CS 1101 Programming Methodology http www comp nus
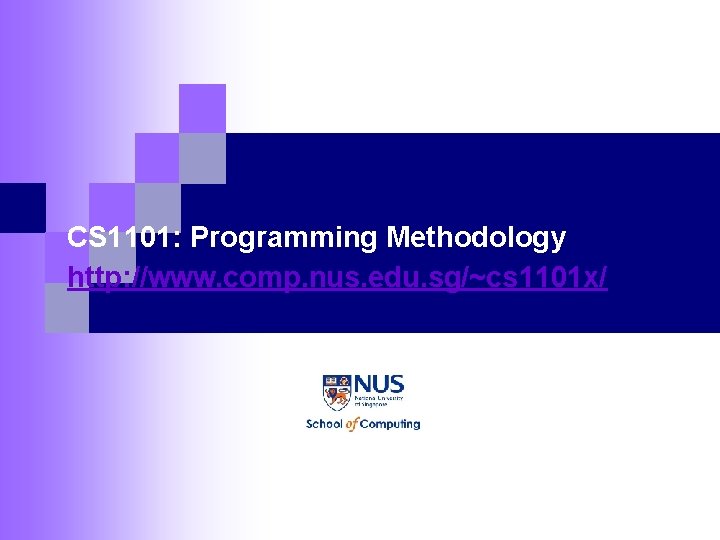
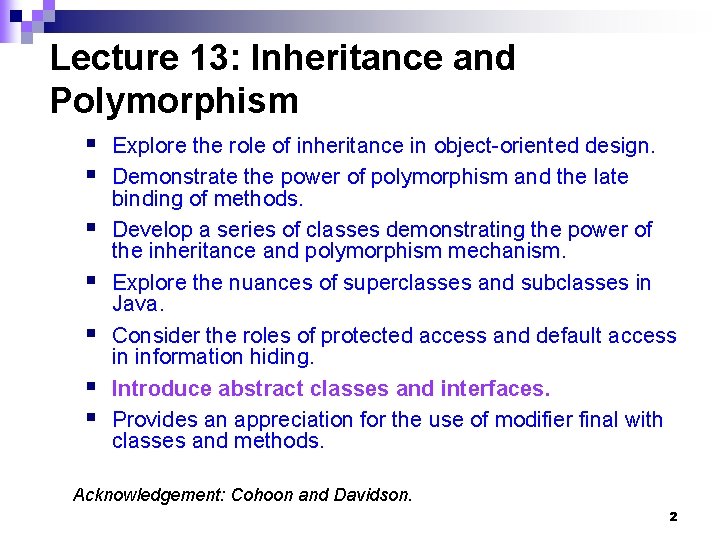
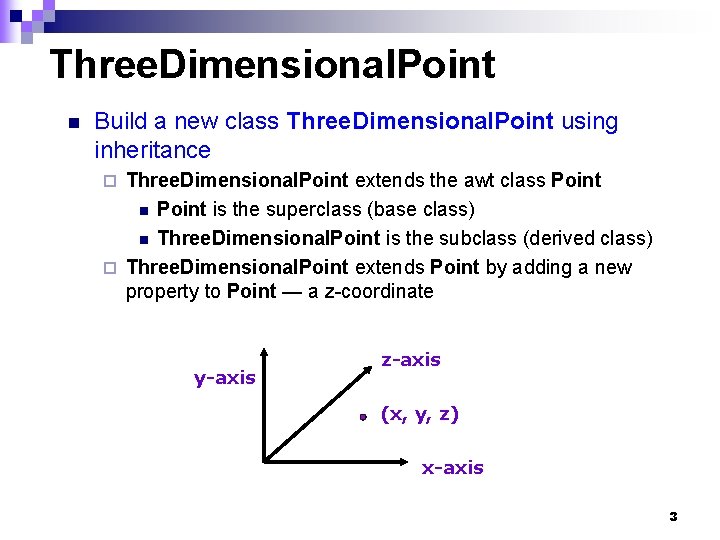
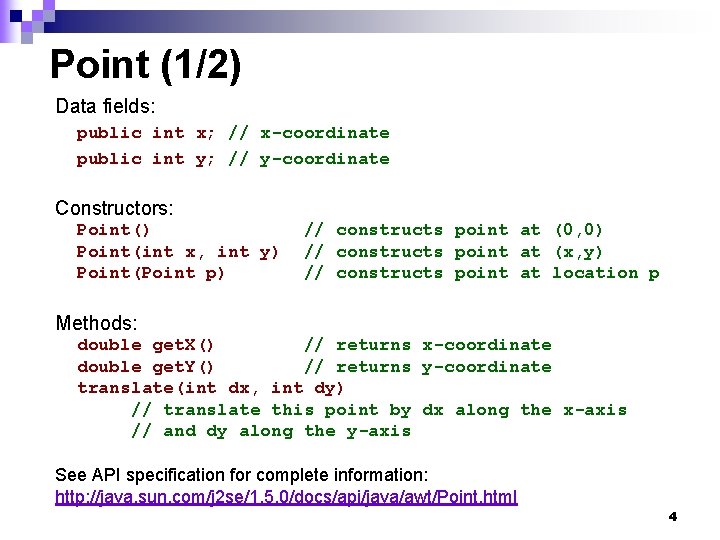
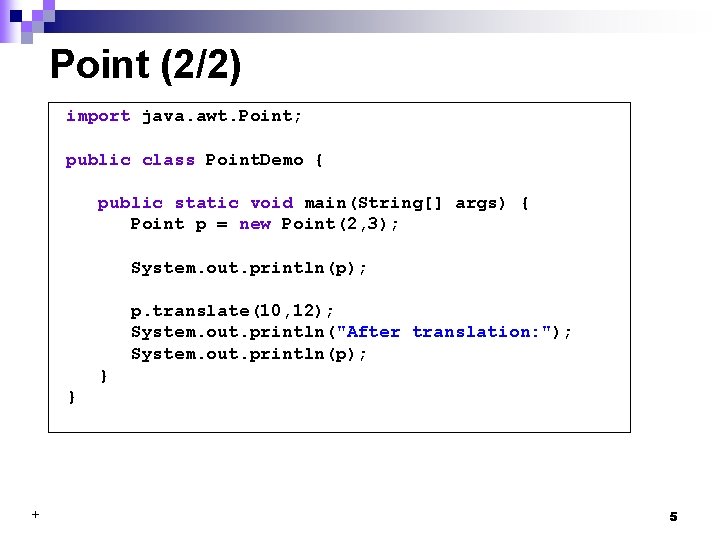
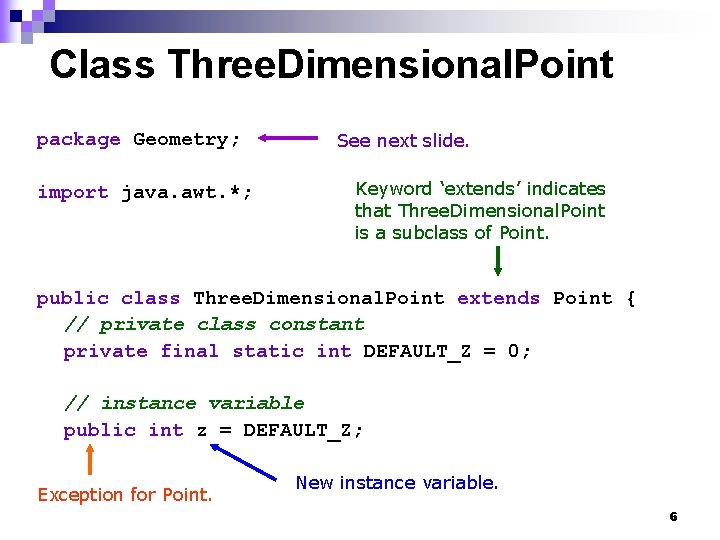
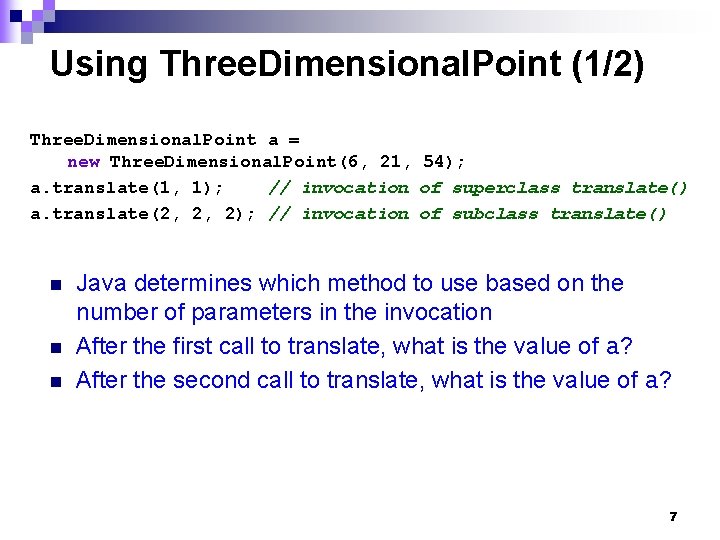
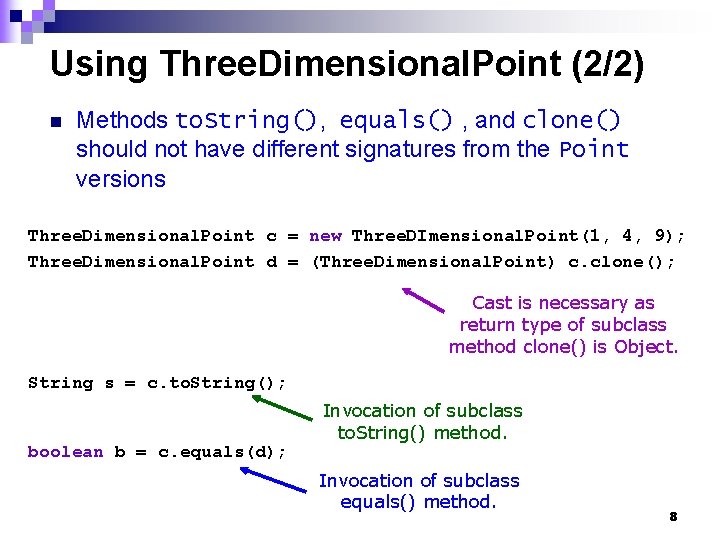
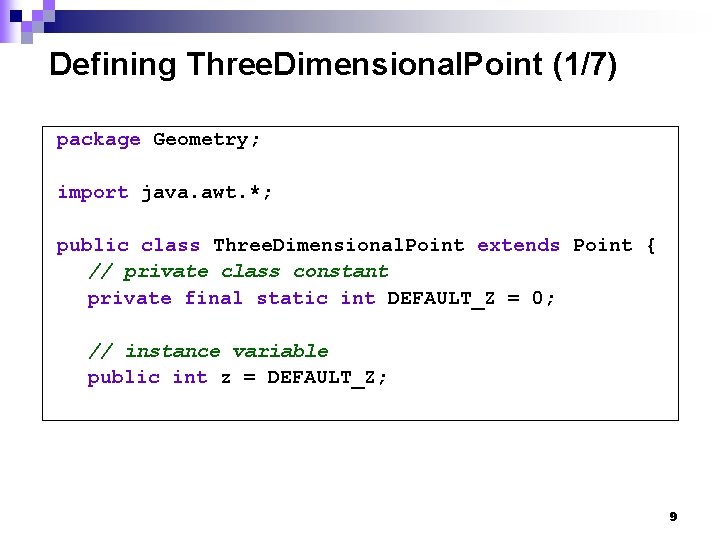
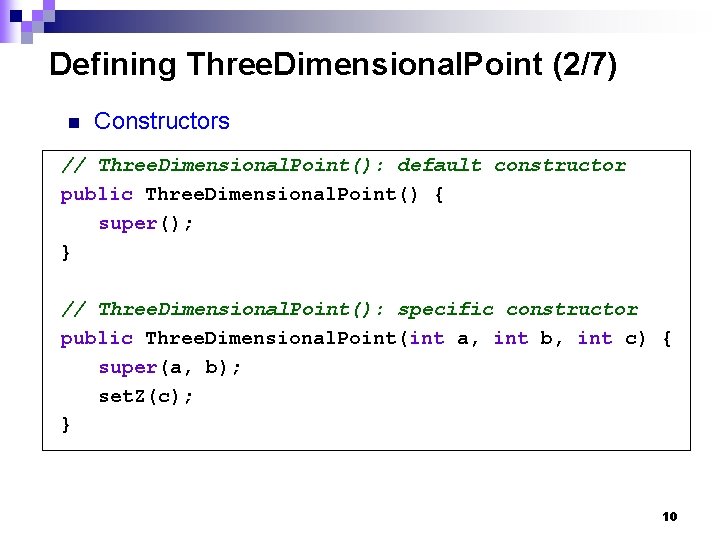
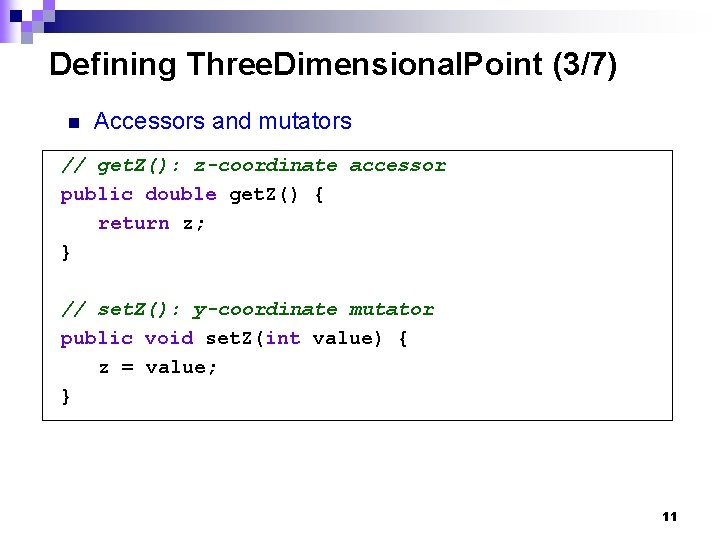
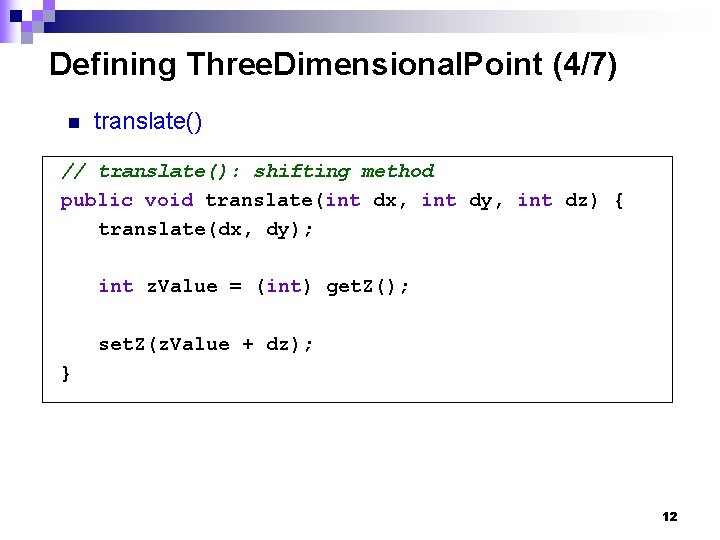
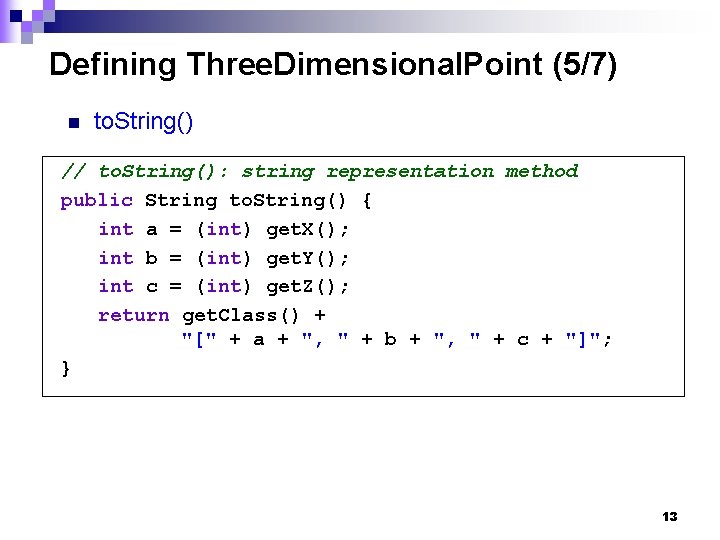
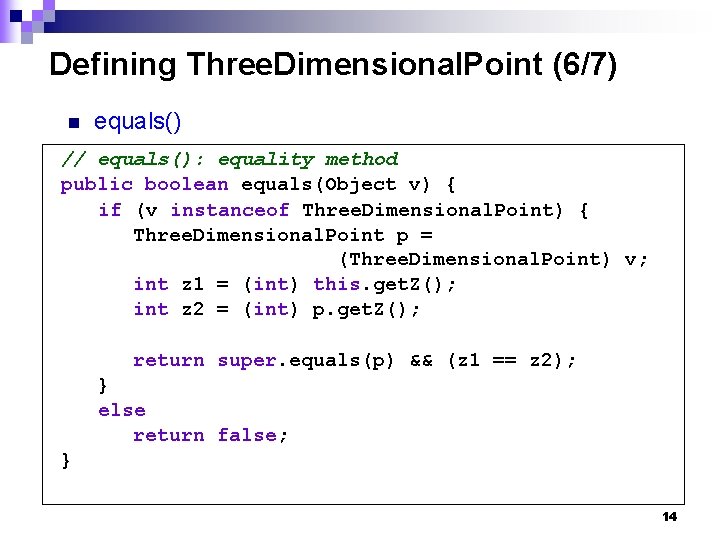
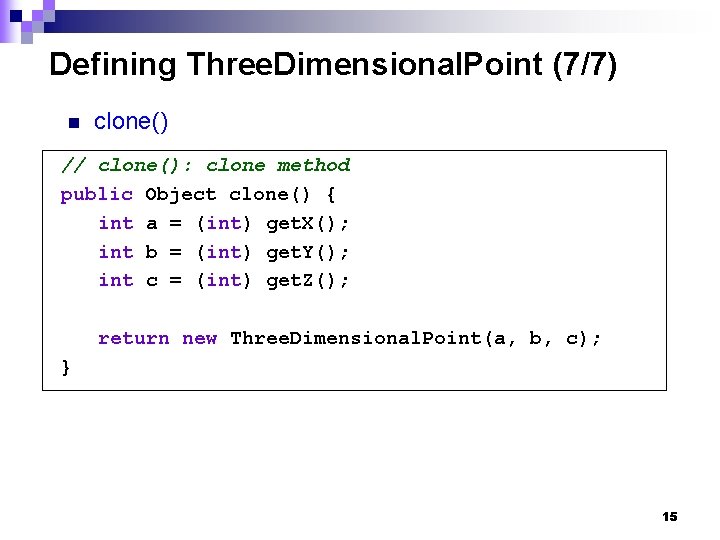
![Three. Dimensional. Point Demo public static void main(String[] args) { Three. Dimensonal. Point p Three. Dimensional. Point Demo public static void main(String[] args) { Three. Dimensonal. Point p](https://slidetodoc.com/presentation_image_h/0ed93bb942b82f663a28fcb2906e0e20/image-16.jpg)
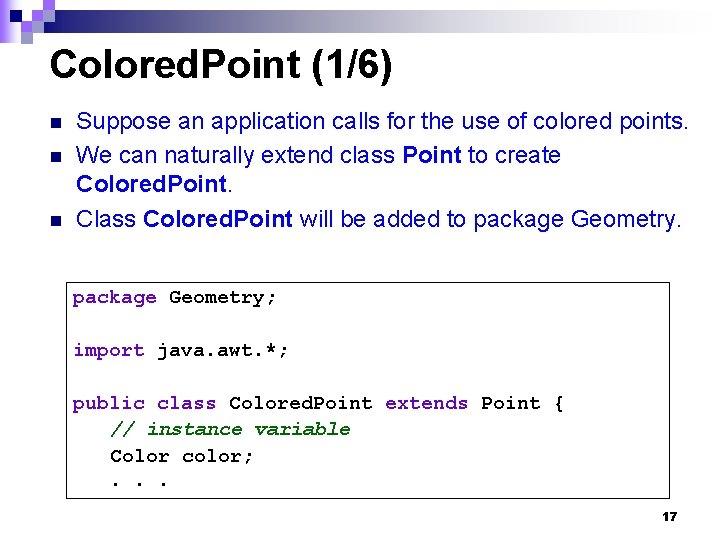
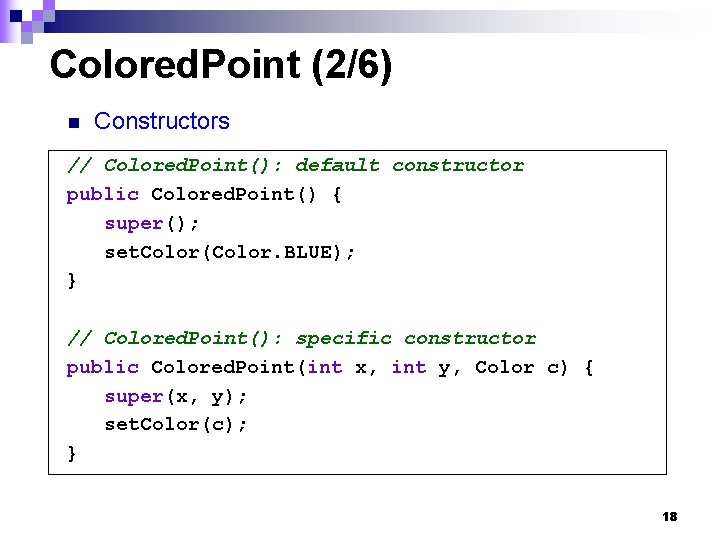
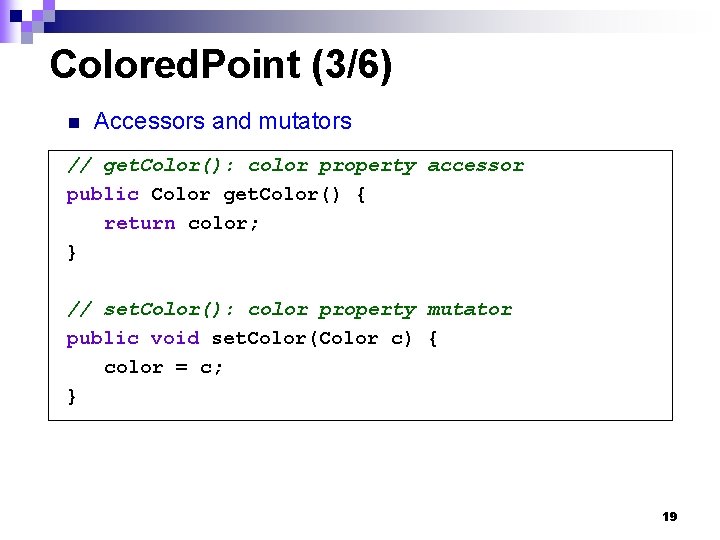
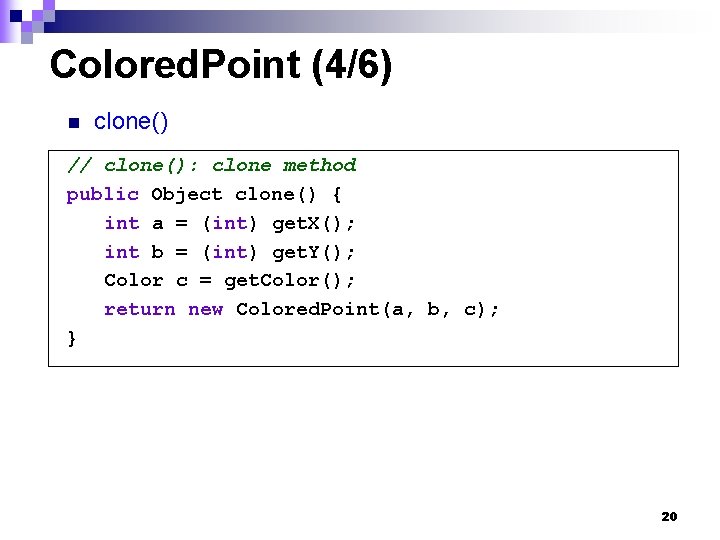
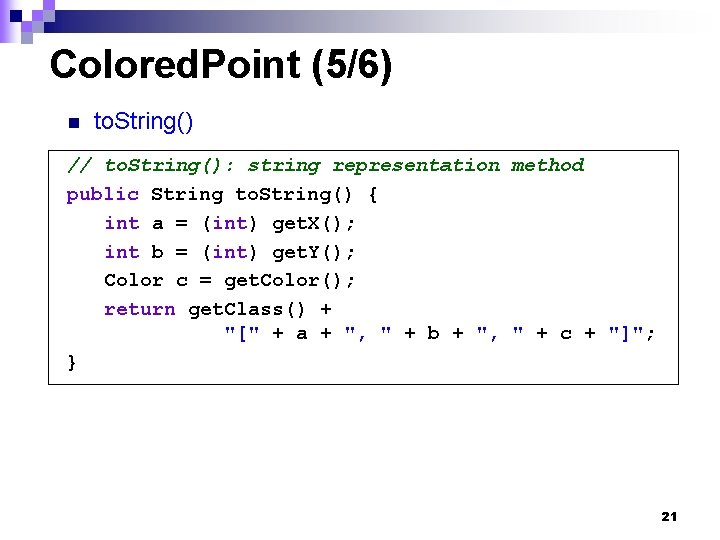
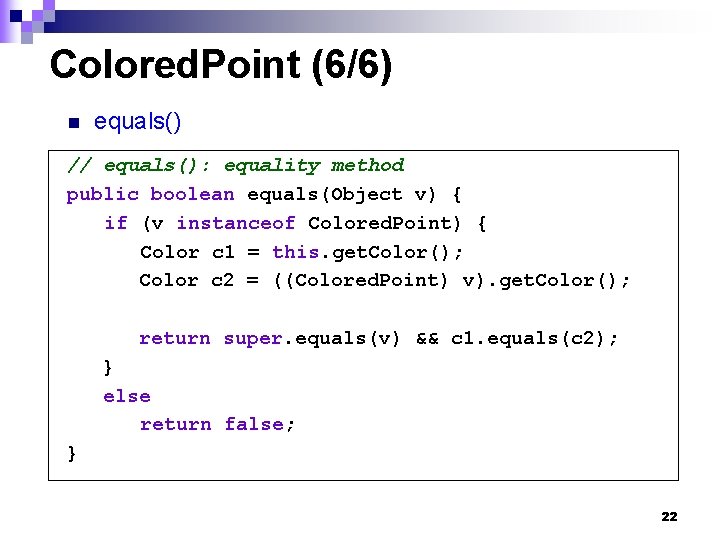
![Colored. Point Demo public static void main(String[] args) { Colored. Point p = new Colored. Point Demo public static void main(String[] args) { Colored. Point p = new](https://slidetodoc.com/presentation_image_h/0ed93bb942b82f663a28fcb2906e0e20/image-23.jpg)
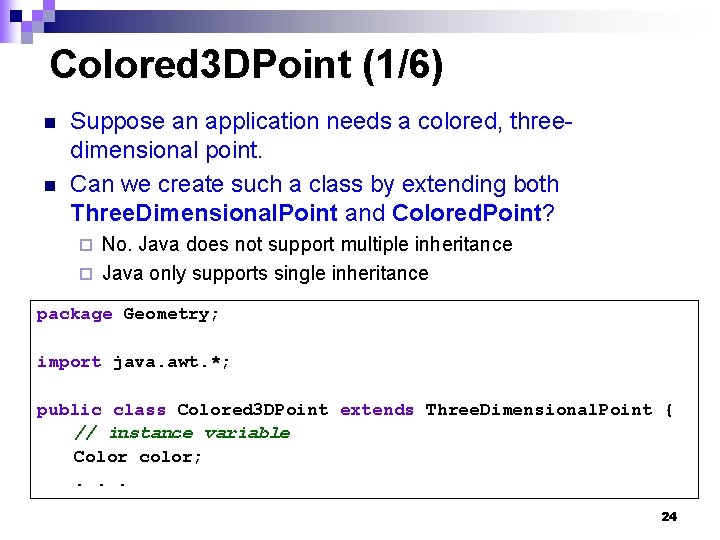
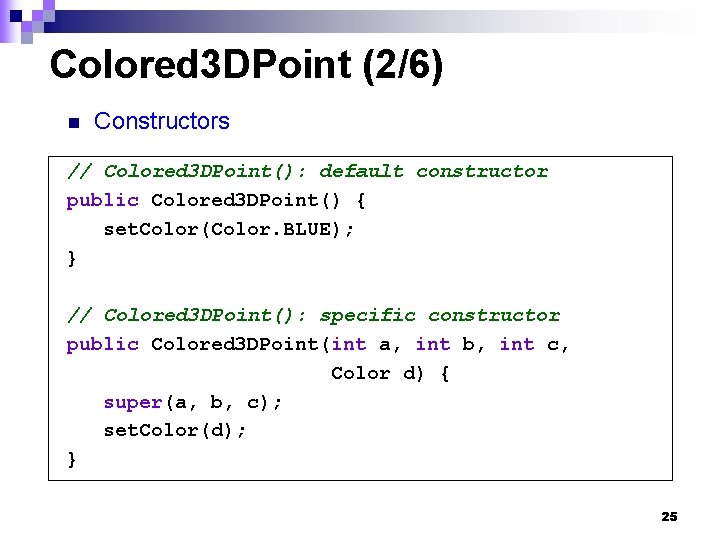
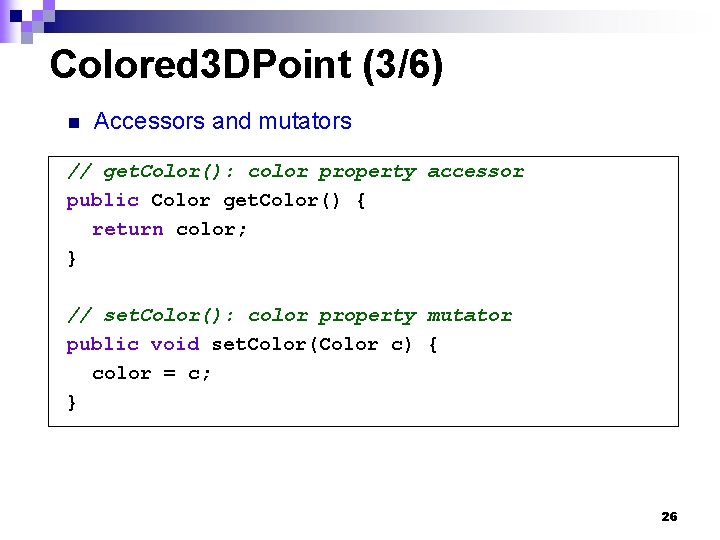
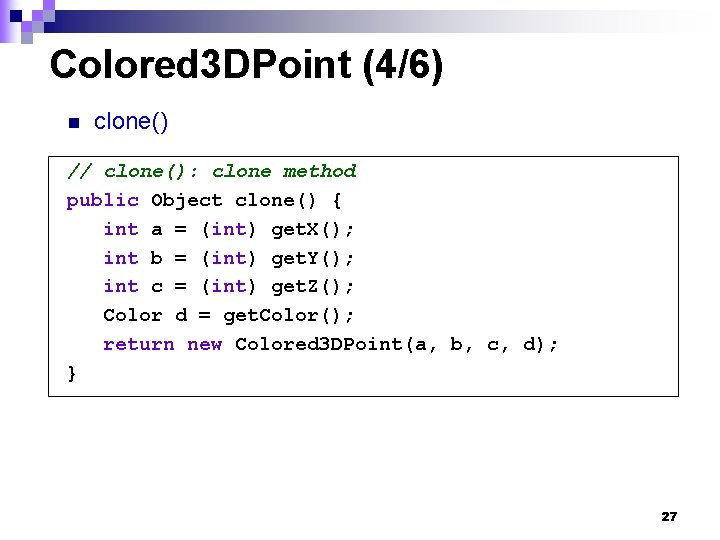
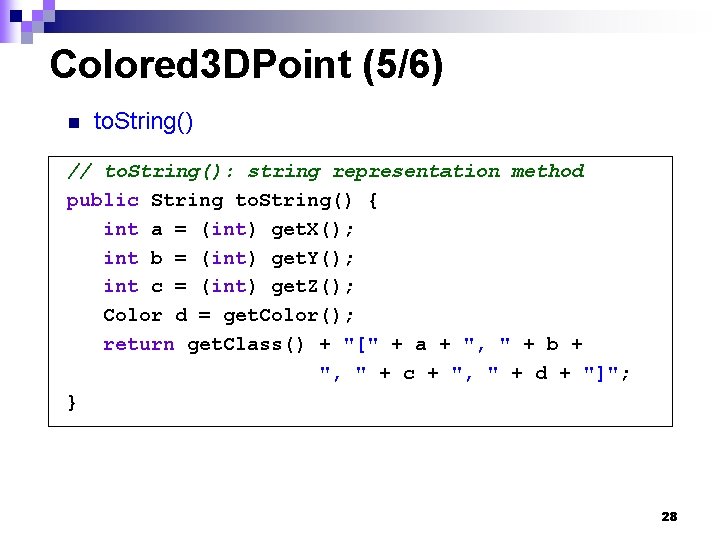
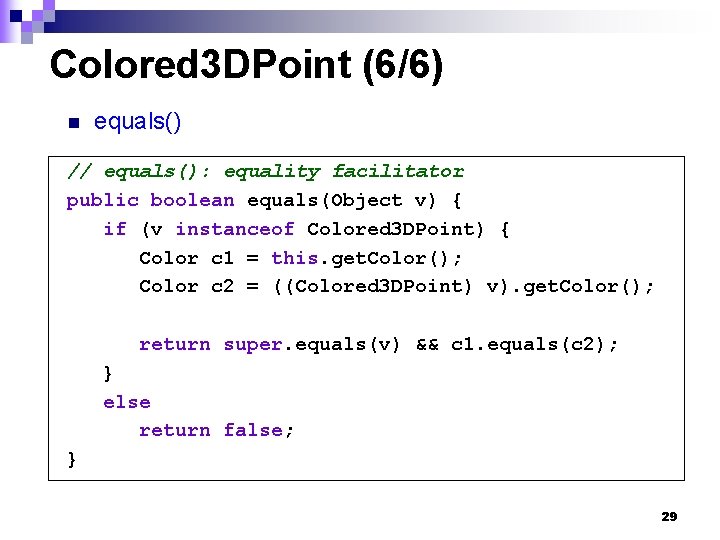
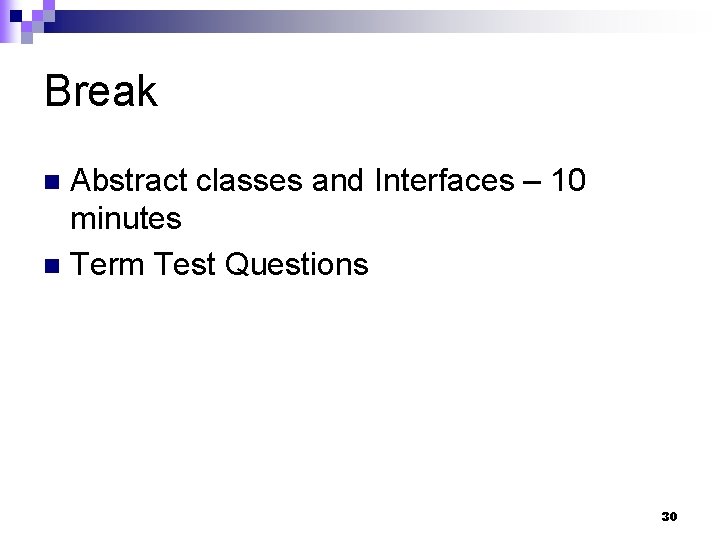
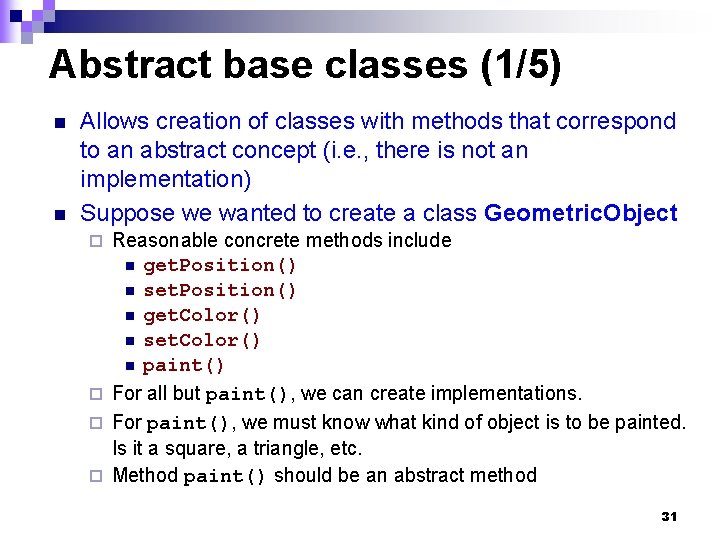
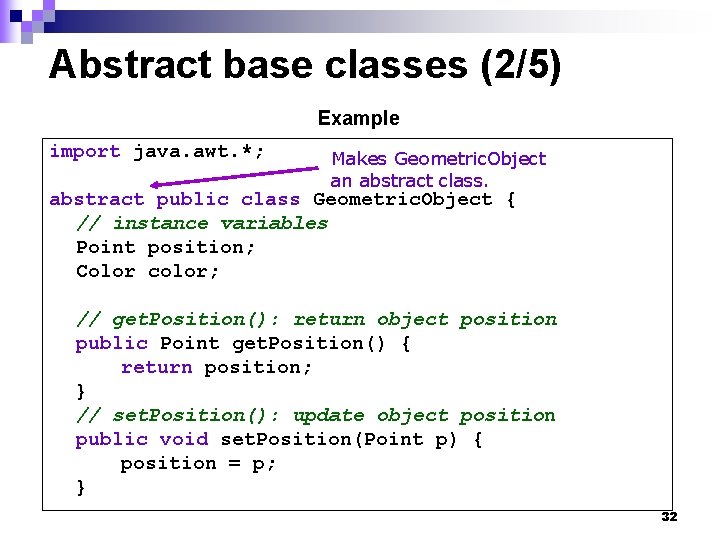
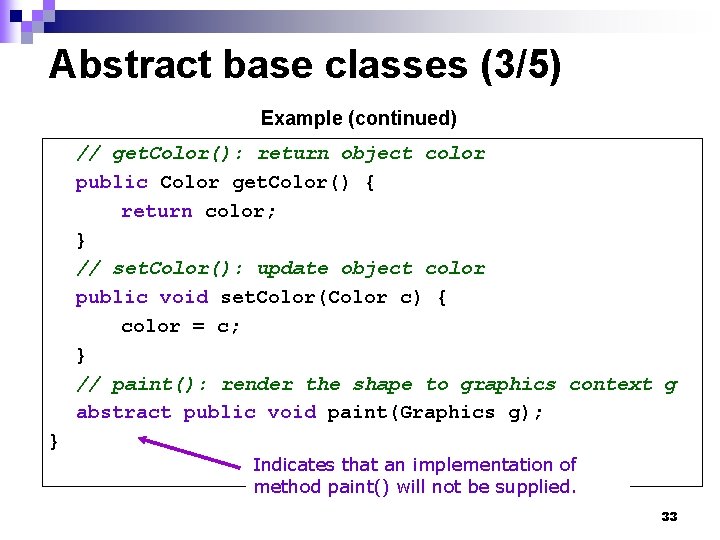
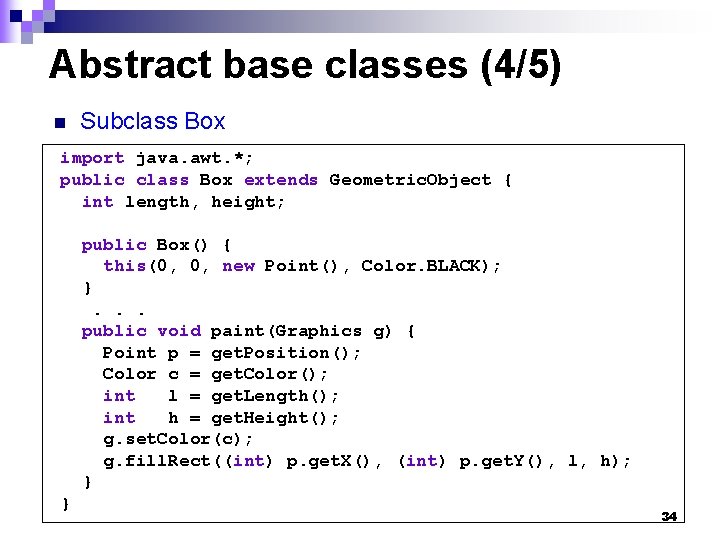
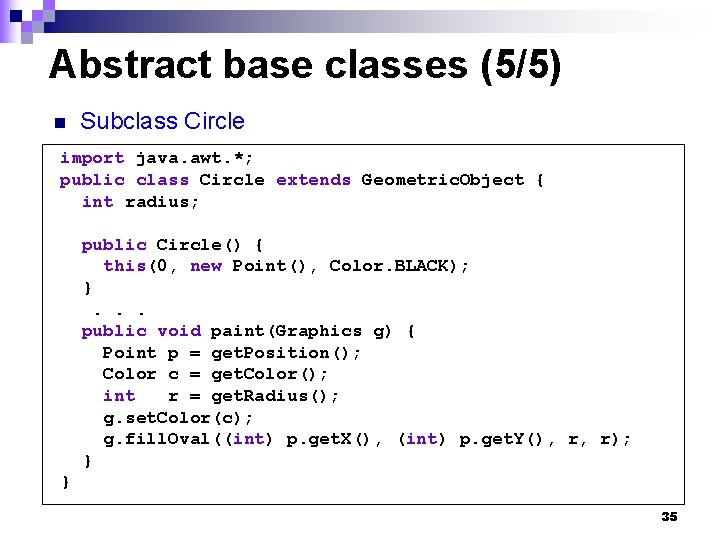
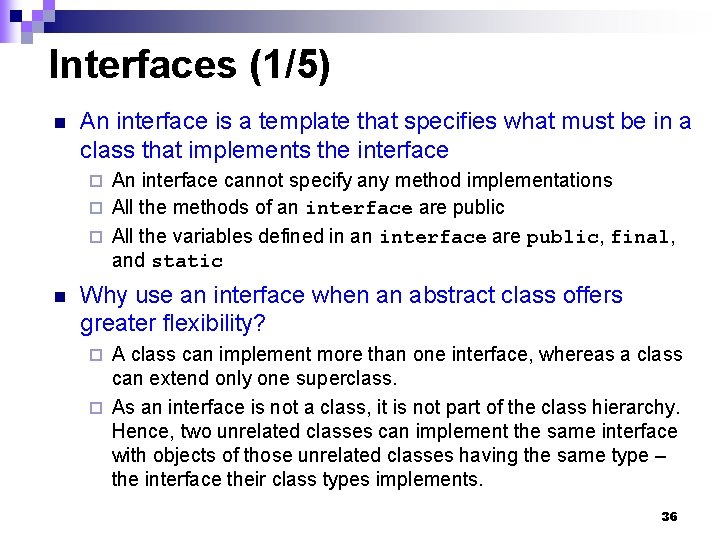
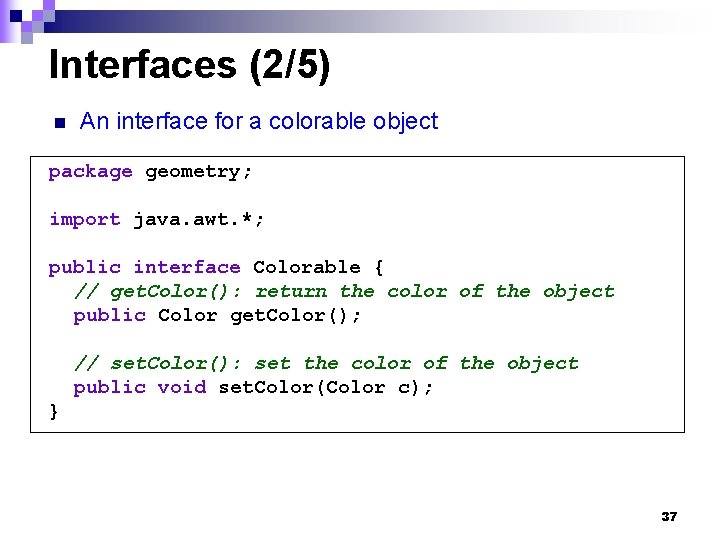
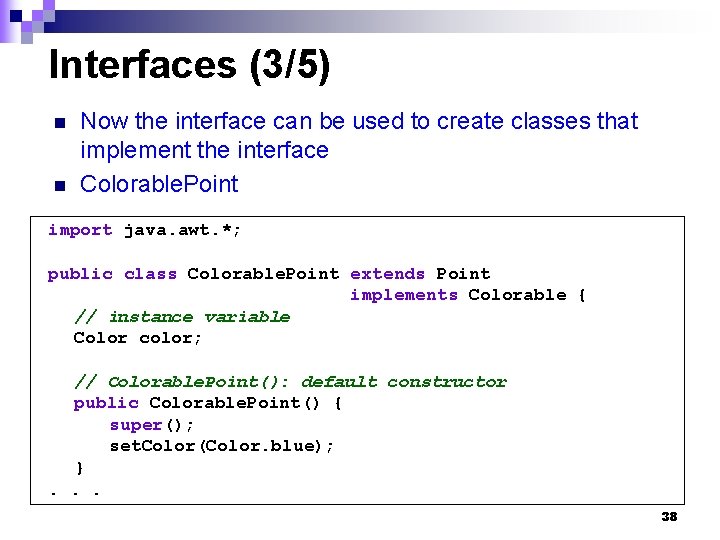
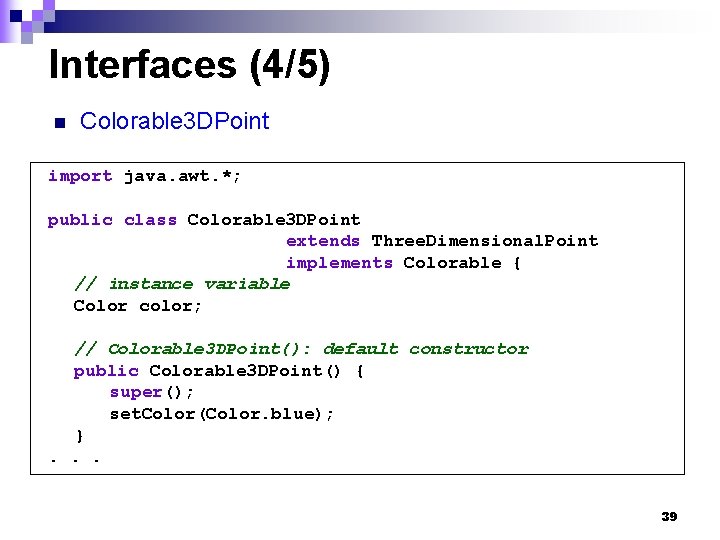
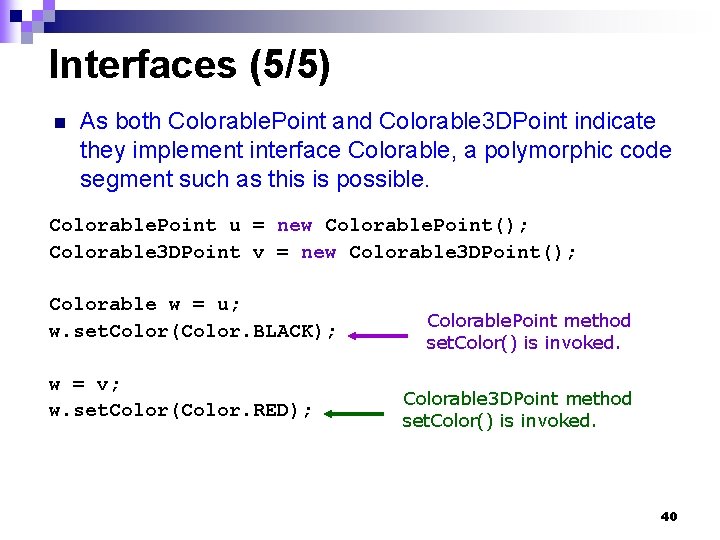
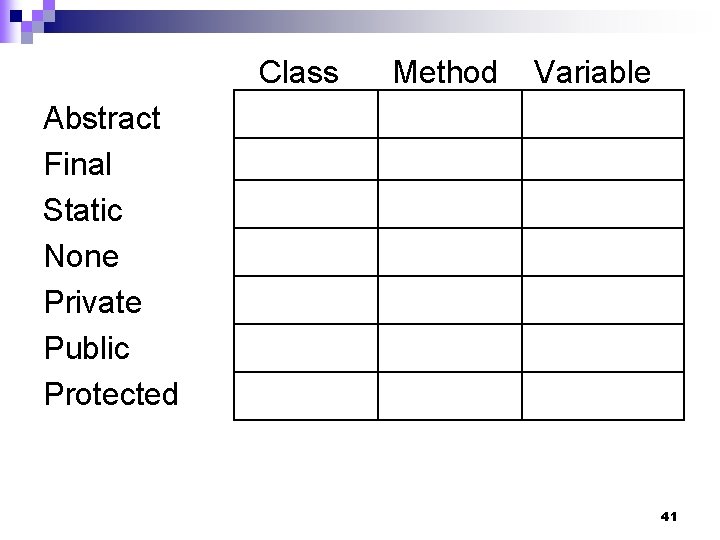
- Slides: 41
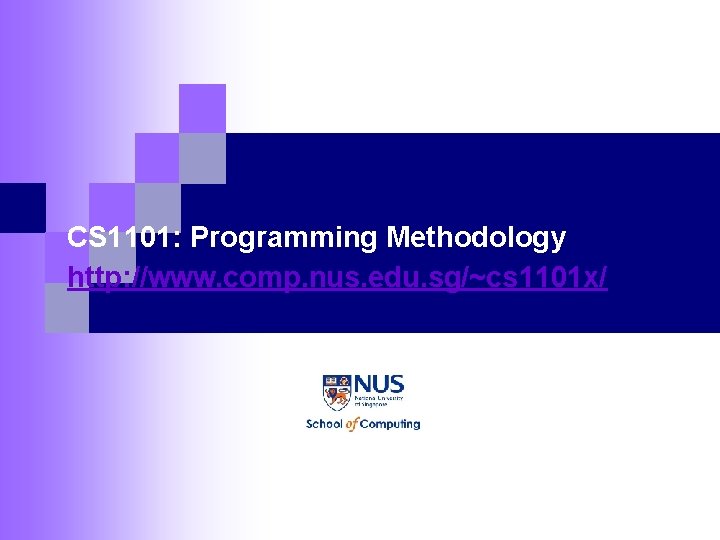
