CS 1101 Programming Methodology http www comp nus
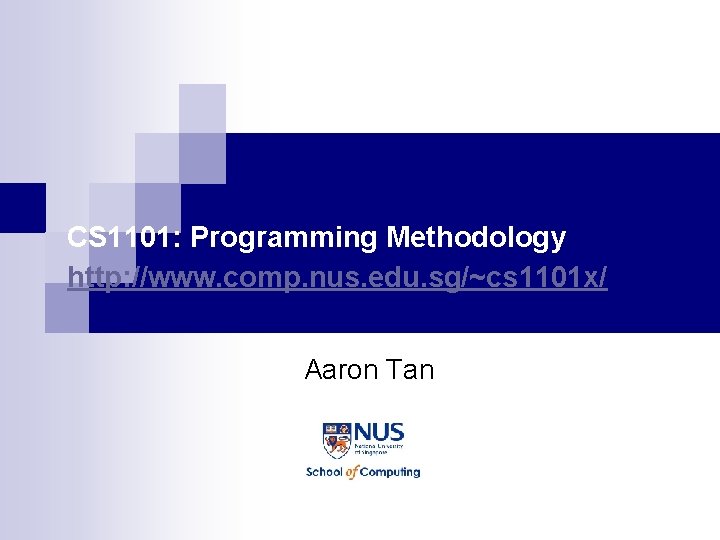
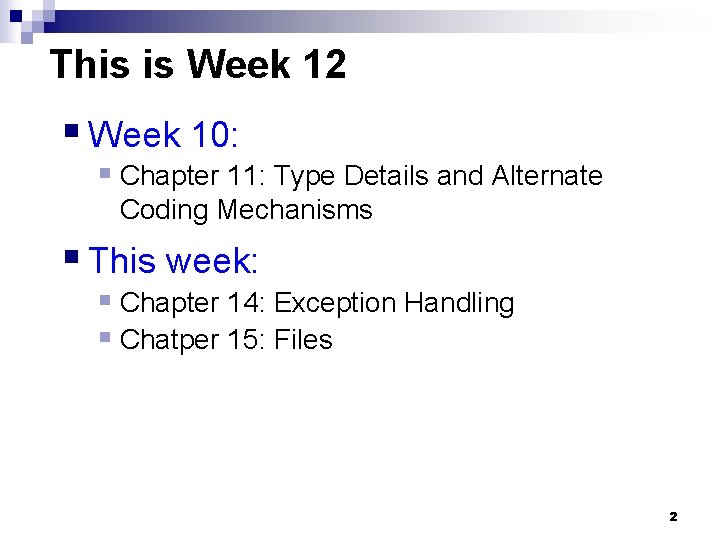
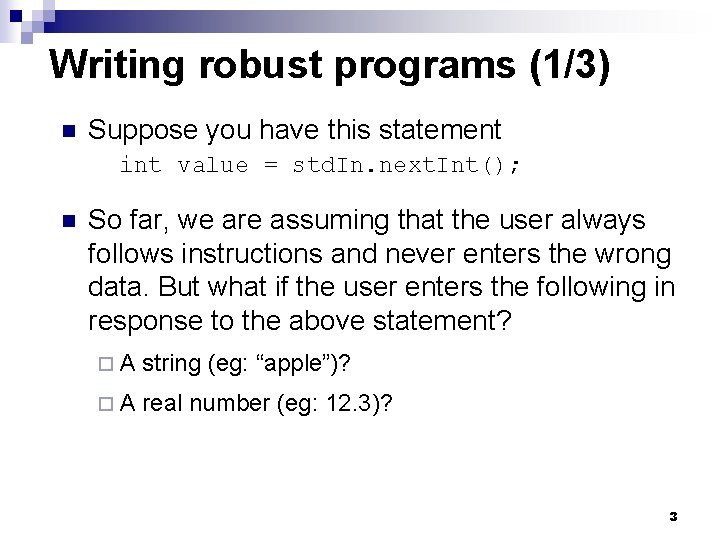
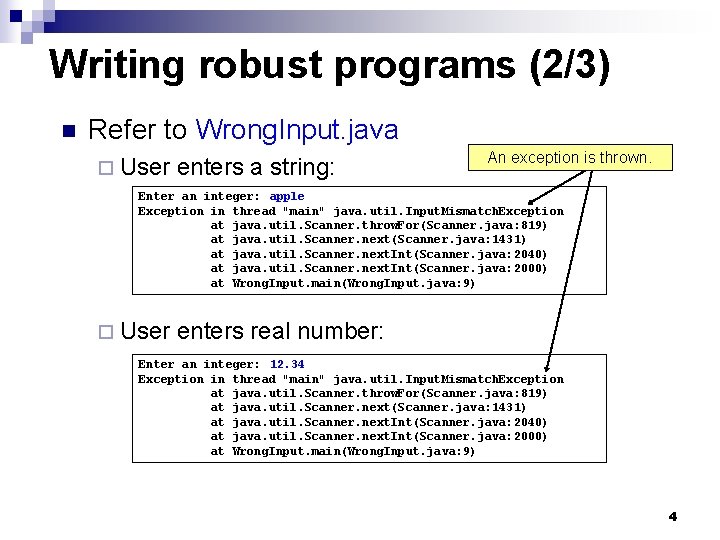
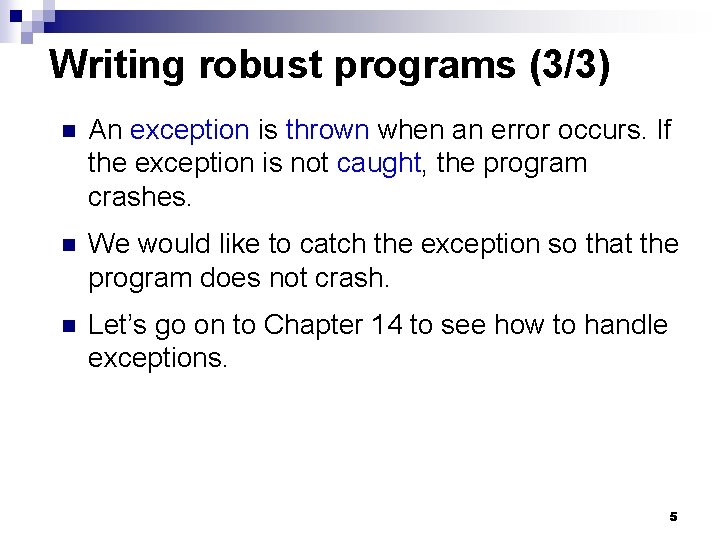
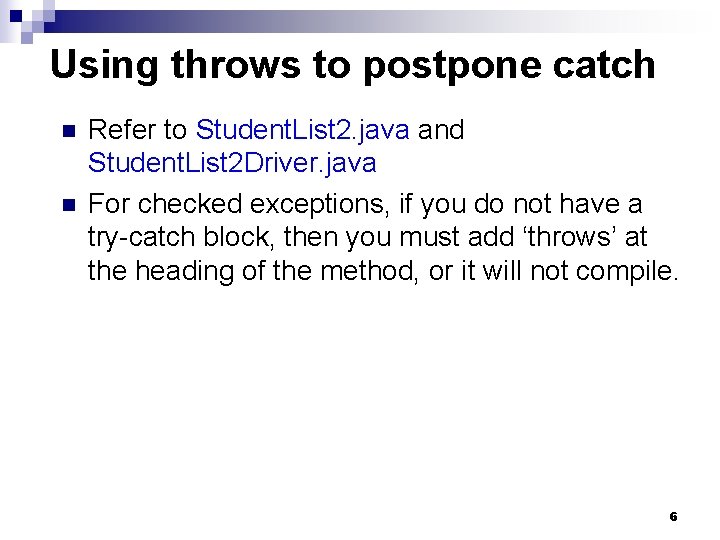
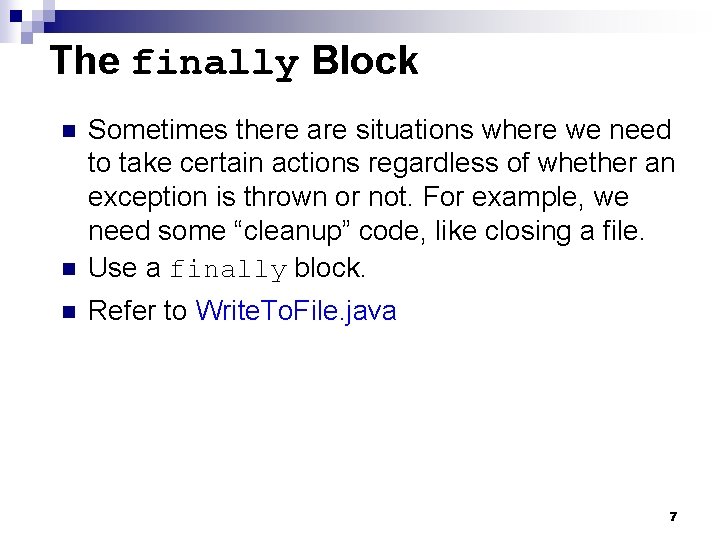
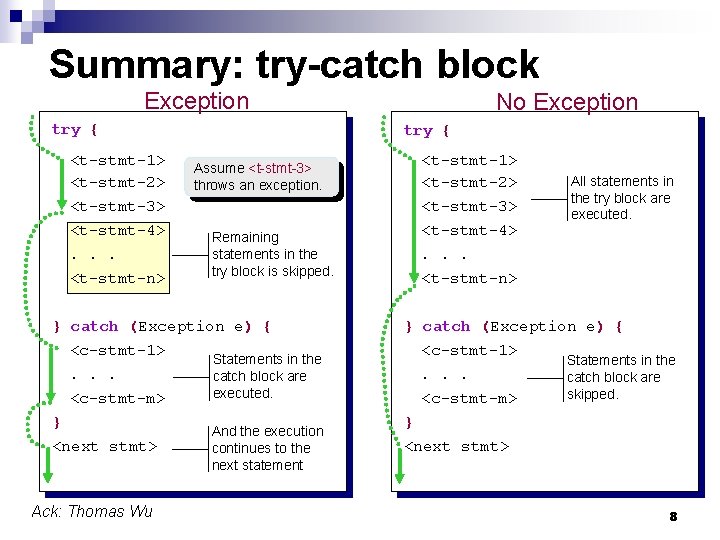
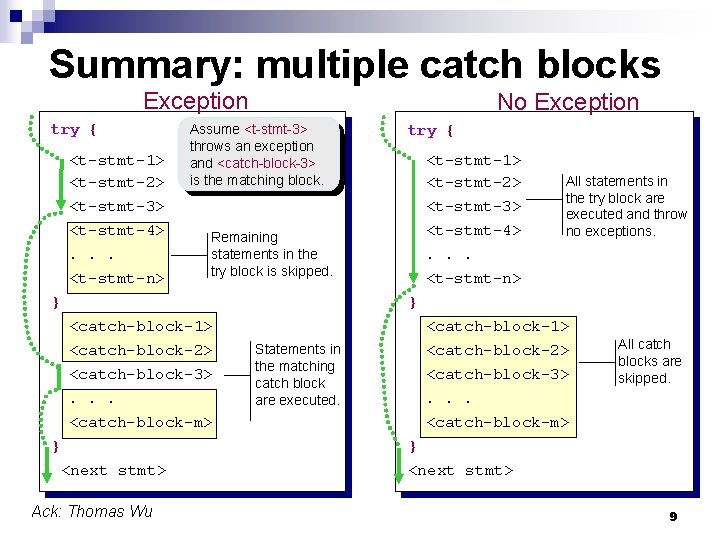
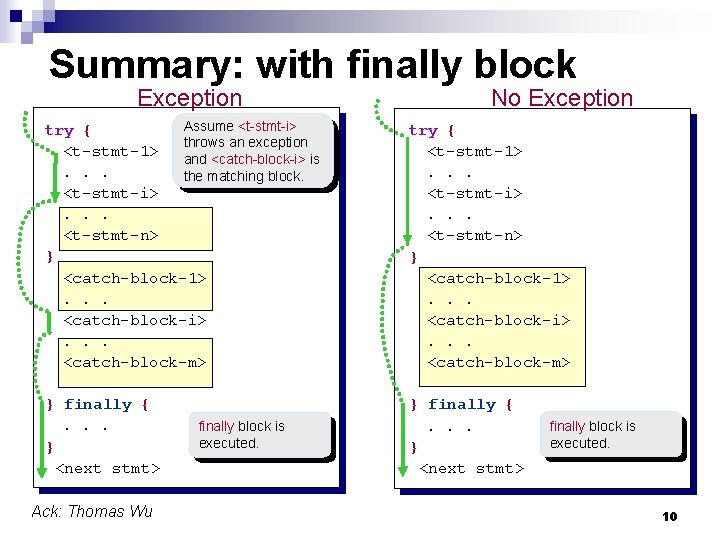
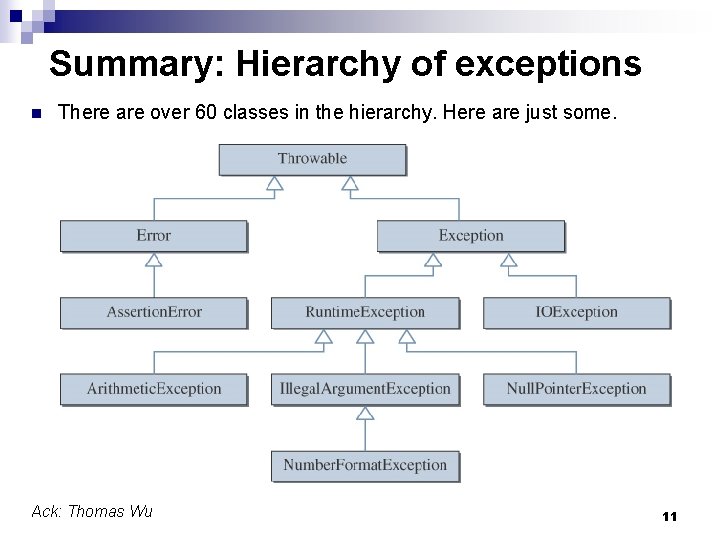
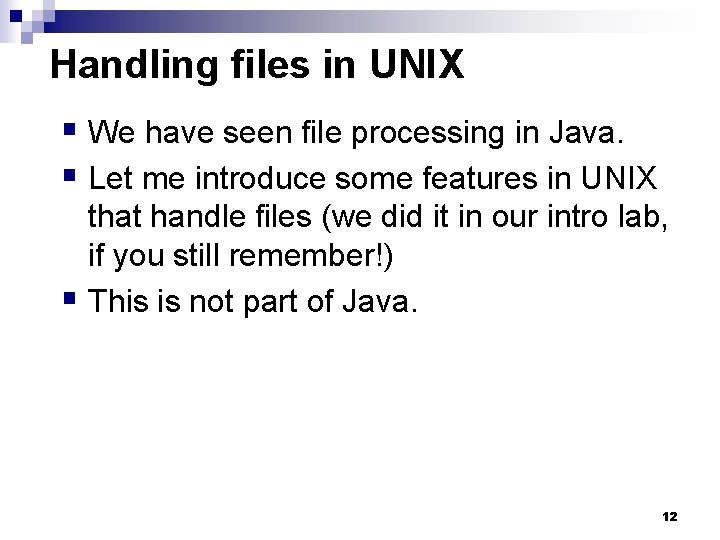
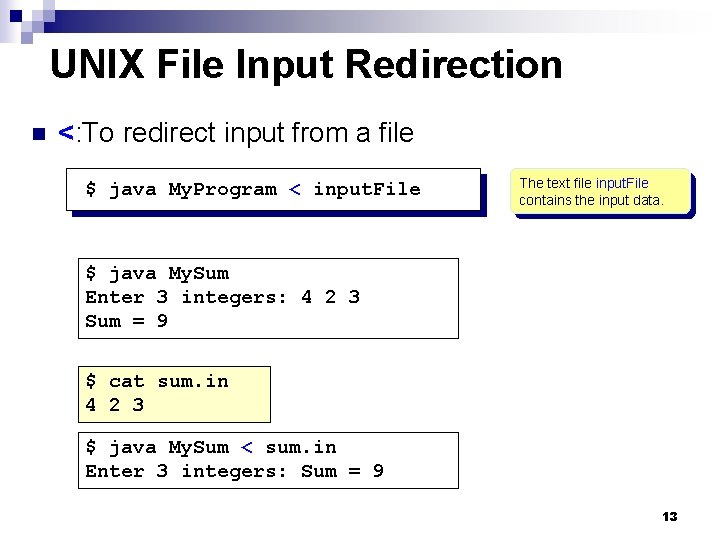
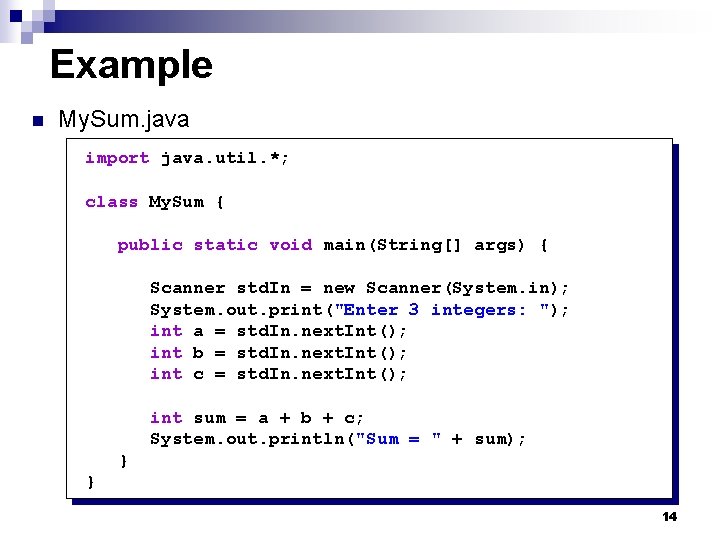
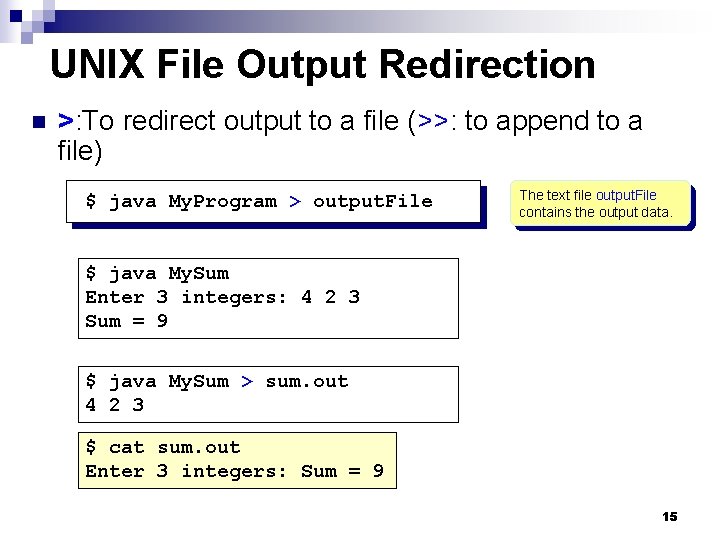
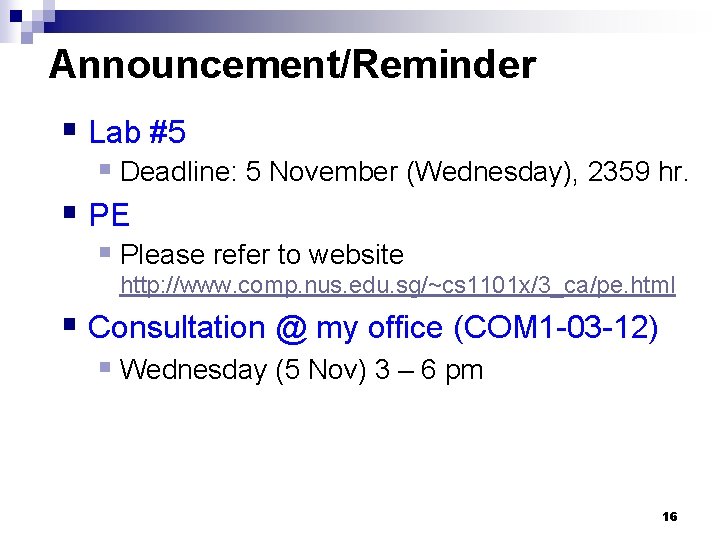
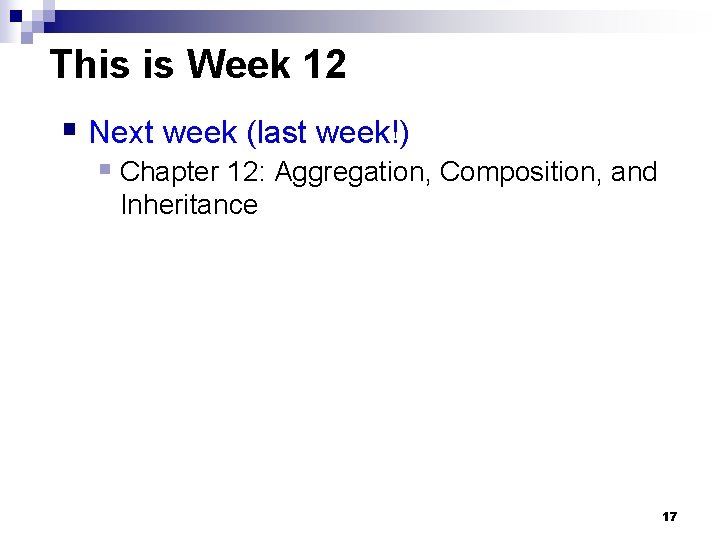
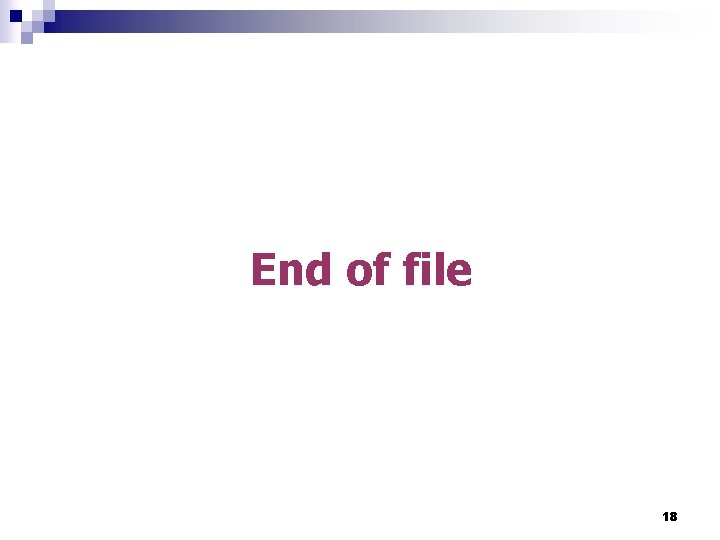
- Slides: 18
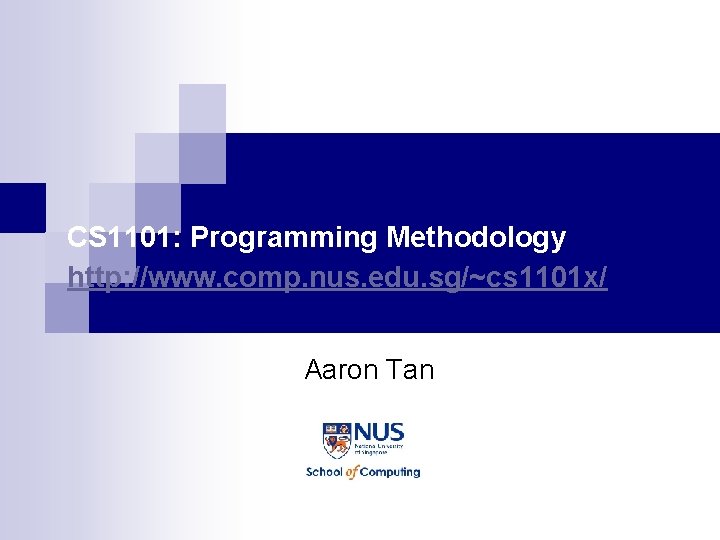
CS 1101: Programming Methodology http: //www. comp. nus. edu. sg/~cs 1101 x/ Aaron Tan
