Intro to Programming Algorithm Design Input Validation Assg
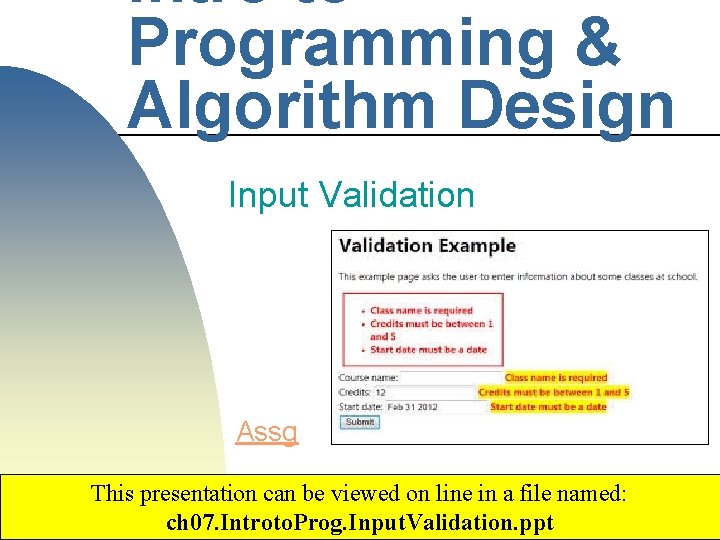
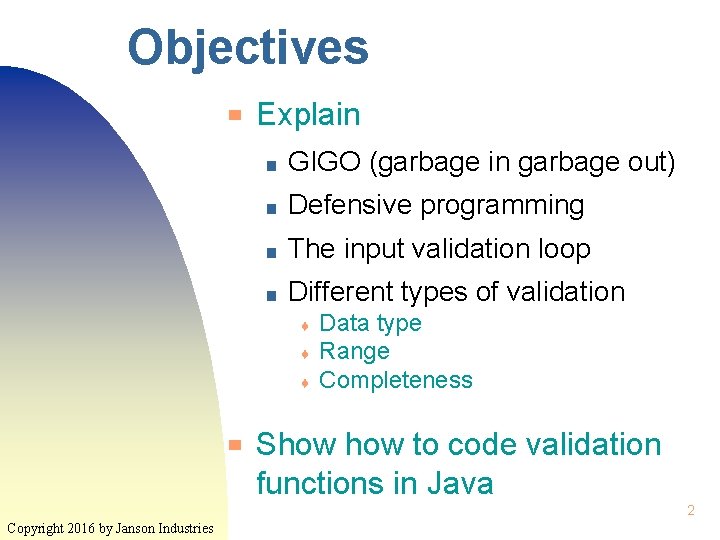
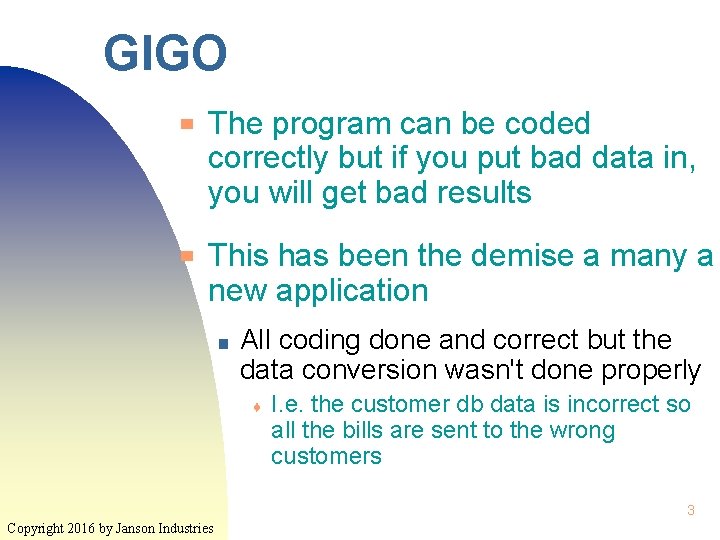
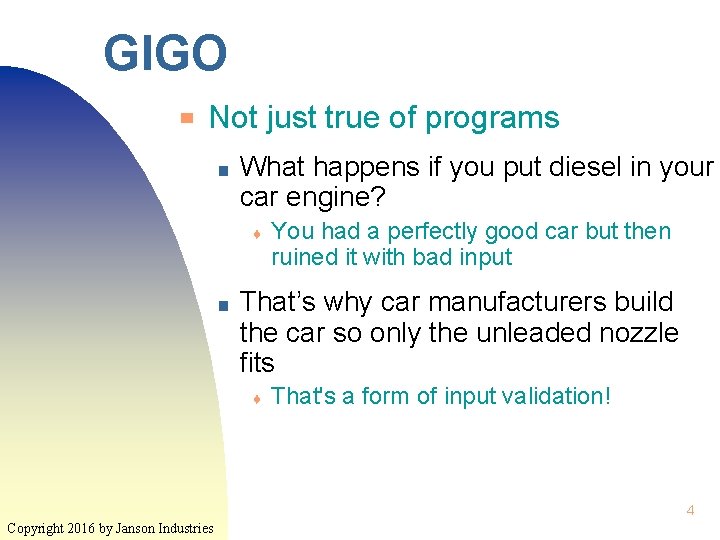
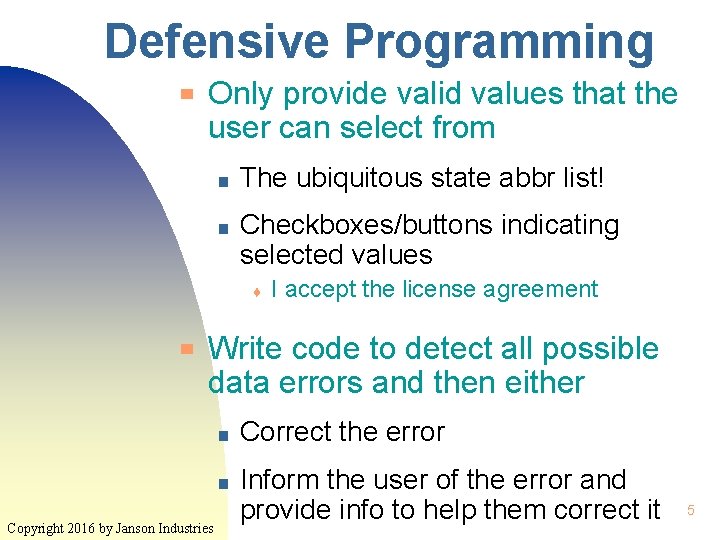
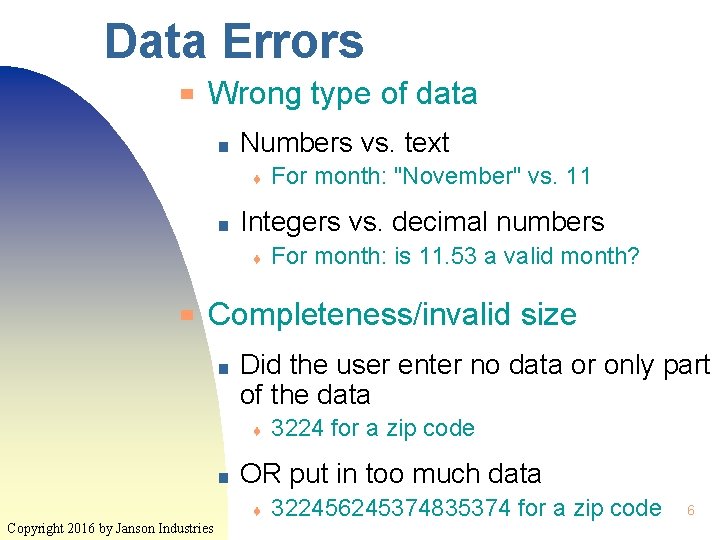
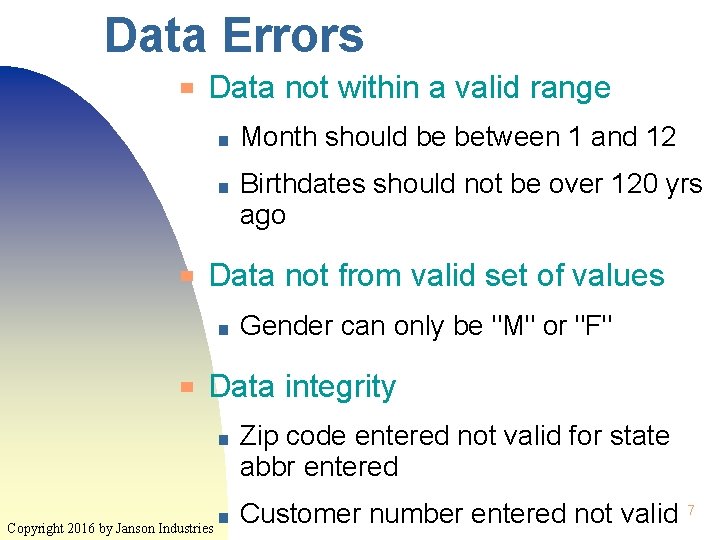
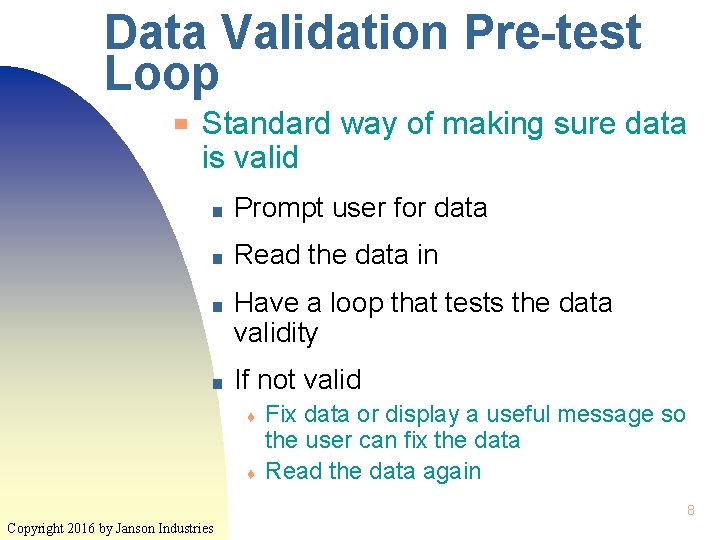
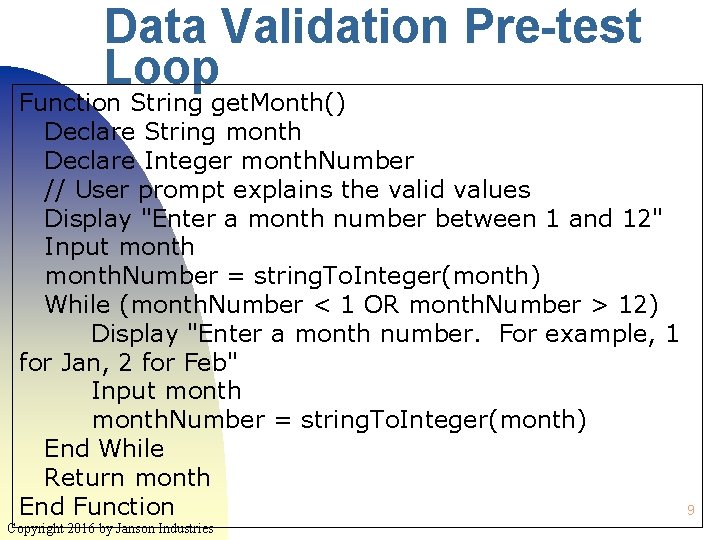
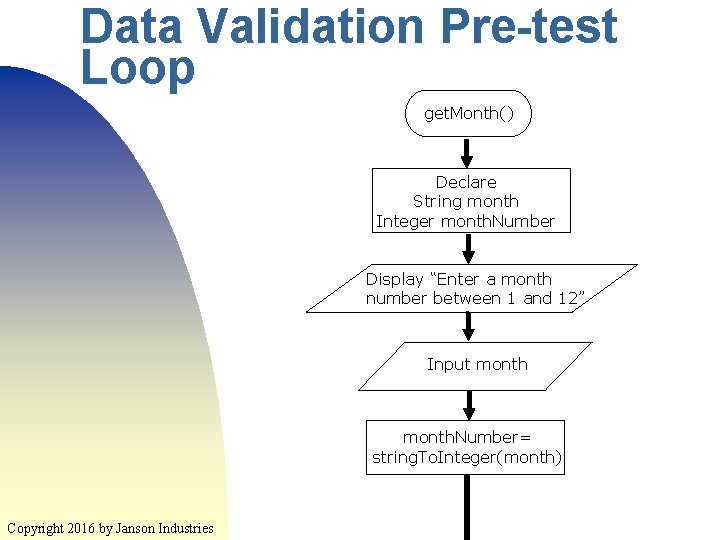
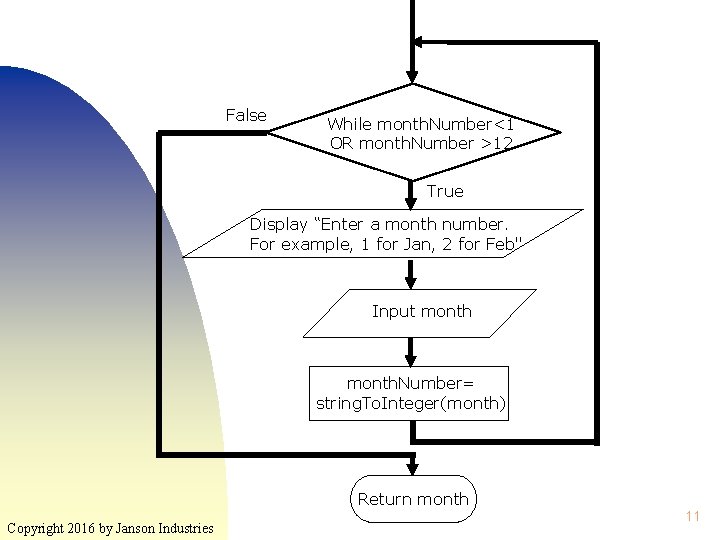
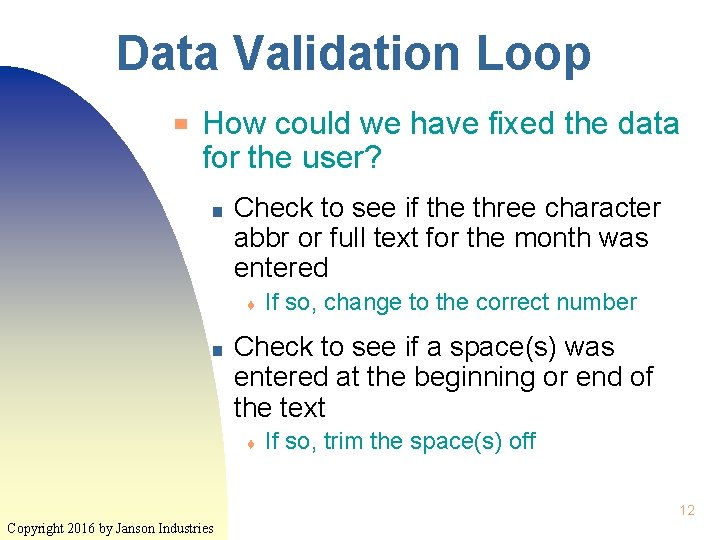
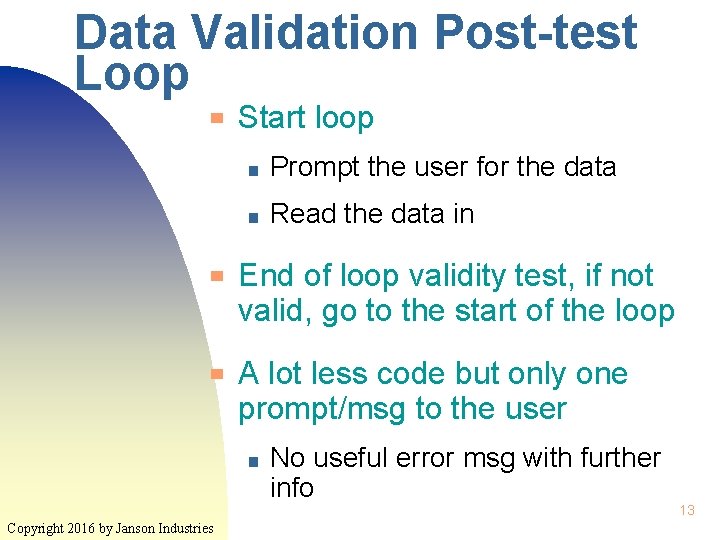
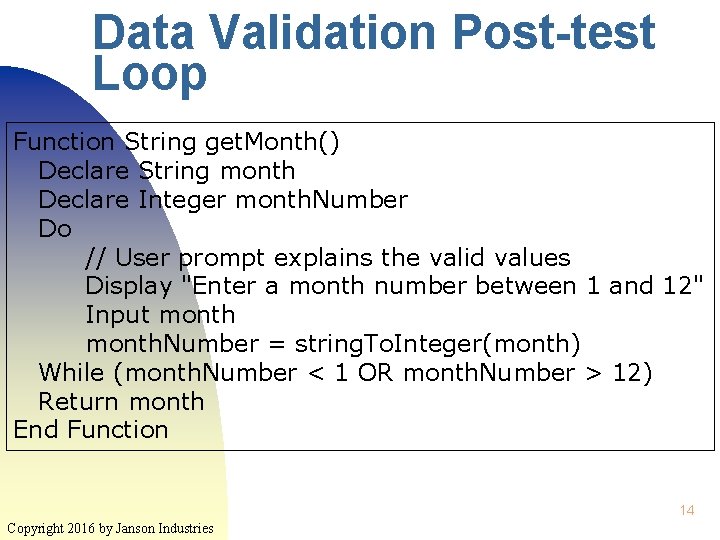
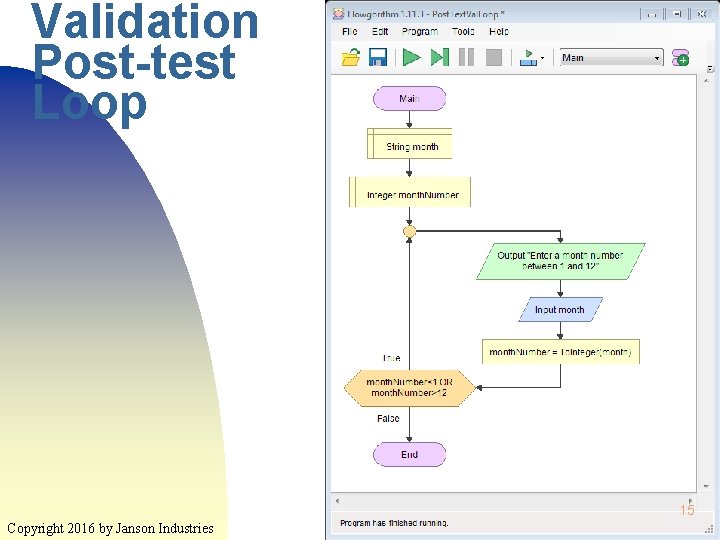
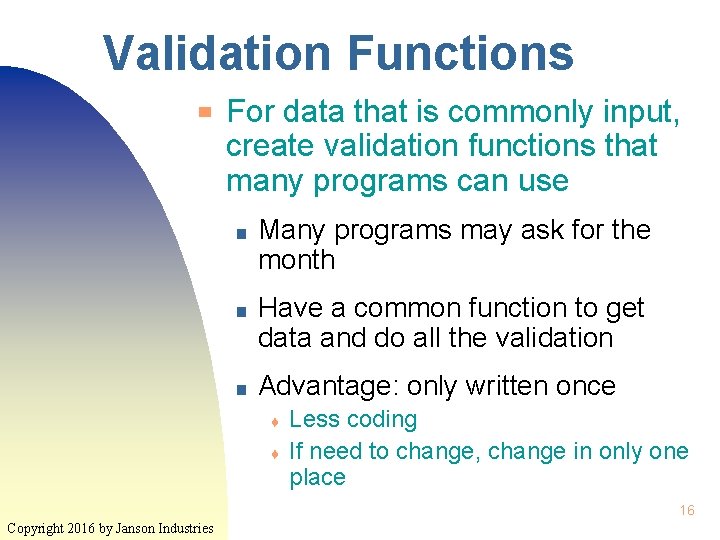
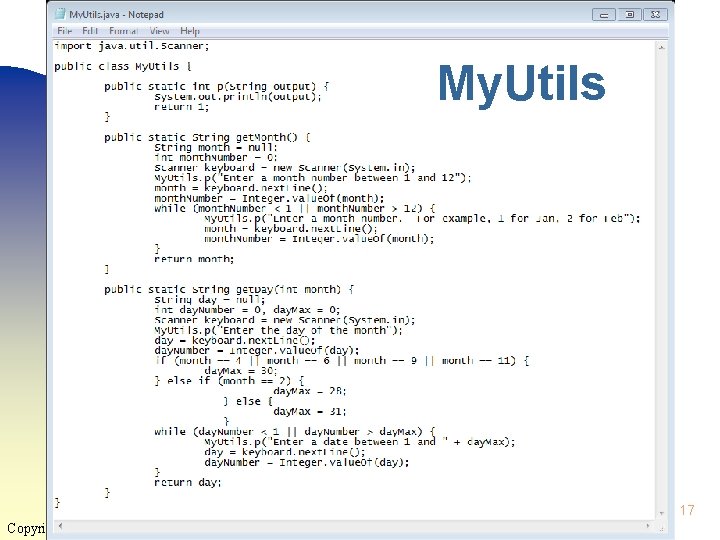
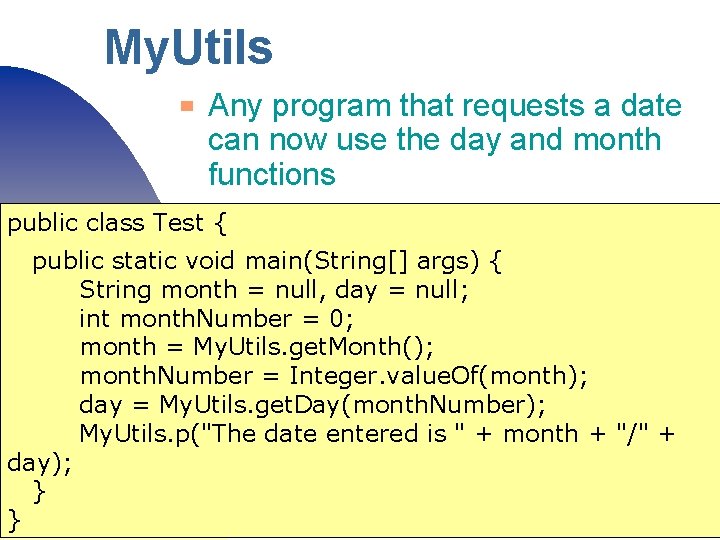
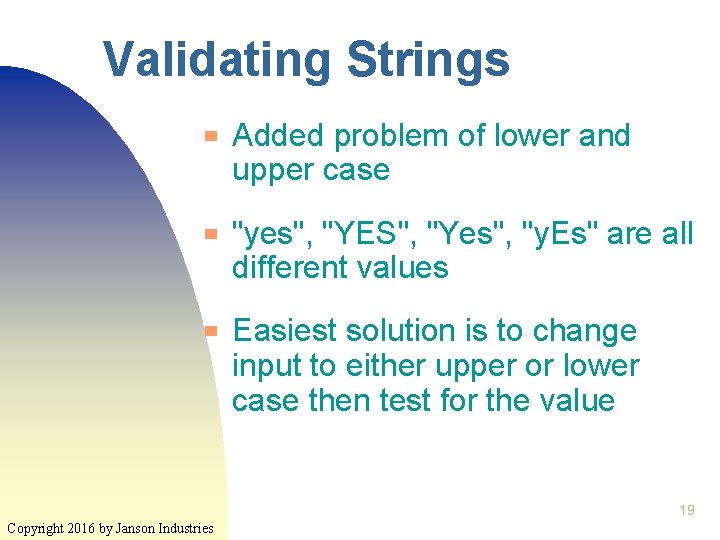
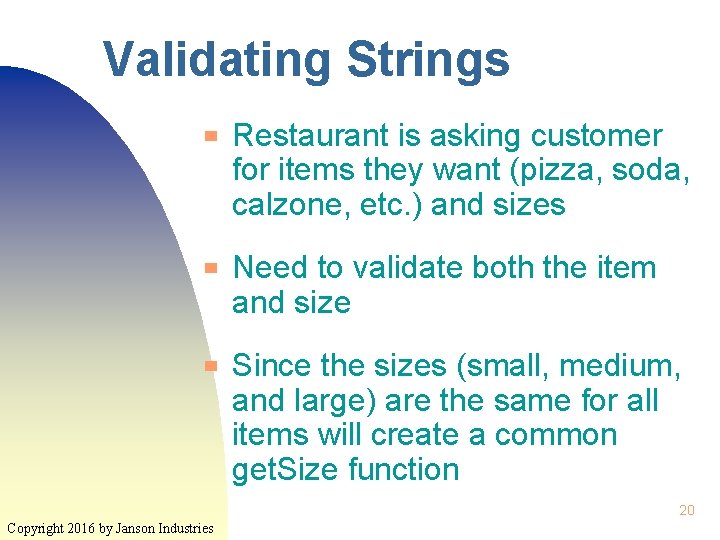
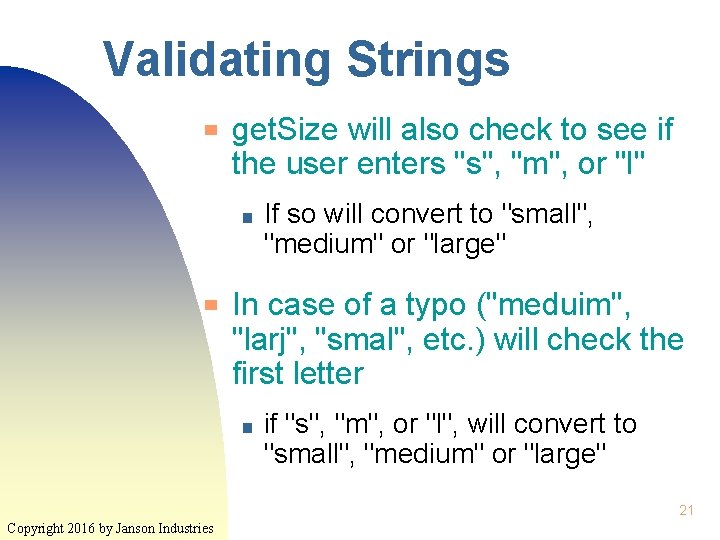
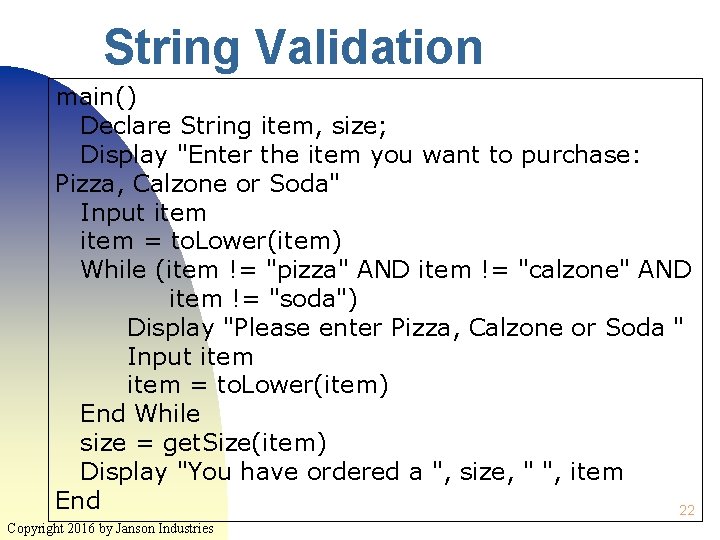
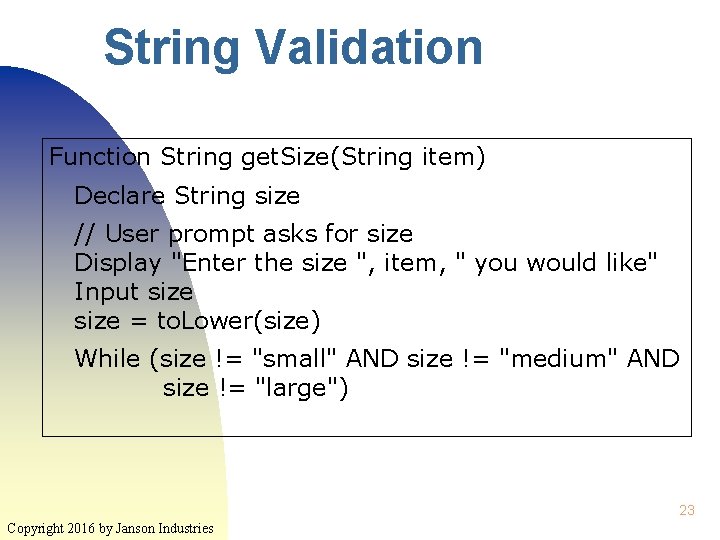
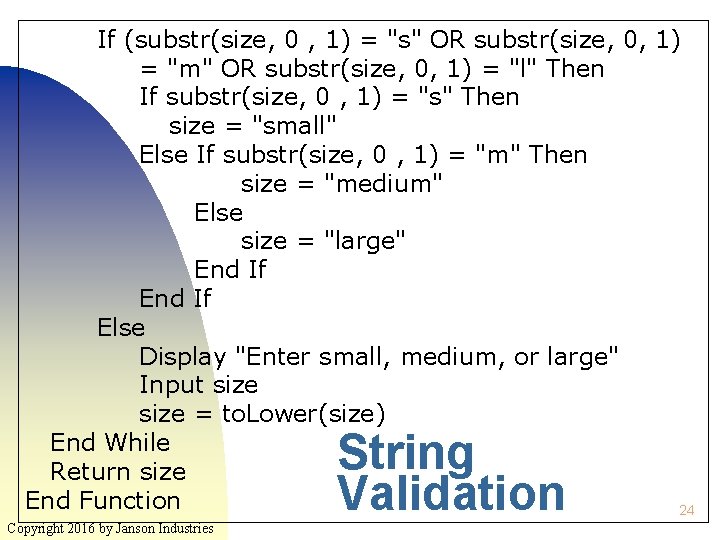
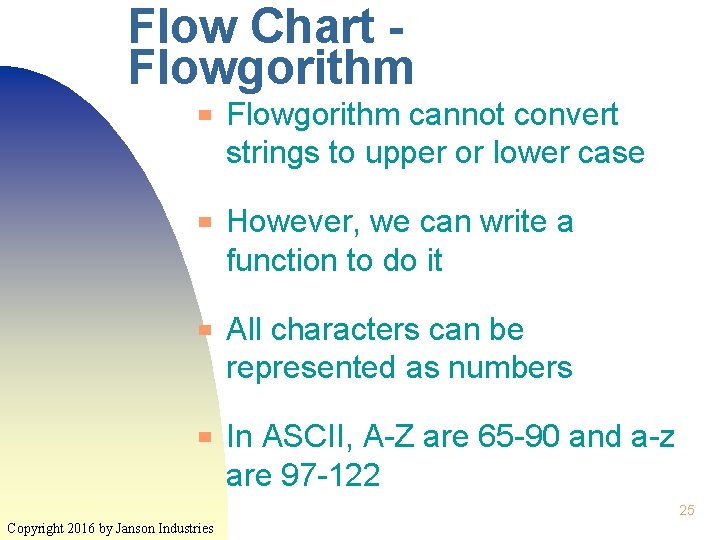
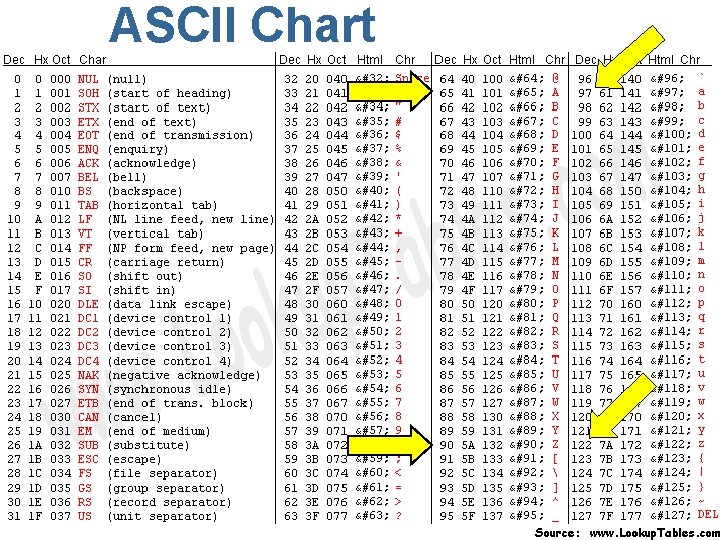
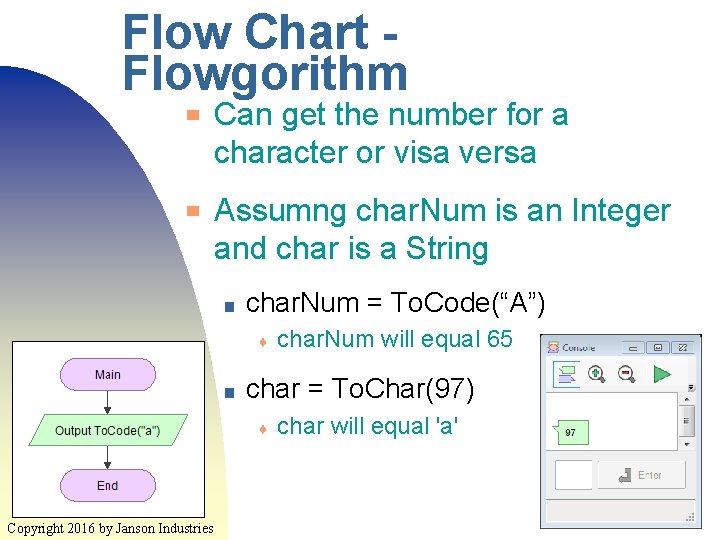
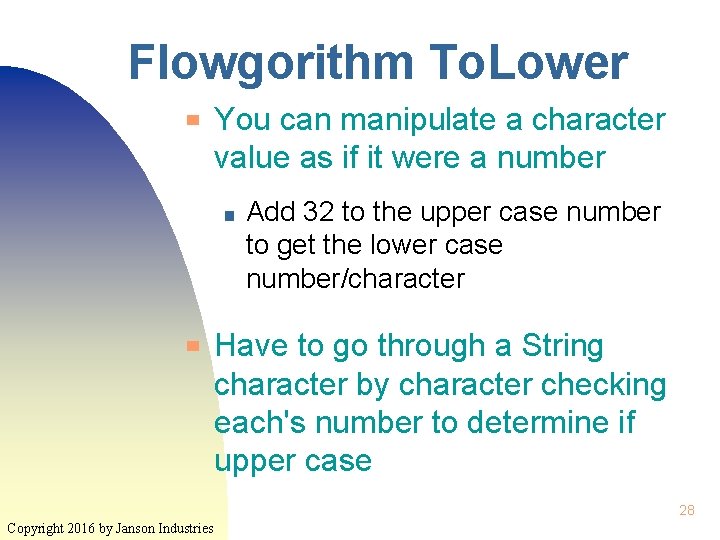
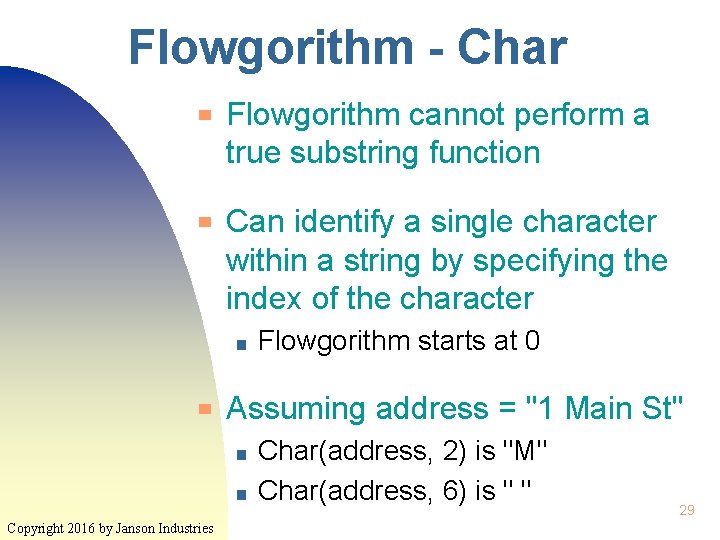
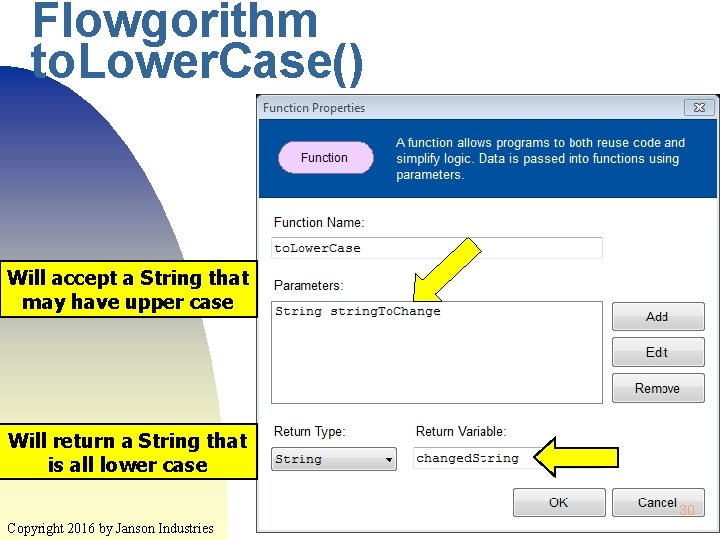
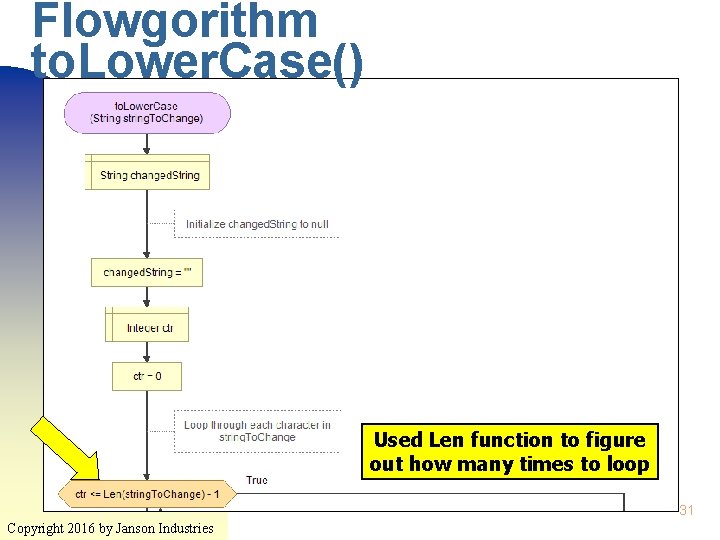
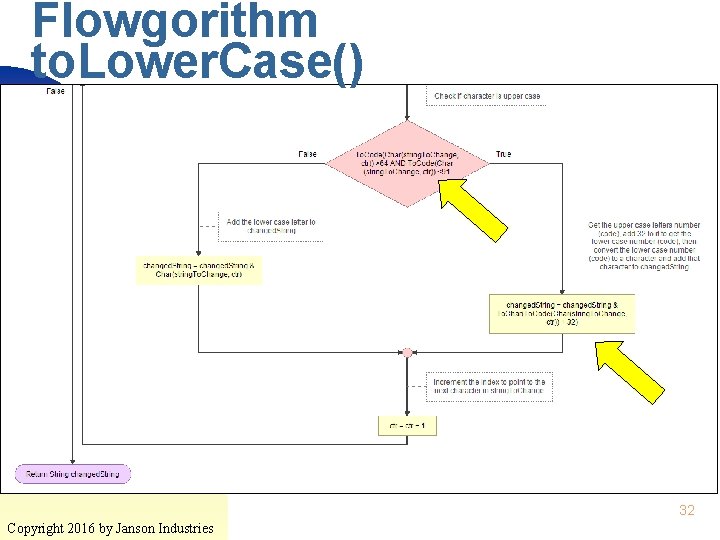
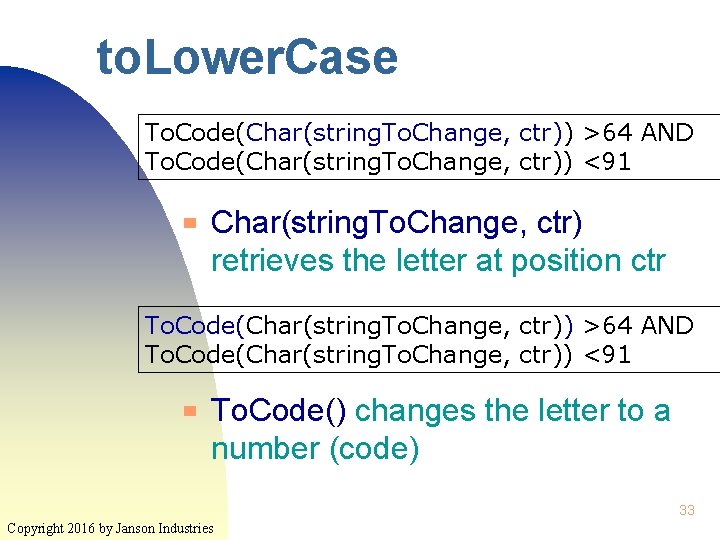
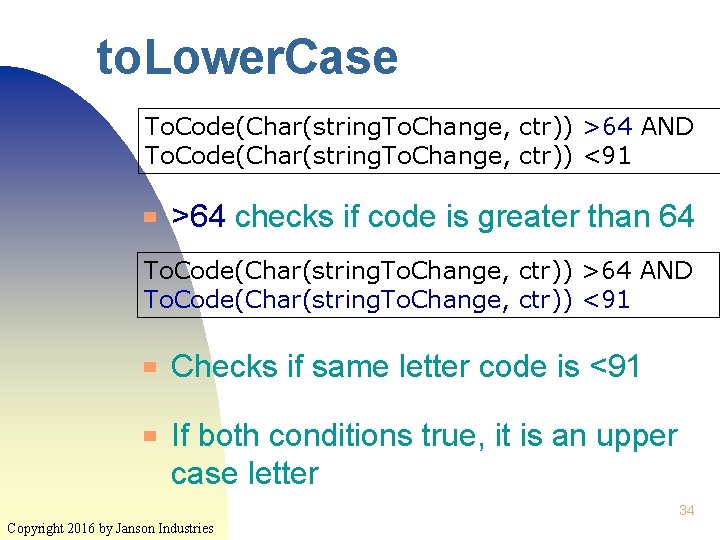
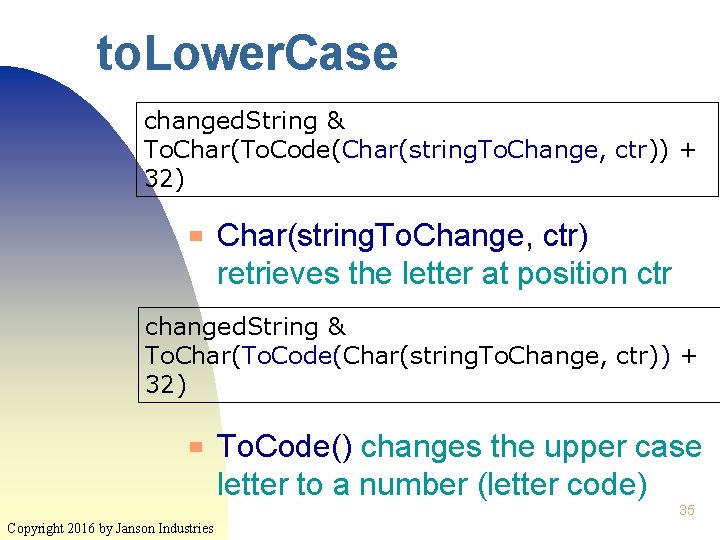
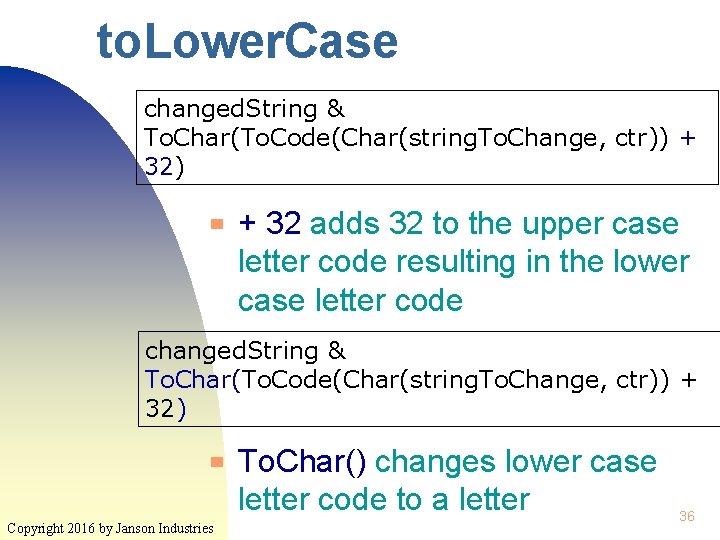
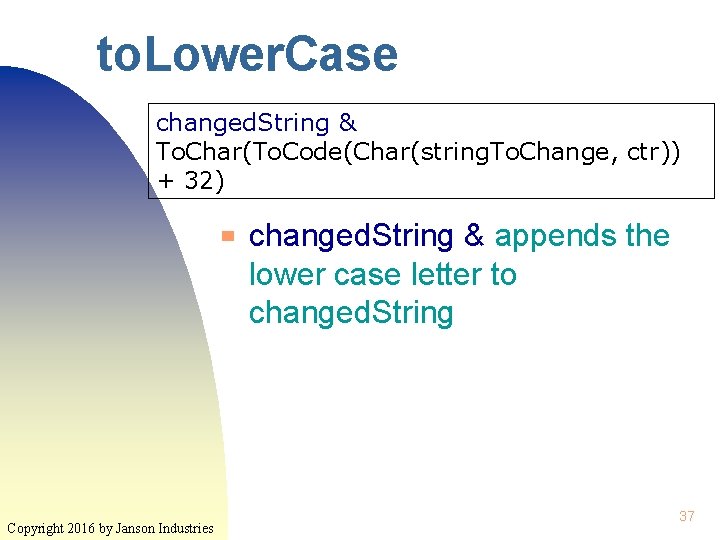
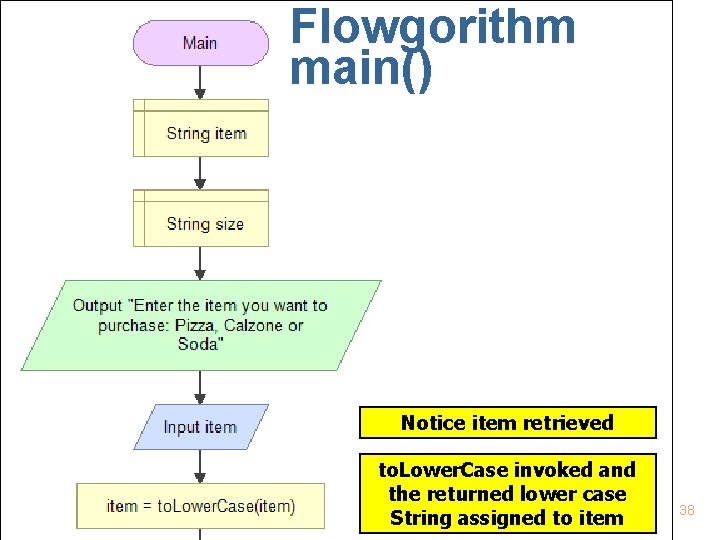
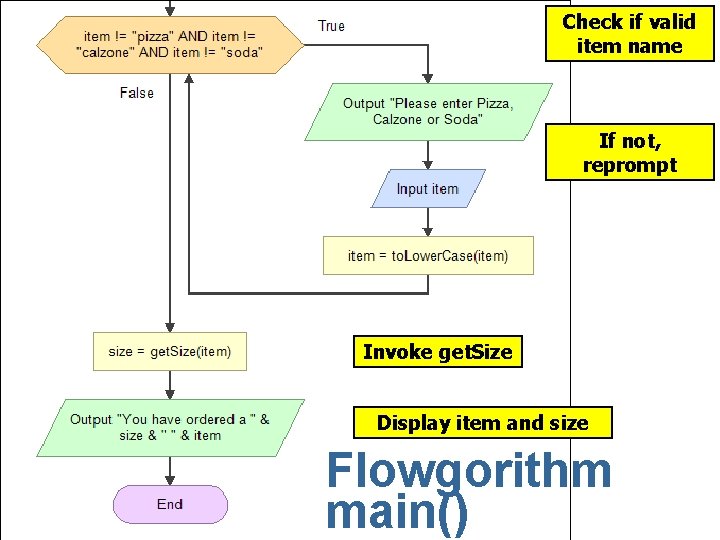
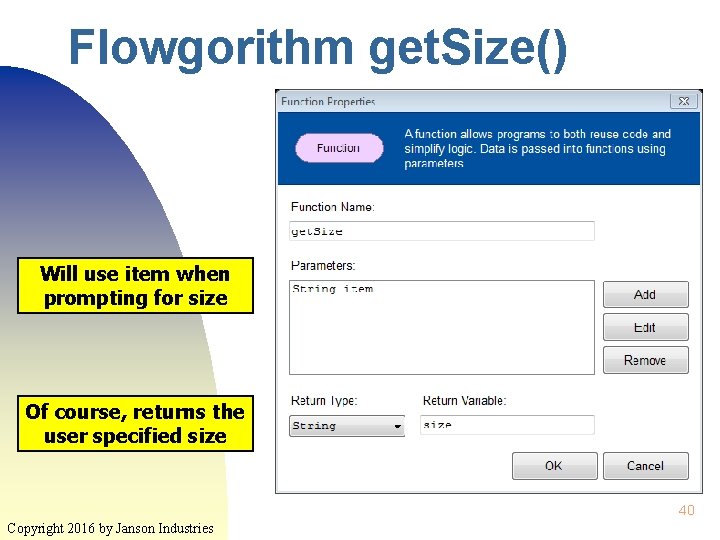
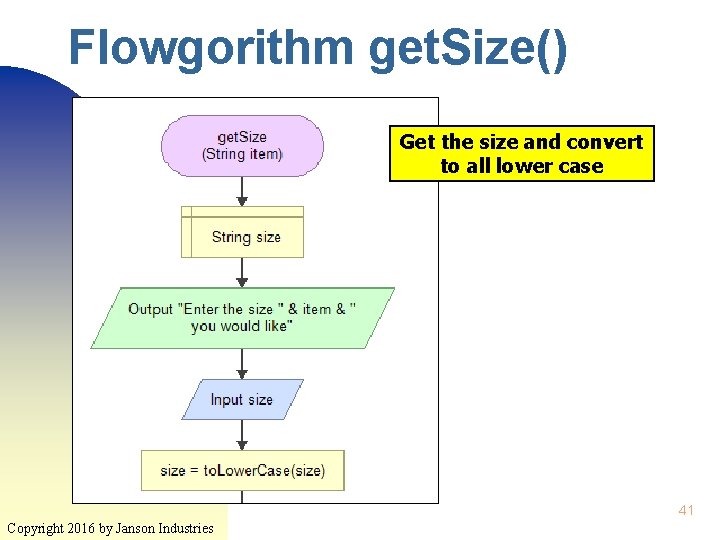
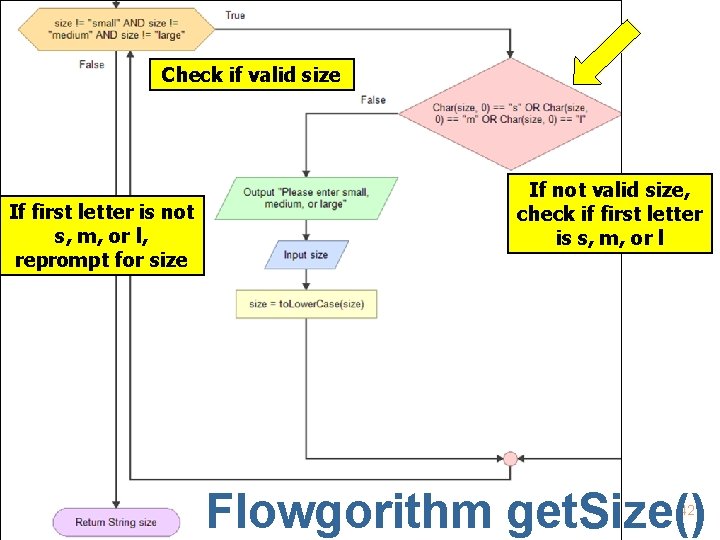
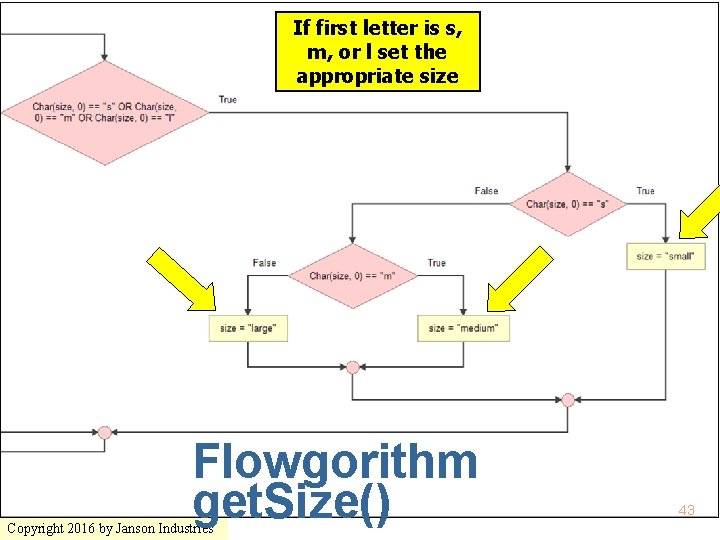
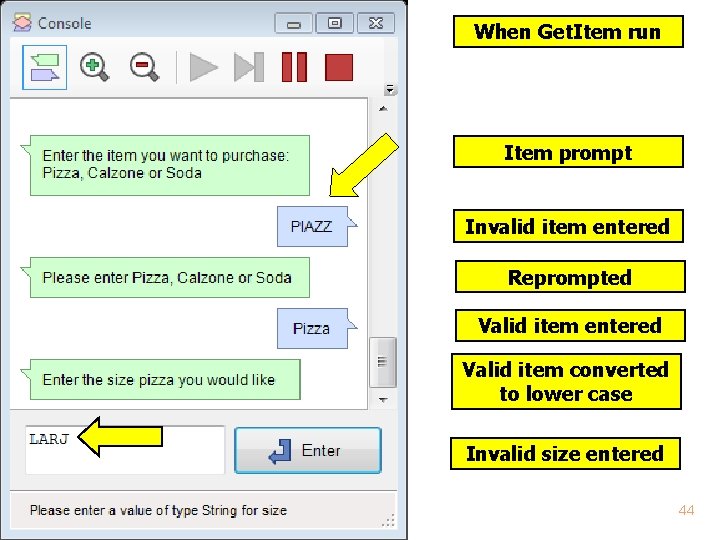
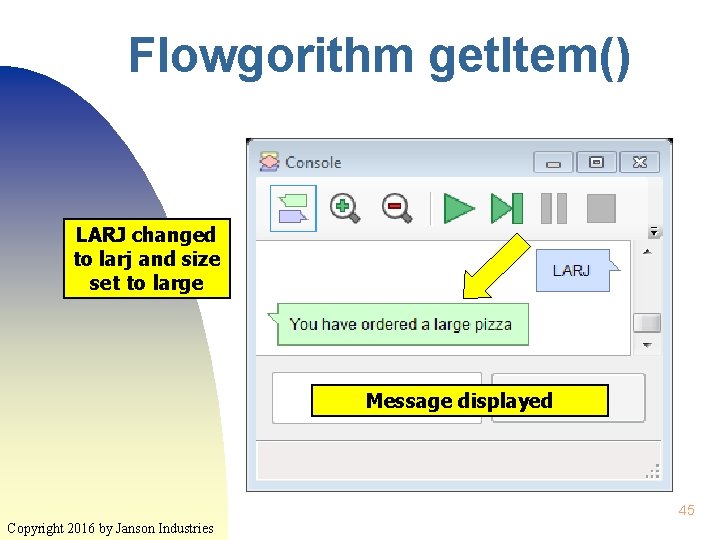
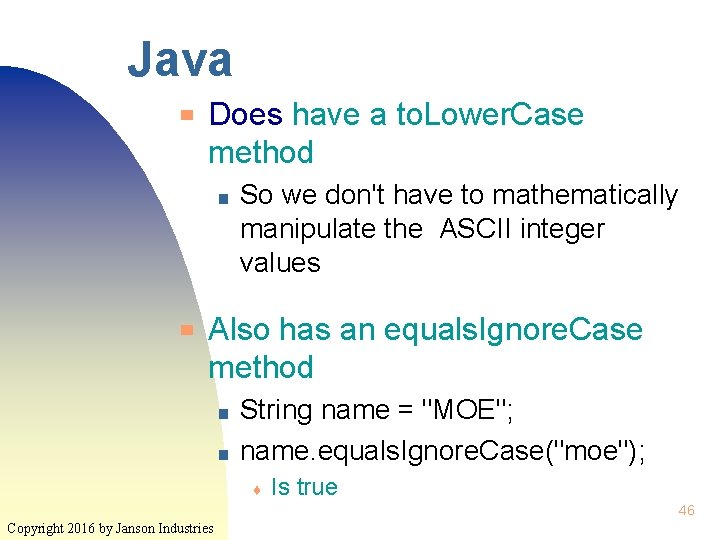
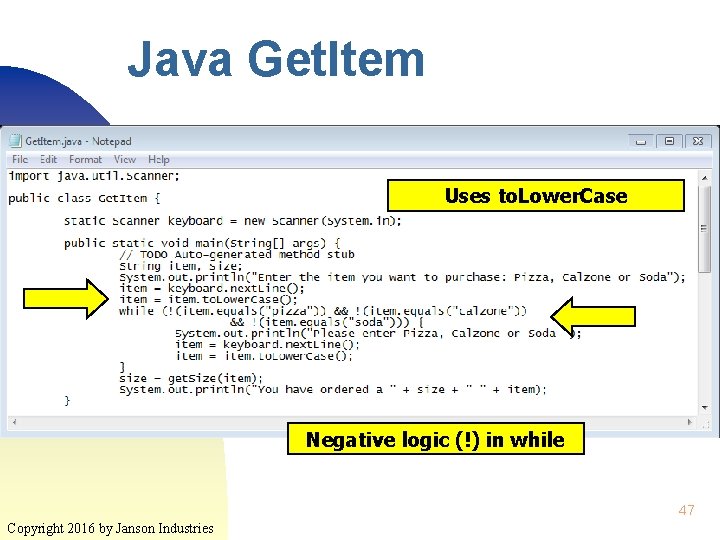
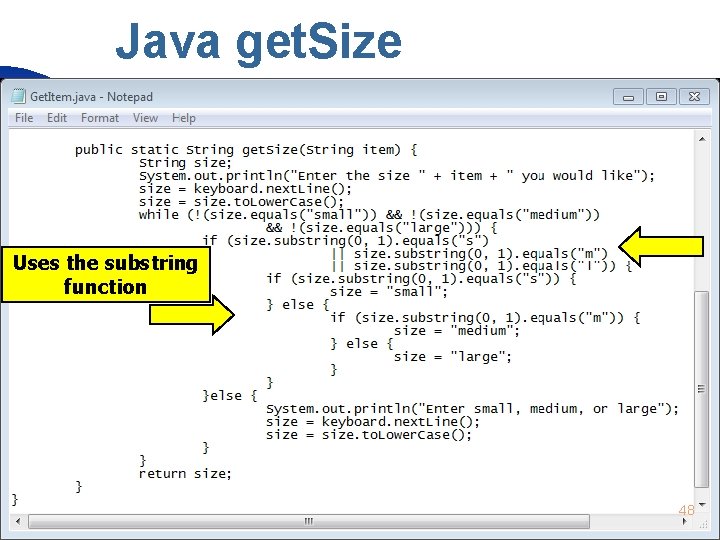
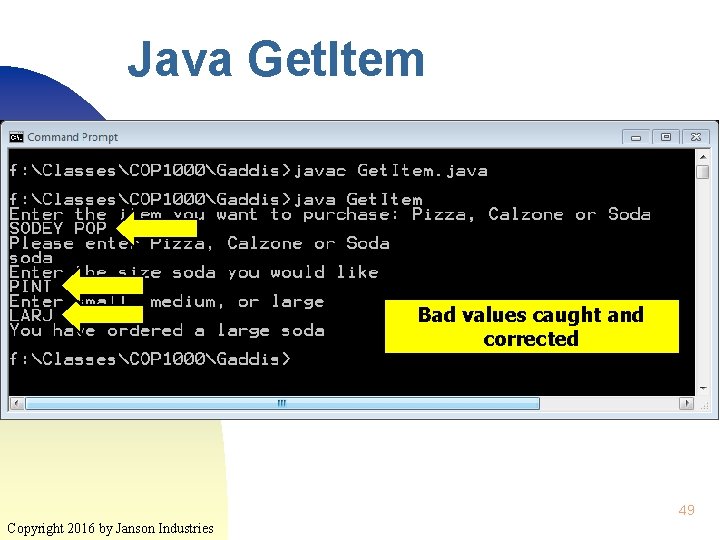
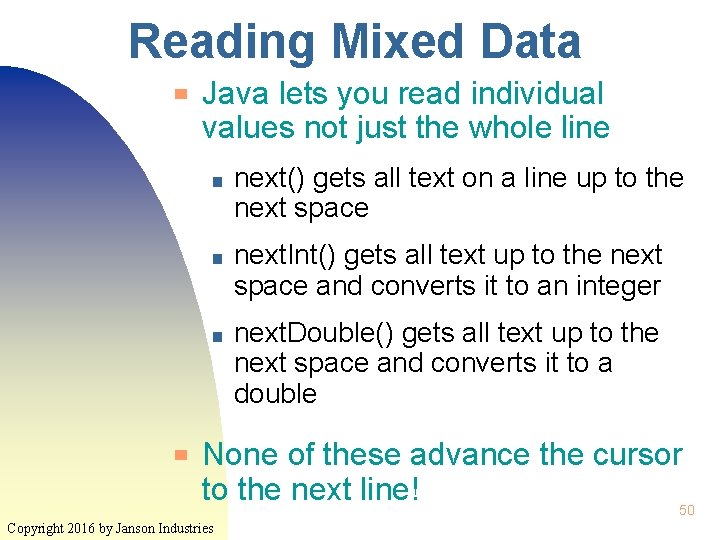
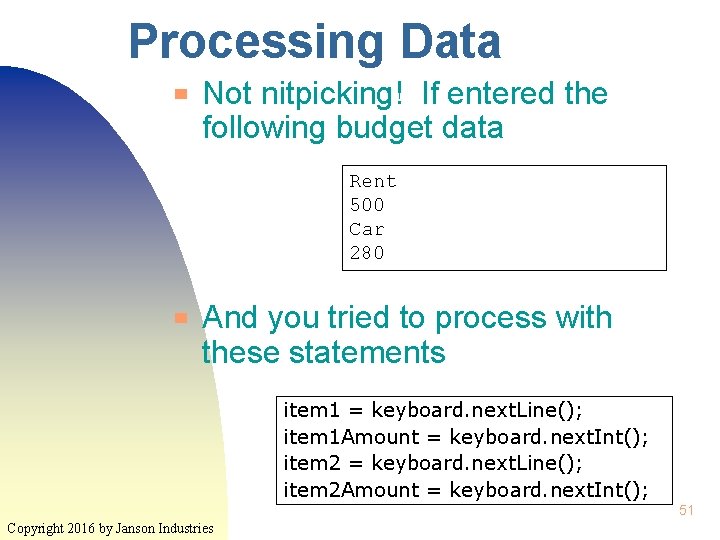
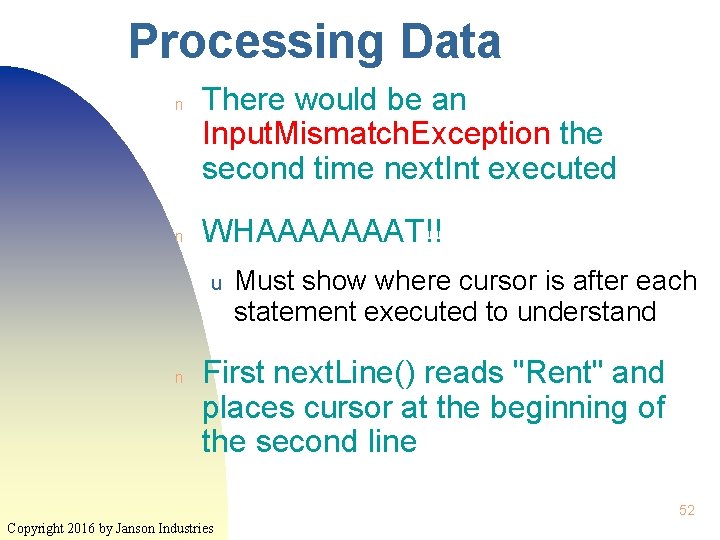
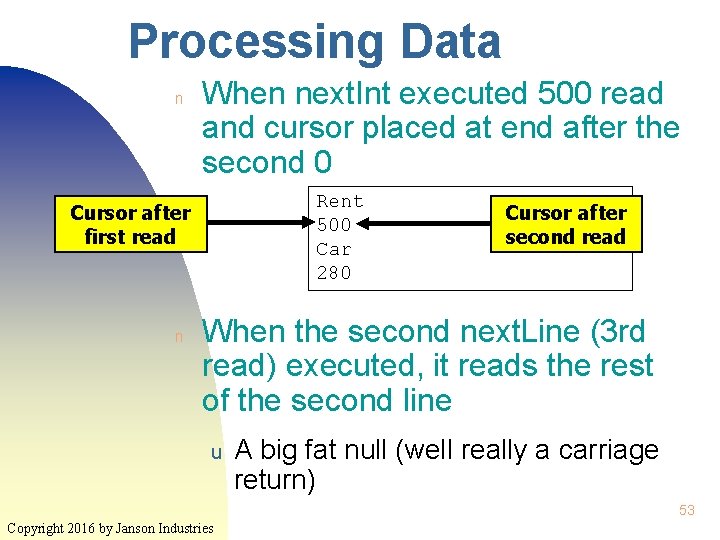
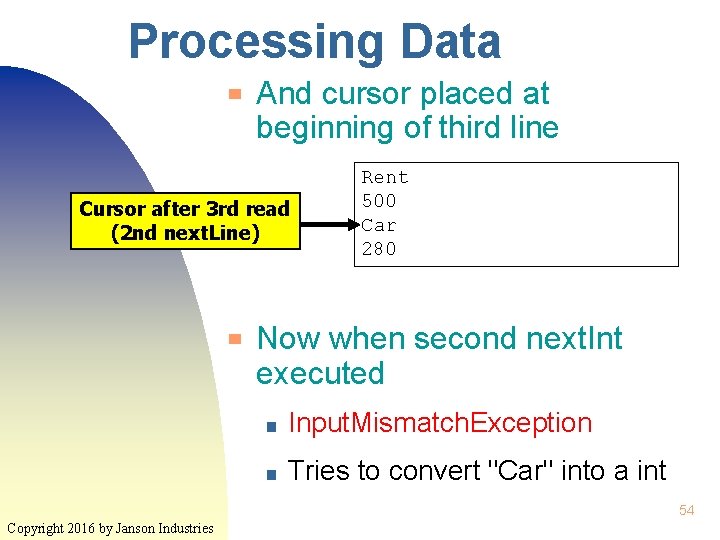
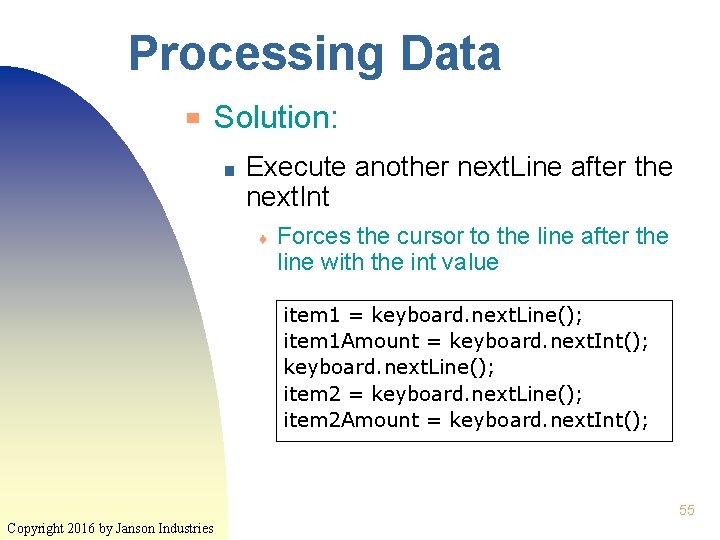
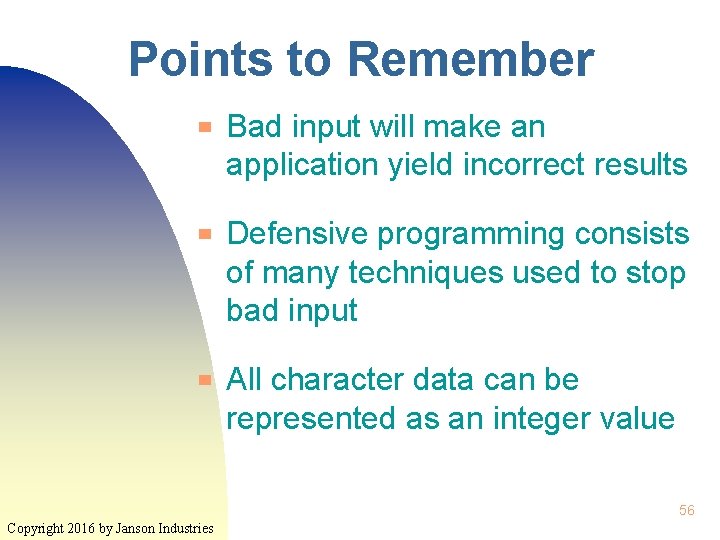
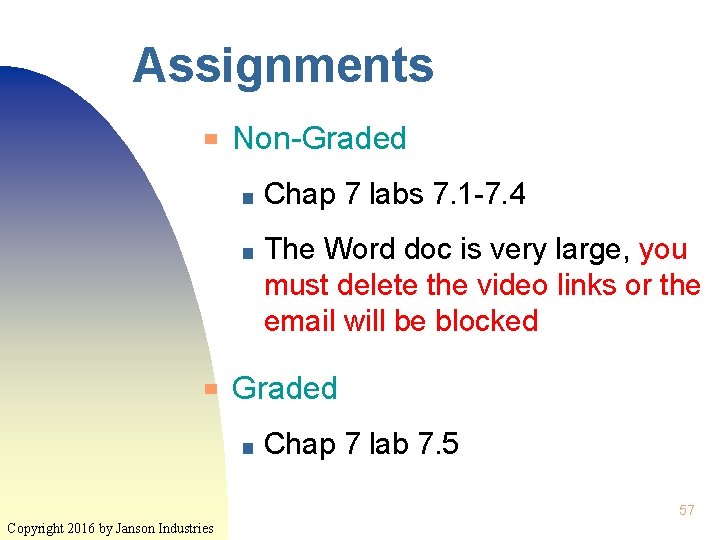
- Slides: 57
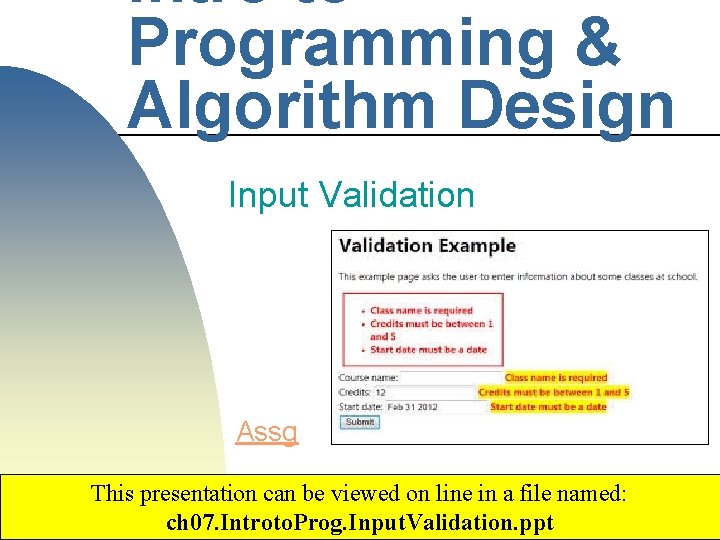
Intro to Programming & Algorithm Design Input Validation Assg This presentation can be viewed on line in a file named: ch 07. Introto. Prog. Input. Validation. ppt Copyright 2003 by Janson Industries 1
