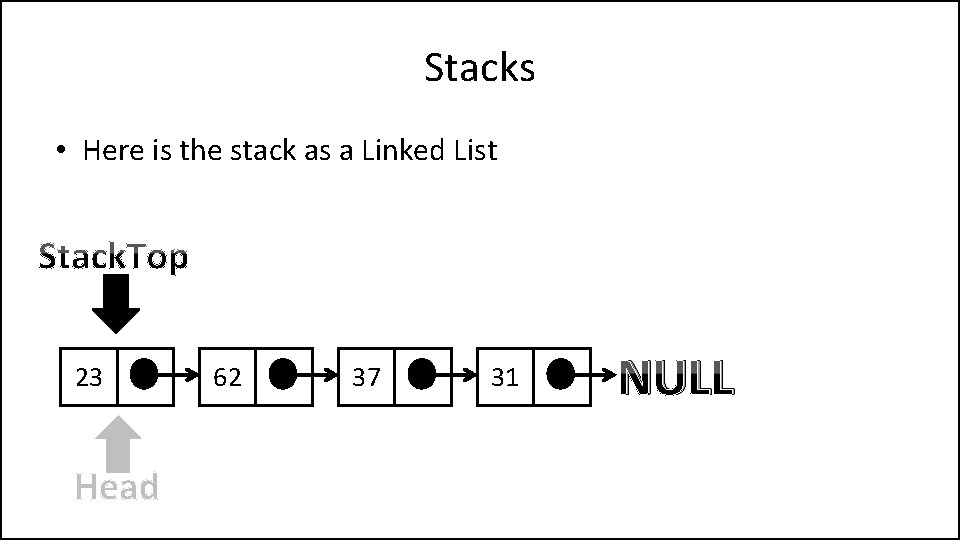
Stacks • Here is the stack as a Linked List 23 Head 62 37 31 NULL
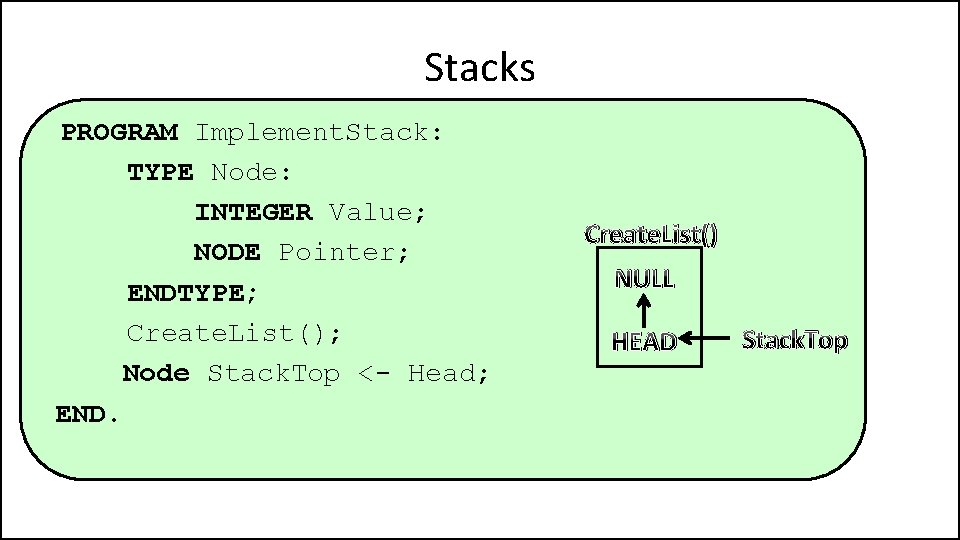
Stacks PROGRAM Implement. Stack: TYPE Node: INTEGER Value; NODE Pointer; ENDTYPE; Create. List(); Node Stack. Top <- Head; END. Create. List() NULL HEAD Stack. Top
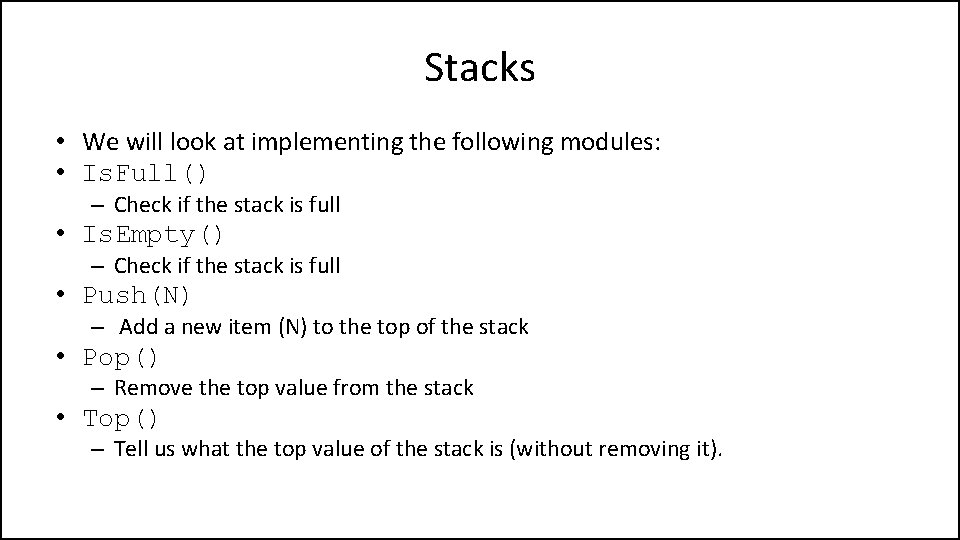
Stacks • We will look at implementing the following modules: • Is. Full() – Check if the stack is full • Is. Empty() – Check if