CS 1101: Programming Methodology http: //www. comp. nus. edu. sg/~cs 1101 x/
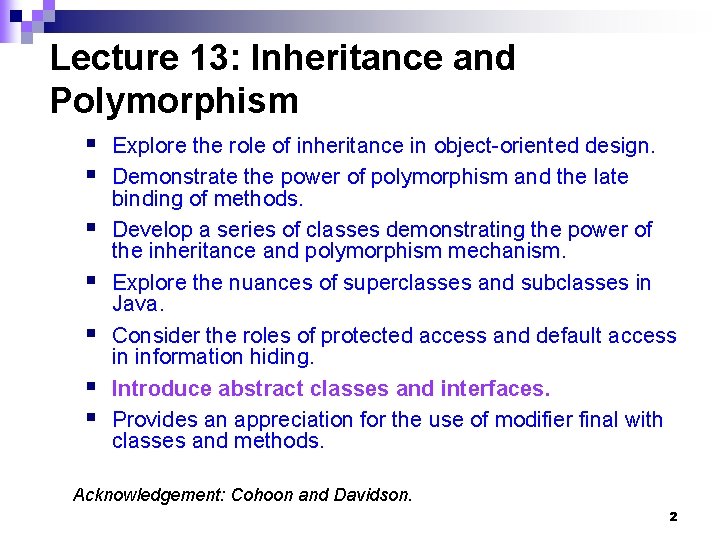
Lecture 13: Inheritance and Polymorphism § Explore the role of inheritance in object-oriented design. § Demonstrate the power of polymorphism and the late § § § binding of methods. Develop a series of classes demonstrating the power of the inheritance and polymorphism mechanism. Explore the nuances of superclasses and subclasses in Java. Consider the roles of protected access and default access in information hiding. Introduce abstract classes and interfaces. Provides an appreciation for the use of modifier final with classes and methods. Acknowledgement: Cohoon and Davidson. 2
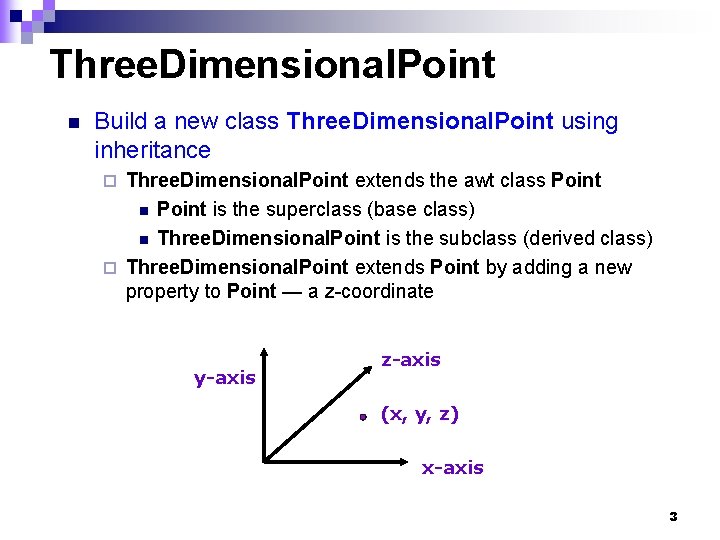
Three. Dimensional. Point n Build a new class Three. Dimensional. Point using inheritance Three. Dimensional. Point extends the awt class Point n Point is the superclass (base class) n Three. Dimensional. Point is the subclass (derived class) ¨ Three. Dimensional. Point extends Point by adding a new property to Point — a z-coordinate ¨ y-axis z-axis (x, y, z) x-axis 3
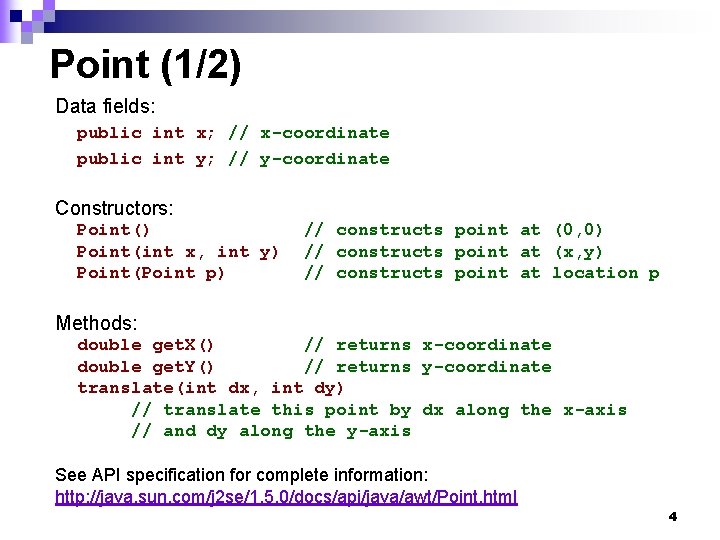
Point (1/2) Data fields: public int x; // x-coordinate public int y; // y-coordinate Constructors: Point() // constructs point at (0, 0) Point(int x, int y) // constructs point at (x, y) Point(Point p) // constructs point at location p Methods: double get. X() // returns x-coordinate double get. Y() // returns y-coordinate translate(int dx, int dy) // translate this point by dx along the x-axis // and dy along the y-axis See API specification for complete information: http: //java. sun. com/j 2 se/1. 5. 0/docs/api/java/awt/Point. html 4
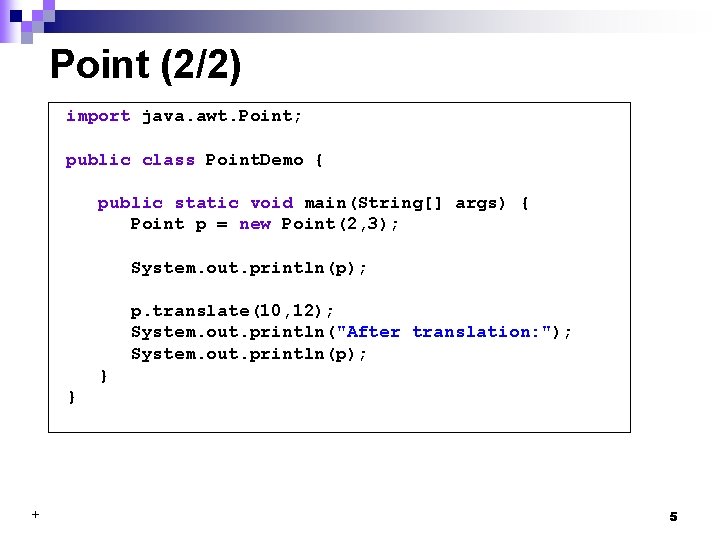
Point (2/2) import java. awt. Point; public class Point. Demo { public static void main(String[] args) { Point p = new Point(2, 3); System. out. println(p); p. translate(10, 12); System. out. println("After translation: "); System. out. println(p); } } + 5
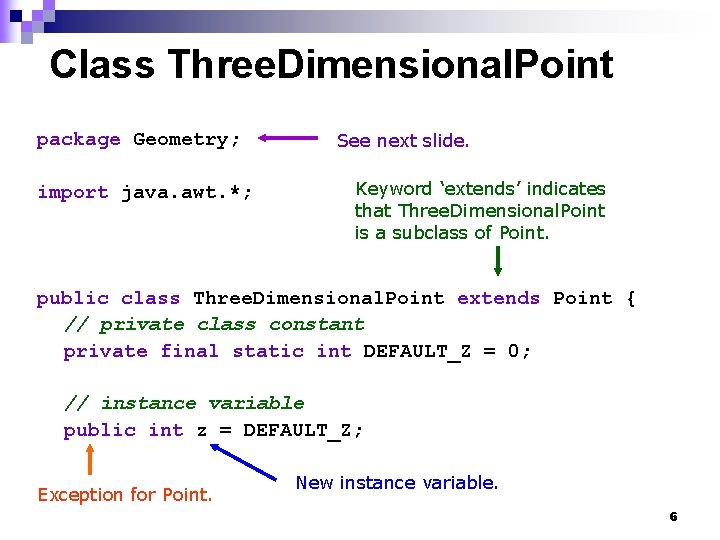
Class Three. Dimensional. Point package Geometry; import java. awt. *; See next slide. Keyword ‘extends’ indicates that Three. Dimensional. Point is a subclass of Point. public class Three. Dimensional. Point extends Point { // private class constant private final static int DEFAULT_Z = 0; // instance variable public int z = DEFAULT_Z; Exception for Point. New instance variable. 6
