Intro to Programming Algorithm Design Input Processing and
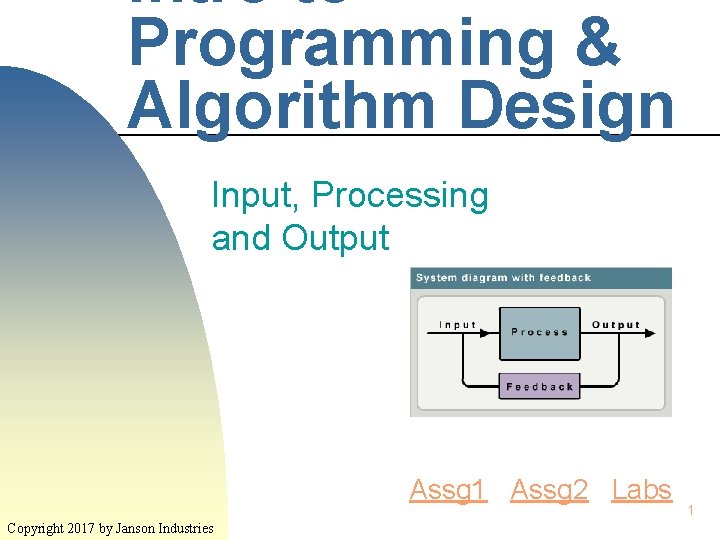
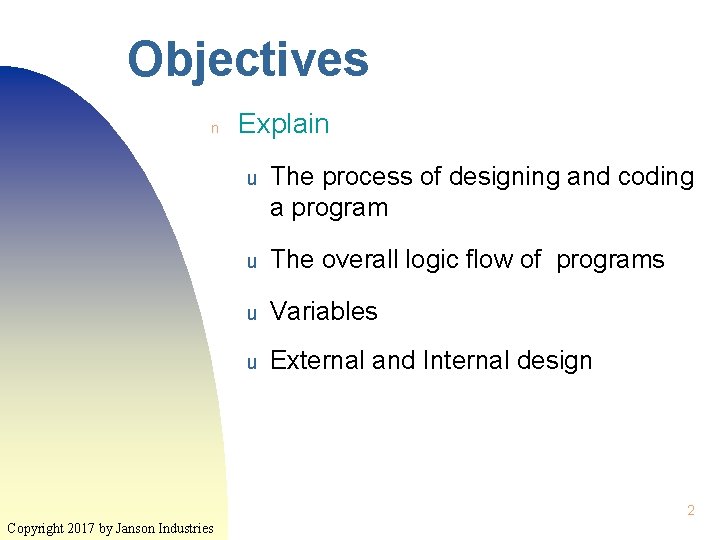
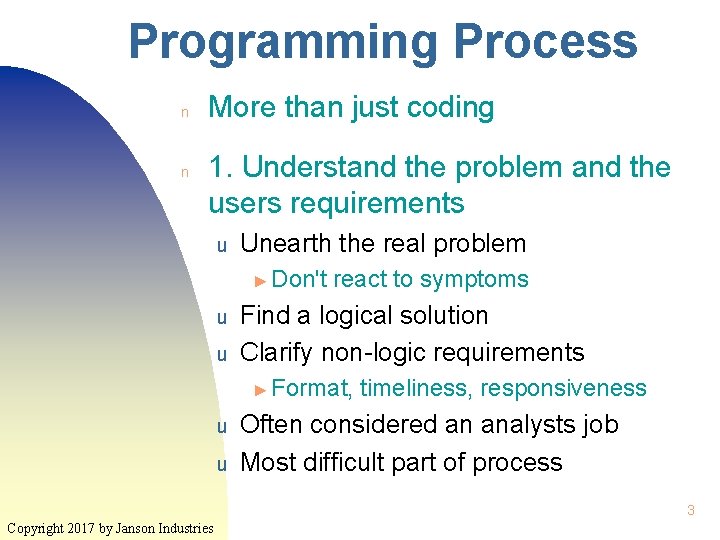
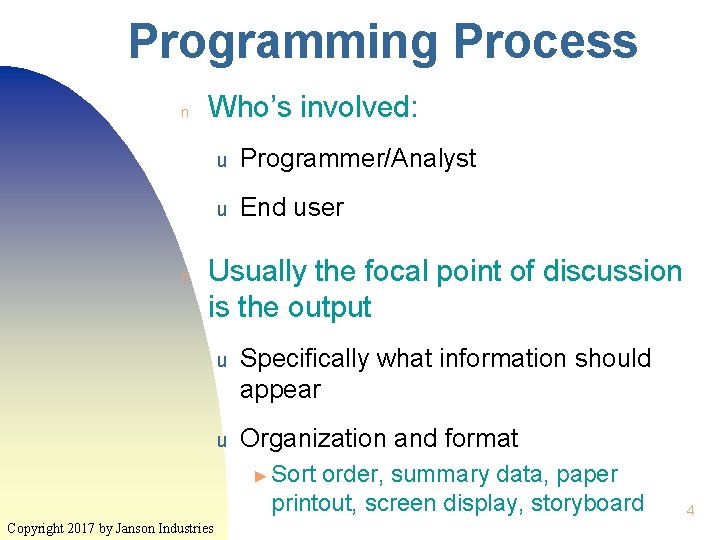
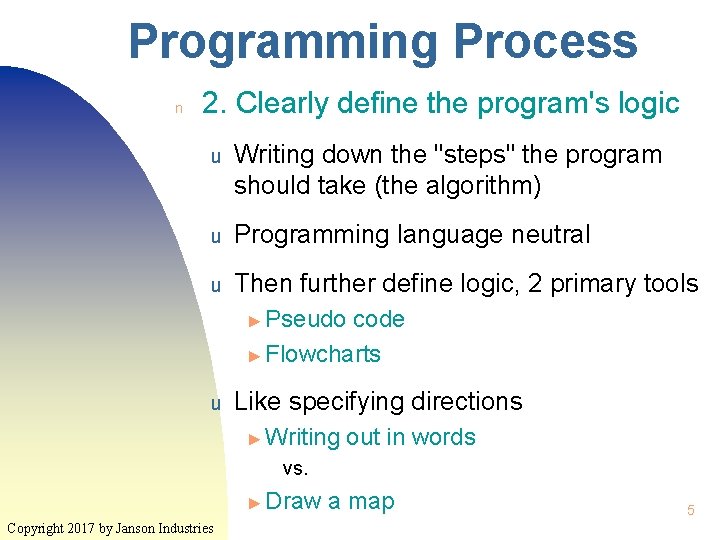
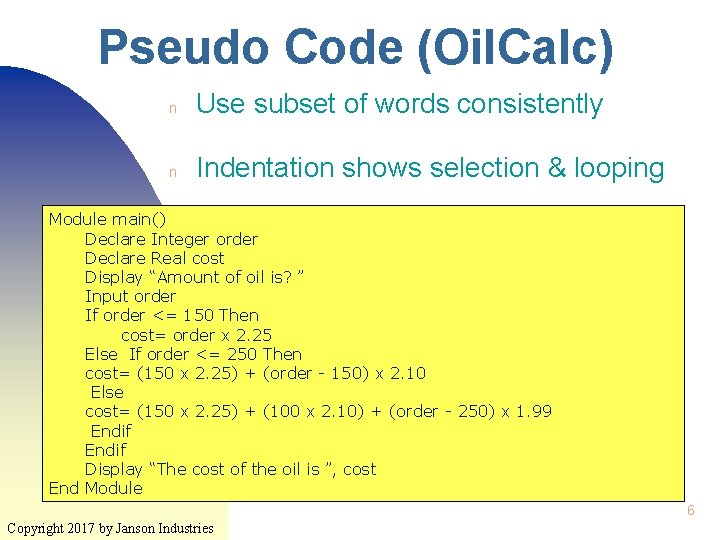
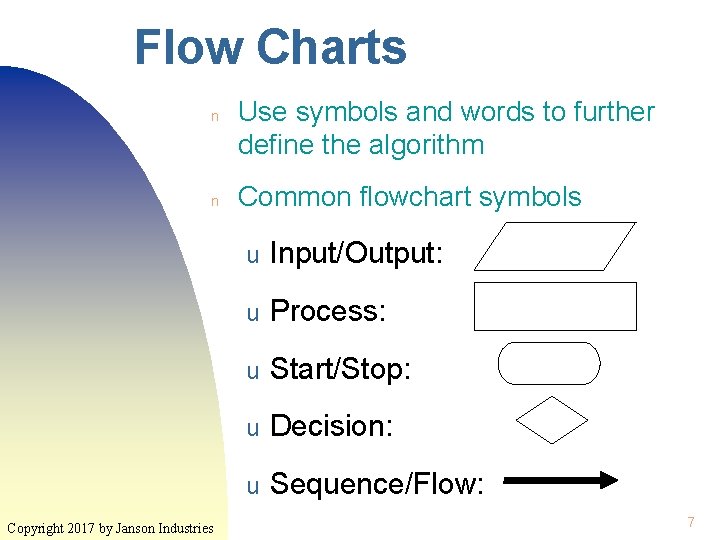
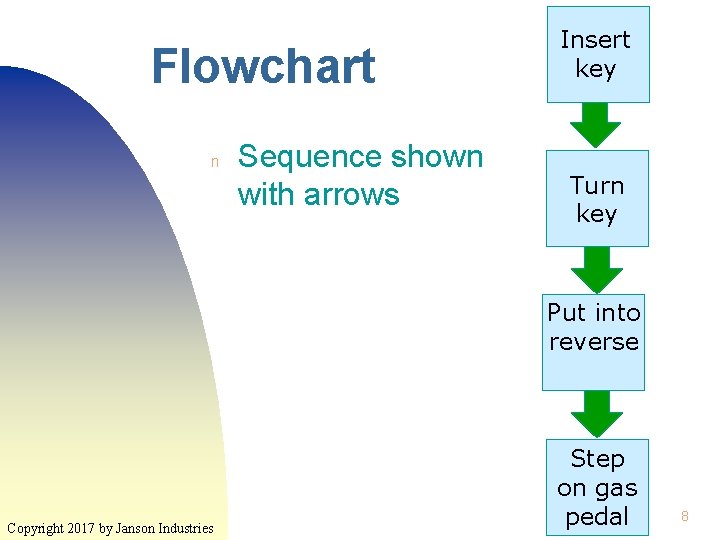
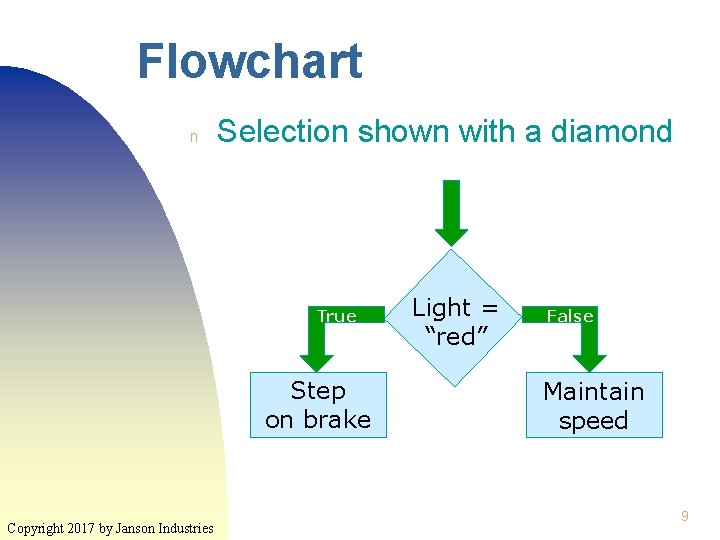
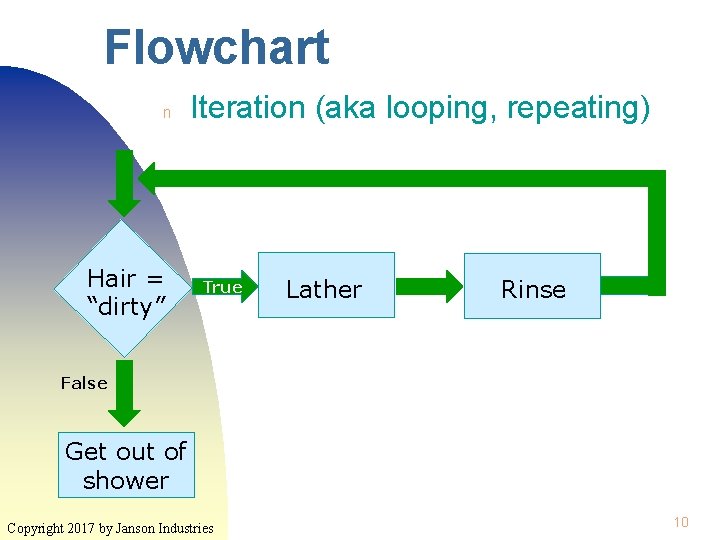
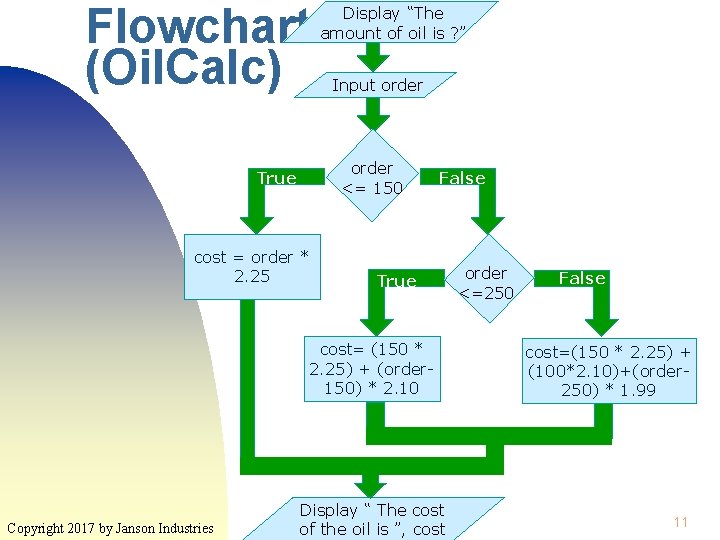
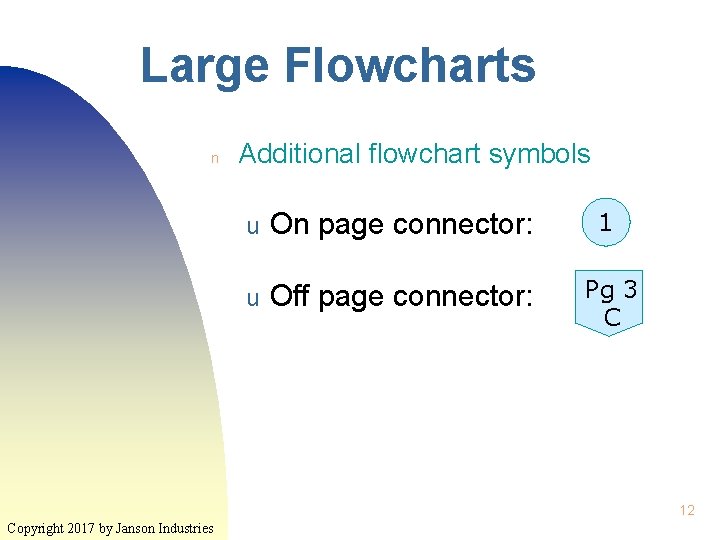
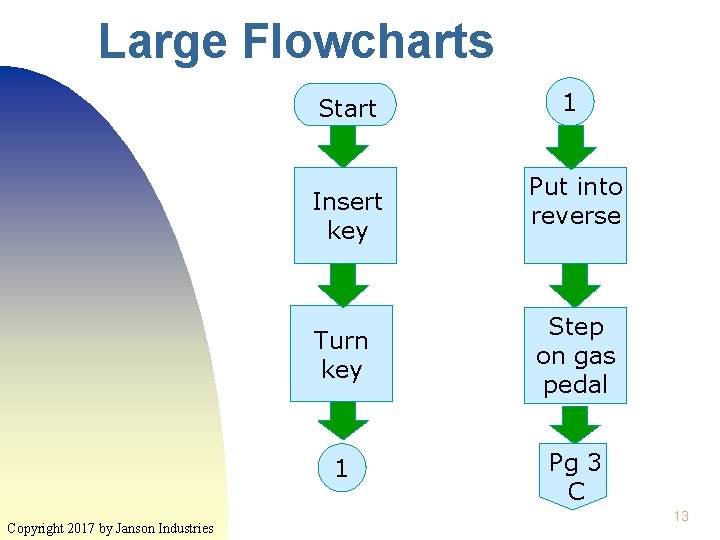
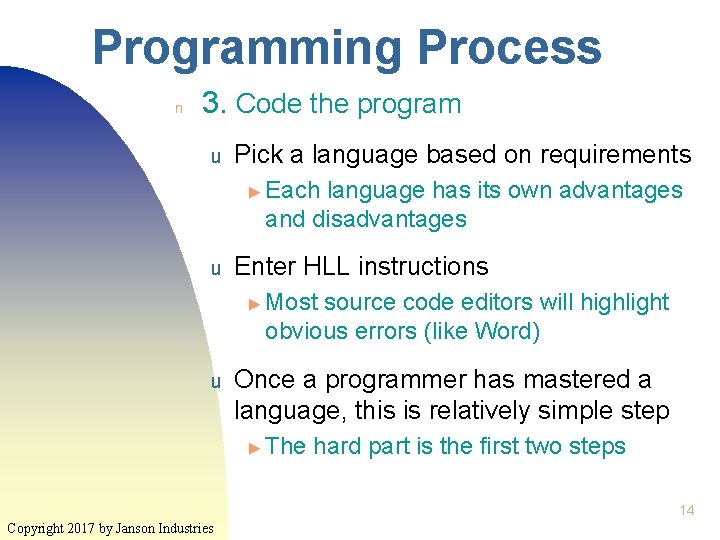
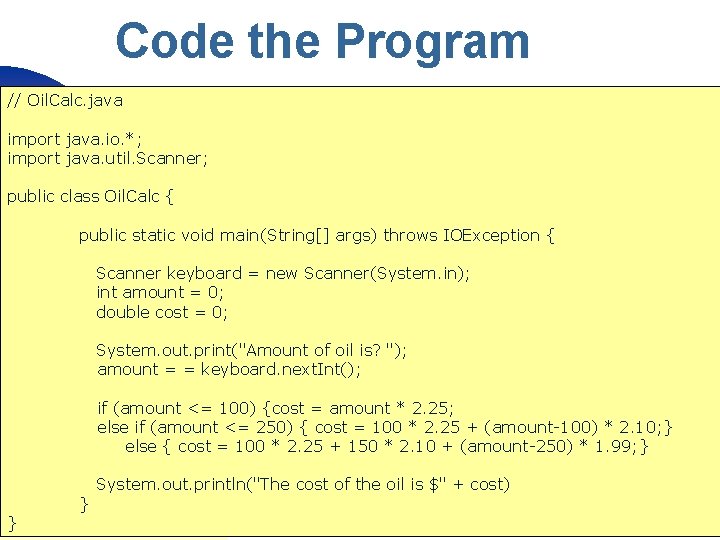
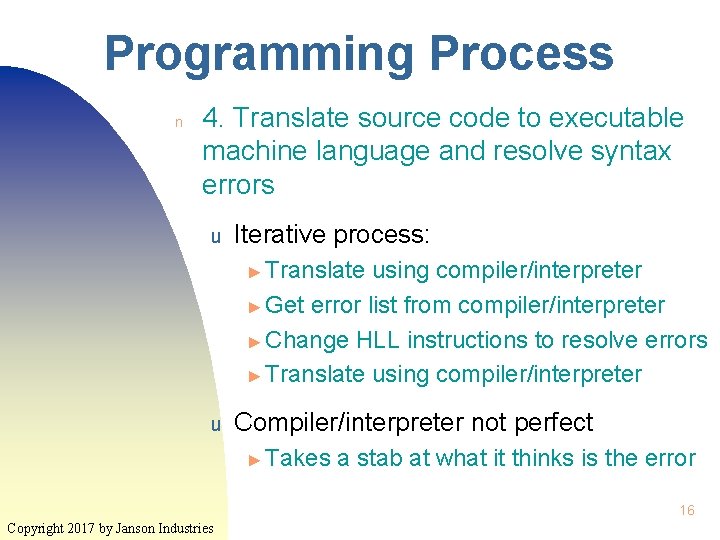
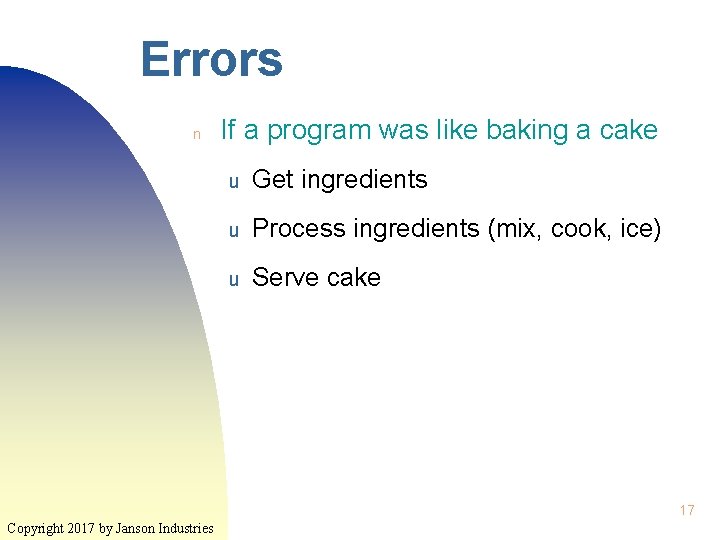
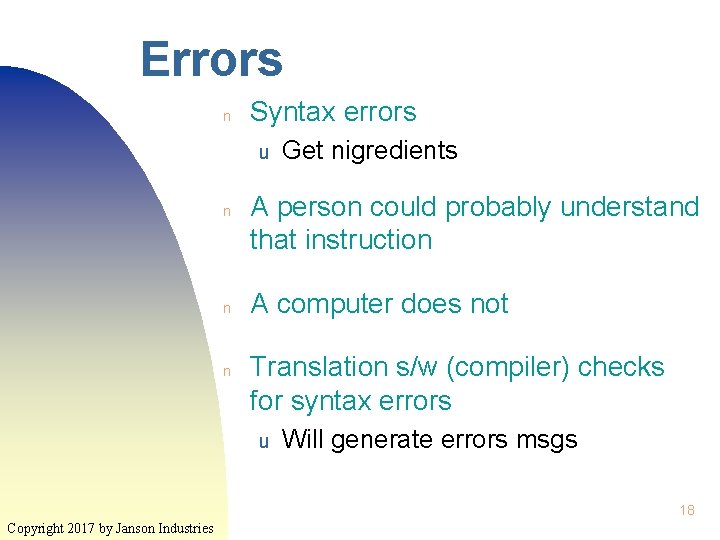
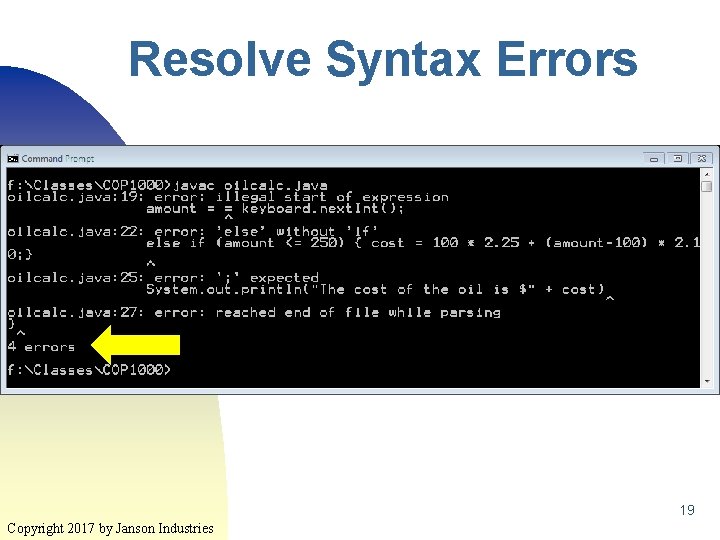
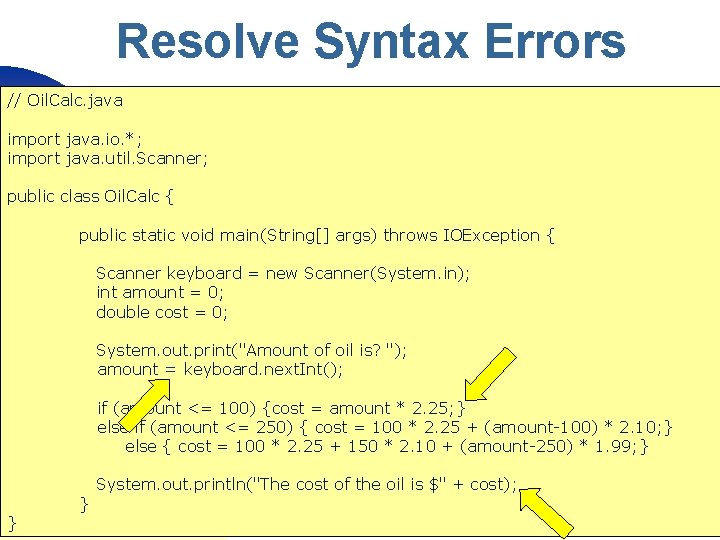
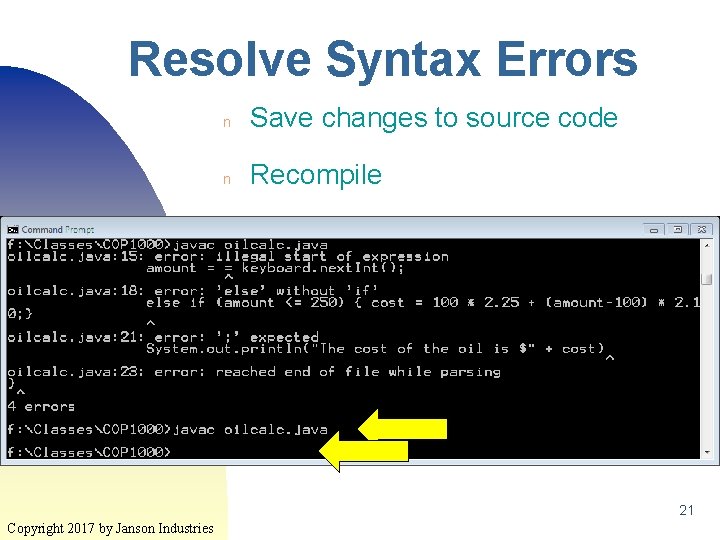
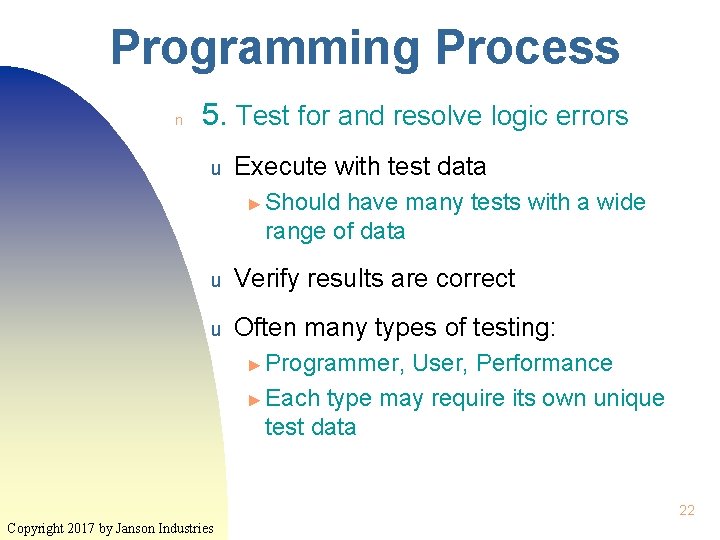
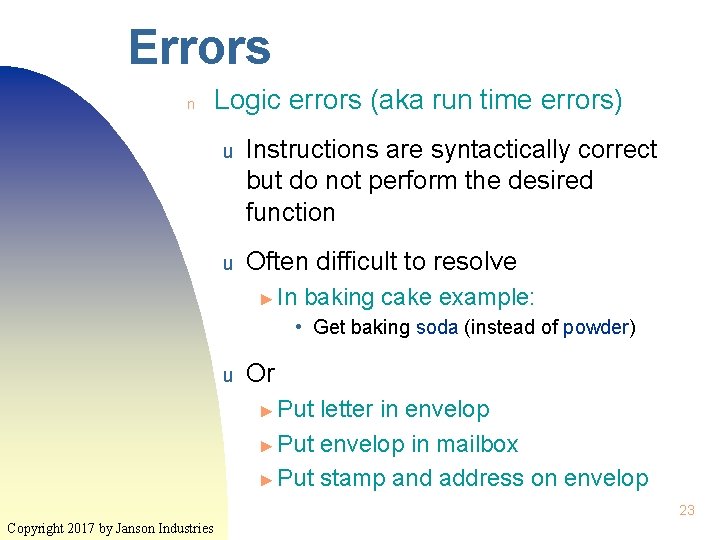
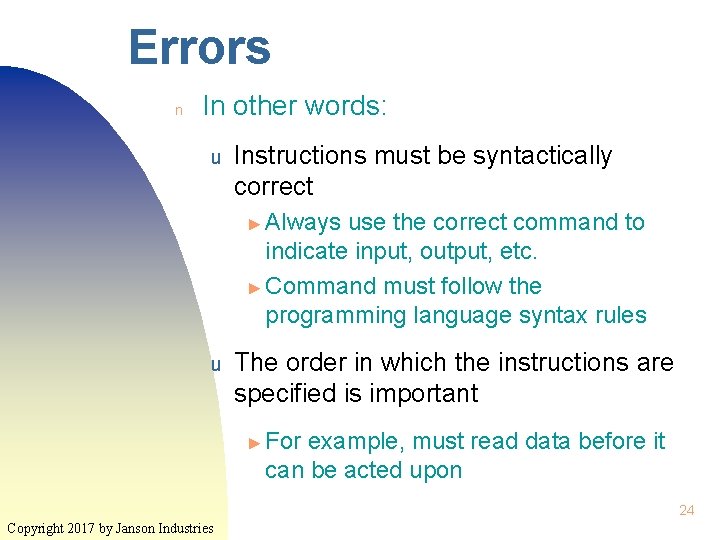
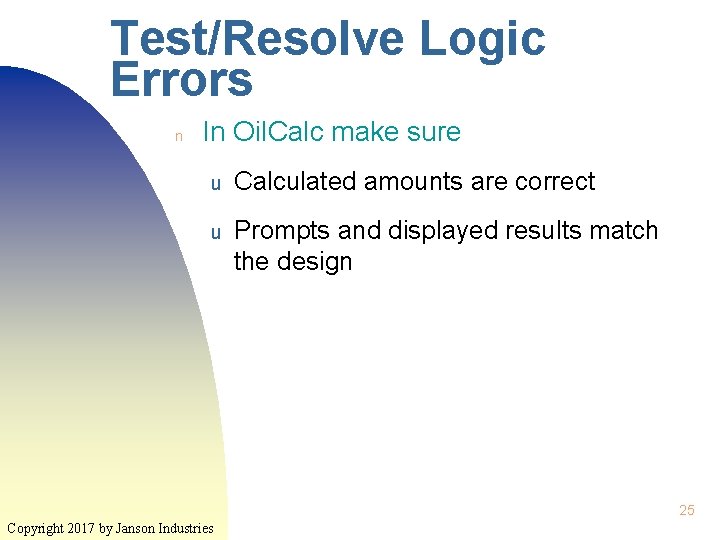
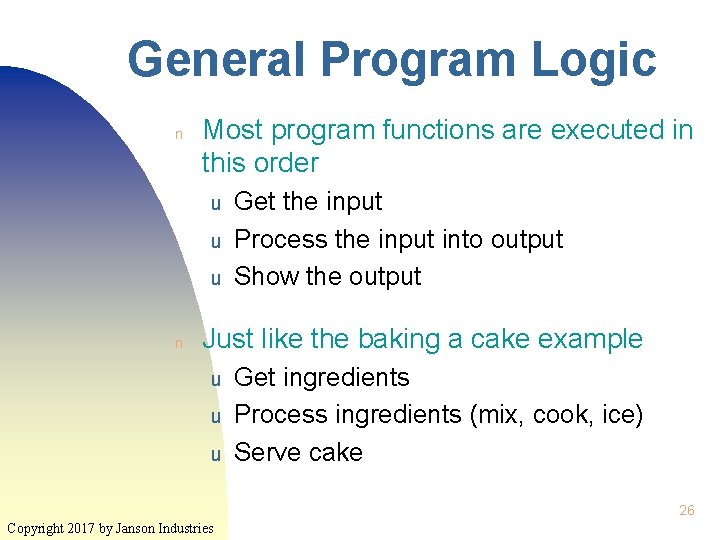
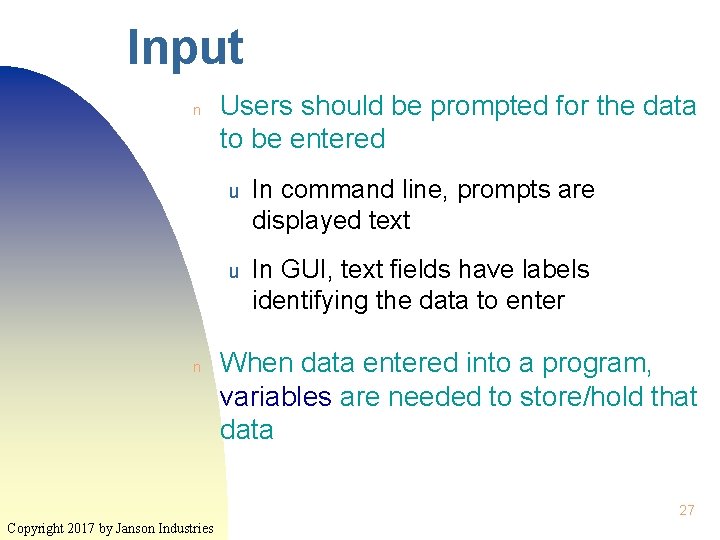
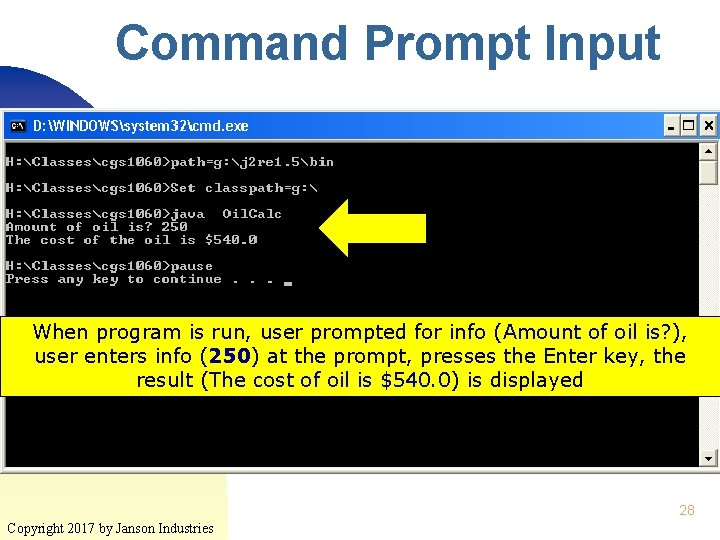
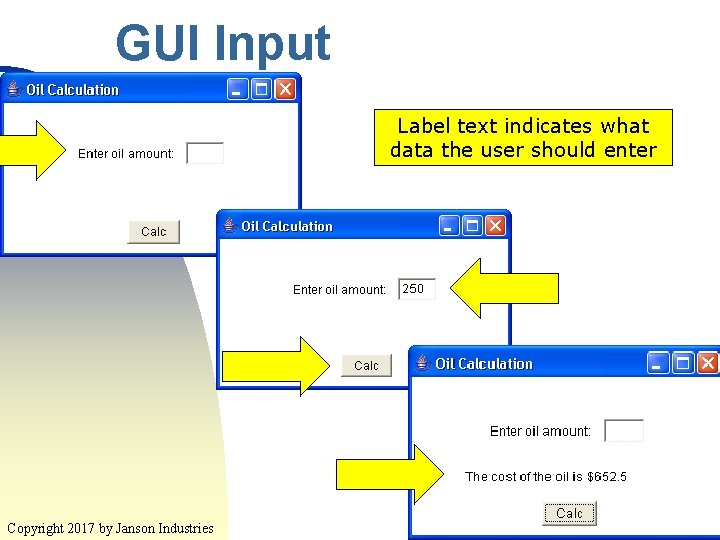
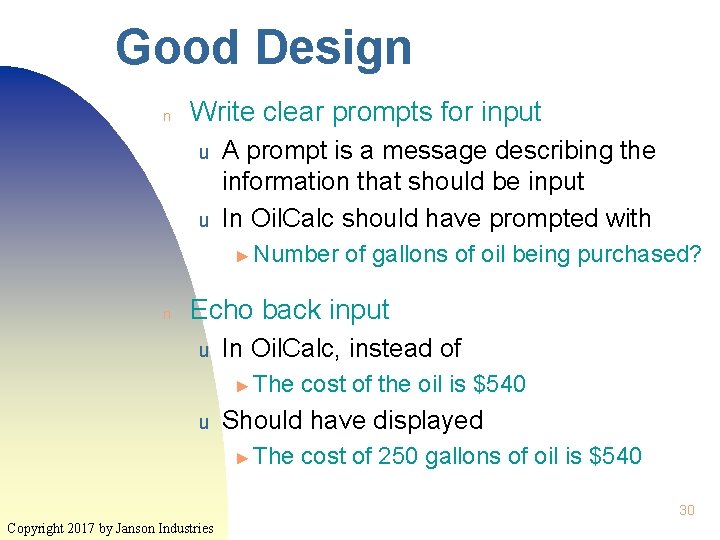
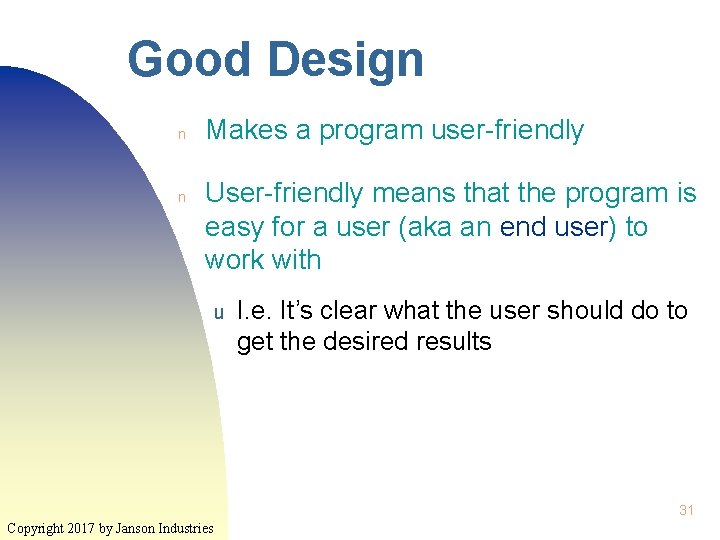
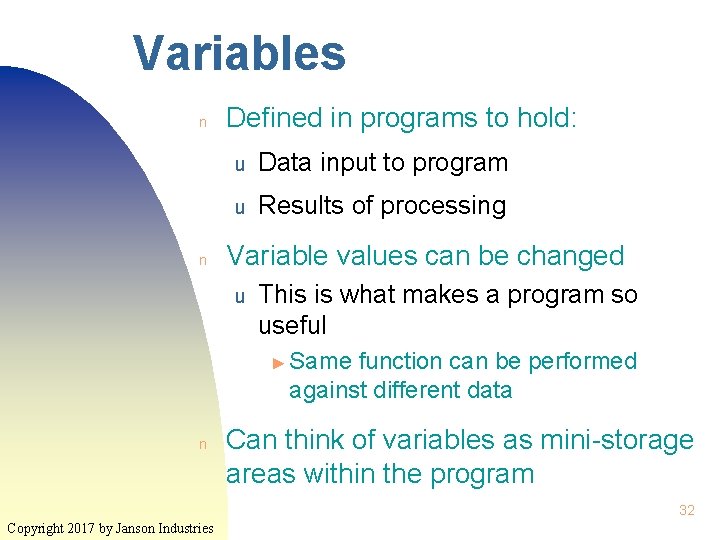
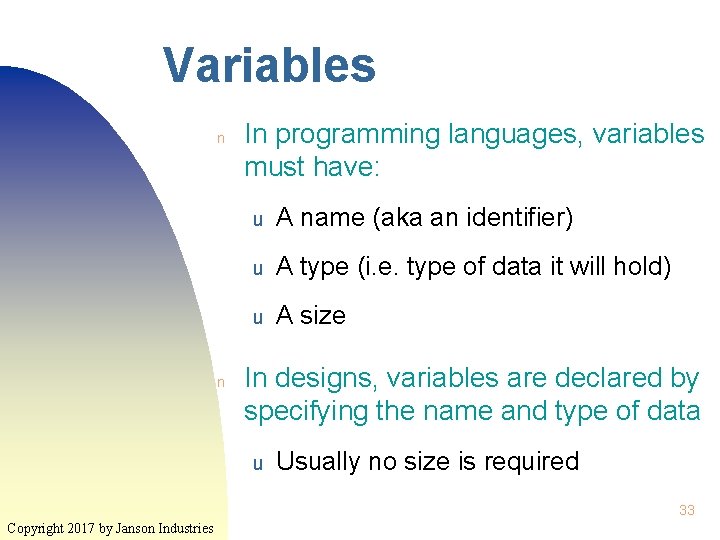
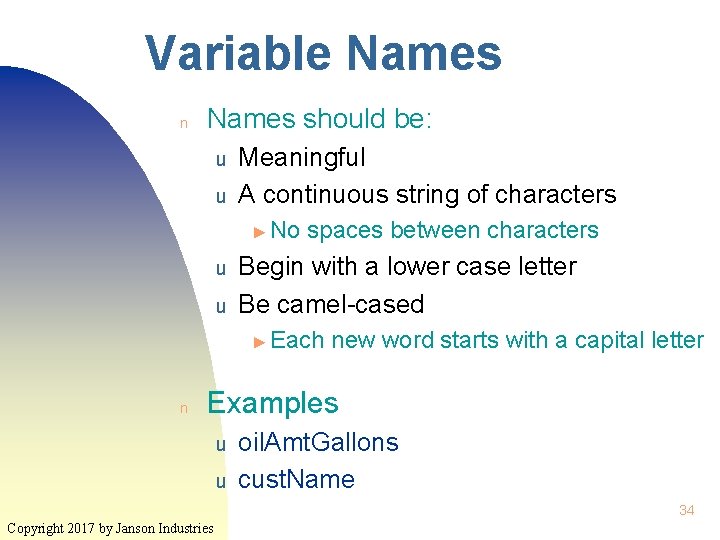
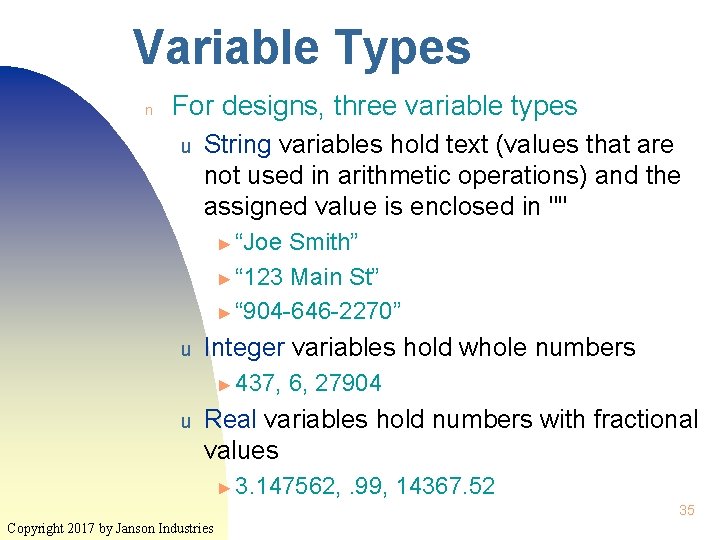
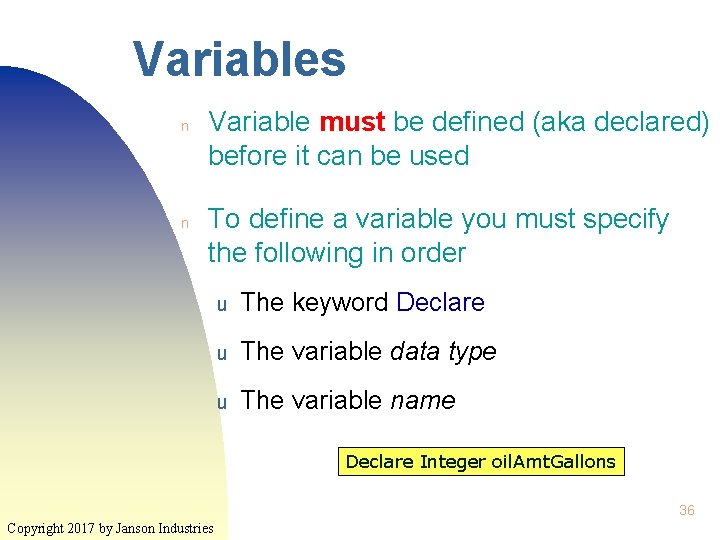
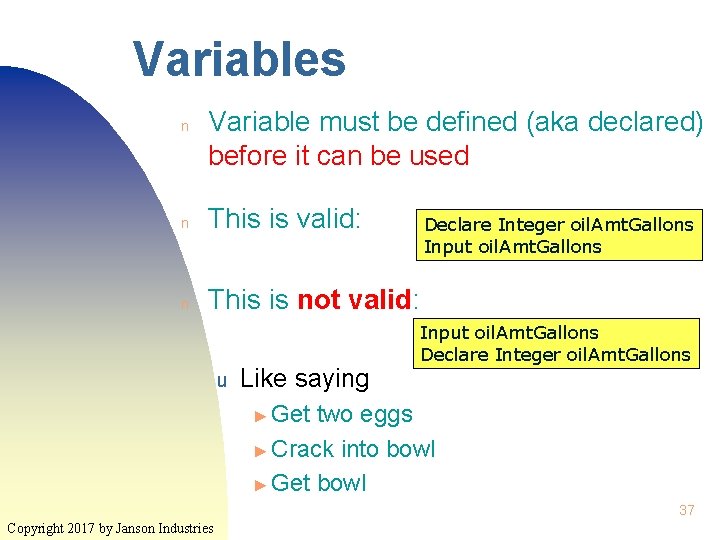
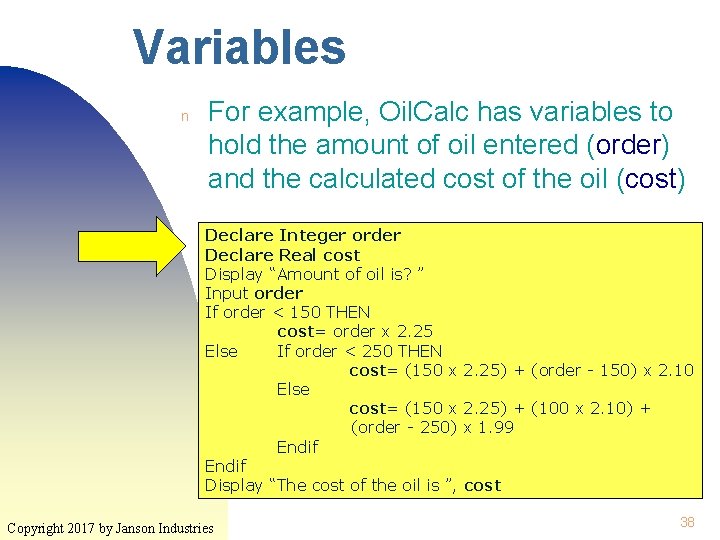
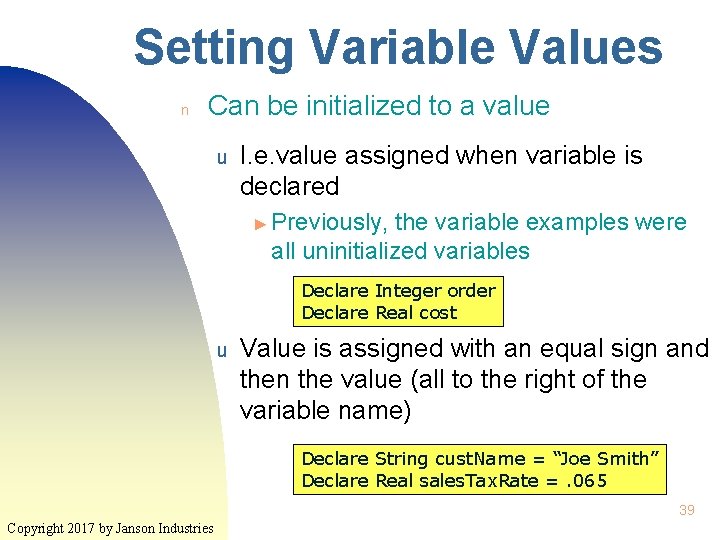
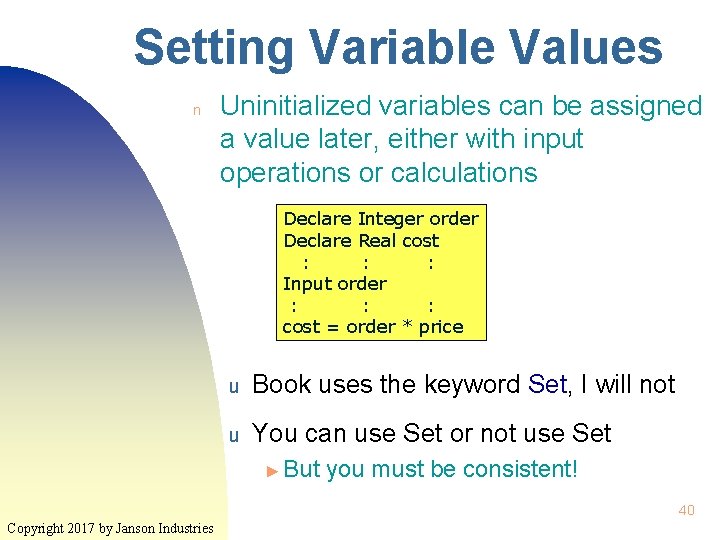
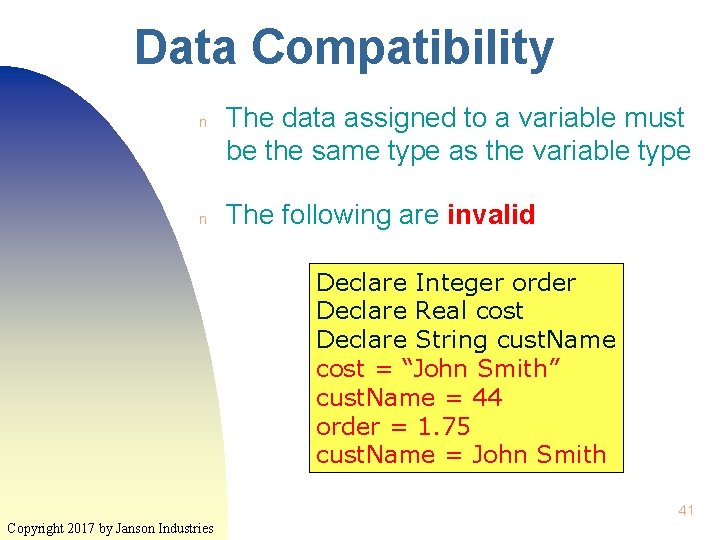
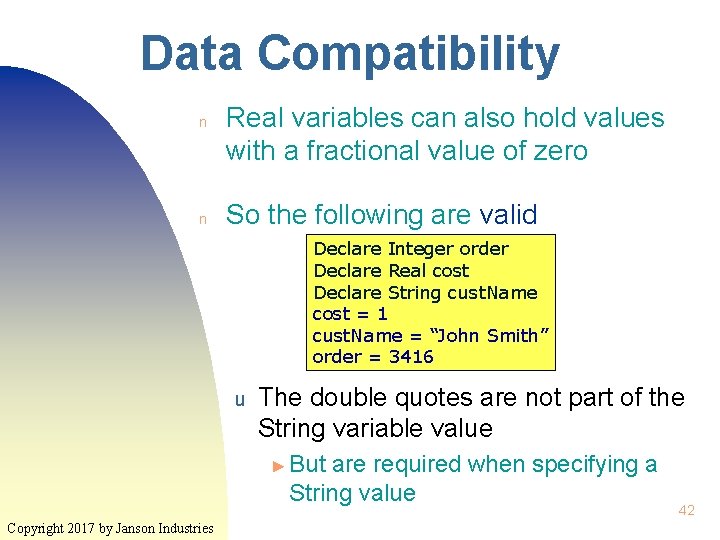
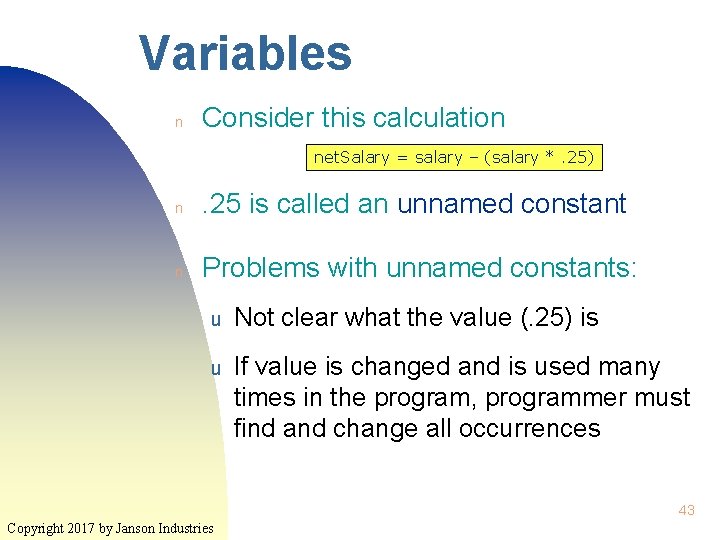
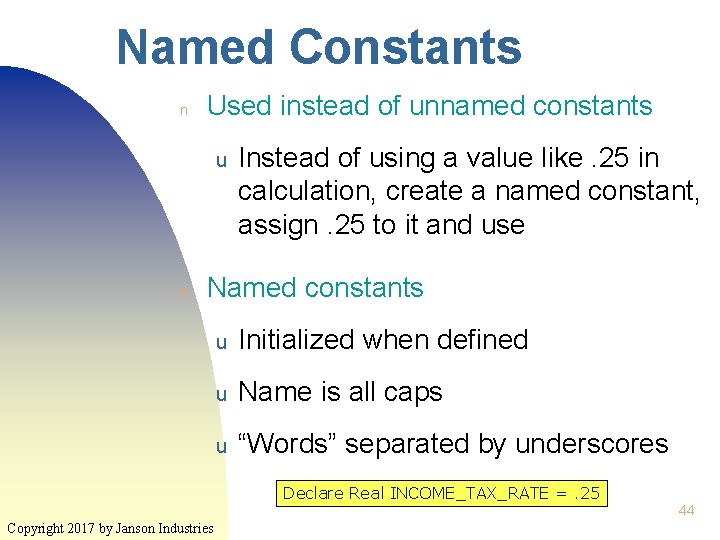
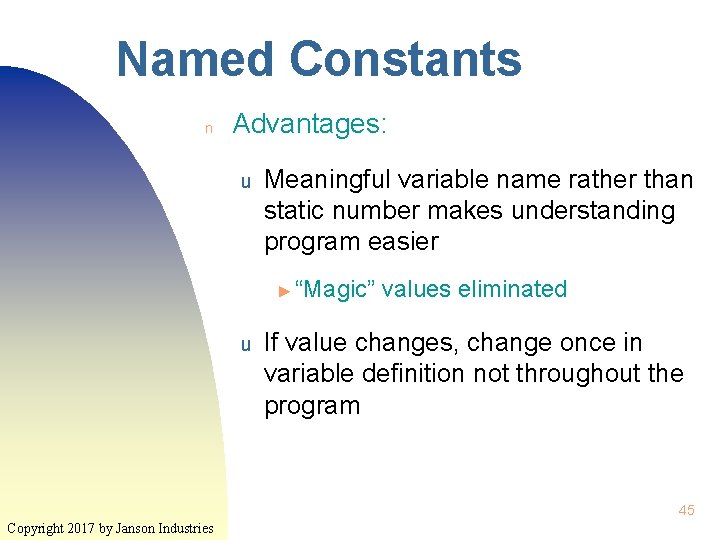
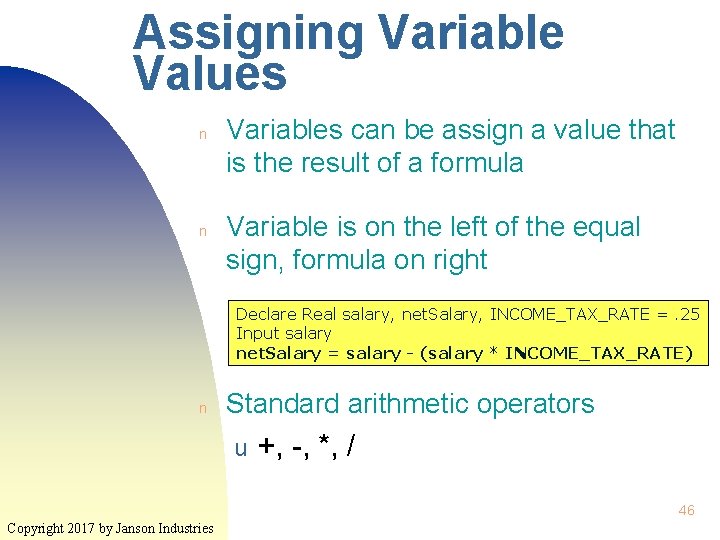
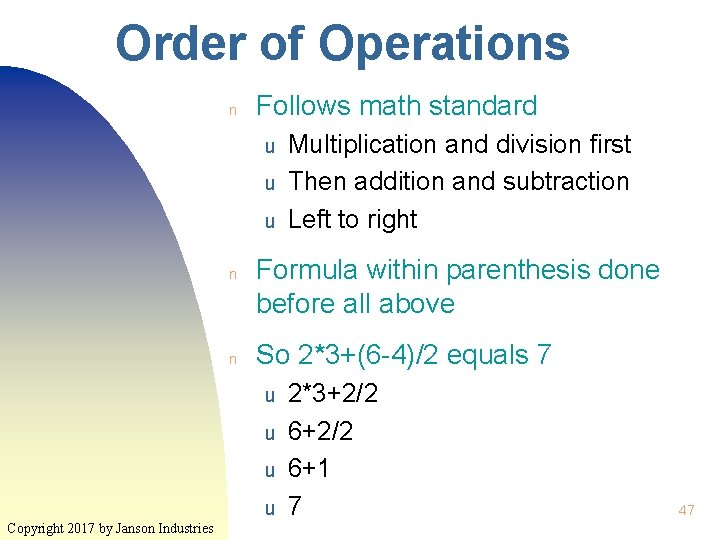
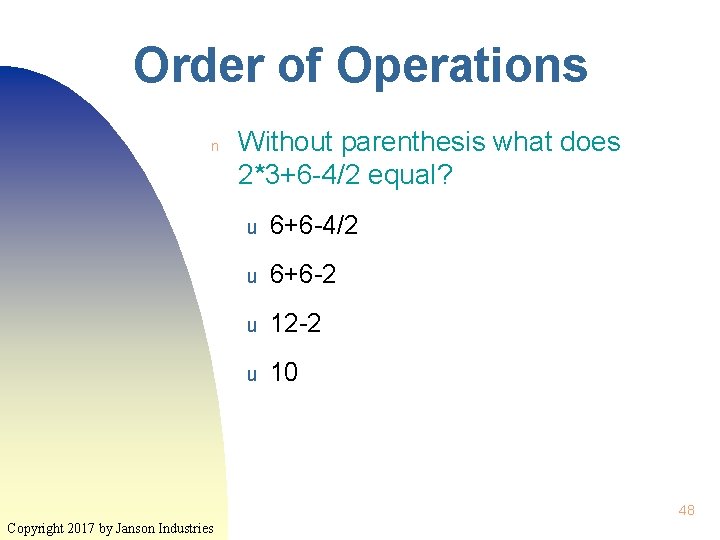
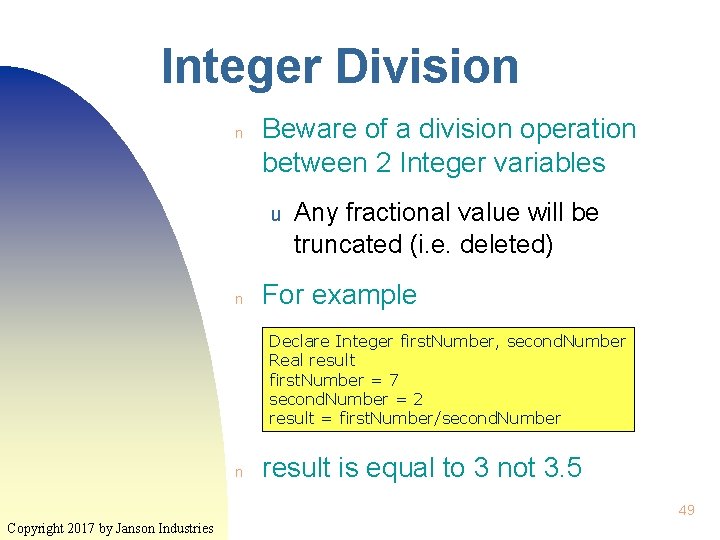
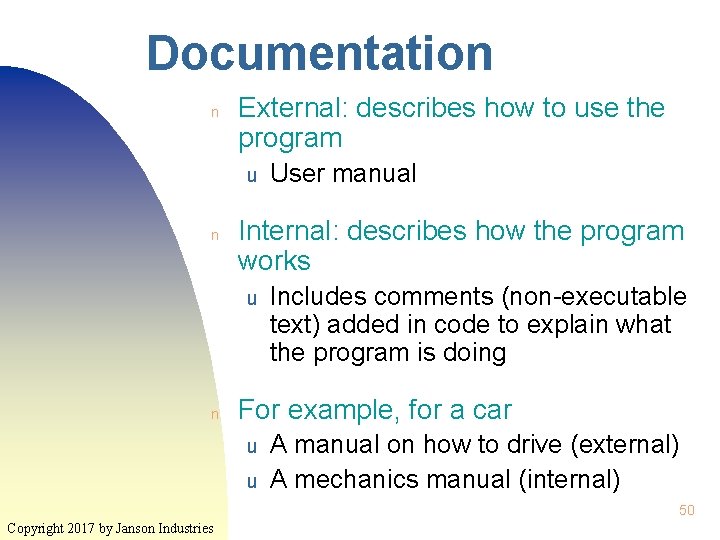
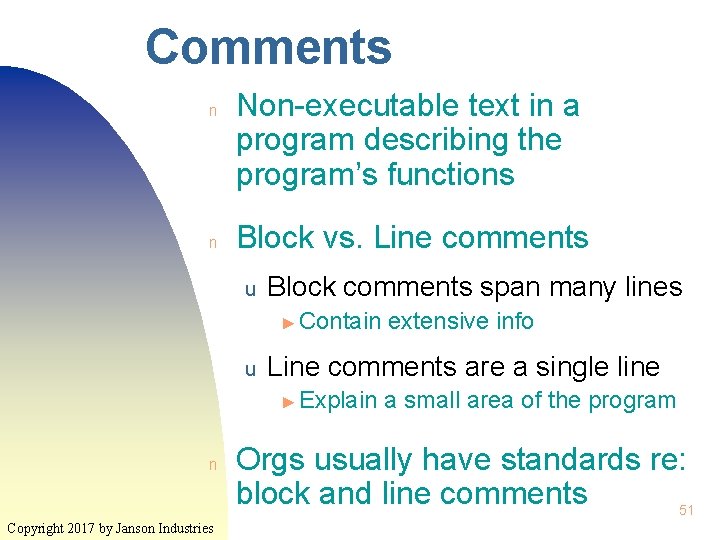
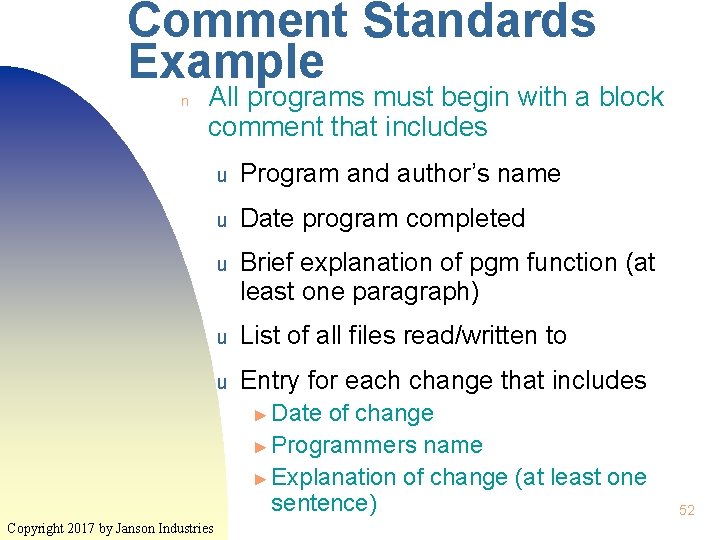
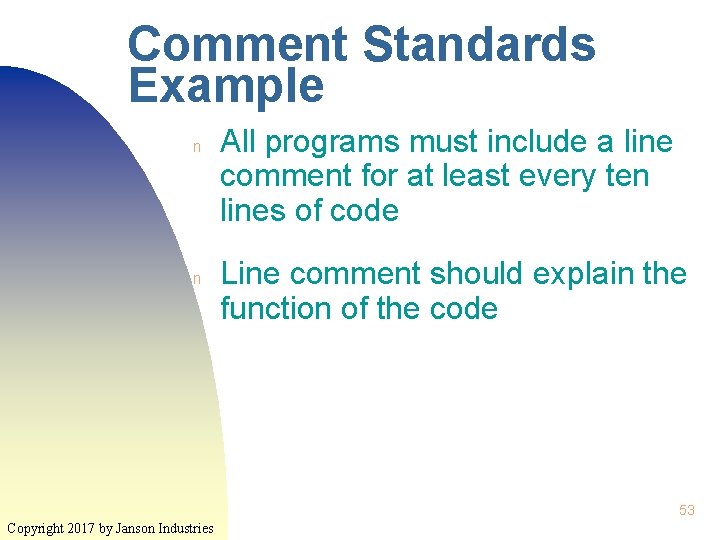
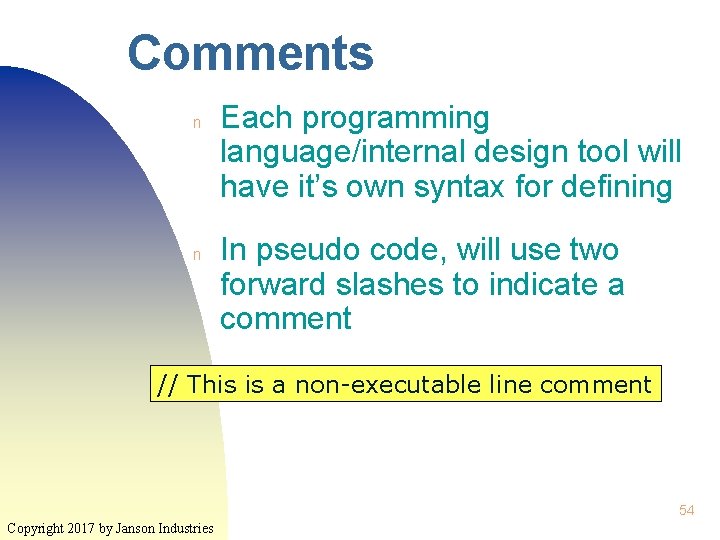
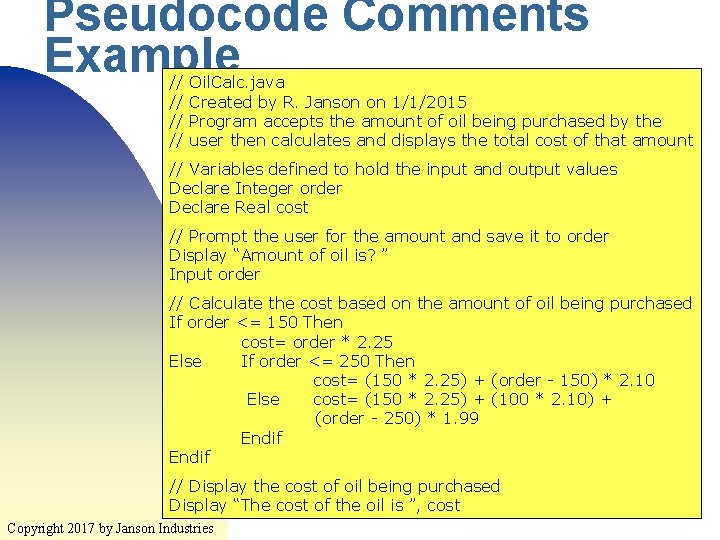
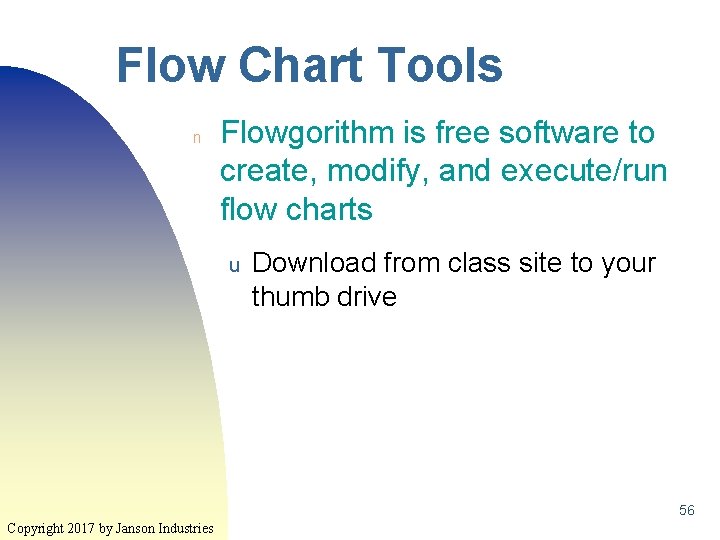
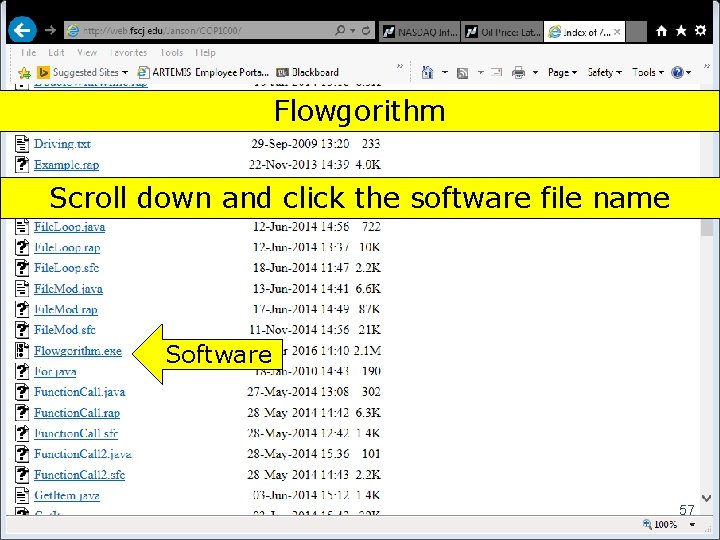
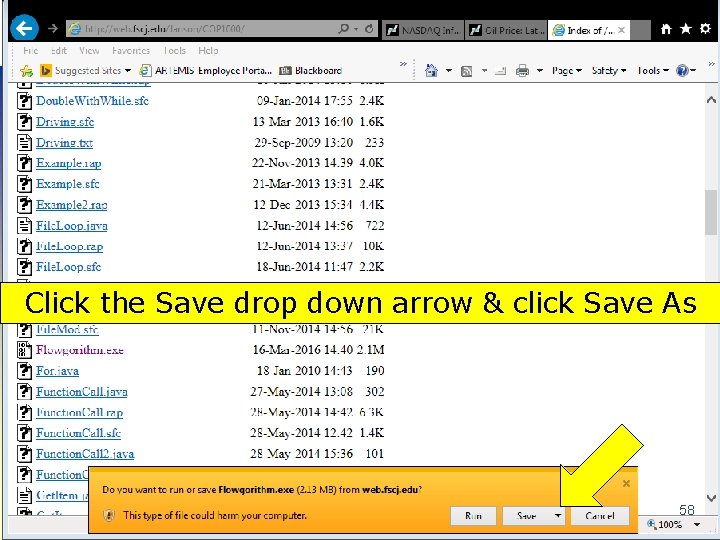
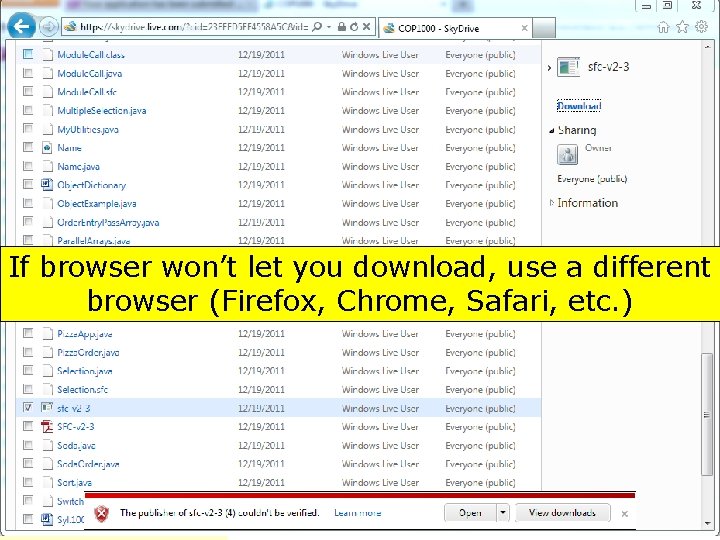
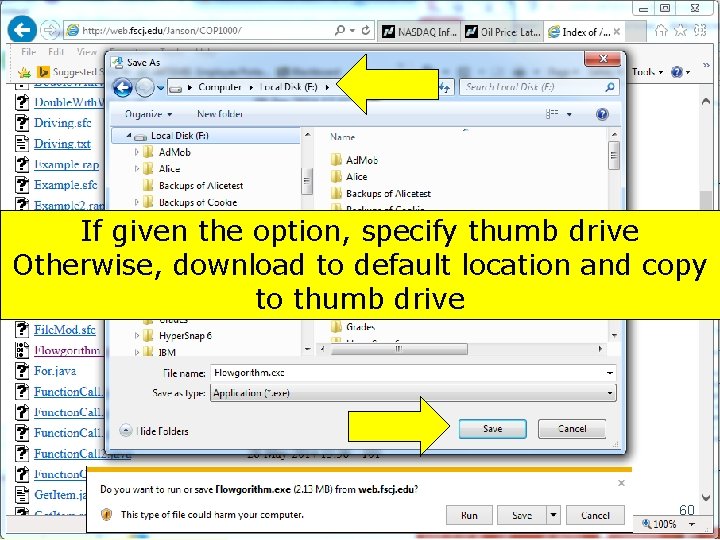
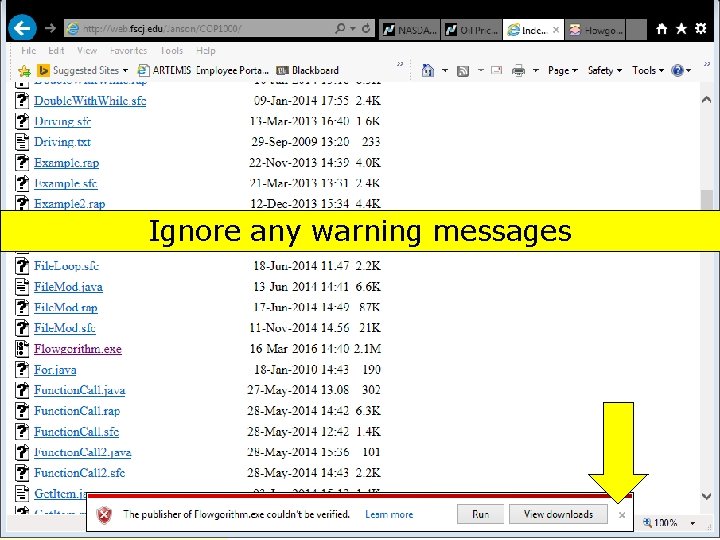
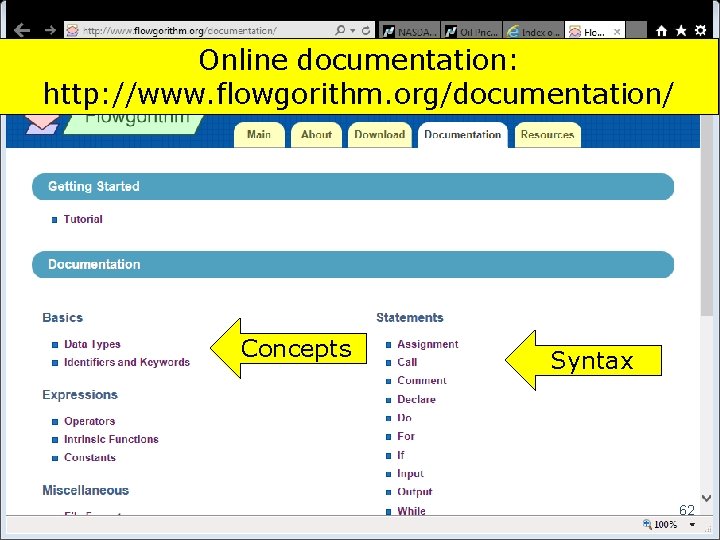
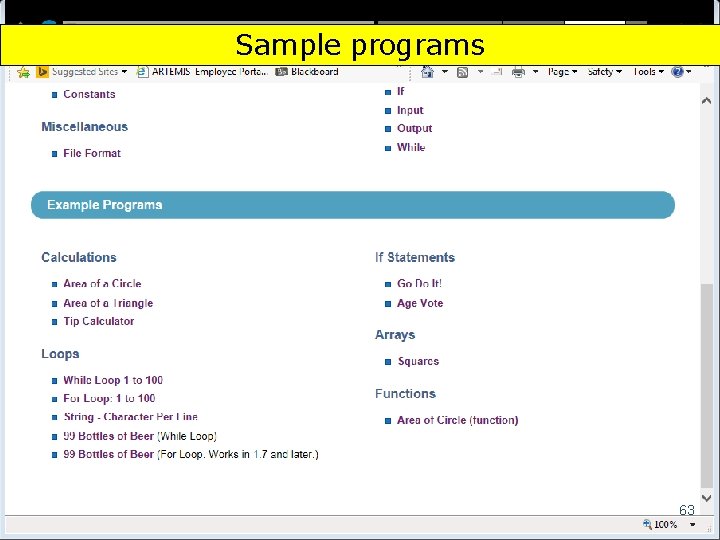
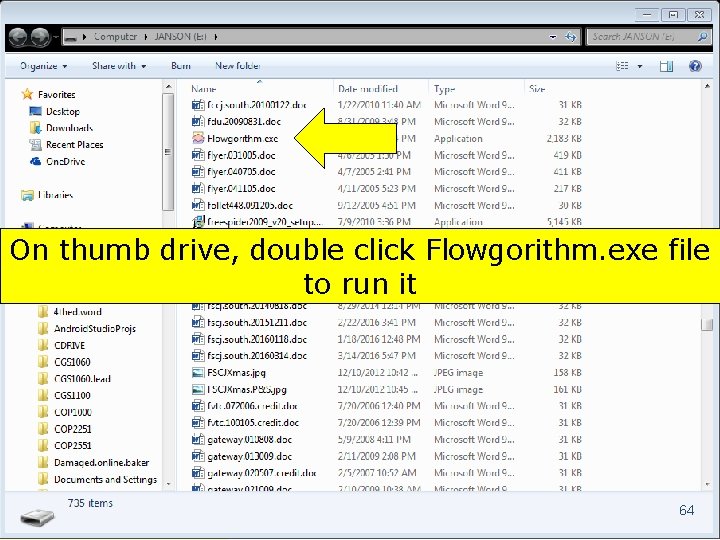
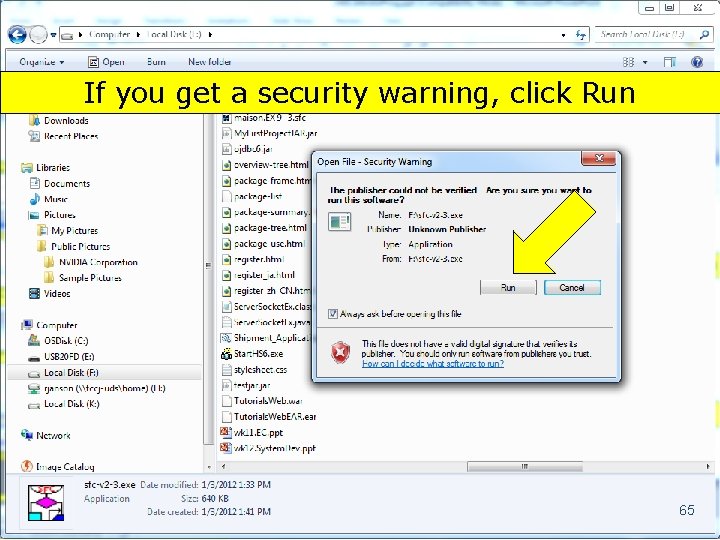
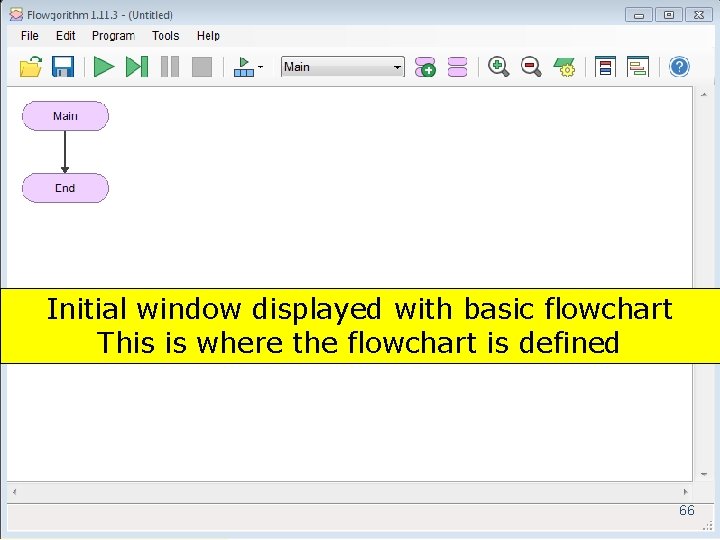
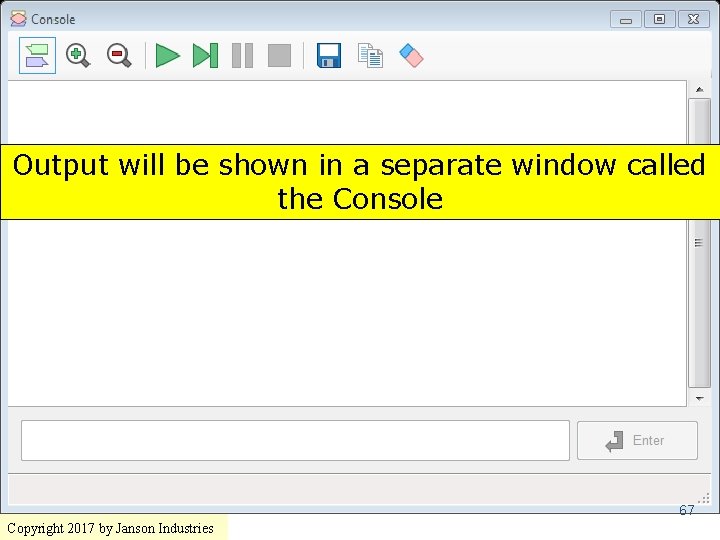
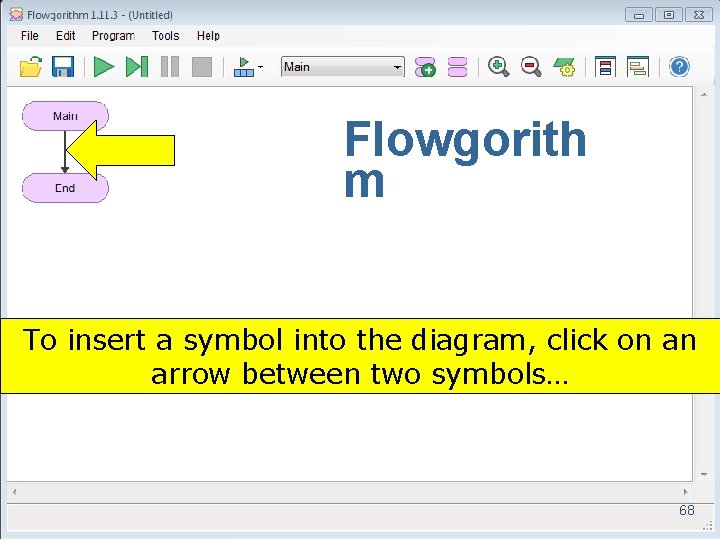
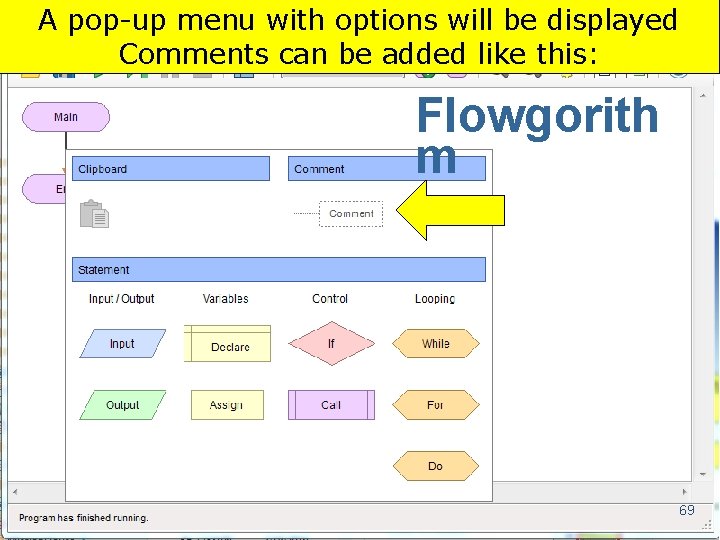
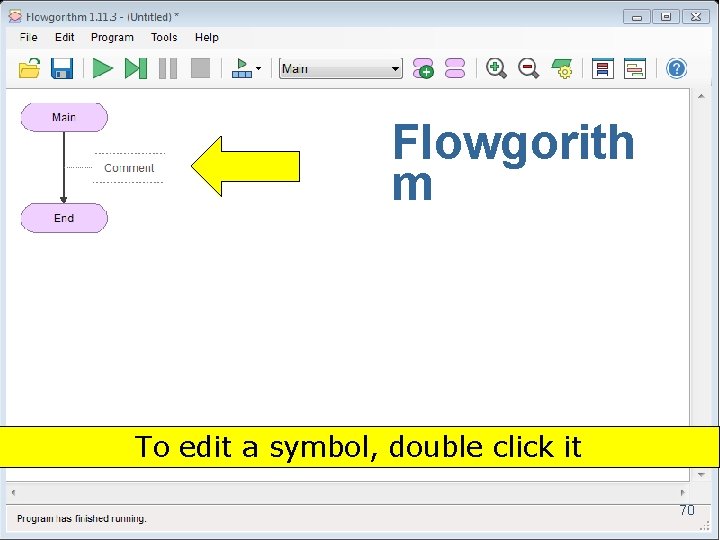
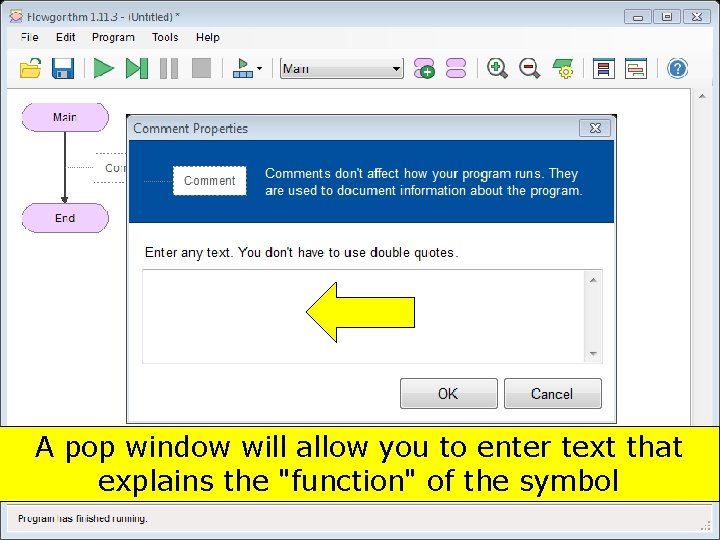
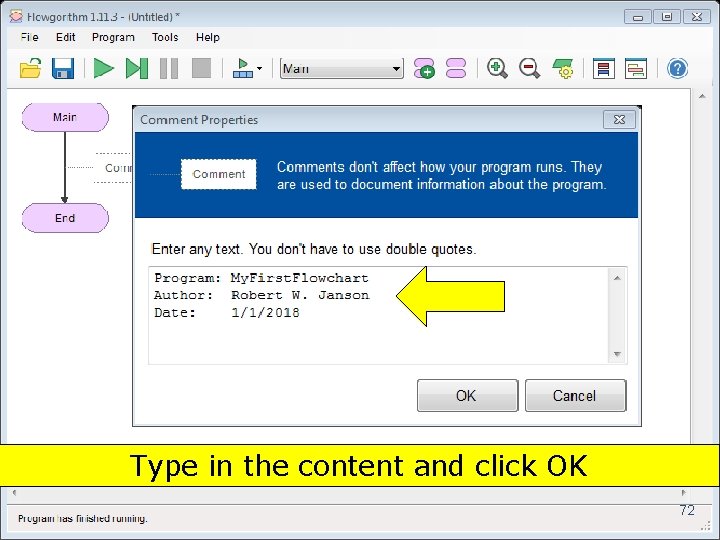
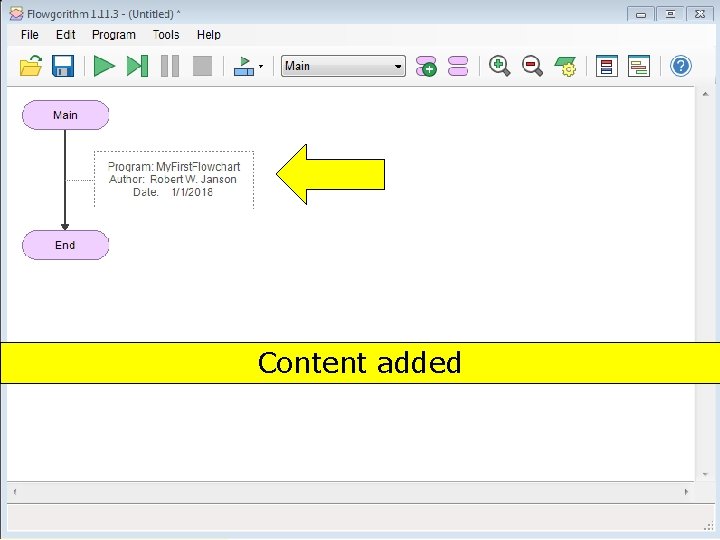
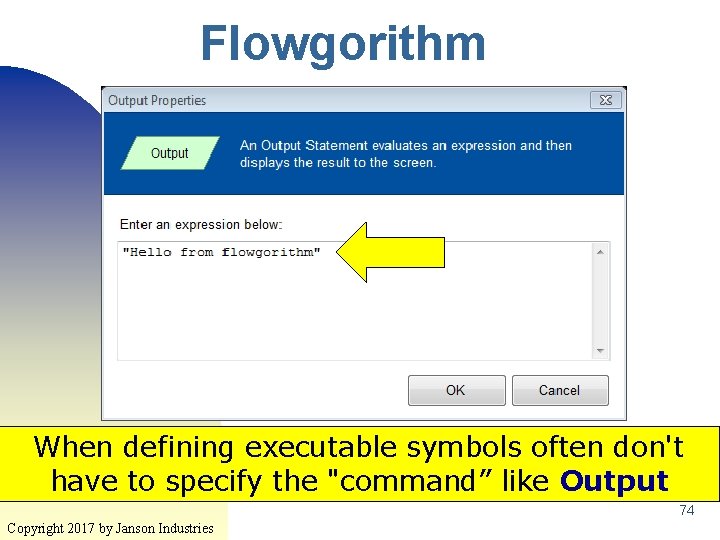
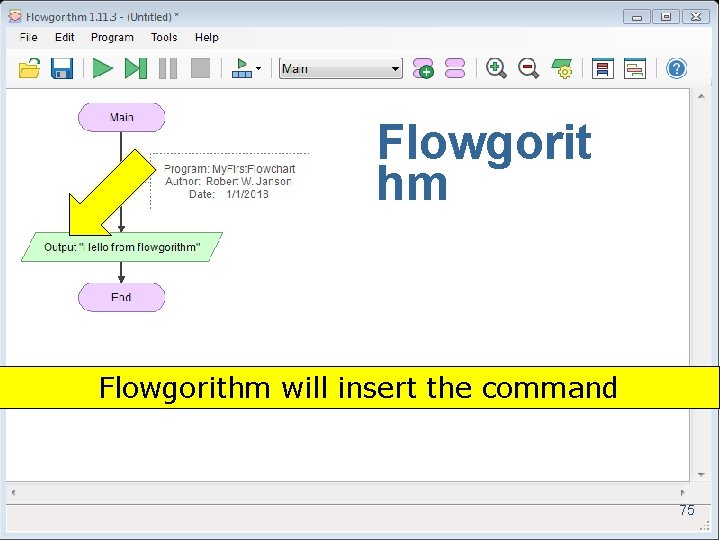
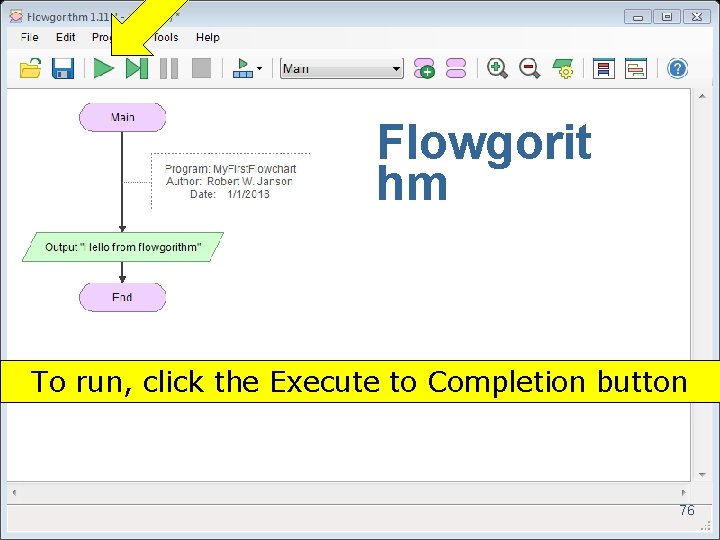
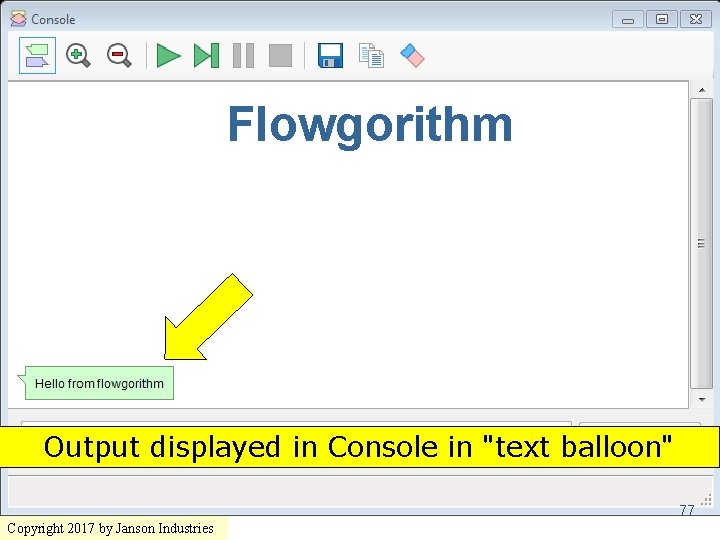
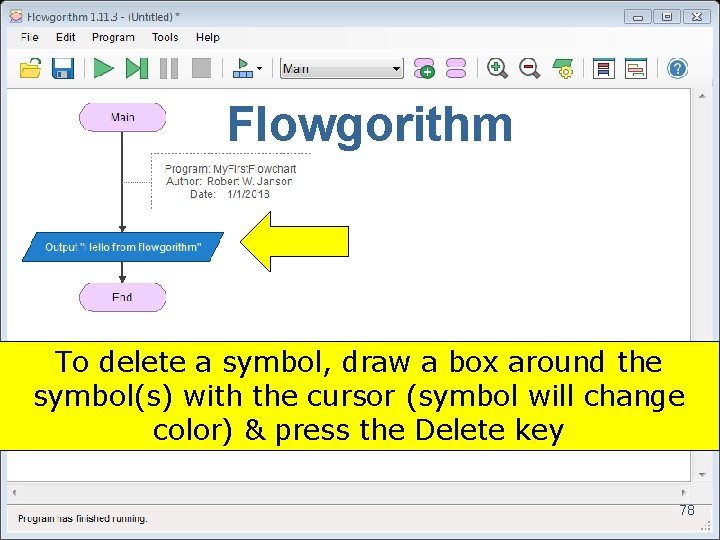
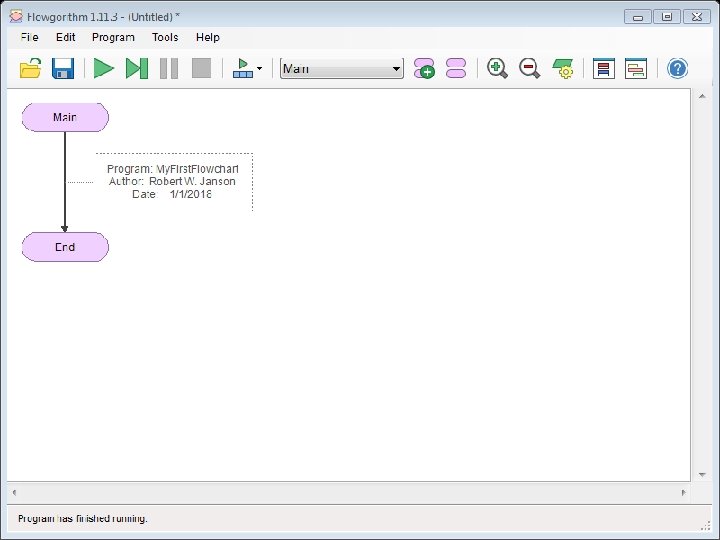
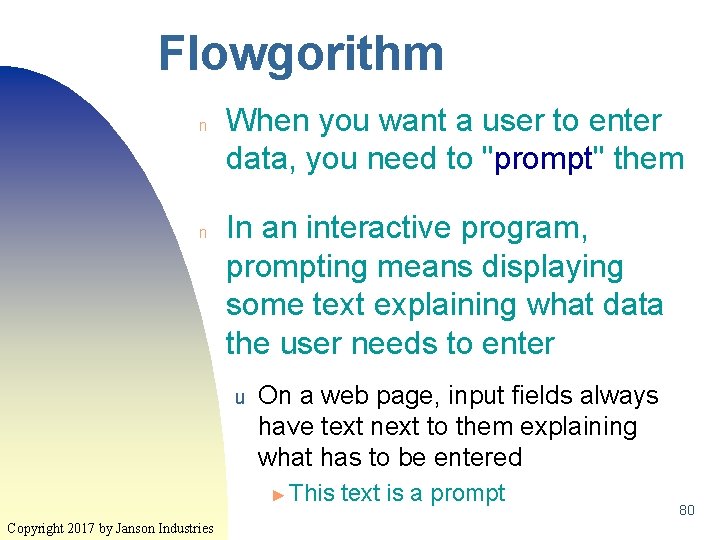
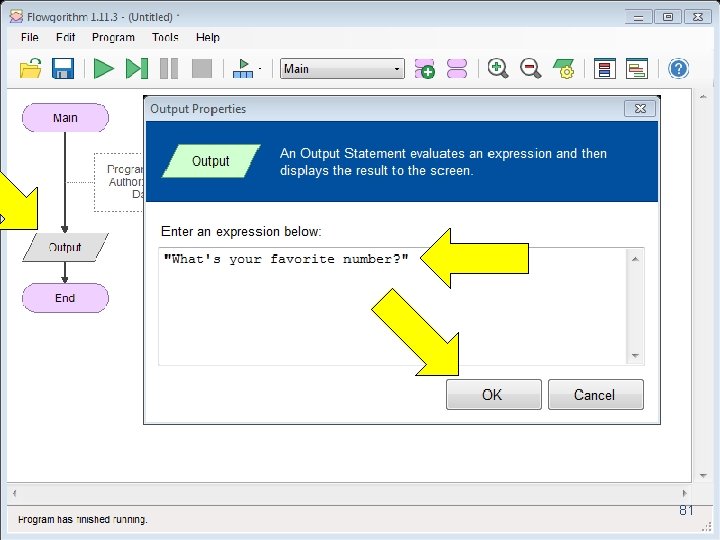
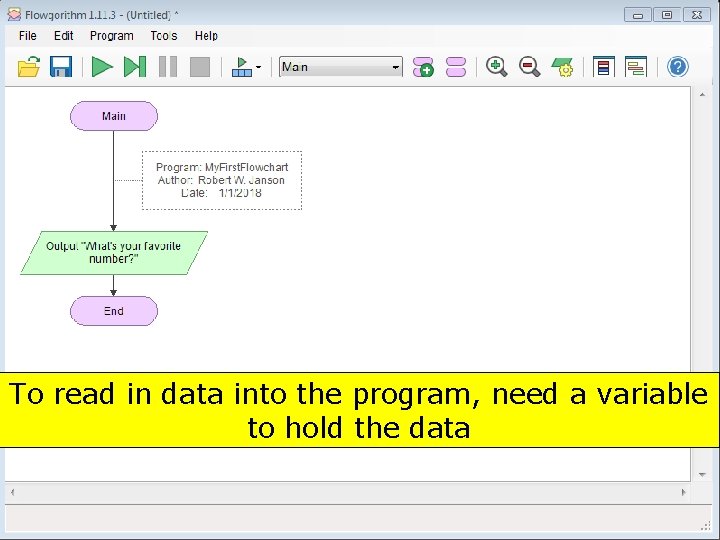
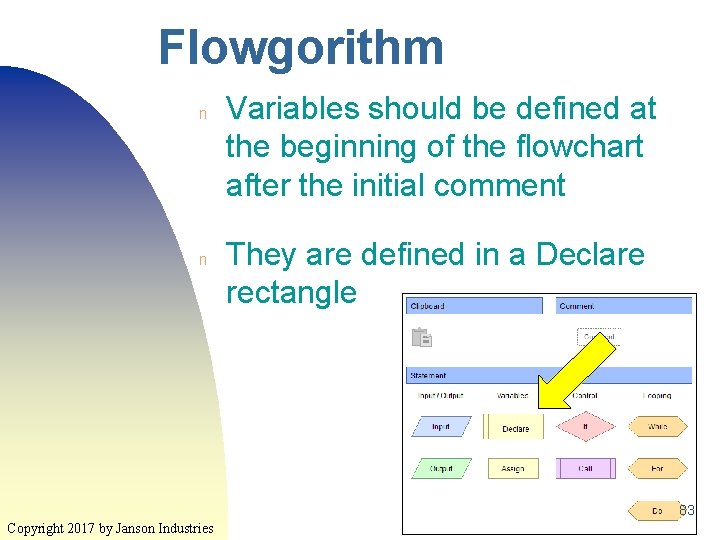
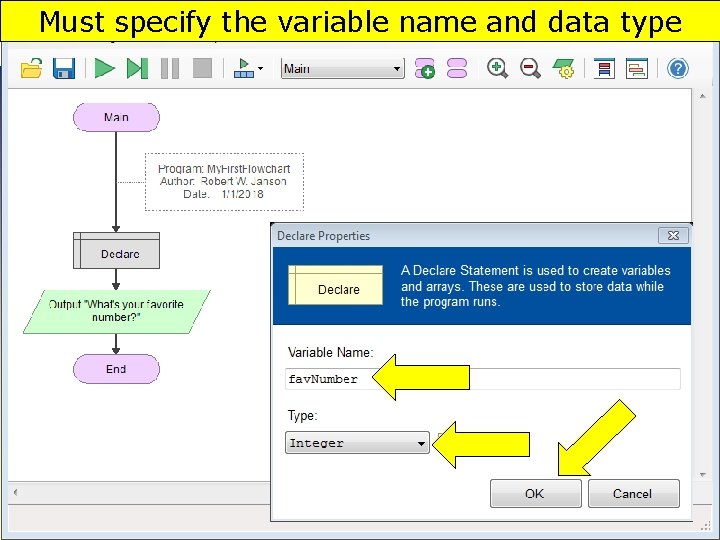
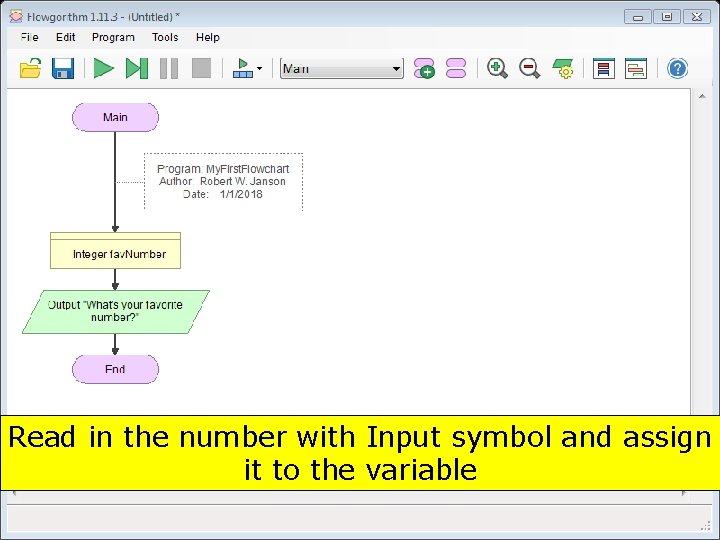
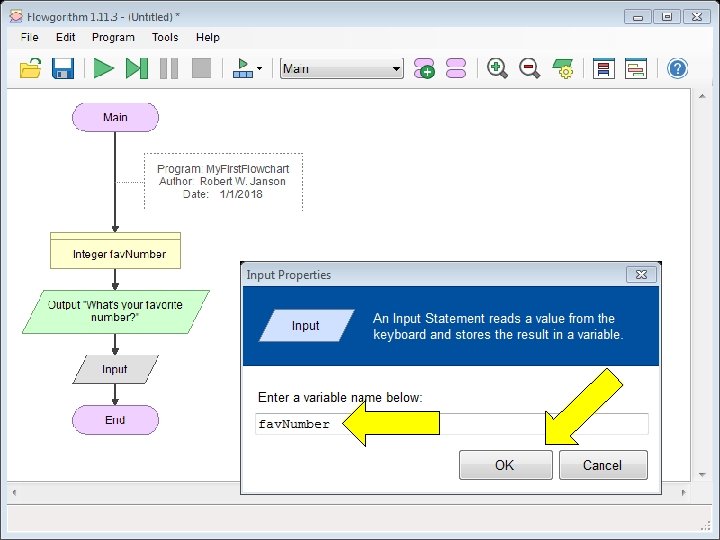
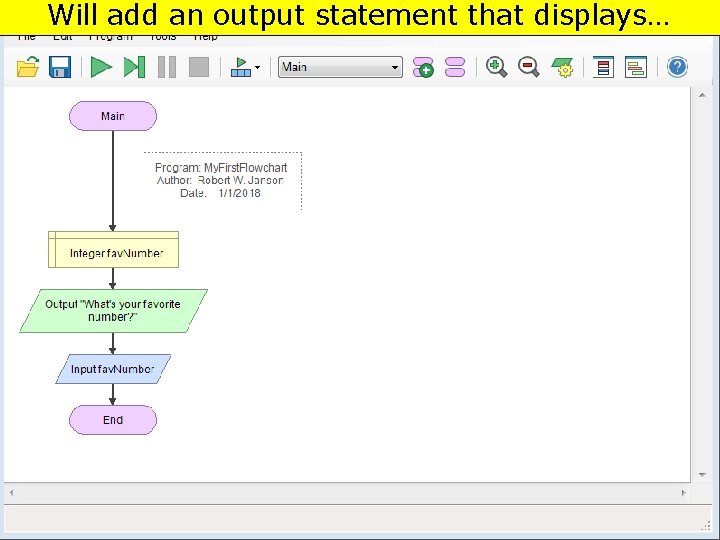
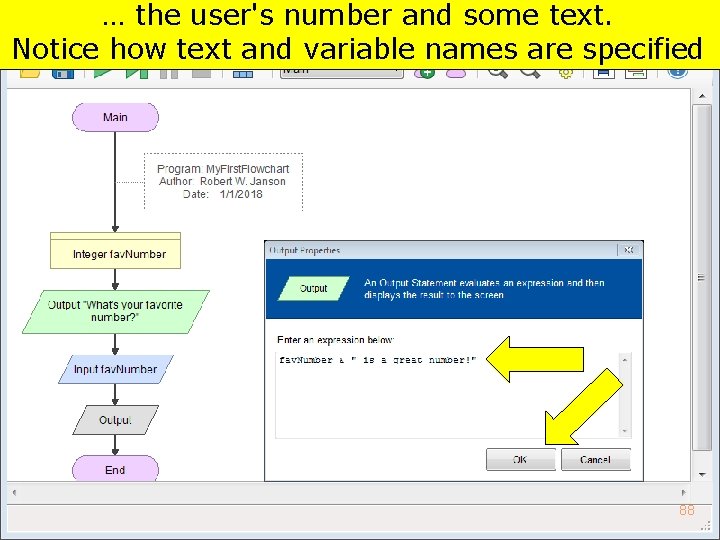
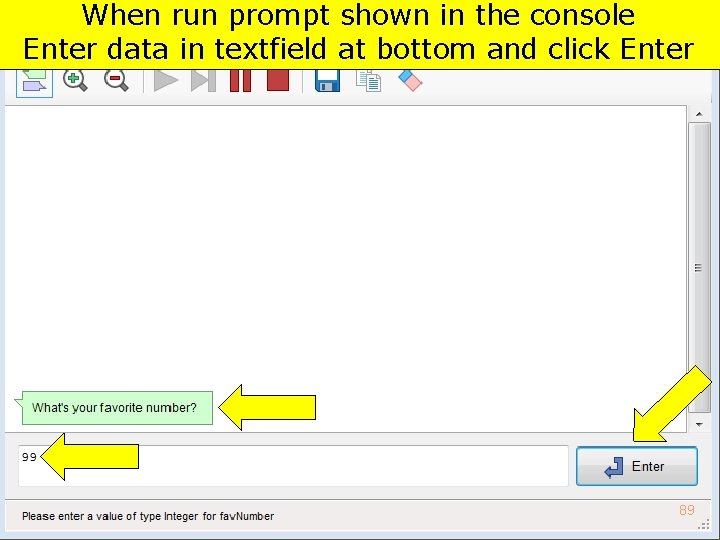
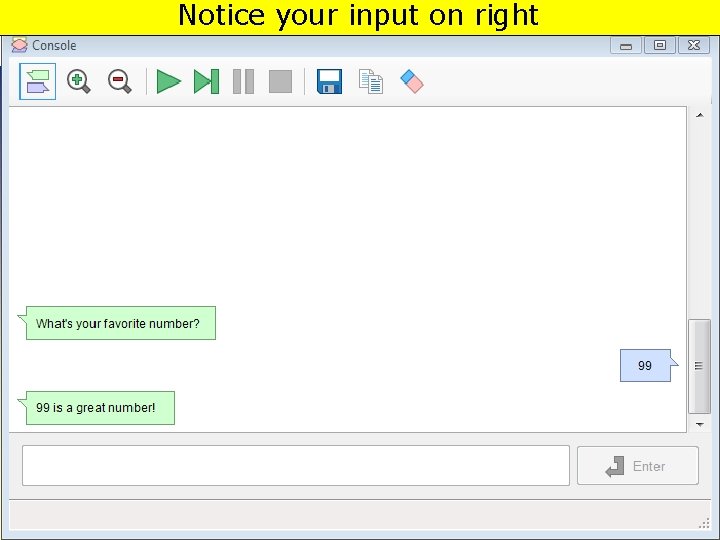
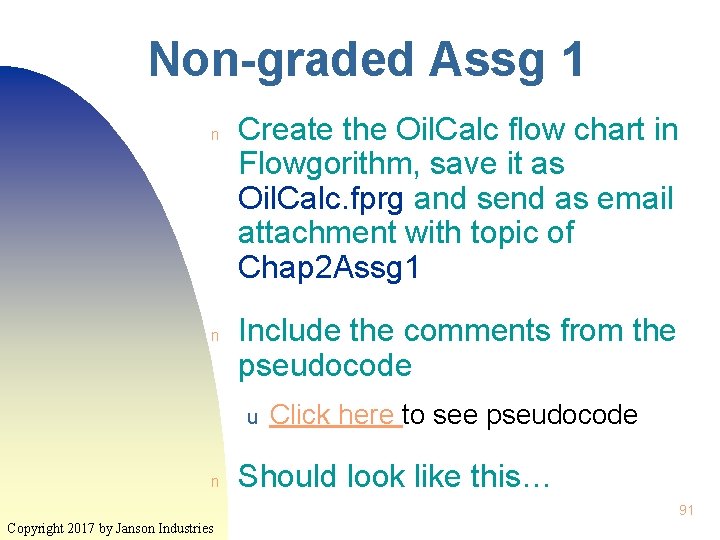
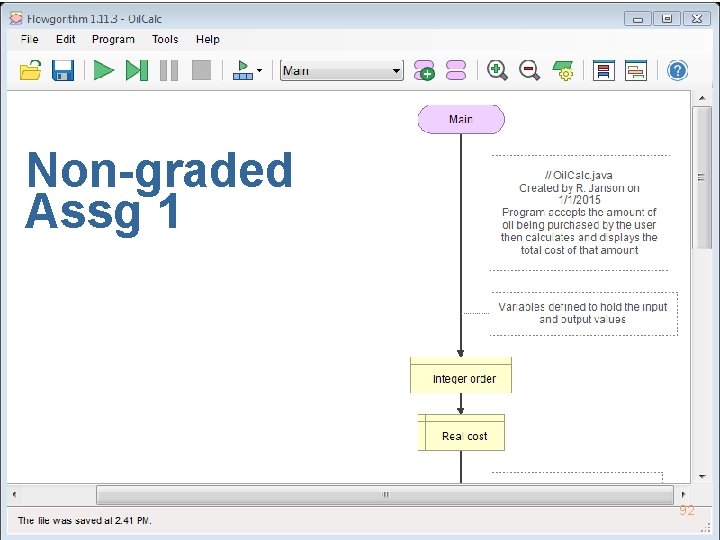
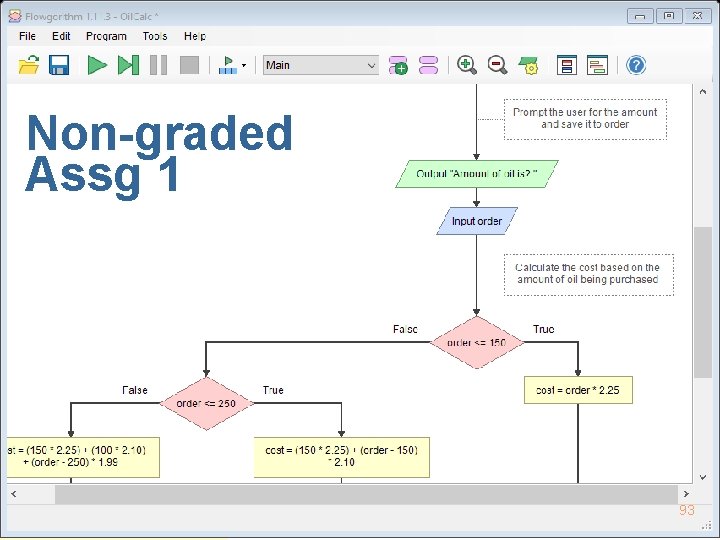
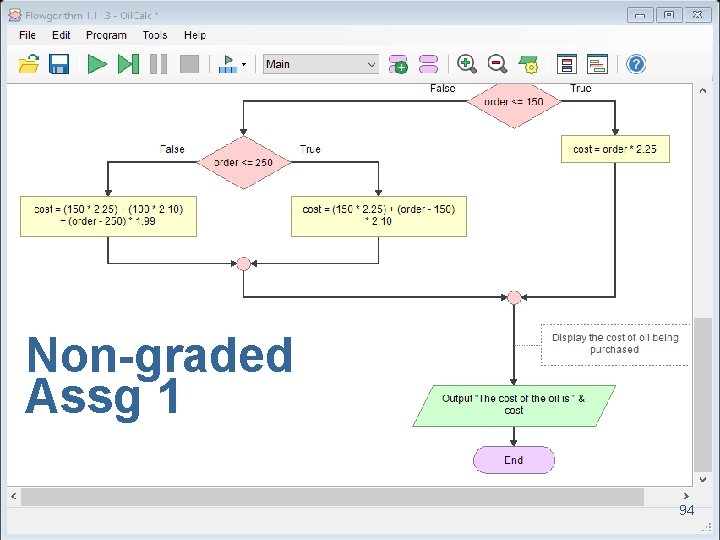
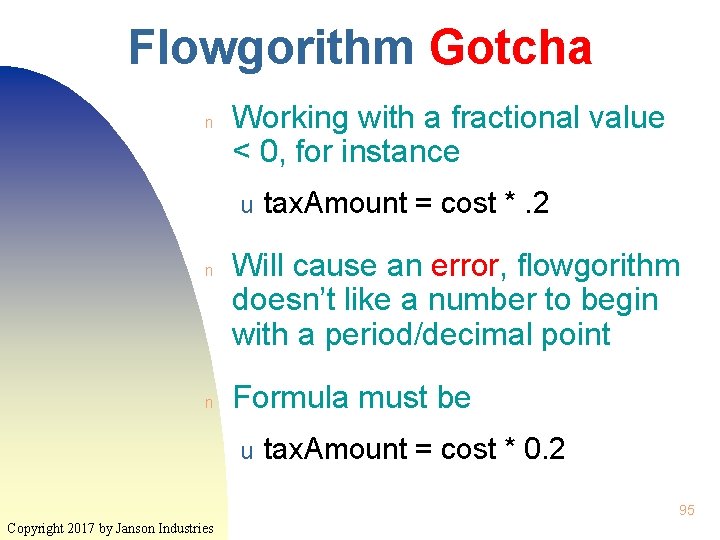
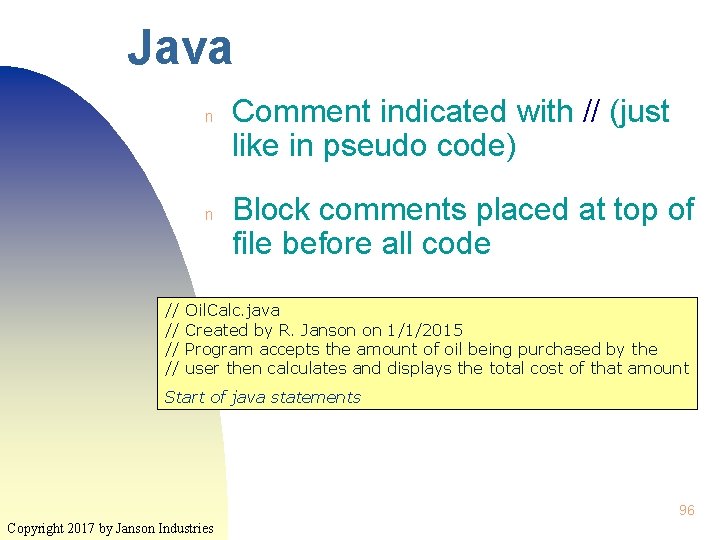
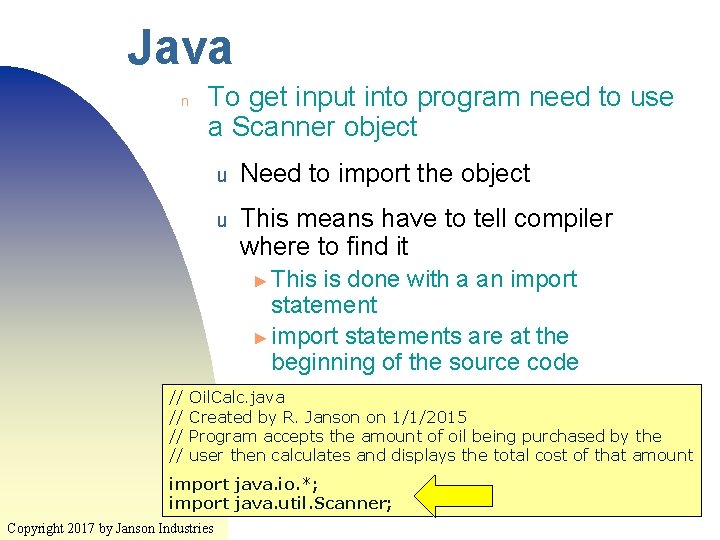
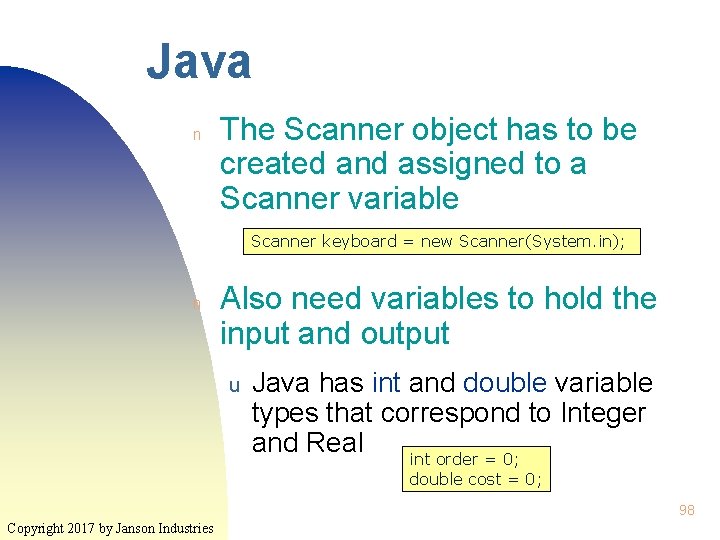
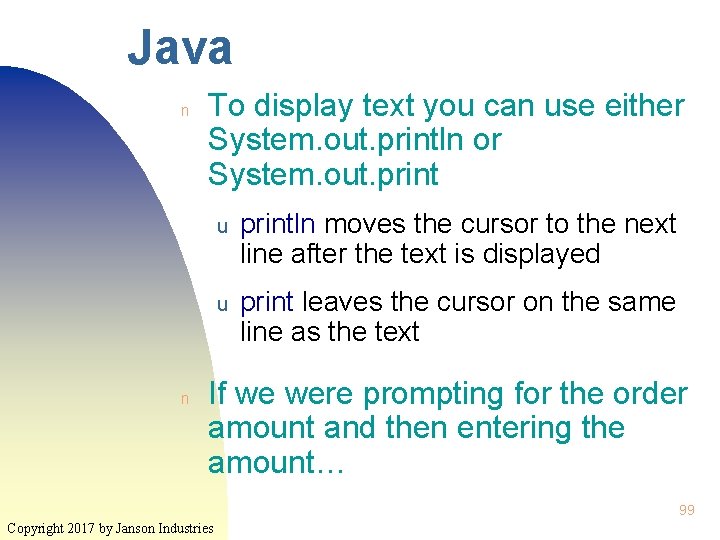
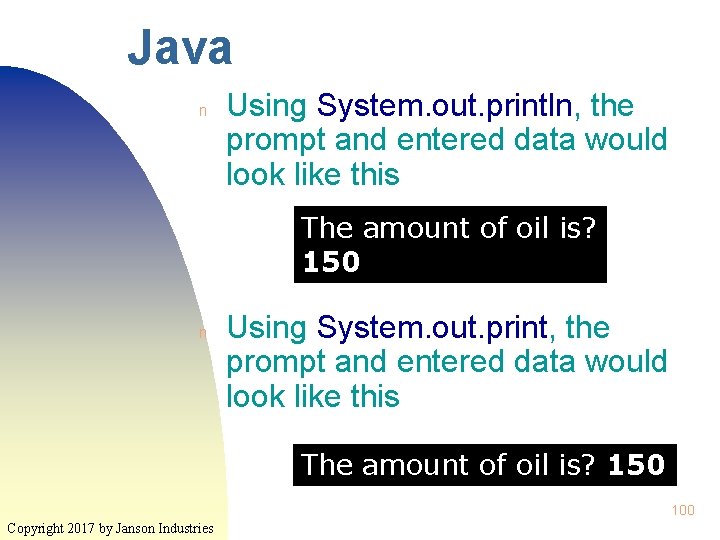
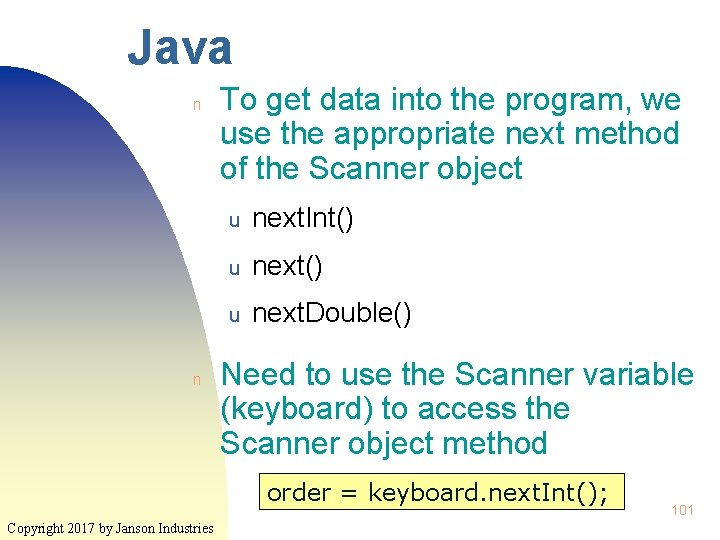
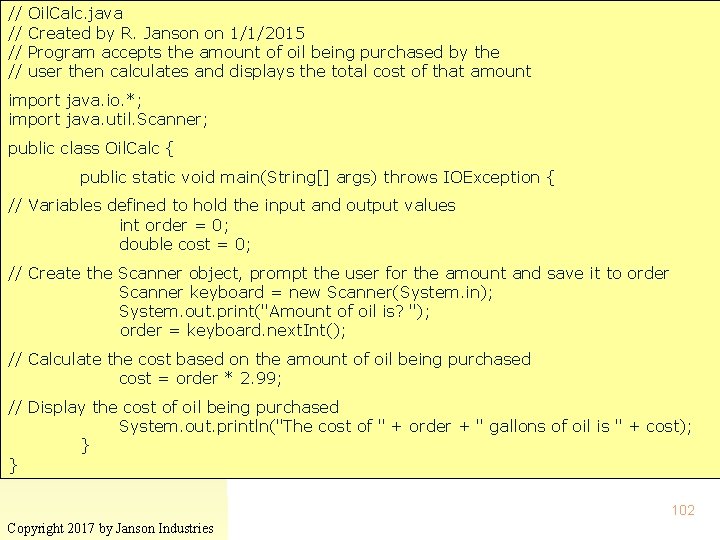
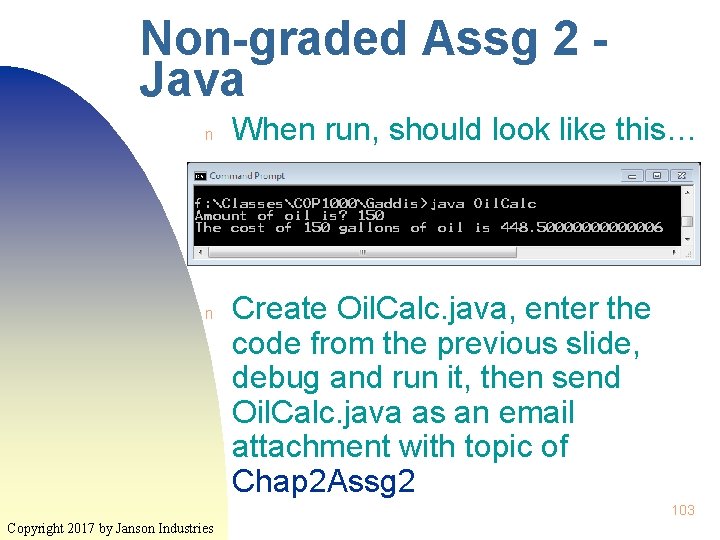
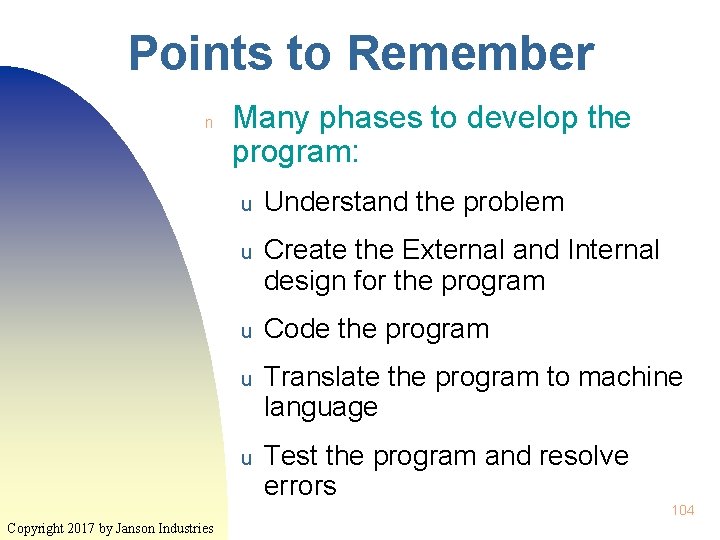
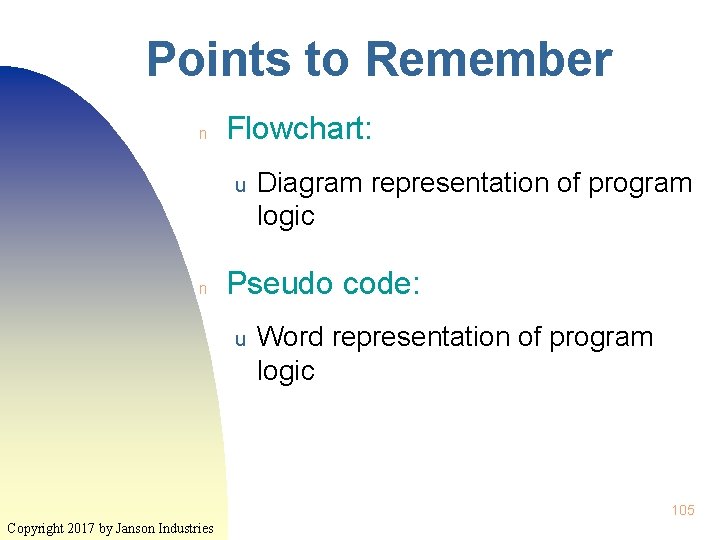
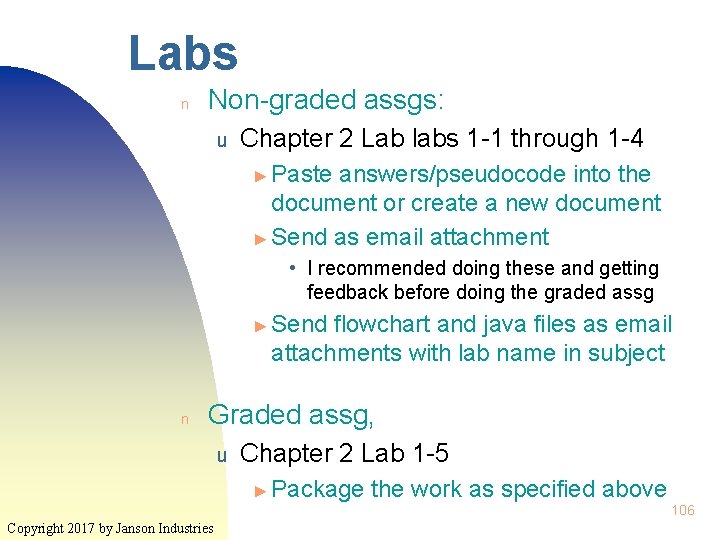
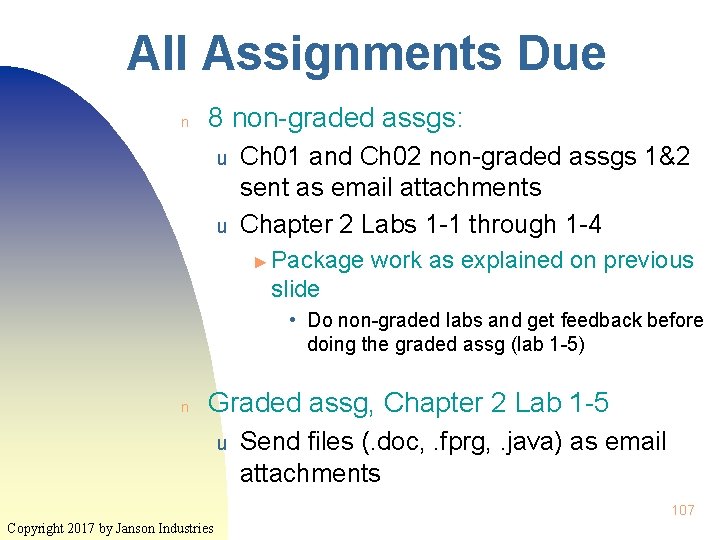
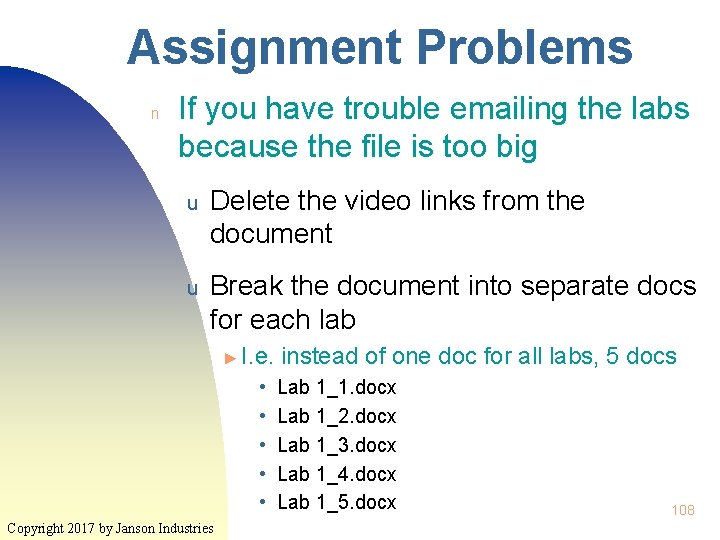
- Slides: 108
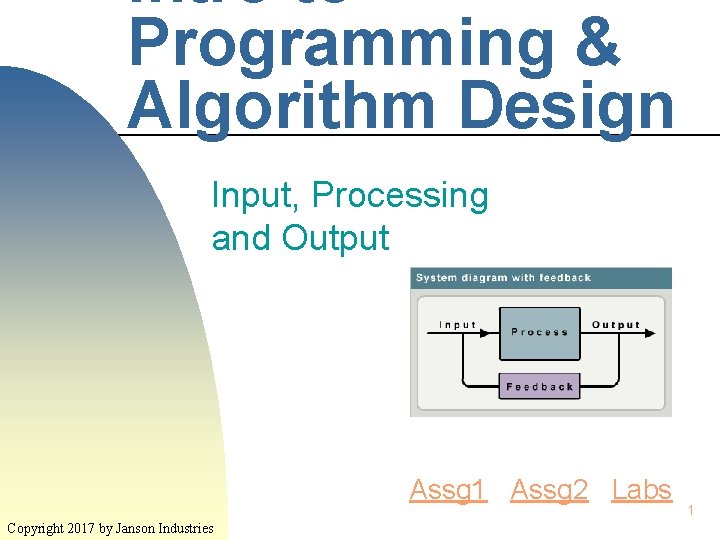
Intro to Programming & Algorithm Design Input, Processing and Output Assg 1 Assg 2 Labs Copyright 2017 by Janson Industries 1
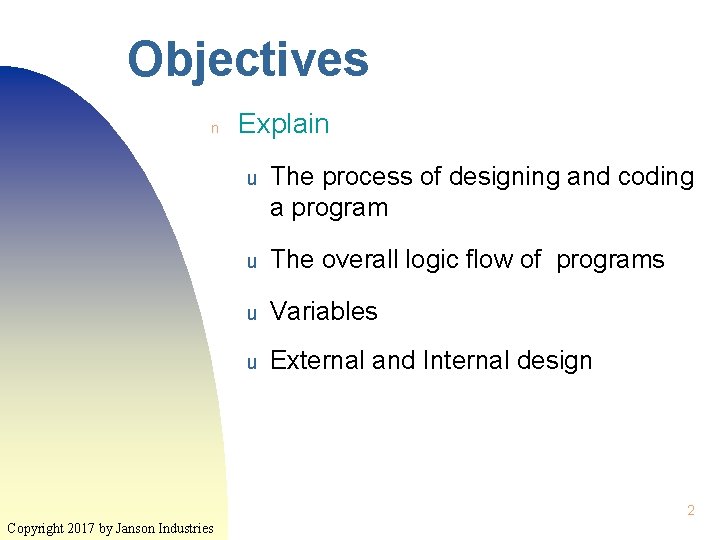