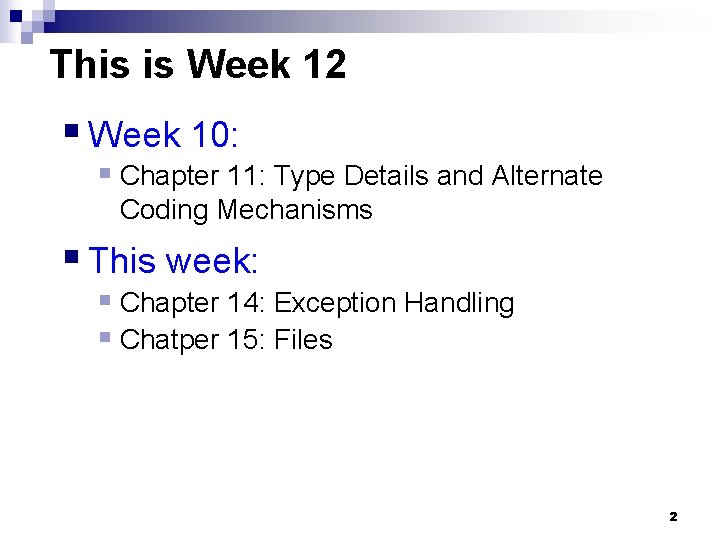
This is Week 12 § Week 10: § Chapter 11: Type Details and Alternate Coding Mechanisms § This week: § Chapter 14: Exception Handling § Chatper 15: Files 2
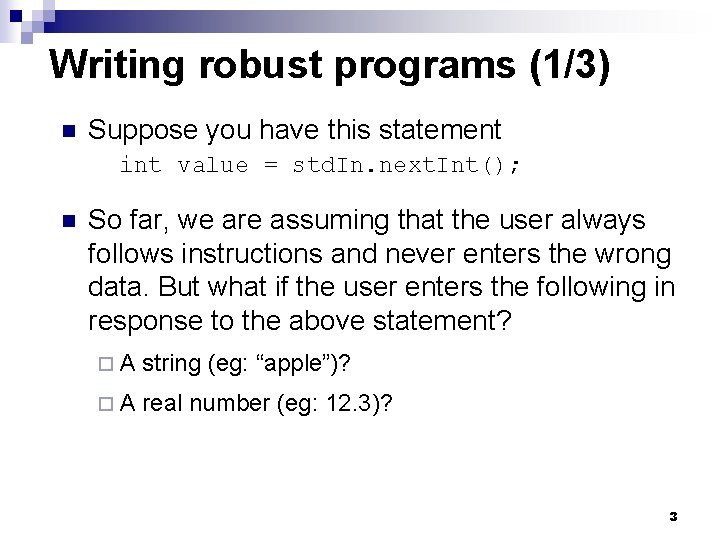
Writing robust programs (1/3) n Suppose you have this statement int value = std. In. next. Int(); n So far, we are assuming that the user always follows instructions and never enters the wrong data. But what if the user enters the following in response to the above statement? ¨A string (eg: “apple”)? ¨A real number (eg: 12. 3)? 3
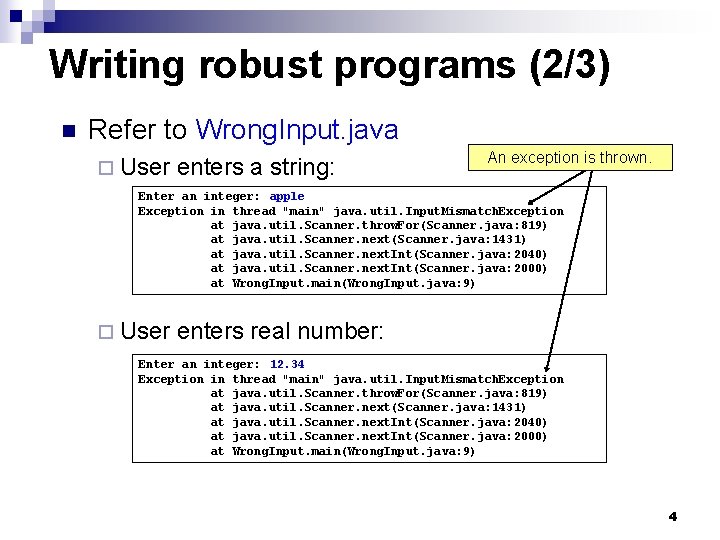
Writing robust programs (2/3) n Refer to Wrong. Input. java ¨ User enters a string: An exception is thrown. Enter an integer: apple Exception in thread "main" java. util. Input. Mismatch. Exception at java. util. Scanner. throw. For(Scanner. java: 819) at java. util. Scanner. next(Scanner. java: 1431) at