the stack is full • Push(N) – Add a new item (N) to the top of the stack • Pop() – Remove the top value from the stack • Top() – Tell us what the top value of the stack is (without removing it).
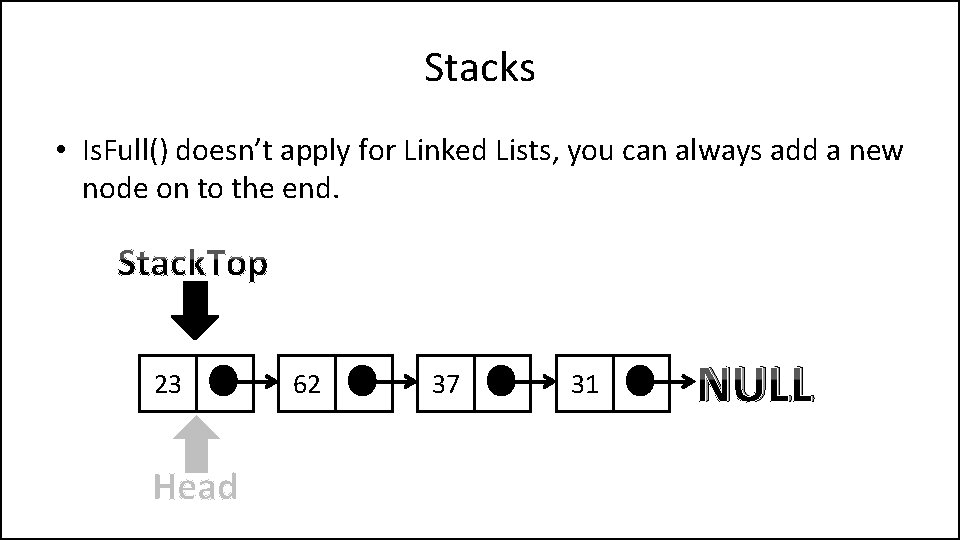
Stacks • Is. Full() doesn’t apply for Linked Lists, you can always add a new node on to the end. 23 Head 62 37 31 NULL
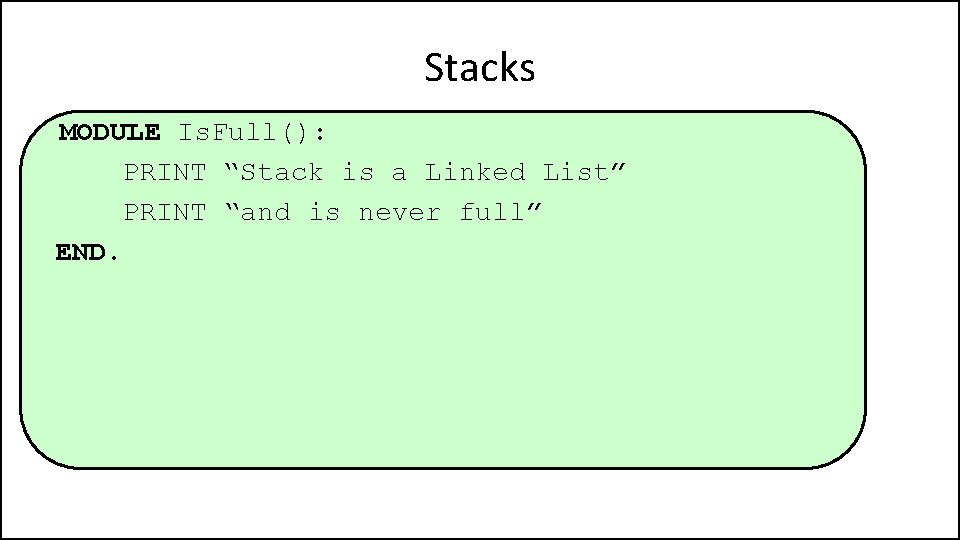
Stacks MODULE Is. Full(): PRINT “Stack is a Linked List” PRINT “and is never full” END.