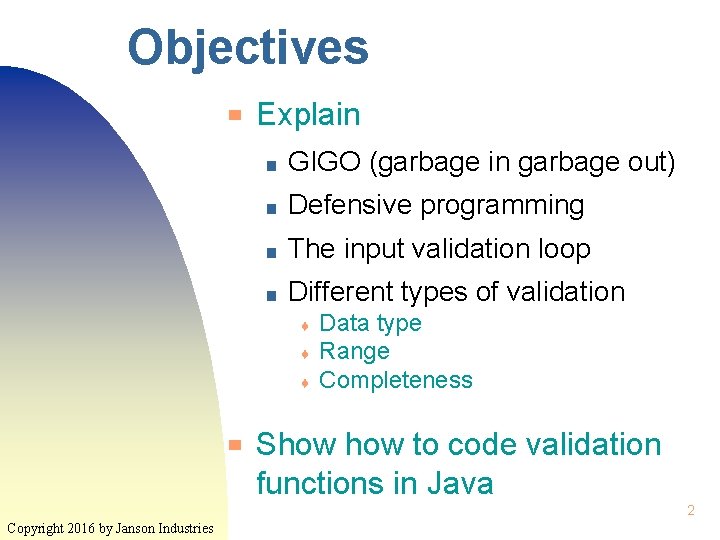
Objectives ▀ Explain ■ GIGO (garbage in garbage out) ■ Defensive programming ■ The input validation loop ■ Different types of validation ♦ ♦ ♦ ▀ Data type Range Completeness Show to code validation functions in Java 2 Copyright 2016 by Janson Industries
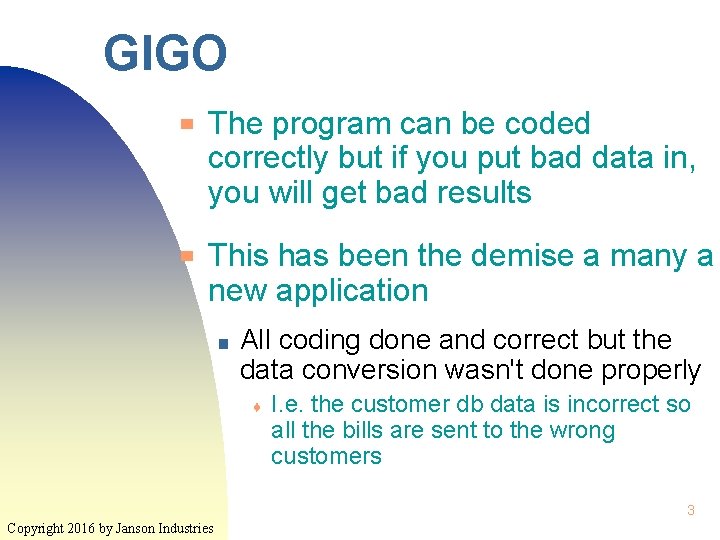
GIGO ▀ ▀ The program can be coded correctly but if you put bad data in, you will get bad results This has been the demise a many a new application ■ All coding done and correct but the data conversion wasn't done properly ♦ I. e. the customer db data is incorrect so all the bills are sent to the wrong customers 3 Copyright 2016 by Janson Industries
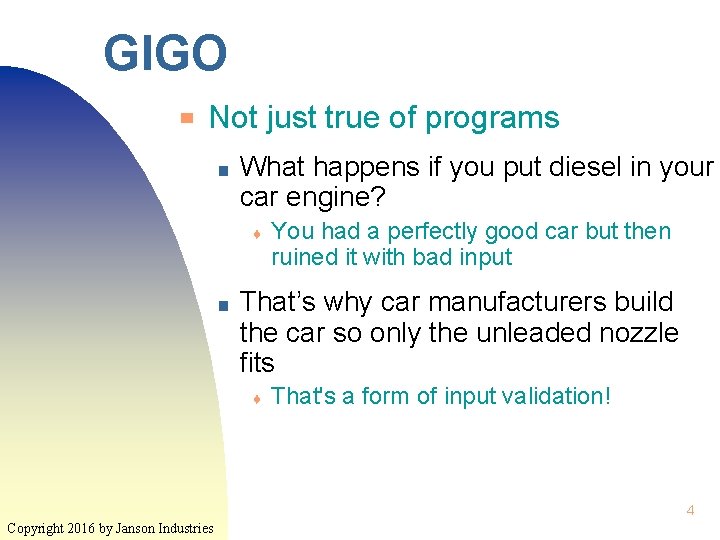
GIGO ▀ Not just true of programs ■ What happens if you put diesel in your car engine? ♦ ■ You had a perfectly good car but then ruined it with bad input That’s why car manufacturers build the car so only the unleaded nozzle fits ♦ That's a form of input validation! 4 Copyright 2016 by Janson Industries
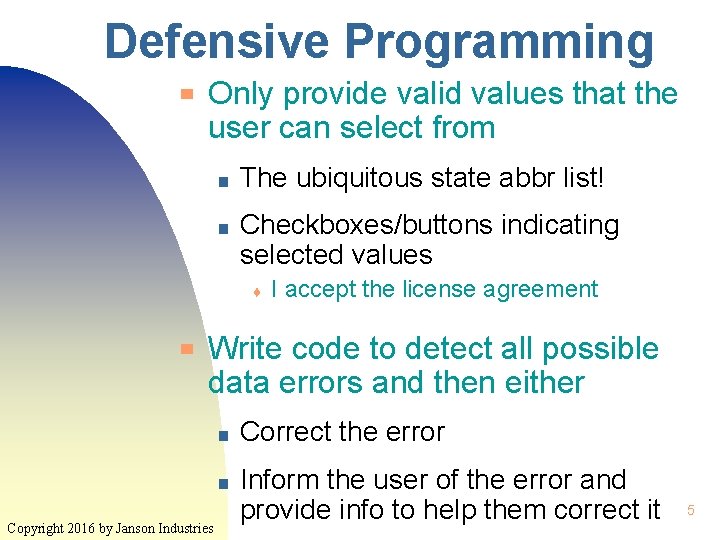
Defensive Programming ▀ Only provide valid values that the user can select from ■ The ubiquitous state abbr list! ■ Checkboxes/buttons indicating selected values ♦ ▀ I accept the license agreement Write code to detect all possible data errors and then either Copyright 2016 by Janson Industries ■ Correct the error ■ Inform the user of the error and provide info to help them correct it 5
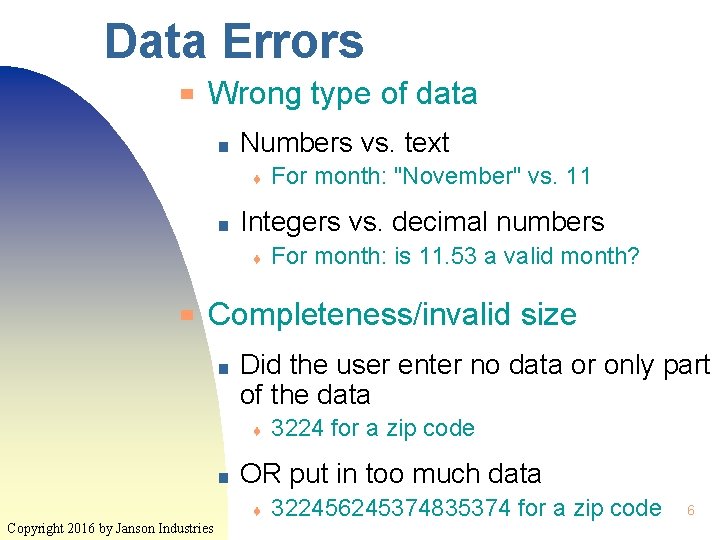
Data Errors ▀ Wrong type of data ■ Numbers vs. text ♦ ■ Integers vs. decimal numbers ♦ ▀ For month: "November" vs. 11 For month: is 11. 53 a valid month? Completeness/invalid size ■ Did the user enter no data or only part of the data ♦ ■ OR put in too much data ♦ Copyright 2016 by Janson Industries 3224 for a zip code 322456245374835374 for a zip code 6
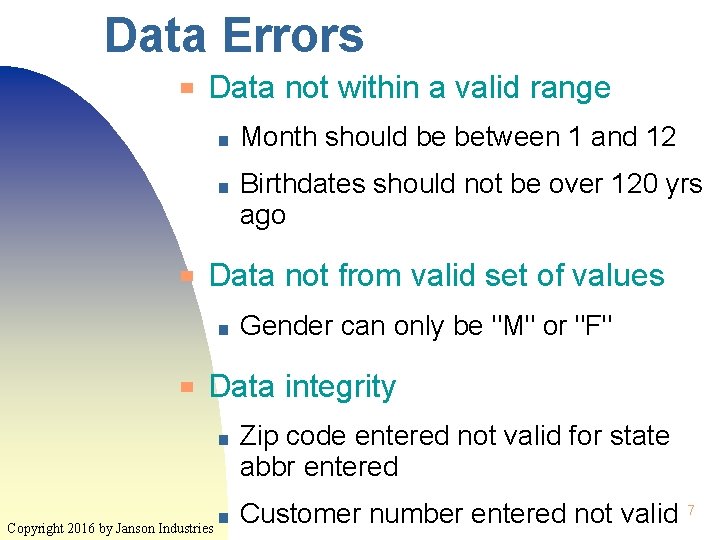
Data Errors ▀ ▀ Data not within a valid range ■ Month should be between 1 and 12 ■ Birthdates should not be over 120 yrs ago Data not from valid set of values ■ ▀ Gender can only be "M" or "F" Data integrity ■ ■ Copyright 2016 by Janson Industries Zip code entered not valid for state abbr entered Customer number entered not valid 7
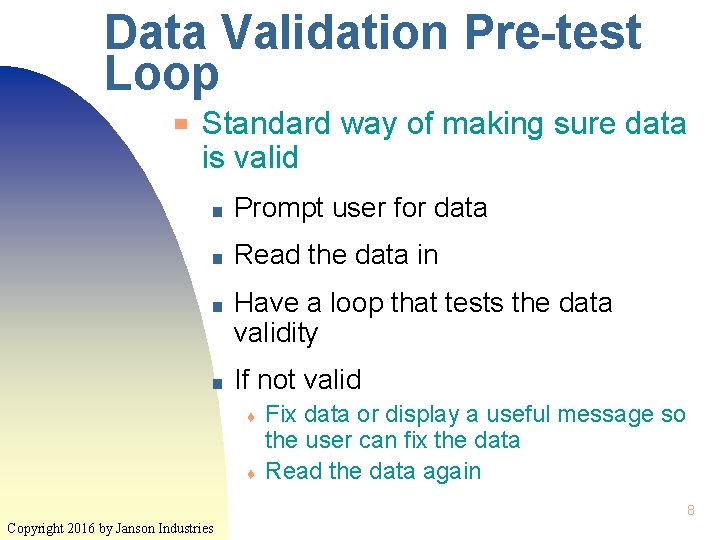
Data Validation Pre-test Loop ▀ Standard way of making sure data is valid ■ Prompt user for data ■ Read the data in ■ Have a loop that tests the data validity ■ If not valid ♦ ♦ Fix data or display a useful message so the user can fix the data Read the data again 8 Copyright 2016 by Janson Industries
