Data Structures and Analysis COMP 410 David Stotts
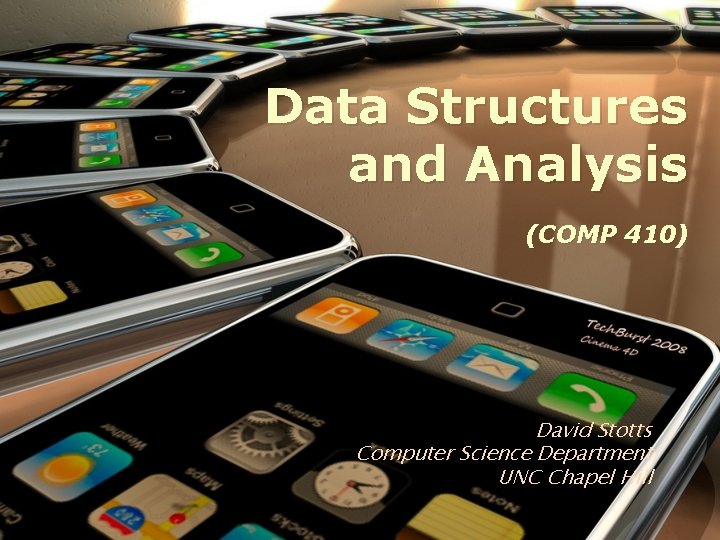
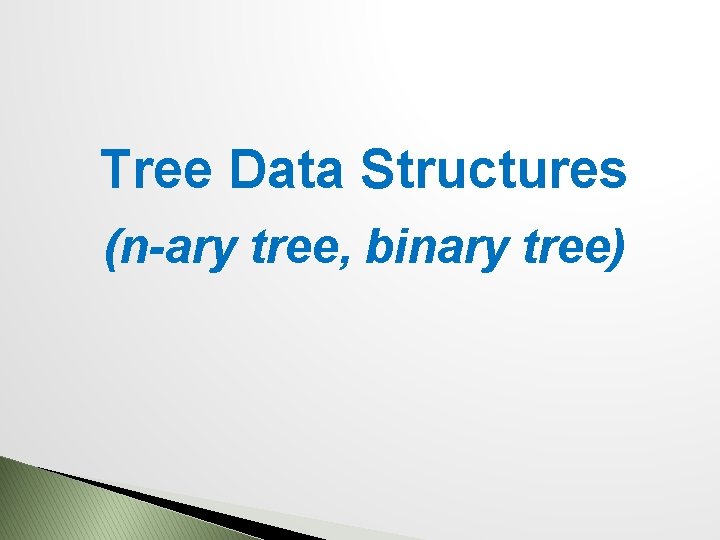
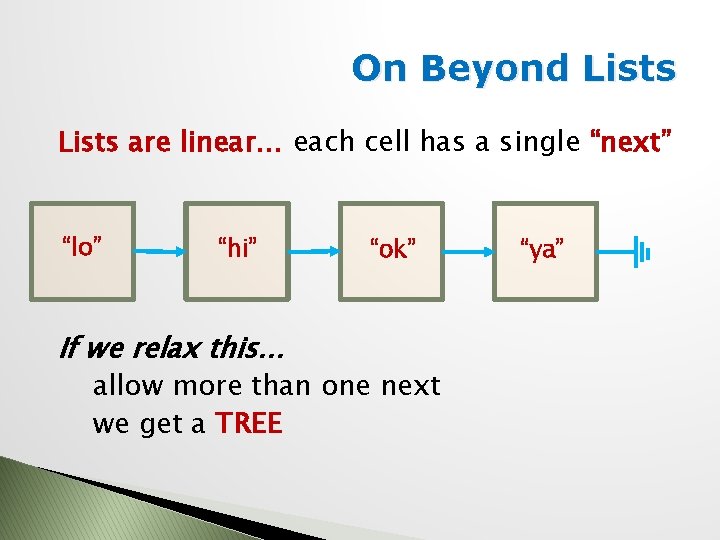
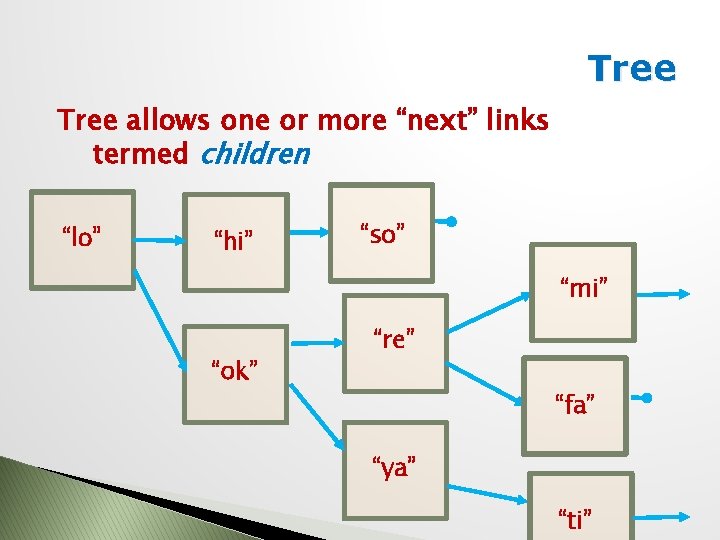
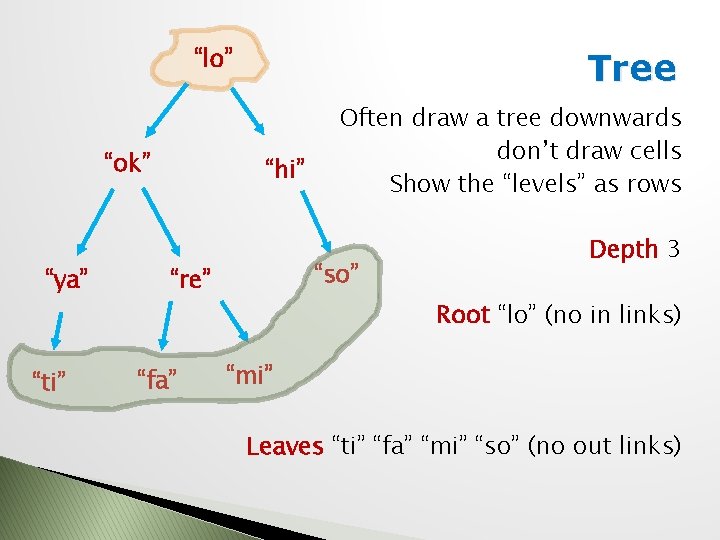
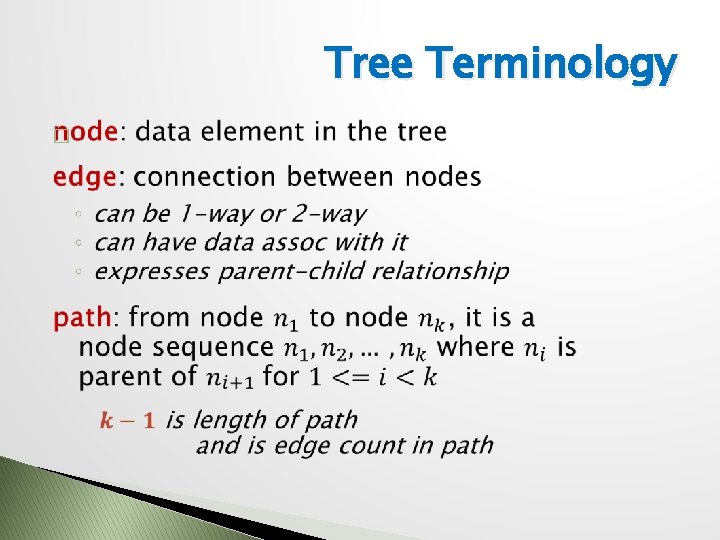
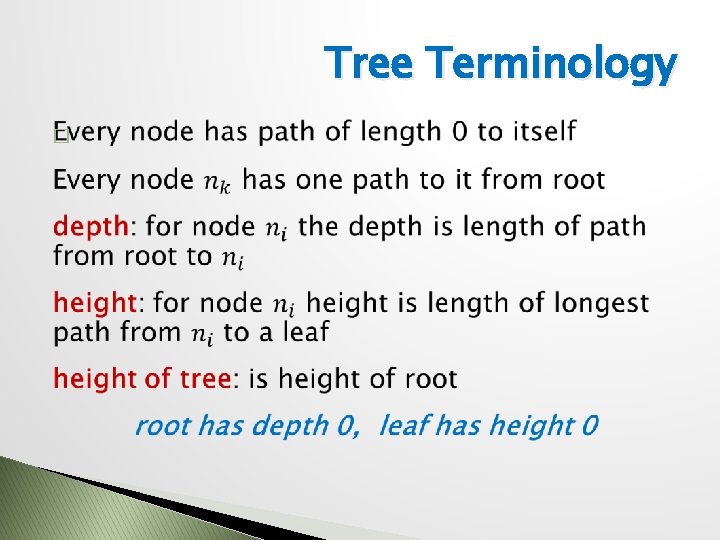
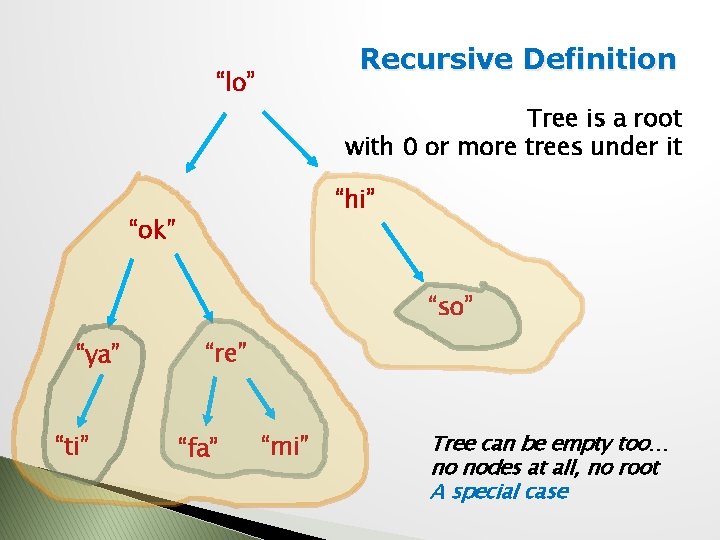
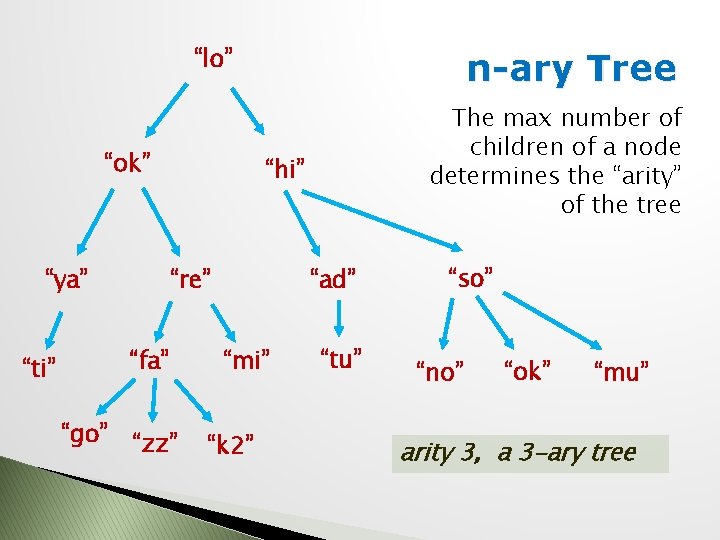
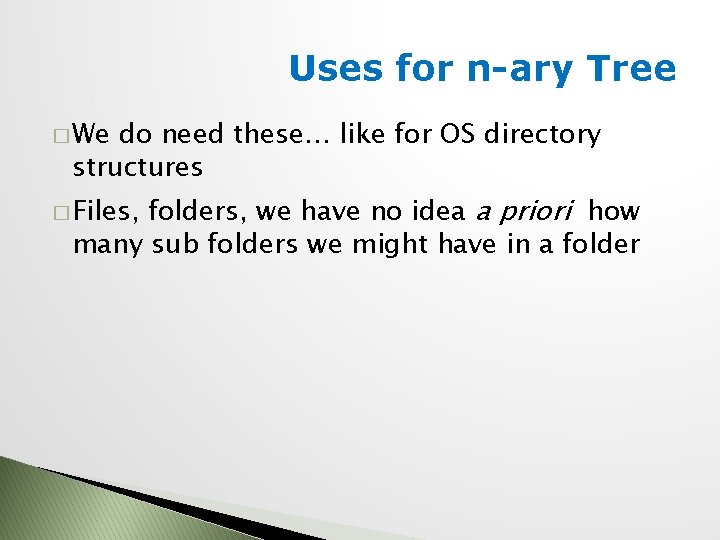
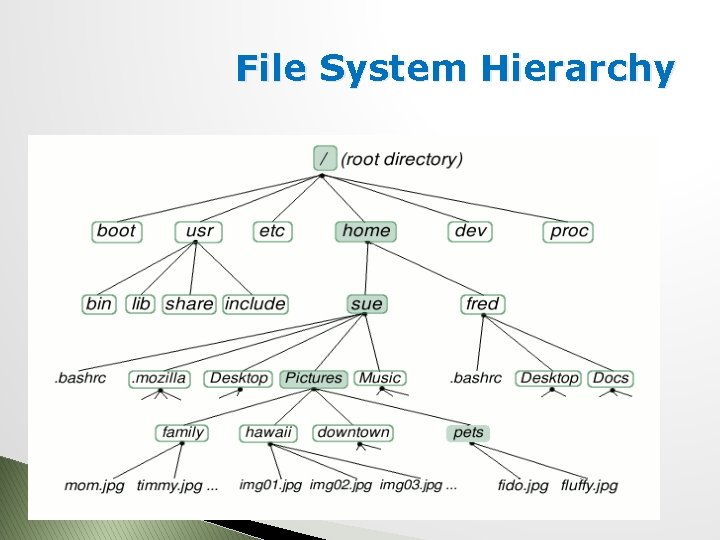
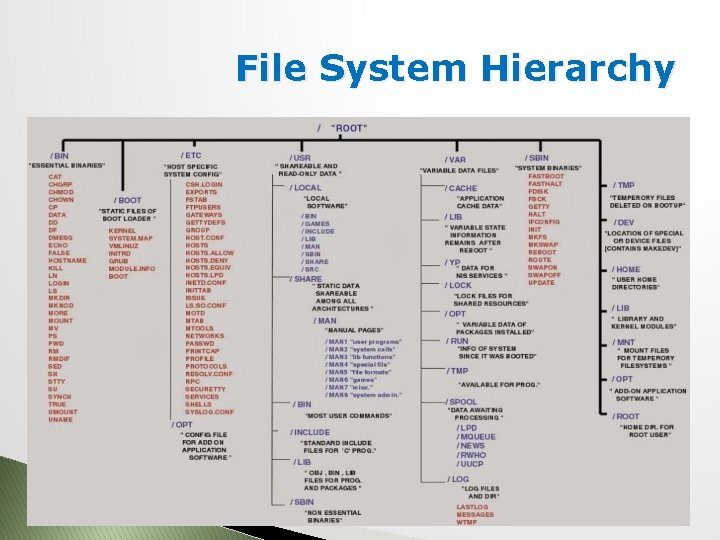
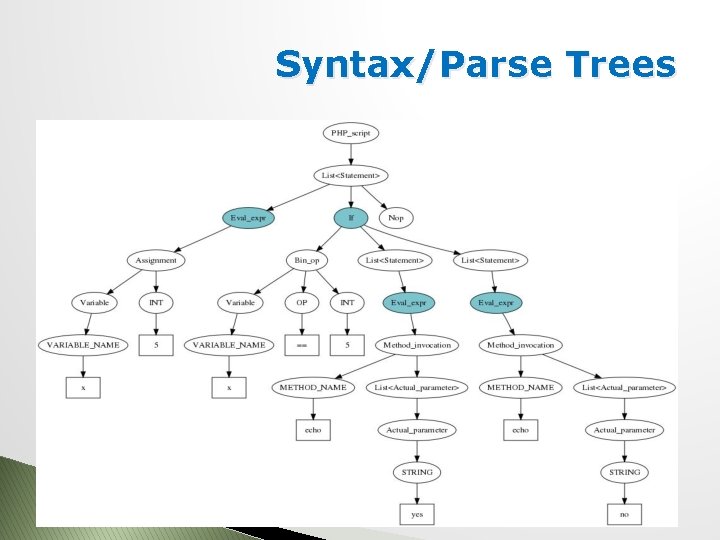
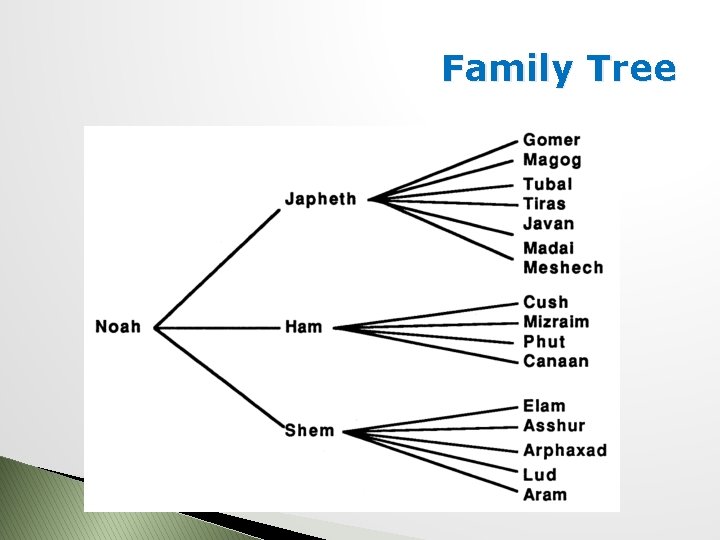
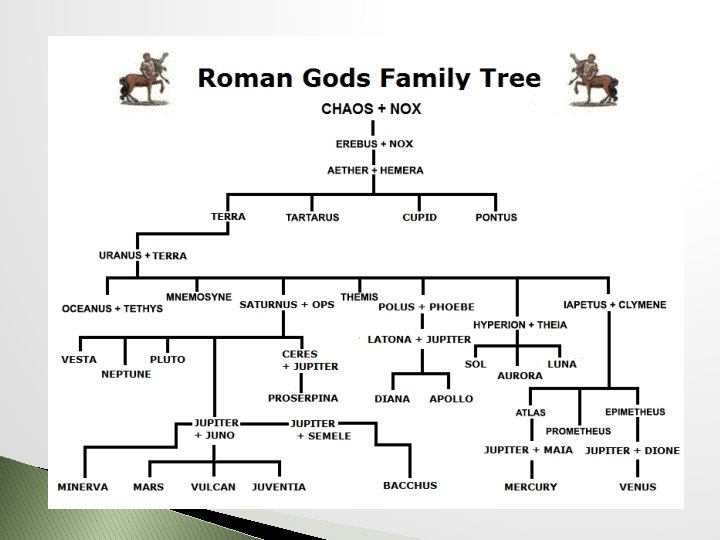
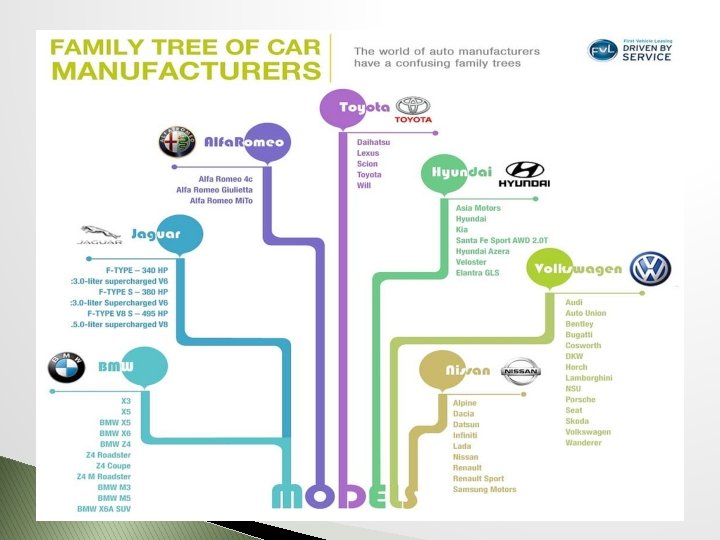
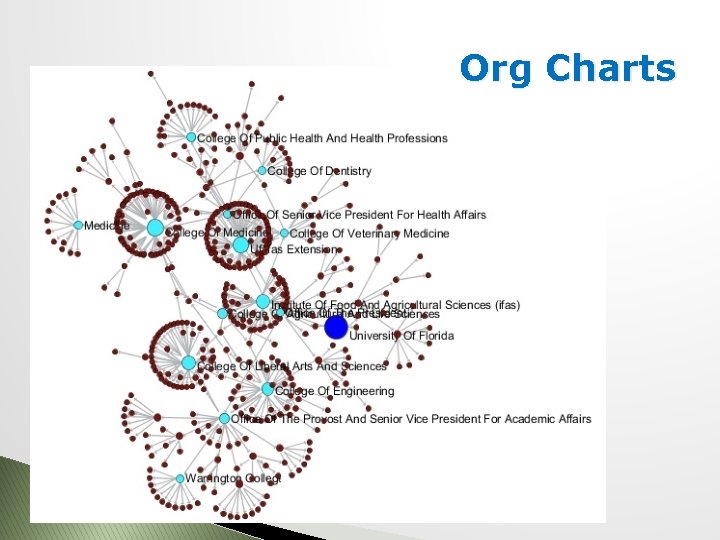
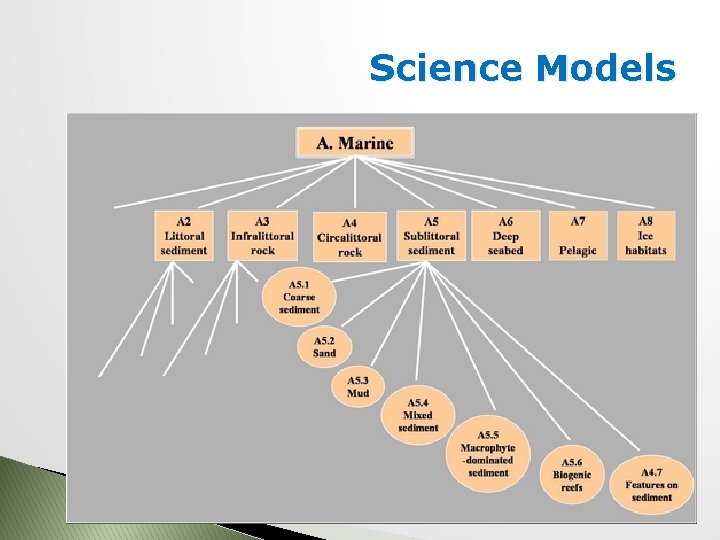
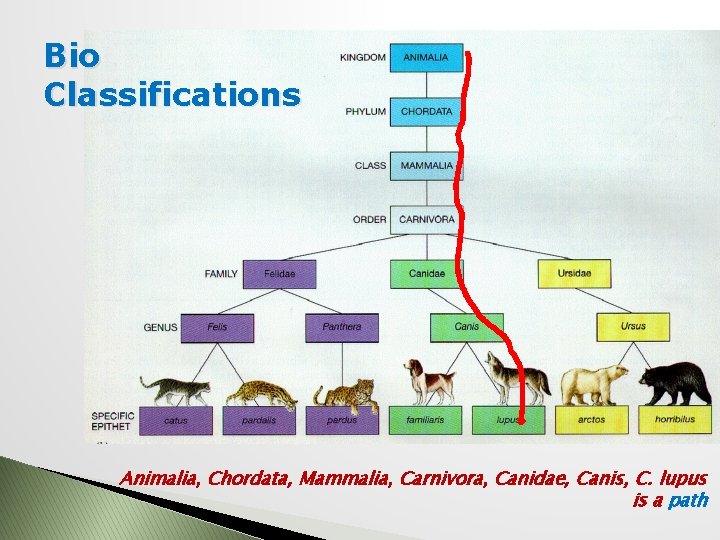
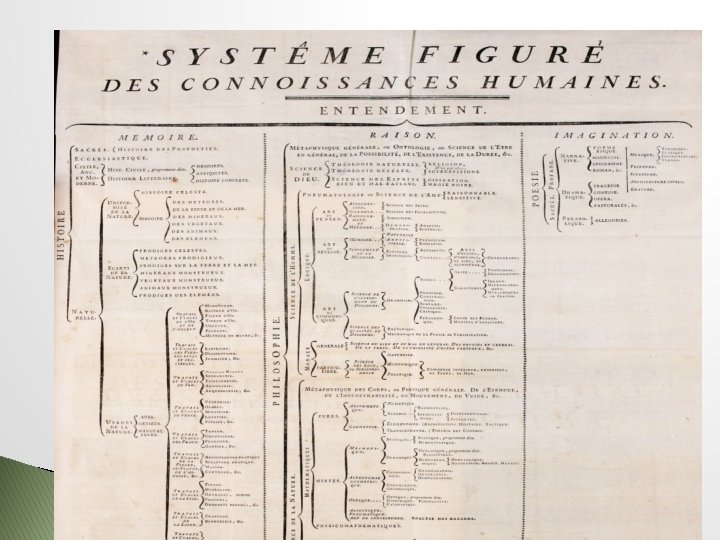
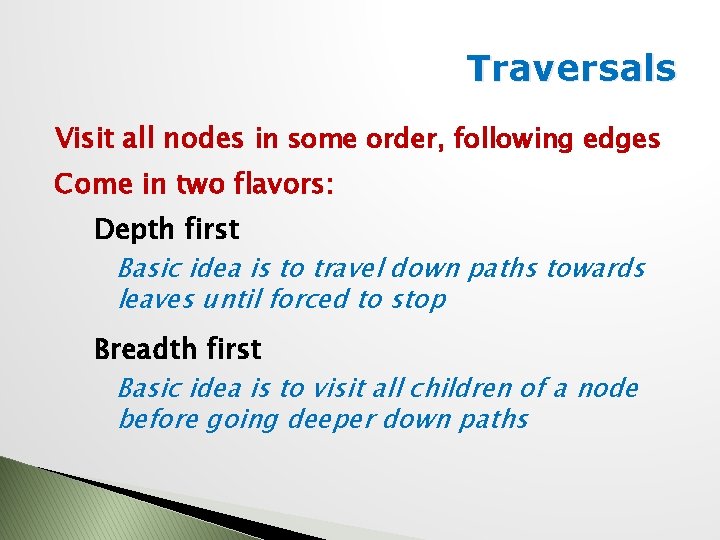
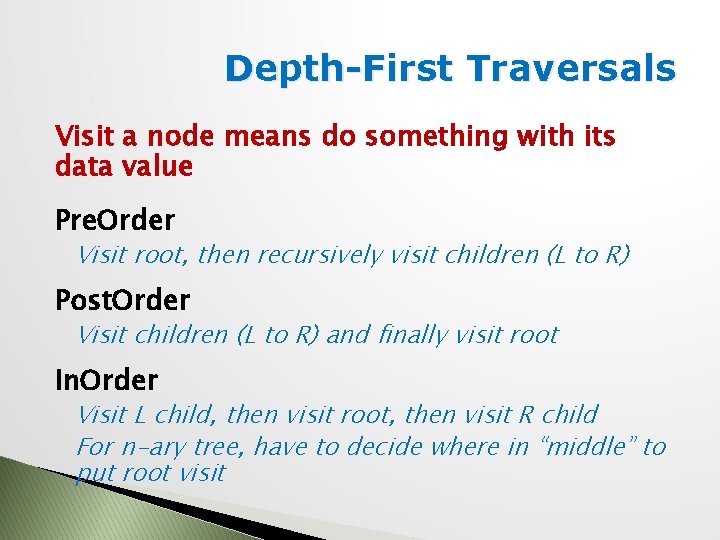
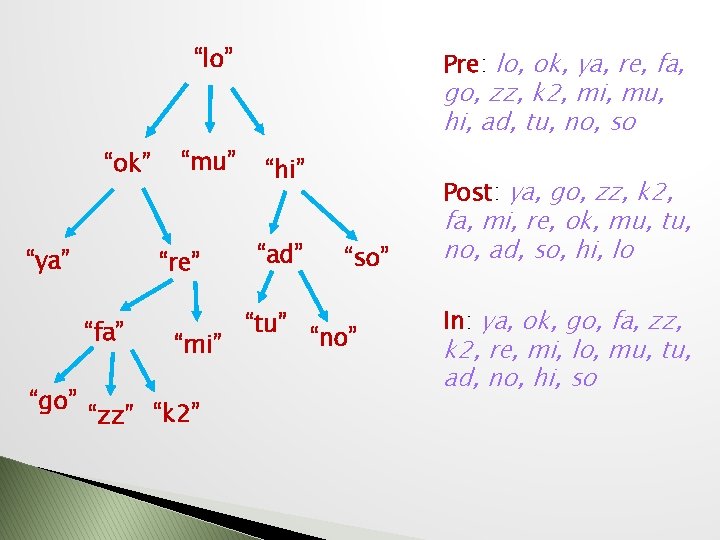
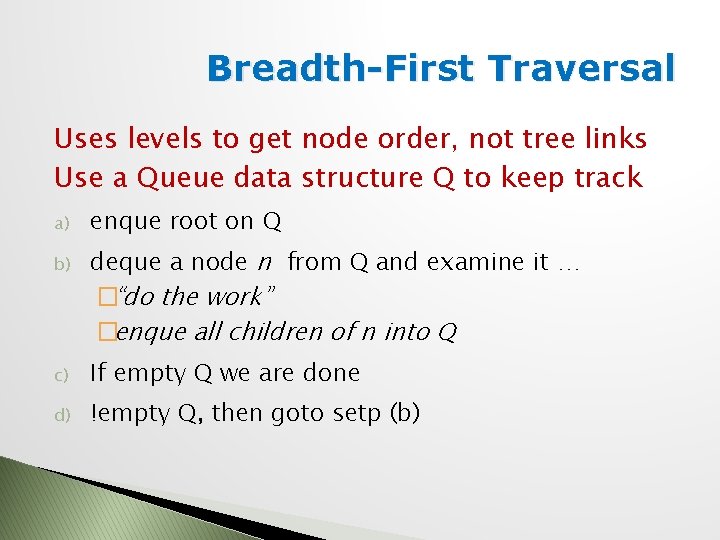
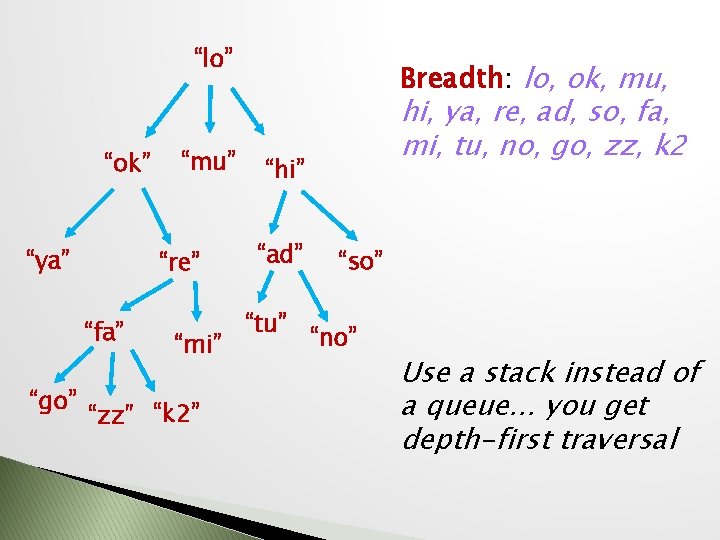
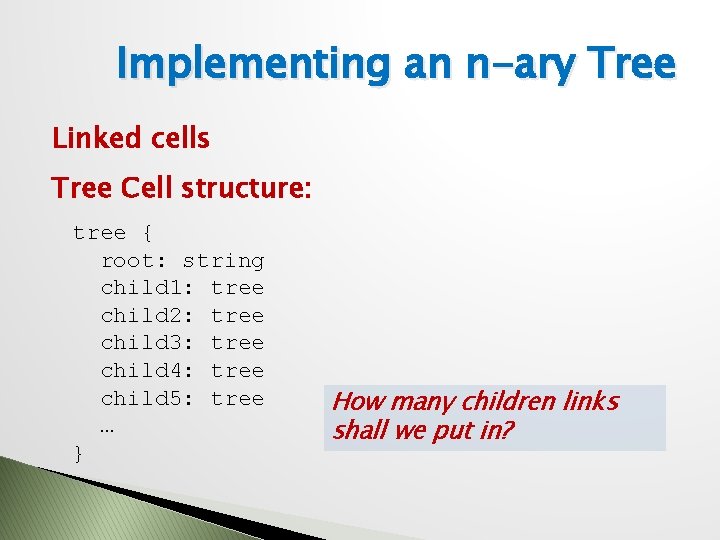
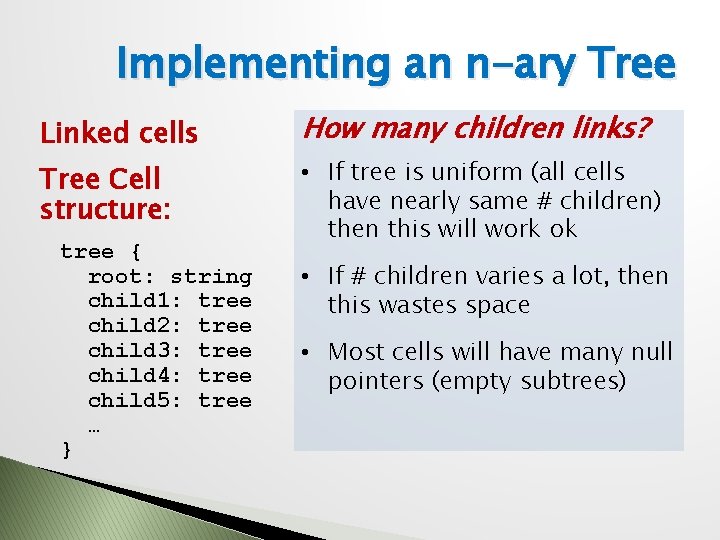
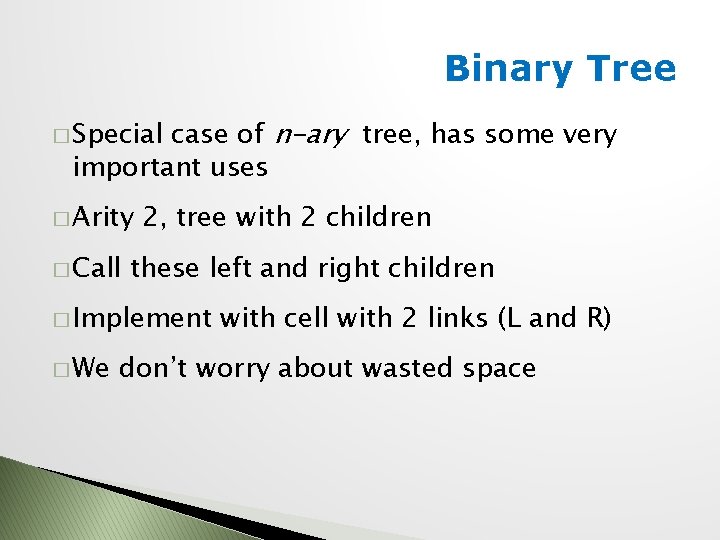
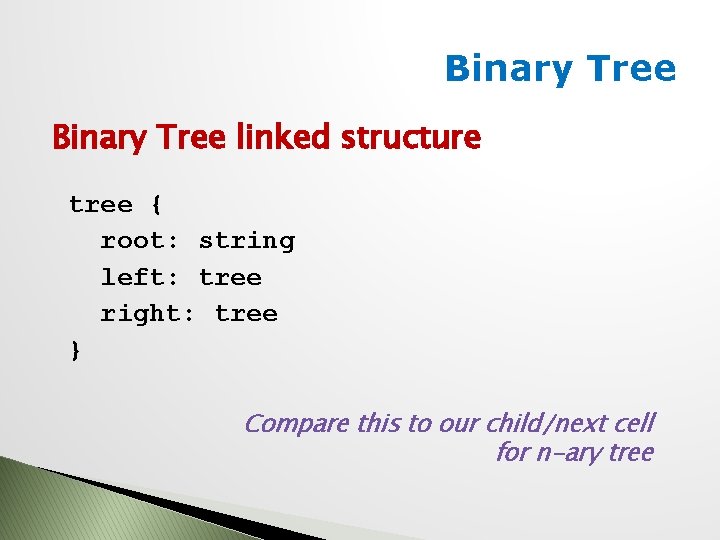