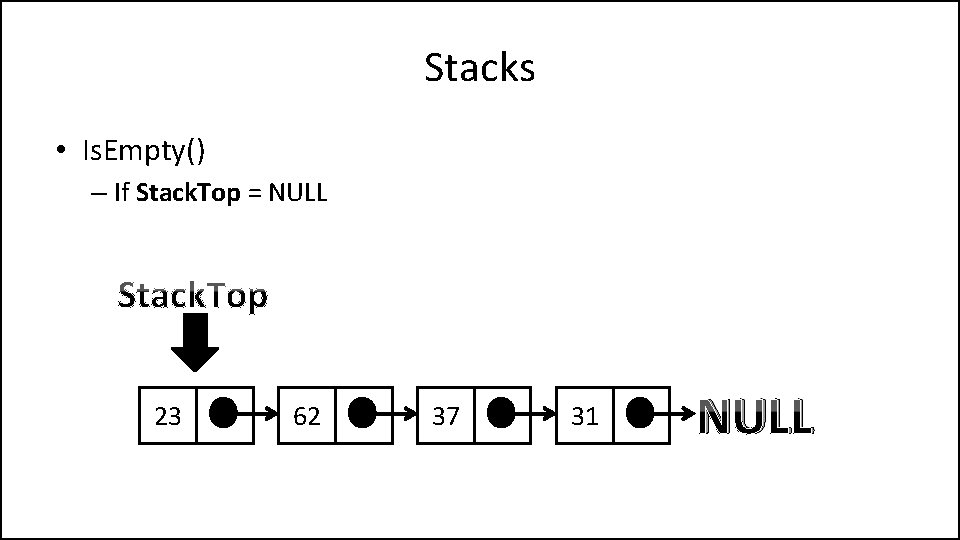
Stacks • Is. Empty() – If Stack. Top = NULL 23 62 37 31 NULL
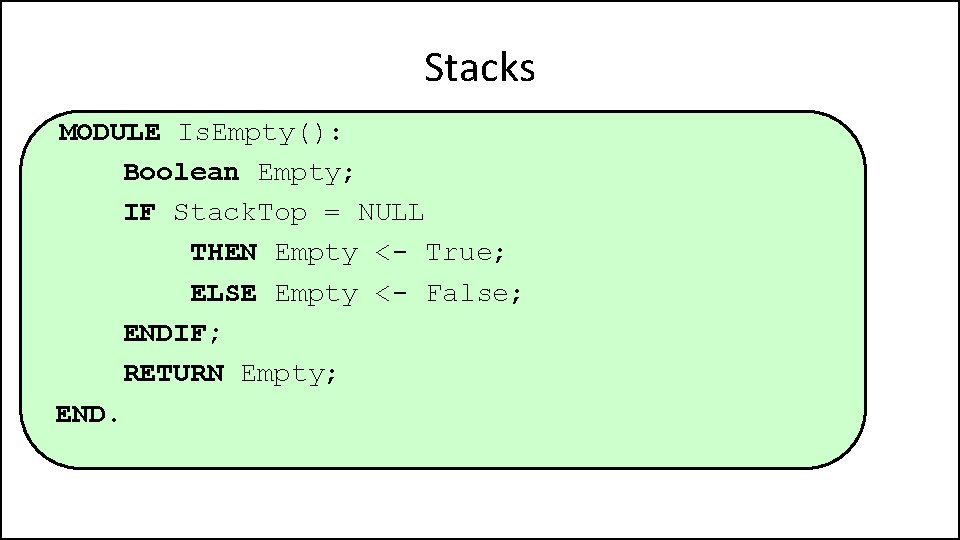
Stacks MODULE Is. Empty(): Boolean Empty; IF Stack. Top = NULL THEN Empty <- True; ELSE Empty <- False; ENDIF; RETURN Empty; END.