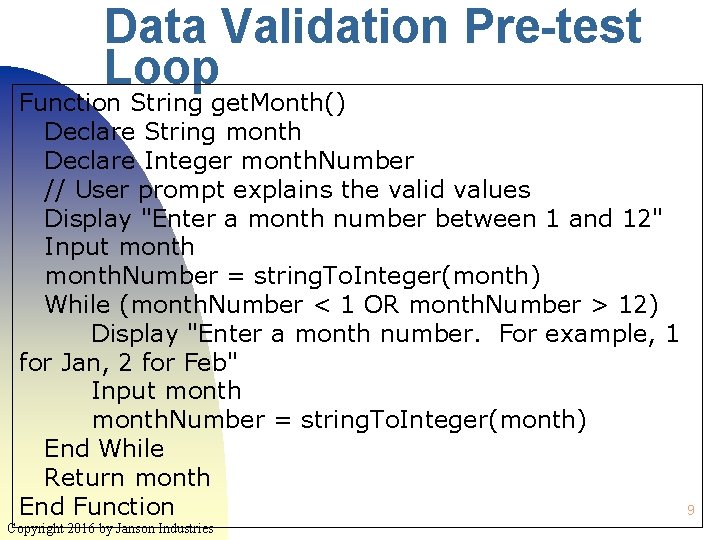
Data Validation Pre-test Loop Function String get. Month() Declare String month Declare Integer month. Number // User prompt explains the valid values Display "Enter a month number between 1 and 12" Input month. Number = string. To. Integer(month) While (month. Number < 1 OR month. Number > 12) Display "Enter a month number. For example, 1 for Jan, 2 for Feb" Input month. Number = string. To. Integer(month) End While Return month End Function 9 Copyright 2016 by Janson Industries
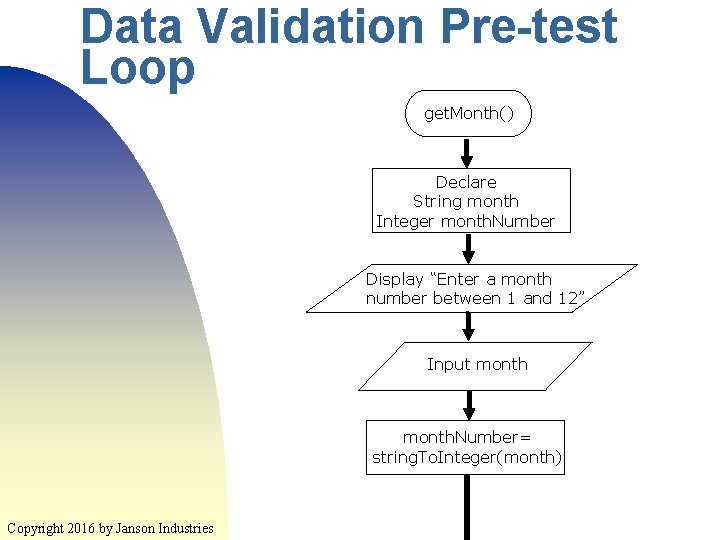
Data Validation Pre-test Loop get. Month() Declare String month Integer month. Number Display “Enter a month number between 1 and 12” Input month. Number= string. To. Integer(month) Copyright 2016 by Janson Industries
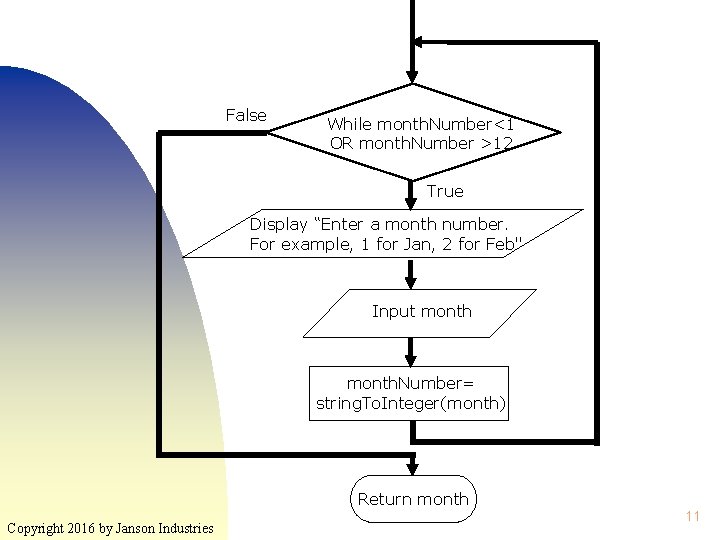
False While month. Number<1 OR month. Number >12 True Display “Enter a month number. For example, 1 for Jan, 2 for Feb" Input month. Number= string. To. Integer(month) Return month Copyright 2016 by Janson Industries 11
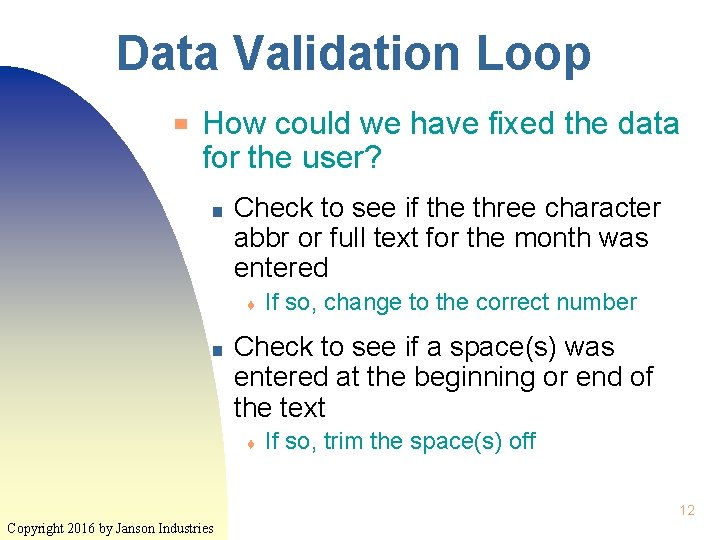
Data Validation Loop ▀ How could we have fixed the data for the user? ■ Check to see if the three character abbr or full text for the month was entered ♦ ■ If so, change to the correct number Check to see if a space(s) was entered at the beginning or end of the text ♦ If so, trim the space(s) off 12 Copyright 2016 by Janson Industries
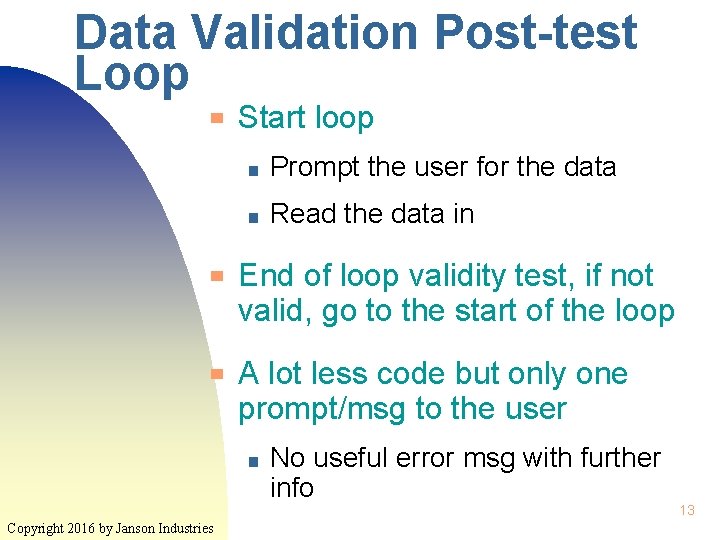
Data Validation Post-test Loop ▀ ▀ ▀ Start loop ■ Prompt the user for the data ■ Read the data in End of loop validity test, if not valid, go to the start of the loop A lot less code but only one prompt/msg to the user ■ Copyright 2016 by Janson Industries No useful error msg with further info 13
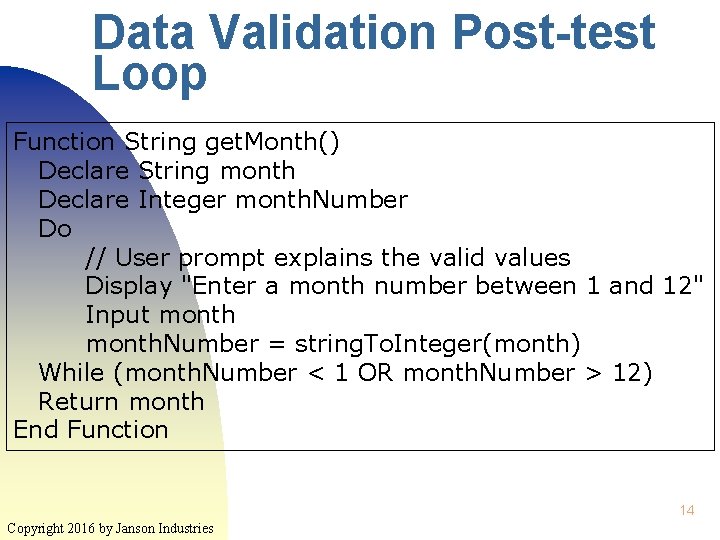
Data Validation Post-test Loop Function String get. Month() Declare String month Declare Integer month. Number Do // User prompt explains the valid values Display "Enter a month number between 1 and 12" Input month. Number = string. To. Integer(month) While (month. Number < 1 OR month. Number > 12) Return month End Function 14 Copyright 2016 by Janson Industries
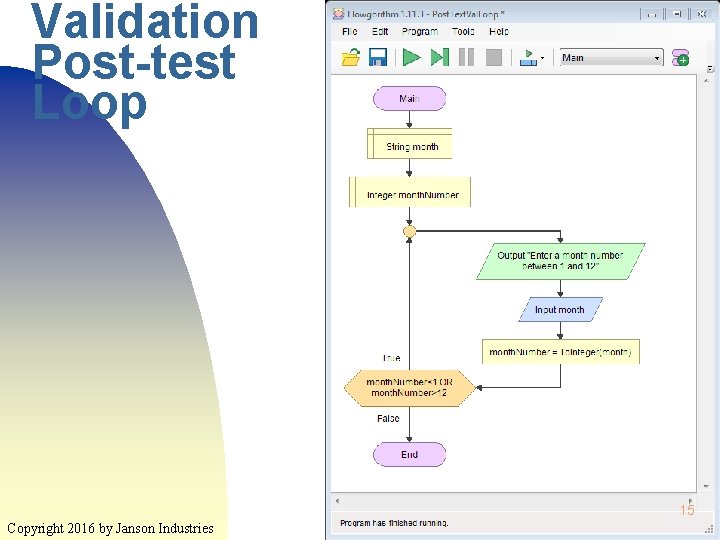
Validation Post-test Loop 15 Copyright 2016 by Janson Industries
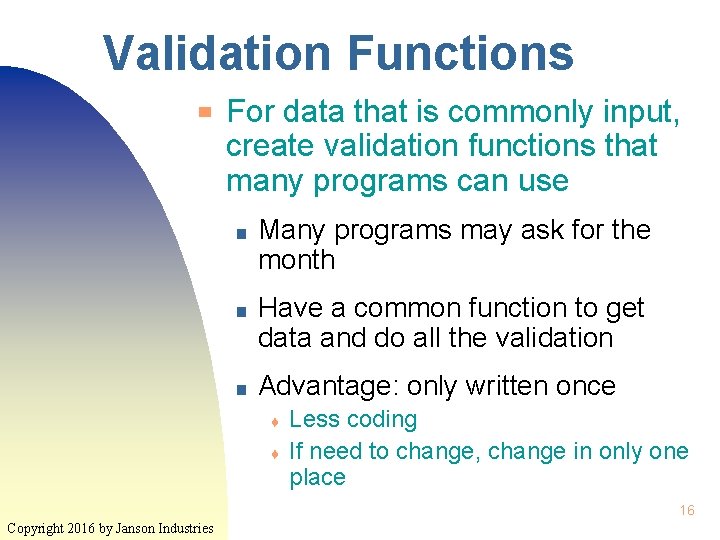
Validation Functions ▀ For data that is commonly input, create validation functions that many programs can use ■ Many programs may ask for the month ■ Have a common function to get data and do all the validation ■ Advantage: only written once ♦ ♦ Less coding If need to change, change in only one place 16 Copyright 2016 by Janson Industries