Objectives n Explain u The process of designing and coding a program u The overall logic flow of programs u Variables u External and Internal design 2 Copyright 2017 by Janson Industries
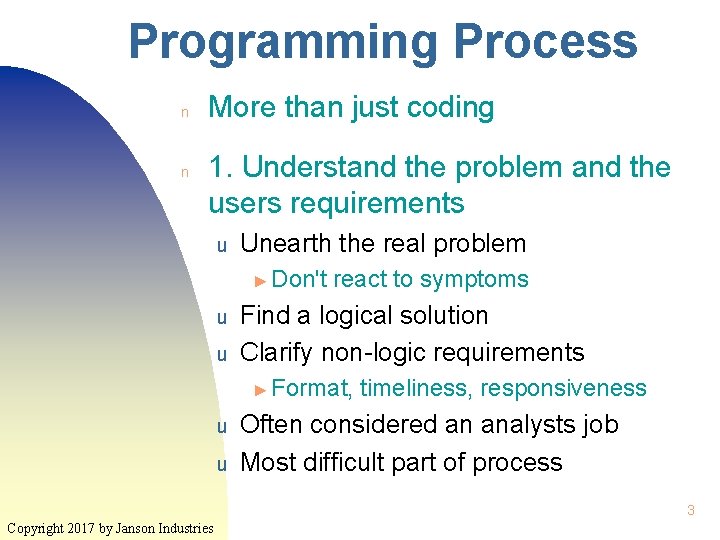
Programming Process n n More than just coding 1. Understand the problem and the users requirements u Unearth the real problem ► Don't u u react to symptoms Find a logical solution Clarify non-logic requirements ► Format, u u timeliness, responsiveness Often considered an analysts job Most difficult part of process 3 Copyright 2017 by Janson Industries
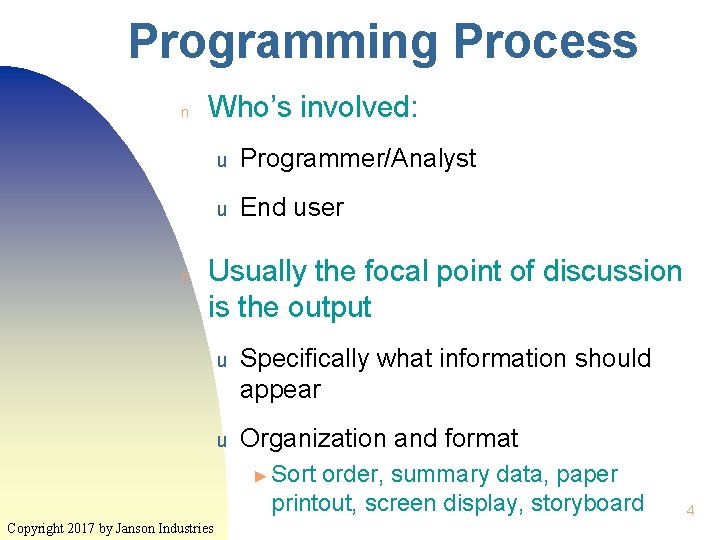
Programming Process n n Who’s involved: u Programmer/Analyst u End user Usually the focal point of discussion is the output u Specifically what information should appear u Organization and format ► Sort order, summary data, paper printout, screen display, storyboard Copyright 2017 by Janson Industries 4
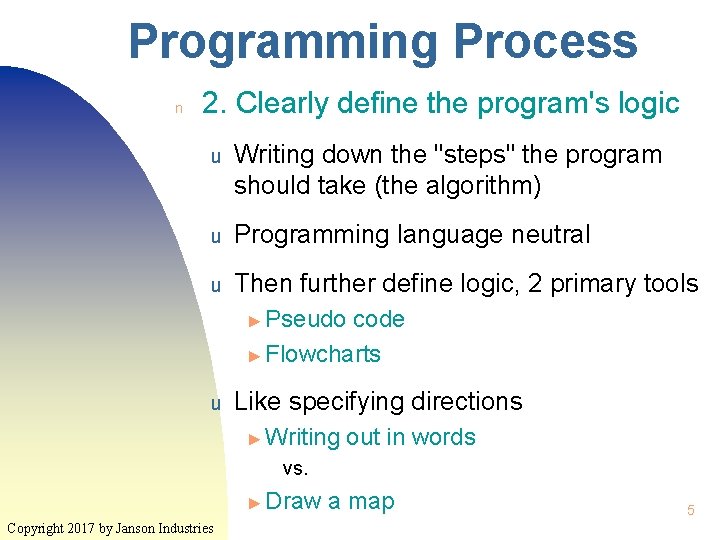
Programming Process n 2. Clearly define the program's logic u Writing down the "steps" the program should take (the algorithm) u Programming language neutral u Then further define logic, 2 primary tools ► Pseudo code ► Flowcharts u Like specifying directions ► Writing out in words vs. ► Draw Copyright 2017 by Janson Industries a map 5
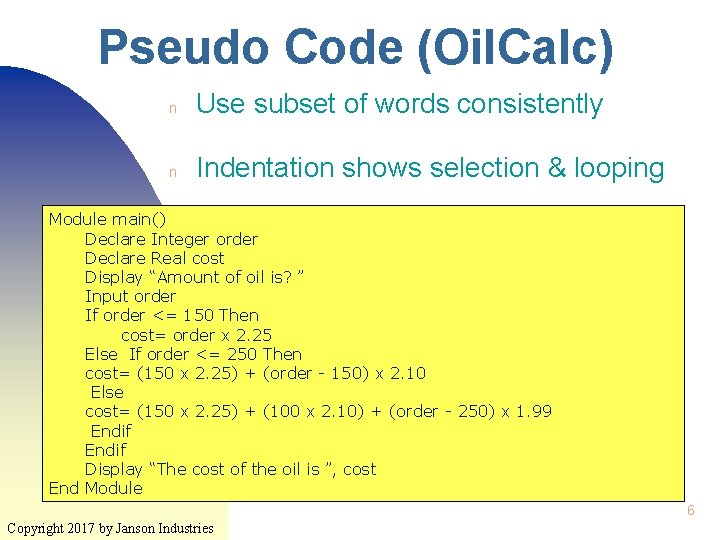
Pseudo Code (Oil. Calc) n Use subset of words consistently n Indentation shows selection & looping Module main() Declare Integer order Declare Real cost Display “Amount of oil is? ” Input order If order <= 150 Then cost= order x 2. 25 Else If order <= 250 Then cost= (150 x 2. 25) + (order - 150) x 2. 10 Else cost= (150 x 2. 25) + (100 x 2. 10) + (order - 250) x 1. 99 Endif Display “The cost of the oil is ”, cost End Module 6 Copyright 2017 by Janson Industries
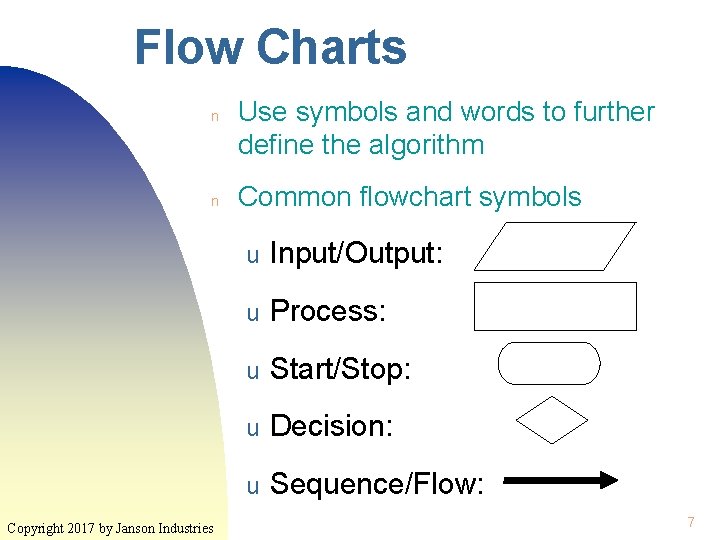
Flow Charts n n Copyright 2017 by Janson Industries Use symbols and words to further define the algorithm Common flowchart symbols u Input/Output: u Process: u Start/Stop: u Decision: u Sequence/Flow: 7
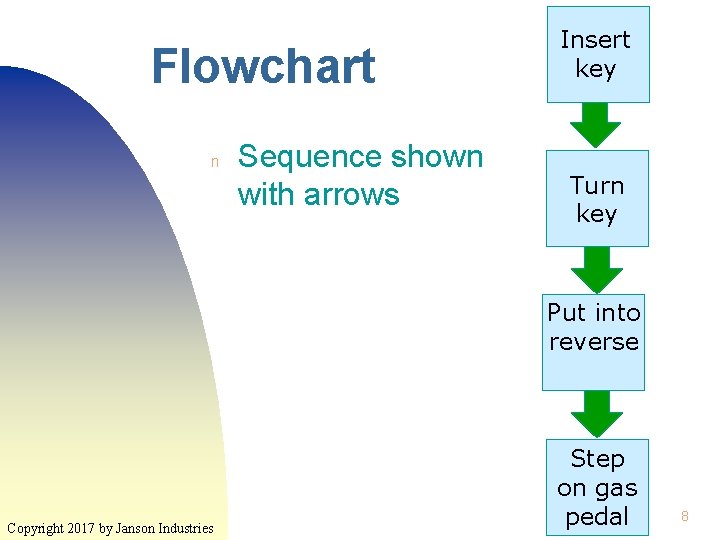
Flowchart n Sequence shown with arrows Insert key Turn key Put into reverse Copyright 2017 by Janson Industries Step on gas pedal 8
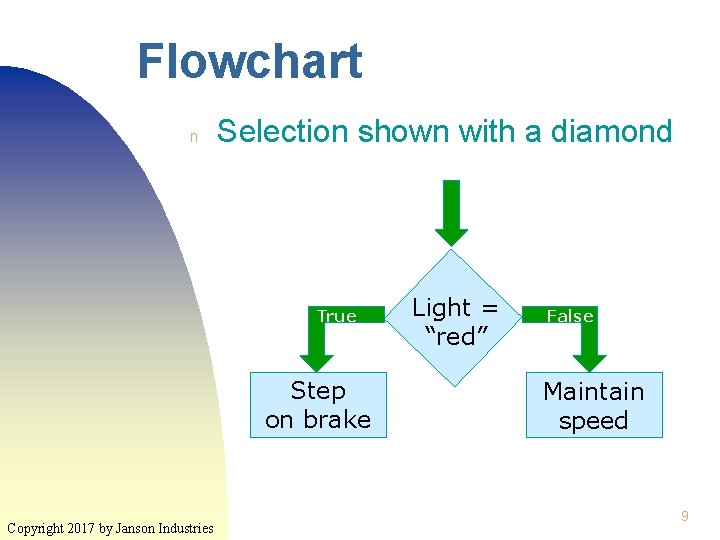
Flowchart n Selection shown with a diamond True Step on brake Copyright 2017 by Janson Industries Light = “red” False Maintain speed 9
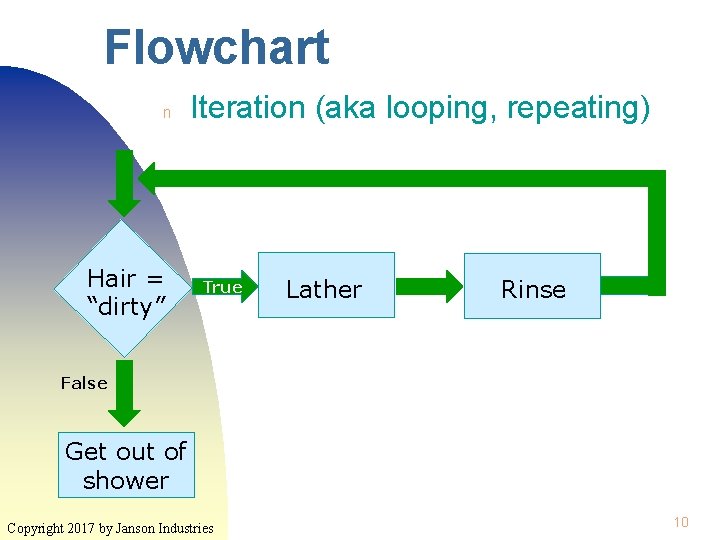
Flowchart n Hair = “dirty” Iteration (aka looping, repeating) True Lather Rinse False Get out of shower Copyright 2017 by Janson Industries 10
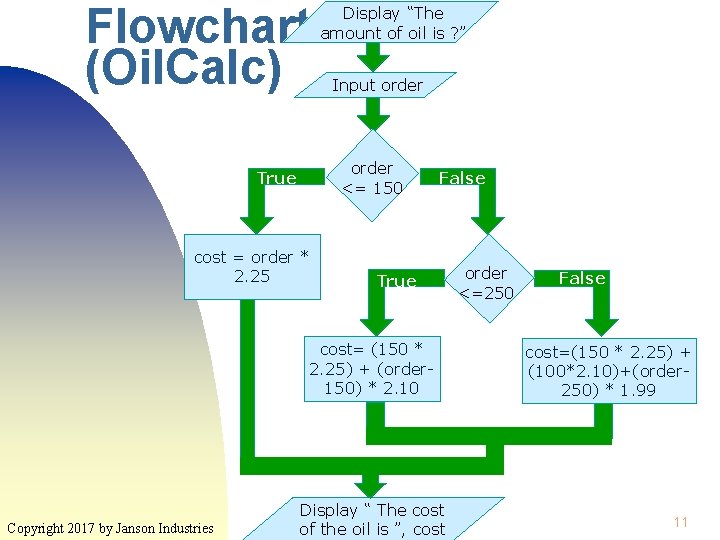
Flowchart (Oil. Calc) Display “The amount of oil is ? ” Input order <= 150 True cost = order * 2. 25 False True cost= (150 * 2. 25) + (order 150) * 2. 10 Copyright 2017 by Janson Industries Display “ The cost of the oil is ”, cost order <=250 False cost=(150 * 2. 25) + (100*2. 10)+(order 250) * 1. 99 11
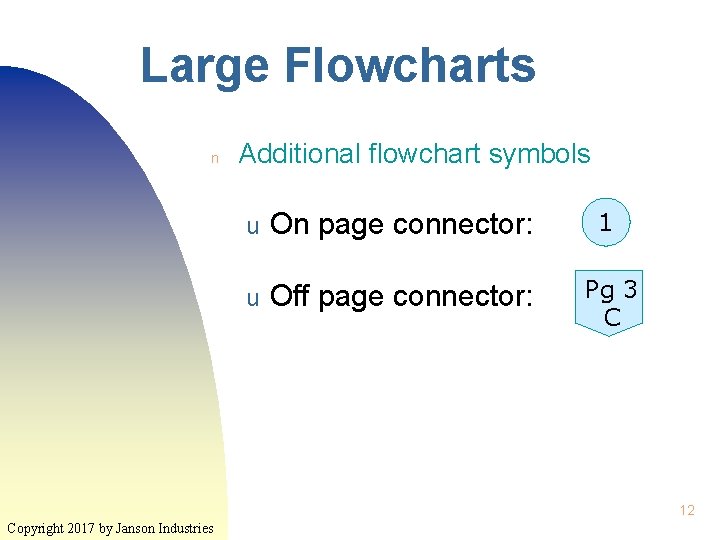
Large Flowcharts n Additional flowchart symbols u On page connector: 1 u Off page connector: Pg 3 C 12 Copyright 2017 by Janson Industries
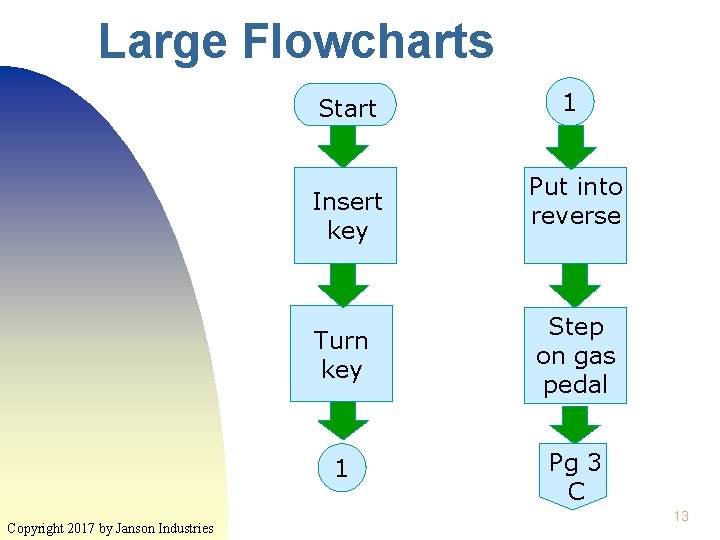
Large Flowcharts Start Insert key Copyright 2017 by Janson Industries 1 Put into reverse Turn key Step on gas pedal 1 Pg 3 C 13
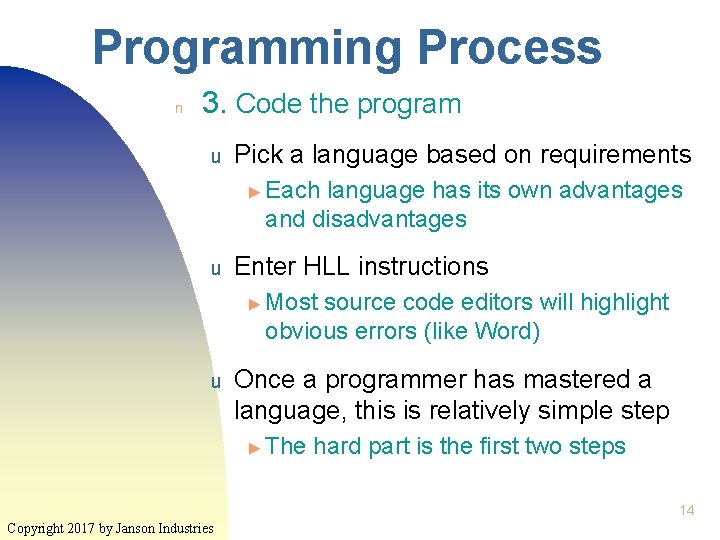
Programming Process n 3. Code the program u Pick a language based on requirements ► Each language has its own advantages and disadvantages u Enter HLL instructions ► Most source code editors will highlight obvious errors (like Word) u Once a programmer has mastered a language, this is relatively simple step ► The hard part is the first two steps 14 Copyright 2017 by Janson Industries
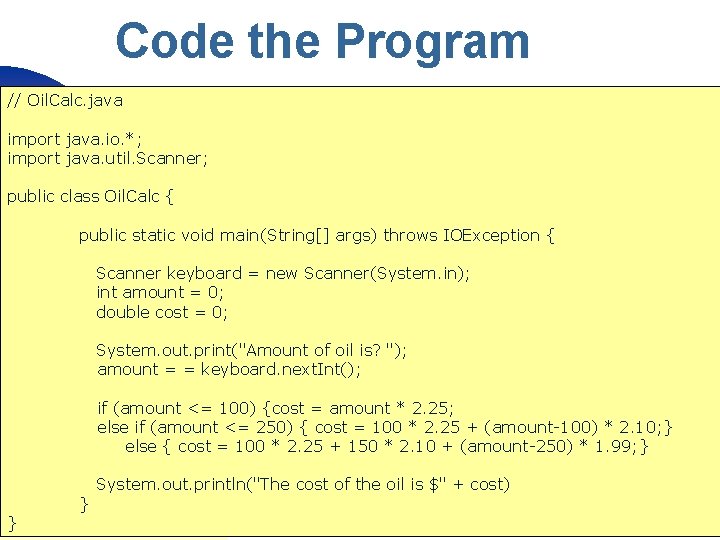
Code the Program // Oil. Calc. java import java. io. *; import java. util. Scanner; public class Oil. Calc { public static void main(String[] args) throws IOException { Scanner keyboard = new Scanner(System. in); int amount = 0; double cost = 0; System. out. print("Amount of oil is? "); amount = = keyboard. next. Int(); if (amount <= 100) {cost = amount * 2. 25; else if (amount <= 250) { cost = 100 * 2. 25 + (amount-100) * 2. 10; } else { cost = 100 * 2. 25 + 150 * 2. 10 + (amount-250) * 1. 99; } } System. out. println("The cost of the oil is $" + cost) } Copyright 2017 by Janson Industries 15
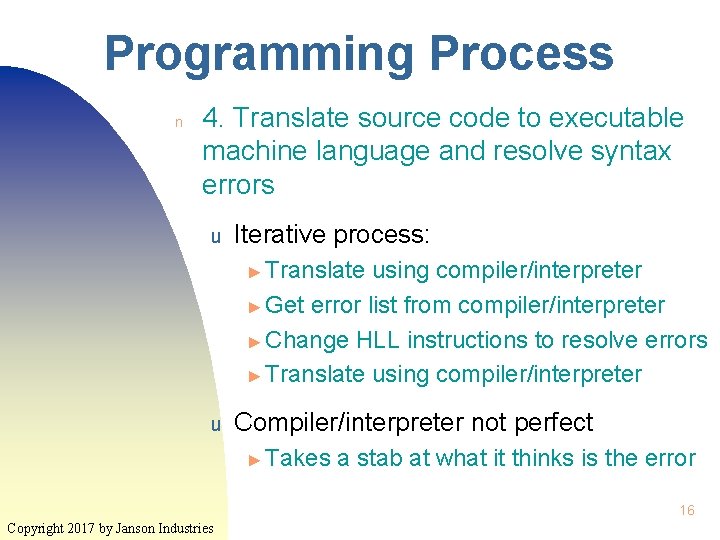
Programming Process n 4. Translate source code to executable machine language and resolve syntax errors u Iterative process: ► Translate using compiler/interpreter ► Get error list from compiler/interpreter ► Change HLL instructions to resolve errors ► Translate using compiler/interpreter u Compiler/interpreter not perfect ► Takes a stab at what it thinks is the error 16 Copyright 2017 by Janson Industries