java. util. Scanner. next. Int(Scanner. java: 2040) at java. util. Scanner. next. Int(Scanner. java: 2000) at Wrong. Input. main(Wrong. Input. java: 9) ¨ User enters real number: Enter an integer: 12. 34 Exception in thread "main" java. util. Input. Mismatch. Exception at java. util. Scanner. throw. For(Scanner. java: 819) at java. util. Scanner. next(Scanner. java: 1431) at java. util. Scanner. next. Int(Scanner. java: 2040) at java. util. Scanner. next. Int(Scanner. java: 2000) at Wrong. Input. main(Wrong. Input. java: 9) 4
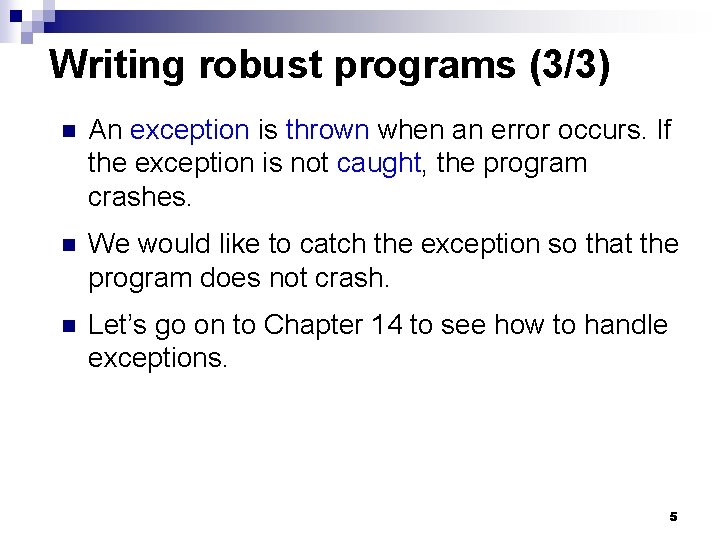
Writing robust programs (3/3) n An exception is thrown when an error occurs. If the exception is not caught, the program crashes. n We would like to catch the exception so that the program does not crash. n Let’s go on to Chapter 14 to see how to handle exceptions. 5
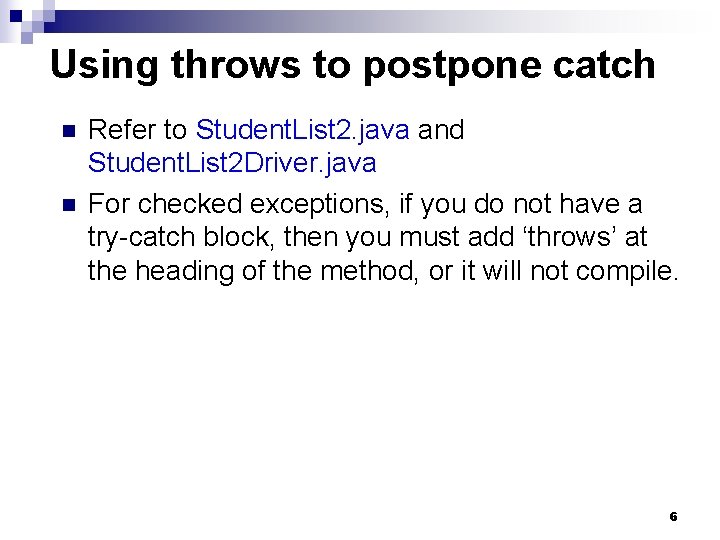