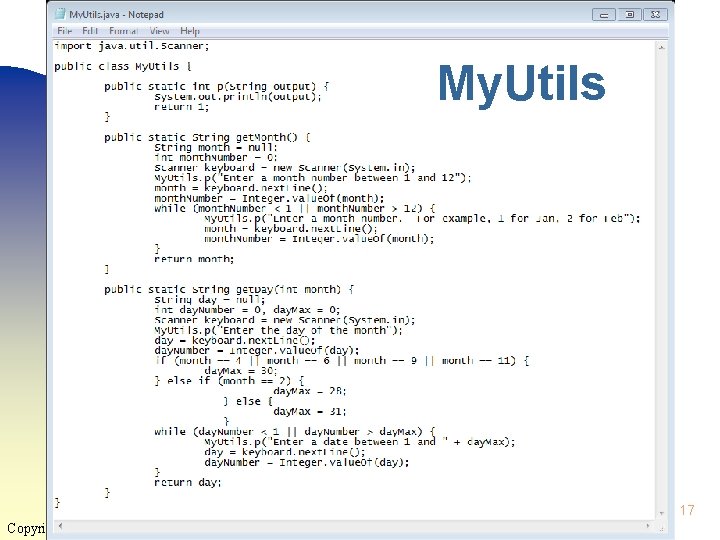
My. Utils 17 Copyright 2016 by Janson Industries
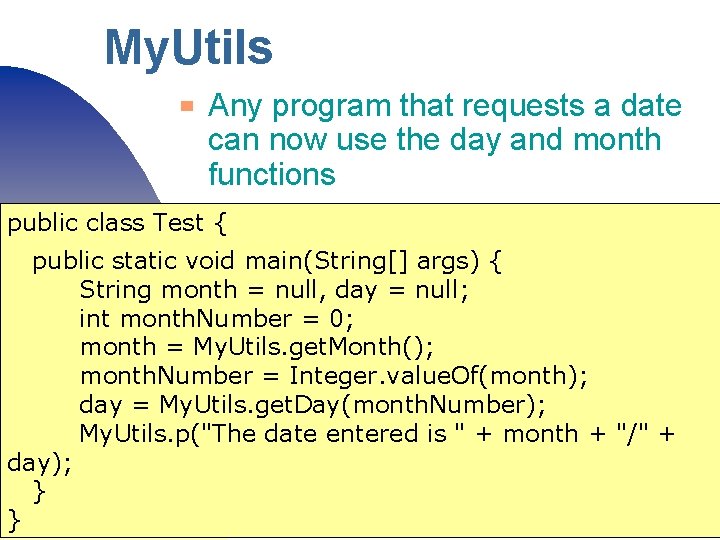
My. Utils ▀ Any program that requests a date can now use the day and month functions public class Test { public static void main(String[] args) { String month = null, day = null; int month. Number = 0; month = My. Utils. get. Month(); month. Number = Integer. value. Of(month); day = My. Utils. get. Day(month. Number); My. Utils. p("The date entered is " + month + "/" + day); } 18 } Copyright 2016 by Janson Industries
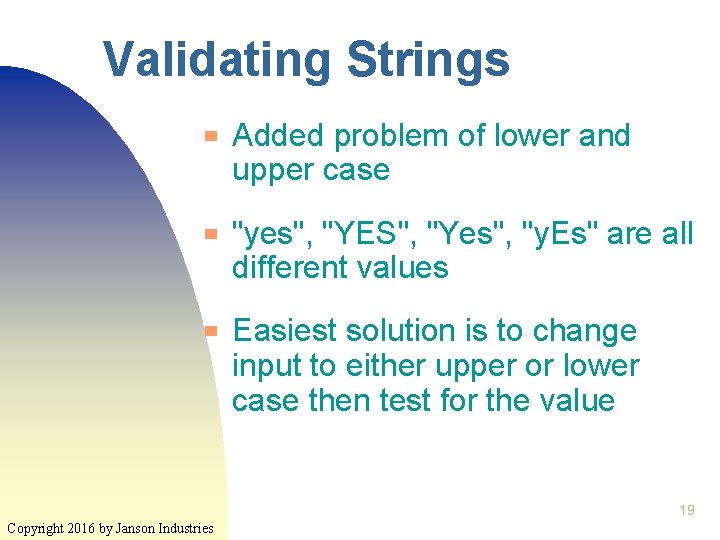
Validating Strings ▀ ▀ ▀ Added problem of lower and upper case "yes", "YES", "Yes", "y. Es" are all different values Easiest solution is to change input to either upper or lower case then test for the value 19 Copyright 2016 by Janson Industries
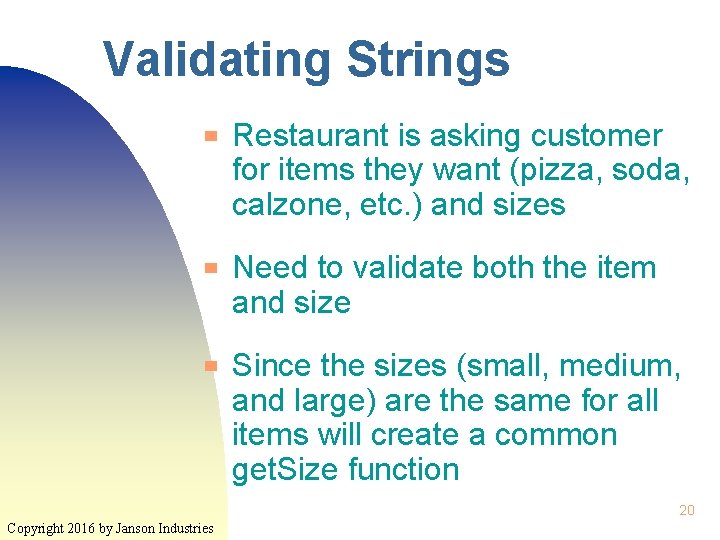
Validating Strings ▀ ▀ ▀ Restaurant is asking customer for items they want (pizza, soda, calzone, etc. ) and sizes Need to validate both the item and size Since the sizes (small, medium, and large) are the same for all items will create a common get. Size function 20 Copyright 2016 by Janson Industries
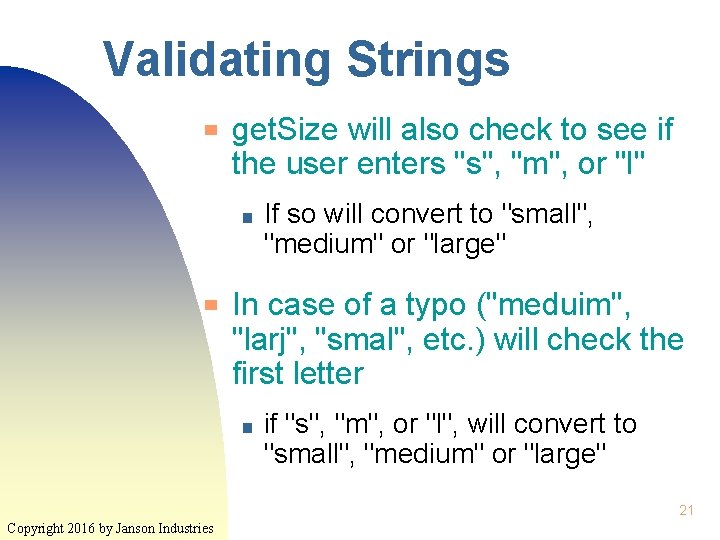
Validating Strings ▀ get. Size will also check to see if the user enters "s", "m", or "l" ■ ▀ If so will convert to "small", "medium" or "large" In case of a typo ("meduim", "larj", "smal", etc. ) will check the first letter ■ if "s", "m", or "l", will convert to "small", "medium" or "large" 21 Copyright 2016 by Janson Industries
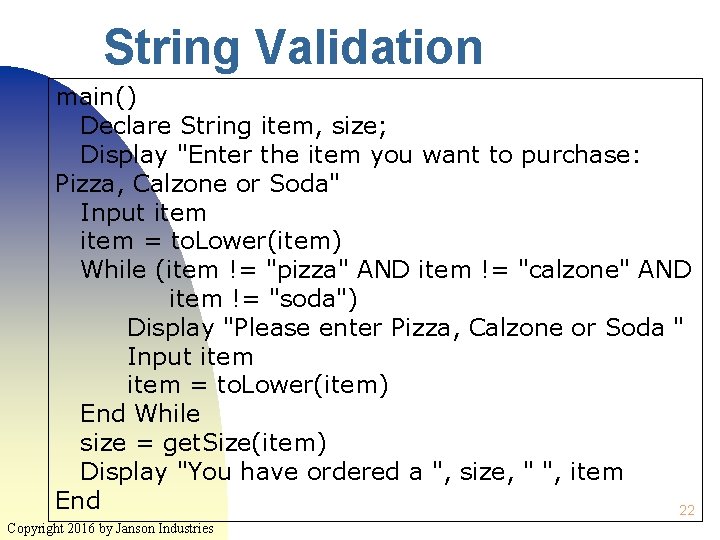
String Validation main() Declare String item, size; Display "Enter the item you want to purchase: Pizza, Calzone or Soda" Input item = to. Lower(item) While (item != "pizza" AND item != "calzone" AND item != "soda") Display "Please enter Pizza, Calzone or Soda " Input item = to. Lower(item) End While size = get. Size(item) Display "You have ordered a ", size, " ", item End 22 Copyright 2016 by Janson Industries
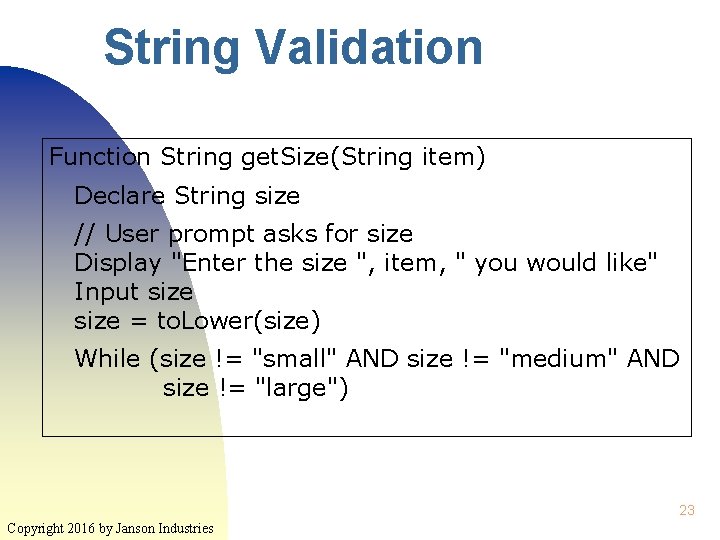
String Validation Function String get. Size(String item) Declare String size // User prompt asks for size Display "Enter the size ", item, " you would like" Input size = to. Lower(size) While (size != "small" AND size != "medium" AND size != "large") 23 Copyright 2016 by Janson Industries
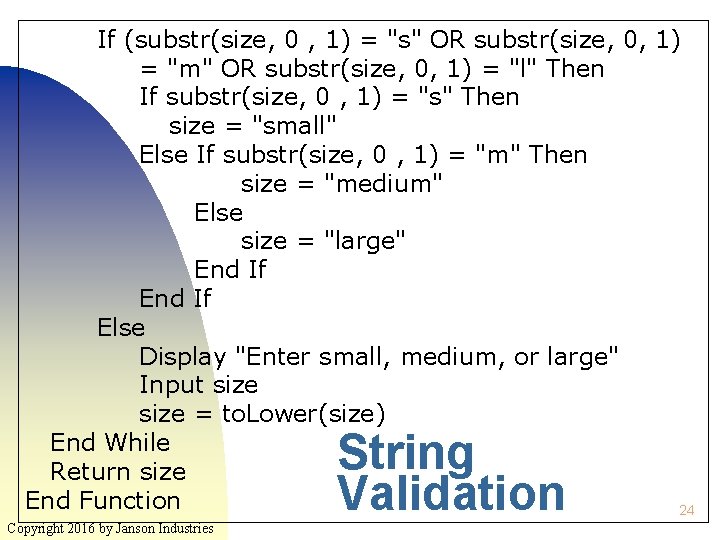