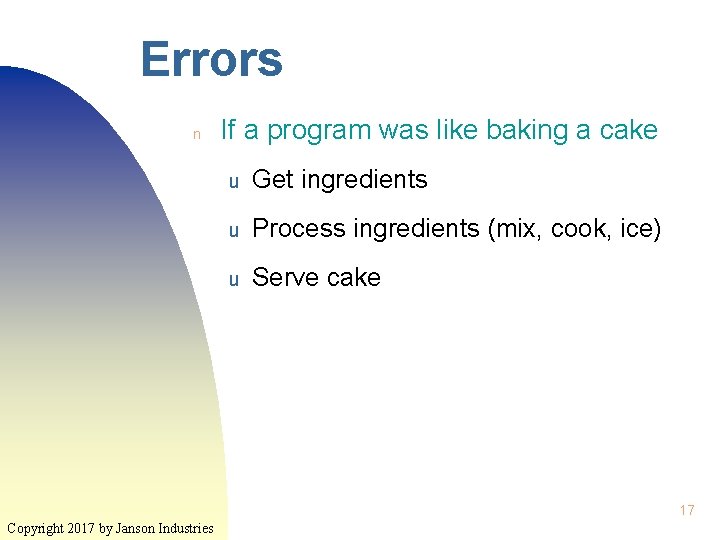
Errors n If a program was like baking a cake u Get ingredients u Process ingredients (mix, cook, ice) u Serve cake 17 Copyright 2017 by Janson Industries
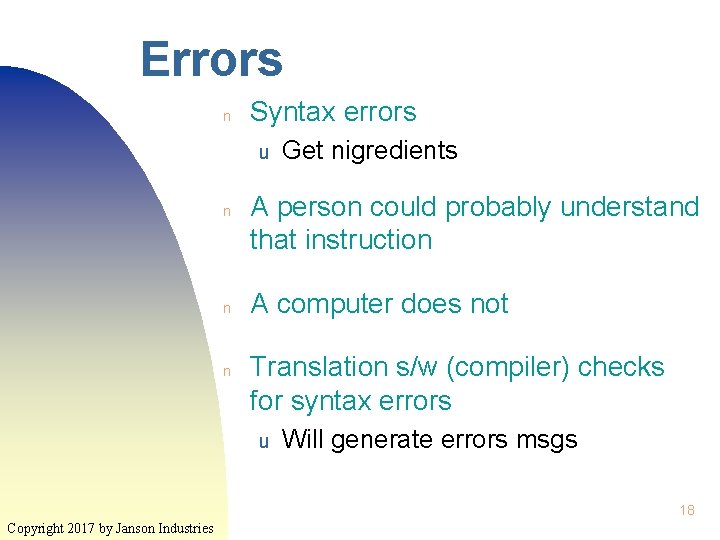
Errors n Syntax errors u n n n Get nigredients A person could probably understand that instruction A computer does not Translation s/w (compiler) checks for syntax errors u Will generate errors msgs 18 Copyright 2017 by Janson Industries
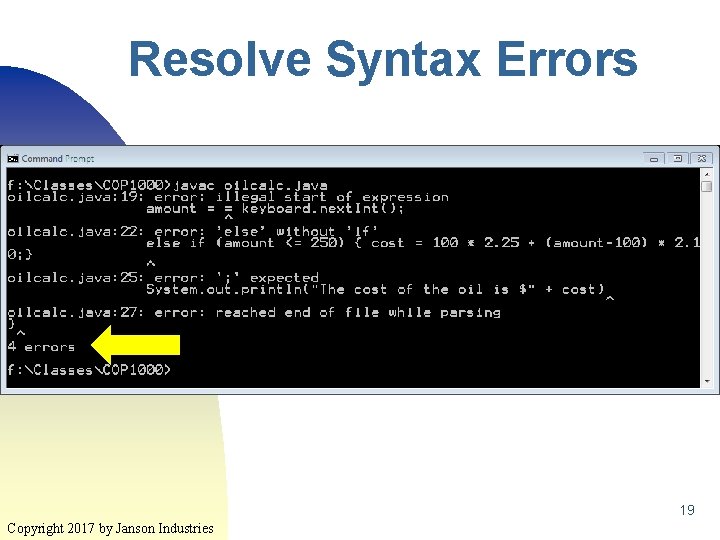
Resolve Syntax Errors 19 Copyright 2017 by Janson Industries
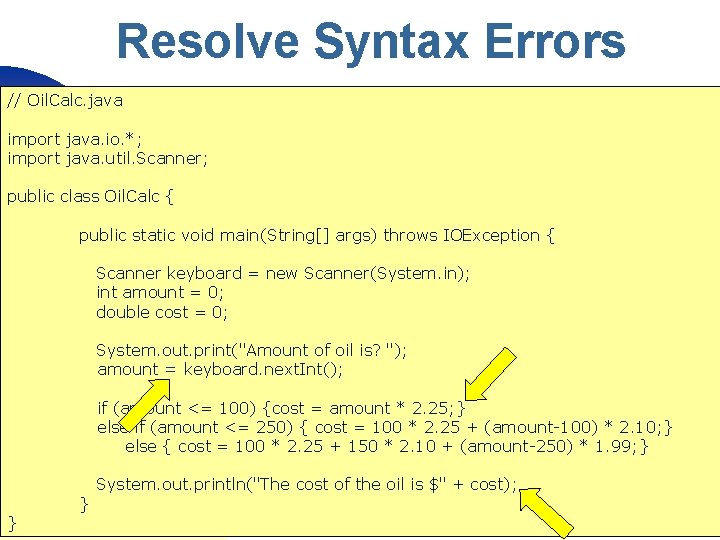
Resolve Syntax Errors // Oil. Calc. java import java. io. *; import java. util. Scanner; public class Oil. Calc { public static void main(String[] args) throws IOException { Scanner keyboard = new Scanner(System. in); int amount = 0; double cost = 0; System. out. print("Amount of oil is? "); amount = keyboard. next. Int(); if (amount <= 100) {cost = amount * 2. 25; } else if (amount <= 250) { cost = 100 * 2. 25 + (amount-100) * 2. 10; } else { cost = 100 * 2. 25 + 150 * 2. 10 + (amount-250) * 1. 99; } } System. out. println("The cost of the oil is $" + cost); } Copyright 2017 by Janson Industries 20
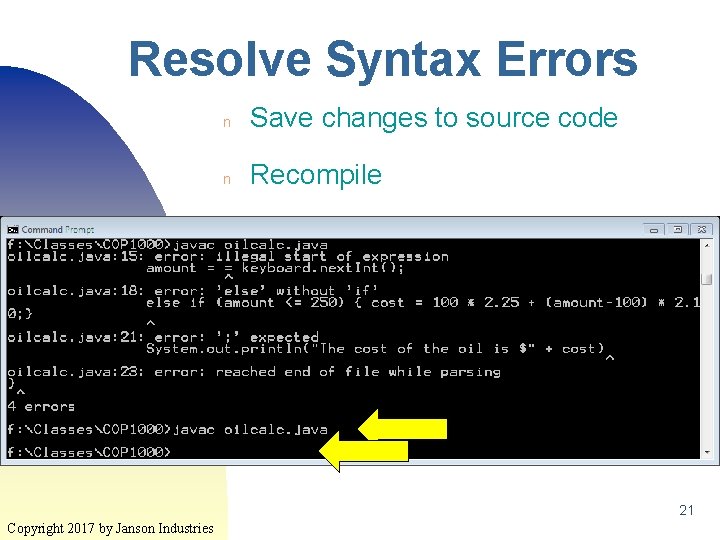
Resolve Syntax Errors n Save changes to source code n Recompile 21 Copyright 2017 by Janson Industries
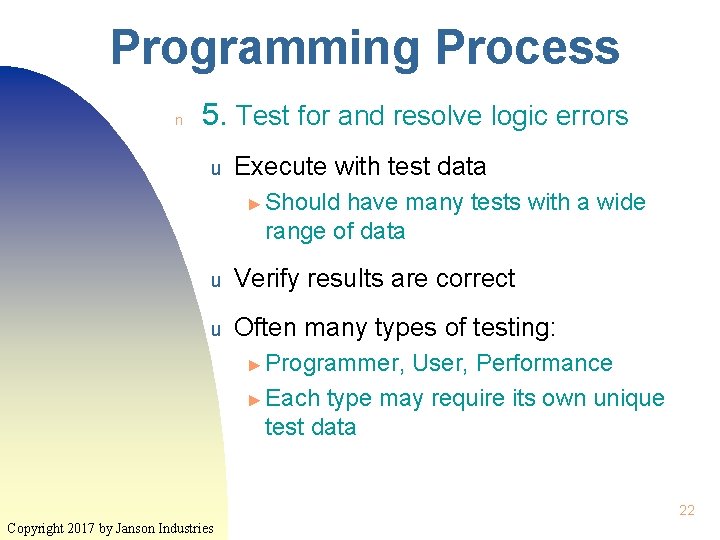
Programming Process n 5. Test for and resolve logic errors u Execute with test data ► Should have many tests with a wide range of data u Verify results are correct u Often many types of testing: ► Programmer, User, Performance ► Each type may require its own unique test data 22 Copyright 2017 by Janson Industries
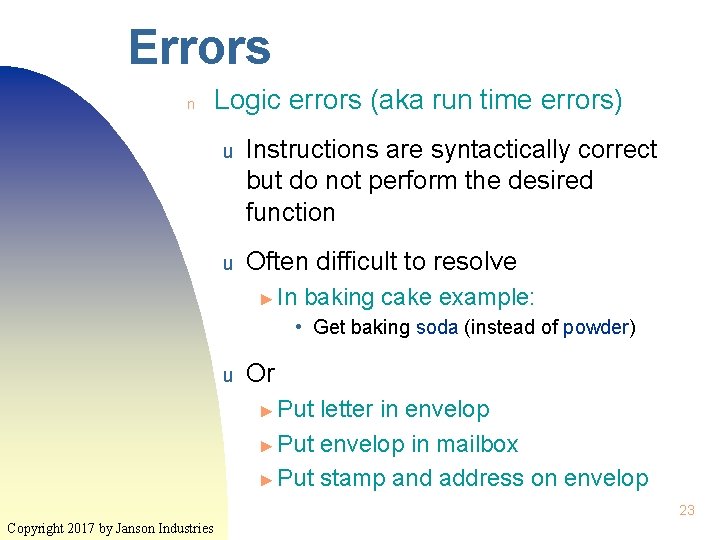
Errors n Logic errors (aka run time errors) u Instructions are syntactically correct but do not perform the desired function u Often difficult to resolve ► In baking cake example: • Get baking soda (instead of powder) u Or ► Put letter in envelop ► Put envelop in mailbox ► Put stamp and address on envelop 23 Copyright 2017 by Janson Industries
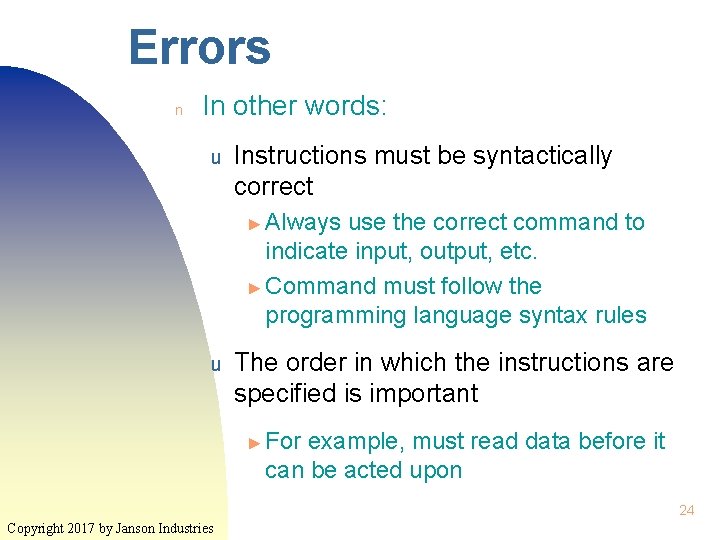
Errors n In other words: u Instructions must be syntactically correct ► Always use the correct command to indicate input, output, etc. ► Command must follow the programming language syntax rules u The order in which the instructions are specified is important ► For example, must read data before it can be acted upon 24 Copyright 2017 by Janson Industries
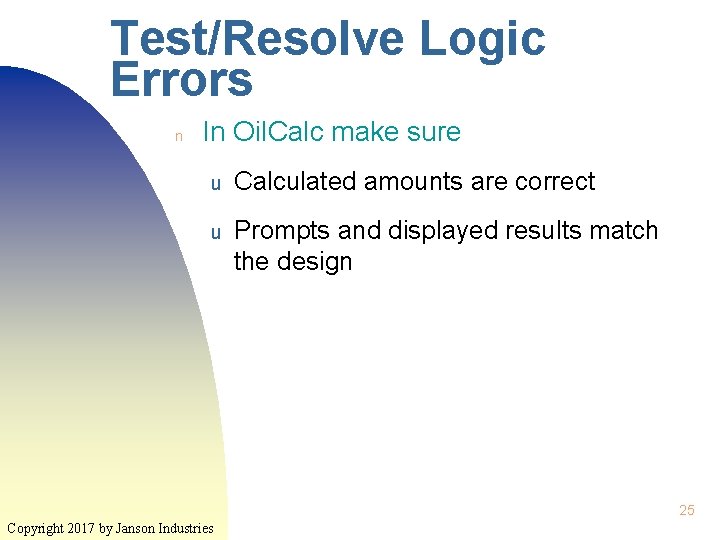
Test/Resolve Logic Errors n In Oil. Calc make sure u Calculated amounts are correct u Prompts and displayed results match the design 25 Copyright 2017 by Janson Industries
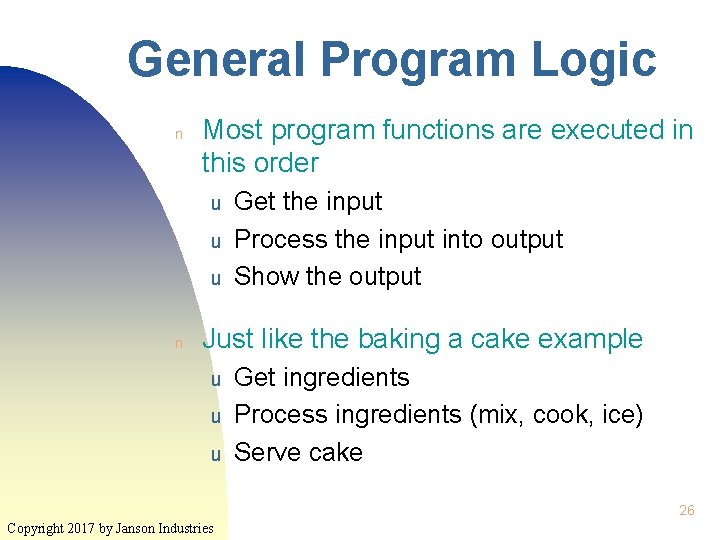
General Program Logic n Most program functions are executed in this order u u u n Get the input Process the input into output Show the output Just like the baking a cake example u u u Get ingredients Process ingredients (mix, cook, ice) Serve cake 26 Copyright 2017 by Janson Industries
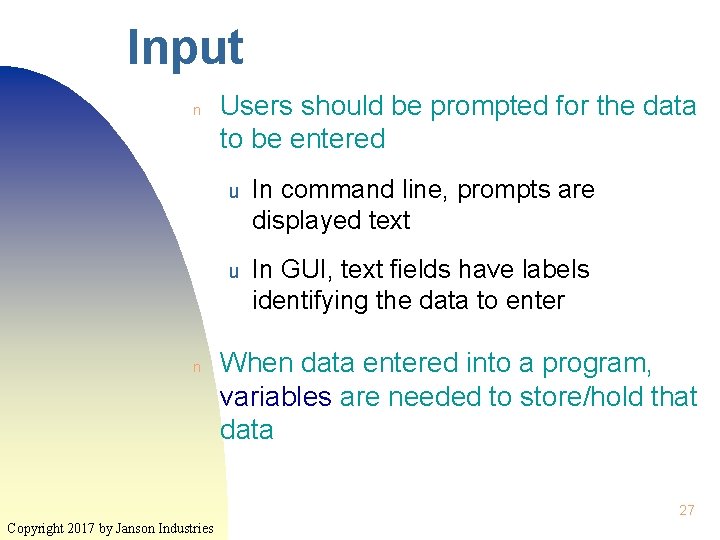
Input n n Users should be prompted for the data to be entered u In command line, prompts are displayed text u In GUI, text fields have labels identifying the data to enter When data entered into a program, variables are needed to store/hold that data 27 Copyright 2017 by Janson Industries
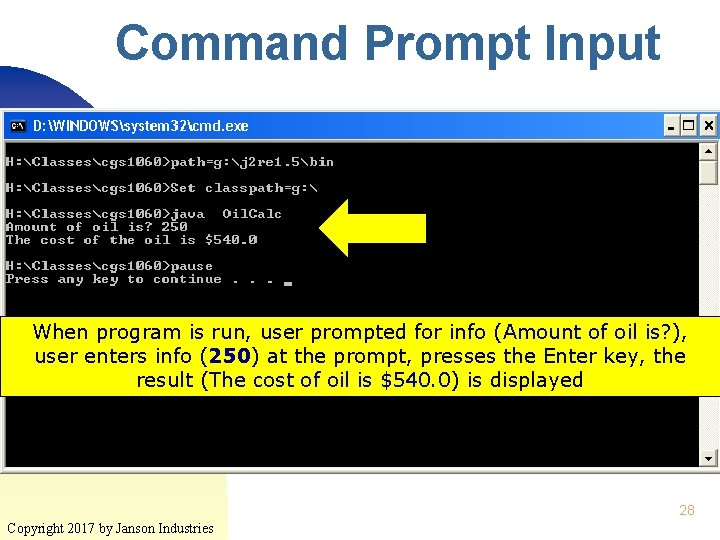
Command Prompt Input When program is run, user prompted for info (Amount of oil is? ), user enters info (250) at the prompt, presses the Enter key, the result (The cost of oil is $540. 0) is displayed 28 Copyright 2017 by Janson Industries
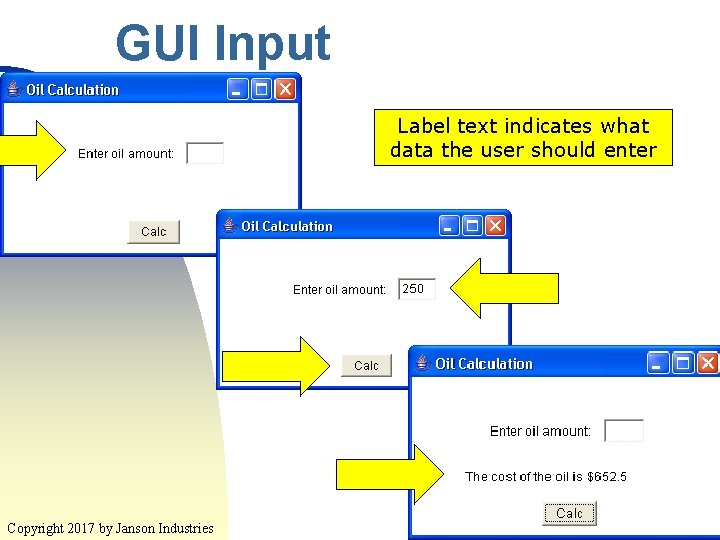
GUI Input Label text indicates what data the user should enter 29 Copyright 2017 by Janson Industries
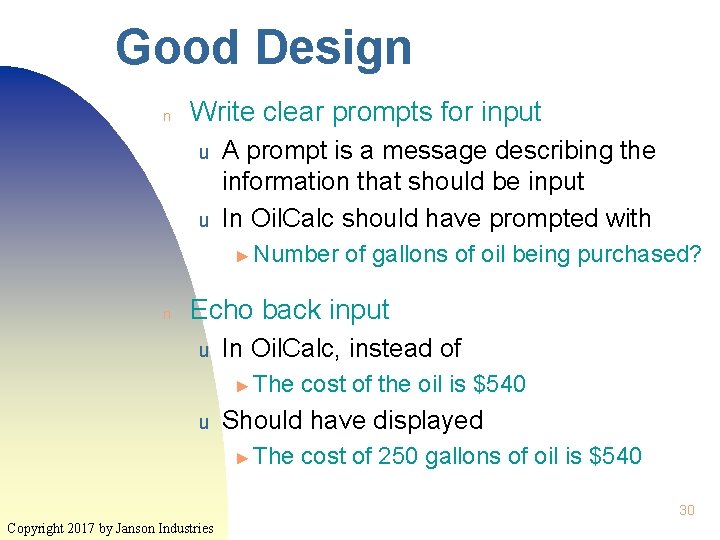
Good Design n Write clear prompts for input u u A prompt is a message describing the information that should be input In Oil. Calc should have prompted with ► Number n of gallons of oil being purchased? Echo back input u In Oil. Calc, instead of ► The u cost of the oil is $540 Should have displayed ► The cost of 250 gallons of oil is $540 30 Copyright 2017 by Janson Industries
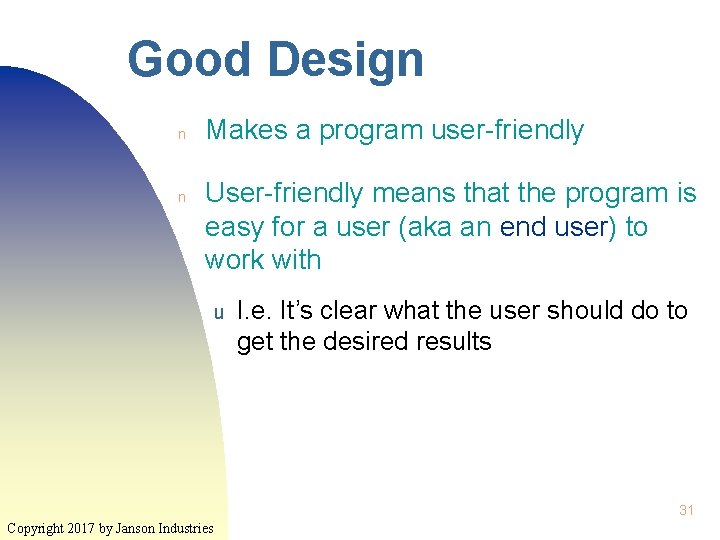
Good Design n n Makes a program user-friendly User-friendly means that the program is easy for a user (aka an end user) to work with u I. e. It’s clear what the user should do to get the desired results 31 Copyright 2017 by Janson Industries
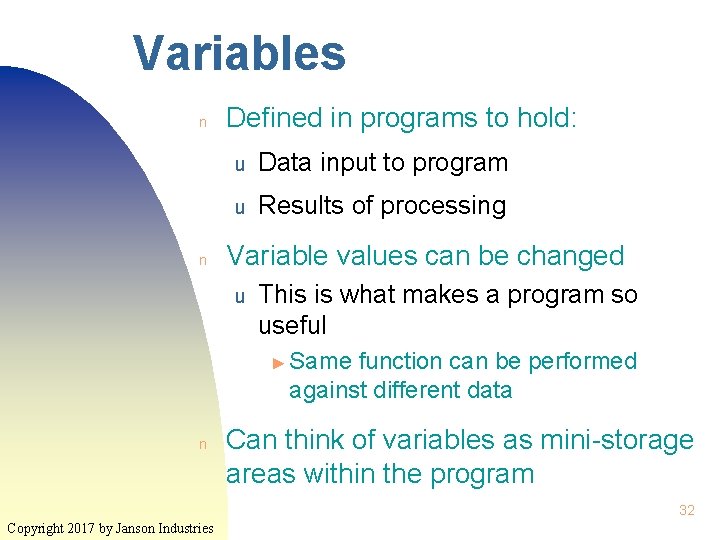
Variables n n Defined in programs to hold: u Data input to program u Results of processing Variable values can be changed u This is what makes a program so useful ► Same function can be performed against different data n Can think of variables as mini-storage areas within the program 32 Copyright 2017 by Janson Industries