Using throws to postpone catch n n Refer to Student. List 2. java and Student. List 2 Driver. java For checked exceptions, if you do not have a try-catch block, then you must add ‘throws’ at the heading of the method, or it will not compile. 6
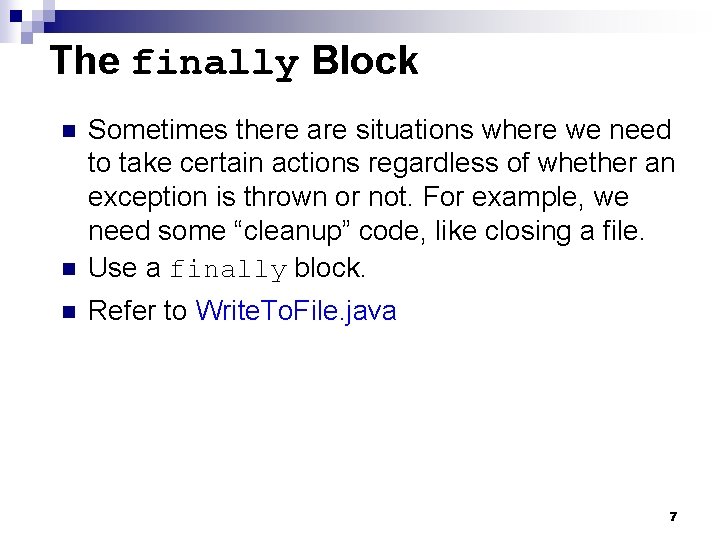
The finally Block n Sometimes there are situations where we need to take certain actions regardless of whether an exception is thrown or not. For example, we need some “cleanup” code, like closing a file. Use a finally block. n Refer to Write. To. File. java n 7
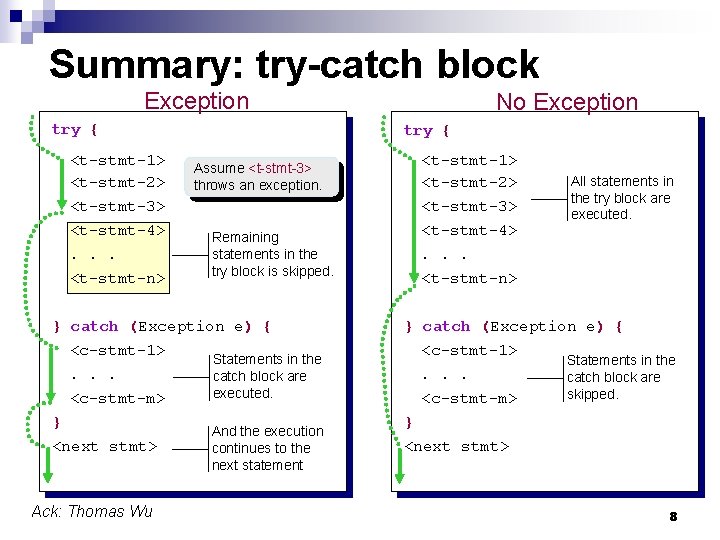
Summary: try-catch block Exception try { <t-stmt-1> <t-stmt-2> <t-stmt-3> <t-stmt-4>. . . <t-stmt-n> No Exception try { Assume <t-stmt-3> throws an exception. Remaining statements in the try block is skipped. } catch (Exception e) { <c-stmt-1> Statements in the. . . catch block are executed. <c-stmt-m> } And the execution <next stmt> continues to the <t-stmt-1> <t-stmt-2> <t-stmt-3> <t-stmt-4>. . . <t-stmt-n> All statements in the try block are executed. } catch (Exception e) { <c-stmt-1> Statements in the. . . catch block are skipped. <c-stmt-m> } <next stmt> next statement Ack: Thomas Wu 8
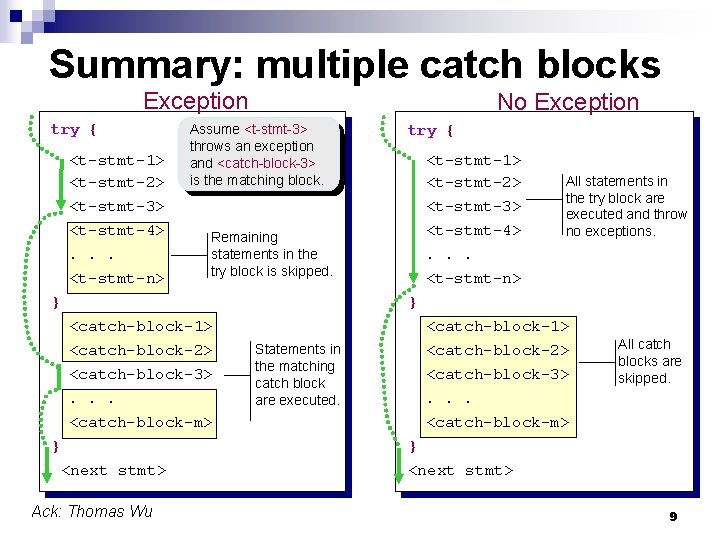
Summary: multiple catch blocks Exception try { <t-stmt-1> <t-stmt-2> <t-stmt-3> <t-stmt-4>. . . <t-stmt-n> No Exception Assume <t-stmt-3> throws an exception and <catch-block-3> is the matching block. try { <t-stmt-1> <t-stmt-2> <t-stmt-3> <t-stmt-4>. . . <t-stmt-n> Remaining statements in the try block is skipped. } All statements in the try block are executed and throw no exceptions. } <catch-block-1> <catch-block-2> <catch-block-3>. . . <catch-block-m> } <next stmt> Ack: Thomas Wu Statements in the matching catch block are executed. <catch-block-1> <catch-block-2> <catch-block-3>. . . <catch-block-m> All catch blocks are skipped. } <next stmt> 9