If (substr(size, 0 , 1) = "s" OR substr(size, 0, 1) = "m" OR substr(size, 0, 1) = "l" Then If substr(size, 0 , 1) = "s" Then size = "small" Else If substr(size, 0 , 1) = "m" Then size = "medium" Else size = "large" End If Else Display "Enter small, medium, or large" Input size = to. Lower(size) End While Return size End Function 24 String Validation Copyright 2016 by Janson Industries
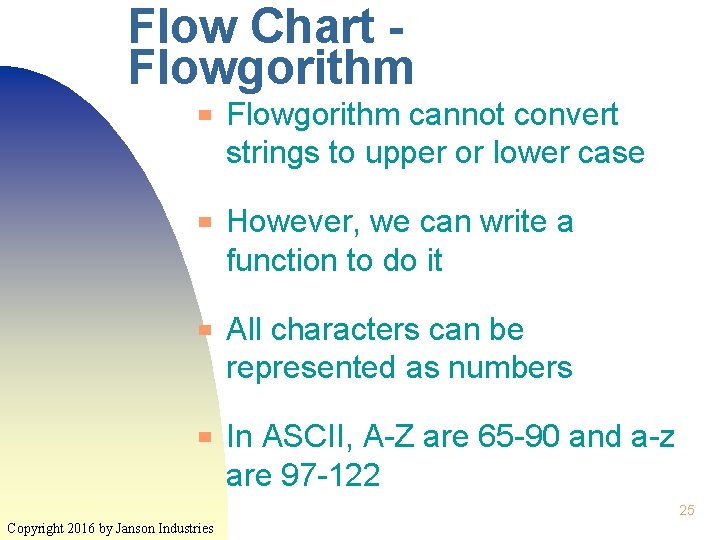
Flow Chart Flowgorithm ▀ ▀ Flowgorithm cannot convert strings to upper or lower case However, we can write a function to do it All characters can be represented as numbers In ASCII, A-Z are 65 -90 and a-z are 97 -122 25 Copyright 2016 by Janson Industries
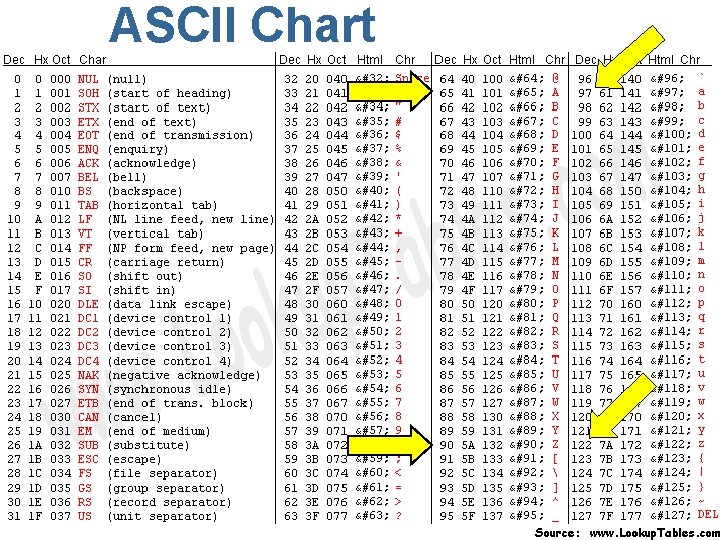
ASCII Chart 26 Copyright 2016 by Janson Industries
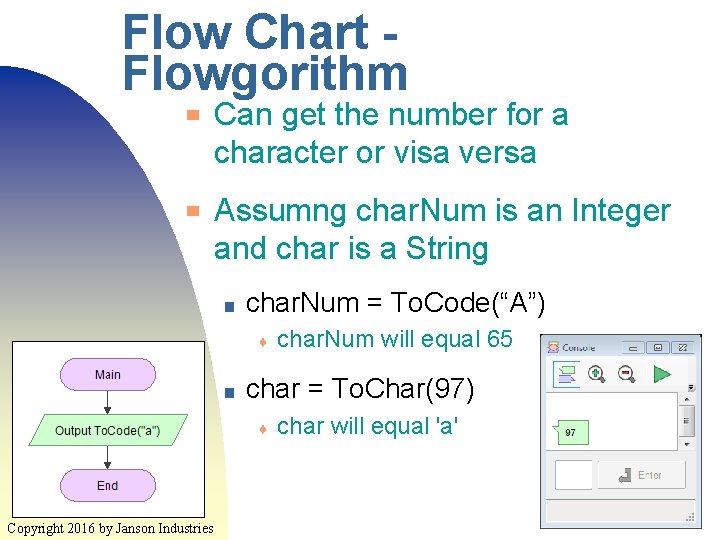
Flow Chart Flowgorithm ▀ ▀ Can get the number for a character or visa versa Assumng char. Num is an Integer and char is a String ■ char. Num = To. Code(“A”) ♦ ■ char. Num will equal 65 char = To. Char(97) ♦ char will equal 'a' 27 Copyright 2016 by Janson Industries
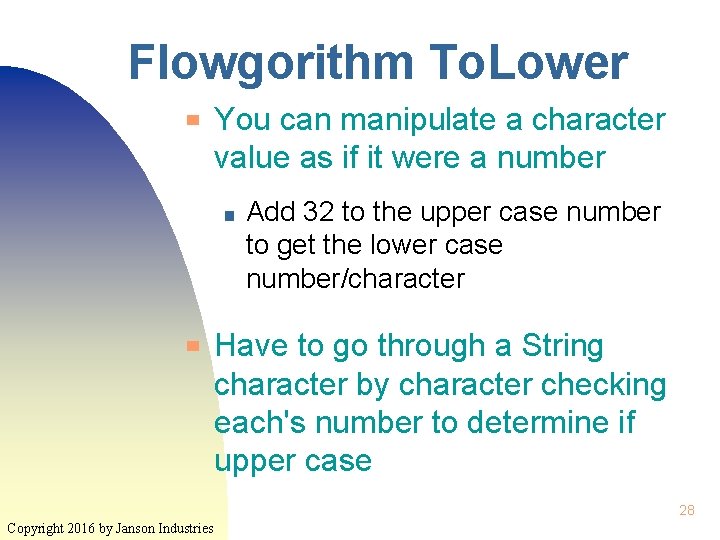
Flowgorithm To. Lower ▀ You can manipulate a character value as if it were a number ■ ▀ Add 32 to the upper case number to get the lower case number/character Have to go through a String character by character checking each's number to determine if upper case 28 Copyright 2016 by Janson Industries
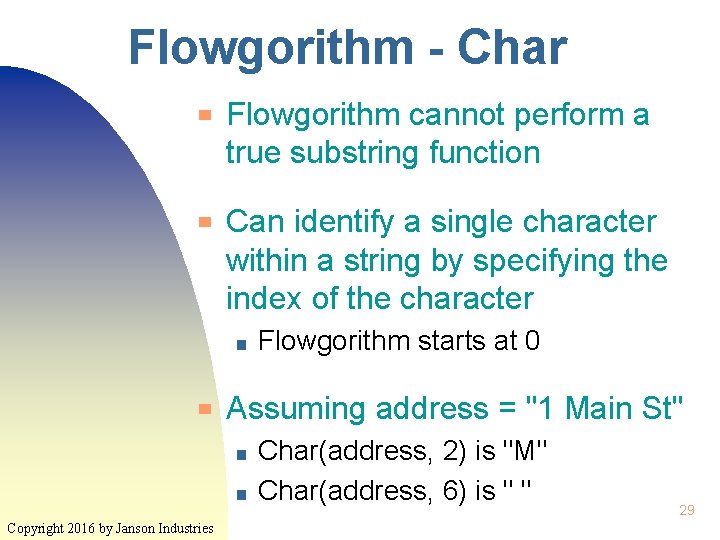
Flowgorithm - Char ▀ ▀ Flowgorithm cannot perform a true substring function Can identify a single character within a string by specifying the index of the character ■ ▀ Assuming address = "1 Main St" ■ ■ Copyright 2016 by Janson Industries Flowgorithm starts at 0 Char(address, 2) is "M" Char(address, 6) is " " 29
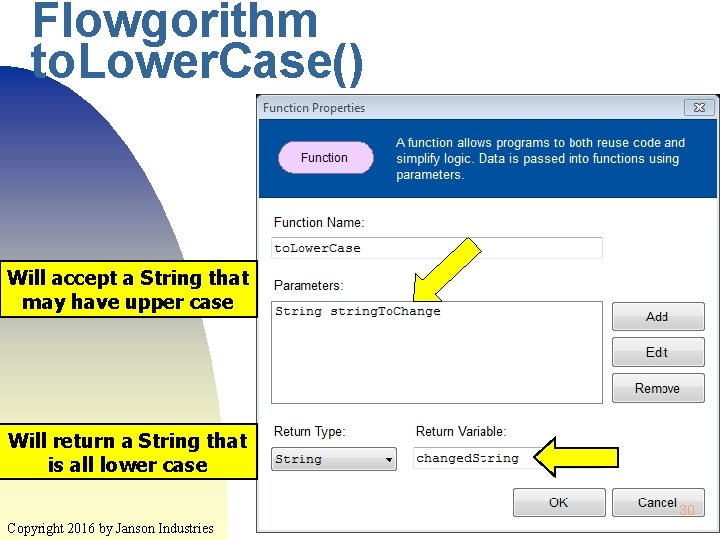
Flowgorithm to. Lower. Case() Will accept a String that may have upper case Will return a String that is all lower case 30 Copyright 2016 by Janson Industries
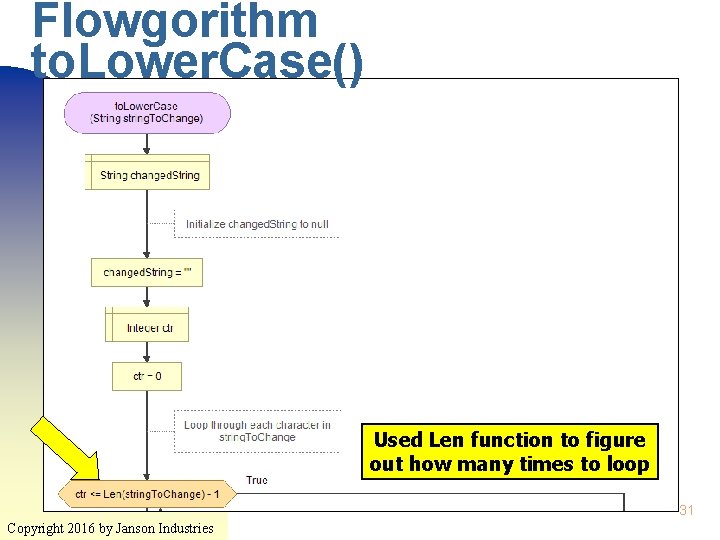
Flowgorithm to. Lower. Case() Used Len function to figure out how many times to loop 31 Copyright 2016 by Janson Industries
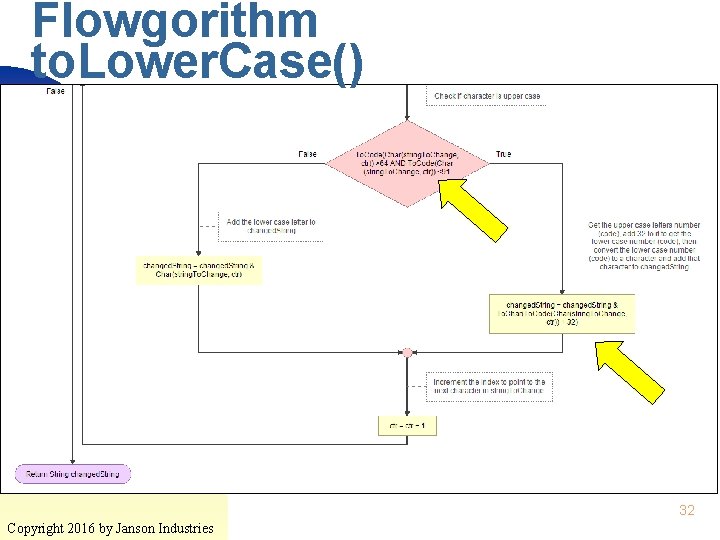
Flowgorithm to. Lower. Case() 32 Copyright 2016 by Janson Industries
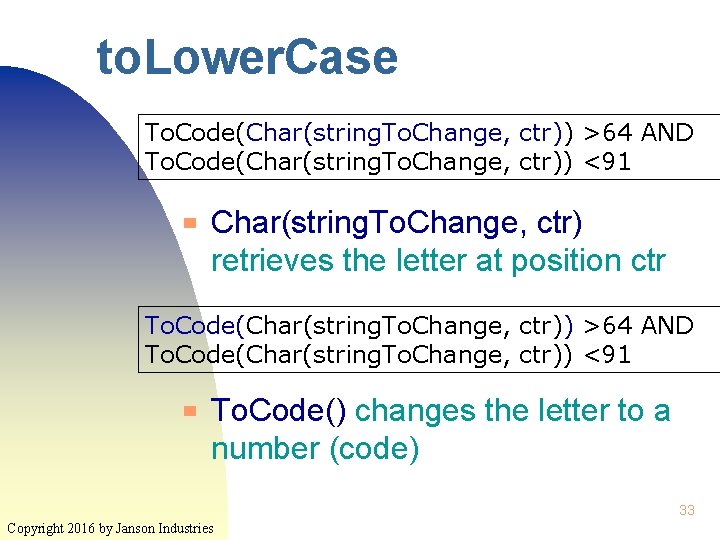
to. Lower. Case To. Code(Char(string. To. Change, ctr)) >64 AND To. Code(Char(string. To. Change, ctr)) <91 ▀ Char(string. To. Change, ctr) retrieves the letter at position ctr To. Code(Char(string. To. Change, ctr)) >64 AND To. Code(Char(string. To. Change, ctr)) <91 ▀ To. Code() changes the letter to a number (code) 33 Copyright 2016 by Janson Industries
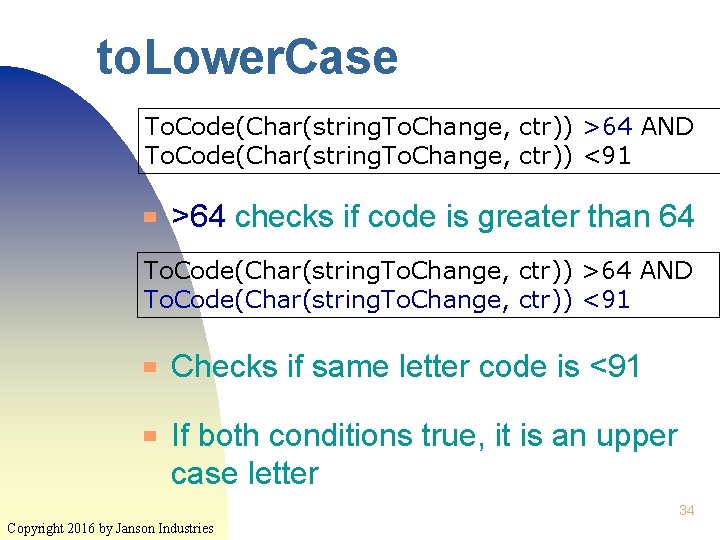