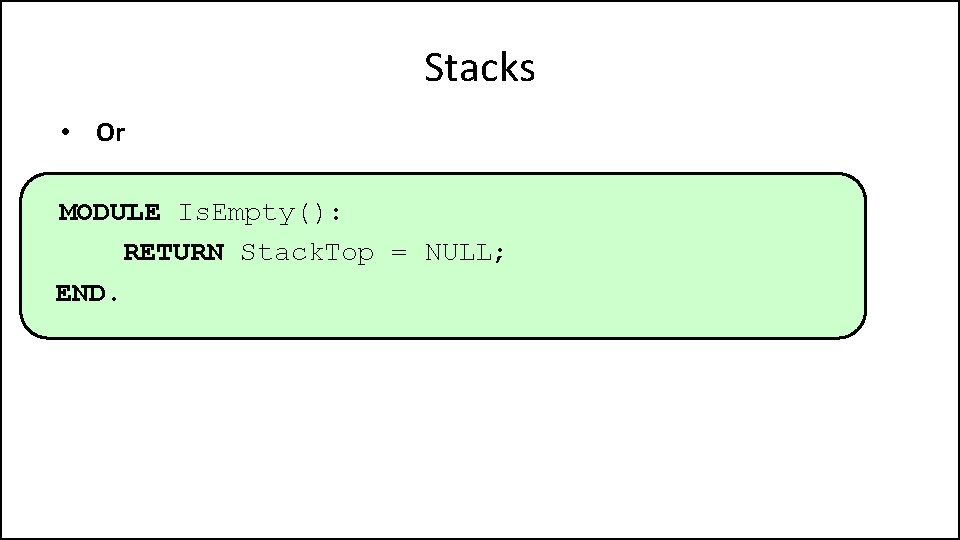
Stacks • Or MODULE Is. Empty(): RETURN Stack. Top = NULL; END.
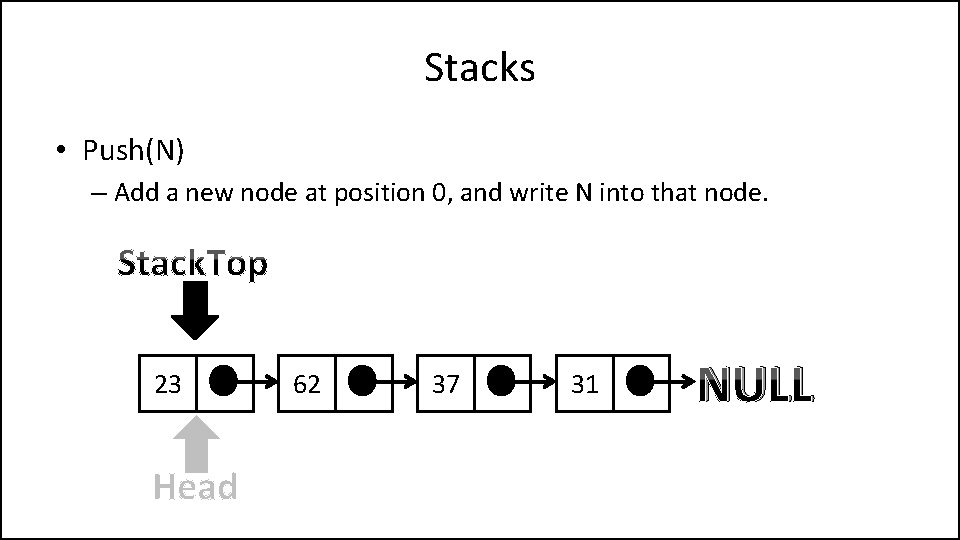
Stacks • Push(N) – Add a new node at position 0, and write N into that node. 23 Head 62 37 31 NULL
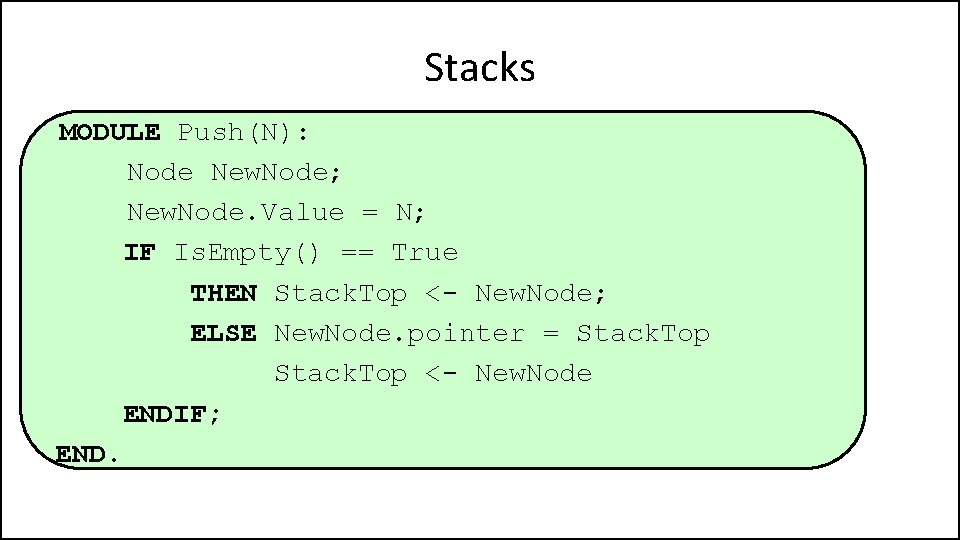
Stacks MODULE Push(N): Node New. Node; New. Node. Value = N; IF Is. Empty() == True THEN Stack. Top <- New. Node; ELSE New. Node. pointer = Stack. Top <- New. Node ENDIF; END.
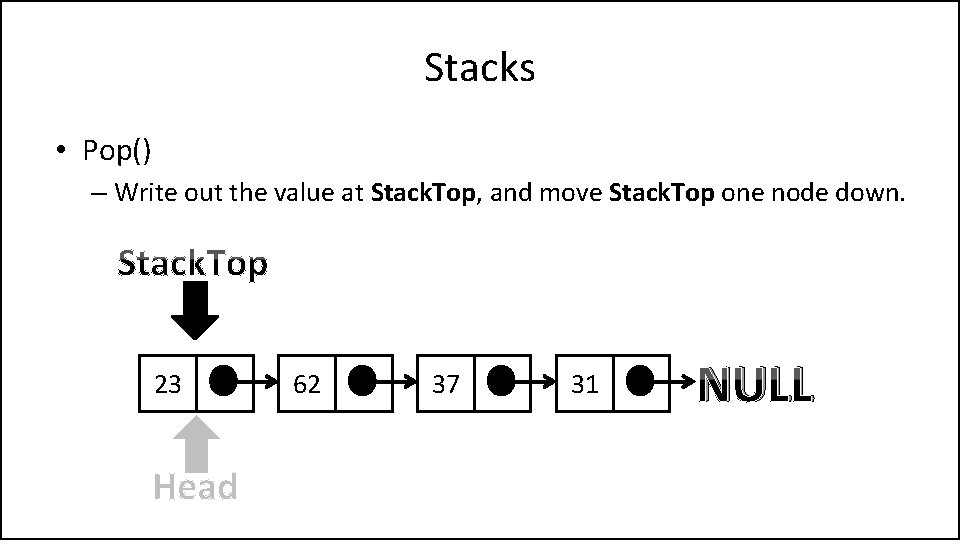