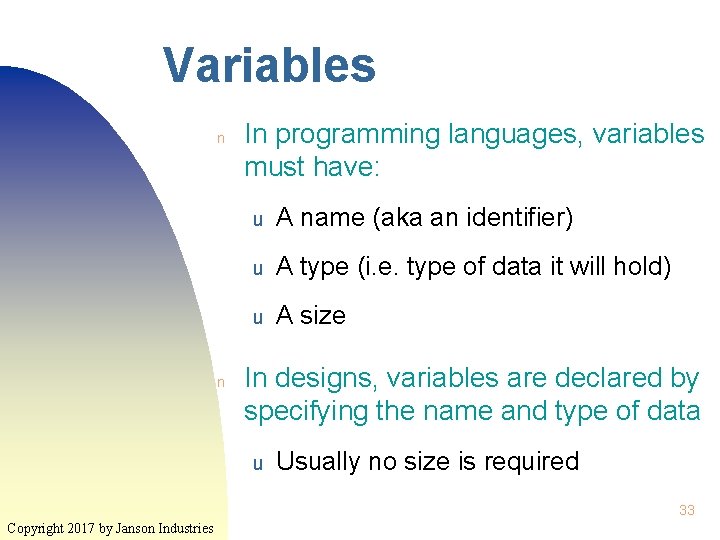
Variables n n In programming languages, variables must have: u A name (aka an identifier) u A type (i. e. type of data it will hold) u A size In designs, variables are declared by specifying the name and type of data u Usually no size is required 33 Copyright 2017 by Janson Industries
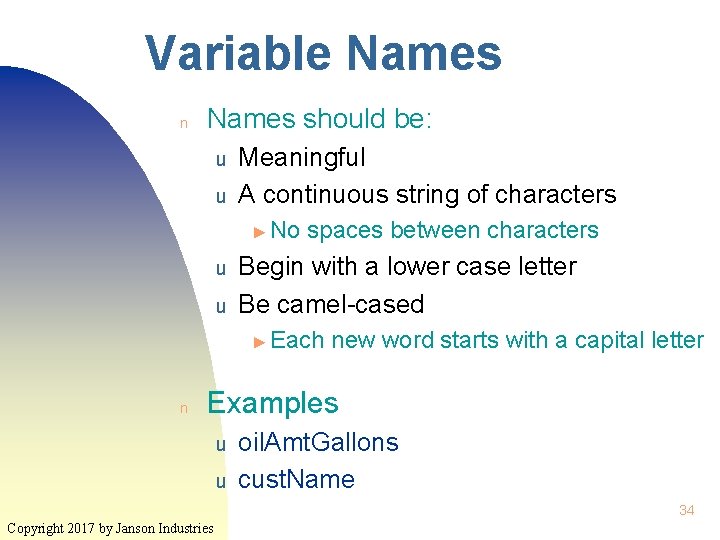
Variable Names n Names should be: u u Meaningful A continuous string of characters ► No u u spaces between characters Begin with a lower case letter Be camel-cased ► Each n new word starts with a capital letter Examples u u oil. Amt. Gallons cust. Name 34 Copyright 2017 by Janson Industries
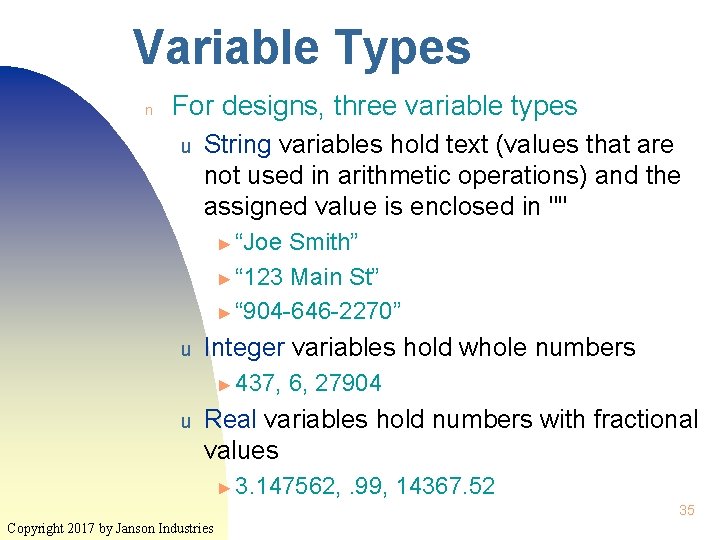
Variable Types n For designs, three variable types u String variables hold text (values that are not used in arithmetic operations) and the assigned value is enclosed in "" ► “Joe Smith” ► “ 123 Main St” ► “ 904 -646 -2270” u Integer variables hold whole numbers ► 437, u 6, 27904 Real variables hold numbers with fractional values ► 3. 147562, . 99, 14367. 52 35 Copyright 2017 by Janson Industries
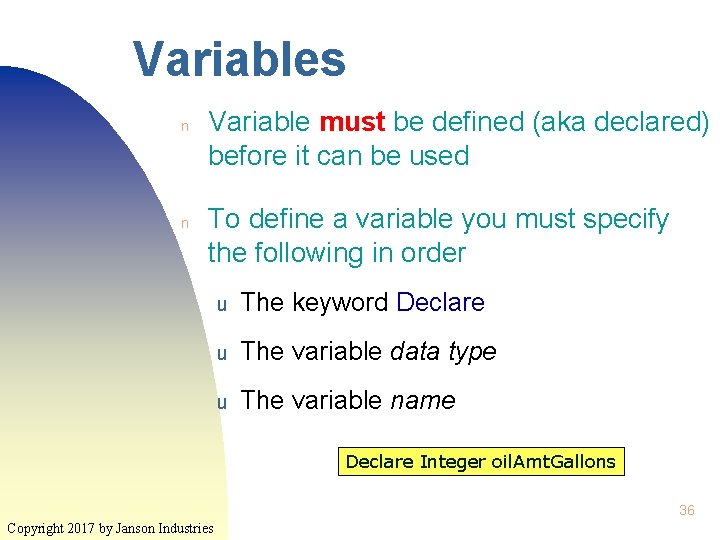
Variables n n Variable must be defined (aka declared) before it can be used To define a variable you must specify the following in order u The keyword Declare u The variable data type u The variable name Declare Integer oil. Amt. Gallons 36 Copyright 2017 by Janson Industries
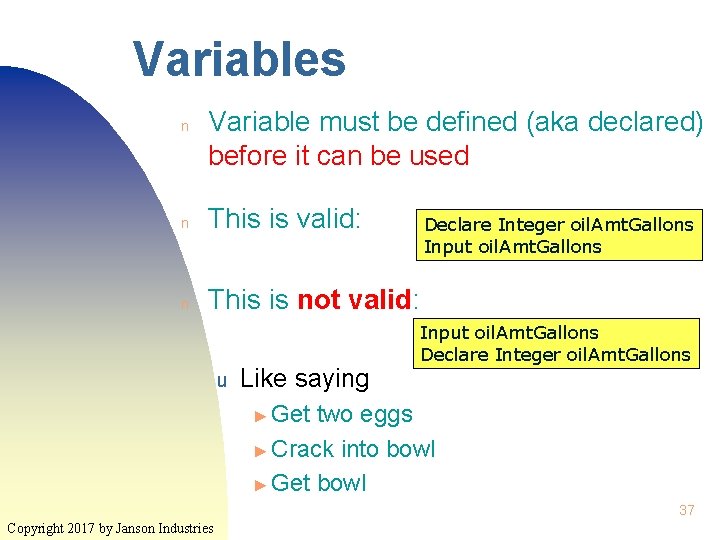
Variables n Variable must be defined (aka declared) before it can be used n This is valid: n This is not valid: u Like saying Declare Integer oil. Amt. Gallons Input oil. Amt. Gallons Declare Integer oil. Amt. Gallons ► Get two eggs ► Crack into bowl ► Get bowl 37 Copyright 2017 by Janson Industries
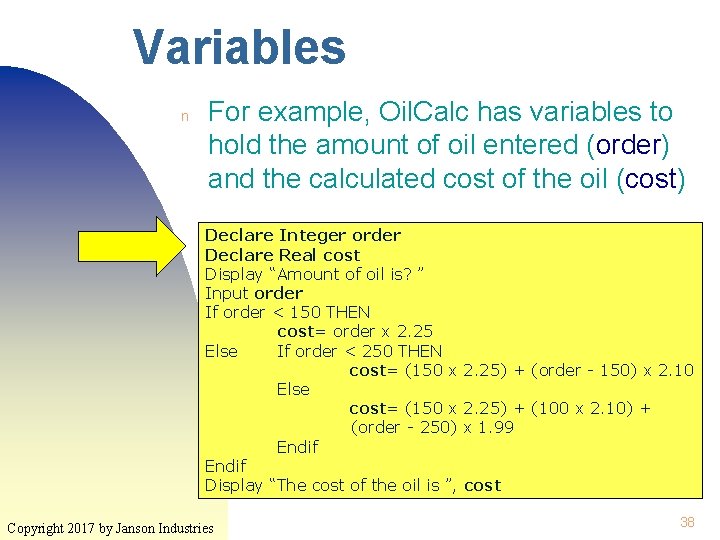
Variables n For example, Oil. Calc has variables to hold the amount of oil entered (order) and the calculated cost of the oil (cost) Declare Integer order Declare Real cost Display “Amount of oil is? ” Input order If order < 150 THEN cost= order x 2. 25 Else If order < 250 THEN cost= (150 x Else cost= (150 x (order - 250) Endif Display “The cost of the oil is ”, Copyright 2017 by Janson Industries 2. 25) + (order - 150) x 2. 10 2. 25) + (100 x 2. 10) + x 1. 99 cost 38
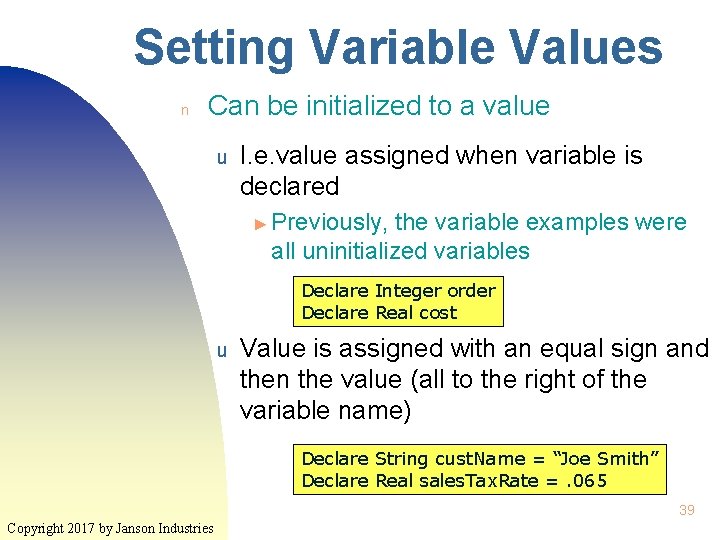
Setting Variable Values n Can be initialized to a value u I. e. value assigned when variable is declared ► Previously, the variable examples were all uninitialized variables Declare Integer order Declare Real cost u Value is assigned with an equal sign and then the value (all to the right of the variable name) Declare String cust. Name = “Joe Smith” Declare Real sales. Tax. Rate =. 065 39 Copyright 2017 by Janson Industries
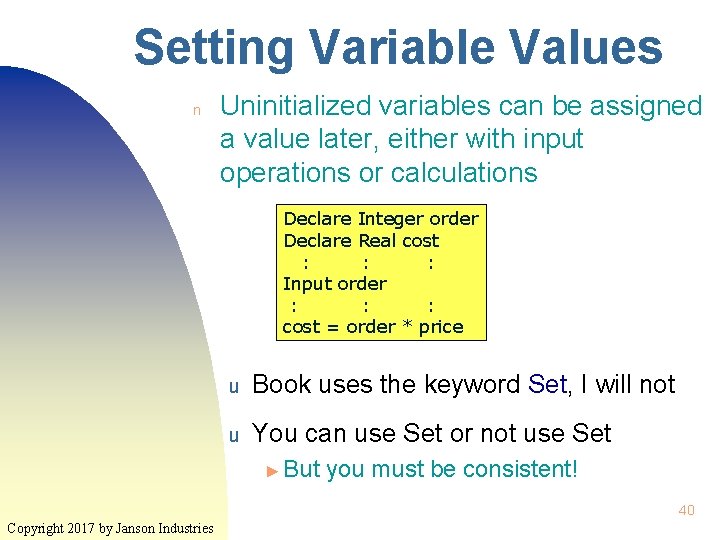
Setting Variable Values n Uninitialized variables can be assigned a value later, either with input operations or calculations Declare Integer order Declare Real cost : : : Input order : : : cost = order * price u Book uses the keyword Set, I will not u You can use Set or not use Set ► But you must be consistent! 40 Copyright 2017 by Janson Industries
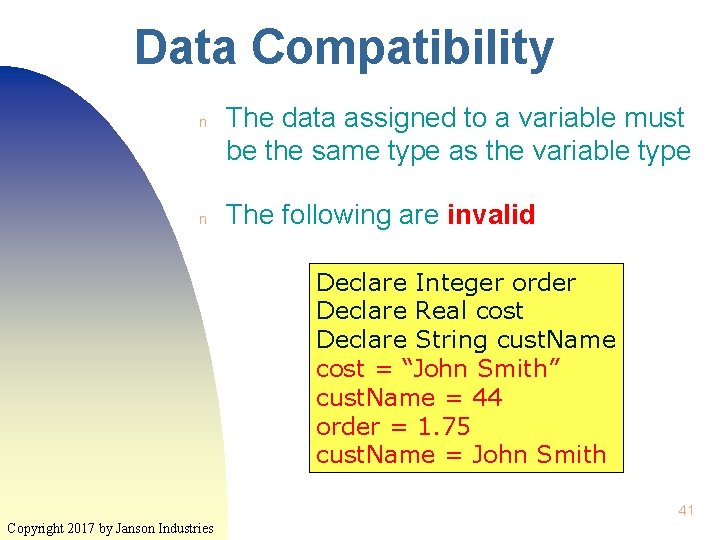
Data Compatibility n n The data assigned to a variable must be the same type as the variable type The following are invalid Declare Integer order Declare Real cost Declare String cust. Name cost = “John Smith” cust. Name = 44 order = 1. 75 cust. Name = John Smith 41 Copyright 2017 by Janson Industries
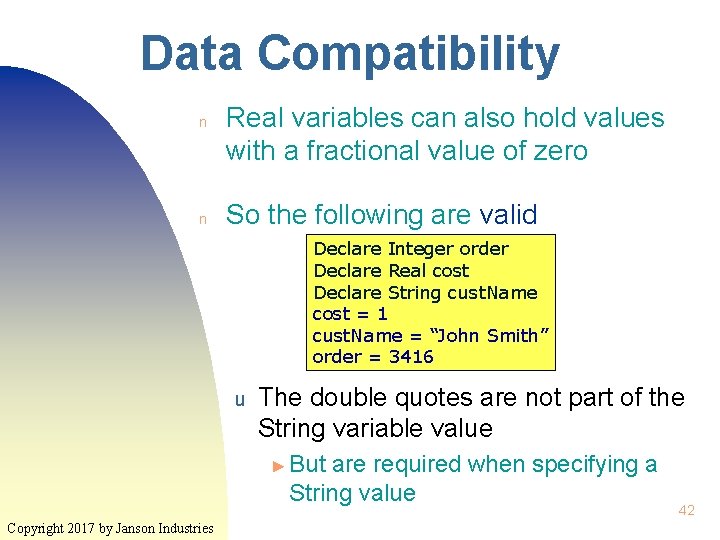
Data Compatibility n n Real variables can also hold values with a fractional value of zero So the following are valid Declare Integer order Declare Real cost Declare String cust. Name cost = 1 cust. Name = “John Smith” order = 3416 u The double quotes are not part of the String variable value ► But are required when specifying a String value Copyright 2017 by Janson Industries 42
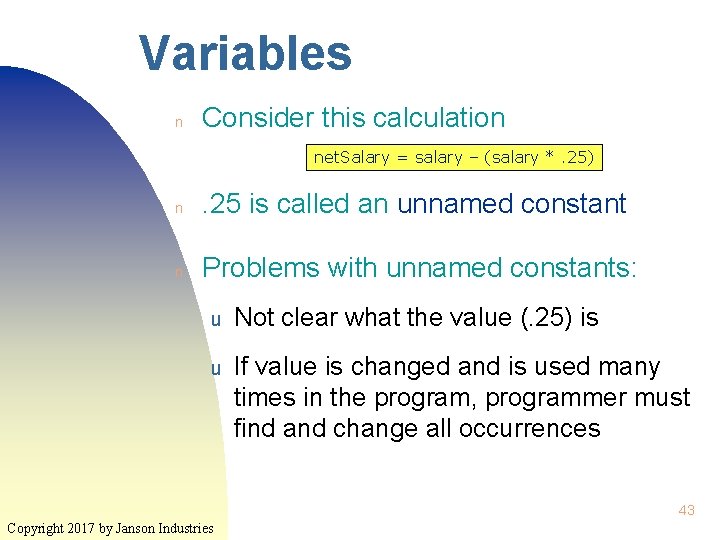
Variables n Consider this calculation net. Salary = salary – (salary *. 25) n . 25 is called an unnamed constant n Problems with unnamed constants: u Not clear what the value (. 25) is u If value is changed and is used many times in the program, programmer must find and change all occurrences 43 Copyright 2017 by Janson Industries
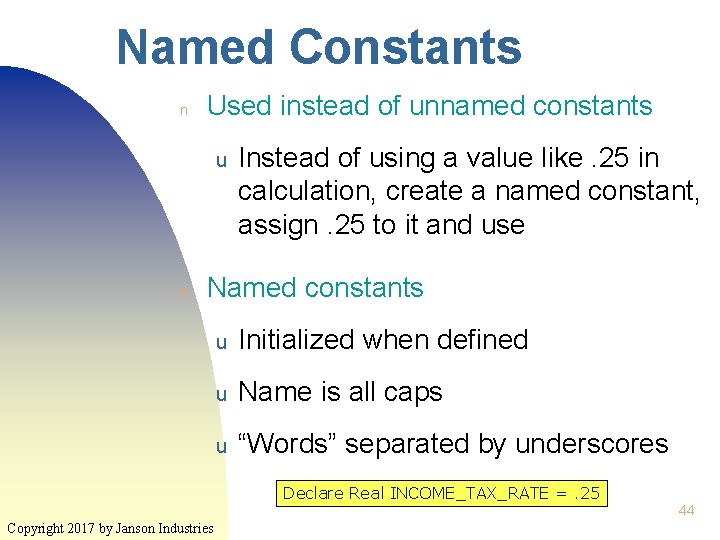
Named Constants n Used instead of unnamed constants u n Instead of using a value like. 25 in calculation, create a named constant, assign. 25 to it and use Named constants u Initialized when defined u Name is all caps u “Words” separated by underscores Declare Real INCOME_TAX_RATE =. 25 Copyright 2017 by Janson Industries 44