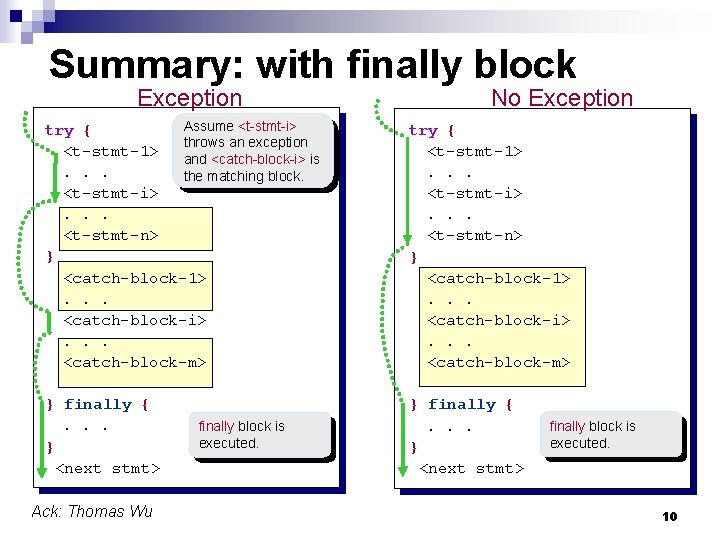
Summary: with finally block Exception No Exception Assume <t-stmt-i> try { throws an exception <t-stmt-1> and <catch-block-i> is. . . the matching block. <t-stmt-i>. . . <t-stmt-n> } <catch-block-1>. . . <catch-block-i>. . . <catch-block-m> try { <t-stmt-1>. . . <t-stmt-i>. . . <t-stmt-n> } <catch-block-1>. . . <catch-block-i>. . . <catch-block-m> } finally {. . . } <next stmt> Ack: Thomas Wu finally block is executed. 10
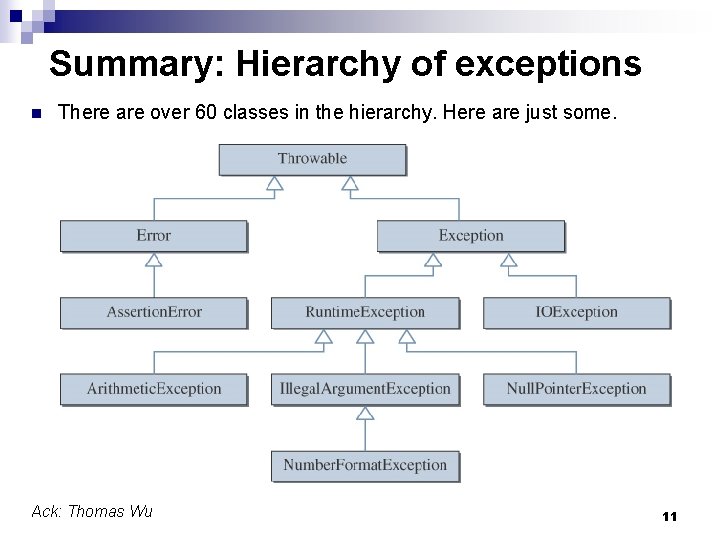
Summary: Hierarchy of exceptions n There are over 60 classes in the hierarchy. Here are just some. Ack: Thomas Wu 11
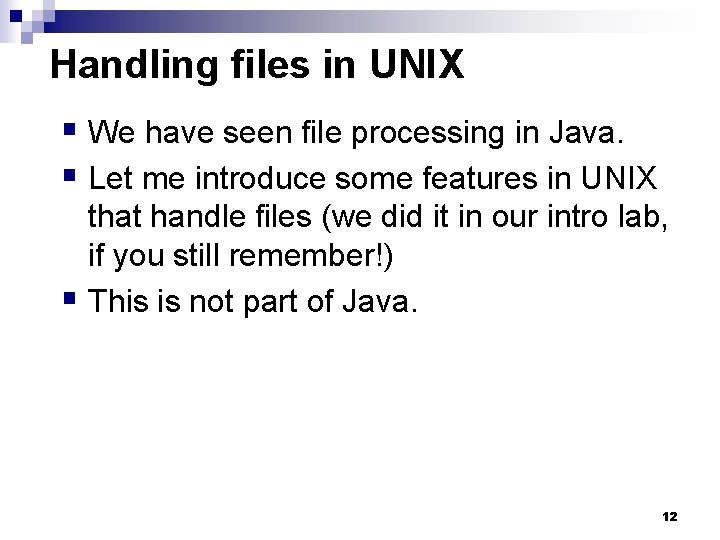
Handling files in UNIX § We have seen file processing in Java. § Let me introduce some features in UNIX that handle files (we did it in our intro lab, if you still remember!) § This is not part of Java. 12
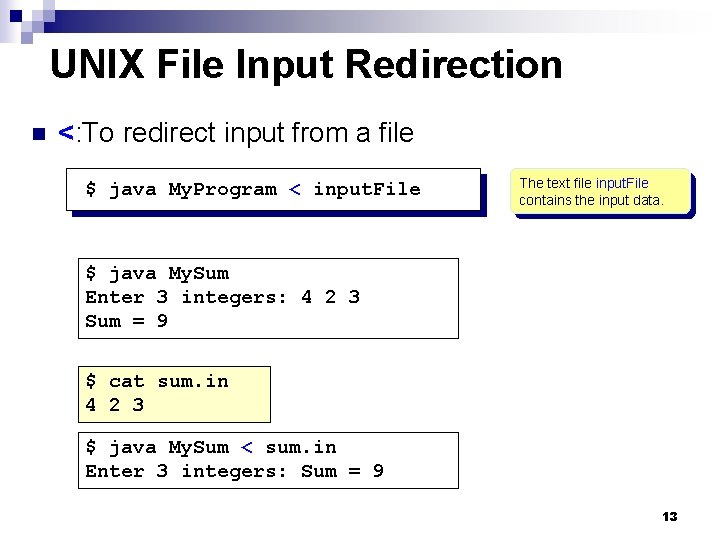