to. Lower. Case To. Code(Char(string. To. Change, ctr)) >64 AND To. Code(Char(string. To. Change, ctr)) <91 ▀ >64 checks if code is greater than 64 To. Code(Char(string. To. Change, ctr)) >64 AND To. Code(Char(string. To. Change, ctr)) <91 ▀ ▀ Checks if same letter code is <91 If both conditions true, it is an upper case letter 34 Copyright 2016 by Janson Industries
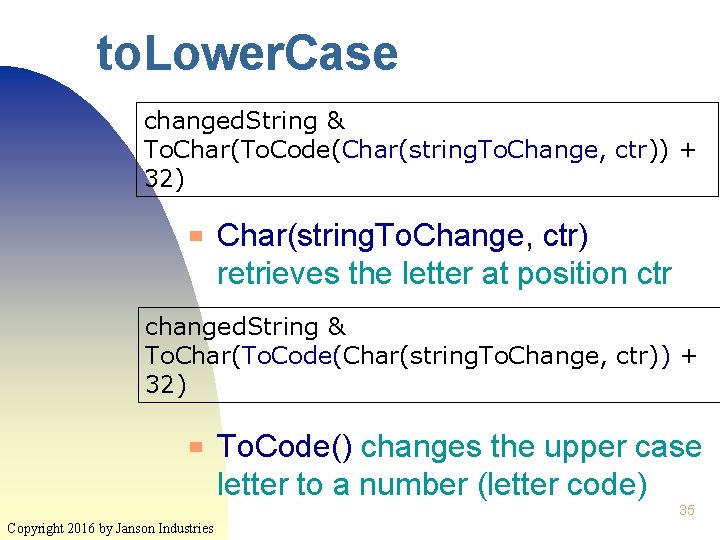
to. Lower. Case changed. String & To. Char(To. Code(Char(string. To. Change, ctr)) + 32) ▀ Char(string. To. Change, ctr) retrieves the letter at position ctr changed. String & To. Char(To. Code(Char(string. To. Change, ctr)) + 32) ▀ To. Code() changes the upper case letter to a number (letter code) 35 Copyright 2016 by Janson Industries
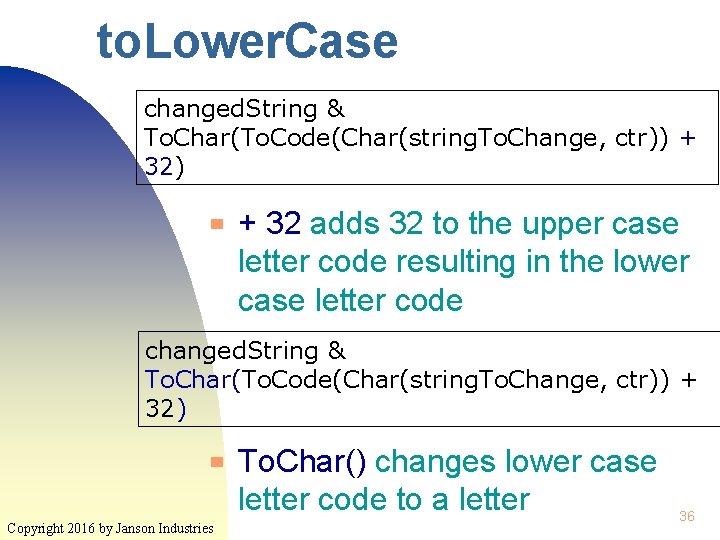
to. Lower. Case changed. String & To. Char(To. Code(Char(string. To. Change, ctr)) + 32) ▀ + 32 adds 32 to the upper case letter code resulting in the lower case letter code changed. String & To. Char(To. Code(Char(string. To. Change, ctr)) + 32) ▀ Copyright 2016 by Janson Industries To. Char() changes lower case letter code to a letter 36
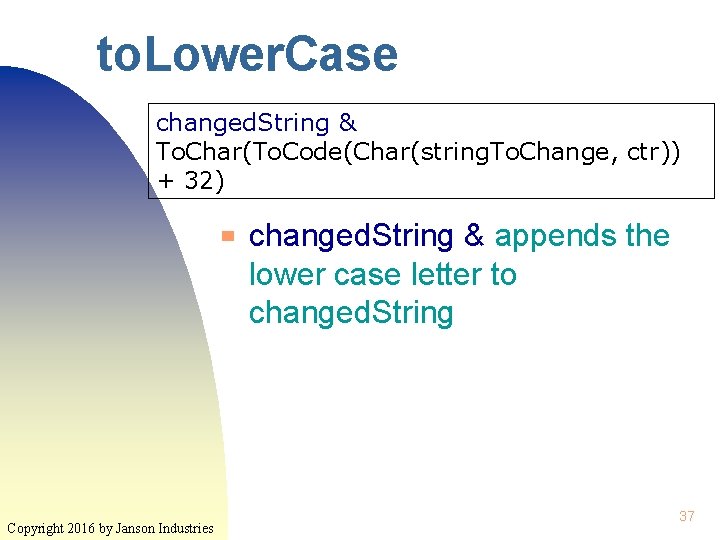
to. Lower. Case changed. String & To. Char(To. Code(Char(string. To. Change, ctr)) + 32) ▀ Copyright 2016 by Janson Industries changed. String & appends the lower case letter to changed. String 37
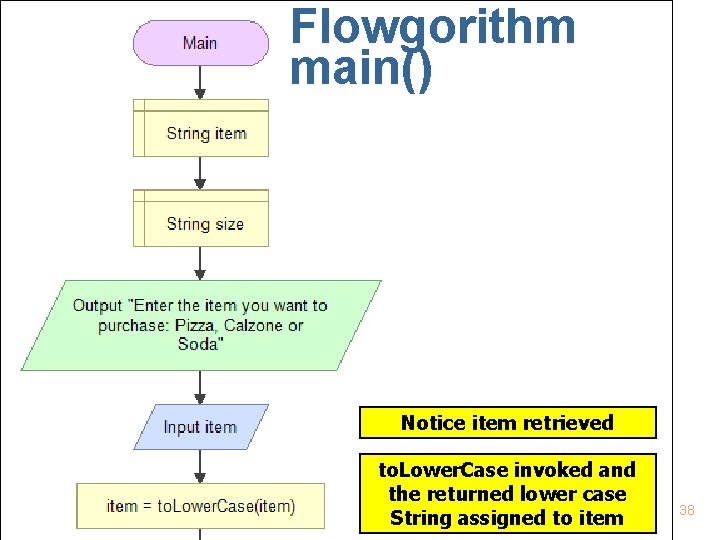
Flowgorithm main() Notice item retrieved Copyright 2016 by Janson Industries to. Lower. Case invoked and the returned lower case String assigned to item 38
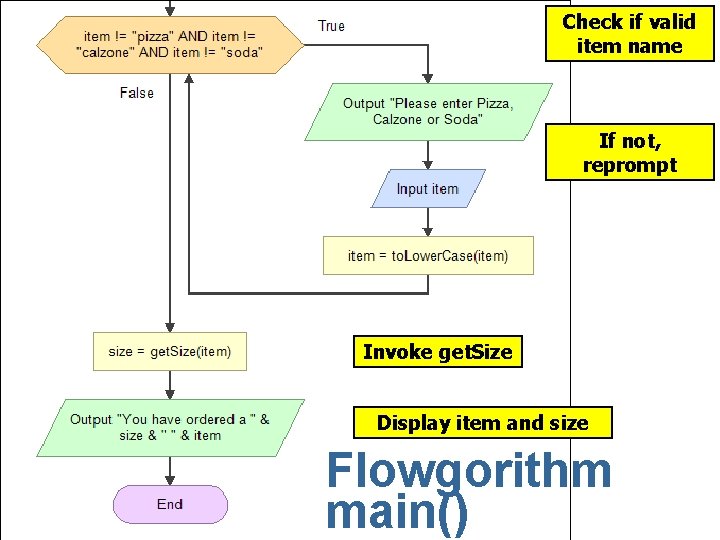
Check if valid item name If not, reprompt Invoke get. Size Display item and size Copyright 2016 by Janson Industries Flowgorithm main()
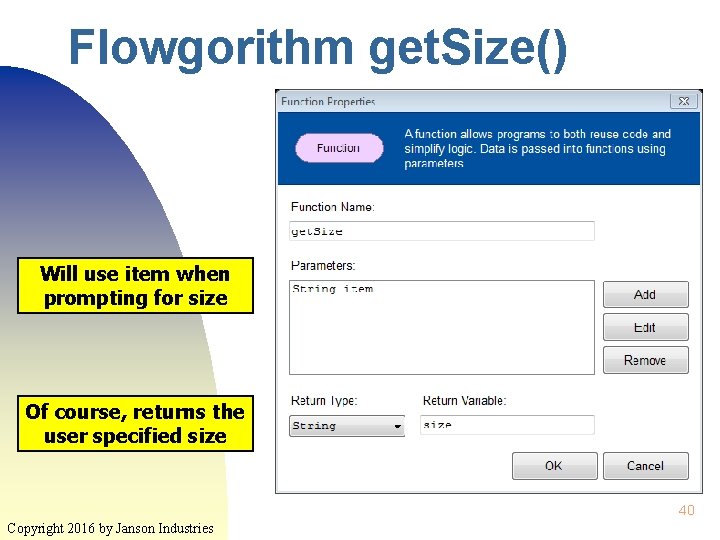
Flowgorithm get. Size() Will use item when prompting for size Of course, returns the user specified size 40 Copyright 2016 by Janson Industries
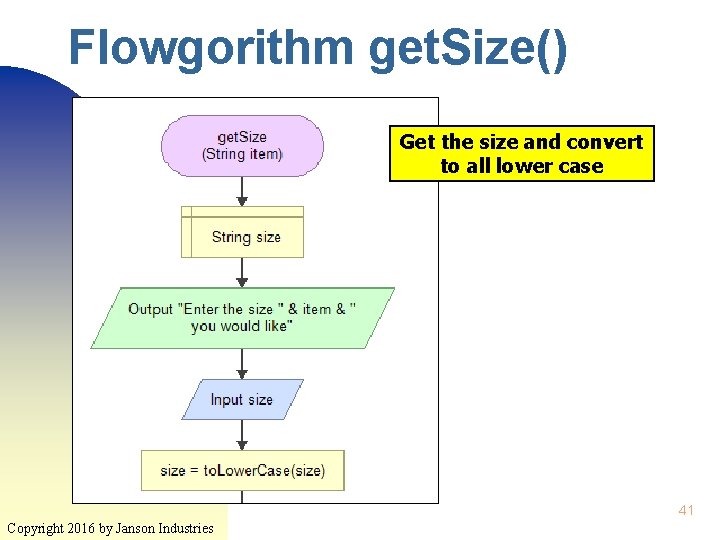
Flowgorithm get. Size() Get the size and convert to all lower case 41 Copyright 2016 by Janson Industries
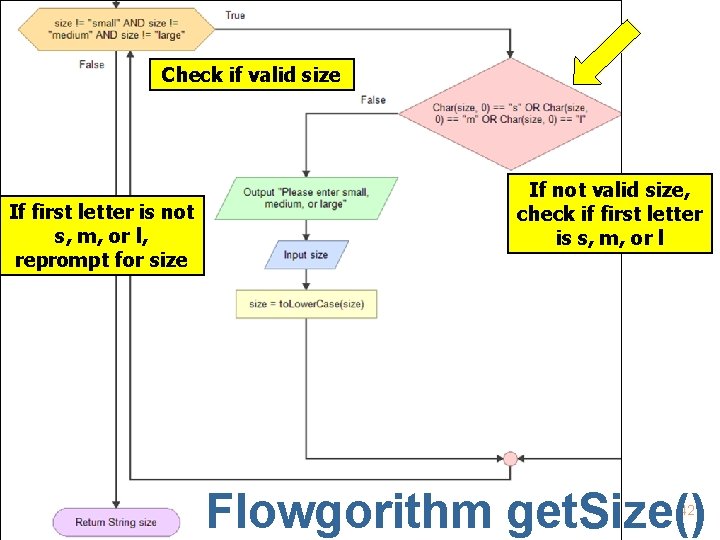
Check if valid size If not valid size, check if first letter is s, m, or l If first letter is not s, m, or l, reprompt for size Flowgorithm get. Size() 42 Copyright 2016 by Janson Industries
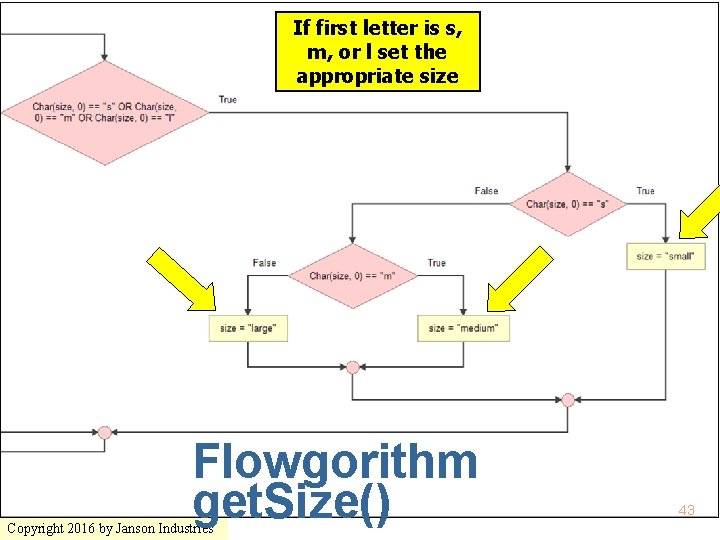
If first letter is s, m, or l set the appropriate size Flowgorithm get. Size() Copyright 2016 by Janson Industries 43
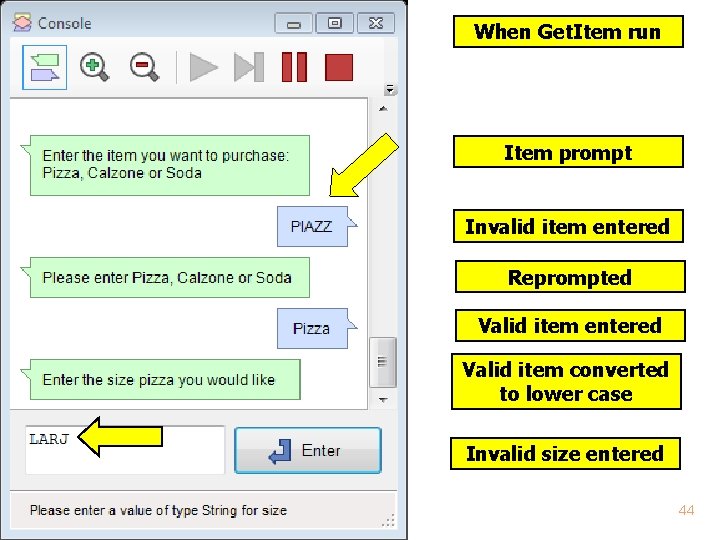
When Get. Item run Item prompt Invalid item entered Reprompted Valid item entered Valid item converted to lower case Invalid size entered 44 Copyright 2016 by Janson Industries
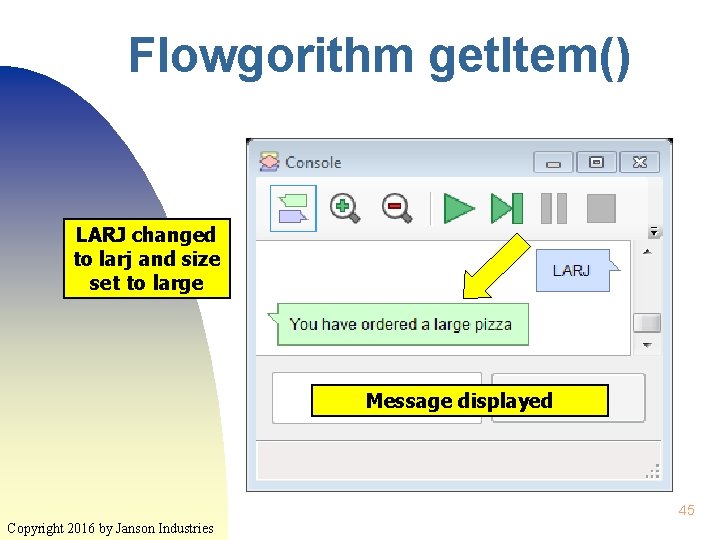