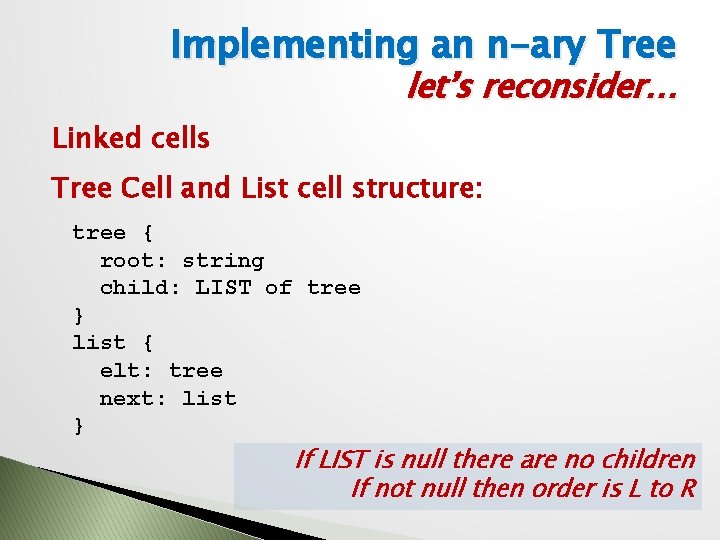
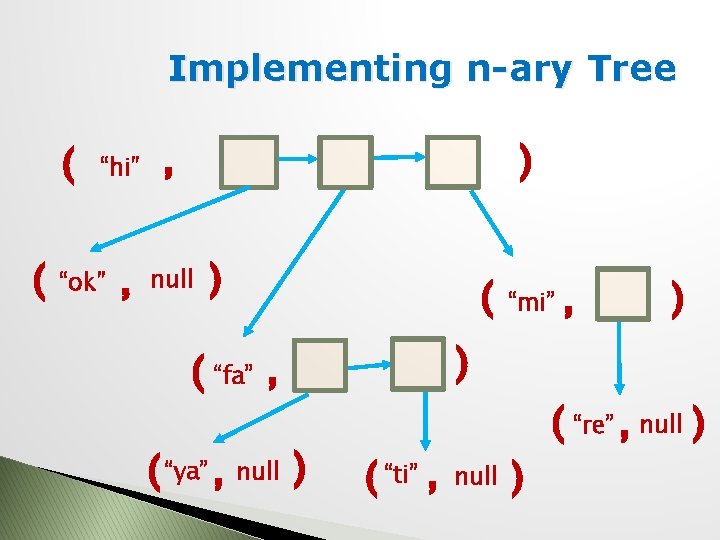
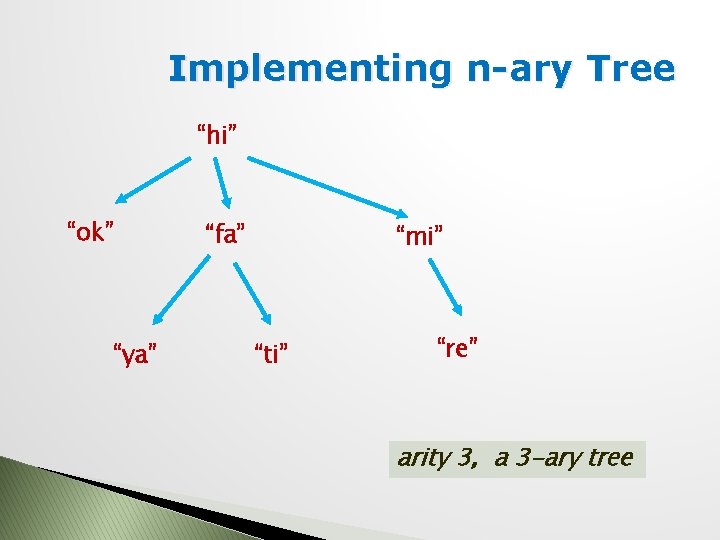
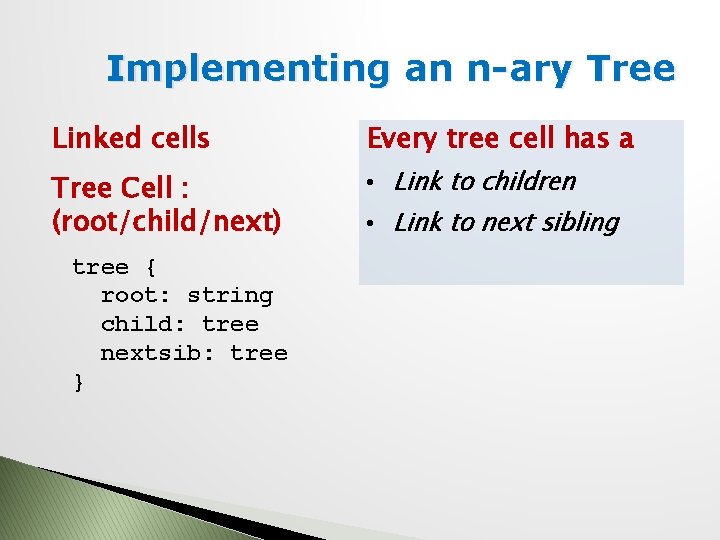
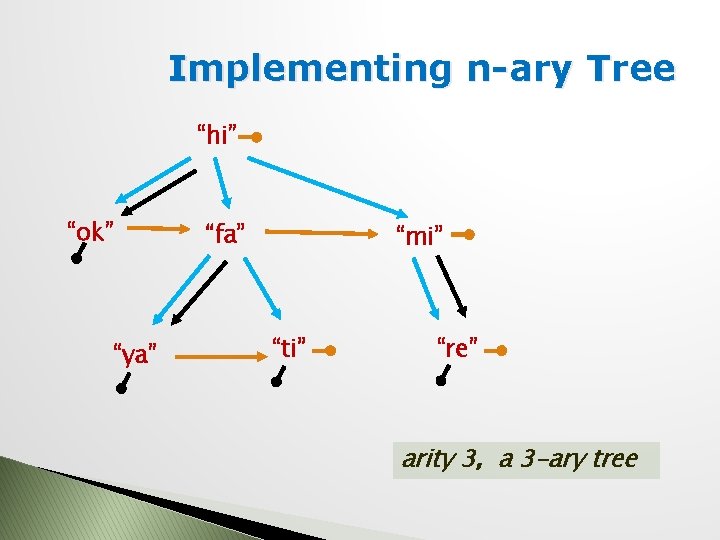
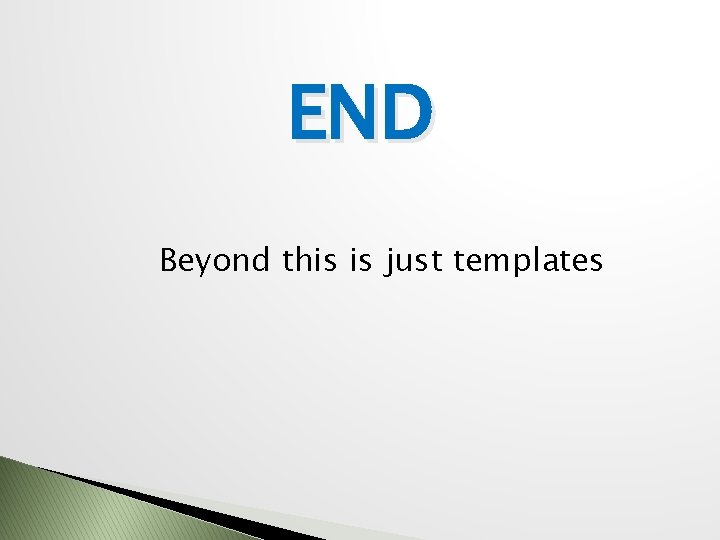
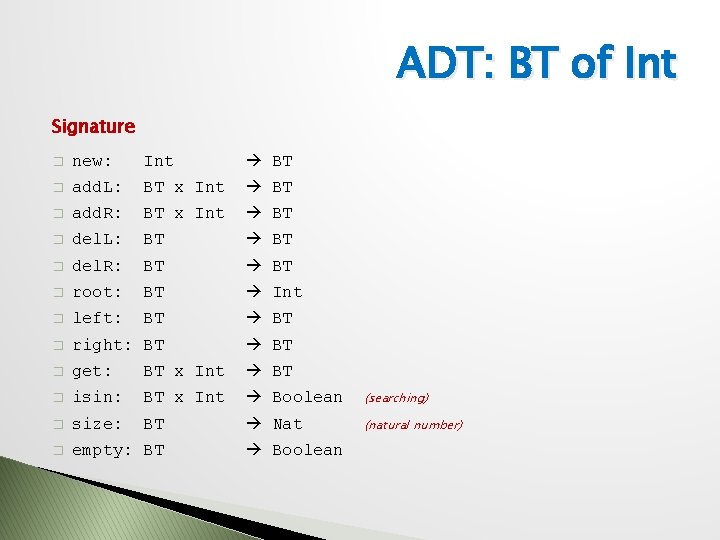
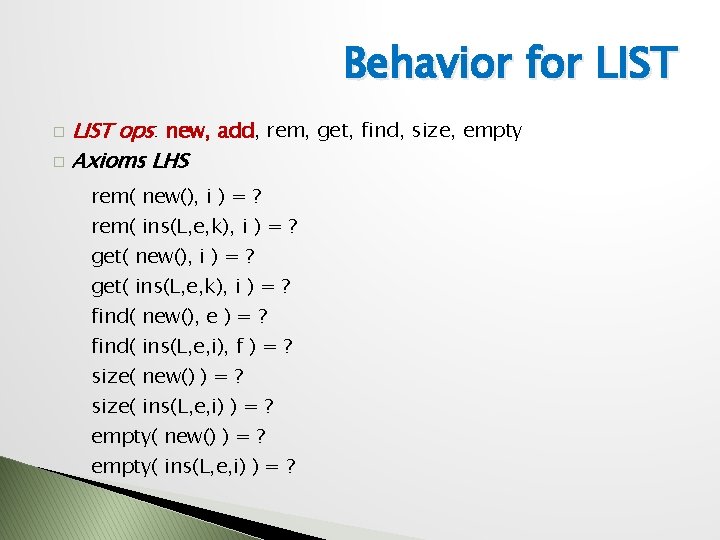
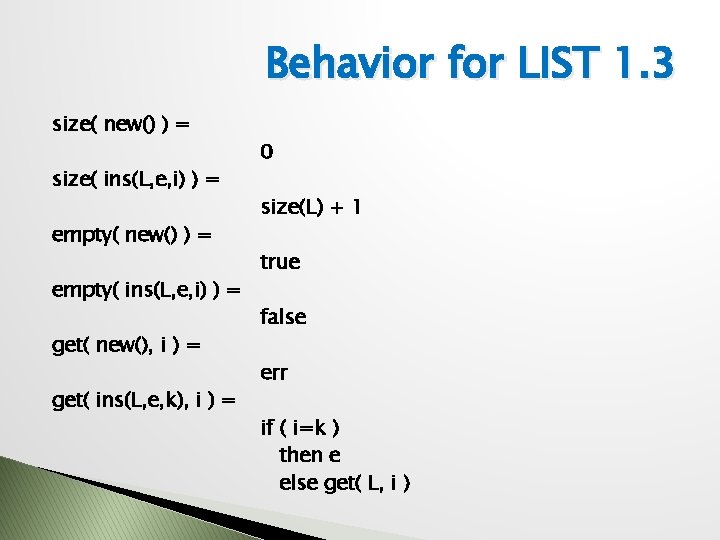
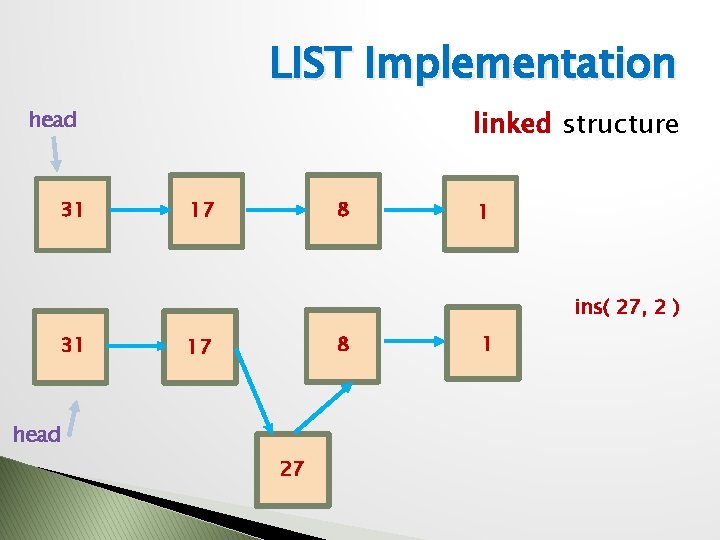
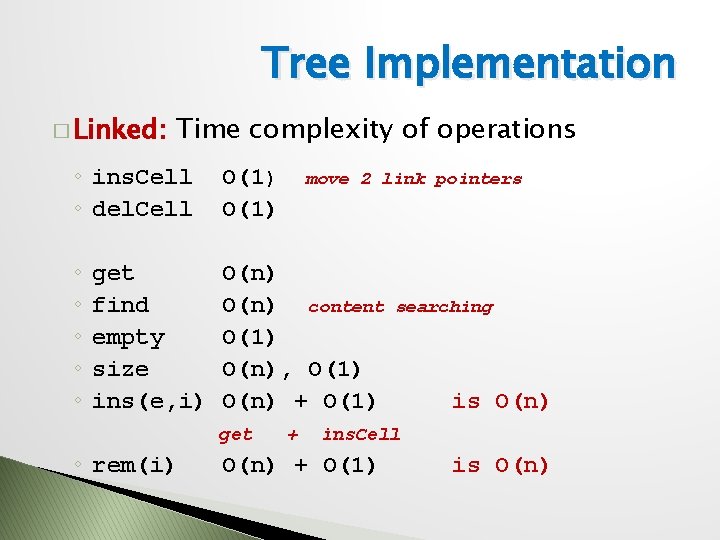
- Slides: 40
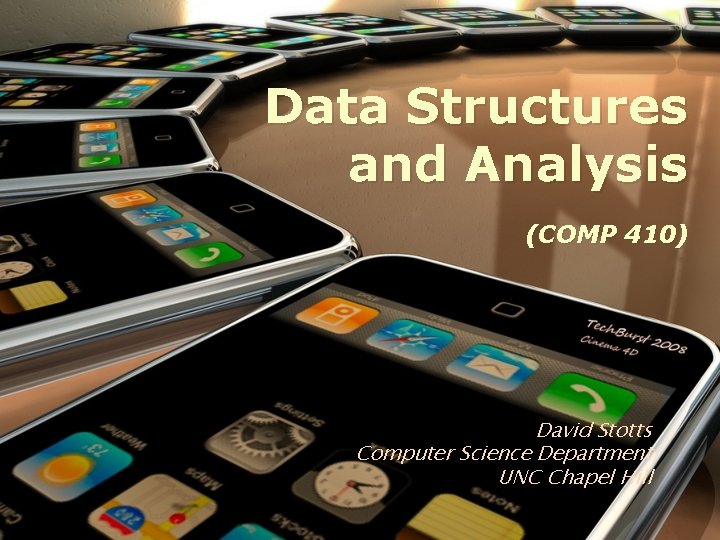
Data Structures and Analysis (COMP 410) David Stotts Computer Science Department UNC Chapel Hill
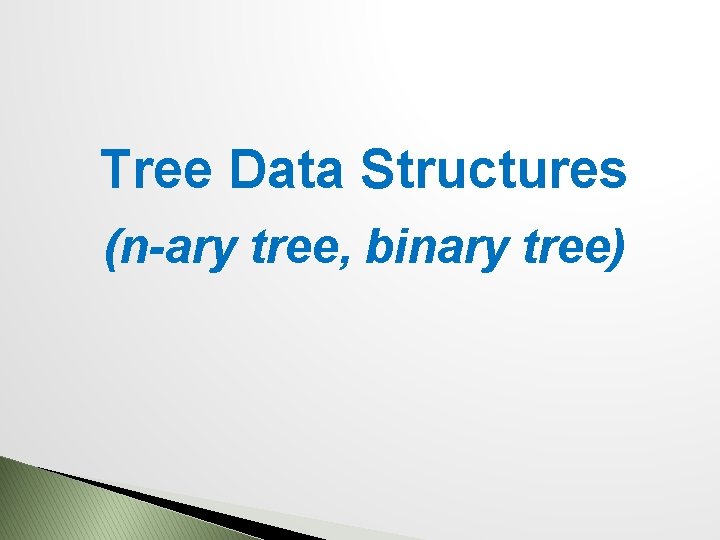
Tree Data Structures (n-ary tree, binary tree)
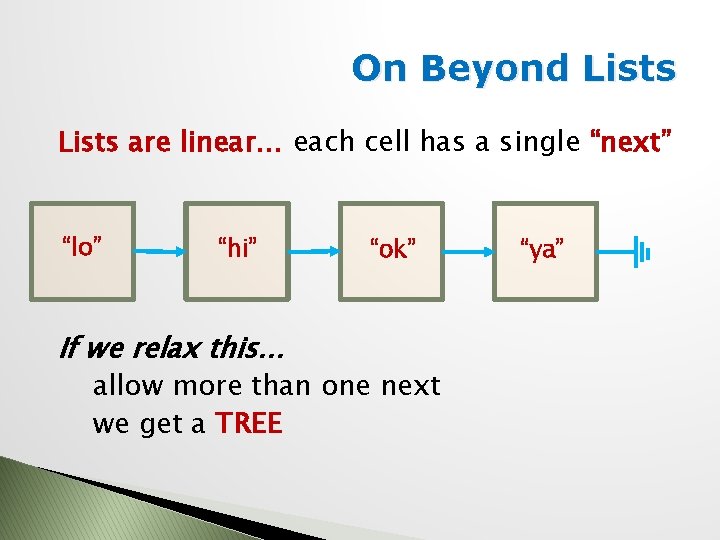
On Beyond Lists are linear… each cell has a single “next” “lo” “hi” If we relax this… “ok” allow more than one next we get a TREE “ya”
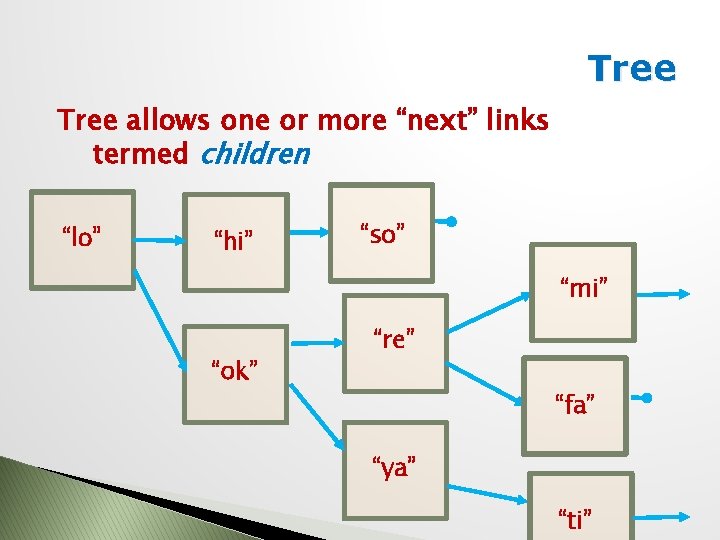
Tree allows one or more “next” links termed children “lo” “hi” “so” “mi” “ok” “re” “fa” “ya” “ti”
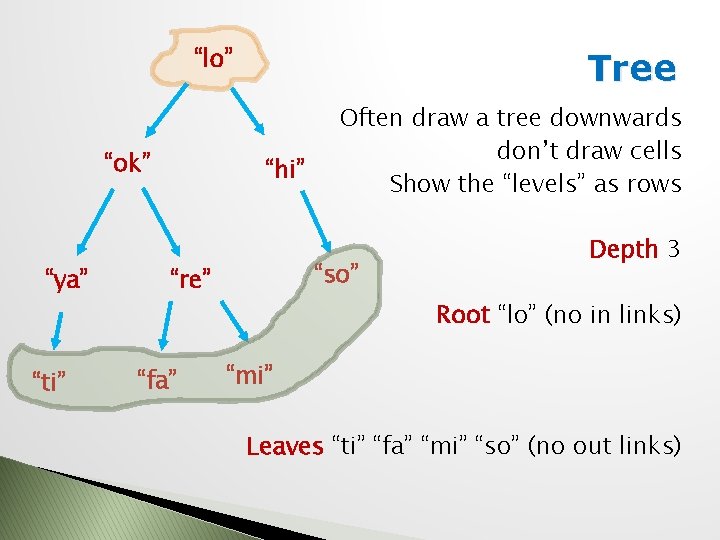
“lo” “ok” “ya” “ti” Tree “hi” “so” “re” “fa” Often draw a tree downwards don’t draw cells Show the “levels” as rows Depth 3 Root “lo” (no in links) “mi” Leaves “ti” “fa” “mi” “so” (no out links)