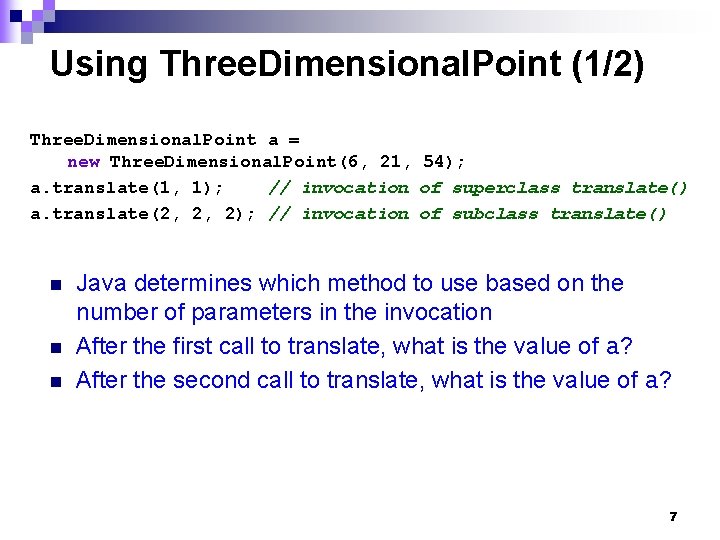
Using Three. Dimensional. Point (1/2) Three. Dimensional. Point a = new Three. Dimensional. Point(6, 21, 54); a. translate(1, 1); // invocation of superclass translate() a. translate(2, 2, 2); // invocation of subclass translate() n n n Java determines which method to use based on the number of parameters in the invocation After the first call to translate, what is the value of a? After the second call to translate, what is the value of a? 7
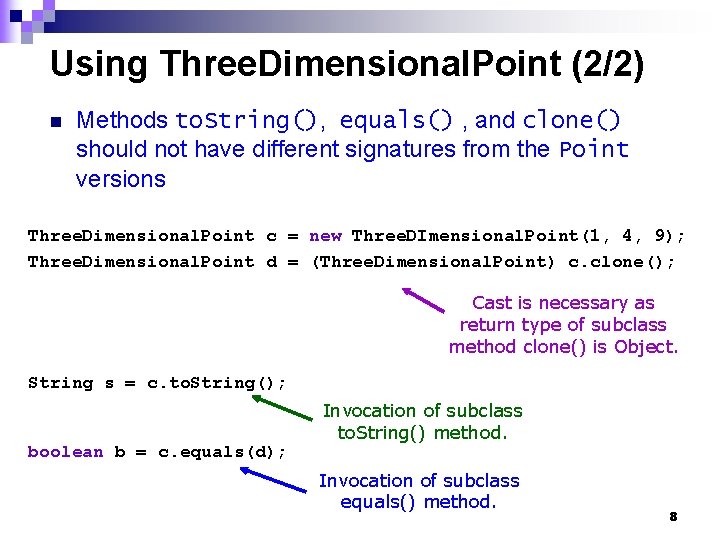
Using Three. Dimensional. Point (2/2) n Methods to. String(), equals() , and clone() should not have different signatures from the Point versions Three. Dimensional. Point c = new Three. DImensional. Point(1, 4, 9); Three. Dimensional. Point d = (Three. Dimensional. Point) c. clone(); Cast is necessary as return type of subclass method clone() is Object. String s = c. to. String(); boolean b = c. equals(d); Invocation of subclass to. String() method. Invocation of subclass equals() method. 8
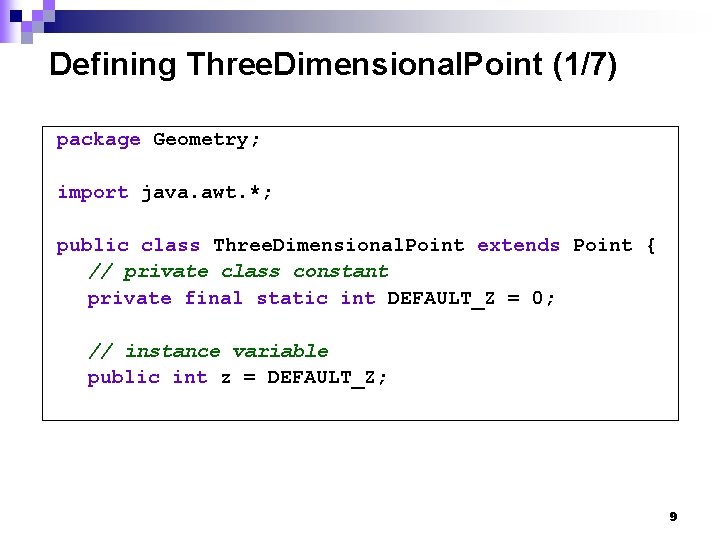
Defining Three. Dimensional. Point (1/7) package Geometry; import java. awt. *; public class Three. Dimensional. Point extends Point { // private class constant private final static int DEFAULT_Z = 0; // instance variable public int z = DEFAULT_Z; 9
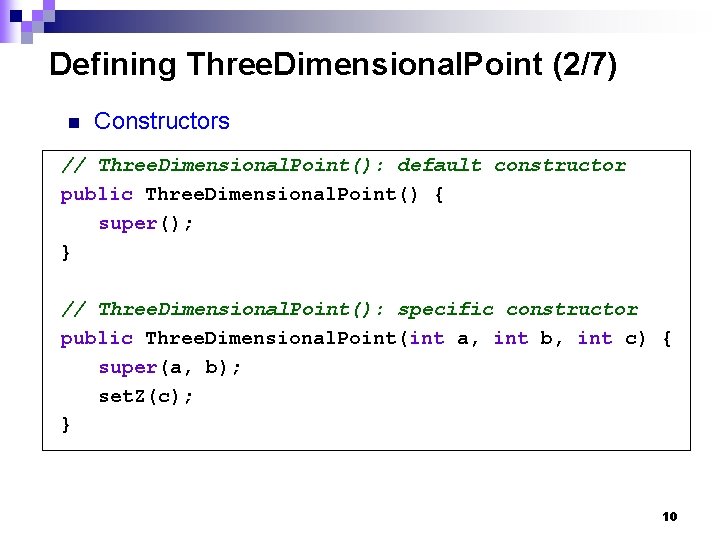
Defining Three. Dimensional. Point (2/7) n Constructors // Three. Dimensional. Point(): default constructor public Three. Dimensional. Point() { super(); } // Three. Dimensional. Point(): specific constructor public Three. Dimensional. Point(int a, int b, int c) { super(a, b); set. Z(c); } 10
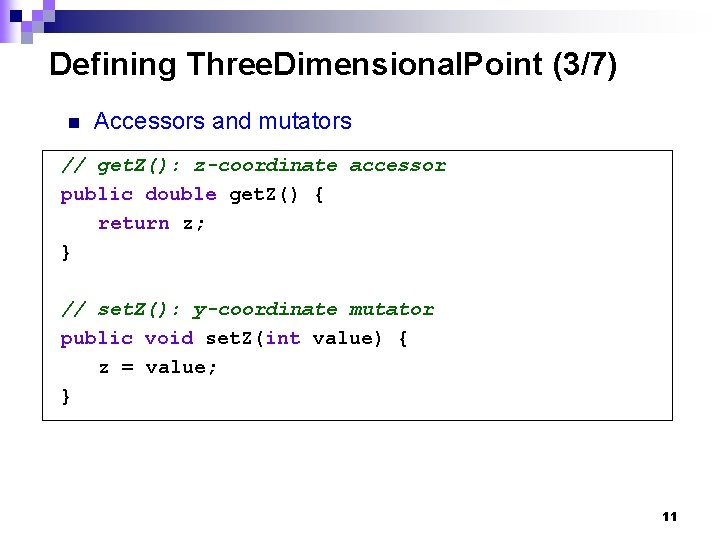
Defining Three. Dimensional. Point (3/7) n Accessors and mutators // get. Z(): z-coordinate accessor public double get. Z() { return z; } // set. Z(): y-coordinate mutator public void set. Z(int value) { z = value; } 11
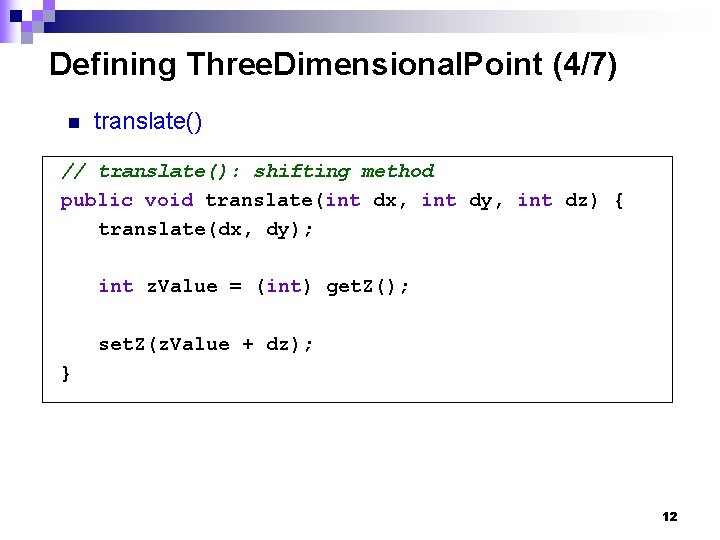
Defining Three. Dimensional. Point (4/7) n translate() // translate(): shifting method public void translate(int dx, int dy, int dz) { translate(dx, dy); int z. Value = (int) get. Z(); set. Z(z. Value + dz); } 12