Flowgorithm get. Item() LARJ changed to larj and size set to large Message displayed 45 Copyright 2016 by Janson Industries
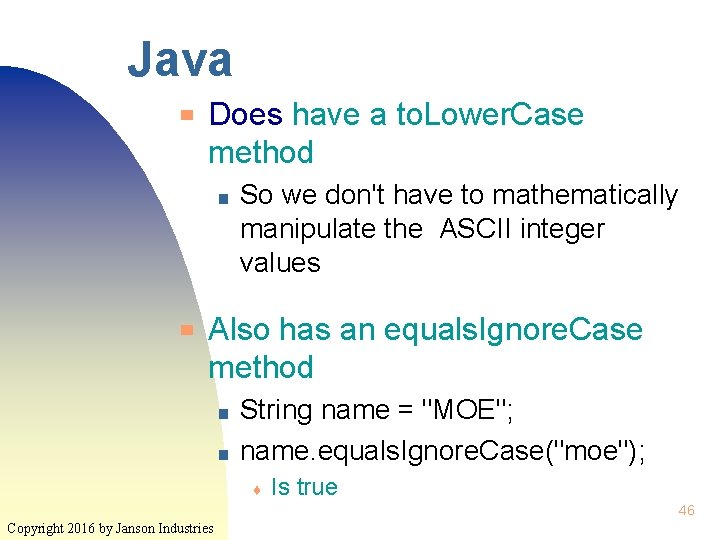
Java ▀ Does have a to. Lower. Case method ■ ▀ So we don't have to mathematically manipulate the ASCII integer values Also has an equals. Ignore. Case method ■ ■ String name = "MOE"; name. equals. Ignore. Case("moe"); ♦ Is true 46 Copyright 2016 by Janson Industries
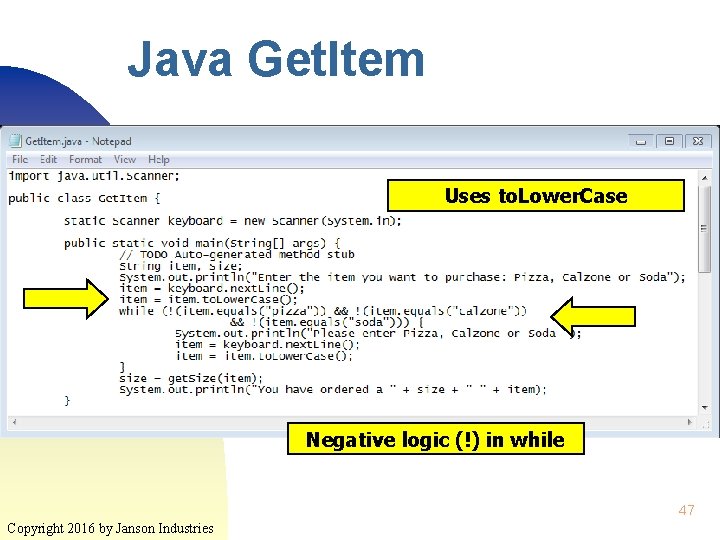
Java Get. Item Uses to. Lower. Case Negative logic (!) in while 47 Copyright 2016 by Janson Industries
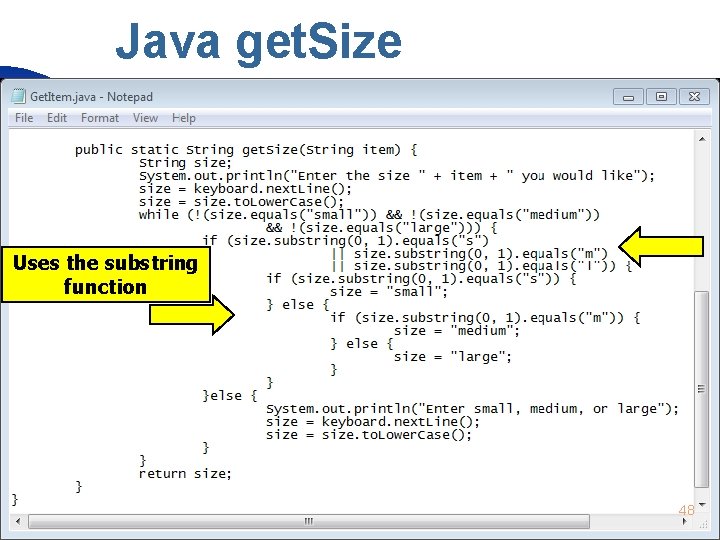
Java get. Size Uses the substring function 48 Copyright 2016 by Janson Industries
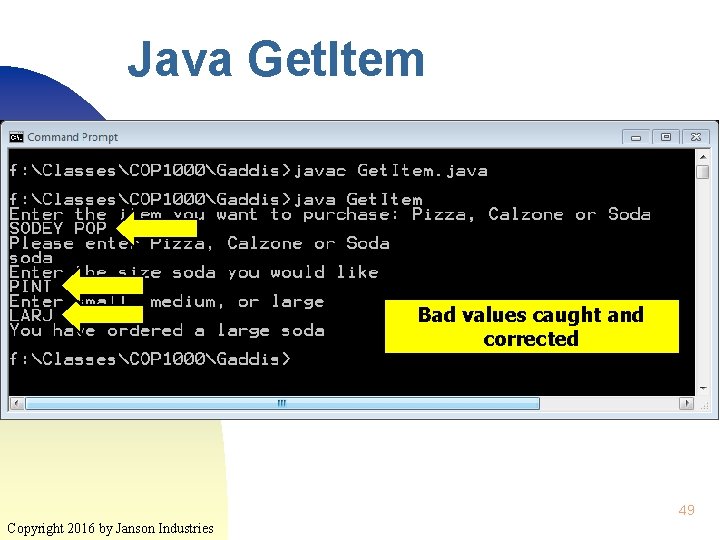
Java Get. Item Bad values caught and corrected 49 Copyright 2016 by Janson Industries
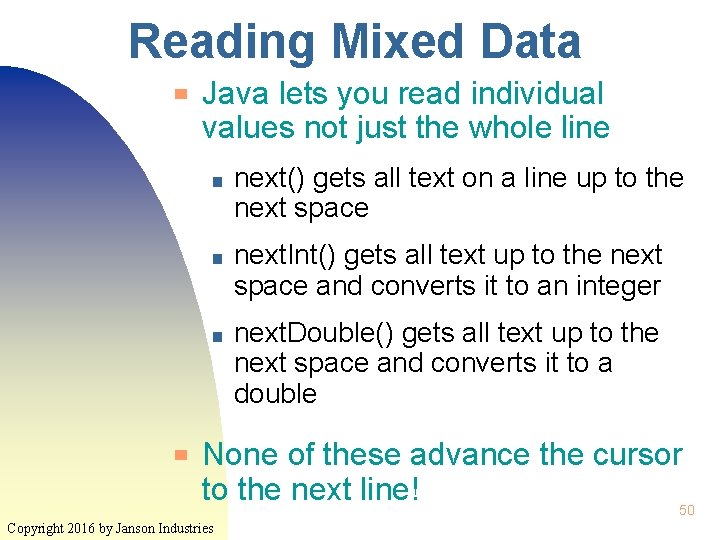
Reading Mixed Data ▀ ▀ Java lets you read individual values not just the whole line ■ next() gets all text on a line up to the next space ■ next. Int() gets all text up to the next space and converts it to an integer ■ next. Double() gets all text up to the next space and converts it to a double None of these advance the cursor to the next line! 50 Copyright 2016 by Janson Industries
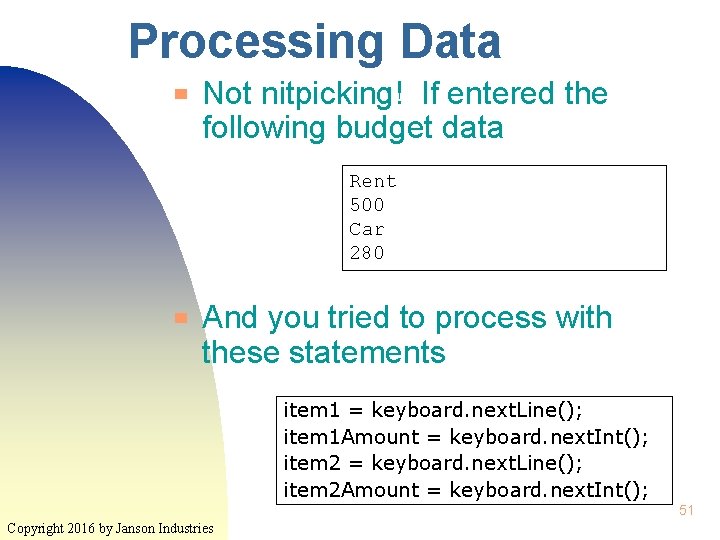
Processing Data ▀ Not nitpicking! If entered the following budget data Rent 500 Car 280 ▀ And you tried to process with these statements item 1 = keyboard. next. Line(); item 1 Amount = keyboard. next. Int(); item 2 = keyboard. next. Line(); item 2 Amount = keyboard. next. Int(); 51 Copyright 2016 by Janson Industries
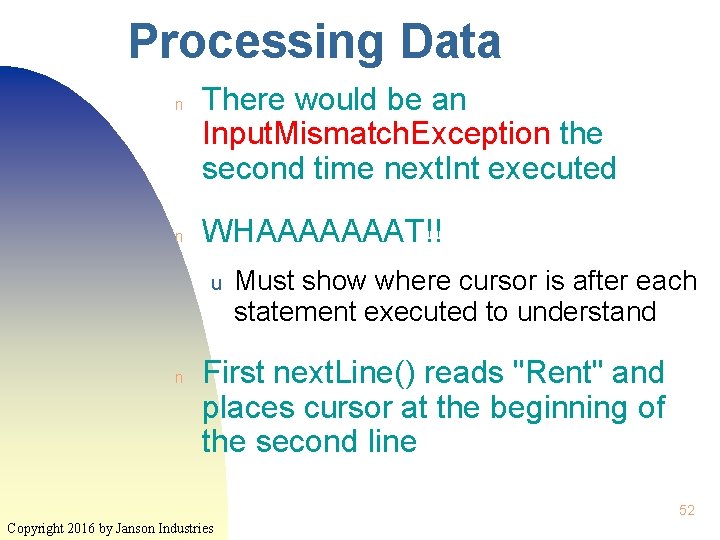
Processing Data n n There would be an Input. Mismatch. Exception the second time next. Int executed WHAAAAAAAT!! u n Must show where cursor is after each statement executed to understand First next. Line() reads "Rent" and places cursor at the beginning of the second line 52 Copyright 2016 by Janson Industries
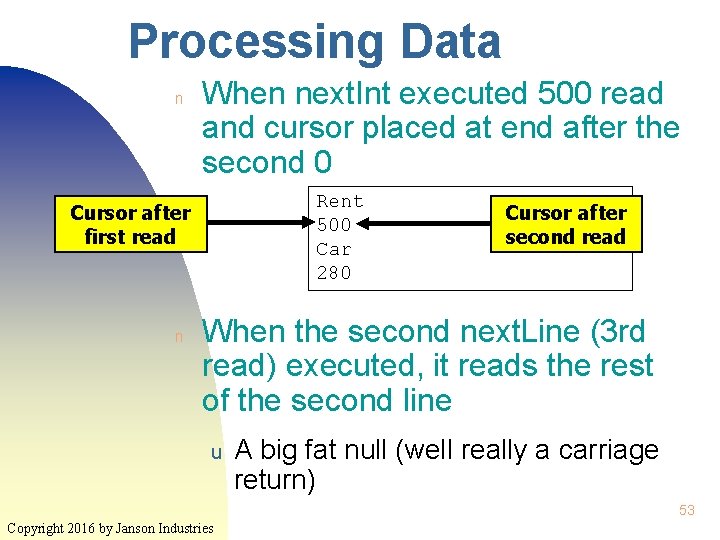
Processing Data n When next. Int executed 500 read and cursor placed at end after the second 0 Rent 500 Car 280 Cursor after first read n Cursor after second read When the second next. Line (3 rd read) executed, it reads the rest of the second line u A big fat null (well really a carriage return) 53 Copyright 2016 by Janson Industries
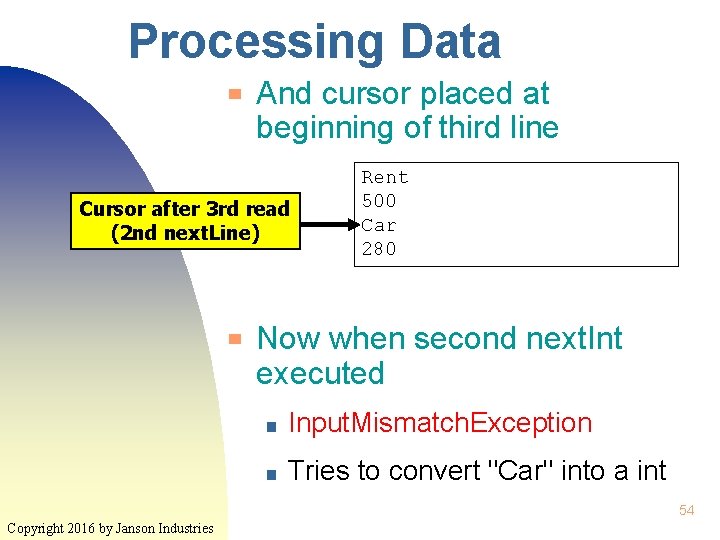
Processing Data ▀ And cursor placed at beginning of third line Cursor after 3 rd read (2 nd next. Line) ▀ Rent 500 Car 280 Now when second next. Int executed ■ Input. Mismatch. Exception ■ Tries to convert "Car" into a int 54 Copyright 2016 by Janson Industries