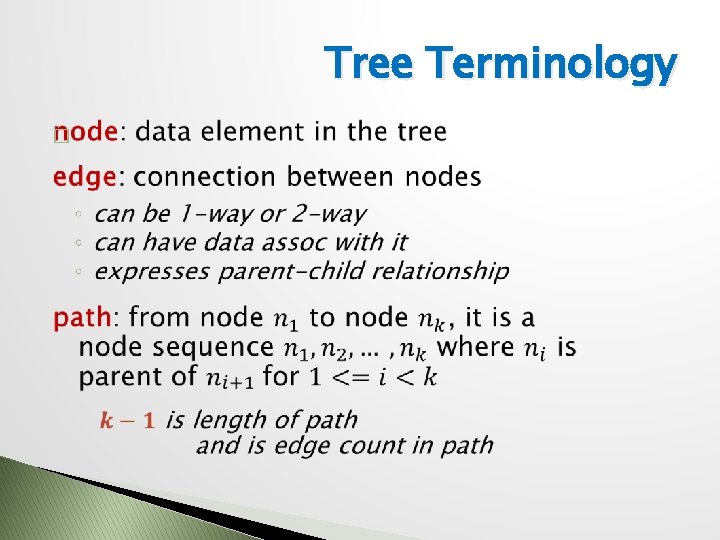
Tree Terminology �
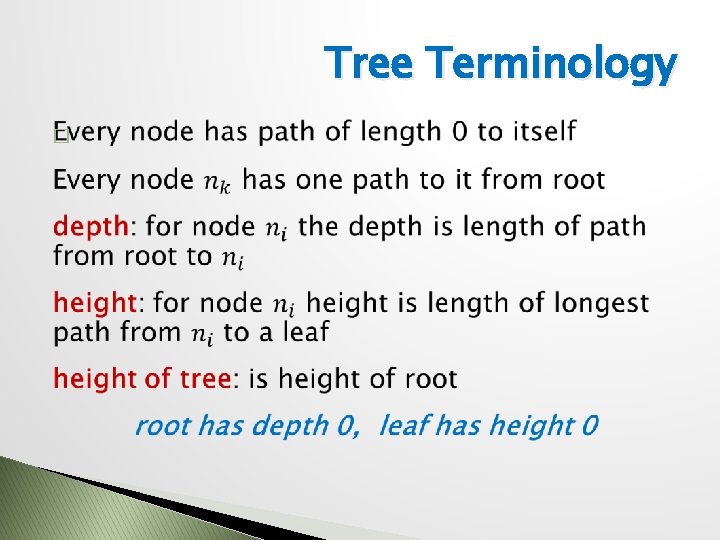
Tree Terminology �
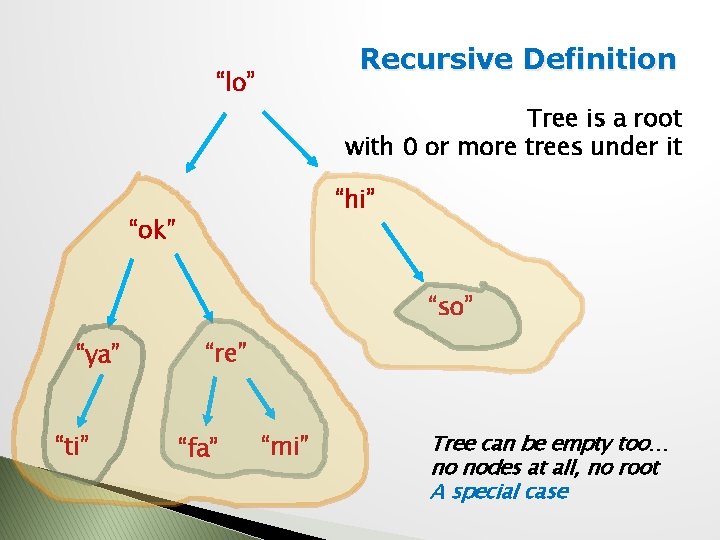
Recursive Definition “lo” Tree is a root with 0 or more trees under it “hi” “ok” “so” “ya” “ti” “re” “fa” “mi” Tree can be empty too… no nodes at all, no root A special case
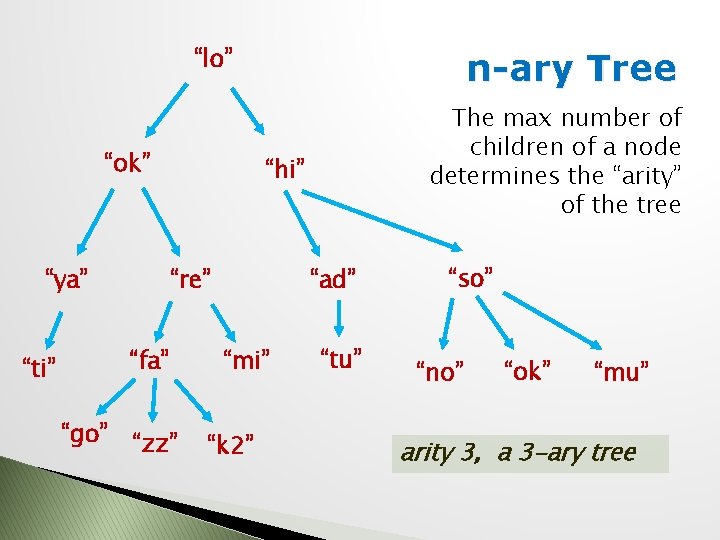
“lo” “ok” “ya” “go” The max number of children of a node determines the “arity” of the tree “hi” “re” “fa” “ti” n-ary Tree “zz” “ad” “mi” “k 2” “tu” “so” “no” “ok” “mu” arity 3, a 3 -ary tree
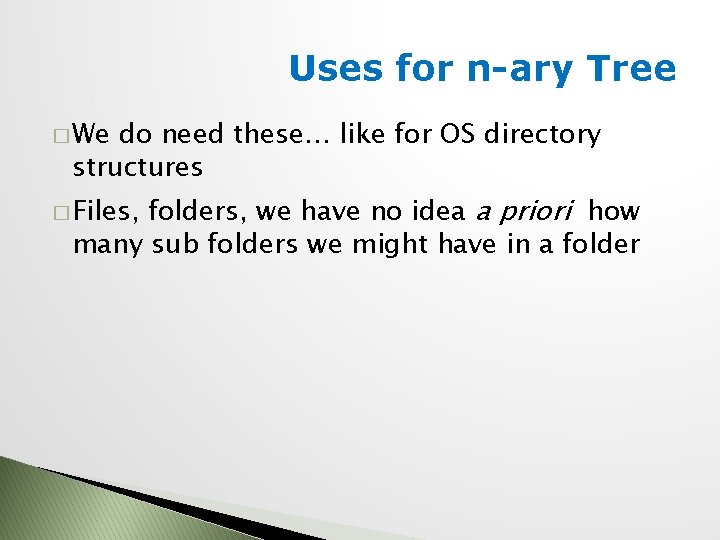
Uses for n-ary Tree � We do need these… like for OS directory structures folders, we have no idea a priori how many sub folders we might have in a folder � Files,
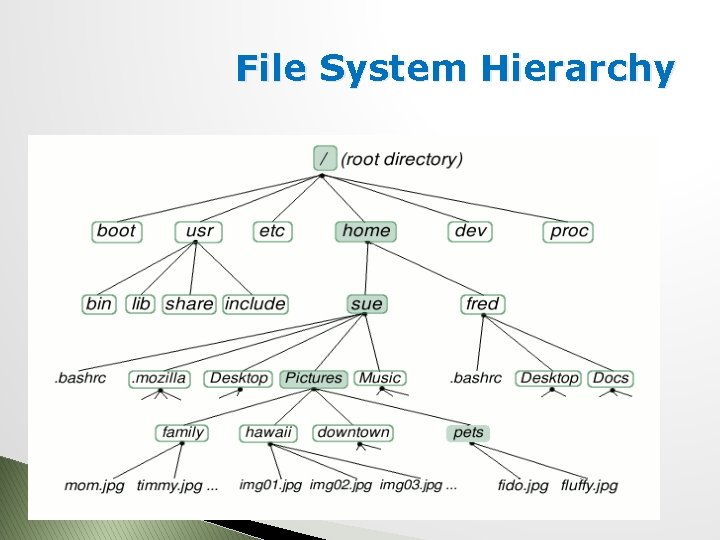
File System Hierarchy
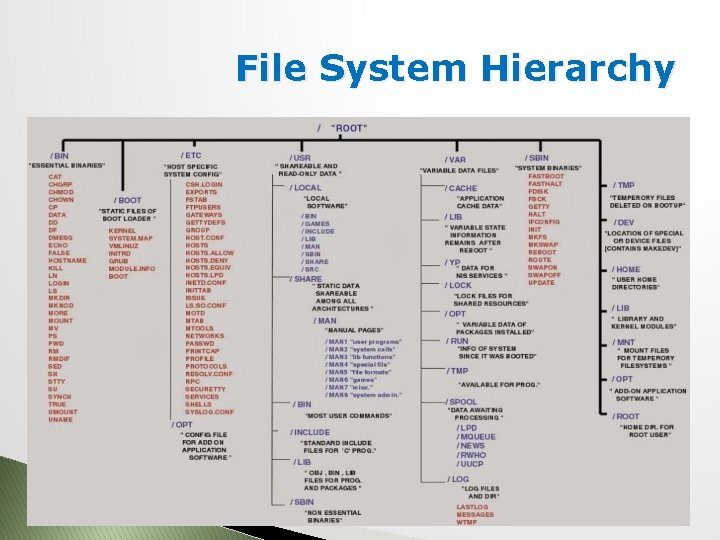
File System Hierarchy
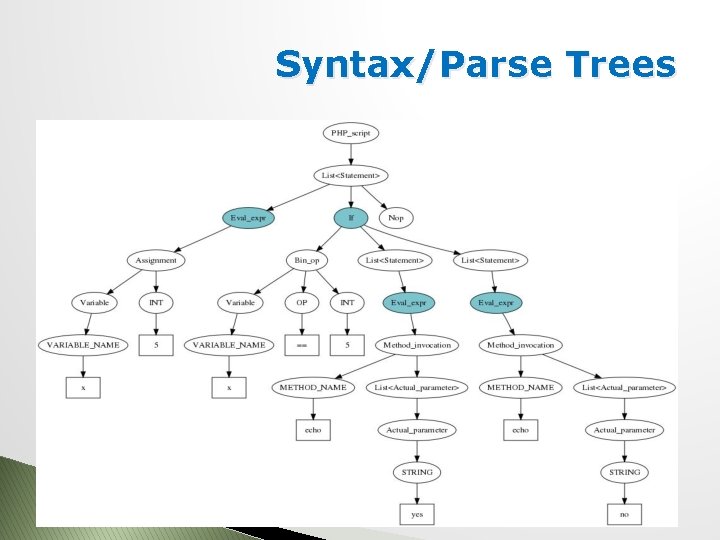
Syntax/Parse Trees
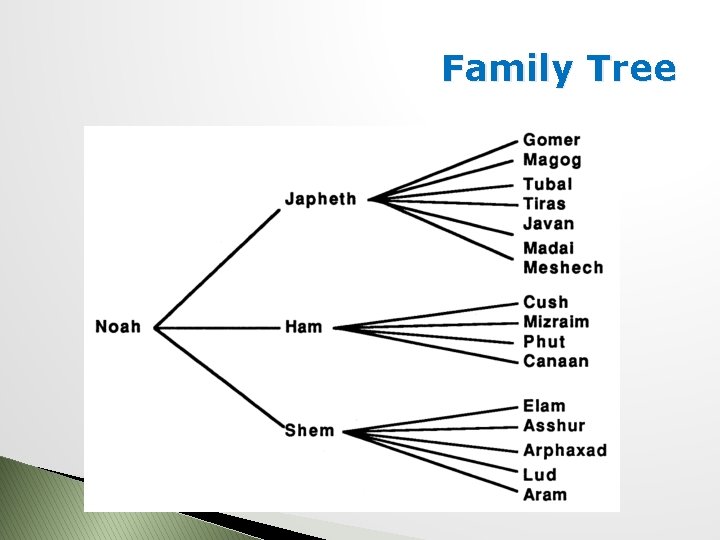
Family Tree
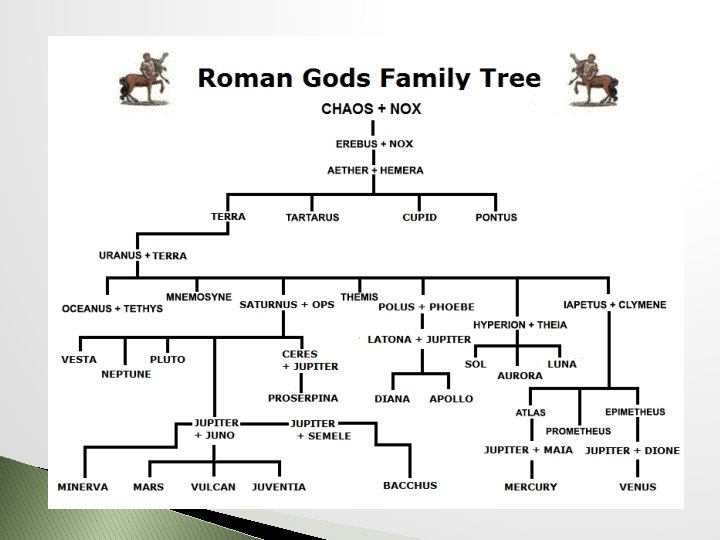
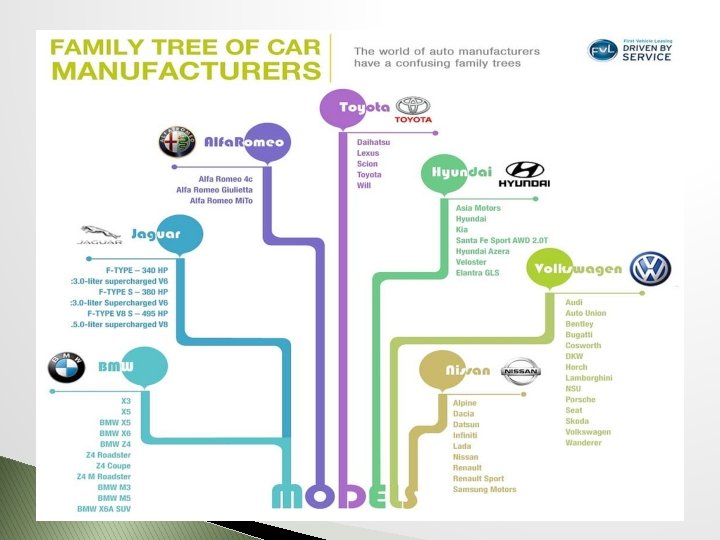
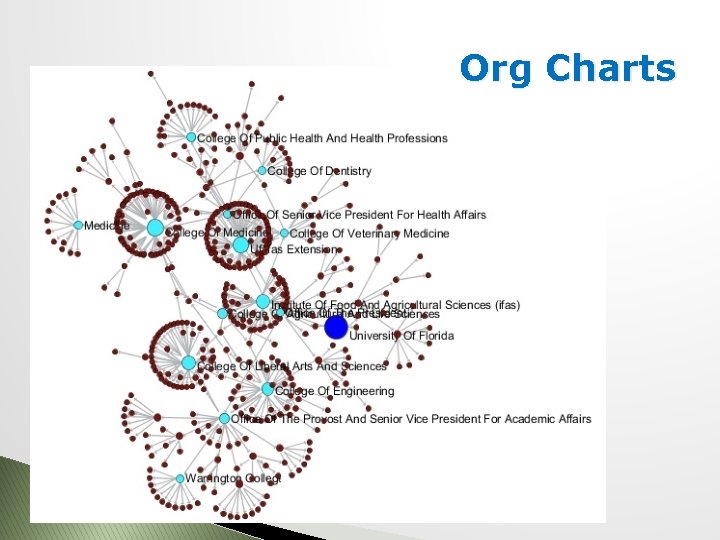
Org Charts
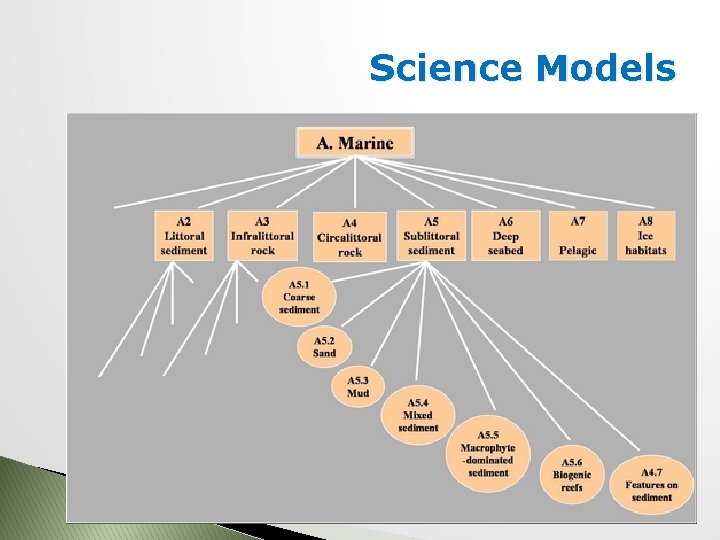
Science Models