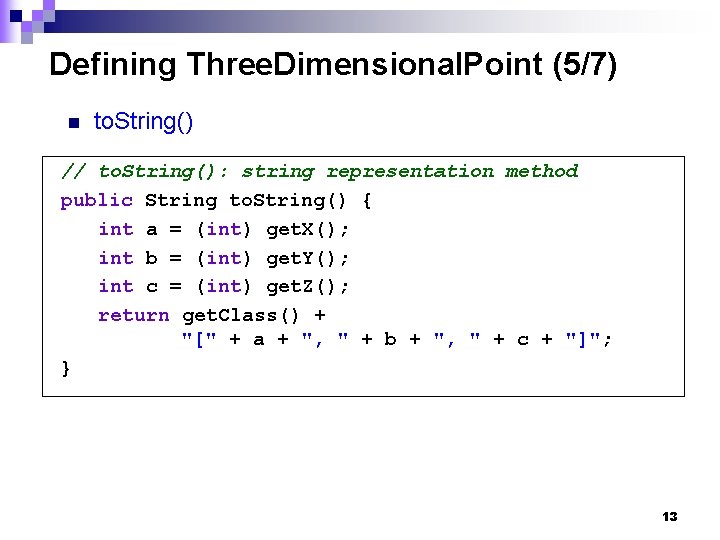
Defining Three. Dimensional. Point (5/7) n to. String() // to. String(): string representation method public String to. String() { int a = (int) get. X(); int b = (int) get. Y(); int c = (int) get. Z(); return get. Class() + "[" + a + ", " + b + ", " + c + "]"; } 13
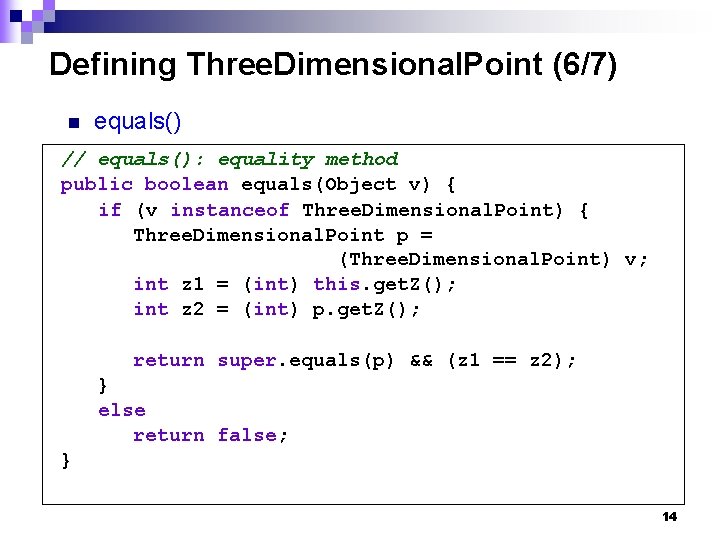
Defining Three. Dimensional. Point (6/7) n equals() // equals(): equality method public boolean equals(Object v) { if (v instanceof Three. Dimensional. Point) { Three. Dimensional. Point p = (Three. Dimensional. Point) v; int z 1 = (int) this. get. Z(); int z 2 = (int) p. get. Z(); return super. equals(p) && (z 1 == z 2); } else return false; } 14
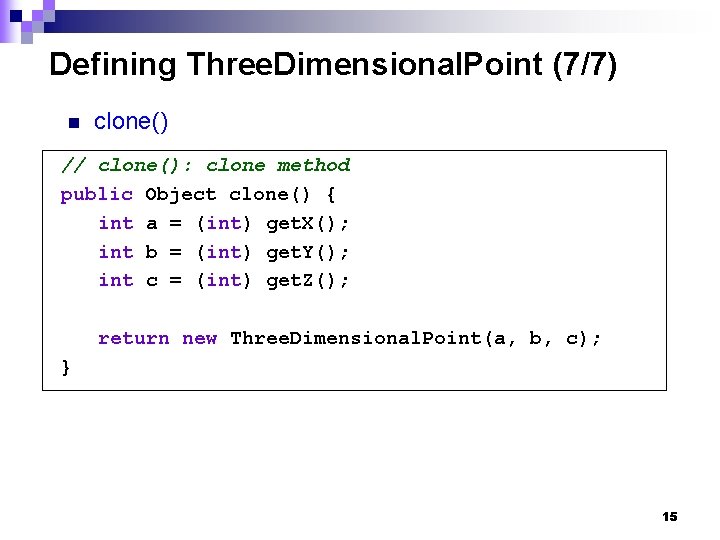
Defining Three. Dimensional. Point (7/7) n clone() // clone(): clone method public Object clone() { int a = (int) get. X(); int b = (int) get. Y(); int c = (int) get. Z(); return new Three. Dimensional. Point(a, b, c); } 15
![Three Dimensional Point Demo public static void mainString args Three Dimensonal Point p Three. Dimensional. Point Demo public static void main(String[] args) { Three. Dimensonal. Point p](https://slidetodoc.com/presentation_image_h/0ed93bb942b82f663a28fcb2906e0e20/image-16.jpg)
Three. Dimensional. Point Demo public static void main(String[] args) { Three. Dimensonal. Point p = new Three. Dimensional. Point(2, 3, 8); System. out. println(p); p. translate(1, 2, 3); System. out. println("After translation: "); System. out. println(p); } + 16
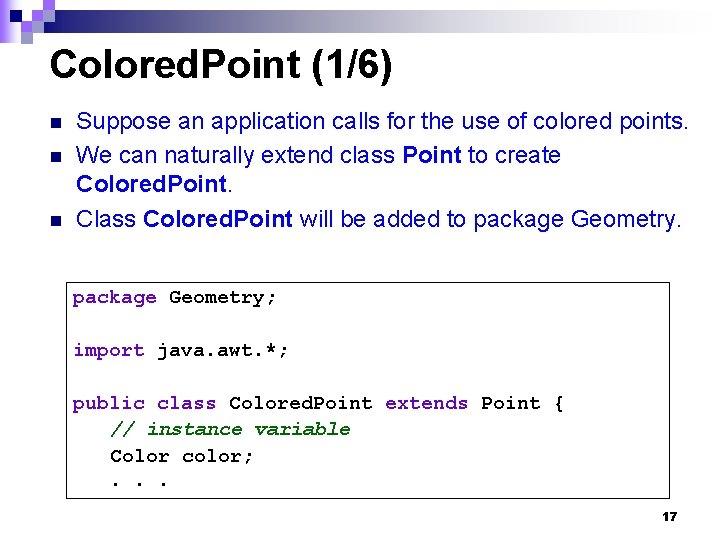
Colored. Point (1/6) n n n Suppose an application calls for the use of colored points. We can naturally extend class Point to create Colored. Point. Class Colored. Point will be added to package Geometry; import java. awt. *; public class Colored. Point extends Point { // instance variable Color color; . . . 17
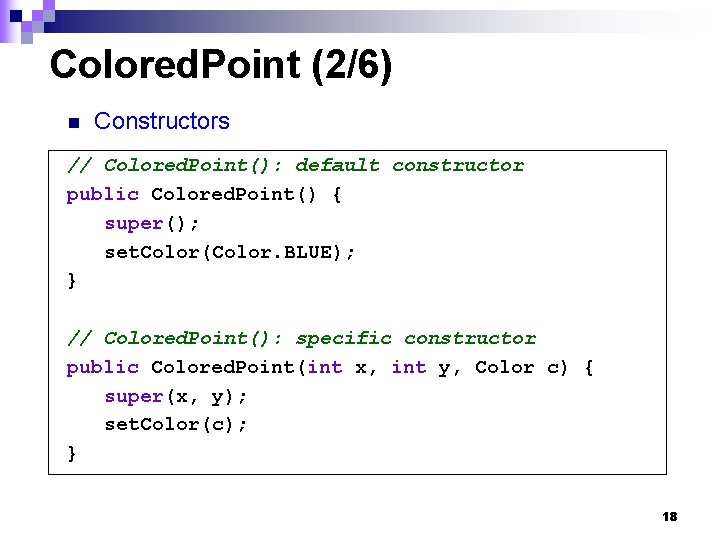
Colored. Point (2/6) n Constructors // Colored. Point(): default constructor public Colored. Point() { super(); set. Color(Color. BLUE); } // Colored. Point(): specific constructor public Colored. Point(int x, int y, Color c) { super(x, y); set. Color(c); } 18
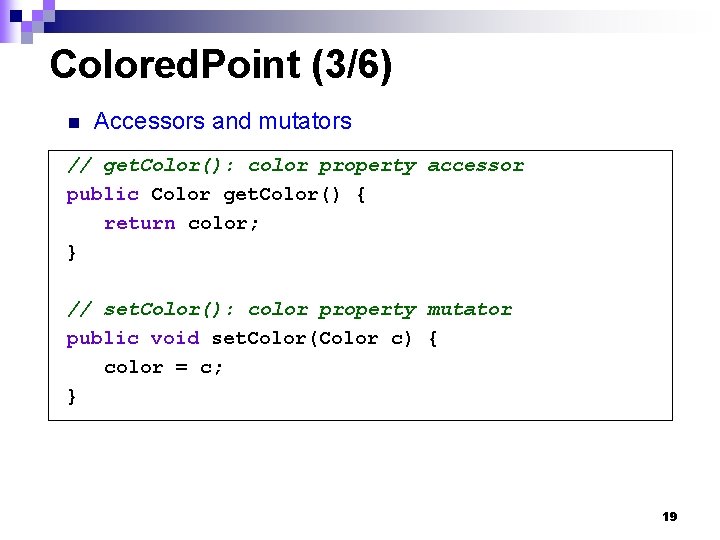