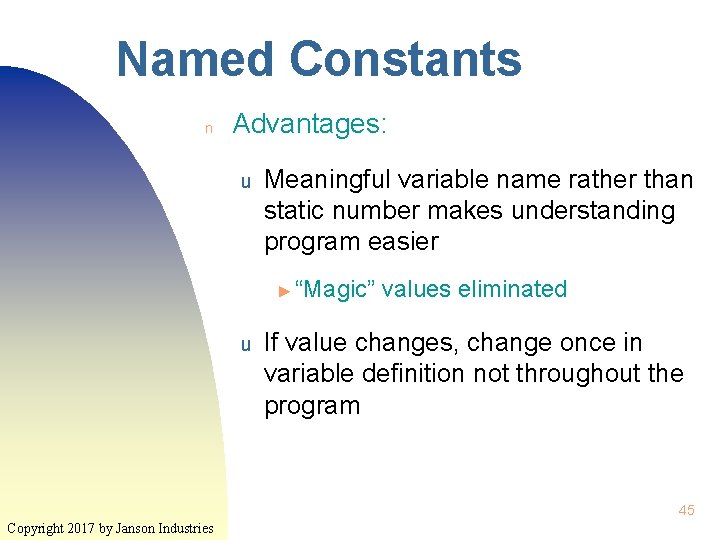
Named Constants n Advantages: u Meaningful variable name rather than static number makes understanding program easier ► “Magic” u values eliminated If value changes, change once in variable definition not throughout the program 45 Copyright 2017 by Janson Industries
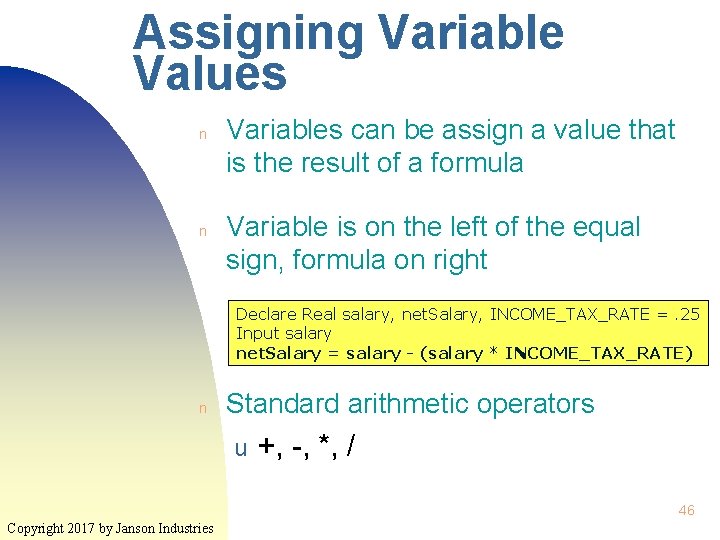
Assigning Variable Values n n Variables can be assign a value that is the result of a formula Variable is on the left of the equal sign, formula on right Declare Real salary, net. Salary, INCOME_TAX_RATE =. 25 Input salary net. Salary = salary - (salary * INCOME_TAX_RATE) n Standard arithmetic operators u +, -, *, / 46 Copyright 2017 by Janson Industries
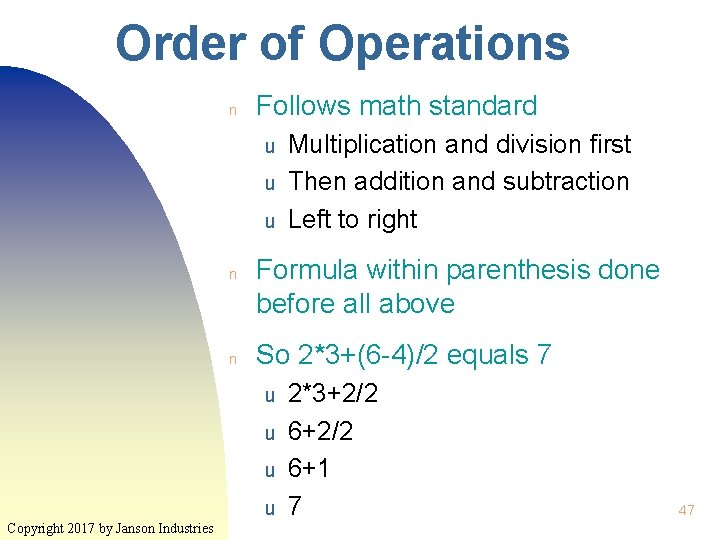
Order of Operations n Follows math standard u u u n n Formula within parenthesis done before all above So 2*3+(6 -4)/2 equals 7 u u Copyright 2017 by Janson Industries Multiplication and division first Then addition and subtraction Left to right 2*3+2/2 6+1 7 47
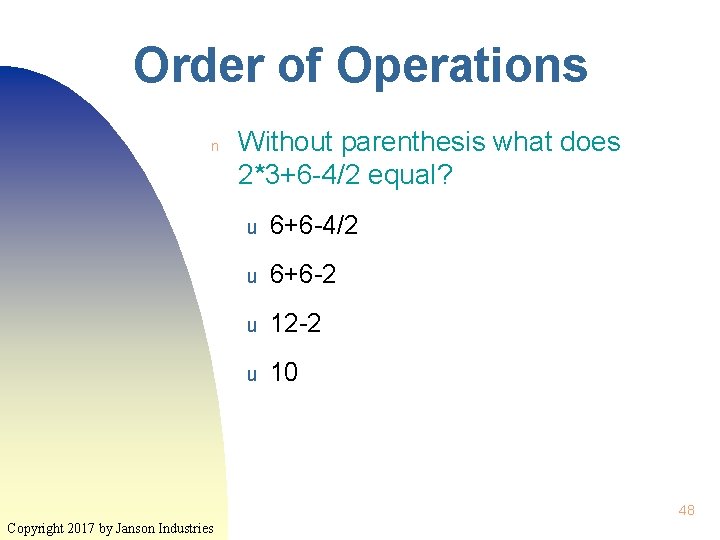
Order of Operations n Without parenthesis what does 2*3+6 -4/2 equal? u 6+6 -4/2 u 6+6 -2 u 12 -2 u 10 48 Copyright 2017 by Janson Industries
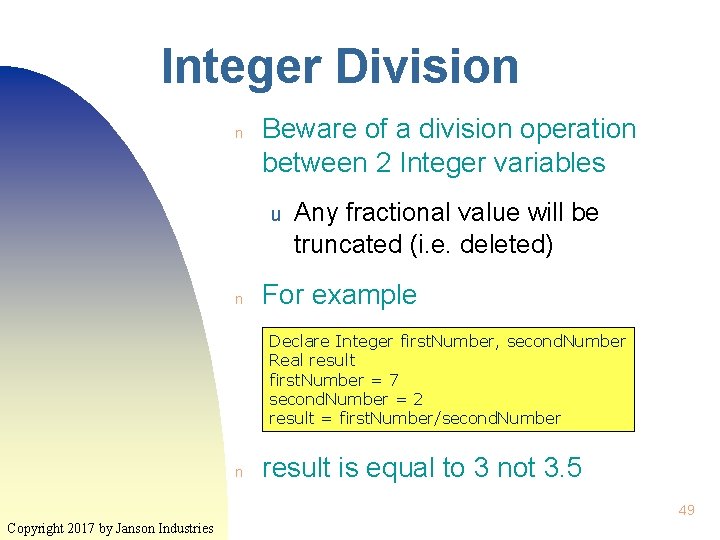
Integer Division n Beware of a division operation between 2 Integer variables u n Any fractional value will be truncated (i. e. deleted) For example Declare Integer first. Number, second. Number Real result first. Number = 7 second. Number = 2 result = first. Number/second. Number n result is equal to 3 not 3. 5 49 Copyright 2017 by Janson Industries
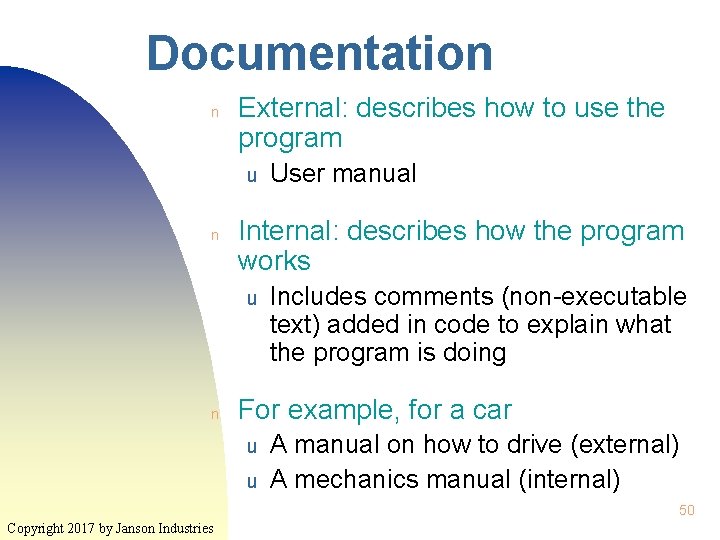
Documentation n External: describes how to use the program u n Internal: describes how the program works u n User manual Includes comments (non-executable text) added in code to explain what the program is doing For example, for a car u u A manual on how to drive (external) A mechanics manual (internal) 50 Copyright 2017 by Janson Industries
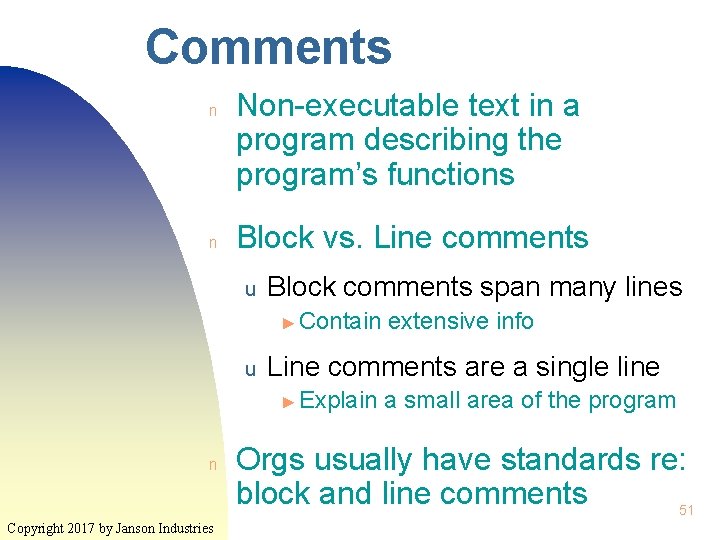
Comments n n Non-executable text in a program describing the program’s functions Block vs. Line comments u Block comments span many lines ► Contain u Line comments are a single line ► Explain n Copyright 2017 by Janson Industries extensive info a small area of the program Orgs usually have standards re: block and line comments 51
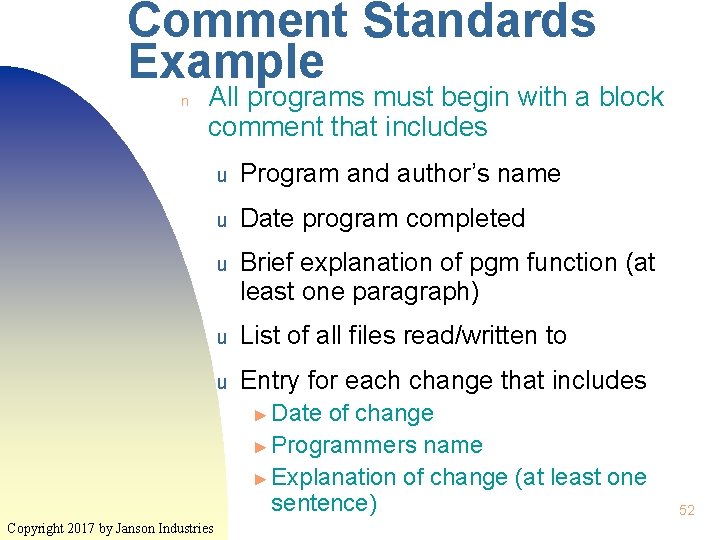
Comment Standards Example n All programs must begin with a block comment that includes u Program and author’s name u Date program completed u Brief explanation of pgm function (at least one paragraph) u List of all files read/written to u Entry for each change that includes ► Date of change ► Programmers name ► Explanation of change (at least one sentence) Copyright 2017 by Janson Industries 52
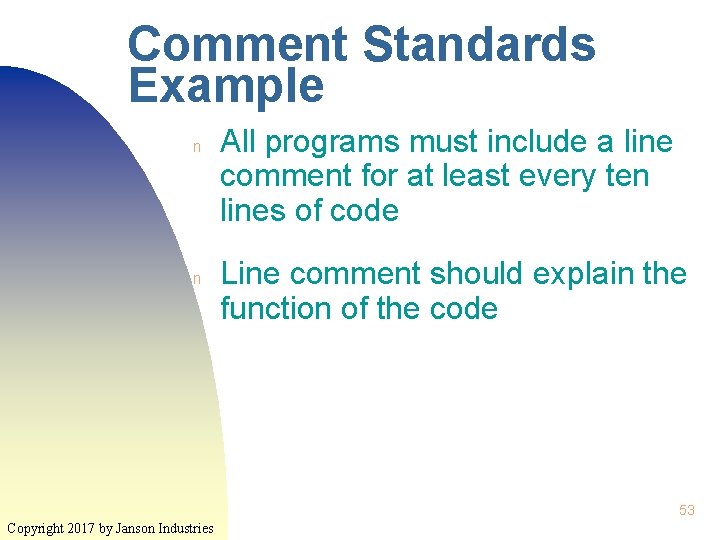
Comment Standards Example n n All programs must include a line comment for at least every ten lines of code Line comment should explain the function of the code 53 Copyright 2017 by Janson Industries
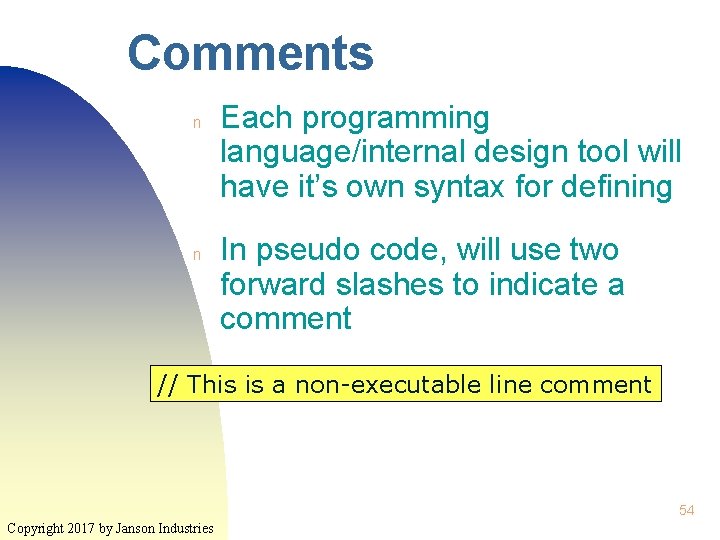
Comments n n Each programming language/internal design tool will have it’s own syntax for defining In pseudo code, will use two forward slashes to indicate a comment // This is a non-executable line comment 54 Copyright 2017 by Janson Industries
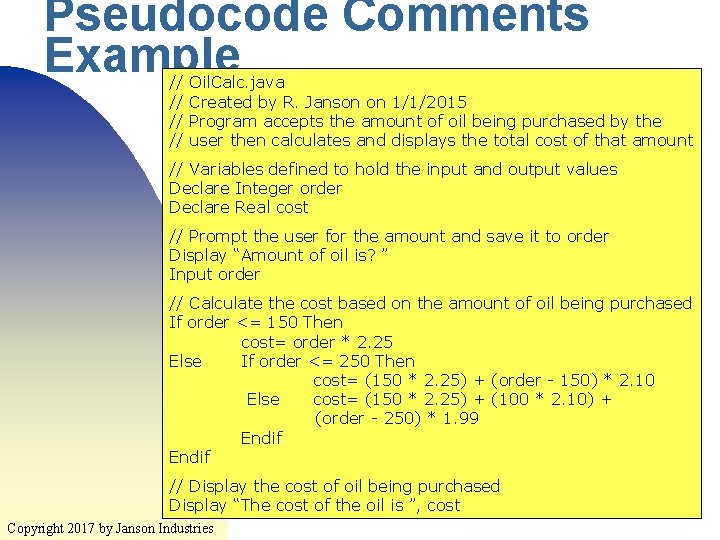
Pseudocode Comments Example // // Oil. Calc. java Created by R. Janson on 1/1/2015 Program accepts the amount of oil being purchased by the user then calculates and displays the total cost of that amount // Variables defined to hold the input and output values Declare Integer order Declare Real cost // Prompt the user for the amount and save it to order Display “Amount of oil is? ” Input order // Calculate the cost based on the amount of oil being purchased If order <= 150 Then cost= order * 2. 25 Else If order <= 250 Then cost= (150 * 2. 25) + (order - 150) * 2. 10 Else cost= (150 * 2. 25) + (100 * 2. 10) + (order - 250) * 1. 99 Endif // Display the cost of oil being purchased Display “The cost of the oil is ”, cost Copyright 2017 by Janson Industries 55
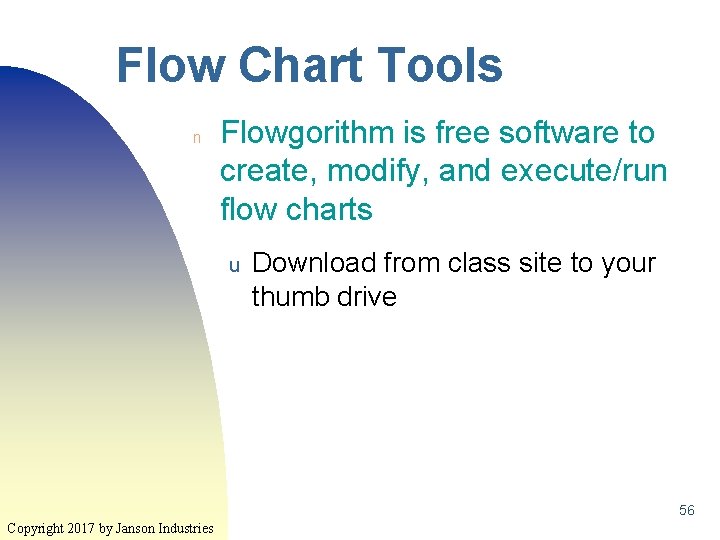
Flow Chart Tools n Flowgorithm is free software to create, modify, and execute/run flow charts u Download from class site to your thumb drive 56 Copyright 2017 by Janson Industries
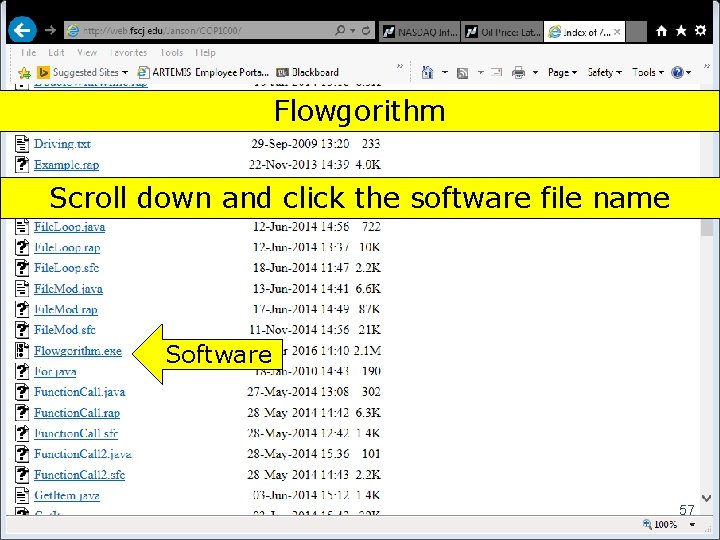
Flowgorithm Scroll down and click the software file name Software 57 Copyright 2017 by Janson Industries
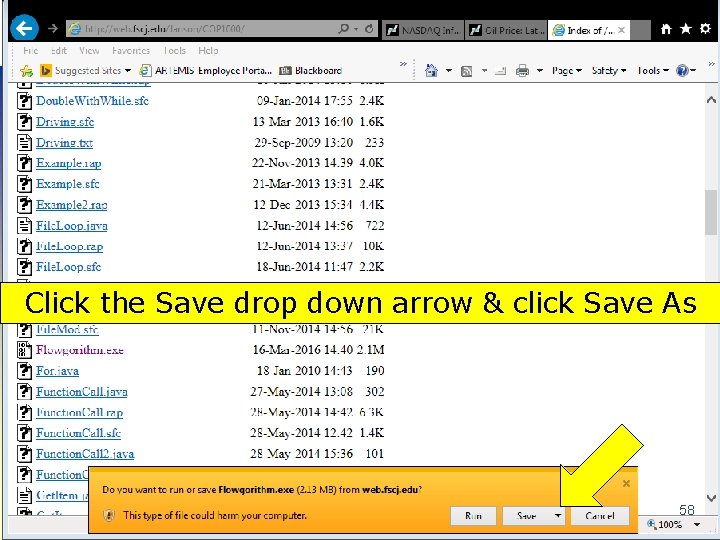
Click the Save drop down arrow & click Save As 58 Copyright 2017 by Janson Industries
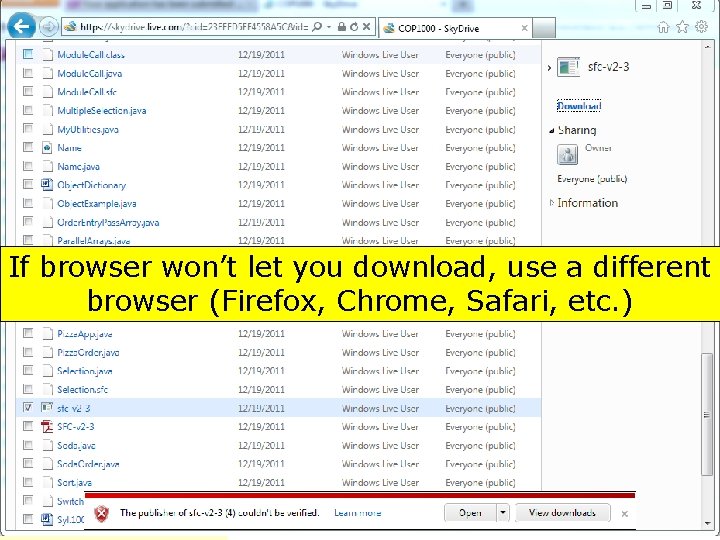