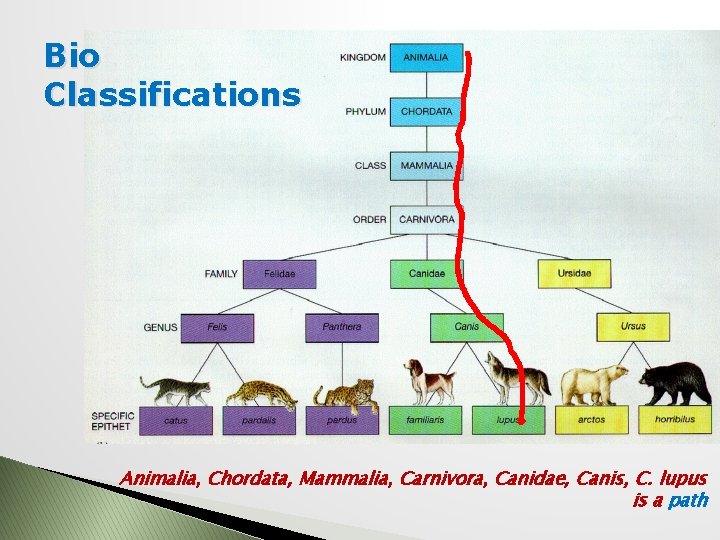
Bio Classifications Animalia, Chordata, Mammalia, Carnivora, Canidae, Canis, C. lupus is a path
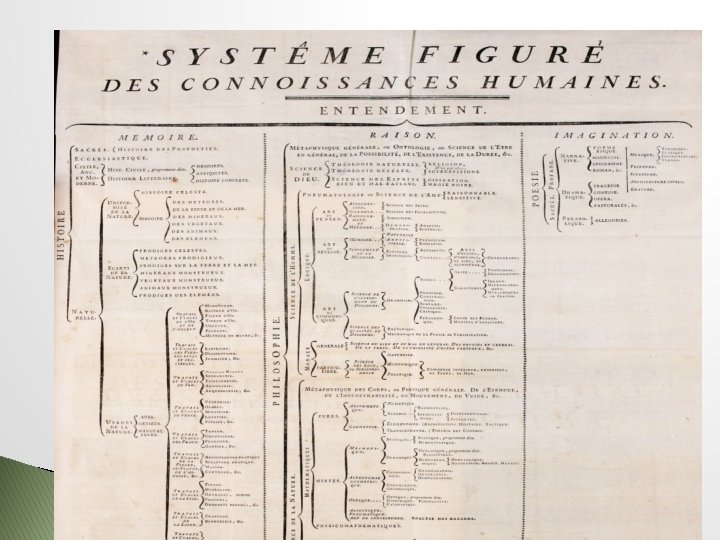
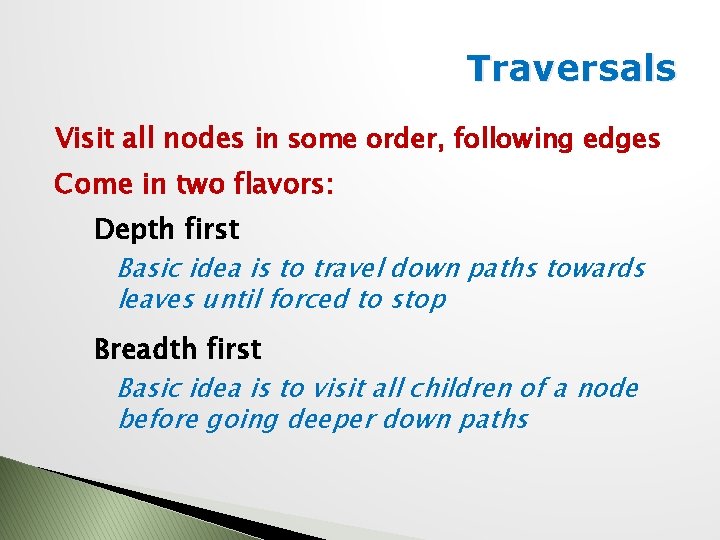
Traversals Visit all nodes in some order, following edges Come in two flavors: Depth first Basic idea is to travel down paths towards leaves until forced to stop Breadth first Basic idea is to visit all children of a node before going deeper down paths
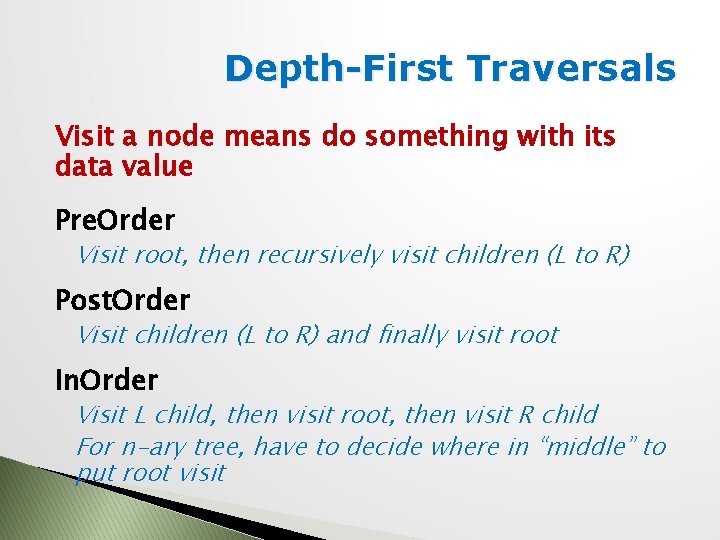
Depth-First Traversals Visit a node means do something with its data value Pre. Order Visit root, then recursively visit children (L to R) Post. Order Visit children (L to R) and finally visit root In. Order Visit L child, then visit root, then visit R child For n-ary tree, have to decide where in “middle” to put root visit
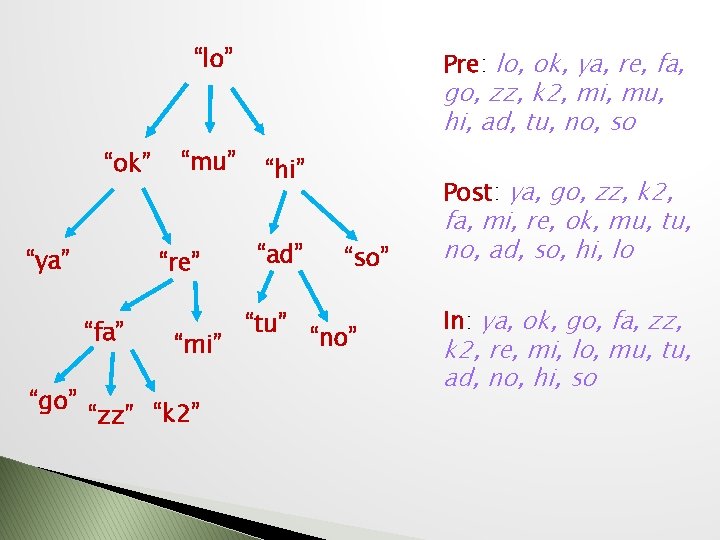
“lo” “ok” “ya” “re” “fa” “go” “mu” “mi” “zz” “k 2” Pre: lo, ok, ya, re, fa, go, zz, k 2, mi, mu, hi, ad, tu, no, so “hi” “ad” “tu” Post: ya, go, zz, k 2, “so” “no” fa, mi, re, ok, mu, tu, no, ad, so, hi, lo In: ya, ok, go, fa, zz, k 2, re, mi, lo, mu, tu, ad, no, hi, so
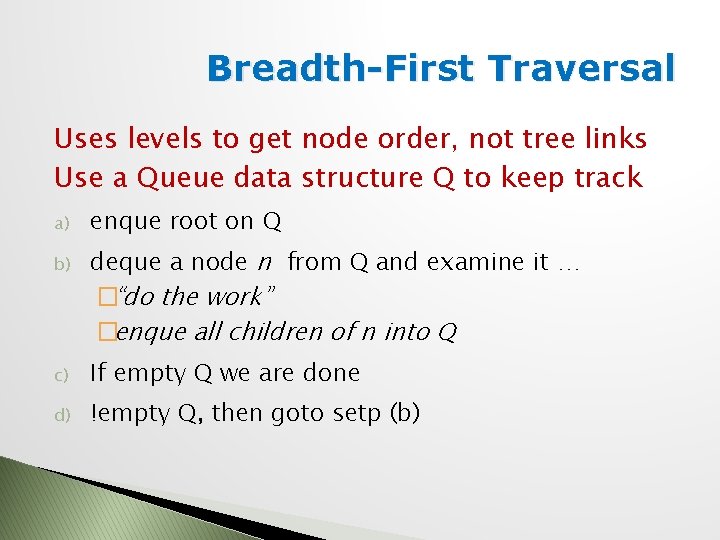