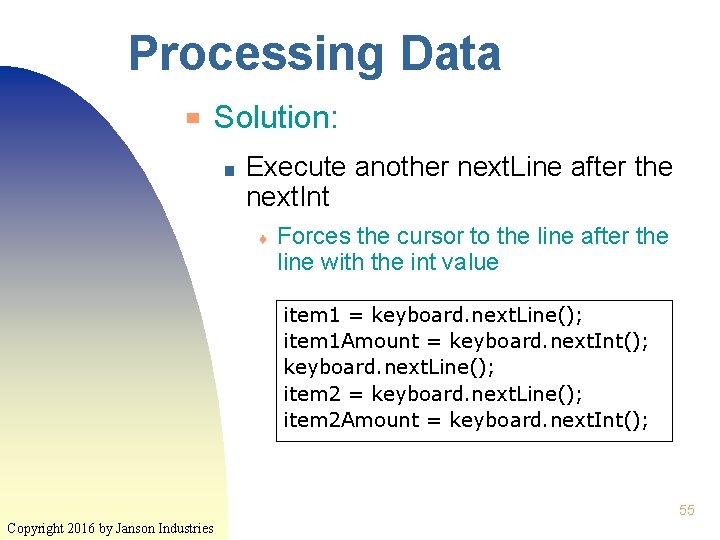
Processing Data ▀ Solution: ■ Execute another next. Line after the next. Int ♦ Forces the cursor to the line after the line with the int value item 1 = keyboard. next. Line(); item 1 Amount = keyboard. next. Int(); keyboard. next. Line(); item 2 = keyboard. next. Line(); item 2 Amount = keyboard. next. Int(); 55 Copyright 2016 by Janson Industries
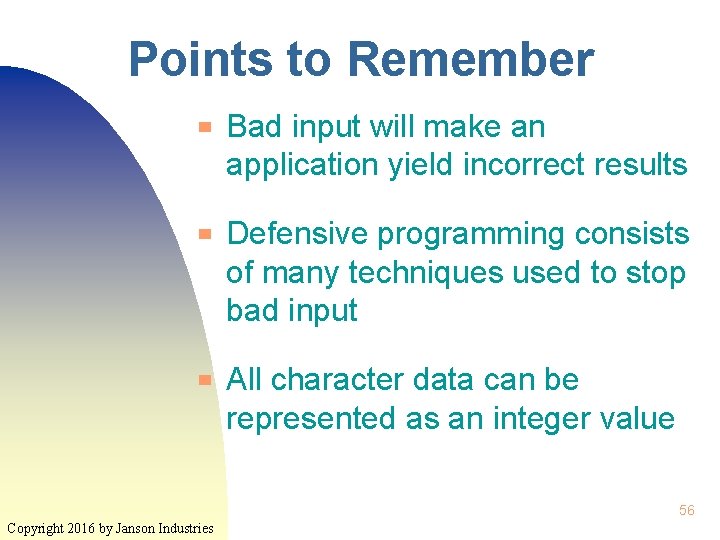
Points to Remember ▀ ▀ ▀ Bad input will make an application yield incorrect results Defensive programming consists of many techniques used to stop bad input All character data can be represented as an integer value 56 Copyright 2016 by Janson Industries
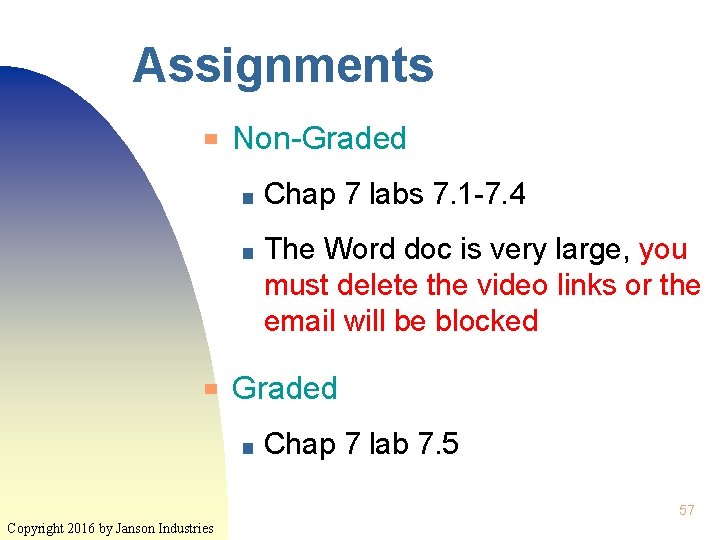
Assignments ▀ ▀ Non-Graded ■ Chap 7 labs 7. 1 -7. 4 ■ The Word doc is very large, you must delete the video links or the email will be blocked Graded ■ Chap 7 lab 7. 5 57 Copyright 2016 by Janson Industries
Assg main oü
Flowgorithm char
An input validation loop is sometimes called
User interface input and output design
Ist spring design and validation
A nested model for visualization design and validation
Rhythm in floral design
Input output peripheral
Finely tuned input
Perbedaan linear programming dan integer programming
Greedy programming vs dynamic programming
System programming definition
Linear vs integer programming
Definisi linear
Analysis of algorithms
Counting primitive operations
Dynamic programming
Genetic programming vs genetic algorithm
Genetic programming vs genetic algorithm
Elements of dynamic programming
Dynamic programming algorithm
Difference between a star and ao star
Sweep line algorithm codeforces
Guidelines for data entry screen design
Input design objectives
Objectives of input design
Forward reference table (frt) is arranged like ?
Modular design programming
Interior design programming
Modular design programming
Reliability design in dynamic programming
Akamai waf bypass
Is unit testing verification or validation
Validationrule wpf
Concurrent validation
Moderation and validation of assessment
Twic nexgen
Struts validation
Protocol for process validation
Arelle software
Method verification vs validation
Method verification vs validation
Material obligation
Why we use validation set
Jsf validation
Black box and white box testing
Integrative validation definition
Software validation example
Validation nach richard
Check digit
Jocelyn barker
4 benaderingswijze dementie
Business process validation
Verification and validation
Frnti
Clinical validation denials
Validation authority
Sam j. levine