UNIX File Input Redirection n <: To redirect input from a file $ java My. Program < input. File The text file input. File contains the input data. $ java My. Sum Enter 3 integers: 4 2 3 Sum = 9 $ cat sum. in 4 2 3 $ java My. Sum < sum. in Enter 3 integers: Sum = 9 13
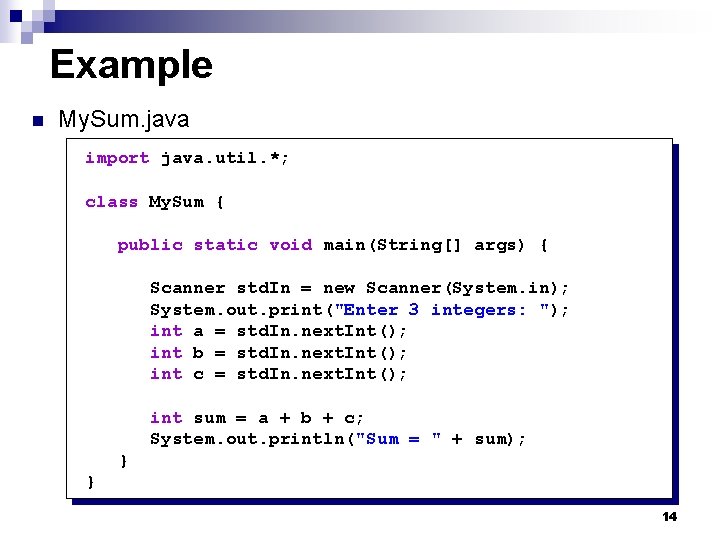
Example n My. Sum. java import java. util. *; class My. Sum { public static void main(String[] args) { Scanner std. In = new Scanner(System. in); System. out. print("Enter 3 integers: "); int a = std. In. next. Int(); int b = std. In. next. Int(); int c = std. In. next. Int(); int sum = a + b + c; System. out. println("Sum = " + sum); } } 14
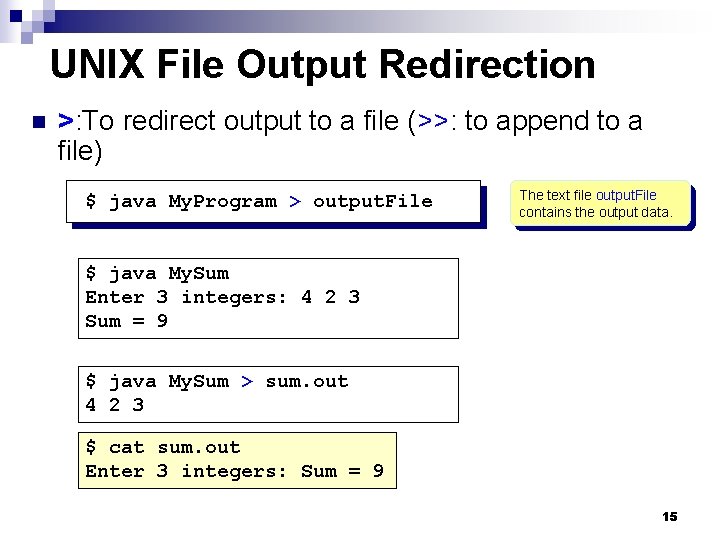
UNIX File Output Redirection n >: To redirect output to a file (>>: to append to a file) $ java My. Program > output. File The text file output. File contains the output data. $ java My. Sum Enter 3 integers: 4 2 3 Sum = 9 $ java My. Sum > sum. out 4 2 3 $ cat sum. out Enter 3 integers: Sum = 9 15