Breadth-First Traversal Uses levels to get node order, not tree links Use a Queue data structure Q to keep track a) b) enque root on Q deque a node n from Q and examine it … �“do the work” �enque all children of n into Q c) If empty Q we are done d) !empty Q, then goto setp (b)
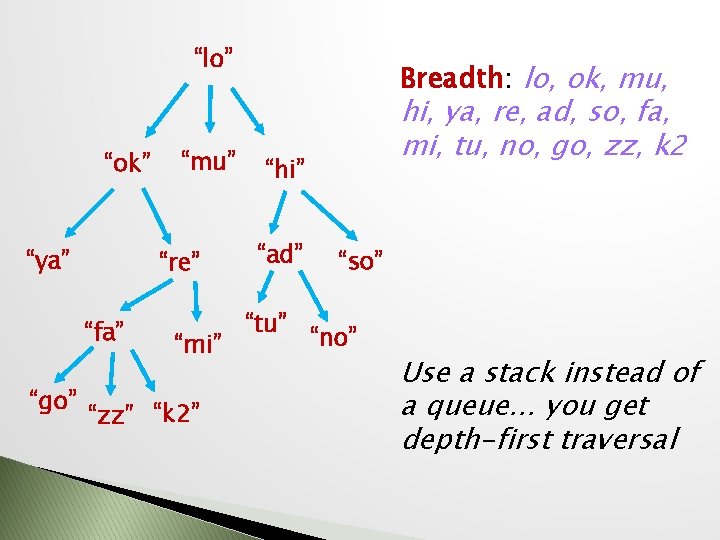
“lo” “ok” “ya” “re” “fa” “go” “mu” “mi” “zz” “k 2” Breadth: lo, ok, mu, hi, ya, re, ad, so, fa, mi, tu, no, go, zz, k 2 “hi” “ad” “tu” “so” “no” Use a stack instead of a queue… you get depth-first traversal
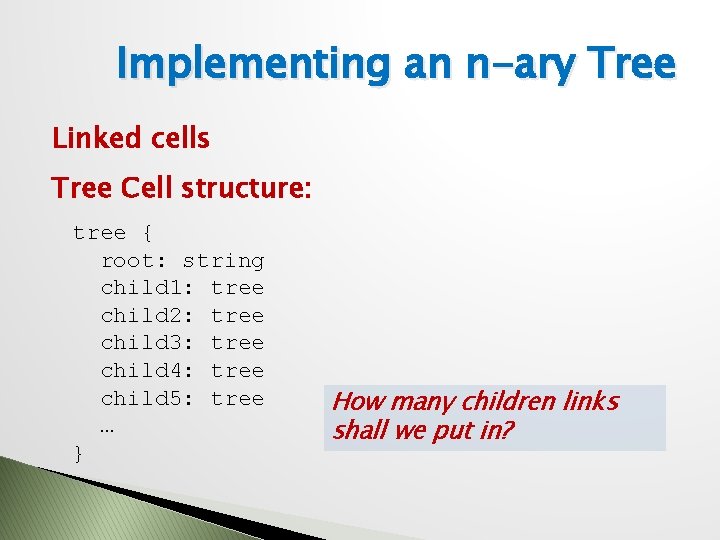
Implementing an n-ary Tree Linked cells Tree Cell structure: tree { root: string child 1: tree child 2: tree child 3: tree child 4: tree child 5: tree … } How many children links shall we put in?
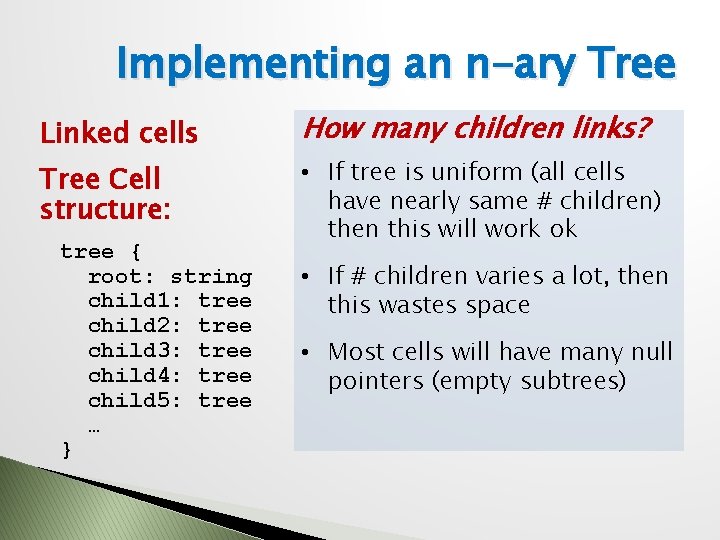
Implementing an n-ary Tree Linked cells Tree Cell structure: tree { root: string child 1: tree child 2: tree child 3: tree child 4: tree child 5: tree … } How many children links? • If tree is uniform (all cells have nearly same # children) then this will work ok • If # children varies a lot, then this wastes space • Most cells will have many null pointers (empty subtrees)
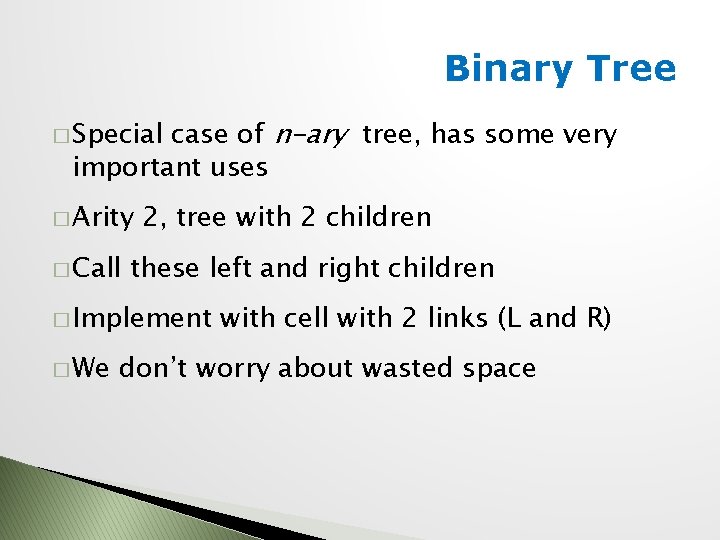