If browser won’t let you download, use a different browser (Firefox, Chrome, Safari, etc. ) 59 Copyright 2017 by Janson Industries
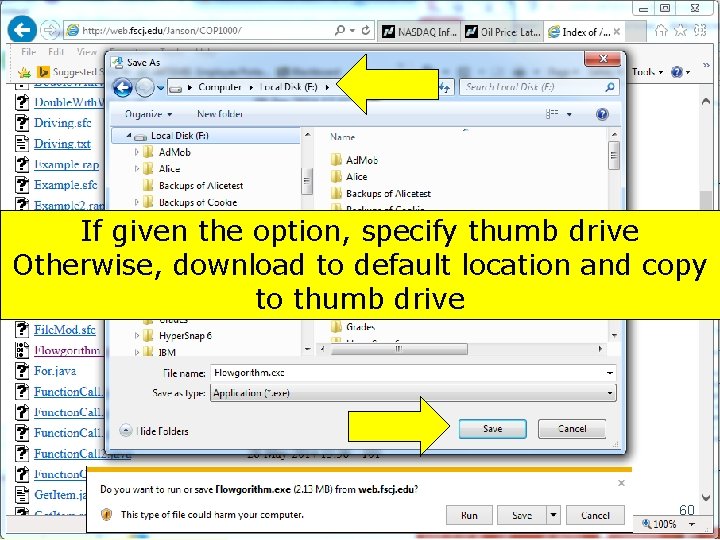
If given the option, specify thumb drive Otherwise, download to default location and copy to thumb drive 60 Copyright 2017 by Janson Industries
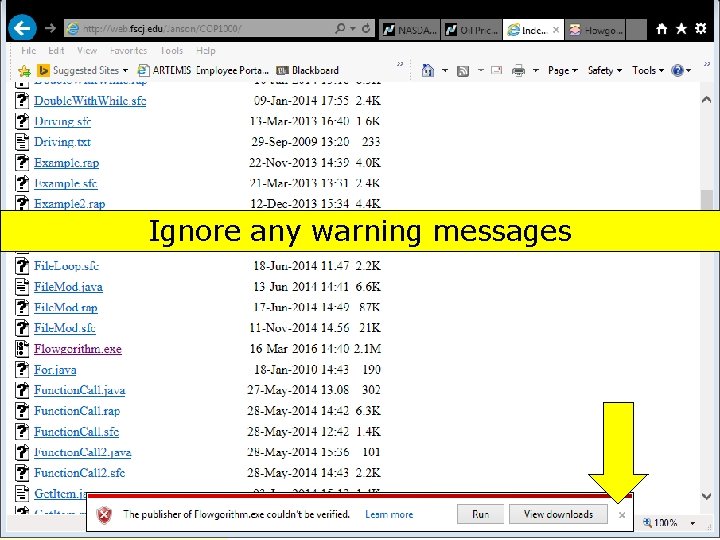
Ignore any warning messages 61 Copyright 2017 by Janson Industries
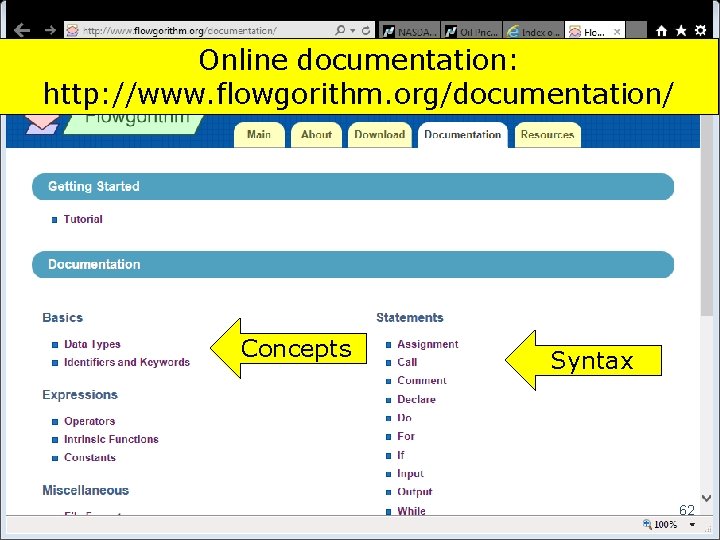
Online documentation: http: //www. flowgorithm. org/documentation/ Concepts Syntax 62 Copyright 2017 by Janson Industries
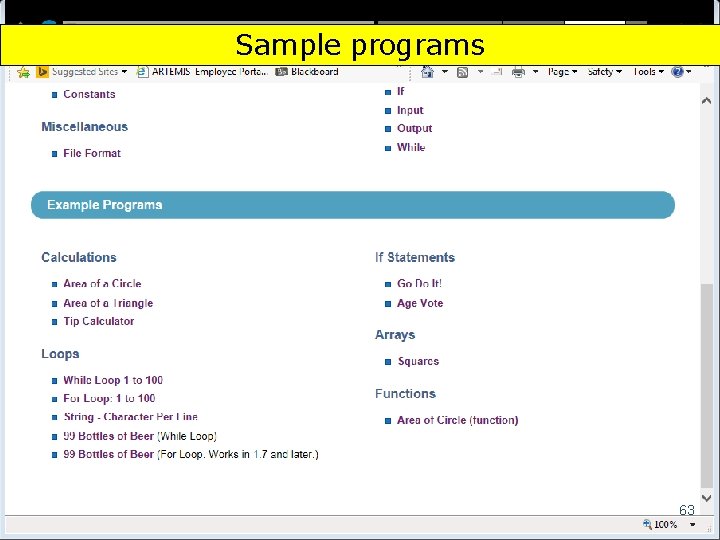
Sample programs 63 Copyright 2017 by Janson Industries
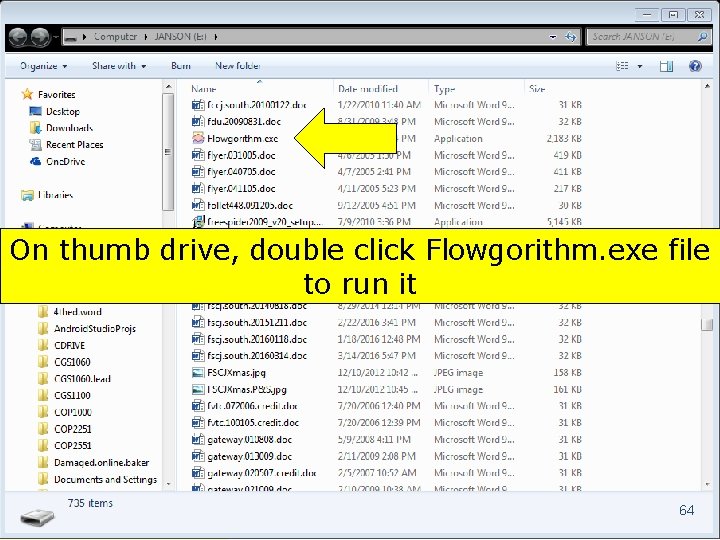
On thumb drive, double click Flowgorithm. exe file to run it 64 Copyright 2017 by Janson Industries
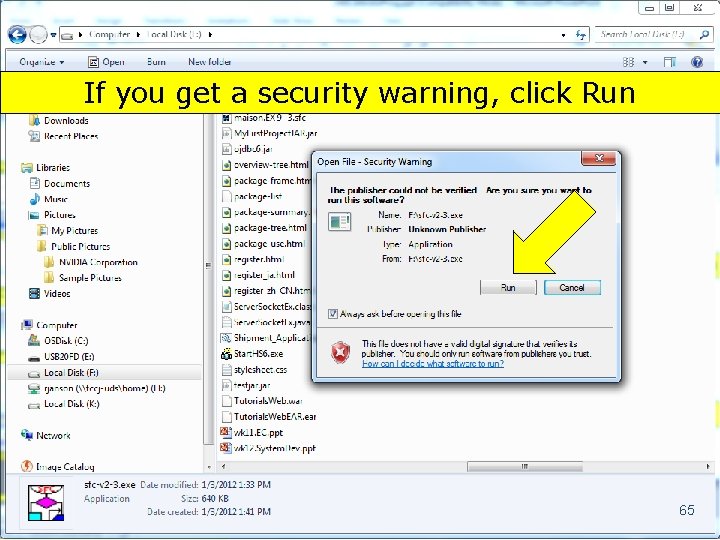
If you get a security warning, click Run 65 Copyright 2017 by Janson Industries
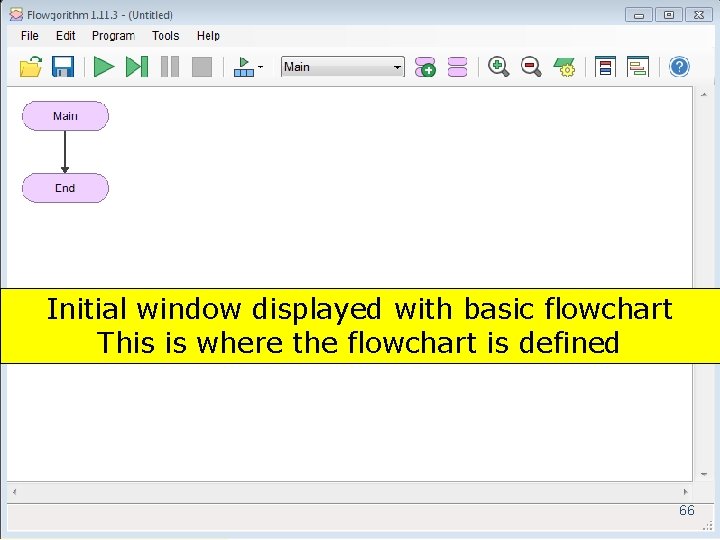
Initial window displayed with basic flowchart This is where the flowchart is defined 66 Copyright 2017 by Janson Industries
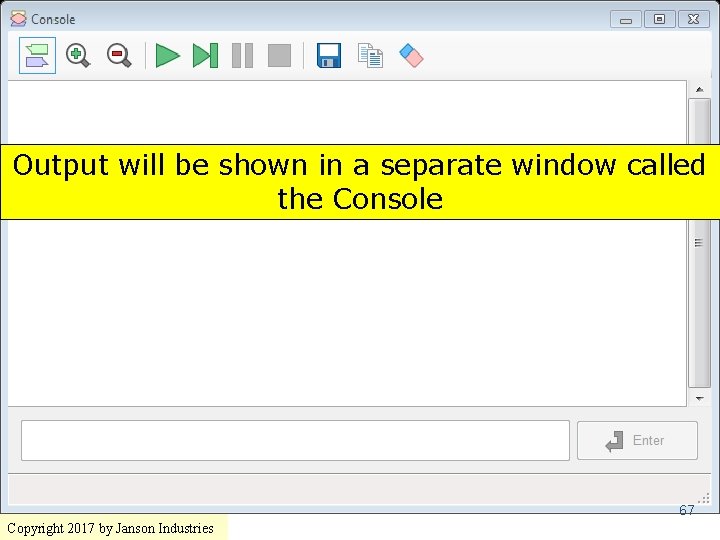
Output will be shown in a separate window called the Console 67 Copyright 2017 by Janson Industries
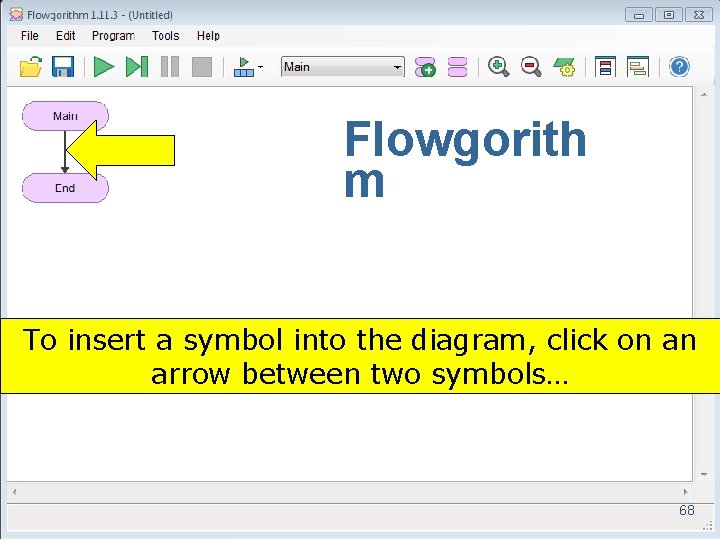
Flowgorith m To insert a symbol into the diagram, click on an arrow between two symbols… 68 Copyright 2017 by Janson Industries
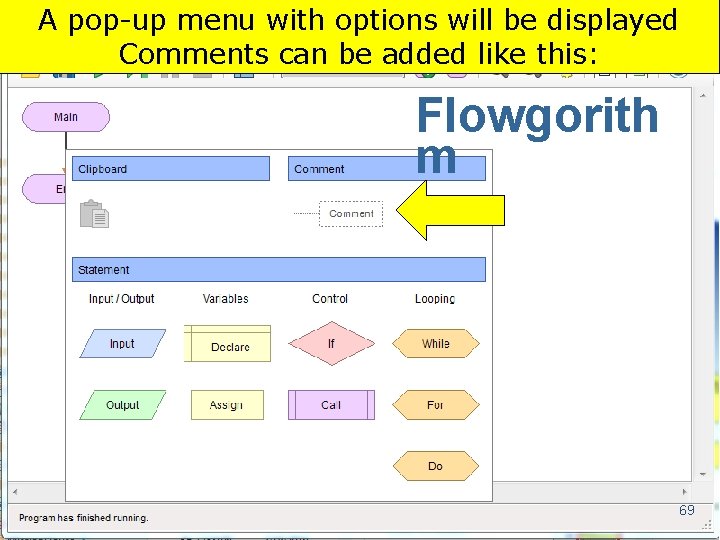
A pop-up menu with options will be displayed Comments can be added like this: Flowgorith m 69 Copyright 2017 by Janson Industries
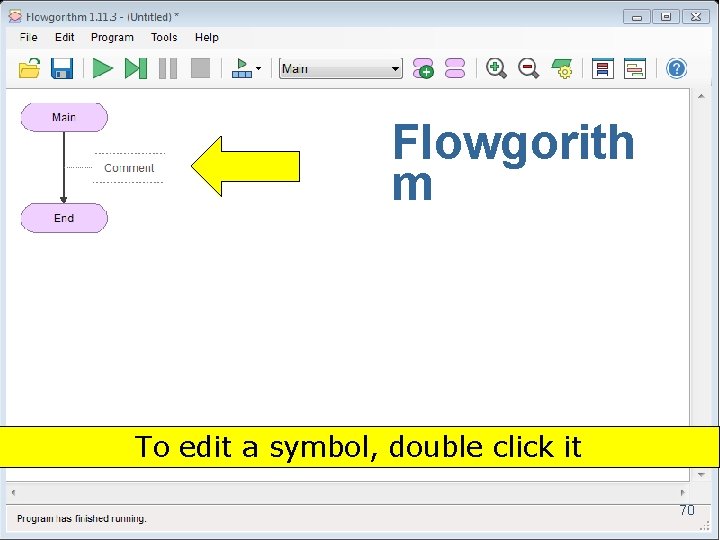
Flowgorith m To edit a symbol, double click it 70 Copyright 2017 by Janson Industries
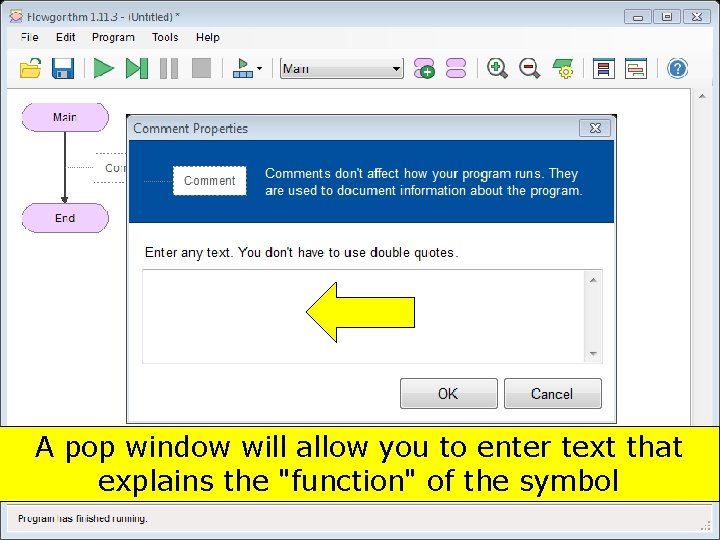
A pop window will allow you to enter text that explains the "function" of the symbol 71 Copyright 2017 by Janson Industries
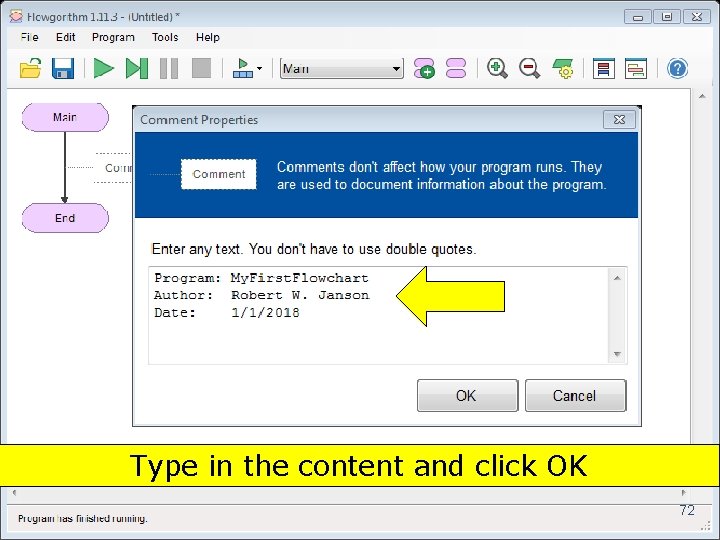
Type in the content and click OK 72 Copyright 2017 by Janson Industries
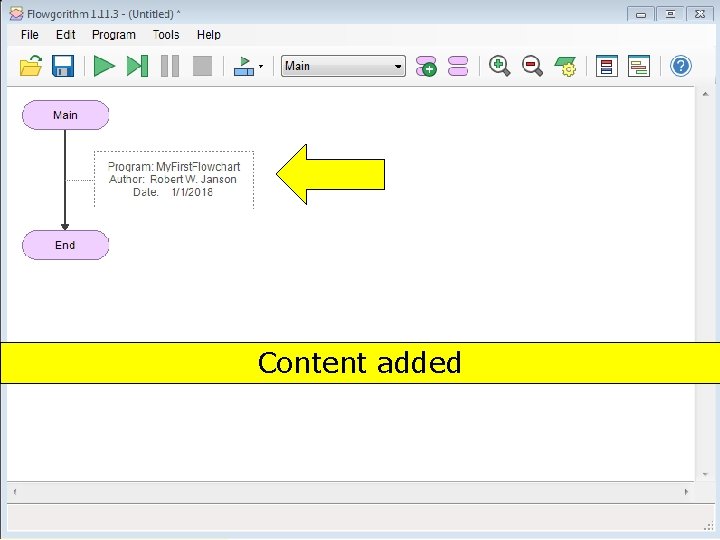
Content added 73 Copyright 2017 by Janson Industries
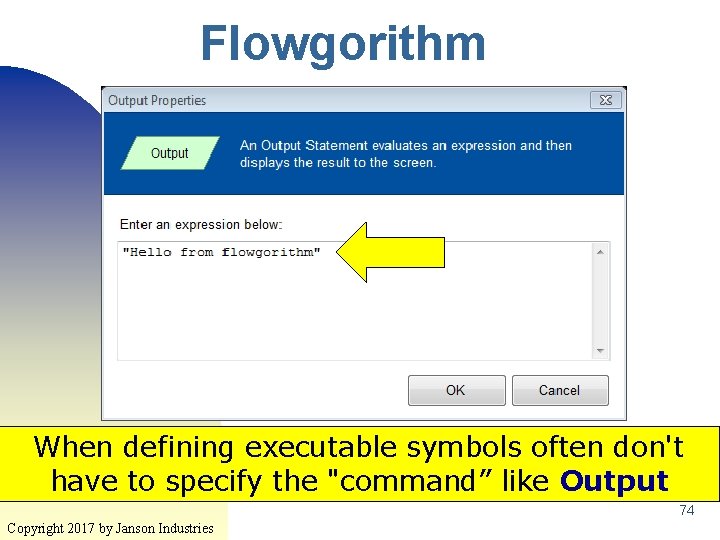
Flowgorithm When defining executable symbols often don't have to specify the "command” like Output 74 Copyright 2017 by Janson Industries
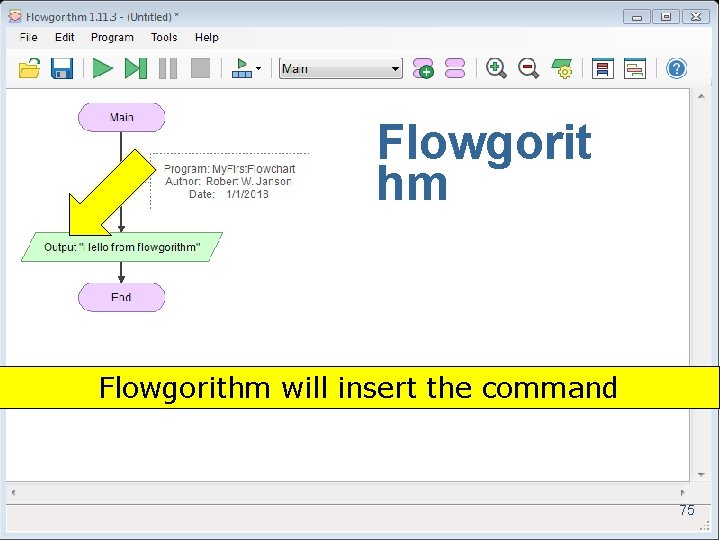
Flowgorit hm Flowgorithm will insert the command 75 Copyright 2017 by Janson Industries
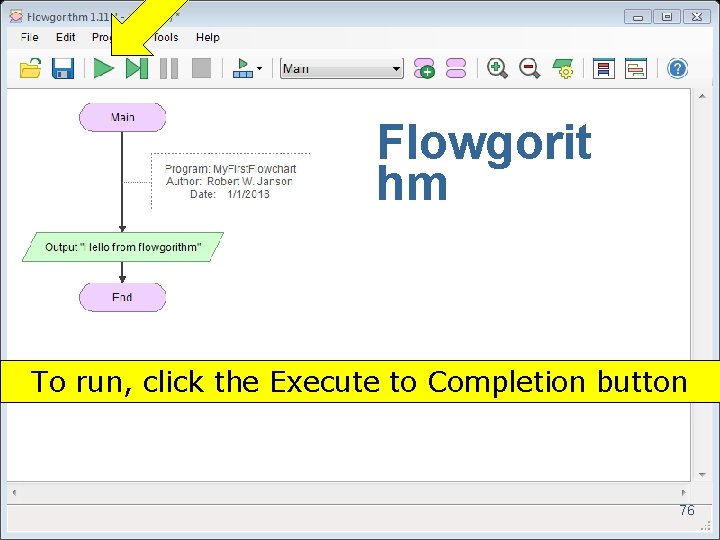
Flowgorit hm To run, click the Execute to Completion button 76 Copyright 2017 by Janson Industries
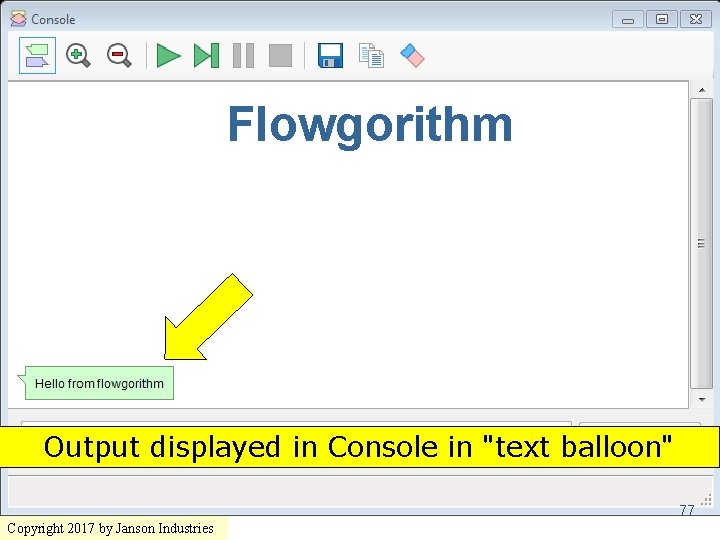
Flowgorithm Output displayed in Console in "text balloon" 77 Copyright 2017 by Janson Industries
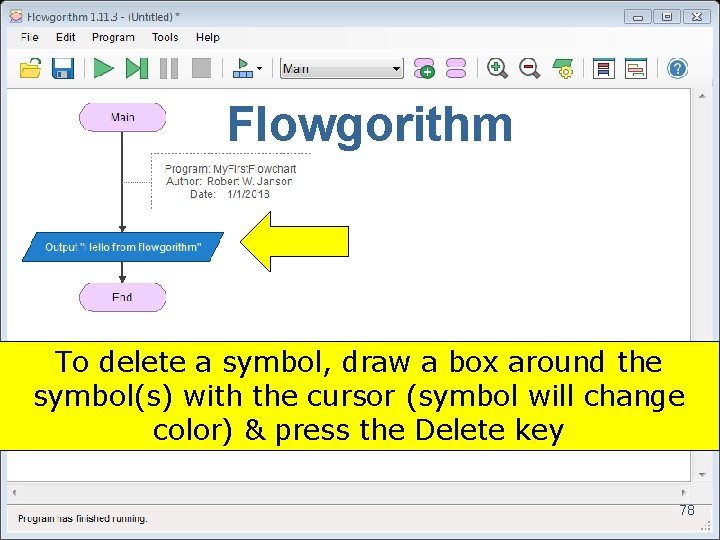
Flowgorithm To delete a symbol, draw a box around the symbol(s) with the cursor (symbol will change color) & press the Delete key 78 Copyright 2017 by Janson Industries
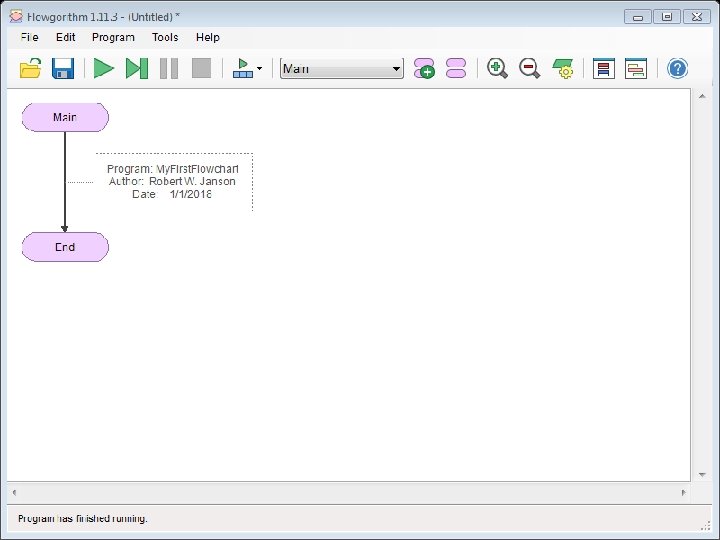
79 Copyright 2017 by Janson Industries
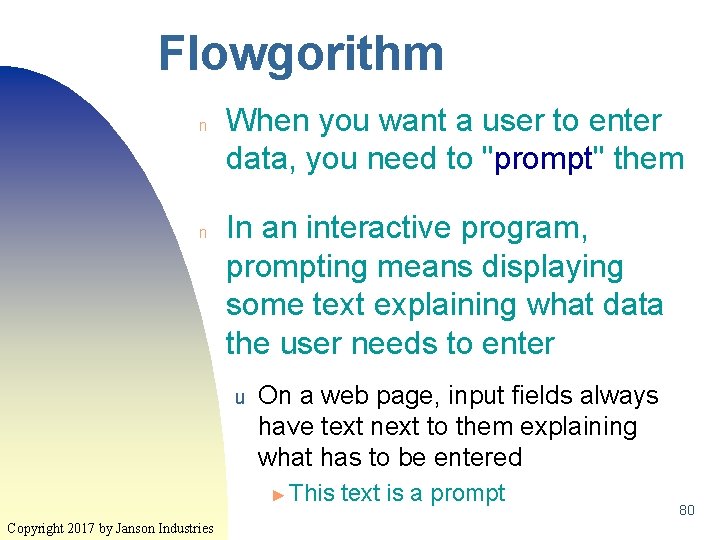
Flowgorithm n n When you want a user to enter data, you need to "prompt" them In an interactive program, prompting means displaying some text explaining what data the user needs to enter u On a web page, input fields always have text next to them explaining what has to be entered ► This Copyright 2017 by Janson Industries text is a prompt 80
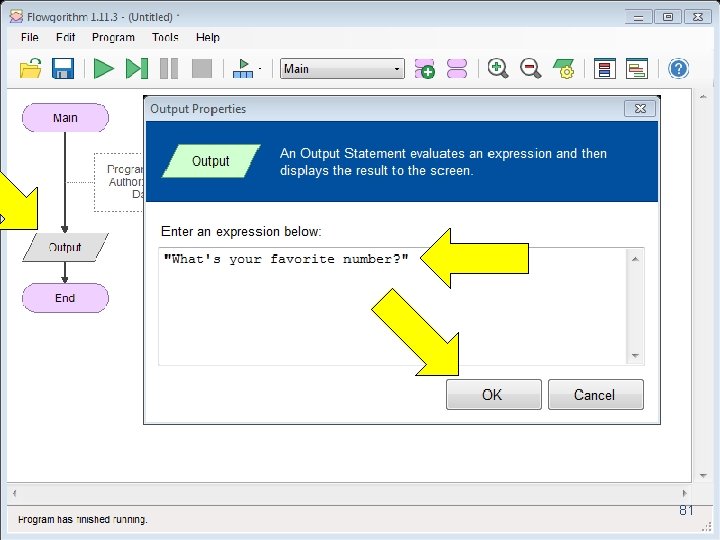
81 Copyright 2017 by Janson Industries
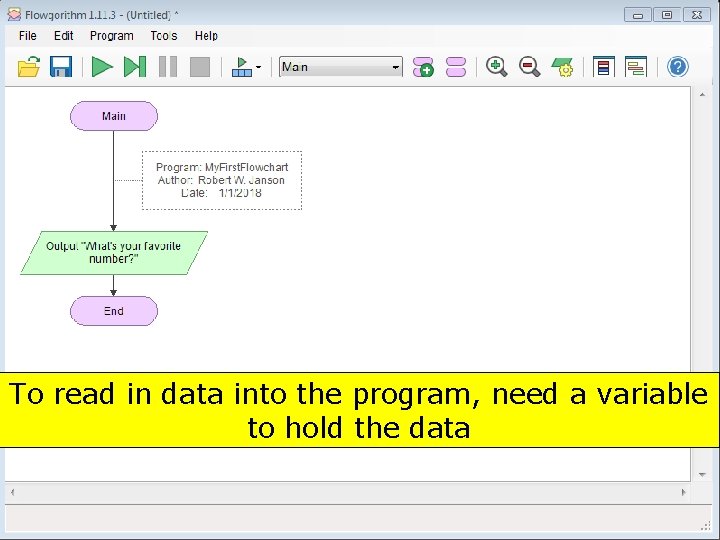
To read in data into the program, need a variable to hold the data 82 Copyright 2017 by Janson Industries
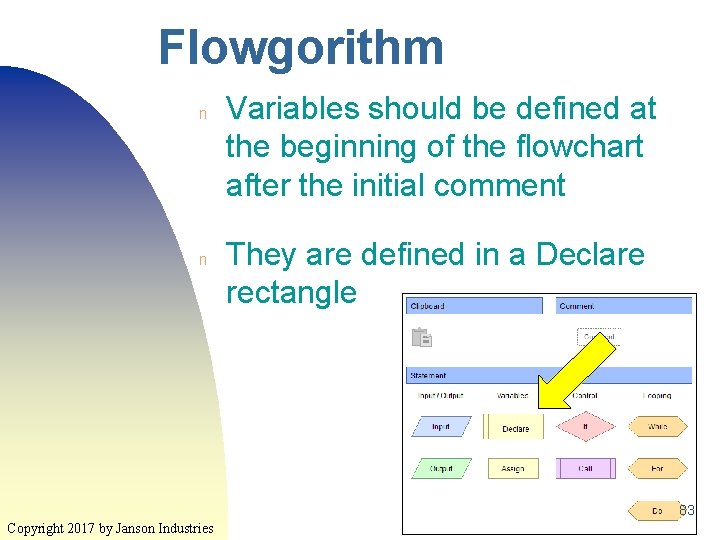
Flowgorithm n n Variables should be defined at the beginning of the flowchart after the initial comment They are defined in a Declare rectangle 83 Copyright 2017 by Janson Industries
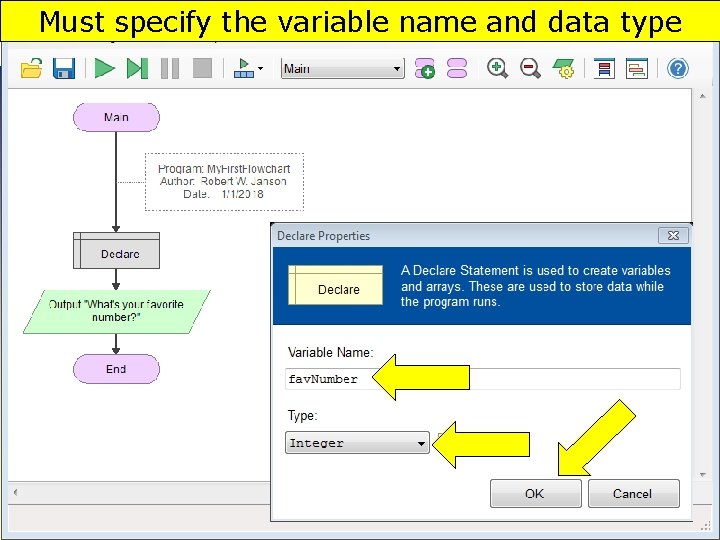
Must specify the variable name and data type 84 Copyright 2017 by Janson Industries
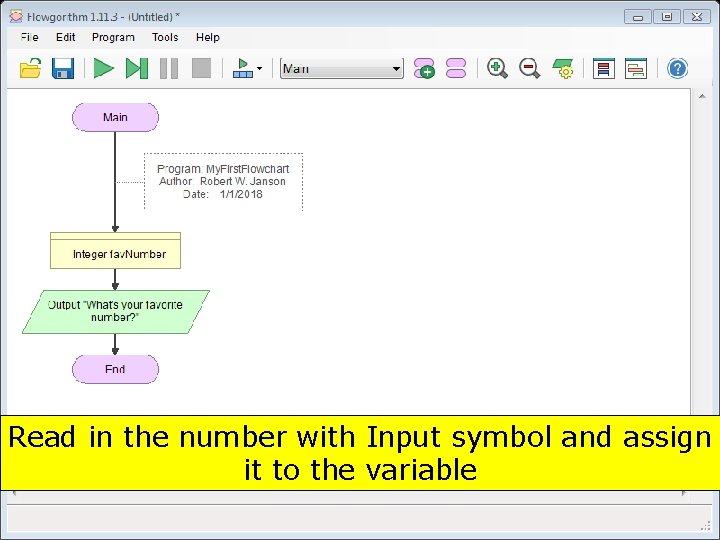
Read in the number with Input symbol and assign it to the variable 85 Copyright 2017 by Janson Industries
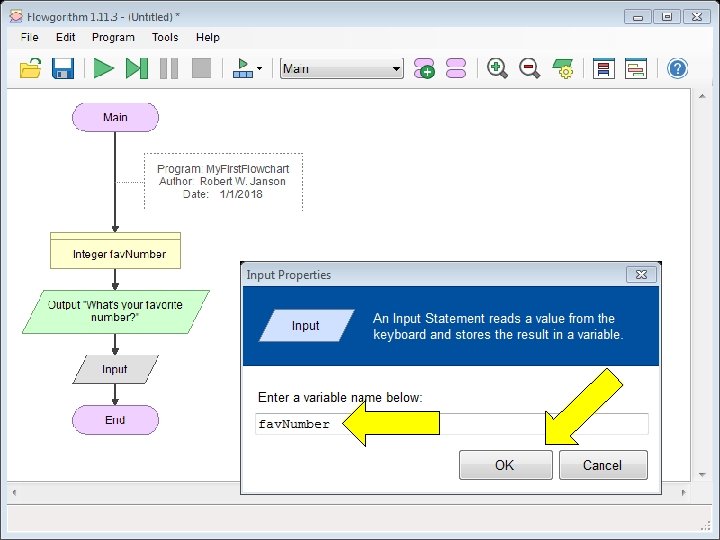
86 Copyright 2017 by Janson Industries
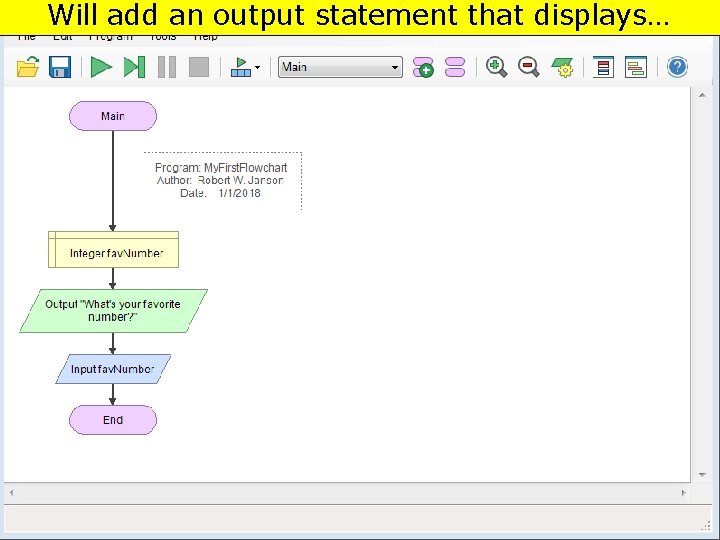
Will add an output statement that displays… 87 Copyright 2017 by Janson Industries
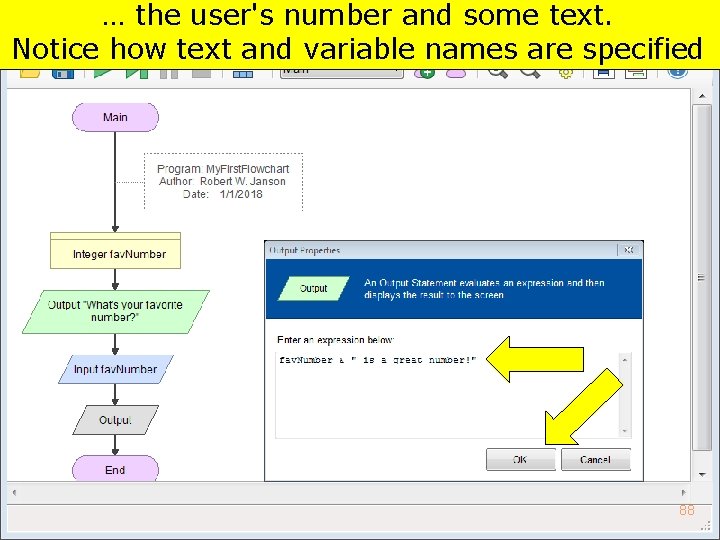
… the user's number and some text. Notice how text and variable names are specified 88 Copyright 2017 by Janson Industries
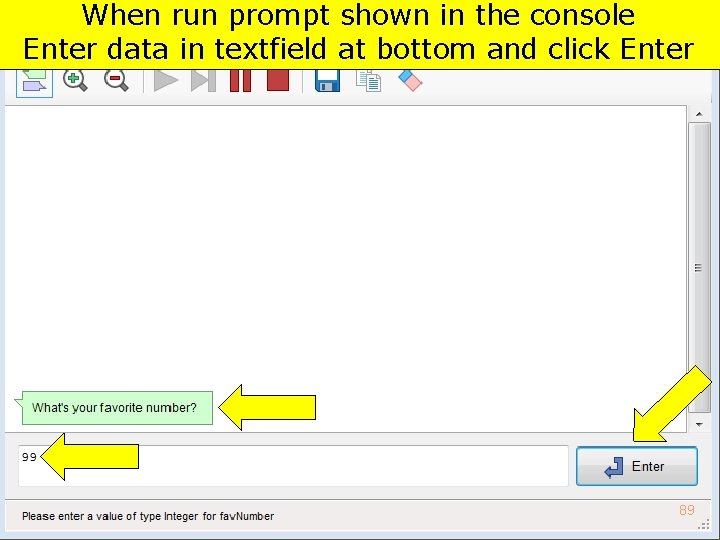
When run prompt shown in the console Enter data in textfield at bottom and click Enter 89 Copyright 2017 by Janson Industries
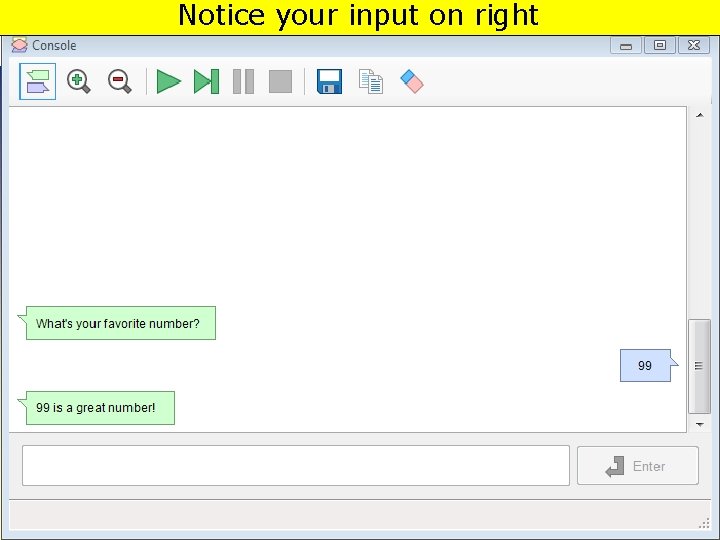
Notice your input on right 90 Copyright 2017 by Janson Industries
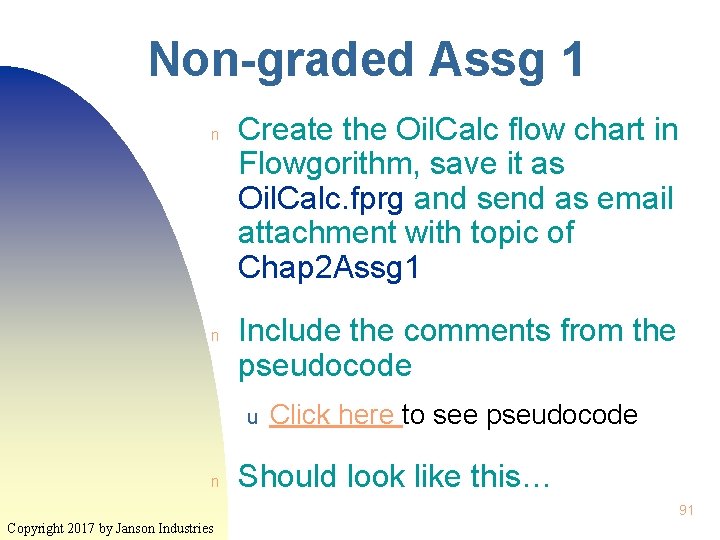