Colored. Point (3/6) n Accessors and mutators // get. Color(): color property accessor public Color get. Color() { return color; } // set. Color(): color property mutator public void set. Color(Color c) { color = c; } 19
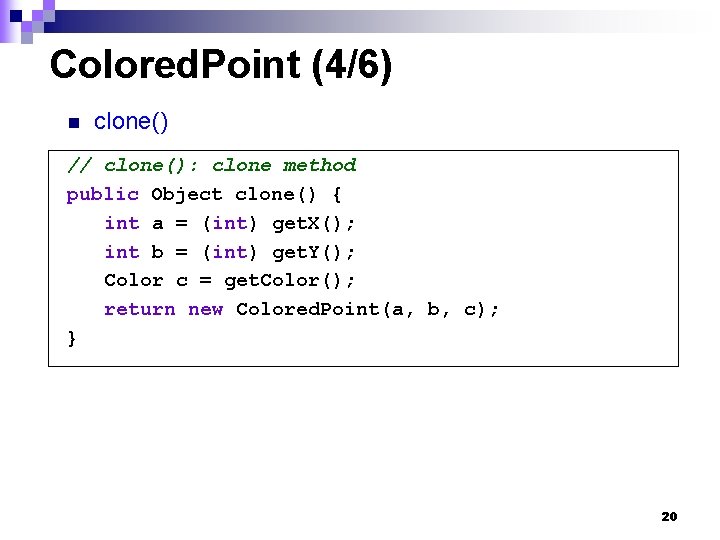
Colored. Point (4/6) n clone() // clone(): clone method public Object clone() { int a = (int) get. X(); int b = (int) get. Y(); Color c = get. Color(); return new Colored. Point(a, b, c); } 20
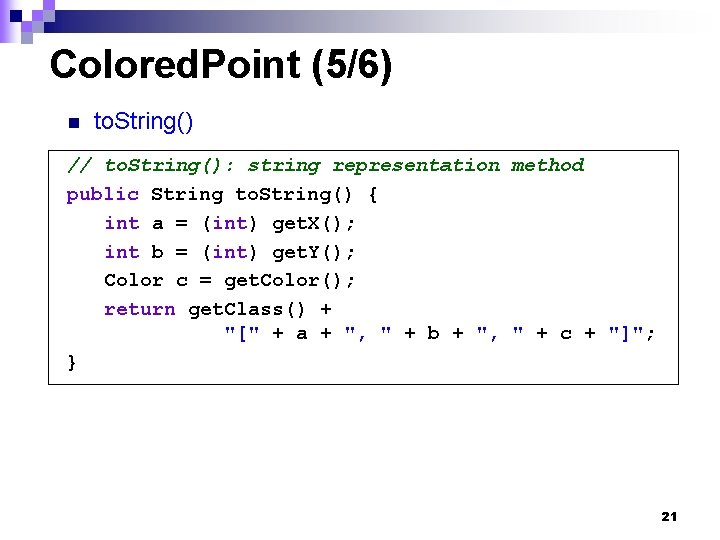
Colored. Point (5/6) n to. String() // to. String(): string representation method public String to. String() { int a = (int) get. X(); int b = (int) get. Y(); Color c = get. Color(); return get. Class() + "[" + a + ", " + b + ", " + c + "]"; } 21
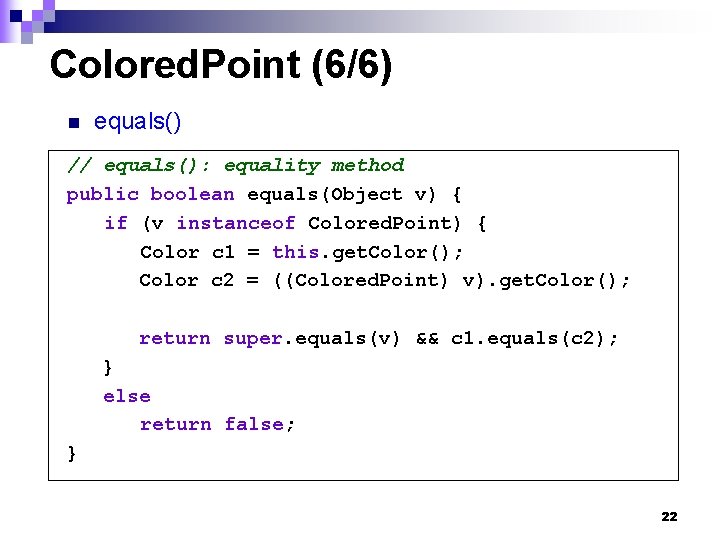
Colored. Point (6/6) n equals() // equals(): equality method public boolean equals(Object v) { if (v instanceof Colored. Point) { Color c 1 = this. get. Color(); Color c 2 = ((Colored. Point) v). get. Color(); return super. equals(v) && c 1. equals(c 2); } else return false; } 22
![Colored Point Demo public static void mainString args Colored Point p new Colored. Point Demo public static void main(String[] args) { Colored. Point p = new](https://slidetodoc.com/presentation_image_h/0ed93bb942b82f663a28fcb2906e0e20/image-23.jpg)
Colored. Point Demo public static void main(String[] args) { Colored. Point p = new Colored. Point(6, 2, Color. RED); System. out. println(p); p. translate(3, 1); p. set. Color(Color. GREEN); System. out. println("Updated point: "); System. out. println(p); } + 23
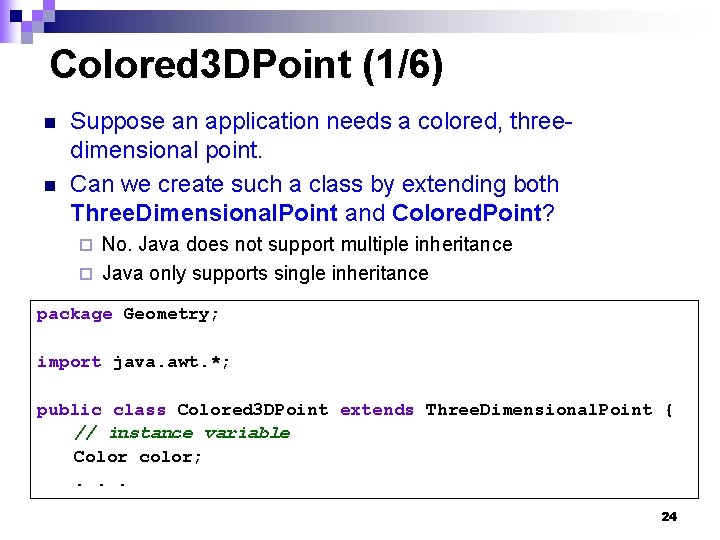
Colored 3 DPoint (1/6) n n Suppose an application needs a colored, threedimensional point. Can we create such a class by extending both Three. Dimensional. Point and Colored. Point? No. Java does not support multiple inheritance ¨ Java only supports single inheritance ¨ package Geometry; import java. awt. *; public class Colored 3 DPoint extends Three. Dimensional. Point { // instance variable Color color; . . . 24
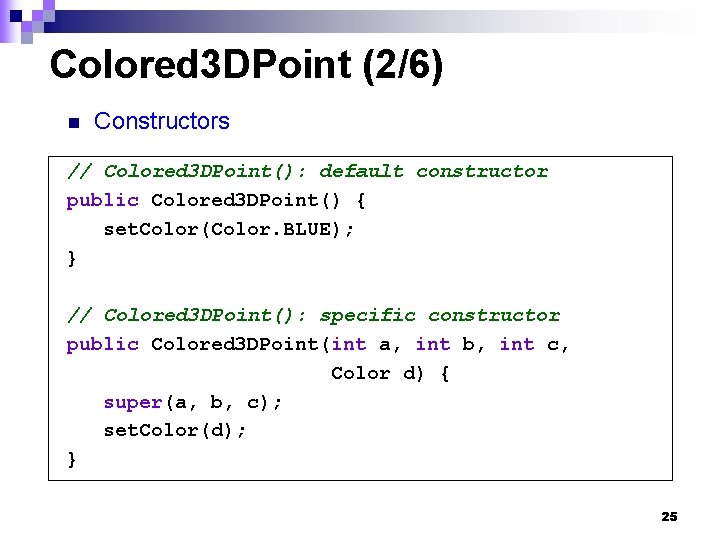
Colored 3 DPoint (2/6) n Constructors // Colored 3 DPoint(): default constructor public Colored 3 DPoint() { set. Color(Color. BLUE); } // Colored 3 DPoint(): specific constructor public Colored 3 DPoint(int a, int b, int c, Color d) { super(a, b, c); set. Color(d); } 25