Binary Tree case of n-ary tree, has some very important uses � Special � Arity � Call 2, tree with 2 children these left and right children � Implement � We with cell with 2 links (L and R) don’t worry about wasted space
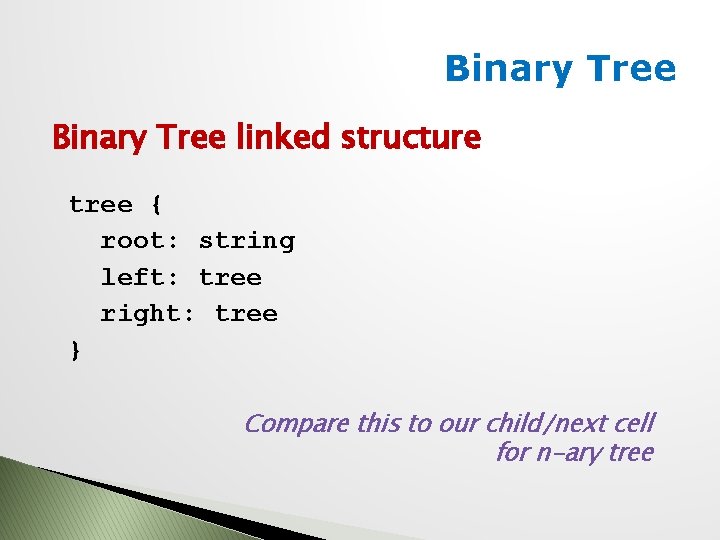
Binary Tree linked structure tree { root: string left: tree right: tree } Compare this to our child/next cell for n-ary tree
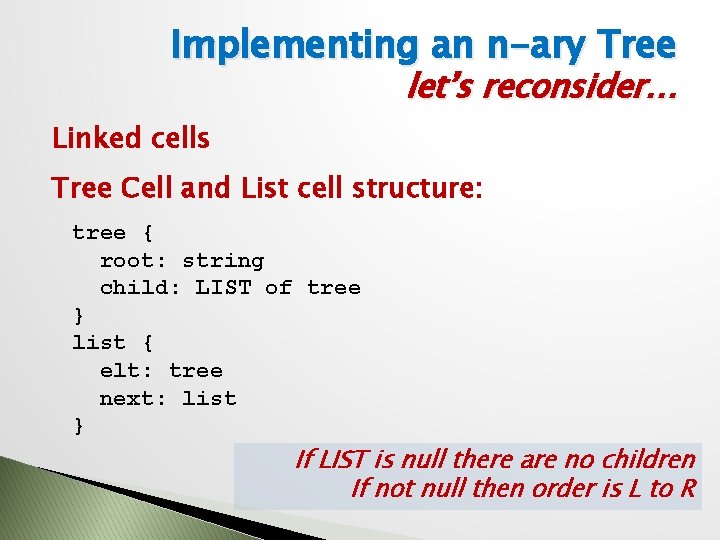
Implementing an n-ary Tree let’s reconsider… Linked cells Tree Cell and List cell structure: tree { root: string child: LIST of tree } list { elt: tree next: list } If LIST is null there are no children If not null then order is L to R
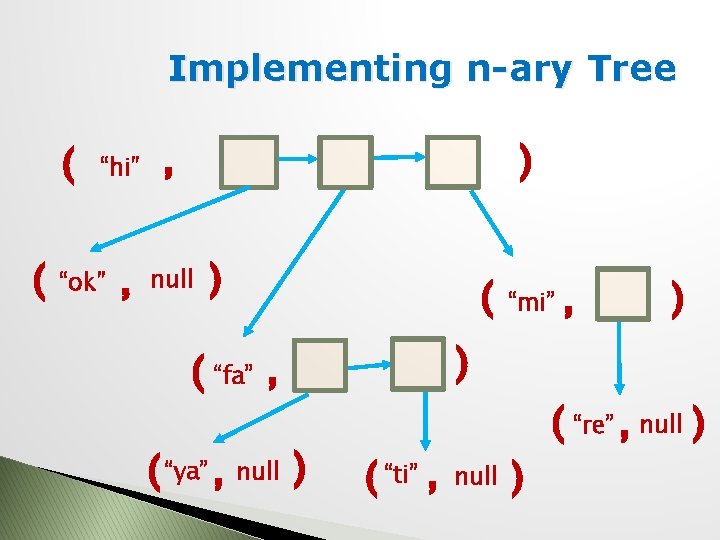
Implementing n-ary Tree ( ( “hi” , , null “ok” ) ) ( “fa” , ( “ya” , null ) “mi” ( “ti” , null ) , ) ( “re” , null )
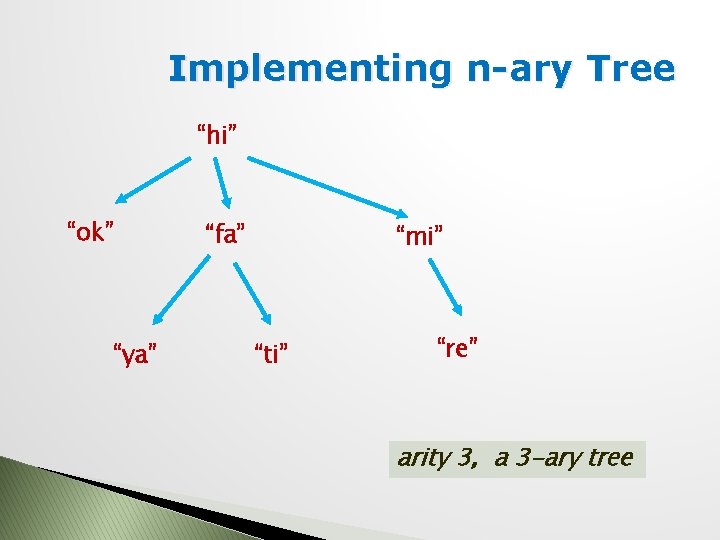
Implementing n-ary Tree “hi” “ok” “ya” “fa” “mi” “ti” “re” arity 3, a 3 -ary tree
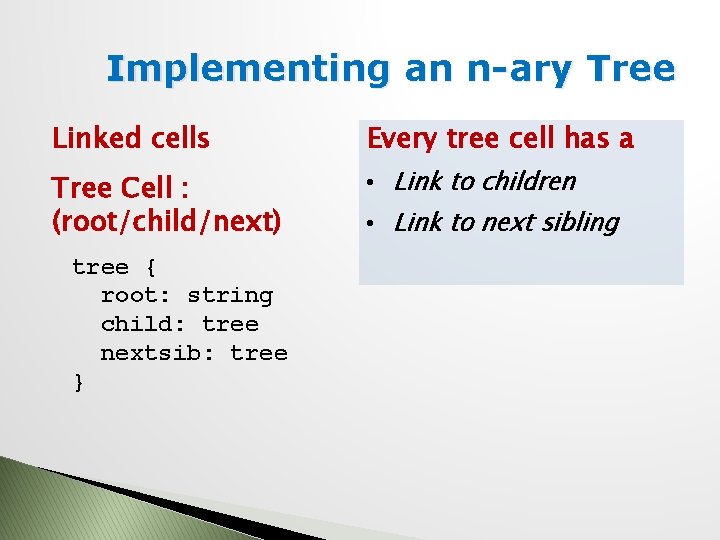
Implementing an n-ary Tree Linked cells Every tree cell has a Tree Cell : (root/child/next) • Link to children tree { root: string child: tree nextsib: tree } • Link to next sibling
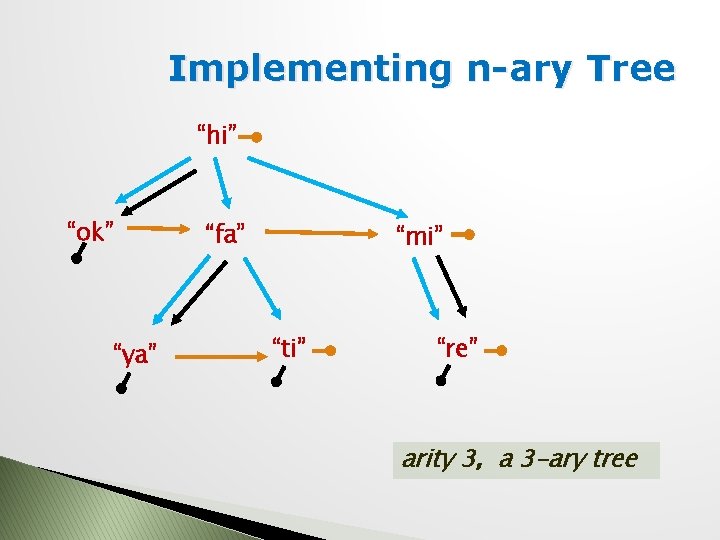
Implementing n-ary Tree “hi” “ok” “ya” “fa” “mi” “ti” “re” arity 3, a 3 -ary tree