Non-graded Assg 1 n n Create the Oil. Calc flow chart in Flowgorithm, save it as Oil. Calc. fprg and send as email attachment with topic of Chap 2 Assg 1 Include the comments from the pseudocode u n Click here to see pseudocode Should look like this… 91 Copyright 2017 by Janson Industries
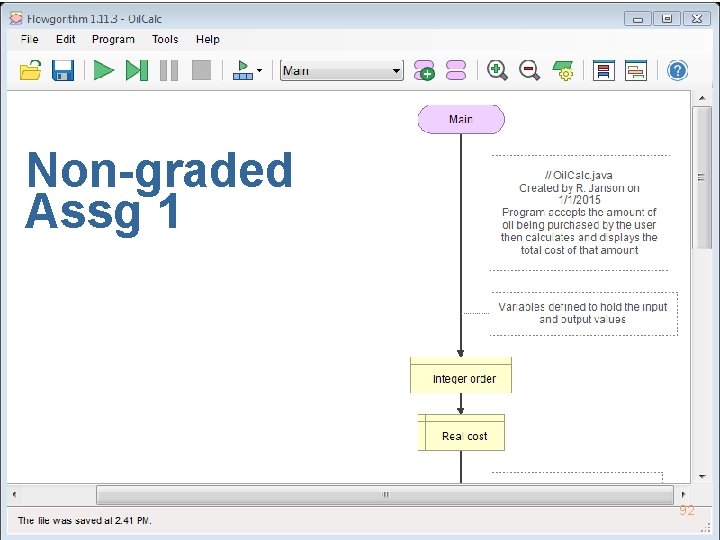
Non-graded Assg 1 92 Copyright 2017 by Janson Industries
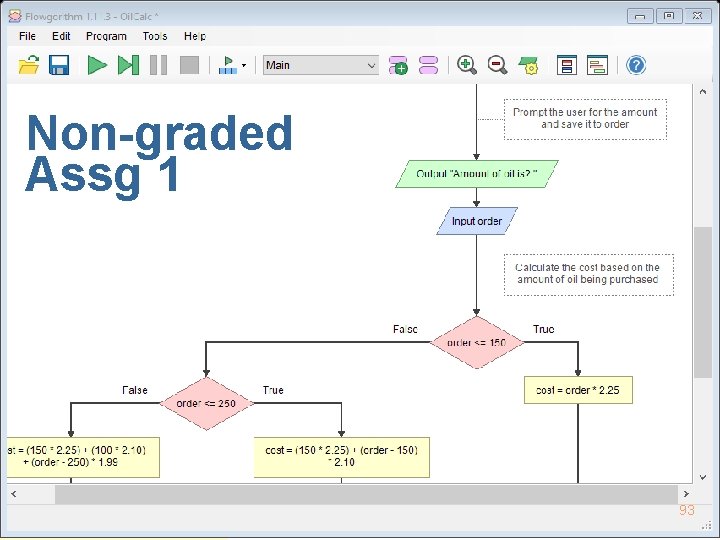
Non-graded Assg 1 93 Copyright 2017 by Janson Industries
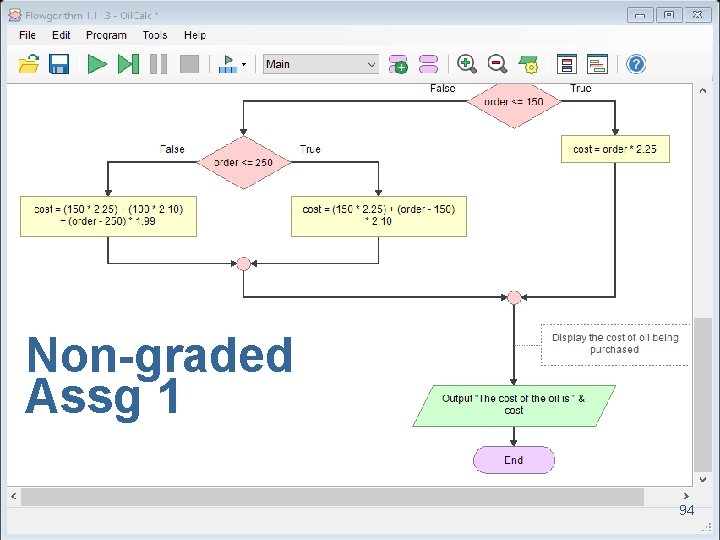
Non-graded Assg 1 94 Copyright 2017 by Janson Industries
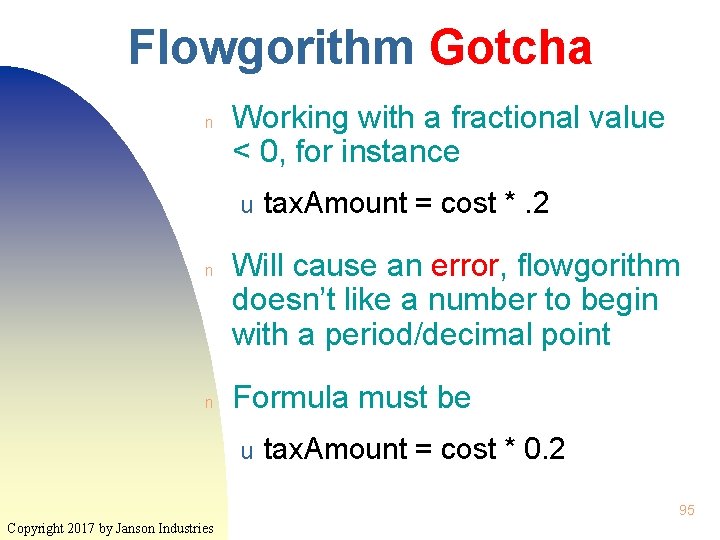
Flowgorithm Gotcha n Working with a fractional value < 0, for instance u n n tax. Amount = cost *. 2 Will cause an error, flowgorithm doesn’t like a number to begin with a period/decimal point Formula must be u tax. Amount = cost * 0. 2 95 Copyright 2017 by Janson Industries
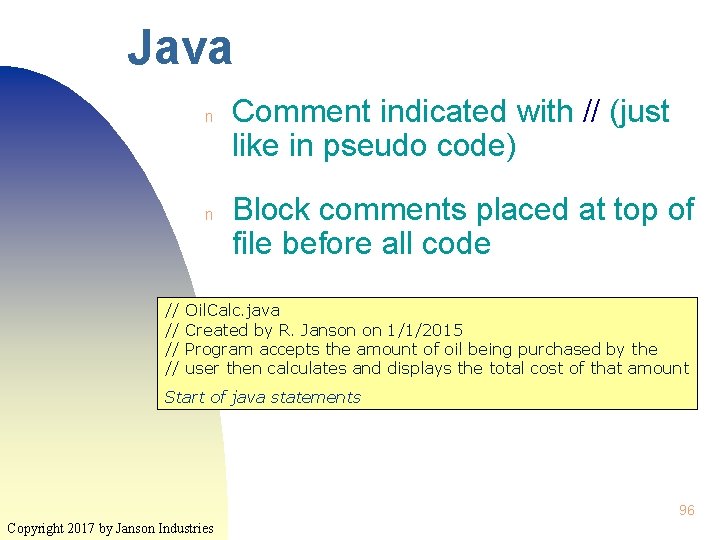
Java n n // // Comment indicated with // (just like in pseudo code) Block comments placed at top of file before all code Oil. Calc. java Created by R. Janson on 1/1/2015 Program accepts the amount of oil being purchased by the user then calculates and displays the total cost of that amount Start of java statements 96 Copyright 2017 by Janson Industries
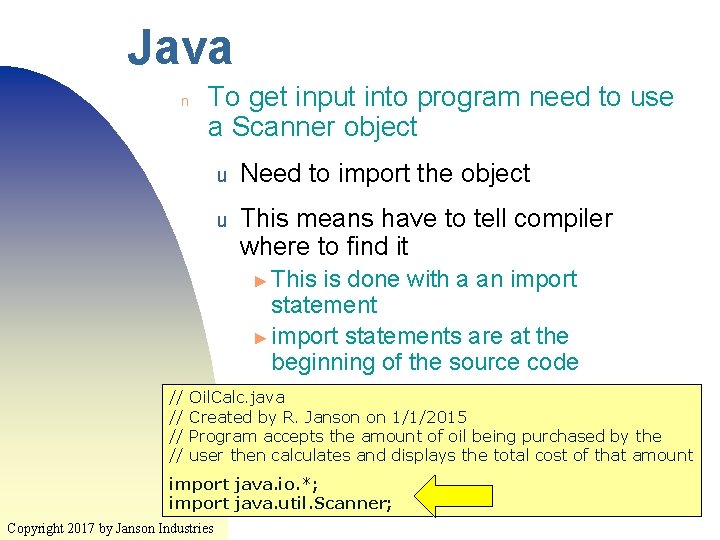
Java n To get input into program need to use a Scanner object u Need to import the object u This means have to tell compiler where to find it ► This is done with a an import statement ► import statements are at the beginning of the source code // // Oil. Calc. java Created by R. Janson on 1/1/2015 Program accepts the amount of oil being purchased by the user then calculates and displays the total cost of that amount import java. io. *; import java. util. Scanner; Copyright 2017 by Janson Industries 97
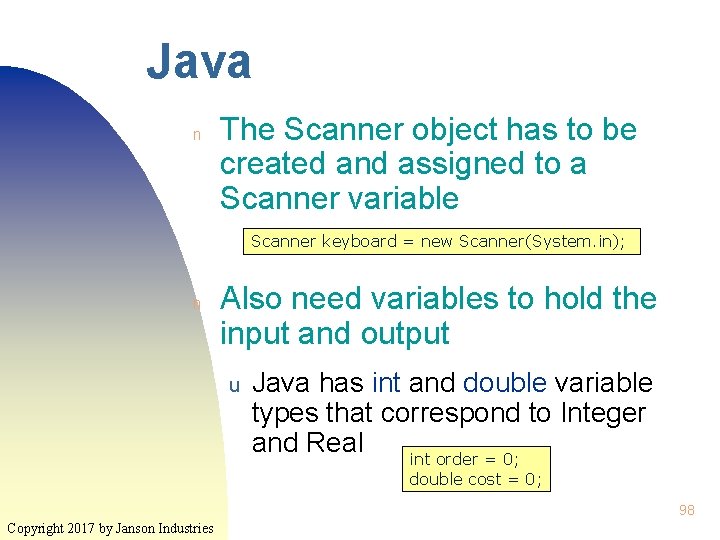
Java n The Scanner object has to be created and assigned to a Scanner variable Scanner keyboard = new Scanner(System. in); n Also need variables to hold the input and output u Java has int and double variable types that correspond to Integer and Real int order = 0; double cost = 0; 98 Copyright 2017 by Janson Industries
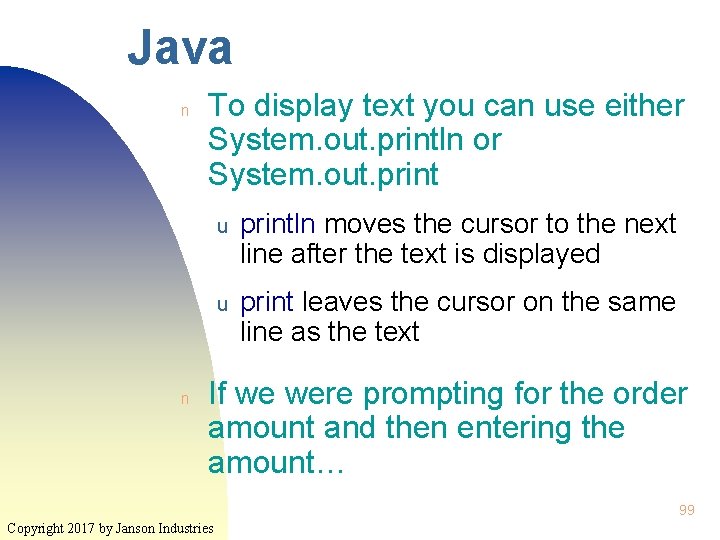
Java n n To display text you can use either System. out. println or System. out. print u println moves the cursor to the next line after the text is displayed u print leaves the cursor on the same line as the text If we were prompting for the order amount and then entering the amount… 99 Copyright 2017 by Janson Industries
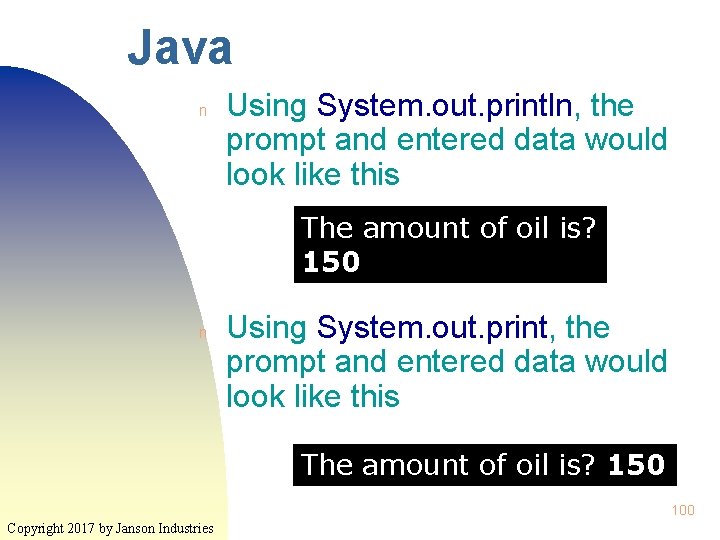
Java n Using System. out. println, the prompt and entered data would look like this The amount of oil is? 150 n Using System. out. print, the prompt and entered data would look like this The amount of oil is? 150 100 Copyright 2017 by Janson Industries
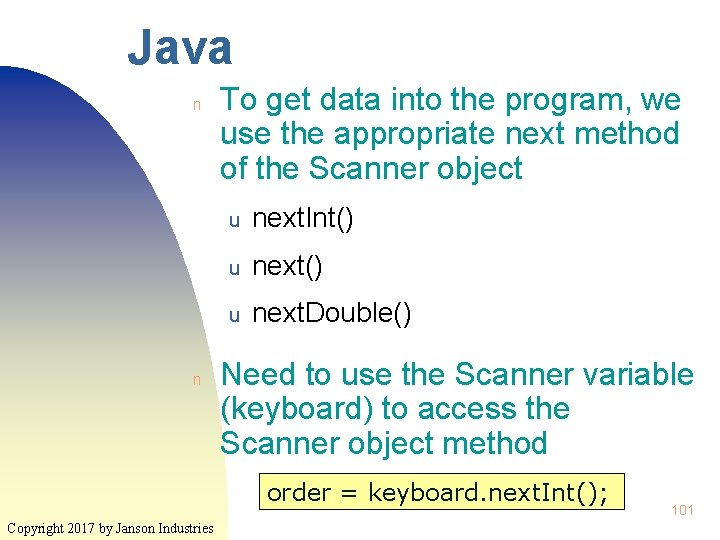
Java n n To get data into the program, we use the appropriate next method of the Scanner object u next. Int() u next. Double() Need to use the Scanner variable (keyboard) to access the Scanner object method order = keyboard. next. Int(); Copyright 2017 by Janson Industries 101
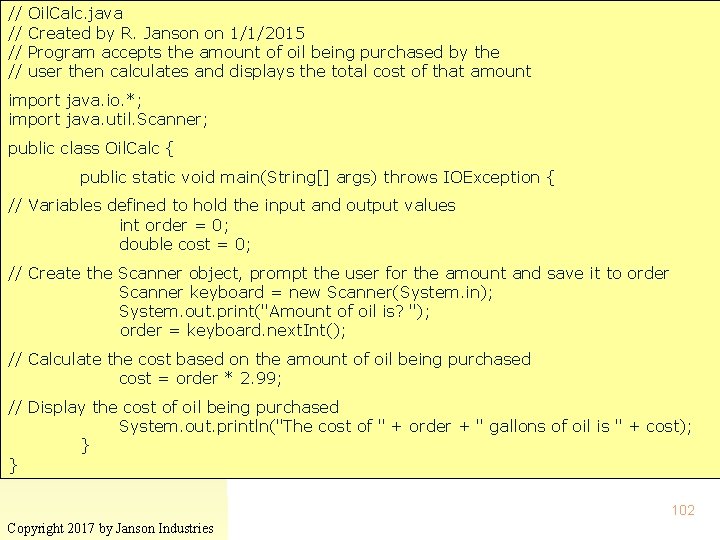
// // Oil. Calc. java Created by R. Janson on 1/1/2015 Program accepts the amount of oil being purchased by the user then calculates and displays the total cost of that amount Java import java. io. *; import java. util. Scanner; public class Oil. Calc { public static void main(String[] args) throws IOException { // Variables defined to hold the input and output values int order = 0; double cost = 0; // Create the Scanner object, prompt the user for the amount and save it to order Scanner keyboard = new Scanner(System. in); System. out. print("Amount of oil is? "); order = keyboard. next. Int(); // Calculate the cost based on the amount of oil being purchased cost = order * 2. 99; // Display the cost of oil being purchased System. out. println("The cost of " + order + " gallons of oil is " + cost); } } 102 Copyright 2017 by Janson Industries
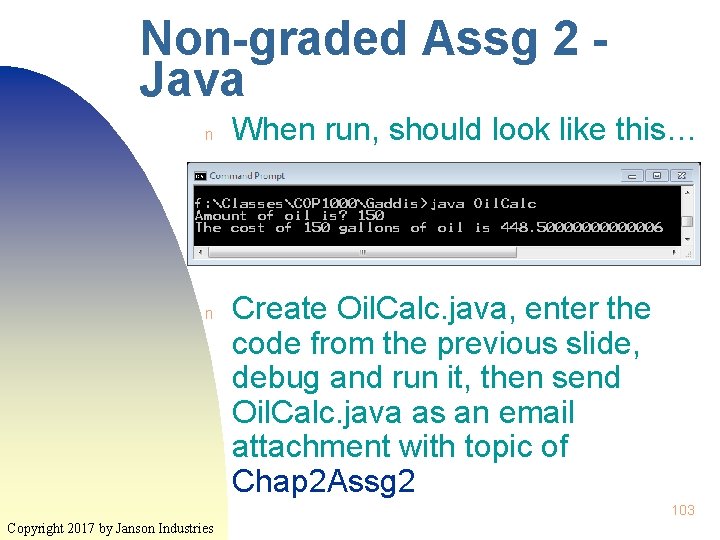
Non-graded Assg 2 Java n n When run, should look like this… Create Oil. Calc. java, enter the code from the previous slide, debug and run it, then send Oil. Calc. java as an email attachment with topic of Chap 2 Assg 2 103 Copyright 2017 by Janson Industries
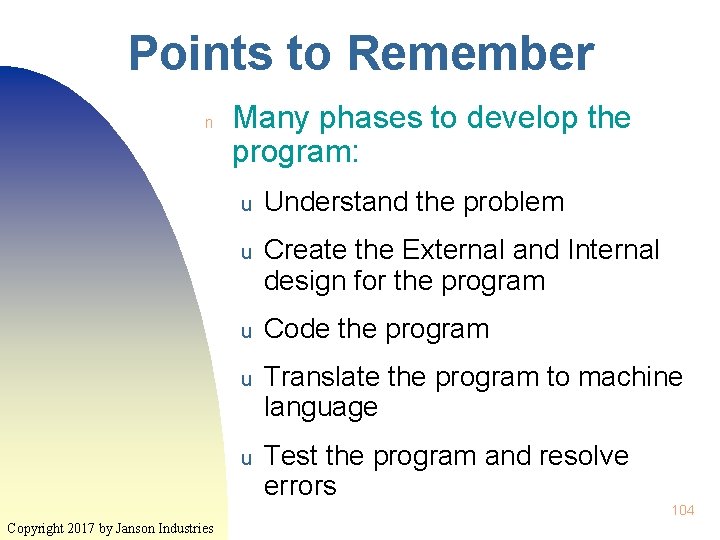
Points to Remember n Many phases to develop the program: u Understand the problem u Create the External and Internal design for the program u Code the program u Translate the program to machine language u Test the program and resolve errors 104 Copyright 2017 by Janson Industries
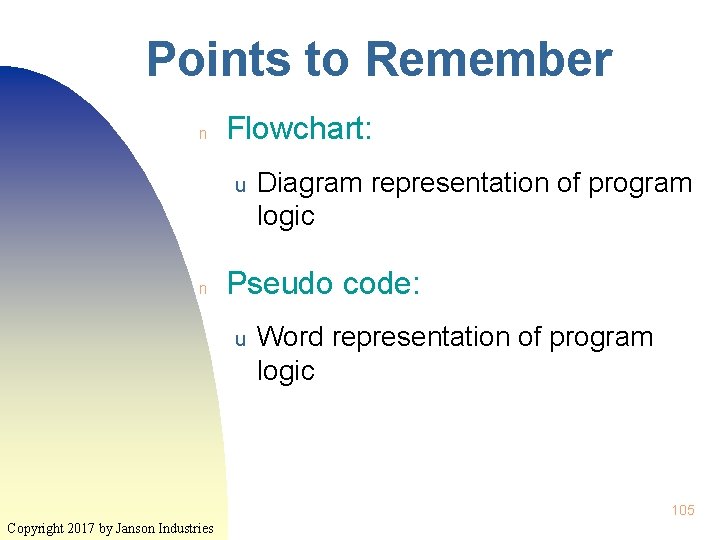