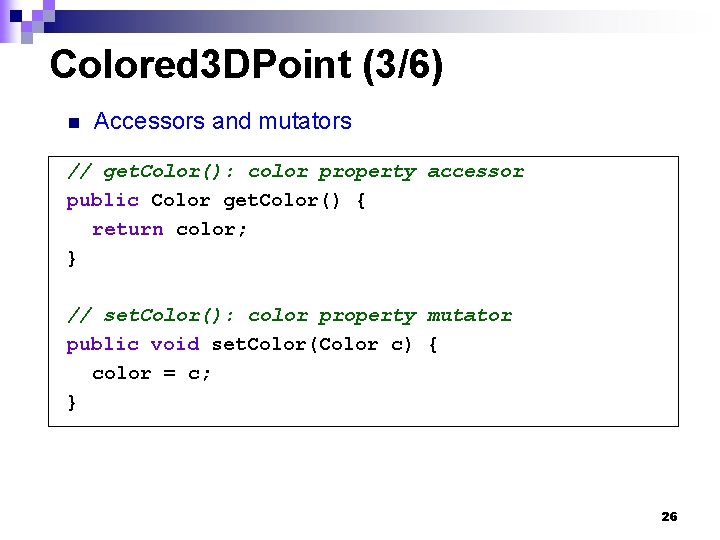
Colored 3 DPoint (3/6) n Accessors and mutators // get. Color(): color property accessor public Color get. Color() { return color; } // set. Color(): color property mutator public void set. Color(Color c) { color = c; } 26
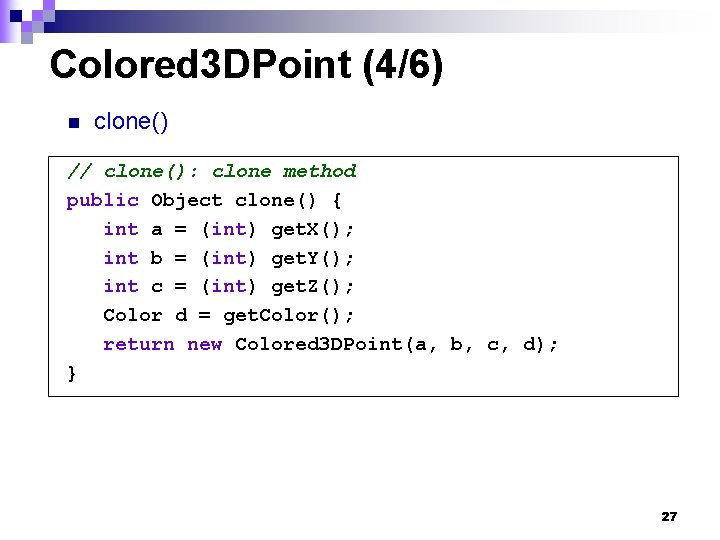
Colored 3 DPoint (4/6) n clone() // clone(): clone method public Object clone() { int a = (int) get. X(); int b = (int) get. Y(); int c = (int) get. Z(); Color d = get. Color(); return new Colored 3 DPoint(a, b, c, d); } 27
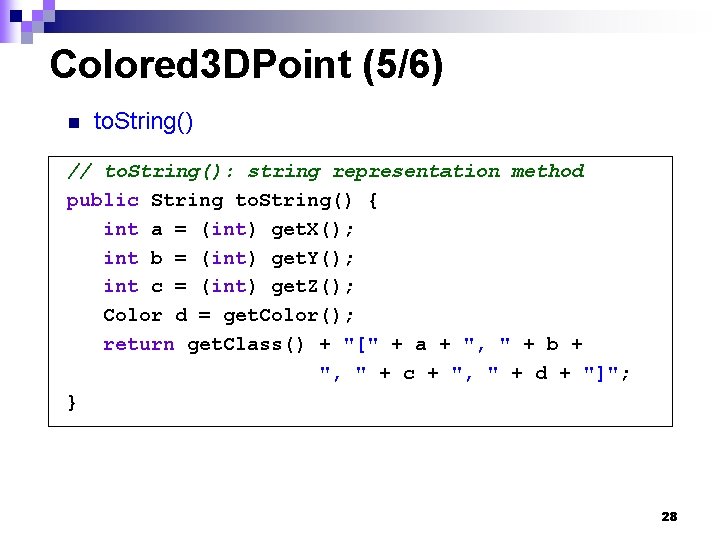
Colored 3 DPoint (5/6) n to. String() // to. String(): string representation method public String to. String() { int a = (int) get. X(); int b = (int) get. Y(); int c = (int) get. Z(); Color d = get. Color(); return get. Class() + "[" + a + ", " + b + ", " + c + ", " + d + "]"; } 28
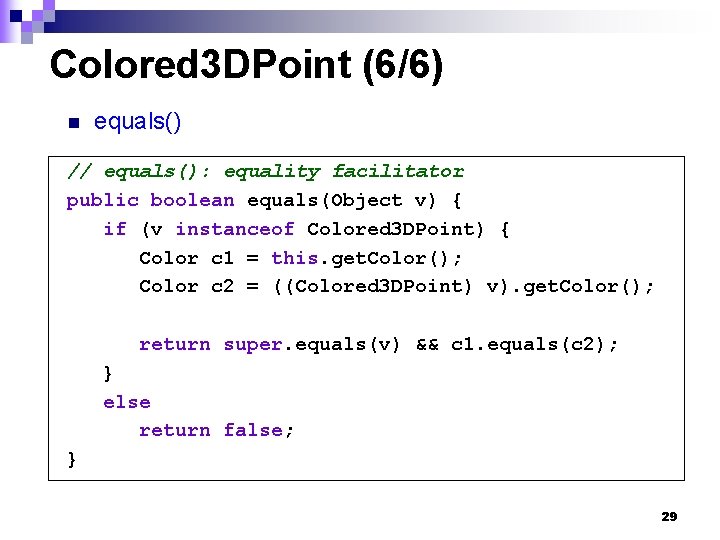
Colored 3 DPoint (6/6) n equals() // equals(): equality facilitator public boolean equals(Object v) { if (v instanceof Colored 3 DPoint) { Color c 1 = this. get. Color(); Color c 2 = ((Colored 3 DPoint) v). get. Color(); return super. equals(v) && c 1. equals(c 2); } else return false; } 29
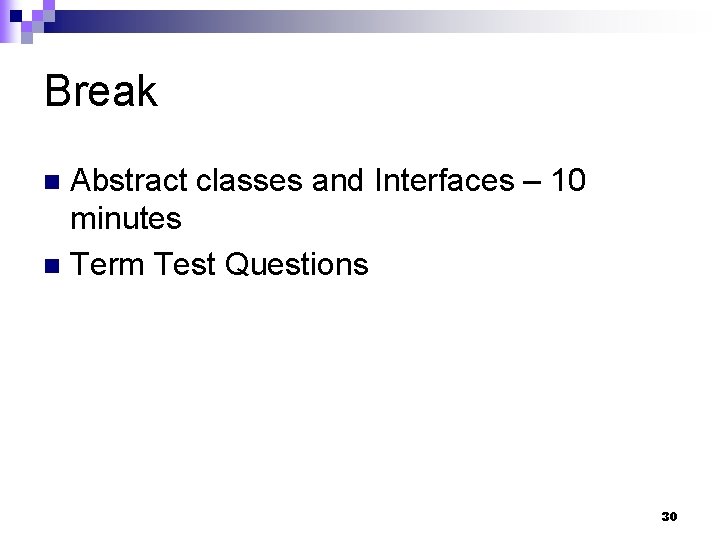
Break Abstract classes and Interfaces – 10 minutes n Term Test Questions n 30
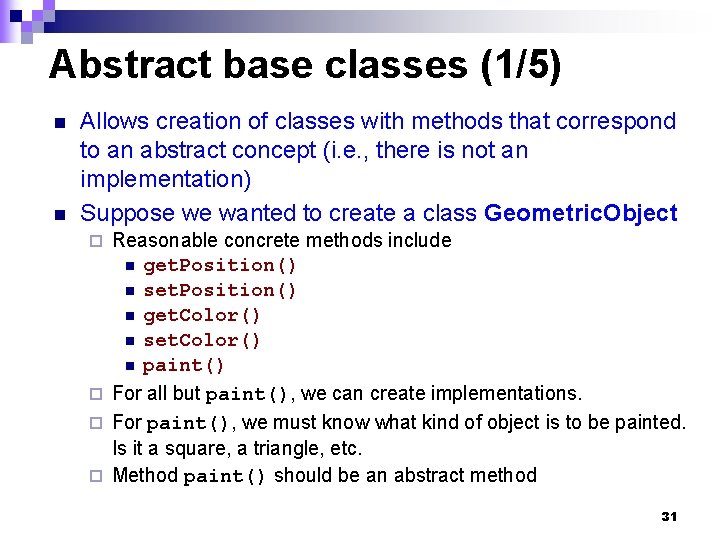
Abstract base classes (1/5) n n Allows creation of classes with methods that correspond to an abstract concept (i. e. , there is not an implementation) Suppose we wanted to create a class Geometric. Object Reasonable concrete methods include n get. Position() n set. Position() n get. Color() n set. Color() n paint() ¨ For all but paint(), we can create implementations. ¨ For paint(), we must know what kind of object is to be painted. Is it a square, a triangle, etc. ¨ Method paint() should be an abstract method ¨ 31
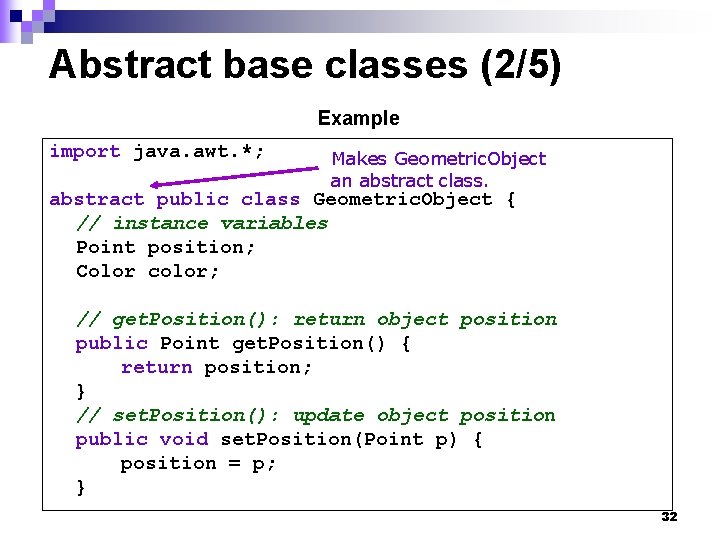
Abstract base classes (2/5) Example import java. awt. *; Makes Geometric. Object an abstract class. abstract public class Geometric. Object { // instance variables Point position; Color color; // get. Position(): return object position public Point get. Position() { return position; } // set. Position(): update object position public void set. Position(Point p) { position = p; } 32