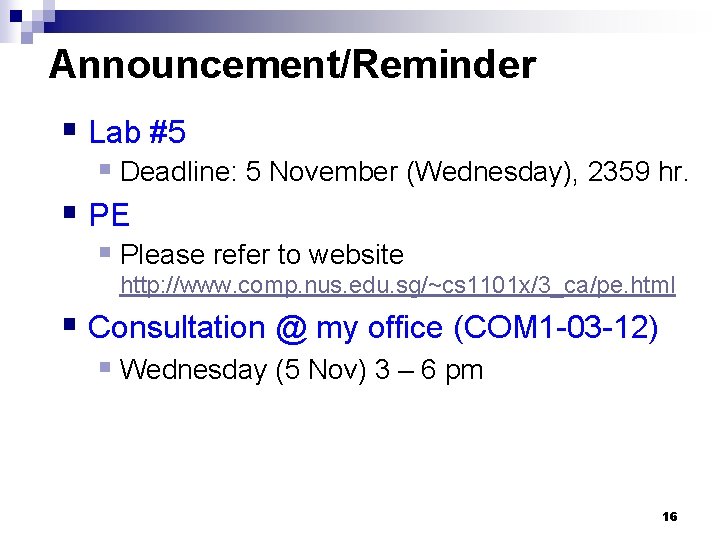
Announcement/Reminder § Lab #5 § Deadline: 5 November (Wednesday), 2359 hr. § PE § Please refer to website http: //www. comp. nus. edu. sg/~cs 1101 x/3_ca/pe. html § Consultation @ my office (COM 1 -03 -12) § Wednesday (5 Nov) 3 – 6 pm 16
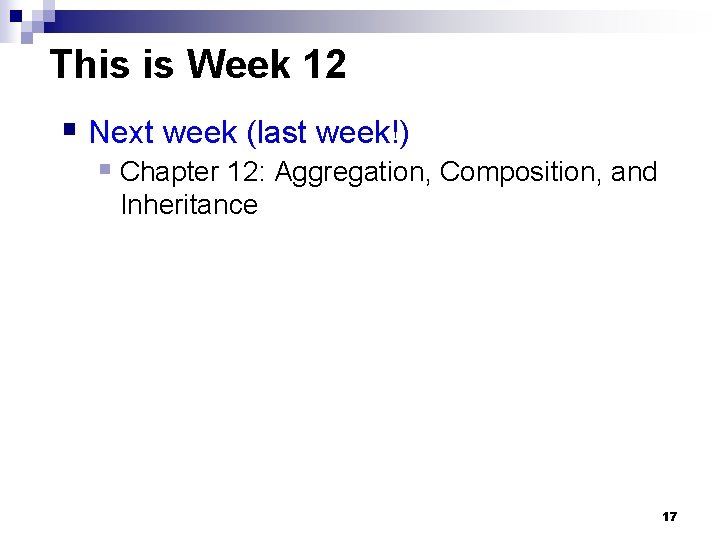
This is Week 12 § Next week (last week!) § Chapter 12: Aggregation, Composition, and Inheritance 17
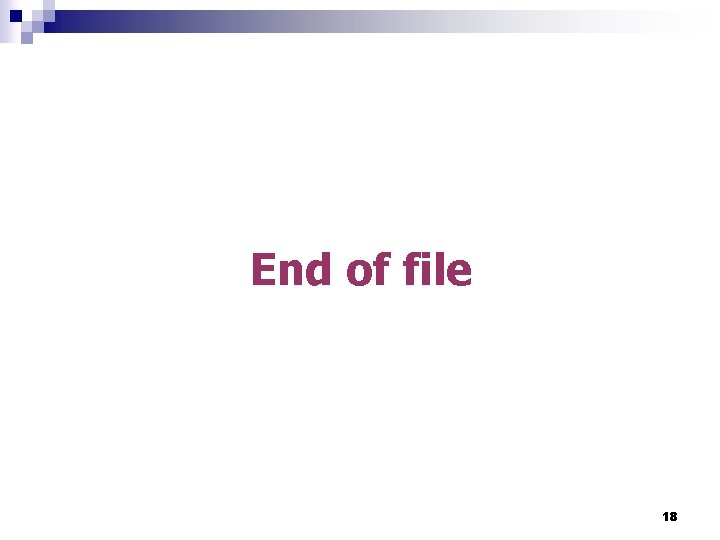
End of file 18
Ivle nus
Salute report example
Cs 1101 programming fundamentals final exam
Cs 1101 programming fundamentals
Cs 1101 programming fundamentals
Sequence detector 1101
29 cfr 1926 class asbestps
Uga pols 1101
Enc 1101 final exam
Sequence detector 1101
Cst 1101
Binary 1101
Cst 1101
Sls 1101
Pols 1101 final exam study guide
Sistema binario suma
Afi 51-1101
Enc 1101 fiu
Sintagma verbal