Points to Remember n Flowchart: u n Diagram representation of program logic Pseudo code: u Word representation of program logic 105 Copyright 2017 by Janson Industries
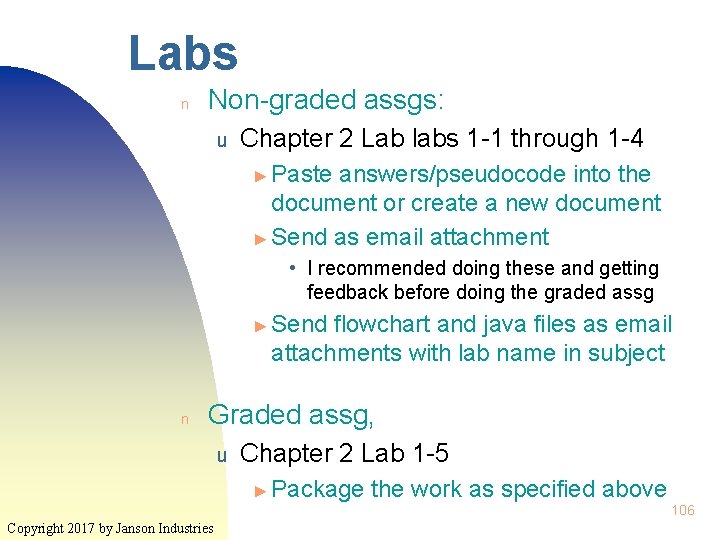
Labs n Non-graded assgs: u Chapter 2 Lab labs 1 -1 through 1 -4 ► Paste answers/pseudocode into the document or create a new document ► Send as email attachment • I recommended doing these and getting feedback before doing the graded assg ► Send flowchart and java files as email attachments with lab name in subject n Graded assg, u Chapter 2 Lab 1 -5 ► Package the work as specified above 106 Copyright 2017 by Janson Industries
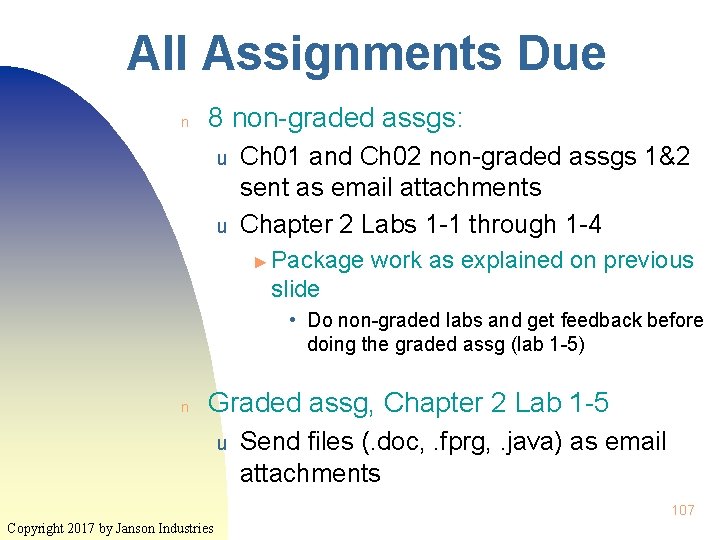
All Assignments Due n 8 non-graded assgs: u u Ch 01 and Ch 02 non-graded assgs 1&2 sent as email attachments Chapter 2 Labs 1 -1 through 1 -4 ► Package work as explained on previous slide • Do non-graded labs and get feedback before doing the graded assg (lab 1 -5) n Graded assg, Chapter 2 Lab 1 -5 u Send files (. doc, . fprg, . java) as email attachments 107 Copyright 2017 by Janson Industries
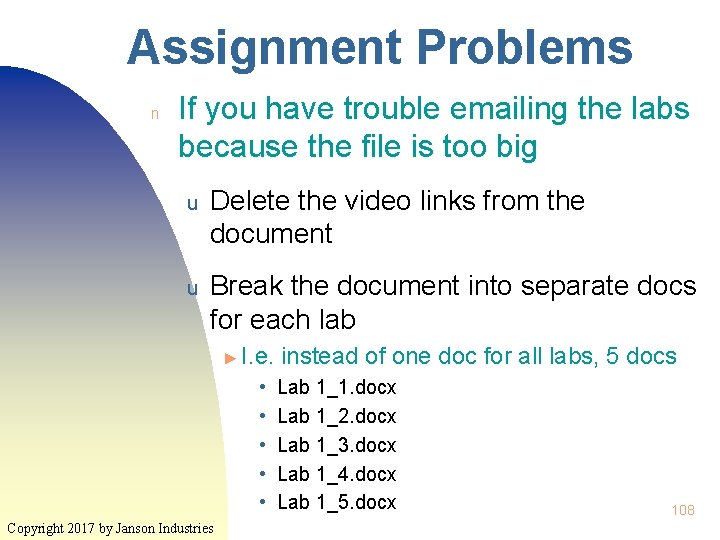
Assignment Problems n If you have trouble emailing the labs because the file is too big u Delete the video links from the document u Break the document into separate docs for each lab ► I. e. • • • Copyright 2017 by Janson Industries instead of one doc for all labs, 5 docs Lab 1_1. docx Lab 1_2. docx Lab 1_3. docx Lab 1_4. docx Lab 1_5. docx 108
What is form control in system analysis and design
Finely tuned input
Hardware input processing and output devices
Information system input processing output
Chapter 2 input processing and output
Algorithmic input output
How do you write an input and output algorithm?
Neighborhood averaging in image processing
Examples of secondary processing
Batch processing vs interactive processing
Floral arrangement shapes
Peripheral output adalah... *
Compare a* and ao* algorithm
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
Runtime programming
Linear vs integer programming
Perbedaan linear programming dan integer programming
The keyboard mouse scanner digital camera or
Processing information input
Char flowgorithm
Steps in dynamic programming
Genetic programming vs genetic algorithm
Genetic programming vs genetic algorithm
Features of dynamic programming
Dynamic programming algorithm
Bottom-up processing examples
Gloria suarez
Top-down processing vs bottom-up processing
What is point processing in digital image processing
Histogram processing in digital image processing
Parallel processing vs concurrent processing
Nonlinear image processing
Point processing in image processing
Gonzalez
Top-down processing example
Mpp algorithm in image processing
Line sweep algorithm
Materials: engineering, science, processing and design
Pearson physics
Algorithm design and analysis process
Brute force algorithm examples
Algorithm design and problem solving
Problem solving and algorithm design
Introduction conclusion paragraphs
The two households in romeo and juliet
Body paragraph romeo and juliet
Thermochemistry
Titles for introductions
Basic principles of input design
The objectives of input design are
Objectives of input design
Advanced assembler directives in system programming
Top down modular design
Interior design programming
Modular design programming
Reliability design in dynamic programming
Compiler design mcq
Algorithm design paradigm
Algorithm design techniques
Algorithm design techniques
Algorithm design techniques with examples
Advanced algorithm analysis
Critical path length of task dependency graph
Thematic format in research
Exposé sur victor hugo
Types of hook sentences
Where is the hook located in the introduction paragraph
How to write a comparative essay introduction
Introduction body conclusion
Intro qr codes
States of matter
Introduction of advertisement
Tillbaka till vintergatan intro
Introduction body conclusion example
Introduction to reverse engineering
Essay on relationship
What are the four parts of a body paragraph
Organic chemistry introduction
Intro to offensive security
Utvecklingssamtal engelska
Materialgruppenmanagement definition
Lord of the flies thesis
Leq format
Description d'un paysage exemple
Intro paragraph format
Andrew ng intro machine learning
Intro to verilog
Severance intro
Ifs managers examples
Antonino virgillito
Whats an introduction paragraph
Introduction about digestive system
Kfc introduction
Body conclusion
Intro to vlsi
Intro to vectors
Intro to typography
Sound of music intro
Intro to reverse engineering
Intro to polynomials
Intro to mis
Dns in computer networks
Intro to business chapter 10 test answers
Paragraph to ex
Funnel introduction paragraph
Random forest intro
Funnel paragraph example
Format berita package