Stacks • Pop() – Write out the value at Stack. Top, and move Stack. Top one node down. 23 Head 62 37 31 NULL
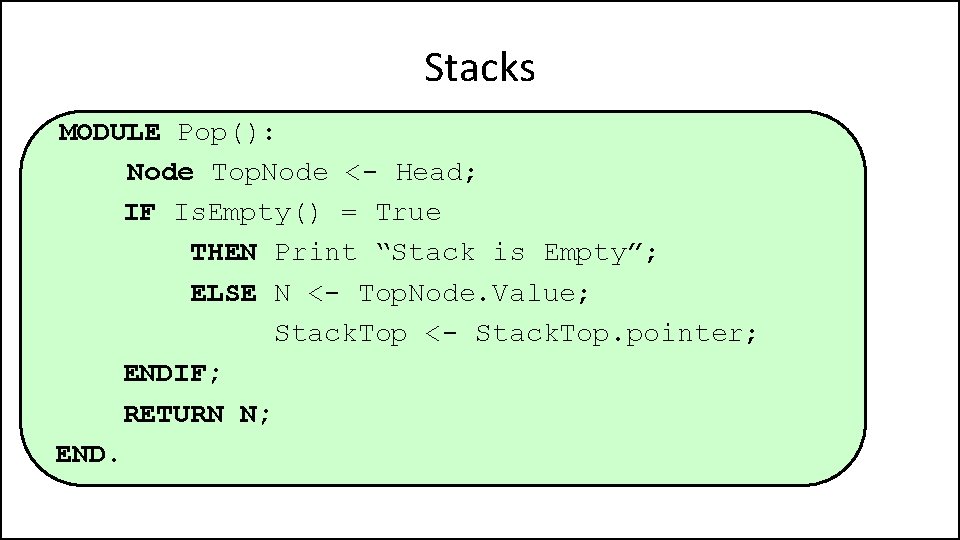
Stacks MODULE Pop(): Node Top. Node <- Head; IF Is. Empty() = True THEN Print “Stack is Empty”; ELSE N <- Top. Node. Value; Stack. Top <- Stack. Top. pointer; ENDIF; RETURN N; END.
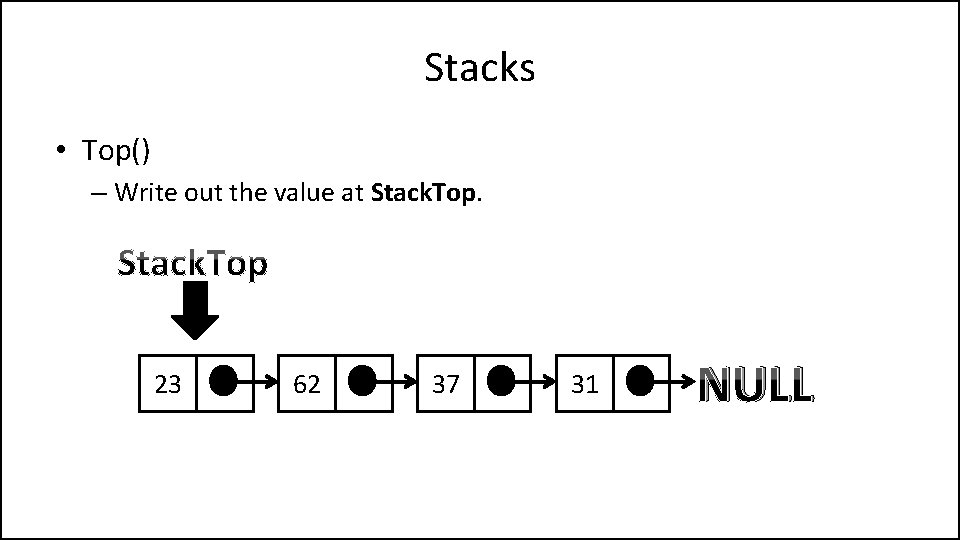
Stacks • Top() – Write out the value at Stack. Top. 23 62 37 31 NULL
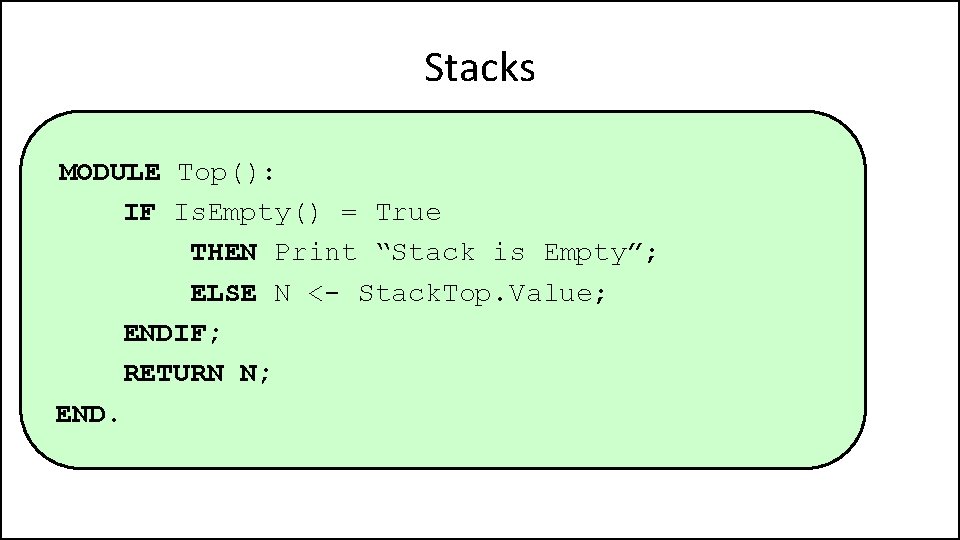