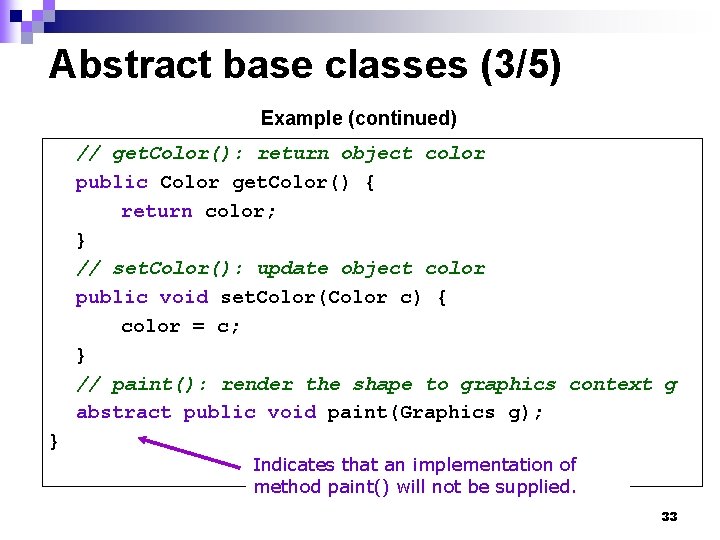
Abstract base classes (3/5) Example (continued) // get. Color(): return object color public Color get. Color() { return color; } // set. Color(): update object color public void set. Color(Color c) { color = c; } // paint(): render the shape to graphics context g abstract public void paint(Graphics g); } Indicates that an implementation of method paint() will not be supplied. 33
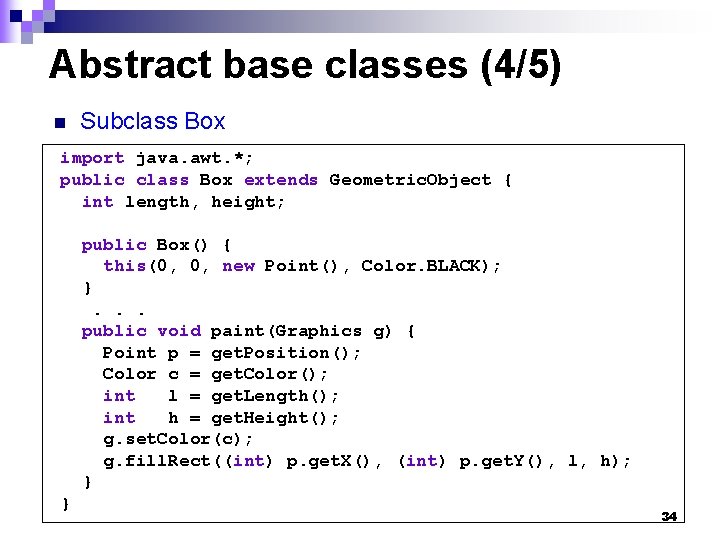
Abstract base classes (4/5) n Subclass Box import java. awt. *; public class Box extends Geometric. Object { int length, height; public Box() { this(0, 0, new Point(), Color. BLACK); } . . . public void paint(Graphics g) { Point p = get. Position(); Color c = get. Color(); int l = get. Length(); int h = get. Height(); g. set. Color(c); g. fill. Rect((int) p. get. X(), (int) p. get. Y(), l, h); } } 34
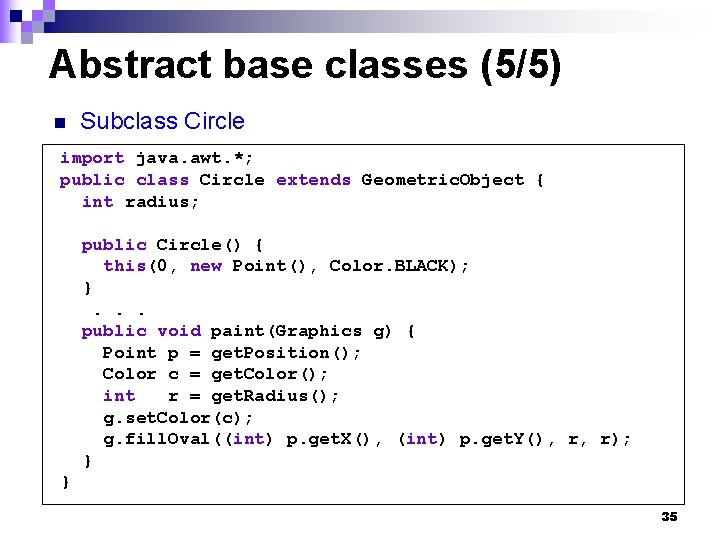
Abstract base classes (5/5) n Subclass Circle import java. awt. *; public class Circle extends Geometric. Object { int radius; public Circle() { this(0, new Point(), Color. BLACK); } . . . public void paint(Graphics g) { Point p = get. Position(); Color c = get. Color(); int r = get. Radius(); g. set. Color(c); g. fill. Oval((int) p. get. X(), (int) p. get. Y(), r, r); } } 35
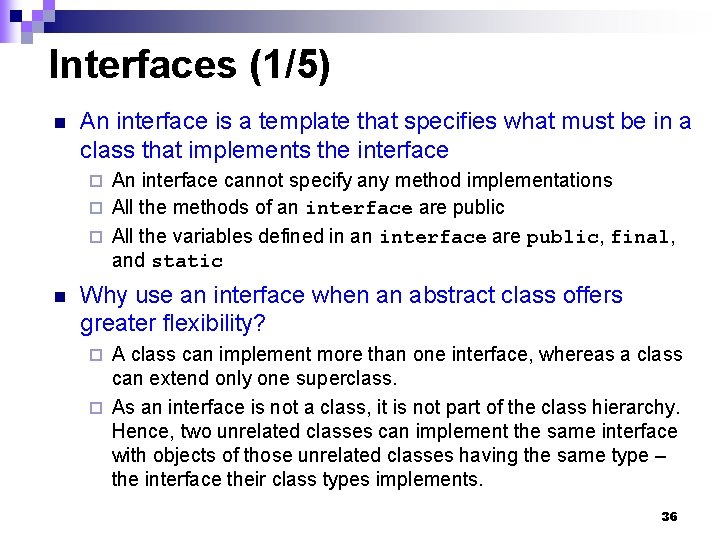
Interfaces (1/5) n An interface is a template that specifies what must be in a class that implements the interface An interface cannot specify any method implementations ¨ All the methods of an interface are public ¨ All the variables defined in an interface are public, final, and static ¨ n Why use an interface when an abstract class offers greater flexibility? A class can implement more than one interface, whereas a class can extend only one superclass. ¨ As an interface is not a class, it is not part of the class hierarchy. Hence, two unrelated classes can implement the same interface with objects of those unrelated classes having the same type – the interface their class types implements. ¨ 36
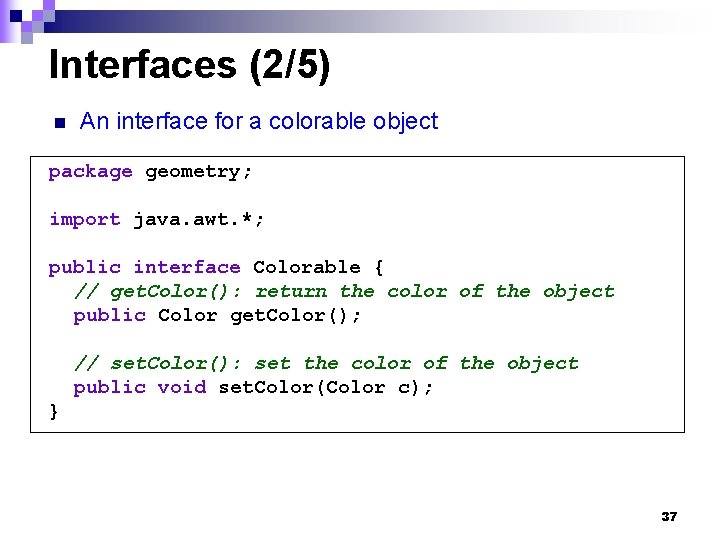
Interfaces (2/5) n An interface for a colorable object package geometry; import java. awt. *; public interface Colorable { // get. Color(): return the color of the object public Color get. Color(); // set. Color(): set the color of the object public void set. Color(Color c); } 37