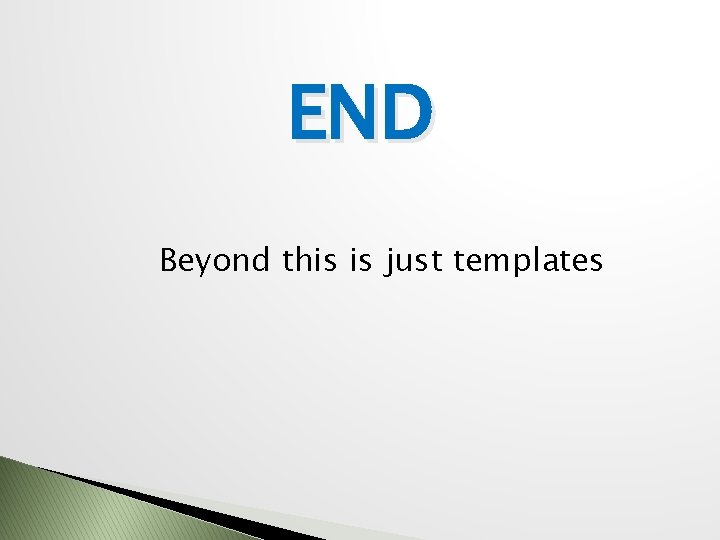
END Beyond this is just templates
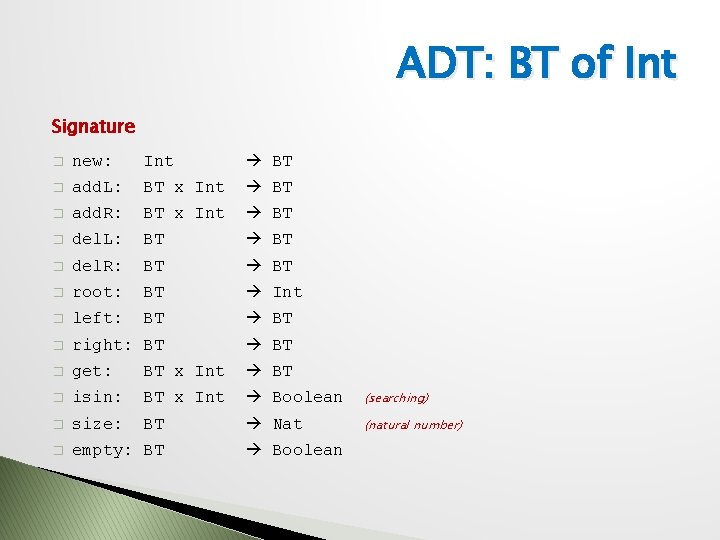
ADT: BT of Int Signature � new: Int BT � add. L: BT x Int BT � add. R: BT x Int BT � del. L: BT � del. R: BT � root: BT Int � left: BT � right: BT � get: BT x Int BT � isin: BT x Int Boolean (searching) � size: BT Nat (natural number) � empty: BT Boolean
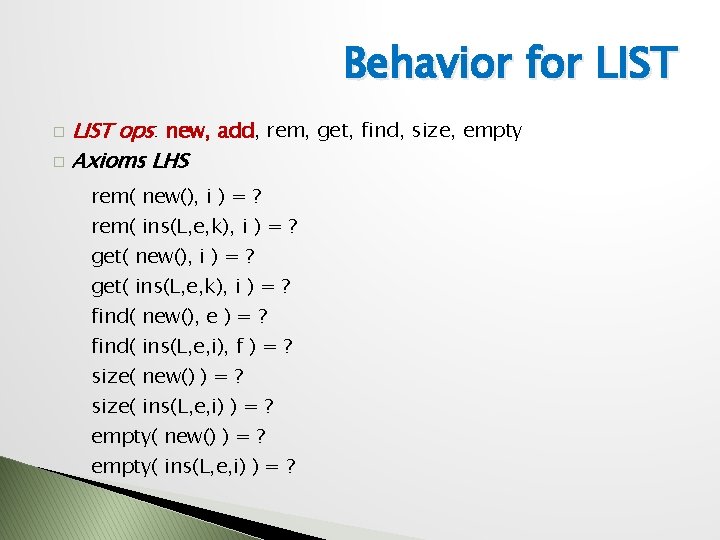
Behavior for LIST ops: new, add, rem, get, find, size, empty � Axioms LHS � rem( new(), i ) = ? rem( ins(L, e, k), i ) = ? get( new(), i ) = ? get( ins(L, e, k), i ) = ? find( new(), e ) = ? find( ins(L, e, i), f ) = ? size( new() ) = ? size( ins(L, e, i) ) = ? empty( new() ) = ? empty( ins(L, e, i) ) = ?
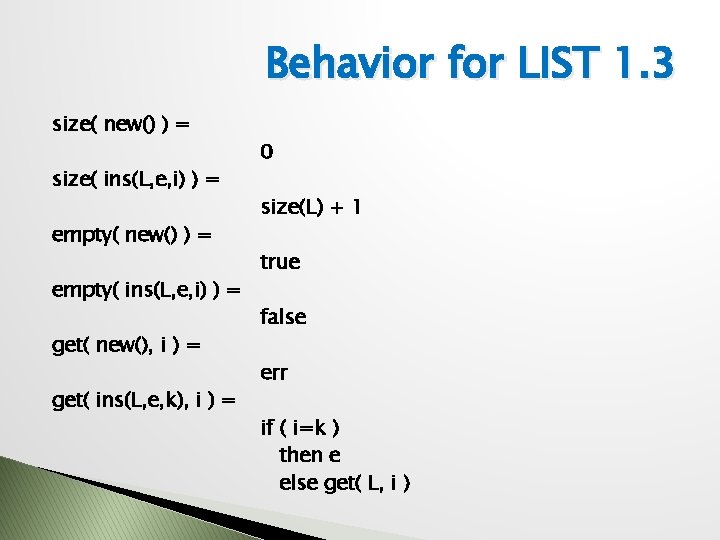
Behavior for LIST 1. 3 size( new() ) = size( ins(L, e, i) ) = empty( new() ) = empty( ins(L, e, i) ) = get( new(), i ) = get( ins(L, e, k), i ) = 0 size(L) + 1 true false err if ( i=k ) then e else get( L, i )
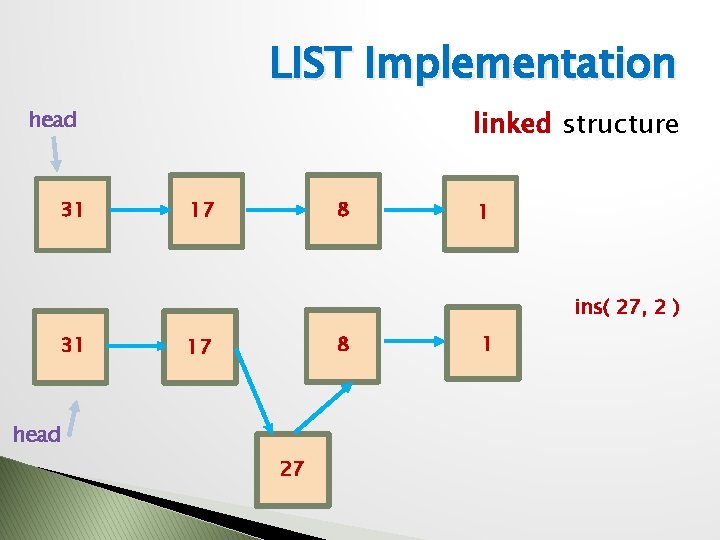
LIST Implementation linked structure head 31 17 8 1 ins( 27, 2 ) 31 8 17 head 27 1
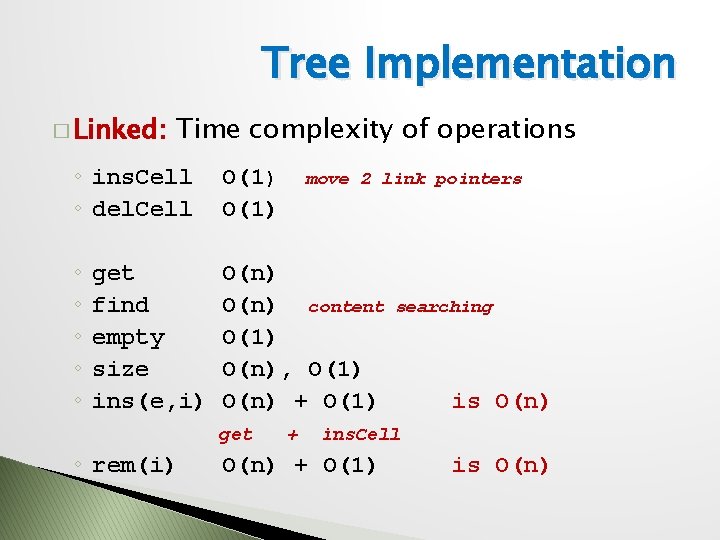