Stacks MODULE Top(): IF Is. Empty() = True THEN Print “Stack is Empty”; ELSE N <- Stack. Top. Value; ENDIF; RETURN N; END.
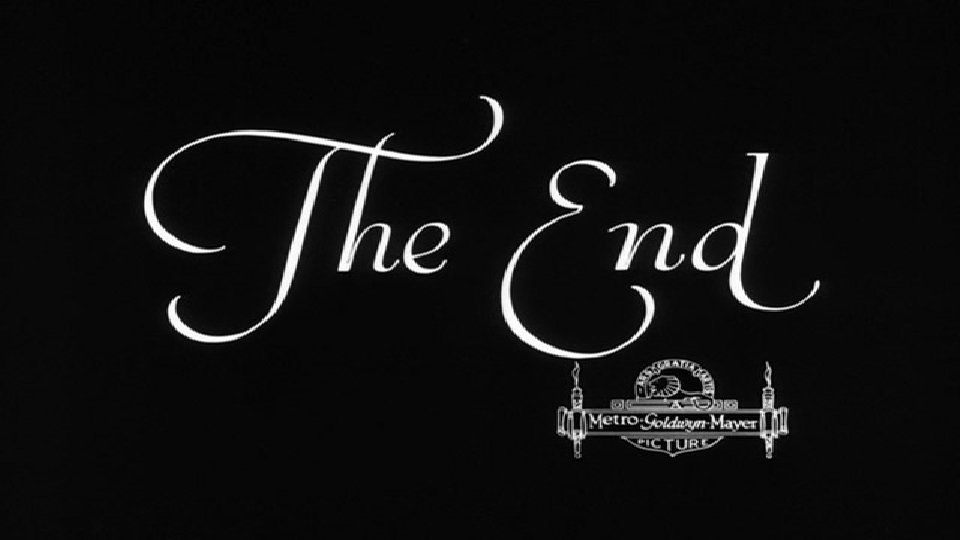
etc.
Damian gordon
Index.of.secret
Damian gordon
Coe 202
Perbedaan single linked list dan double linked list
Advantage of linked list
Introduction to linked list
Topological sort can be implemented by?
Bombay nursing home registration
Bohlen-serrano agreement tagalog
Topological sort can be implemented by?
Stackguard implemented as a gccpatch
Jiyo implemented a strategy
Windowok inc implemented a new pay policy
Origins of vc and datagram networks
Threads cannot be implemented as a library
Who implemented glasnost and perestroika
Threads cannot be implemented as a library
A switch in a datagram network uses
Virtual memory is commonly implemented by
Data structures using java
Stacks internet
6 stacks
Coastal landforms diagram
Exercises on stacks and queues
Caves arches stacks and stumps diagram
Java stacks and queues
What are stacks
Speed stacks spreads nationally in 1998.