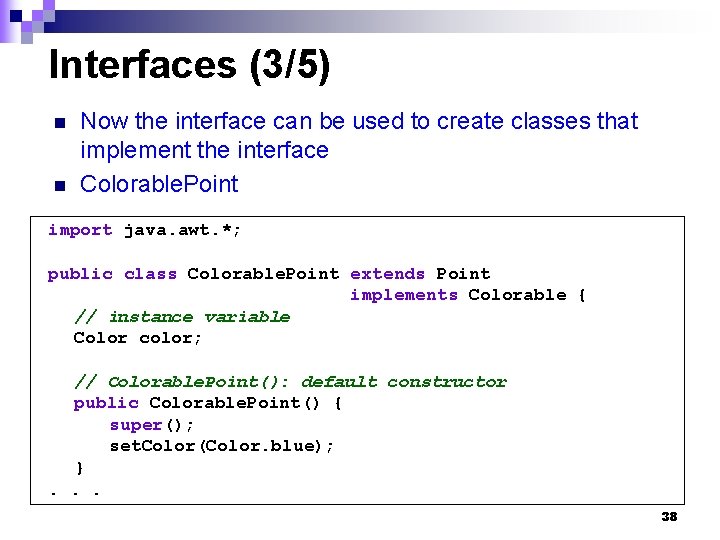
Interfaces (3/5) n n Now the interface can be used to create classes that implement the interface Colorable. Point import java. awt. *; public class Colorable. Point extends Point implements Colorable { // instance variable Color color; // Colorable. Point(): default constructor public Colorable. Point() { super(); set. Color(Color. blue); } . . . 38
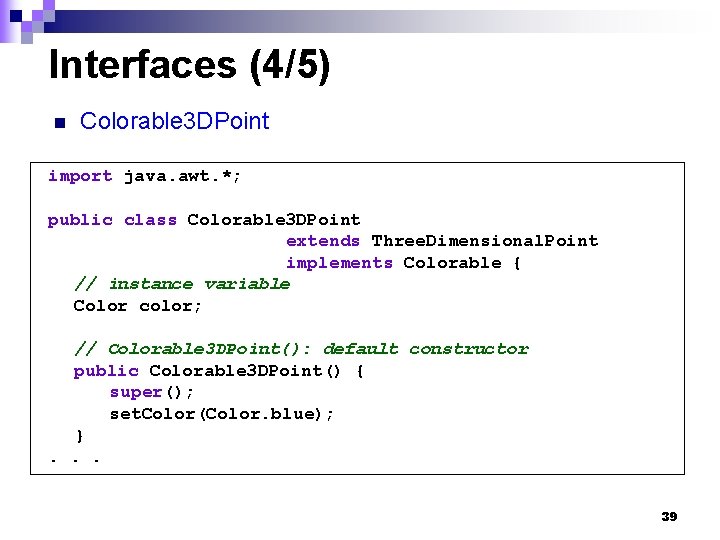
Interfaces (4/5) n Colorable 3 DPoint import java. awt. *; public class Colorable 3 DPoint extends Three. Dimensional. Point implements Colorable { // instance variable Color color; // Colorable 3 DPoint(): default constructor public Colorable 3 DPoint() { super(); set. Color(Color. blue); } . . . 39
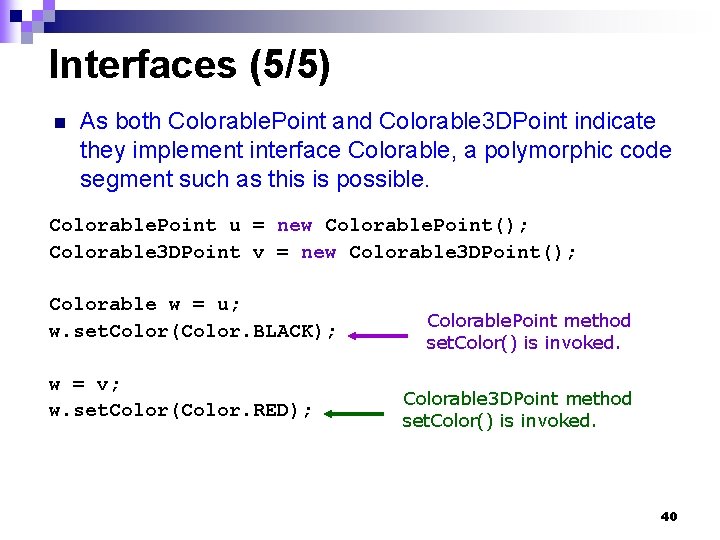
Interfaces (5/5) n As both Colorable. Point and Colorable 3 DPoint indicate they implement interface Colorable, a polymorphic code segment such as this is possible. Colorable. Point u = new Colorable. Point(); Colorable 3 DPoint v = new Colorable 3 DPoint(); Colorable w = u; w. set. Color(Color. BLACK); w = v; w. set. Color(Color. RED); Colorable. Point method set. Color() is invoked. Colorable 3 DPoint method set. Color() is invoked. 40
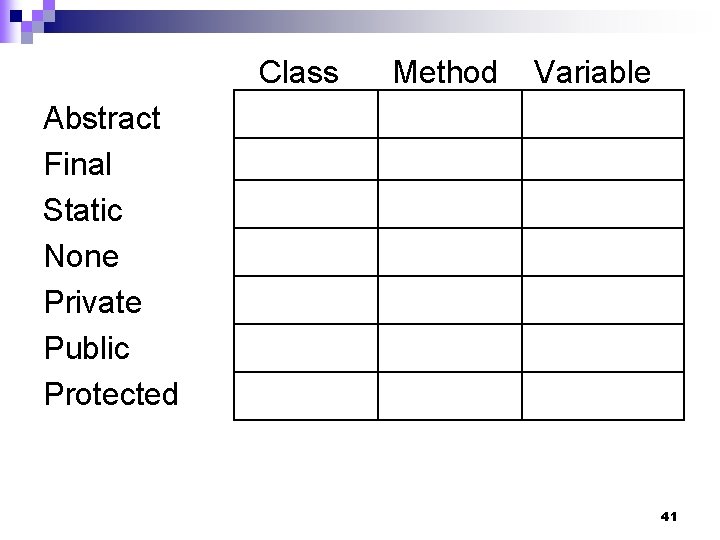
Class Method Variable Abstract Final Static None Private Public Protected 41
Cs 1101 programming fundamentals final exam
Cs 1101 final
Cs 1101 final
Cs 1101 programming fundamentals
Cs 1101 programming fundamentals
Sequence detector 1101
Osha 1926 asbestos
Uga pols 1101
Enc 1101 final exam
Sequence detector 1101
Cst 1101
1101 binary to decimal
Cst 1101
Sls 1101
Pols 1101 final exam
Sistema binario suma
Afi 51-1101
Enc 1101 fiu
Que es sintagma verbal
Cst 1101
Nus cs1101s
Iso 1101:2012
1010 1101
Cs 1101 final
Cs 1101 final
Garland science
Gaylene rogers lonergan
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
What is system programming
Integer programming vs linear programming
Perbedaan linear programming dan integer programming
Sanjay jain nus
Nus proxy
Nus library e resources
Nus soc ffmpeg
Cj koh library
Nus entrepreneurship centre
Central library rbr
Nus cs1010
Nus soc course schedule
Nus high school timetable