Tree Implementation � Linked: Time complexity of operations ◦ ins. Cell ◦ del. Cell O(1) ◦ ◦ ◦ O(n) content O(1) O(n), O(1) O(n) + O(1) get find empty size ins(e, i) get ◦ rem(i) move 2 link pointers + searching is O(n) ins. Cell O(n) + O(1) is O(n)
Comp 410
Homologous structures examples
Inverted indexing
Ved yöntemi
Kov-21 skl
15-410 cmu
Iat 410
Si-410
Si-410
6-12/410
Pengertian pangkat akar dan logaritma
The bradley family owns 410 acres
What is cross projection sketch
Section 16–3 the process of speciation (pages 404–410)
Ipm 410-3 (shikha) price
Svsd 410
Si 410
Buss 410
Alunda innebandy
Cse 410
Cpsc 410
Barracuda web filter 410
Comp set data
Professor ajit diwan
Kevin wayne princeton
Data structures and algorithms tutorial
Conditional macro expansion in system software
Assembler algorithm and data structures
Information retrieval data structures and algorithms
Data structures and abstractions with java
Data structures and algorithms bits pilani
Adts, data structures, and problem solving with c++
Ajit diwan iit bombay
Data structures and algorithm
Data structures and algorithms
Data structures and algorithms
Waterloo data structures and algorithms
Information retrieval data structures and algorithms
Ephemeral data structure
Data structures and algorithms
Procedures of data collection